Definite Loops A repetition structure that executes code
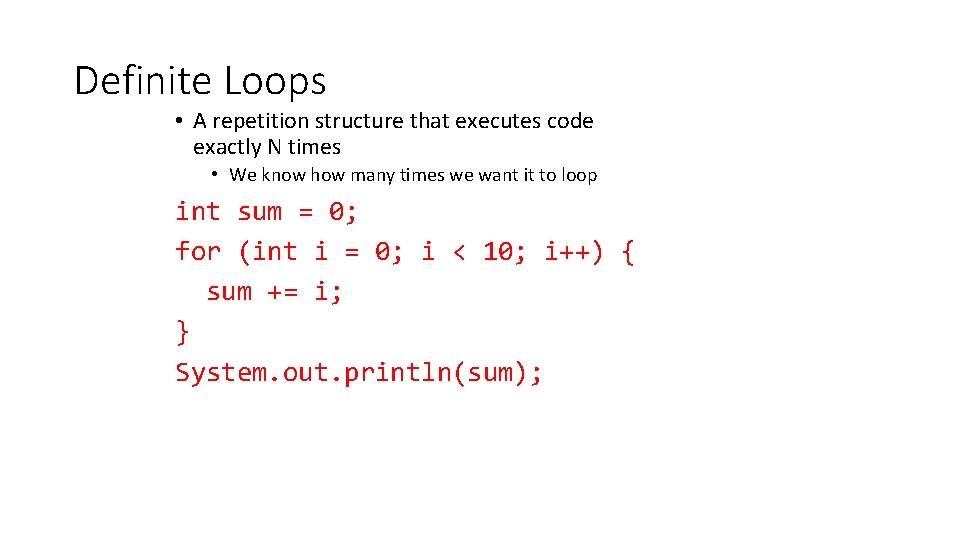
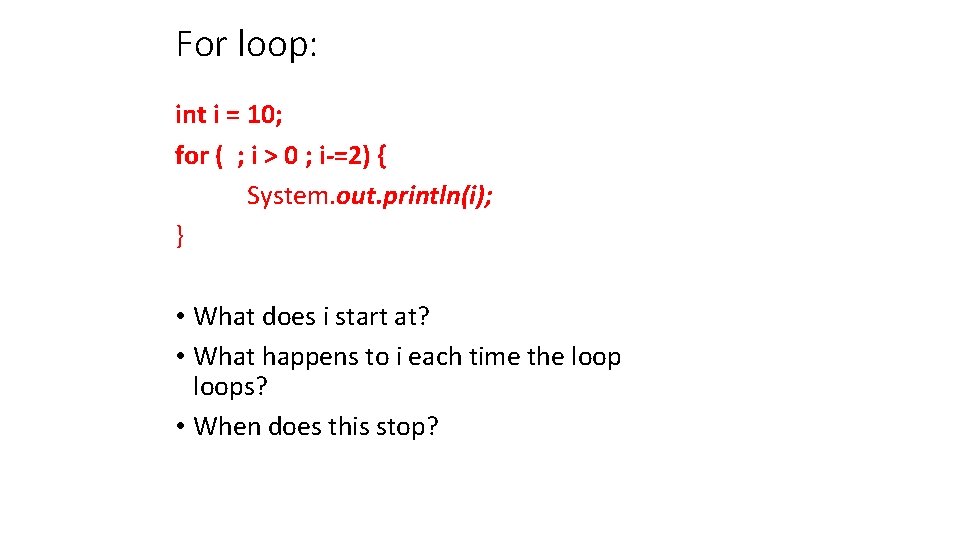
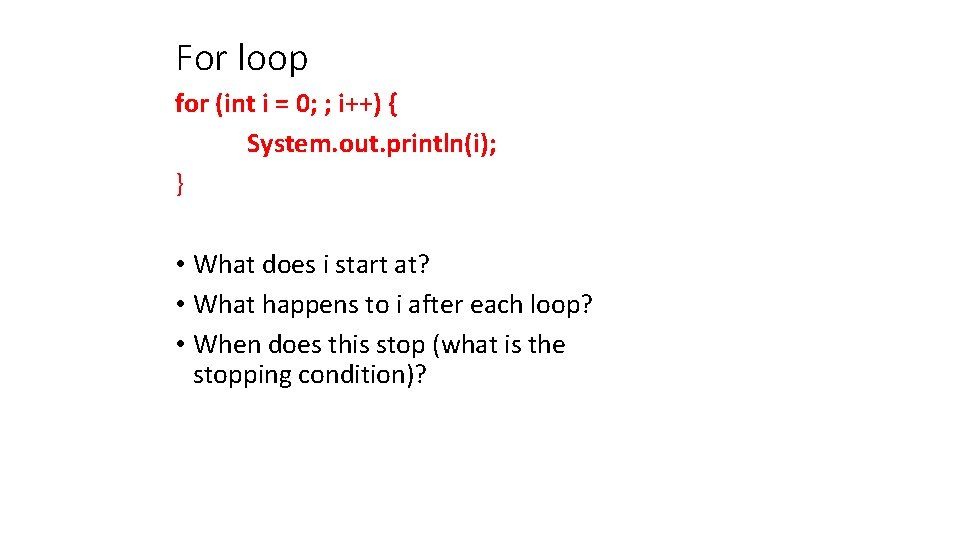
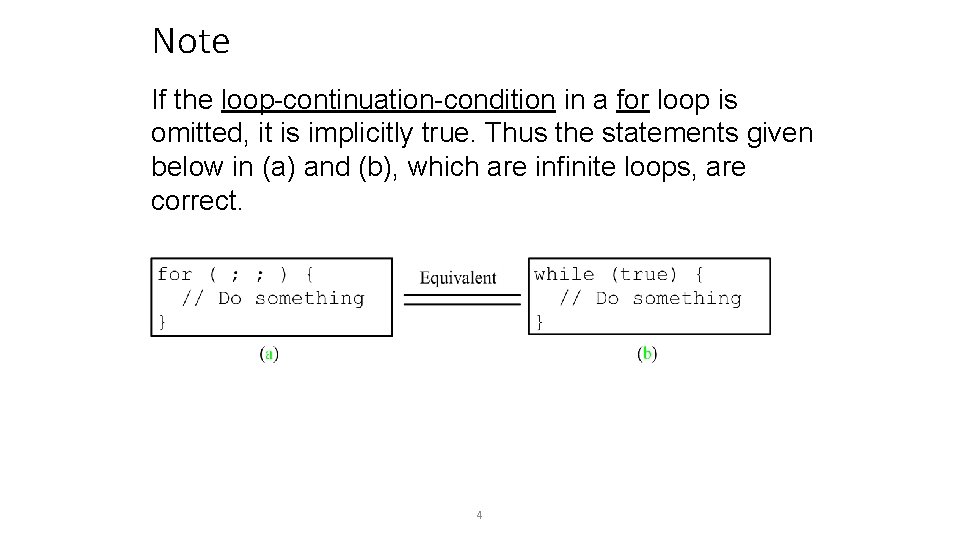
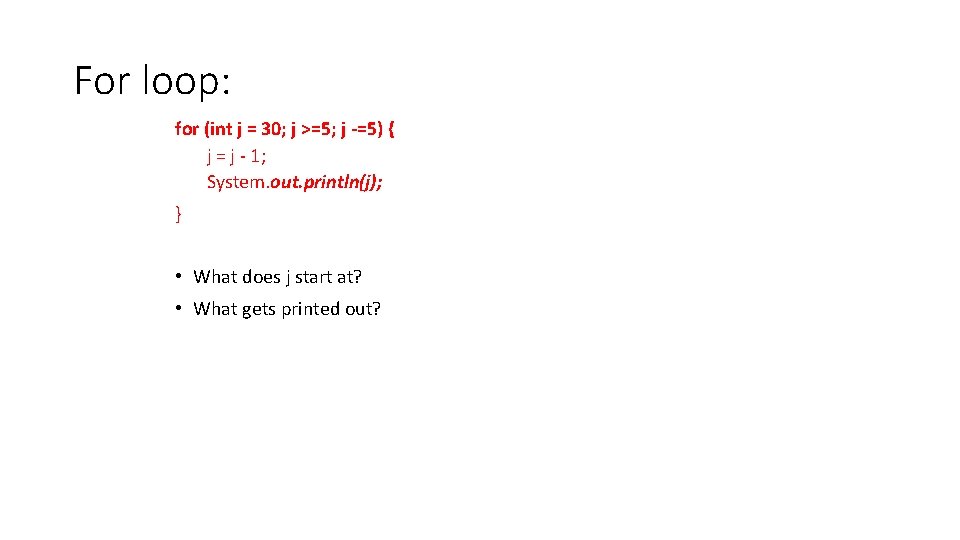
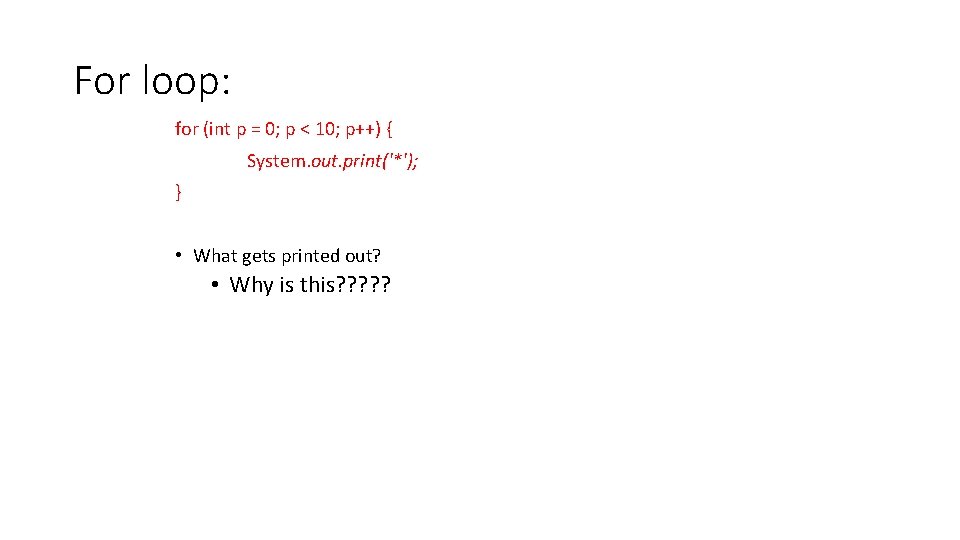
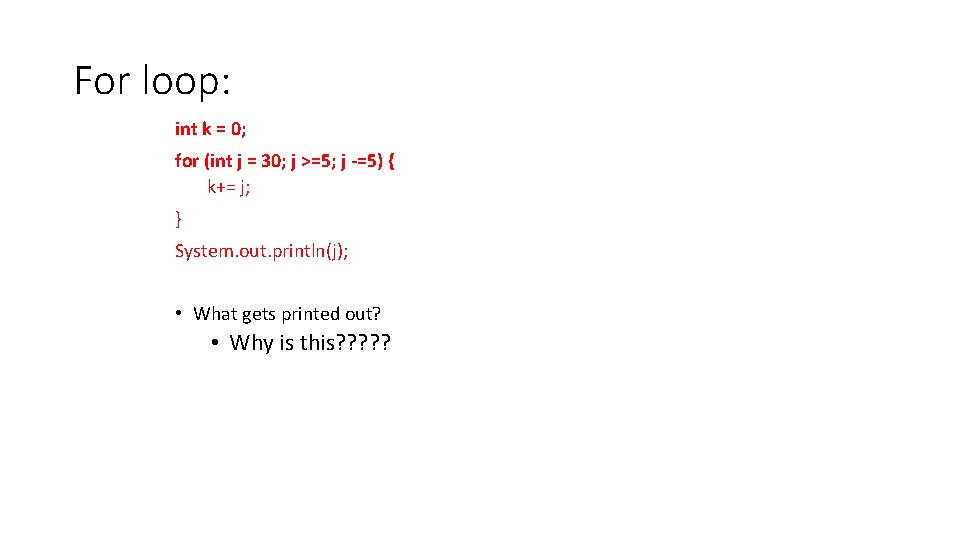
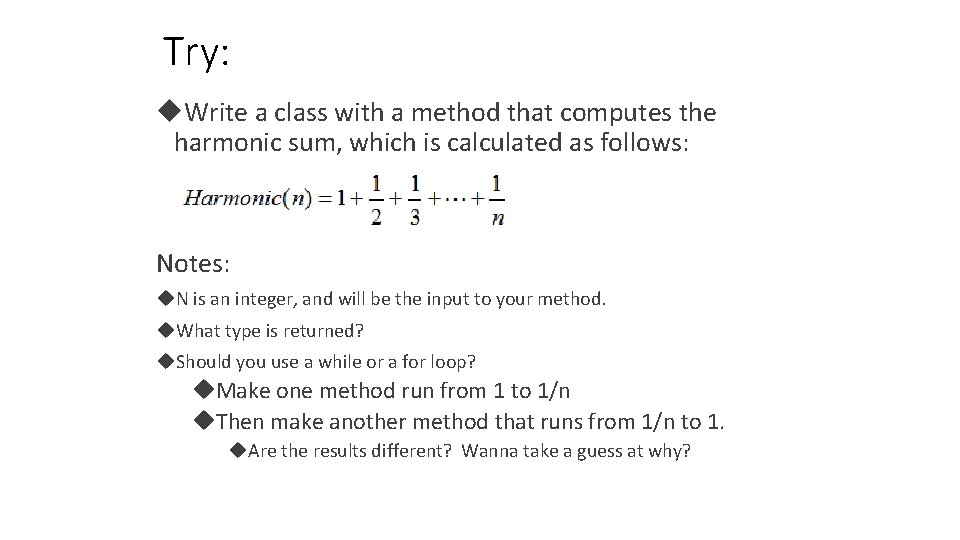
![public class Harmonic. Sum { public static void main (String[] args) { } System. public class Harmonic. Sum { public static void main (String[] args) { } System.](https://slidetodoc.com/presentation_image_h/1fe857b9aadd70833259bd20f83ac39b/image-9.jpg)
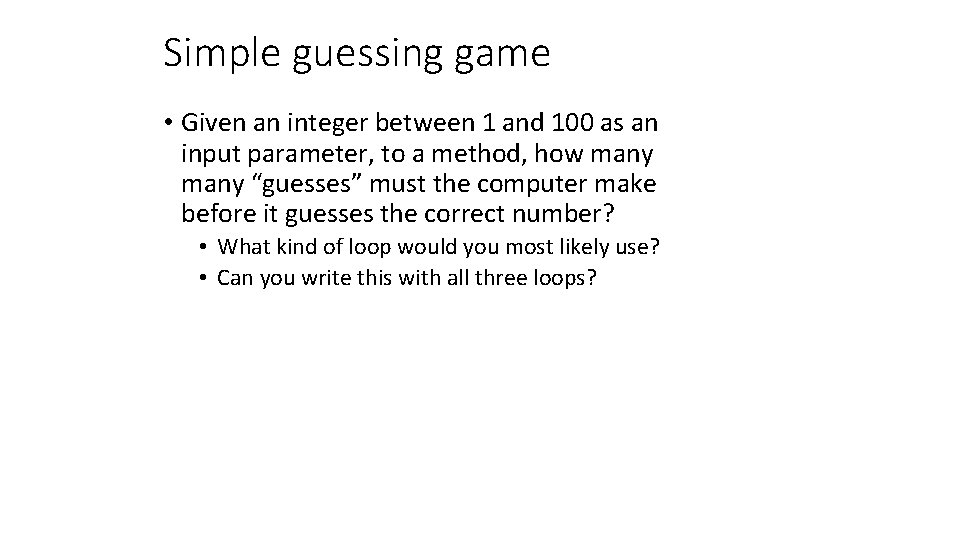
![import java. util. Random; public class Hello 2 { public static void main (String[] import java. util. Random; public class Hello 2 { public static void main (String[]](https://slidetodoc.com/presentation_image_h/1fe857b9aadd70833259bd20f83ac39b/image-11.jpg)
- Slides: 11
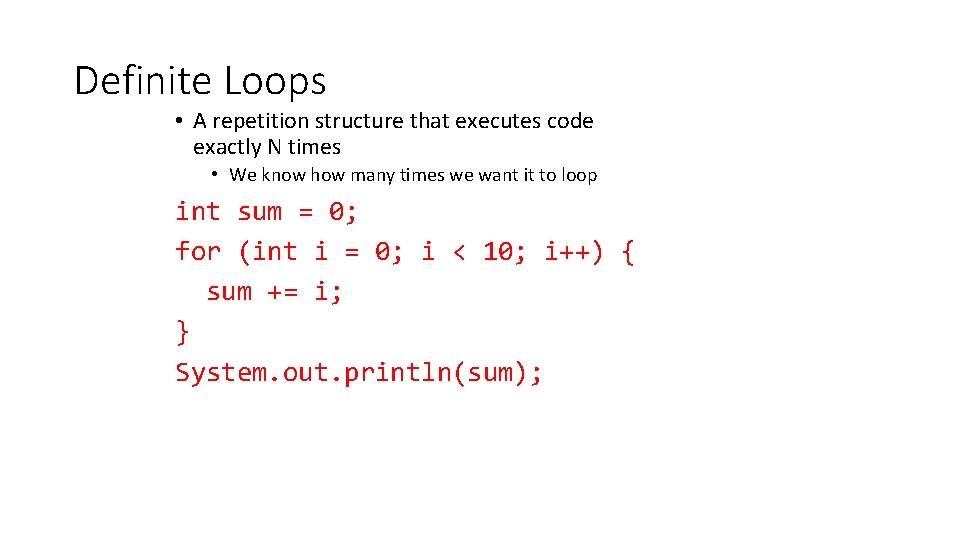
Definite Loops • A repetition structure that executes code exactly N times • We know how many times we want it to loop int sum = 0; for (int i = 0; i < 10; i++) { sum += i; } System. out. println(sum);
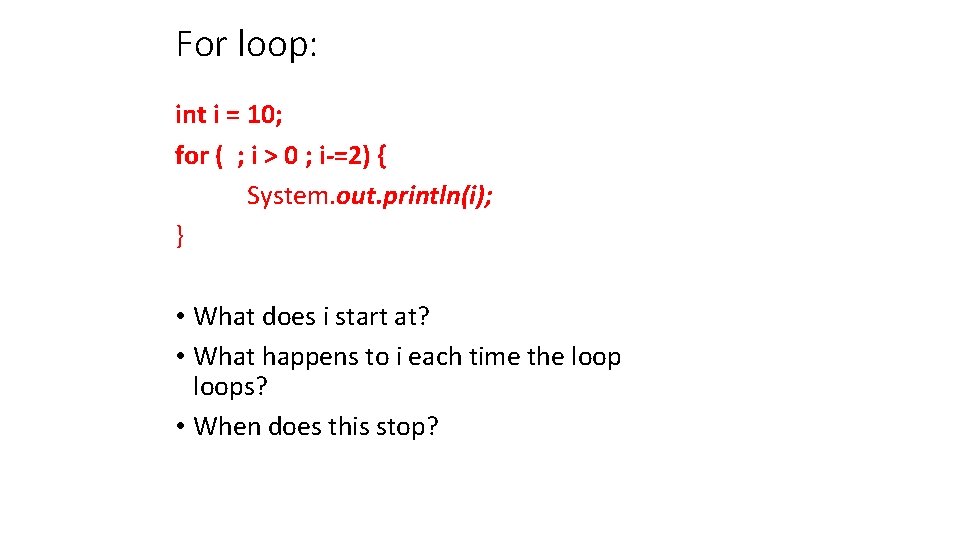
For loop: int i = 10; for ( ; i > 0 ; i-=2) { System. out. println(i); } • What does i start at? • What happens to i each time the loops? • When does this stop?
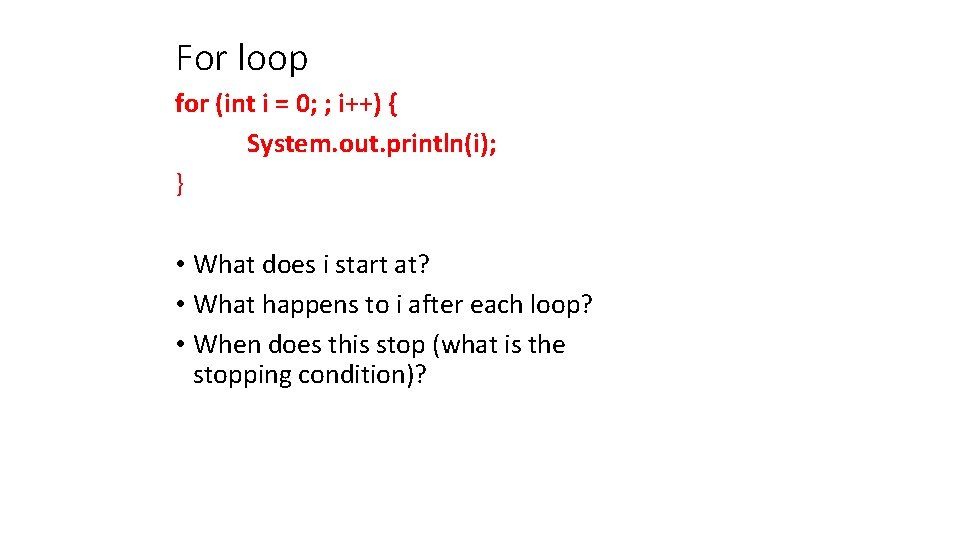
For loop for (int i = 0; ; i++) { System. out. println(i); } • What does i start at? • What happens to i after each loop? • When does this stop (what is the stopping condition)?
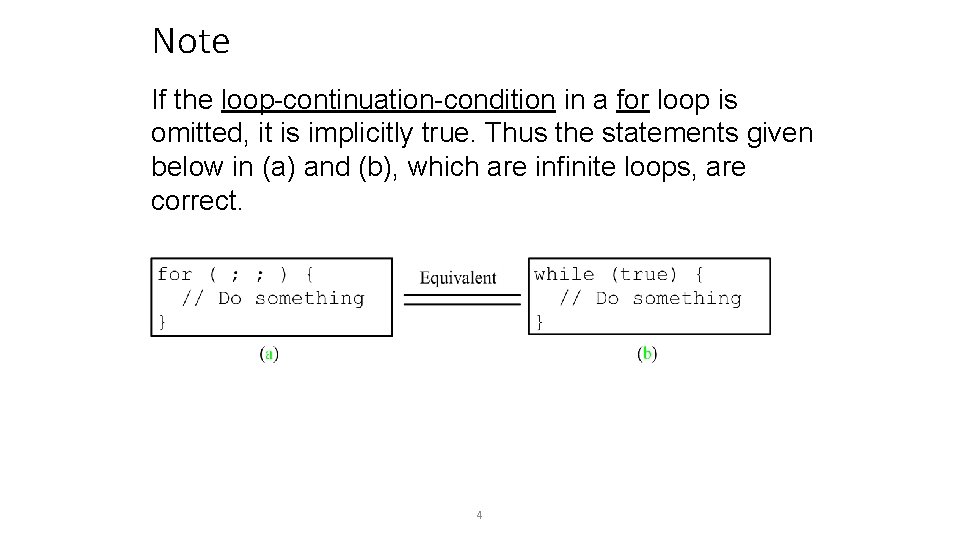
Note If the loop-continuation-condition in a for loop is omitted, it is implicitly true. Thus the statements given below in (a) and (b), which are infinite loops, are correct. 4
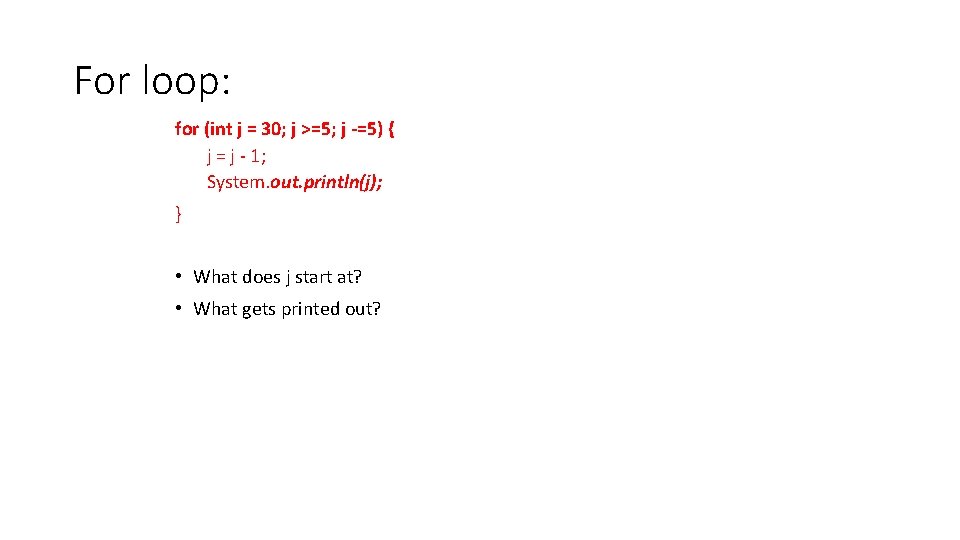
For loop: for (int j = 30; j >=5; j -=5) { j = j - 1; System. out. println(j); } • What does j start at? • What gets printed out?
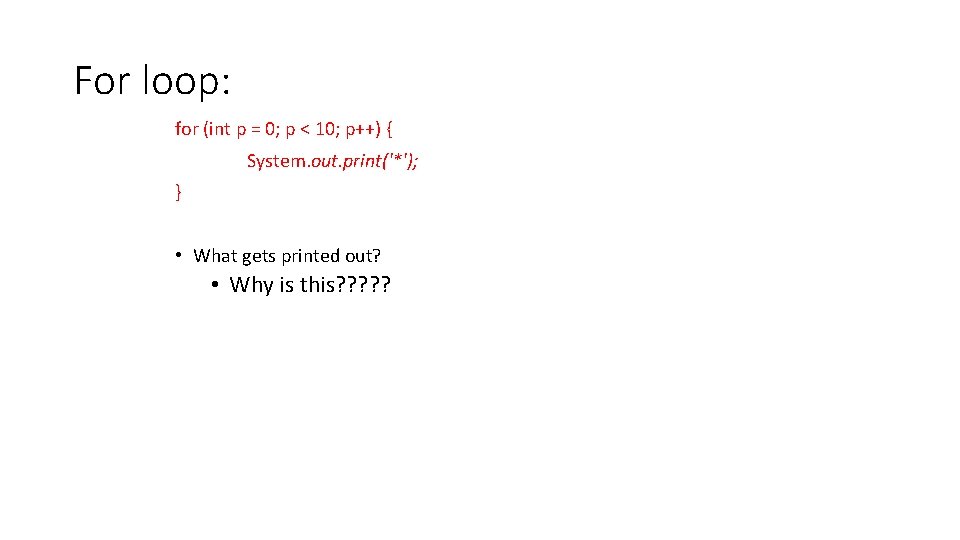
For loop: for (int p = 0; p < 10; p++) { System. out. print('*'); } • What gets printed out? • Why is this? ? ?
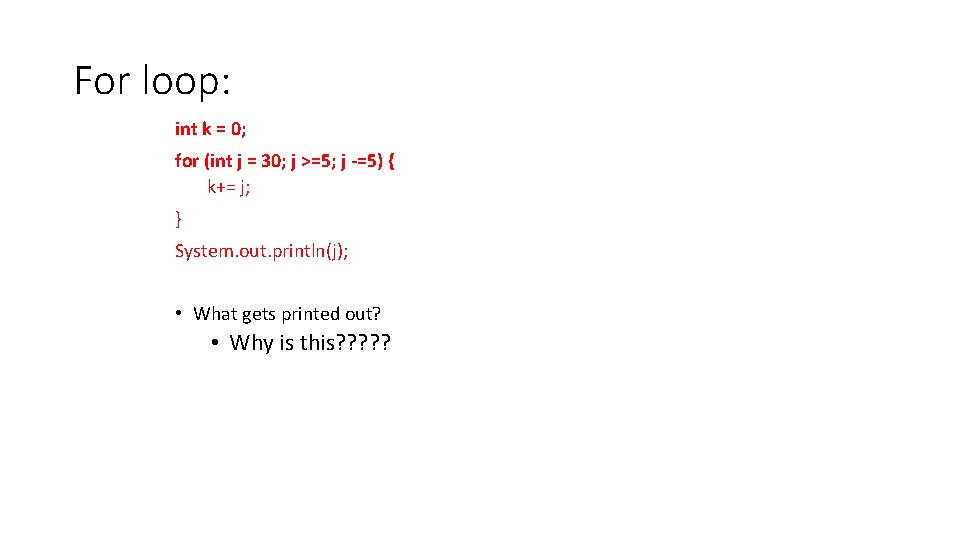
For loop: int k = 0; for (int j = 30; j >=5; j -=5) { k+= j; } System. out. println(j); • What gets printed out? • Why is this? ? ?
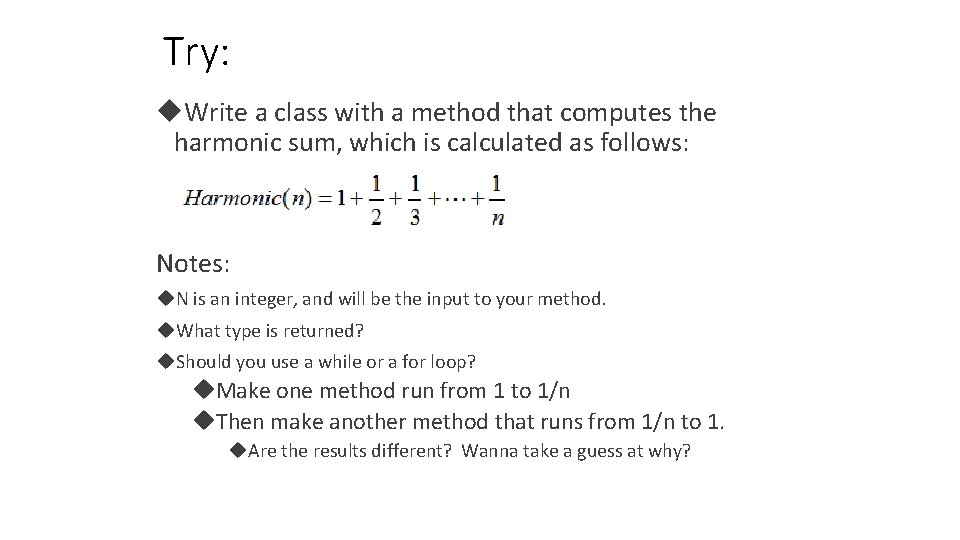
Try: Write a class with a method that computes the harmonic sum, which is calculated as follows: Notes: N is an integer, and will be the input to your method. What type is returned? Should you use a while or a for loop? Make one method run from 1 to 1/n Then make another method that runs from 1/n to 1. Are the results different? Wanna take a guess at why?
![public class Harmonic Sum public static void main String args System public class Harmonic. Sum { public static void main (String[] args) { } System.](https://slidetodoc.com/presentation_image_h/1fe857b9aadd70833259bd20f83ac39b/image-9.jpg)
public class Harmonic. Sum { public static void main (String[] args) { } System. out. println(HSLeft. To. Right(50000)); System. out. println(HSRight. To. Left(50000)); public static double HSLeft. To. Right(int max. Denom) { } // for left-to-right double sum. L 2 R = 0. 0; for (int n = 1; n <= max. Denom; n++) { sum. L 2 R += (double)(1. 0/n); } return (sum. L 2 R); public static double HSRight. To. Left(int max. Denom) { } } // for right-to-left double sum. R 2 L = 0. 0; for (int n = max. Denom; n >= 1; n--) { sum. R 2 L += (double)(1. 0/n); } return (sum. R 2 L);
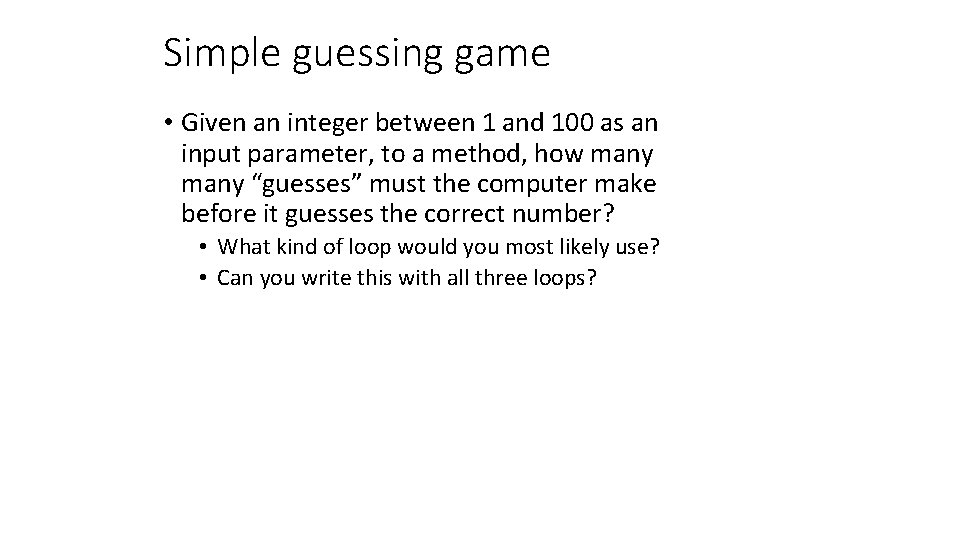
Simple guessing game • Given an integer between 1 and 100 as an input parameter, to a method, how many “guesses” must the computer make before it guesses the correct number? • What kind of loop would you most likely use? • Can you write this with all three loops?
![import java util Random public class Hello 2 public static void main String import java. util. Random; public class Hello 2 { public static void main (String[]](https://slidetodoc.com/presentation_image_h/1fe857b9aadd70833259bd20f83ac39b/image-11.jpg)
import java. util. Random; public class Hello 2 { public static void main (String[] args) { System. out. println("While " + while. Guess(72)); System. out. println("Rec " +rec. Guess(42)); System. out. println("for " +for. Guess(27)); } public static int while. Guess(int g) { Random r = new Random(); int ct = 1; int x = r. next. Int(100); while (x != g) { x = r. next. Int(100); ct ++; } return(ct); } public static int rec. Guess(int g) { Random r = new Random(); int x = r. next. Int(100); if (x == g) { return(1); } else { return(1 + rec. Guess(g)); } } public static int for. Guess(int g) { Random r = new Random(); int ct = 1; for (int x=r. next. Int(100); x != g; x = r. next. Int(100), ct++); return(ct); }