Declaring a Class the h file pragma once
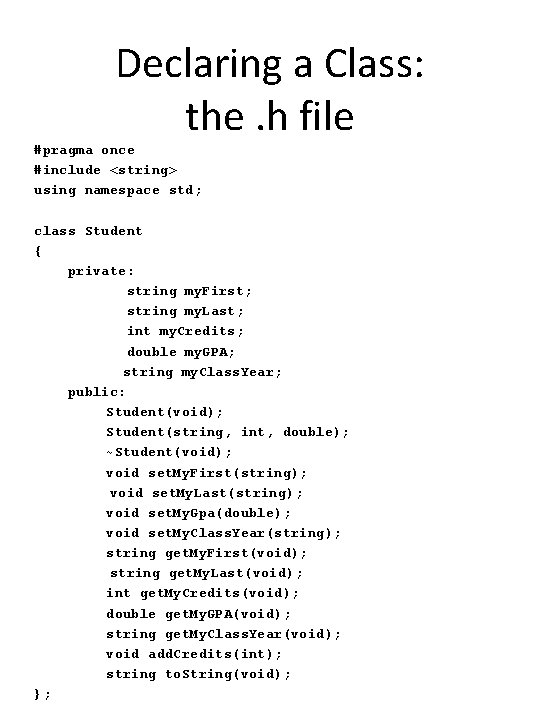
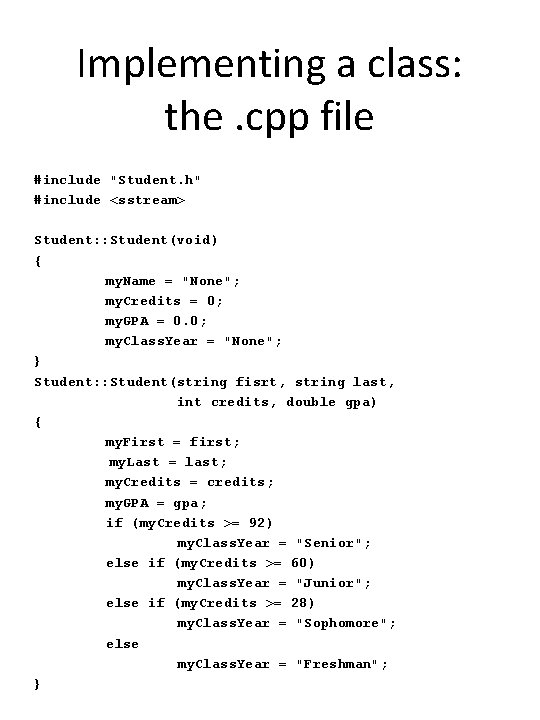
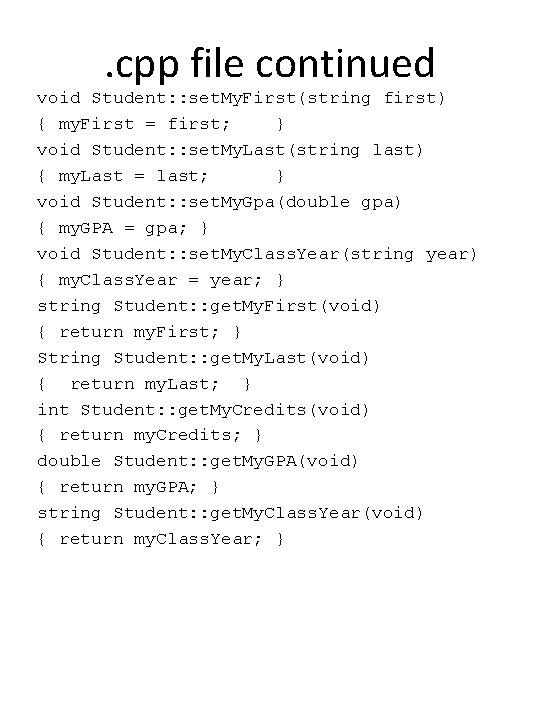
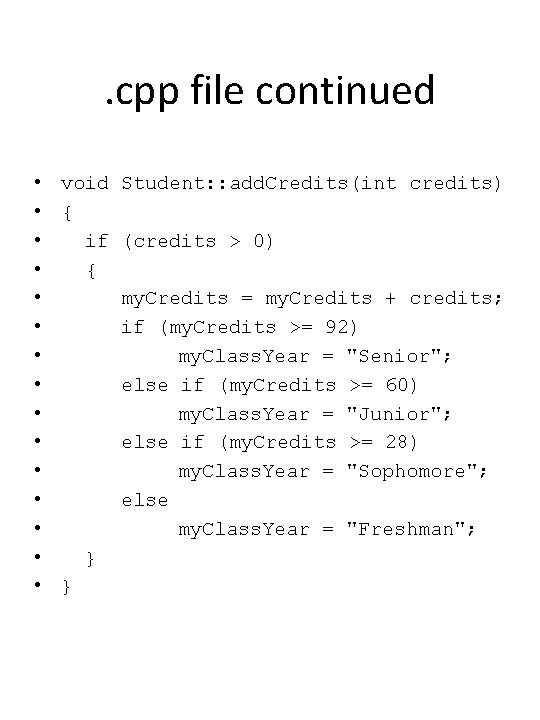
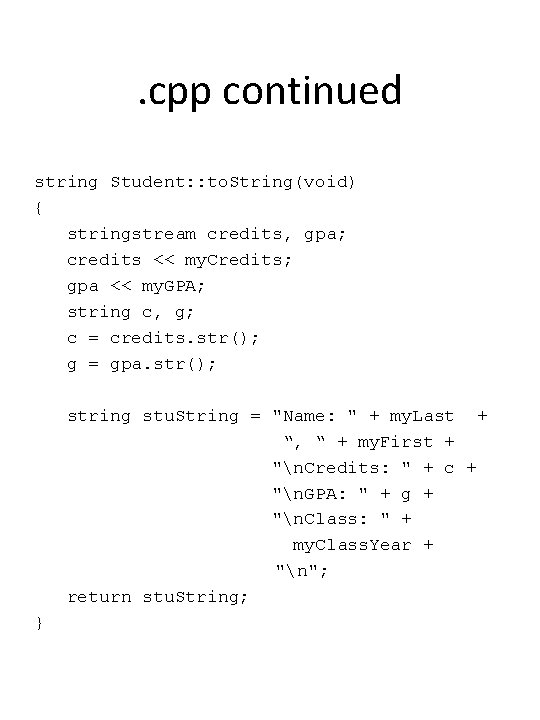
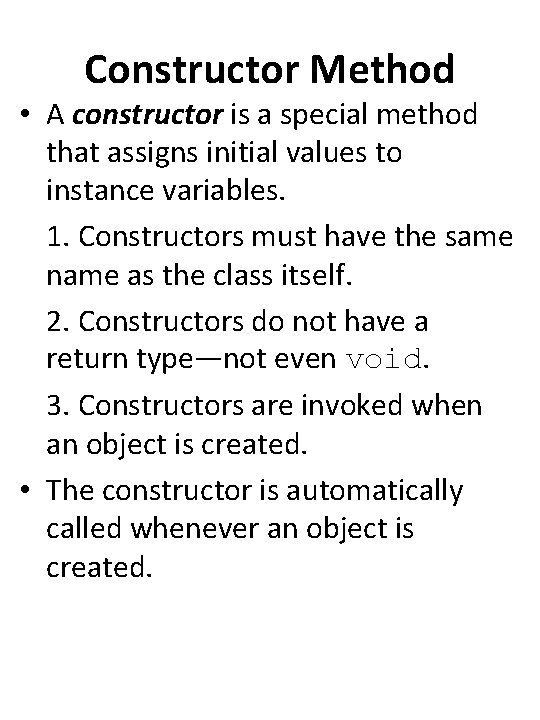
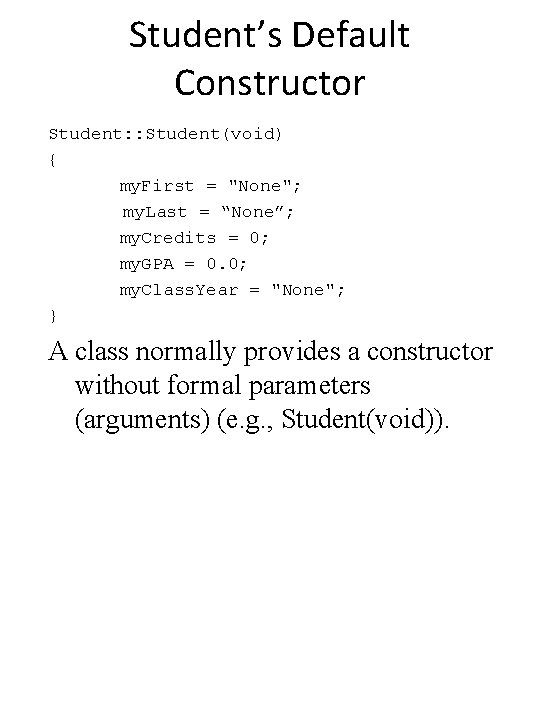
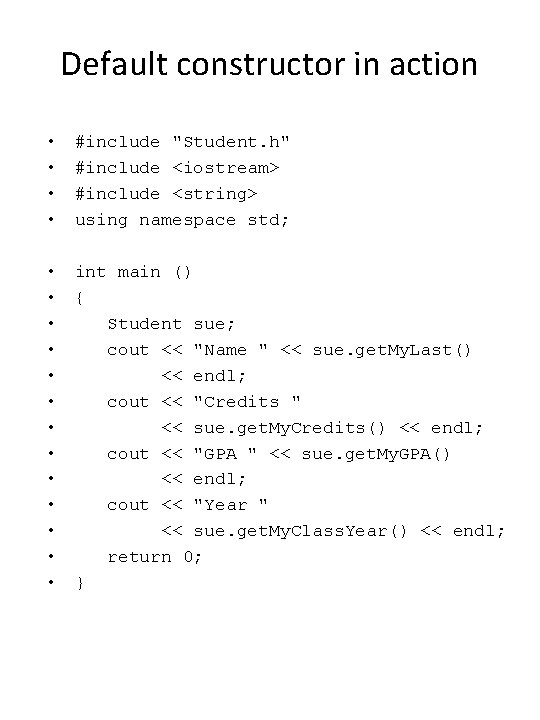
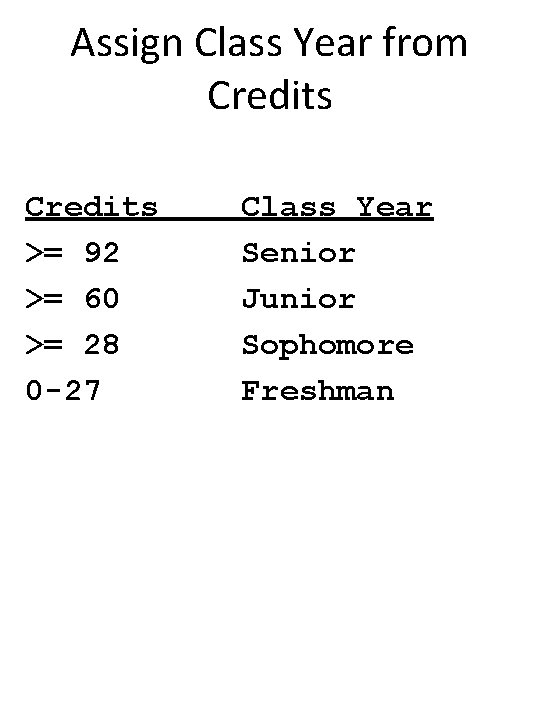
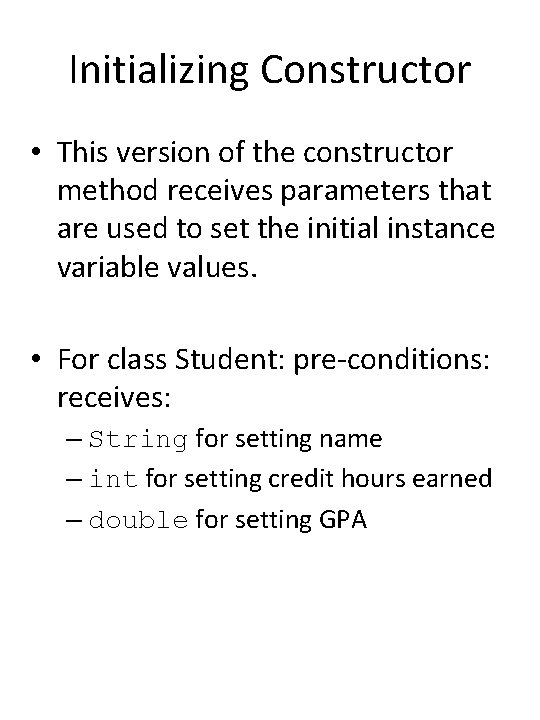
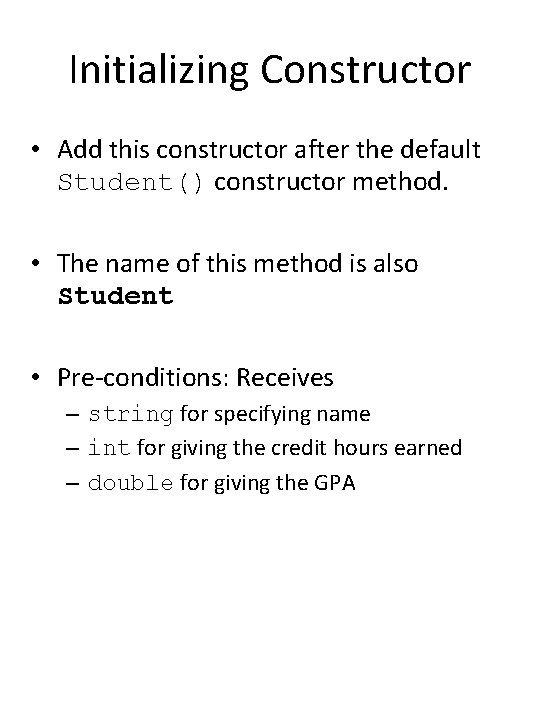
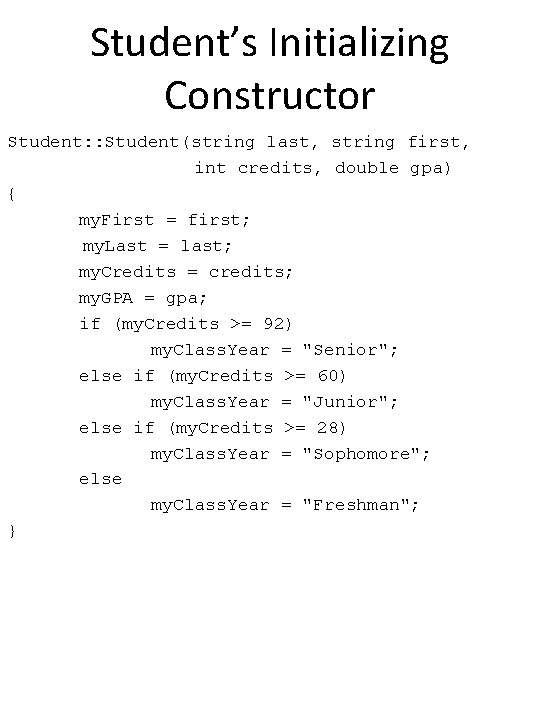
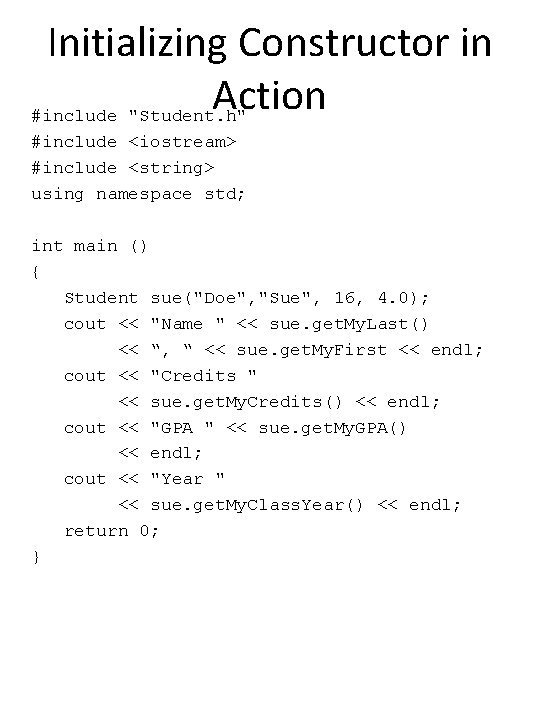
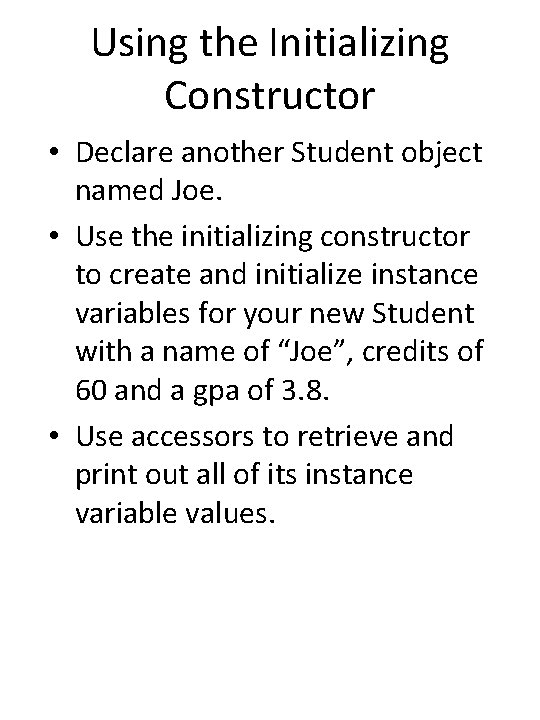
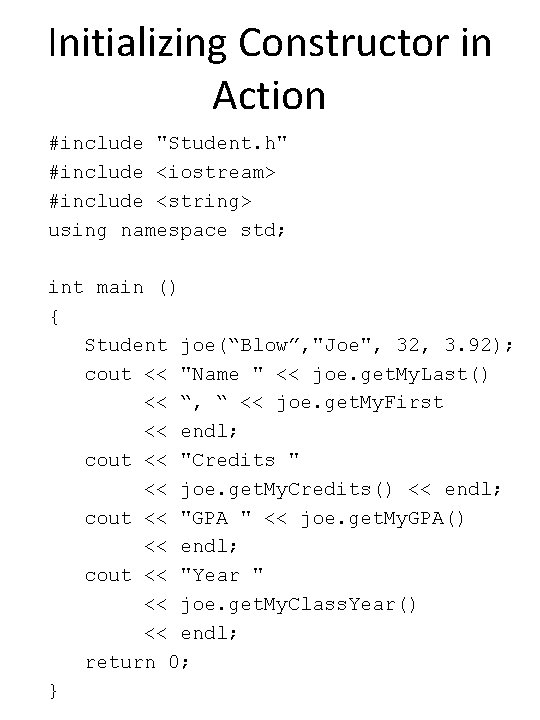
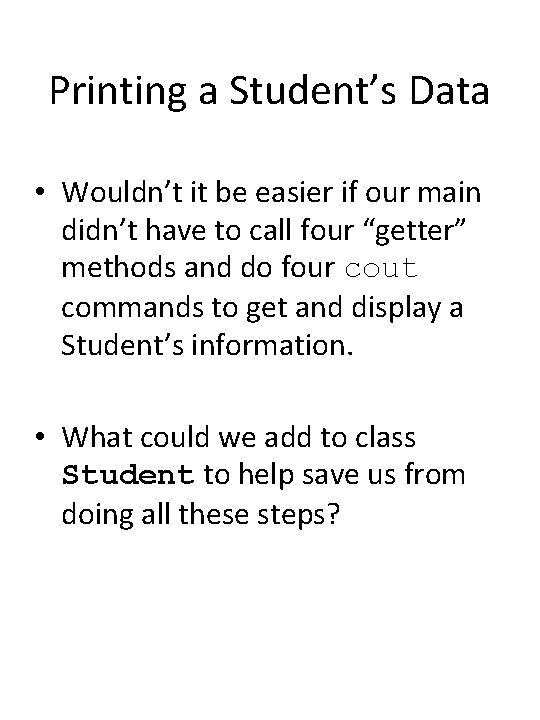
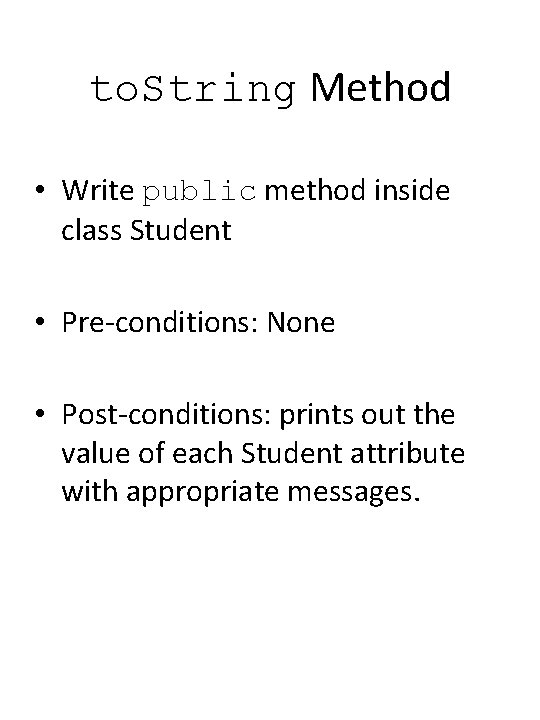
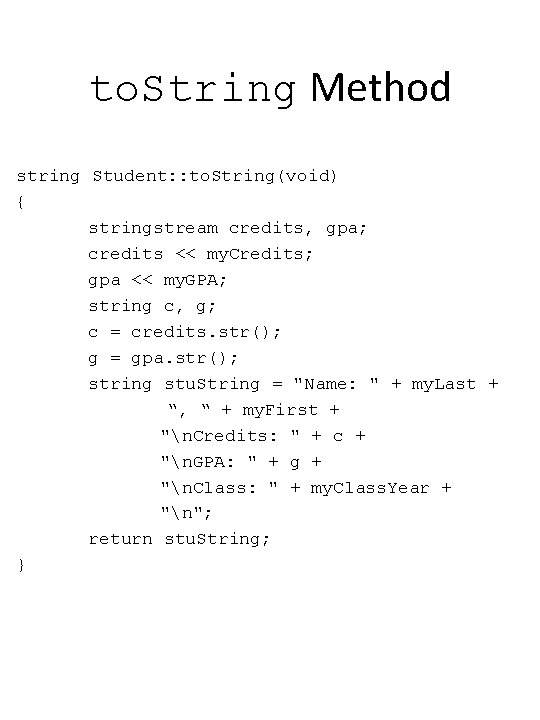
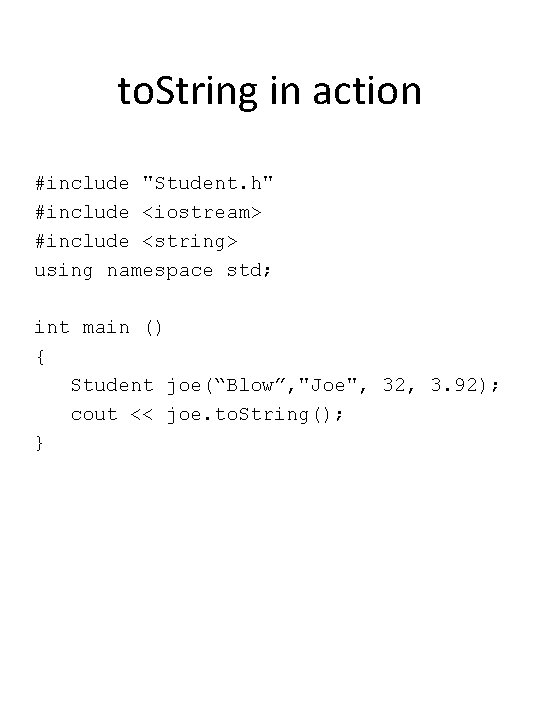
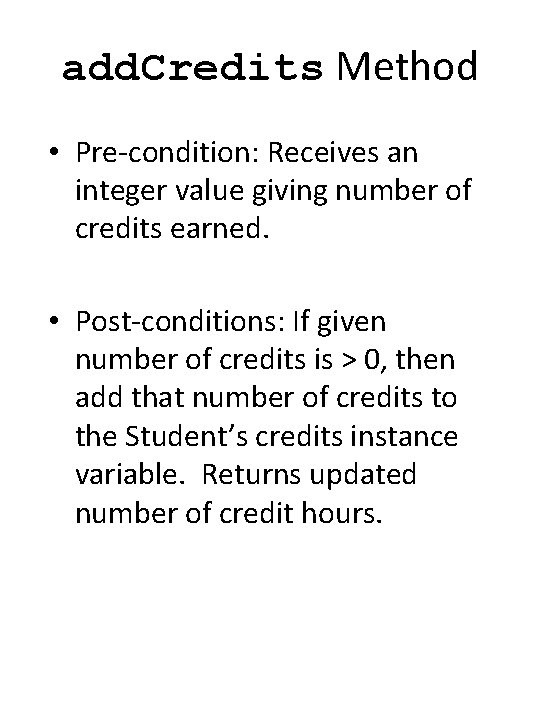
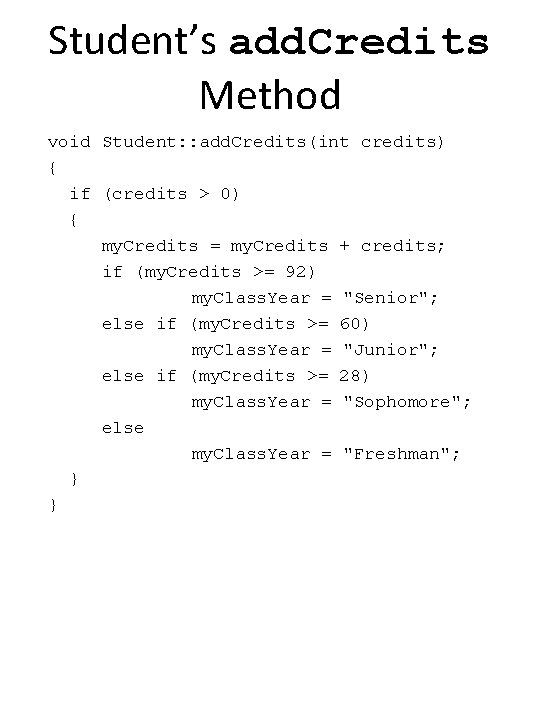
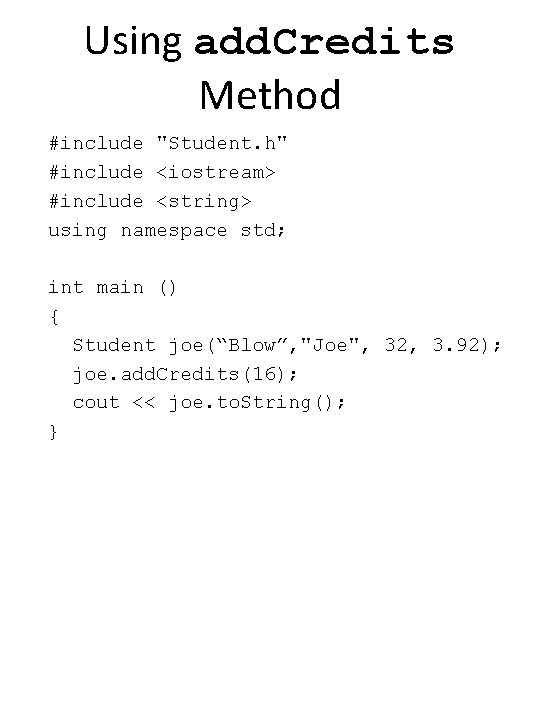
- Slides: 22
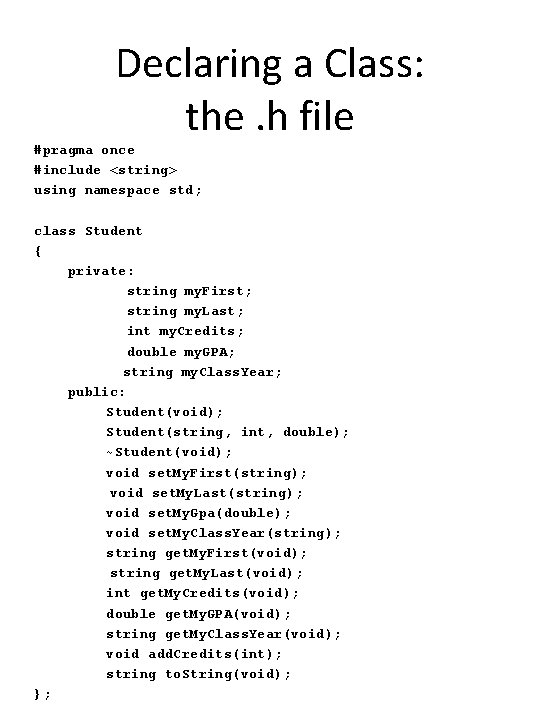
Declaring a Class: the. h file #pragma once #include <string> using namespace std; class Student { private: string my. First; string my. Last; int my. Credits; double my. GPA; string my. Class. Year; public: Student(void); Student(string, int, double); ~Student(void); void set. My. First(string); void set. My. Last(string); void set. My. Gpa(double); void set. My. Class. Year(string); string get. My. First(void); string get. My. Last(void); int get. My. Credits(void); double get. My. GPA(void); string get. My. Class. Year(void); void add. Credits(int); string to. String(void); };
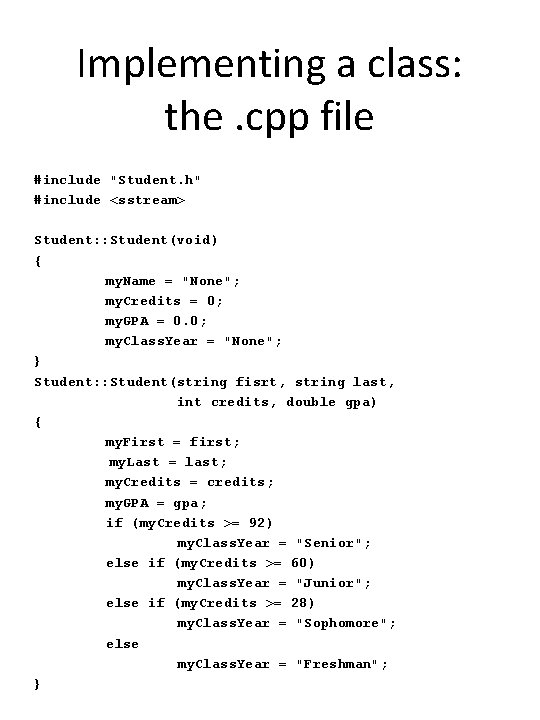
Implementing a class: the. cpp file #include "Student. h" #include <sstream> Student: : Student(void) { my. Name = "None"; my. Credits = 0; my. GPA = 0. 0; my. Class. Year = "None"; } Student: : Student(string fisrt, string last, int credits, double gpa) { my. First = first; my. Last = last; my. Credits = credits; my. GPA = gpa; if (my. Credits >= 92) my. Class. Year = "Senior"; else if (my. Credits >= 60) my. Class. Year = "Junior"; else if (my. Credits >= 28) my. Class. Year = "Sophomore"; else my. Class. Year = "Freshman"; }
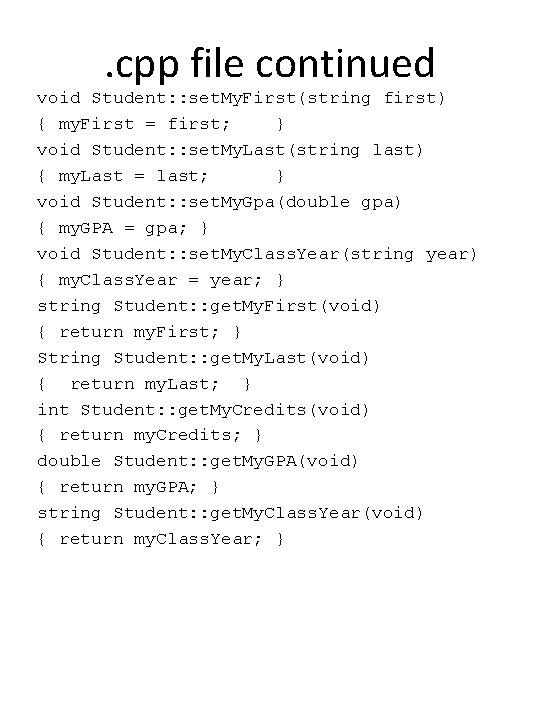
. cpp file continued void Student: : set. My. First(string first) { my. First = first; } void Student: : set. My. Last(string last) { my. Last = last; } void Student: : set. My. Gpa(double gpa) { my. GPA = gpa; } void Student: : set. My. Class. Year(string year) { my. Class. Year = year; } string Student: : get. My. First(void) { return my. First; } String Student: : get. My. Last(void) { return my. Last; } int Student: : get. My. Credits(void) { return my. Credits; } double Student: : get. My. GPA(void) { return my. GPA; } string Student: : get. My. Class. Year(void) { return my. Class. Year; }
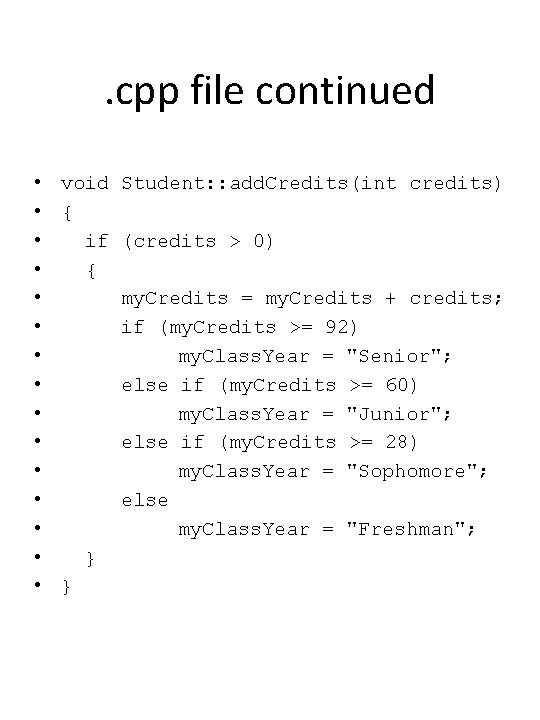
. cpp file continued • void Student: : add. Credits(int credits) • { • if (credits > 0) • { • my. Credits = my. Credits + credits; • if (my. Credits >= 92) • my. Class. Year = "Senior"; • else if (my. Credits >= 60) • my. Class. Year = "Junior"; • else if (my. Credits >= 28) • my. Class. Year = "Sophomore"; • else • my. Class. Year = "Freshman"; • }
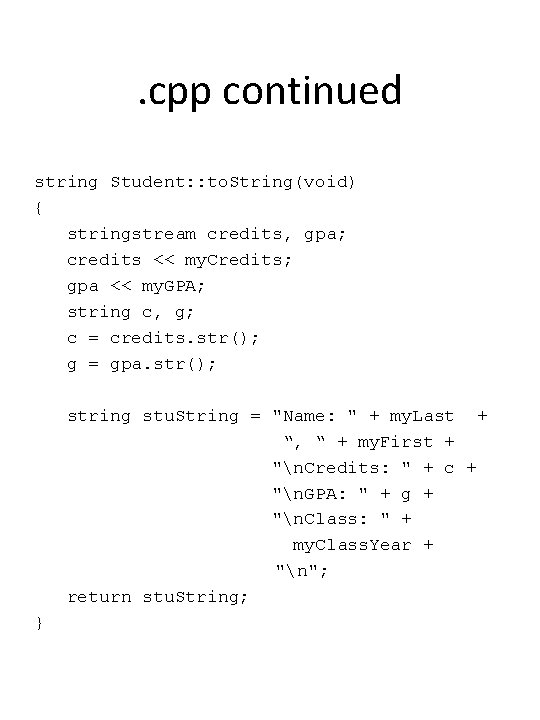
. cpp continued string Student: : to. String(void) { stringstream credits, gpa; credits << my. Credits; gpa << my. GPA; string c, g; c = credits. str(); g = gpa. str(); string stu. String = "Name: " + my. Last + “, “ + my. First + "n. Credits: " + c + "n. GPA: " + g + "n. Class: " + my. Class. Year + "n"; return stu. String; }
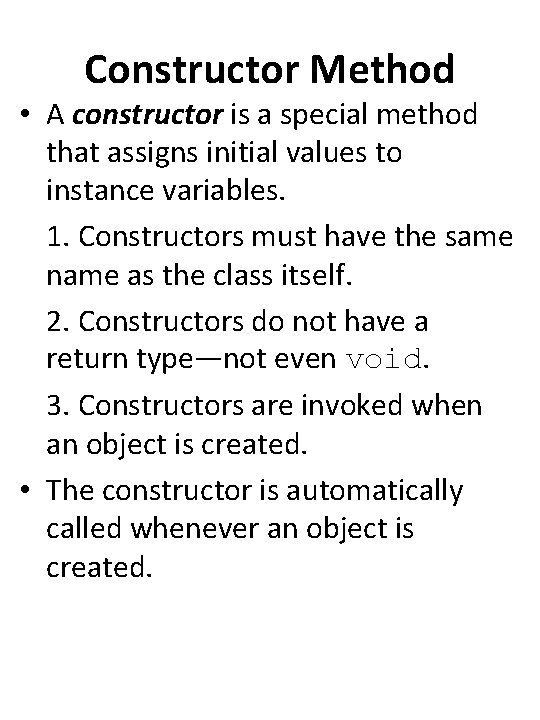
Constructor Method • A constructor is a special method that assigns initial values to instance variables. 1. Constructors must have the same name as the class itself. 2. Constructors do not have a return type—not even void. 3. Constructors are invoked when an object is created. • The constructor is automatically called whenever an object is created.
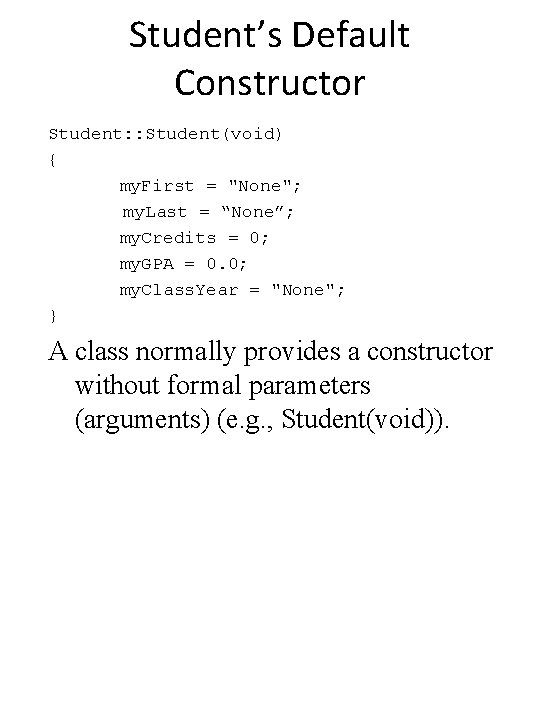
Student’s Default Constructor Student: : Student(void) { my. First = "None"; my. Last = “None”; my. Credits = 0; my. GPA = 0. 0; my. Class. Year = "None"; } A class normally provides a constructor without formal parameters (arguments) (e. g. , Student(void)).
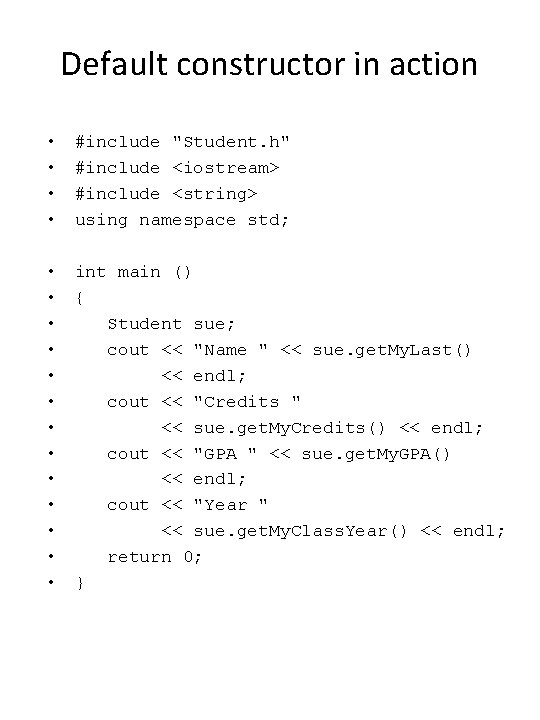
Default constructor in action • • #include "Student. h" #include <iostream> #include <string> using namespace std; • • • • int main () { Student sue; cout << "Name " << sue. get. My. Last() << endl; cout << "Credits " << sue. get. My. Credits() << endl; cout << "GPA " << sue. get. My. GPA() << endl; cout << "Year " << sue. get. My. Class. Year() << endl; return 0; }
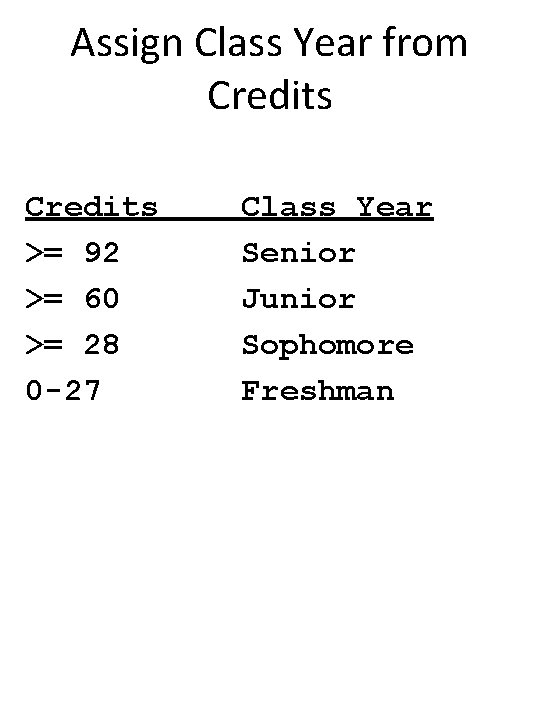
Assign Class Year from Credits >= 92 >= 60 >= 28 0 -27 Class Year Senior Junior Sophomore Freshman
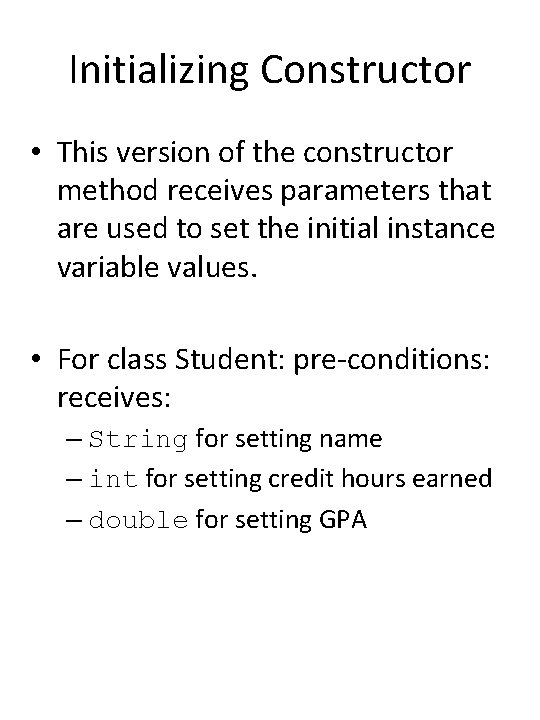
Initializing Constructor • This version of the constructor method receives parameters that are used to set the initial instance variable values. • For class Student: pre-conditions: receives: – String for setting name – int for setting credit hours earned – double for setting GPA
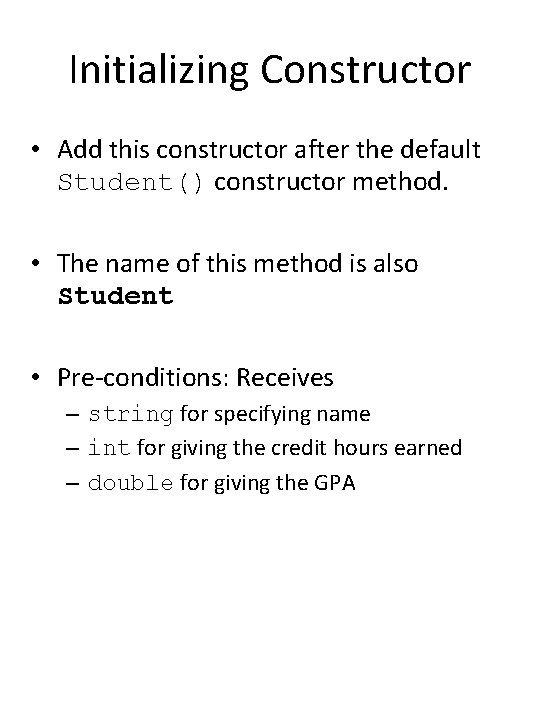
Initializing Constructor • Add this constructor after the default Student() constructor method. • The name of this method is also Student • Pre-conditions: Receives – string for specifying name – int for giving the credit hours earned – double for giving the GPA
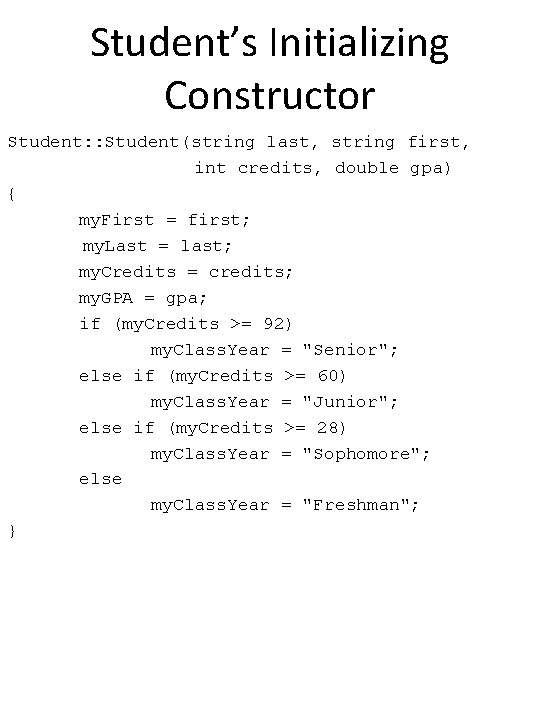
Student’s Initializing Constructor Student: : Student(string last, string first, int credits, double gpa) { my. First = first; my. Last = last; my. Credits = credits; my. GPA = gpa; if (my. Credits >= 92) my. Class. Year = "Senior"; else if (my. Credits >= 60) my. Class. Year = "Junior"; else if (my. Credits >= 28) my. Class. Year = "Sophomore"; else my. Class. Year = "Freshman"; }
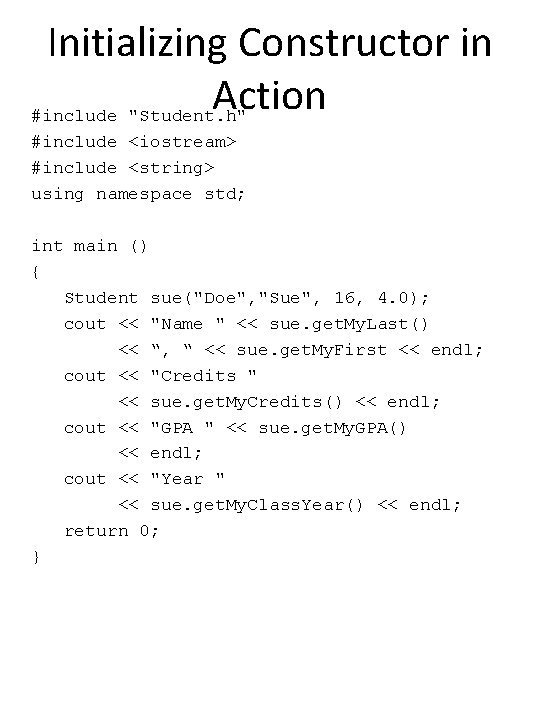
Initializing Constructor in Action #include "Student. h" #include <iostream> #include <string> using namespace std; int main () { Student sue("Doe", "Sue", 16, 4. 0); cout << "Name " << sue. get. My. Last() << “, “ << sue. get. My. First << endl; cout << "Credits " << sue. get. My. Credits() << endl; cout << "GPA " << sue. get. My. GPA() << endl; cout << "Year " << sue. get. My. Class. Year() << endl; return 0; }
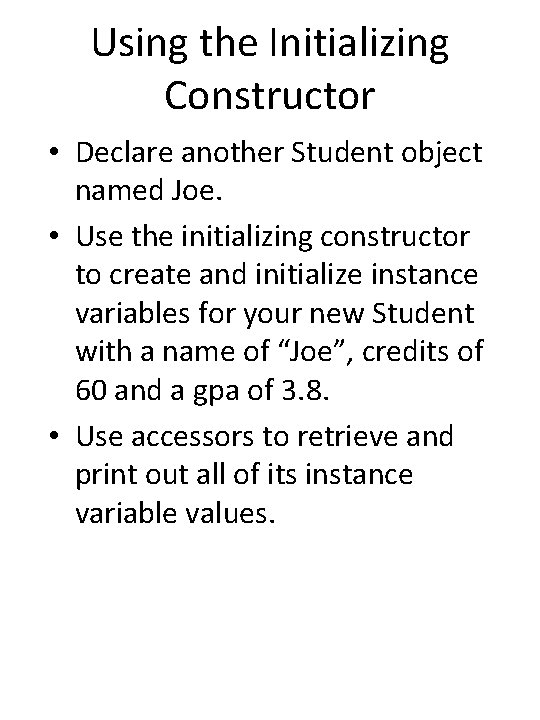
Using the Initializing Constructor • Declare another Student object named Joe. • Use the initializing constructor to create and initialize instance variables for your new Student with a name of “Joe”, credits of 60 and a gpa of 3. 8. • Use accessors to retrieve and print out all of its instance variable values.
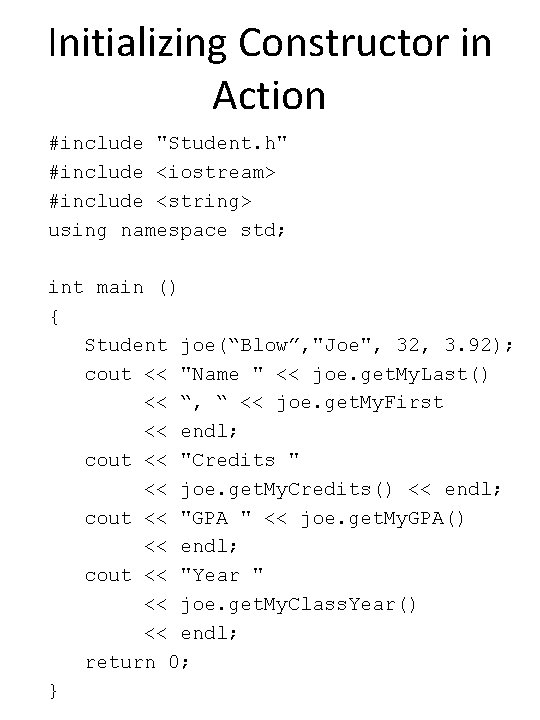
Initializing Constructor in Action #include "Student. h" #include <iostream> #include <string> using namespace std; int main () { Student joe(“Blow”, "Joe", 32, 3. 92); cout << "Name " << joe. get. My. Last() << “, “ << joe. get. My. First << endl; cout << "Credits " << joe. get. My. Credits() << endl; cout << "GPA " << joe. get. My. GPA() << endl; cout << "Year " << joe. get. My. Class. Year() << endl; return 0; }
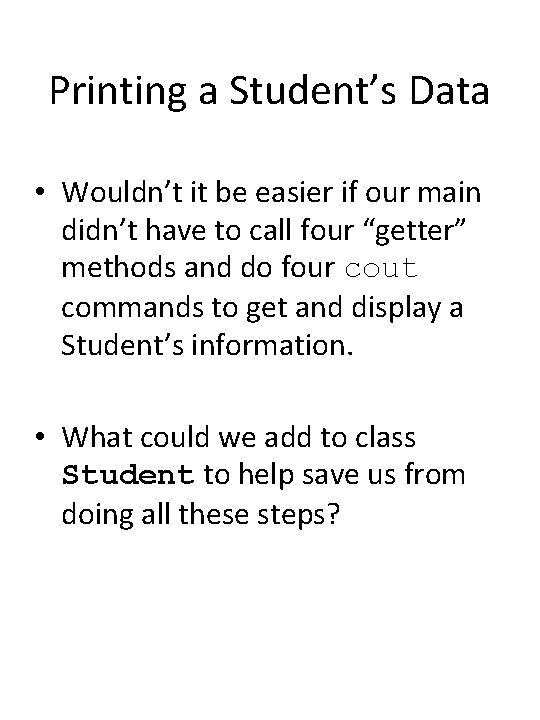
Printing a Student’s Data • Wouldn’t it be easier if our main didn’t have to call four “getter” methods and do four cout commands to get and display a Student’s information. • What could we add to class Student to help save us from doing all these steps?
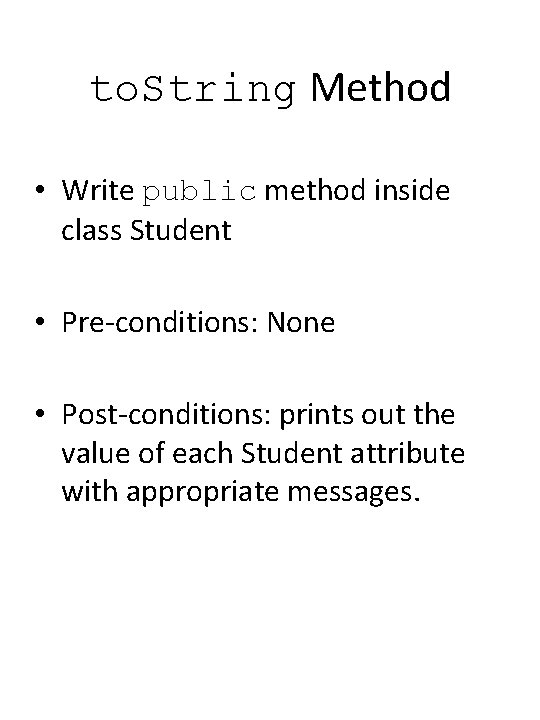
to. String Method • Write public method inside class Student • Pre-conditions: None • Post-conditions: prints out the value of each Student attribute with appropriate messages.
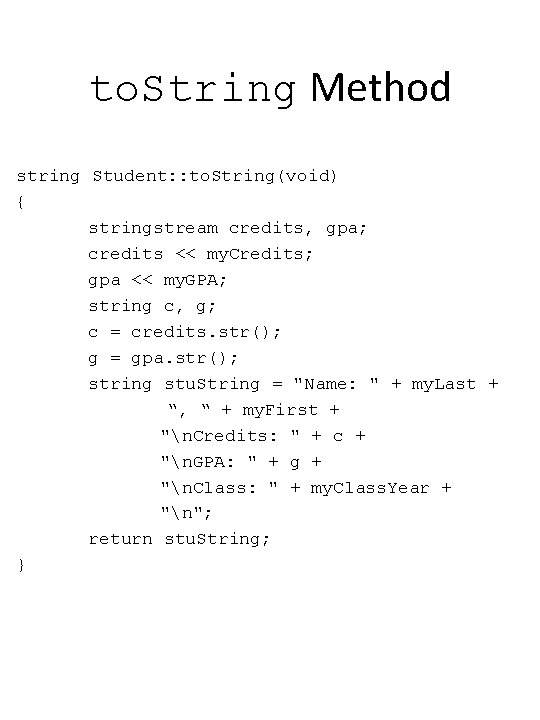
to. String Method string Student: : to. String(void) { stringstream credits, gpa; credits << my. Credits; gpa << my. GPA; string c, g; c = credits. str(); g = gpa. str(); string stu. String = "Name: " + my. Last + “, “ + my. First + "n. Credits: " + c + "n. GPA: " + g + "n. Class: " + my. Class. Year + "n"; return stu. String; }
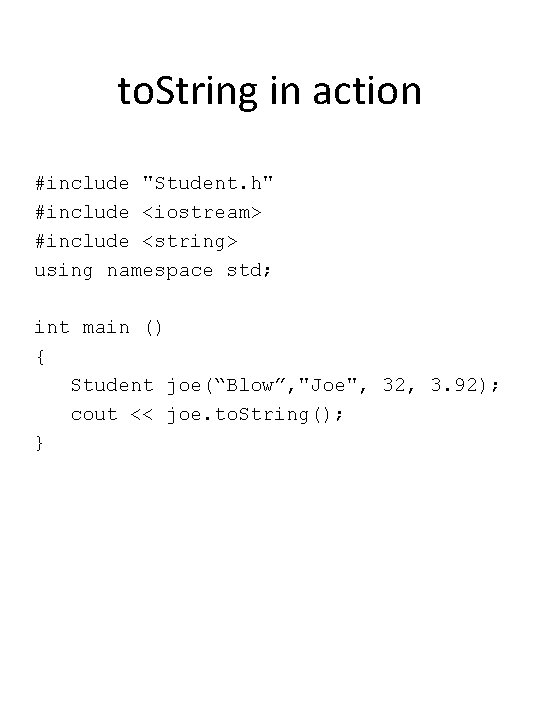
to. String in action #include "Student. h" #include <iostream> #include <string> using namespace std; int main () { Student joe(“Blow”, "Joe", 32, 3. 92); cout << joe. to. String(); }
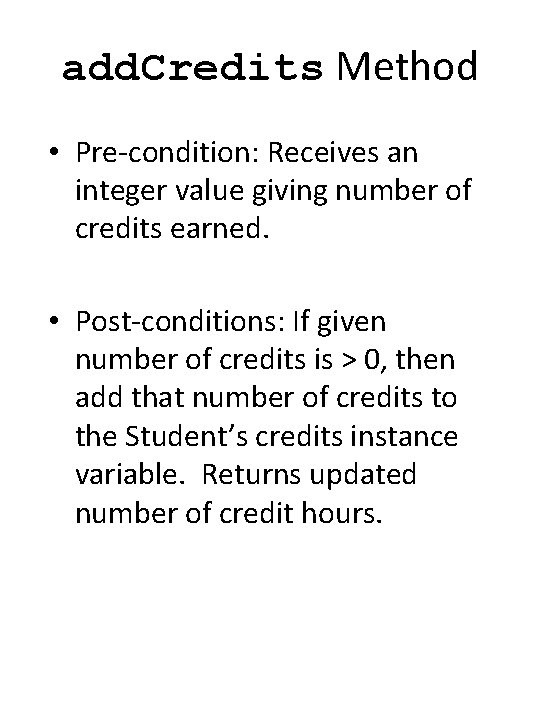
add. Credits Method • Pre-condition: Receives an integer value giving number of credits earned. • Post-conditions: If given number of credits is > 0, then add that number of credits to the Student’s credits instance variable. Returns updated number of credit hours.
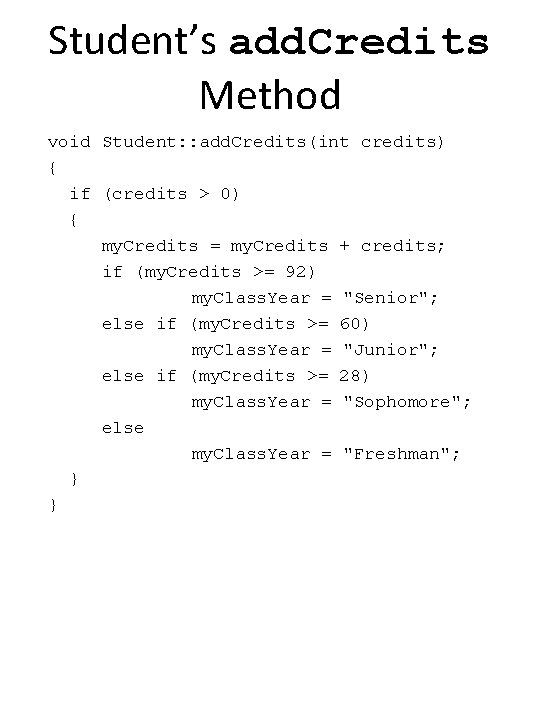
Student’s add. Credits Method void Student: : add. Credits(int credits) { if (credits > 0) { my. Credits = my. Credits + credits; if (my. Credits >= 92) my. Class. Year = "Senior"; else if (my. Credits >= 60) my. Class. Year = "Junior"; else if (my. Credits >= 28) my. Class. Year = "Sophomore"; else my. Class. Year = "Freshman"; } }
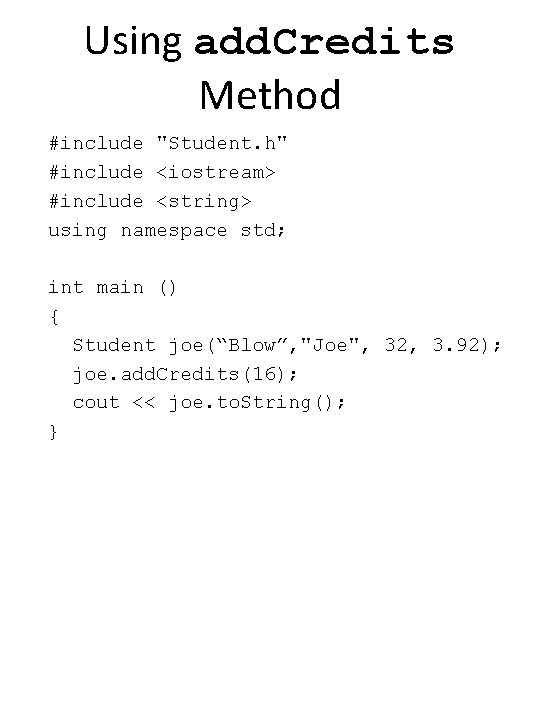
Using add. Credits Method #include "Student. h" #include <iostream> #include <string> using namespace std; int main () { Student joe(“Blow”, "Joe", 32, 3. 92); joe. add. Credits(16); cout << joe. to. String(); }