CSE321 Programming Languages Introduction to Functional Programming POSTECH
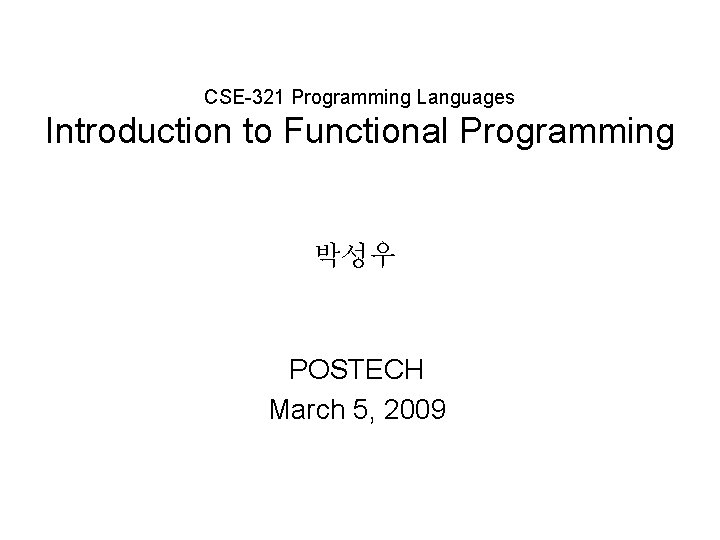
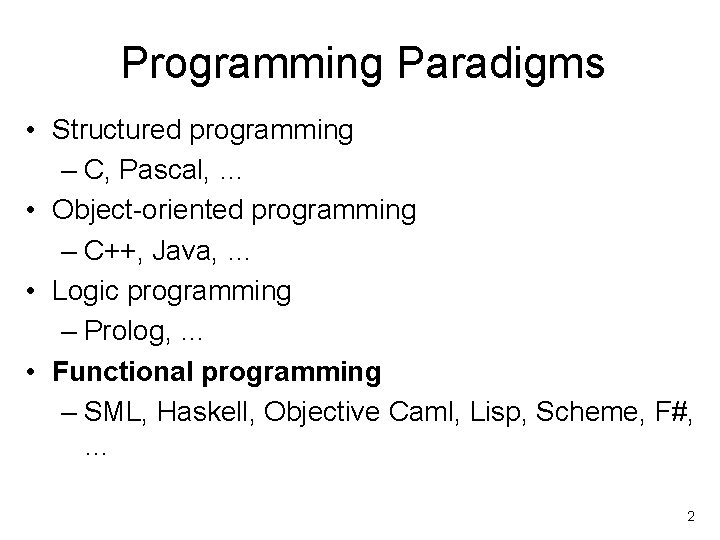
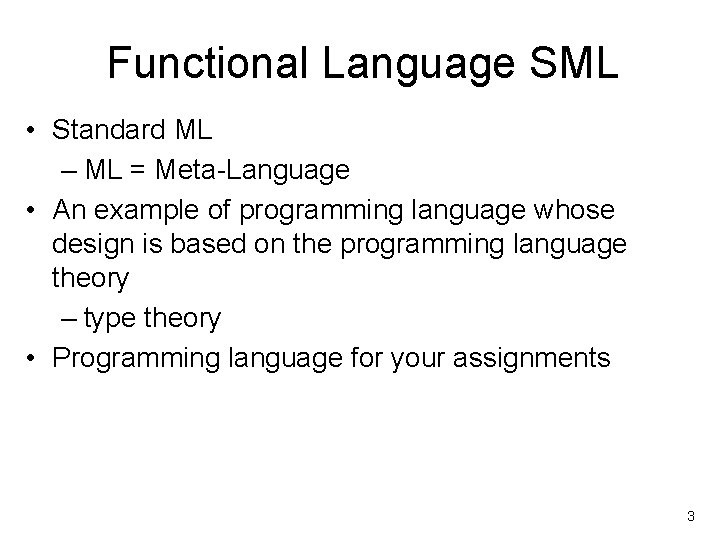
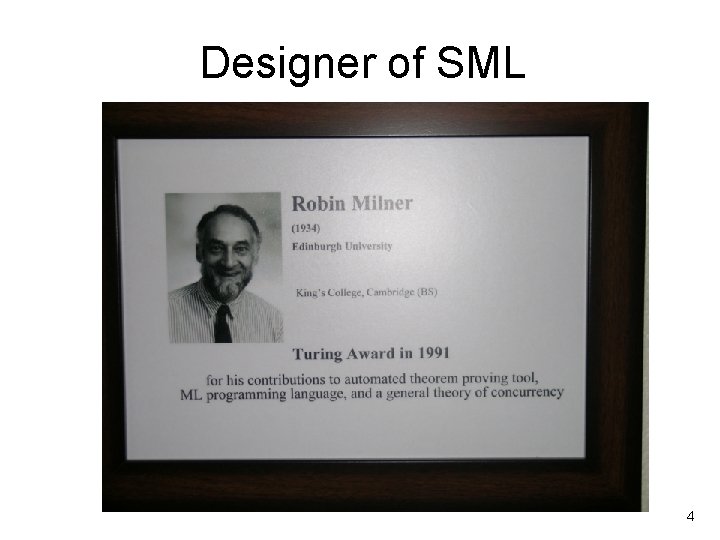
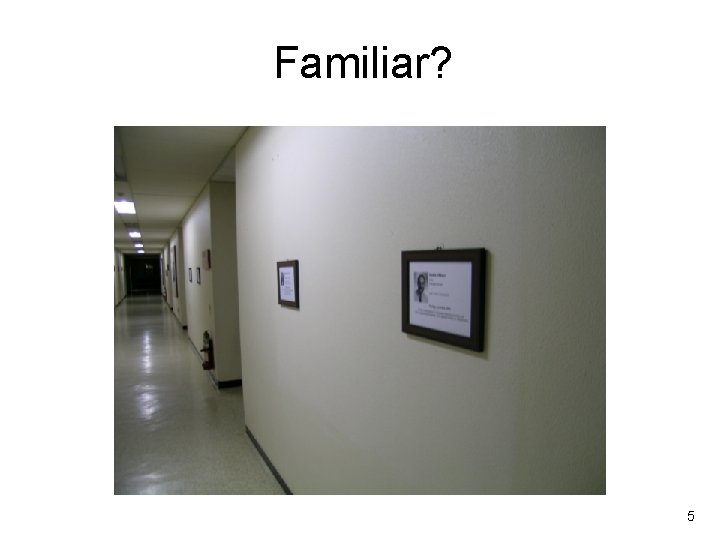
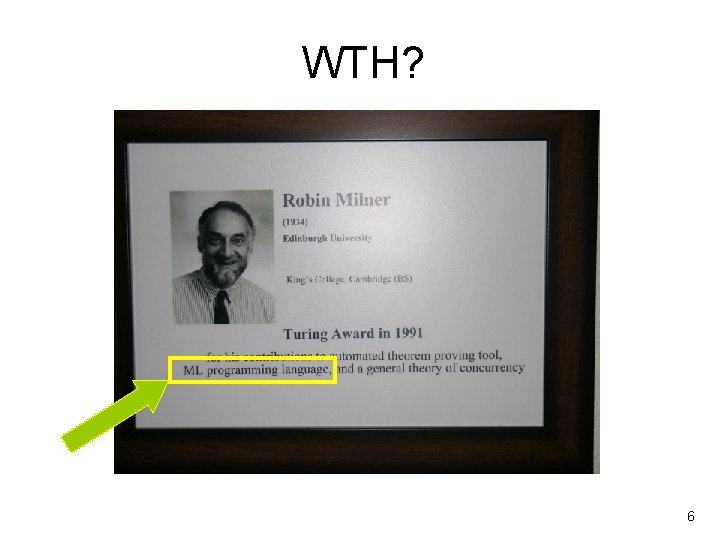
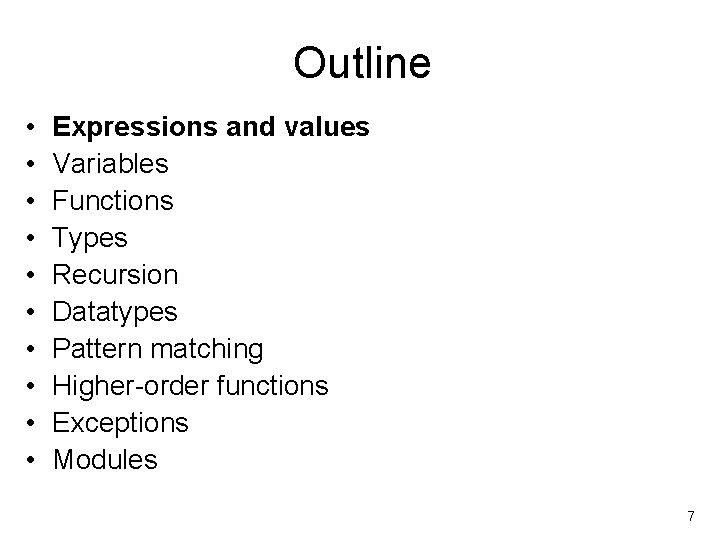
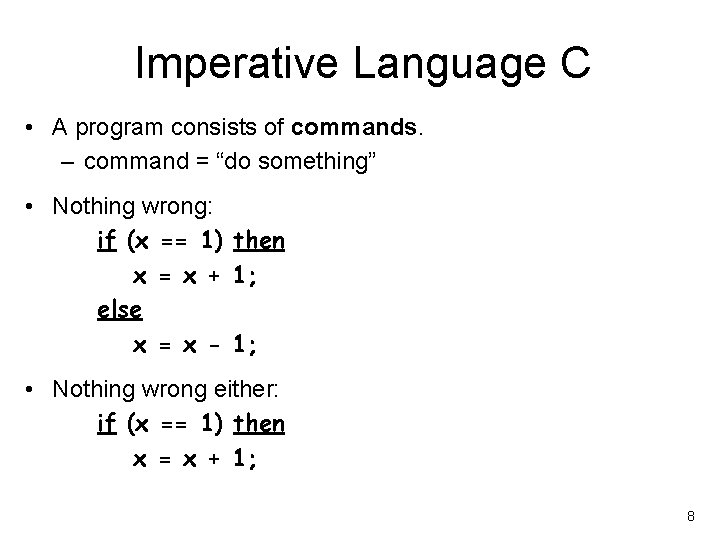
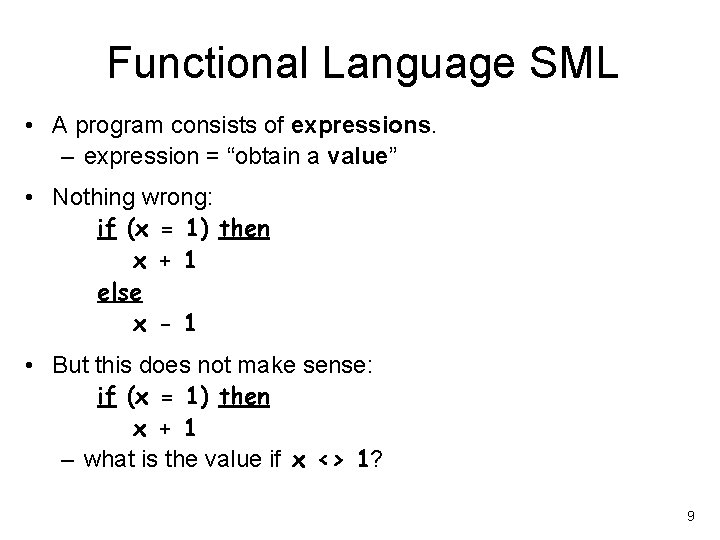
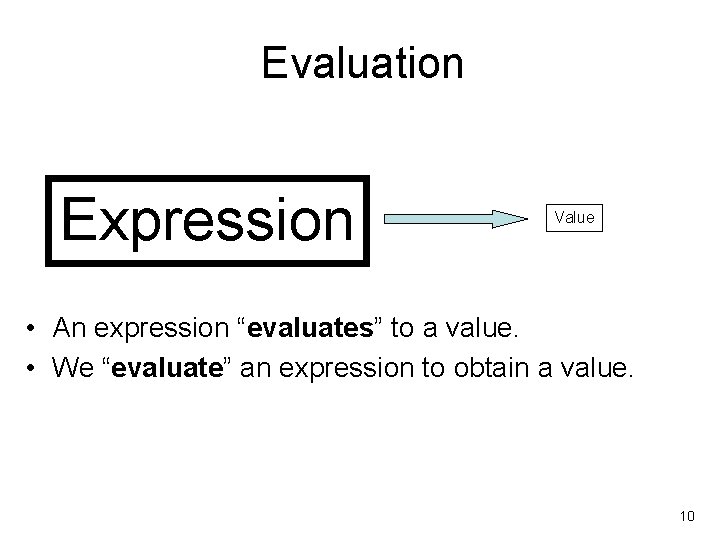
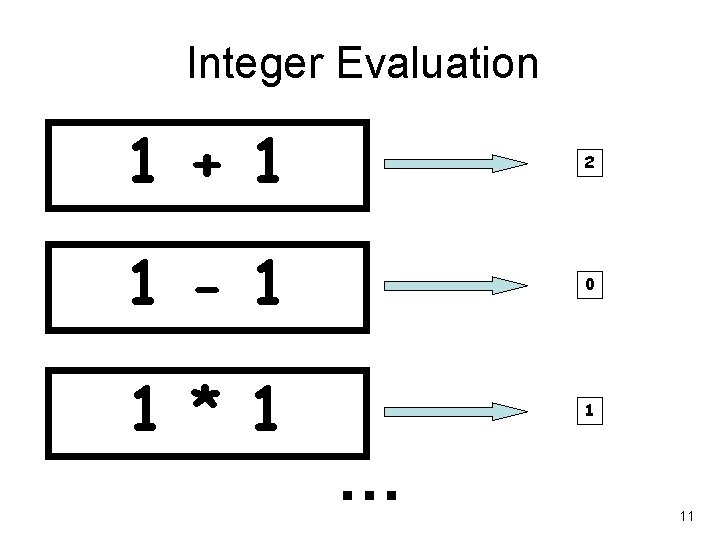
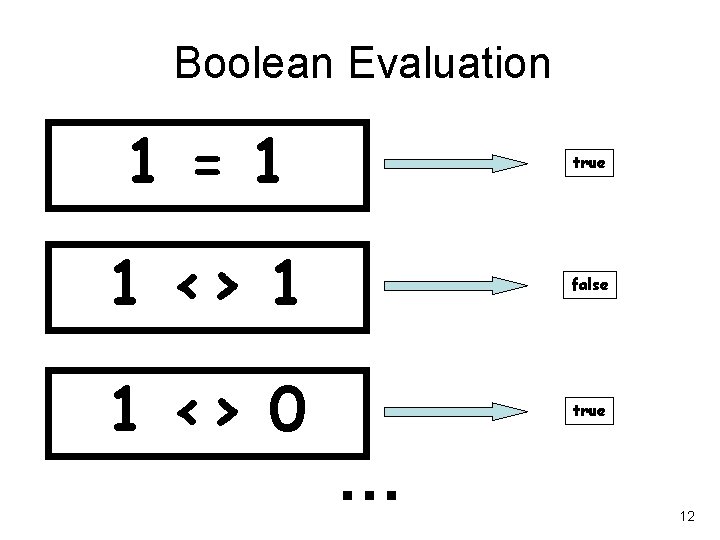
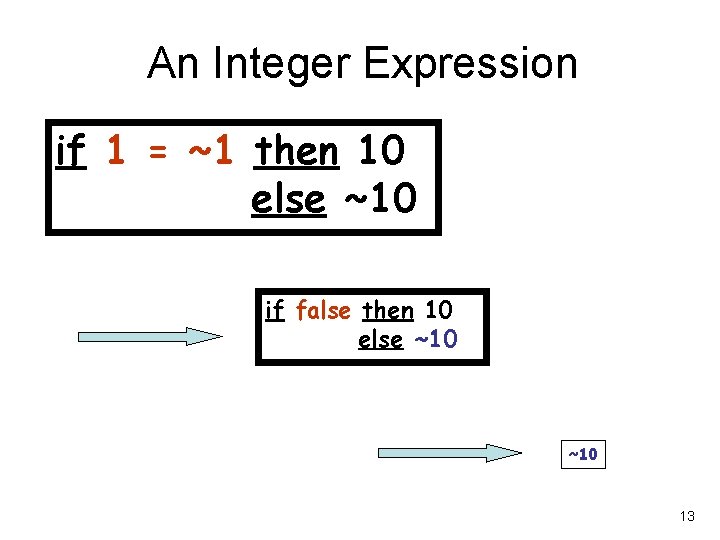
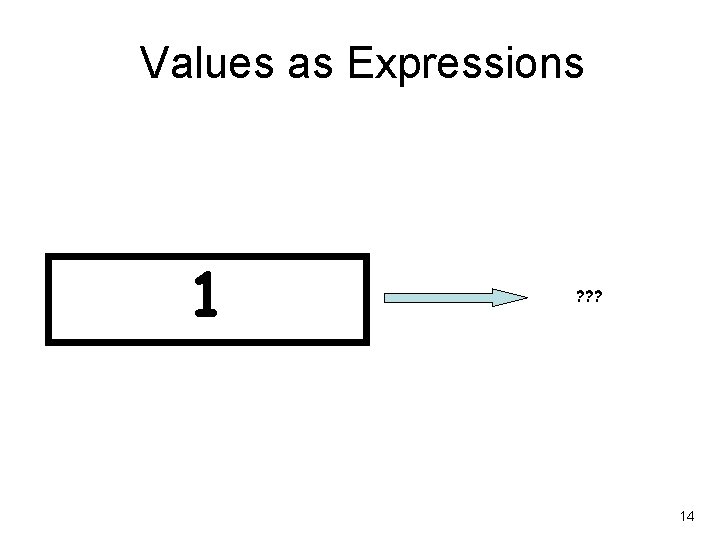
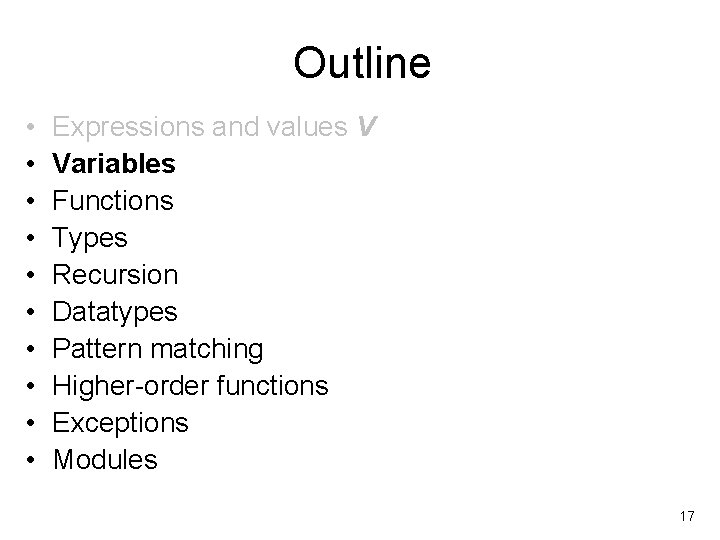
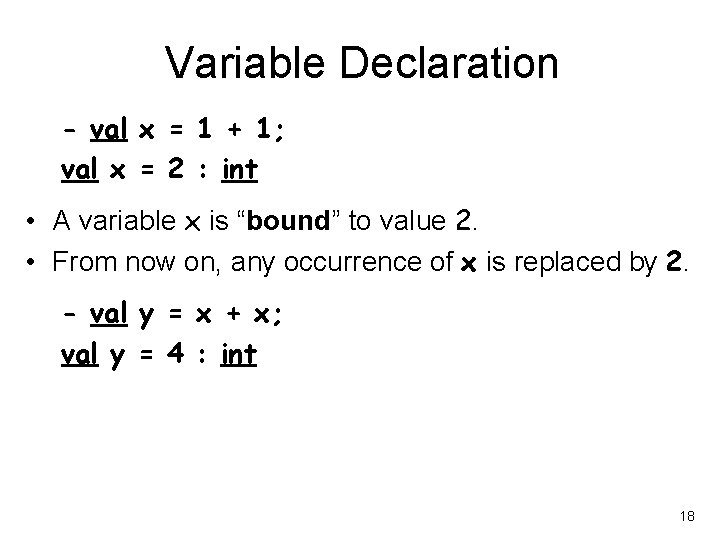
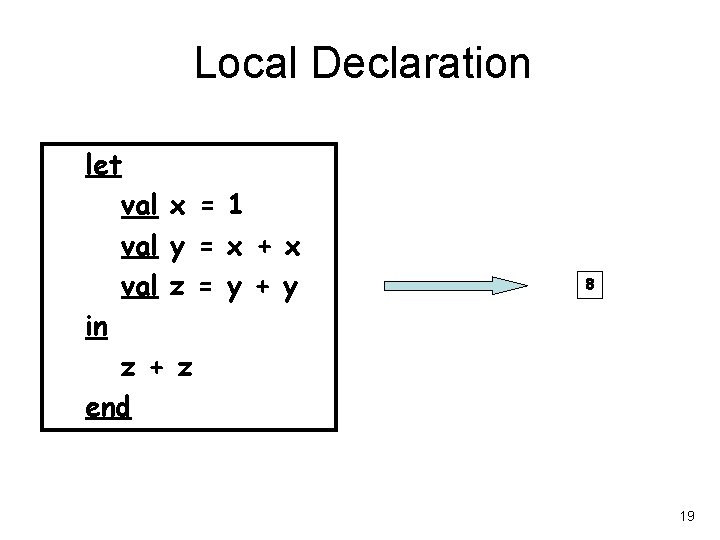
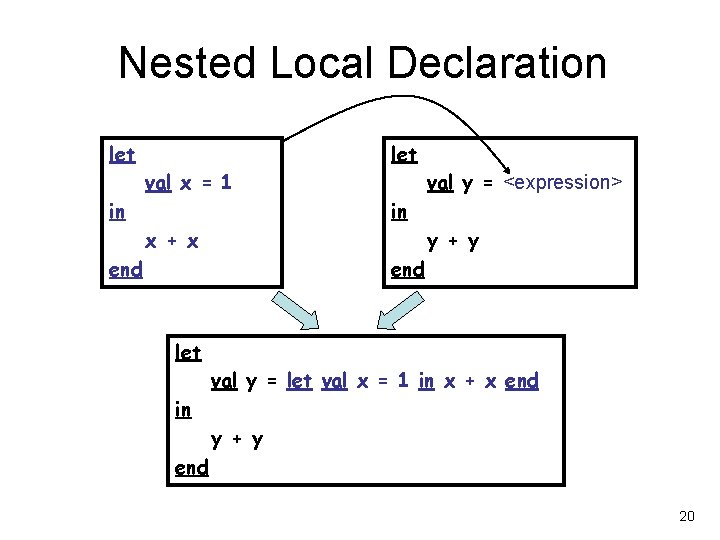
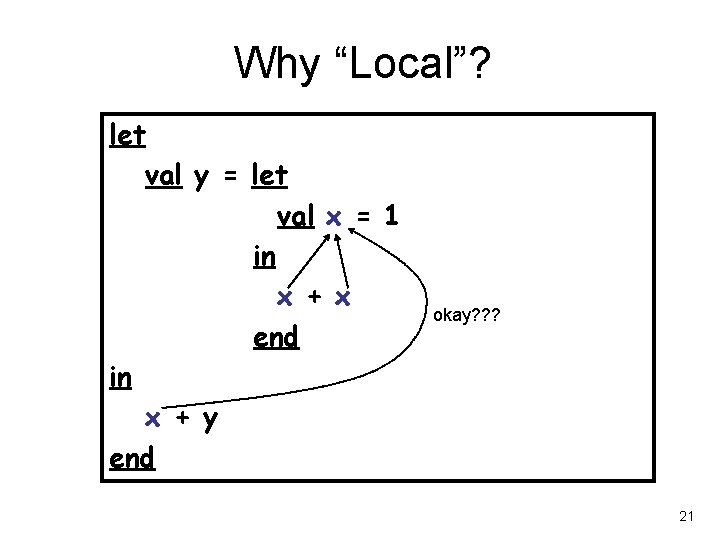
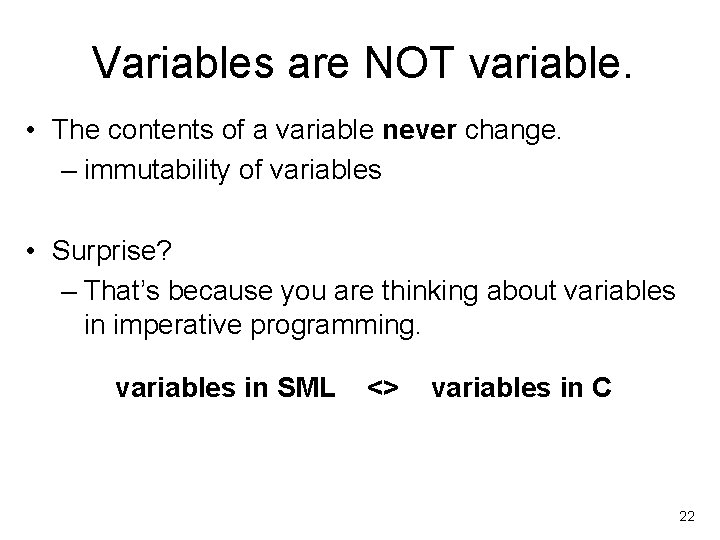
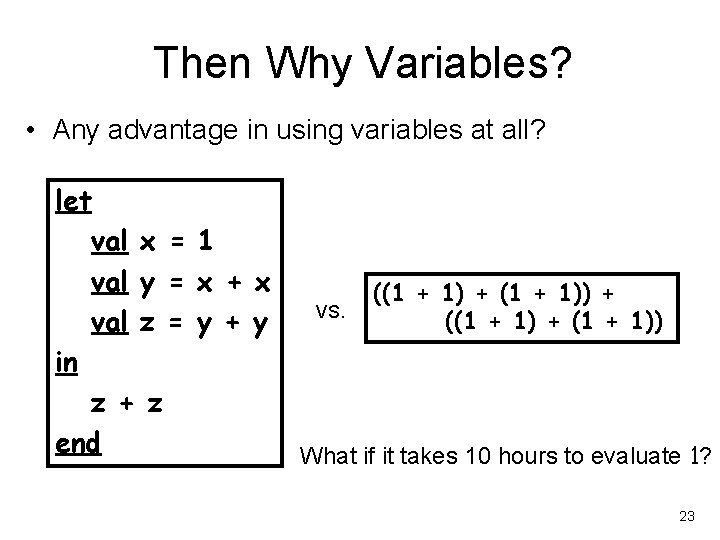
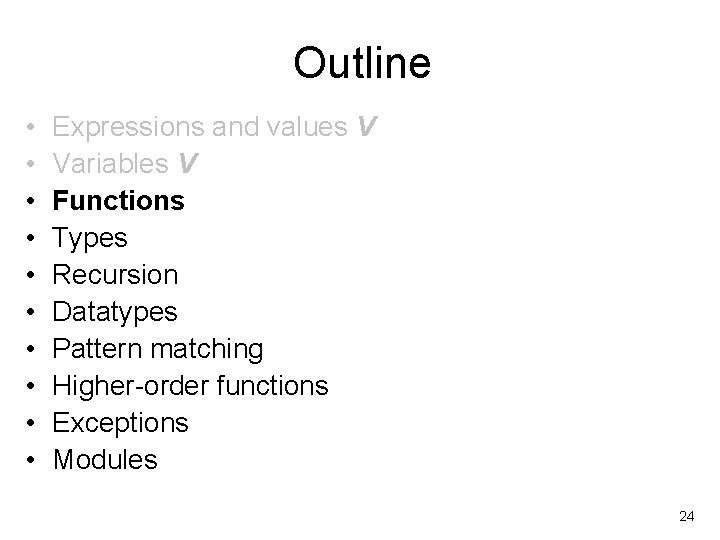
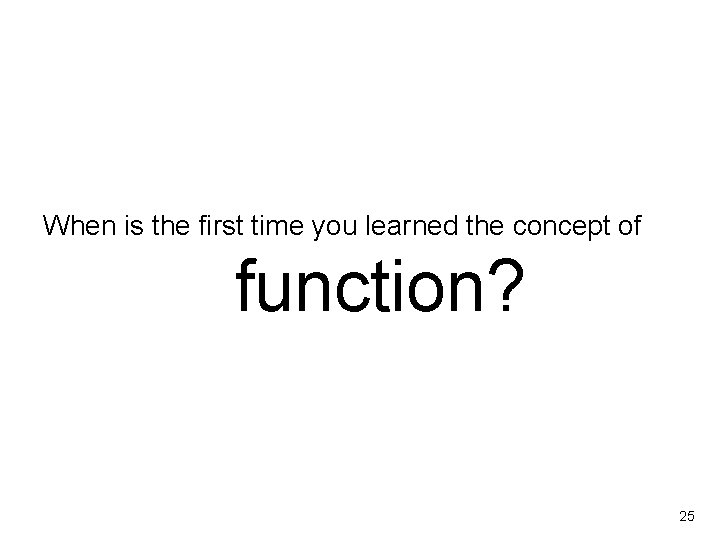
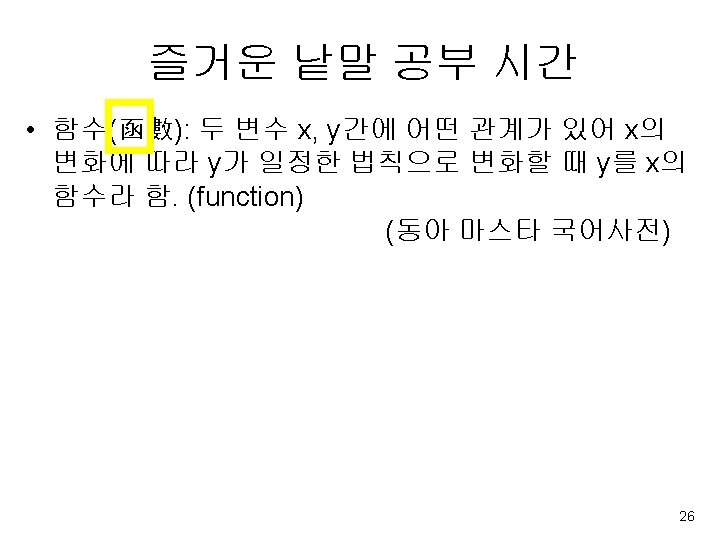
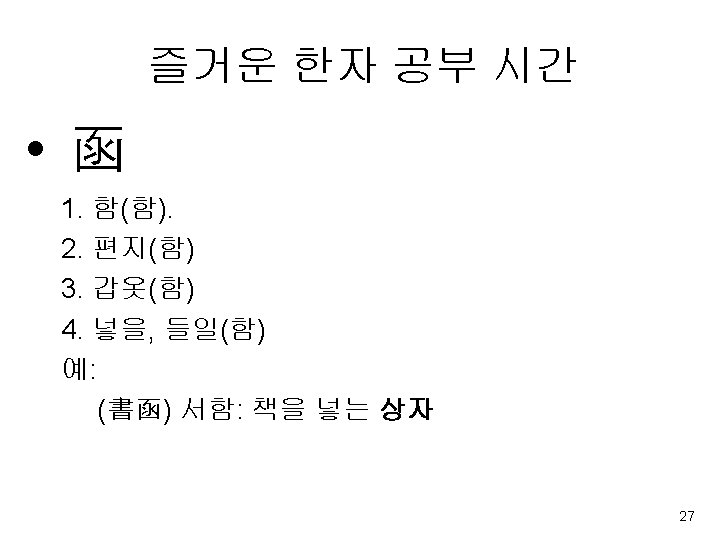
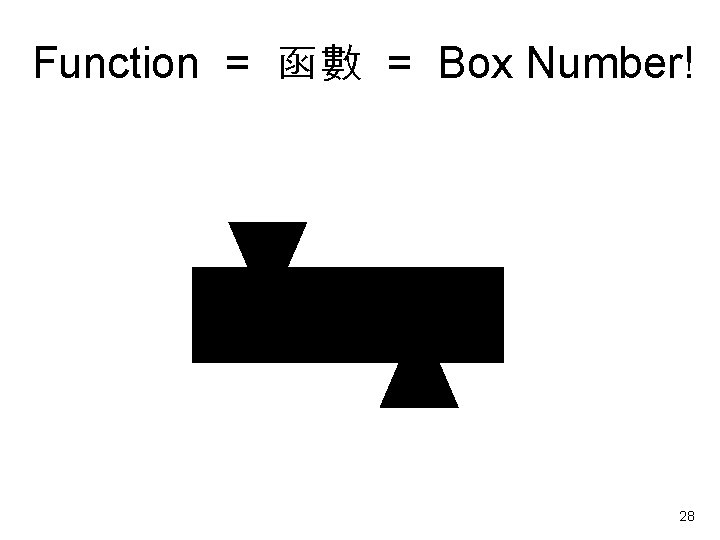
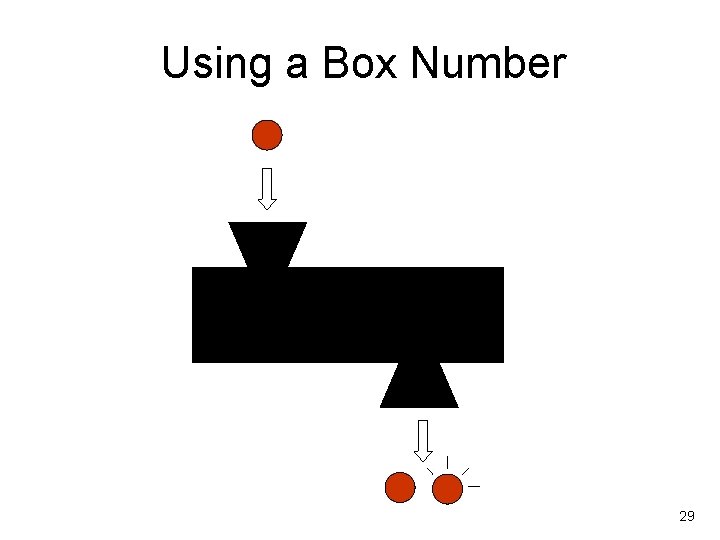
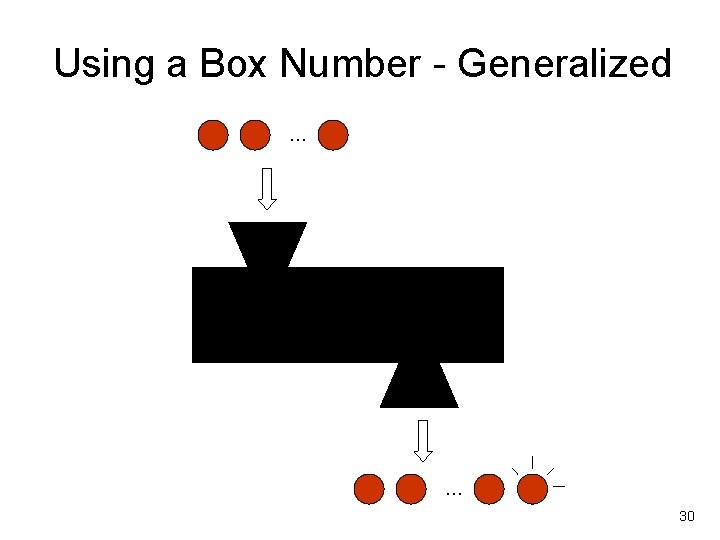
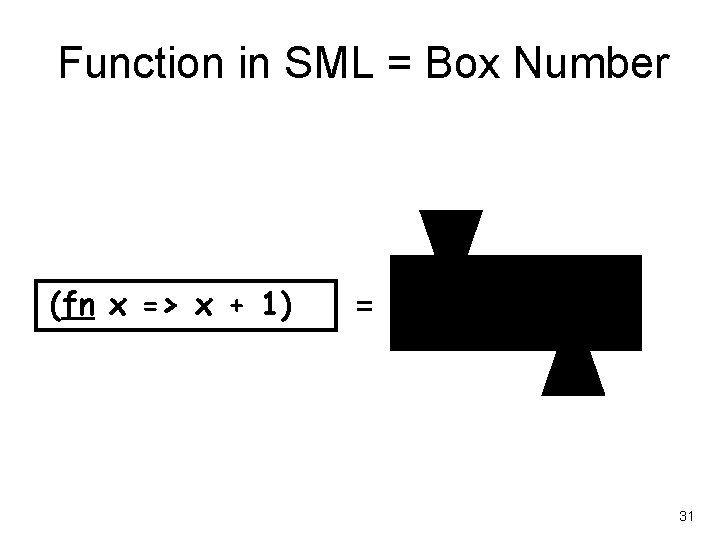
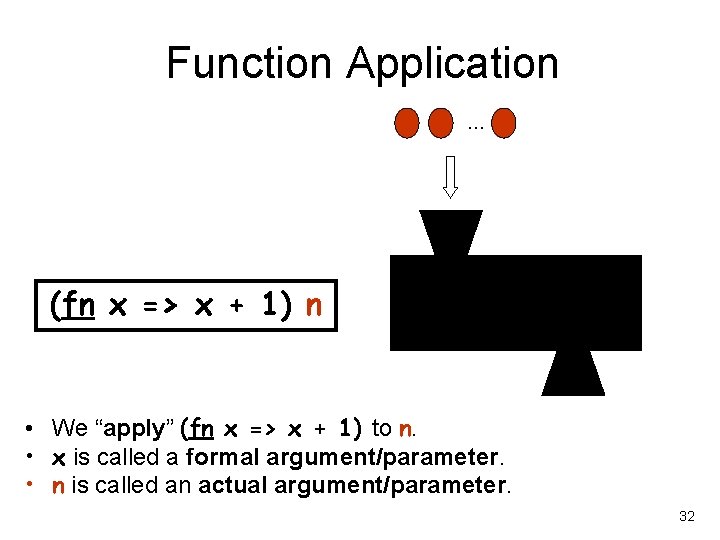
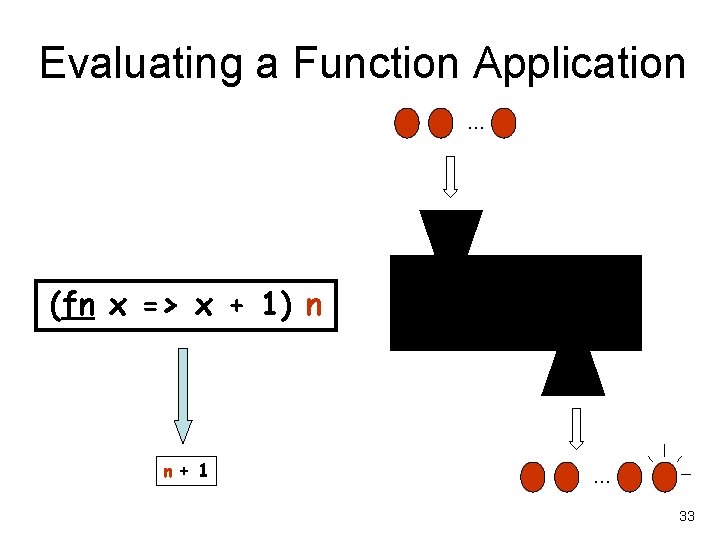
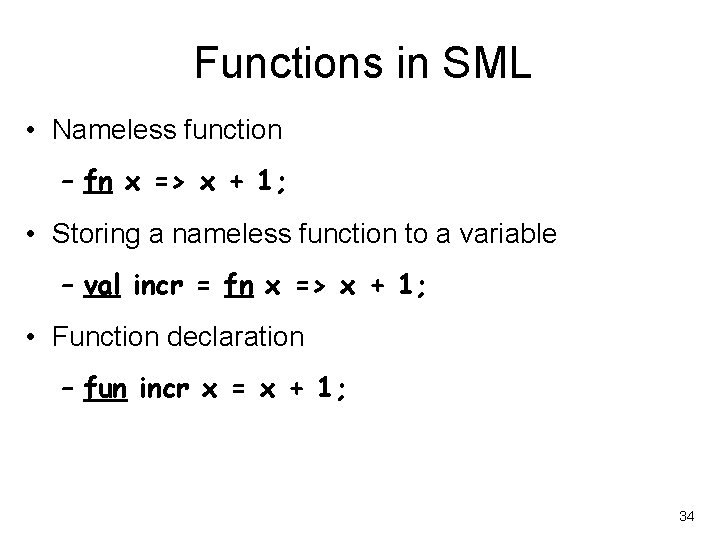
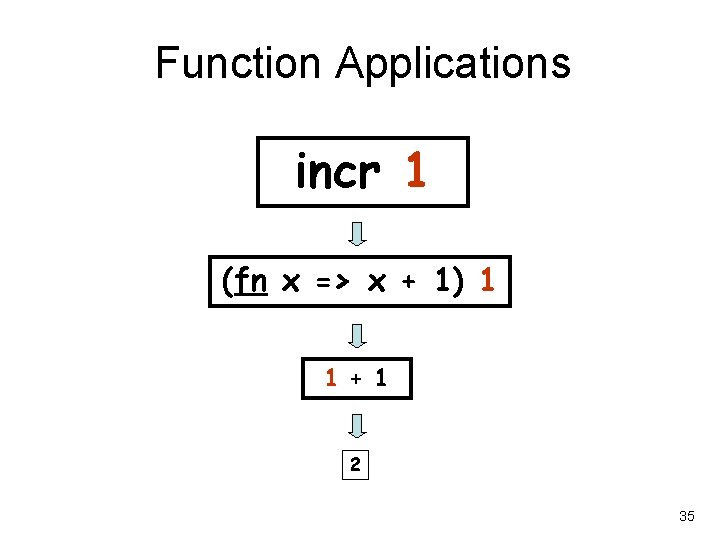
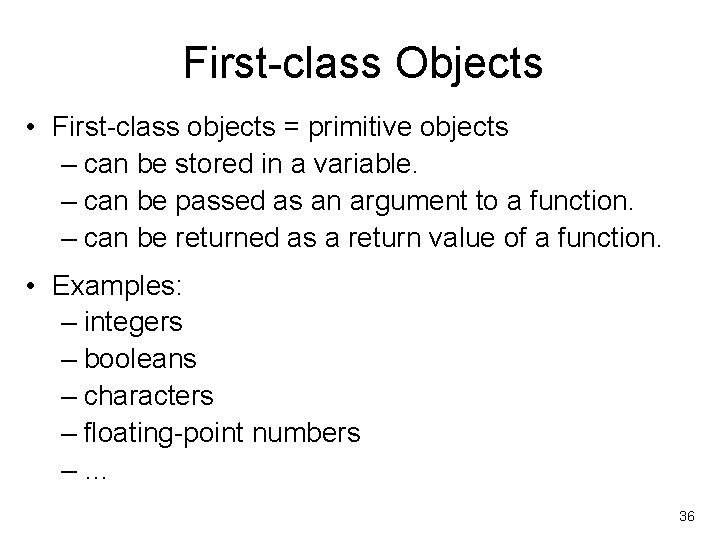
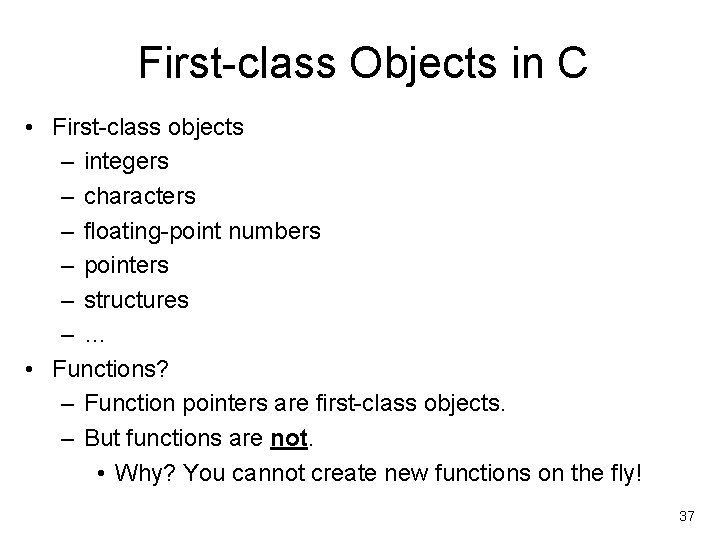
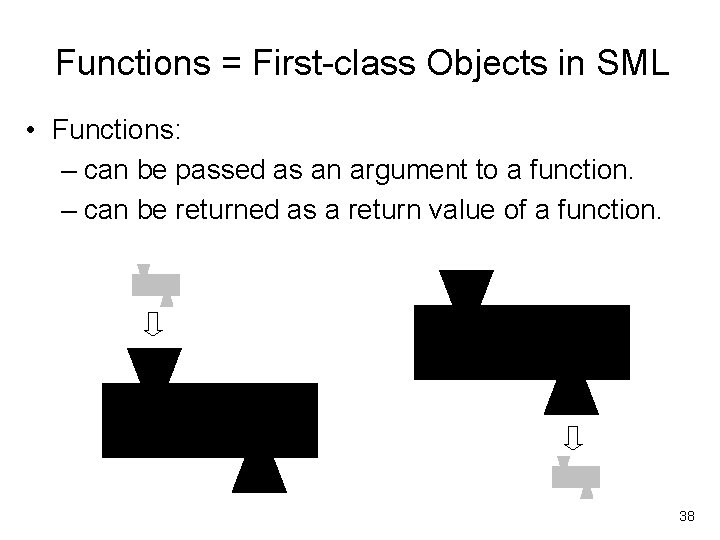
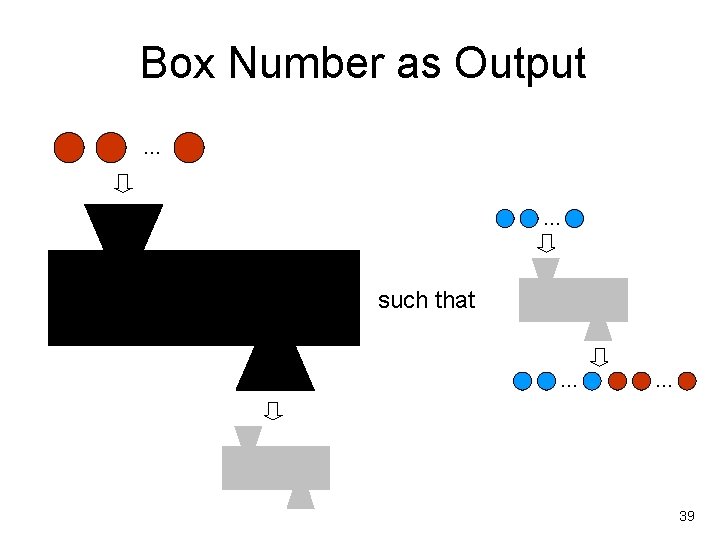
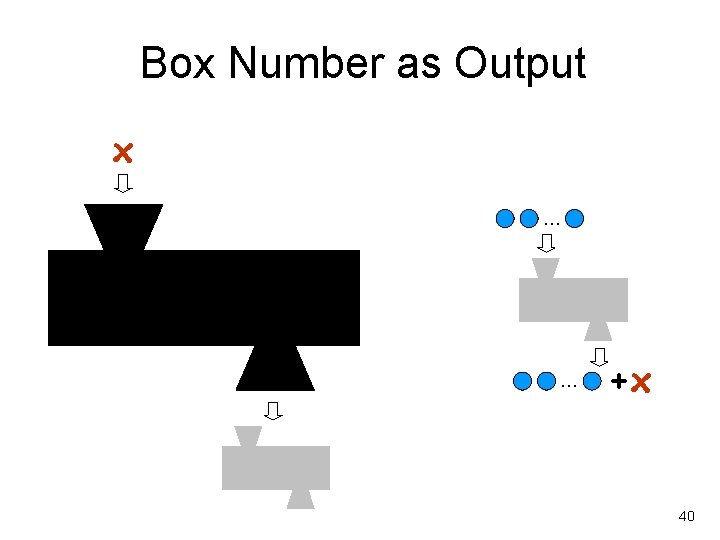
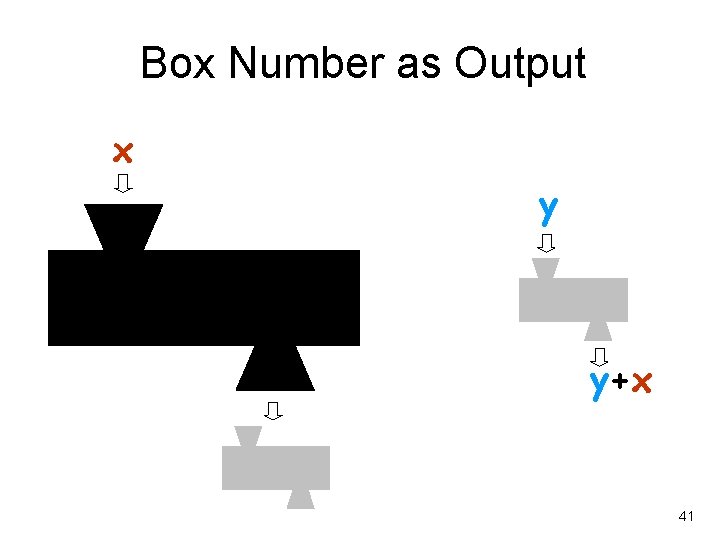
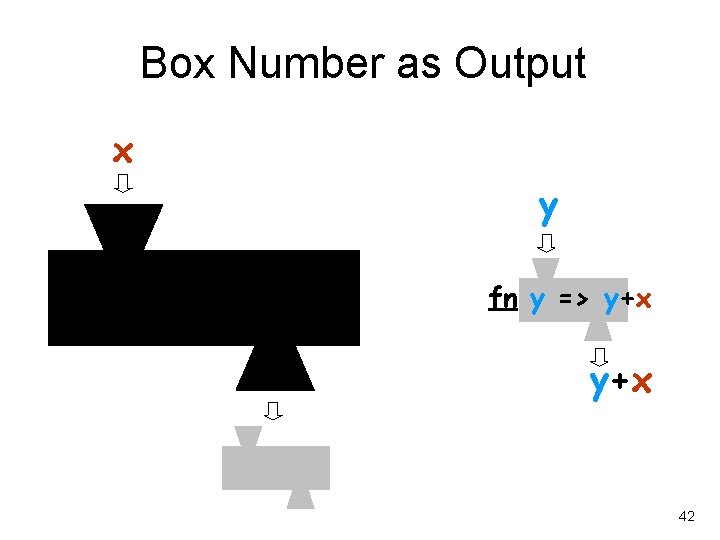
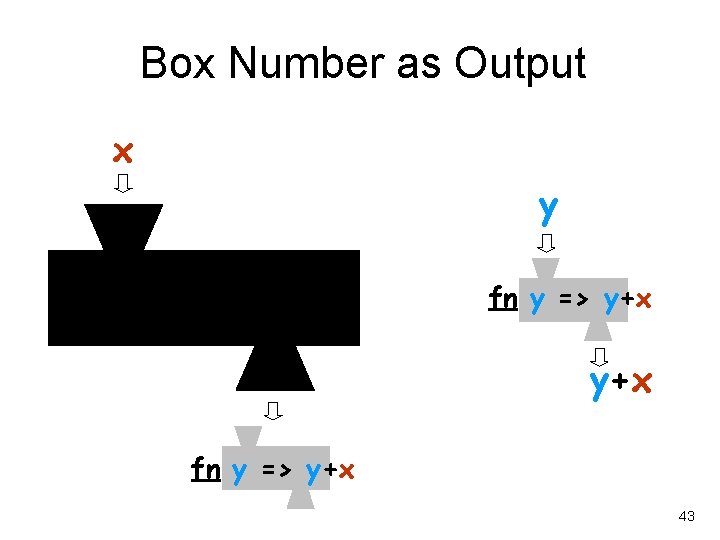
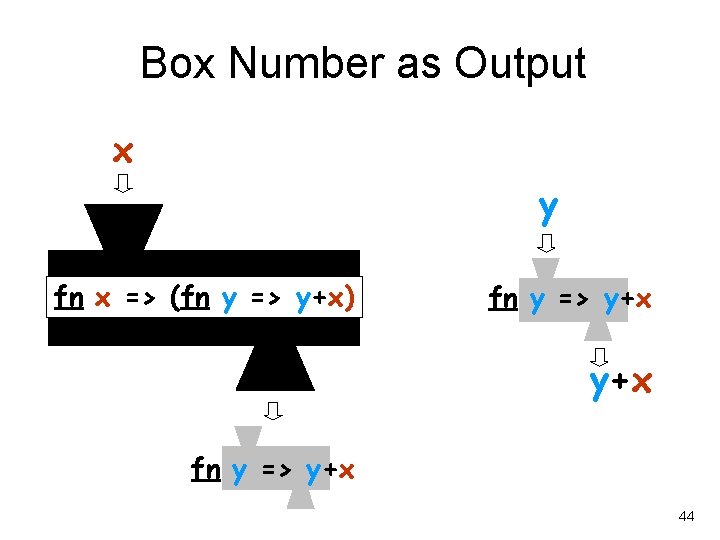
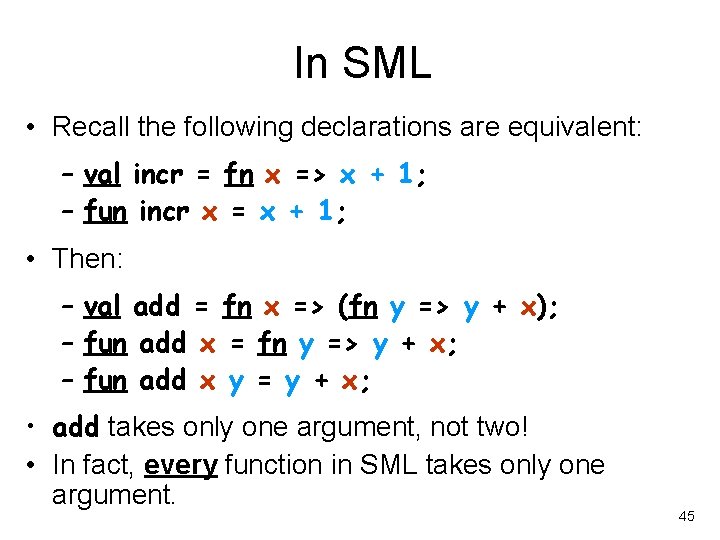
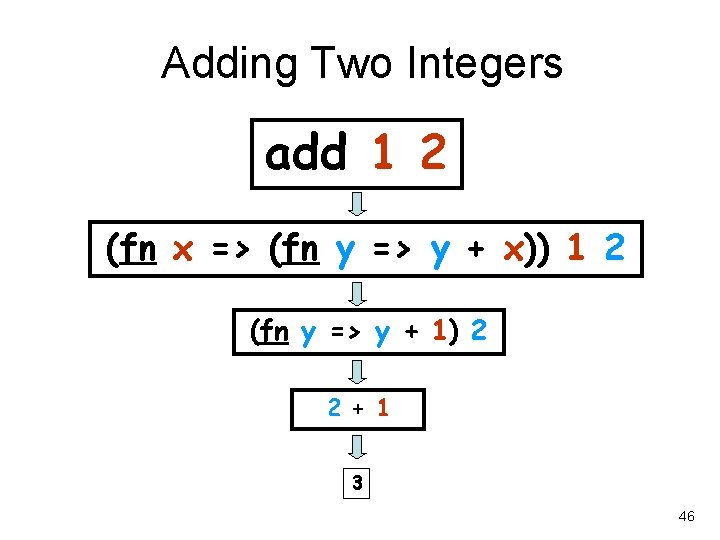
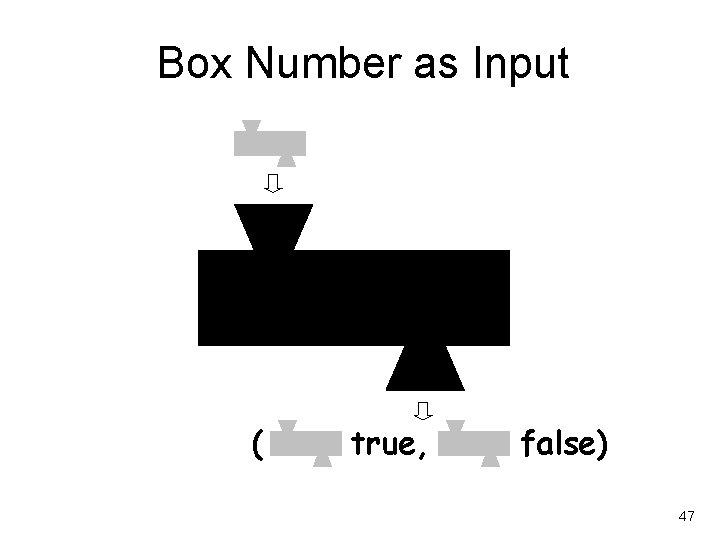
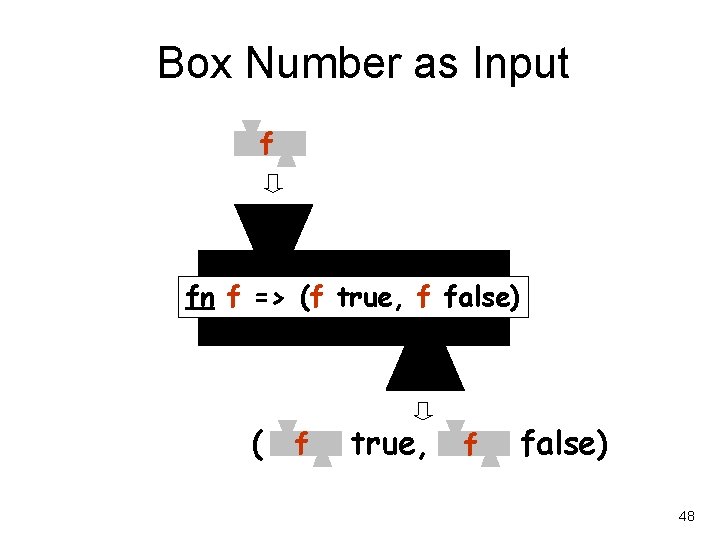
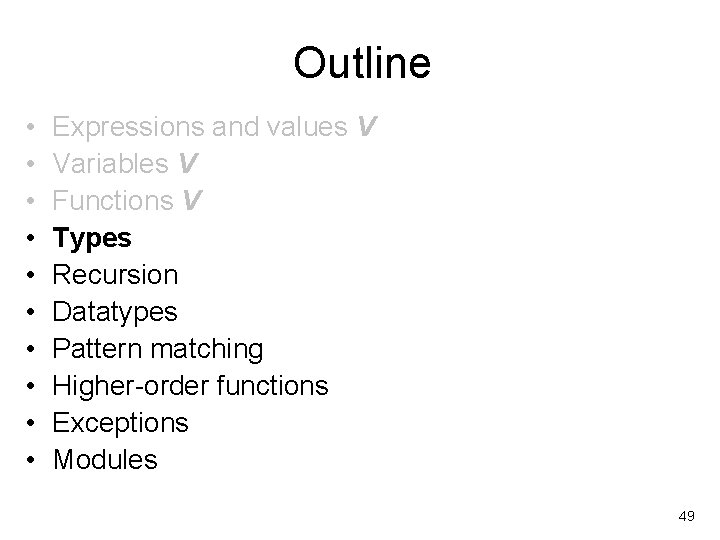
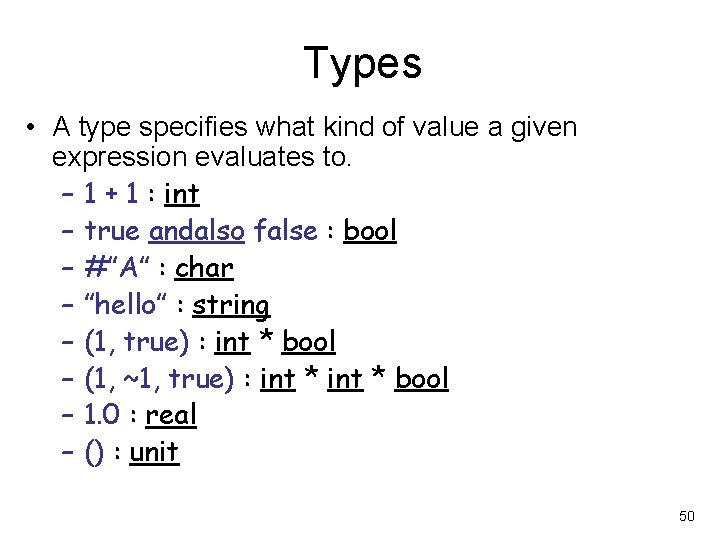
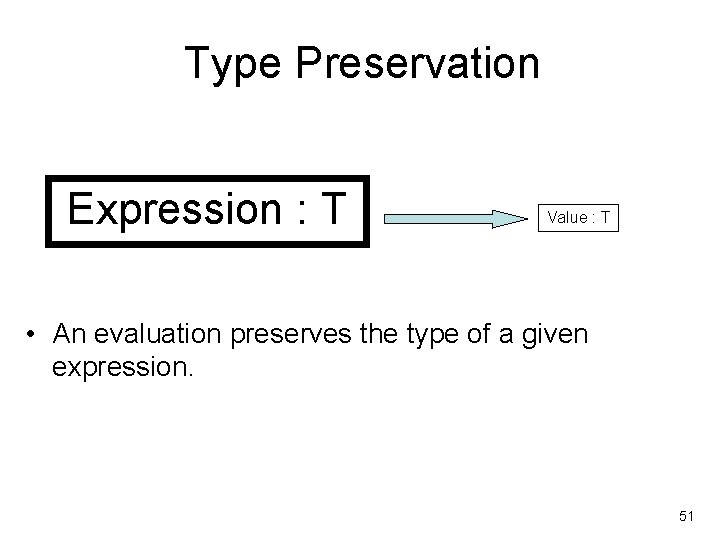
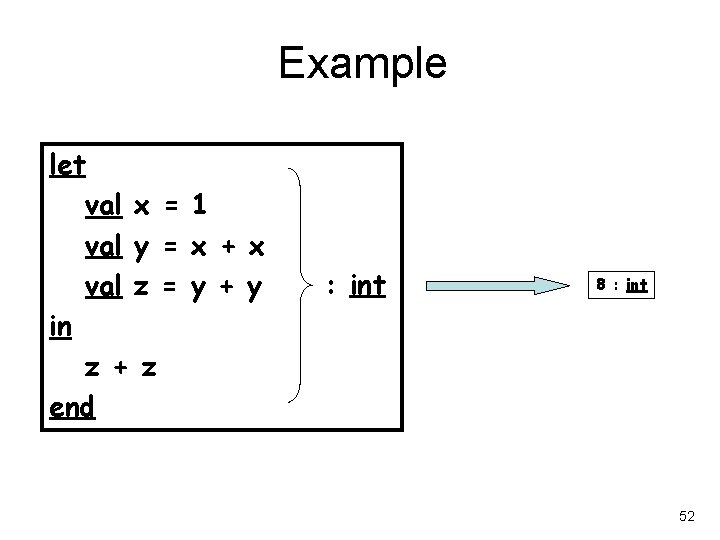
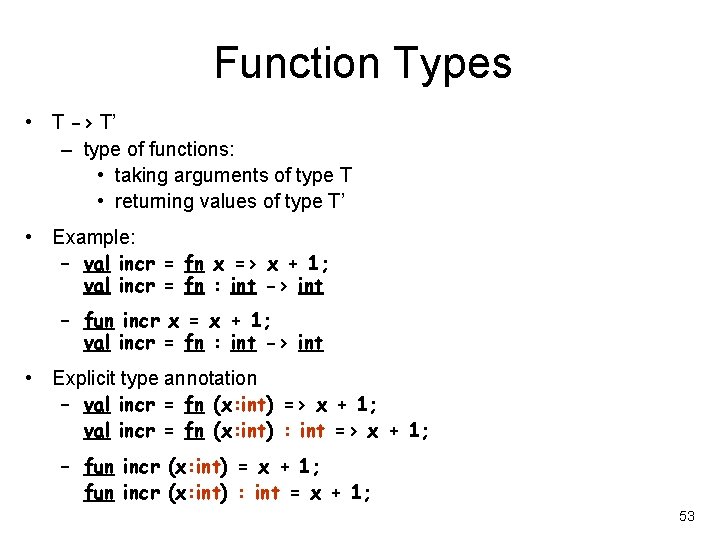
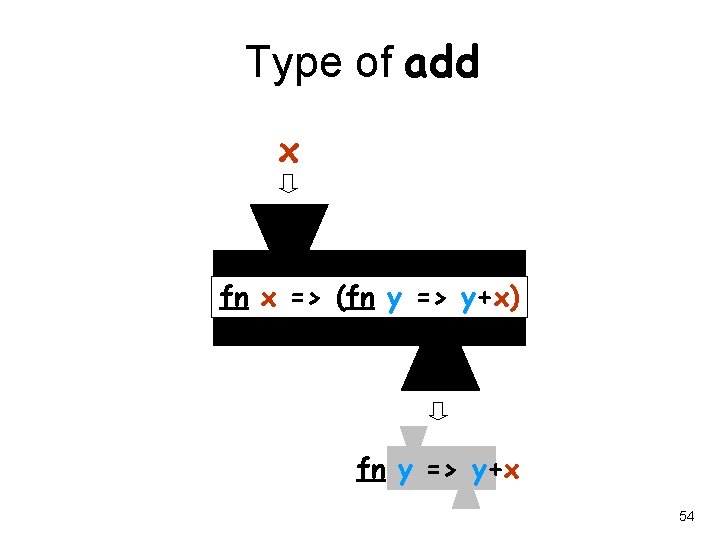
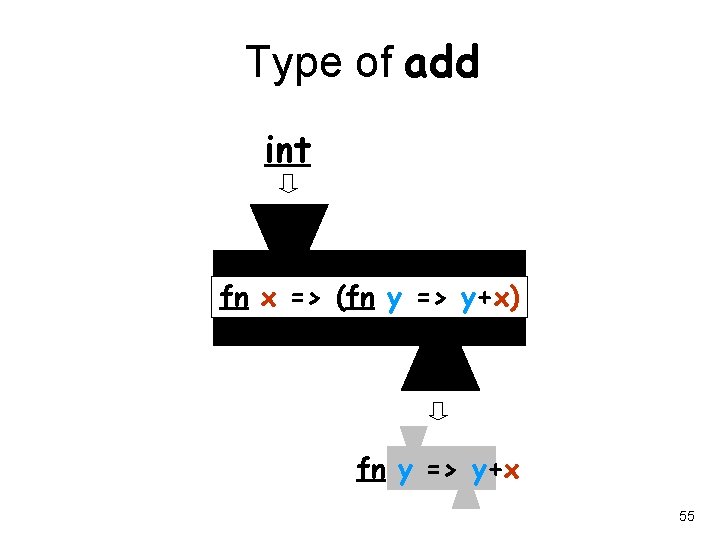
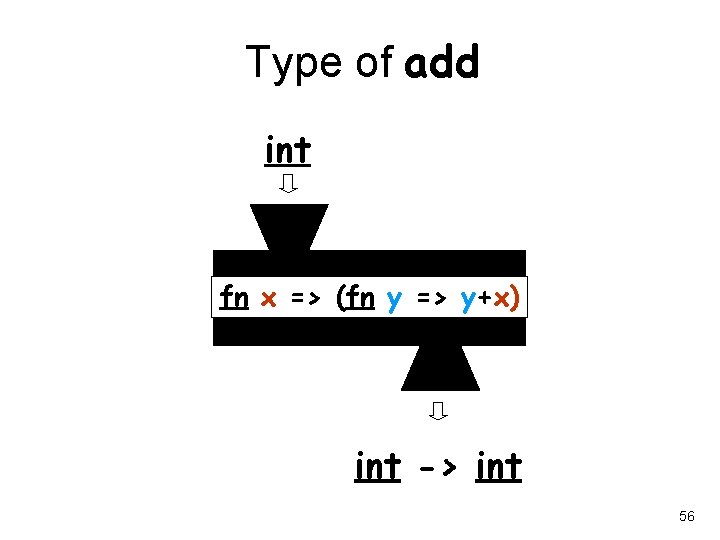
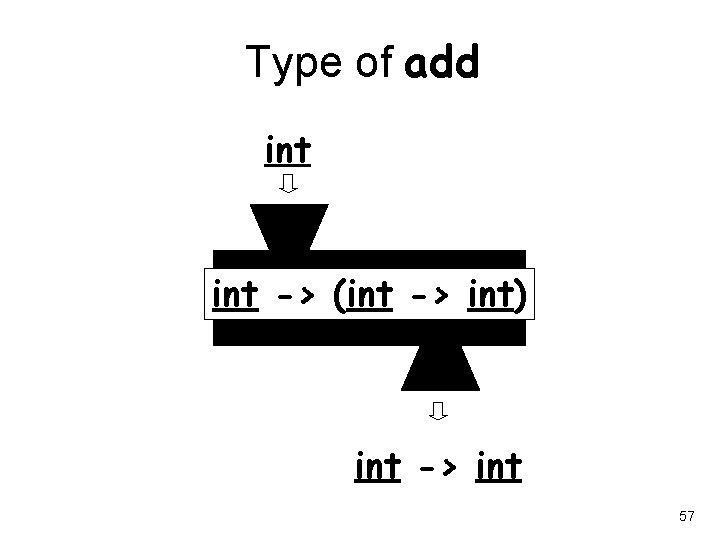
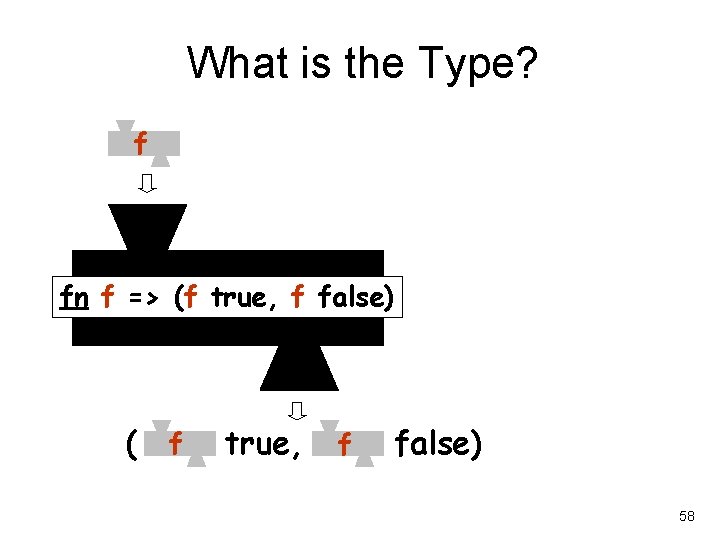
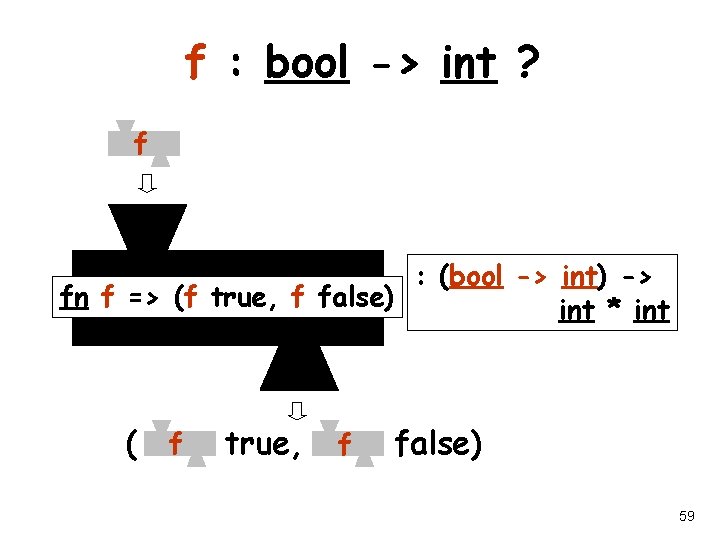
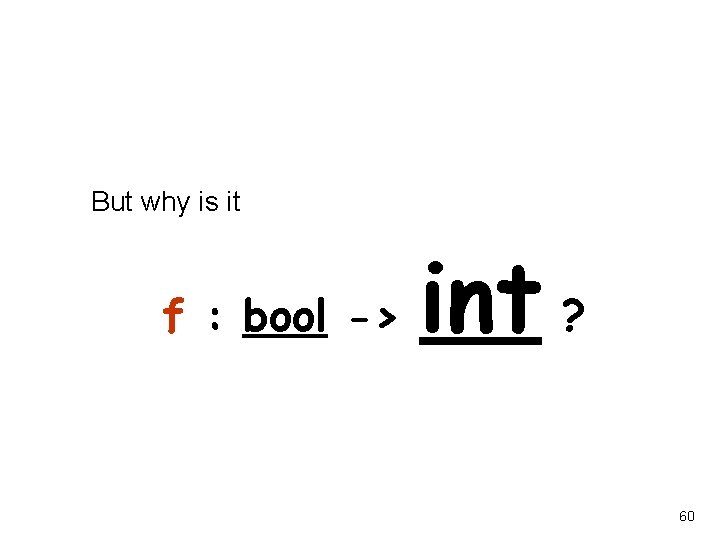
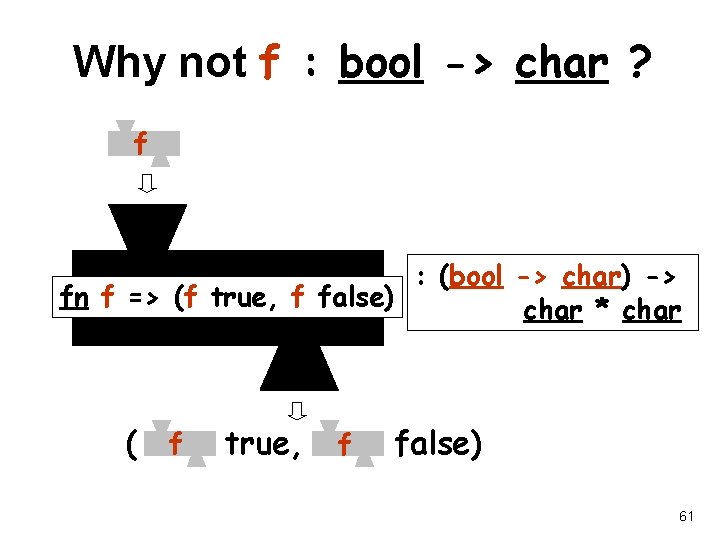
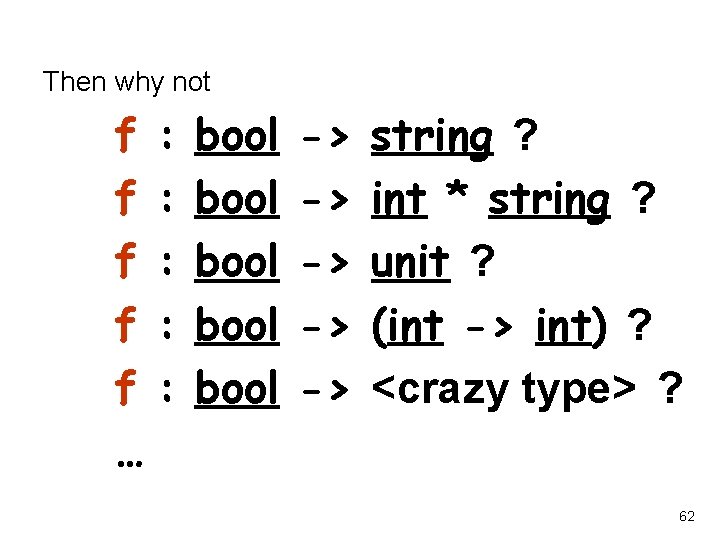
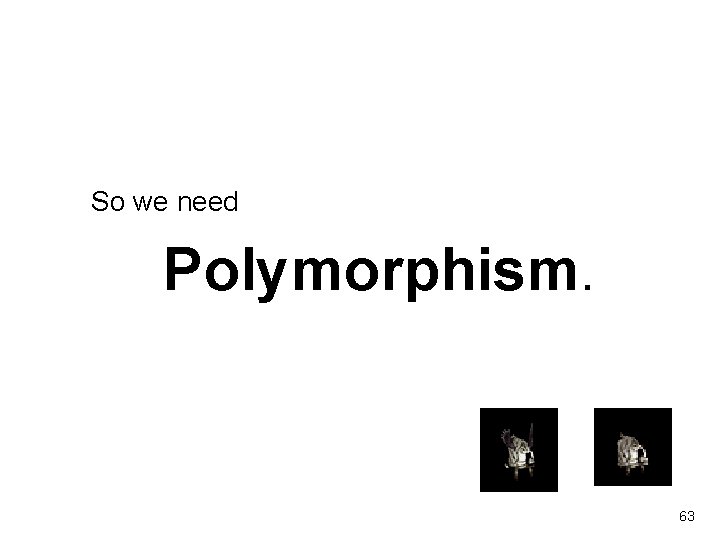
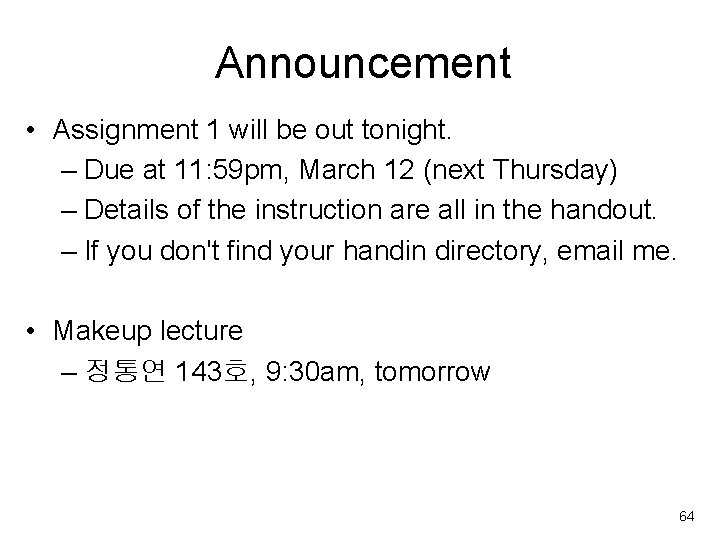
- Slides: 62
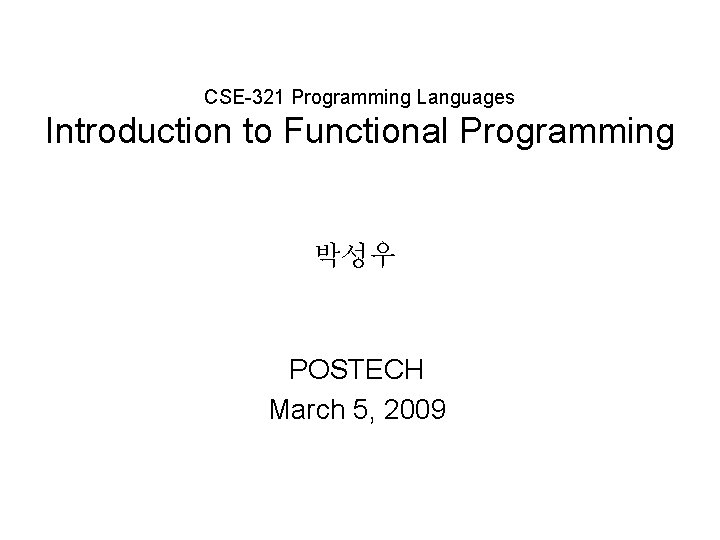
CSE-321 Programming Languages Introduction to Functional Programming 박성우 POSTECH March 5, 2009
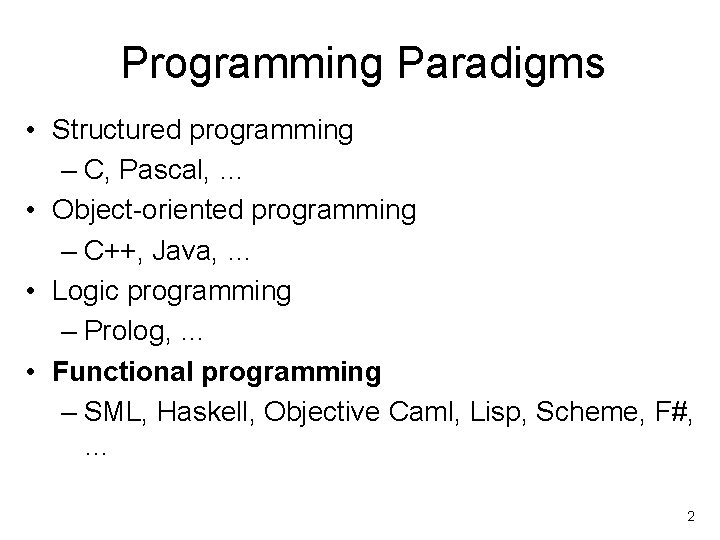
Programming Paradigms • Structured programming – C, Pascal, … • Object-oriented programming – C++, Java, … • Logic programming – Prolog, … • Functional programming – SML, Haskell, Objective Caml, Lisp, Scheme, F#, … 2
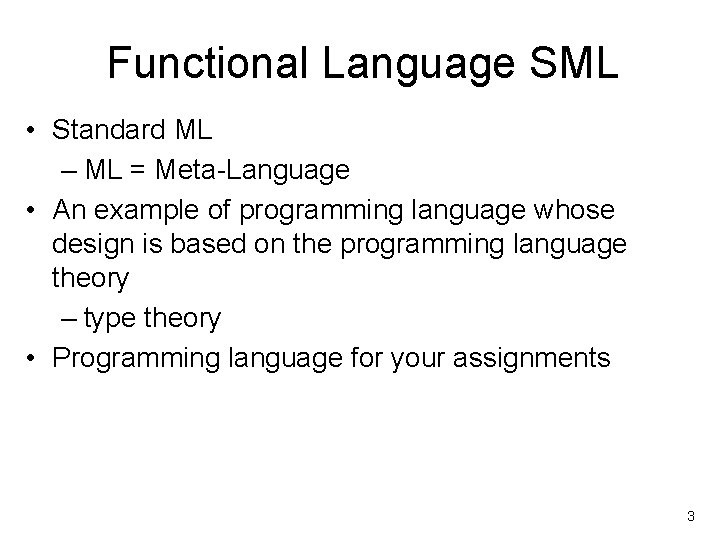
Functional Language SML • Standard ML – ML = Meta-Language • An example of programming language whose design is based on the programming language theory – type theory • Programming language for your assignments 3
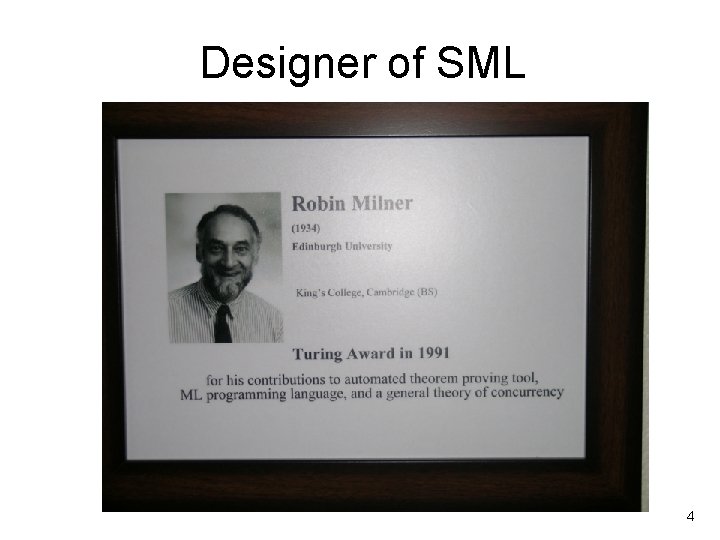
Designer of SML 4
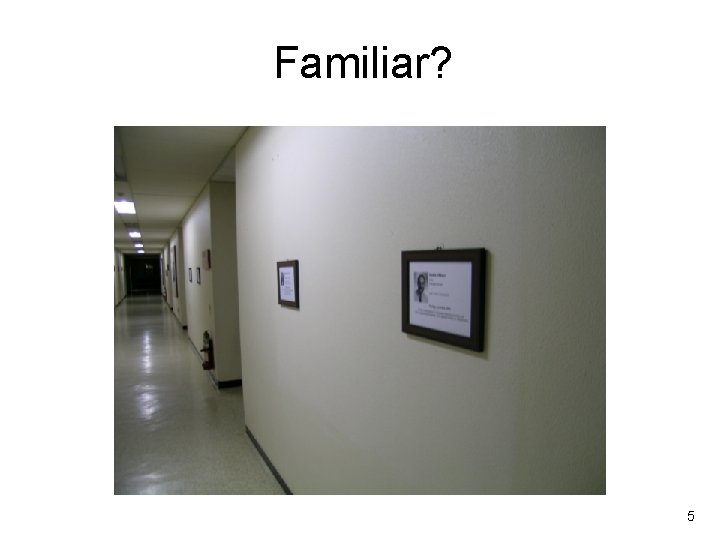
Familiar? 5
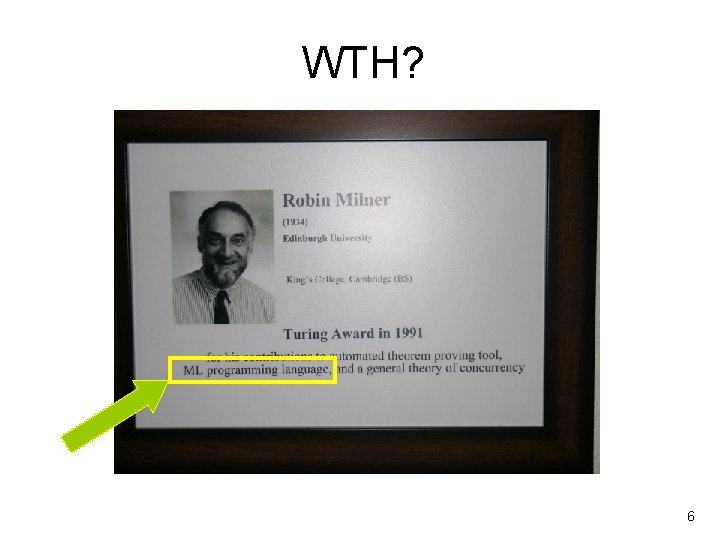
WTH? 6
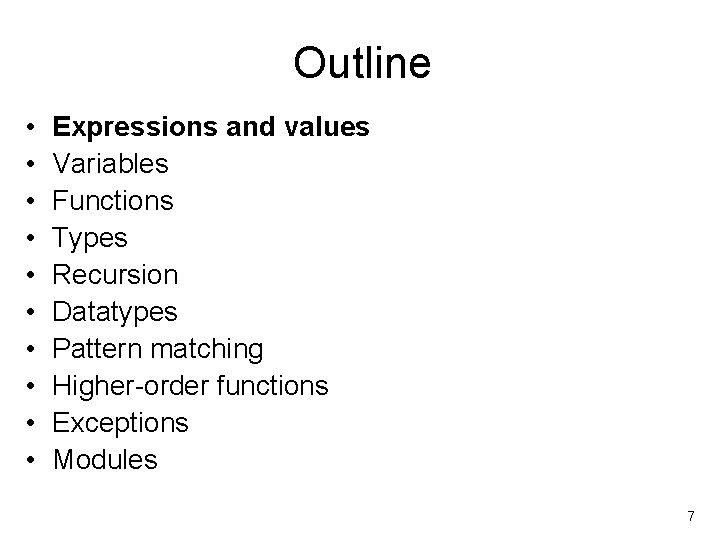
Outline • • • Expressions and values Variables Functions Types Recursion Datatypes Pattern matching Higher-order functions Exceptions Modules 7
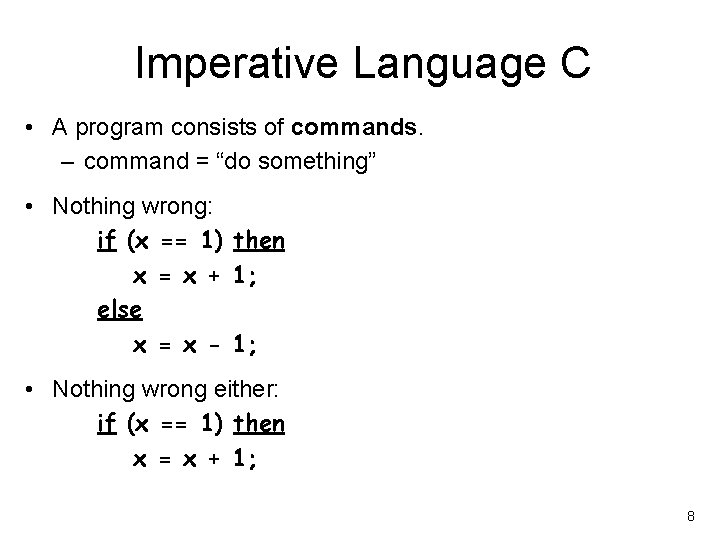
Imperative Language C • A program consists of commands. – command = “do something” • Nothing wrong: if (x == 1) then x = x + 1; else x = x - 1; • Nothing wrong either: if (x == 1) then x = x + 1; 8
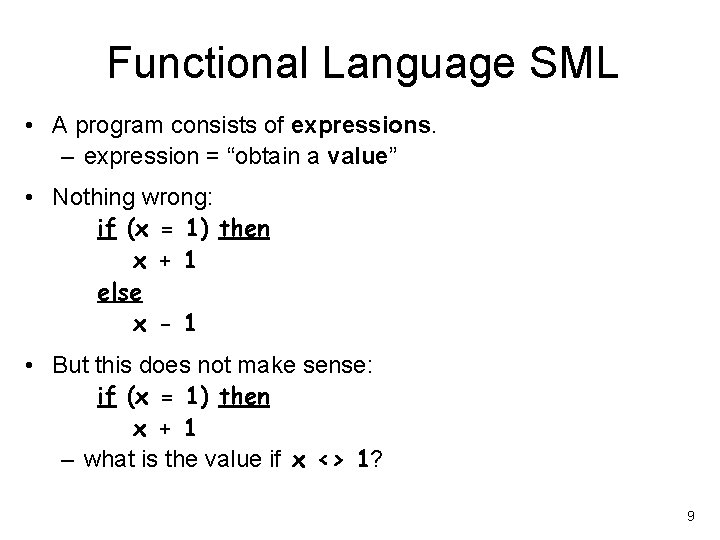
Functional Language SML • A program consists of expressions. – expression = “obtain a value” • Nothing wrong: if (x = 1) then x + 1 else x - 1 • But this does not make sense: if (x = 1) then x + 1 – what is the value if x <> 1? 9
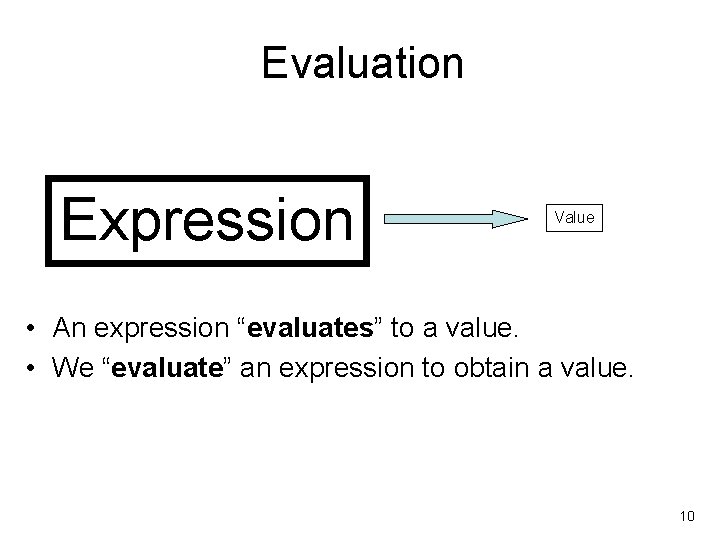
Evaluation Expression Value • An expression “evaluates” to a value. • We “evaluate” an expression to obtain a value. 10
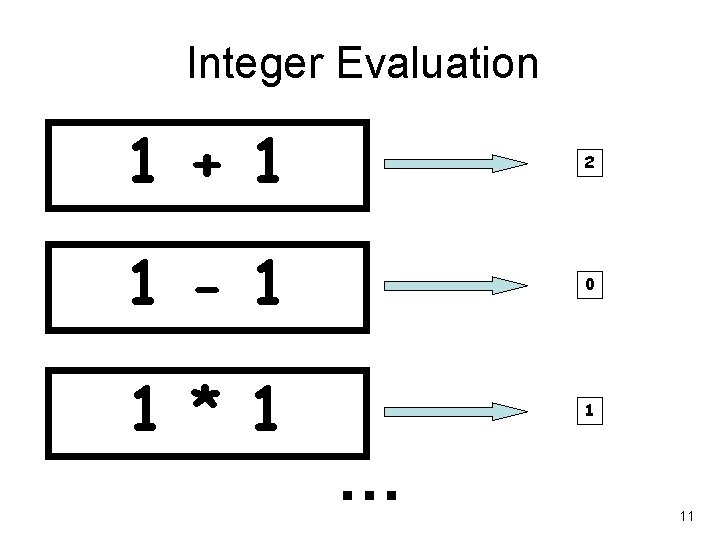
Integer Evaluation 1 + 1 2 1 - 1 0 1 * 1 1 … 11
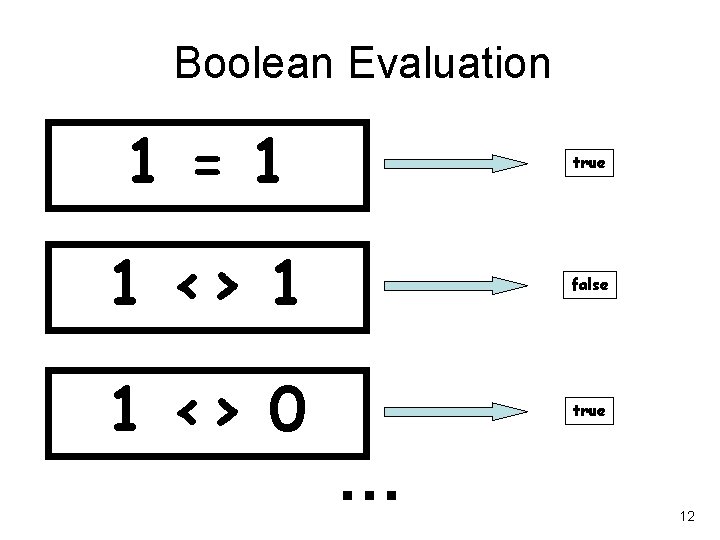
Boolean Evaluation 1 = 1 true 1 <> 1 false 1 <> 0 true … 12
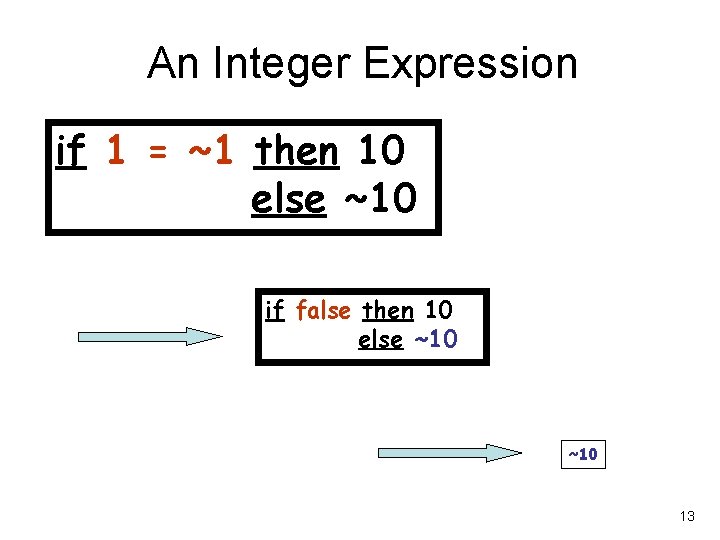
An Integer Expression if 1 = ~1 then 10 else ~10 if false then 10 else ~10 13
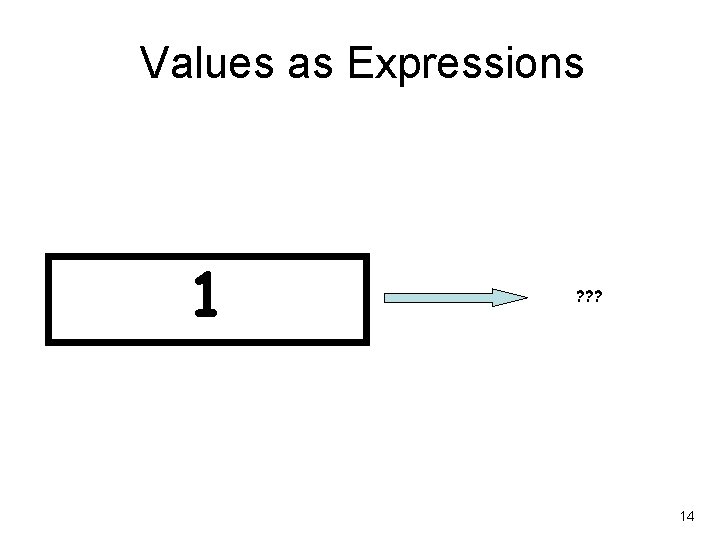
Values as Expressions 1 ? ? ? 14
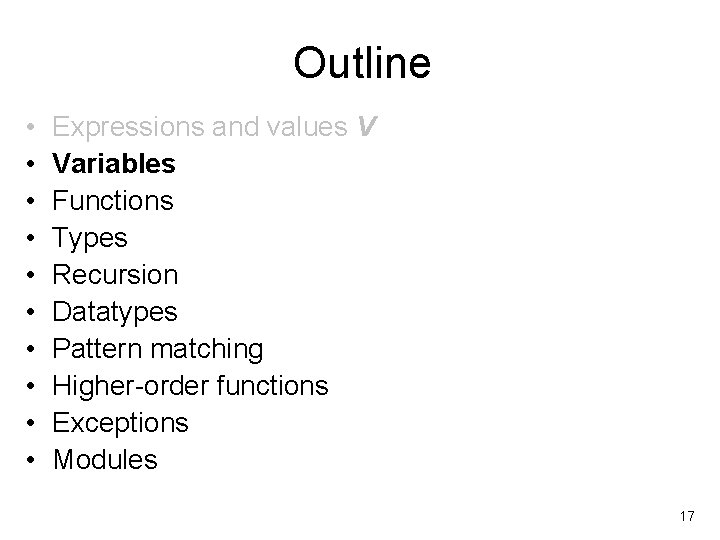
Outline • • • Expressions and values V Variables Functions Types Recursion Datatypes Pattern matching Higher-order functions Exceptions Modules 17
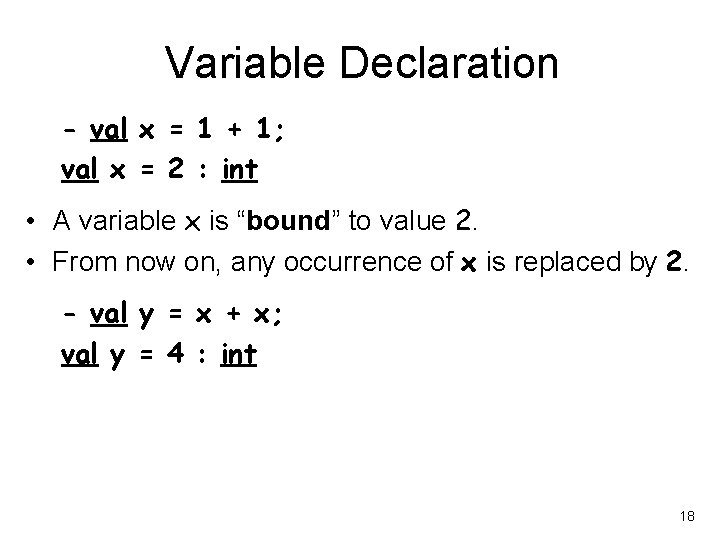
Variable Declaration - val x = 1 + 1; val x = 2 : int • A variable x is “bound” to value 2. • From now on, any occurrence of x is replaced by 2. - val y = x + x; val y = 4 : int 18
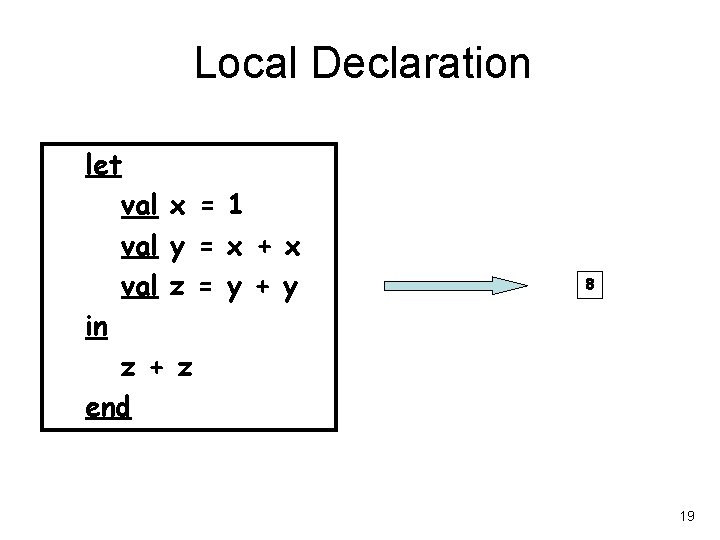
Local Declaration let val x = 1 val y = x + x val z = y + y in z + z end 8 19
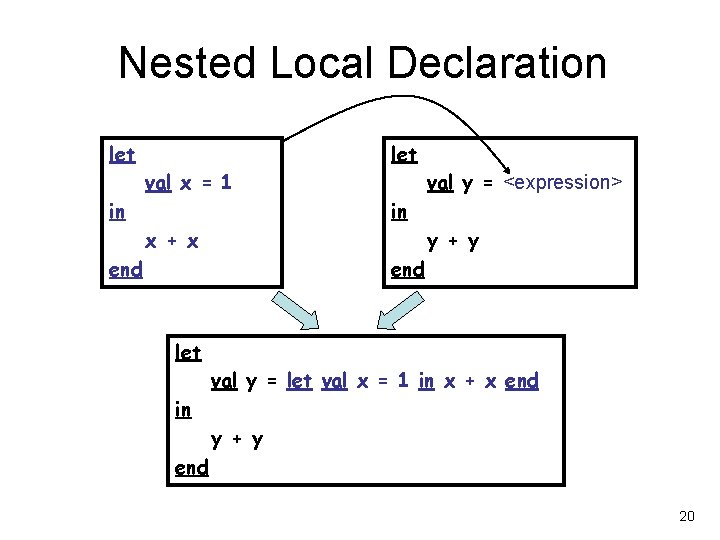
Nested Local Declaration let val x = 1 in val y = <expression> in x + x y + y end let val y = let val x = 1 in x + x end in y + y end 20
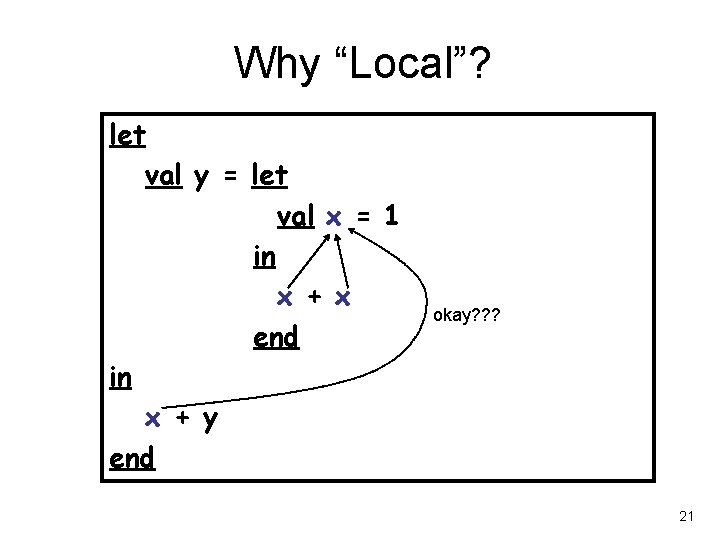
Why “Local”? let val y = let val x = 1 in x + x end in x + y end okay? ? ? 21
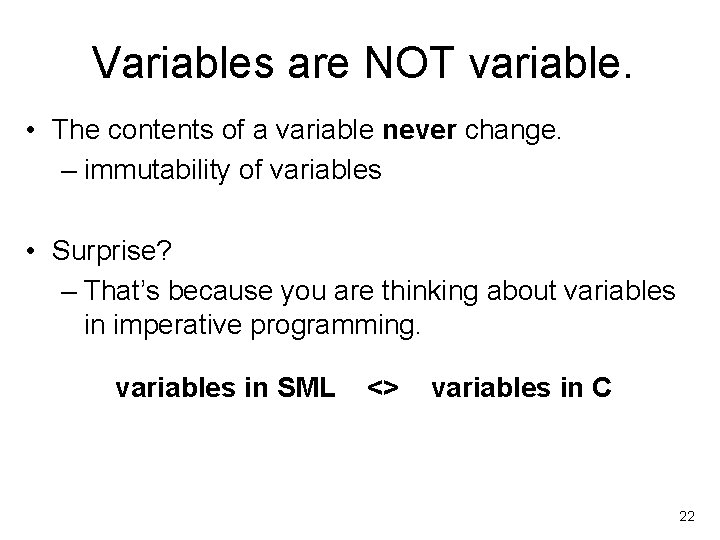
Variables are NOT variable. • The contents of a variable never change. – immutability of variables • Surprise? – That’s because you are thinking about variables in imperative programming. variables in SML <> variables in C 22
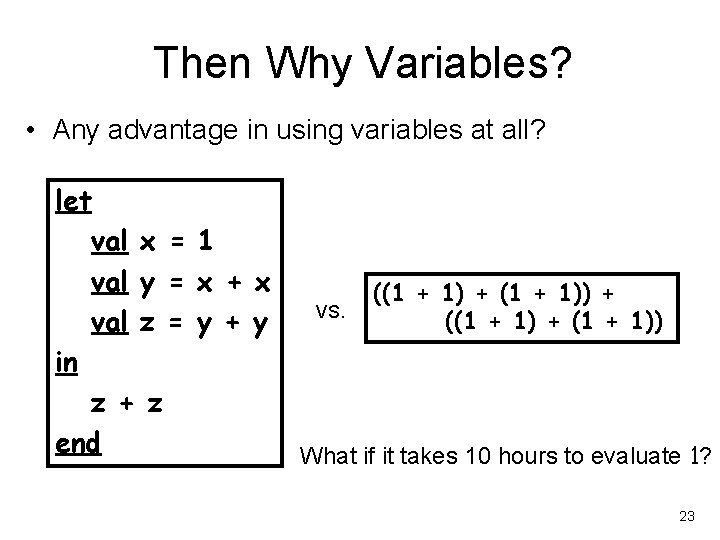
Then Why Variables? • Any advantage in using variables at all? let val x = 1 val y = x + x val z = y + y in z + z end VS. ((1 + 1) + (1 + 1)) + ((1 + 1) + (1 + 1)) What if it takes 10 hours to evaluate 1? 23
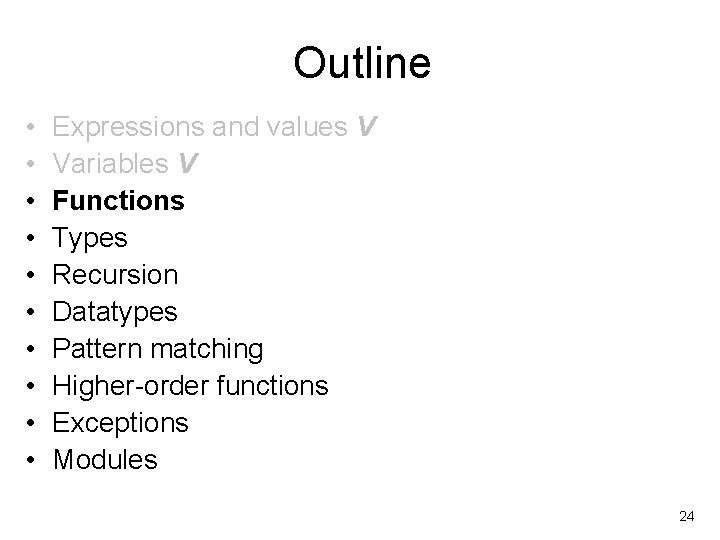
Outline • • • Expressions and values V Variables V Functions Types Recursion Datatypes Pattern matching Higher-order functions Exceptions Modules 24
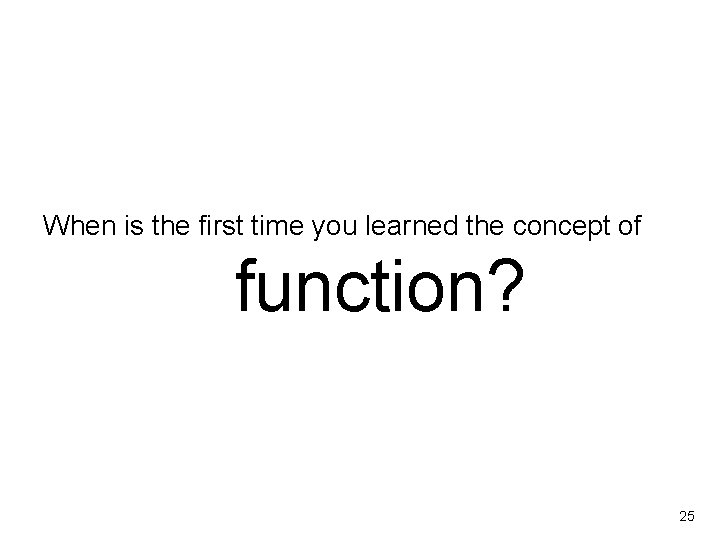
When is the first time you learned the concept of function? 25
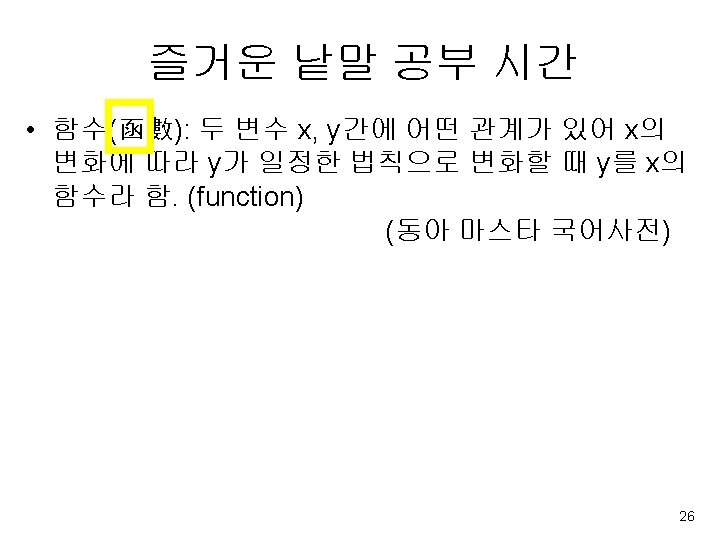
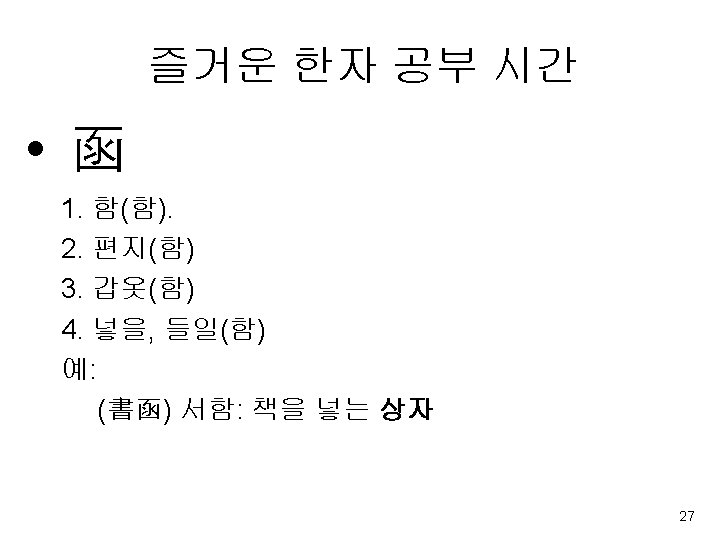
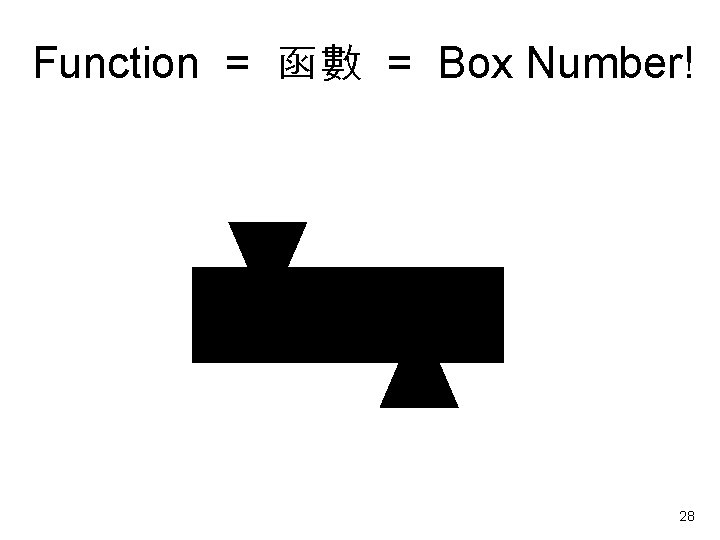
Function = 函數 = Box Number! 28
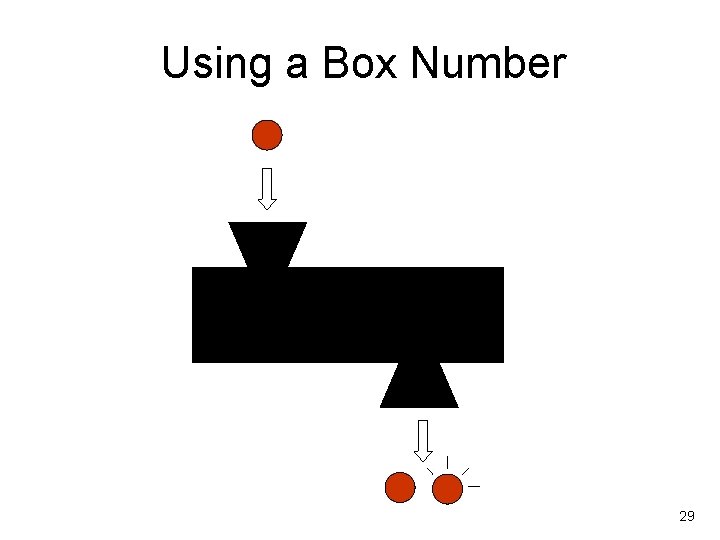
Using a Box Number 29
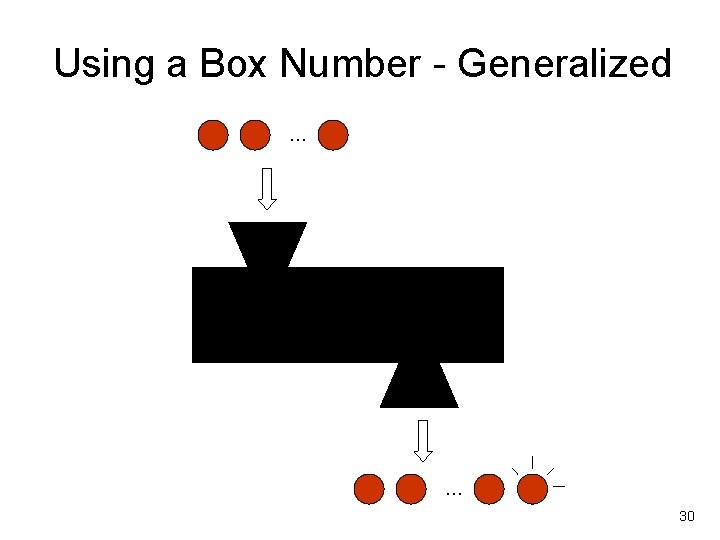
Using a Box Number - Generalized … … 30
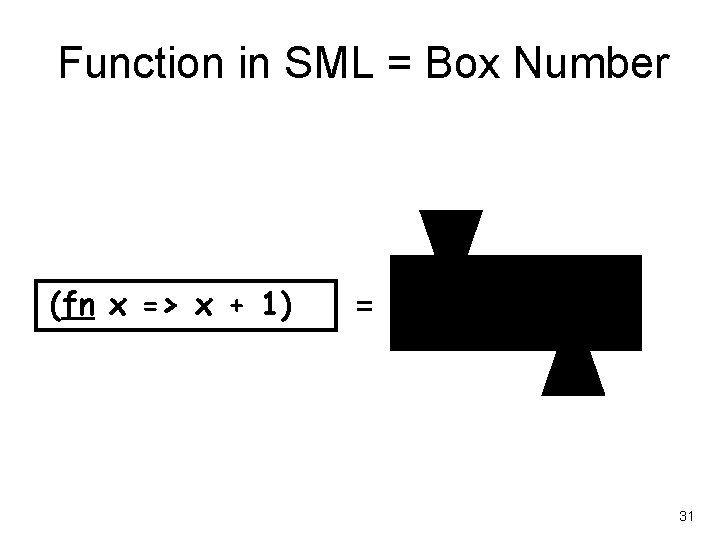
Function in SML = Box Number (fn x => x + 1) n = 31
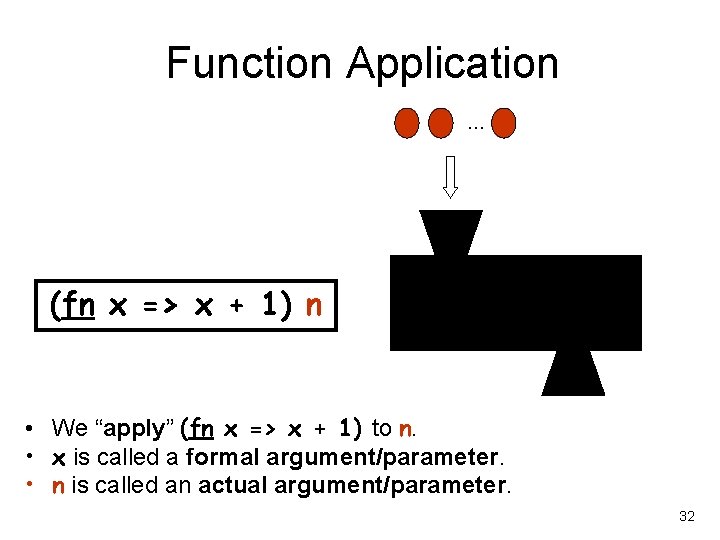
Function Application … (fn x => x + 1) n • We “apply” (fn x => x + 1) to n. • x is called a formal argument/parameter. • n is called an actual argument/parameter. 32
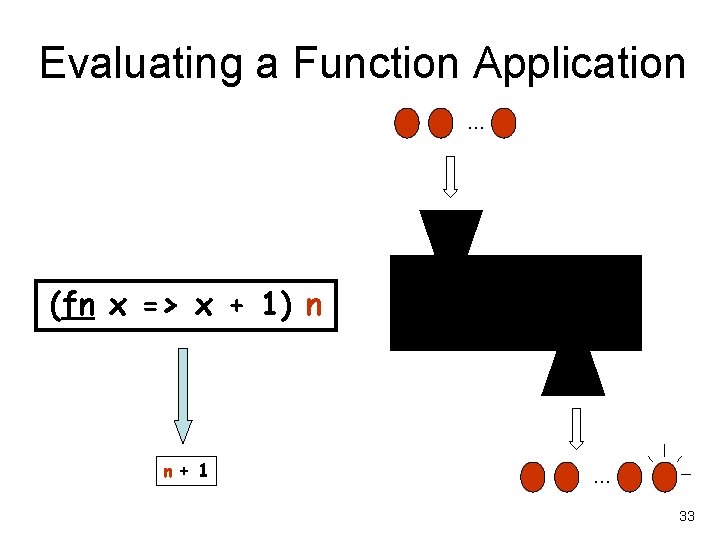
Evaluating a Function Application … (fn x => x + 1) n n+ 1 … 33
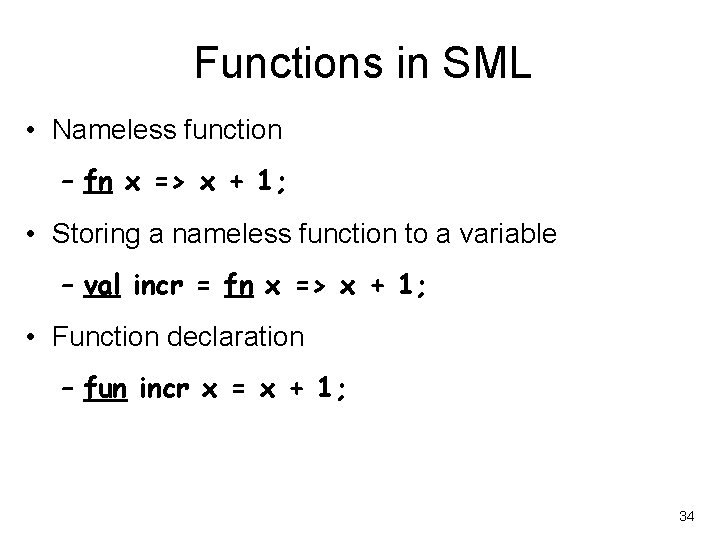
Functions in SML • Nameless function – fn x => x + 1; • Storing a nameless function to a variable – val incr = fn x => x + 1; • Function declaration – fun incr x = x + 1; 34
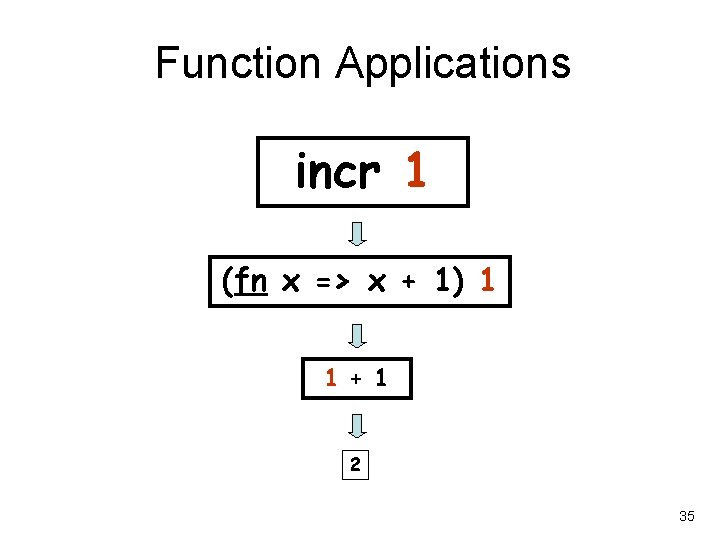
Function Applications incr 1 (fn x => x + 1) 1 1 + 1 2 35
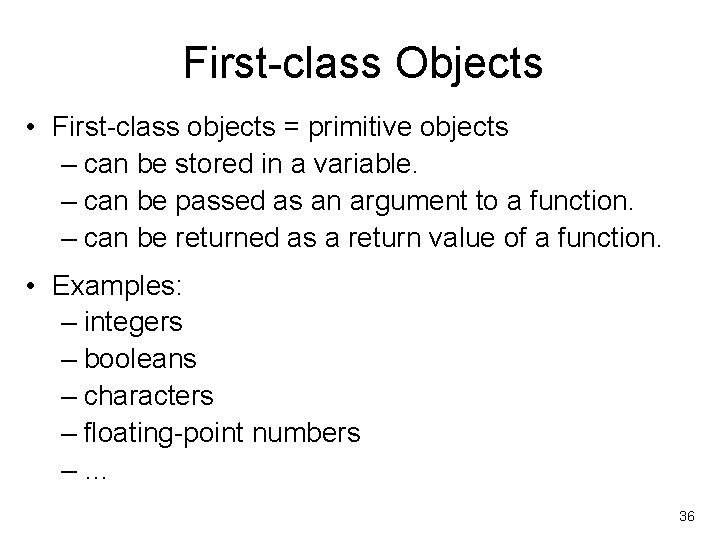
First-class Objects • First-class objects = primitive objects – can be stored in a variable. – can be passed as an argument to a function. – can be returned as a return value of a function. • Examples: – integers – booleans – characters – floating-point numbers –… 36
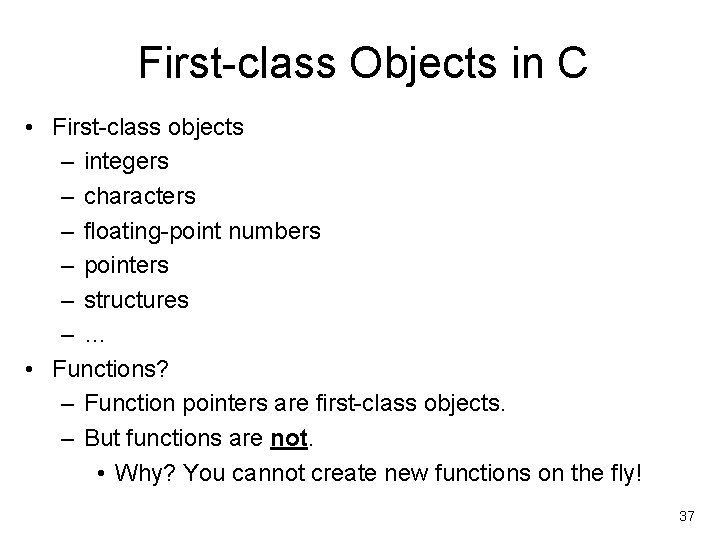
First-class Objects in C • First-class objects – integers – characters – floating-point numbers – pointers – structures –… • Functions? – Function pointers are first-class objects. – But functions are not. • Why? You cannot create new functions on the fly! 37
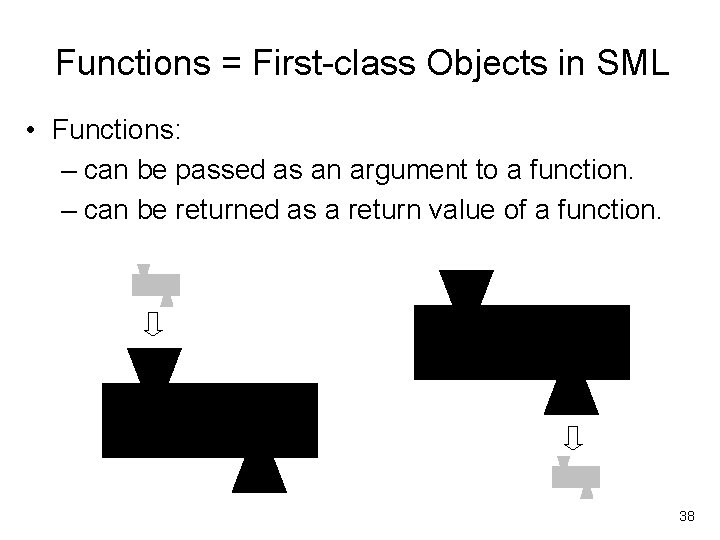
Functions = First-class Objects in SML • Functions: – can be passed as an argument to a function. – can be returned as a return value of a function. 38
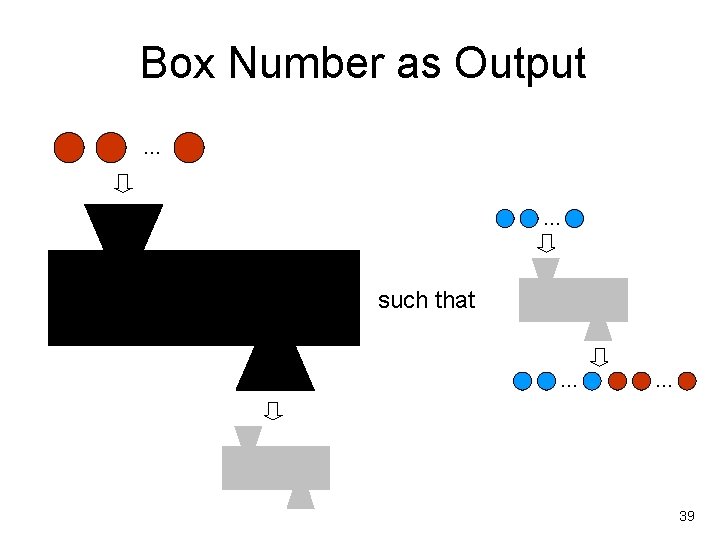
Box Number as Output … … such that … … 39
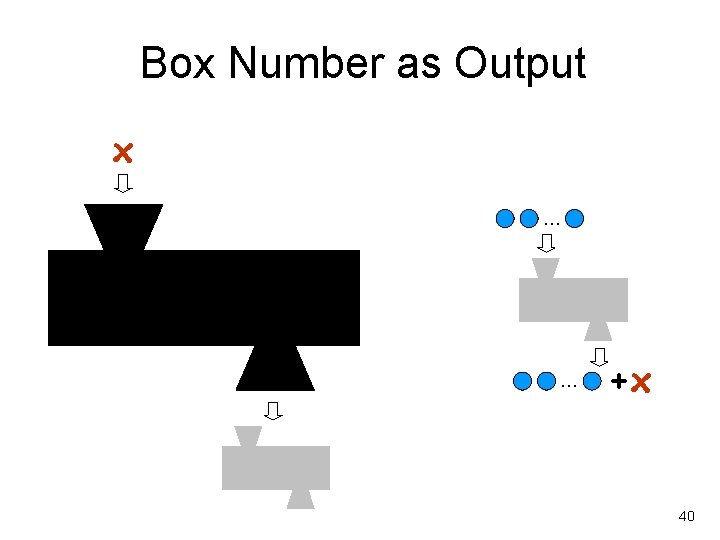
Box Number as Output x … … +x 40
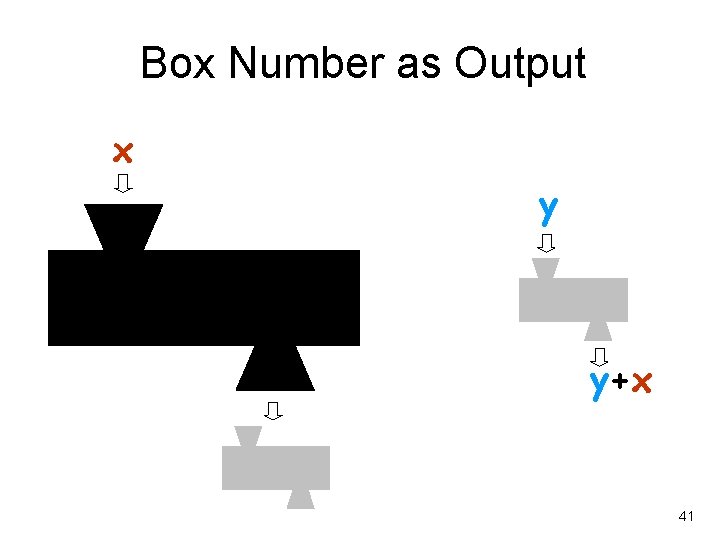
Box Number as Output x y y+x 41
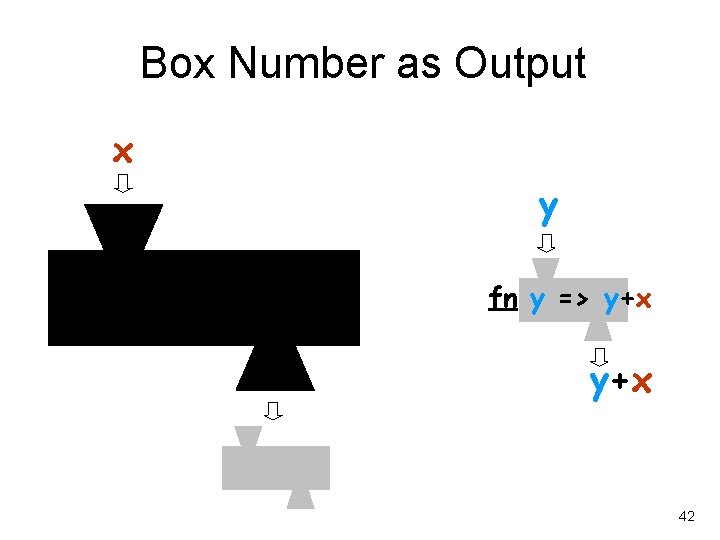
Box Number as Output x y fn y => y+x 42
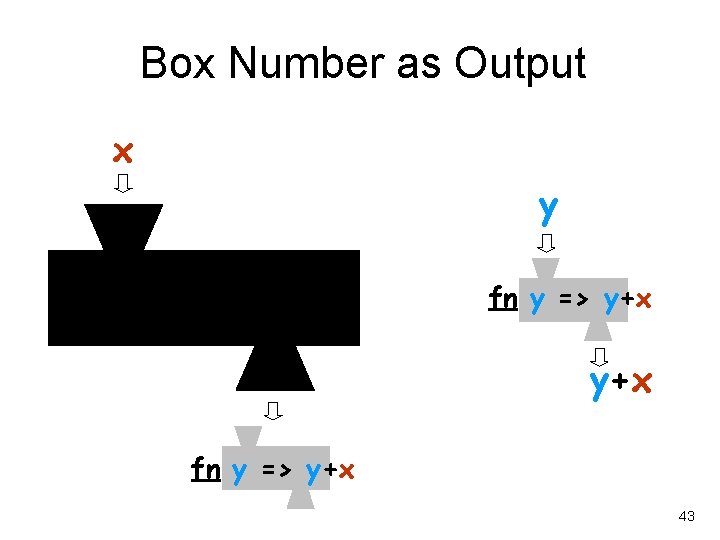
Box Number as Output x y fn y => y+x 43
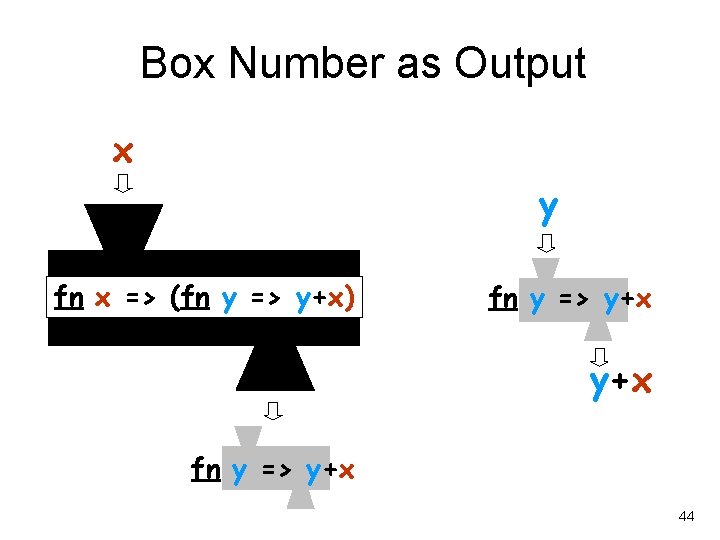
Box Number as Output x y fn x => (fn y => y+x) fn y => y+x 44
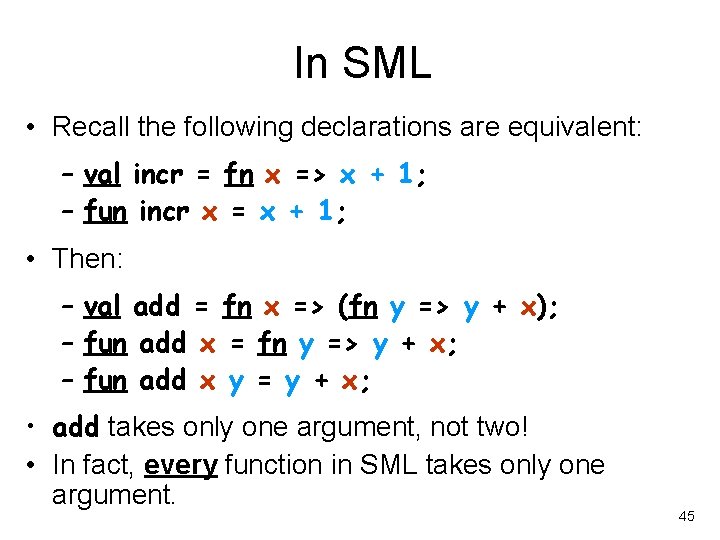
In SML • Recall the following declarations are equivalent: – val incr = fn x => x + 1; – fun incr x = x + 1; • Then: – val add = fn x => (fn y => y + x); – fun add x = fn y => y + x; – fun add x y = y + x; • add takes only one argument, not two! • In fact, every function in SML takes only one argument. 45
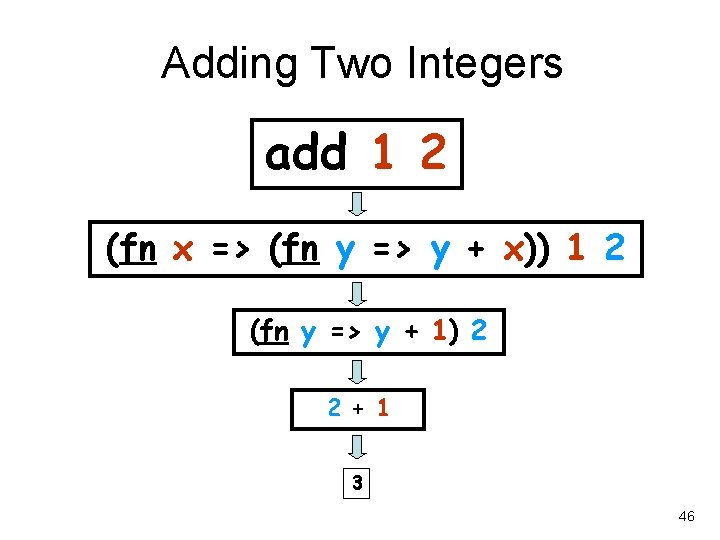
Adding Two Integers add 1 2 (fn x => (fn y => y + x)) 1 2 (fn y => y + 1) 2 2 + 1 3 46
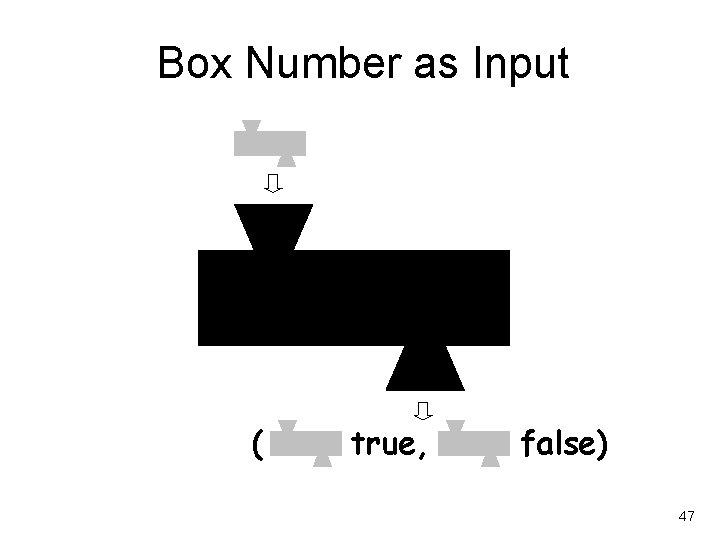
Box Number as Input ( true, false) 47
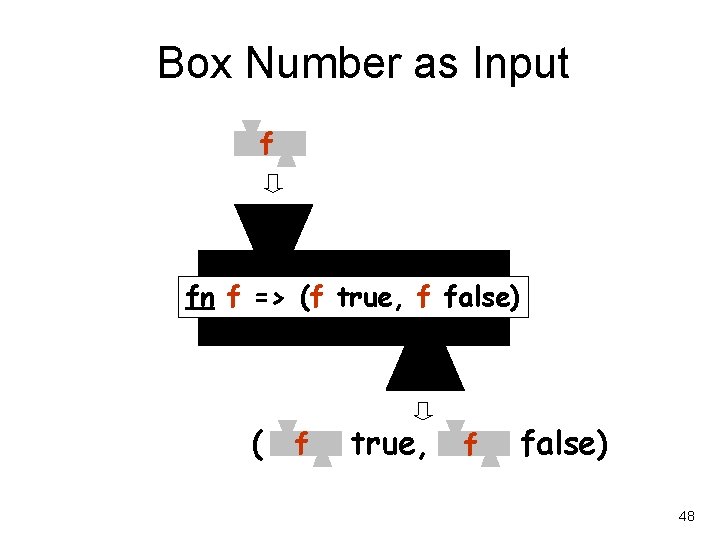
Box Number as Input f fn f => (f true, f false) ( f true, f false) 48
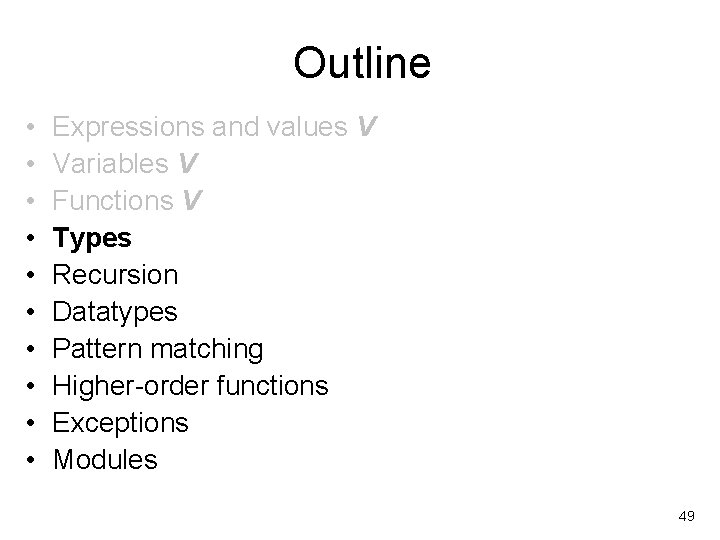
Outline • • • Expressions and values V Variables V Functions V Types Recursion Datatypes Pattern matching Higher-order functions Exceptions Modules 49
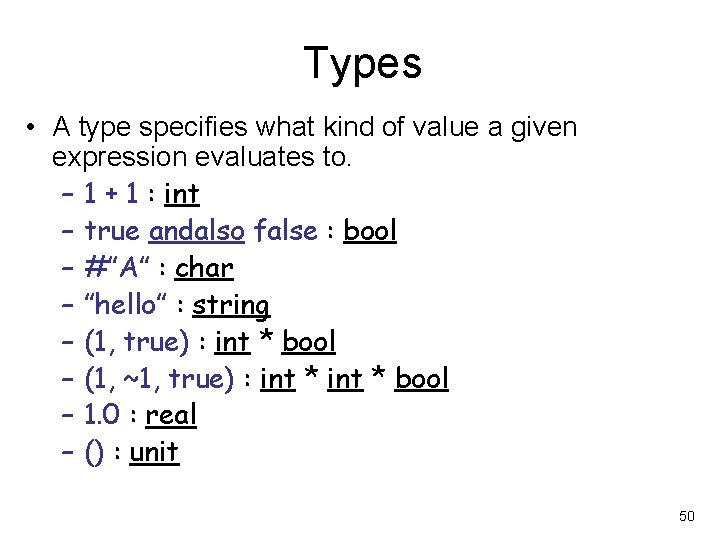
Types • A type specifies what kind of value a given expression evaluates to. – 1 + 1 : int – true andalso false : bool – #”A” : char – ”hello” : string – (1, true) : int * bool – (1, ~1, true) : int * bool – 1. 0 : real – () : unit 50
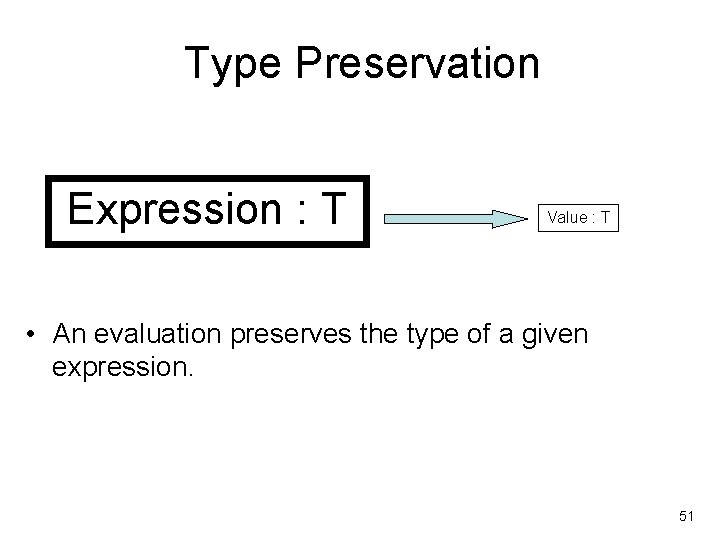
Type Preservation Expression : T Value : T • An evaluation preserves the type of a given expression. 51
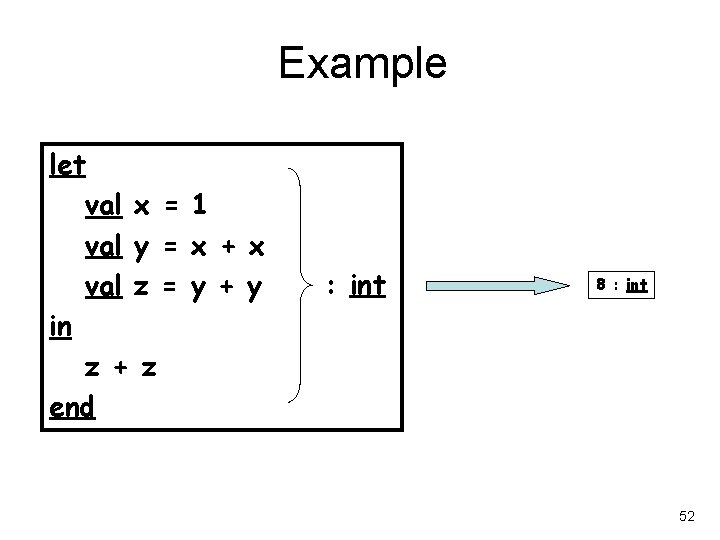
Example let val x = 1 val y = x + x val z = y + y in z + z end : int 8 : int 52
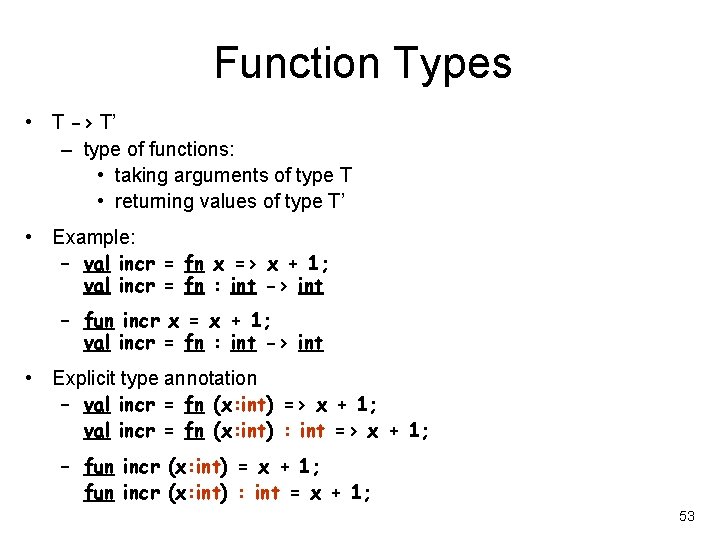
Function Types • T -> T’ – type of functions: • taking arguments of type T • returning values of type T’ • Example: – val incr = fn x => x + 1; val incr = fn : int -> int – fun incr x = x + 1; val incr = fn : int -> int • Explicit type annotation – val incr = fn (x: int) => x + 1; val incr = fn (x: int) : int => x + 1; – fun incr (x: int) = x + 1; fun incr (x: int) : int = x + 1; 53
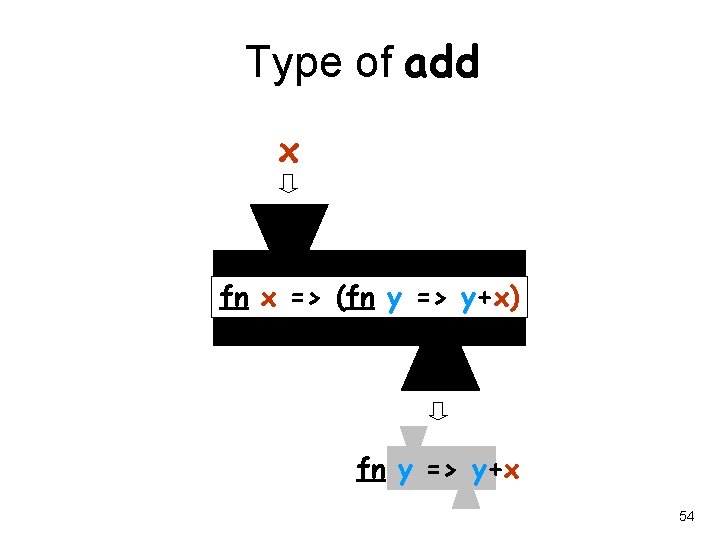
Type of add x fn x => (fn y => y+x) fn y => y+x 54
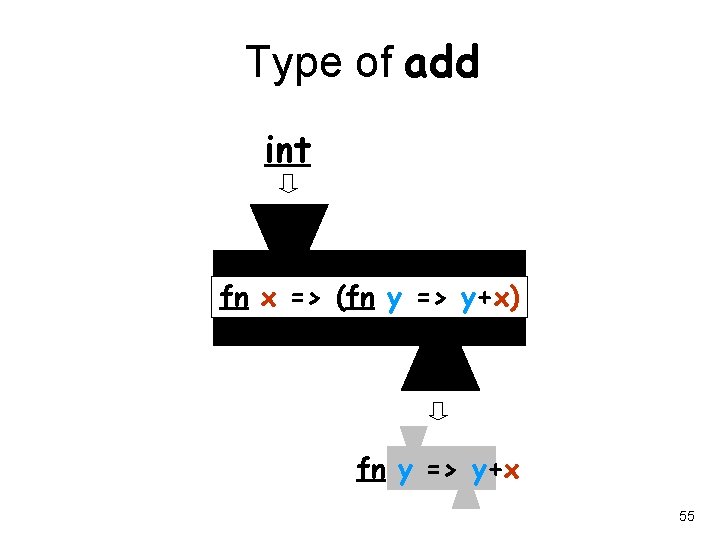
Type of add int fn x => (fn y => y+x) fn y => y+x 55
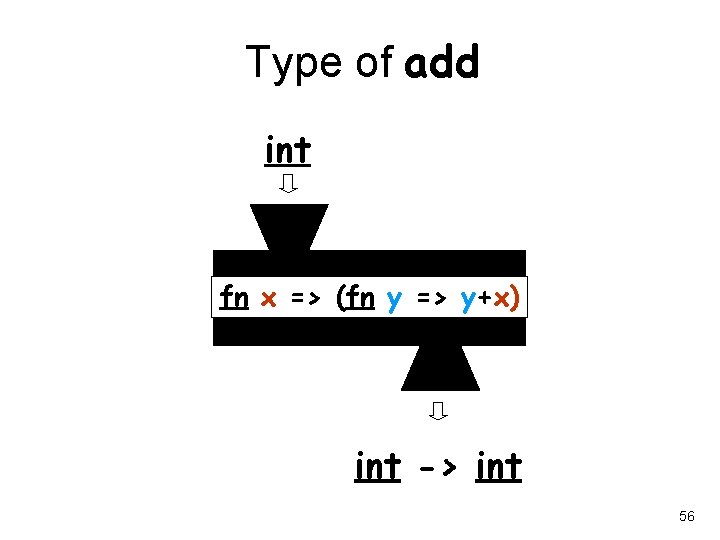
Type of add int fn x => (fn y => y+x) int -> int 56
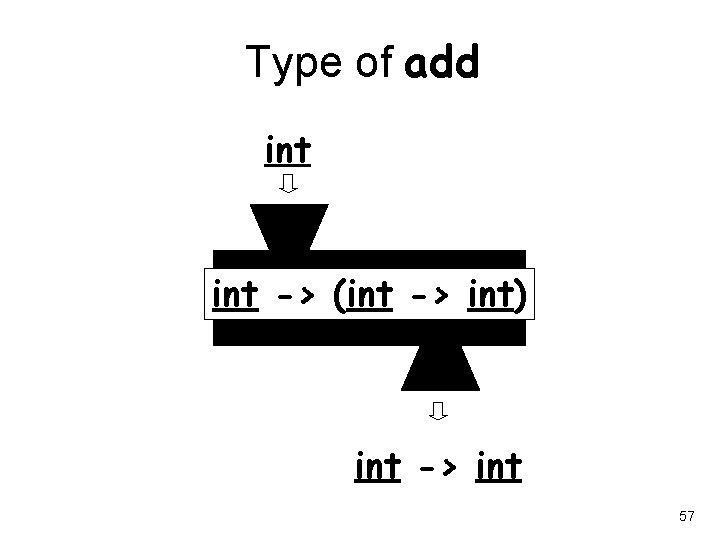
Type of add int -> (int -> int) int -> int 57
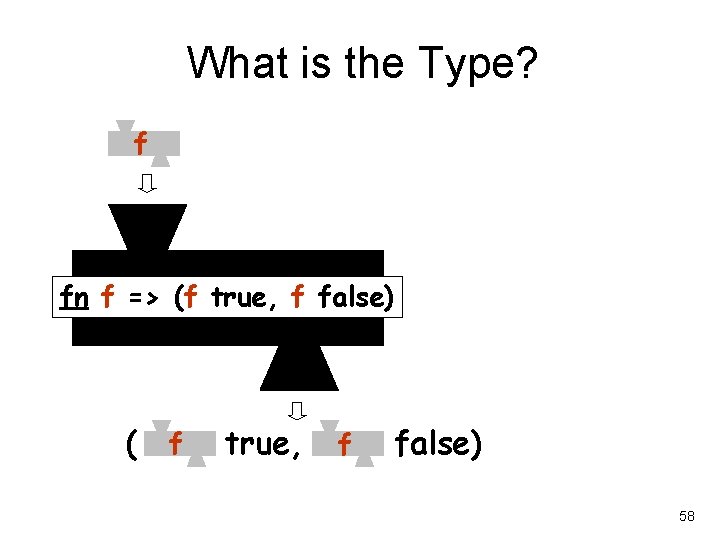
What is the Type? f fn f => (f true, f false) ( f true, f false) 58
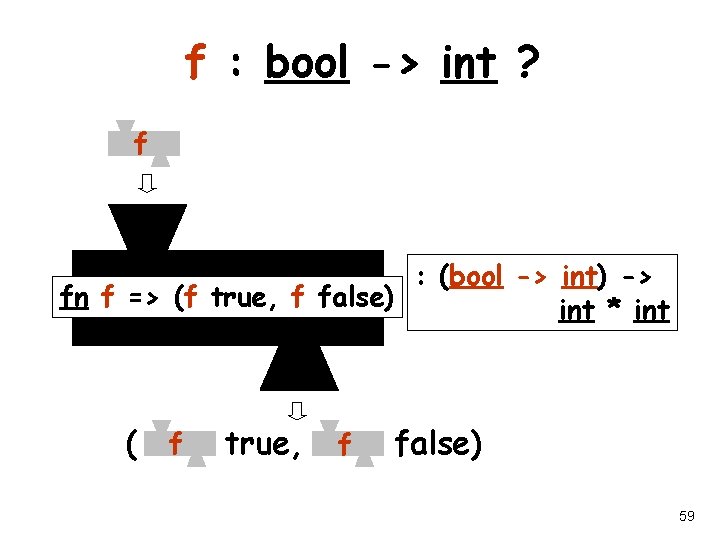
f : bool -> int ? f : (bool -> int) -> fn f => (f true, f false) int * int ( f true, f false) 59
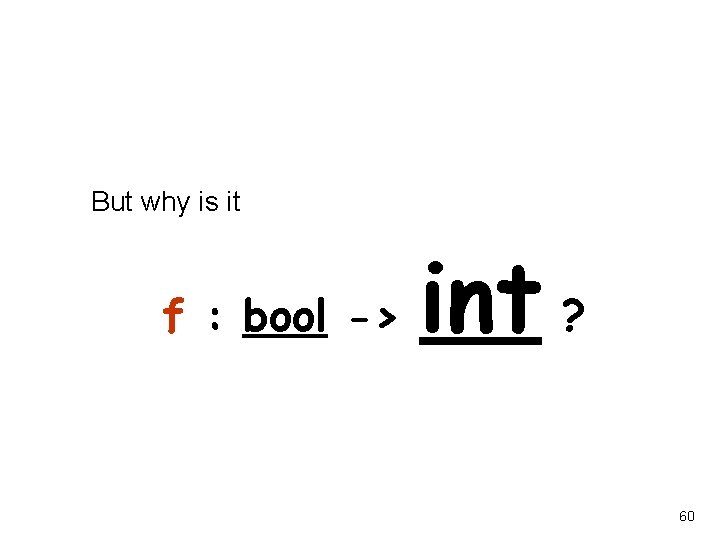
But why is it f : bool -> int ? 60
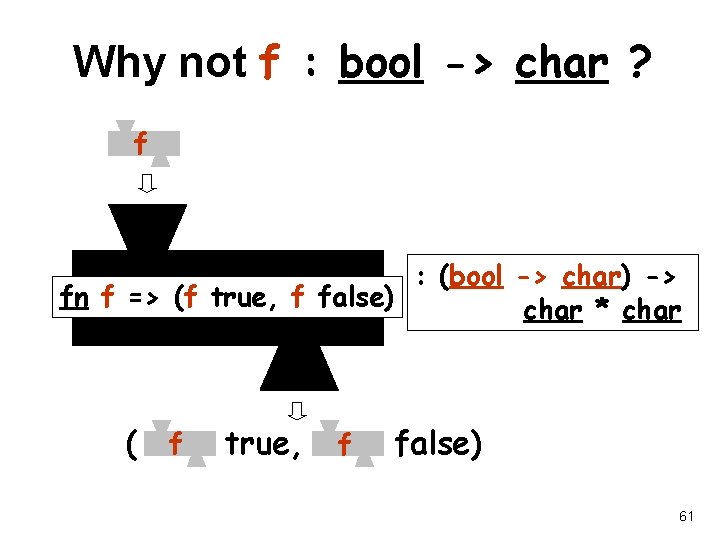
Why not f : bool -> char ? f : (bool -> char) -> fn f => (f true, f false) char * char ( f true, f false) 61
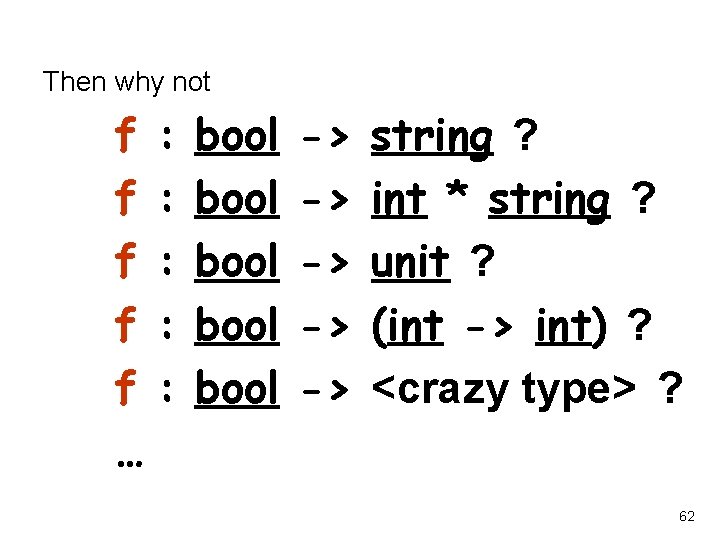
Then why not f : f : f : … bool bool -> -> -> string ? int * string ? unit ? (int -> int) ? <crazy type> ? 62
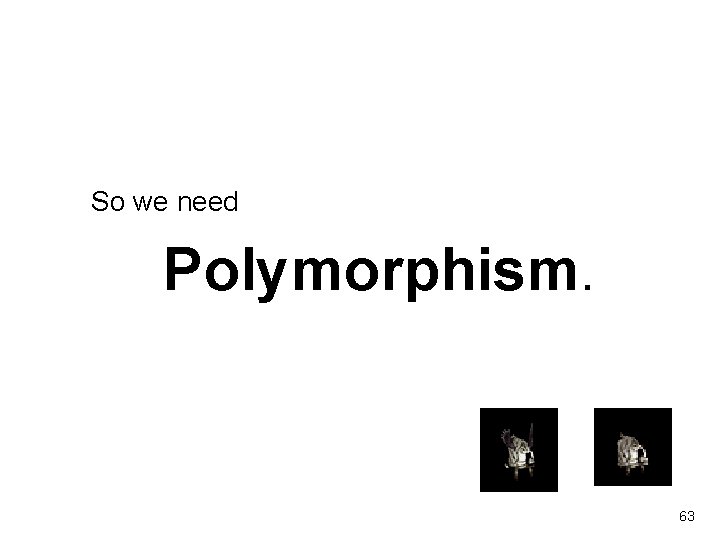
So we need Polymorphism. 63
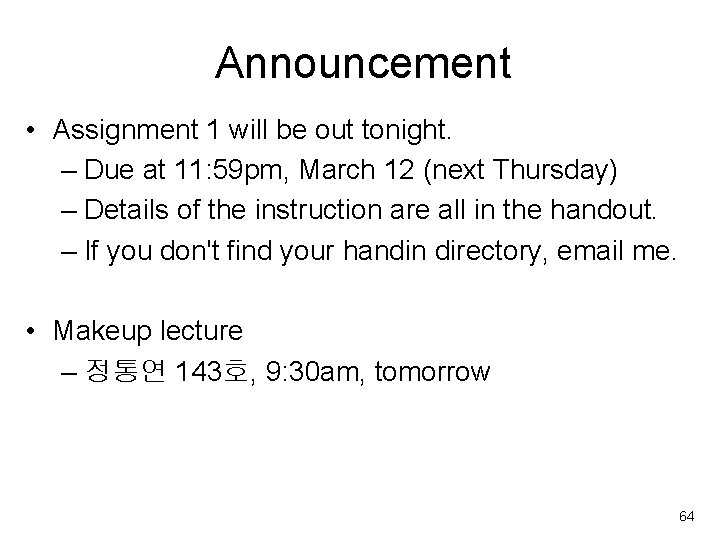
Announcement • Assignment 1 will be out tonight. – Due at 11: 59 pm, March 12 (next Thursday) – Details of the instruction are all in the handout. – If you don't find your handin directory, email me. • Makeup lecture – 정통연 143호, 9: 30 am, tomorrow 64