ECE 491 Senior Design I Lecture 4 Sequential
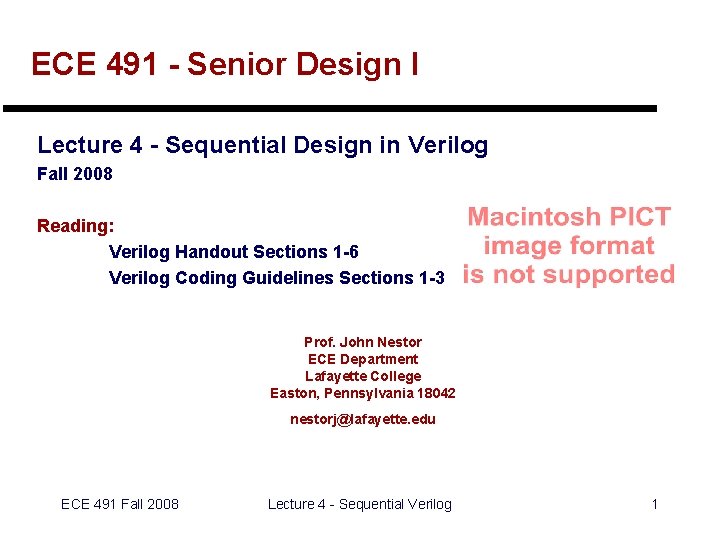
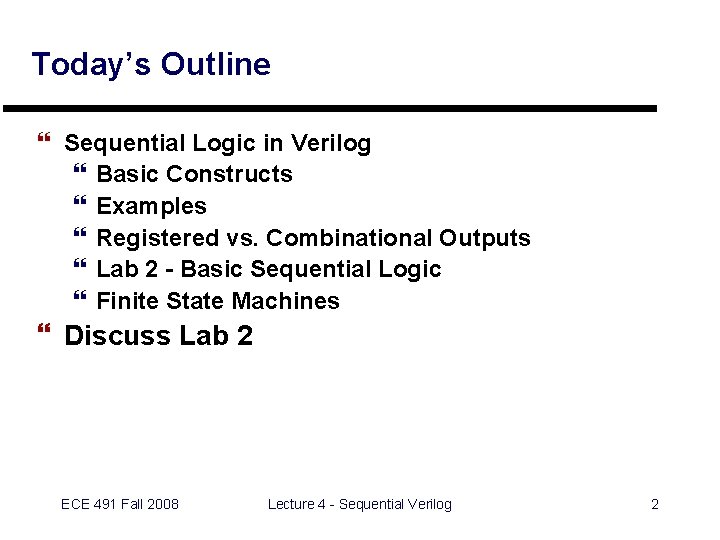
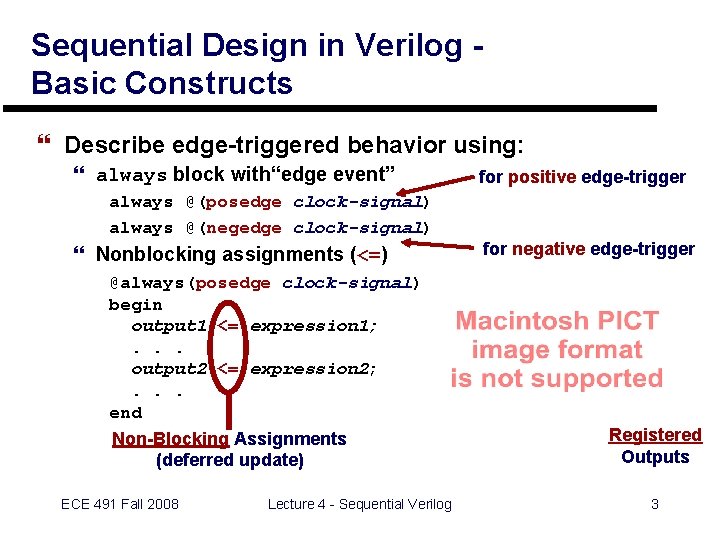
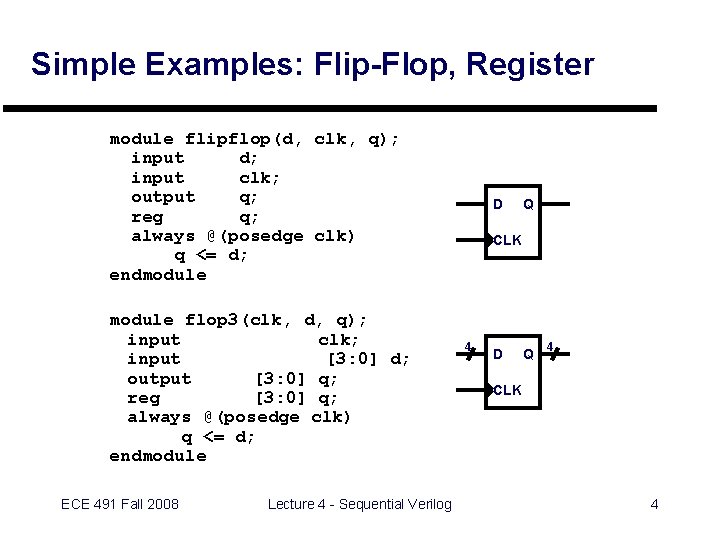
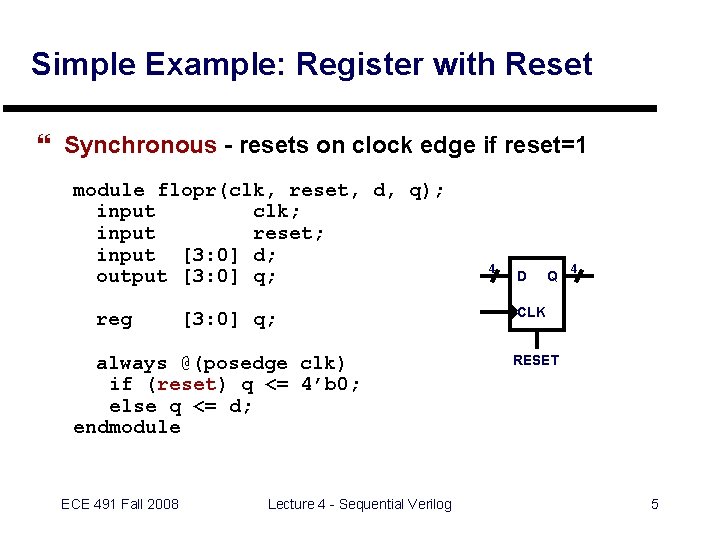
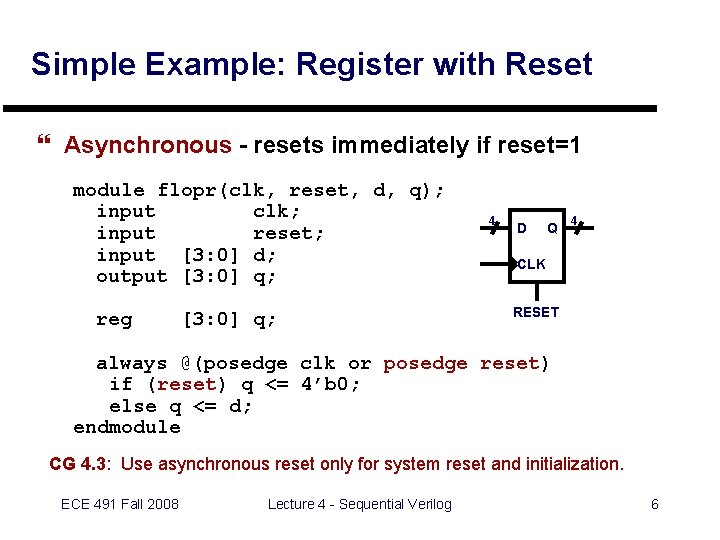
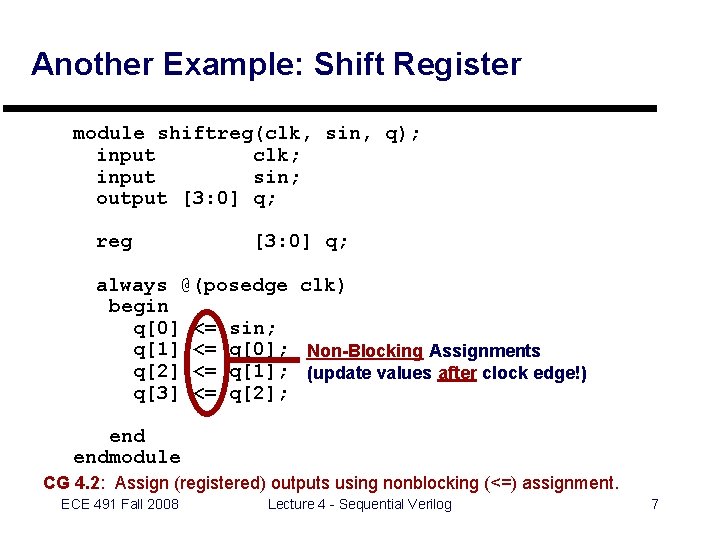
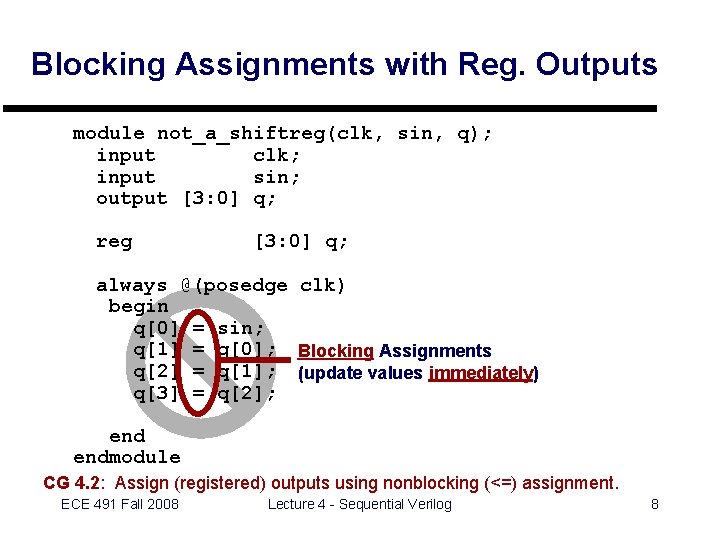
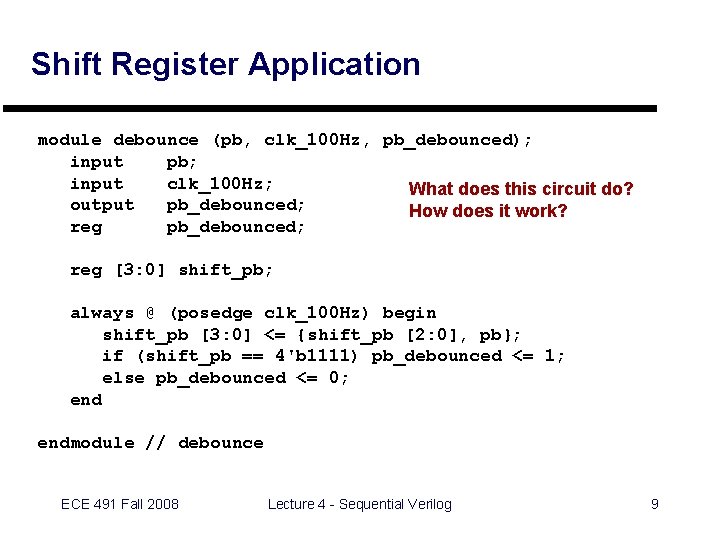
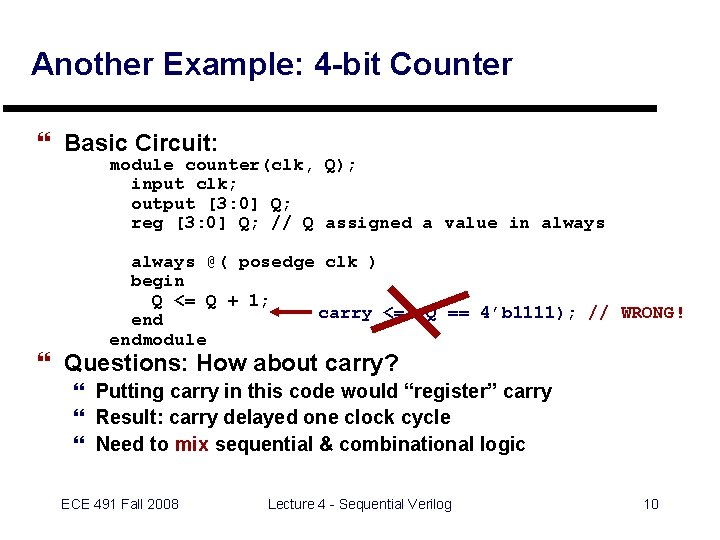
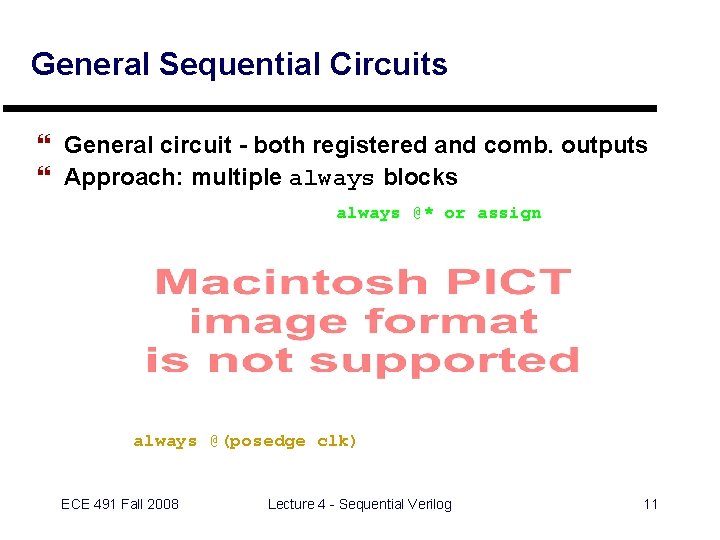
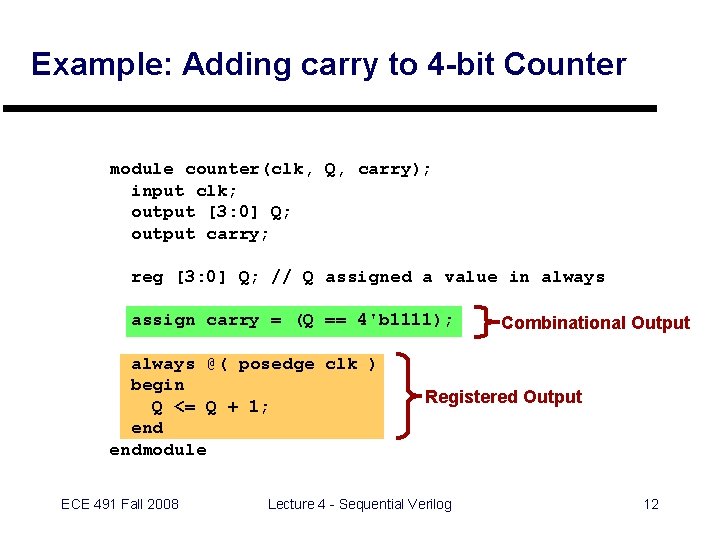
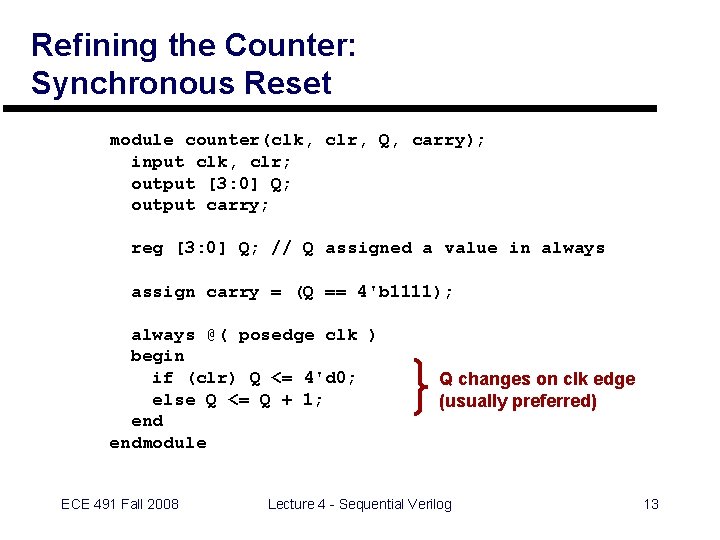
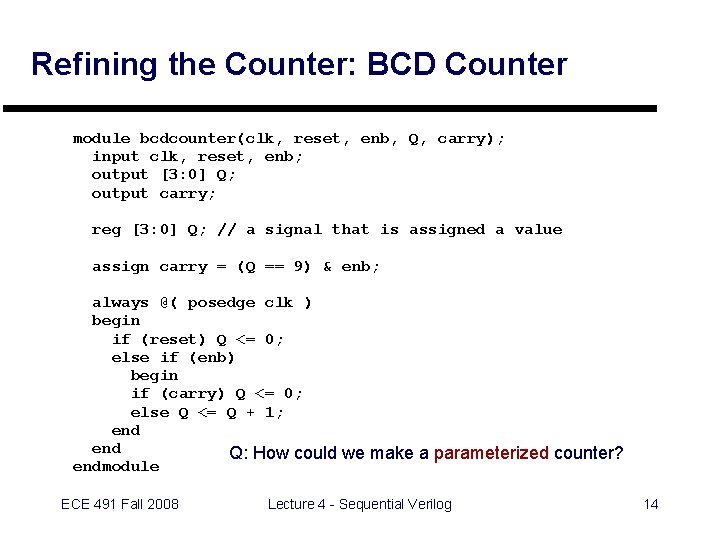
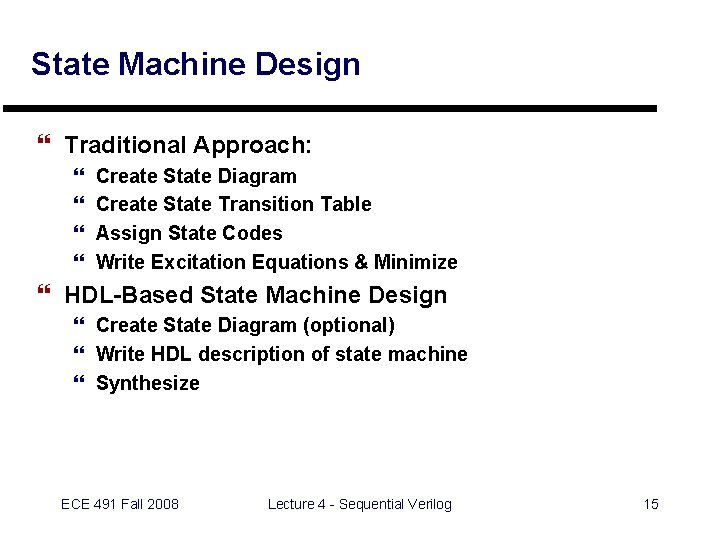
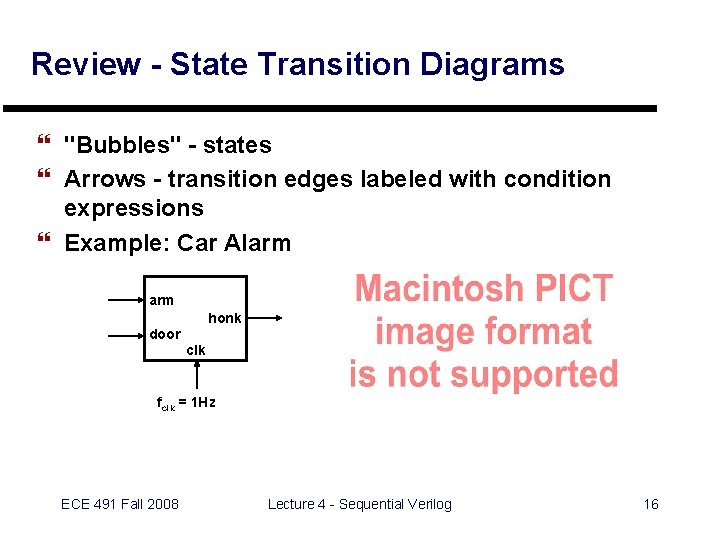
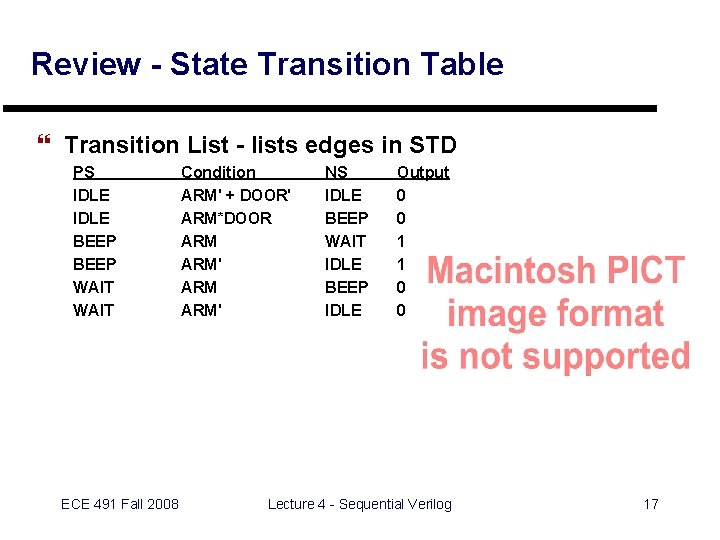
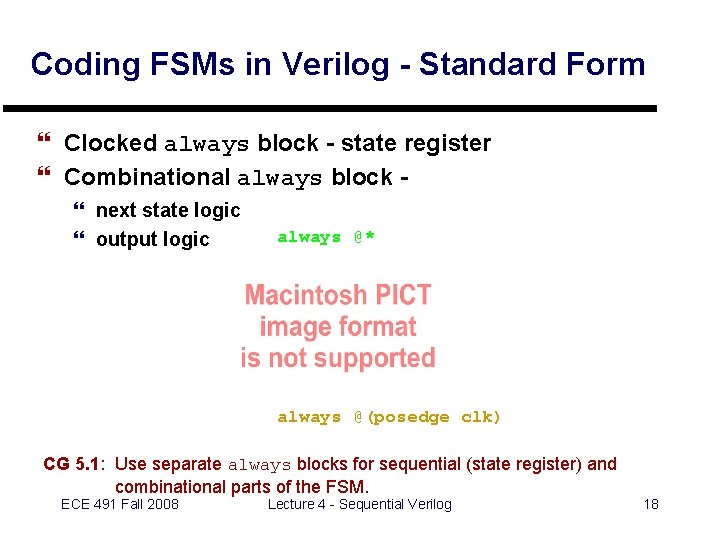
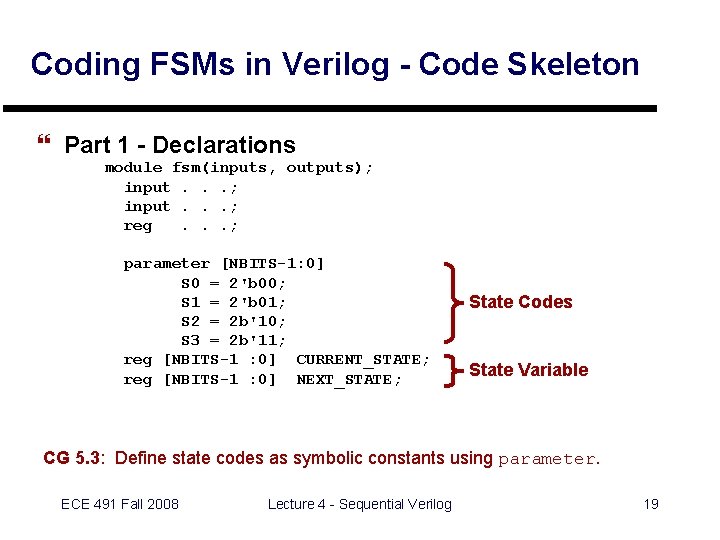
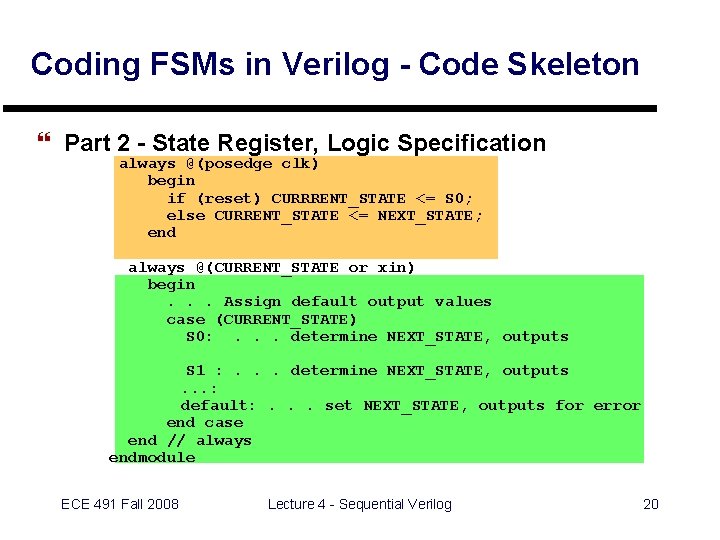
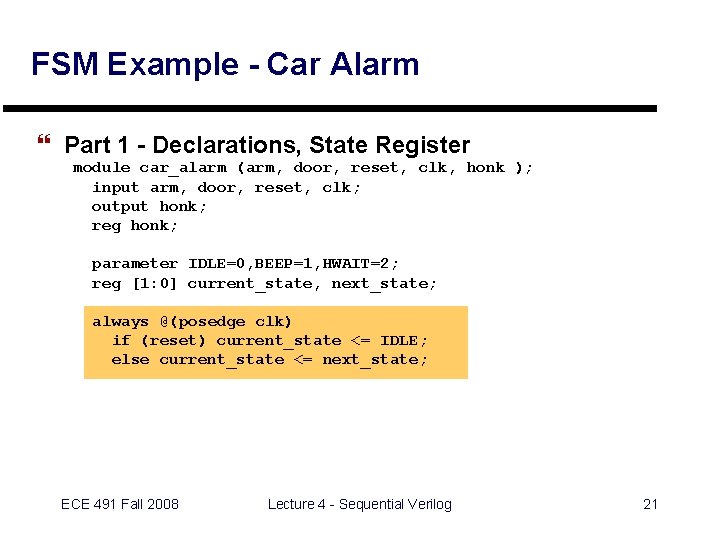
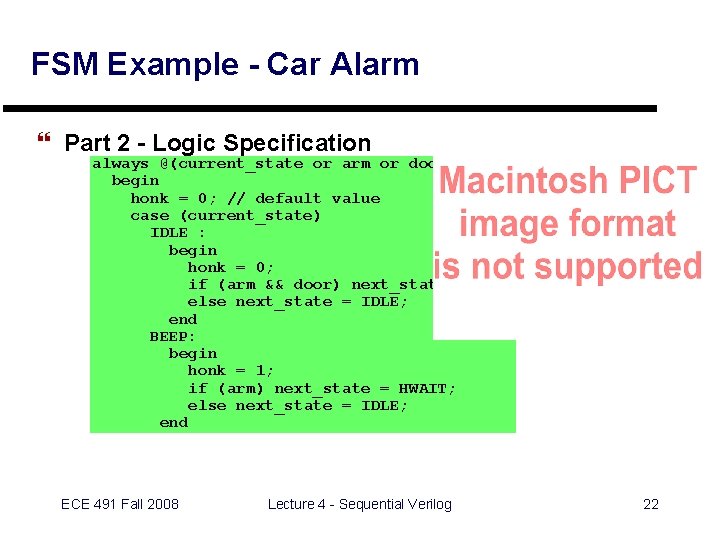
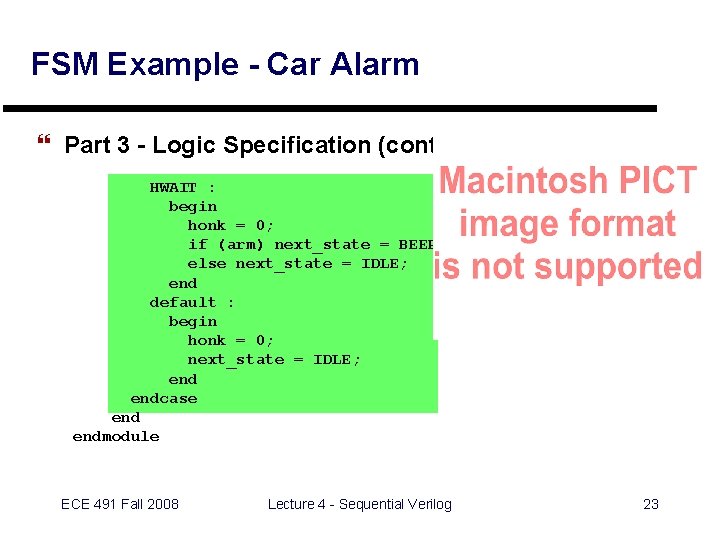
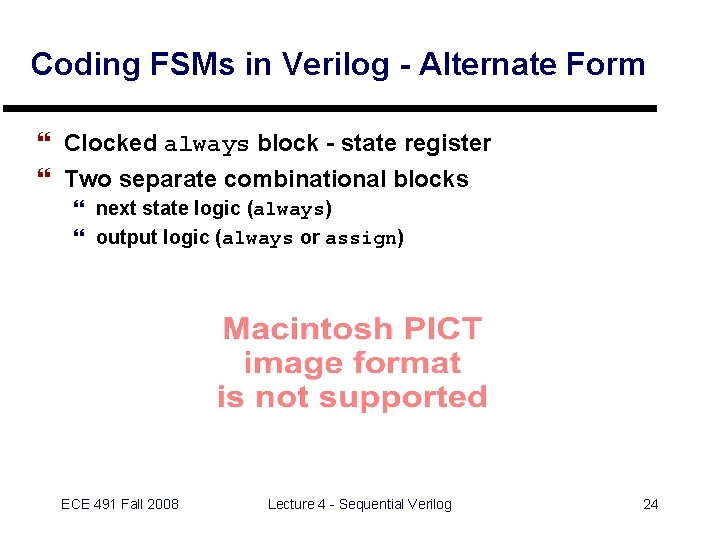
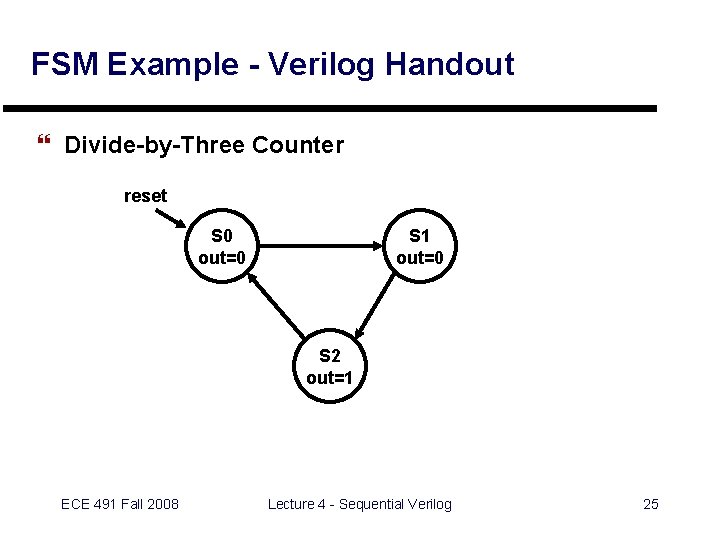
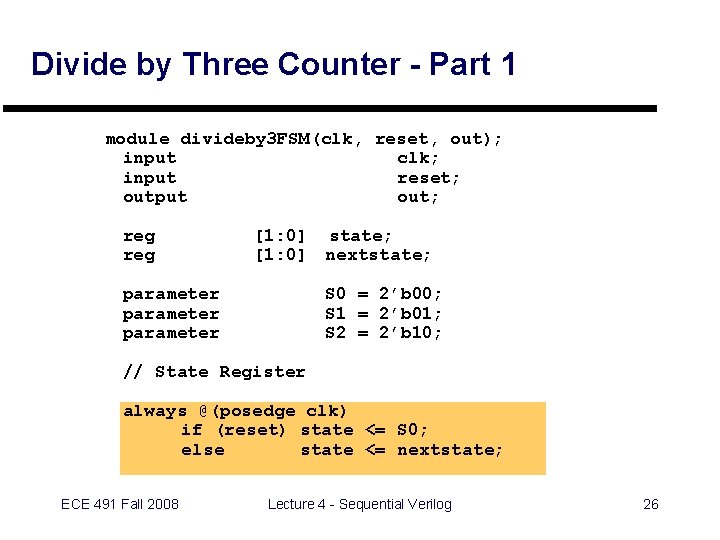
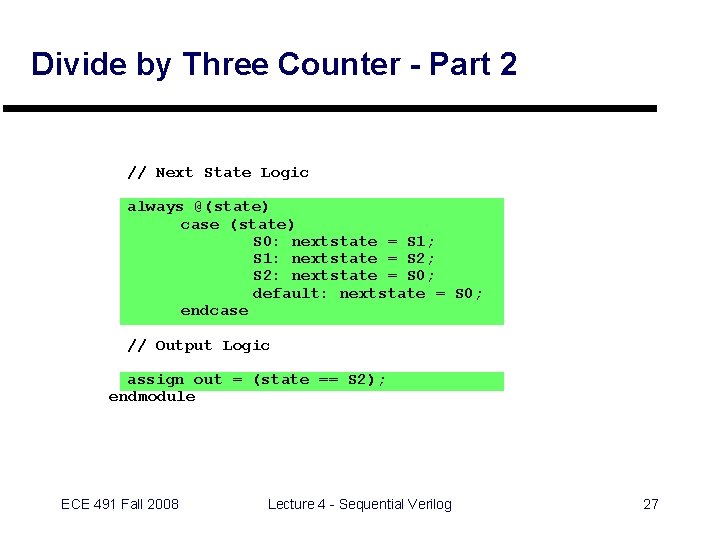
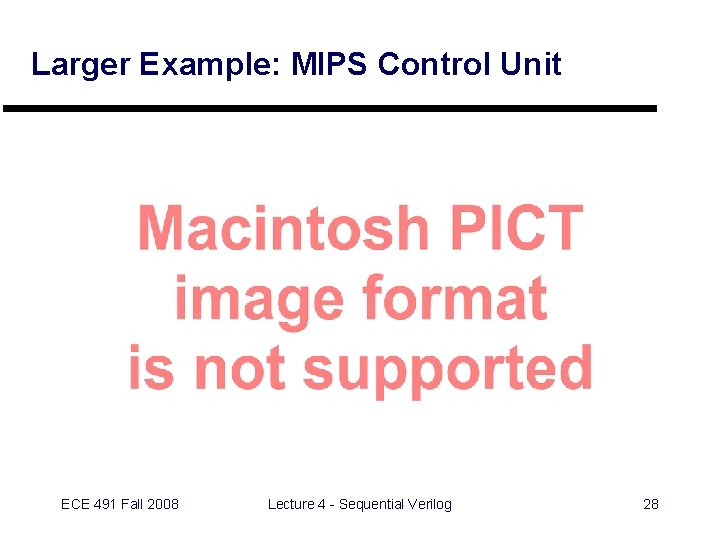
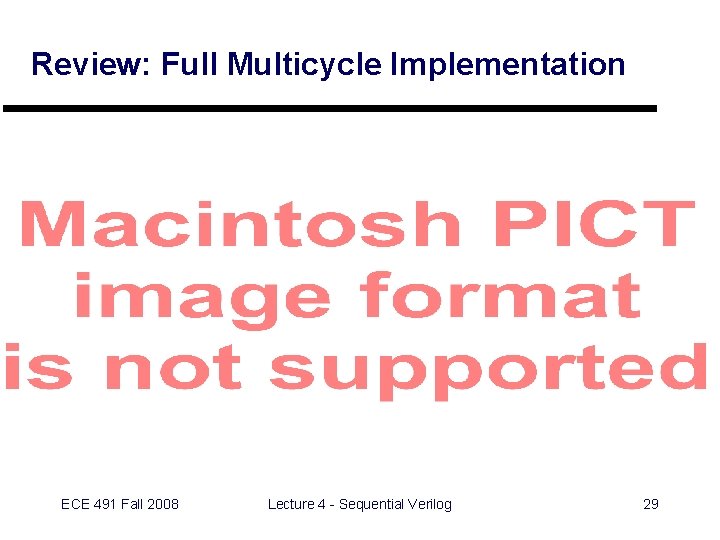
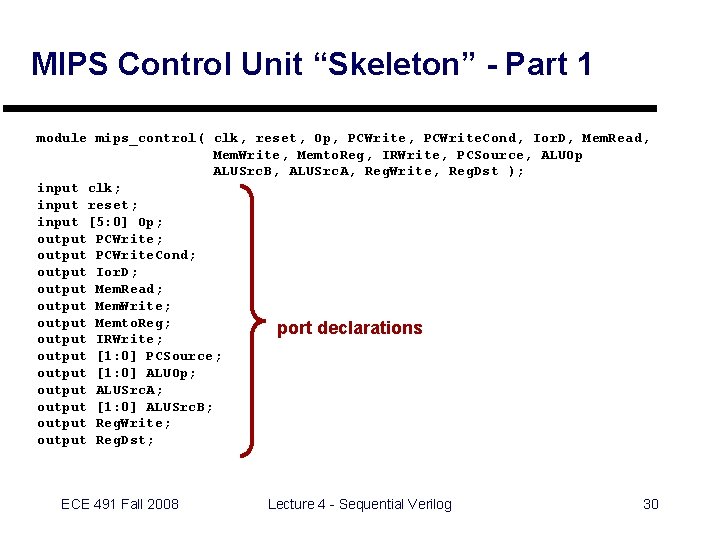
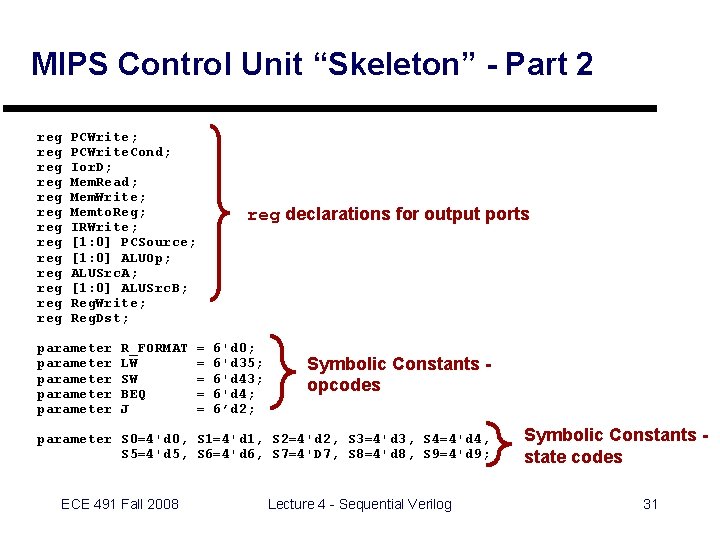
![MIPS Control Unit - Part 3 reg [3: 0] current_state, next_state; always @(negedge clk) MIPS Control Unit - Part 3 reg [3: 0] current_state, next_state; always @(negedge clk)](https://slidetodoc.com/presentation_image_h2/641281e45dab0ad713902a9fe63852bc/image-32.jpg)
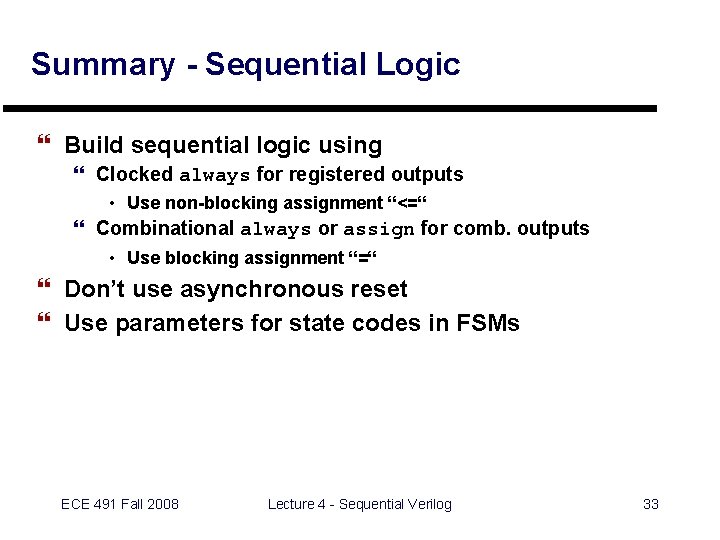
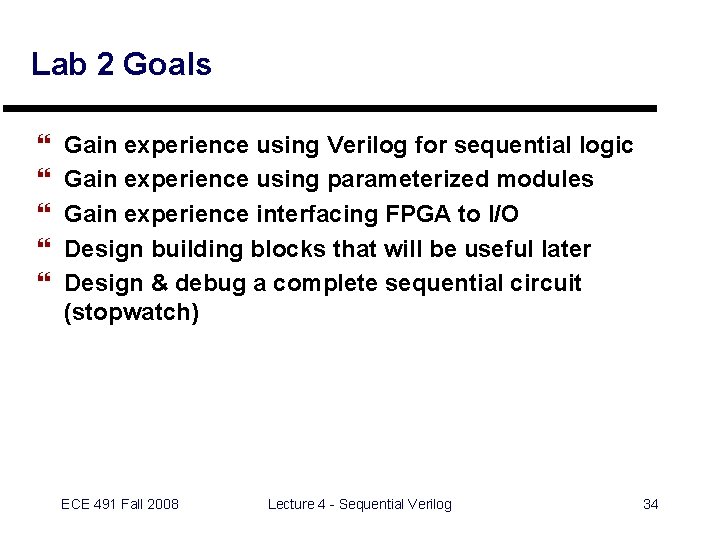
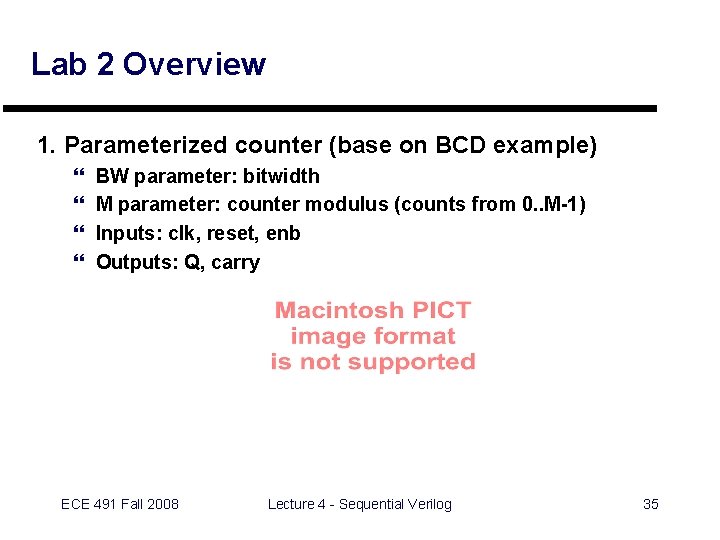
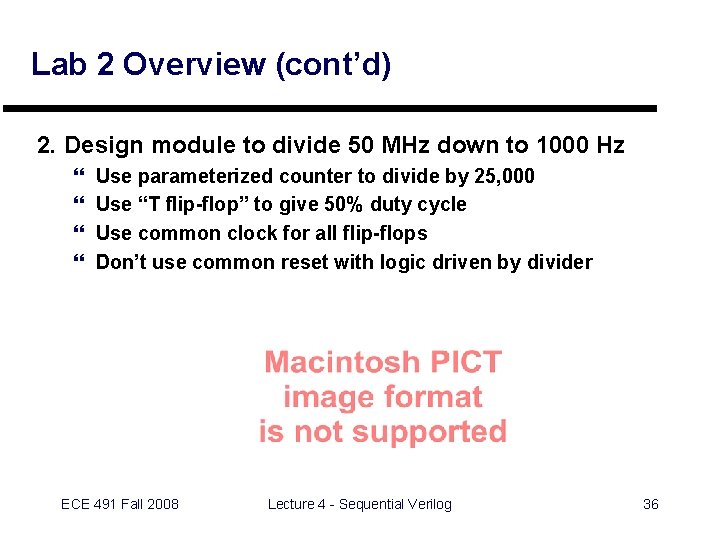
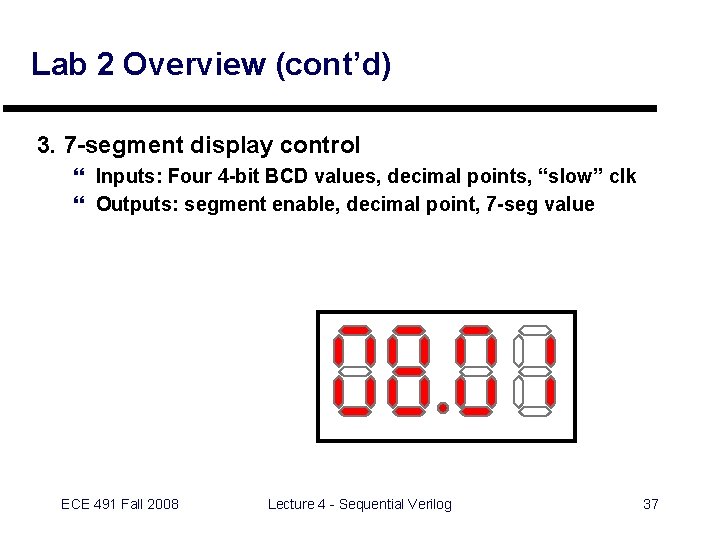
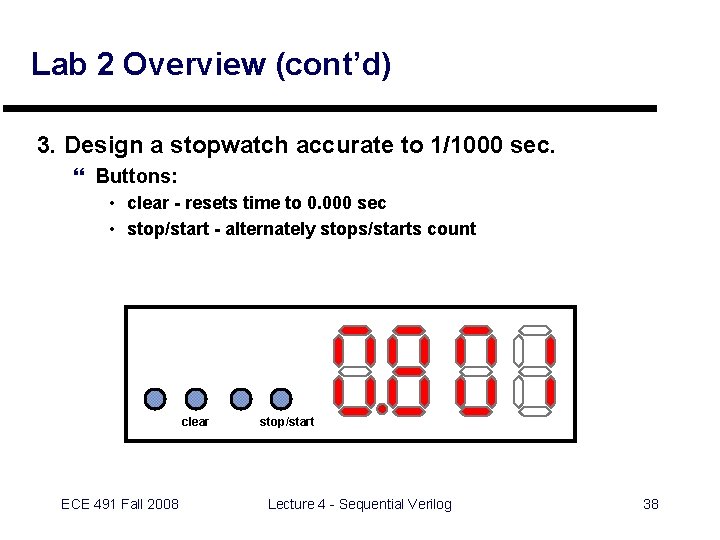
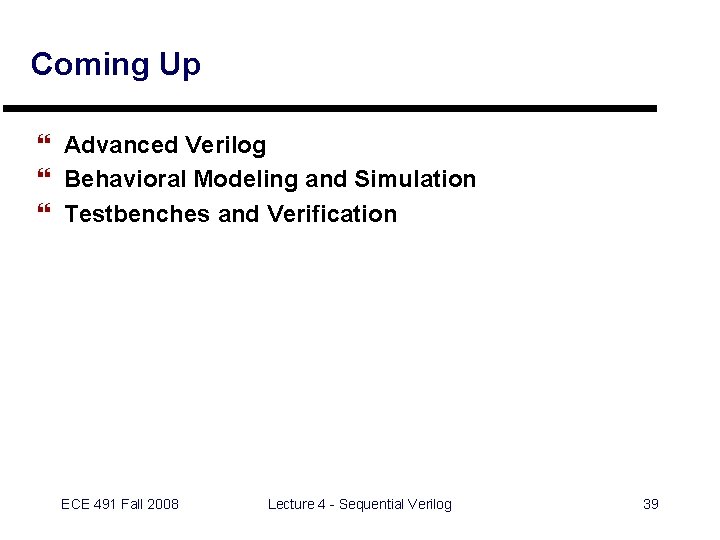
- Slides: 39
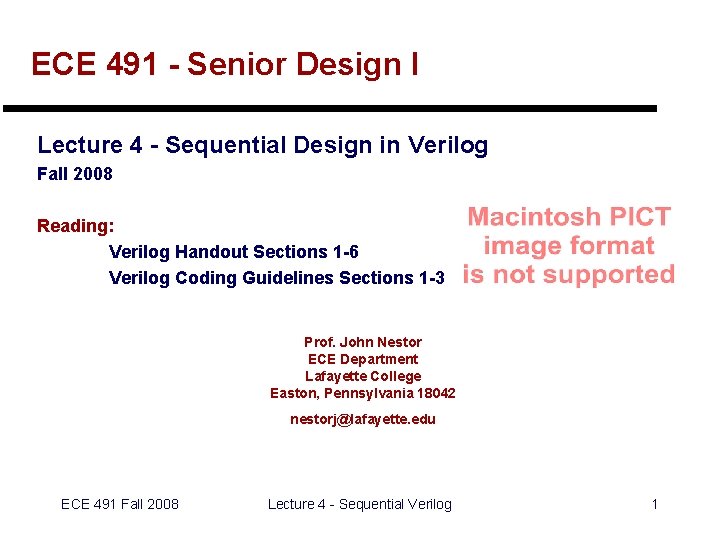
ECE 491 - Senior Design I Lecture 4 - Sequential Design in Verilog Fall 2008 Reading: Verilog Handout Sections 1 -6 Verilog Coding Guidelines Sections 1 -3 Prof. John Nestor ECE Department Lafayette College Easton, Pennsylvania 18042 nestorj@lafayette. edu ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 1
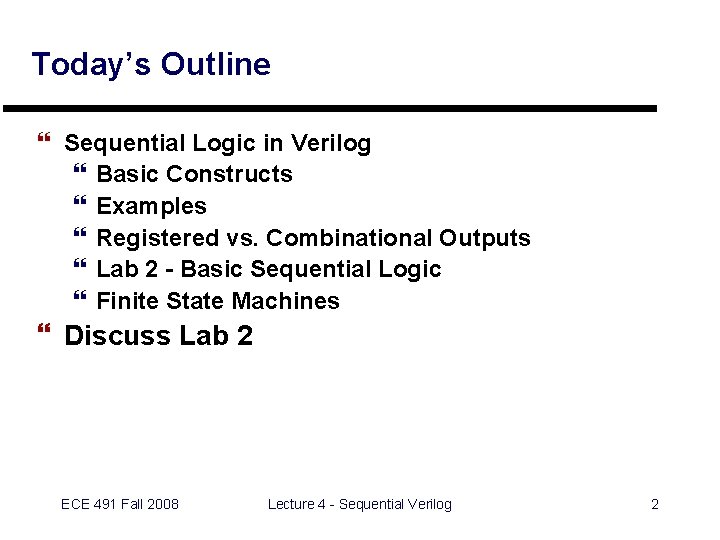
Today’s Outline } Sequential Logic in Verilog } Basic Constructs } Examples } Registered vs. Combinational Outputs } Lab 2 - Basic Sequential Logic } Finite State Machines } Discuss Lab 2 ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 2
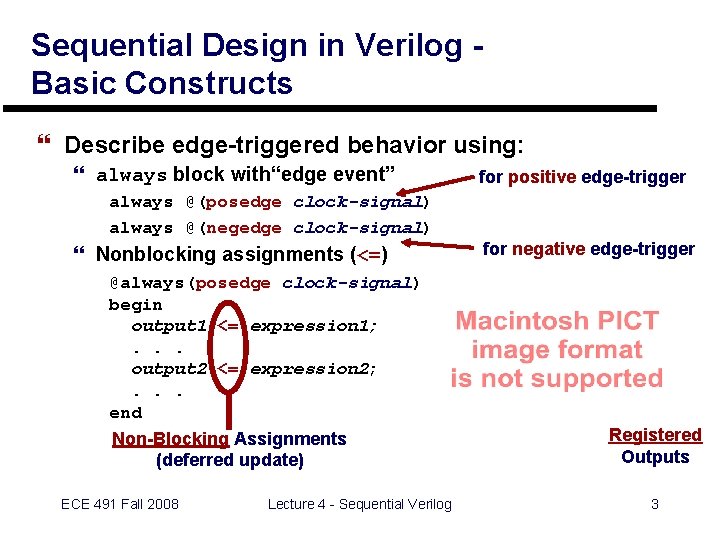
Sequential Design in Verilog Basic Constructs } Describe edge-triggered behavior using: } always block with“edge event” for positive edge-trigger always @(posedge clock-signal) always @(negedge clock-signal) } Nonblocking assignments (<=) for negative edge-trigger @always(posedge clock-signal) begin output 1 <= expression 1; . . . output 2 <= expression 2; . . . end Non-Blocking Assignments (deferred update) ECE 491 Fall 2008 Lecture 4 - Sequential Verilog Registered Outputs 3
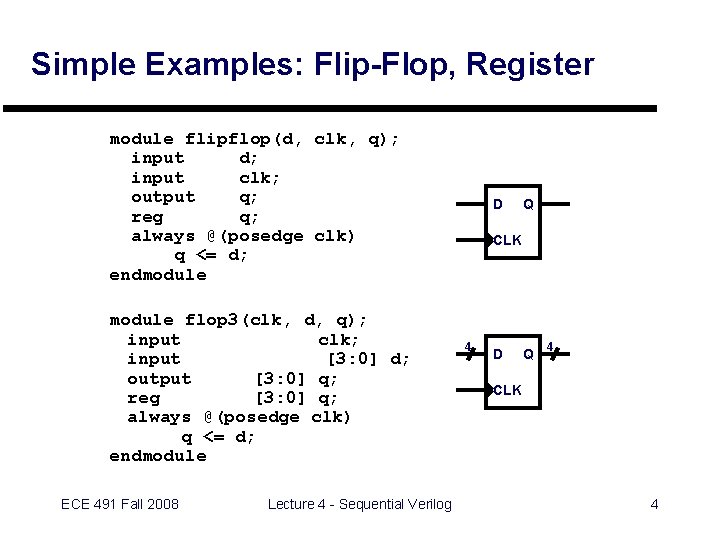
Simple Examples: Flip-Flop, Register module flipflop(d, clk, q); input d; input clk; output q; reg q; always @(posedge clk) q <= d; endmodule flop 3(clk, d, q); input clk; input [3: 0] d; output [3: 0] q; reg [3: 0] q; always @(posedge clk) q <= d; endmodule ECE 491 Fall 2008 Lecture 4 - Sequential Verilog D Q CLK 4 D Q 4 CLK 4
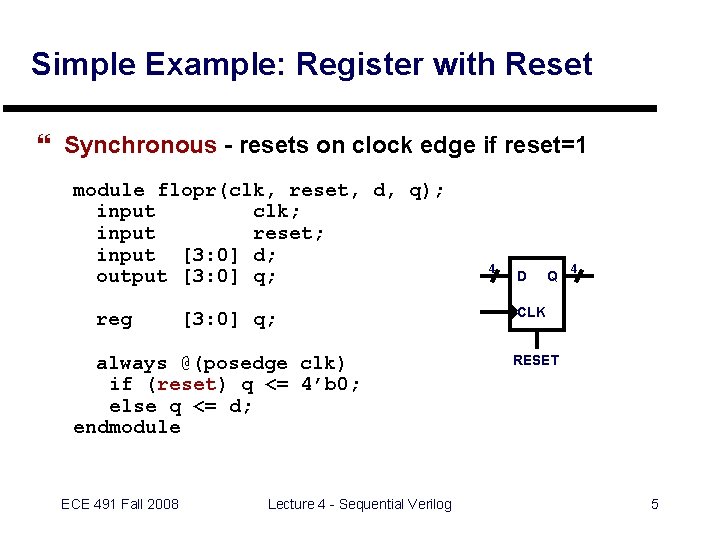
Simple Example: Register with Reset } Synchronous - resets on clock edge if reset=1 module flopr(clk, reset, d, q); input clk; input reset; input [3: 0] d; output [3: 0] q; reg [3: 0] q; always @(posedge clk) if (reset) q <= 4’b 0; else q <= d; endmodule ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 4 D Q 4 CLK RESET 5
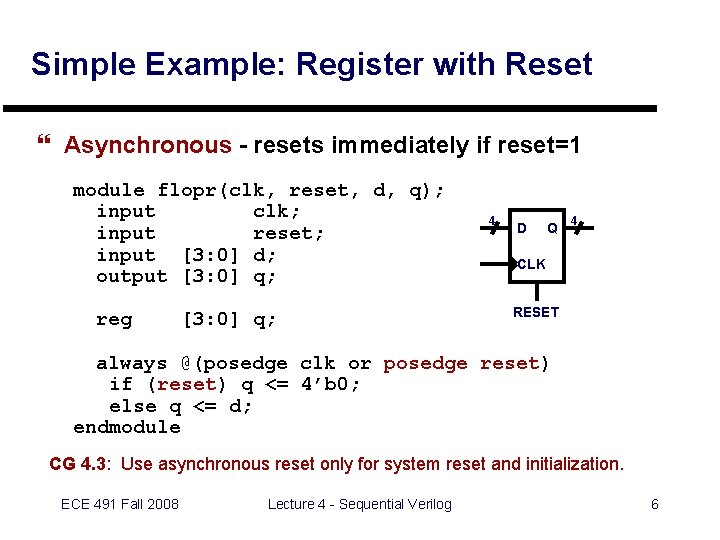
Simple Example: Register with Reset } Asynchronous - resets immediately if reset=1 module flopr(clk, reset, d, q); input clk; input reset; input [3: 0] d; output [3: 0] q; reg [3: 0] q; 4 D Q 4 CLK RESET always @(posedge clk or posedge reset) if (reset) q <= 4’b 0; else q <= d; endmodule CG 4. 3: Use asynchronous reset only for system reset and initialization. ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 6
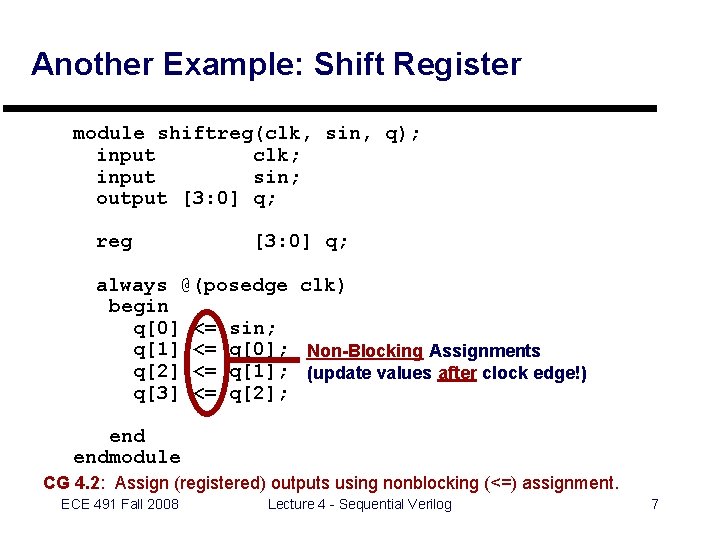
Another Example: Shift Register module shiftreg(clk, sin, q); input clk; input sin; output [3: 0] q; reg [3: 0] q; always @(posedge clk) begin q[0] <= sin; q[1] <= q[0]; Non-Blocking Assignments q[2] <= q[1]; (update values after clock edge!) q[3] <= q[2]; endmodule CG 4. 2: Assign (registered) outputs using nonblocking (<=) assignment. ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 7
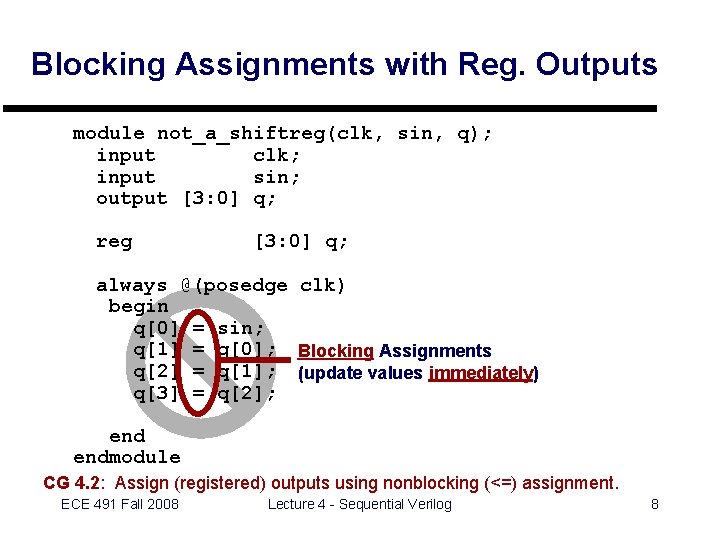
Blocking Assignments with Reg. Outputs module not_a_shiftreg(clk, sin, q); input clk; input sin; output [3: 0] q; reg [3: 0] q; always @(posedge clk) begin q[0] = sin; q[1] = q[0]; Blocking Assignments q[2] = q[1]; (update values immediately) q[3] = q[2]; endmodule CG 4. 2: Assign (registered) outputs using nonblocking (<=) assignment. ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 8
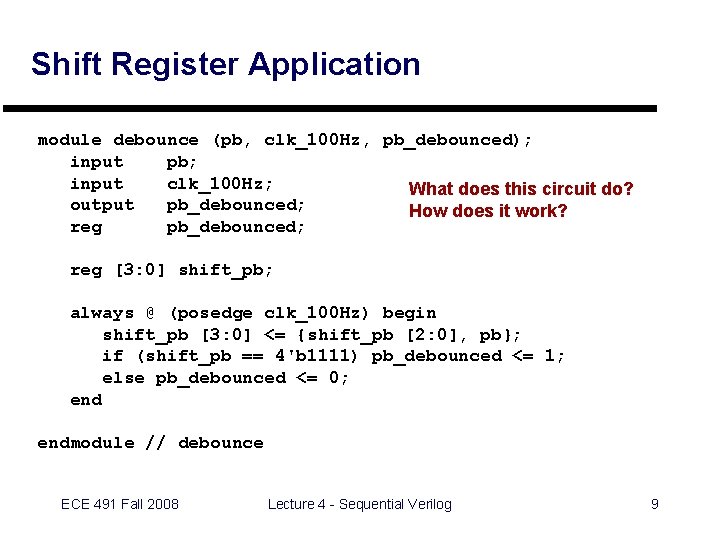
Shift Register Application module debounce (pb, clk_100 Hz, pb_debounced); input pb; input clk_100 Hz; What does this circuit do? output pb_debounced; How does it work? reg pb_debounced; reg [3: 0] shift_pb; always @ (posedge clk_100 Hz) begin shift_pb [3: 0] <= {shift_pb [2: 0], pb}; if (shift_pb == 4'b 1111) pb_debounced <= 1; else pb_debounced <= 0; endmodule // debounce ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 9
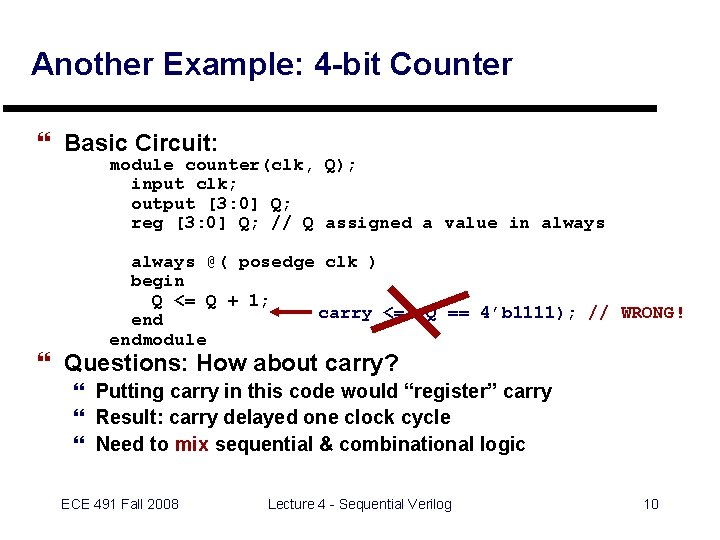
Another Example: 4 -bit Counter } Basic Circuit: module counter(clk, Q); input clk; output [3: 0] Q; reg [3: 0] Q; // Q assigned a value in always @( posedge clk ) begin Q <= Q + 1; carry <= (Q == 4’b 1111); // WRONG! endmodule } Questions: How about carry? } Putting carry in this code would “register” carry } Result: carry delayed one clock cycle } Need to mix sequential & combinational logic ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 10
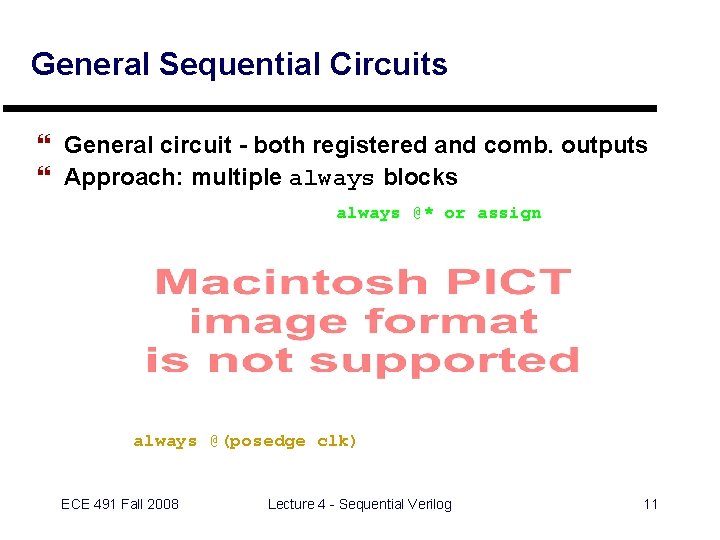
General Sequential Circuits } General circuit - both registered and comb. outputs } Approach: multiple always blocks always @* or assign always @(posedge clk) ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 11
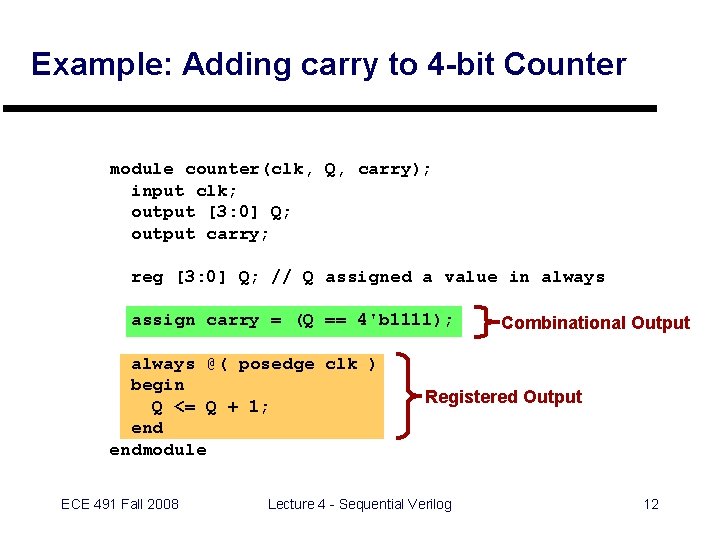
Example: Adding carry to 4 -bit Counter module counter(clk, Q, carry); input clk; output [3: 0] Q; output carry; reg [3: 0] Q; // Q assigned a value in always assign carry = (Q == 4'b 1111); always @( posedge clk ) begin Q <= Q + 1; endmodule ECE 491 Fall 2008 Combinational Output Registered Output Lecture 4 - Sequential Verilog 12
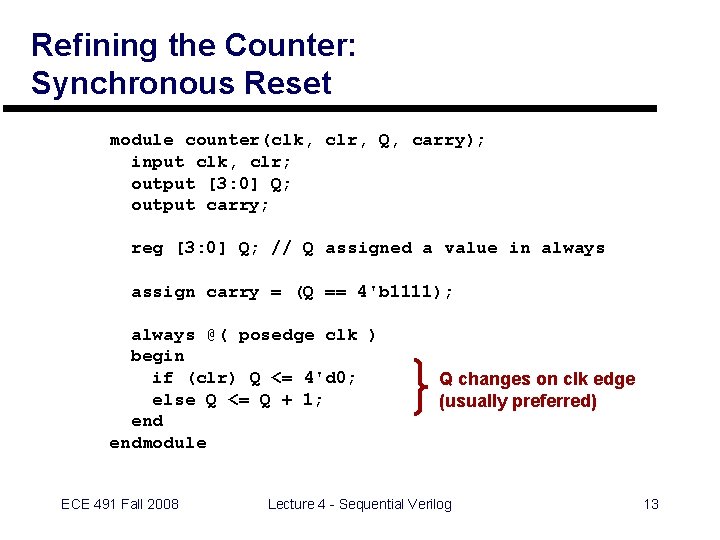
Refining the Counter: Synchronous Reset module counter(clk, clr, Q, carry); input clk, clr; output [3: 0] Q; output carry; reg [3: 0] Q; // Q assigned a value in always assign carry = (Q == 4'b 1111); always @( posedge clk ) begin if (clr) Q <= 4'd 0; else Q <= Q + 1; endmodule ECE 491 Fall 2008 Q changes on clk edge (usually preferred) Lecture 4 - Sequential Verilog 13
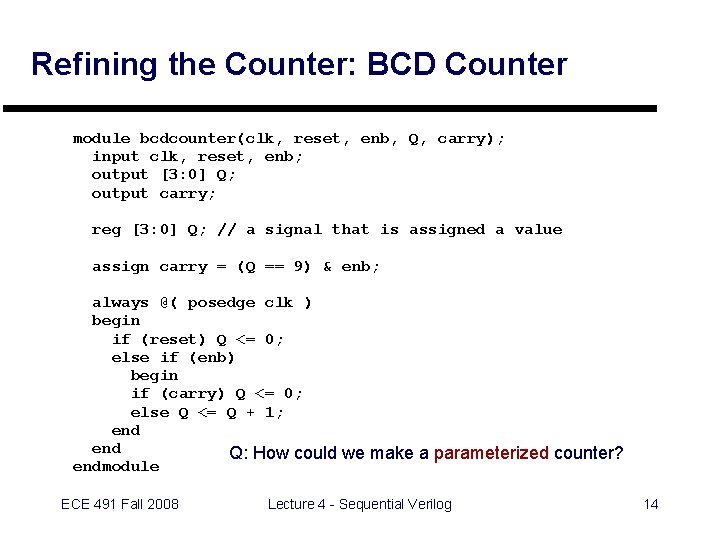
Refining the Counter: BCD Counter module bcdcounter(clk, reset, enb, Q, carry); input clk, reset, enb; output [3: 0] Q; output carry; reg [3: 0] Q; // a signal that is assigned a value assign carry = (Q == 9) & enb; always @( posedge clk ) begin if (reset) Q <= 0; else if (enb) begin if (carry) Q <= 0; else Q <= Q + 1; end Q: How could we make a parameterized counter? endmodule ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 14
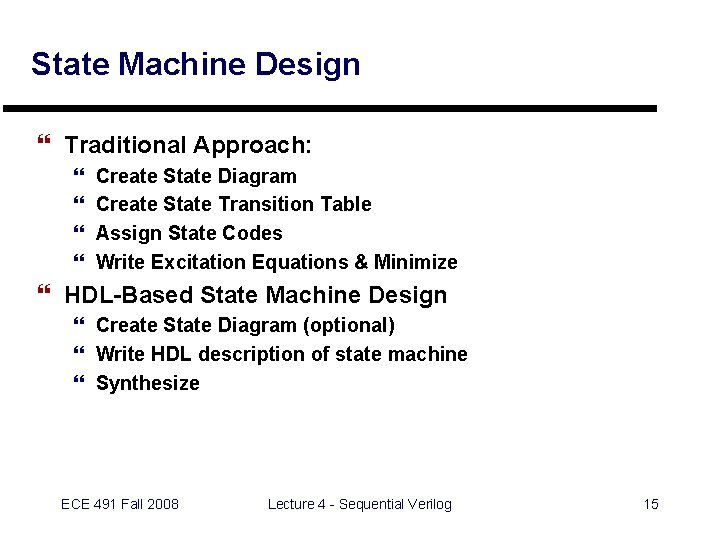
State Machine Design } Traditional Approach: } } Create State Diagram Create State Transition Table Assign State Codes Write Excitation Equations & Minimize } HDL-Based State Machine Design } Create State Diagram (optional) } Write HDL description of state machine } Synthesize ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 15
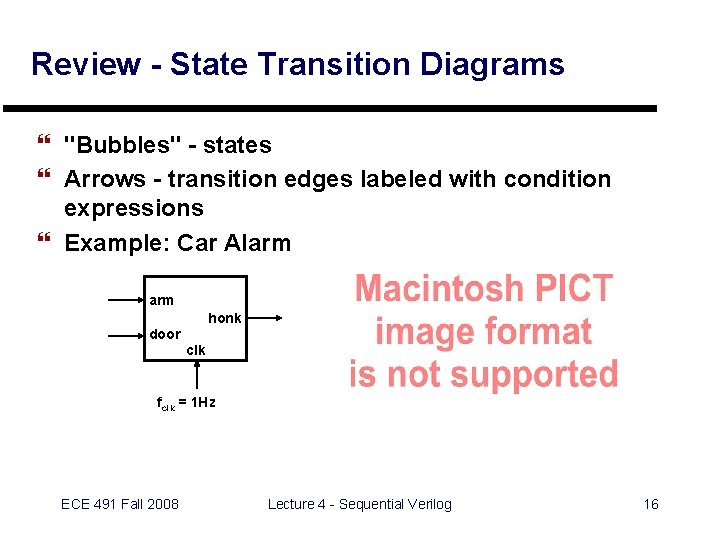
Review - State Transition Diagrams } "Bubbles" - states } Arrows - transition edges labeled with condition expressions } Example: Car Alarm honk door clk fclk = 1 Hz ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 16
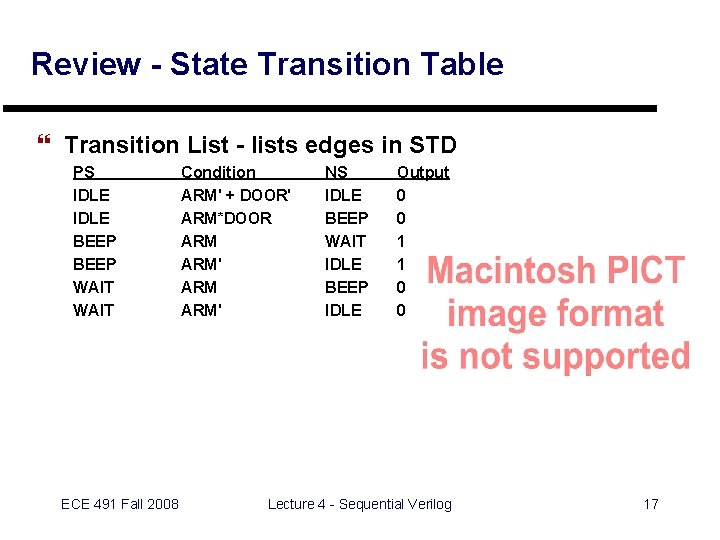
Review - State Transition Table } Transition List - lists edges in STD PS IDLE BEEP WAIT ECE 491 Fall 2008 Condition ARM' + DOOR' ARM*DOOR ARM ARM' NS IDLE BEEP WAIT IDLE BEEP IDLE Output 0 0 1 1 0 0 Lecture 4 - Sequential Verilog 17
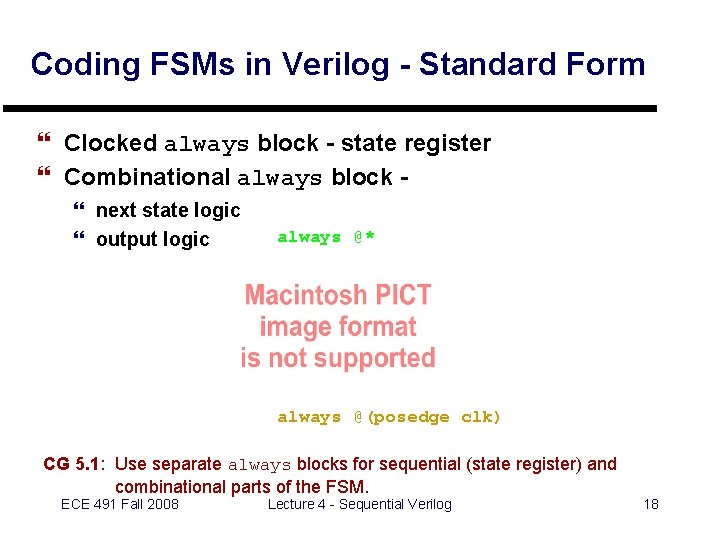
Coding FSMs in Verilog - Standard Form } Clocked always block - state register } Combinational always block } next state logic } output logic always @* always @(posedge clk) CG 5. 1: Use separate always blocks for sequential (state register) and combinational parts of the FSM. ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 18
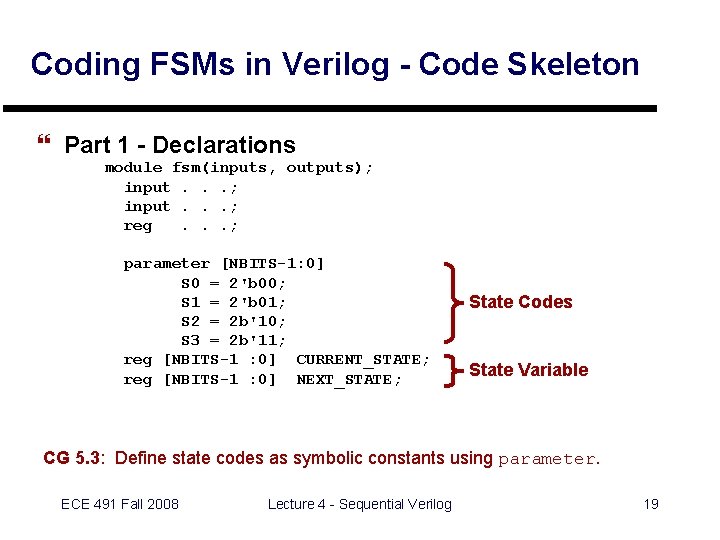
Coding FSMs in Verilog - Code Skeleton } Part 1 - Declarations module fsm(inputs, outputs); input. . . ; reg. . . ; parameter [NBITS-1: 0] S 0 = 2'b 00; S 1 = 2'b 01; S 2 = 2 b'10; S 3 = 2 b'11; reg [NBITS-1 : 0] CURRENT_STATE; reg [NBITS-1 : 0] NEXT_STATE; State Codes State Variable CG 5. 3: Define state codes as symbolic constants using parameter. ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 19
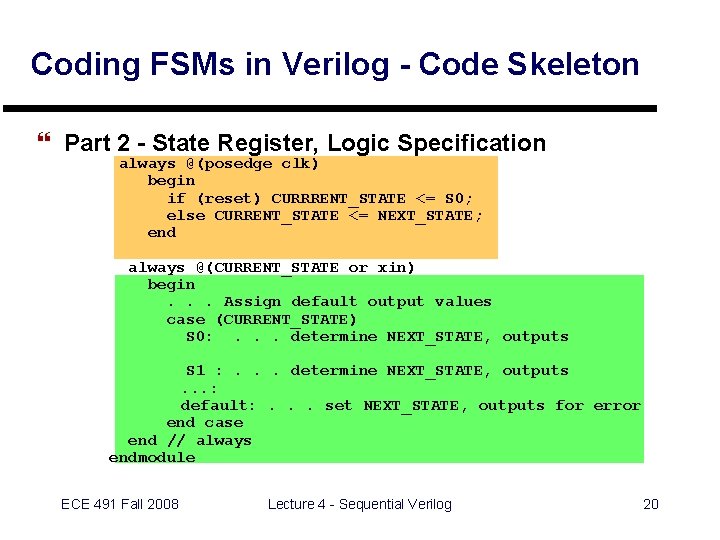
Coding FSMs in Verilog - Code Skeleton } Part 2 - State Register, Logic Specification always @(posedge clk) begin if (reset) CURRRENT_STATE <= S 0; else CURRENT_STATE <= NEXT_STATE; end always @(CURRENT_STATE or xin) begin. . . Assign default output values case (CURRENT_STATE) S 0: . . . determine NEXT_STATE, outputs S 1 : . . . determine NEXT_STATE, outputs. . . : default: . . . set NEXT_STATE, outputs for error end case end // always endmodule ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 20
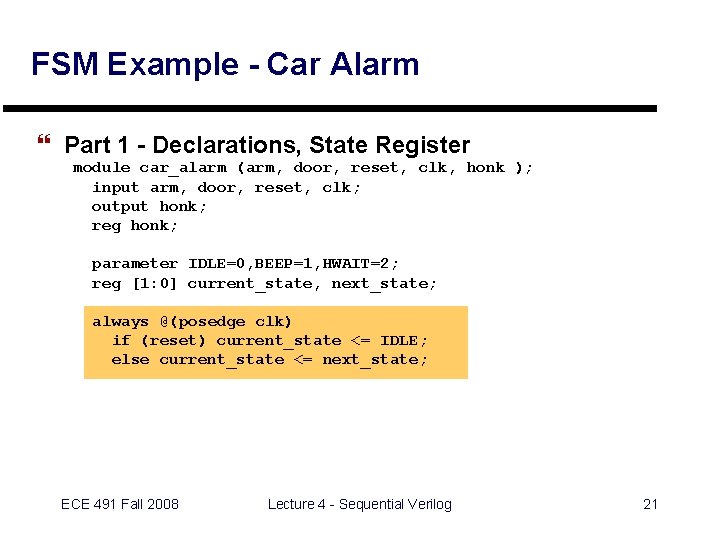
FSM Example - Car Alarm } Part 1 - Declarations, State Register module car_alarm (arm, door, reset, clk, honk ); input arm, door, reset, clk; output honk; reg honk; parameter IDLE=0, BEEP=1, HWAIT=2; reg [1: 0] current_state, next_state; always @(posedge clk) if (reset) current_state <= IDLE; else current_state <= next_state; ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 21
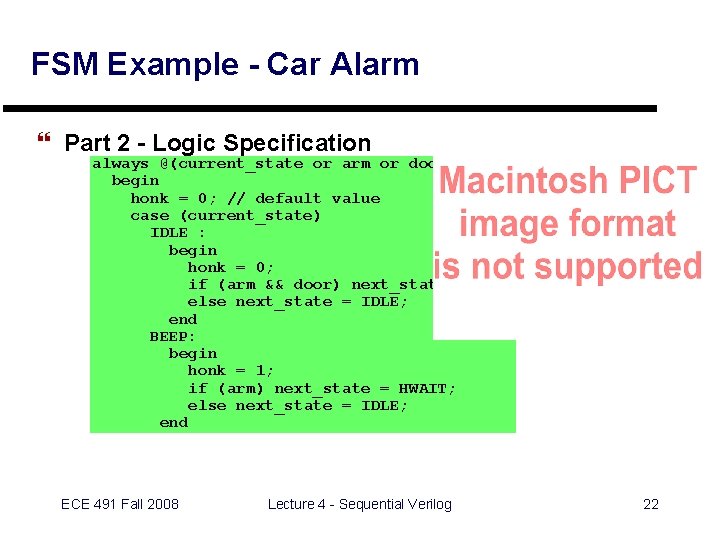
FSM Example - Car Alarm } Part 2 - Logic Specification always @(current_state or arm or door) begin honk = 0; // default value case (current_state) IDLE : begin honk = 0; if (arm && door) next_state = BEEP; else next_state = IDLE; end BEEP: begin honk = 1; if (arm) next_state = HWAIT; else next_state = IDLE; end ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 22
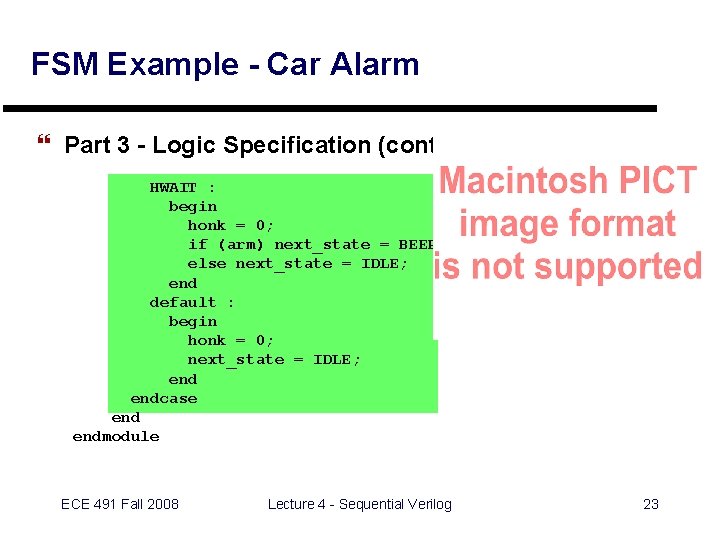
FSM Example - Car Alarm } Part 3 - Logic Specification (cont’d) HWAIT : begin honk = 0; if (arm) next_state = BEEP; else next_state = IDLE; end default : begin honk = 0; next_state = IDLE; endcase endmodule ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 23
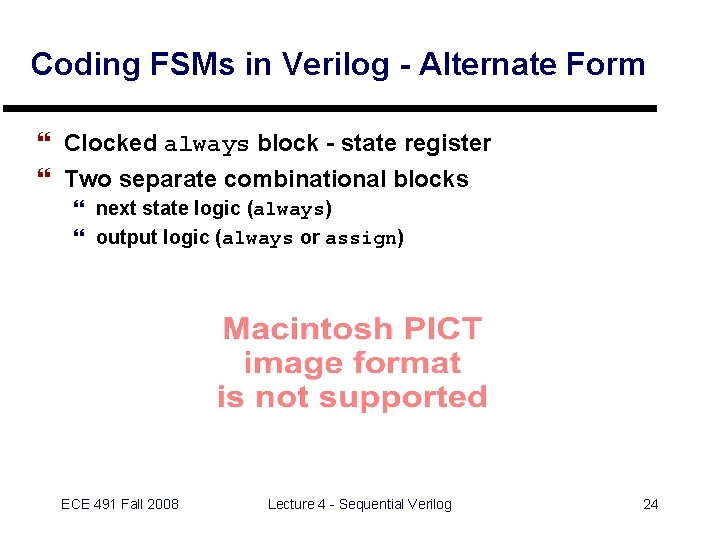
Coding FSMs in Verilog - Alternate Form } Clocked always block - state register } Two separate combinational blocks } next state logic (always) } output logic (always or assign) ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 24
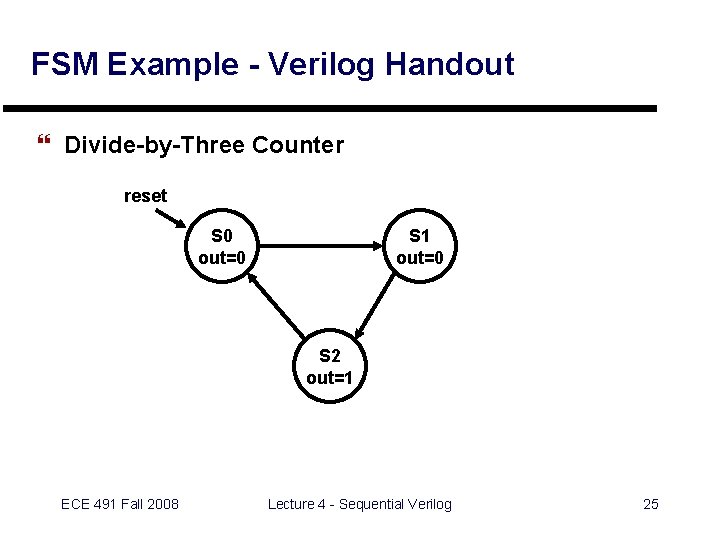
FSM Example - Verilog Handout } Divide-by-Three Counter reset S 0 out=0 S 1 out=0 S 2 out=1 ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 25
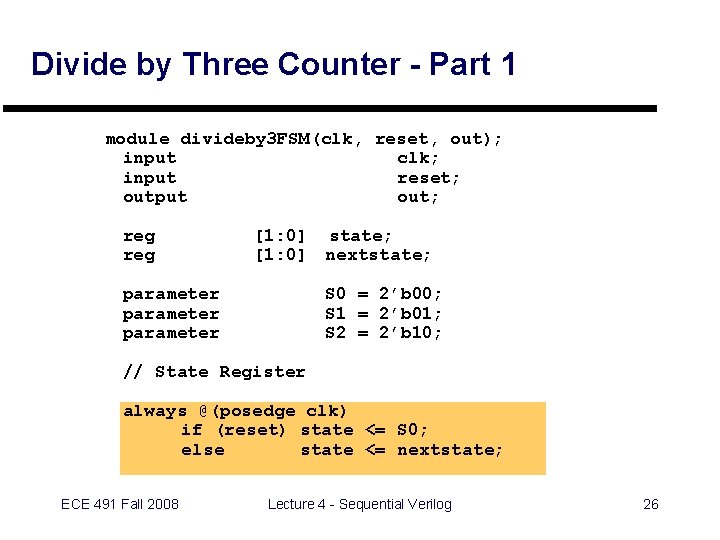
Divide by Three Counter - Part 1 module divideby 3 FSM(clk, reset, out); input clk; input reset; output out; reg [1: 0] state; [1: 0] nextstate; parameter S 0 = 2’b 00; S 1 = 2’b 01; S 2 = 2’b 10; // State Register always @(posedge clk) if (reset) state <= S 0; else state <= nextstate; ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 26
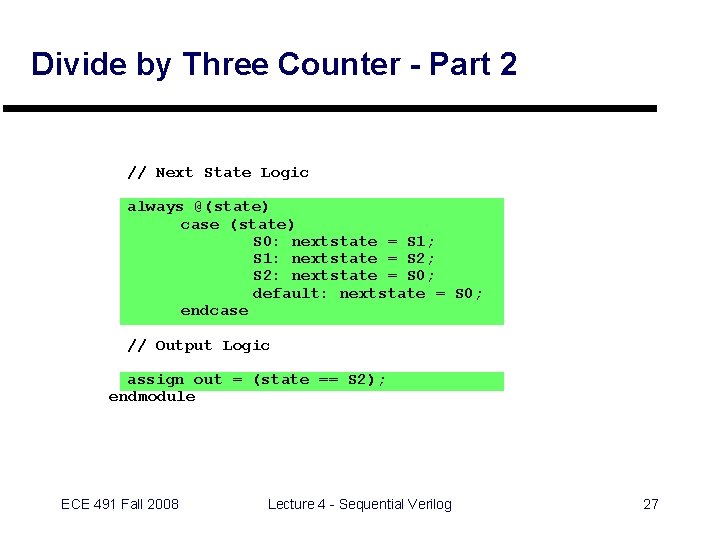
Divide by Three Counter - Part 2 // Next State Logic always @(state) case (state) S 0: nextstate = S 1; S 1: nextstate = S 2; S 2: nextstate = S 0; default: nextstate = S 0; endcase // Output Logic assign out = (state == S 2); endmodule ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 27
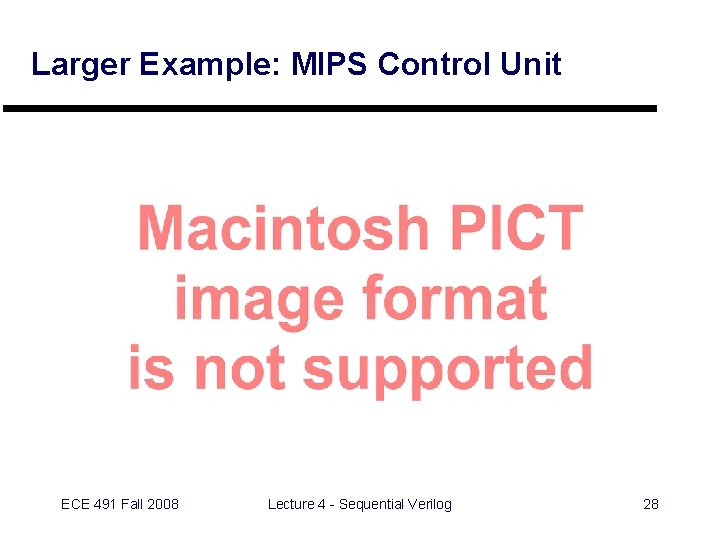
Larger Example: MIPS Control Unit ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 28
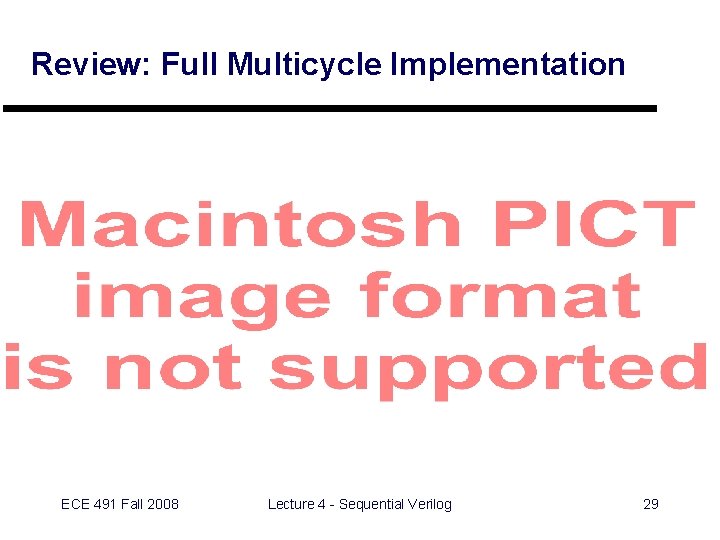
Review: Full Multicycle Implementation ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 29
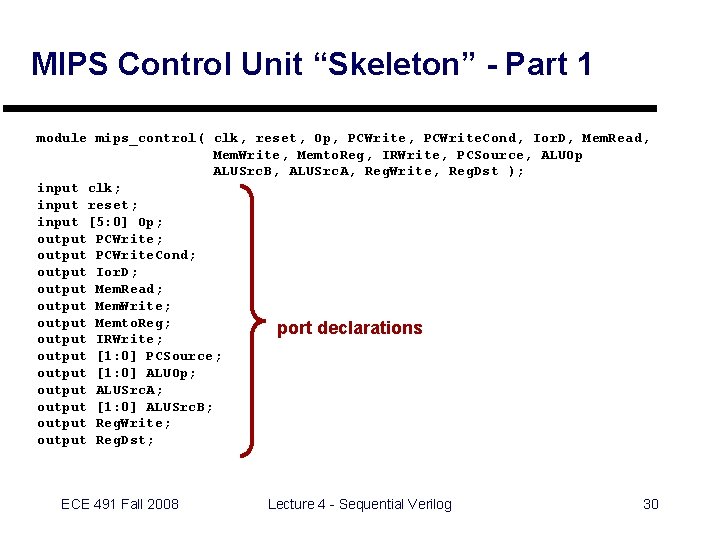
MIPS Control Unit “Skeleton” - Part 1 module mips_control( clk, reset, Op, PCWrite. Cond, Ior. D, Mem. Read, Mem. Write, Memto. Reg, IRWrite, PCSource, ALUOp ALUSrc. B, ALUSrc. A, Reg. Write, Reg. Dst ); input clk; input reset; input [5: 0] Op; output PCWrite. Cond; output Ior. D; output Mem. Read; output Mem. Write; output Memto. Reg; port declarations output IRWrite; output [1: 0] PCSource; output [1: 0] ALUOp; output ALUSrc. A; output [1: 0] ALUSrc. B; output Reg. Write; output Reg. Dst; ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 30
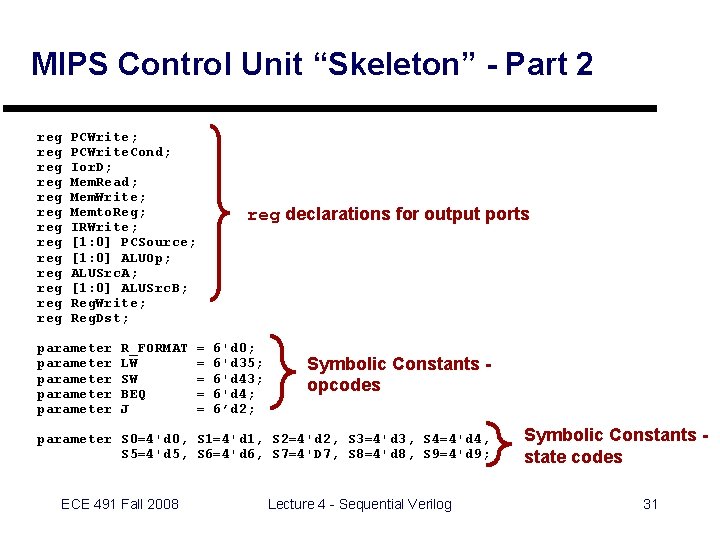
MIPS Control Unit “Skeleton” - Part 2 reg reg reg reg PCWrite; PCWrite. Cond; Ior. D; Mem. Read; Mem. Write; Memto. Reg; IRWrite; [1: 0] PCSource; [1: 0] ALUOp; ALUSrc. A; [1: 0] ALUSrc. B; Reg. Write; Reg. Dst; parameter parameter R_FORMAT LW SW BEQ J = = = reg declarations for output ports 6'd 0; 6'd 35; 6'd 43; 6'd 4; 6’d 2; Symbolic Constants opcodes parameter S 0=4'd 0, S 1=4'd 1, S 2=4'd 2, S 3=4'd 3, S 4=4'd 4, S 5=4'd 5, S 6=4'd 6, S 7=4'D 7, S 8=4'd 8, S 9=4'd 9; ECE 491 Fall 2008 Lecture 4 - Sequential Verilog Symbolic Constants state codes 31
![MIPS Control Unit Part 3 reg 3 0 currentstate nextstate always negedge clk MIPS Control Unit - Part 3 reg [3: 0] current_state, next_state; always @(negedge clk)](https://slidetodoc.com/presentation_image_h2/641281e45dab0ad713902a9fe63852bc/image-32.jpg)
MIPS Control Unit - Part 3 reg [3: 0] current_state, next_state; always @(negedge clk) begin if (reset) current_state <= S 0; else current_state <= next_state; end always @(current_state or Op) begin // default values PCWrite = 1'b 0; PCWrite. Cond = 1'b 0; Ior. D = 1'bx; Mem. Read = 1'b 0; Mem. Write = 1'b 0; Default Memto. Reg = 1'bx; IRWrite = 1'b 0; Values PCSource = 2'bxx; ALUOp = 2'bxx; ALUSrc. A = 1'bx; ALUSrc. B = 2'bxx; Reg. Write = 1'b 0; Reg. Dst = 1'bx; More Default Values case (current_state) S 0: begin Mem. Read = 1'b 1; ALUSrc. A = 1'b 0; Ior. D = 1'b 0; IRWrite = 1'b 1; ALUSrc. B = 2'b 01; State S 0 ALUOp = 2'b 00; PCWrite = 1'b 1; PCSource = 2'b 00; next_state = S 1; end … (More states endcase end here) endmodule Full example at http: //cadapplets. lafayette. edu/ece 313/examples/mips_multi/control_multi. v ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 32
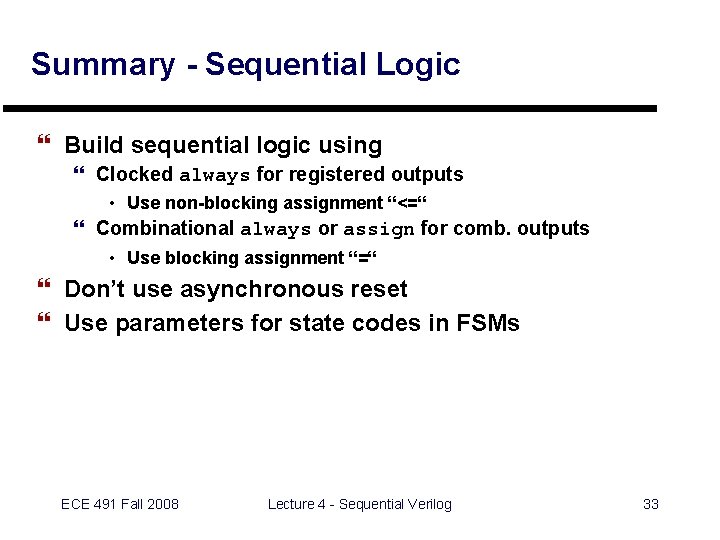
Summary - Sequential Logic } Build sequential logic using } Clocked always for registered outputs • Use non-blocking assignment “<=“ } Combinational always or assign for comb. outputs • Use blocking assignment “=“ } Don’t use asynchronous reset } Use parameters for state codes in FSMs ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 33
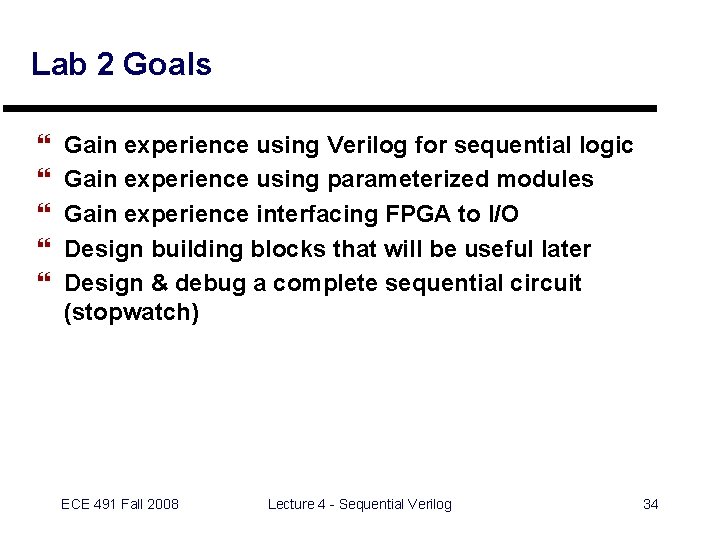
Lab 2 Goals } } } Gain experience using Verilog for sequential logic Gain experience using parameterized modules Gain experience interfacing FPGA to I/O Design building blocks that will be useful later Design & debug a complete sequential circuit (stopwatch) ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 34
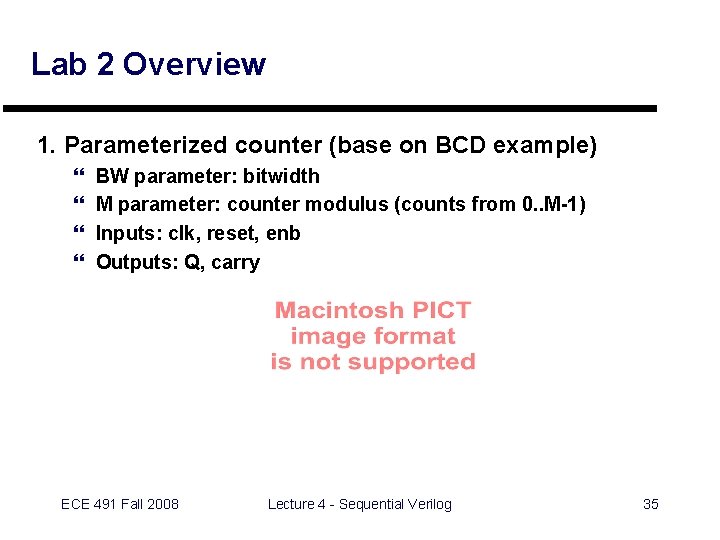
Lab 2 Overview 1. Parameterized counter (base on BCD example) } } BW parameter: bitwidth M parameter: counter modulus (counts from 0. . M-1) Inputs: clk, reset, enb Outputs: Q, carry ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 35
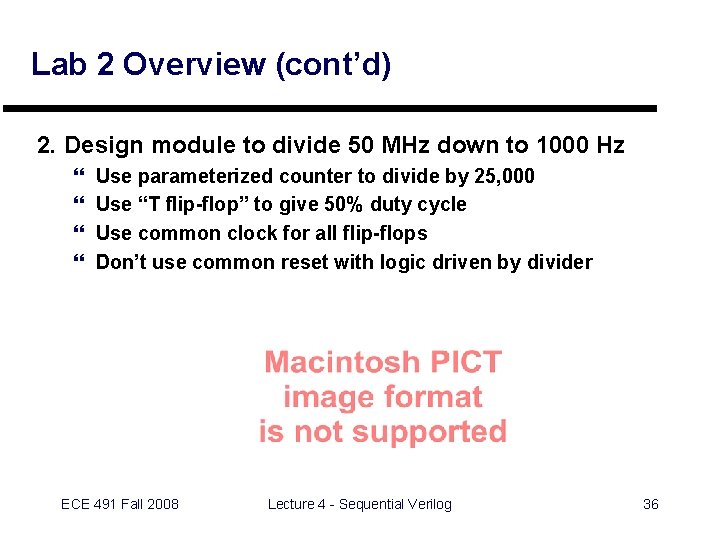
Lab 2 Overview (cont’d) 2. Design module to divide 50 MHz down to 1000 Hz } } Use parameterized counter to divide by 25, 000 Use “T flip-flop” to give 50% duty cycle Use common clock for all flip-flops Don’t use common reset with logic driven by divider ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 36
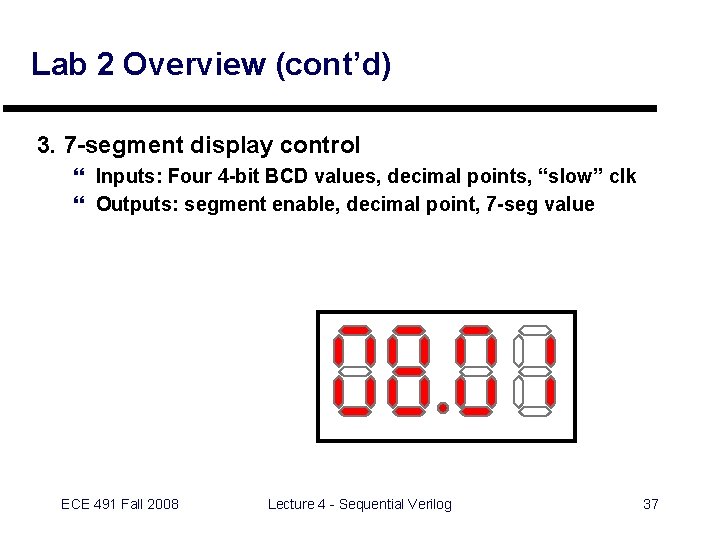
Lab 2 Overview (cont’d) 3. 7 -segment display control } Inputs: Four 4 -bit BCD values, decimal points, “slow” clk } Outputs: segment enable, decimal point, 7 -seg value ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 37
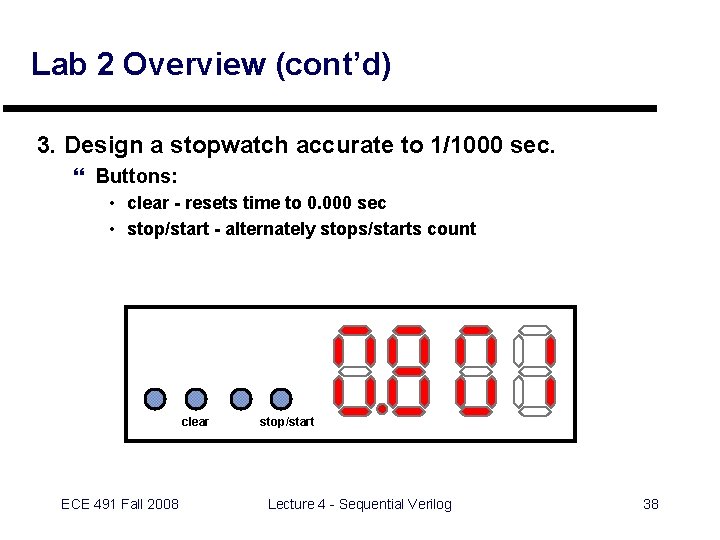
Lab 2 Overview (cont’d) 3. Design a stopwatch accurate to 1/1000 sec. } Buttons: • clear - resets time to 0. 000 sec • stop/start - alternately stops/starts count clear ECE 491 Fall 2008 stop/start Lecture 4 - Sequential Verilog 38
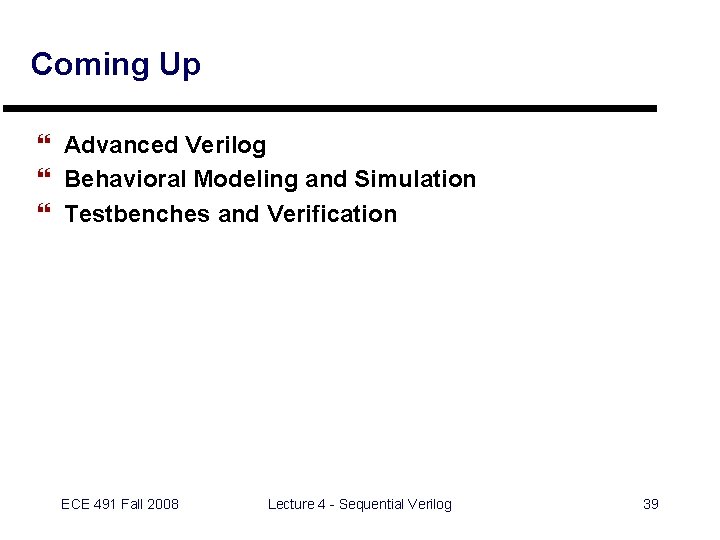
Coming Up } Advanced Verilog } Behavioral Modeling and Simulation } Testbenches and Verification ECE 491 Fall 2008 Lecture 4 - Sequential Verilog 39