DATA HANDLING DATA HANDLING C Data Types Fundamental
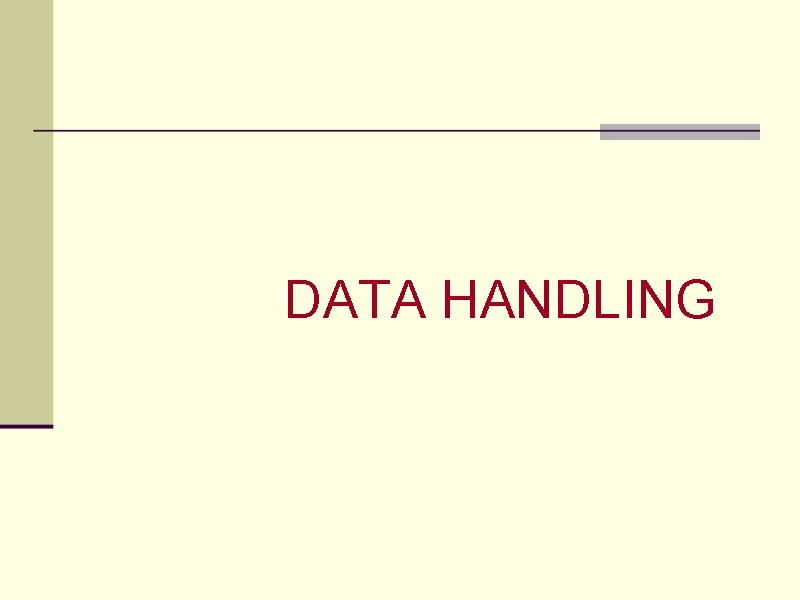
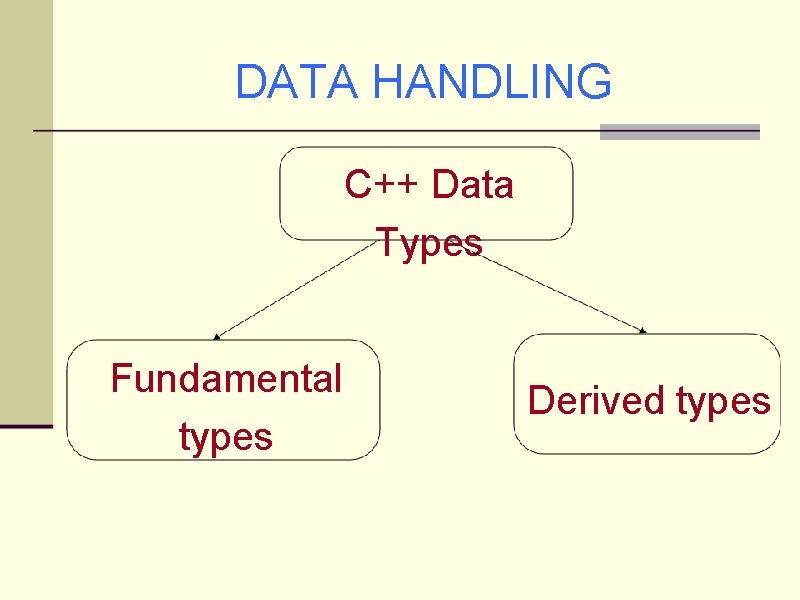
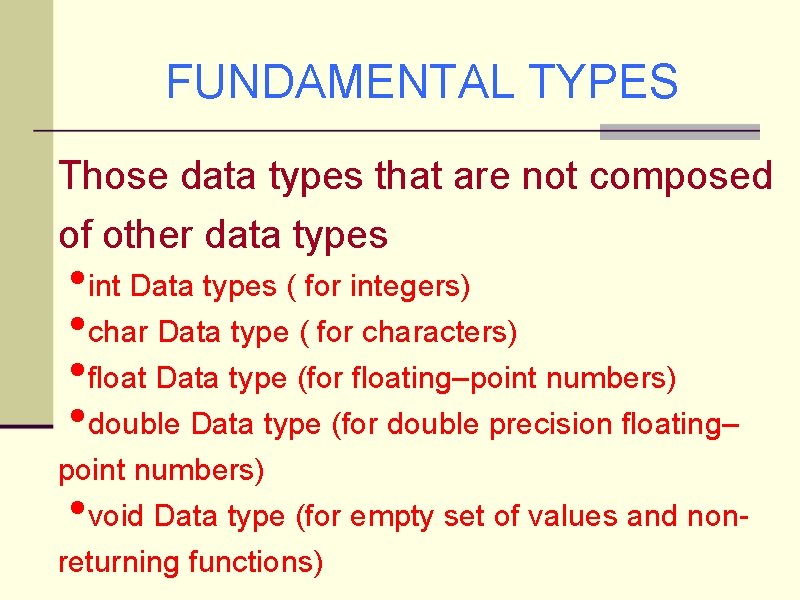
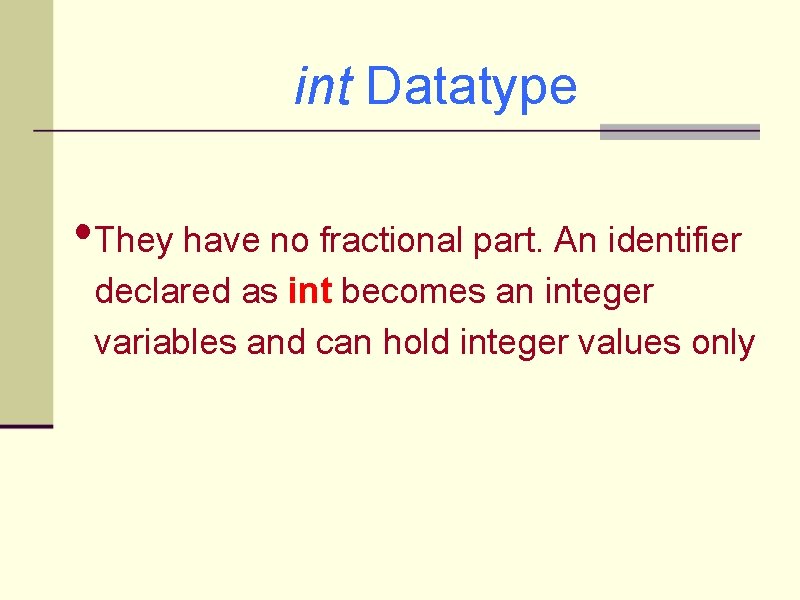
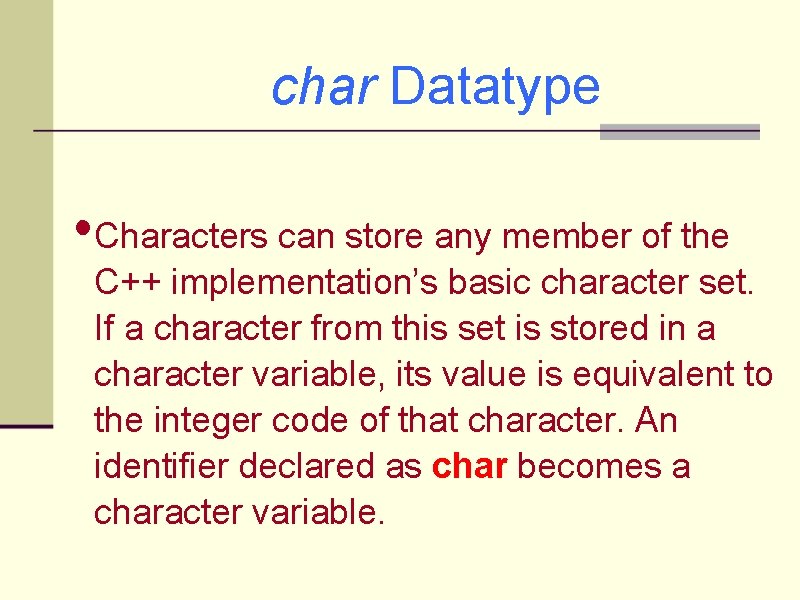
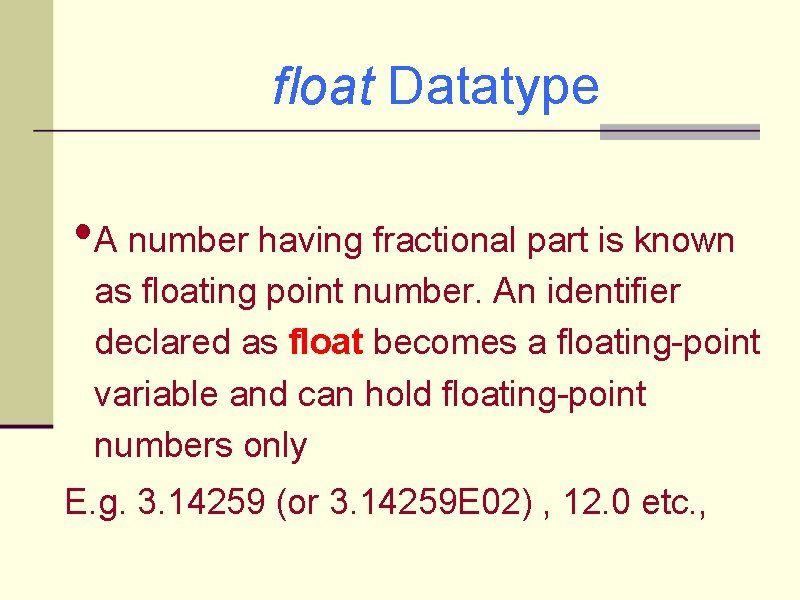
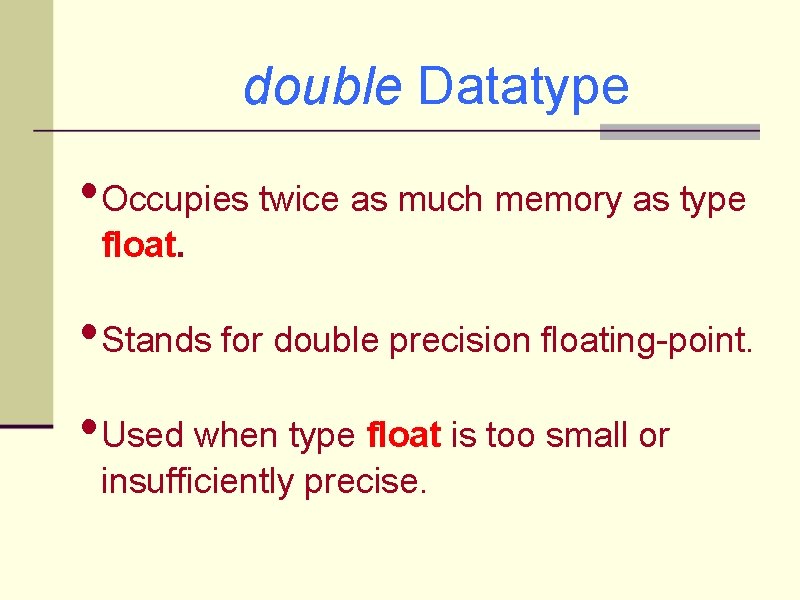
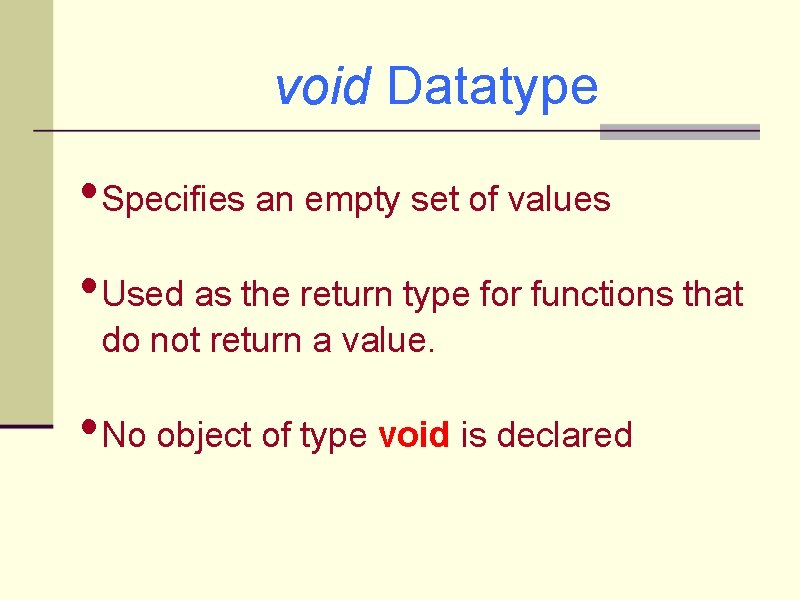
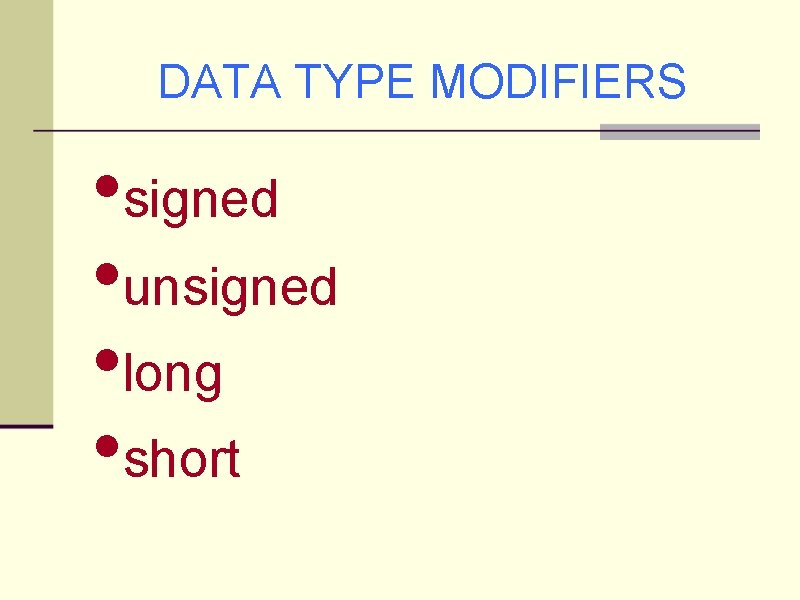
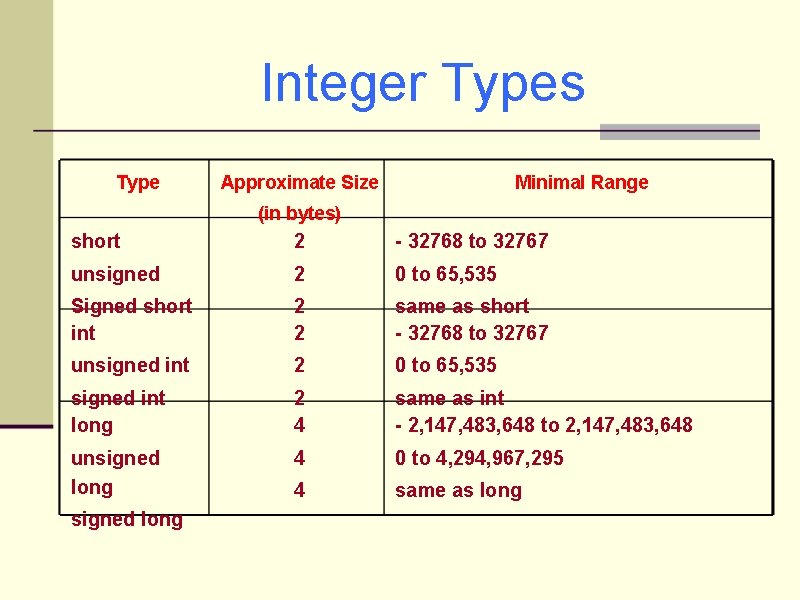
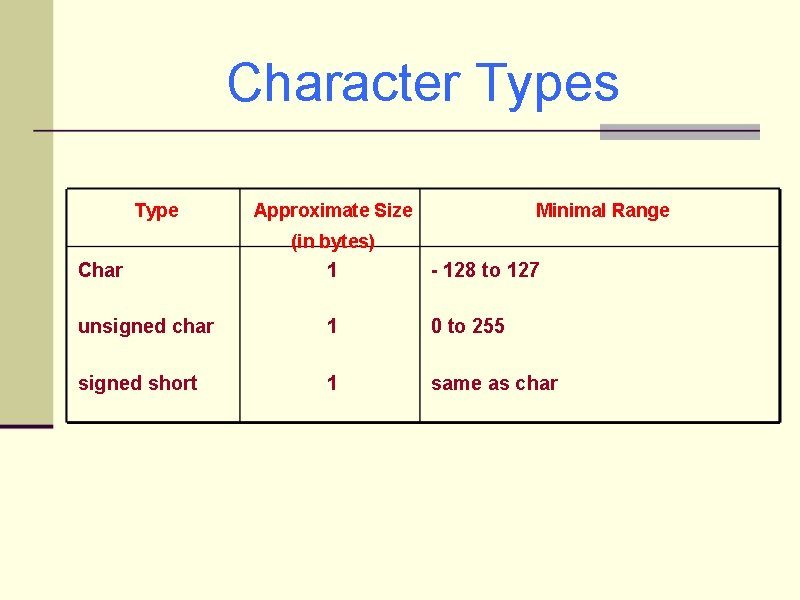
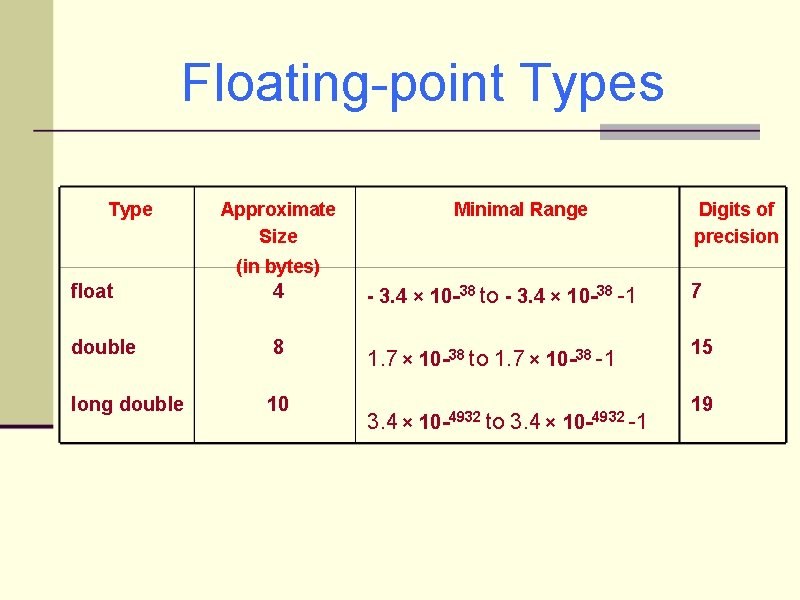
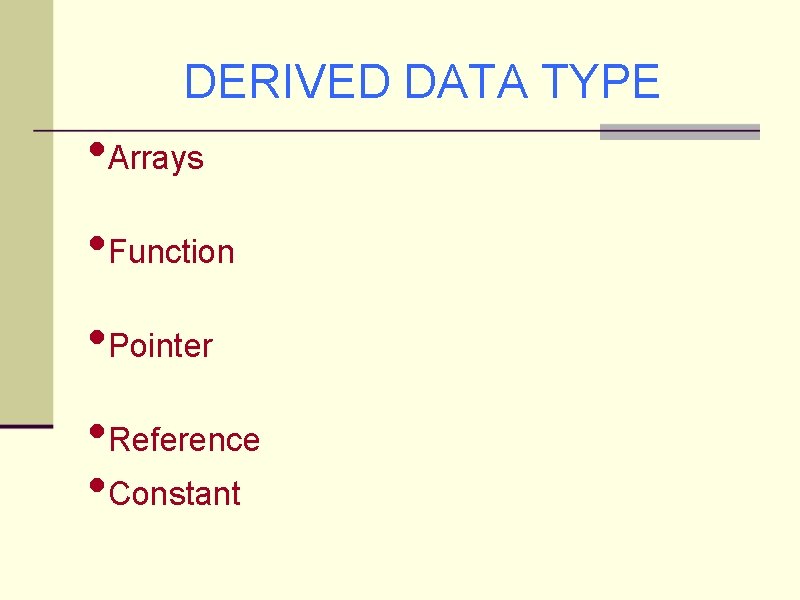
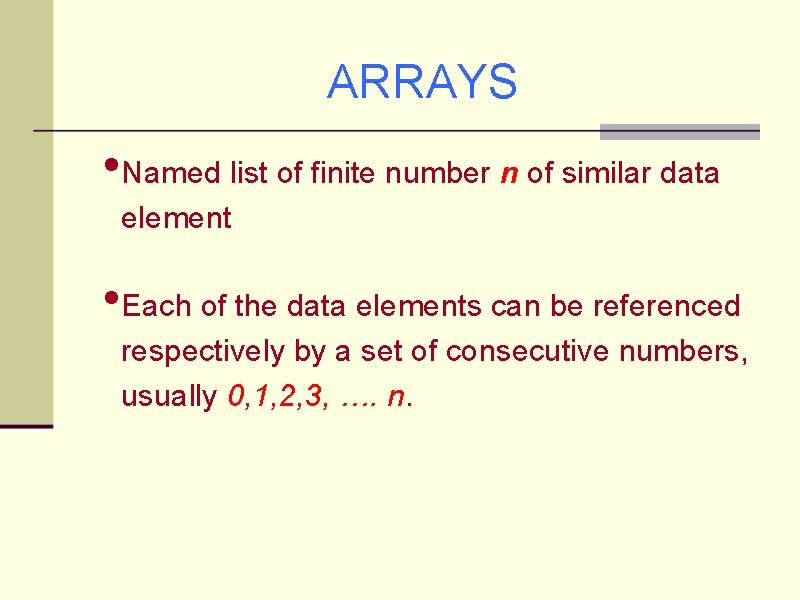
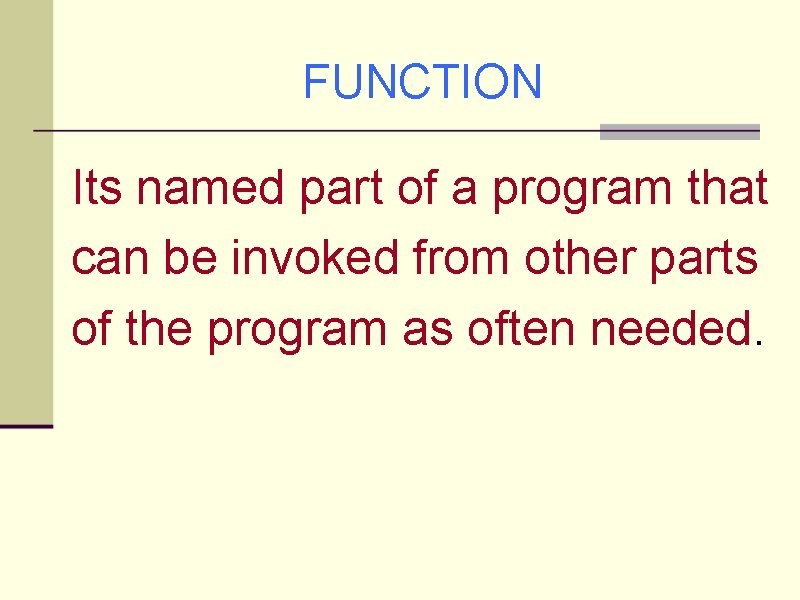
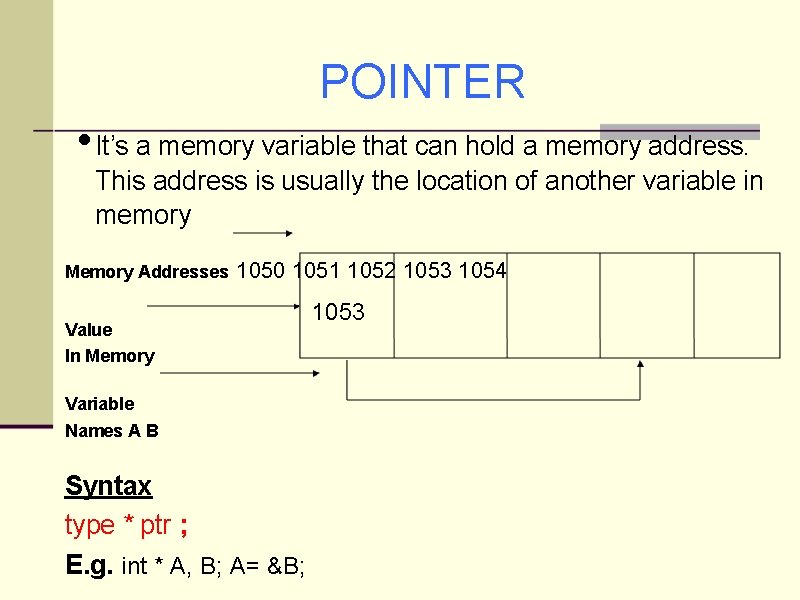
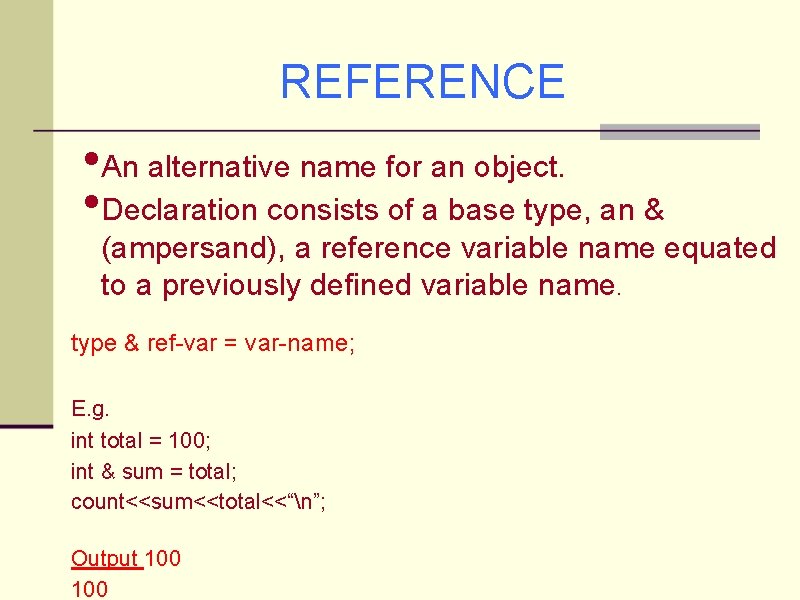
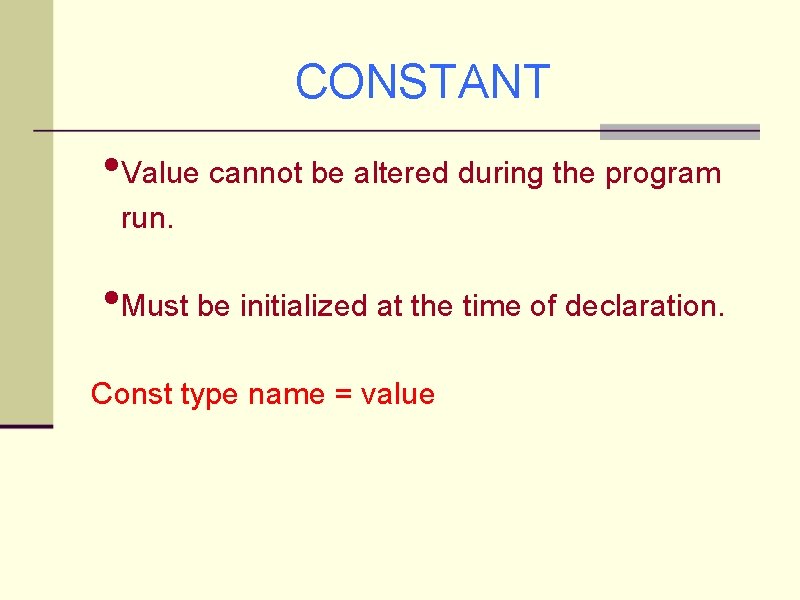
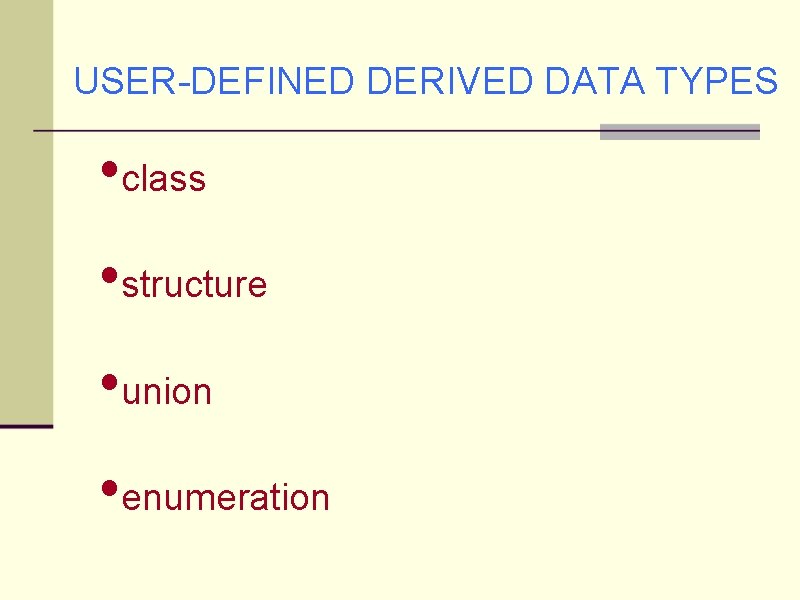
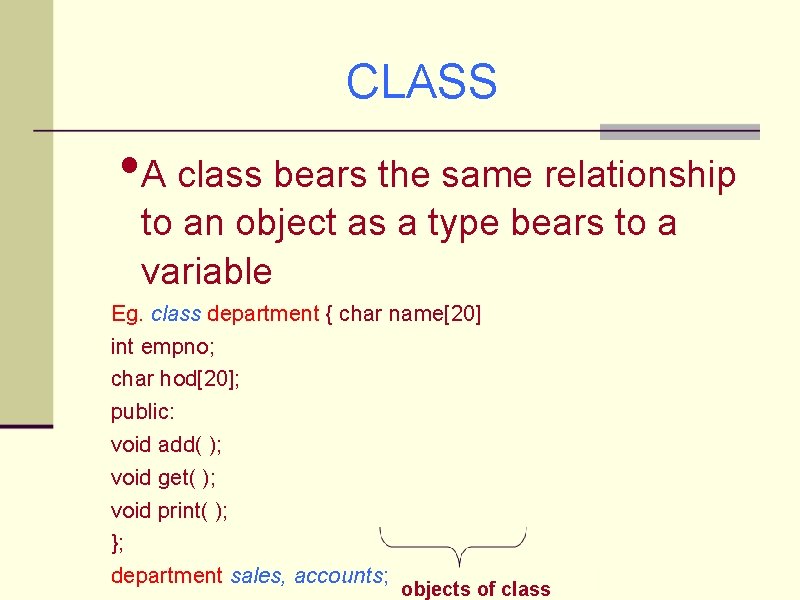
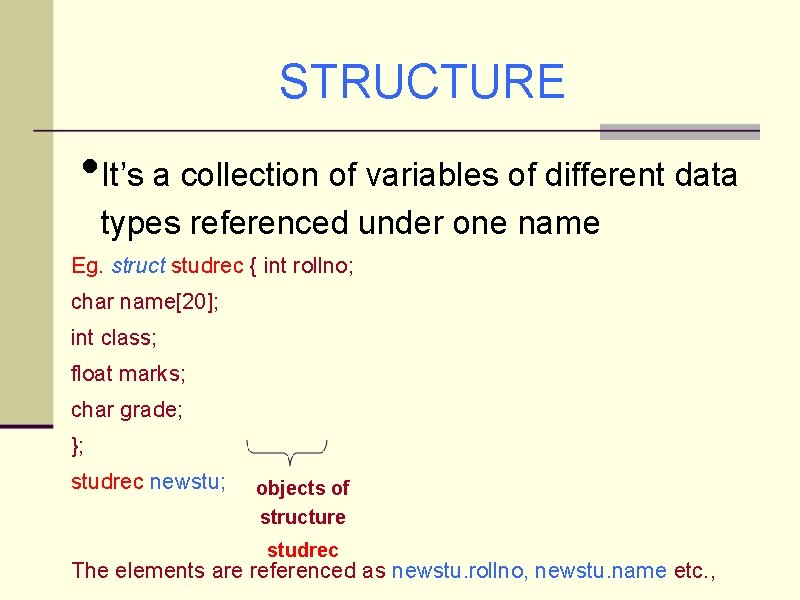
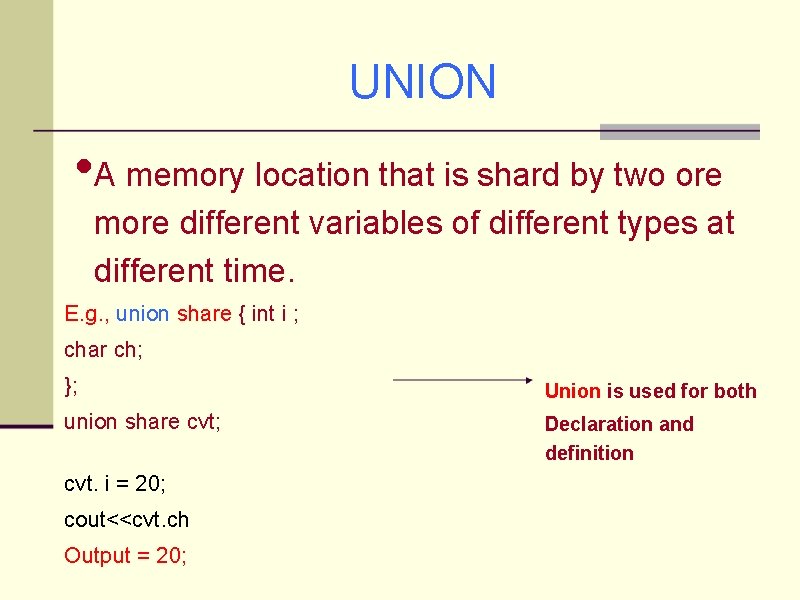
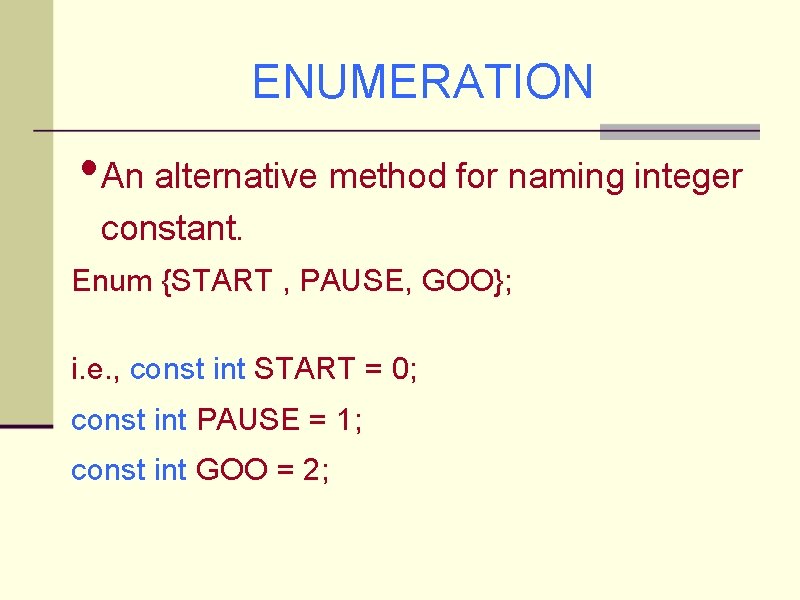
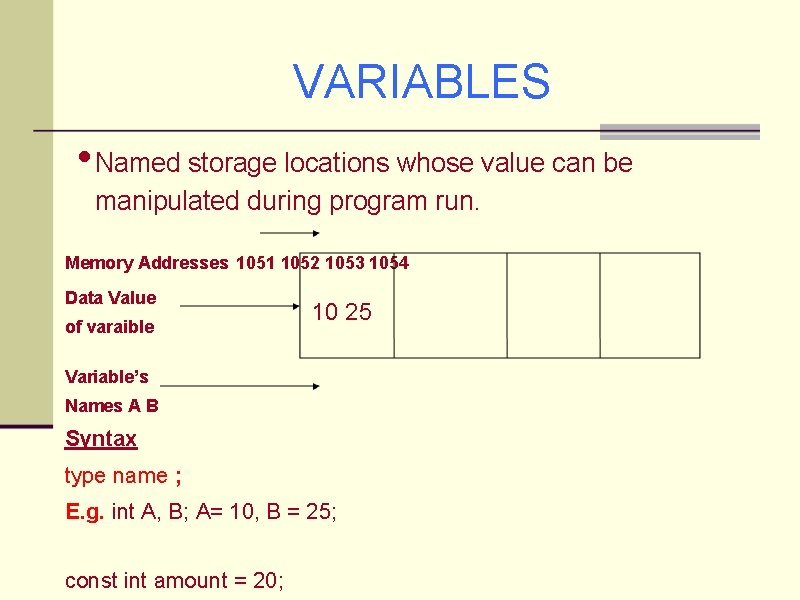
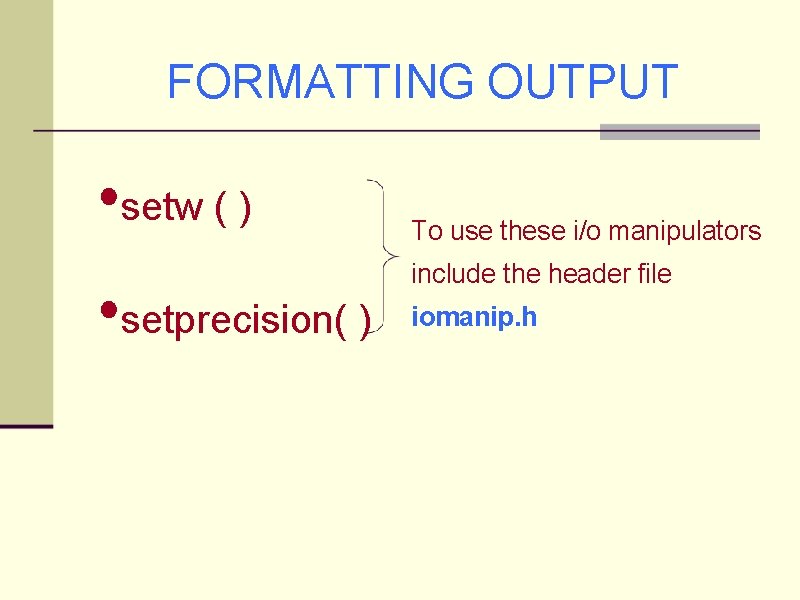
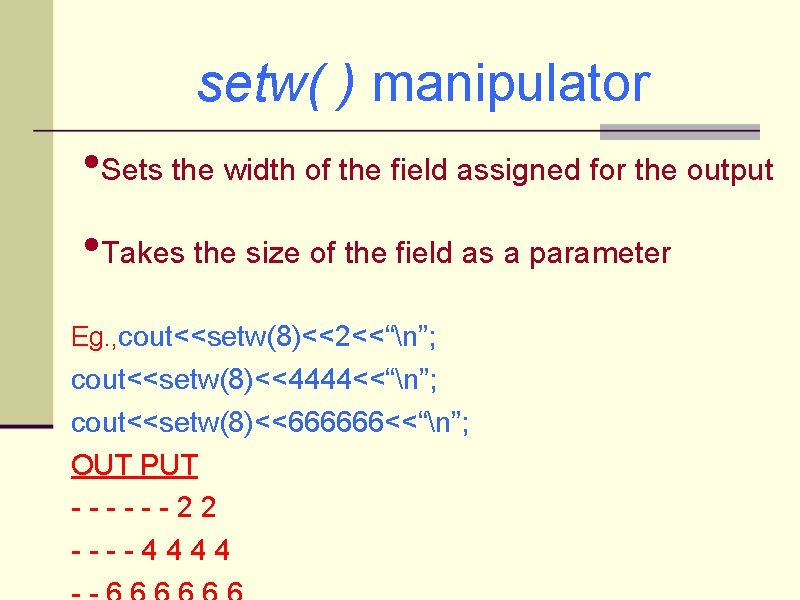
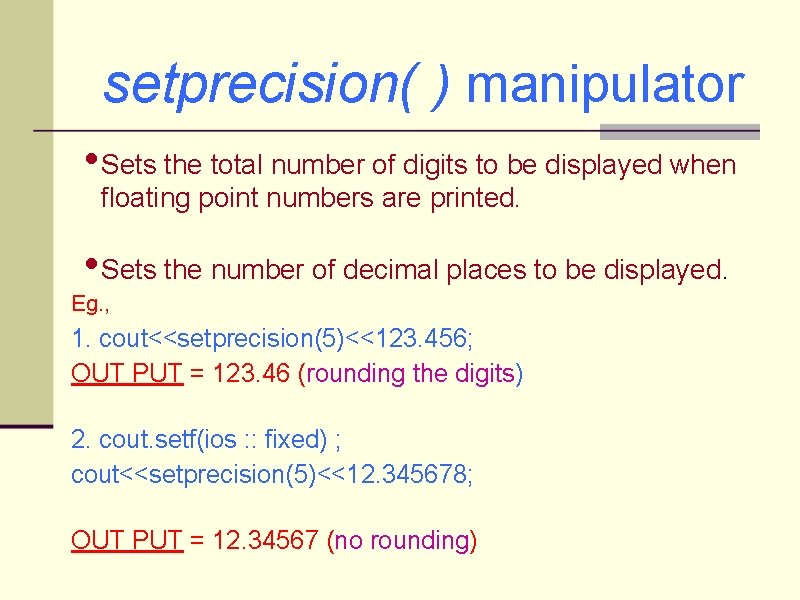
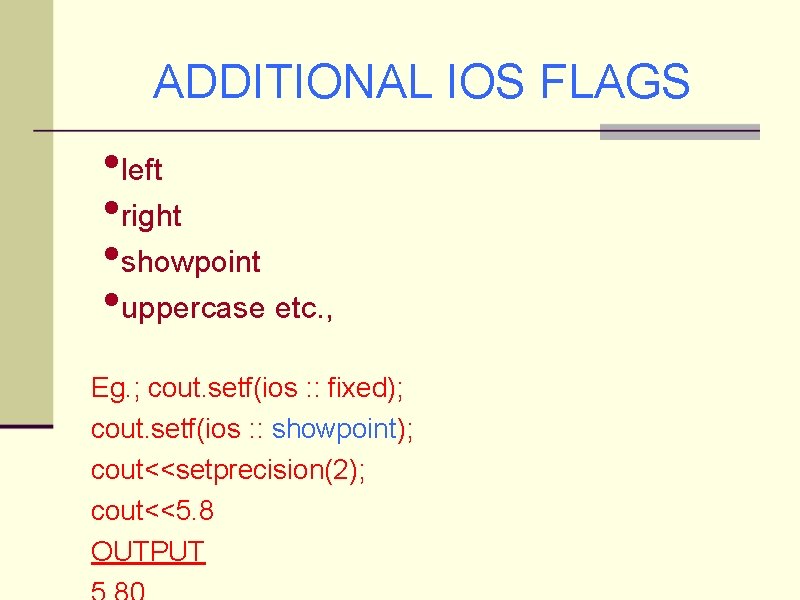
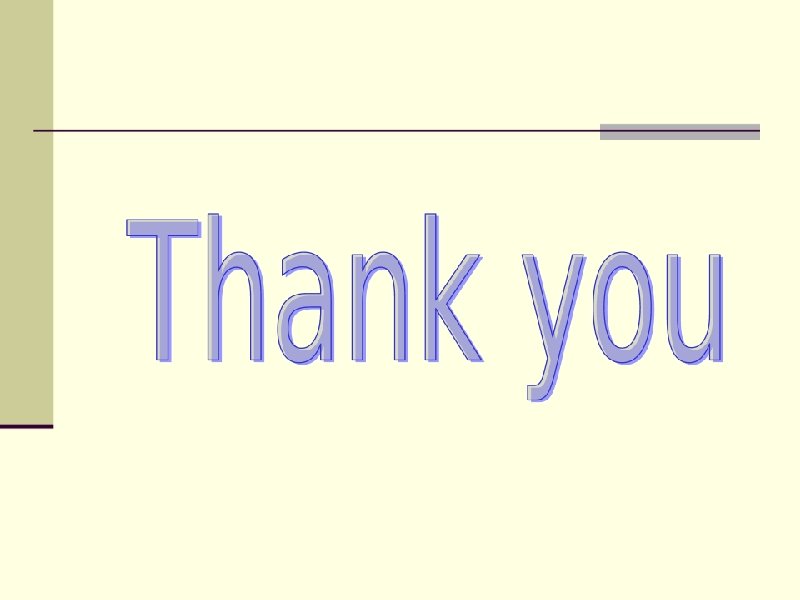
- Slides: 29
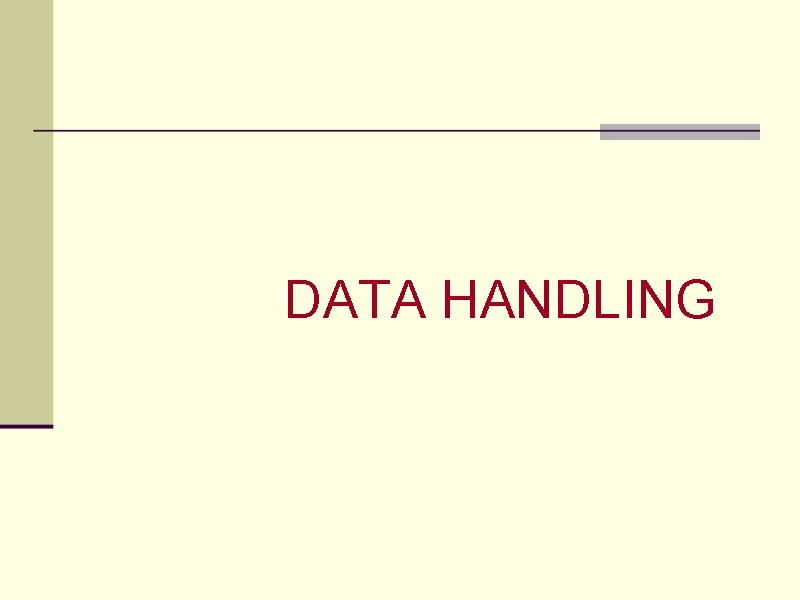
DATA HANDLING
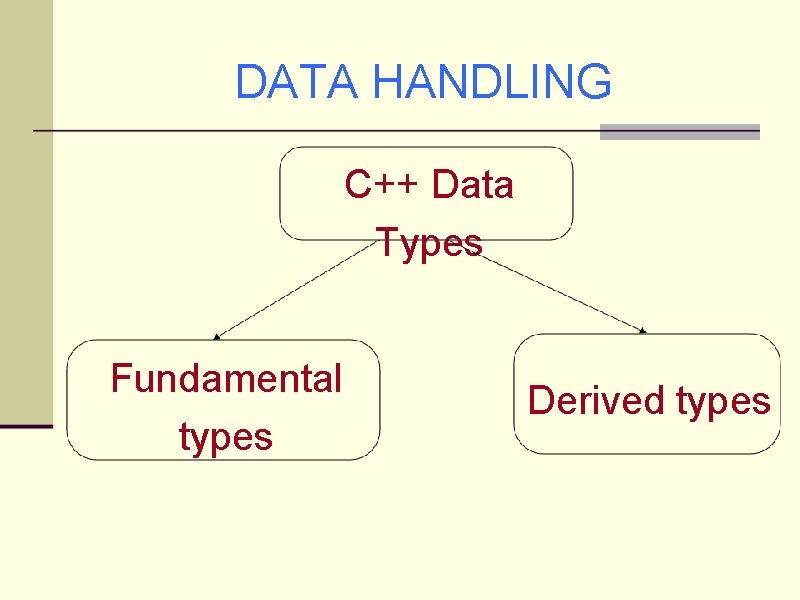
DATA HANDLING C++ Data Types Fundamental types Derived types
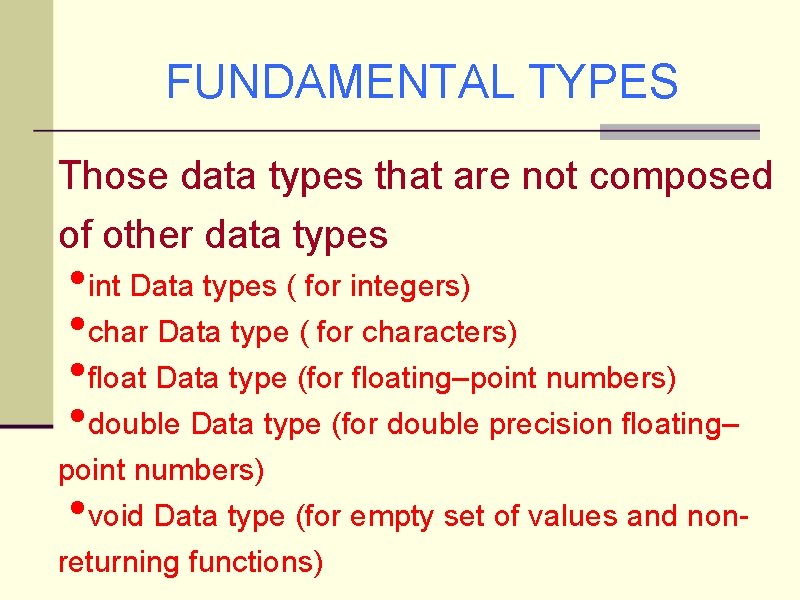
FUNDAMENTAL TYPES Those data types that are not composed of other data types • int Data types ( for integers) • char Data type ( for characters) • float Data type (for floating–point numbers) • double Data type (for double precision floating– point numbers) void Data type (for empty set of values and nonreturning functions) •
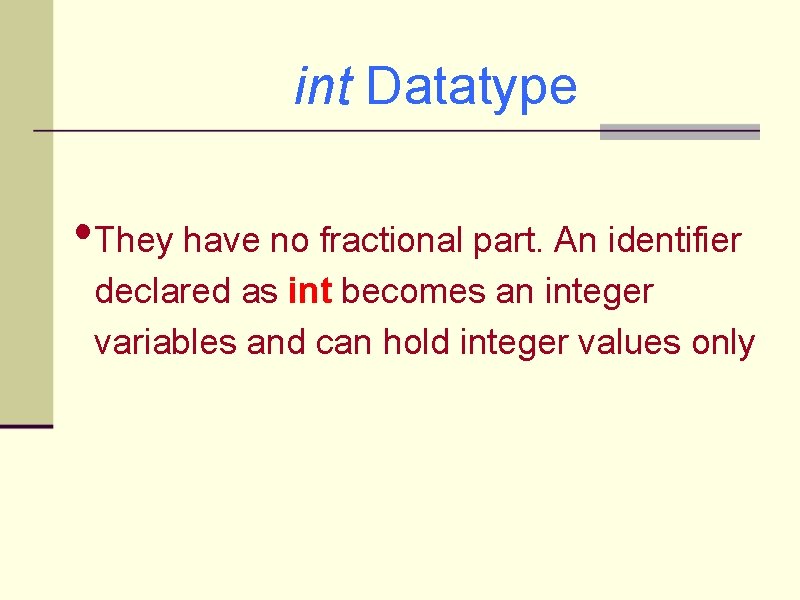
int Datatype • They have no fractional part. An identifier declared as int becomes an integer variables and can hold integer values only
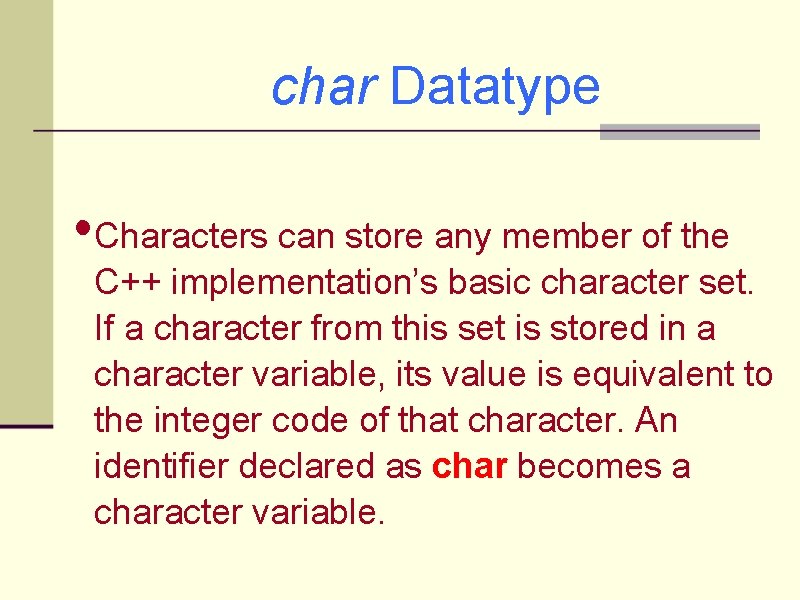
char Datatype • Characters can store any member of the C++ implementation’s basic character set. If a character from this set is stored in a character variable, its value is equivalent to the integer code of that character. An identifier declared as char becomes a character variable.
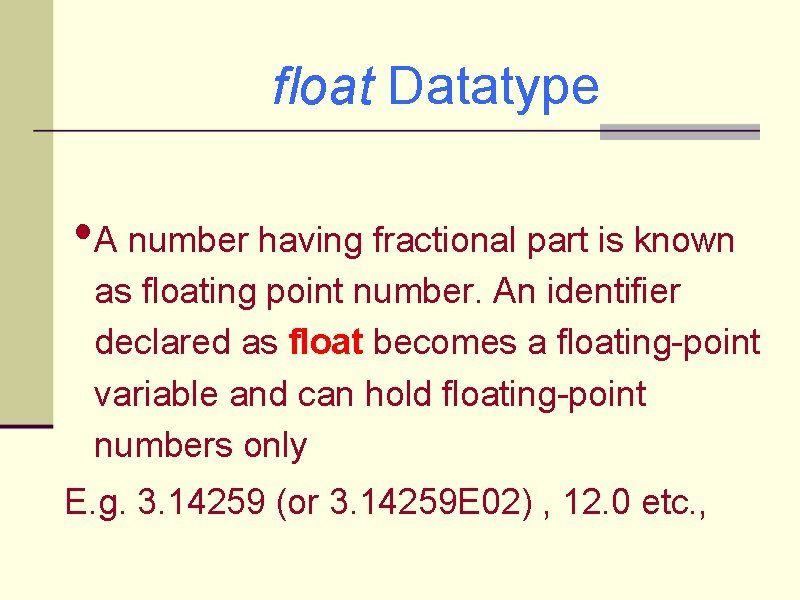
float Datatype • A number having fractional part is known as floating point number. An identifier declared as float becomes a floating-point variable and can hold floating-point numbers only E. g. 3. 14259 (or 3. 14259 E 02) , 12. 0 etc. ,
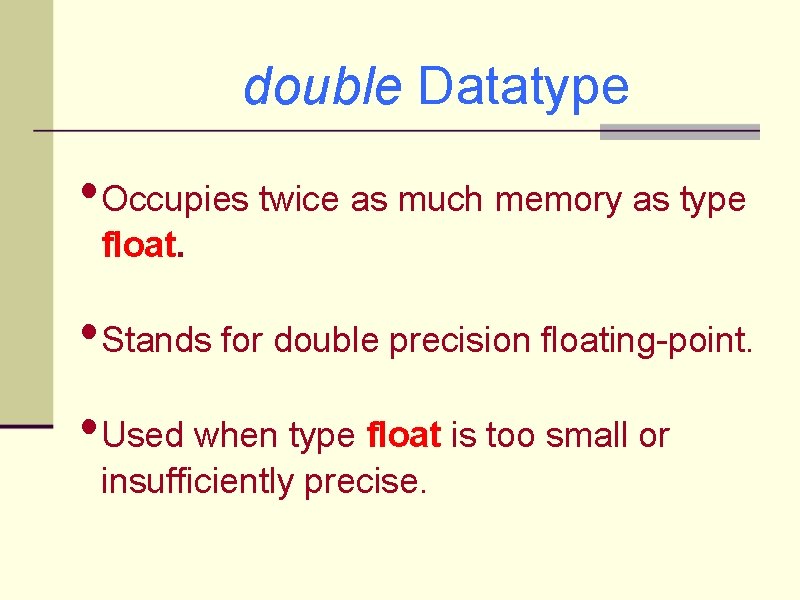
double Datatype • Occupies twice as much memory as type float. • Stands for double precision floating-point. • Used when type float is too small or insufficiently precise.
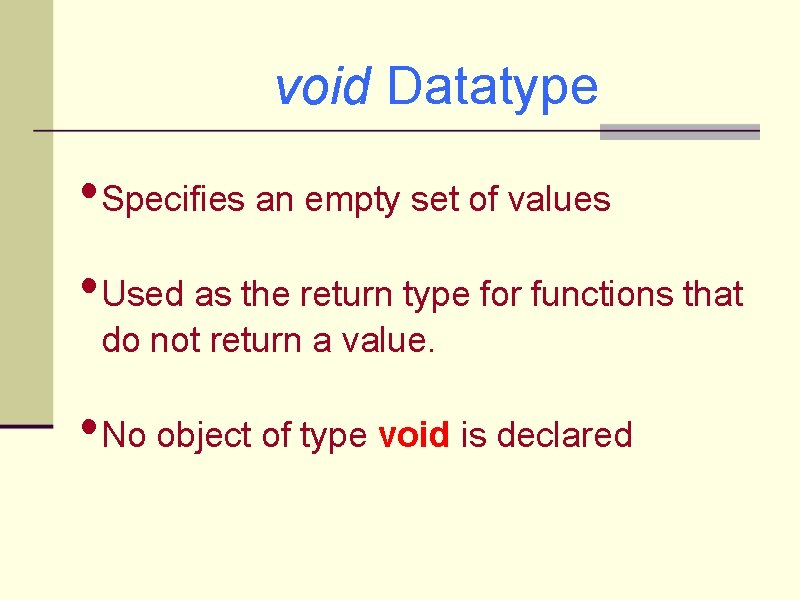
void Datatype • Specifies an empty set of values • Used as the return type for functions that do not return a value. • No object of type void is declared
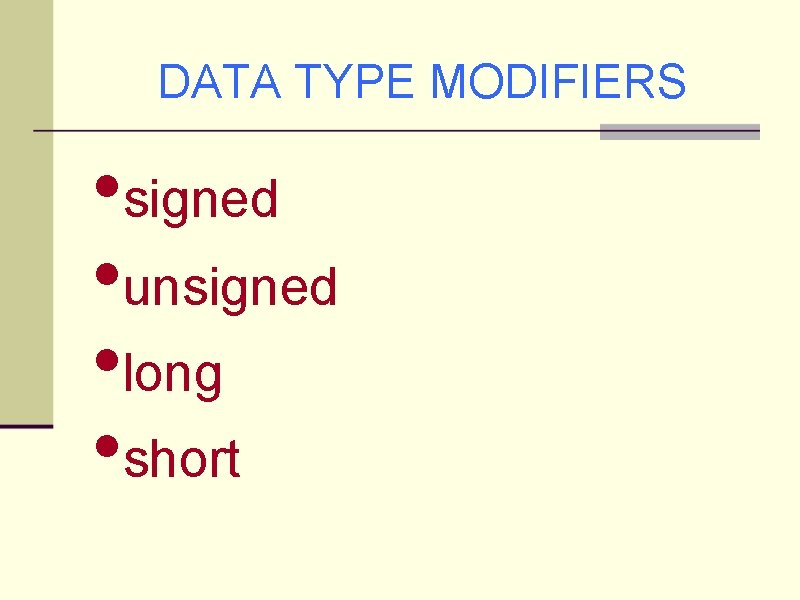
DATA TYPE MODIFIERS • signed • unsigned • long • short
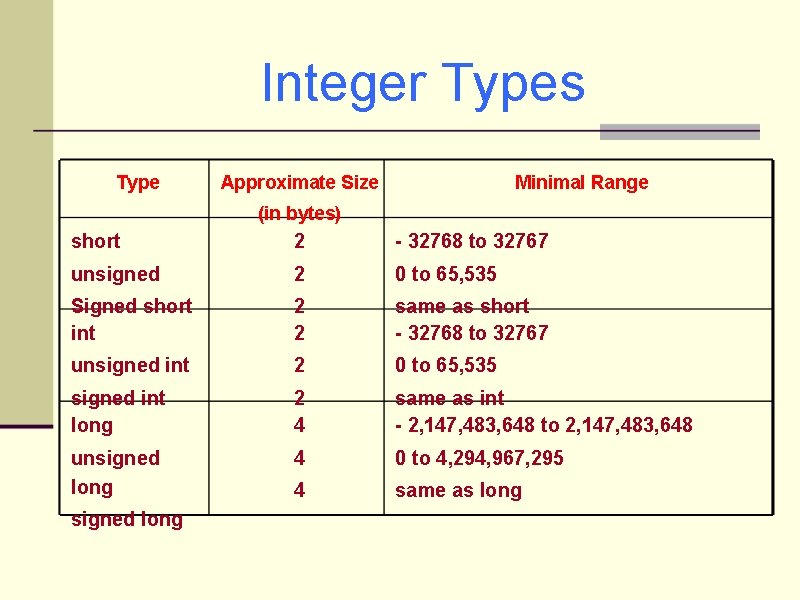
Integer Types Type Approximate Size Minimal Range (in bytes) short 2 - 32768 to 32767 unsigned 2 0 to 65, 535 Signed short int 2 2 same as short - 32768 to 32767 unsigned int 2 0 to 65, 535 signed int long 2 4 same as int - 2, 147, 483, 648 to 2, 147, 483, 648 unsigned long 4 0 to 4, 294, 967, 295 4 same as long signed long
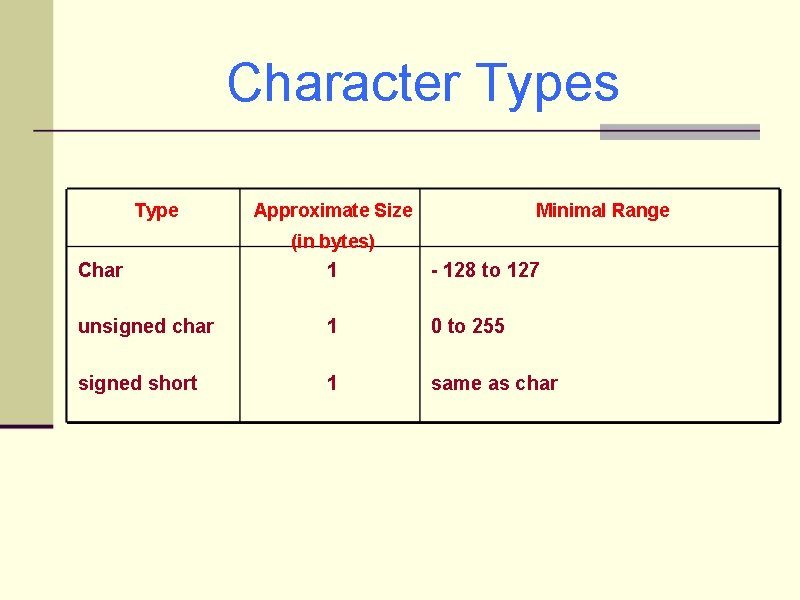
Character Types Type Approximate Size Minimal Range (in bytes) Char 1 - 128 to 127 unsigned char 1 0 to 255 signed short 1 same as char
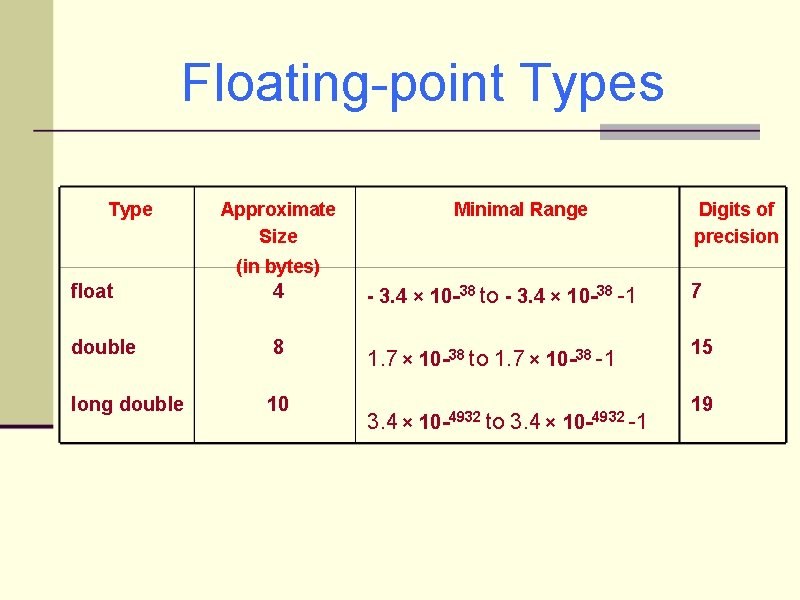
Floating-point Types Type Approximate Size Minimal Range Digits of precision (in bytes) float 4 double 8 long double 10 - 3. 4 × 10 -38 to - 3. 4 × 10 -38 -1 1. 7 × 10 -38 to 1. 7 × 10 -38 -1 3. 4 × 10 -4932 to 3. 4 × 10 -4932 -1 7 15 19
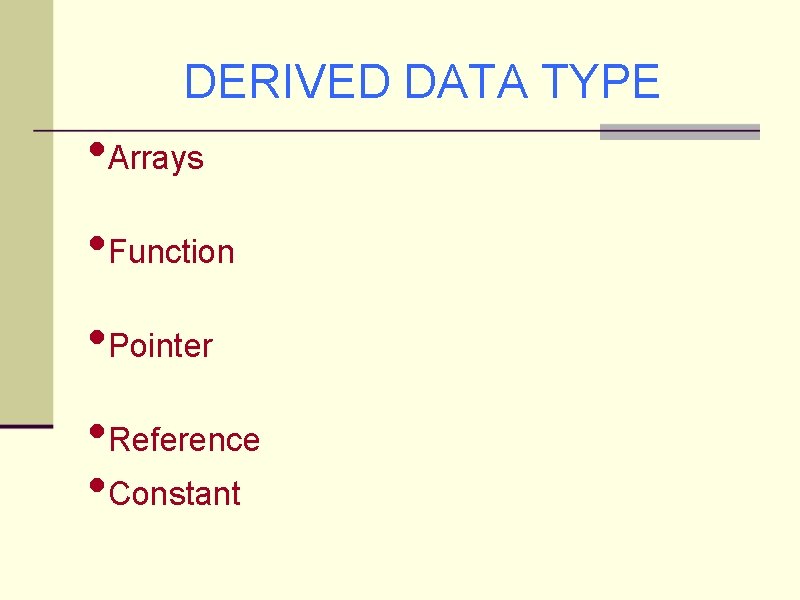
DERIVED DATA TYPE • Arrays • Function • Pointer • Reference • Constant
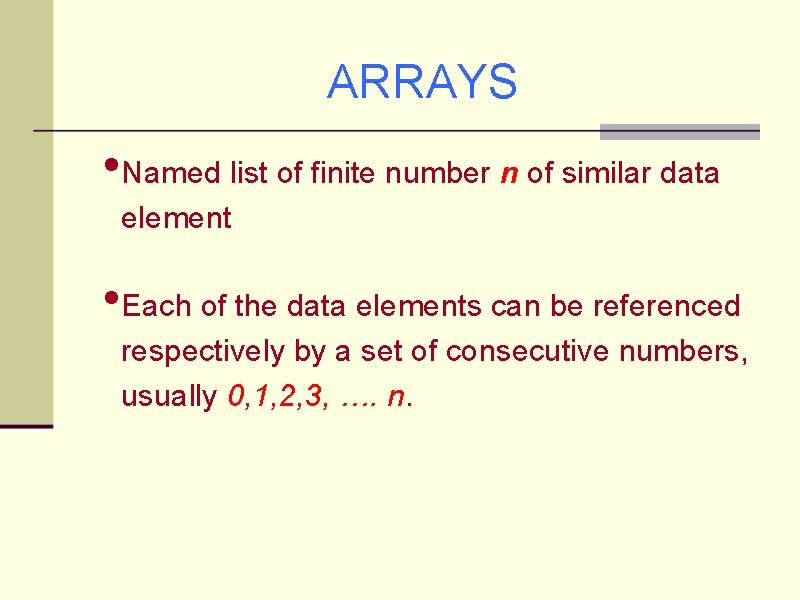
ARRAYS • Named list of finite number n of similar data element • Each of the data elements can be referenced respectively by a set of consecutive numbers, usually 0, 1, 2, 3, …. n.
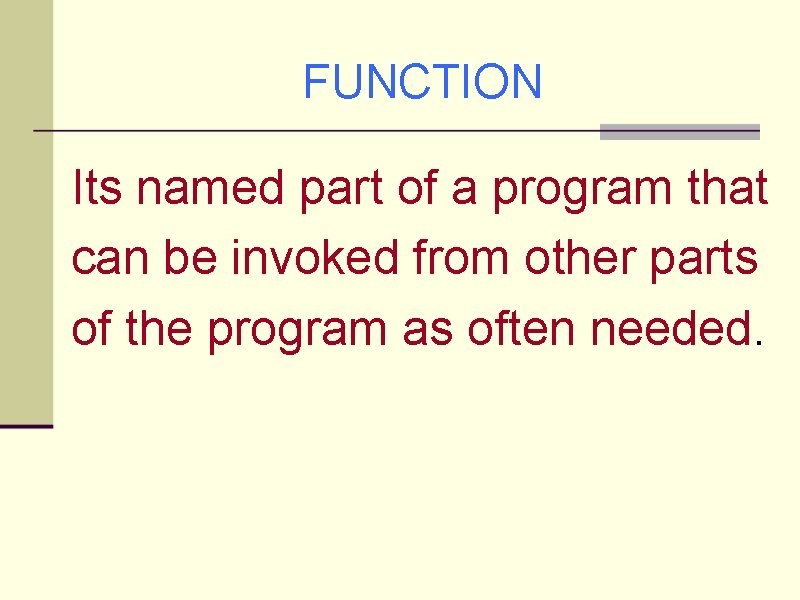
FUNCTION Its named part of a program that can be invoked from other parts of the program as often needed.
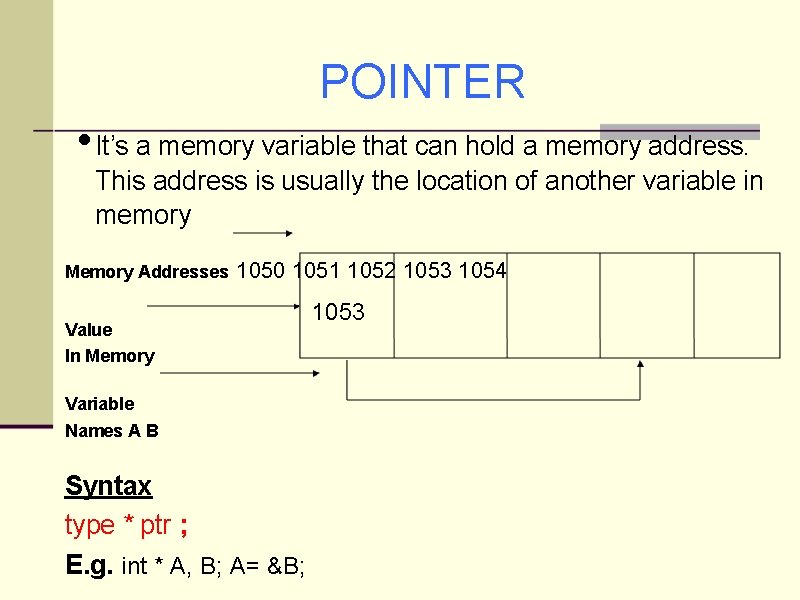
POINTER • It’s a memory variable that can hold a memory address. This address is usually the location of another variable in memory Memory Addresses 1050 1051 1052 1053 1054 Value In Memory Variable Names A B Syntax type * ptr ; E. g. int * A, B; A= &B; 1053
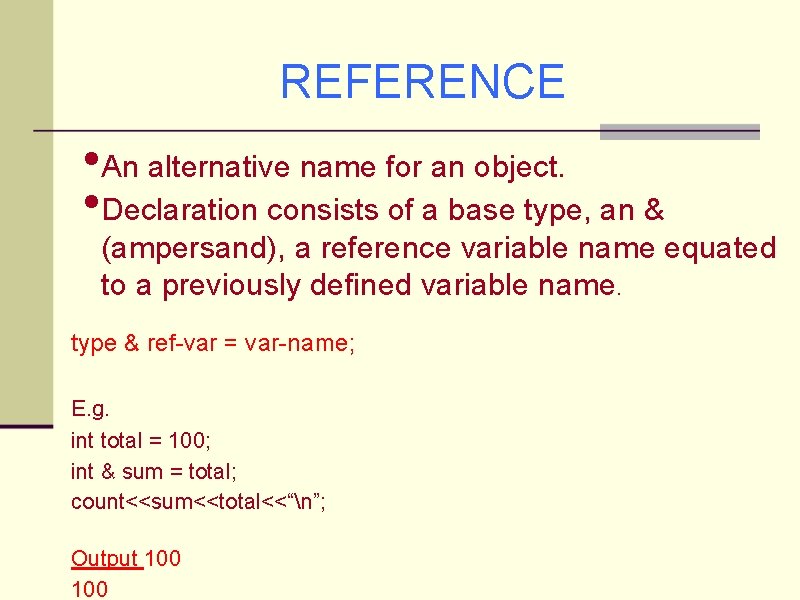
REFERENCE • An alternative name for an object. • Declaration consists of a base type, an & (ampersand), a reference variable name equated to a previously defined variable name. type & ref-var = var-name; E. g. int total = 100; int & sum = total; count<<sum<<total<<“n”; Output 100
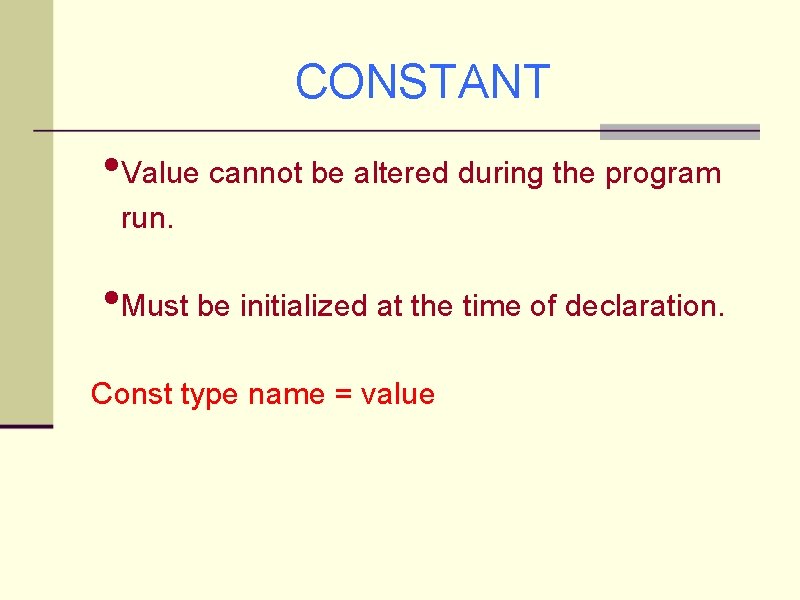
CONSTANT • Value cannot be altered during the program run. • Must be initialized at the time of declaration. Const type name = value
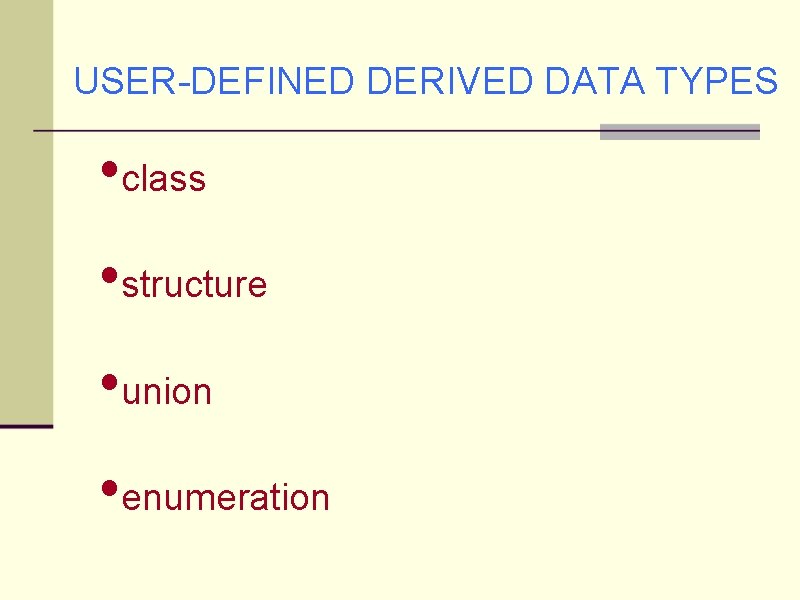
USER-DEFINED DERIVED DATA TYPES • class • structure • union • enumeration
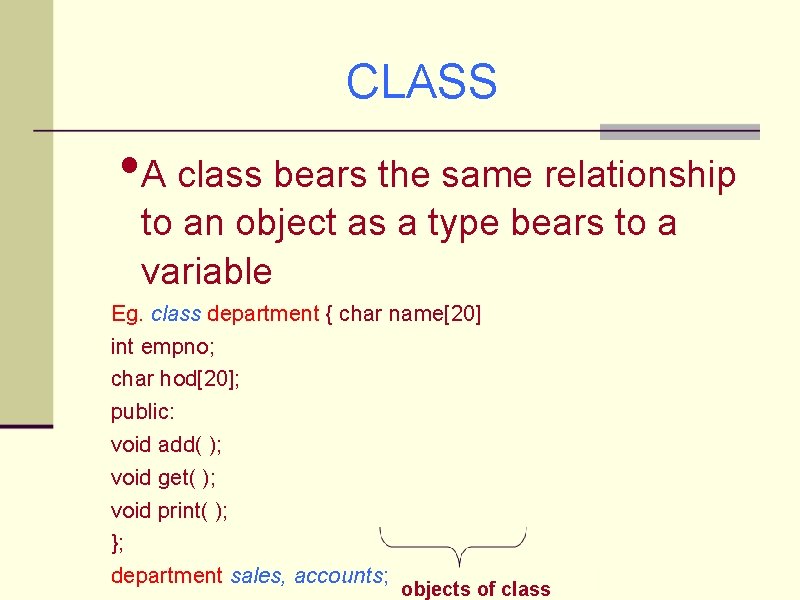
CLASS • A class bears the same relationship to an object as a type bears to a variable Eg. class department { char name[20] int empno; char hod[20]; public: void add( ); void get( ); void print( ); }; department sales, accounts; objects of class
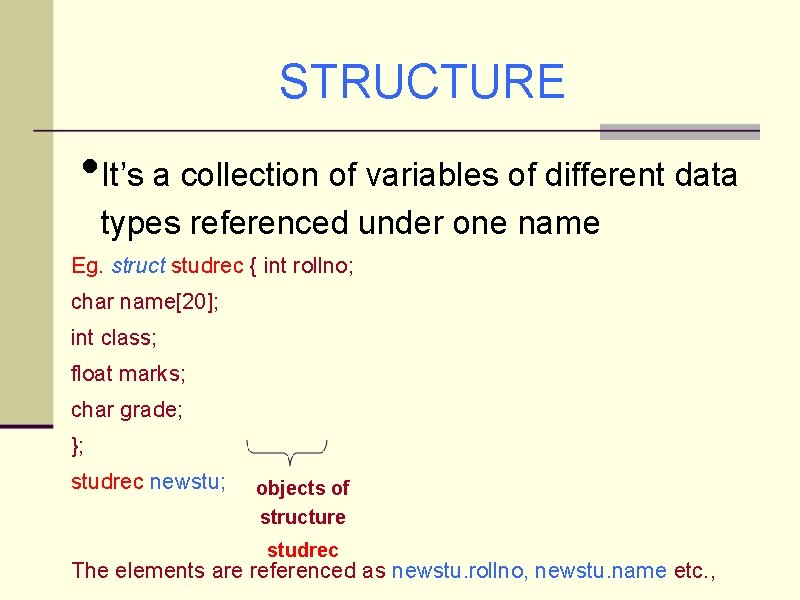
STRUCTURE • It’s a collection of variables of different data types referenced under one name Eg. struct studrec { int rollno; char name[20]; int class; float marks; char grade; }; studrec newstu; objects of structure studrec The elements are referenced as newstu. rollno, newstu. name etc. ,
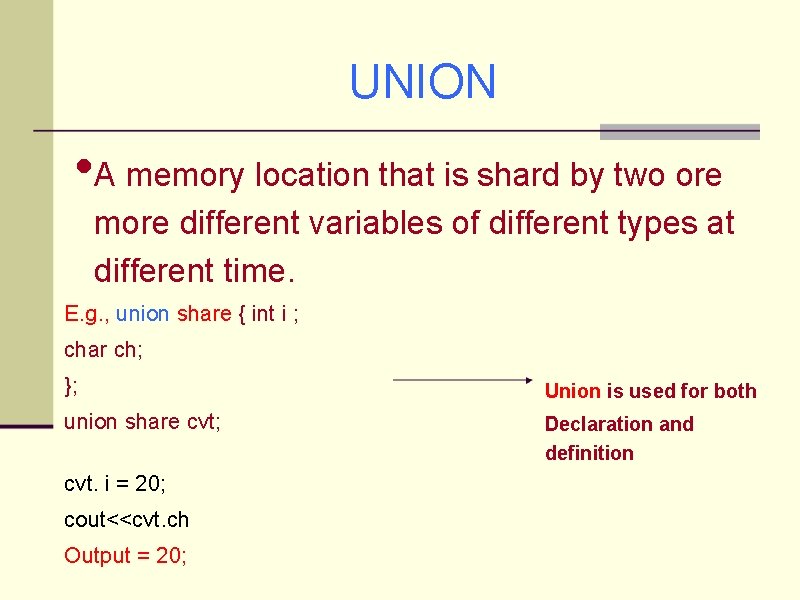
UNION • A memory location that is shard by two ore more different variables of different types at different time. E. g. , union share { int i ; char ch; }; Union is used for both union share cvt; Declaration and definition cvt. i = 20; cout<<cvt. ch Output = 20;
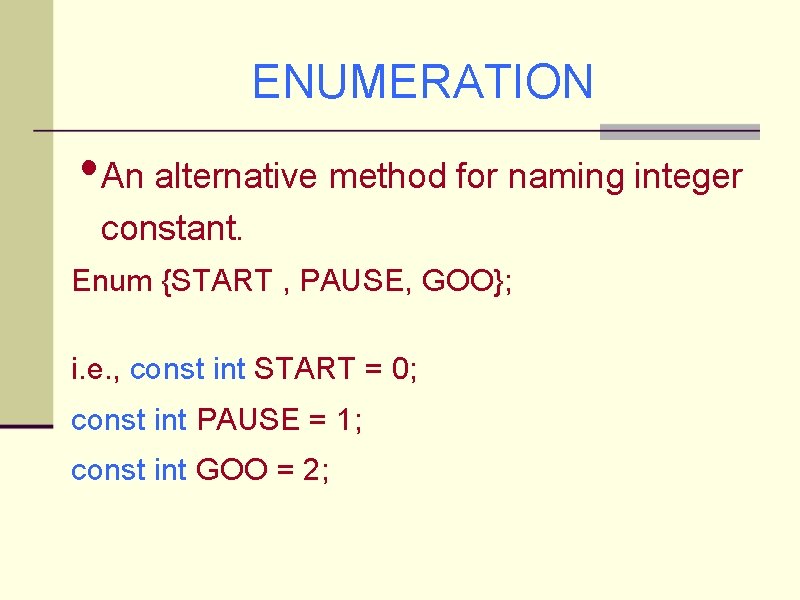
ENUMERATION • An alternative method for naming integer constant. Enum {START , PAUSE, GOO}; i. e. , const int START = 0; const int PAUSE = 1; const int GOO = 2;
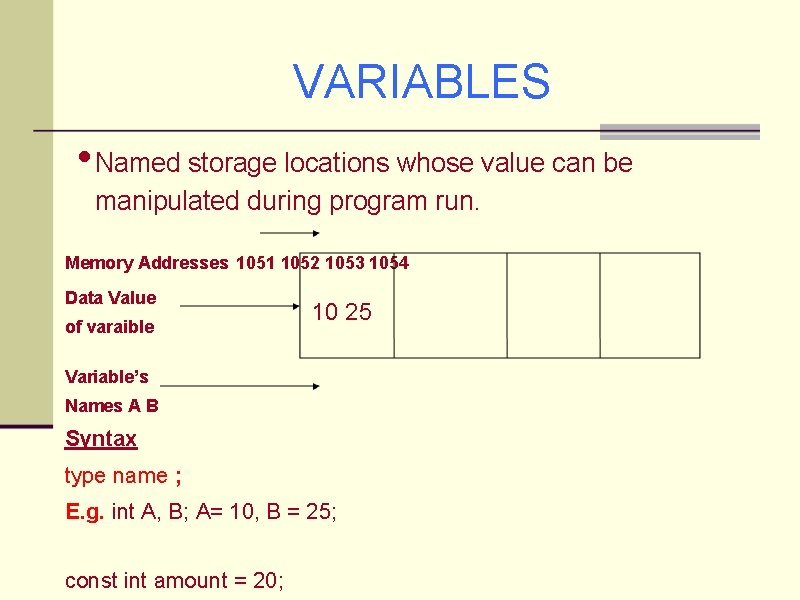
VARIABLES • Named storage locations whose value can be manipulated during program run. Memory Addresses 1051 1052 1053 1054 Data Value of varaible 10 25 Variable’s Names A B Syntax type name ; E. g. int A, B; A= 10, B = 25; const int amount = 20;
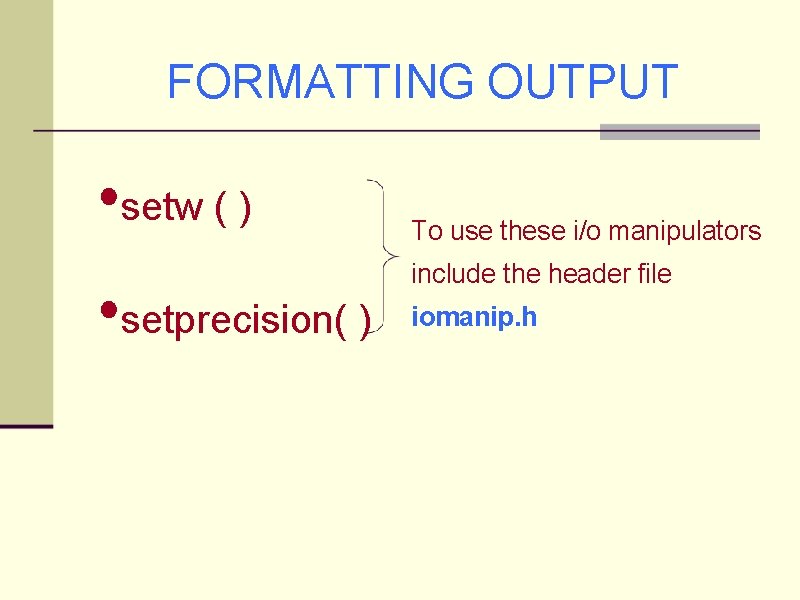
FORMATTING OUTPUT • setw ( ) • setprecision( ) To use these i/o manipulators include the header file iomanip. h
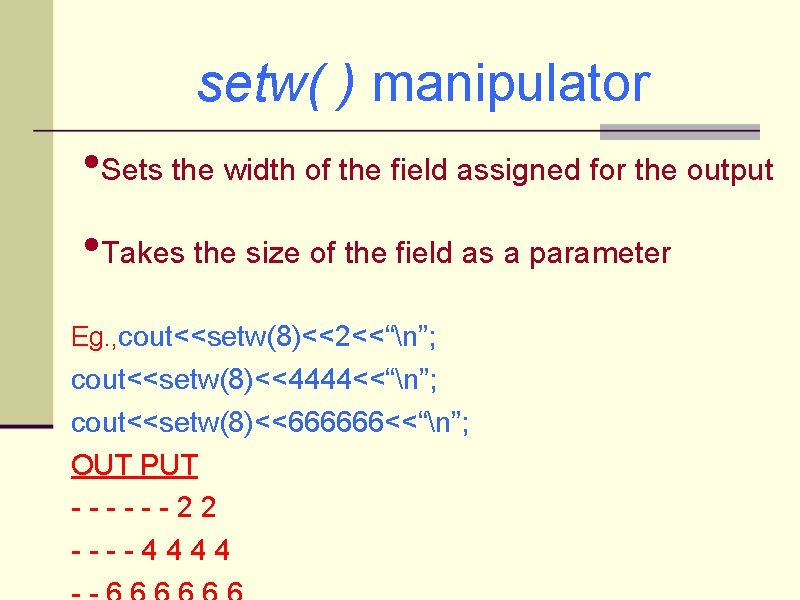
setw( ) manipulator • Sets the width of the field assigned for the output • Takes the size of the field as a parameter Eg. , cout<<setw(8)<<2<<“n”; cout<<setw(8)<<4444<<“n”; cout<<setw(8)<<666666<<“n”; OUT PUT ------22 ----4444
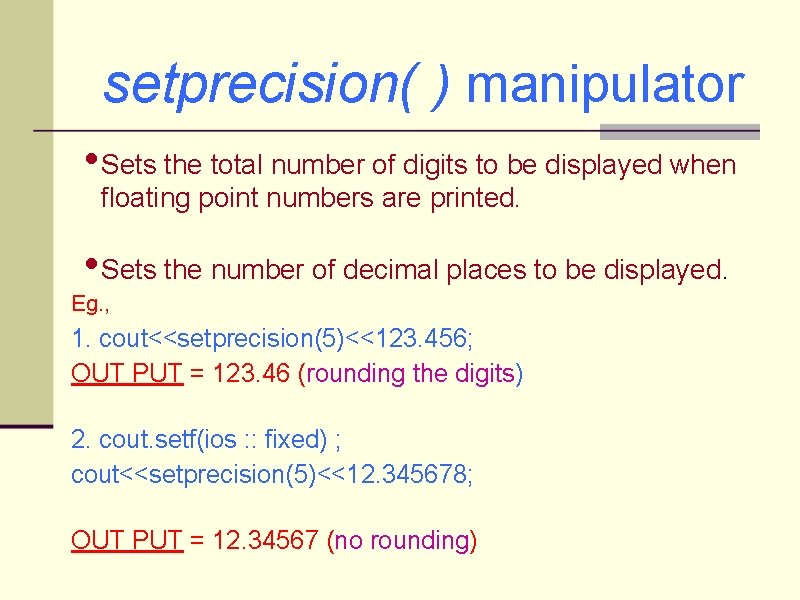
setprecision( ) manipulator • Sets the total number of digits to be displayed when floating point numbers are printed. • Sets the number of decimal places to be displayed. Eg. , 1. cout<<setprecision(5)<<123. 456; OUT PUT = 123. 46 (rounding the digits) 2. cout. setf(ios : : fixed) ; cout<<setprecision(5)<<12. 345678; OUT PUT = 12. 34567 (no rounding)
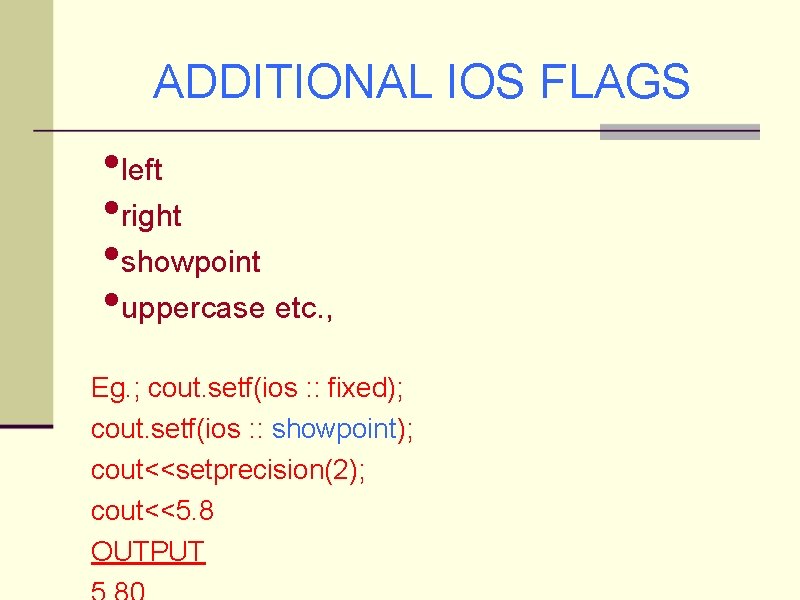
ADDITIONAL IOS FLAGS • left • right • showpoint • uppercase etc. , Eg. ; cout. setf(ios : : fixed); cout. setf(ios : : showpoint); cout<<setprecision(2); cout<<5. 8 OUTPUT
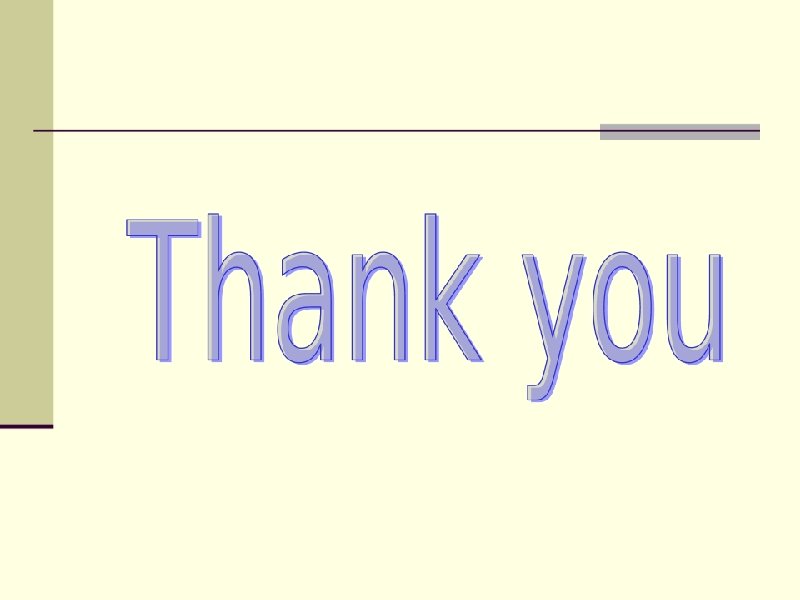