DoWhile Statement Is a looping control structure in
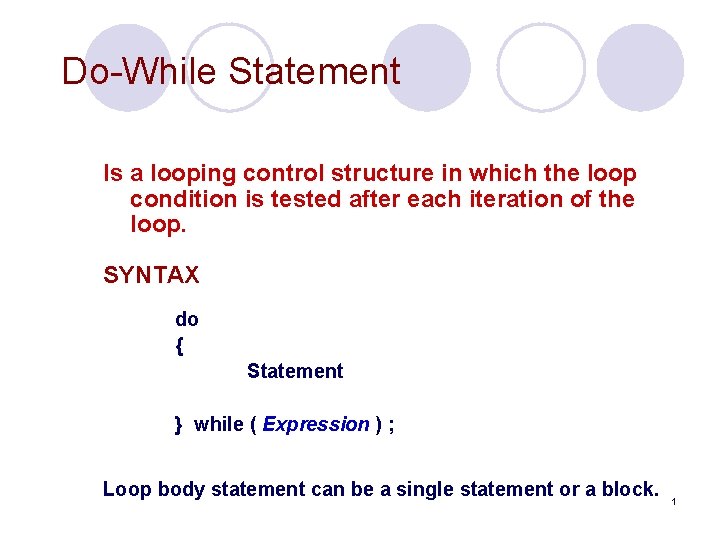
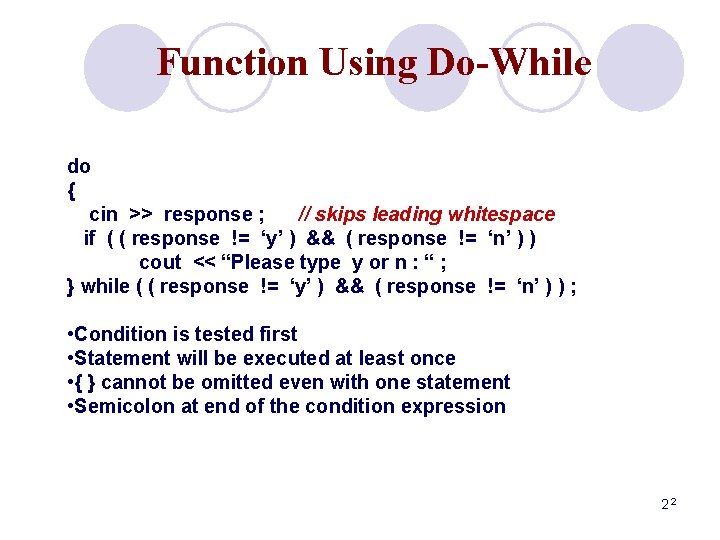
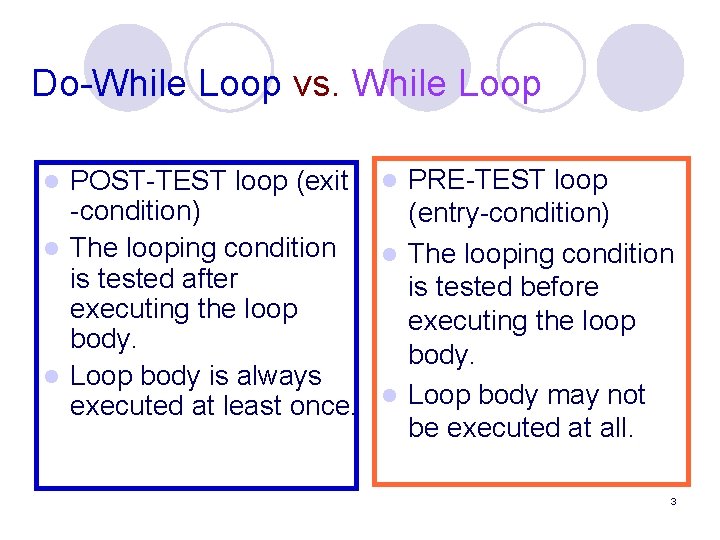
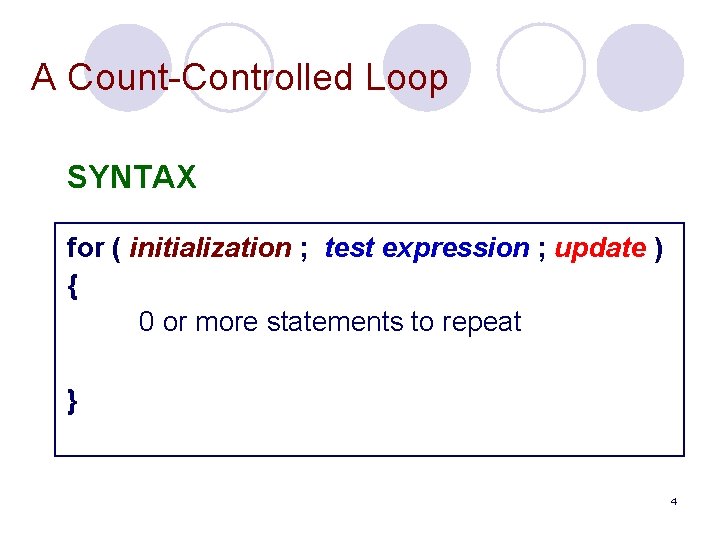
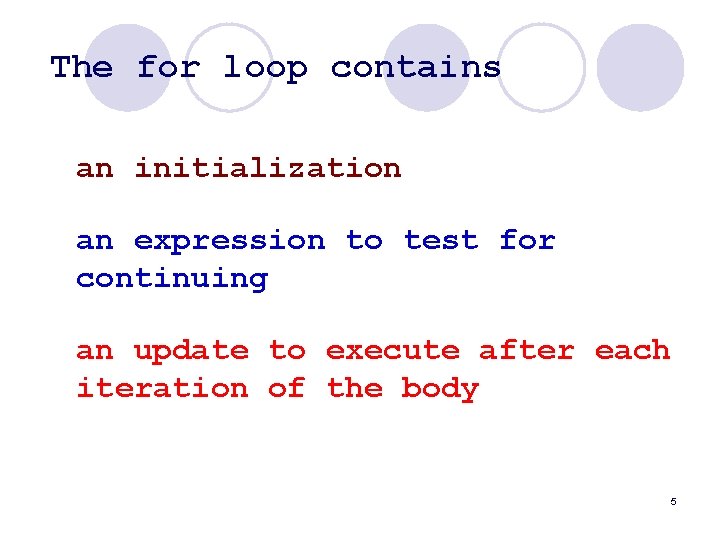
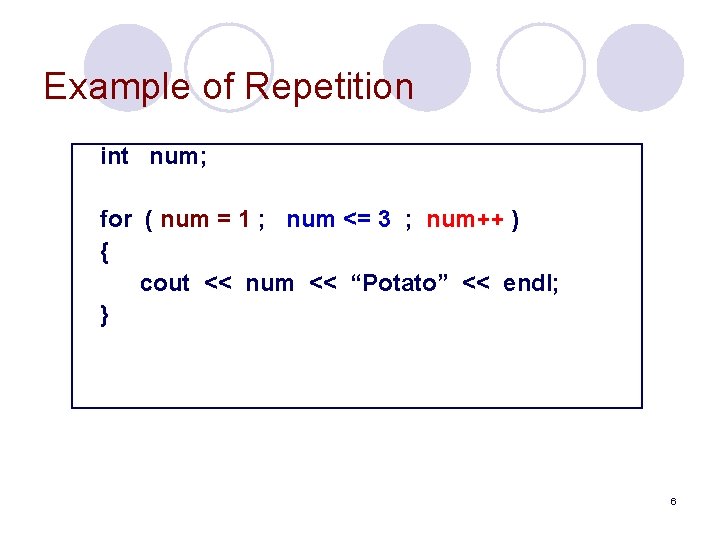
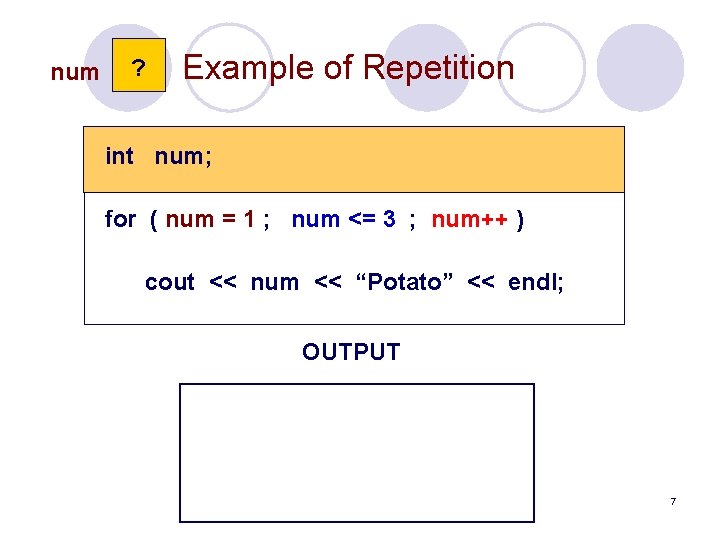
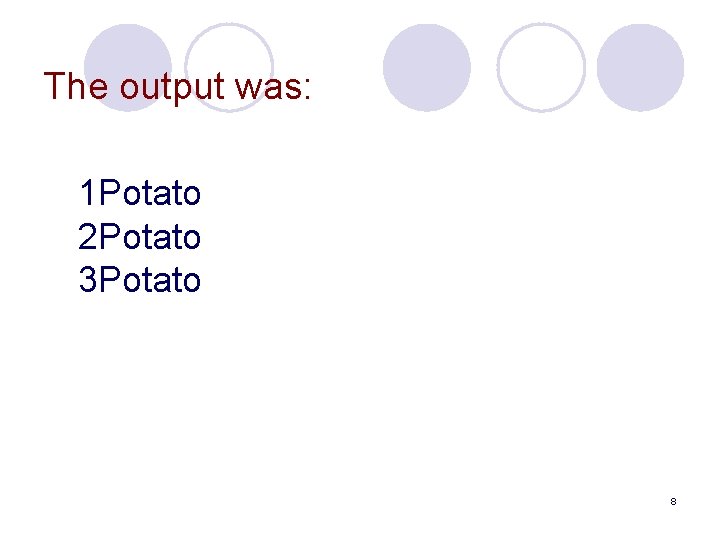
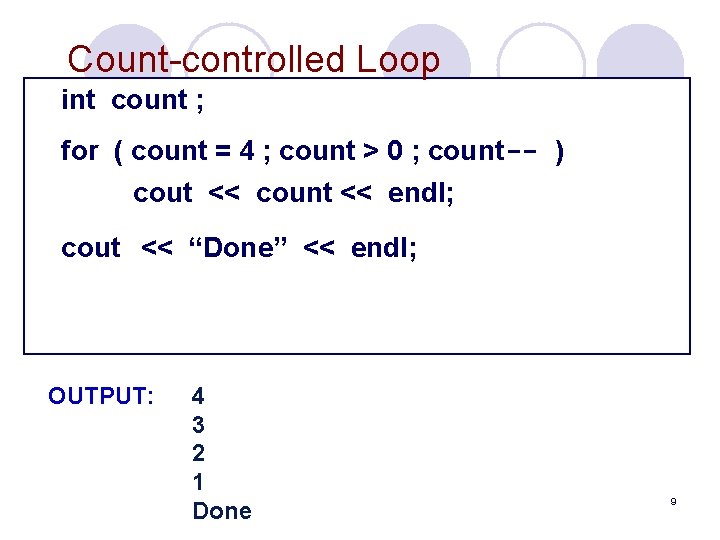
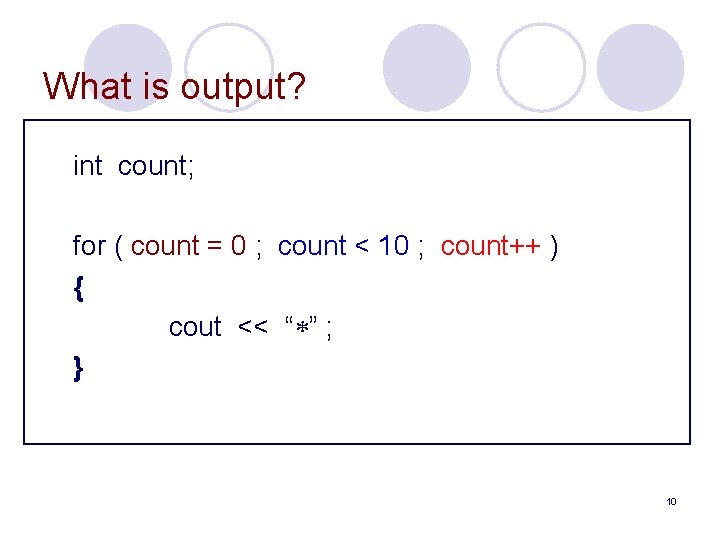
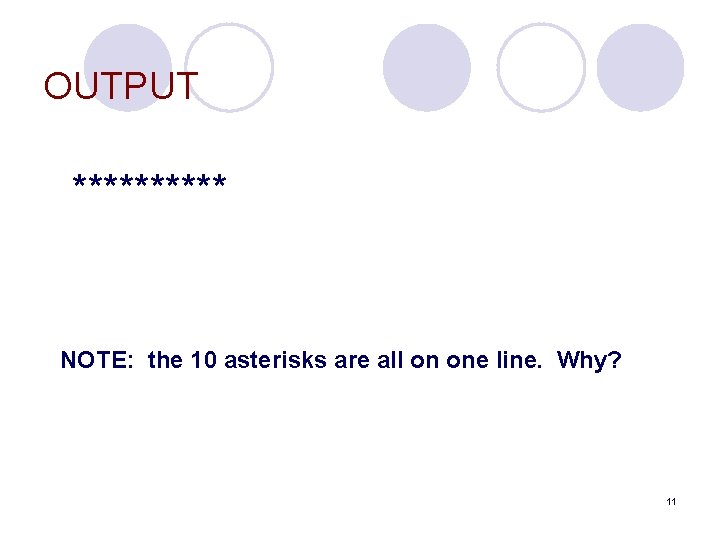
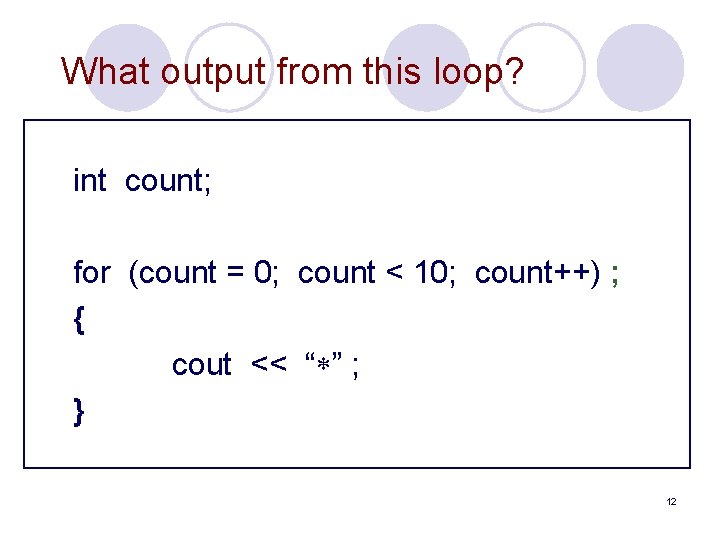
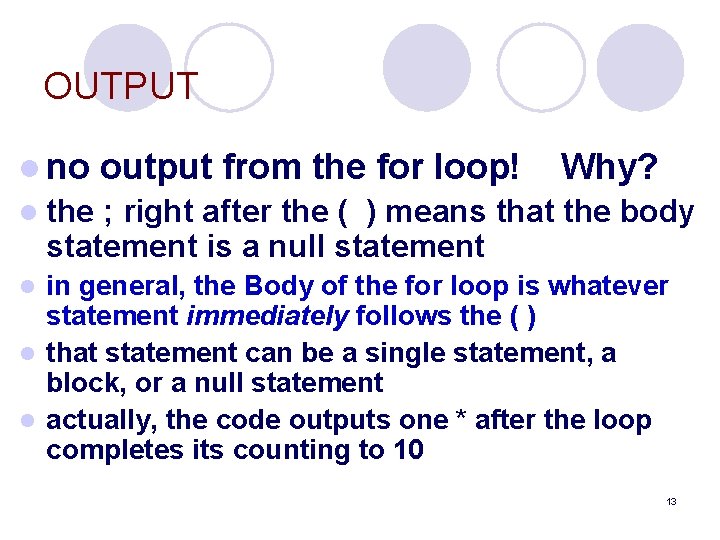
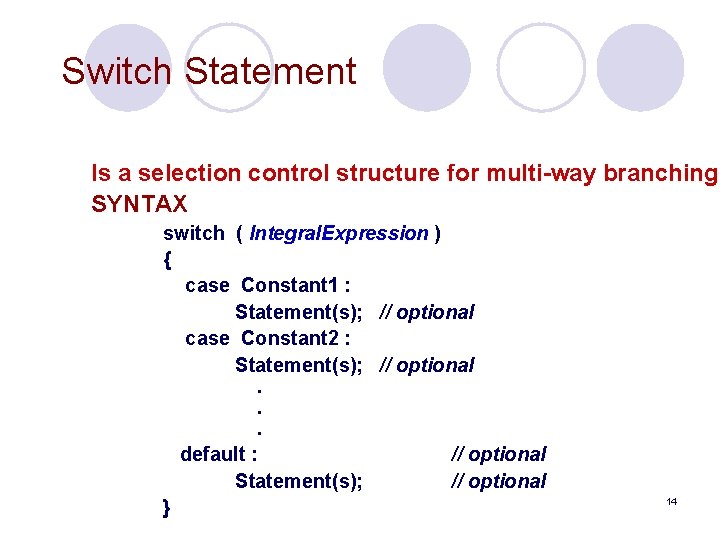
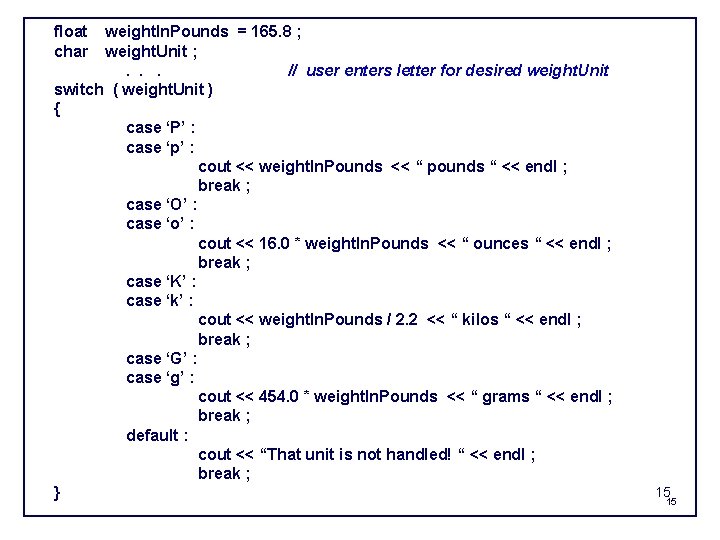
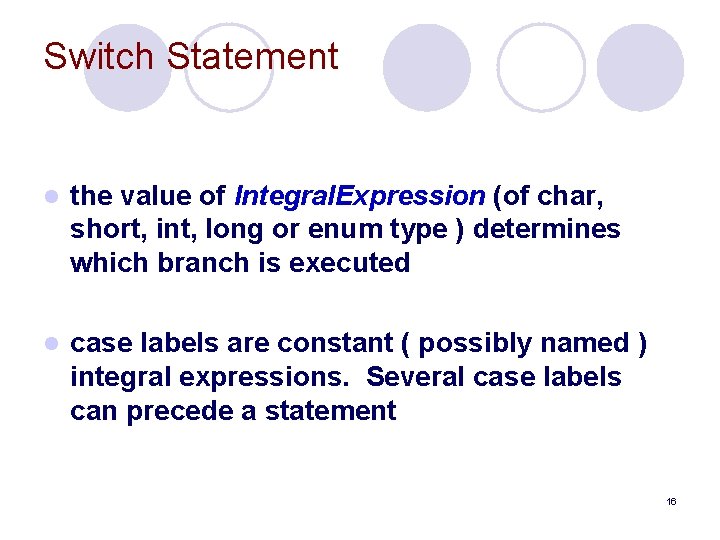
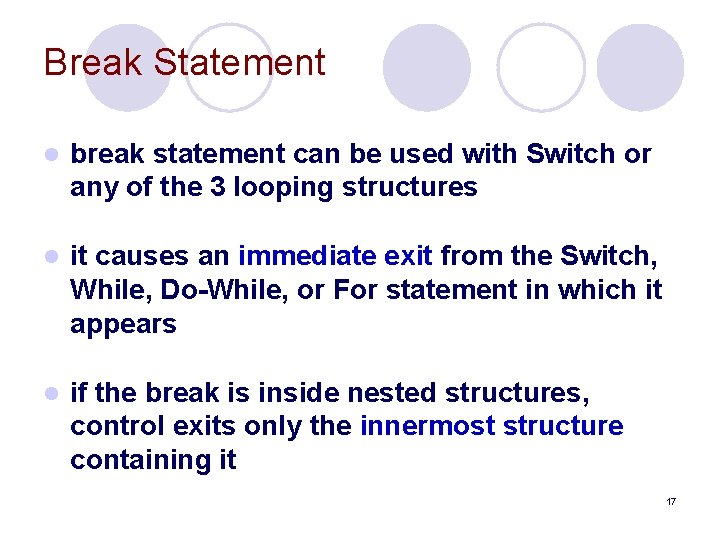
- Slides: 17
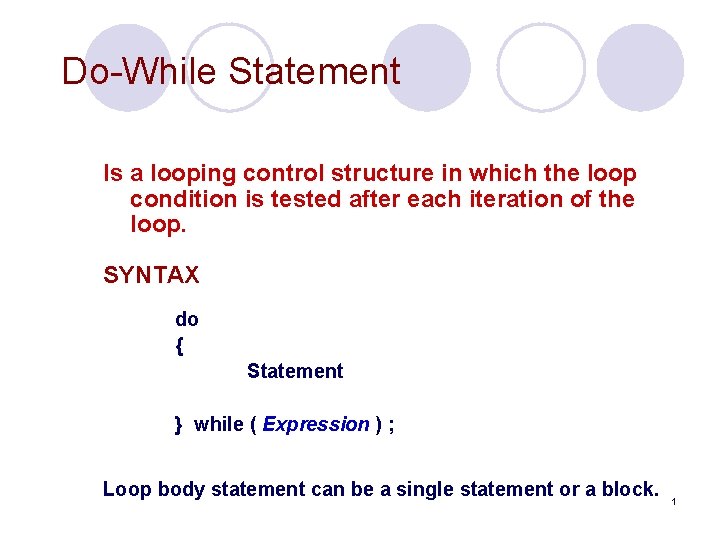
Do-While Statement Is a looping control structure in which the loop condition is tested after each iteration of the loop. SYNTAX do { Statement } while ( Expression ) ; Loop body statement can be a single statement or a block. 1
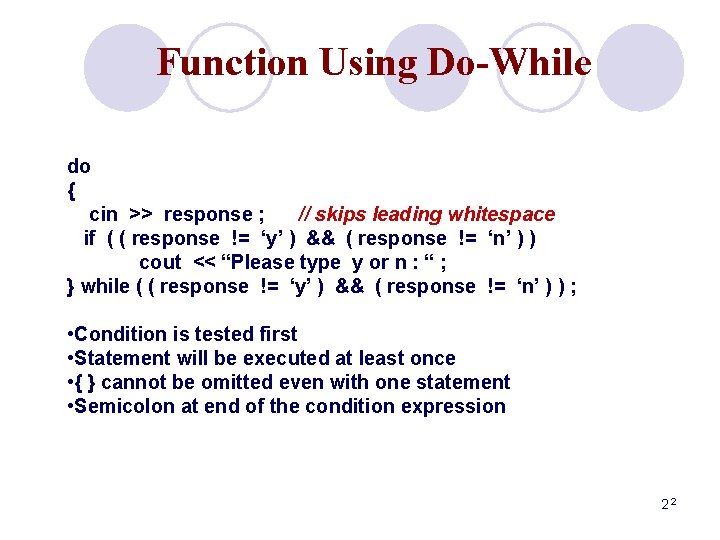
Function Using Do-While do { cin >> response ; // skips leading whitespace if ( ( response != ‘y’ ) && ( response != ‘n’ ) ) cout << “Please type y or n : “ ; } while ( ( response != ‘y’ ) && ( response != ‘n’ ) ) ; • Condition is tested first • Statement will be executed at least once • { } cannot be omitted even with one statement • Semicolon at end of the condition expression 22
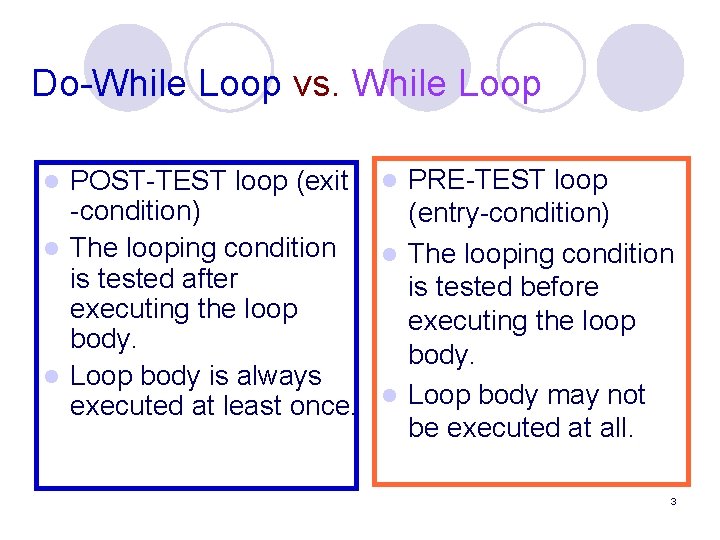
Do-While Loop vs. While Loop POST-TEST loop (exit l PRE-TEST loop -condition) (entry-condition) l The looping condition is tested after is tested before executing the loop body. l Loop body is always executed at least once. l Loop body may not be executed at all. l 3
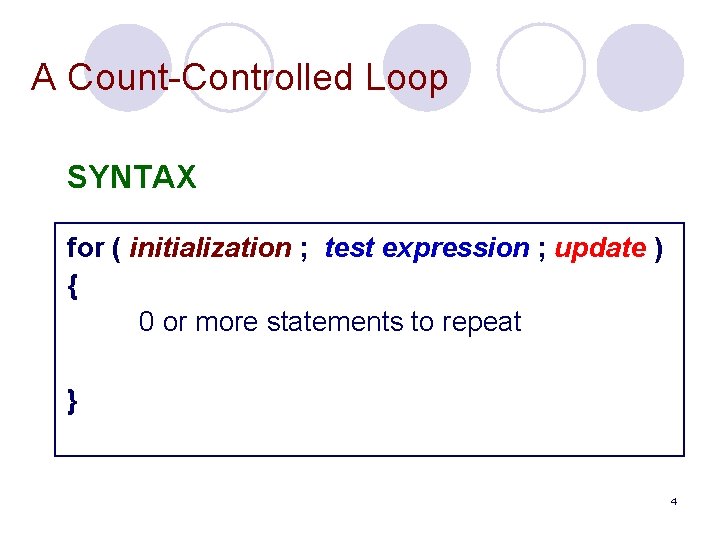
A Count-Controlled Loop SYNTAX for ( initialization ; test expression ; update ) { 0 or more statements to repeat } 4
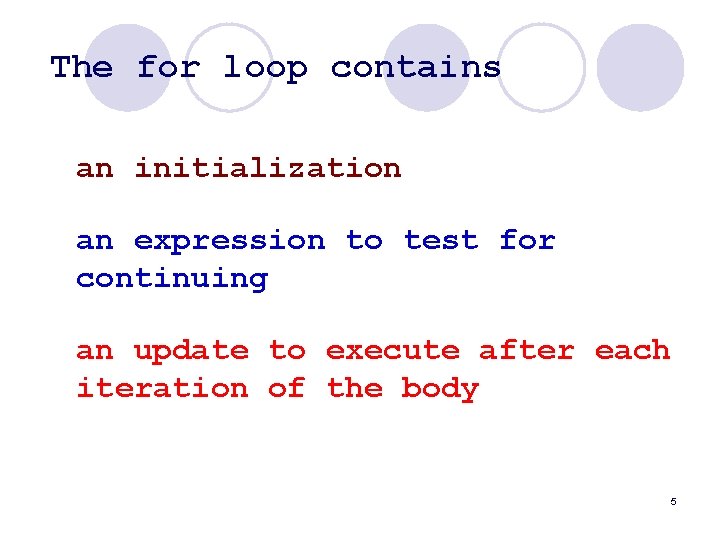
The for loop contains an initialization an expression to test for continuing an update to execute after each iteration of the body 5
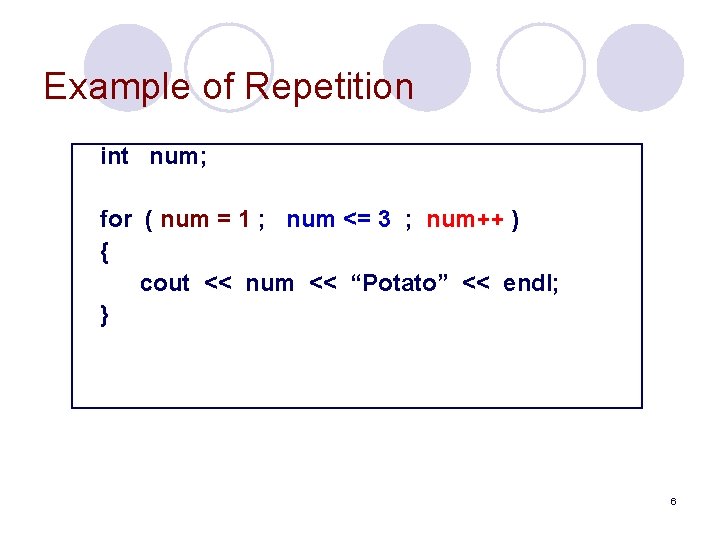
Example of Repetition int num; for ( num = 1 ; num <= 3 ; num++ ) { cout << num << “Potato” << endl; } 6
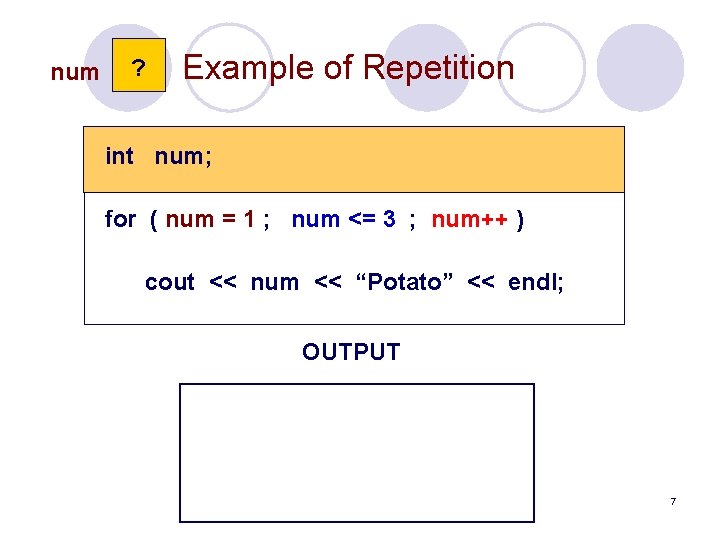
num ? Example of Repetition int num; for ( num = 1 ; num <= 3 ; num++ ) cout << num << “Potato” << endl; OUTPUT 7
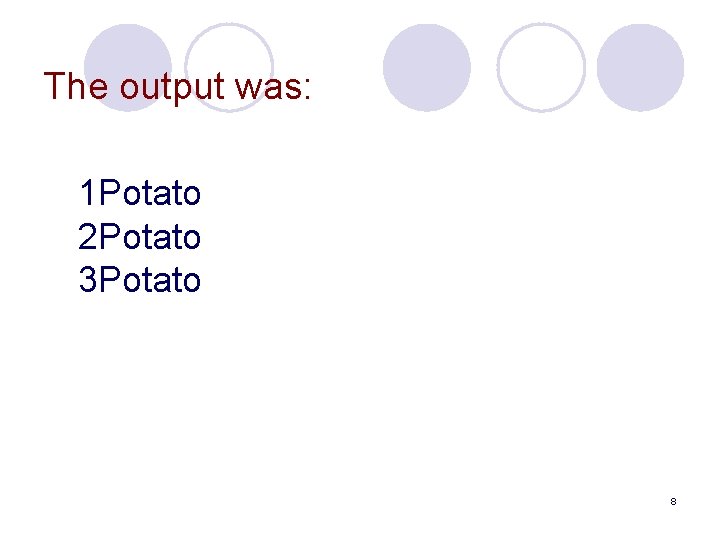
The output was: 1 Potato 2 Potato 3 Potato 8
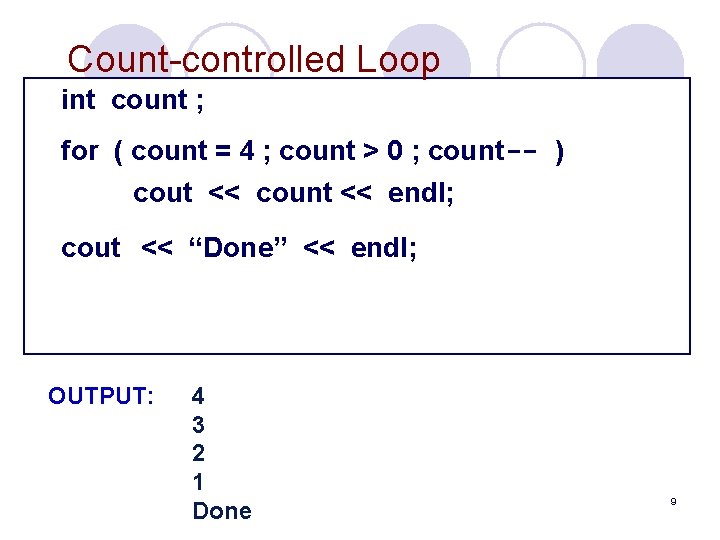
Count-controlled Loop int count ; for ( count = 4 ; count > 0 ; count-- ) cout << count << endl; cout << “Done” << endl; OUTPUT: 4 3 2 1 Done 9
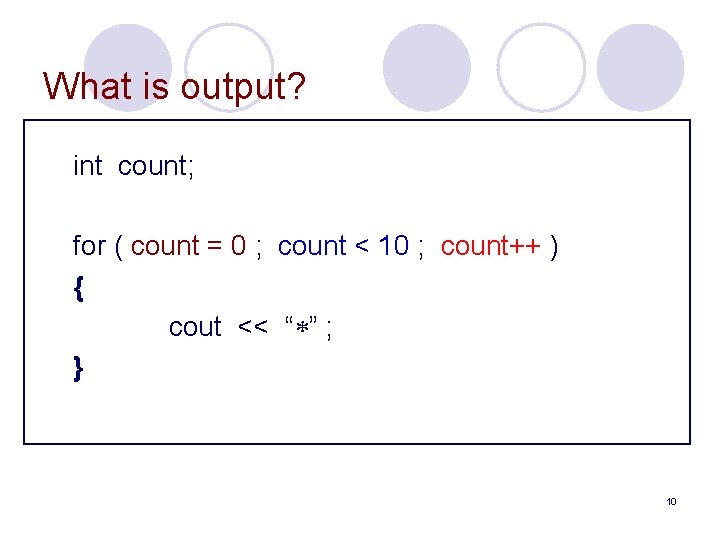
What is output? int count; for ( count = 0 ; count < 10 ; count++ ) { cout << “*” ; } 10
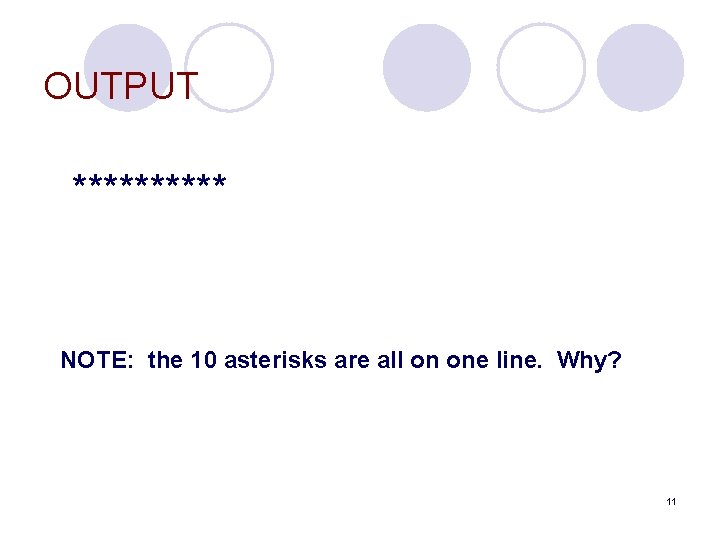
OUTPUT ***** NOTE: the 10 asterisks are all on one line. Why? 11
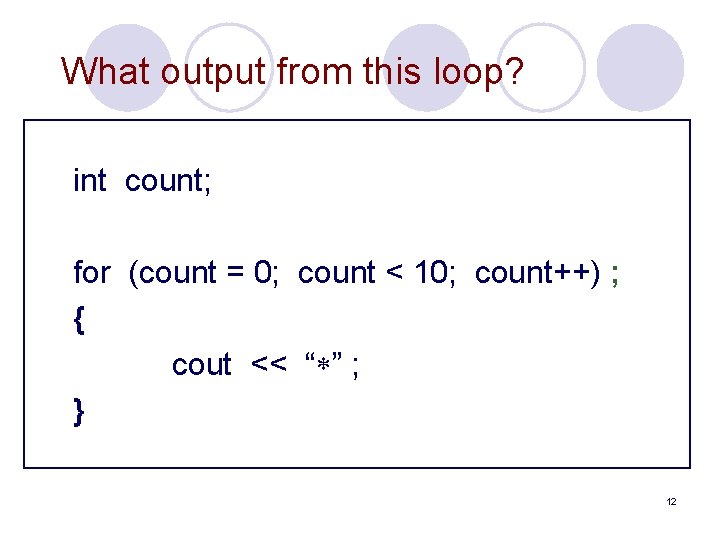
What output from this loop? int count; for (count = 0; count < 10; count++) ; { cout << “*” ; } 12
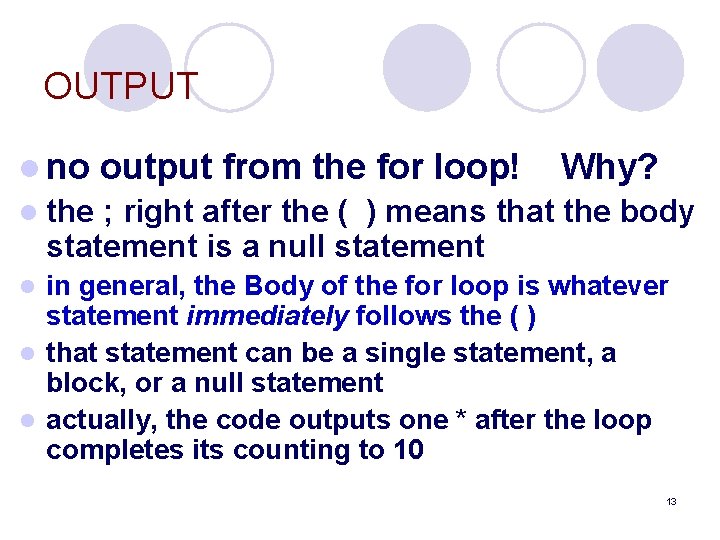
OUTPUT l no output from the for loop! Why? l the ; right after the ( ) means that the body statement is a null statement in general, the Body of the for loop is whatever statement immediately follows the ( ) l that statement can be a single statement, a block, or a null statement l actually, the code outputs one * after the loop completes its counting to 10 l 13
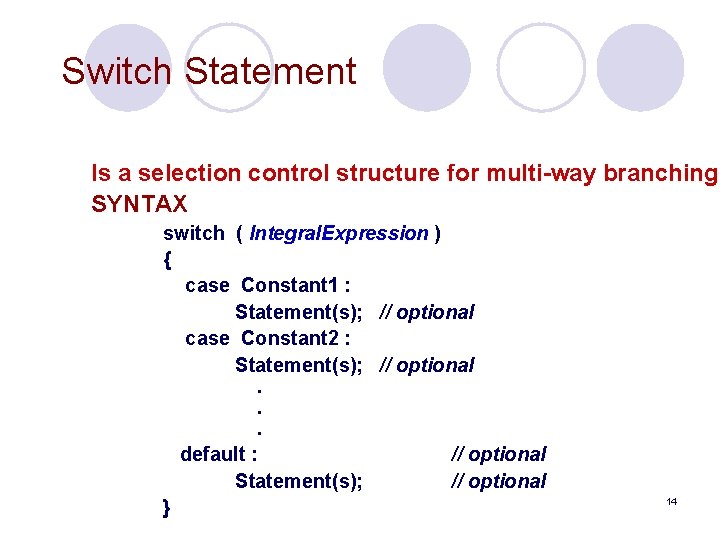
Switch Statement Is a selection control structure for multi-way branching. SYNTAX switch ( Integral. Expression ) { case Constant 1 : Statement(s); // optional case Constant 2 : Statement(s); // optional. . . default : Statement(s); } // optional 14
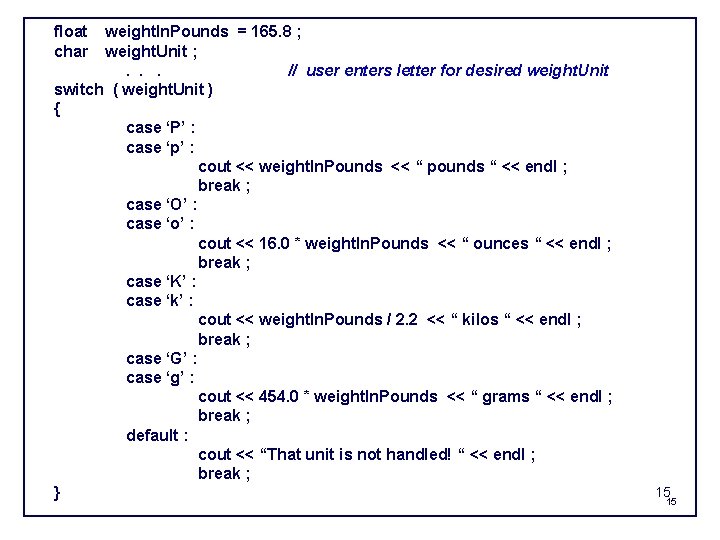
float char weight. In. Pounds = 165. 8 ; weight. Unit ; . . . // user enters letter for desired weight. Unit switch ( weight. Unit ) { case ‘P’ : case ‘p’ : cout << weight. In. Pounds << “ pounds “ << endl ; break ; case ‘O’ : case ‘o’ : cout << 16. 0 * weight. In. Pounds << “ ounces “ << endl ; break ; case ‘K’ : case ‘k’ : cout << weight. In. Pounds / 2. 2 << “ kilos “ << endl ; break ; case ‘G’ : case ‘g’ : cout << 454. 0 * weight. In. Pounds << “ grams “ << endl ; break ; default : cout << “That unit is not handled! “ << endl ; break ; } 15 15
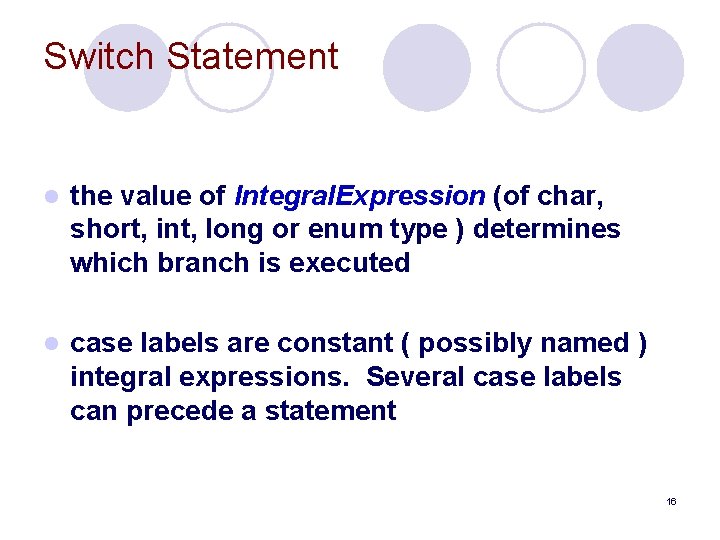
Switch Statement l the value of Integral. Expression (of char, short, int, long or enum type ) determines which branch is executed l case labels are constant ( possibly named ) integral expressions. Several case labels can precede a statement 16
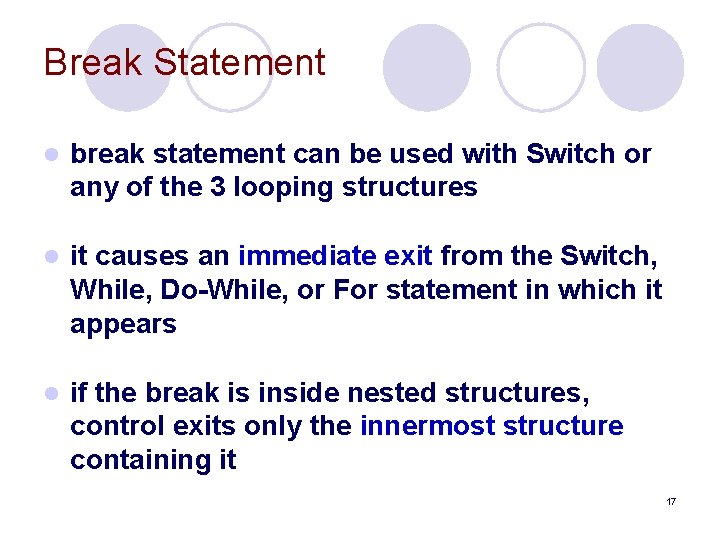
Break Statement l break statement can be used with Switch or any of the 3 looping structures l it causes an immediate exit from the Switch, While, Do-While, or For statement in which it appears l if the break is inside nested structures, control exits only the innermost structure containing it 17