Dijkstras Algorithm Correctness Implement Q How to do
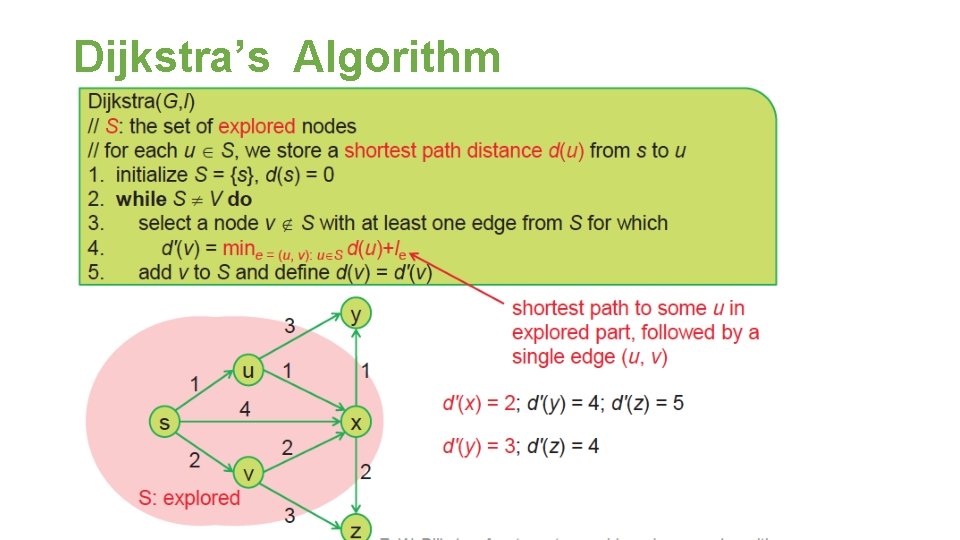
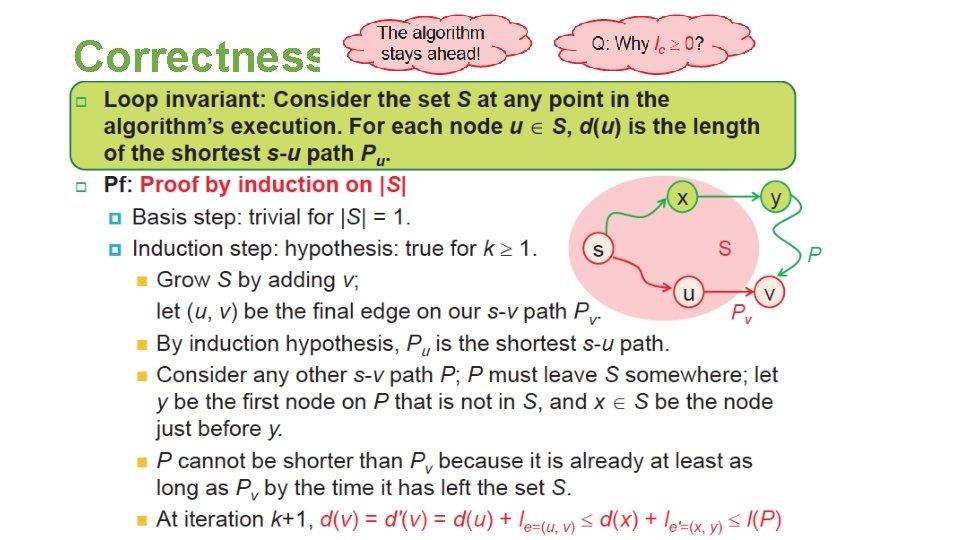
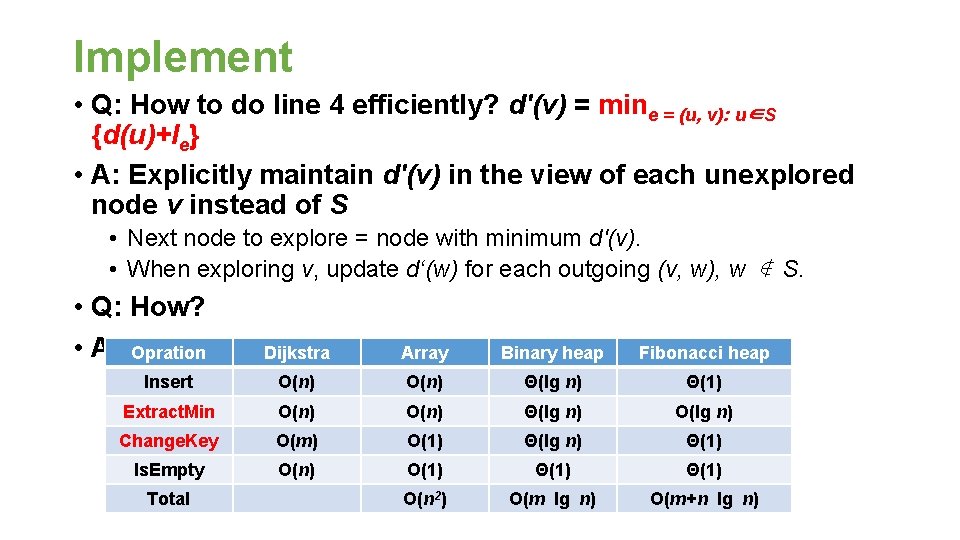
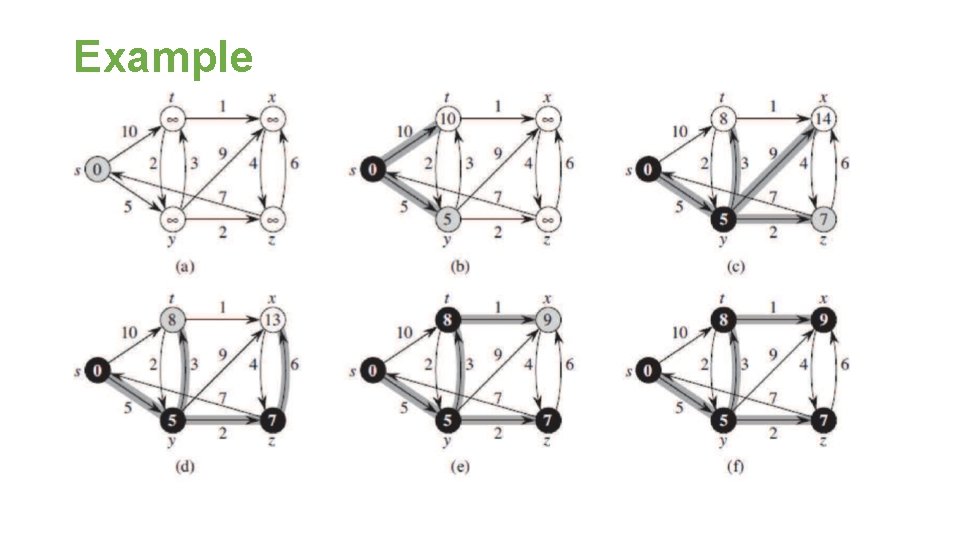
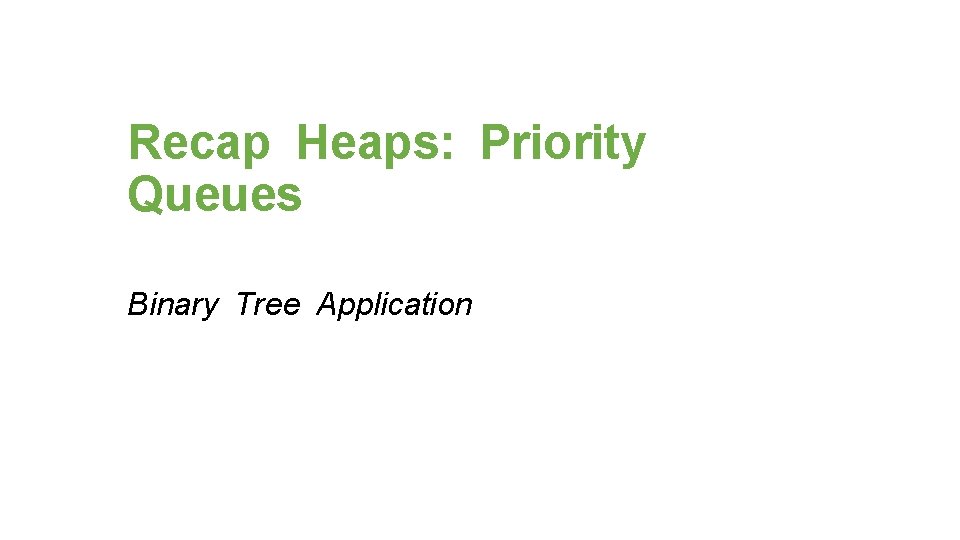
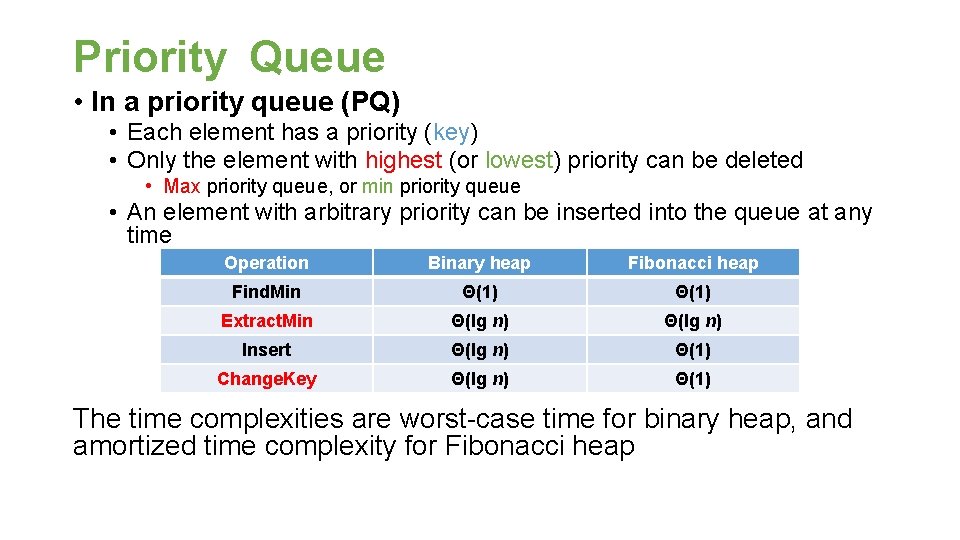
![Heap • Definition: A max (min) heap is • A max (min) tree: key[parent] Heap • Definition: A max (min) heap is • A max (min) tree: key[parent]](https://slidetodoc.com/presentation_image_h2/7d7ce8f2d1181a6a25c7e82bc9504723/image-7.jpg)
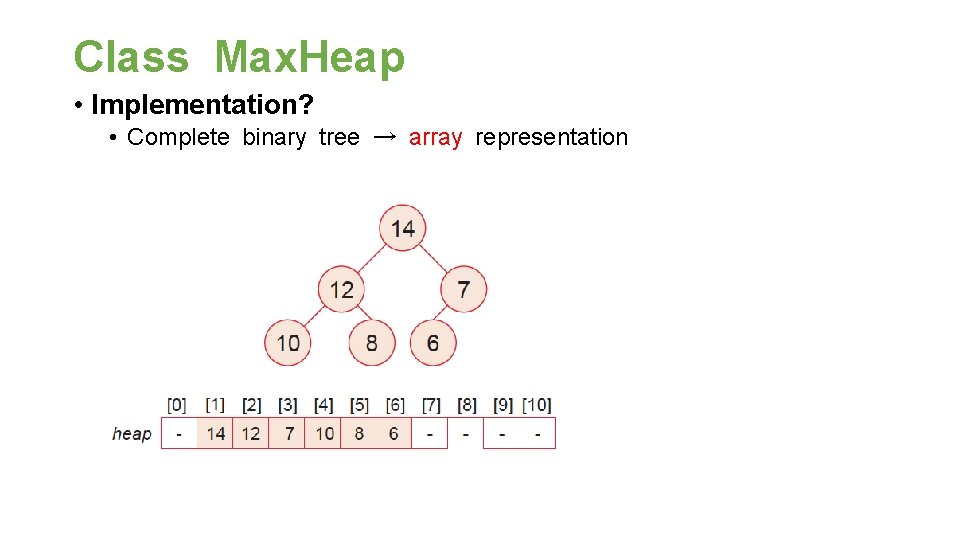
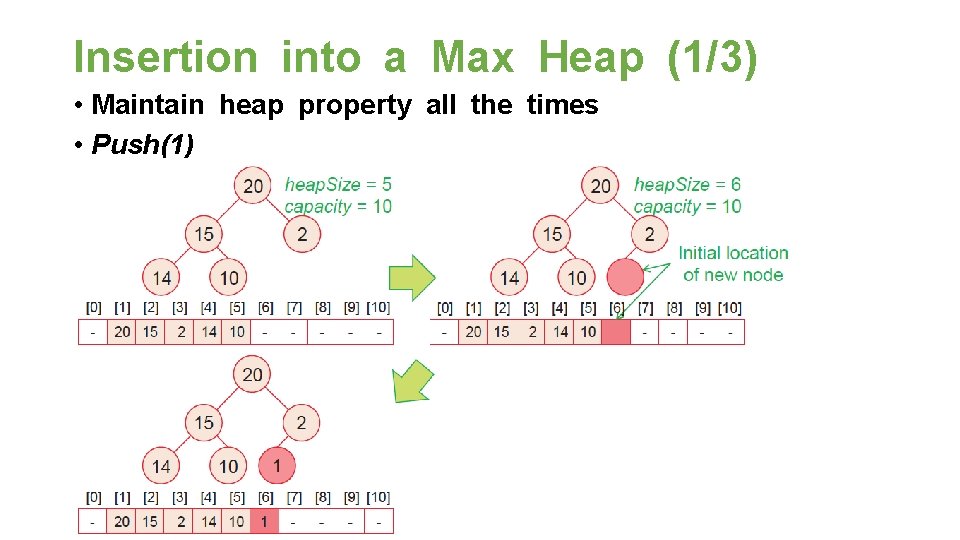
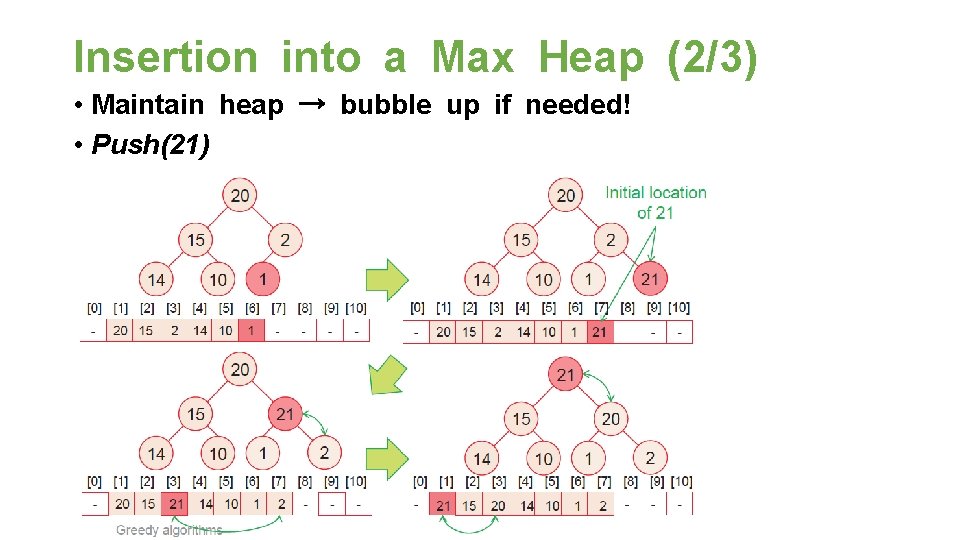
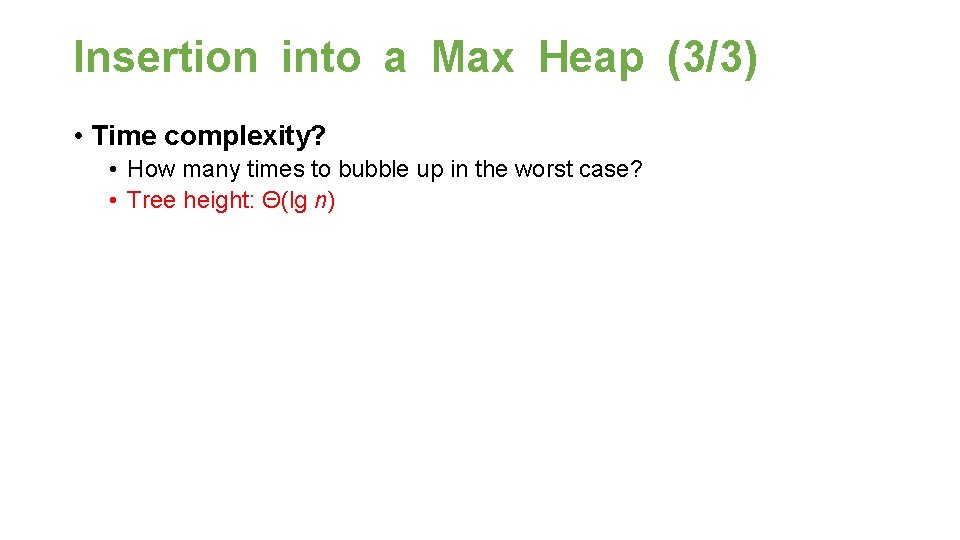
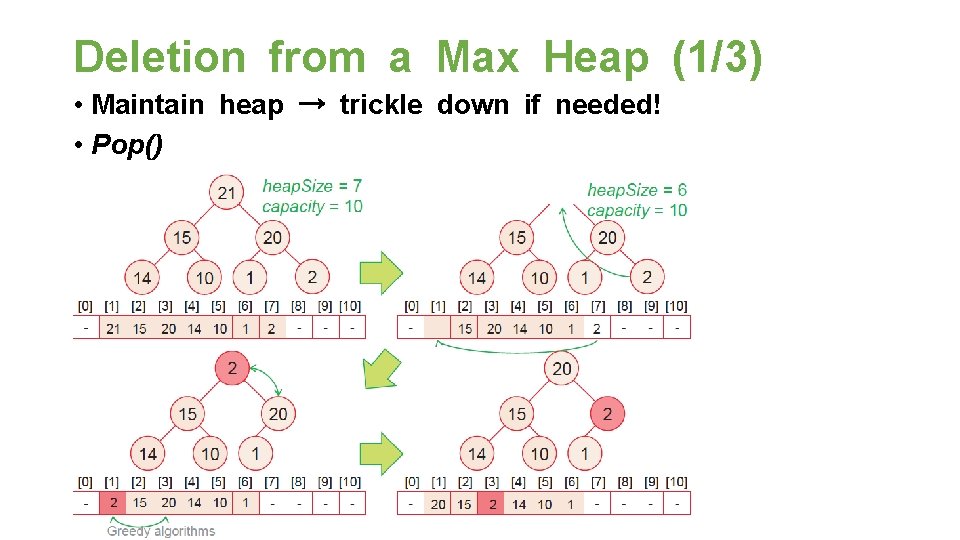
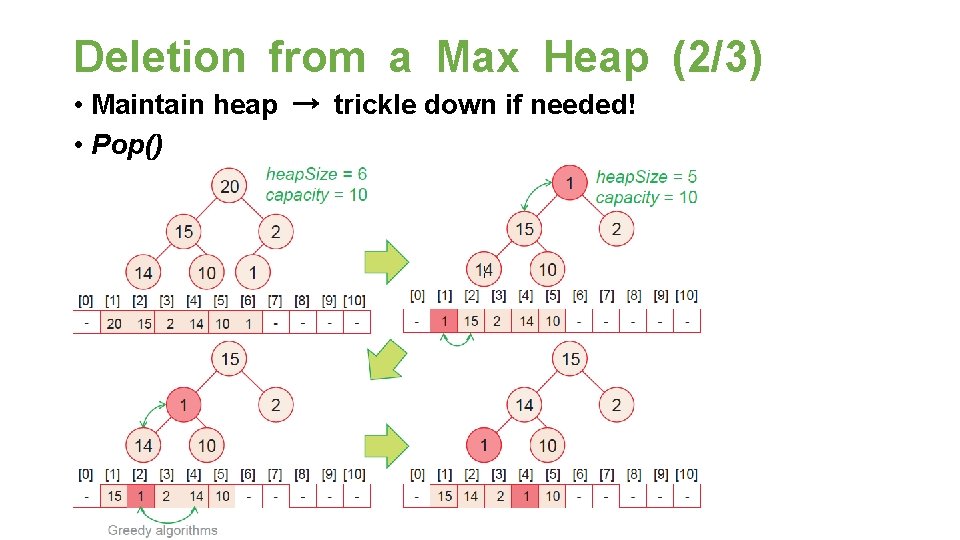
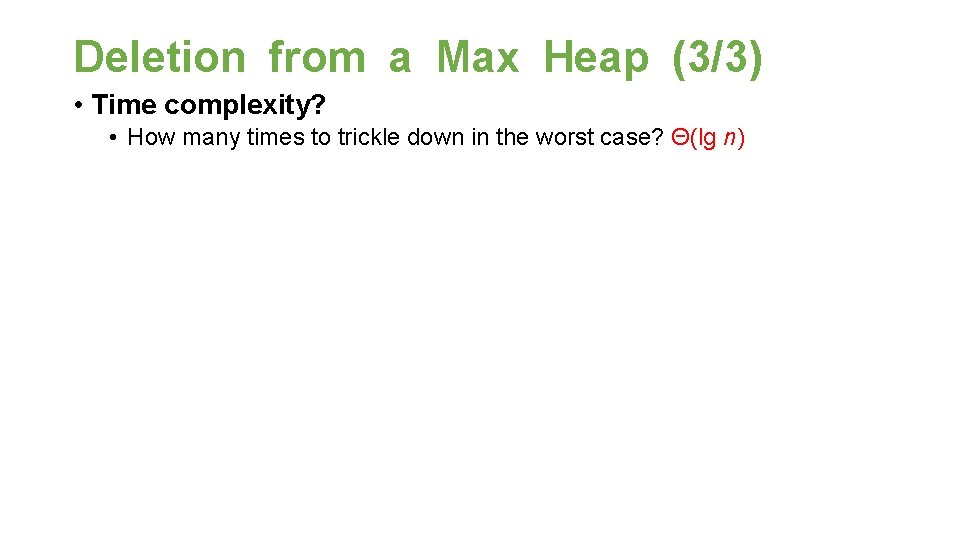
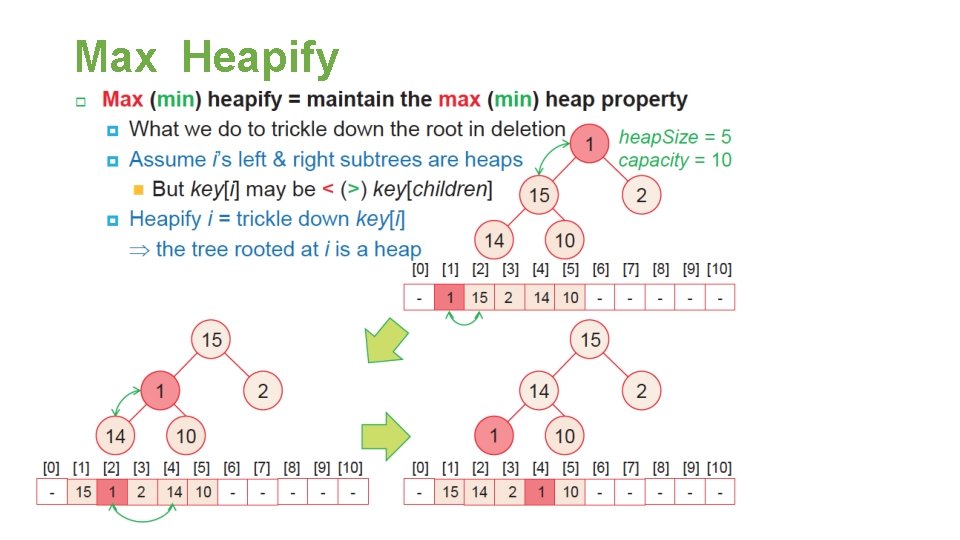
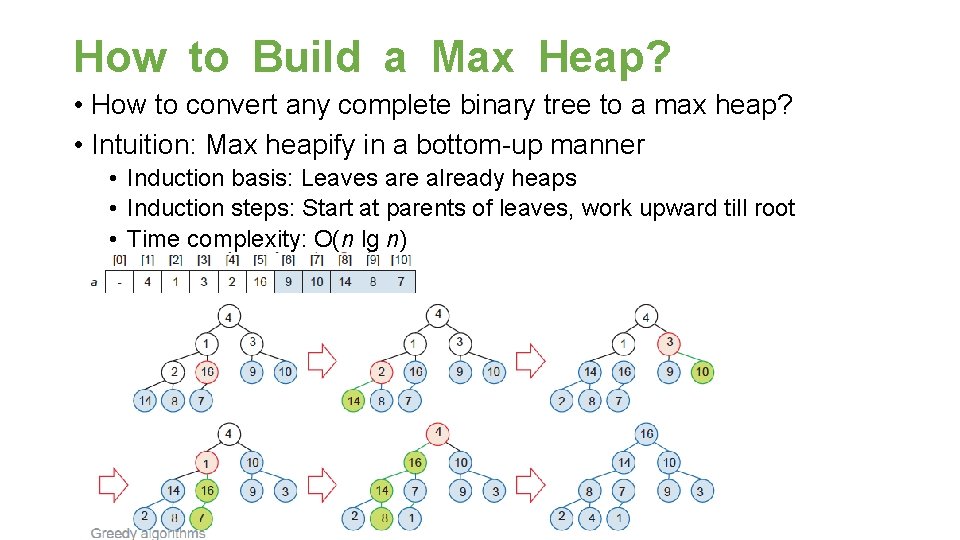
- Slides: 16
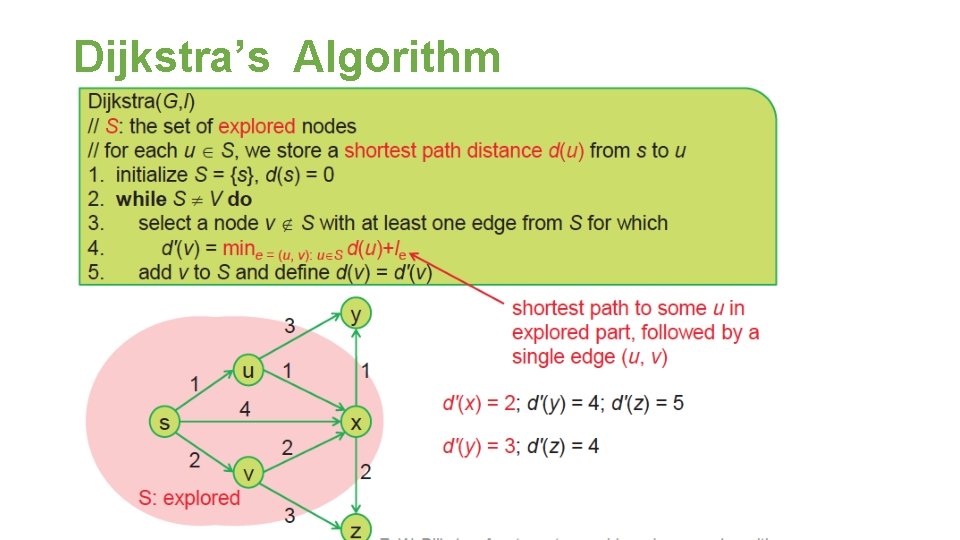
Dijkstra’s Algorithm
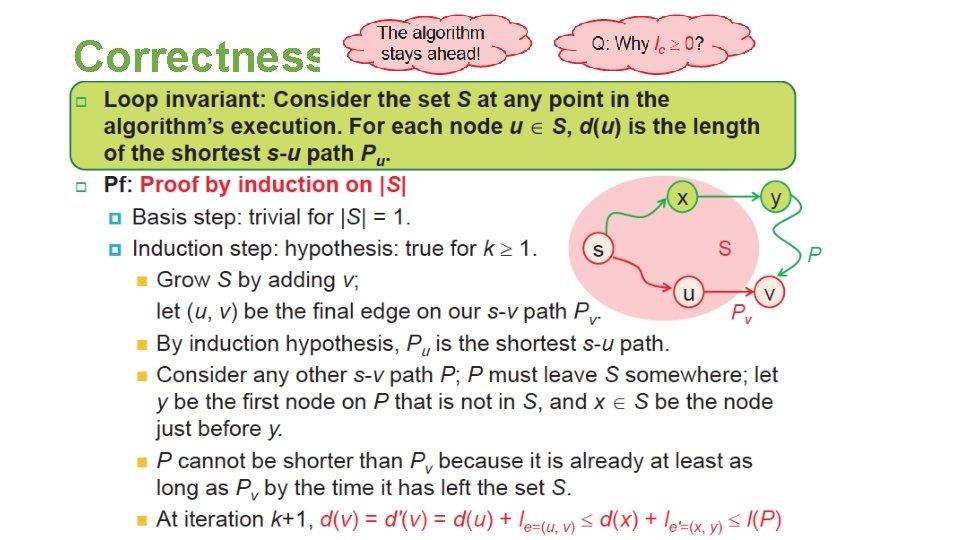
Correctness
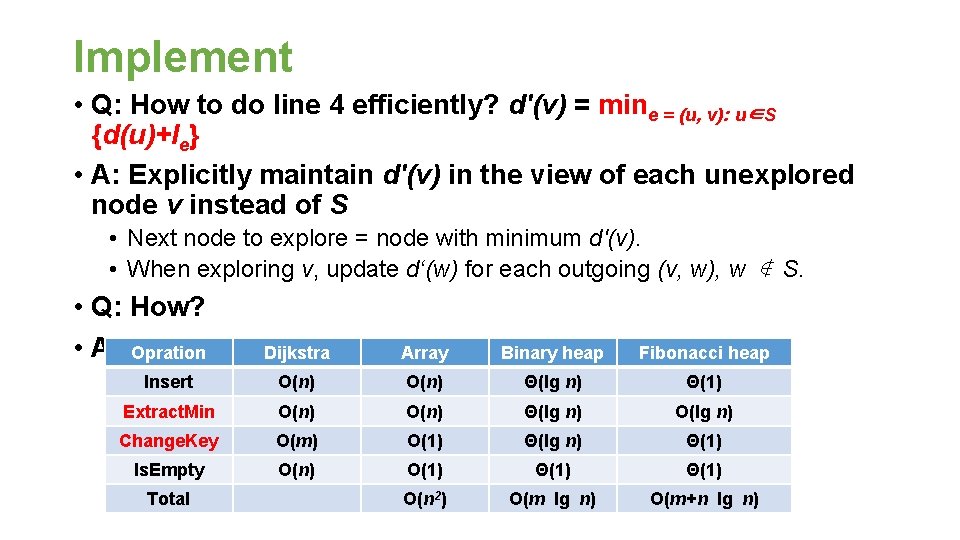
Implement • Q: How to do line 4 efficiently? d'(v) = mine = (u, v): u∈S {d(u)+le} • A: Explicitly maintain d'(v) in the view of each unexplored node v instead of S • Next node to explore = node with minimum d'(v). • When exploring v, update d‘(w) for each outgoing (v, w), w ∉ S. • Q: How? • A: Implement a min priority Opration Dijkstra Array queue Binarynicely heap Fibonacci heap Insert O(n) Θ(lg n) Θ(1) Extract. Min O(n) Θ(lg n) O(lg n) Change. Key O(m) O(1) Θ(lg n) Θ(1) Is. Empty O(n) O(1) Θ(1) O(n 2) O(m lg n) O(m+n lg n) Total
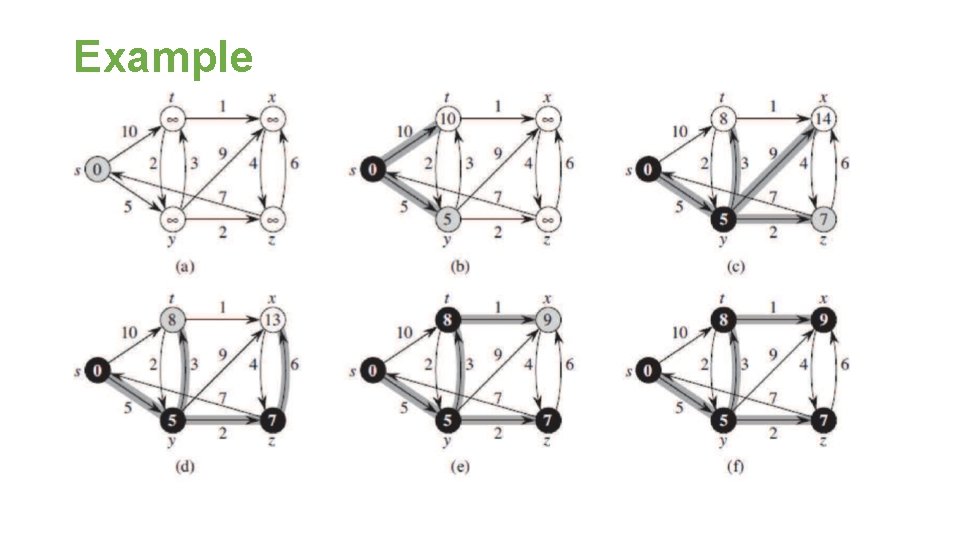
Example
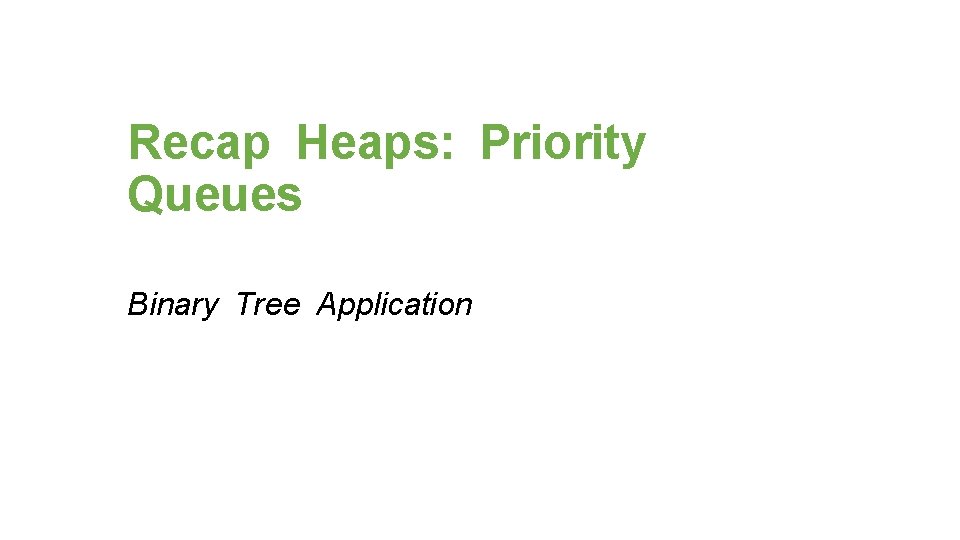
Recap Heaps: Priority Queues Binary Tree Application
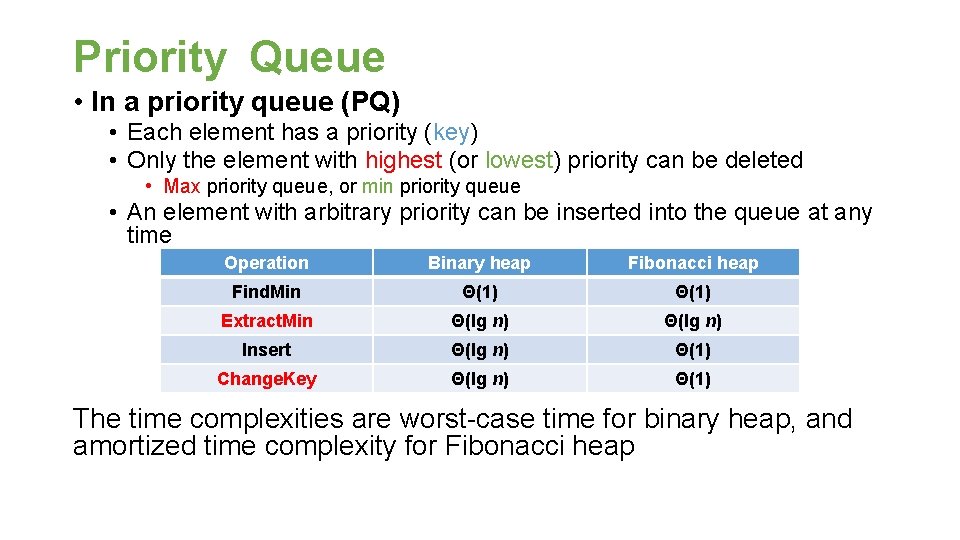
Priority Queue • In a priority queue (PQ) • Each element has a priority (key) • Only the element with highest (or lowest) priority can be deleted • Max priority queue, or min priority queue • An element with arbitrary priority can be inserted into the queue at any time Operation Binary heap Fibonacci heap Find. Min Θ(1) Extract. Min Θ(lg n) Insert Θ(lg n) Θ(1) Change. Key Θ(lg n) Θ(1) The time complexities are worst-case time for binary heap, and amortized time complexity for Fibonacci heap
![Heap Definition A max min heap is A max min tree keyparent Heap • Definition: A max (min) heap is • A max (min) tree: key[parent]](https://slidetodoc.com/presentation_image_h2/7d7ce8f2d1181a6a25c7e82bc9504723/image-7.jpg)
Heap • Definition: A max (min) heap is • A max (min) tree: key[parent] >= (<=) key[children] • A complete binary tree • Corollary: Who has the largest (smallest) key in a max (min) heap? • Root! • Example
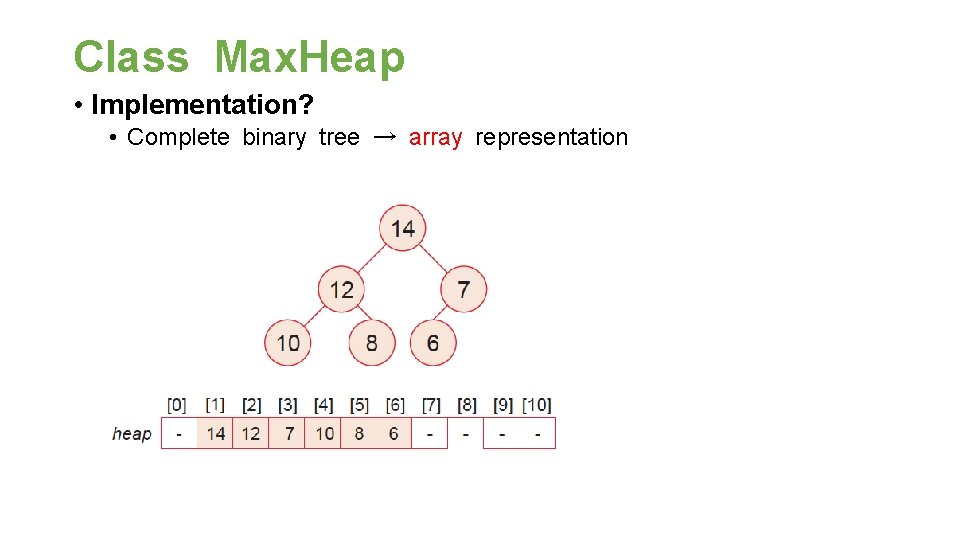
Class Max. Heap • Implementation? • Complete binary tree → array representation
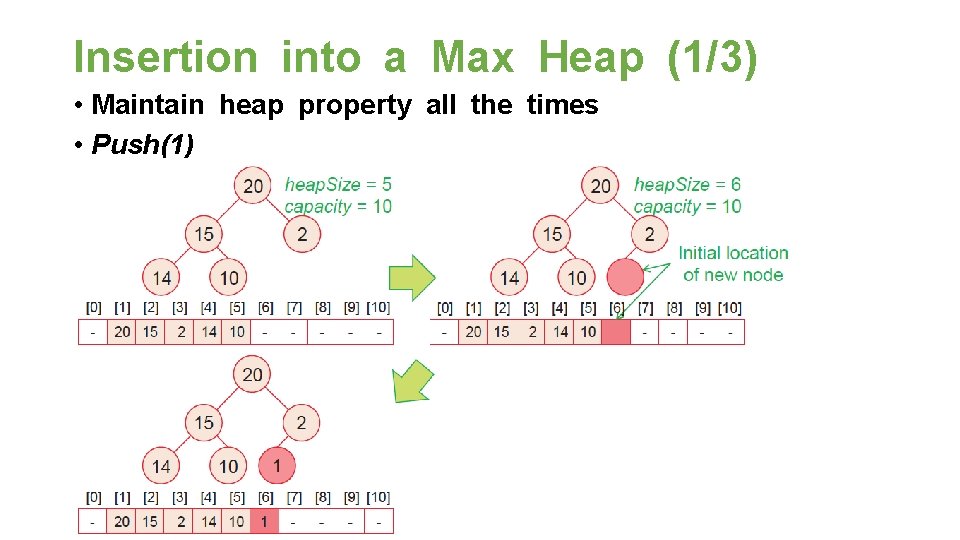
Insertion into a Max Heap (1/3) • Maintain heap property all the times • Push(1)
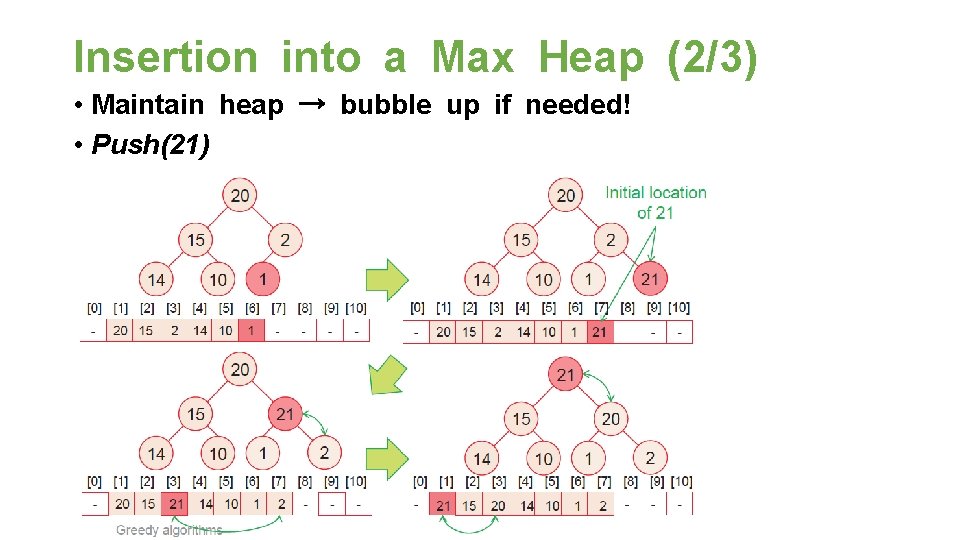
Insertion into a Max Heap (2/3) • Maintain heap → bubble up if needed! • Push(21)
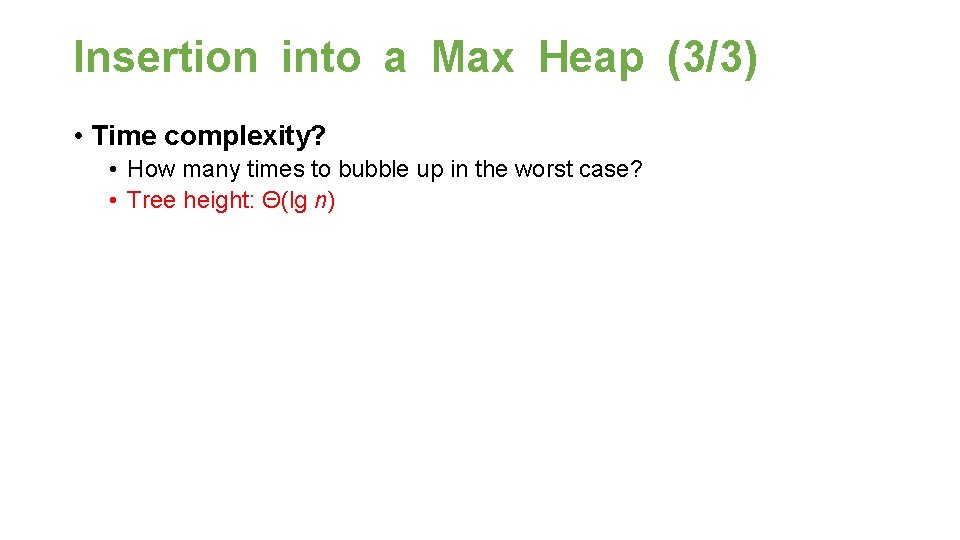
Insertion into a Max Heap (3/3) • Time complexity? • How many times to bubble up in the worst case? • Tree height: Θ(lg n)
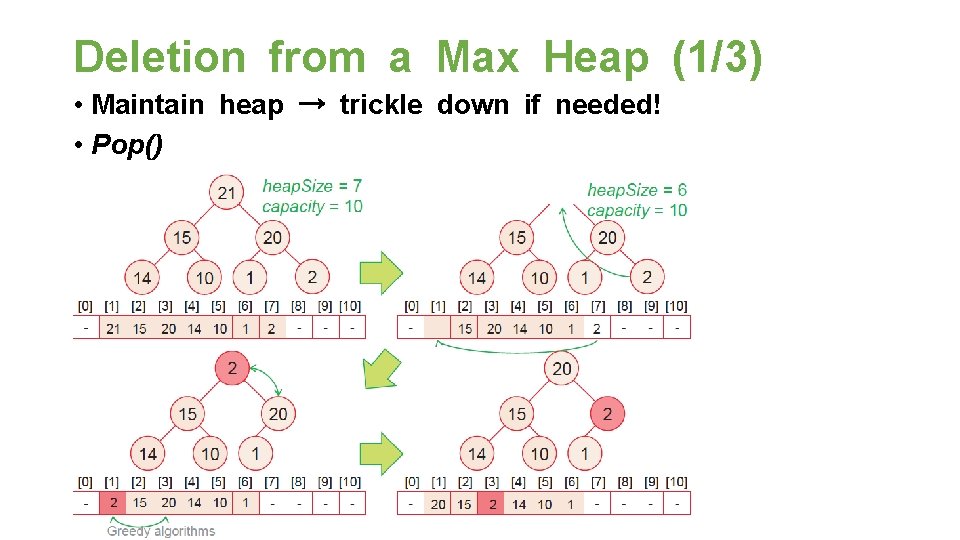
Deletion from a Max Heap (1/3) • Maintain heap → trickle down if needed! • Pop()
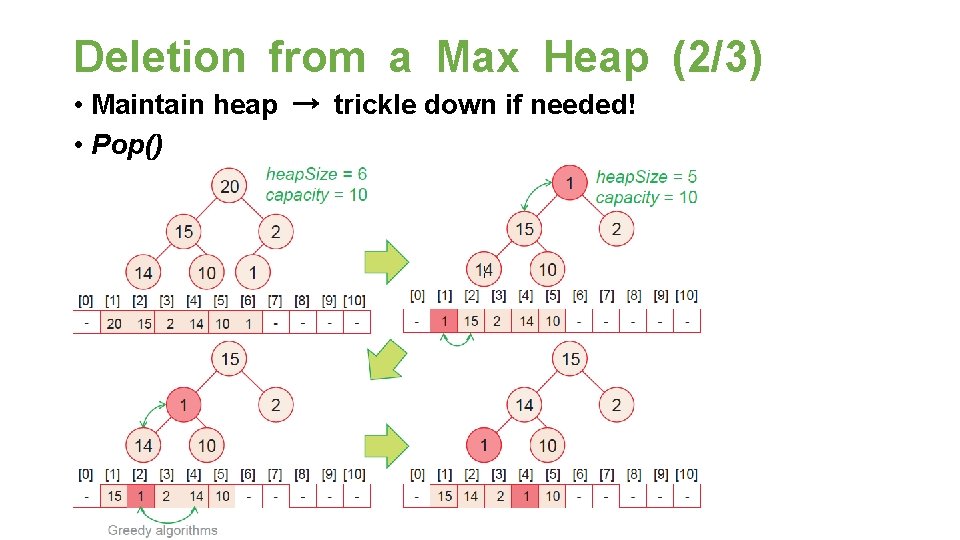
Deletion from a Max Heap (2/3) • Maintain heap → trickle down if needed! • Pop()
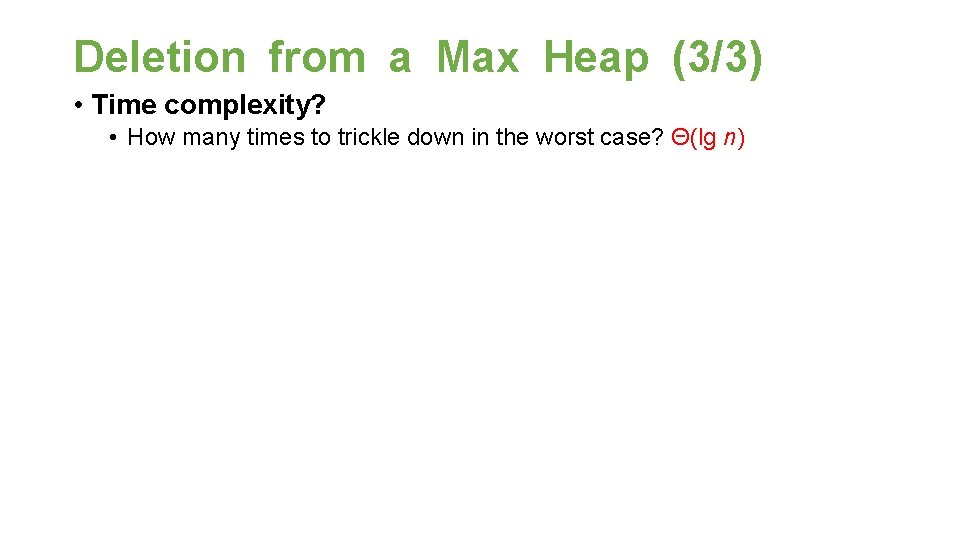
Deletion from a Max Heap (3/3) • Time complexity? • How many times to trickle down in the worst case? Θ(lg n)
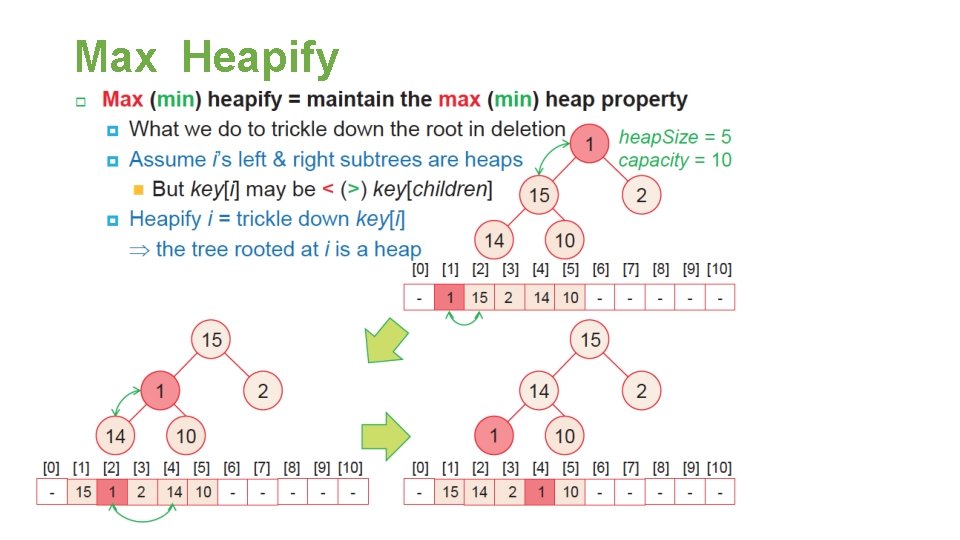
Max Heapify
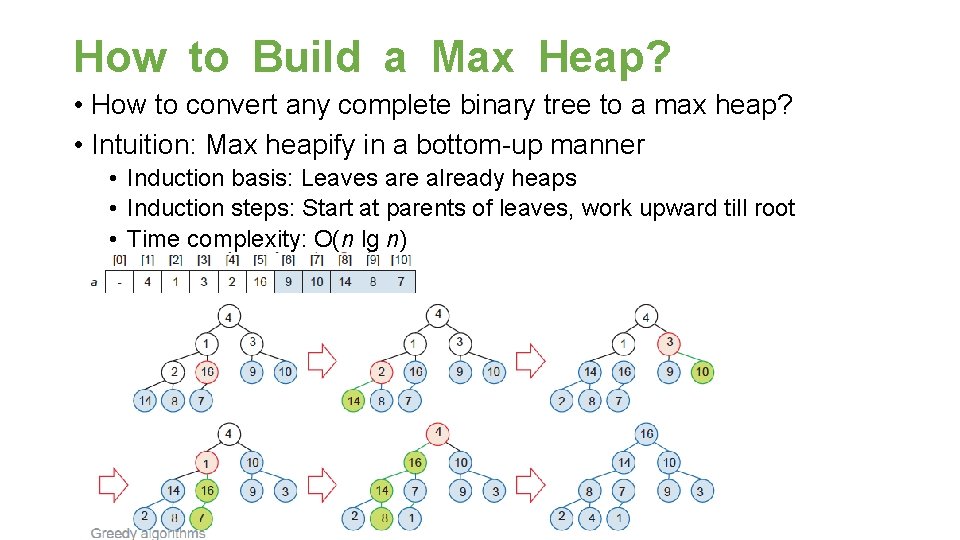
How to Build a Max Heap? • How to convert any complete binary tree to a max heap? • Intuition: Max heapify in a bottom-up manner • Induction basis: Leaves are already heaps • Induction steps: Start at parents of leaves, work upward till root • Time complexity: O(n lg n)
Loop invariant for merge sort
Proof of correctness examples
What is program correctness
Completness in communication
Correctness rules of fragmentation
Correctness and the loop invariant
Bfs proof of correctness
Correctness of fragmentation
Correctness of fragmentation
Entity integrity ensures correctness of the data in a table
Functional correctness
Loop invariant of bubble sort
Divide and conquer
Dynamic connectivity problem
Reliability vs correctness
7c's of communication
V=u+at is dimensionally correct