Data Analysis with ROOT This talk extends the
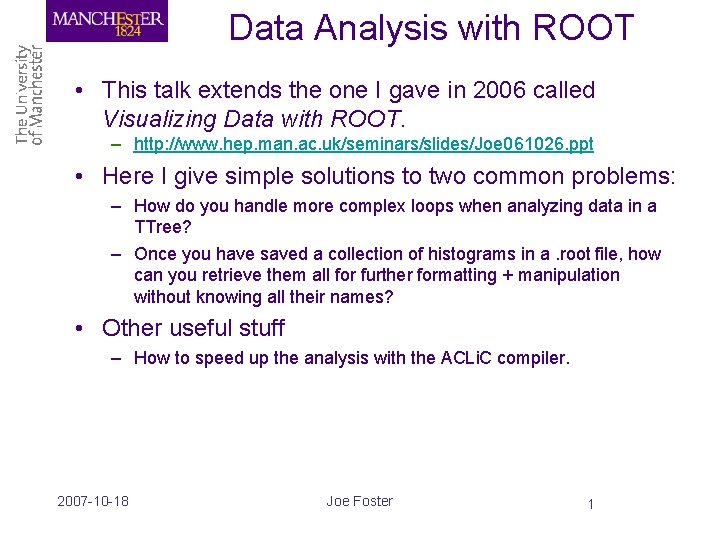
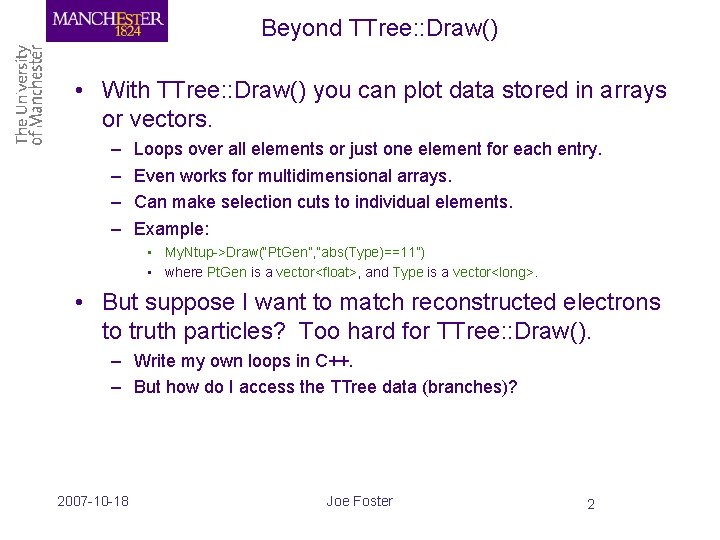
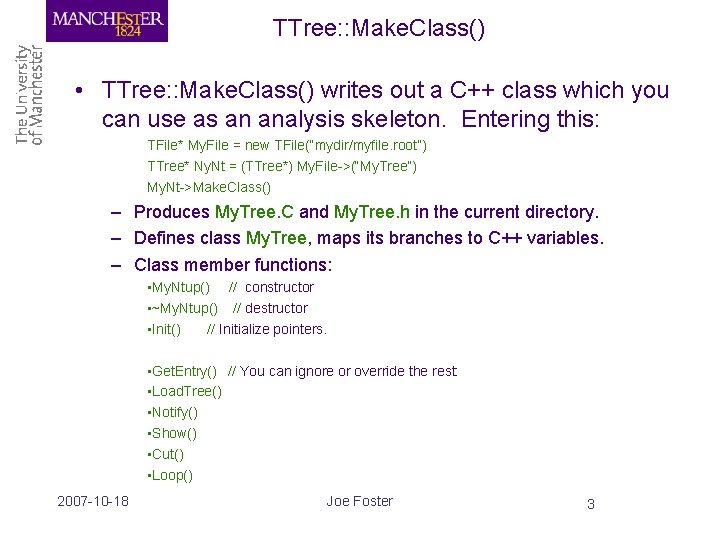
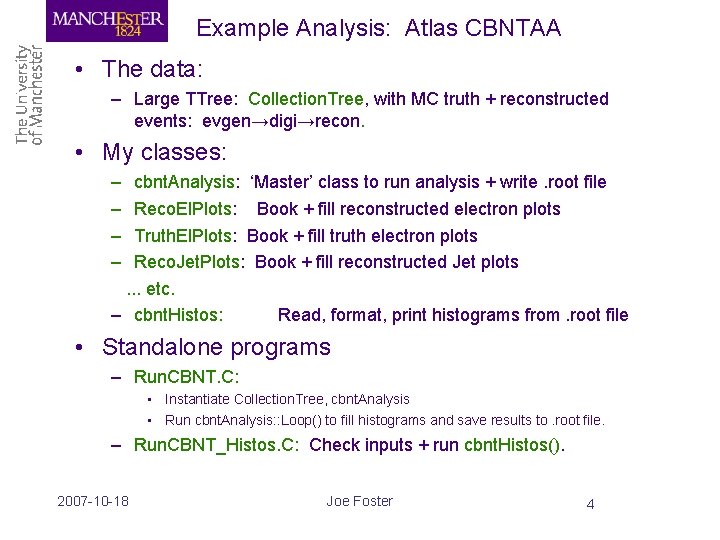
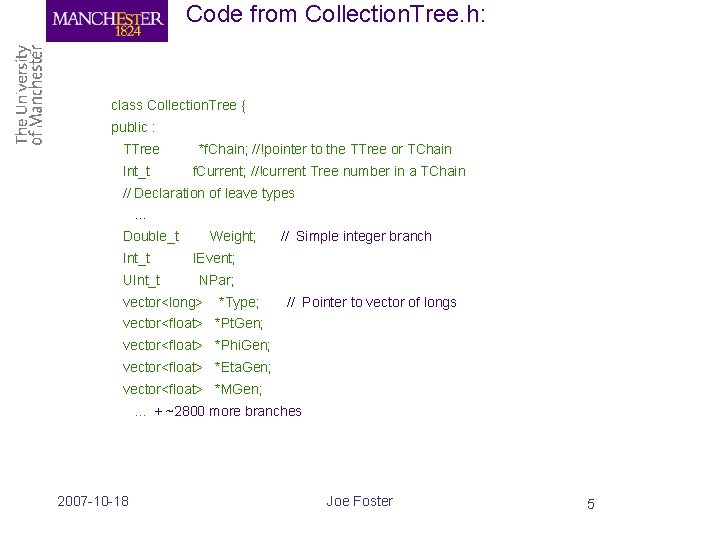
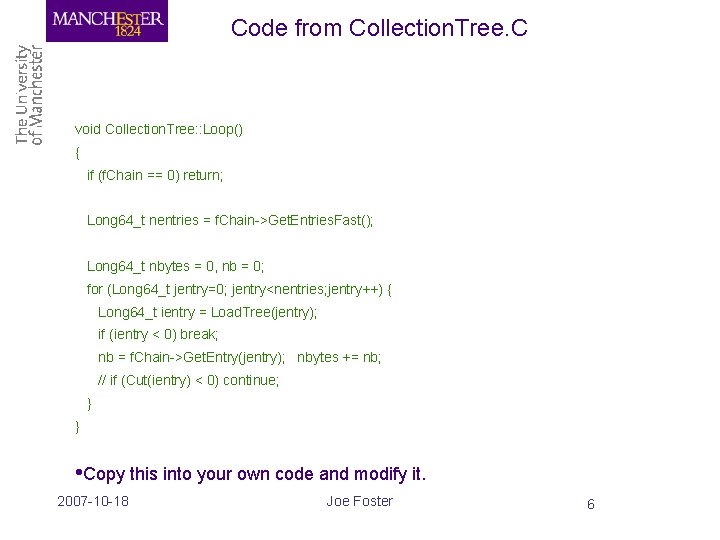
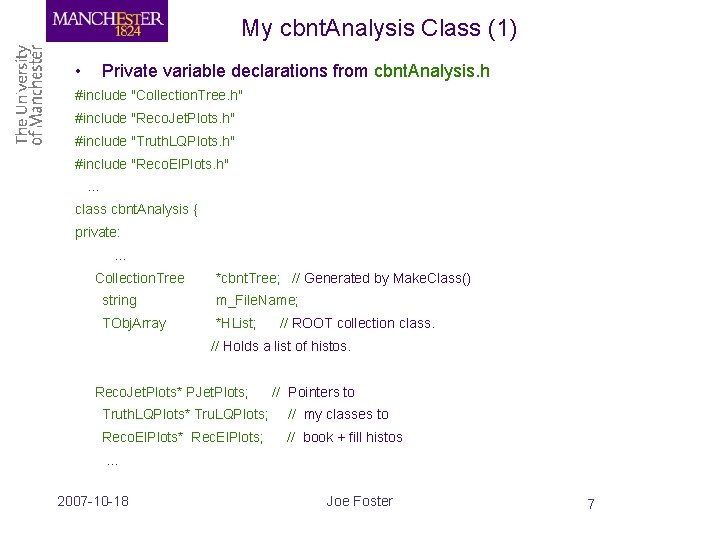
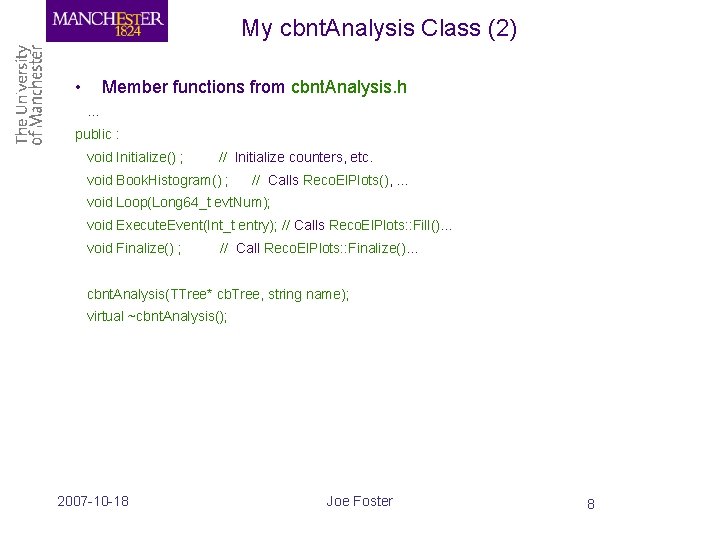
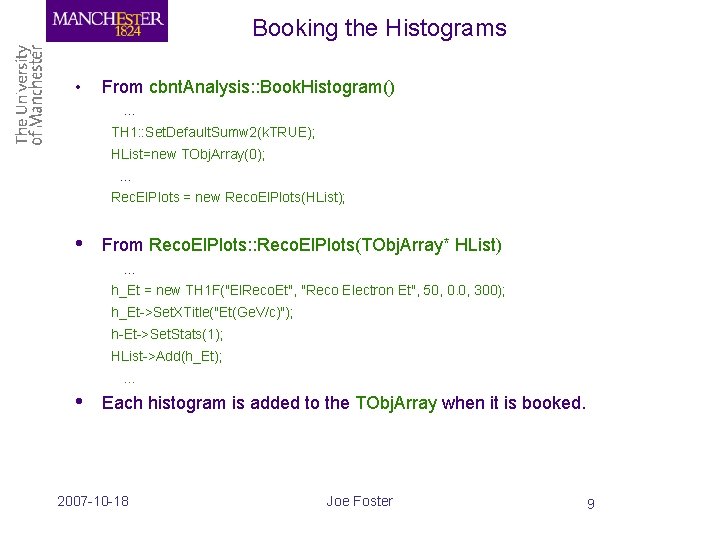
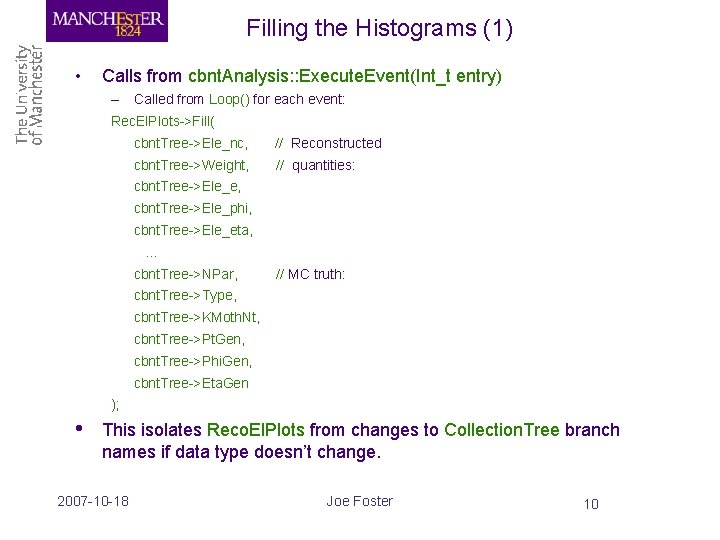
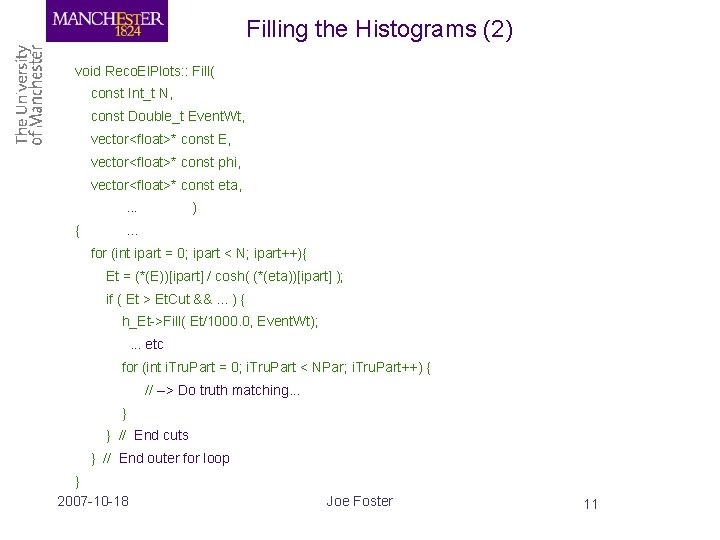
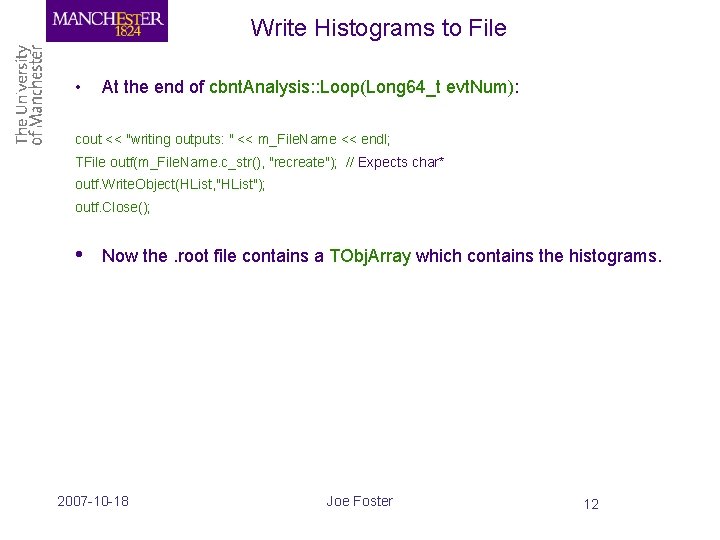
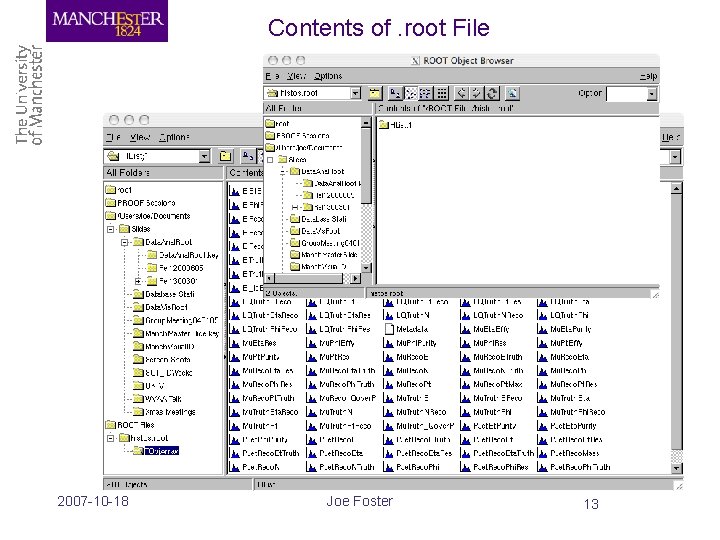
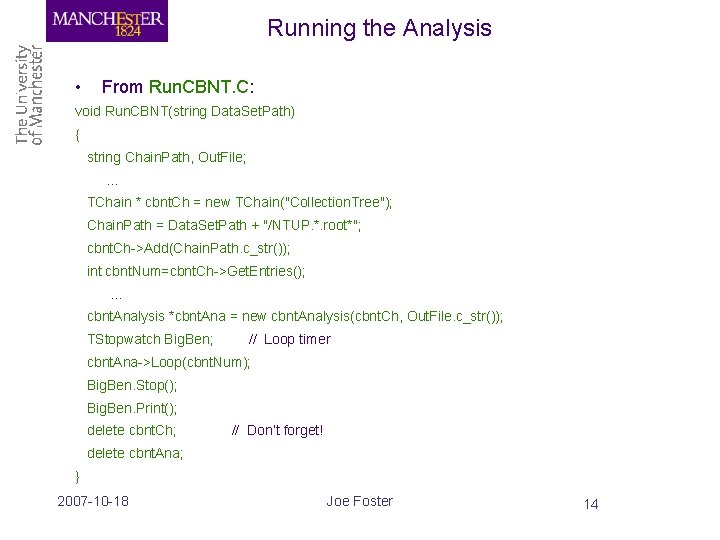
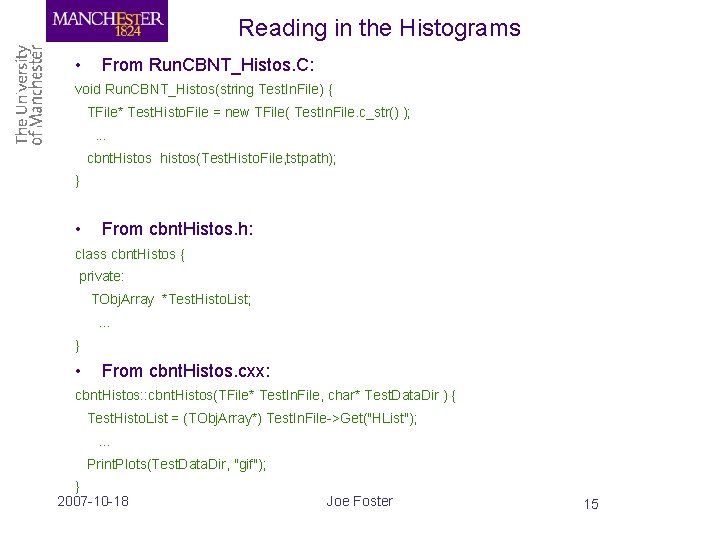
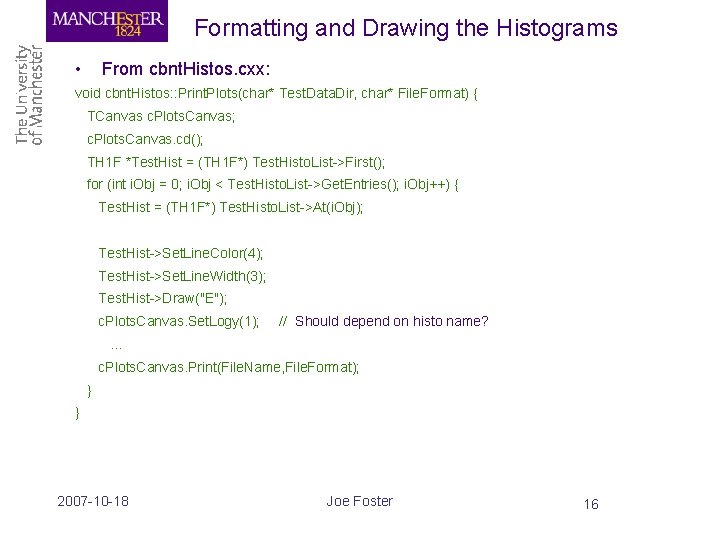
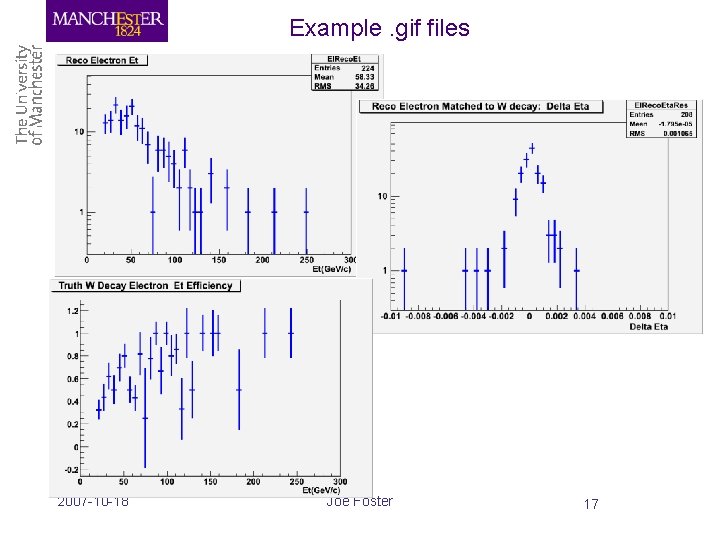
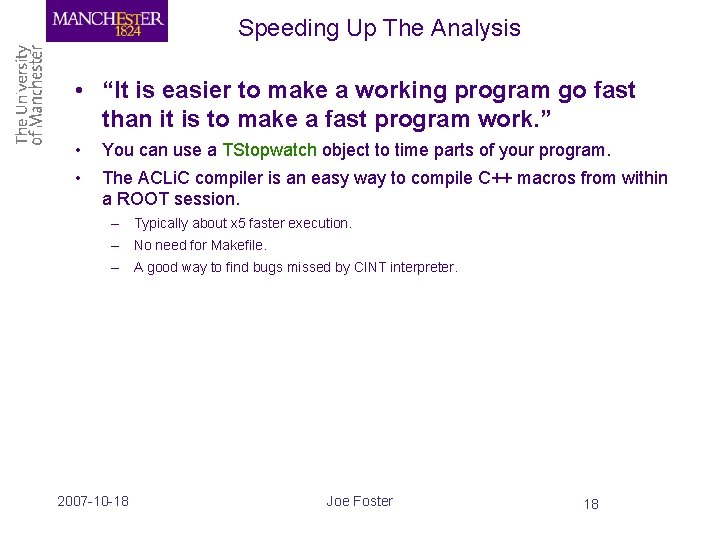
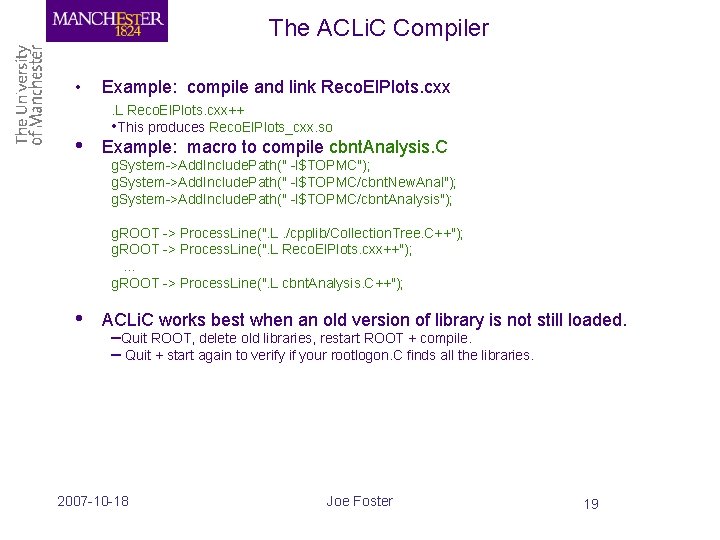
- Slides: 19
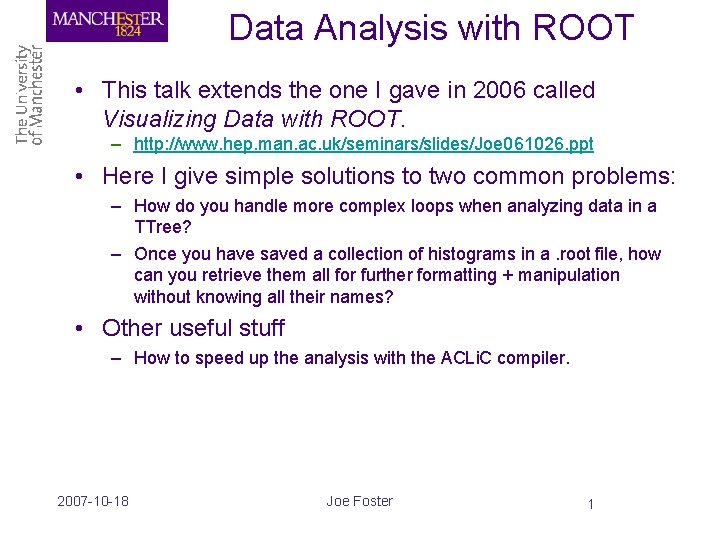
Data Analysis with ROOT • This talk extends the one I gave in 2006 called Visualizing Data with ROOT. – http: //www. hep. man. ac. uk/seminars/slides/Joe 061026. ppt • Here I give simple solutions to two common problems: – How do you handle more complex loops when analyzing data in a TTree? – Once you have saved a collection of histograms in a. root file, how can you retrieve them all for further formatting + manipulation without knowing all their names? • Other useful stuff – How to speed up the analysis with the ACLi. C compiler. 2007 -10 -18 Joe Foster 1
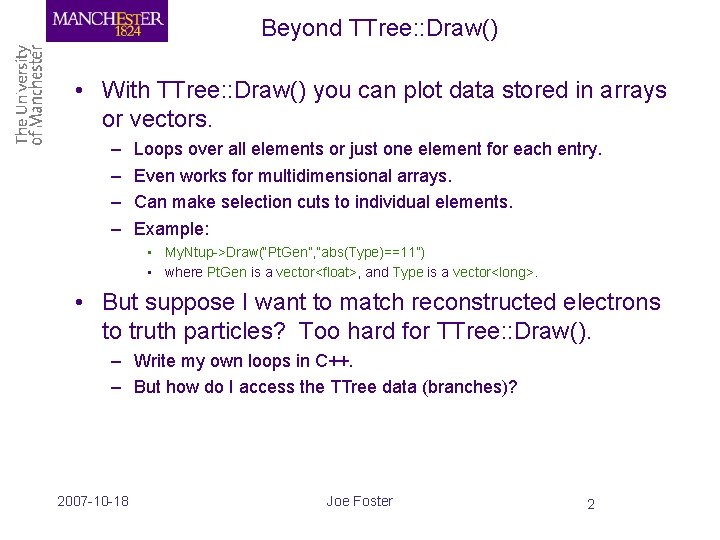
Beyond TTree: : Draw() • With TTree: : Draw() you can plot data stored in arrays or vectors. – – Loops over all elements or just one element for each entry. Even works for multidimensional arrays. Can make selection cuts to individual elements. Example: • My. Ntup->Draw(“Pt. Gen”, ”abs(Type)==11”) • where Pt. Gen is a vector<float>, and Type is a vector<long>. • But suppose I want to match reconstructed electrons to truth particles? Too hard for TTree: : Draw(). – Write my own loops in C++. – But how do I access the TTree data (branches)? 2007 -10 -18 Joe Foster 2
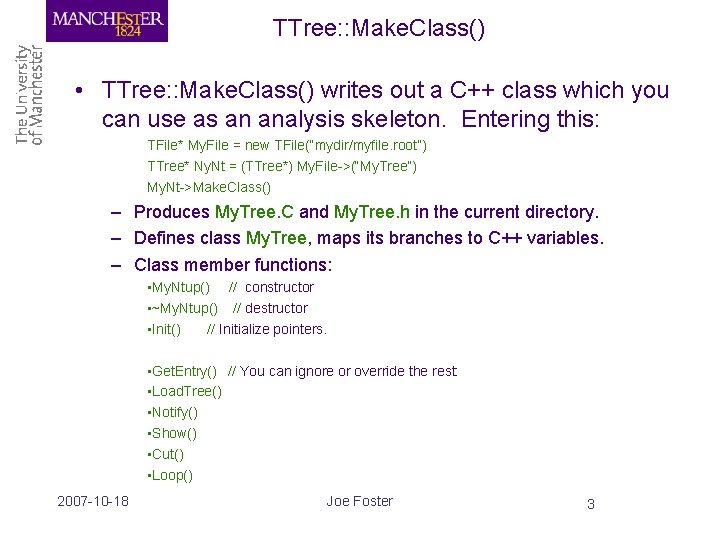
TTree: : Make. Class() • TTree: : Make. Class() writes out a C++ class which you can use as an analysis skeleton. Entering this: TFile* My. File = new TFile(“mydir/myfile. root”) TTree* Ny. Nt = (TTree*) My. File->(“My. Tree”) My. Nt->Make. Class() – Produces My. Tree. C and My. Tree. h in the current directory. – Defines class My. Tree, maps its branches to C++ variables. – Class member functions: • My. Ntup() // constructor • ~My. Ntup() // destructor • Init() // Initialize pointers. • Get. Entry() // You can ignore or override the rest: • Load. Tree() • Notify() • Show() • Cut() • Loop() 2007 -10 -18 Joe Foster 3
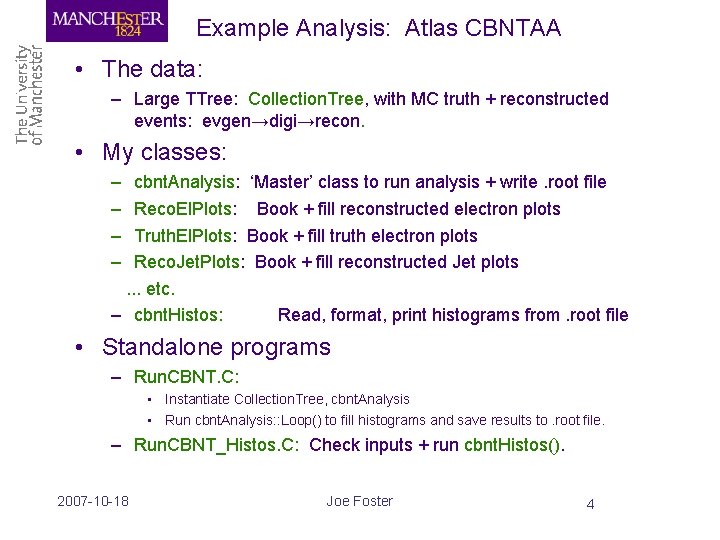
Example Analysis: Atlas CBNTAA • The data: – Large TTree: Collection. Tree, with MC truth + reconstructed events: evgen→digi→recon. • My classes: – – cbnt. Analysis: ‘Master’ class to run analysis + write. root file Reco. El. Plots: Book + fill reconstructed electron plots Truth. El. Plots: Book + fill truth electron plots Reco. Jet. Plots: Book + fill reconstructed Jet plots. . . etc. – cbnt. Histos: Read, format, print histograms from. root file • Standalone programs – Run. CBNT. C: • Instantiate Collection. Tree, cbnt. Analysis • Run cbnt. Analysis: : Loop() to fill histograms and save results to. root file. – Run. CBNT_Histos. C: Check inputs + run cbnt. Histos(). 2007 -10 -18 Joe Foster 4
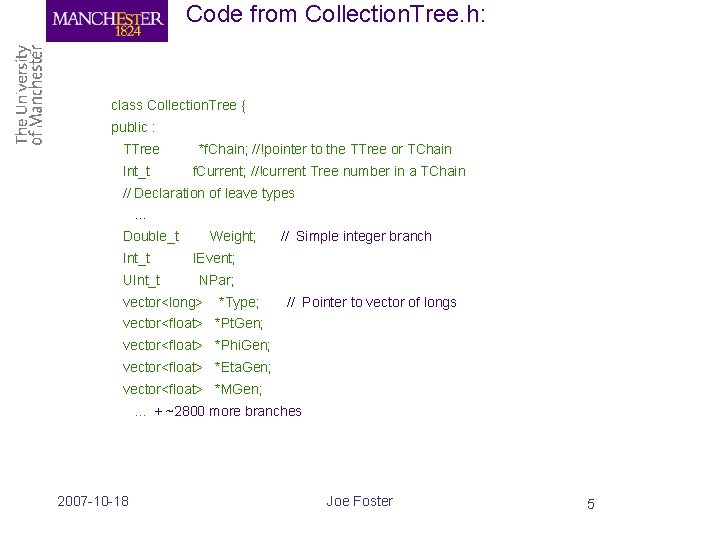
Code from Collection. Tree. h: class Collection. Tree { public : TTree Int_t *f. Chain; //!pointer to the TTree or TChain f. Current; //!current Tree number in a TChain // Declaration of leave types. . . Double_t Int_t UInt_t Weight; // Simple integer branch IEvent; NPar; vector<long> *Type; // Pointer to vector of longs vector<float> *Pt. Gen; vector<float> *Phi. Gen; vector<float> *Eta. Gen; vector<float> *MGen; . . . + ~2800 more branches 2007 -10 -18 Joe Foster 5
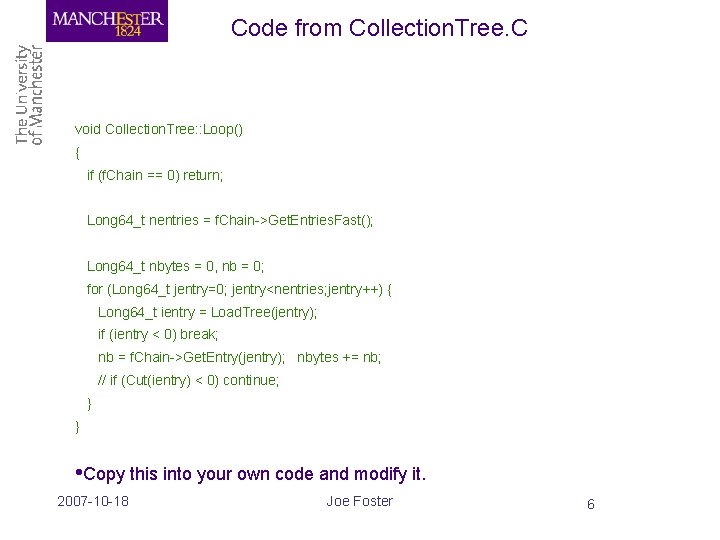
Code from Collection. Tree. C void Collection. Tree: : Loop() { if (f. Chain == 0) return; Long 64_t nentries = f. Chain->Get. Entries. Fast(); Long 64_t nbytes = 0, nb = 0; for (Long 64_t jentry=0; jentry<nentries; jentry++) { Long 64_t ientry = Load. Tree(jentry); if (ientry < 0) break; nb = f. Chain->Get. Entry(jentry); nbytes += nb; // if (Cut(ientry) < 0) continue; } } • Copy this into your own code and modify it. 2007 -10 -18 Joe Foster 6
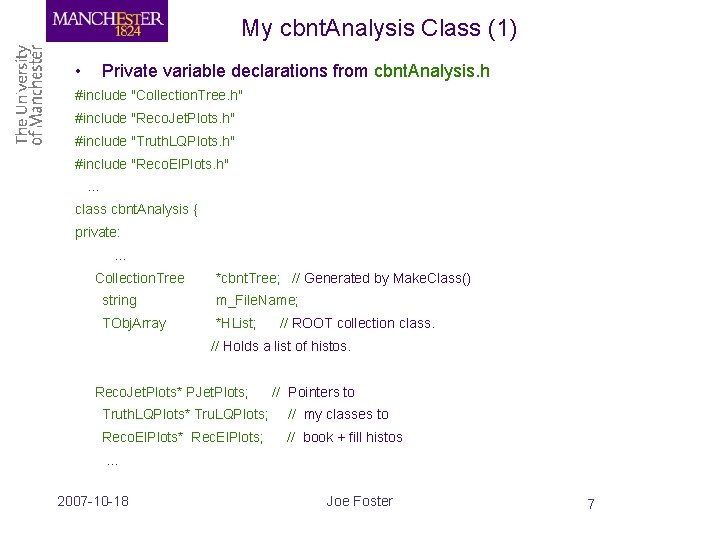
My cbnt. Analysis Class (1) • Private variable declarations from cbnt. Analysis. h #include "Collection. Tree. h" #include "Reco. Jet. Plots. h" #include "Truth. LQPlots. h" #include "Reco. El. Plots. h". . . class cbnt. Analysis { private: . . . Collection. Tree *cbnt. Tree; // Generated by Make. Class() string m_File. Name; TObj. Array *HList; // ROOT collection class. // Holds a list of histos. Reco. Jet. Plots* PJet. Plots; // Pointers to Truth. LQPlots* Tru. LQPlots; // my classes to Reco. El. Plots* Rec. El. Plots; // book + fill histos . . . 2007 -10 -18 Joe Foster 7
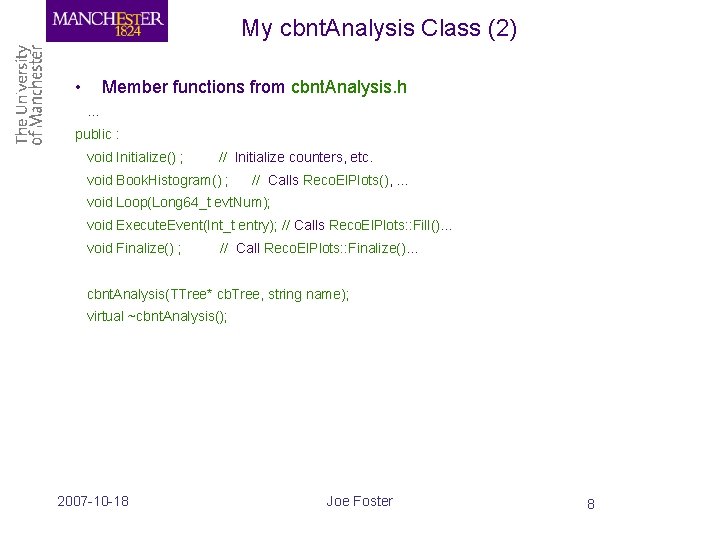
My cbnt. Analysis Class (2) • Member functions from cbnt. Analysis. h. . . public : void Initialize() ; // Initialize counters, etc. void Book. Histogram() ; // Calls Reco. El. Plots(), . . . void Loop(Long 64_t evt. Num); void Execute. Event(Int_t entry); // Calls Reco. El. Plots: : Fill(). . . void Finalize() ; // Call Reco. El. Plots: : Finalize(). . . cbnt. Analysis(TTree* cb. Tree, string name); virtual ~cbnt. Analysis(); 2007 -10 -18 Joe Foster 8
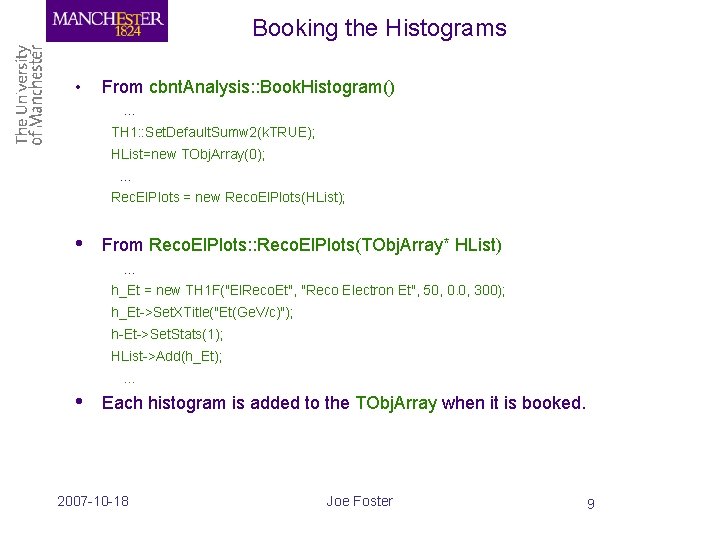
Booking the Histograms • From cbnt. Analysis: : Book. Histogram(). . . TH 1: : Set. Default. Sumw 2(k. TRUE); HList=new TObj. Array(0); . . . Rec. El. Plots = new Reco. El. Plots(HList); • From Reco. El. Plots: : Reco. El. Plots(TObj. Array* HList). . . h_Et = new TH 1 F("El. Reco. Et", "Reco Electron Et", 50, 0. 0, 300); h_Et->Set. XTitle("Et(Ge. V/c)"); h-Et->Set. Stats(1); HList->Add(h_Et); . . . • Each histogram is added to the TObj. Array when it is booked. 2007 -10 -18 Joe Foster 9
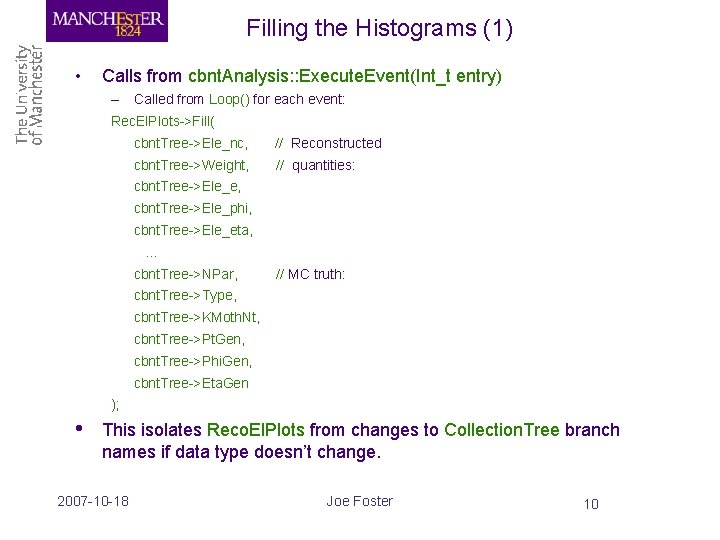
Filling the Histograms (1) • Calls from cbnt. Analysis: : Execute. Event(Int_t entry) – Called from Loop() for each event: Rec. El. Plots->Fill( cbnt. Tree->Ele_nc, // Reconstructed cbnt. Tree->Weight, // quantities: cbnt. Tree->Ele_e, cbnt. Tree->Ele_phi, cbnt. Tree->Ele_eta, . . . cbnt. Tree->NPar, // MC truth: cbnt. Tree->Type, cbnt. Tree->KMoth. Nt, cbnt. Tree->Pt. Gen, cbnt. Tree->Phi. Gen, cbnt. Tree->Eta. Gen ); • This isolates Reco. El. Plots from changes to Collection. Tree branch names if data type doesn’t change. 2007 -10 -18 Joe Foster 10
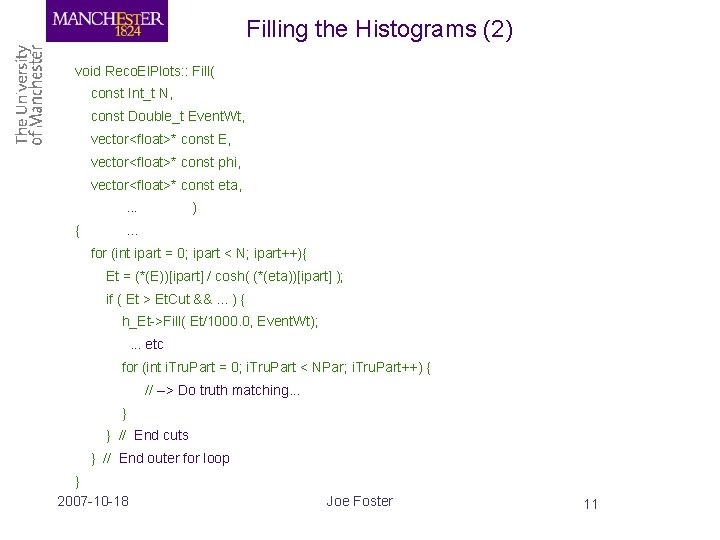
Filling the Histograms (2) void Reco. El. Plots: : Fill( const Int_t N, const Double_t Event. Wt, vector<float>* const E, vector<float>* const phi, vector<float>* const eta, . . . { ) . . . for (int ipart = 0; ipart < N; ipart++){ Et = (*(E))[ipart] / cosh( (*(eta))[ipart] ); if ( Et > Et. Cut &&. . . ) { h_Et->Fill( Et/1000. 0, Event. Wt); . . . etc for (int i. Tru. Part = 0; i. Tru. Part < NPar; i. Tru. Part++) { // --> Do truth matching. . . } } // End cuts } // End outer for loop } 2007 -10 -18 Joe Foster 11
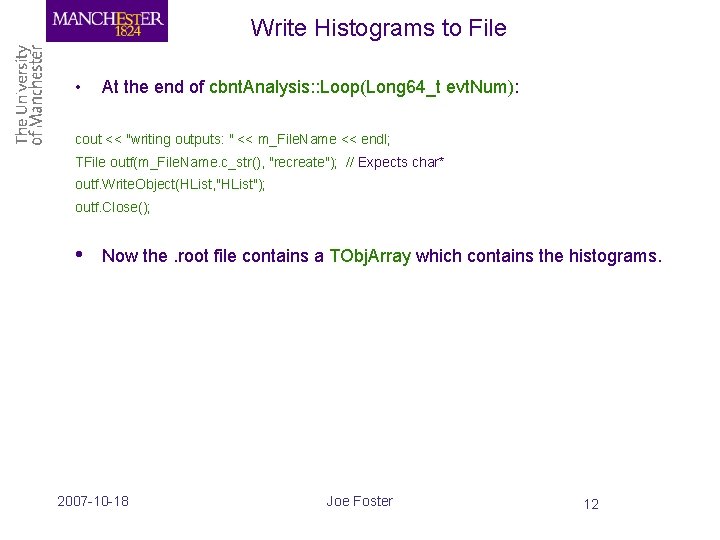
Write Histograms to File • At the end of cbnt. Analysis: : Loop(Long 64_t evt. Num): cout << "writing outputs: " << m_File. Name << endl; TFile outf(m_File. Name. c_str(), "recreate"); // Expects char* outf. Write. Object(HList, "HList"); outf. Close(); • Now the. root file contains a TObj. Array which contains the histograms. 2007 -10 -18 Joe Foster 12
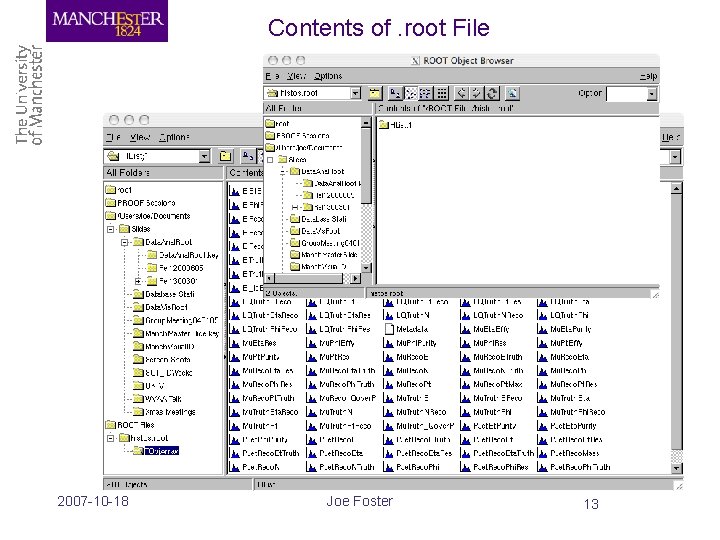
Contents of. root File 2007 -10 -18 Joe Foster 13
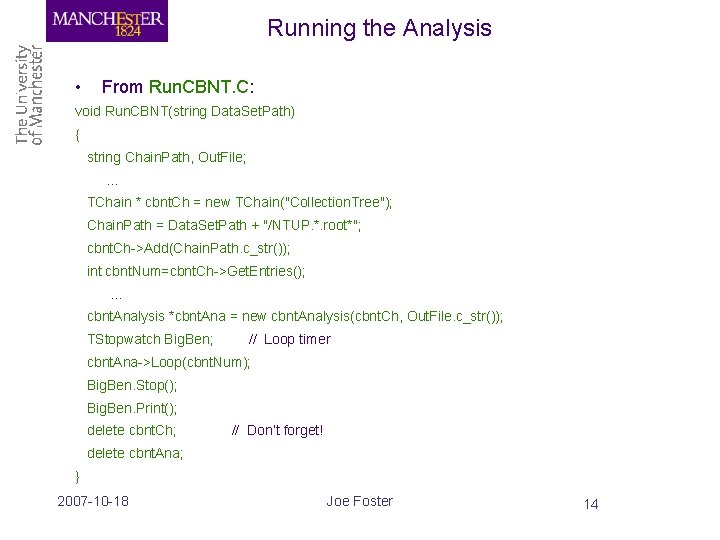
Running the Analysis • From Run. CBNT. C: void Run. CBNT(string Data. Set. Path) { string Chain. Path, Out. File; . . . TChain * cbnt. Ch = new TChain("Collection. Tree"); Chain. Path = Data. Set. Path + "/NTUP. *. root*"; cbnt. Ch->Add(Chain. Path. c_str()); int cbnt. Num=cbnt. Ch->Get. Entries(); . . . cbnt. Analysis *cbnt. Ana = new cbnt. Analysis(cbnt. Ch, Out. File. c_str()); TStopwatch Big. Ben; // Loop timer cbnt. Ana->Loop(cbnt. Num); Big. Ben. Stop(); Big. Ben. Print(); delete cbnt. Ch; // Don’t forget! delete cbnt. Ana; } 2007 -10 -18 Joe Foster 14
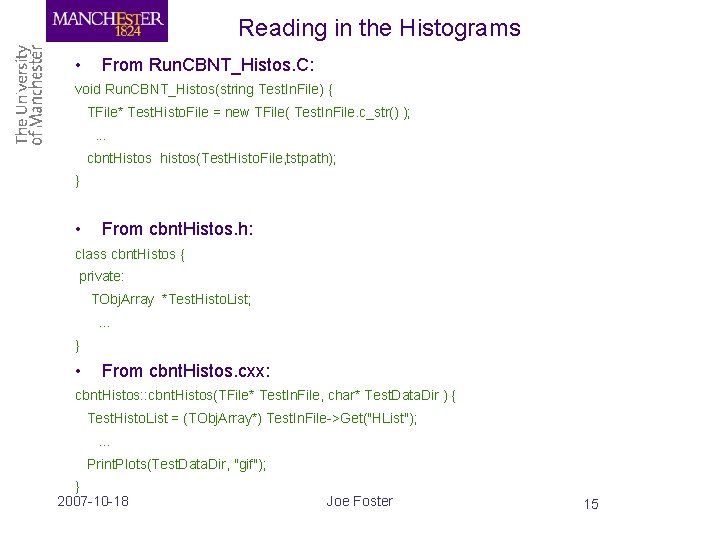
Reading in the Histograms • From Run. CBNT_Histos. C: void Run. CBNT_Histos(string Test. In. File) { TFile* Test. Histo. File = new TFile( Test. In. File. c_str() ); . . . cbnt. Histos histos(Test. Histo. File, tstpath); } • From cbnt. Histos. h: class cbnt. Histos { private: TObj. Array *Test. Histo. List; . . . } • From cbnt. Histos. cxx: cbnt. Histos: : cbnt. Histos(TFile* Test. In. File, char* Test. Data. Dir ) { Test. Histo. List = (TObj. Array*) Test. In. File->Get("HList"); . . . Print. Plots(Test. Data. Dir, "gif"); } 2007 -10 -18 Joe Foster 15
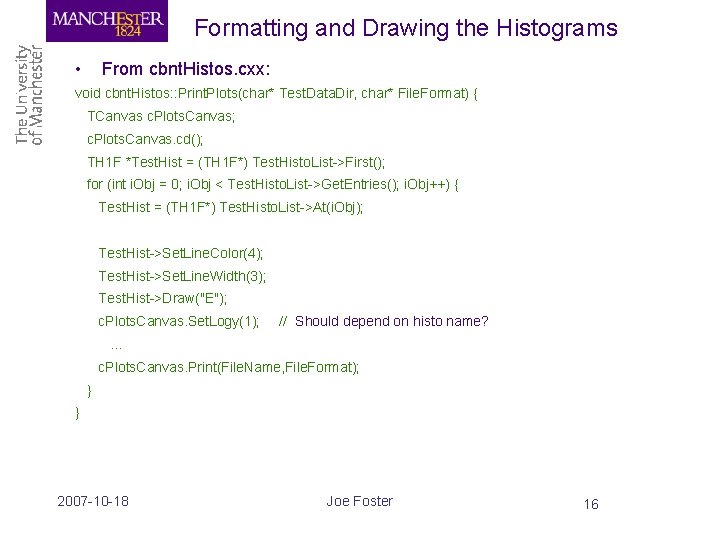
Formatting and Drawing the Histograms • From cbnt. Histos. cxx: void cbnt. Histos: : Print. Plots(char* Test. Data. Dir, char* File. Format) { TCanvas c. Plots. Canvas; c. Plots. Canvas. cd(); TH 1 F *Test. Hist = (TH 1 F*) Test. Histo. List->First(); for (int i. Obj = 0; i. Obj < Test. Histo. List->Get. Entries(); i. Obj++) { Test. Hist = (TH 1 F*) Test. Histo. List->At(i. Obj); Test. Hist->Set. Line. Color(4); Test. Hist->Set. Line. Width(3); Test. Hist->Draw("E"); c. Plots. Canvas. Set. Logy(1); // Should depend on histo name? . . . c. Plots. Canvas. Print(File. Name, File. Format); } } 2007 -10 -18 Joe Foster 16
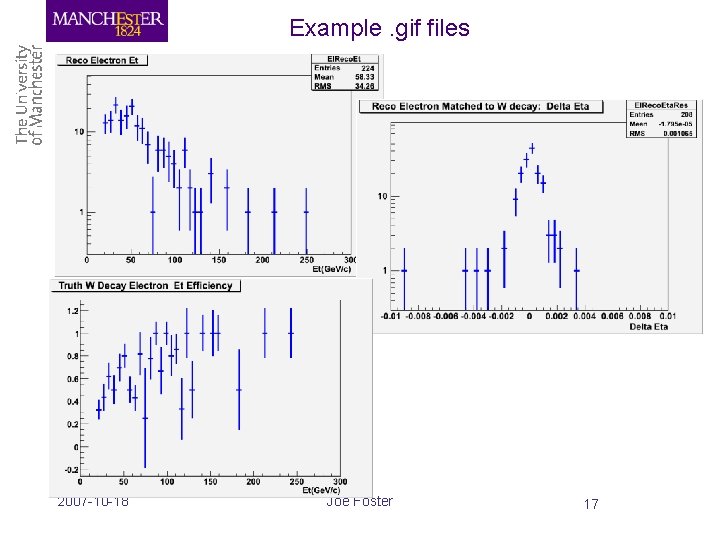
Example. gif files 2007 -10 -18 Joe Foster 17
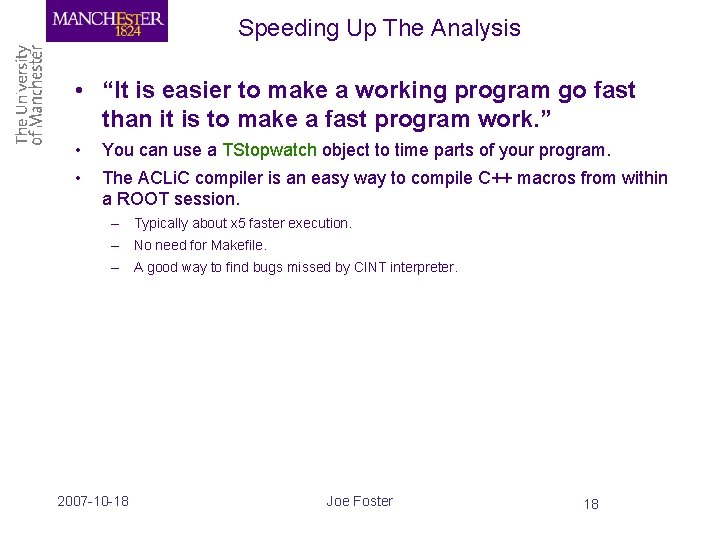
Speeding Up The Analysis • “It is easier to make a working program go fast than it is to make a fast program work. ” • • You can use a TStopwatch object to time parts of your program. The ACLi. C compiler is an easy way to compile C++ macros from within a ROOT session. – Typically about x 5 faster execution. – No need for Makefile. – A good way to find bugs missed by CINT interpreter. 2007 -10 -18 Joe Foster 18
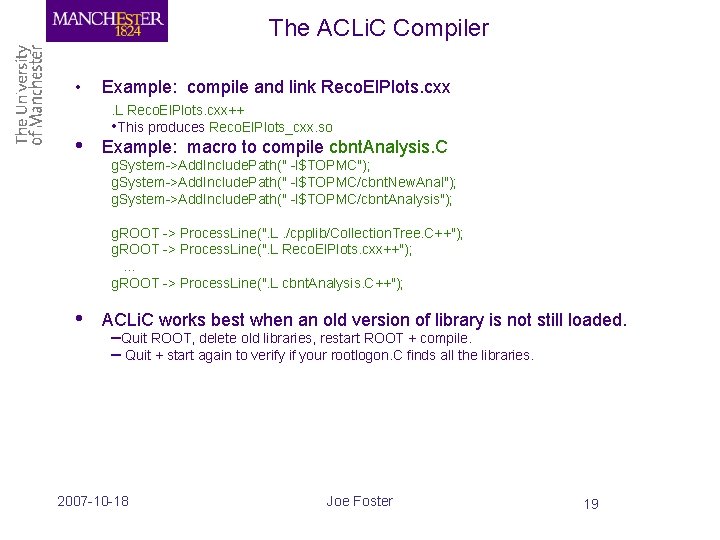
The ACLi. C Compiler • • Example: compile and link Reco. El. Plots. cxx. L Reco. El. Plots. cxx++ • This produces Reco. El. Plots_cxx. so Example: macro to compile cbnt. Analysis. C g. System->Add. Include. Path(" -I$TOPMC"); g. System->Add. Include. Path(" -I$TOPMC/cbnt. New. Anal"); g. System->Add. Include. Path(" -I$TOPMC/cbnt. Analysis"); g. ROOT -> Process. Line(". L. /cpplib/Collection. Tree. C++"); g. ROOT -> Process. Line(". L Reco. El. Plots. cxx++"); . . . g. ROOT -> Process. Line(". L cbnt. Analysis. C++"); • ACLi. C works best when an old version of library is not still loaded. –Quit ROOT, delete old libraries, restart ROOT + compile. – Quit + start again to verify if your rootlogon. C finds all the libraries. 2007 -10 -18 Joe Foster 19
Talk, read talk write template
Amateurs talk tactics professionals talk logistics
Problem talk vs solution talk
Root data analysis
Extends use case
Include extend use case
Thread class hierarchy in java
Pancreas location quadrant
Welcome to the netherlands, a tiny country
Extends high earth atmosphere
Thyrohyoid membrane is pierced by
Public class test subject extends test class
Extends jframe
Runtimeexception extends exception
Definisi use case diagram
Extends jframe java
Intervertebral disc prolapse
Extends vs includes use case
A flat surface that has no thickness and extends forever
Bas relief paper sculpture