Data Programming Design and Modularization IS 101 YCMSC
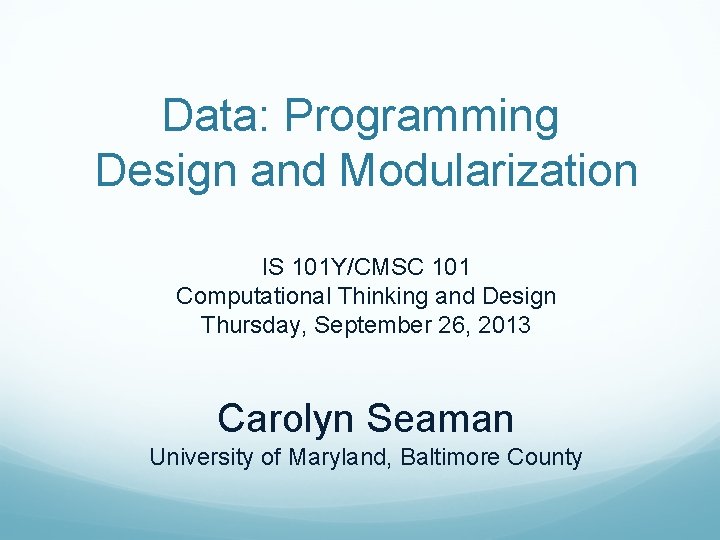
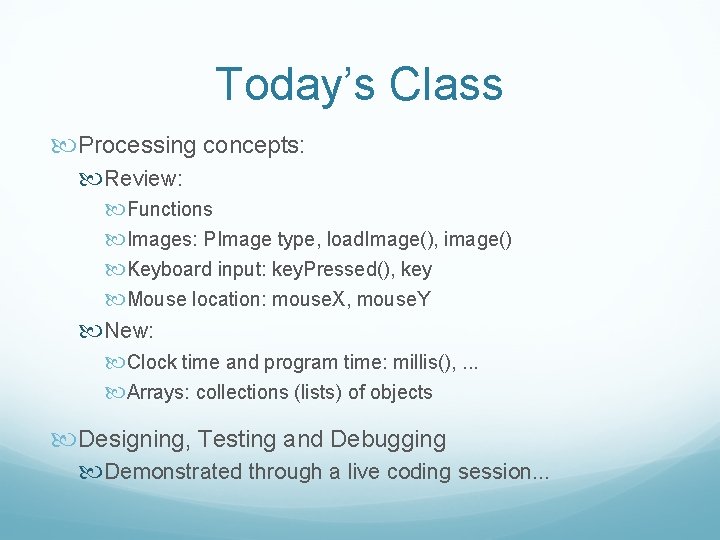
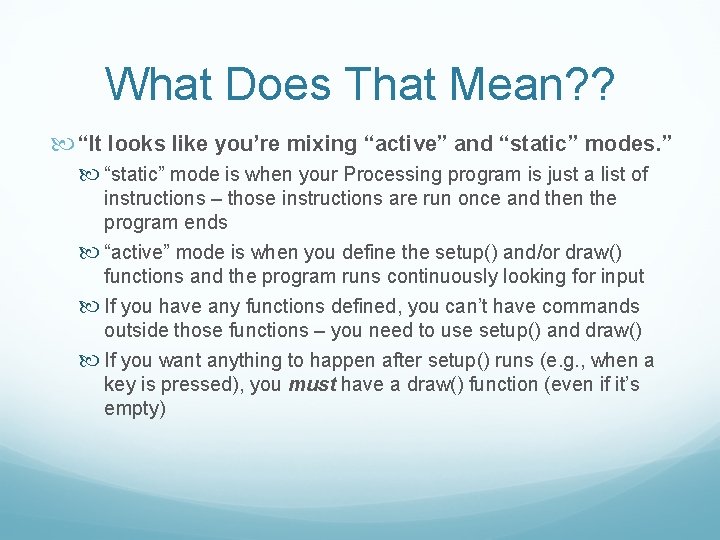
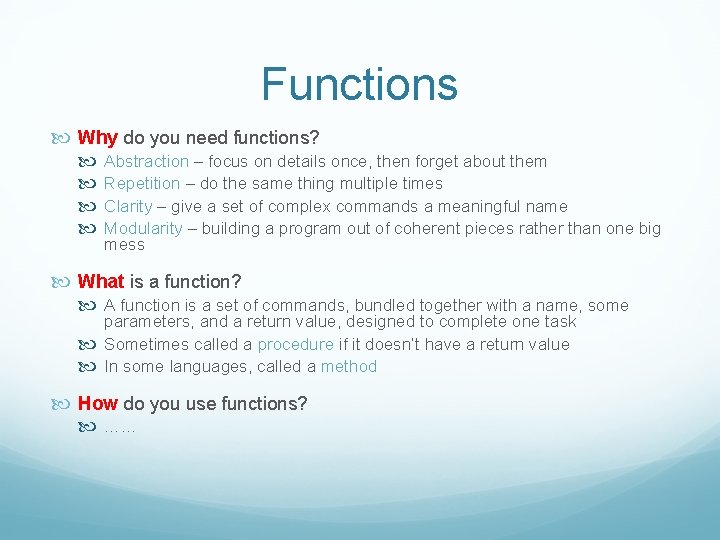
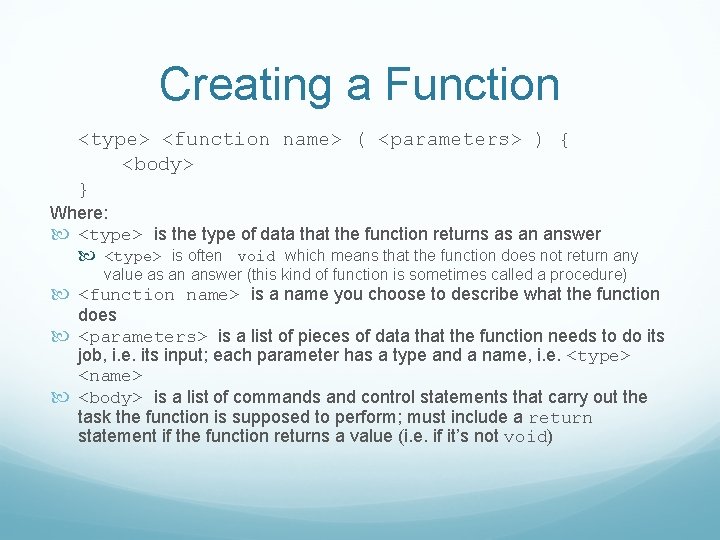
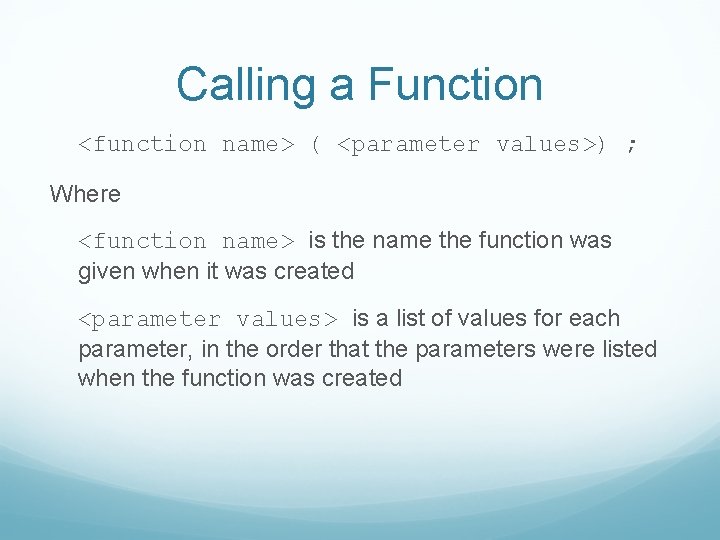
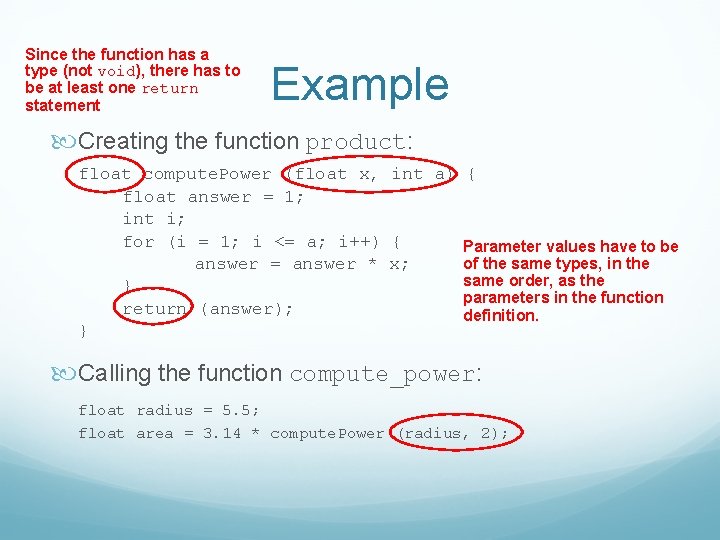
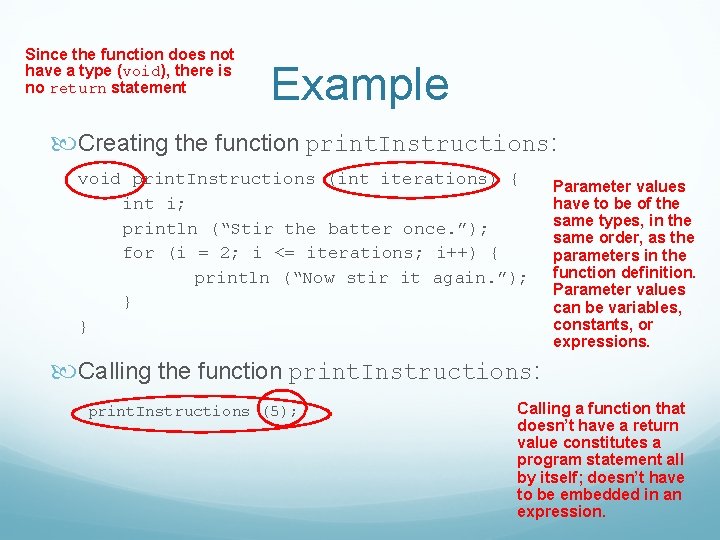
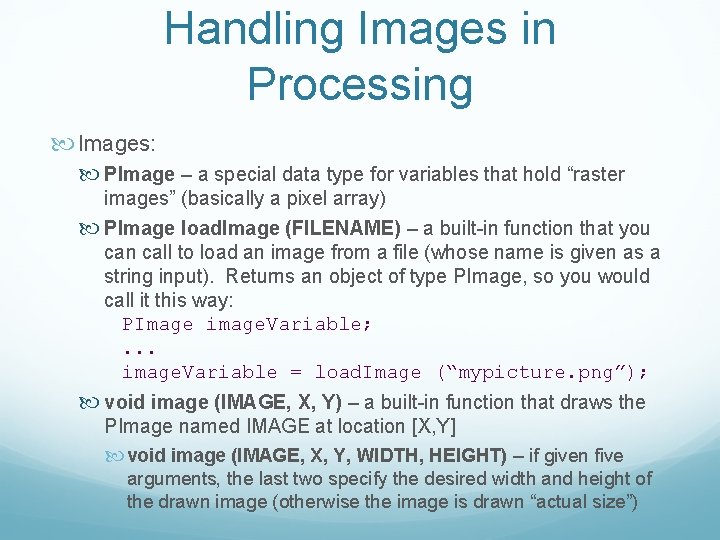
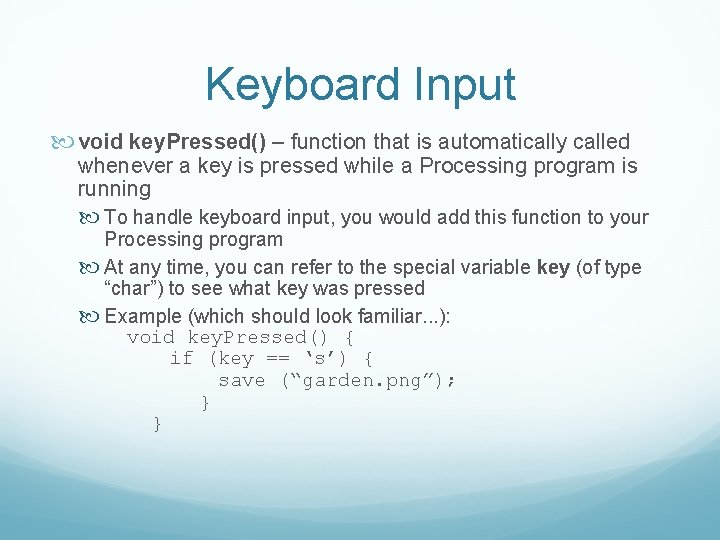
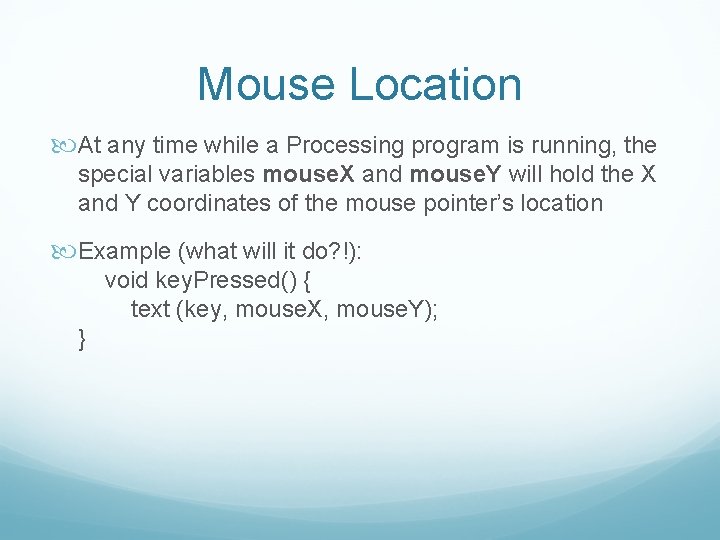
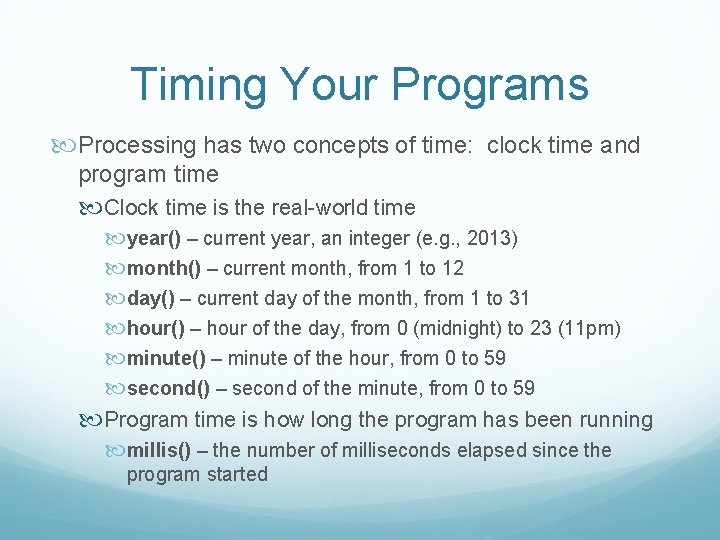
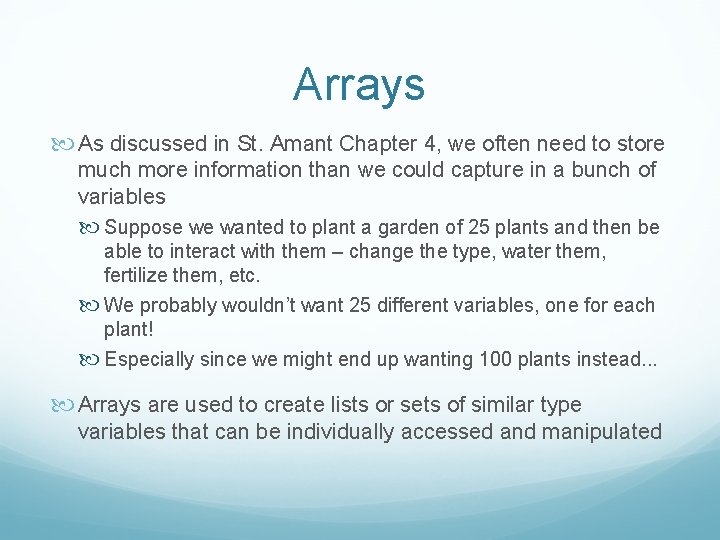
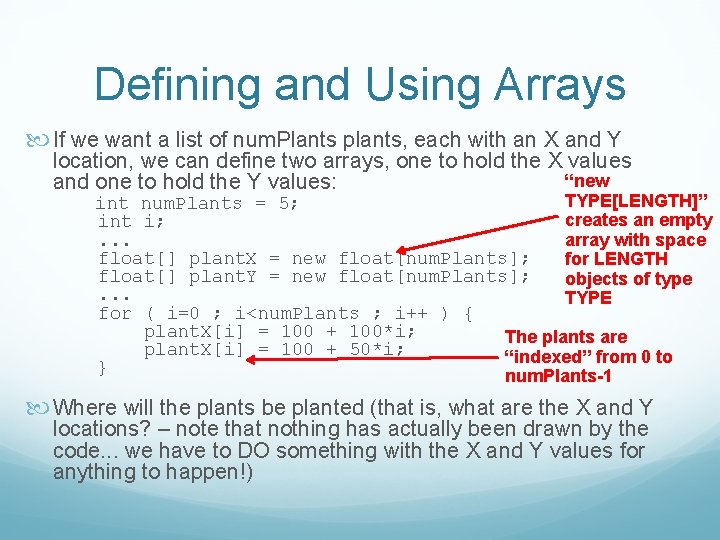
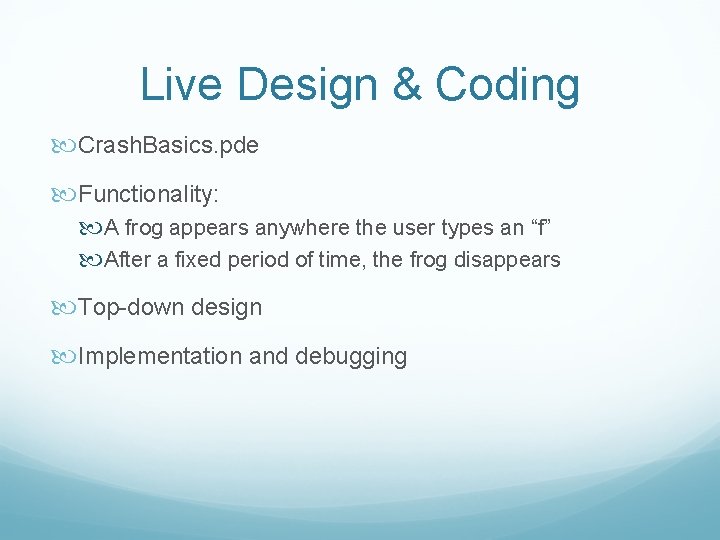
- Slides: 15
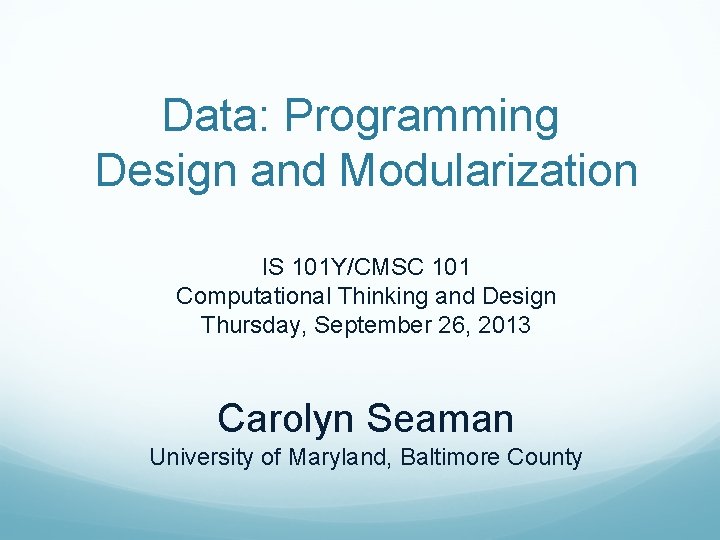
Data: Programming Design and Modularization IS 101 Y/CMSC 101 Computational Thinking and Design Thursday, September 26, 2013 Carolyn Seaman University of Maryland, Baltimore County
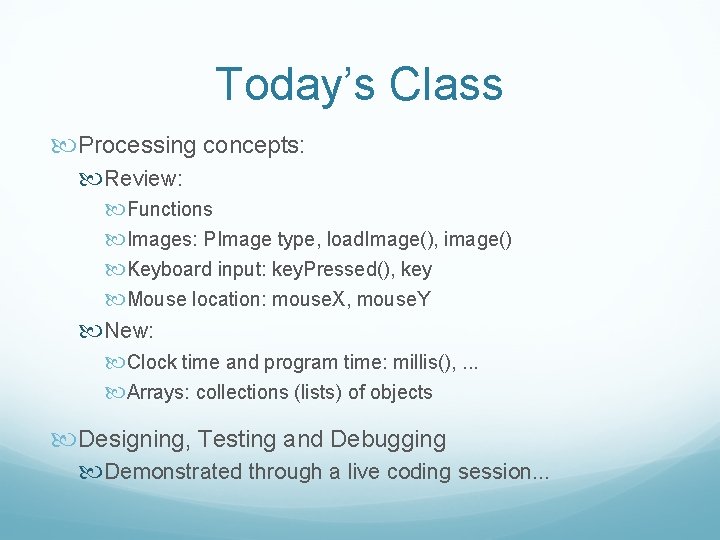
Today’s Class Processing concepts: Review: Functions Images: PImage type, load. Image(), image() Keyboard input: key. Pressed(), key Mouse location: mouse. X, mouse. Y New: Clock time and program time: millis(), . . . Arrays: collections (lists) of objects Designing, Testing and Debugging Demonstrated through a live coding session. . .
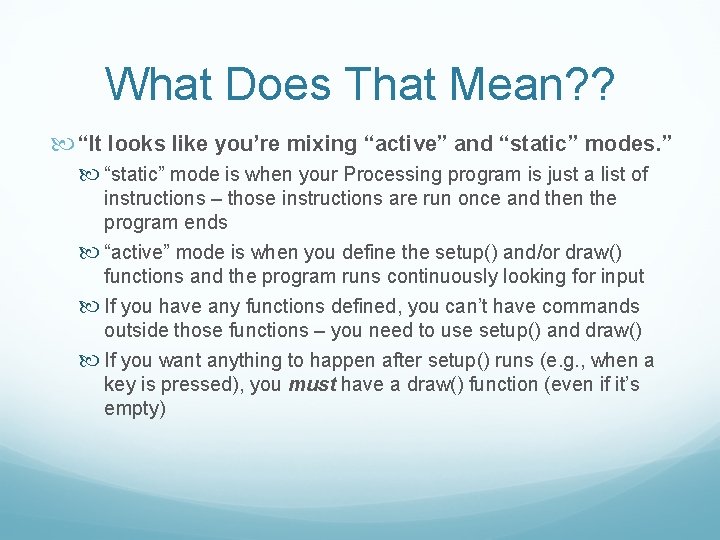
What Does That Mean? ? “It looks like you’re mixing “active” and “static” modes. ” “static” mode is when your Processing program is just a list of instructions – those instructions are run once and then the program ends “active” mode is when you define the setup() and/or draw() functions and the program runs continuously looking for input If you have any functions defined, you can’t have commands outside those functions – you need to use setup() and draw() If you want anything to happen after setup() runs (e. g. , when a key is pressed), you must have a draw() function (even if it’s empty)
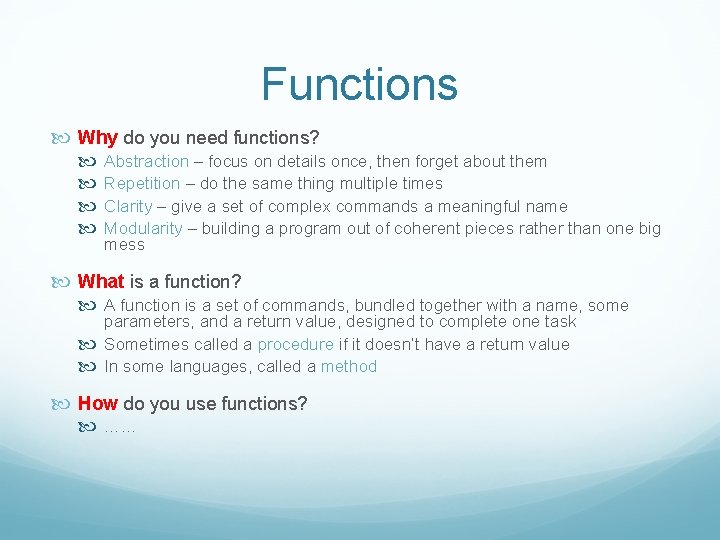
Functions Why do you need functions? Abstraction – focus on details once, then forget about them Repetition – do the same thing multiple times Clarity – give a set of complex commands a meaningful name Modularity – building a program out of coherent pieces rather than one big mess What is a function? A function is a set of commands, bundled together with a name, some parameters, and a return value, designed to complete one task Sometimes called a procedure if it doesn’t have a return value In some languages, called a method How do you use functions? ……
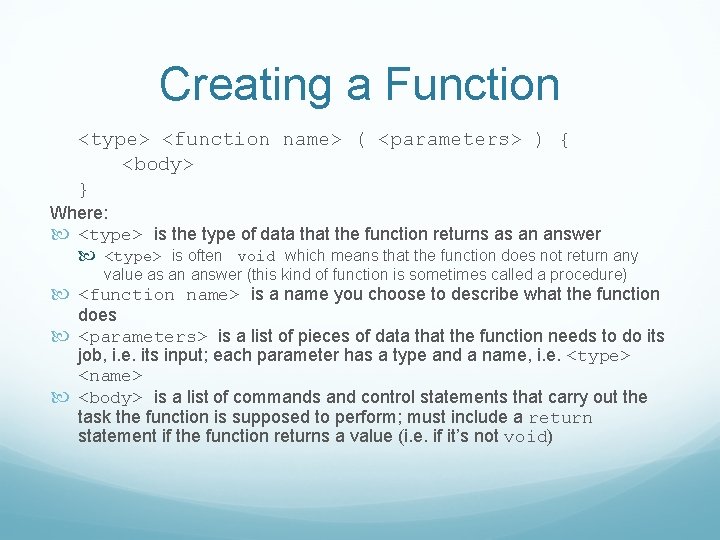
Creating a Function <type> <function name> ( <parameters> ) { <body> } Where: <type> is the type of data that the function returns as an answer <type> is often void which means that the function does not return any value as an answer (this kind of function is sometimes called a procedure) <function name> is a name you choose to describe what the function does <parameters> is a list of pieces of data that the function needs to do its job, i. e. its input; each parameter has a type and a name, i. e. <type> <name> <body> is a list of commands and control statements that carry out the task the function is supposed to perform; must include a return statement if the function returns a value (i. e. if it’s not void)
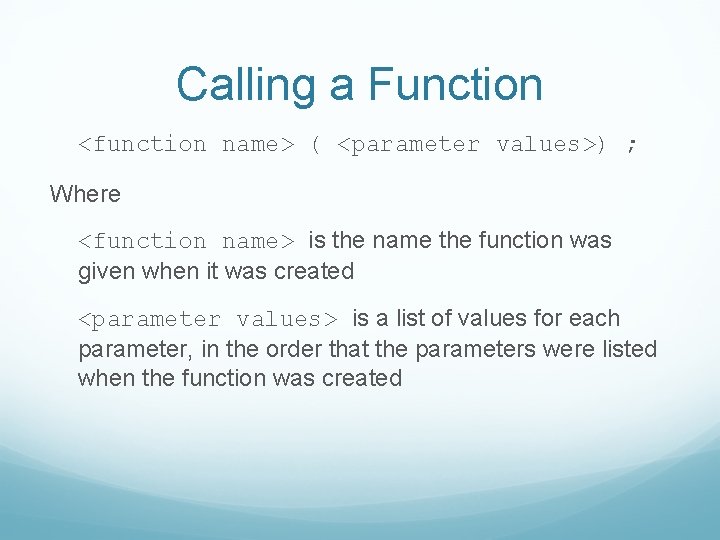
Calling a Function <function name> ( <parameter values>) ; Where <function name> is the name the function was given when it was created <parameter values> is a list of values for each parameter, in the order that the parameters were listed when the function was created
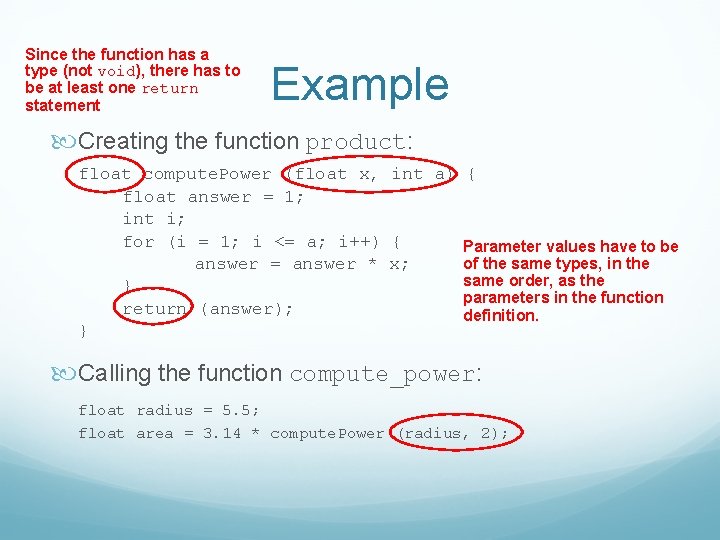
Since the function has a type (not void), there has to be at least one return statement Example Creating the function product: float compute. Power (float x, int a) { float answer = 1; int i; for (i = 1; i <= a; i++) { Parameter values have to be of the same types, in the answer = answer * x; same order, as the } parameters in the function return (answer); definition. } Calling the function compute_power: float radius = 5. 5; float area = 3. 14 * compute. Power (radius, 2);
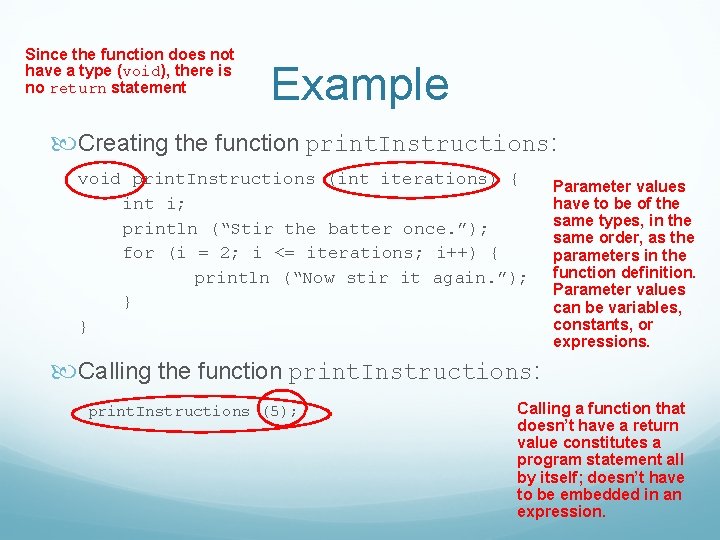
Since the function does not have a type (void), there is no return statement Example Creating the function print. Instructions: void print. Instructions (int iterations) { int i; println (“Stir the batter once. ”); for (i = 2; i <= iterations; i++) { println (“Now stir it again. ”); } } Parameter values have to be of the same types, in the same order, as the parameters in the function definition. Parameter values can be variables, constants, or expressions. Calling the function print. Instructions: print. Instructions (5); Calling a function that doesn’t have a return value constitutes a program statement all by itself; doesn’t have to be embedded in an expression.
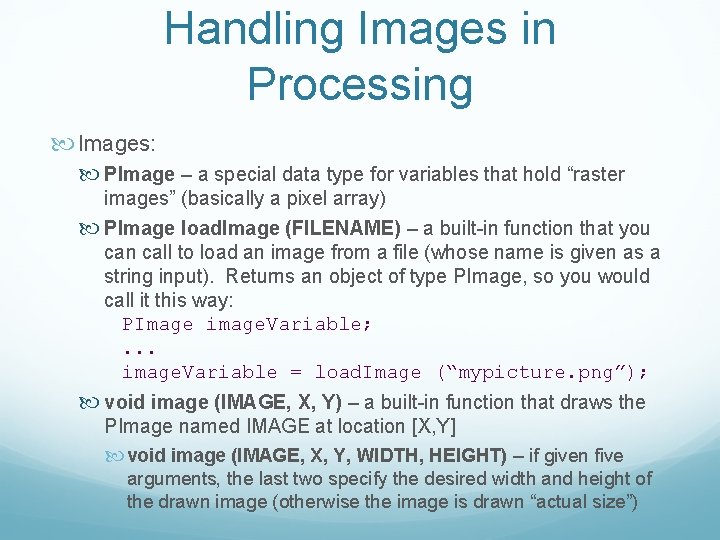
Handling Images in Processing Images: PImage – a special data type for variables that hold “raster images” (basically a pixel array) PImage load. Image (FILENAME) – a built-in function that you can call to load an image from a file (whose name is given as a string input). Returns an object of type PImage, so you would call it this way: PImage image. Variable; . . . image. Variable = load. Image (“mypicture. png”); void image (IMAGE, X, Y) – a built-in function that draws the PImage named IMAGE at location [X, Y] void image (IMAGE, X, Y, WIDTH, HEIGHT) – if given five arguments, the last two specify the desired width and height of the drawn image (otherwise the image is drawn “actual size”)
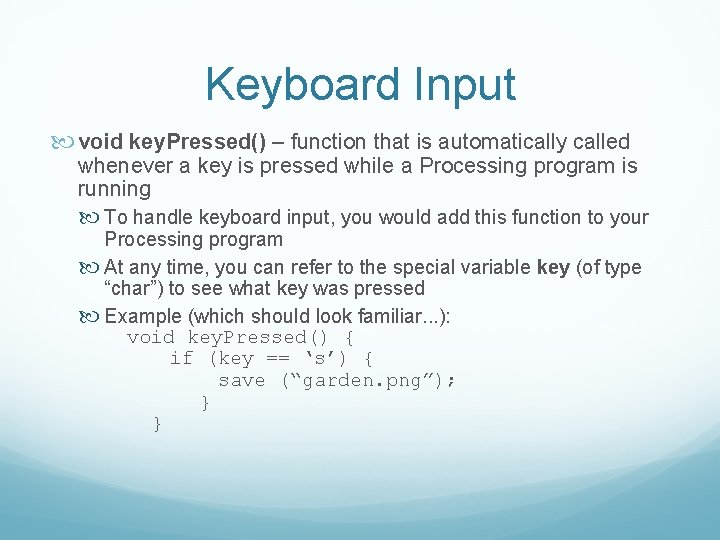
Keyboard Input void key. Pressed() – function that is automatically called whenever a key is pressed while a Processing program is running To handle keyboard input, you would add this function to your Processing program At any time, you can refer to the special variable key (of type “char”) to see what key was pressed Example (which should look familiar. . . ): void key. Pressed() { if (key == ‘s’) { save (“garden. png”); } }
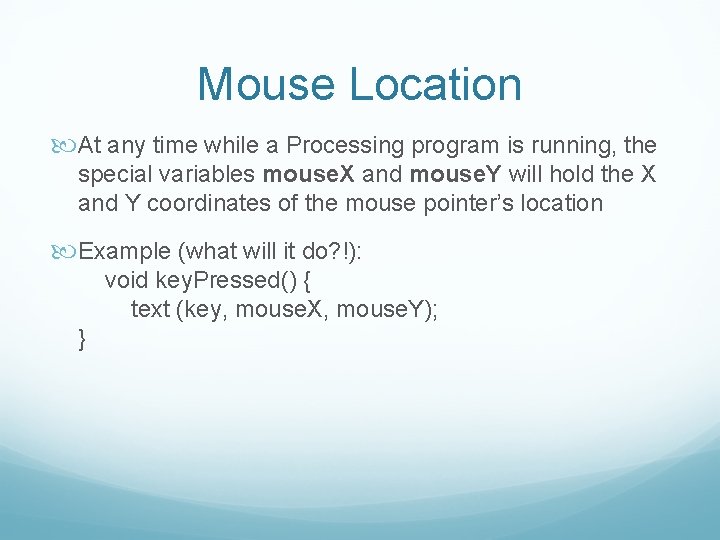
Mouse Location At any time while a Processing program is running, the special variables mouse. X and mouse. Y will hold the X and Y coordinates of the mouse pointer’s location Example (what will it do? !): void key. Pressed() { text (key, mouse. X, mouse. Y); }
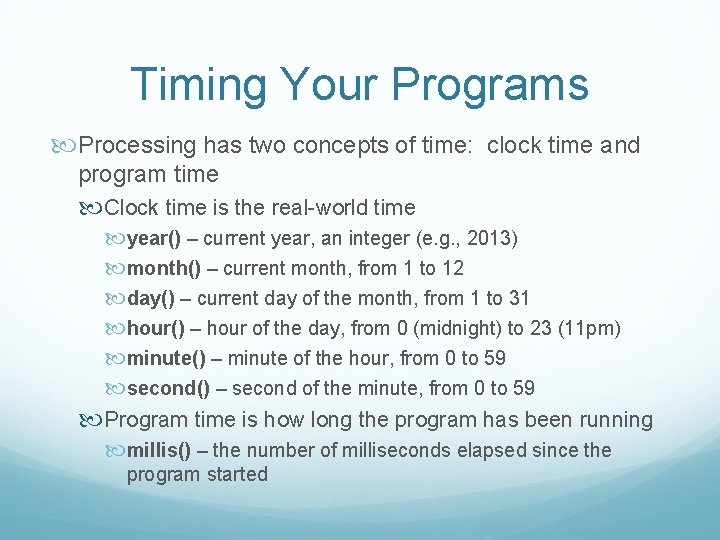
Timing Your Programs Processing has two concepts of time: clock time and program time Clock time is the real-world time year() – current year, an integer (e. g. , 2013) month() – current month, from 1 to 12 day() – current day of the month, from 1 to 31 hour() – hour of the day, from 0 (midnight) to 23 (11 pm) minute() – minute of the hour, from 0 to 59 second() – second of the minute, from 0 to 59 Program time is how long the program has been running millis() – the number of milliseconds elapsed since the program started
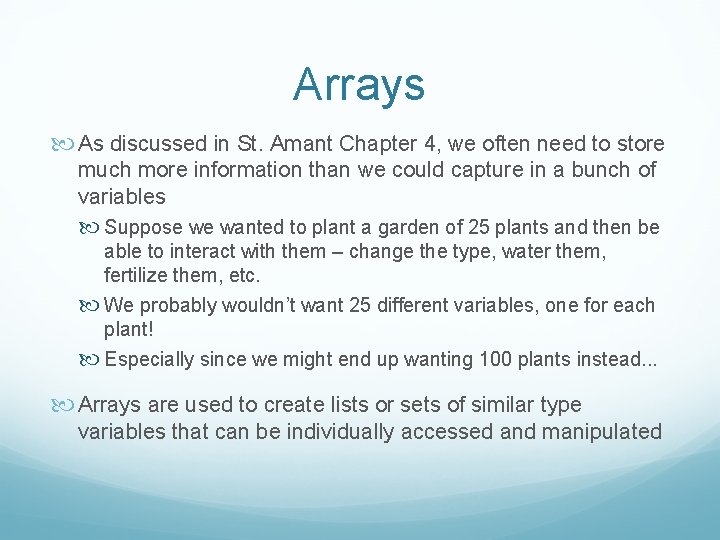
Arrays As discussed in St. Amant Chapter 4, we often need to store much more information than we could capture in a bunch of variables Suppose we wanted to plant a garden of 25 plants and then be able to interact with them – change the type, water them, fertilize them, etc. We probably wouldn’t want 25 different variables, one for each plant! Especially since we might end up wanting 100 plants instead. . . Arrays are used to create lists or sets of similar type variables that can be individually accessed and manipulated
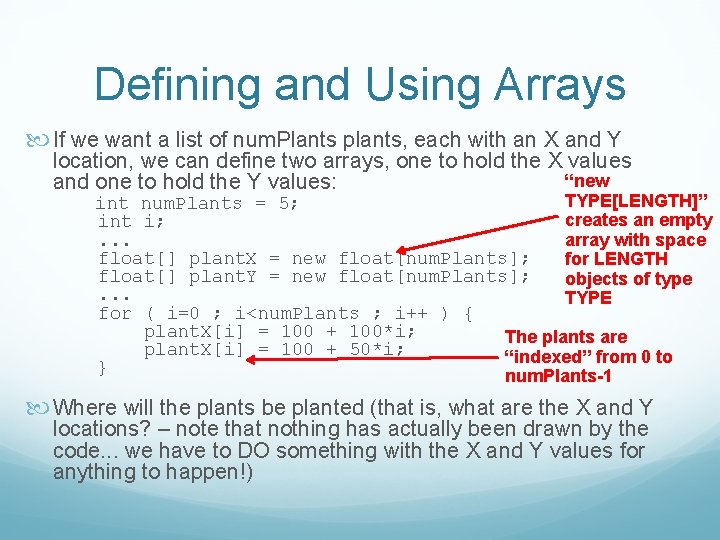
Defining and Using Arrays If we want a list of num. Plants plants, each with an X and Y location, we can define two arrays, one to hold the X values “new and one to hold the Y values: TYPE[LENGTH]” int num. Plants = 5; creates an empty int i; array with space. . . float[] plant. X = new float[num. Plants]; for LENGTH float[] plant. Y = new float[num. Plants]; objects of type. . . TYPE for ( i=0 ; i<num. Plants ; i++ ) { plant. X[i] = 100 + 100*i; The plants are plant. X[i] = 100 + 50*i; “indexed” from 0 to } num. Plants-1 Where will the plants be planted (that is, what are the X and Y locations? – note that nothing has actually been drawn by the code. . . we have to DO something with the X and Y values for anything to happen!)
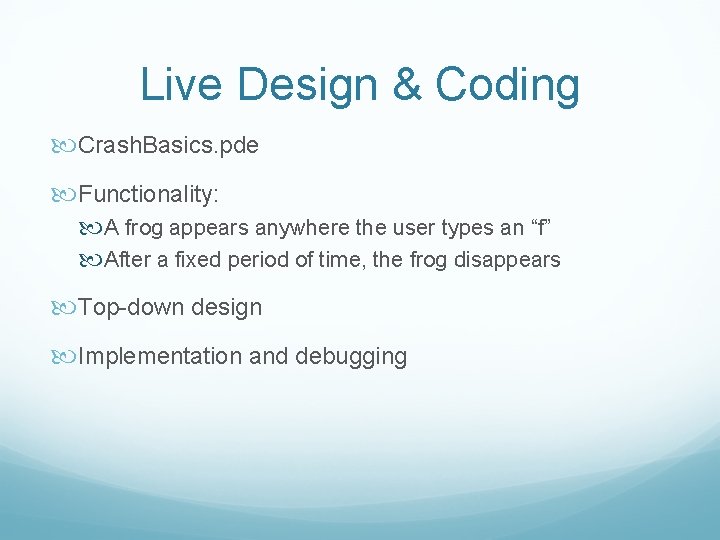
Live Design & Coding Crash. Basics. pde Functionality: A frog appears anywhere the user types an “f” After a fixed period of time, the frog disappears Top-down design Implementation and debugging