CSE 690 GPGPU Lecture 6 Cg Tutorial Klaus
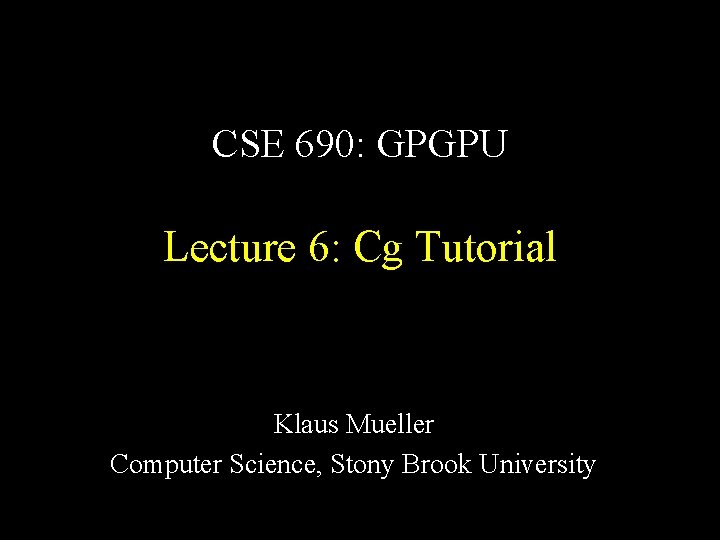
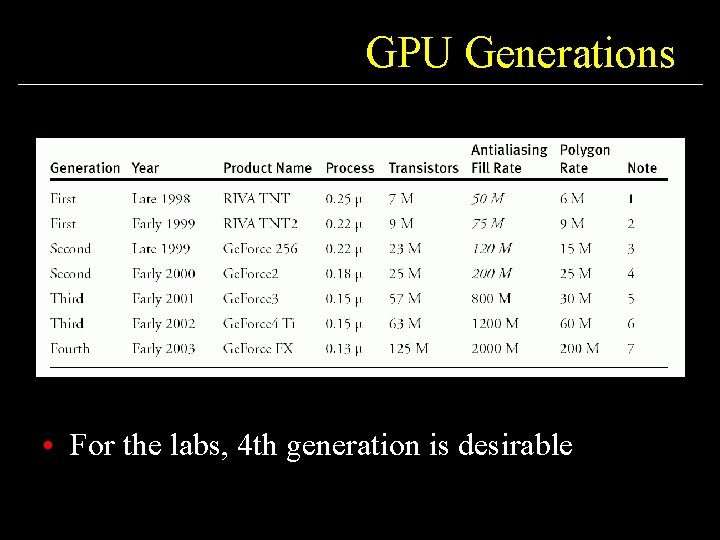
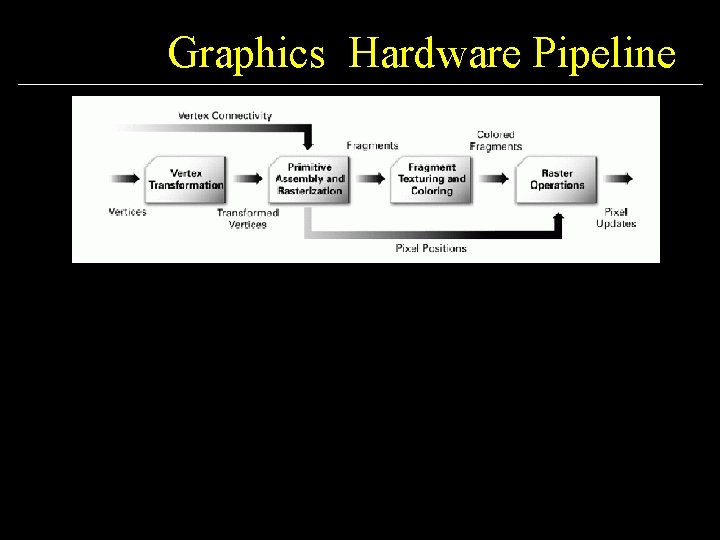
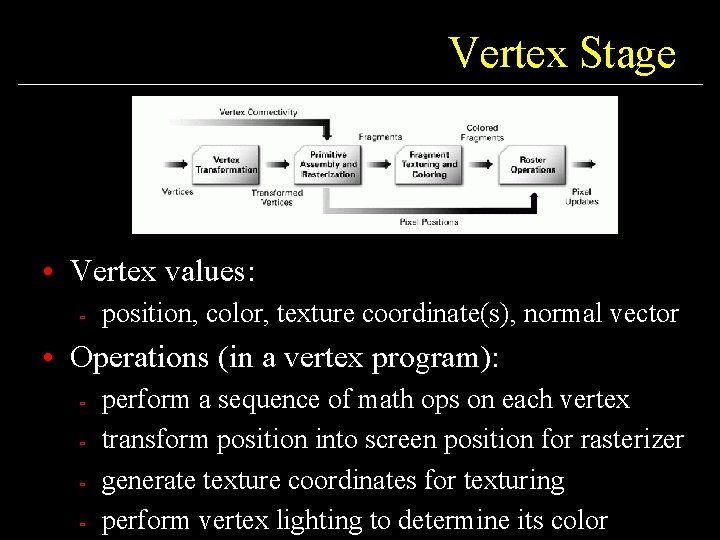
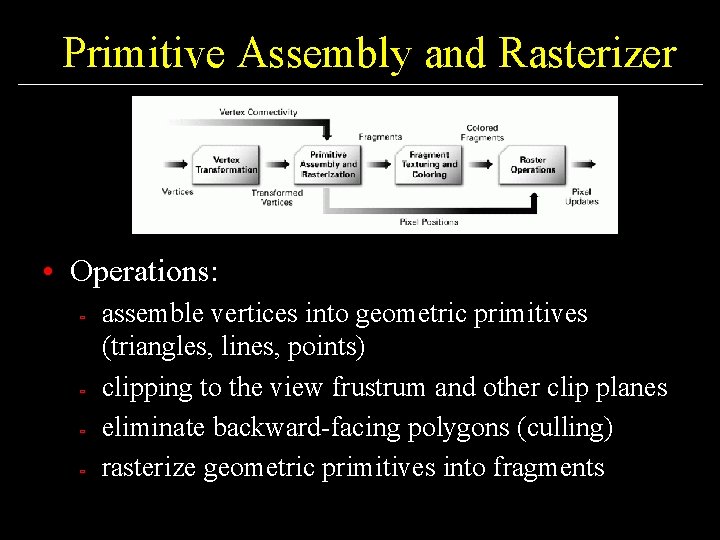
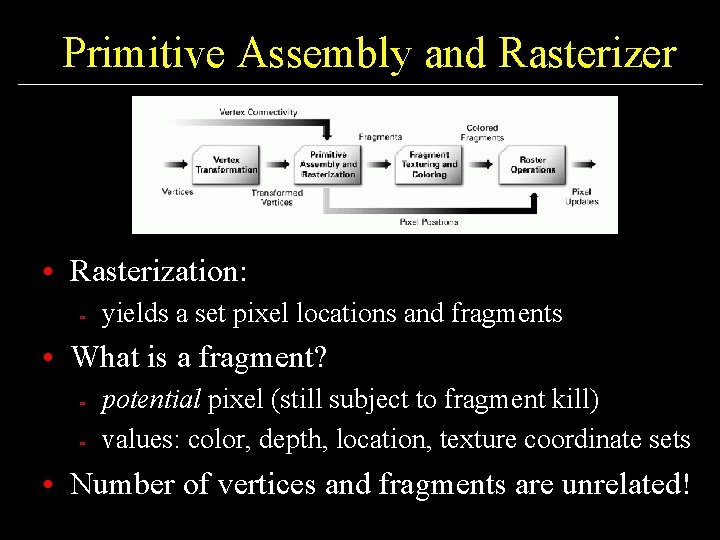
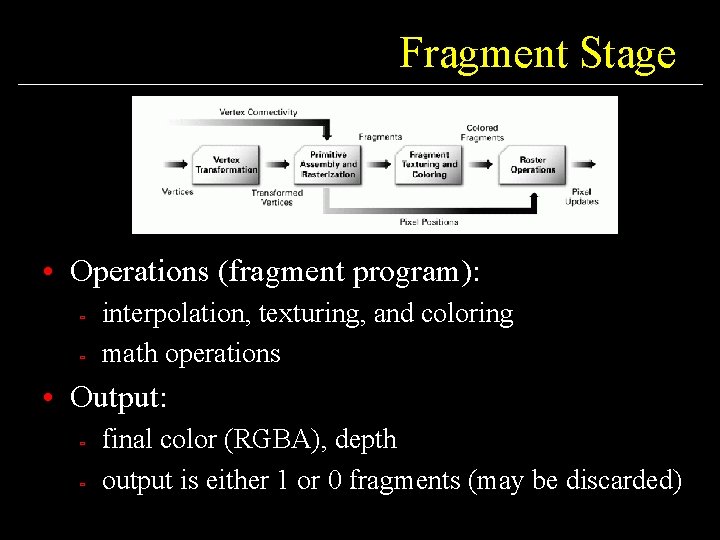
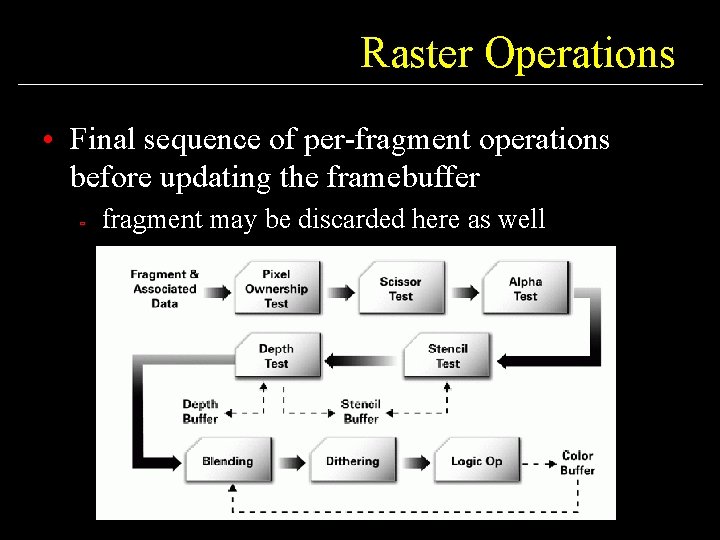
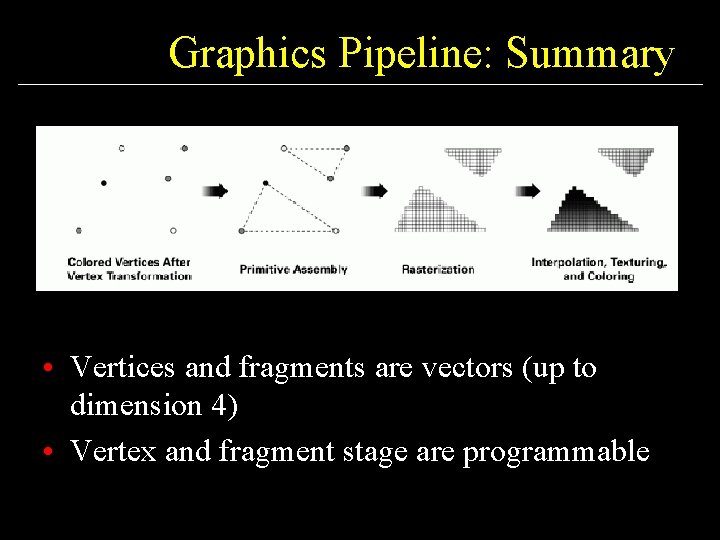
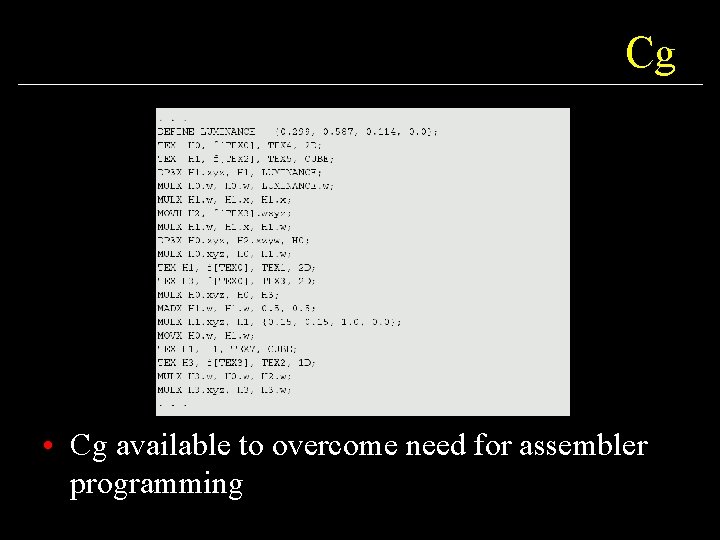
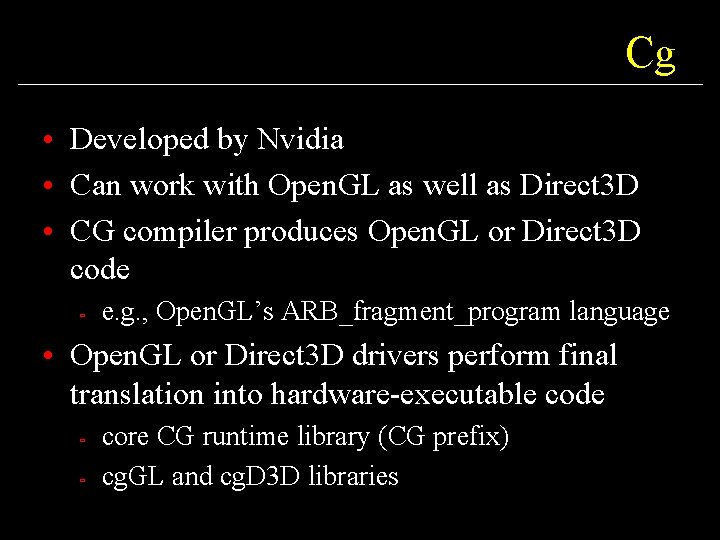
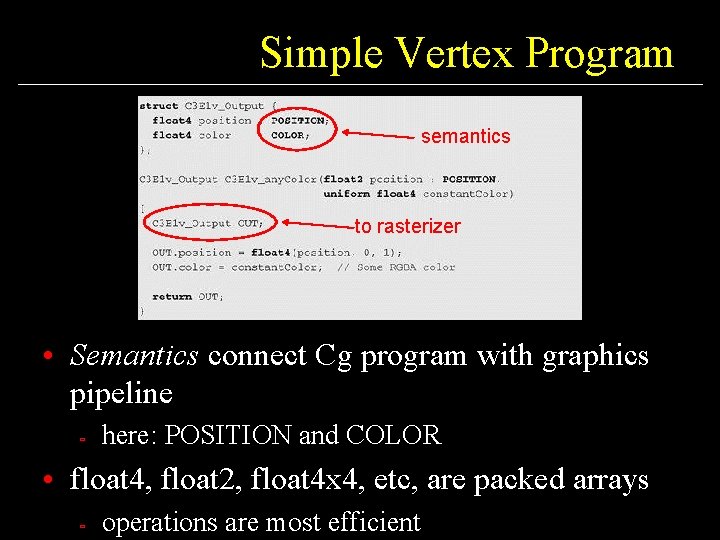
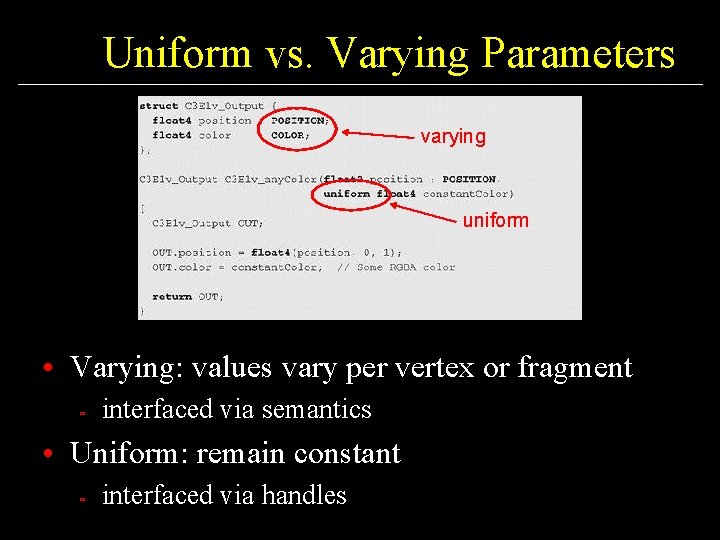
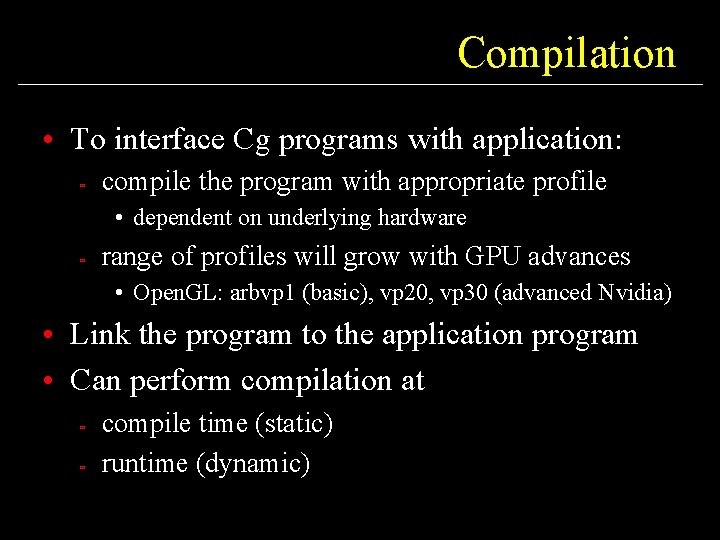
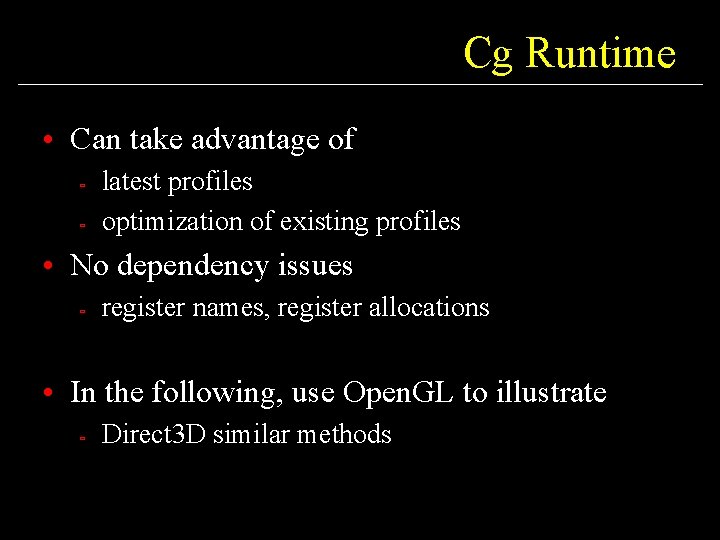
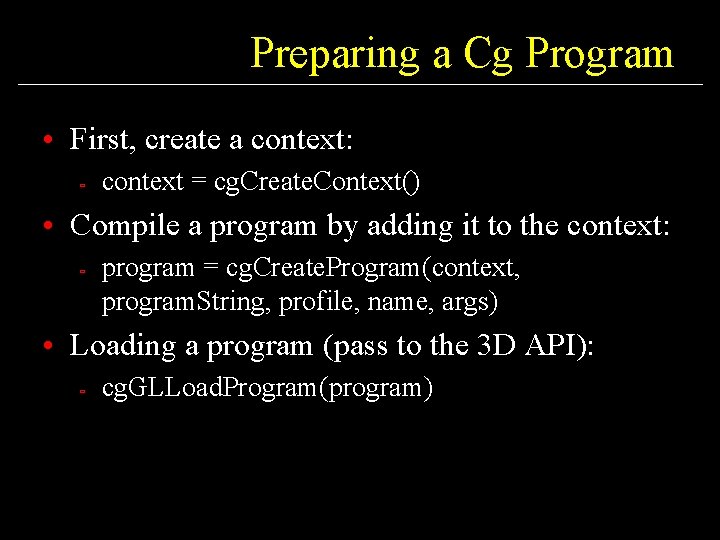
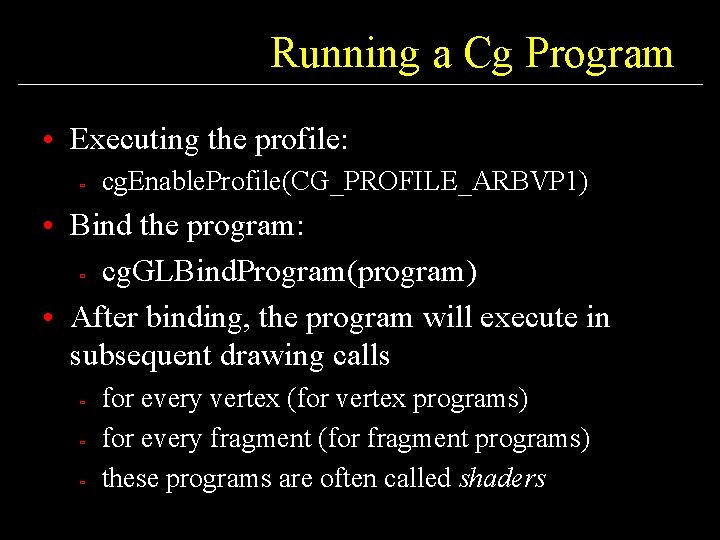
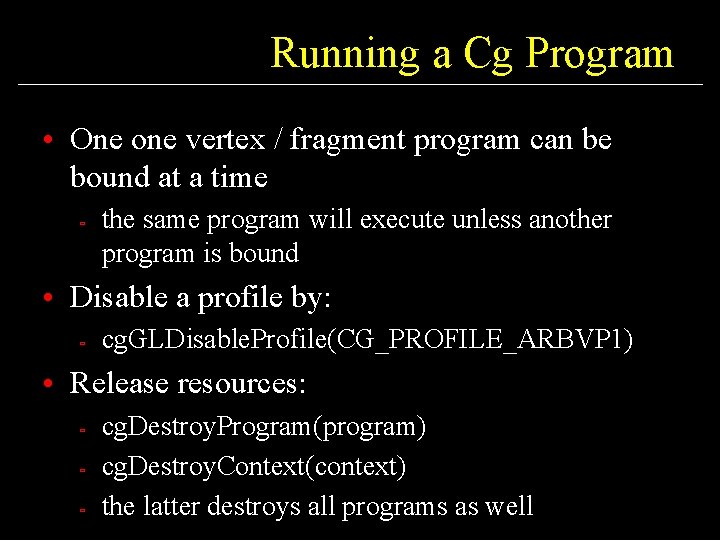
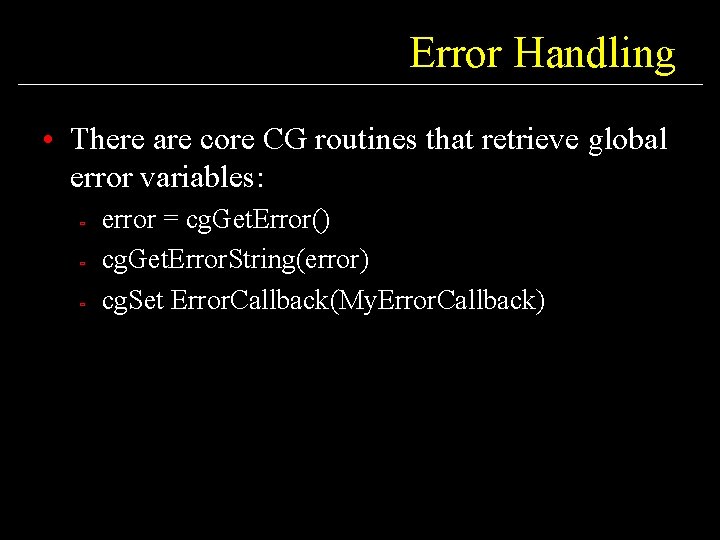
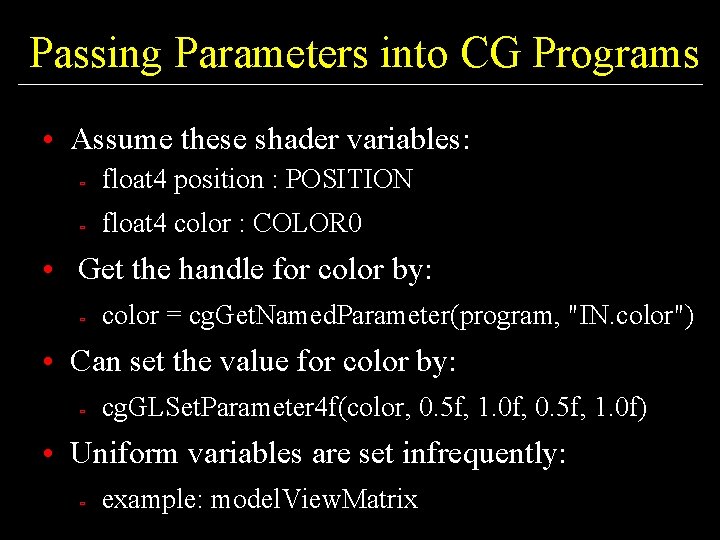
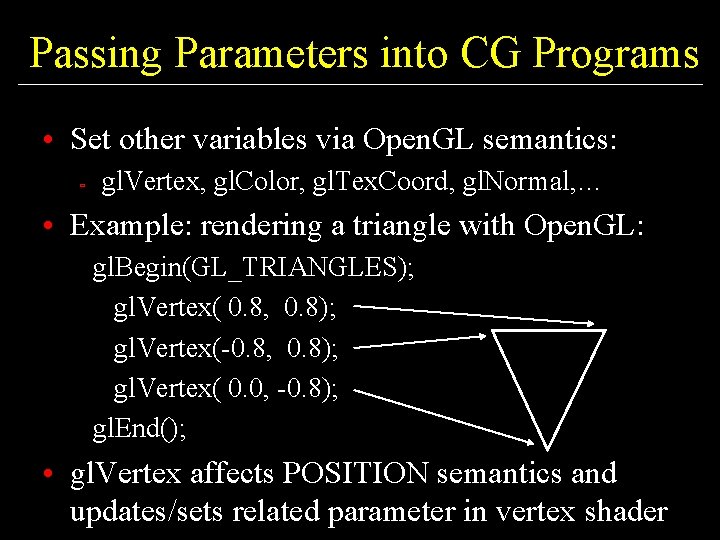
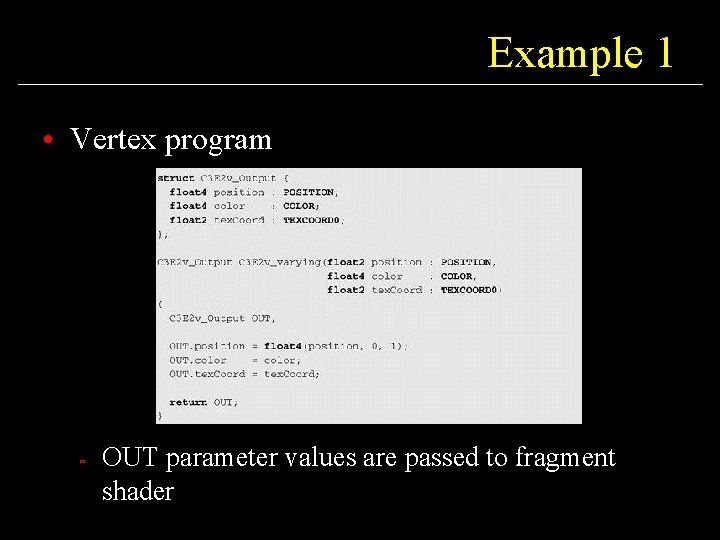
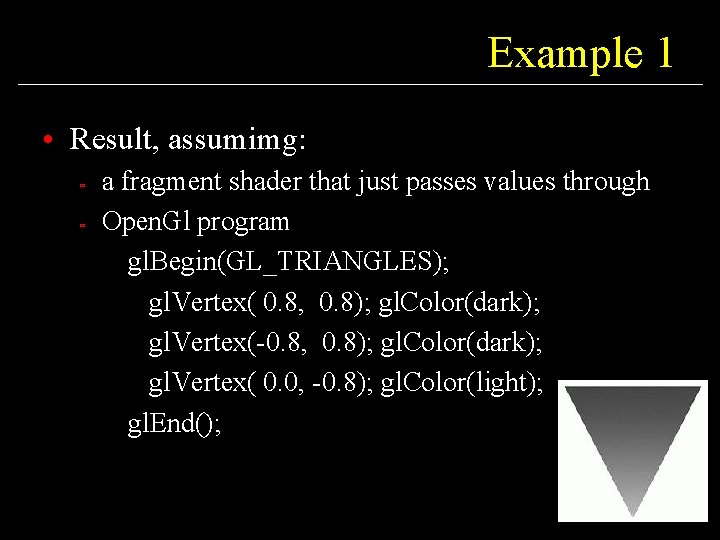
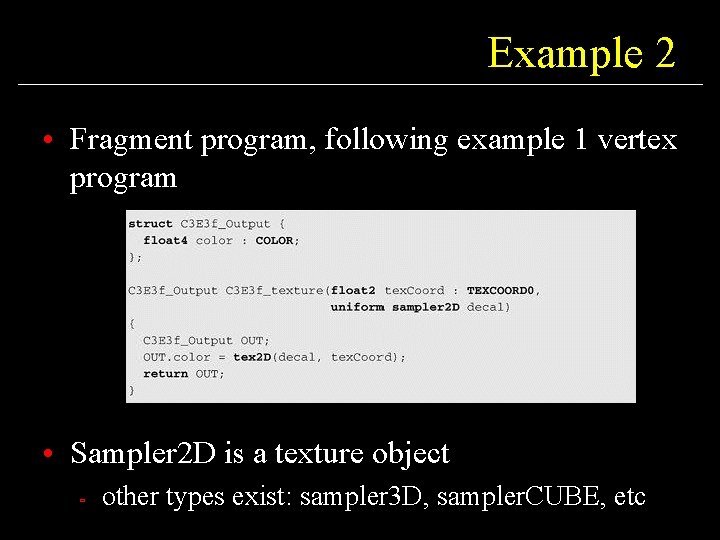
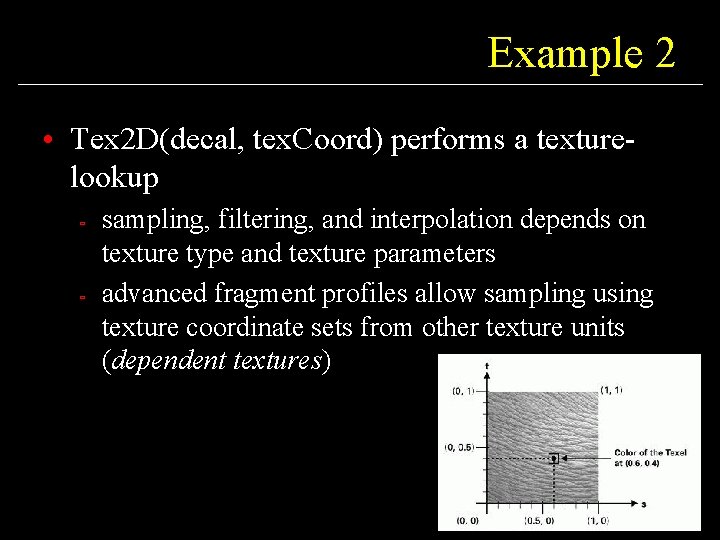
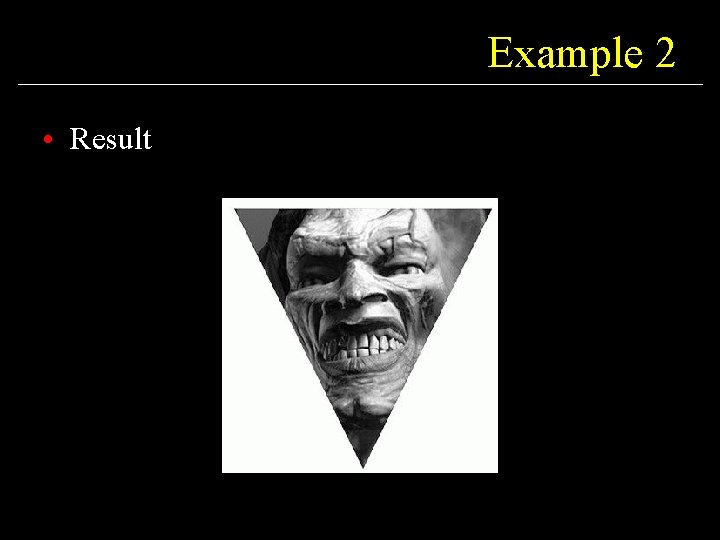
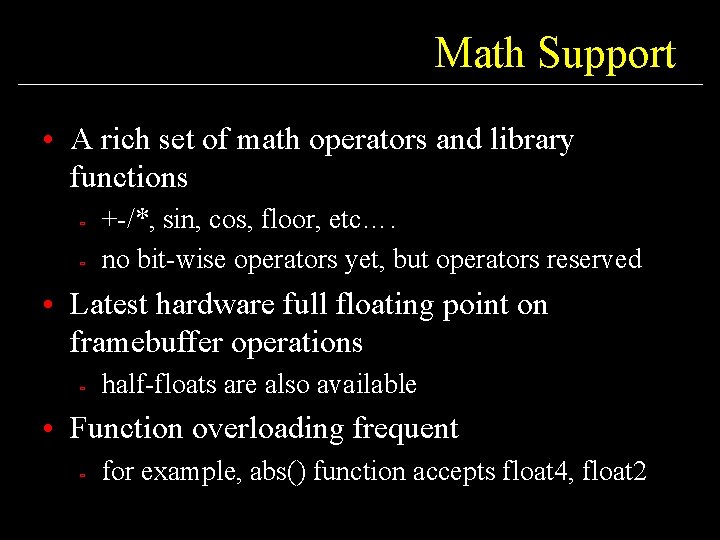
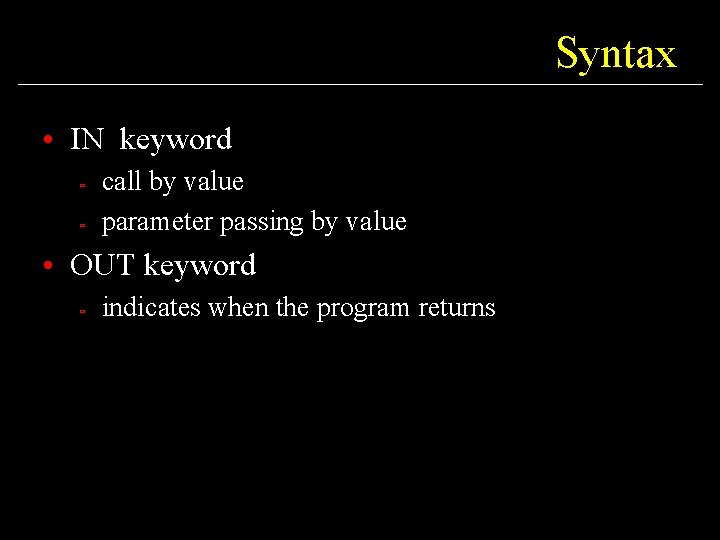
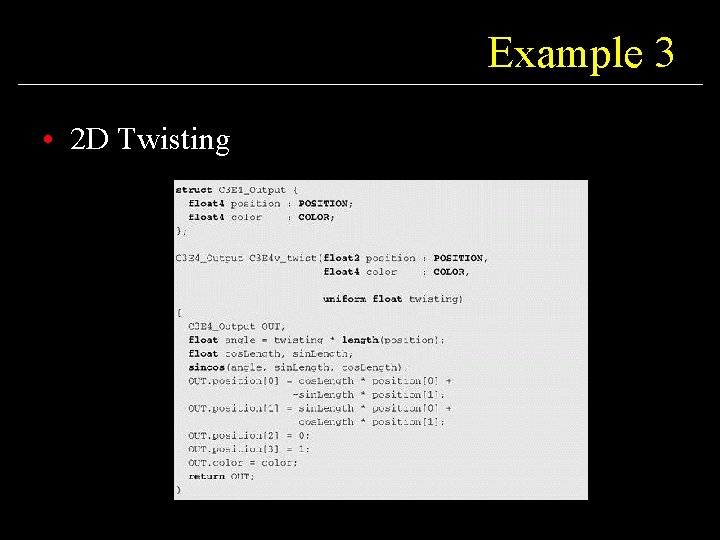
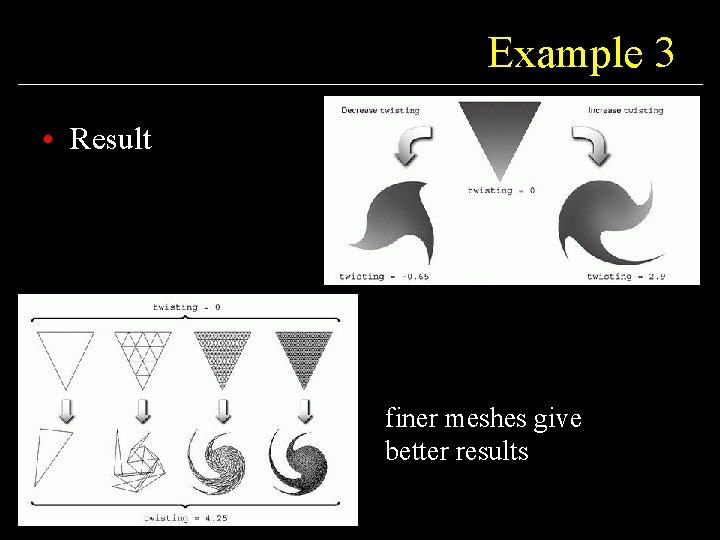
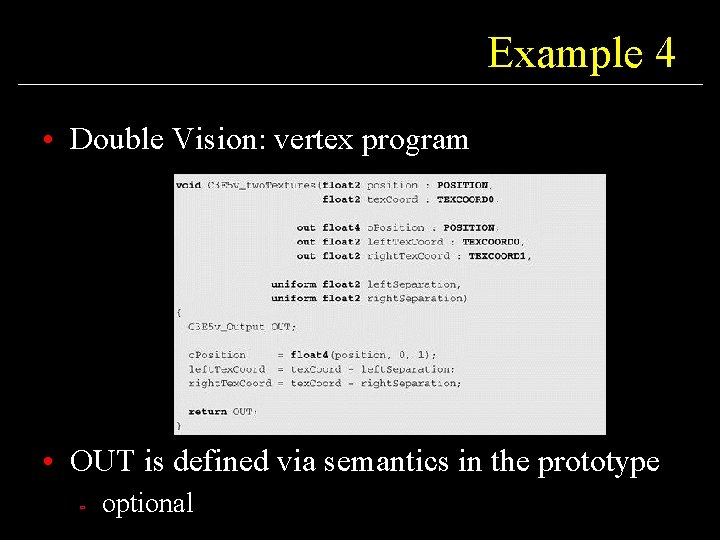
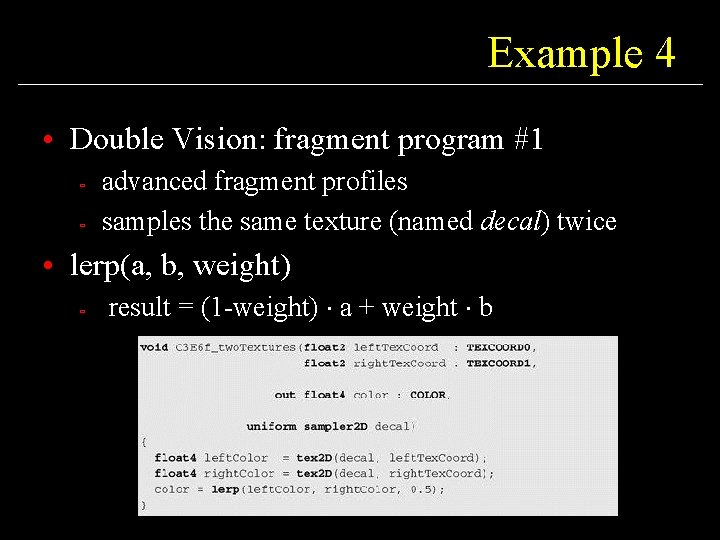
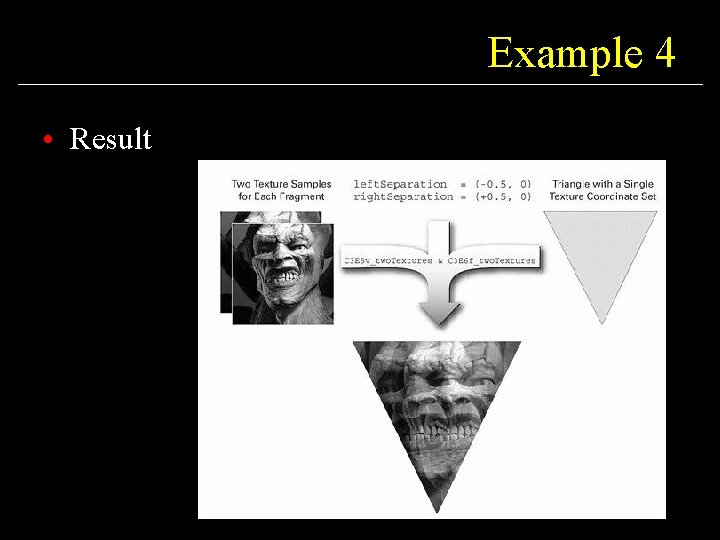
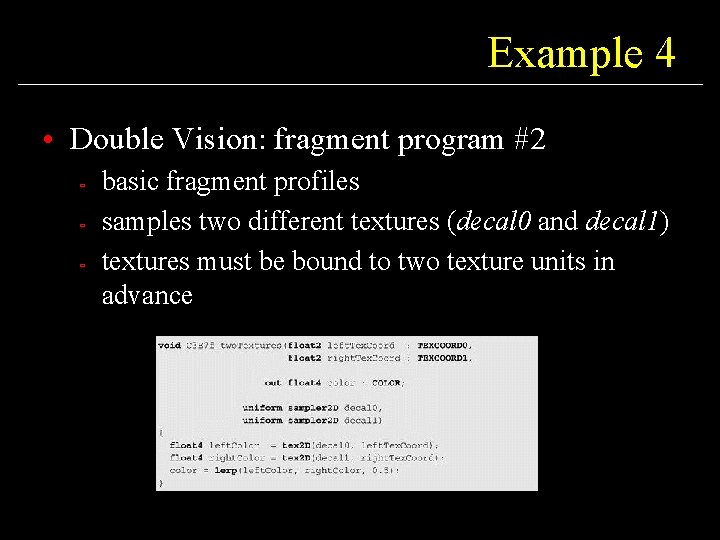
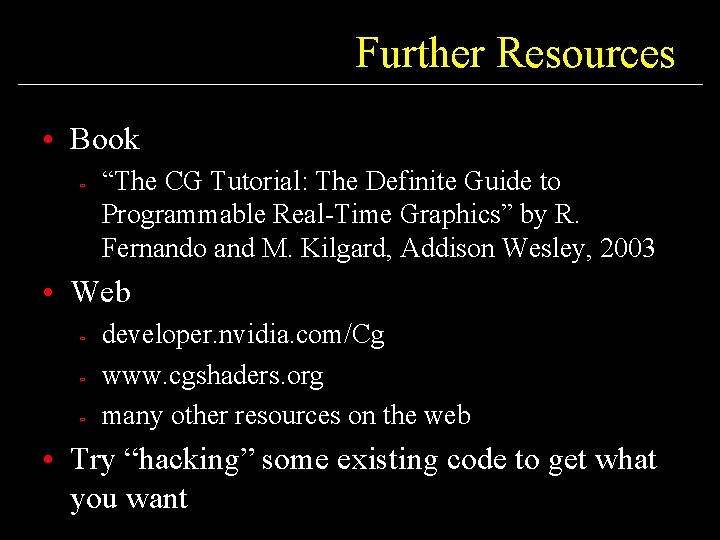
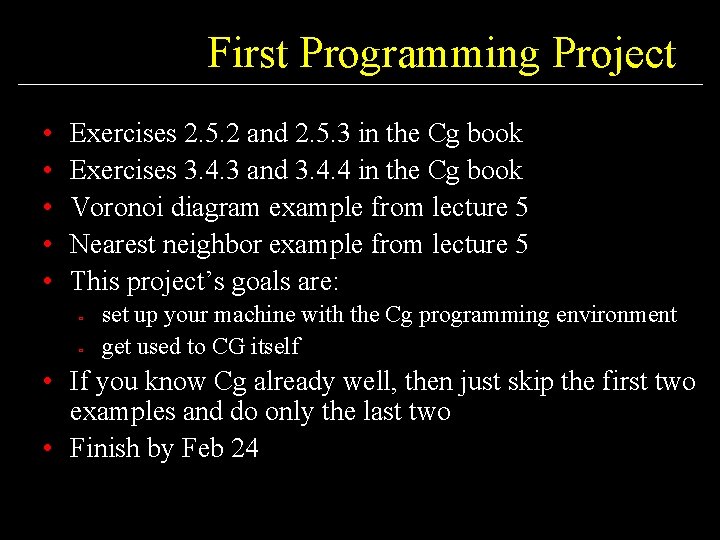
- Slides: 36
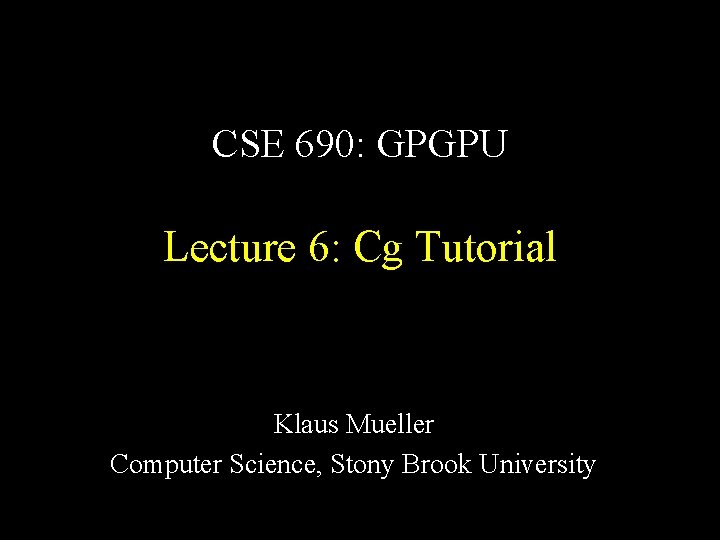
CSE 690: GPGPU Lecture 6: Cg Tutorial Klaus Mueller Computer Science, Stony Brook University
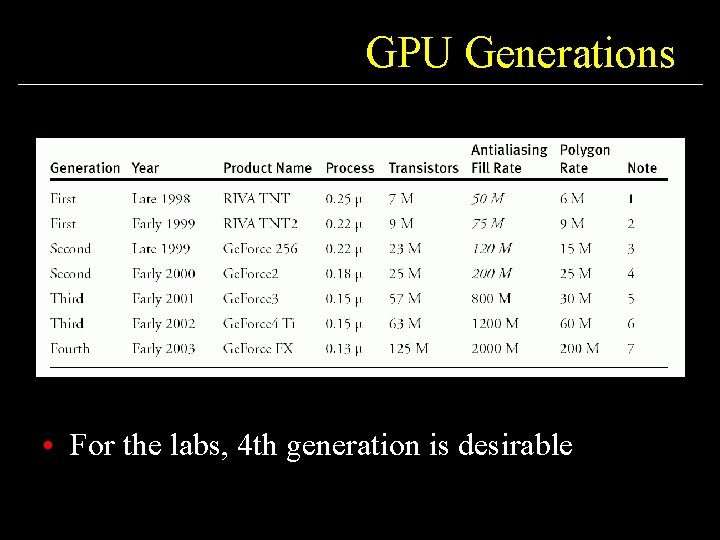
GPU Generations • For the labs, 4 th generation is desirable
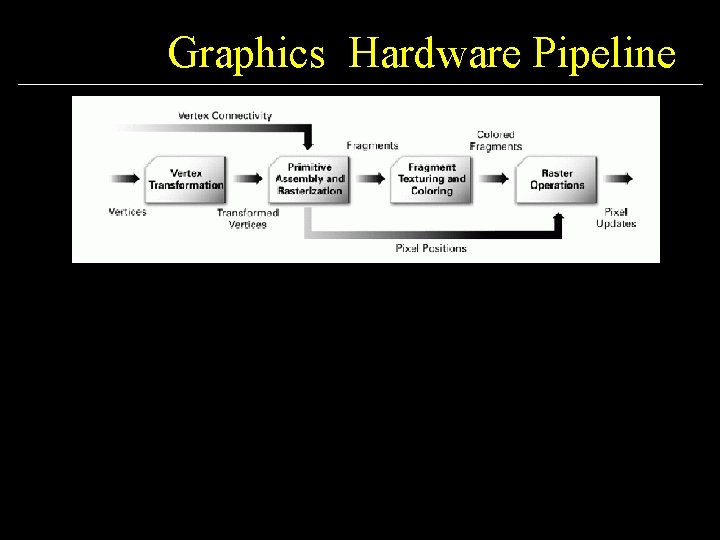
Graphics Hardware Pipeline
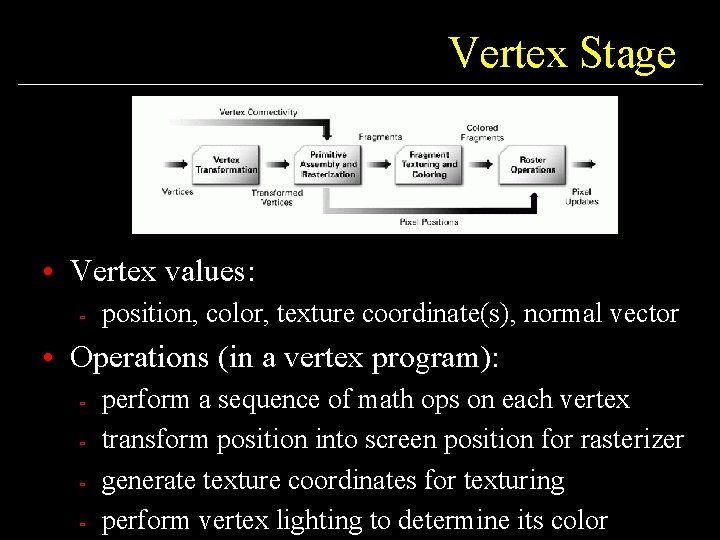
Vertex Stage • Vertex values: ù position, color, texture coordinate(s), normal vector • Operations (in a vertex program): ù ù perform a sequence of math ops on each vertex transform position into screen position for rasterizer generate texture coordinates for texturing perform vertex lighting to determine its color
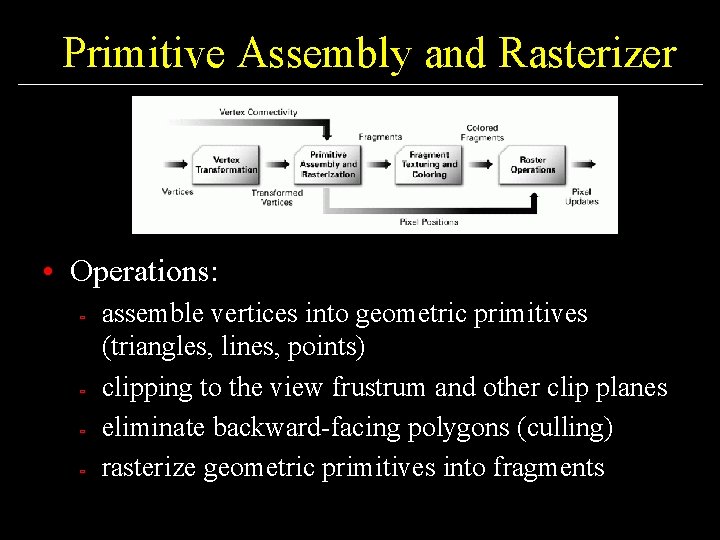
Primitive Assembly and Rasterizer • Operations: ù ù assemble vertices into geometric primitives (triangles, lines, points) clipping to the view frustrum and other clip planes eliminate backward-facing polygons (culling) rasterize geometric primitives into fragments
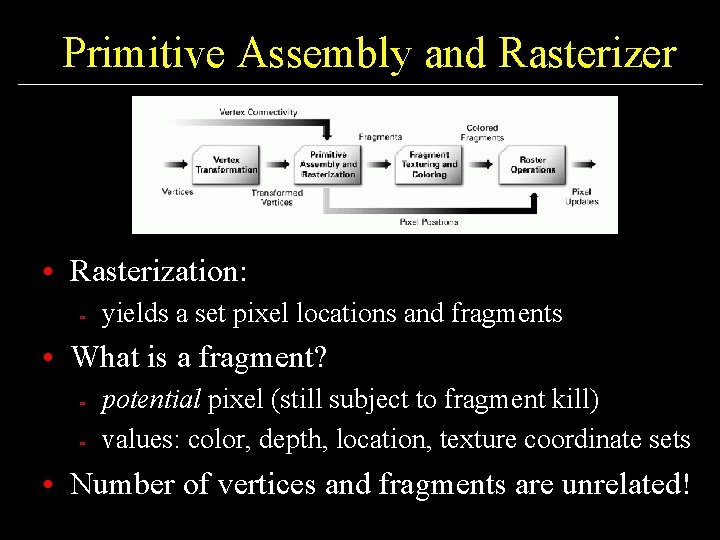
Primitive Assembly and Rasterizer • Rasterization: ù yields a set pixel locations and fragments • What is a fragment? ù ù potential pixel (still subject to fragment kill) values: color, depth, location, texture coordinate sets • Number of vertices and fragments are unrelated!
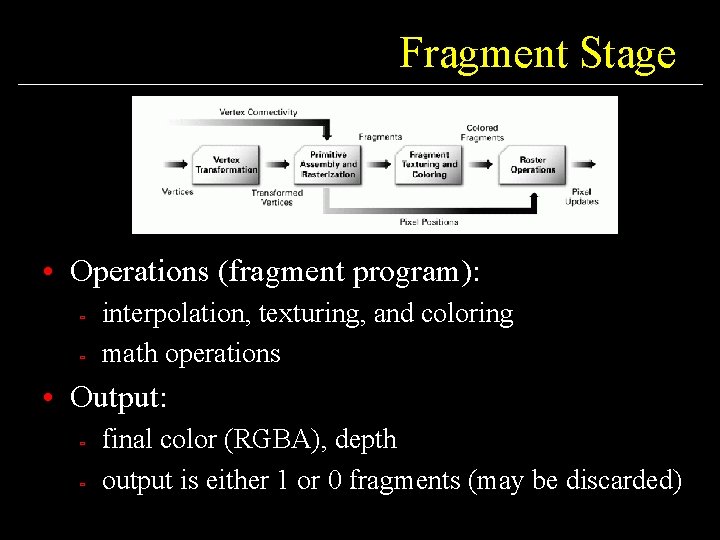
Fragment Stage • Operations (fragment program): ù ù interpolation, texturing, and coloring math operations • Output: ù ù final color (RGBA), depth output is either 1 or 0 fragments (may be discarded)
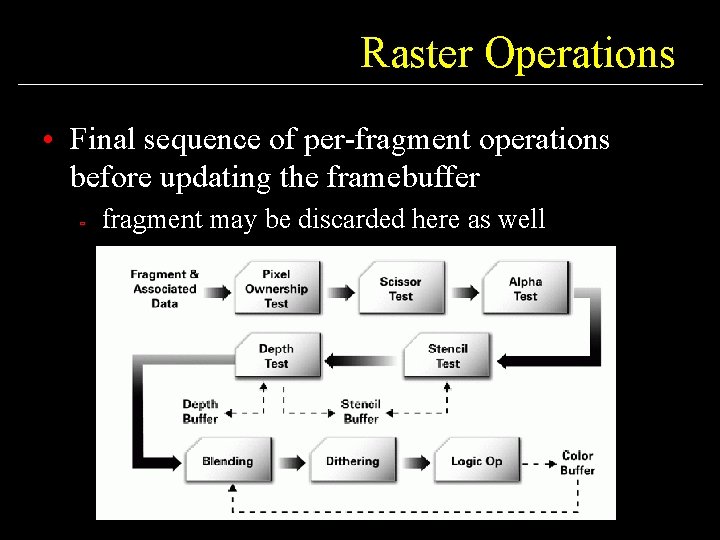
Raster Operations • Final sequence of per-fragment operations before updating the framebuffer ù fragment may be discarded here as well
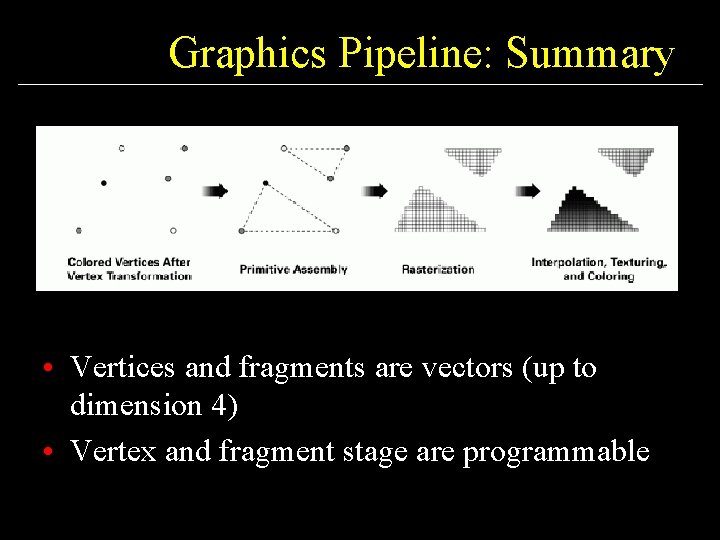
Graphics Pipeline: Summary • Vertices and fragments are vectors (up to dimension 4) • Vertex and fragment stage are programmable
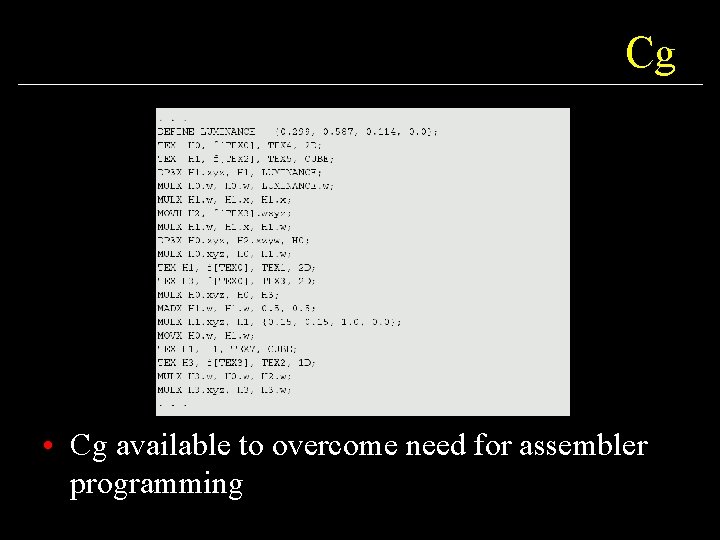
Cg • Cg available to overcome need for assembler programming
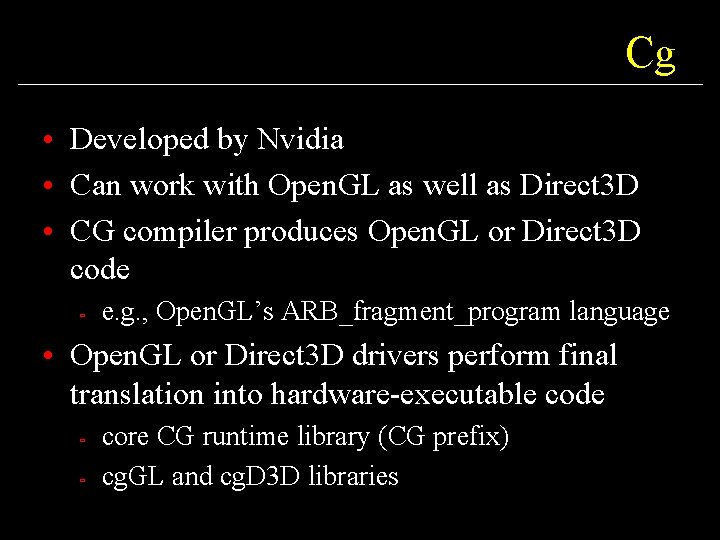
Cg • Developed by Nvidia • Can work with Open. GL as well as Direct 3 D • CG compiler produces Open. GL or Direct 3 D code ù e. g. , Open. GL’s ARB_fragment_program language • Open. GL or Direct 3 D drivers perform final translation into hardware-executable code ù ù core CG runtime library (CG prefix) cg. GL and cg. D 3 D libraries
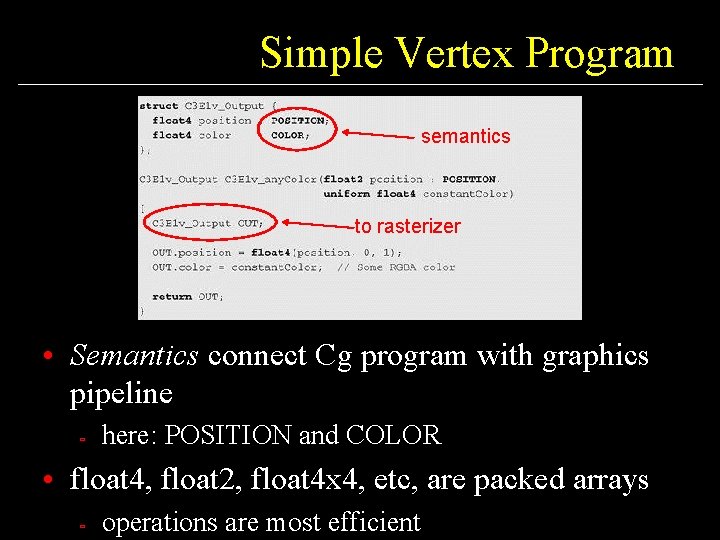
Simple Vertex Program semantics to rasterizer • Semantics connect Cg program with graphics pipeline ù here: POSITION and COLOR • float 4, float 2, float 4 x 4, etc, are packed arrays ù operations are most efficient
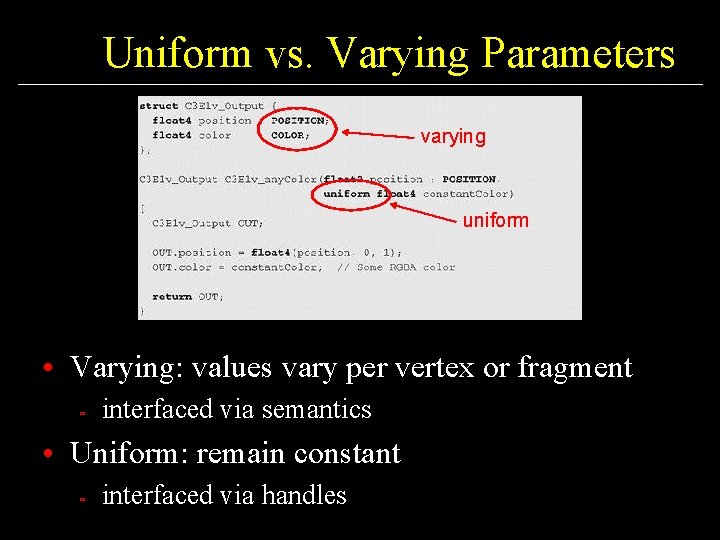
Uniform vs. Varying Parameters varying uniform • Varying: values vary per vertex or fragment ù interfaced via semantics • Uniform: remain constant ù interfaced via handles
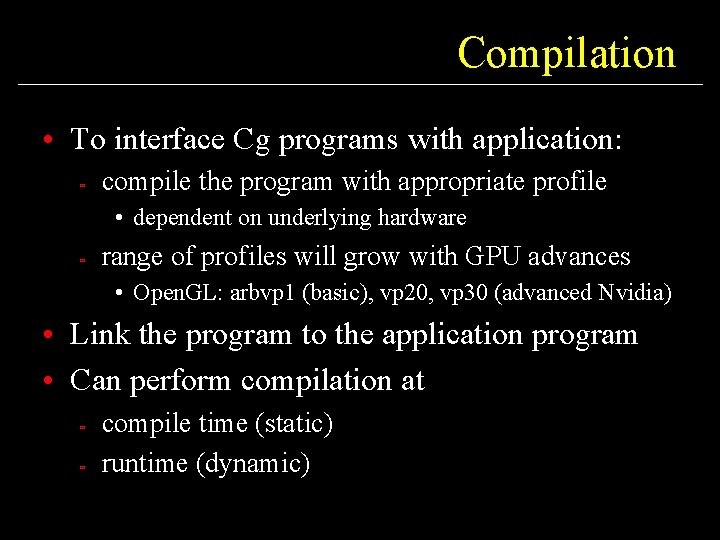
Compilation • To interface Cg programs with application: ù compile the program with appropriate profile • dependent on underlying hardware ù range of profiles will grow with GPU advances • Open. GL: arbvp 1 (basic), vp 20, vp 30 (advanced Nvidia) • Link the program to the application program • Can perform compilation at ù ù compile time (static) runtime (dynamic)
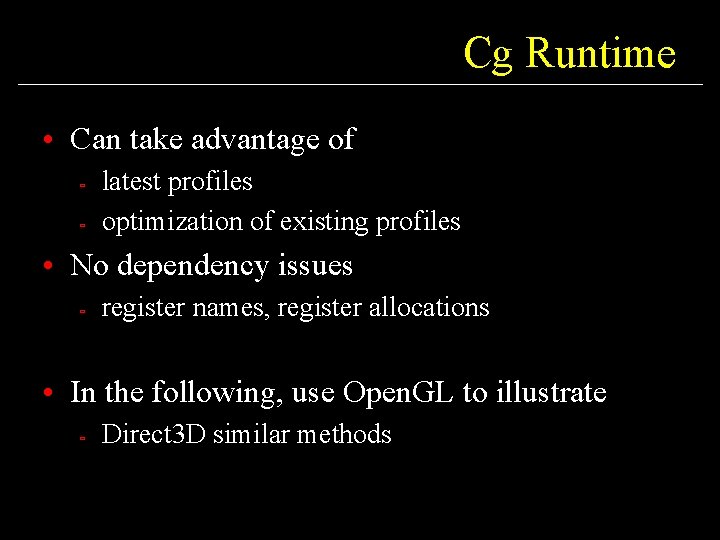
Cg Runtime • Can take advantage of ù ù latest profiles optimization of existing profiles • No dependency issues ù register names, register allocations • In the following, use Open. GL to illustrate ù Direct 3 D similar methods
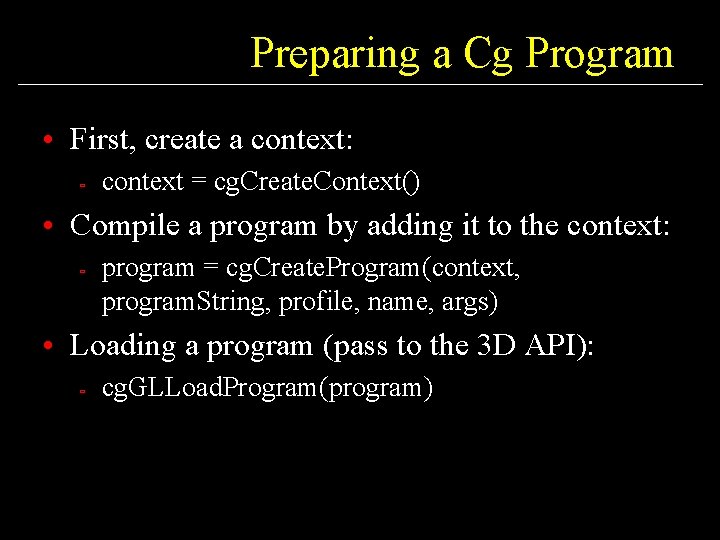
Preparing a Cg Program • First, create a context: ù context = cg. Create. Context() • Compile a program by adding it to the context: ù program = cg. Create. Program(context, program. String, profile, name, args) • Loading a program (pass to the 3 D API): ù cg. GLLoad. Program(program)
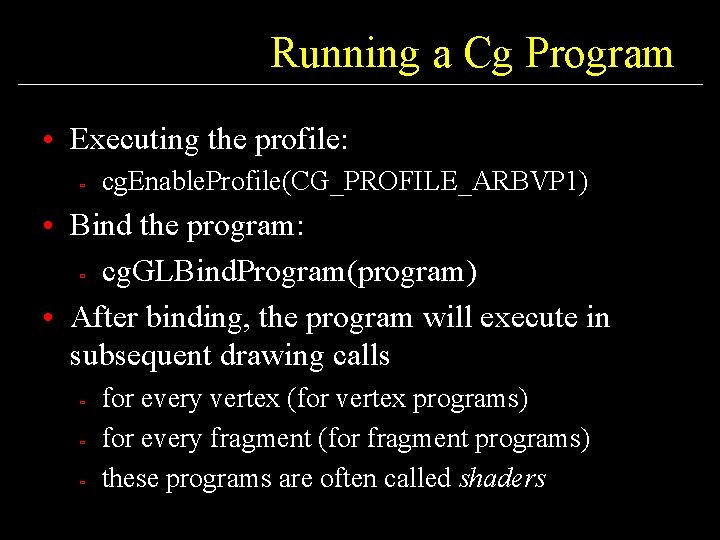
Running a Cg Program • Executing the profile: ù cg. Enable. Profile(CG_PROFILE_ARBVP 1) • Bind the program: ù cg. GLBind. Program(program) • After binding, the program will execute in subsequent drawing calls ù ù ù for every vertex (for vertex programs) for every fragment (for fragment programs) these programs are often called shaders
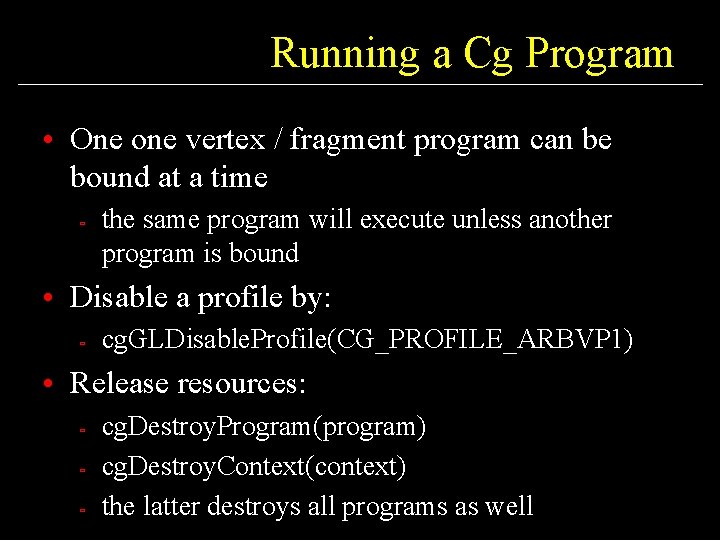
Running a Cg Program • One one vertex / fragment program can be bound at a time ù the same program will execute unless another program is bound • Disable a profile by: ù cg. GLDisable. Profile(CG_PROFILE_ARBVP 1) • Release resources: ù ù ù cg. Destroy. Program(program) cg. Destroy. Context(context) the latter destroys all programs as well
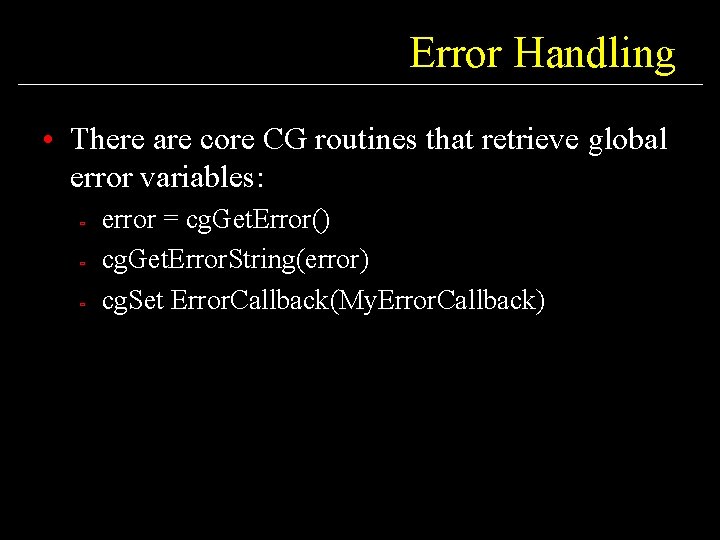
Error Handling • There are core CG routines that retrieve global error variables: ù ù ù error = cg. Get. Error() cg. Get. Error. String(error) cg. Set Error. Callback(My. Error. Callback)
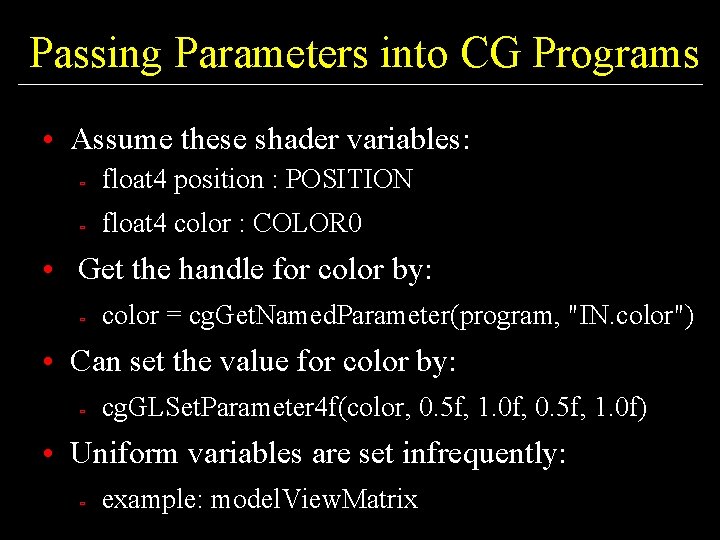
Passing Parameters into CG Programs • Assume these shader variables: ù float 4 position : POSITION ù float 4 color : COLOR 0 • Get the handle for color by: ù color = cg. Get. Named. Parameter(program, "IN. color") • Can set the value for color by: ù cg. GLSet. Parameter 4 f(color, 0. 5 f, 1. 0 f) • Uniform variables are set infrequently: ù example: model. View. Matrix
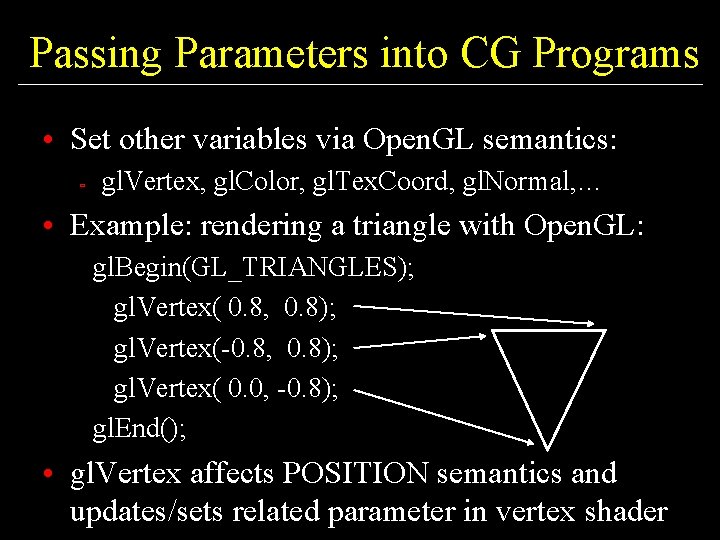
Passing Parameters into CG Programs • Set other variables via Open. GL semantics: ù gl. Vertex, gl. Color, gl. Tex. Coord, gl. Normal, … • Example: rendering a triangle with Open. GL: gl. Begin(GL_TRIANGLES); gl. Vertex( 0. 8, 0. 8); gl. Vertex(-0. 8, 0. 8); gl. Vertex( 0. 0, -0. 8); gl. End(); • gl. Vertex affects POSITION semantics and updates/sets related parameter in vertex shader
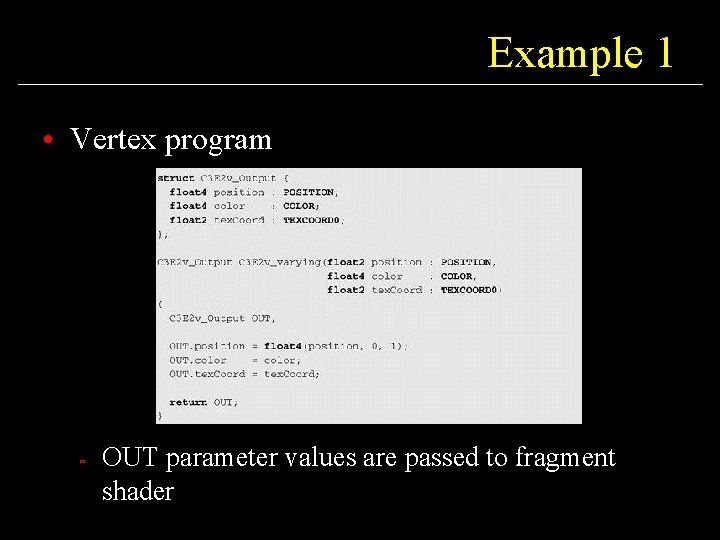
Example 1 • Vertex program ù OUT parameter values are passed to fragment shader
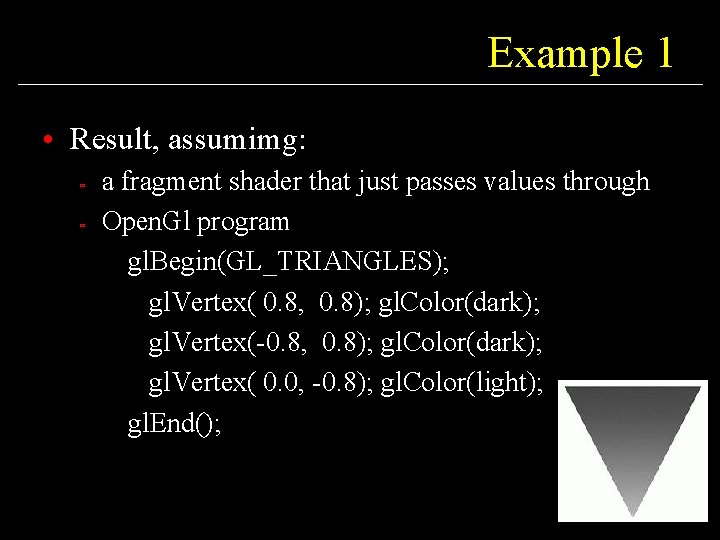
Example 1 • Result, assumimg: ù ù a fragment shader that just passes values through Open. Gl program gl. Begin(GL_TRIANGLES); gl. Vertex( 0. 8, 0. 8); gl. Color(dark); gl. Vertex(-0. 8, 0. 8); gl. Color(dark); gl. Vertex( 0. 0, -0. 8); gl. Color(light); gl. End();
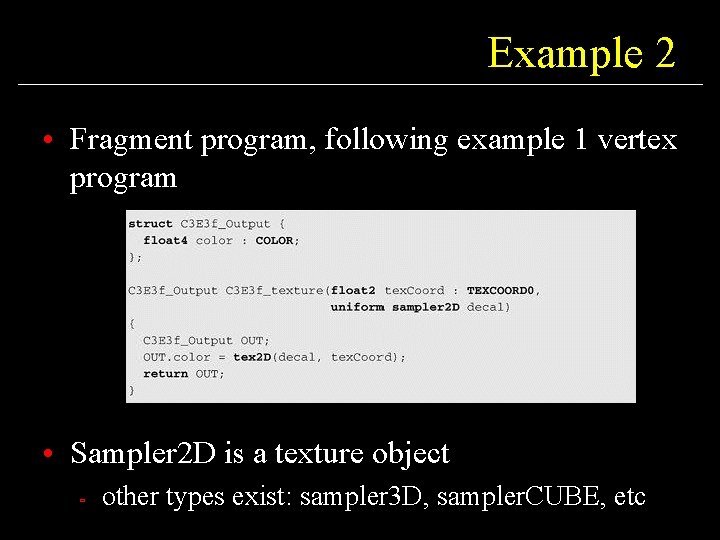
Example 2 • Fragment program, following example 1 vertex program • Sampler 2 D is a texture object ù other types exist: sampler 3 D, sampler. CUBE, etc
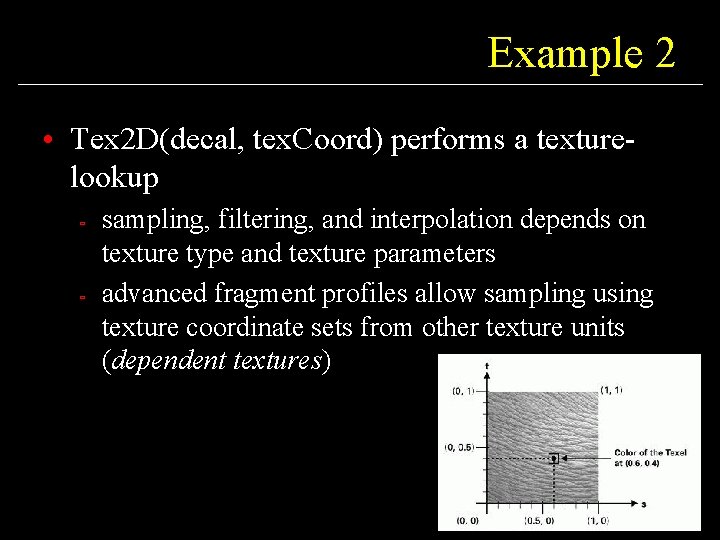
Example 2 • Tex 2 D(decal, tex. Coord) performs a texturelookup ù ù sampling, filtering, and interpolation depends on texture type and texture parameters advanced fragment profiles allow sampling using texture coordinate sets from other texture units (dependent textures)
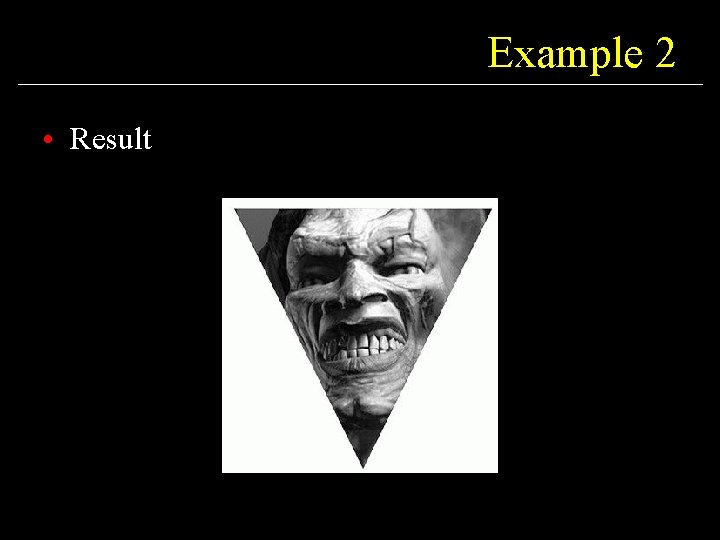
Example 2 • Result
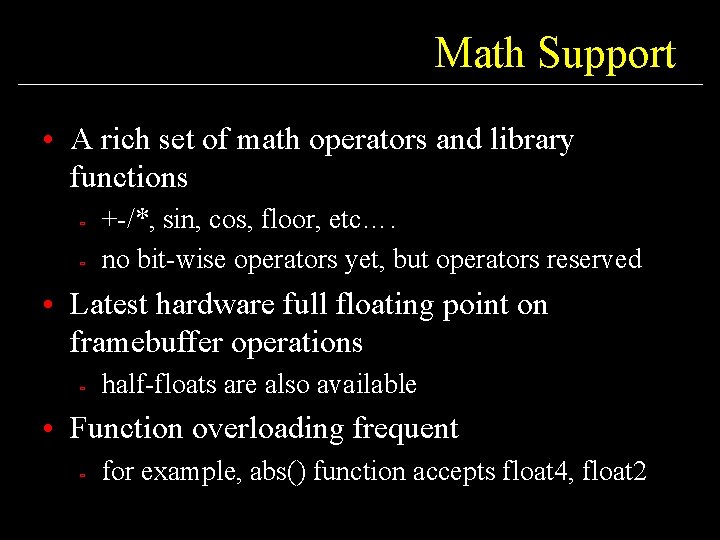
Math Support • A rich set of math operators and library functions ù ù +-/*, sin, cos, floor, etc…. no bit-wise operators yet, but operators reserved • Latest hardware full floating point on framebuffer operations ù half-floats are also available • Function overloading frequent ù for example, abs() function accepts float 4, float 2
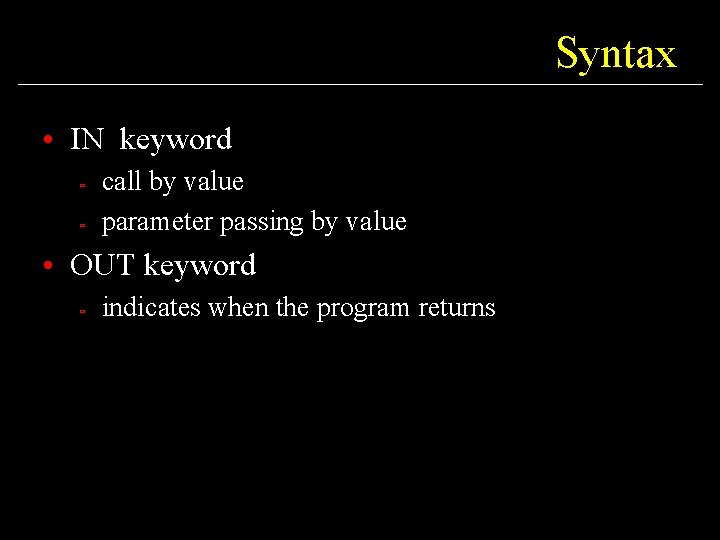
Syntax • IN keyword ù ù call by value parameter passing by value • OUT keyword ù indicates when the program returns
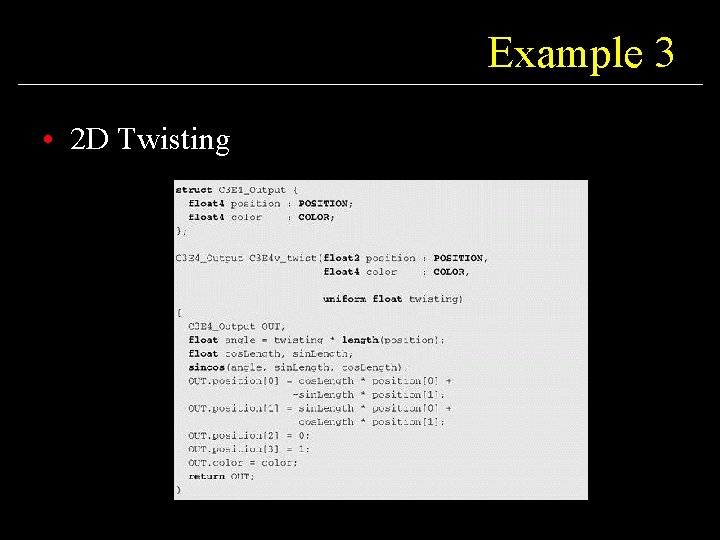
Example 3 • 2 D Twisting
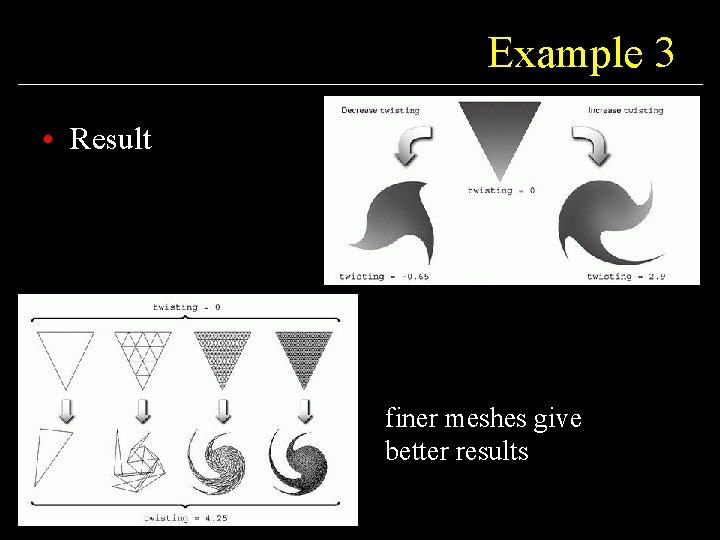
Example 3 • Result finer meshes give better results
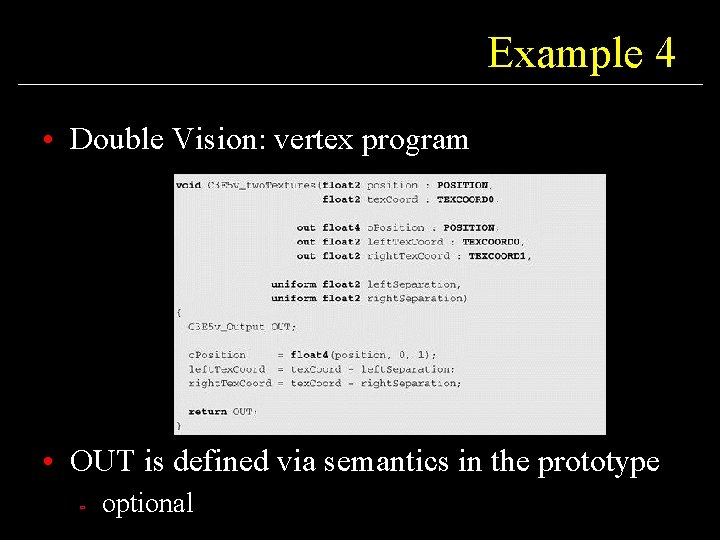
Example 4 • Double Vision: vertex program • OUT is defined via semantics in the prototype ù optional
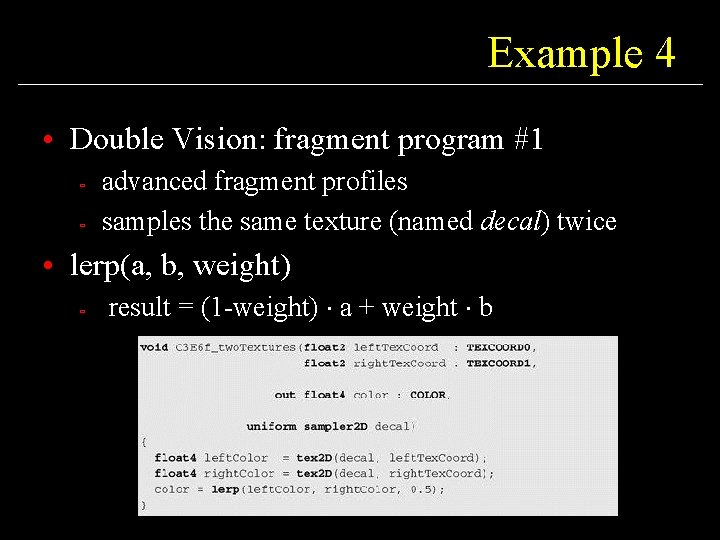
Example 4 • Double Vision: fragment program #1 ù ù advanced fragment profiles samples the same texture (named decal) twice • lerp(a, b, weight) ù result = (1 -weight) × a + weight × b
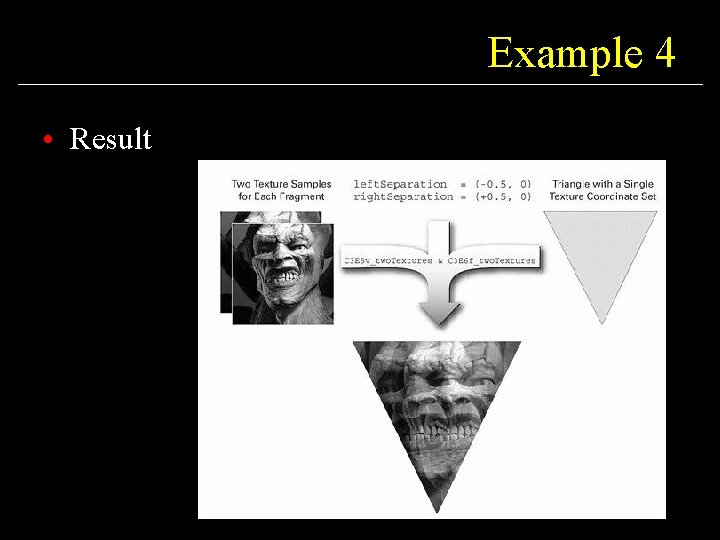
Example 4 • Result
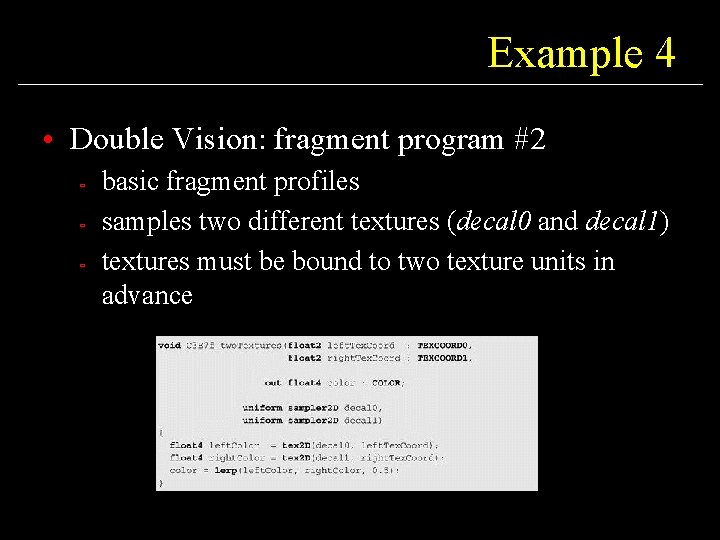
Example 4 • Double Vision: fragment program #2 ù ù ù basic fragment profiles samples two different textures (decal 0 and decal 1) textures must be bound to two texture units in advance
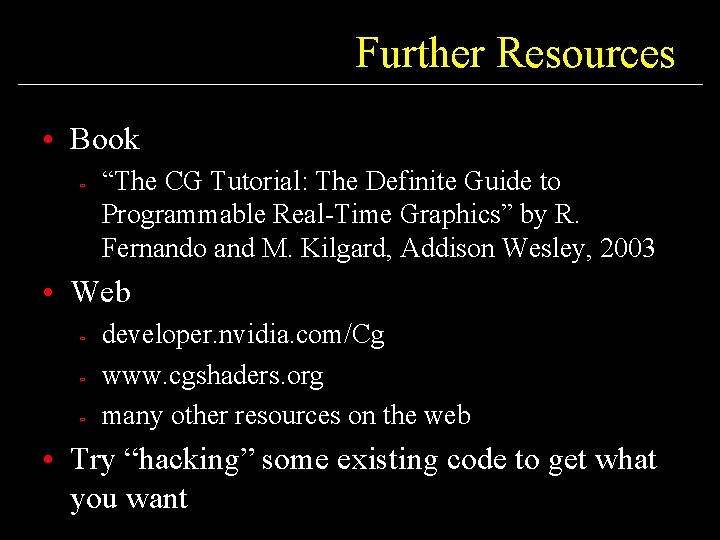
Further Resources • Book ù “The CG Tutorial: The Definite Guide to Programmable Real-Time Graphics” by R. Fernando and M. Kilgard, Addison Wesley, 2003 • Web ù ù ù developer. nvidia. com/Cg www. cgshaders. org many other resources on the web • Try “hacking” some existing code to get what you want
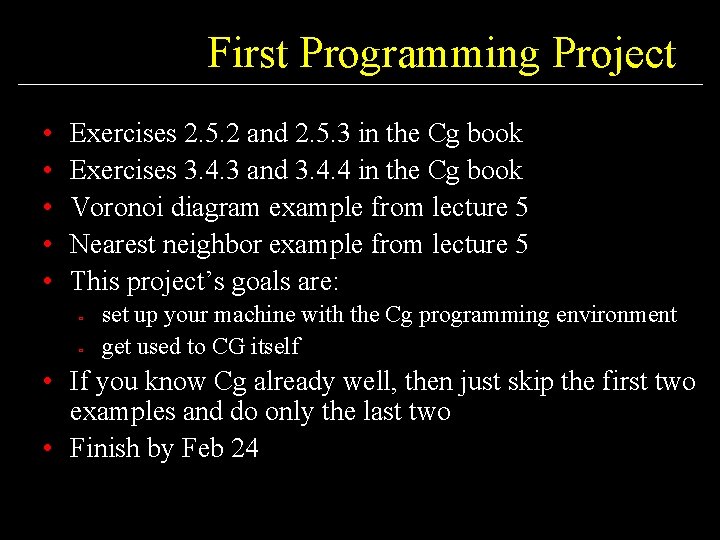
First Programming Project • • • Exercises 2. 5. 2 and 2. 5. 3 in the Cg book Exercises 3. 4. 3 and 3. 4. 4 in the Cg book Voronoi diagram example from lecture 5 Nearest neighbor example from lecture 5 This project’s goals are: ù ù set up your machine with the Cg programming environment get used to CG itself • If you know Cg already well, then just skip the first two examples and do only the last two • Finish by Feb 24