Dependency Injection Inversion of Control and Frameworks OOD
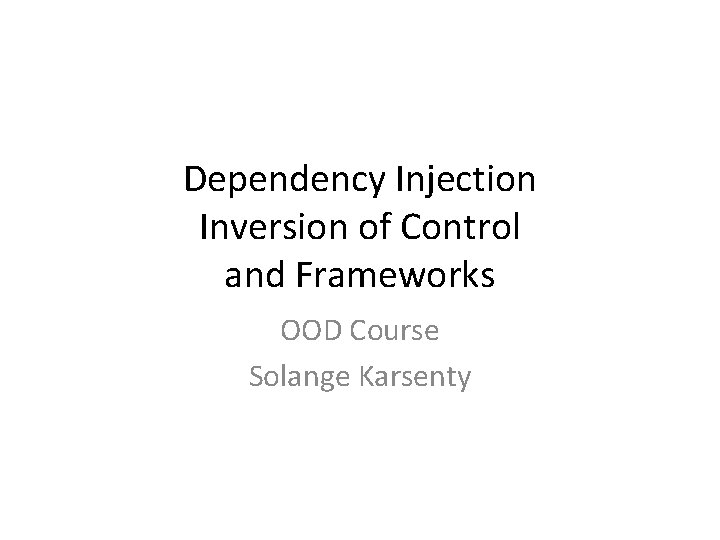
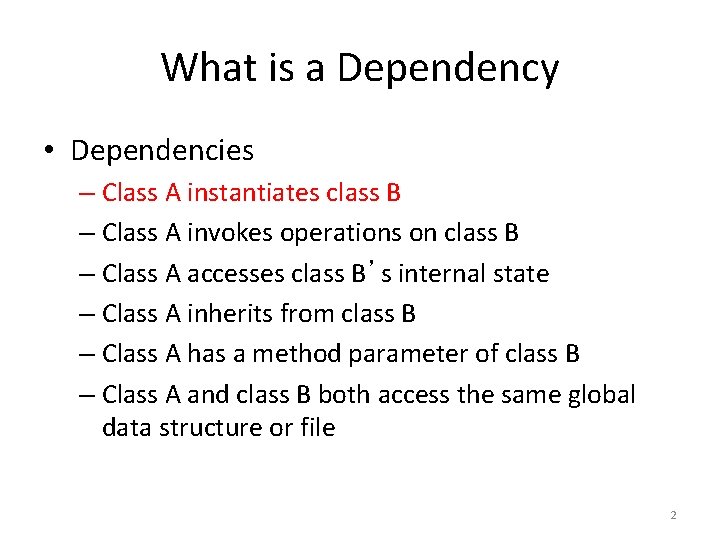
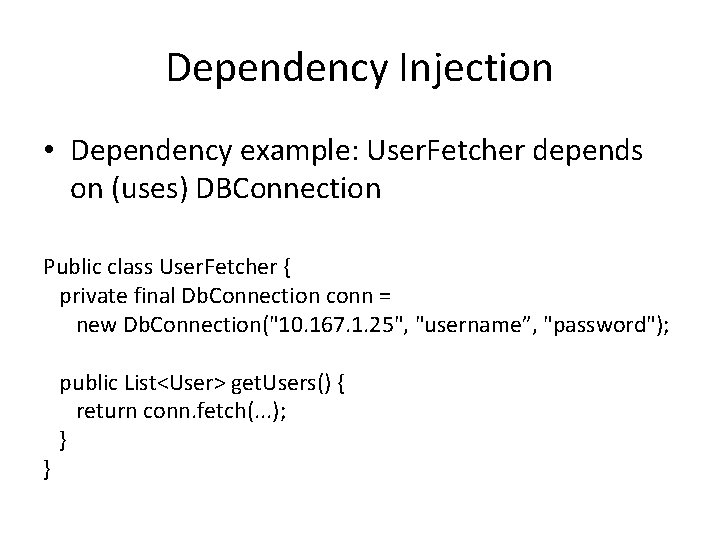
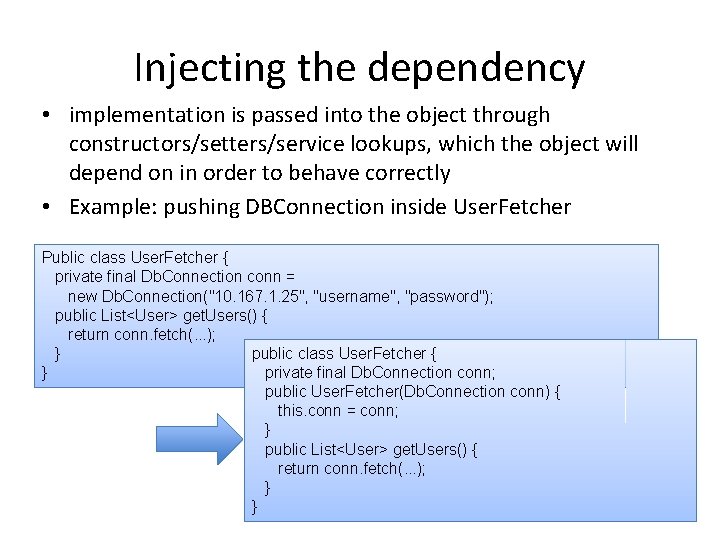
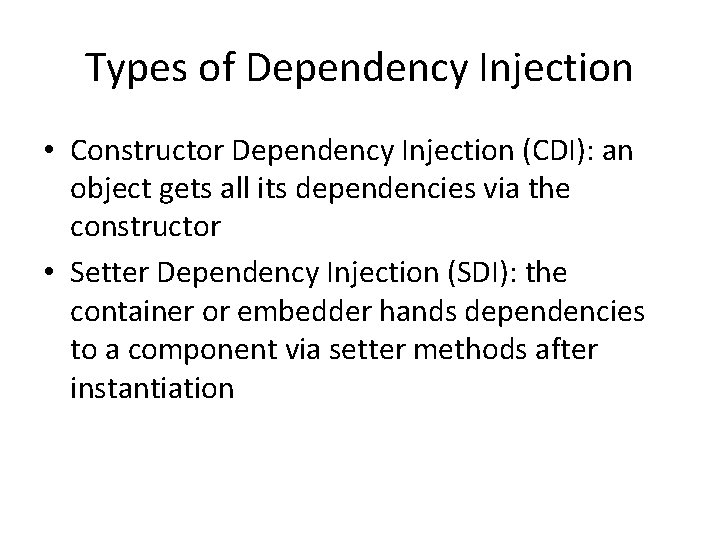
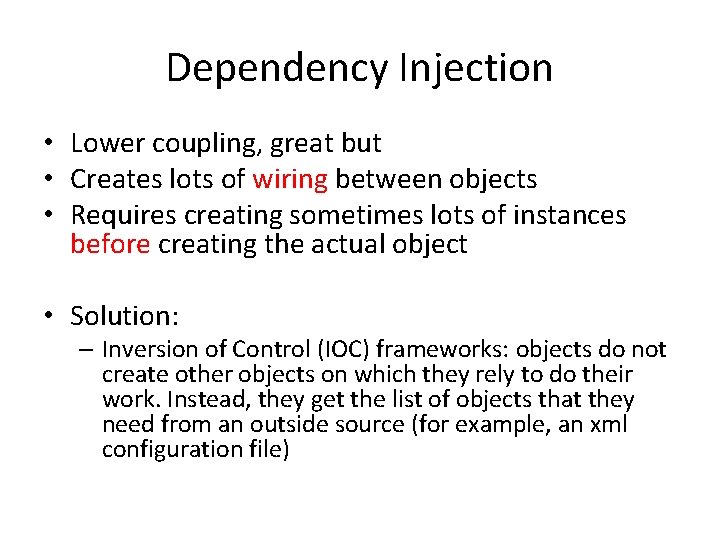
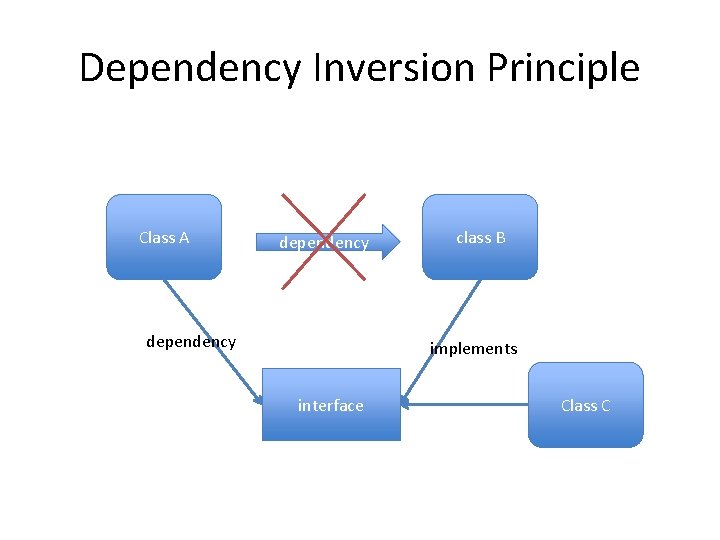
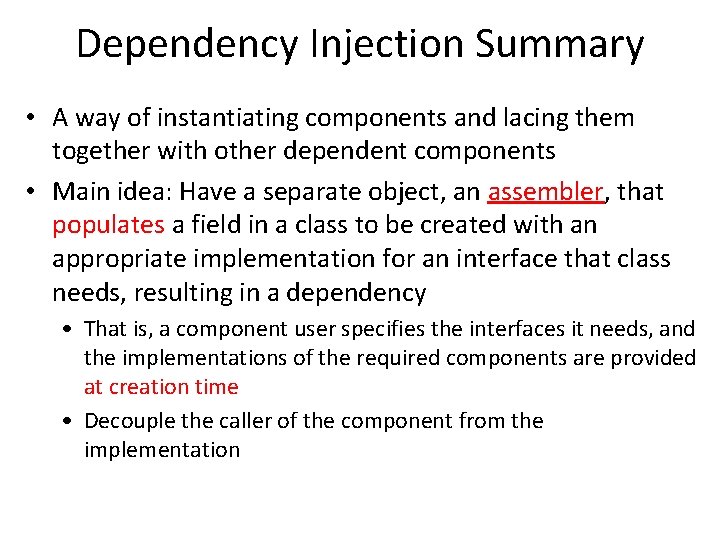
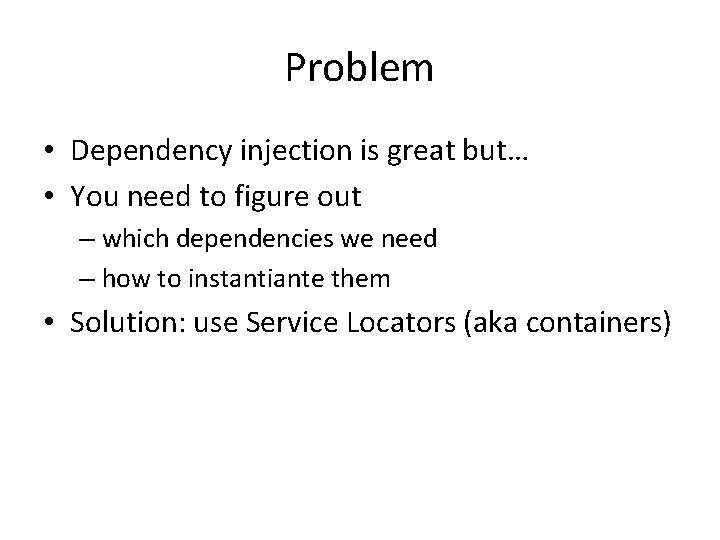
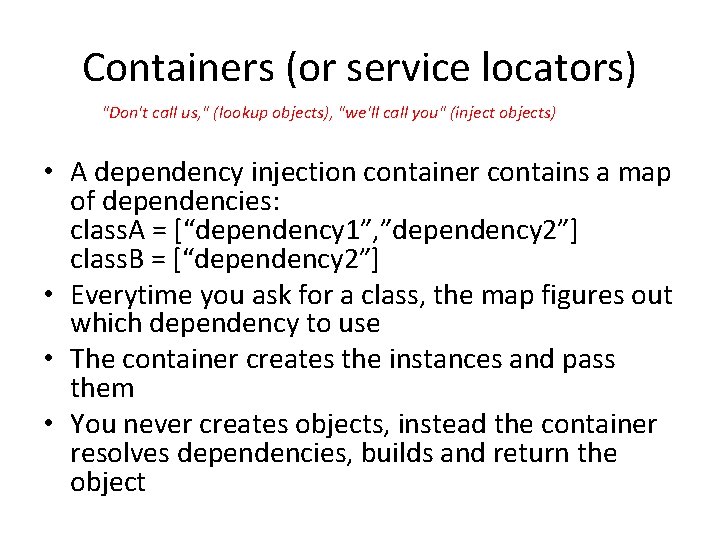
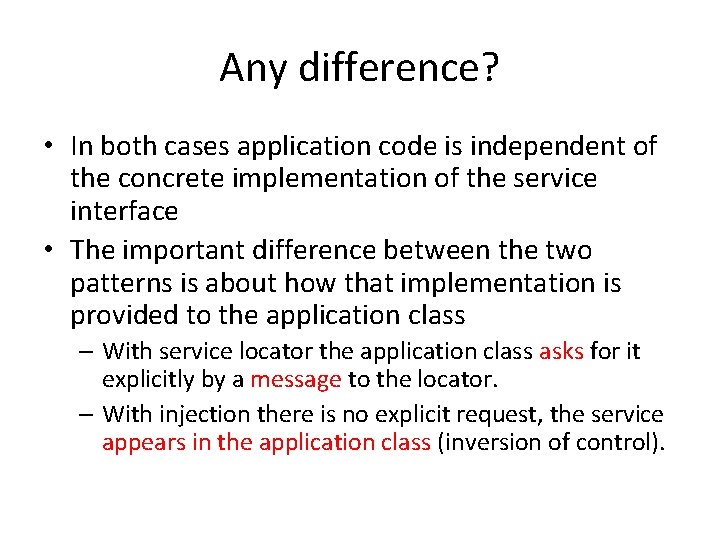
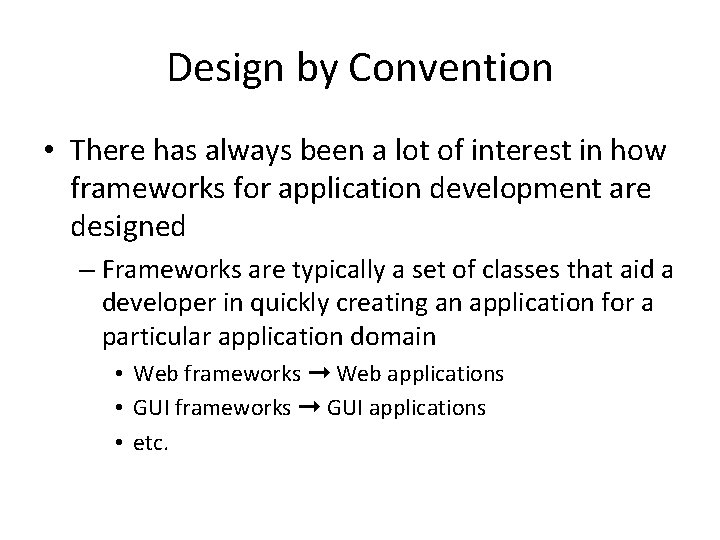
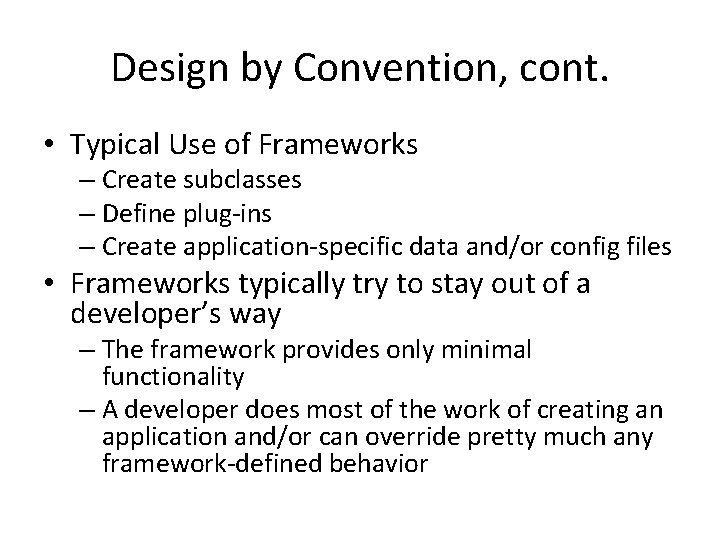
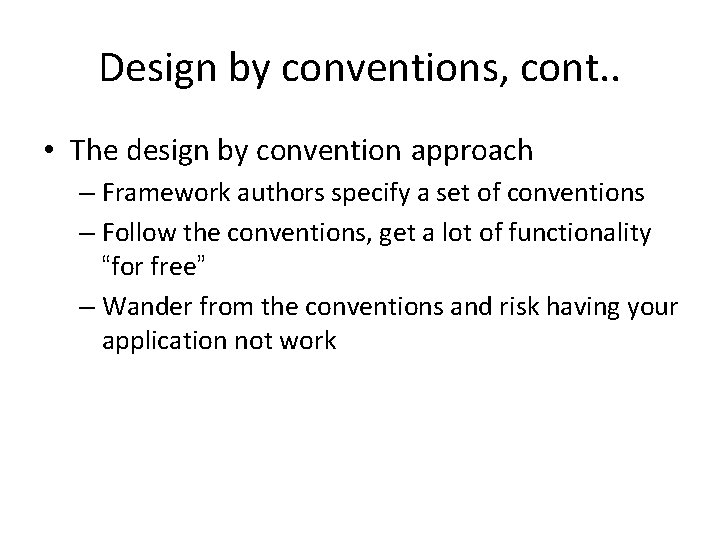
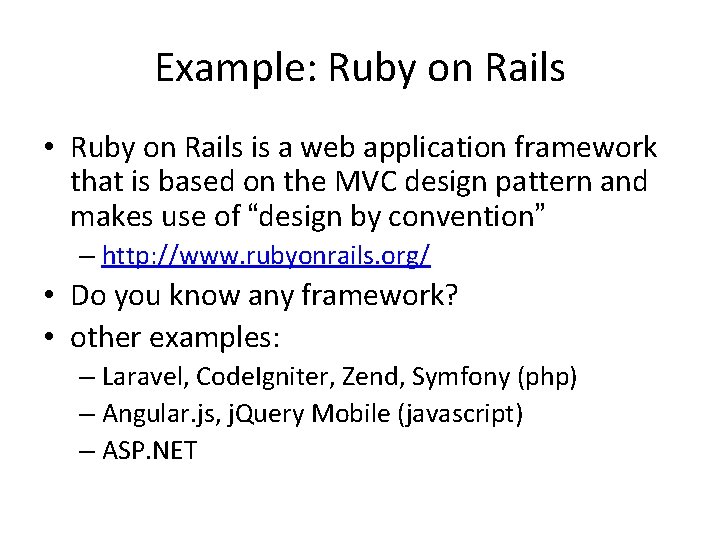
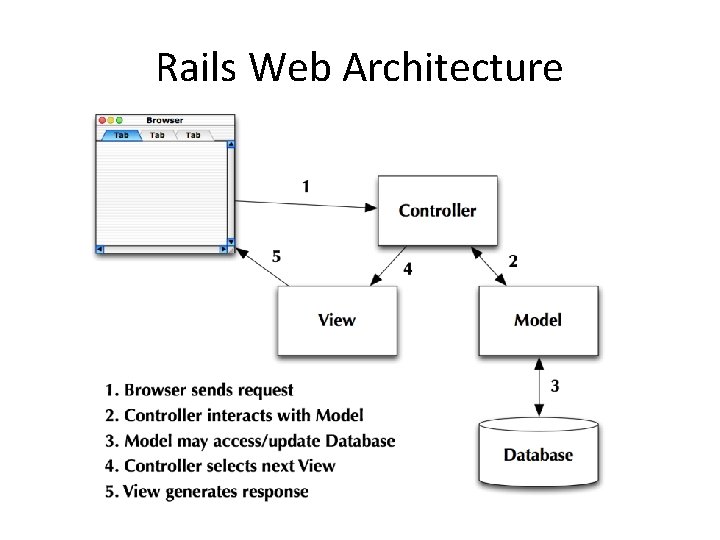
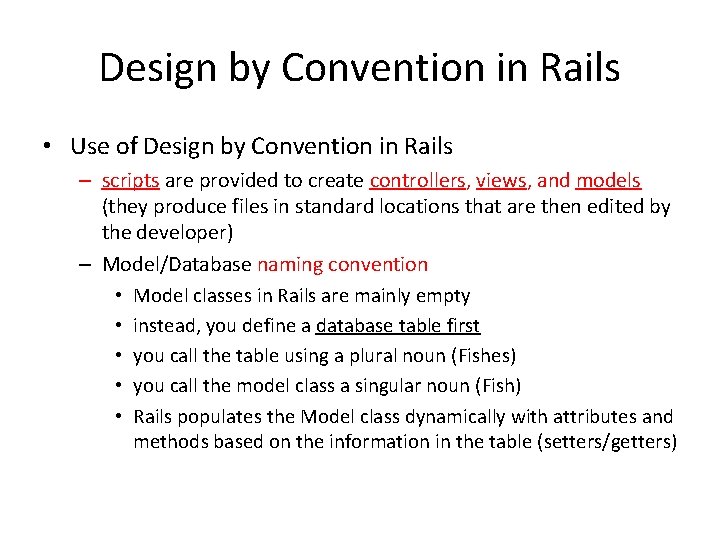
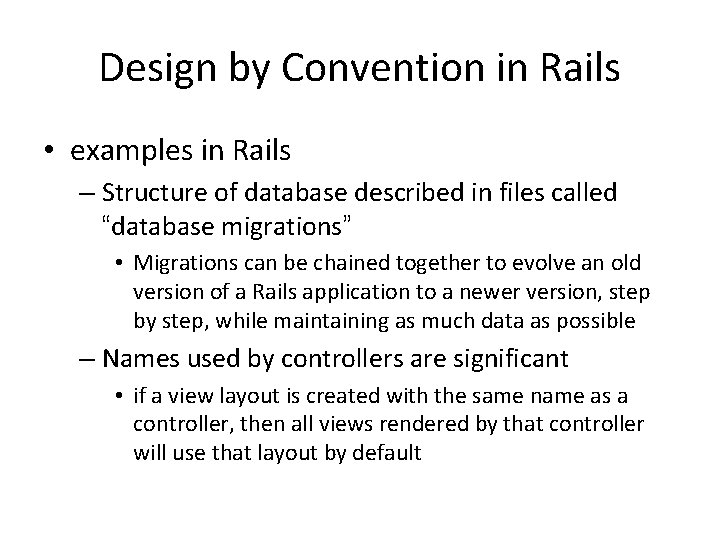
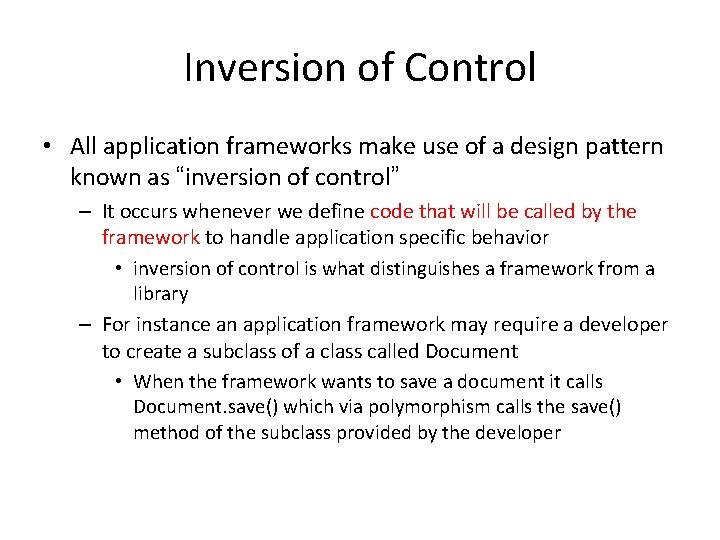
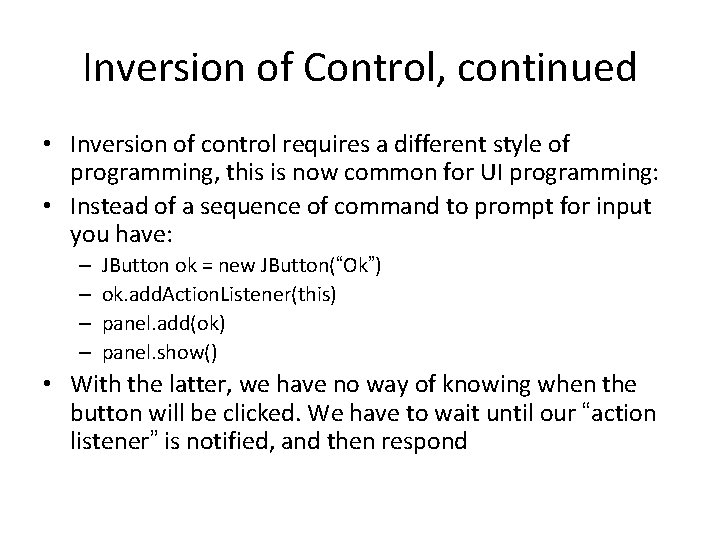
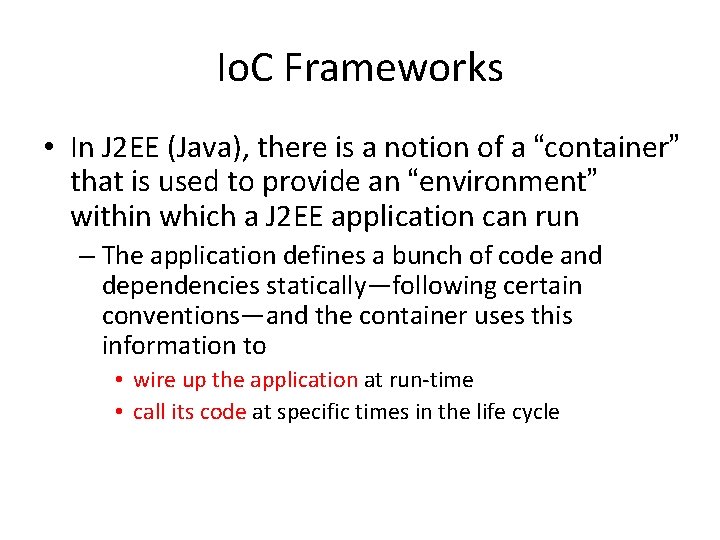
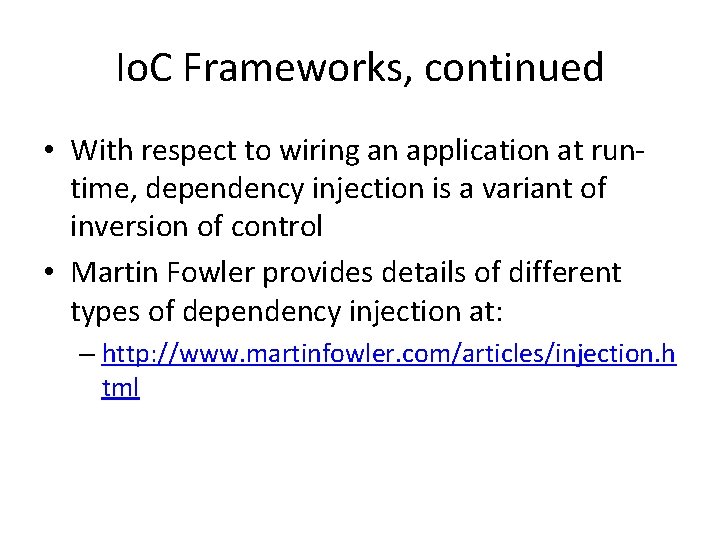
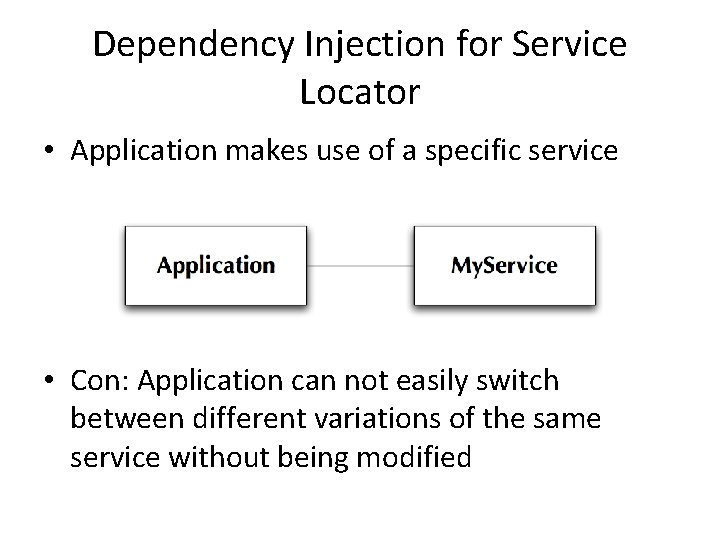
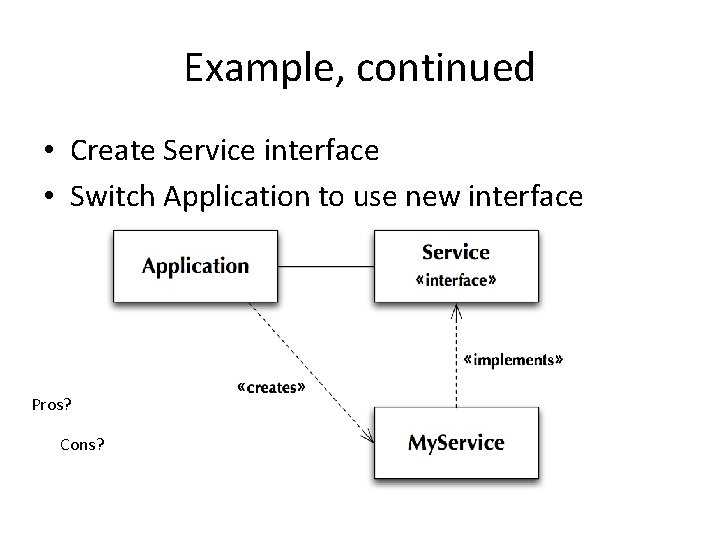
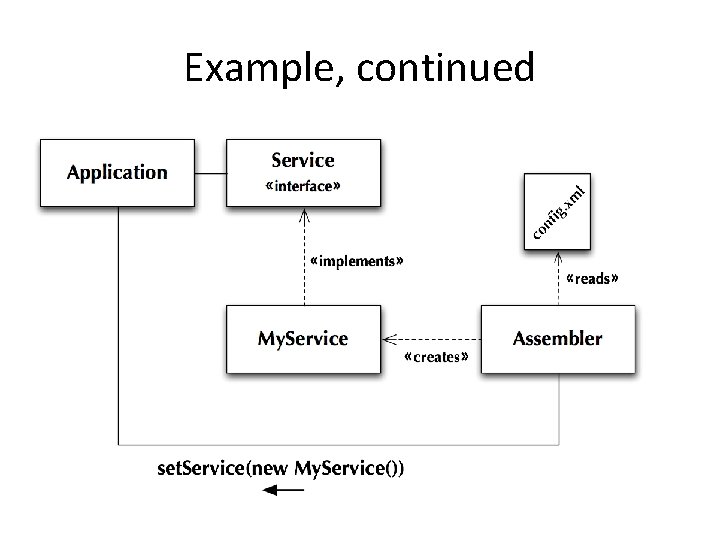
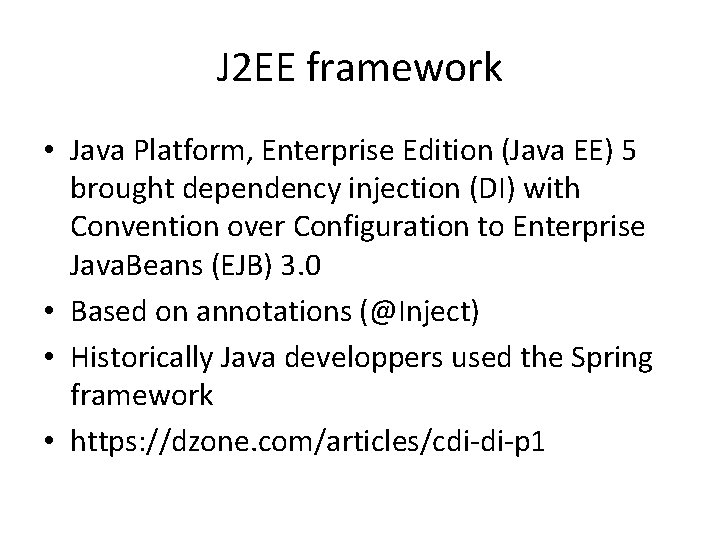
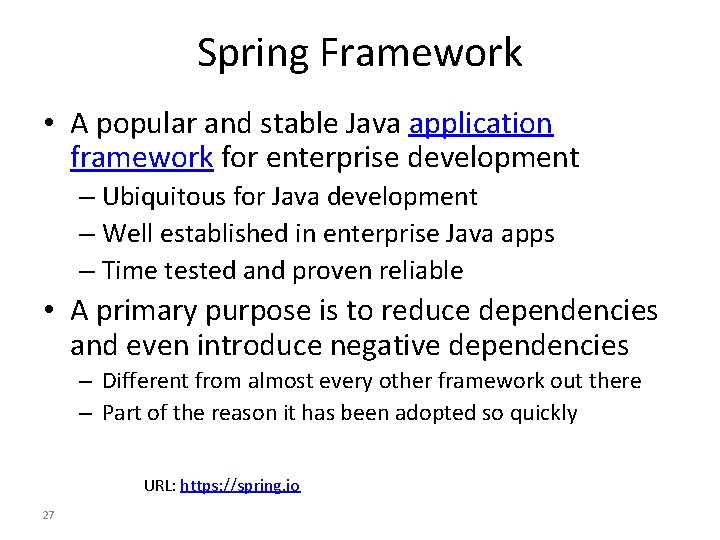
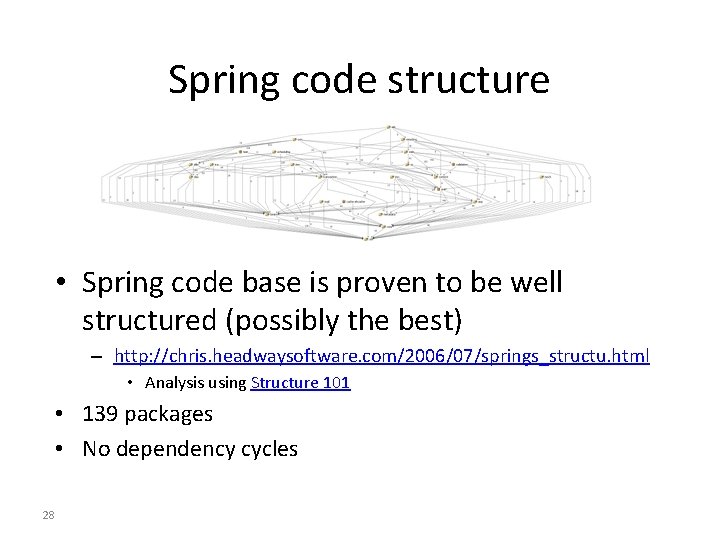
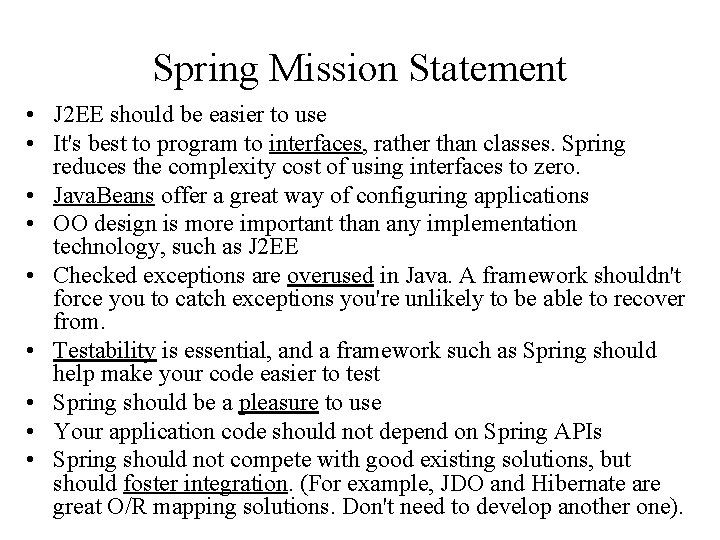
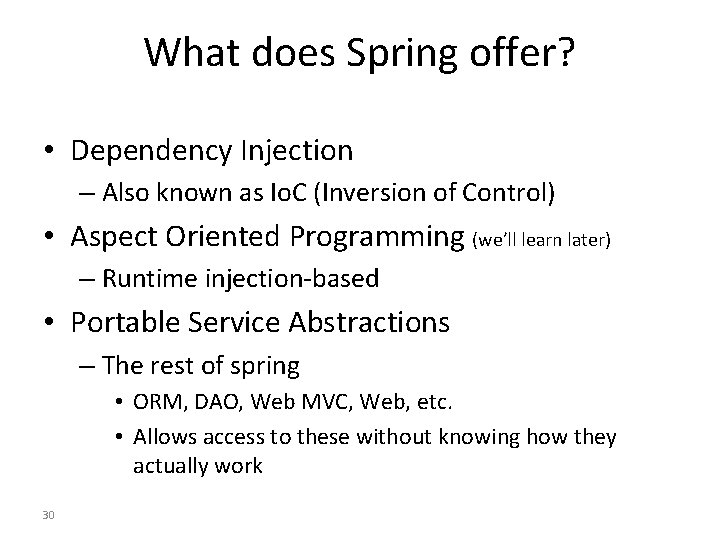
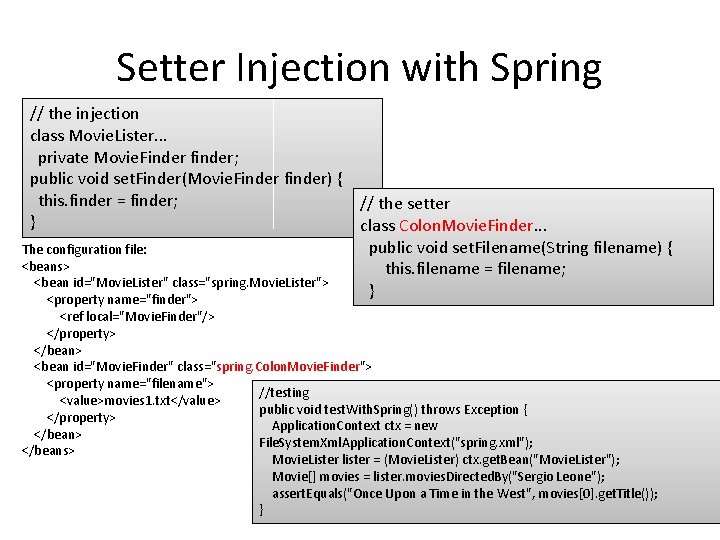
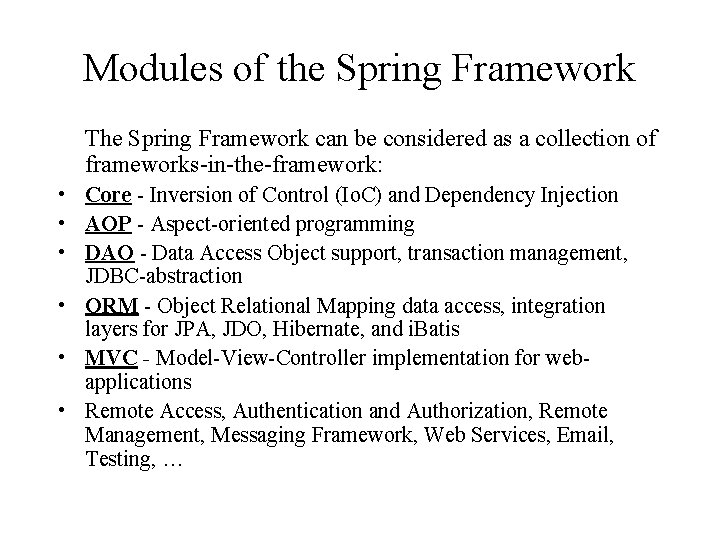
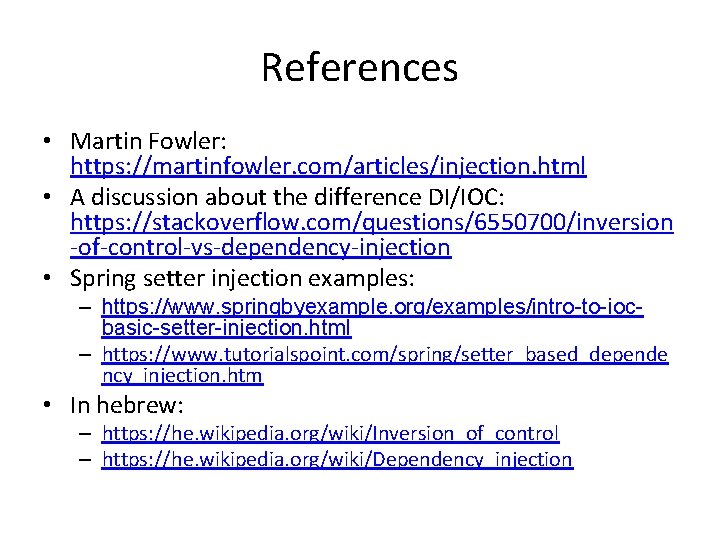
- Slides: 33
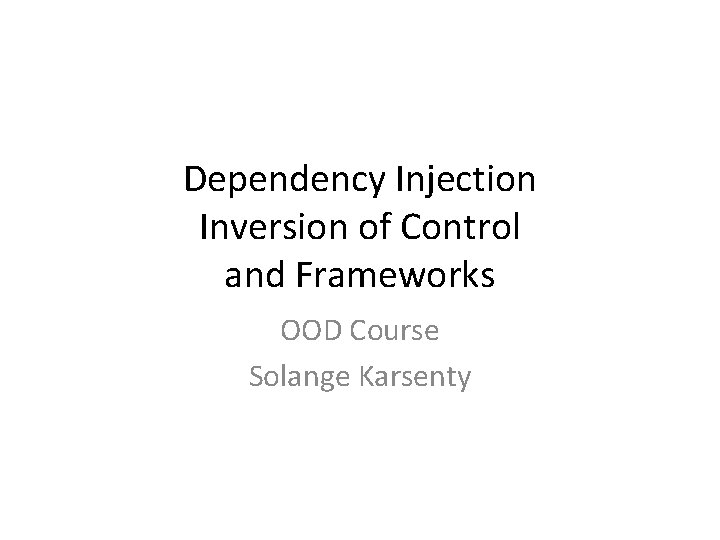
Dependency Injection Inversion of Control and Frameworks OOD Course Solange Karsenty
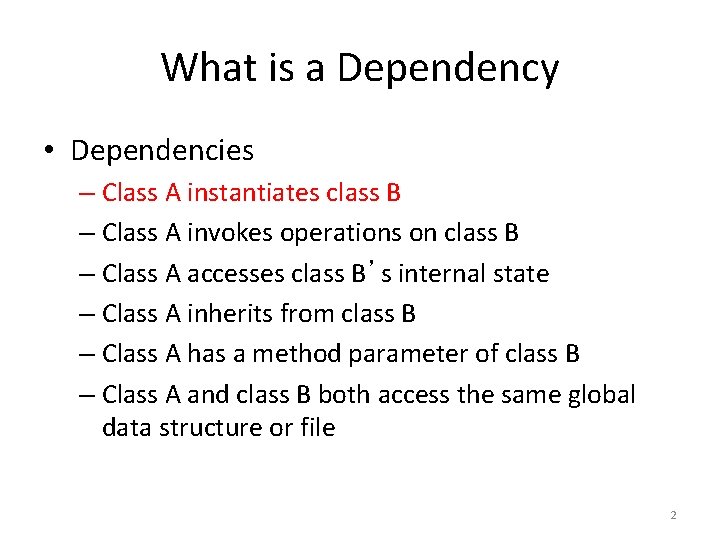
What is a Dependency • Dependencies – Class A instantiates class B – Class A invokes operations on class B – Class A accesses class B’s internal state – Class A inherits from class B – Class A has a method parameter of class B – Class A and class B both access the same global data structure or file 2
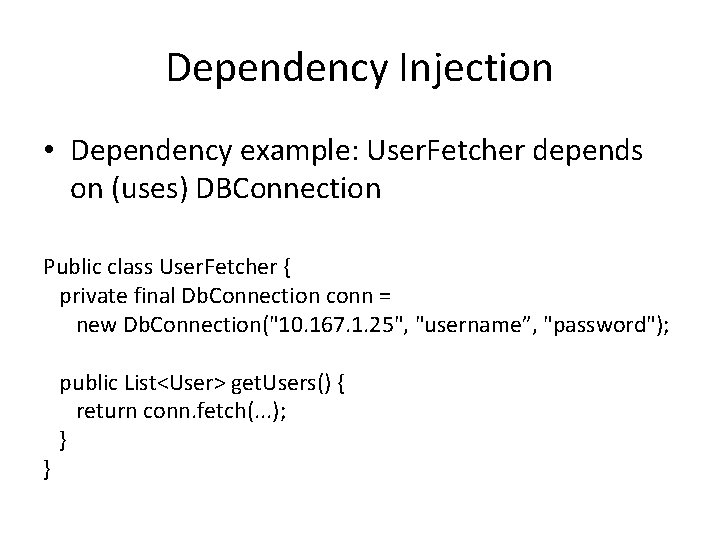
Dependency Injection • Dependency example: User. Fetcher depends on (uses) DBConnection Public class User. Fetcher { private final Db. Connection conn = new Db. Connection("10. 167. 1. 25", "username”, "password"); } public List<User> get. Users() { return conn. fetch(. . . ); }
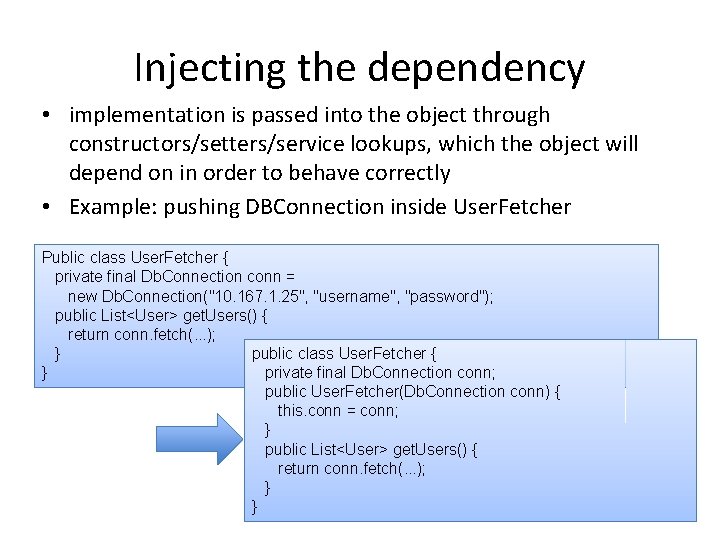
Injecting the dependency • implementation is passed into the object through constructors/setters/service lookups, which the object will depend on in order to behave correctly • Example: pushing DBConnection inside User. Fetcher Public class User. Fetcher { private final Db. Connection conn = new Db. Connection("10. 167. 1. 25", "username", "password"); public List<User> get. Users() { return conn. fetch(. . . ); public class User. Fetcher { } private final Db. Connection conn; } public User. Fetcher(Db. Connection conn) { this. conn = conn; } public List<User> get. Users() { return conn. fetch(. . . ); } }
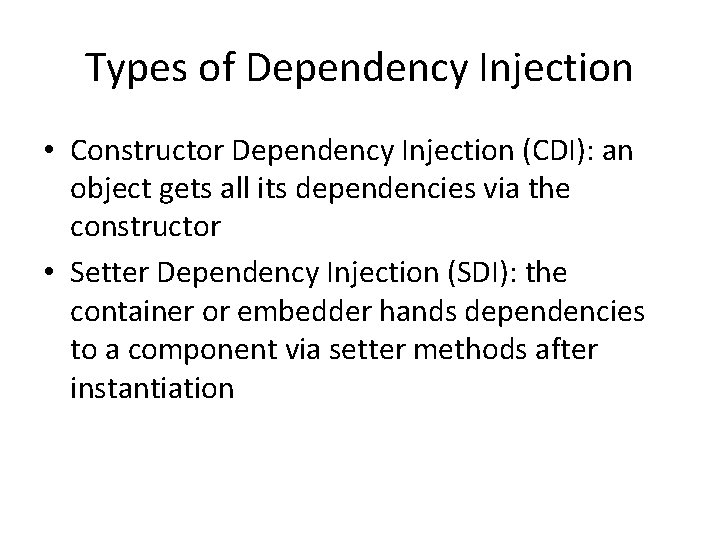
Types of Dependency Injection • Constructor Dependency Injection (CDI): an object gets all its dependencies via the constructor • Setter Dependency Injection (SDI): the container or embedder hands dependencies to a component via setter methods after instantiation
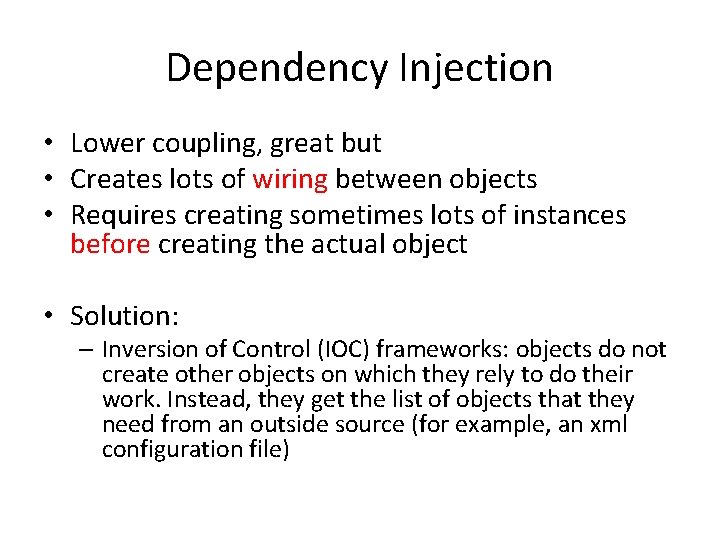
Dependency Injection • Lower coupling, great but • Creates lots of wiring between objects • Requires creating sometimes lots of instances before creating the actual object • Solution: – Inversion of Control (IOC) frameworks: objects do not create other objects on which they rely to do their work. Instead, they get the list of objects that they need from an outside source (for example, an xml configuration file)
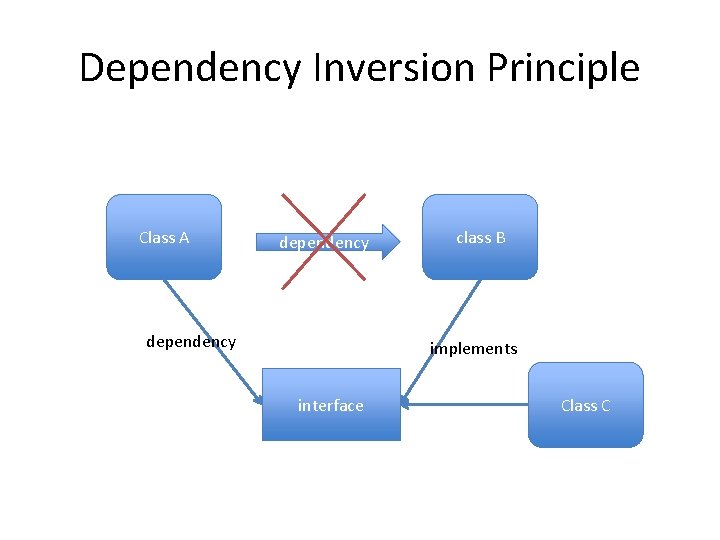
Dependency Inversion Principle Class A dependency class B implements interface Class C
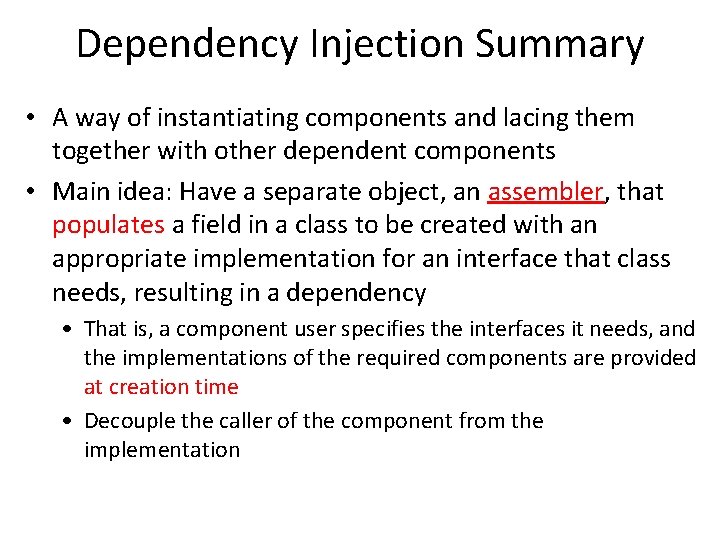
Dependency Injection Summary • A way of instantiating components and lacing them together with other dependent components • Main idea: Have a separate object, an assembler, that populates a field in a class to be created with an appropriate implementation for an interface that class needs, resulting in a dependency • That is, a component user specifies the interfaces it needs, and the implementations of the required components are provided at creation time • Decouple the caller of the component from the implementation
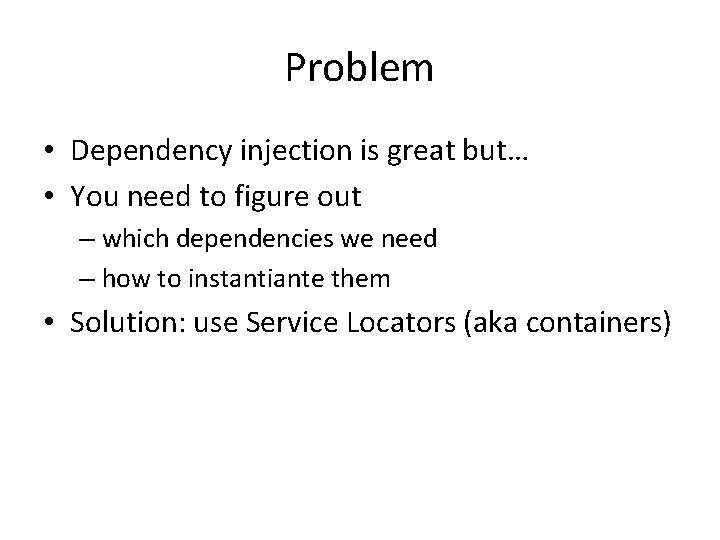
Problem • Dependency injection is great but… • You need to figure out – which dependencies we need – how to instantiante them • Solution: use Service Locators (aka containers)
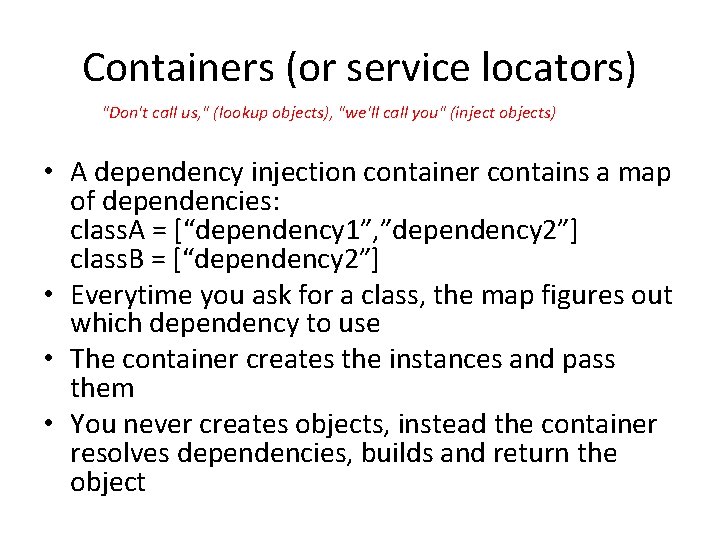
Containers (or service locators) "Don't call us‚" (lookup objects), "we'll call you" (inject objects) • A dependency injection container contains a map of dependencies: class. A = [“dependency 1”, ”dependency 2”] class. B = [“dependency 2”] • Everytime you ask for a class, the map figures out which dependency to use • The container creates the instances and pass them • You never creates objects, instead the container resolves dependencies, builds and return the object
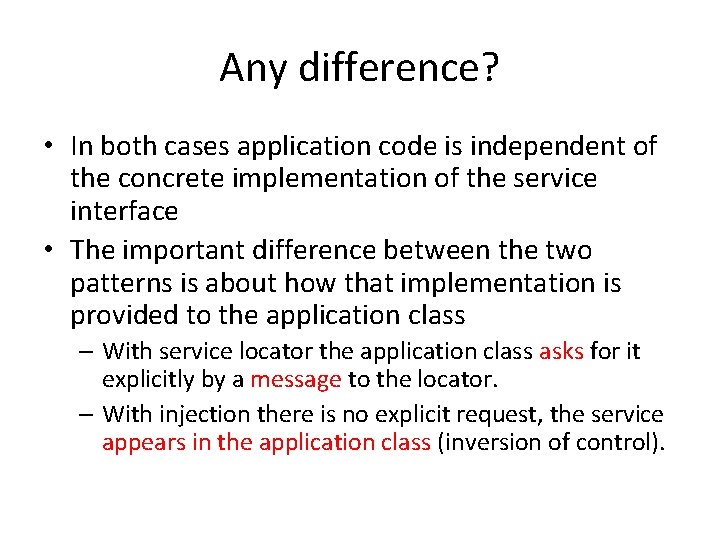
Any difference? • In both cases application code is independent of the concrete implementation of the service interface • The important difference between the two patterns is about how that implementation is provided to the application class – With service locator the application class asks for it explicitly by a message to the locator. – With injection there is no explicit request, the service appears in the application class (inversion of control).
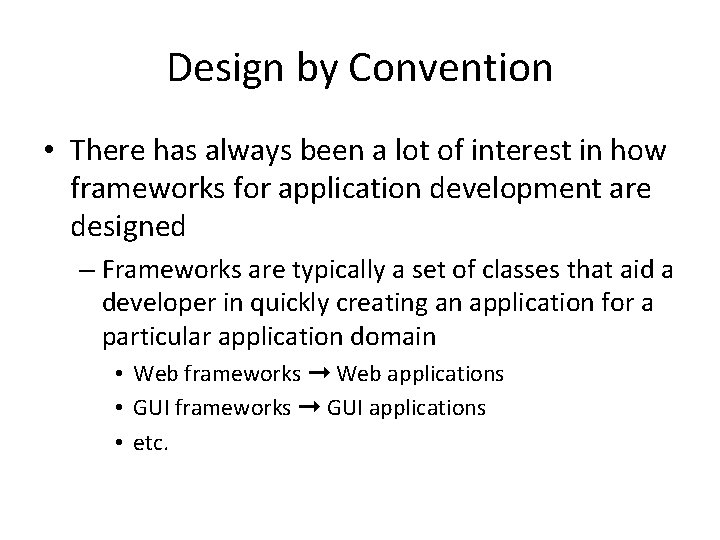
Design by Convention • There has always been a lot of interest in how frameworks for application development are designed – Frameworks are typically a set of classes that aid a developer in quickly creating an application for a particular application domain • Web frameworks ➞ Web applications • GUI frameworks ➞ GUI applications • etc.
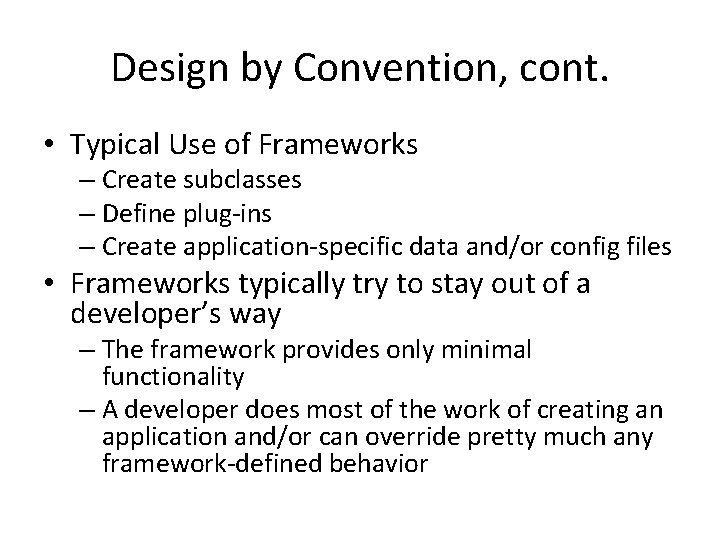
Design by Convention, cont. • Typical Use of Frameworks – Create subclasses – Define plug-ins – Create application-specific data and/or config files • Frameworks typically try to stay out of a developer’s way – The framework provides only minimal functionality – A developer does most of the work of creating an application and/or can override pretty much any framework-defined behavior
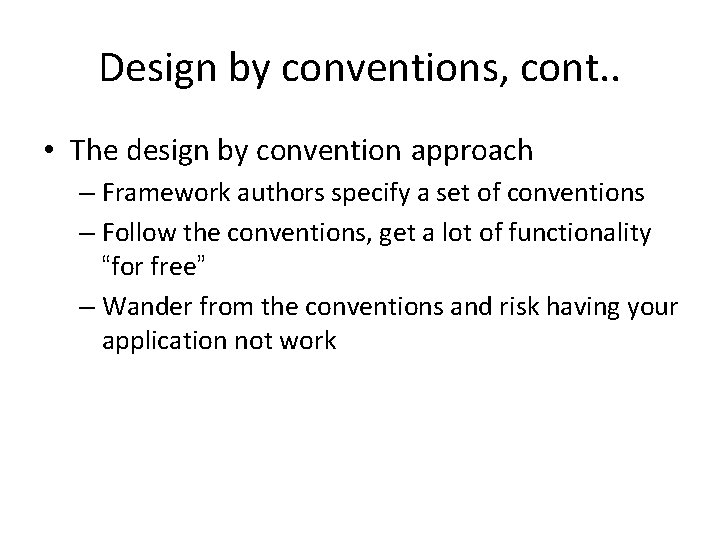
Design by conventions, cont. . • The design by convention approach – Framework authors specify a set of conventions – Follow the conventions, get a lot of functionality “for free” – Wander from the conventions and risk having your application not work
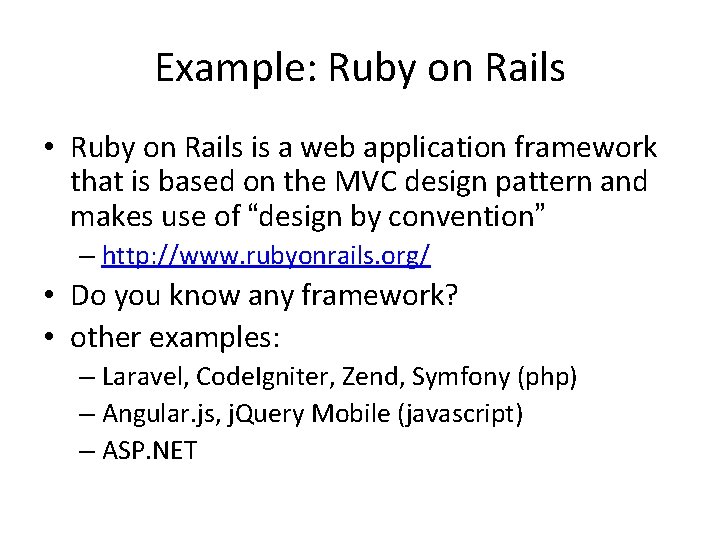
Example: Ruby on Rails • Ruby on Rails is a web application framework that is based on the MVC design pattern and makes use of “design by convention” – http: //www. rubyonrails. org/ • Do you know any framework? • other examples: – Laravel, Code. Igniter, Zend, Symfony (php) – Angular. js, j. Query Mobile (javascript) – ASP. NET
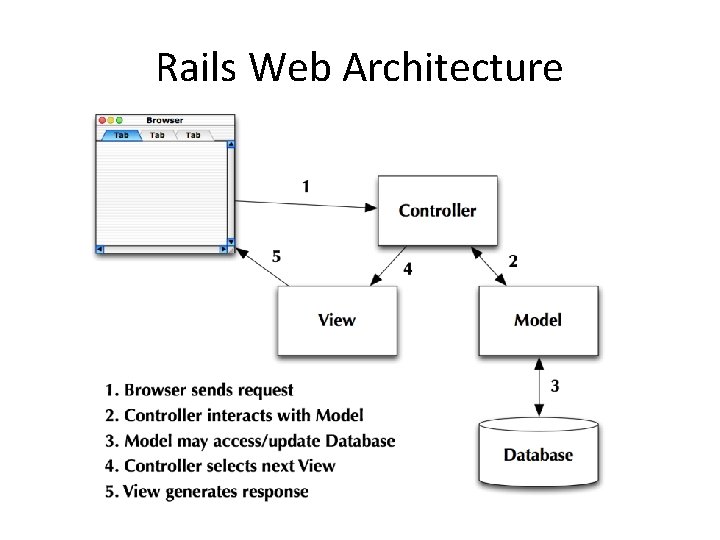
Rails Web Architecture
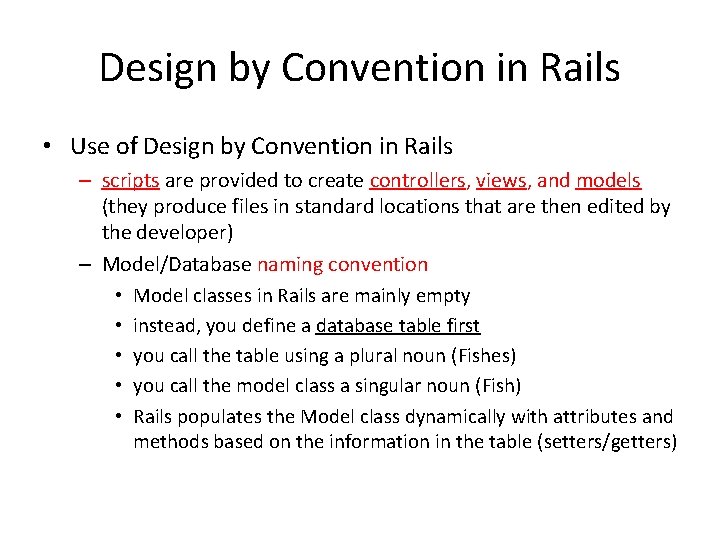
Design by Convention in Rails • Use of Design by Convention in Rails – scripts are provided to create controllers, views, and models (they produce files in standard locations that are then edited by the developer) – Model/Database naming convention • Model classes in Rails are mainly empty • instead, you define a database table first • you call the table using a plural noun (Fishes) • you call the model class a singular noun (Fish) • Rails populates the Model class dynamically with attributes and methods based on the information in the table (setters/getters)
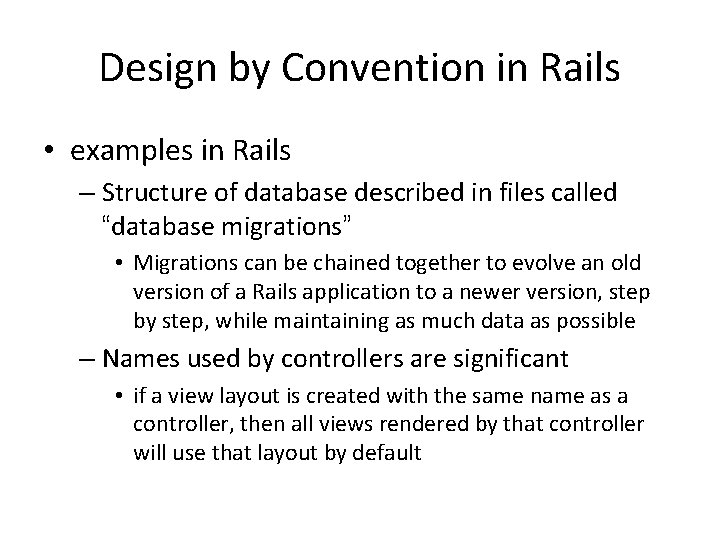
Design by Convention in Rails • examples in Rails – Structure of database described in files called “database migrations” • Migrations can be chained together to evolve an old version of a Rails application to a newer version, step by step, while maintaining as much data as possible – Names used by controllers are significant • if a view layout is created with the same name as a controller, then all views rendered by that controller will use that layout by default
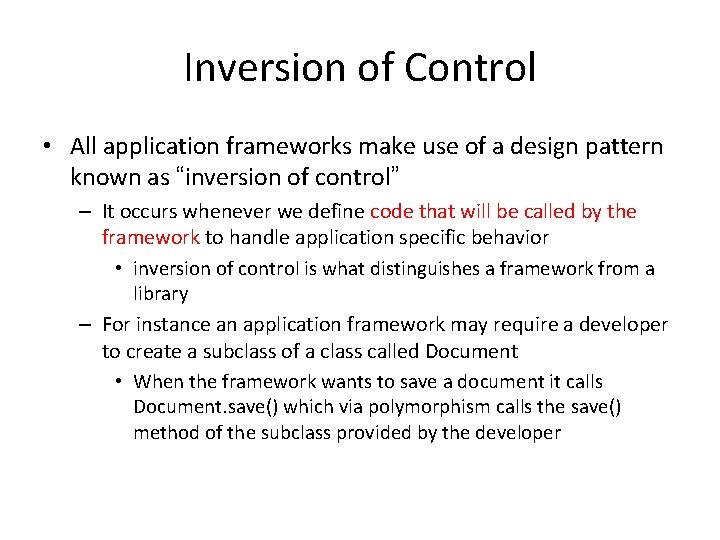
Inversion of Control • All application frameworks make use of a design pattern known as “inversion of control” – It occurs whenever we define code that will be called by the framework to handle application specific behavior • inversion of control is what distinguishes a framework from a library – For instance an application framework may require a developer to create a subclass of a class called Document • When the framework wants to save a document it calls Document. save() which via polymorphism calls the save() method of the subclass provided by the developer
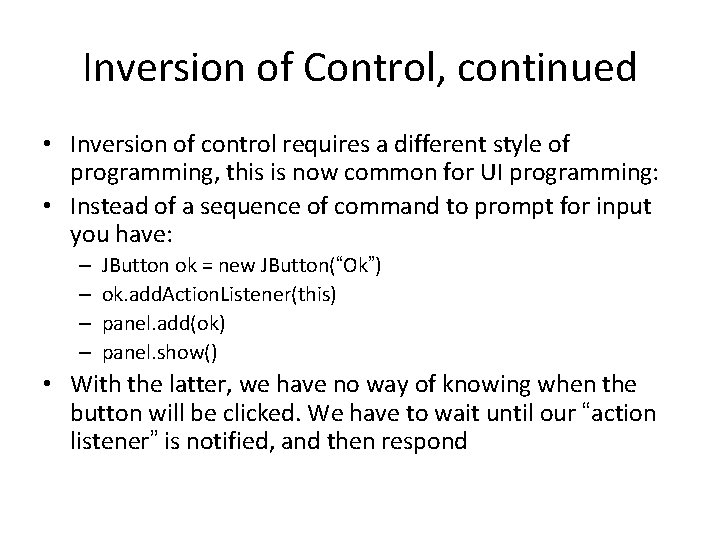
Inversion of Control, continued • Inversion of control requires a different style of programming, this is now common for UI programming: • Instead of a sequence of command to prompt for input you have: – – JButton ok = new JButton(“Ok”) ok. add. Action. Listener(this) panel. add(ok) panel. show() • With the latter, we have no way of knowing when the button will be clicked. We have to wait until our “action listener” is notified, and then respond
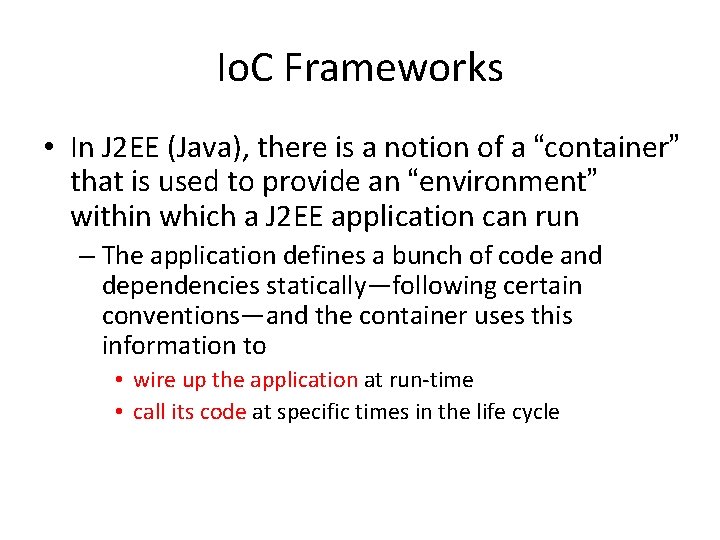
Io. C Frameworks • In J 2 EE (Java), there is a notion of a “container” that is used to provide an “environment” within which a J 2 EE application can run – The application defines a bunch of code and dependencies statically—following certain conventions—and the container uses this information to • wire up the application at run-time • call its code at specific times in the life cycle
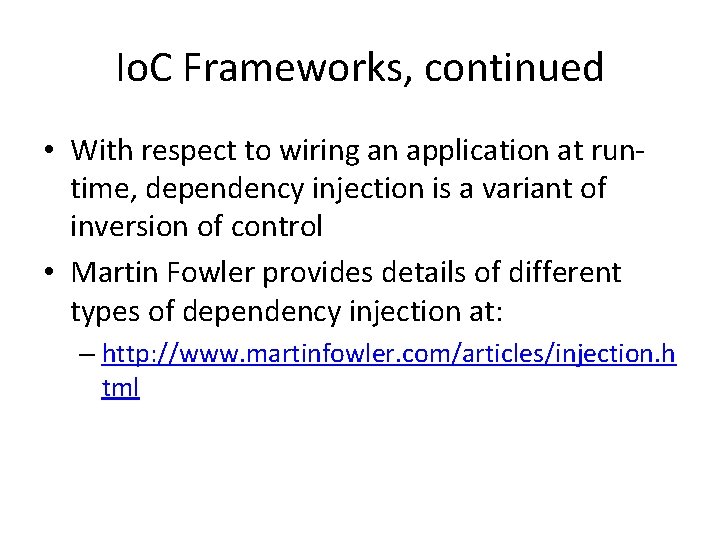
Io. C Frameworks, continued • With respect to wiring an application at runtime, dependency injection is a variant of inversion of control • Martin Fowler provides details of different types of dependency injection at: – http: //www. martinfowler. com/articles/injection. h tml
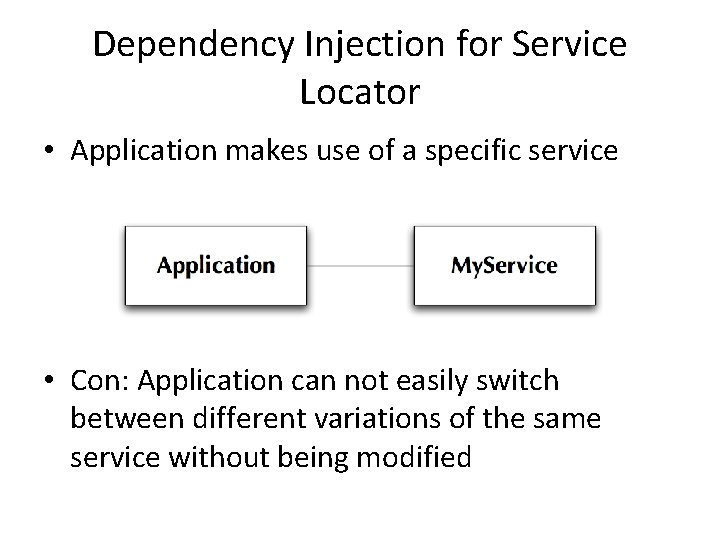
Dependency Injection for Service Locator • Application makes use of a specific service • Con: Application can not easily switch between different variations of the same service without being modified
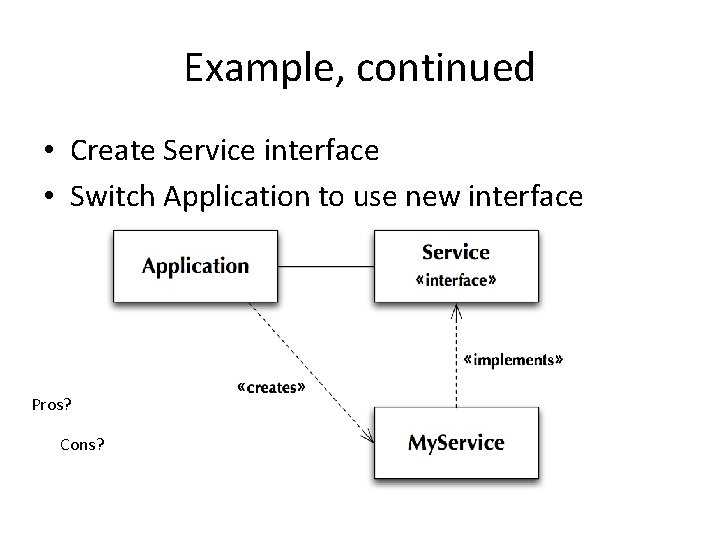
Example, continued • Create Service interface • Switch Application to use new interface Pros? Cons?
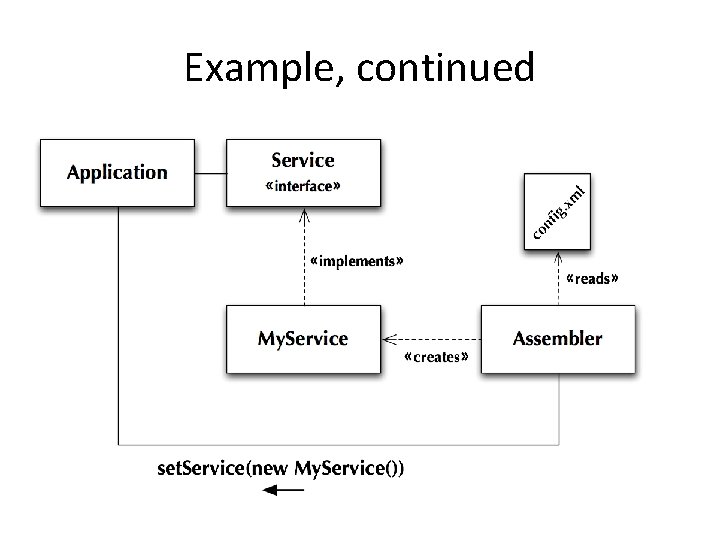
Example, continued
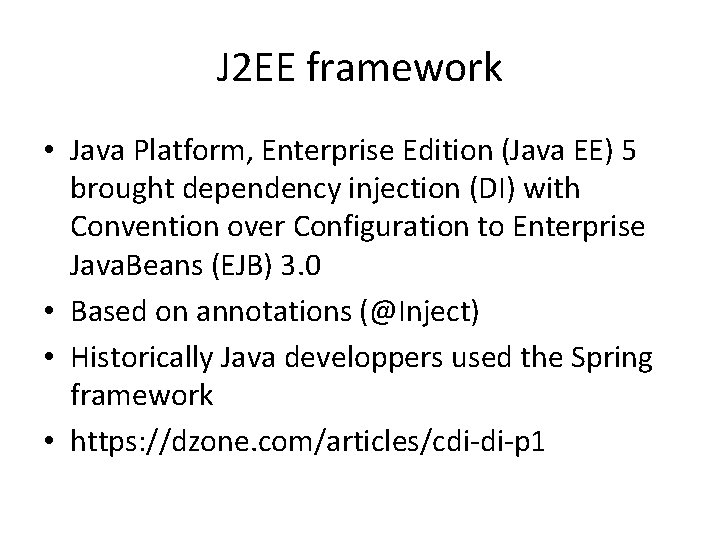
J 2 EE framework • Java Platform, Enterprise Edition (Java EE) 5 brought dependency injection (DI) with Convention over Configuration to Enterprise Java. Beans (EJB) 3. 0 • Based on annotations (@Inject) • Historically Java developpers used the Spring framework • https: //dzone. com/articles/cdi-di-p 1
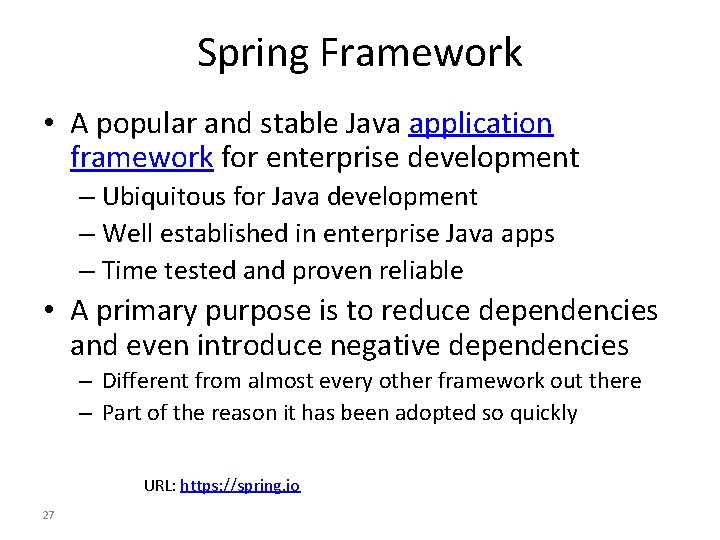
Spring Framework • A popular and stable Java application framework for enterprise development – Ubiquitous for Java development – Well established in enterprise Java apps – Time tested and proven reliable • A primary purpose is to reduce dependencies and even introduce negative dependencies – Different from almost every other framework out there – Part of the reason it has been adopted so quickly URL: https: //spring. io 27
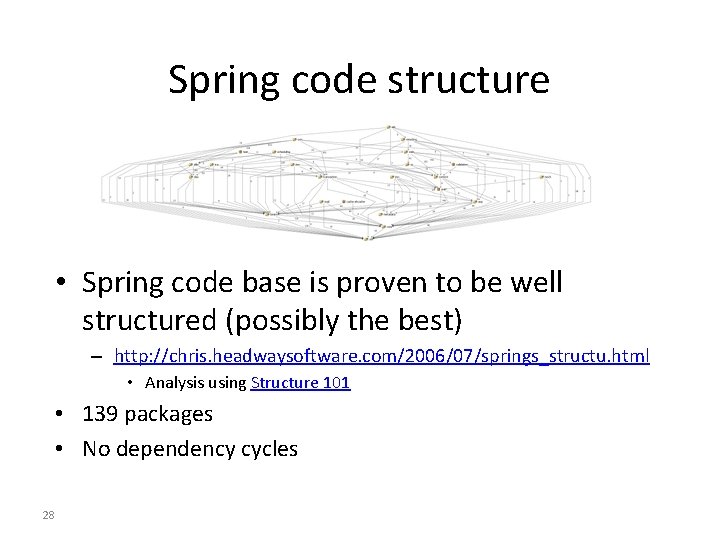
Spring code structure • Spring code base is proven to be well structured (possibly the best) – http: //chris. headwaysoftware. com/2006/07/springs_structu. html • Analysis using Structure 101 • 139 packages • No dependency cycles 28
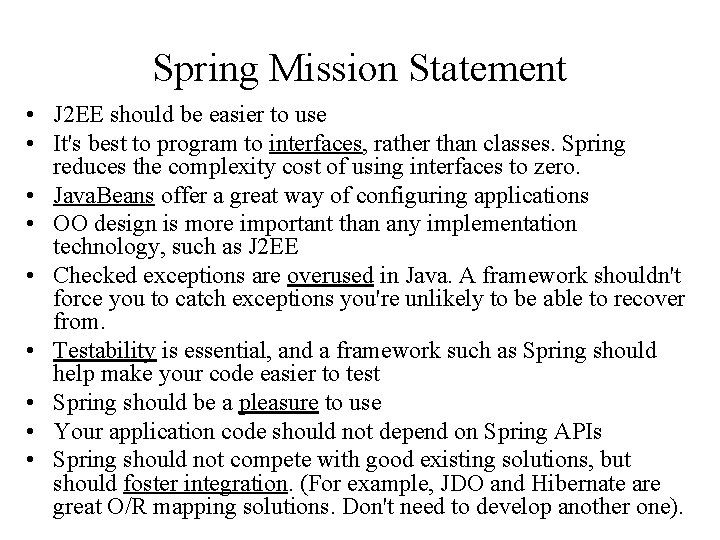
Spring Mission Statement • J 2 EE should be easier to use • It's best to program to interfaces, rather than classes. Spring reduces the complexity cost of using interfaces to zero. • Java. Beans offer a great way of configuring applications • OO design is more important than any implementation technology, such as J 2 EE • Checked exceptions are overused in Java. A framework shouldn't force you to catch exceptions you're unlikely to be able to recover from. • Testability is essential, and a framework such as Spring should help make your code easier to test • Spring should be a pleasure to use • Your application code should not depend on Spring APIs • Spring should not compete with good existing solutions, but should foster integration. (For example, JDO and Hibernate are great O/R mapping solutions. Don't need to develop another one).
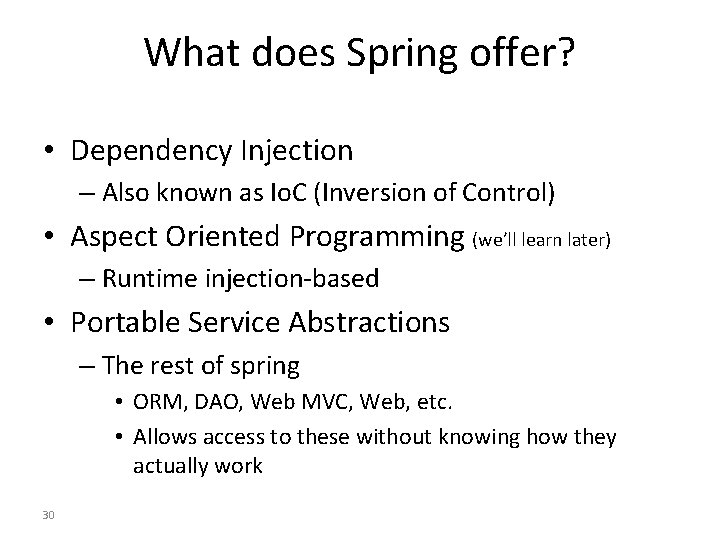
What does Spring offer? • Dependency Injection – Also known as Io. C (Inversion of Control) • Aspect Oriented Programming (we’ll learn later) – Runtime injection-based • Portable Service Abstractions – The rest of spring • ORM, DAO, Web MVC, Web, etc. • Allows access to these without knowing how they actually work 30
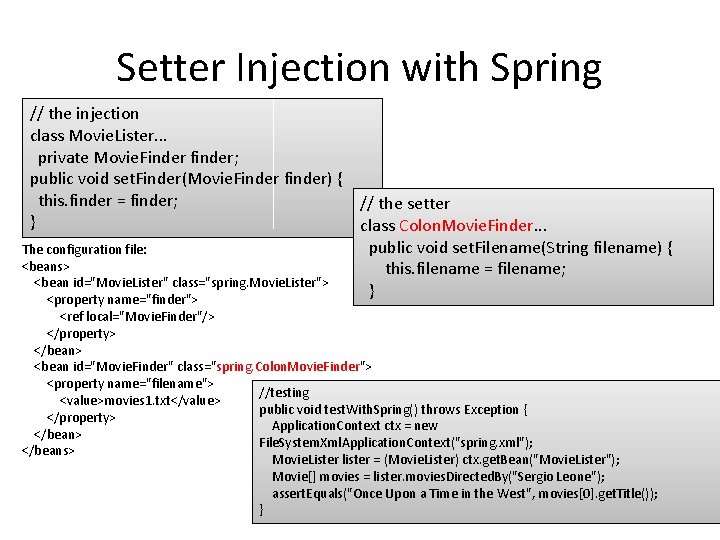
Setter Injection with Spring // the injection class Movie. Lister. . . private Movie. Finder finder; public void set. Finder(Movie. Finder finder) { this. finder = finder; // the setter } class Colon. Movie. Finder. . . public void set. Filename(String filename) { The configuration file: <beans> this. filename = filename; <bean id="Movie. Lister" class="spring. Movie. Lister"> } <property name="finder"> <ref local="Movie. Finder"/> </property> </bean> <bean id="Movie. Finder" class="spring. Colon. Movie. Finder"> <property name="filename"> //testing <value>movies 1. txt</value> public void test. With. Spring() throws Exception { </property> Application. Context ctx = new </bean> File. System. Xml. Application. Context("spring. xml"); </beans> Movie. Lister lister = (Movie. Lister) ctx. get. Bean("Movie. Lister"); Movie[] movies = lister. movies. Directed. By("Sergio Leone"); assert. Equals("Once Upon a Time in the West", movies[0]. get. Title()); }
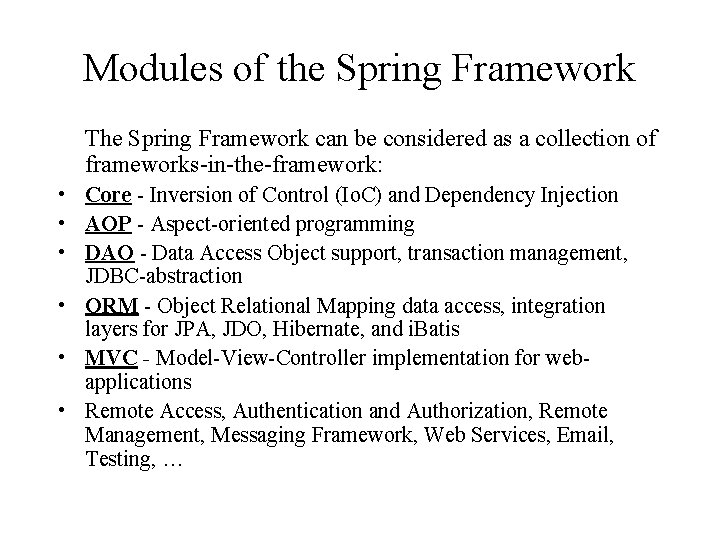
Modules of the Spring Framework The Spring Framework can be considered as a collection of frameworks-in-the-framework: • Core - Inversion of Control (Io. C) and Dependency Injection • AOP - Aspect-oriented programming • DAO - Data Access Object support, transaction management, JDBC-abstraction • ORM - Object Relational Mapping data access, integration layers for JPA, JDO, Hibernate, and i. Batis • MVC - Model-View-Controller implementation for webapplications • Remote Access, Authentication and Authorization, Remote Management, Messaging Framework, Web Services, Email, Testing, …
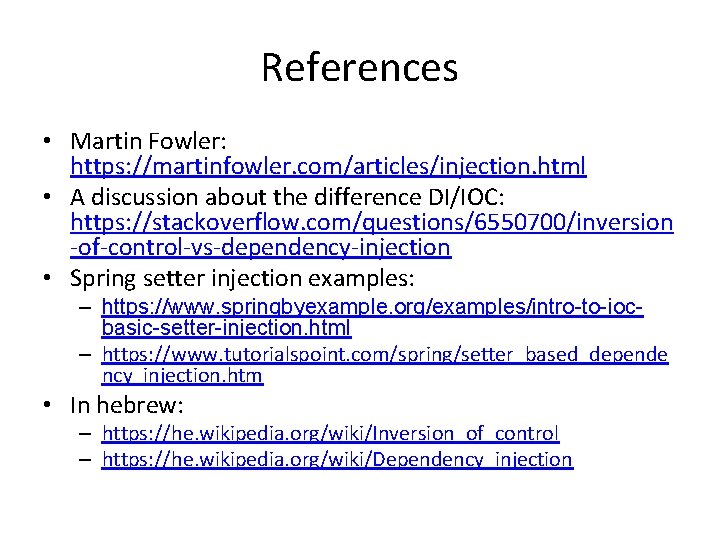
References • Martin Fowler: https: //martinfowler. com/articles/injection. html • A discussion about the difference DI/IOC: https: //stackoverflow. com/questions/6550700/inversion -of-control-vs-dependency-injection • Spring setter injection examples: – https: //www. springbyexample. org/examples/intro-to-iocbasic-setter-injection. html – https: //www. tutorialspoint. com/spring/setter_based_depende ncy_injection. htm • In hebrew: – https: //he. wikipedia. org/wiki/Inversion_of_control – https: //he. wikipedia. org/wiki/Dependency_injection