DMT 245 Introduction to Microcontroller Chapter 2 8051
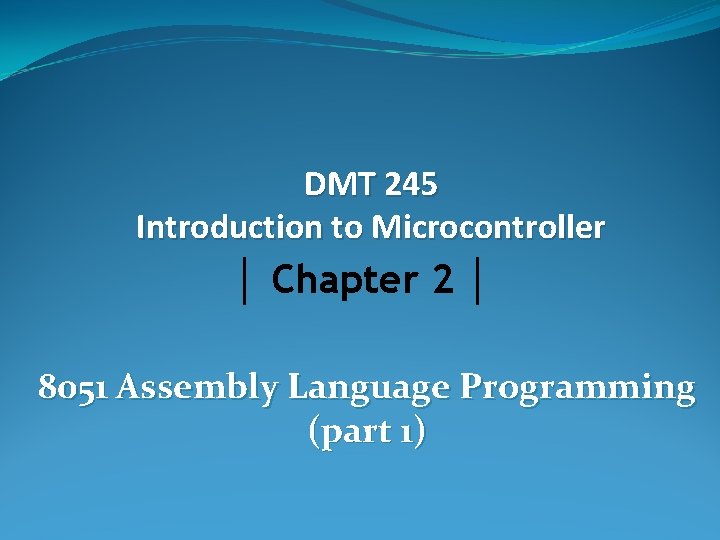
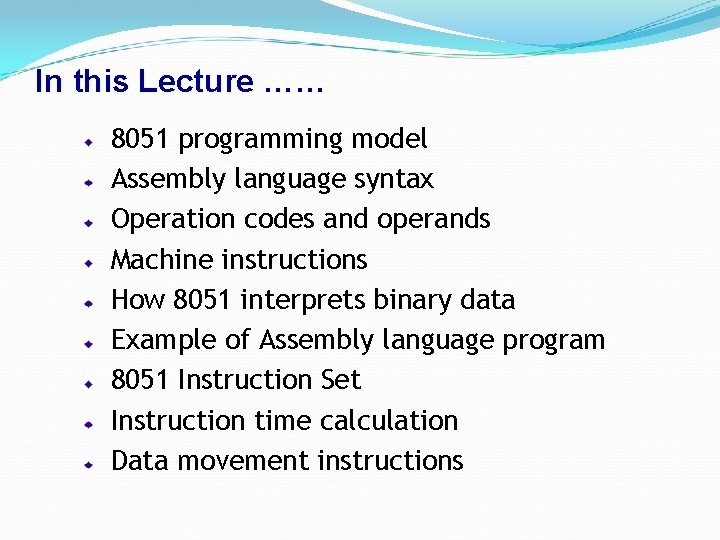
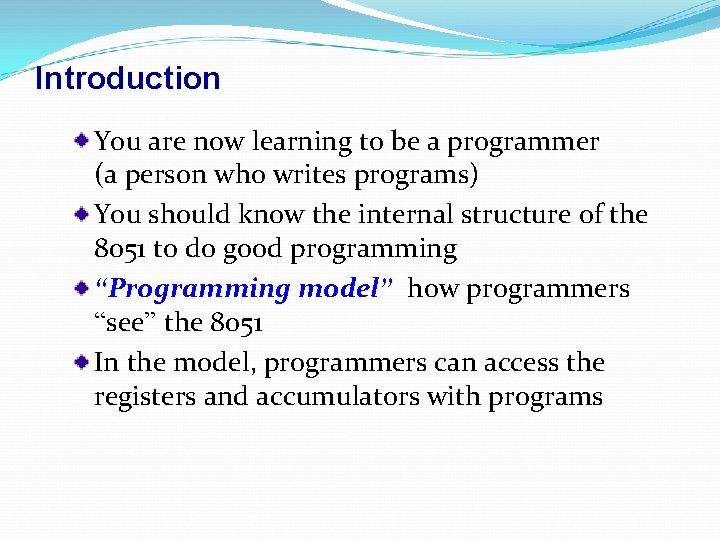
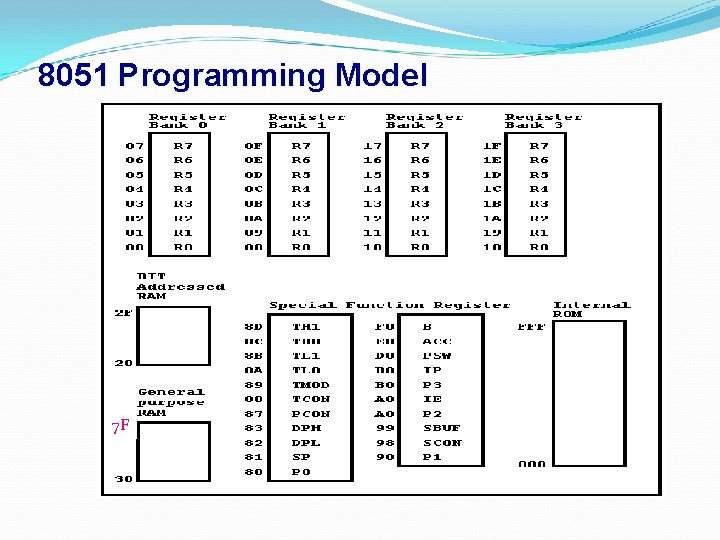
![Assembly Language Syntax = format/rule [label: ] mnemonic [operands] [; comment] Items in square Assembly Language Syntax = format/rule [label: ] mnemonic [operands] [; comment] Items in square](https://slidetodoc.com/presentation_image_h2/6502a6a501d2fc78b9f4ed367dc02a33/image-5.jpg)
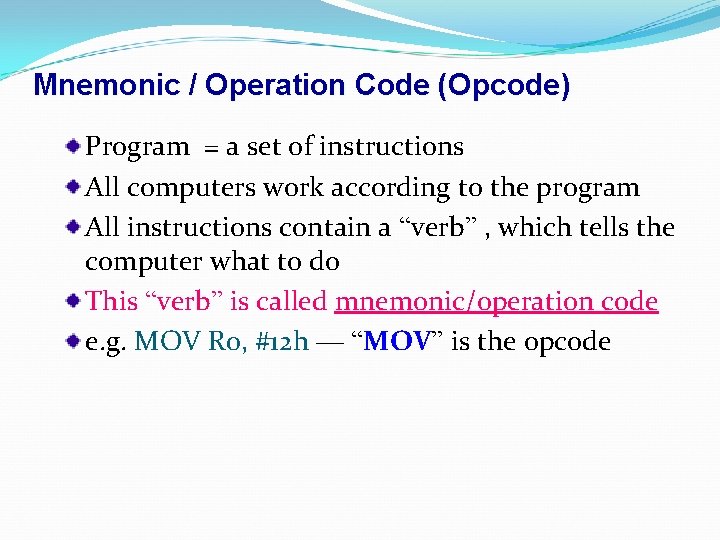
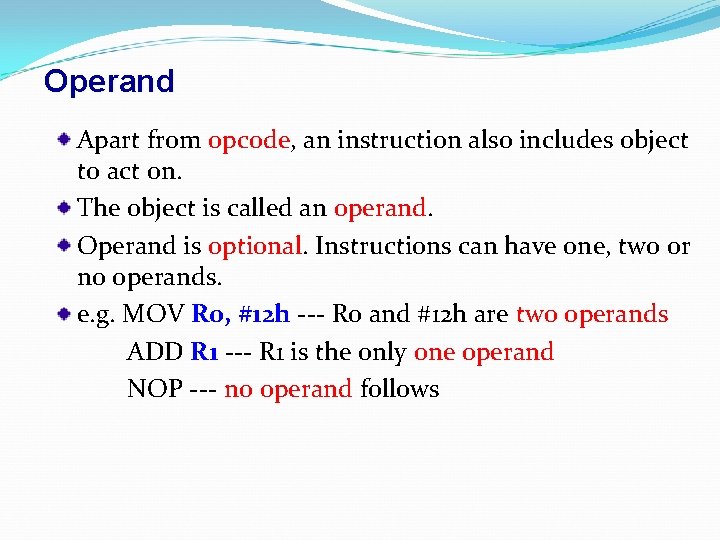
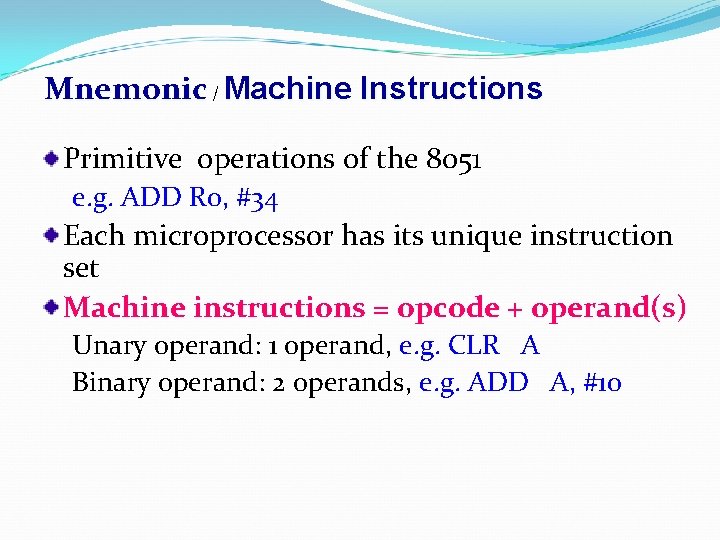
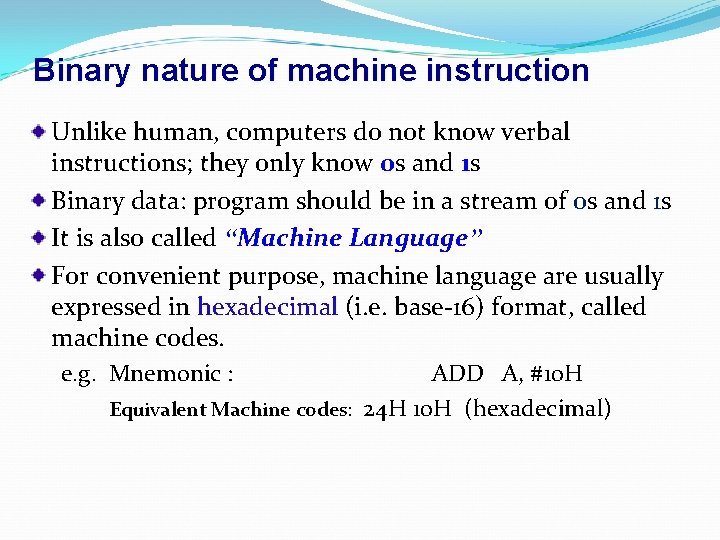
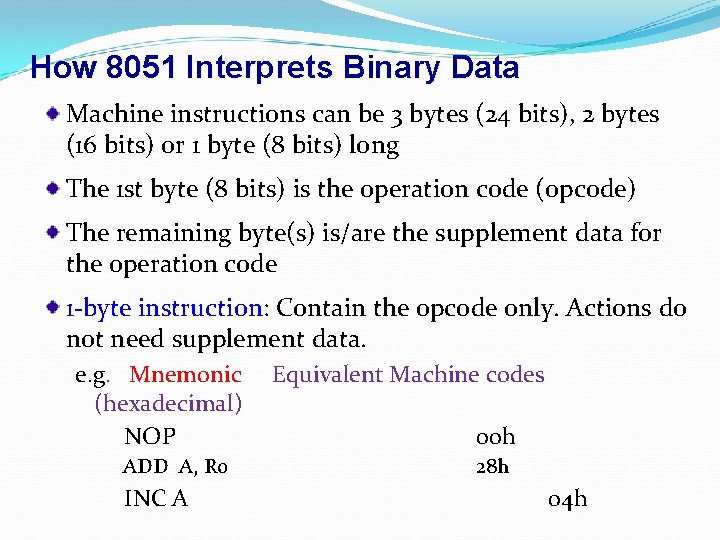
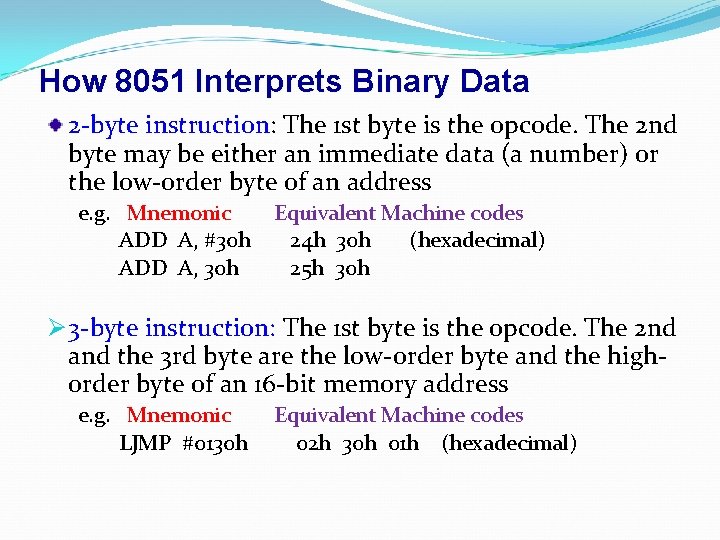
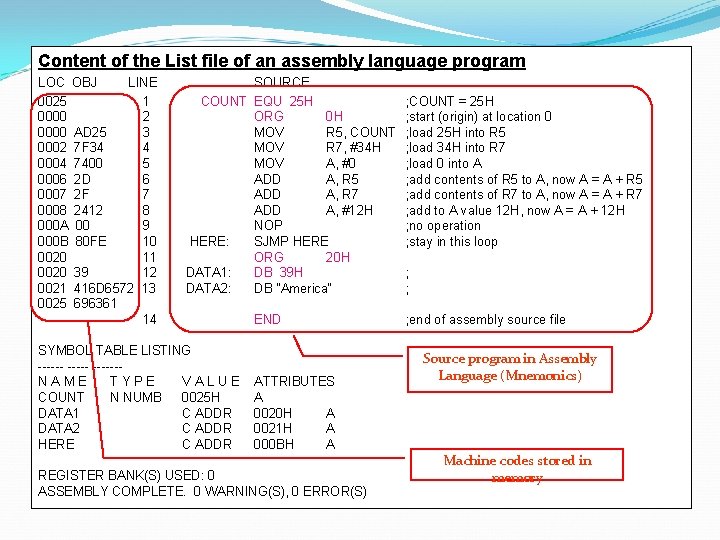
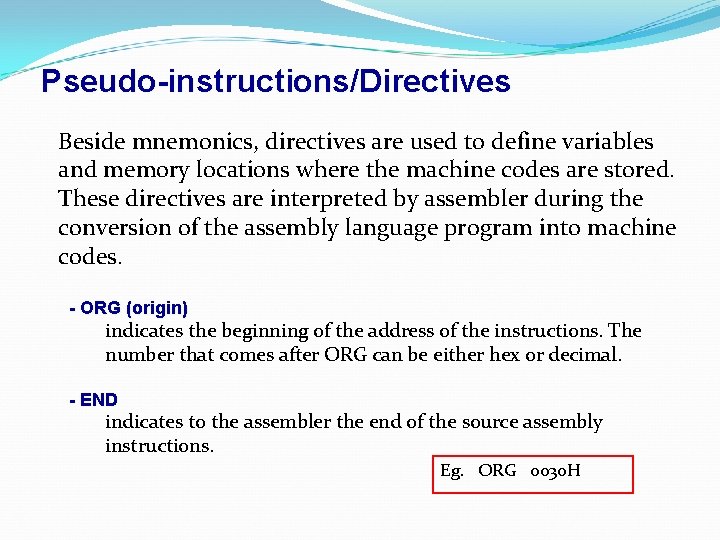
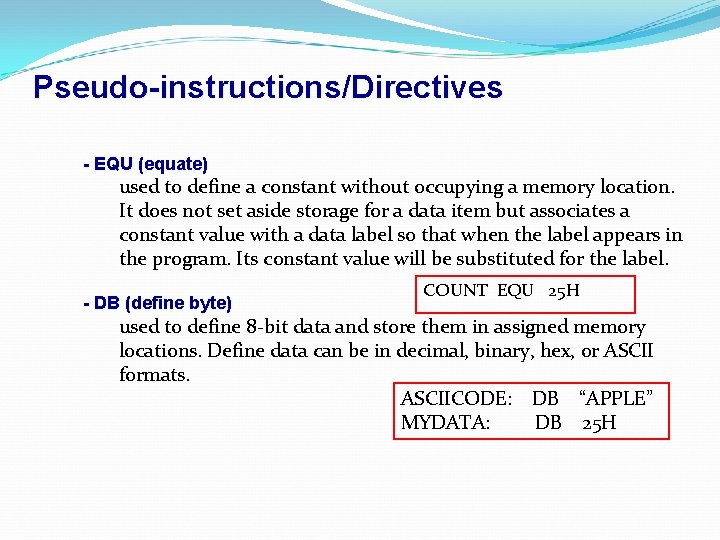
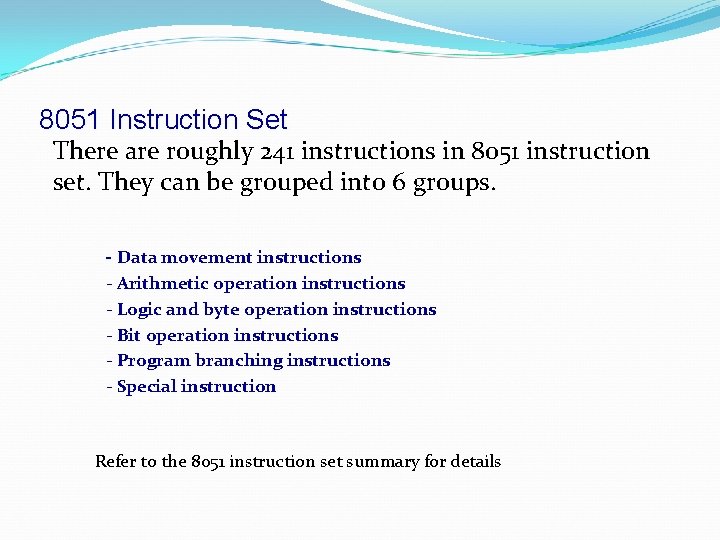
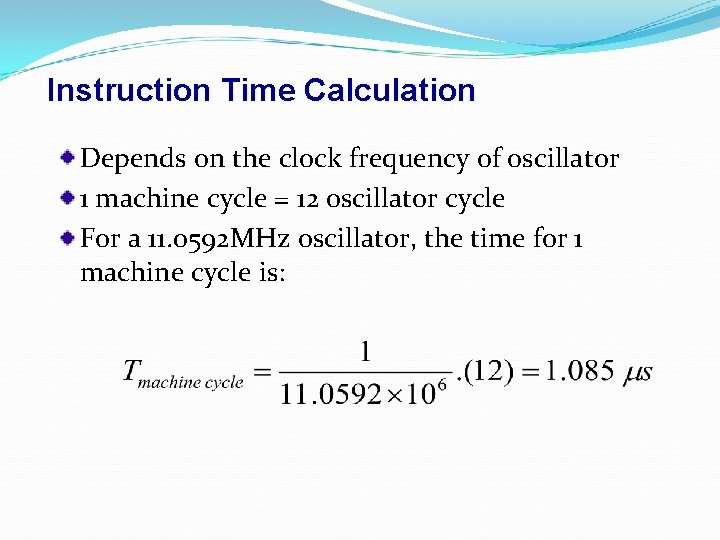
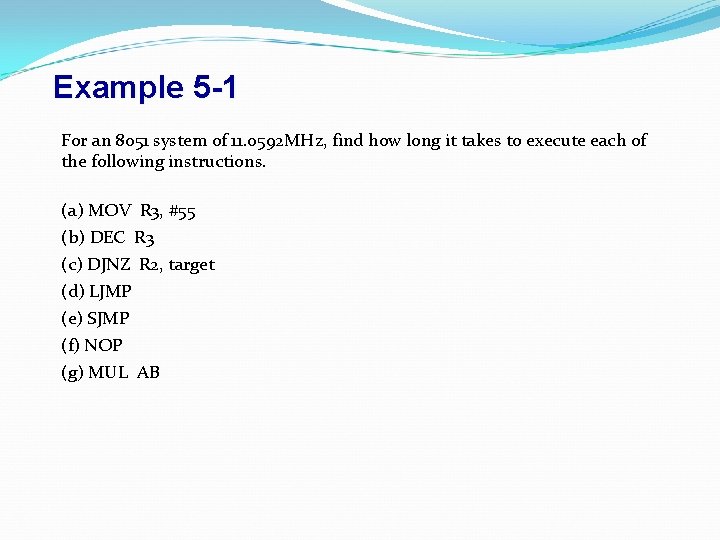
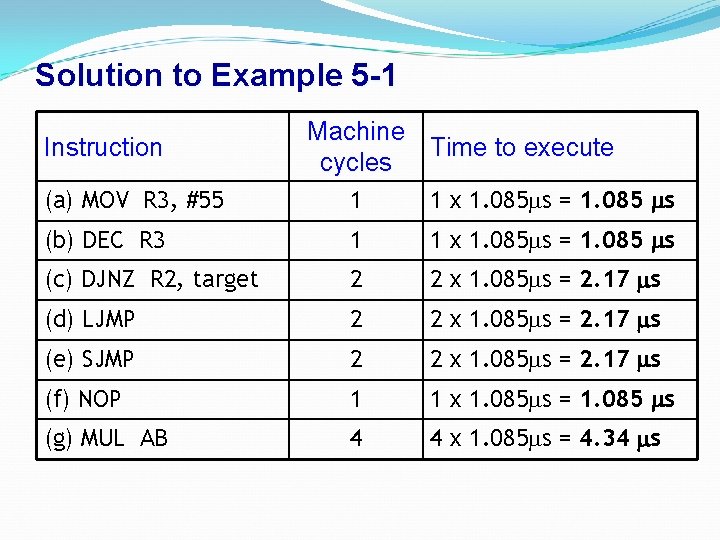
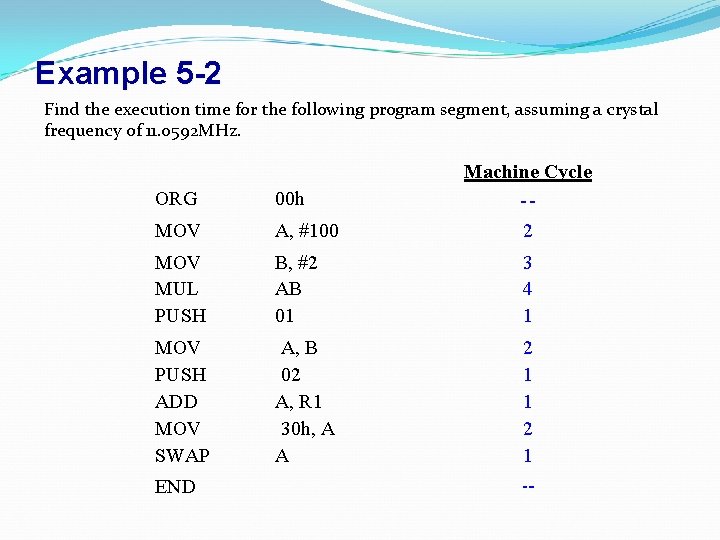
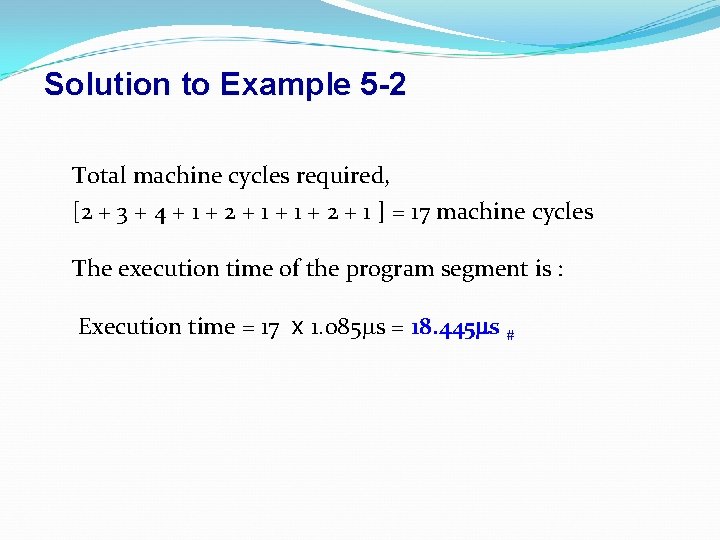
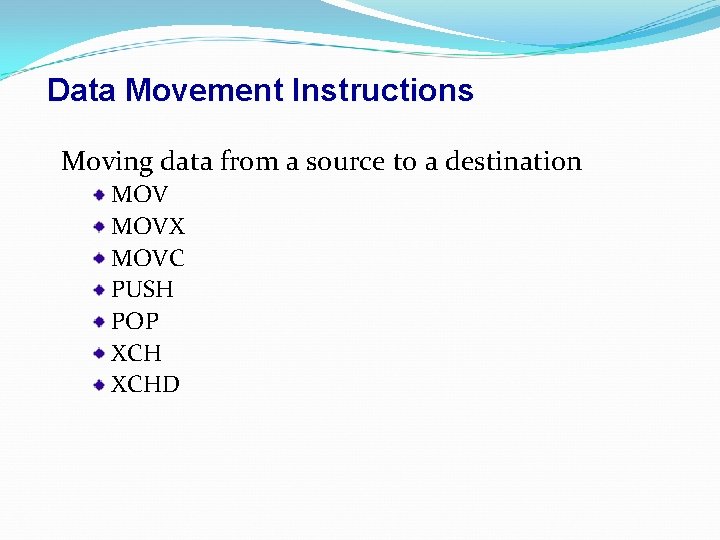
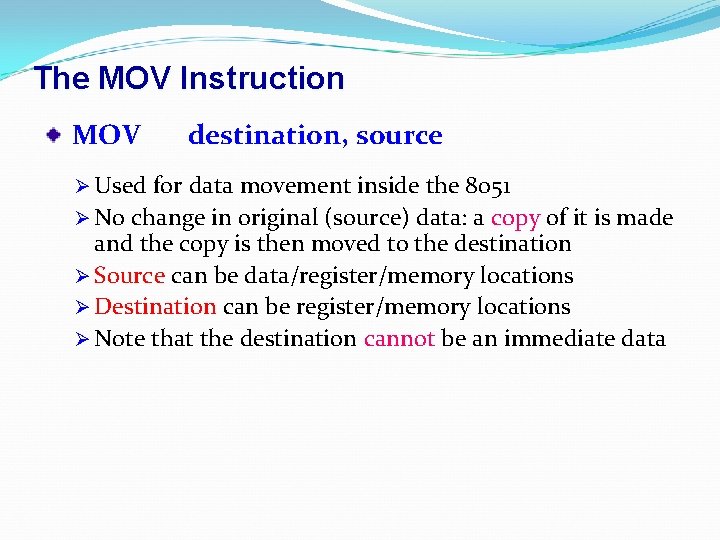
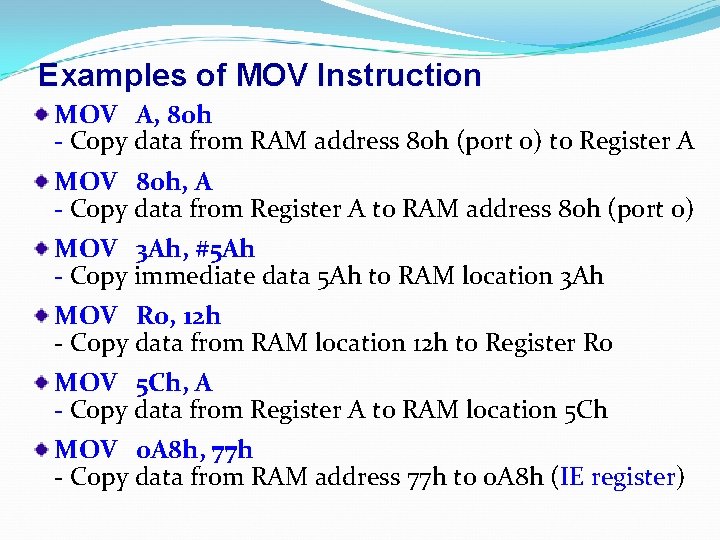
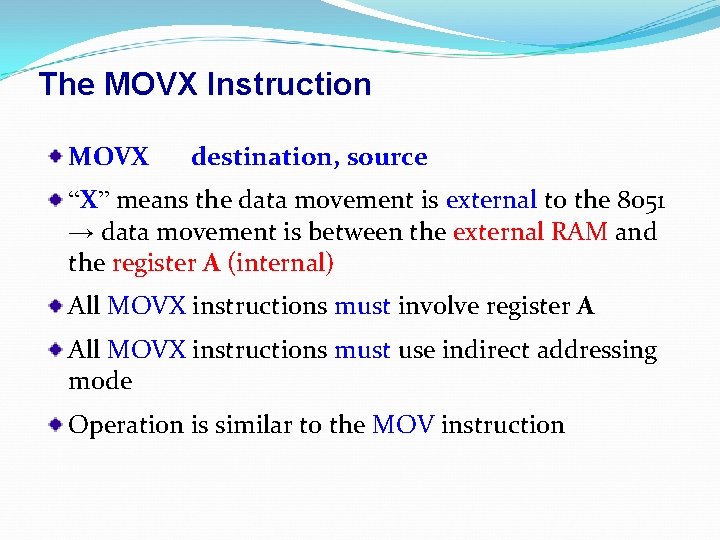
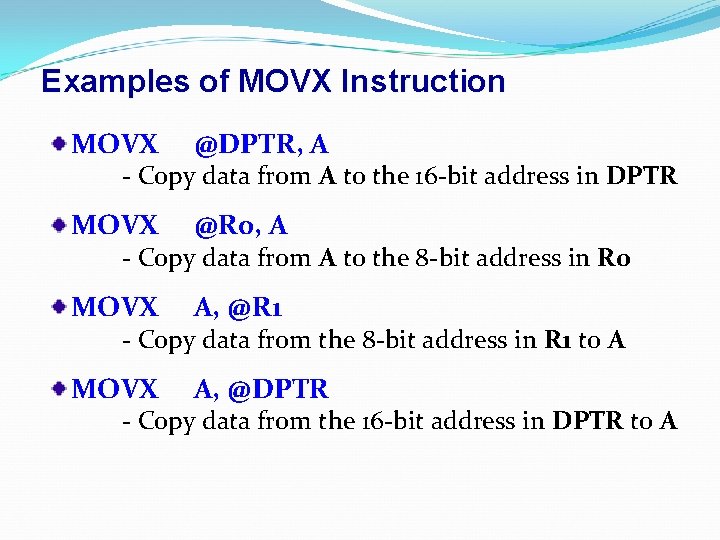
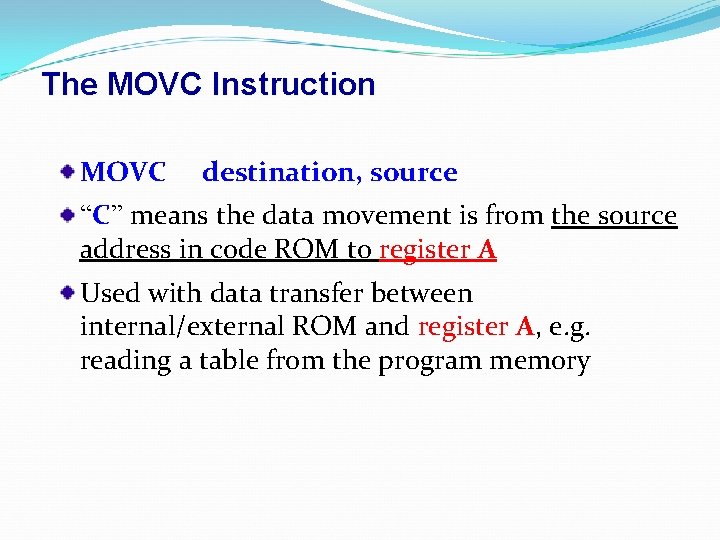
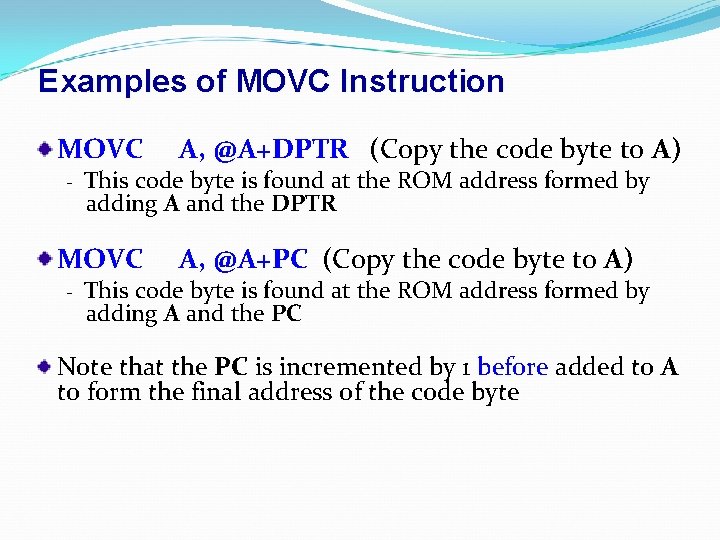
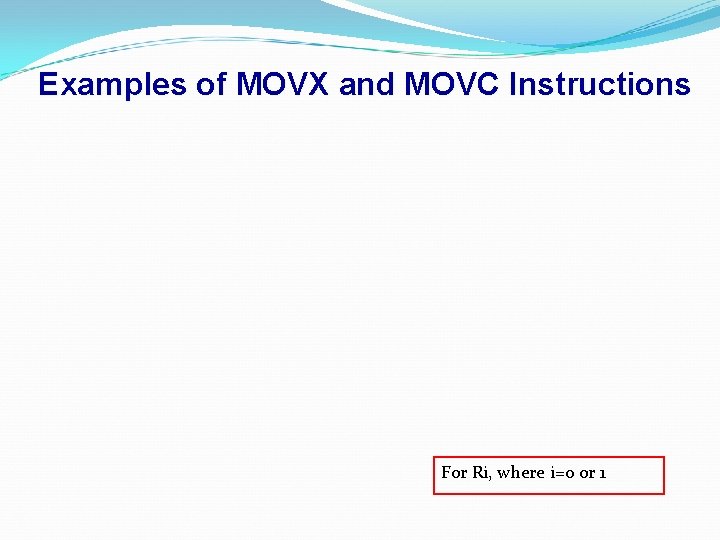
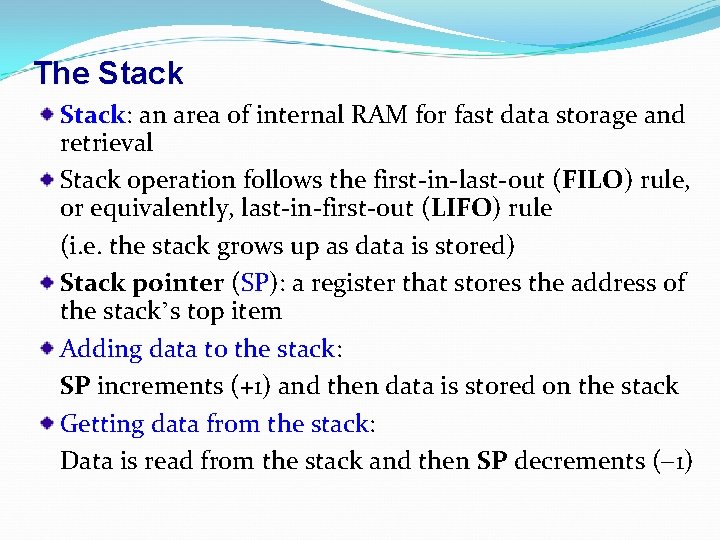
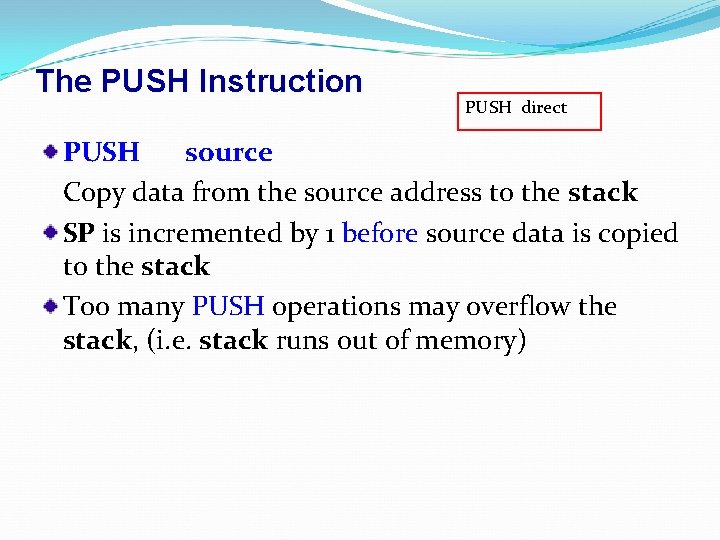
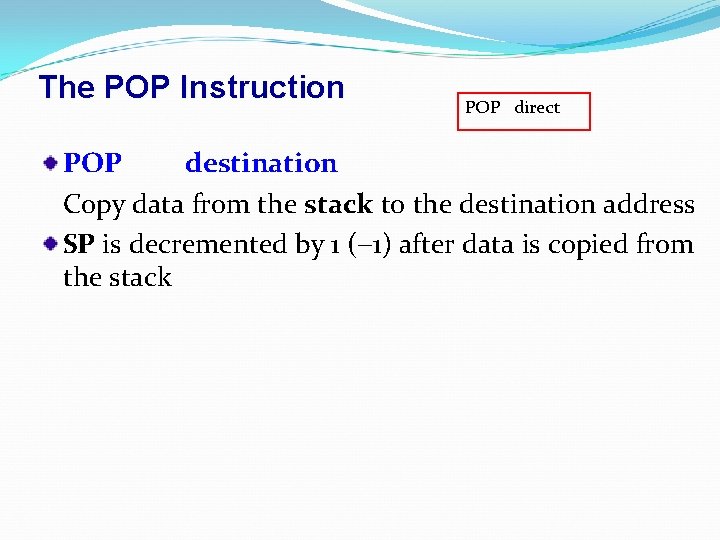
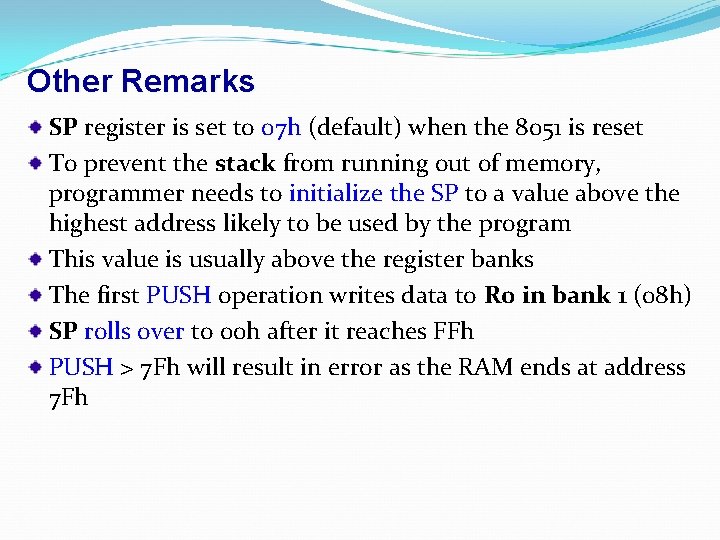
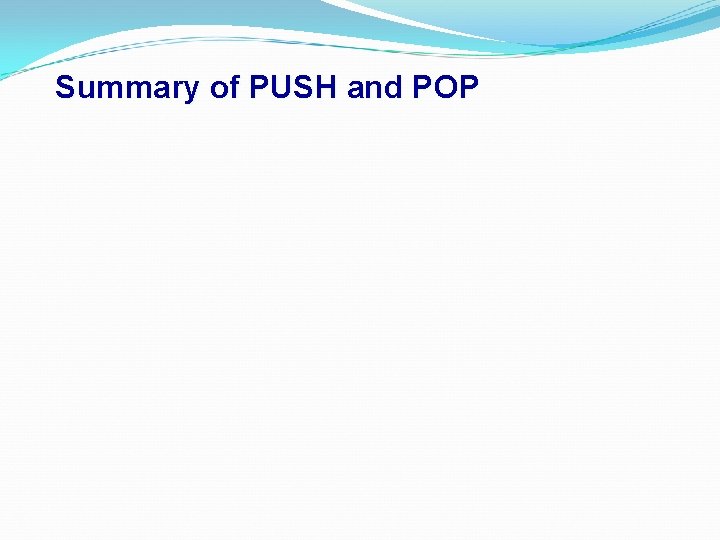
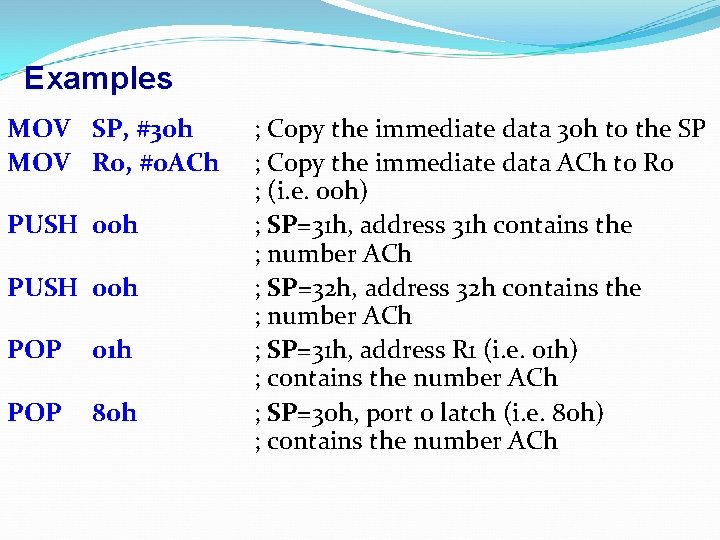
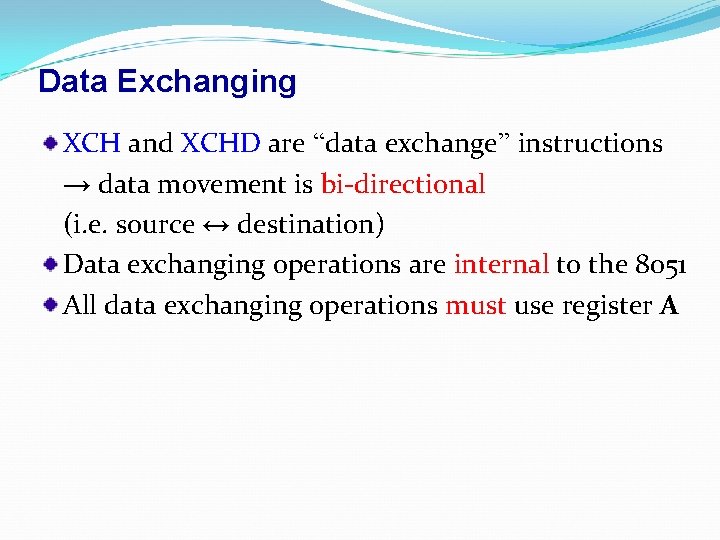
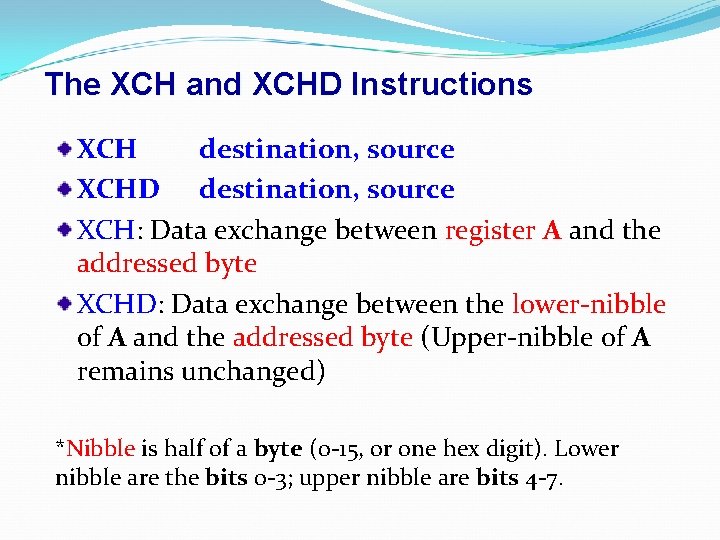
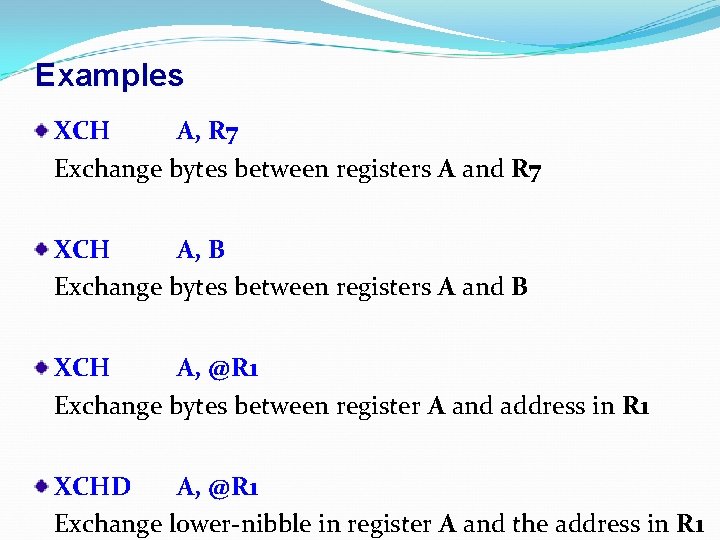
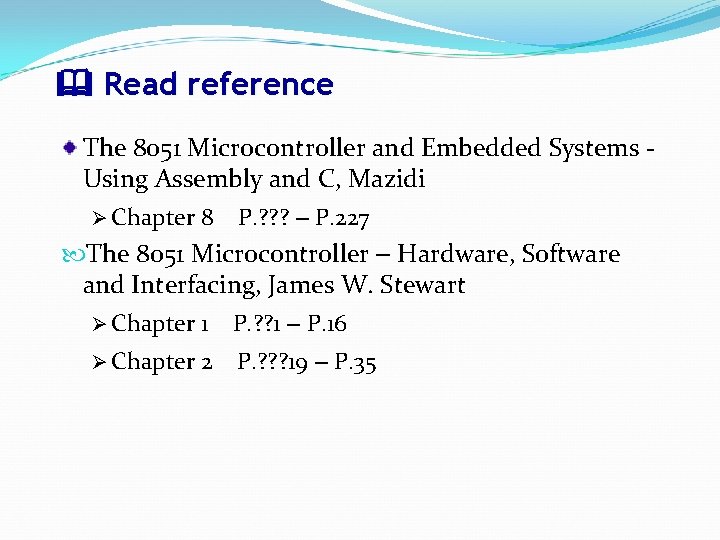
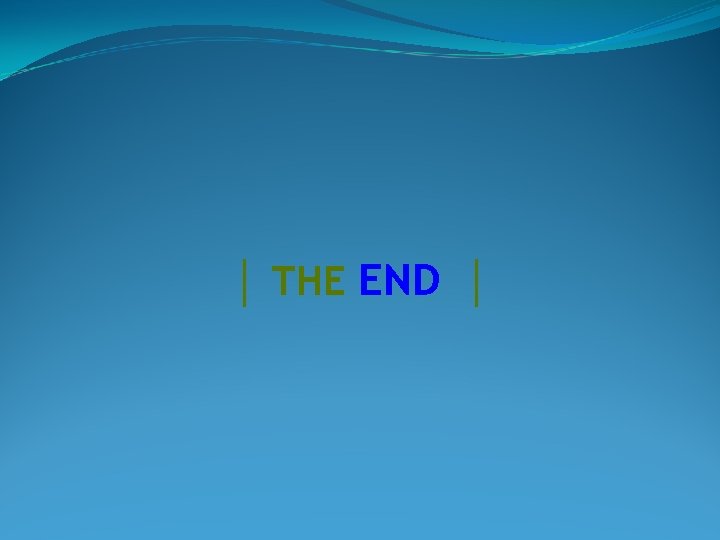
- Slides: 39
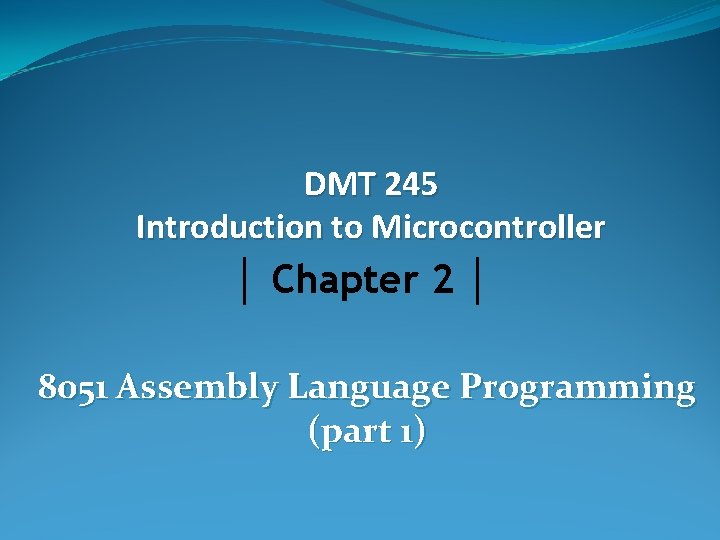
DMT 245 Introduction to Microcontroller │ Chapter 2 │ 8051 Assembly Language Programming (part 1)
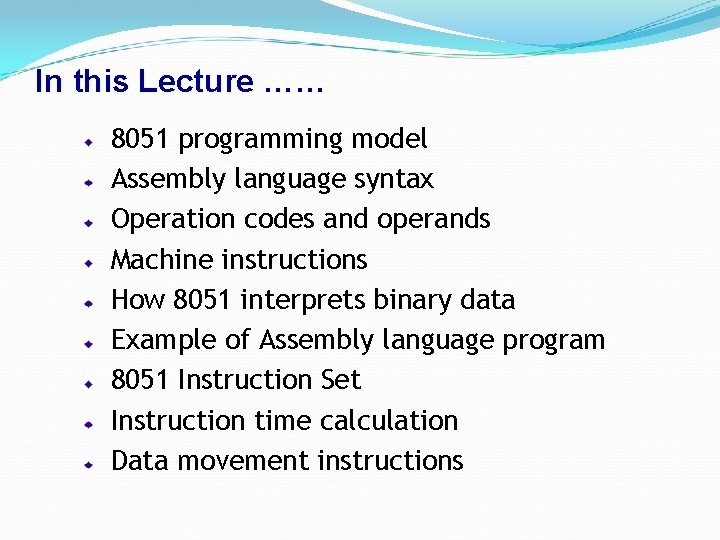
In this Lecture …… 8051 programming model Assembly language syntax Operation codes and operands Machine instructions How 8051 interprets binary data Example of Assembly language program 8051 Instruction Set Instruction time calculation Data movement instructions
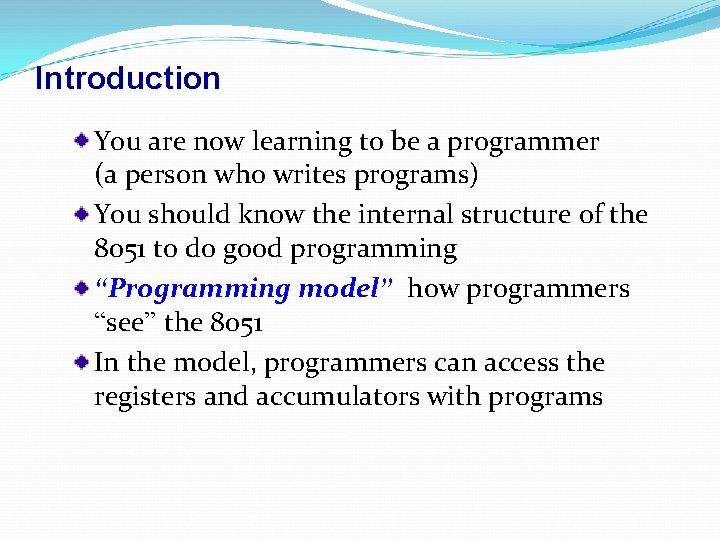
Introduction You are now learning to be a programmer (a person who writes programs) You should know the internal structure of the 8051 to do good programming “Programming model” how programmers “see” the 8051 In the model, programmers can access the registers and accumulators with programs
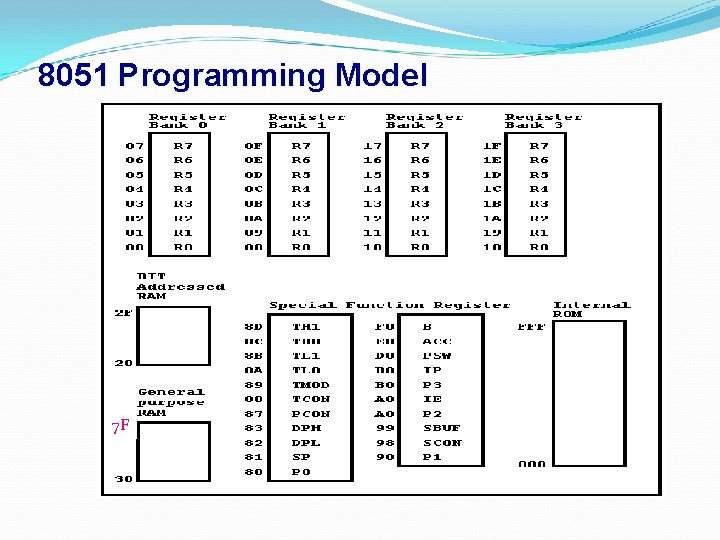
8051 Programming Model 7 F
![Assembly Language Syntax formatrule label mnemonic operands comment Items in square Assembly Language Syntax = format/rule [label: ] mnemonic [operands] [; comment] Items in square](https://slidetodoc.com/presentation_image_h2/6502a6a501d2fc78b9f4ed367dc02a33/image-5.jpg)
Assembly Language Syntax = format/rule [label: ] mnemonic [operands] [; comment] Items in square brackets are optional
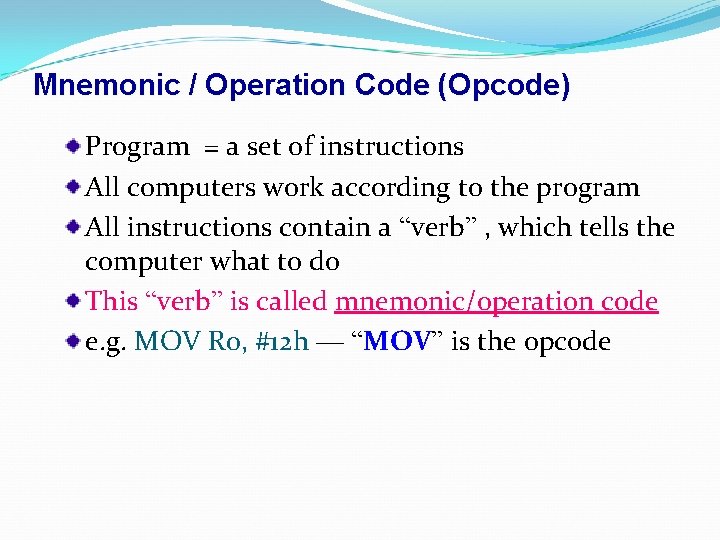
Mnemonic / Operation Code (Opcode) Program = a set of instructions All computers work according to the program All instructions contain a “verb” , which tells the computer what to do This “verb” is called mnemonic/operation code e. g. MOV R 0, #12 h –– “MOV” is the opcode
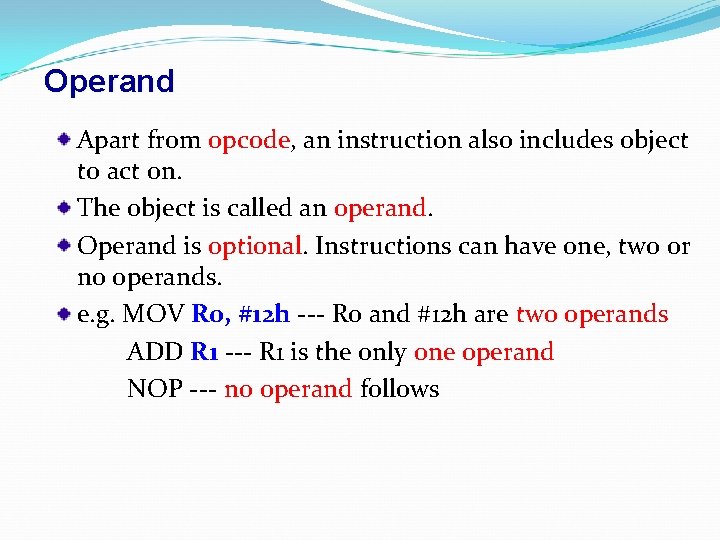
Operand Apart from opcode, an instruction also includes object to act on. The object is called an operand. Operand is optional. Instructions can have one, two or no operands. e. g. MOV R 0, #12 h --- R 0 and #12 h are two operands ADD R 1 --- R 1 is the only one operand NOP --- no operand follows
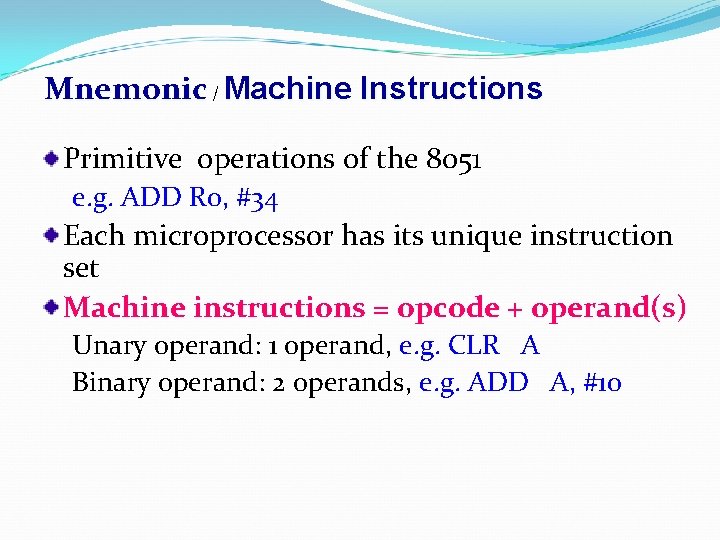
Mnemonic / Machine Instructions Primitive operations of the 8051 e. g. ADD R 0, #34 Each microprocessor has its unique instruction set Machine instructions = opcode + operand(s) Unary operand: 1 operand, e. g. CLR A Binary operand: 2 operands, e. g. ADD A, #10
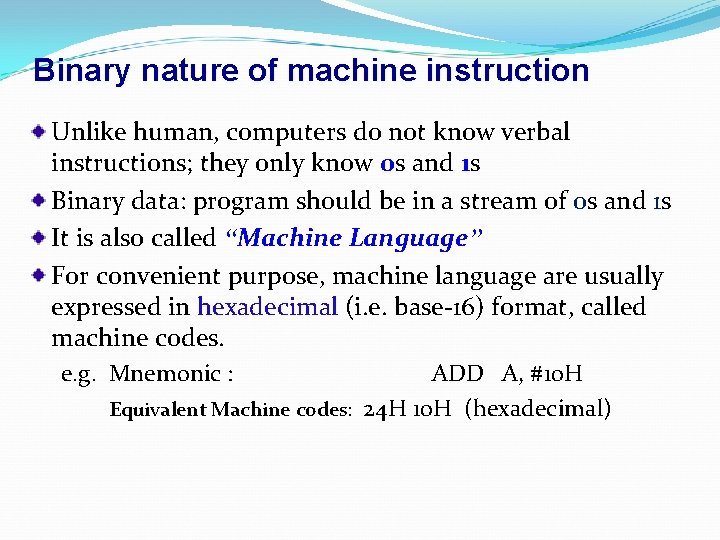
Binary nature of machine instruction Unlike human, computers do not know verbal instructions; they only know 0 s and 1 s Binary data: program should be in a stream of 0 s and 1 s It is also called “Machine Language” For convenient purpose, machine language are usually expressed in hexadecimal (i. e. base-16) format, called machine codes. e. g. Mnemonic : ADD A, #10 H Equivalent Machine codes: 24 H 10 H (hexadecimal)
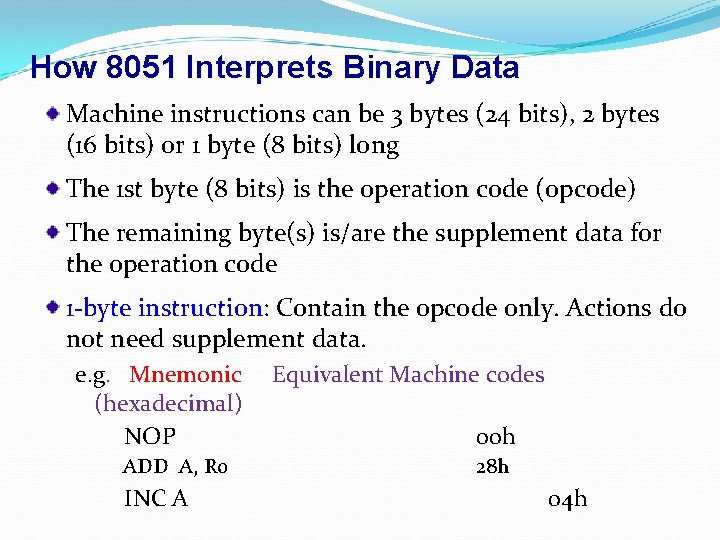
How 8051 Interprets Binary Data Machine instructions can be 3 bytes (24 bits), 2 bytes (16 bits) or 1 byte (8 bits) long The 1 st byte (8 bits) is the operation code (opcode) The remaining byte(s) is/are the supplement data for the operation code 1 -byte instruction: Contain the opcode only. Actions do not need supplement data. e. g. Mnemonic (hexadecimal) NOP ADD A, R 0 INC A Equivalent Machine codes 00 h 28 h 04 h
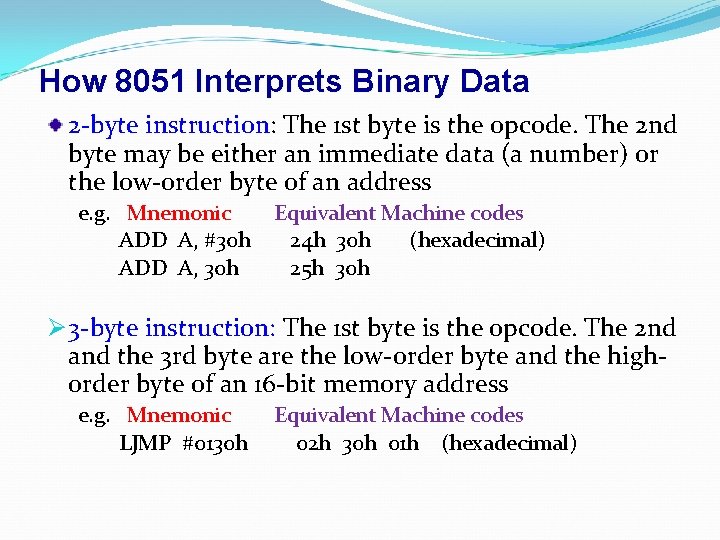
How 8051 Interprets Binary Data 2 -byte instruction: The 1 st byte is the opcode. The 2 nd byte may be either an immediate data (a number) or the low-order byte of an address e. g. Mnemonic ADD A, #30 h ADD A, 30 h Equivalent Machine codes 24 h 30 h (hexadecimal) 25 h 30 h Ø 3 -byte instruction: The 1 st byte is the opcode. The 2 nd and the 3 rd byte are the low-order byte and the highorder byte of an 16 -bit memory address e. g. Mnemonic LJMP #0130 h Equivalent Machine codes 02 h 30 h 01 h (hexadecimal)
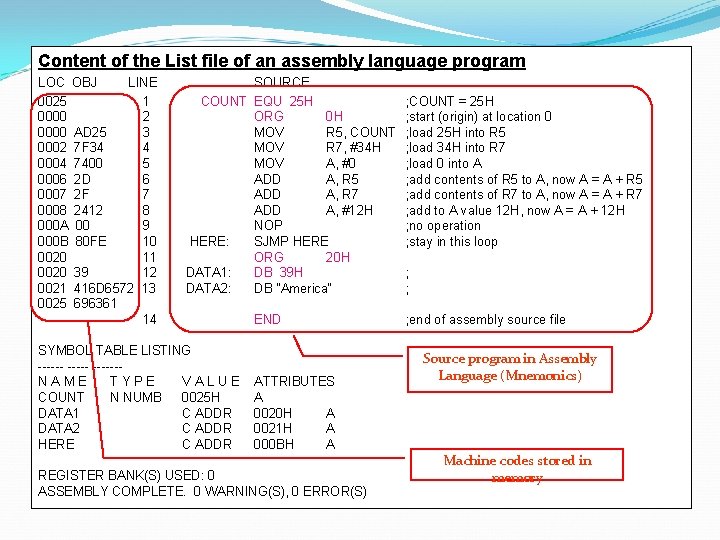
Content of the List file of an assembly language program LOC OBJ LINE 0025 1 0000 2 0000 AD 25 3 0002 7 F 34 4 0004 7400 5 0006 2 D 6 0007 2 F 7 0008 2412 8 000 A 00 9 000 B 80 FE 10 0020 11 0020 39 12 0021 416 D 6572 13 0025 696361 14 SOURCE COUNT EQU 25 H ORG 0 H MOV R 5, COUNT MOV R 7, #34 H MOV A, #0 ADD A, R 5 ADD A, R 7 ADD A, #12 H NOP HERE: SJMP HERE ORG 20 H DATA 1: DB 39 H DATA 2: DB "America“ SYMBOL TABLE LISTING ------NAME TYPE VALUE COUNT N NUMB 0025 H DATA 1 C ADDR DATA 2 C ADDR HERE C ADDR END ATTRIBUTES A 0020 H A 0021 H A 000 BH A REGISTER BANK(S) USED: 0 ASSEMBLY COMPLETE. 0 WARNING(S), 0 ERROR(S) ; COUNT = 25 H ; start (origin) at location 0 ; load 25 H into R 5 ; load 34 H into R 7 ; load 0 into A ; add contents of R 5 to A, now A = A + R 5 ; add contents of R 7 to A, now A = A + R 7 ; add to A value 12 H, now A = A + 12 H ; no operation ; stay in this loop ; ; ; end of assembly source file Source program in Assembly Language (Mnemonics) Machine codes stored in memory
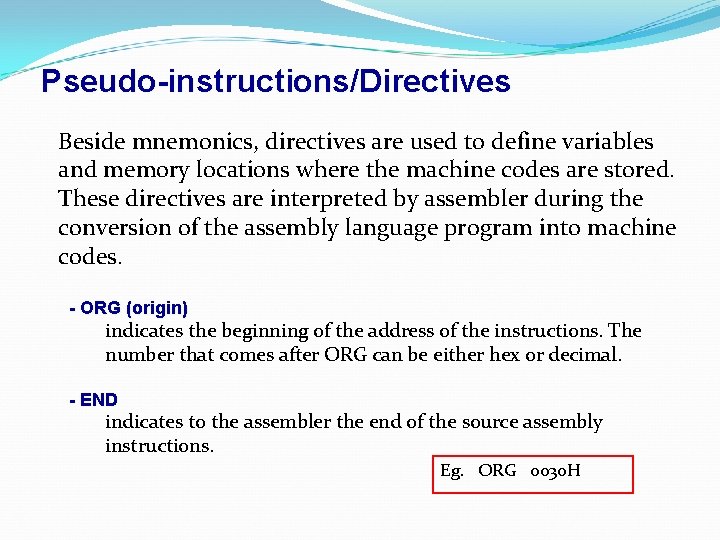
Pseudo-instructions/Directives Beside mnemonics, directives are used to define variables and memory locations where the machine codes are stored. These directives are interpreted by assembler during the conversion of the assembly language program into machine codes. - ORG (origin) indicates the beginning of the address of the instructions. The number that comes after ORG can be either hex or decimal. - END indicates to the assembler the end of the source assembly instructions. Eg. ORG 0030 H
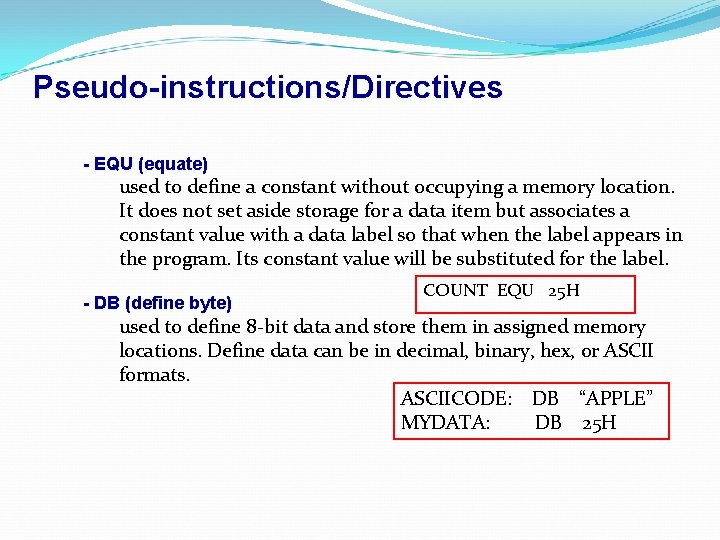
Pseudo-instructions/Directives - EQU (equate) used to define a constant without occupying a memory location. It does not set aside storage for a data item but associates a constant value with a data label so that when the label appears in the program. Its constant value will be substituted for the label. - DB (define byte) COUNT EQU 25 H used to define 8 -bit data and store them in assigned memory locations. Define data can be in decimal, binary, hex, or ASCII formats. ASCIICODE: DB “APPLE” MYDATA: DB 25 H
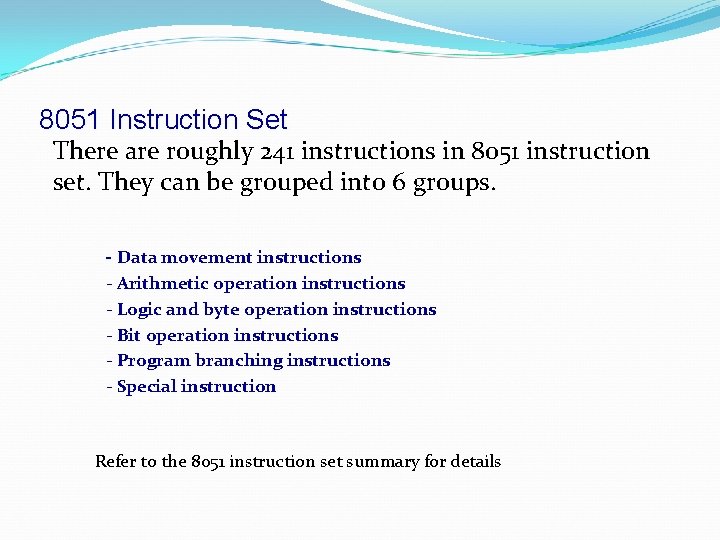
8051 Instruction Set There are roughly 241 instructions in 8051 instruction set. They can be grouped into 6 groups. - Data movement instructions - Arithmetic operation instructions - Logic and byte operation instructions - Bit operation instructions - Program branching instructions - Special instruction Refer to the 8051 instruction set summary for details
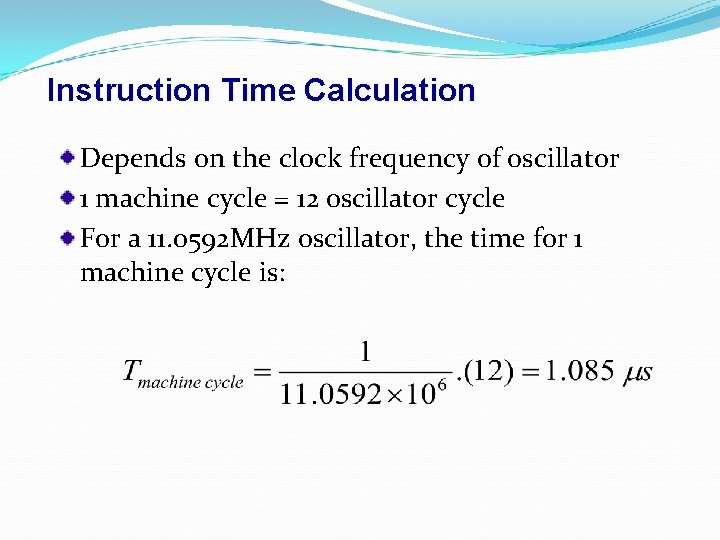
Instruction Time Calculation Depends on the clock frequency of oscillator 1 machine cycle = 12 oscillator cycle For a 11. 0592 MHz oscillator, the time for 1 machine cycle is:
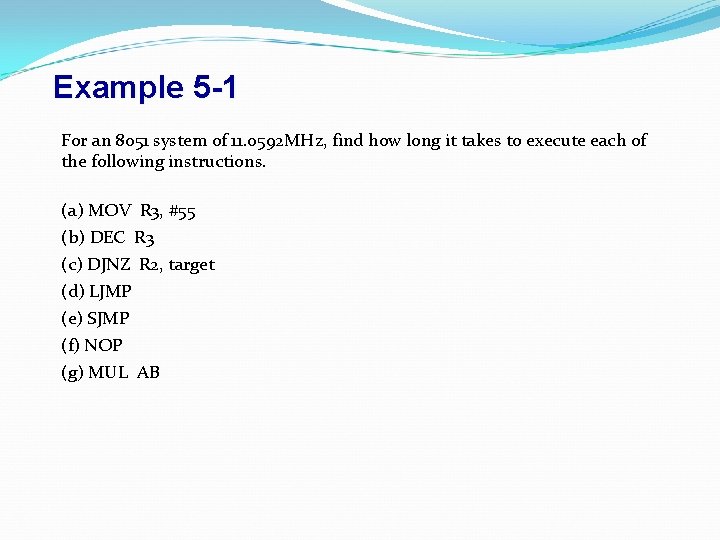
Example 5 -1 For an 8051 system of 11. 0592 MHz, find how long it takes to execute each of the following instructions. (a) MOV R 3, #55 (b) DEC R 3 (c) DJNZ R 2, target (d) LJMP (e) SJMP (f) NOP (g) MUL AB
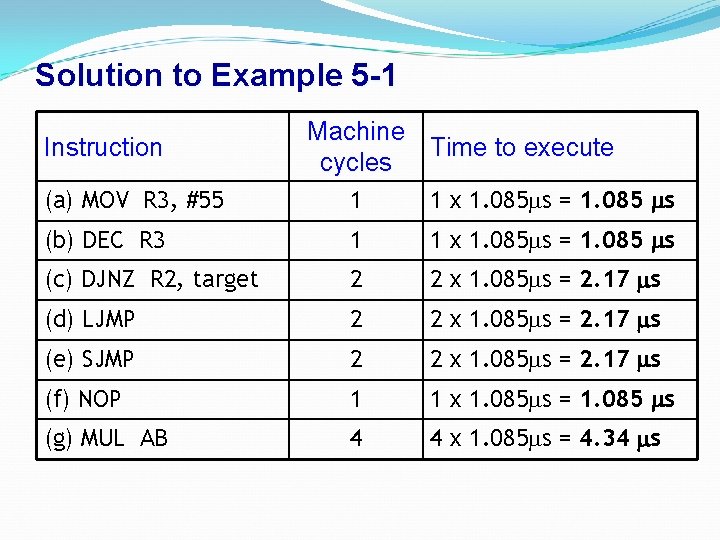
Solution to Example 5 -1 Instruction Machine Time to execute cycles (a) MOV R 3, #55 1 1 x 1. 085 s = 1. 085 s (b) DEC R 3 1 1 x 1. 085 s = 1. 085 s (c) DJNZ R 2, target 2 2 x 1. 085 s = 2. 17 s (d) LJMP 2 2 x 1. 085 s = 2. 17 s (e) SJMP 2 2 x 1. 085 s = 2. 17 s (f) NOP 1 1 x 1. 085 s = 1. 085 s (g) MUL AB 4 4 x 1. 085 s = 4. 34 s
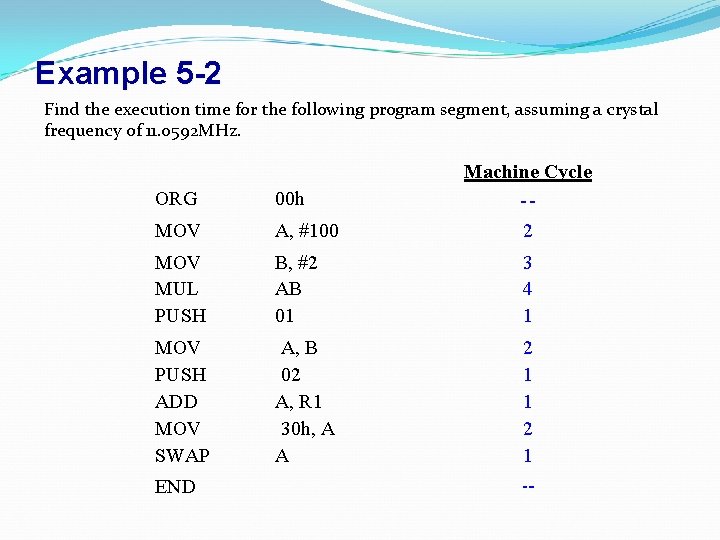
Example 5 -2 Find the execution time for the following program segment, assuming a crystal frequency of 11. 0592 MHz. Machine Cycle ORG 00 h -- MOV A, #100 2 MOV MUL PUSH B, #2 AB 01 3 4 1 MOV PUSH ADD MOV SWAP A, B 02 A, R 1 30 h, A A 2 1 1 2 1 END --
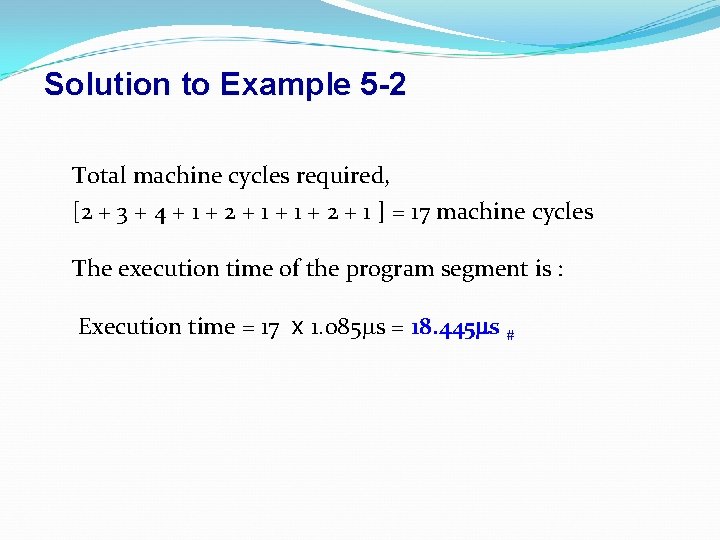
Solution to Example 5 -2 Total machine cycles required, [2 + 3 + 4 + 1 + 2 + 1 ] = 17 machine cycles The execution time of the program segment is : Execution time = 17 x 1. 085 s = 18. 445 s #
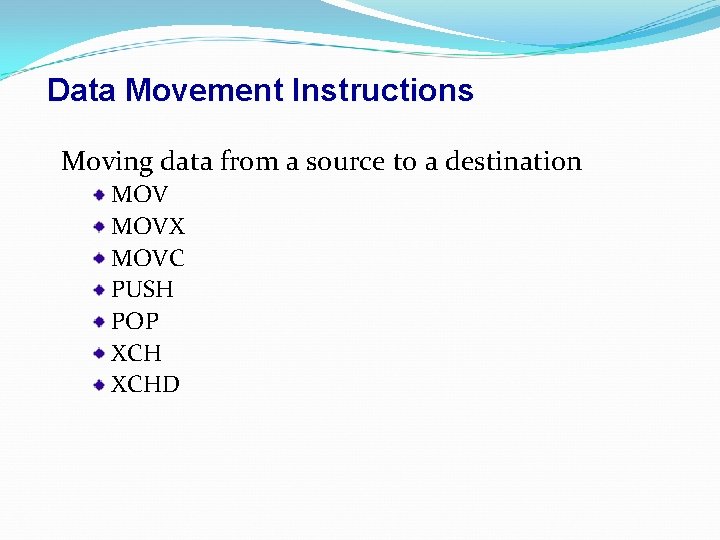
Data Movement Instructions Moving data from a source to a destination MOVX MOVC PUSH POP XCHD
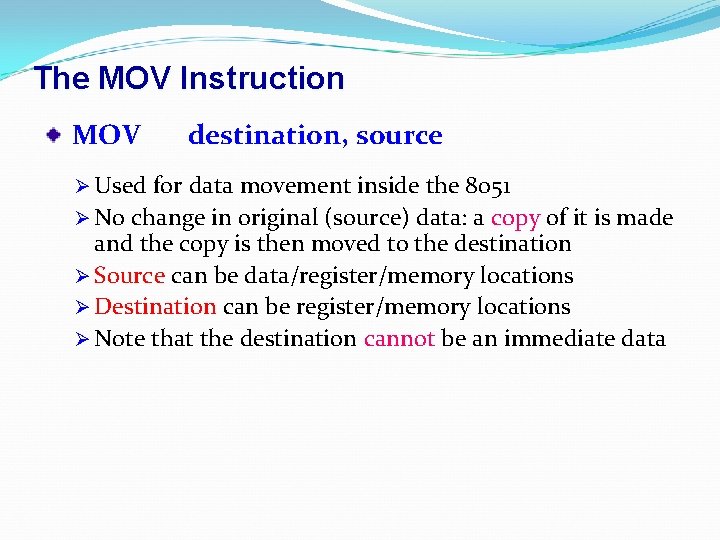
The MOV Instruction MOV Ø Used destination, source for data movement inside the 8051 Ø No change in original (source) data: a copy of it is made and the copy is then moved to the destination Ø Source can be data/register/memory locations Ø Destination can be register/memory locations Ø Note that the destination cannot be an immediate data
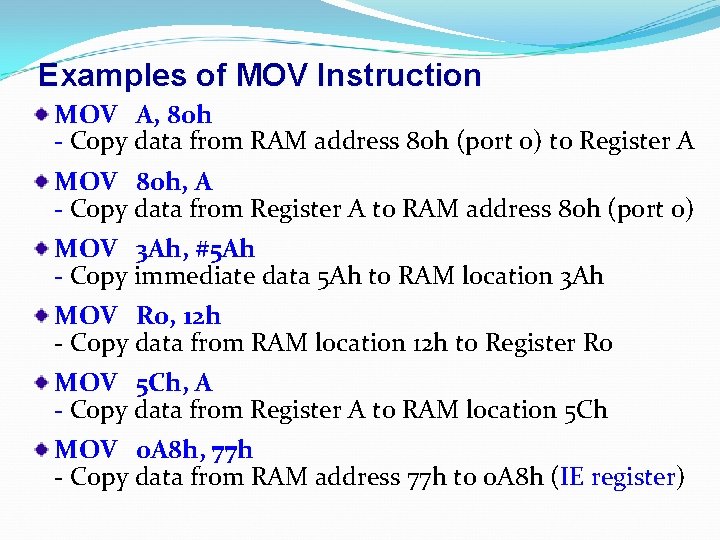
Examples of MOV Instruction MOV A, 80 h - Copy data from RAM address 80 h (port 0) to Register A MOV 80 h, A - Copy data from Register A to RAM address 80 h (port 0) MOV 3 Ah, #5 Ah - Copy immediate data 5 Ah to RAM location 3 Ah MOV R 0, 12 h - Copy data from RAM location 12 h to Register R 0 MOV 5 Ch, A - Copy data from Register A to RAM location 5 Ch MOV 0 A 8 h, 77 h - Copy data from RAM address 77 h to 0 A 8 h (IE register)
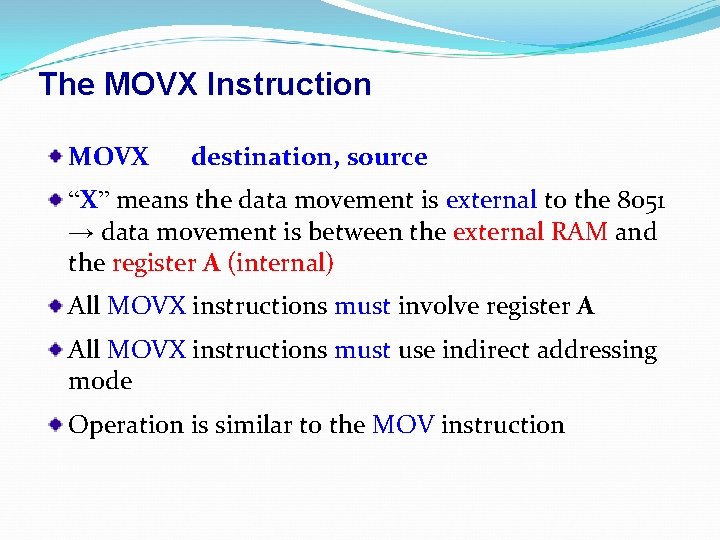
The MOVX Instruction MOVX destination, source “X” means the data movement is external to the 8051 → data movement is between the external RAM and the register A (internal) All MOVX instructions must involve register A All MOVX instructions must use indirect addressing mode Operation is similar to the MOV instruction
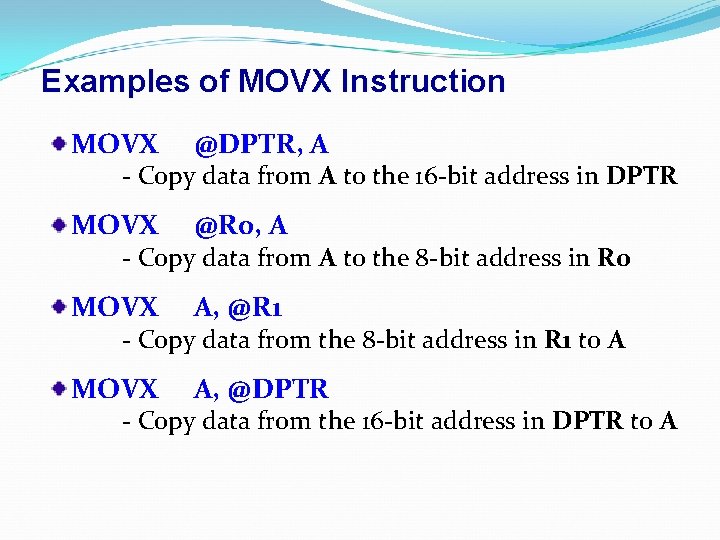
Examples of MOVX Instruction MOVX @DPTR, A MOVX @R 0, A MOVX A, @R 1 MOVX A, @DPTR - Copy data from A to the 16 -bit address in DPTR - Copy data from A to the 8 -bit address in R 0 - Copy data from the 8 -bit address in R 1 to A - Copy data from the 16 -bit address in DPTR to A
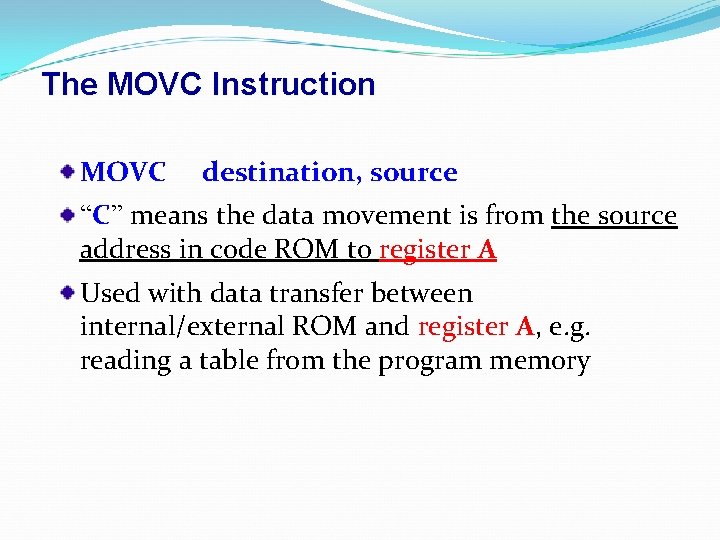
The MOVC Instruction MOVC destination, source “C” means the data movement is from the source address in code ROM to register A Used with data transfer between internal/external ROM and register A, e. g. reading a table from the program memory
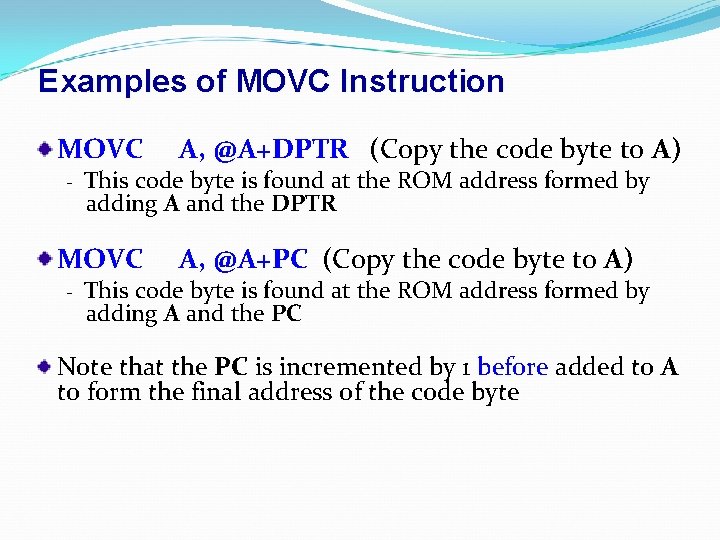
Examples of MOVC Instruction MOVC - This code byte is found at the ROM address formed by adding A and the DPTR MOVC - A, @A+DPTR (Copy the code byte to A) A, @A+PC (Copy the code byte to A) This code byte is found at the ROM address formed by adding A and the PC Note that the PC is incremented by 1 before added to A to form the final address of the code byte
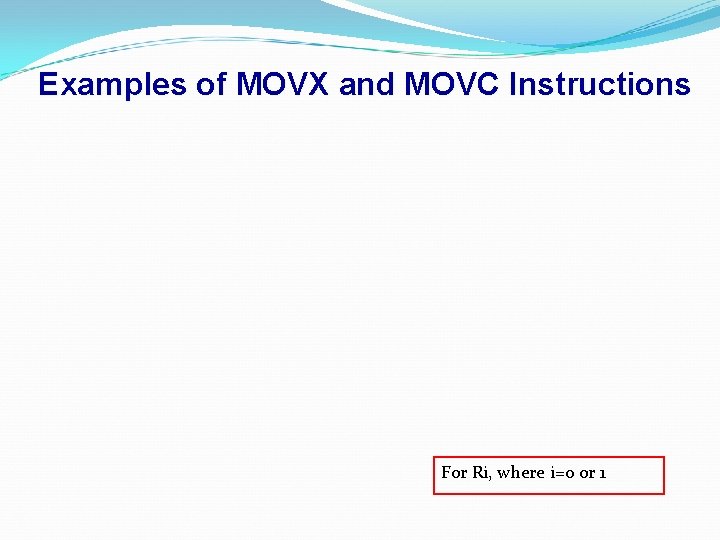
Examples of MOVX and MOVC Instructions For Ri, where i=0 or 1
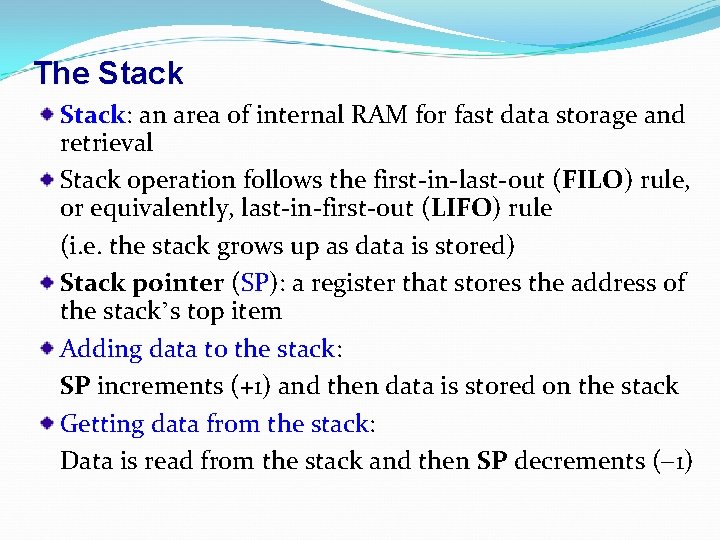
The Stack: an area of internal RAM for fast data storage and retrieval Stack operation follows the first-in-last-out (FILO) rule, or equivalently, last-in-first-out (LIFO) rule (i. e. the stack grows up as data is stored) Stack pointer (SP): a register that stores the address of the stack’s top item Adding data to the stack: SP increments (+1) and then data is stored on the stack Getting data from the stack: Data is read from the stack and then SP decrements ( 1)
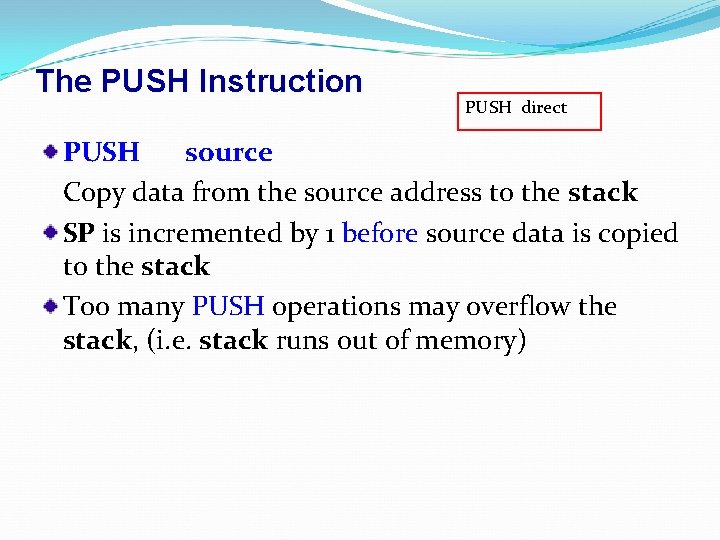
The PUSH Instruction PUSH direct PUSH source Copy data from the source address to the stack SP is incremented by 1 before source data is copied to the stack Too many PUSH operations may overflow the stack, (i. e. stack runs out of memory)
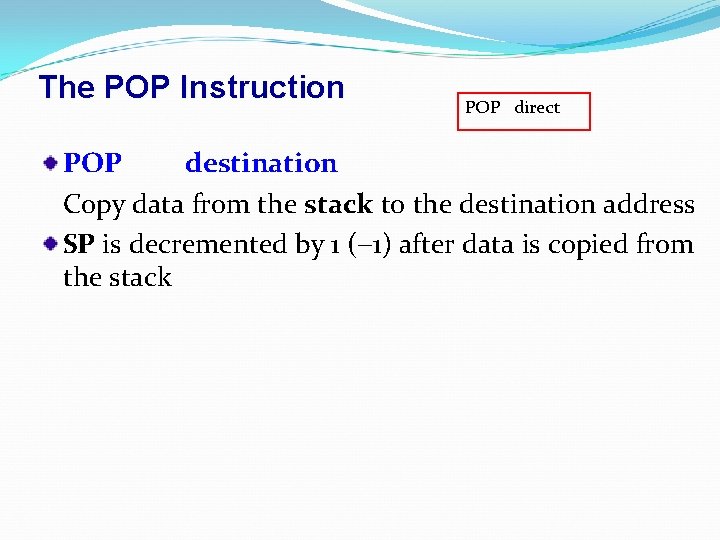
The POP Instruction POP direct POP destination Copy data from the stack to the destination address SP is decremented by 1 ( 1) after data is copied from the stack
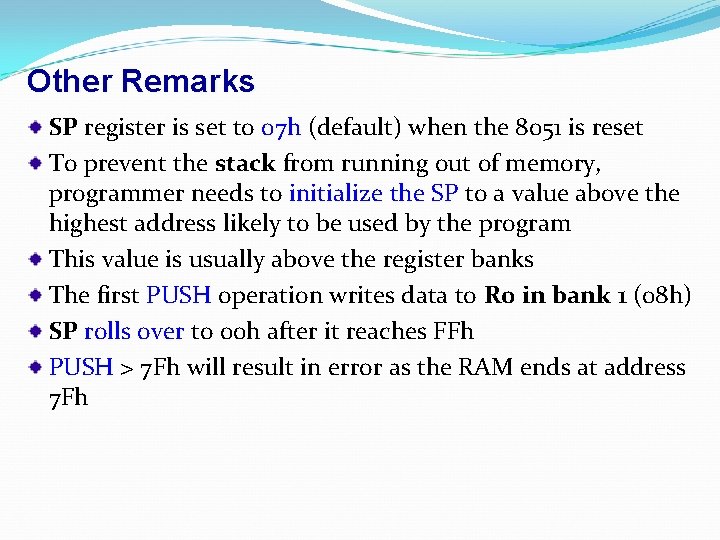
Other Remarks SP register is set to 07 h (default) when the 8051 is reset To prevent the stack from running out of memory, programmer needs to initialize the SP to a value above the highest address likely to be used by the program This value is usually above the register banks The first PUSH operation writes data to R 0 in bank 1 (08 h) SP rolls over to 00 h after it reaches FFh PUSH > 7 Fh will result in error as the RAM ends at address 7 Fh
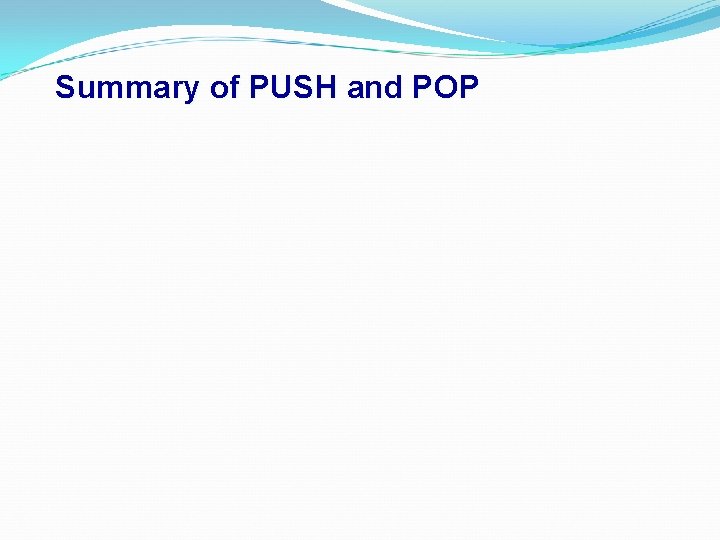
Summary of PUSH and POP
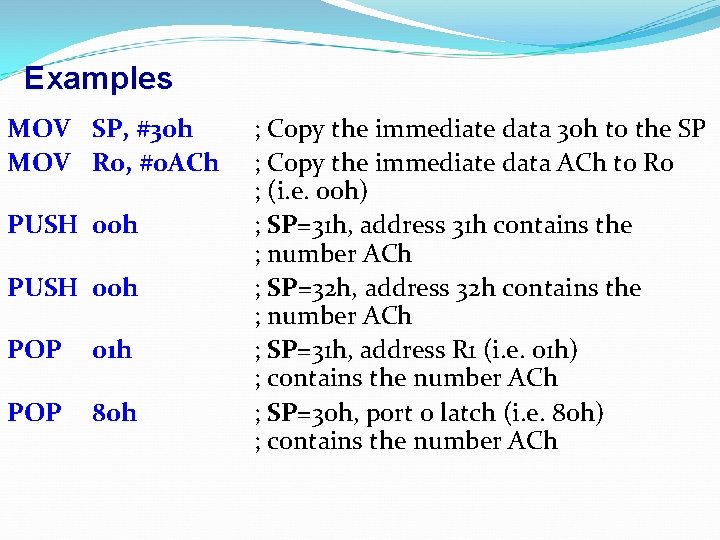
Examples MOV SP, #30 h MOV R 0, #0 ACh PUSH 00 h POP 01 h POP 80 h ; Copy the immediate data 30 h to the SP ; Copy the immediate data ACh to R 0 ; (i. e. 00 h) ; SP=31 h, address 31 h contains the ; number ACh ; SP=32 h, address 32 h contains the ; number ACh ; SP=31 h, address R 1 (i. e. 01 h) ; contains the number ACh ; SP=30 h, port 0 latch (i. e. 80 h) ; contains the number ACh
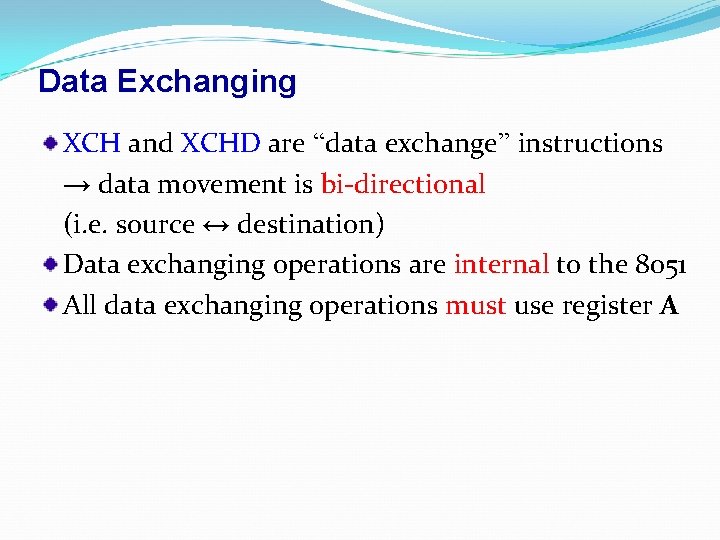
Data Exchanging XCH and XCHD are “data exchange” instructions → data movement is bi-directional (i. e. source ↔ destination) Data exchanging operations are internal to the 8051 All data exchanging operations must use register A
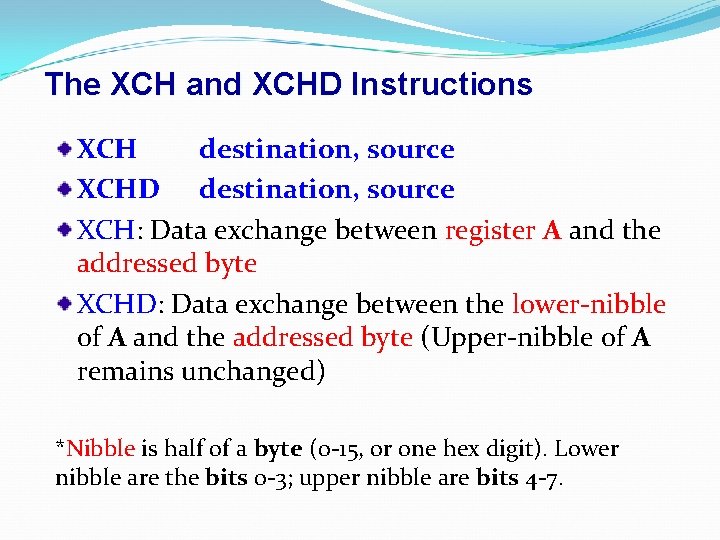
The XCH and XCHD Instructions XCH destination, source XCHD destination, source XCH: Data exchange between register A and the addressed byte XCHD: Data exchange between the lower-nibble of A and the addressed byte (Upper-nibble of A remains unchanged) *Nibble is half of a byte (0 -15, or one hex digit). Lower nibble are the bits 0 -3; upper nibble are bits 4 -7.
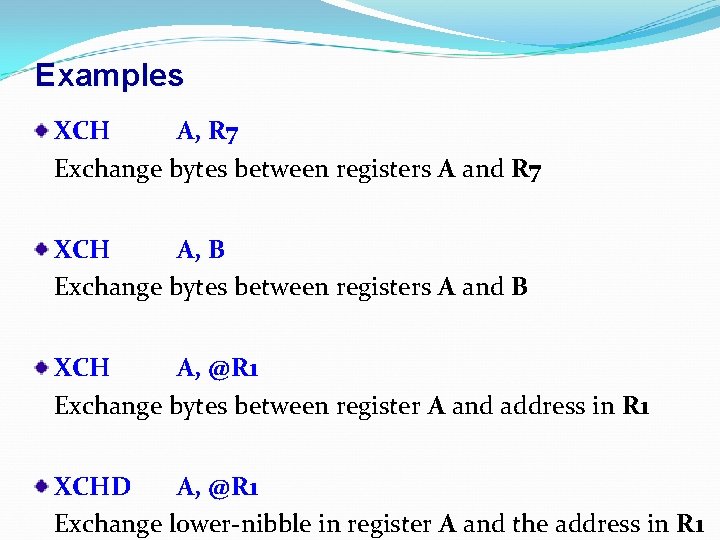
Examples XCH A, R 7 Exchange bytes between registers A and R 7 XCH A, B Exchange bytes between registers A and B XCH A, @R 1 Exchange bytes between register A and address in R 1 XCHD A, @R 1 Exchange lower-nibble in register A and the address in R 1
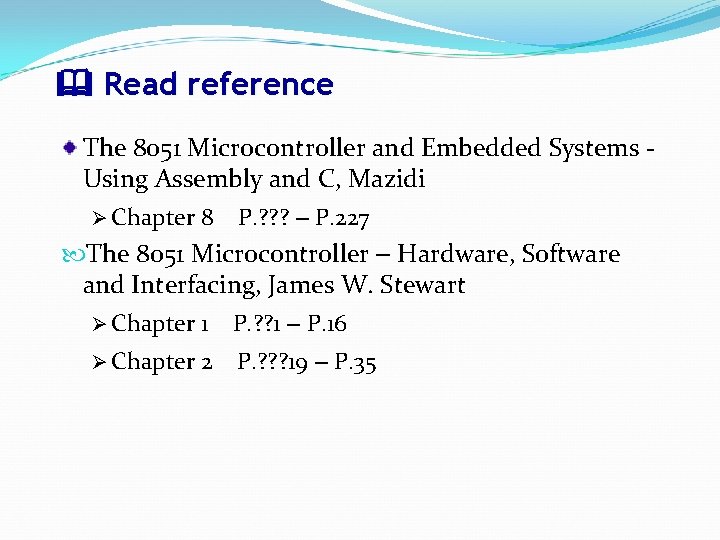
Read reference The 8051 Microcontroller and Embedded Systems Using Assembly and C, Mazidi Ø Chapter 8 P. ? ? ? – P. 227 The 8051 Microcontroller – Hardware, Software and Interfacing, James W. Stewart Ø Chapter 1 P. ? ? 1 – P. 16 Ø Chapter 2 P. ? ? ? 19 – P. 35
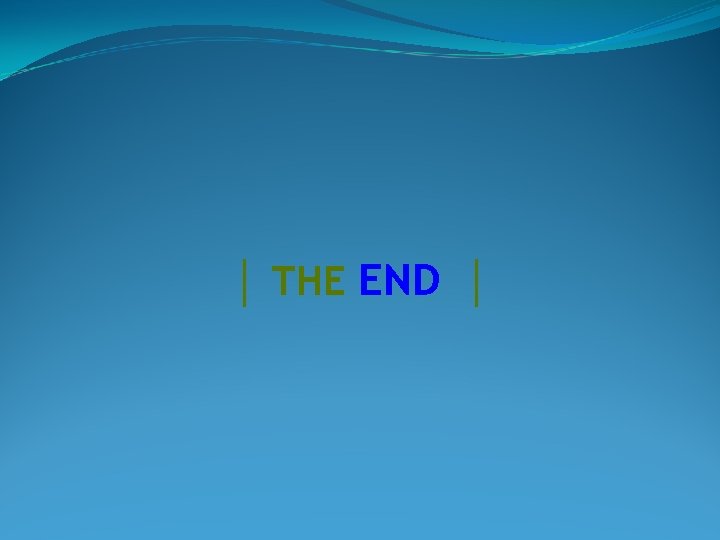
│ THE END │
Pin description of 8051 microcontroller
Assembler directives in 8051 microcontroller
Serial port interrupt in 8051
Basics of microcontroller 8051
Internal memory organization of 8051 microcontroller
1980
8051 addressing modes
Tr
8051 microcontroller instruction set
8050 microcontroller
Interrupt programming in 8051 using assembly language
6 interrupts in 8051
Different modes of operation of timer in 8051
Addressing modes in 8051
Ljmp instruction in 8051 example
Rotate and swap instruction in 8051
Dc motor interfacing with 8051 microcontroller
Components of 8051 microcontroller
8051 microcontroller timers and counters
Programming 8051 timers
Serial communication in 8051 c program
8051 addressing modes
Introduction to semiconductor physics
Dmt 234
Dmt 234
Maid
Dmt 234
The pythagorean theorem
Dmt
Dmt fabrication
Layout dmt
Dmt monitor
Dmt
Arduino introduction ppt
Introduction to microcontroller
Use a number line to solve 245-137
245 angle
245 prime factors
Dental bur design
Far 52-245-1