Data Chapter 6 and 7 Data Persistence Persisting
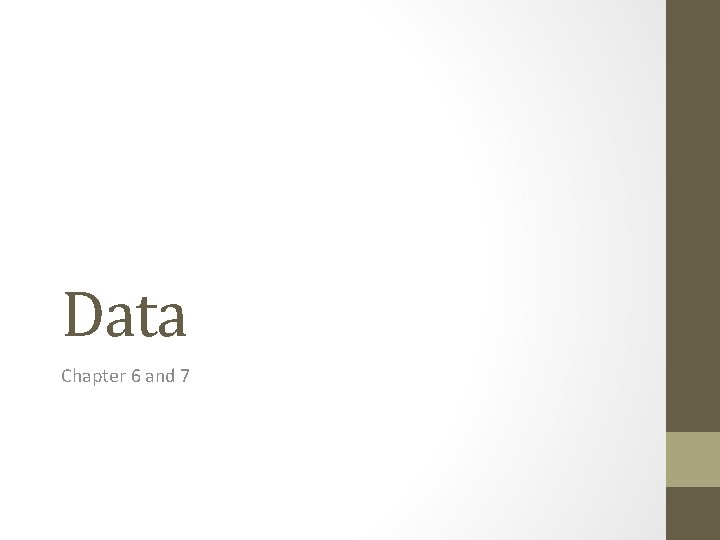
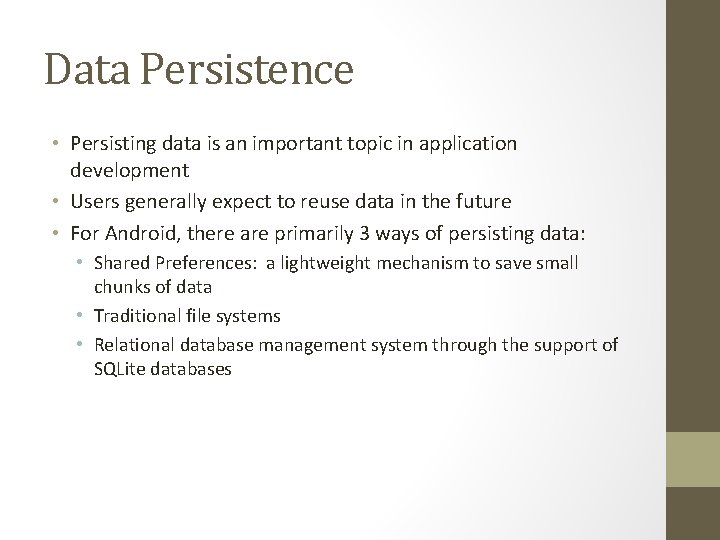
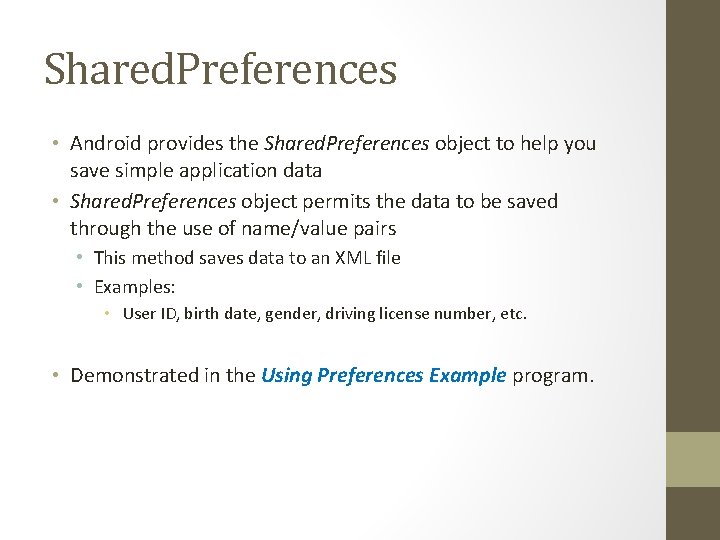
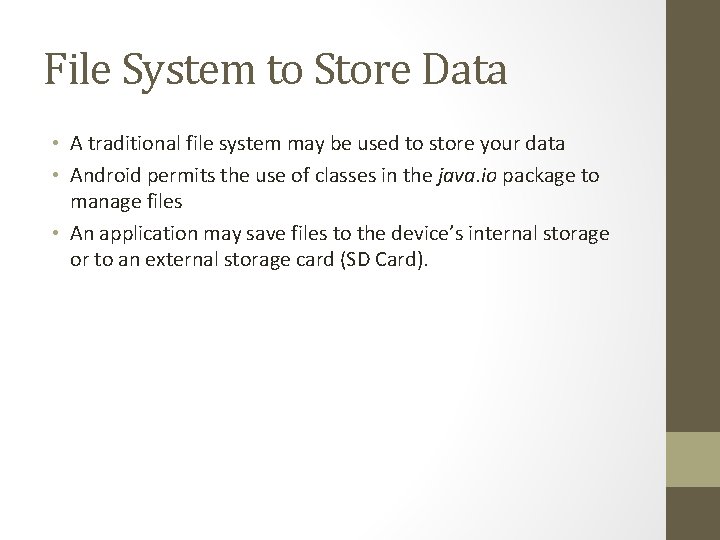
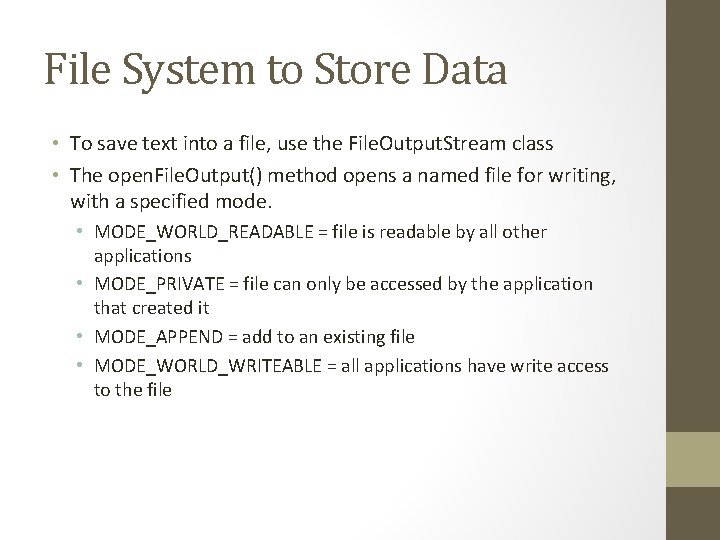
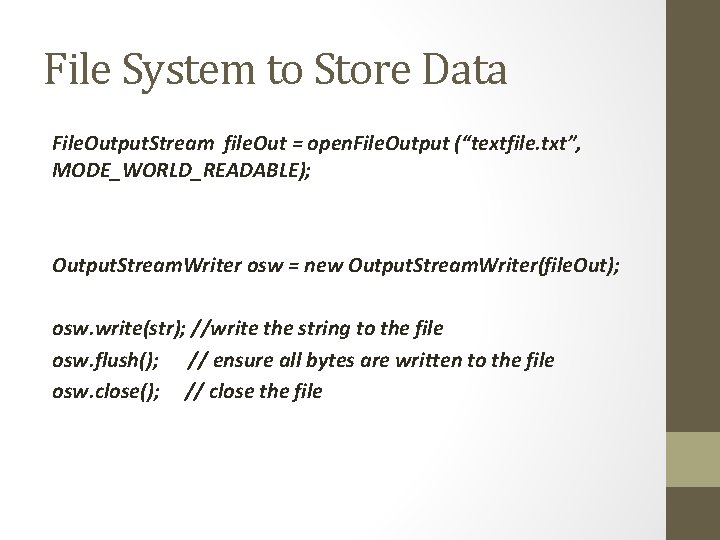
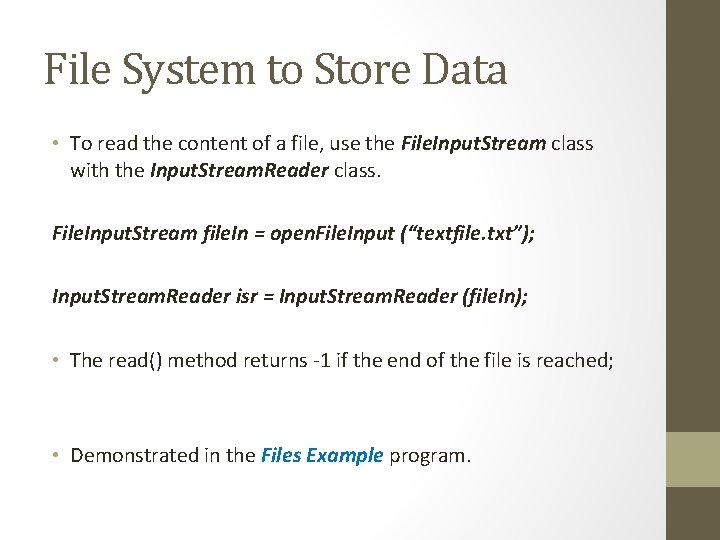
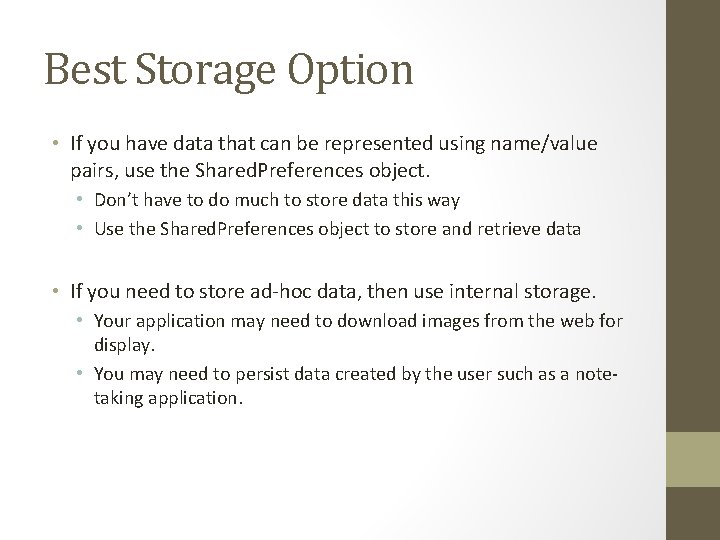
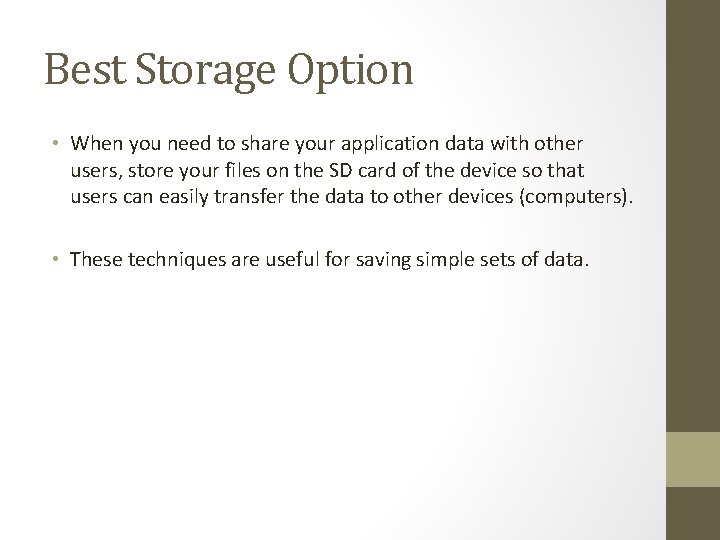
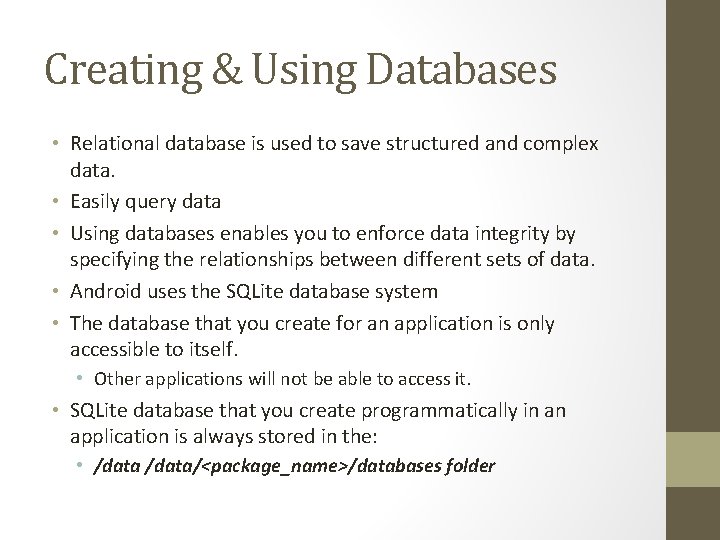
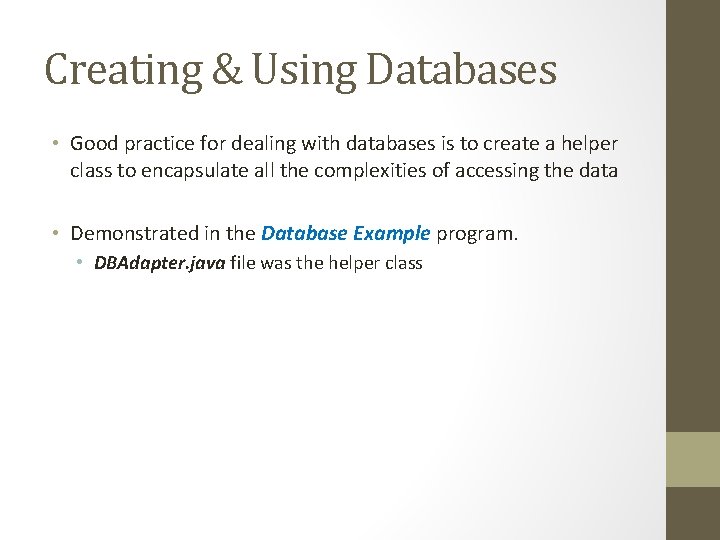
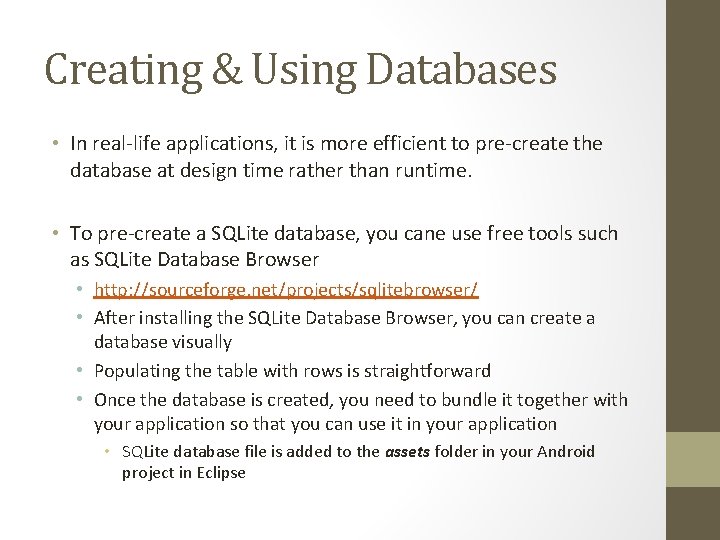
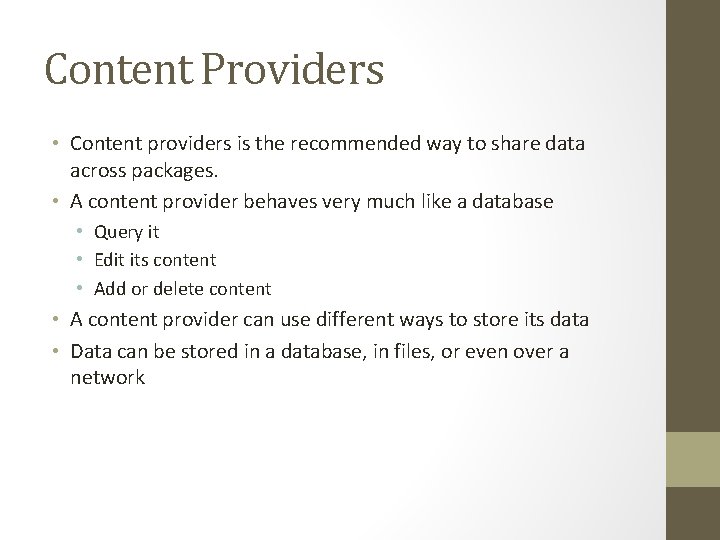
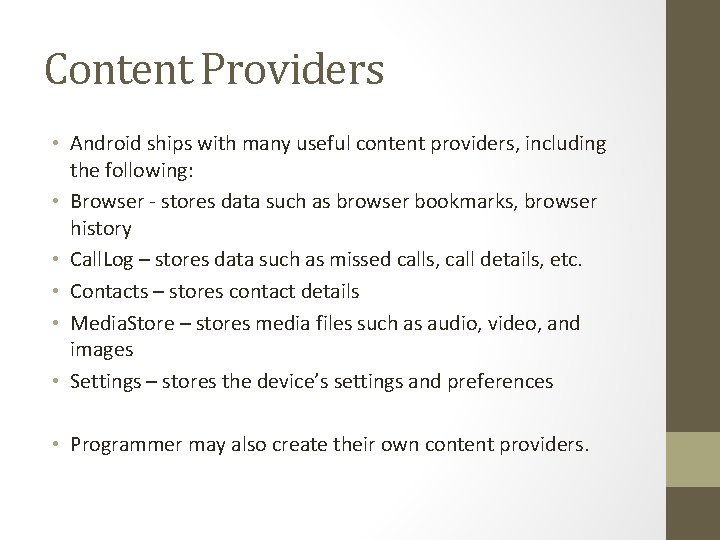
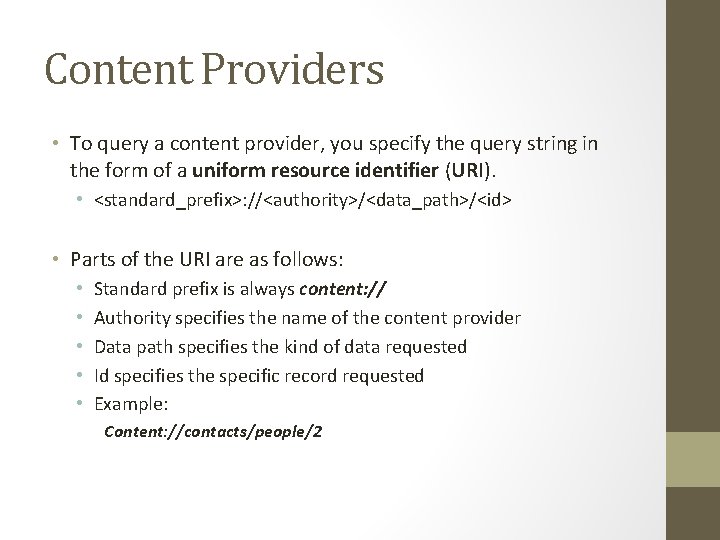
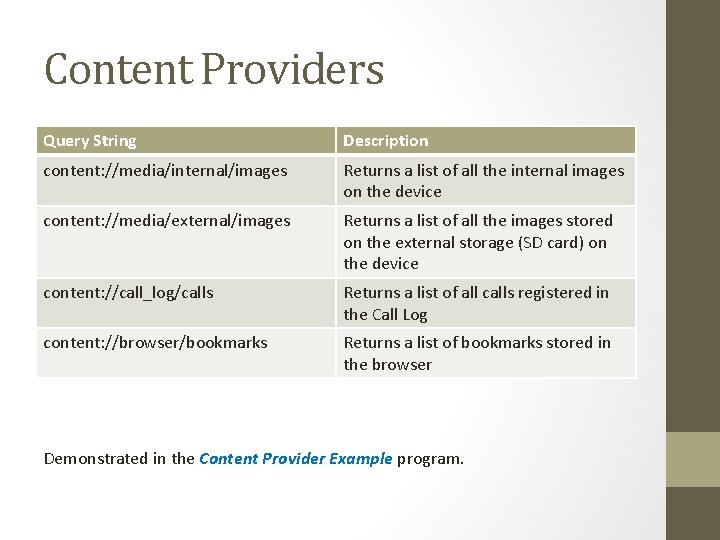
- Slides: 16
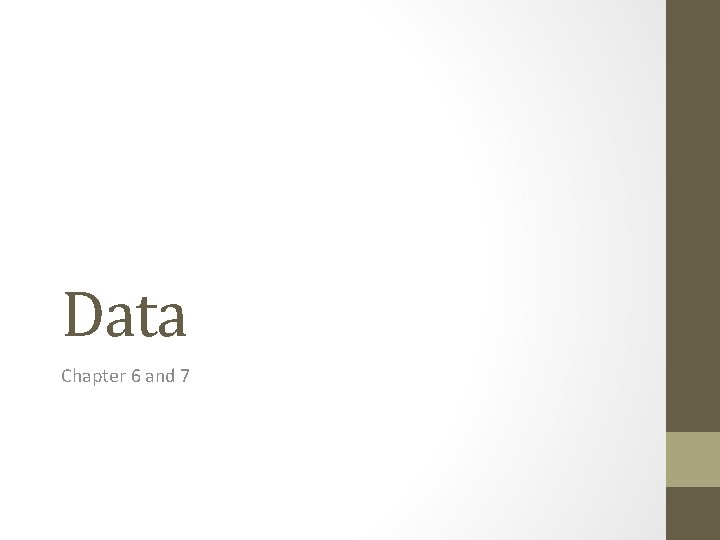
Data Chapter 6 and 7
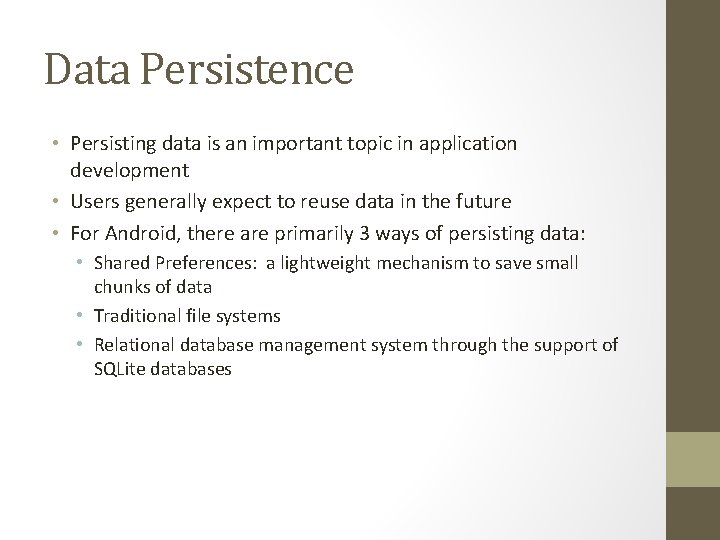
Data Persistence • Persisting data is an important topic in application development • Users generally expect to reuse data in the future • For Android, there are primarily 3 ways of persisting data: • Shared Preferences: a lightweight mechanism to save small chunks of data • Traditional file systems • Relational database management system through the support of SQLite databases
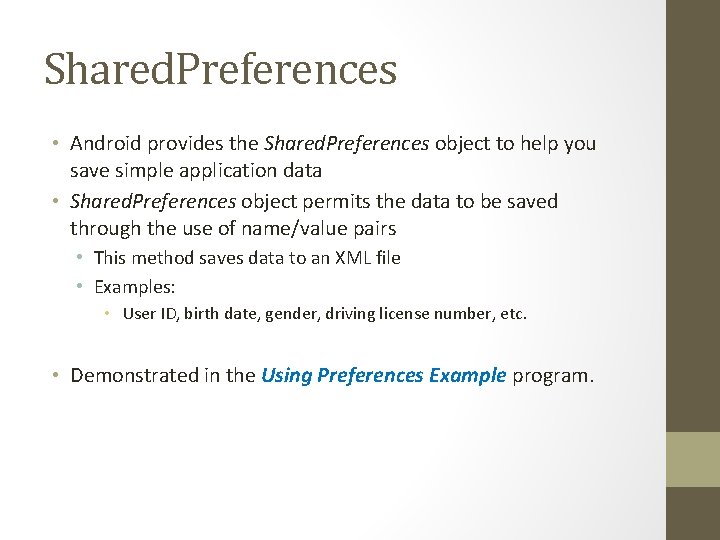
Shared. Preferences • Android provides the Shared. Preferences object to help you save simple application data • Shared. Preferences object permits the data to be saved through the use of name/value pairs • This method saves data to an XML file • Examples: • User ID, birth date, gender, driving license number, etc. • Demonstrated in the Using Preferences Example program.
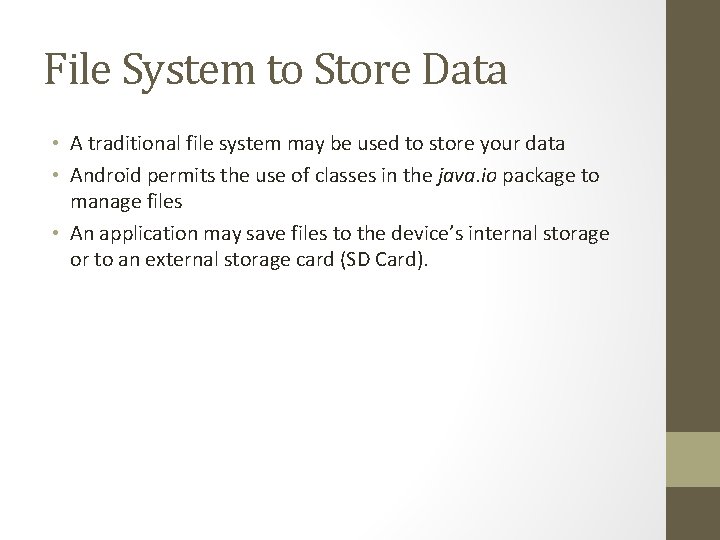
File System to Store Data • A traditional file system may be used to store your data • Android permits the use of classes in the java. io package to manage files • An application may save files to the device’s internal storage or to an external storage card (SD Card).
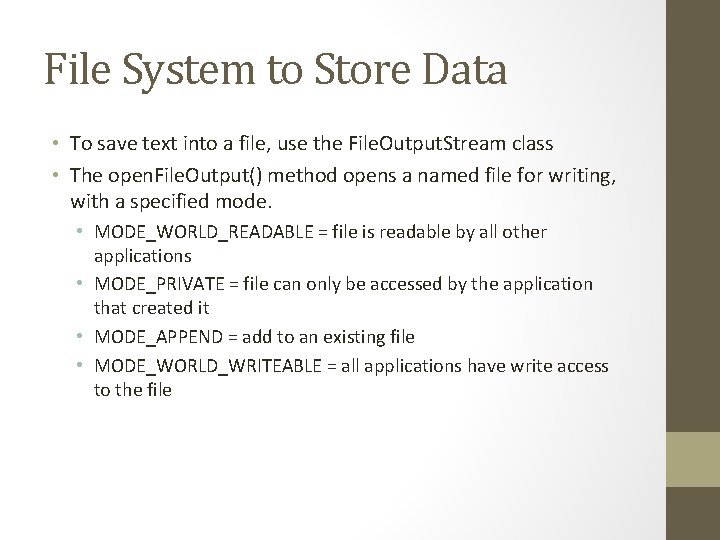
File System to Store Data • To save text into a file, use the File. Output. Stream class • The open. File. Output() method opens a named file for writing, with a specified mode. • MODE_WORLD_READABLE = file is readable by all other applications • MODE_PRIVATE = file can only be accessed by the application that created it • MODE_APPEND = add to an existing file • MODE_WORLD_WRITEABLE = all applications have write access to the file
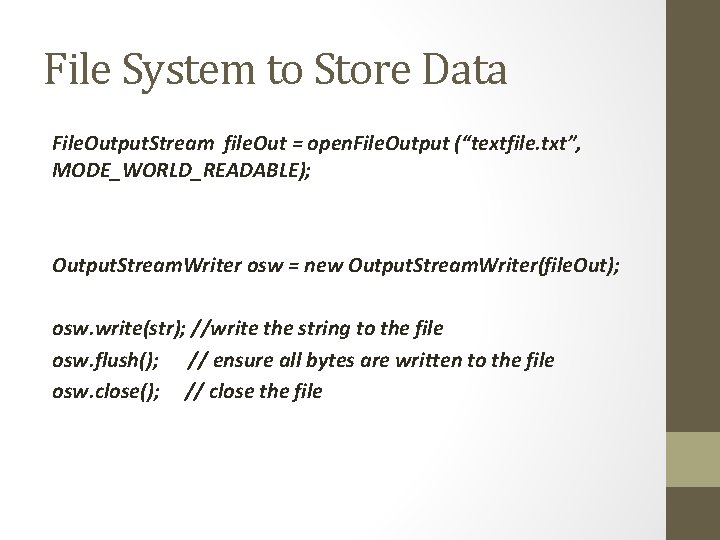
File System to Store Data File. Output. Stream file. Out = open. File. Output (“textfile. txt”, MODE_WORLD_READABLE); Output. Stream. Writer osw = new Output. Stream. Writer(file. Out); osw. write(str); //write the string to the file osw. flush(); // ensure all bytes are written to the file osw. close(); // close the file
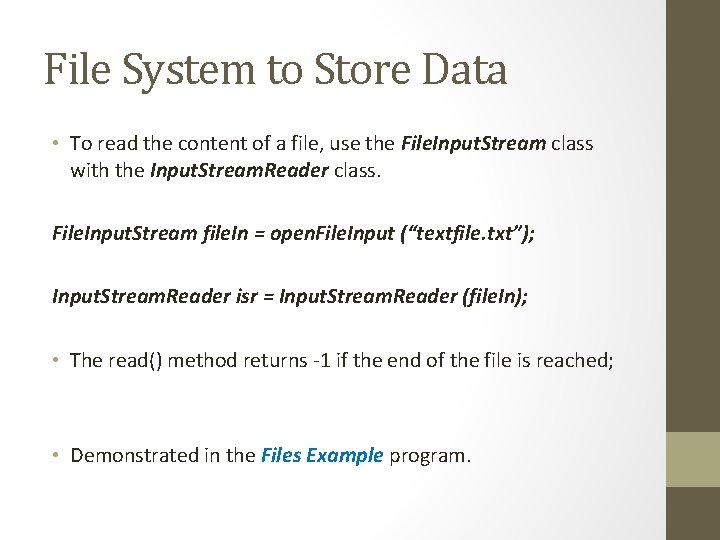
File System to Store Data • To read the content of a file, use the File. Input. Stream class with the Input. Stream. Reader class. File. Input. Stream file. In = open. File. Input (“textfile. txt”); Input. Stream. Reader isr = Input. Stream. Reader (file. In); • The read() method returns -1 if the end of the file is reached; • Demonstrated in the Files Example program.
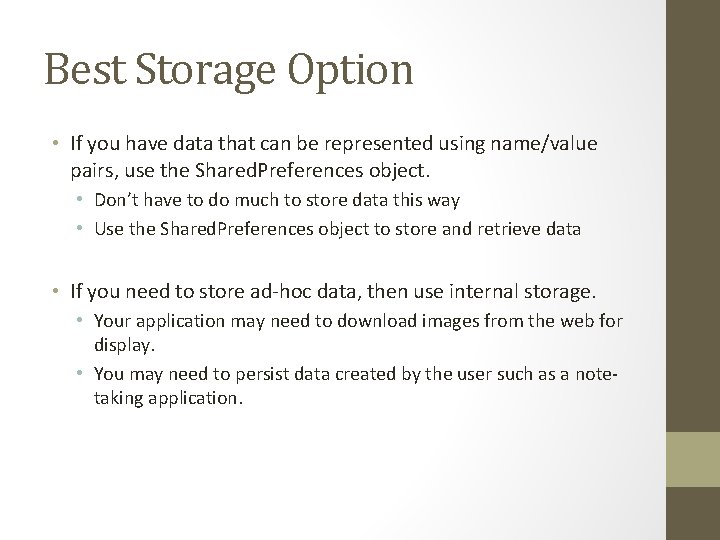
Best Storage Option • If you have data that can be represented using name/value pairs, use the Shared. Preferences object. • Don’t have to do much to store data this way • Use the Shared. Preferences object to store and retrieve data • If you need to store ad-hoc data, then use internal storage. • Your application may need to download images from the web for display. • You may need to persist data created by the user such as a notetaking application.
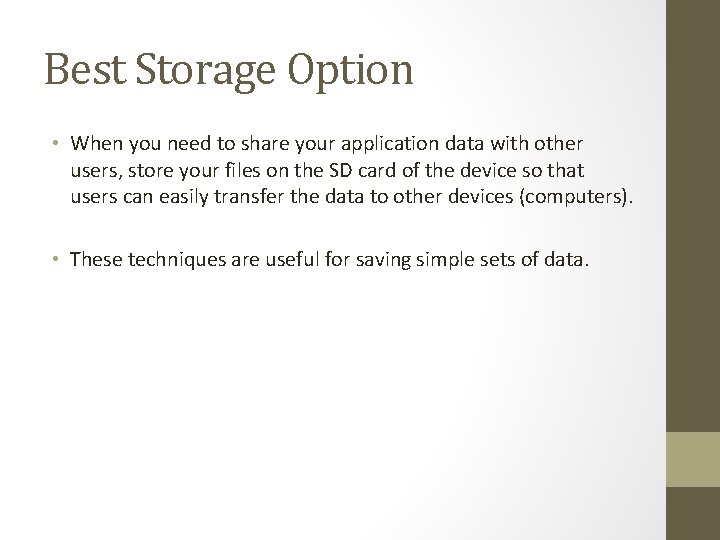
Best Storage Option • When you need to share your application data with other users, store your files on the SD card of the device so that users can easily transfer the data to other devices (computers). • These techniques are useful for saving simple sets of data.
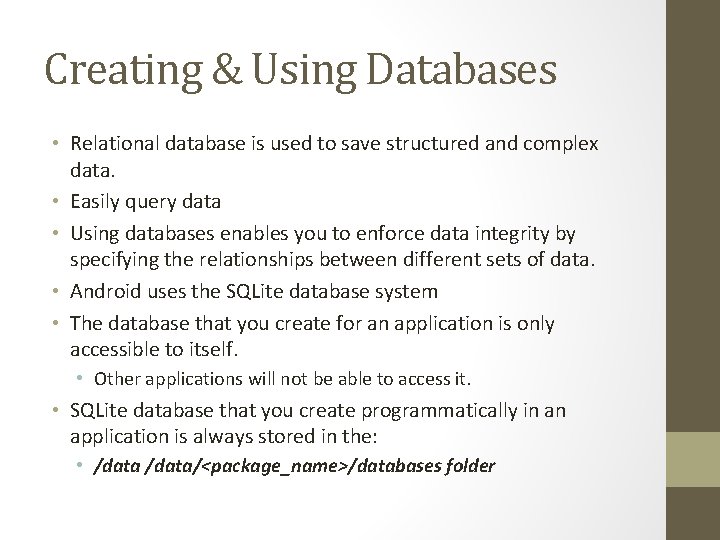
Creating & Using Databases • Relational database is used to save structured and complex data. • Easily query data • Using databases enables you to enforce data integrity by specifying the relationships between different sets of data. • Android uses the SQLite database system • The database that you create for an application is only accessible to itself. • Other applications will not be able to access it. • SQLite database that you create programmatically in an application is always stored in the: • /data/<package_name>/databases folder
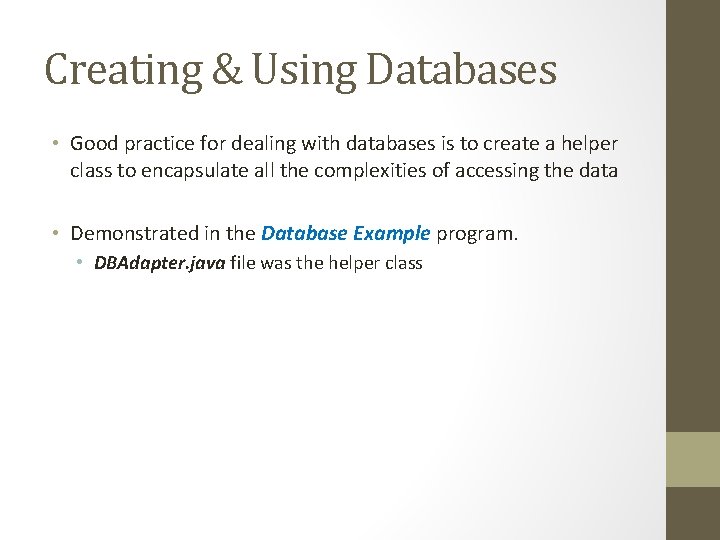
Creating & Using Databases • Good practice for dealing with databases is to create a helper class to encapsulate all the complexities of accessing the data • Demonstrated in the Database Example program. • DBAdapter. java file was the helper class
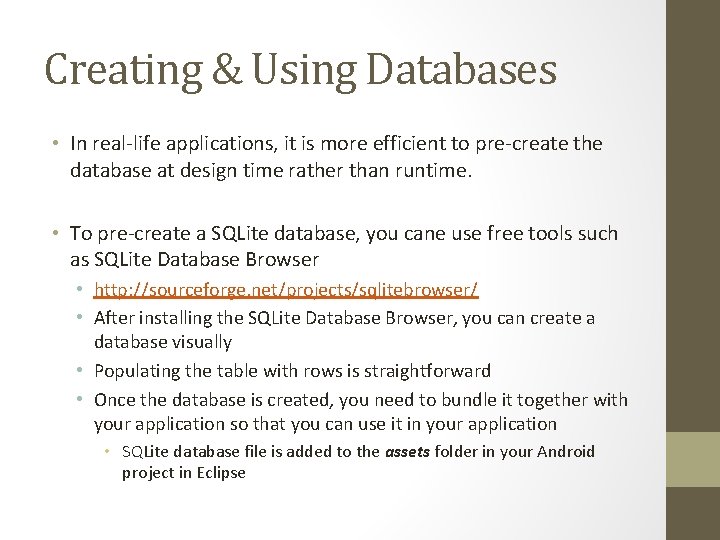
Creating & Using Databases • In real-life applications, it is more efficient to pre-create the database at design time rather than runtime. • To pre-create a SQLite database, you cane use free tools such as SQLite Database Browser • http: //sourceforge. net/projects/sqlitebrowser/ • After installing the SQLite Database Browser, you can create a database visually • Populating the table with rows is straightforward • Once the database is created, you need to bundle it together with your application so that you can use it in your application • SQLite database file is added to the assets folder in your Android project in Eclipse
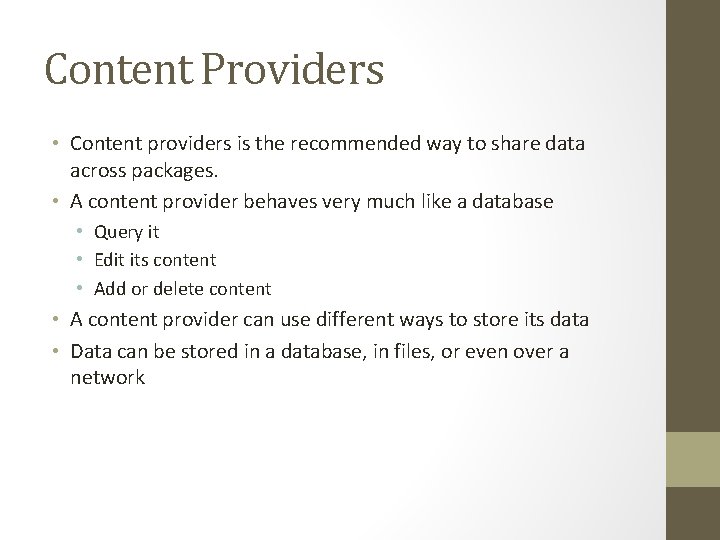
Content Providers • Content providers is the recommended way to share data across packages. • A content provider behaves very much like a database • Query it • Edit its content • Add or delete content • A content provider can use different ways to store its data • Data can be stored in a database, in files, or even over a network
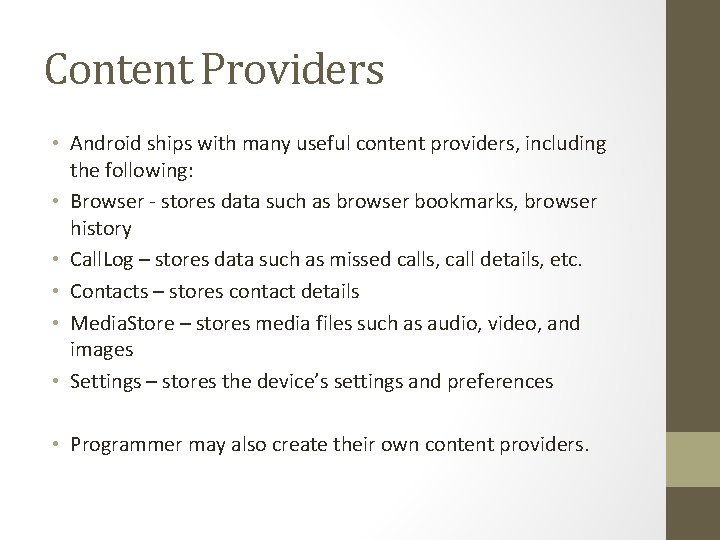
Content Providers • Android ships with many useful content providers, including the following: • Browser - stores data such as browser bookmarks, browser history • Call. Log – stores data such as missed calls, call details, etc. • Contacts – stores contact details • Media. Store – stores media files such as audio, video, and images • Settings – stores the device’s settings and preferences • Programmer may also create their own content providers.
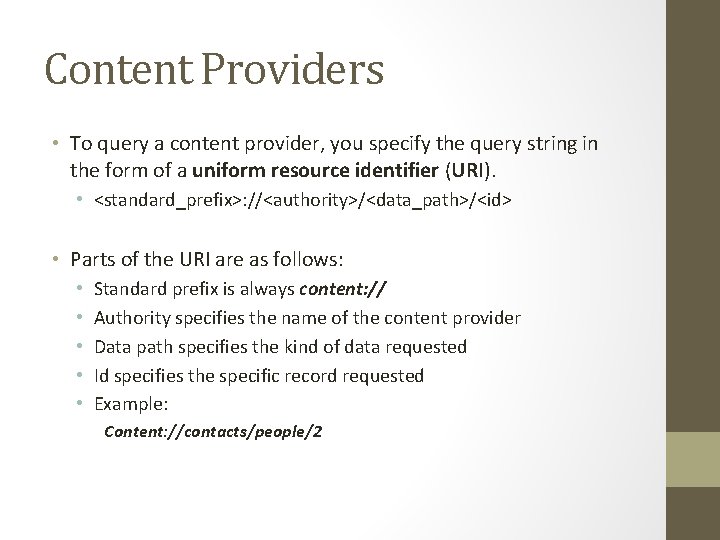
Content Providers • To query a content provider, you specify the query string in the form of a uniform resource identifier (URI). • <standard_prefix>: //<authority>/<data_path>/<id> • Parts of the URI are as follows: • • • Standard prefix is always content: // Authority specifies the name of the content provider Data path specifies the kind of data requested Id specifies the specific record requested Example: Content: //contacts/people/2
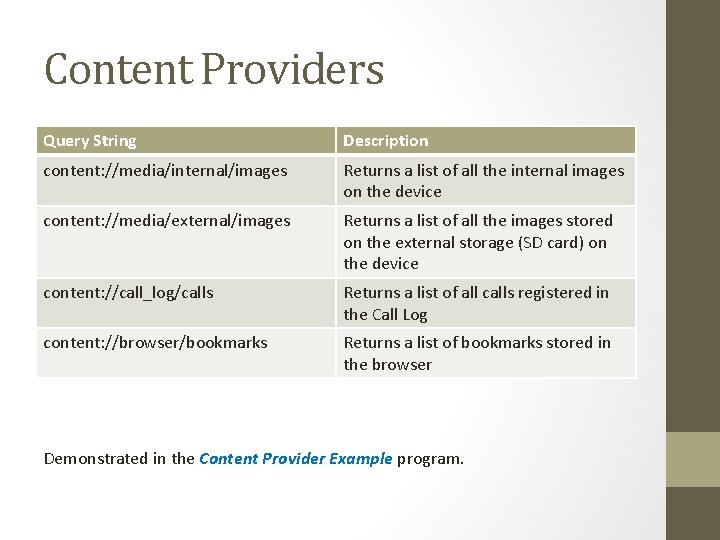
Content Providers Query String Description content: //media/internal/images Returns a list of all the internal images on the device content: //media/external/images Returns a list of all the images stored on the external storage (SD card) on the device content: //call_log/calls Returns a list of all calls registered in the Call Log content: //browser/bookmarks Returns a list of bookmarks stored in the browser Demonstrated in the Content Provider Example program.