Cse 322 Programming Languages and Compilers Lecture 3
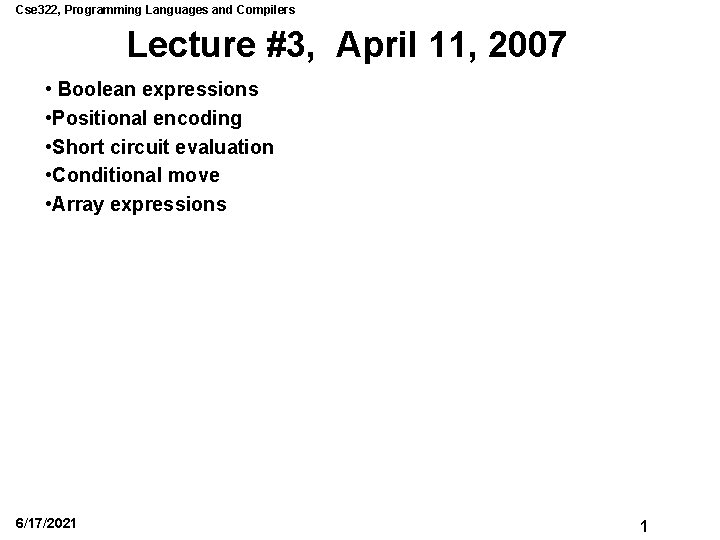
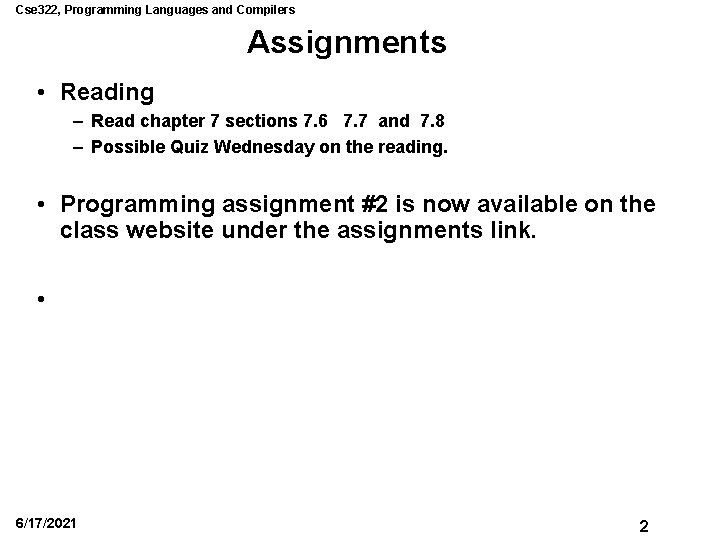
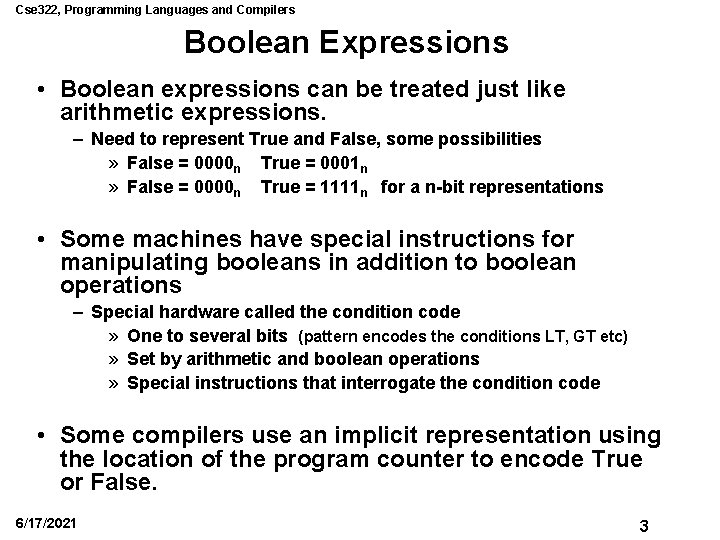
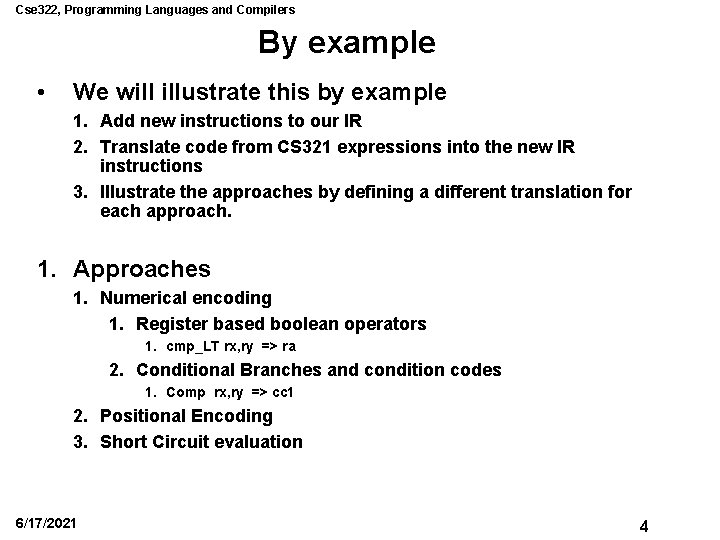
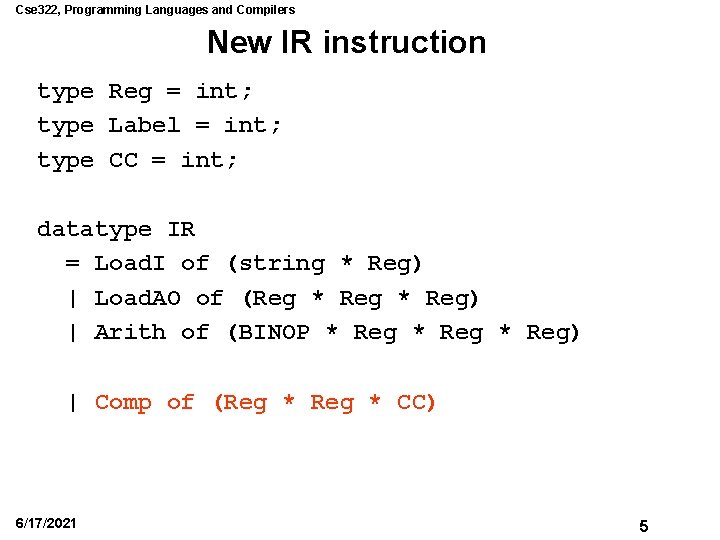
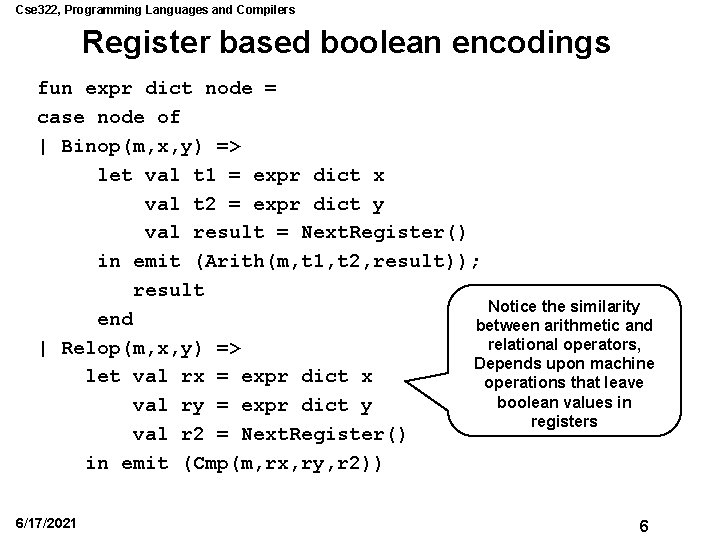
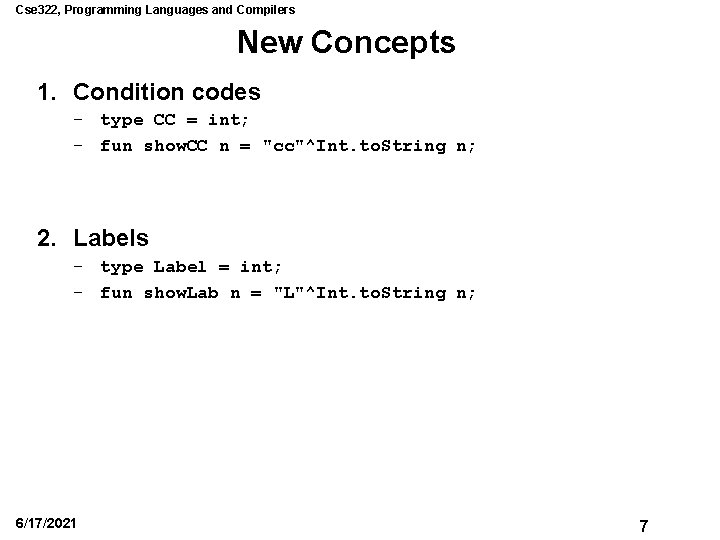
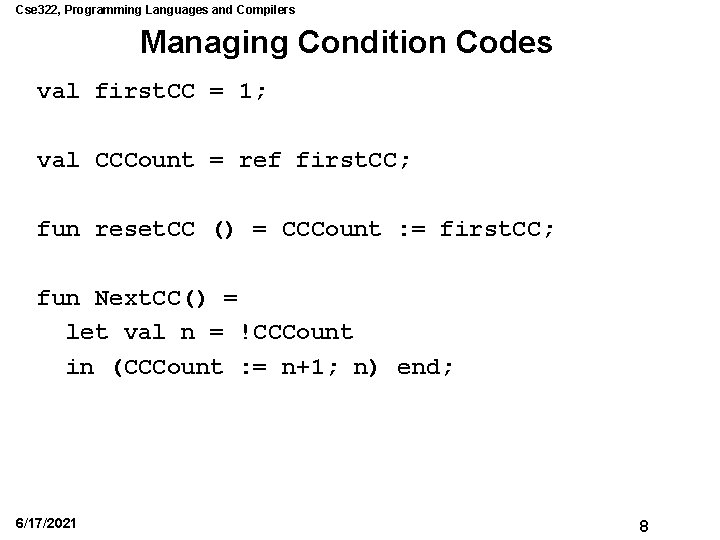
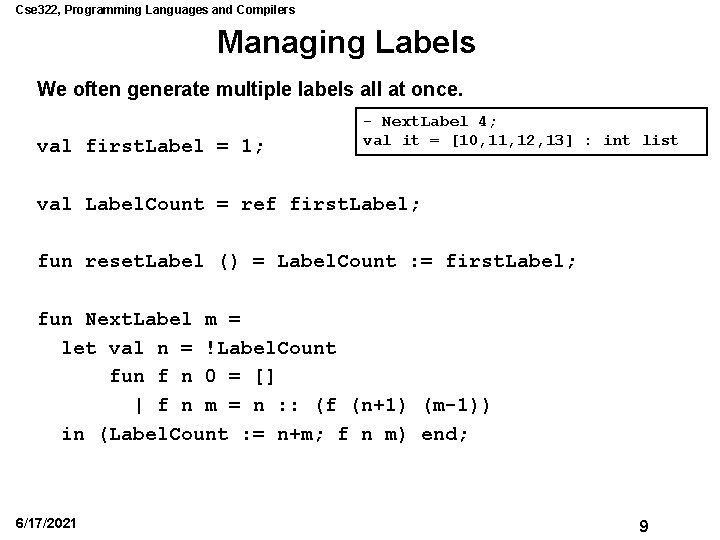
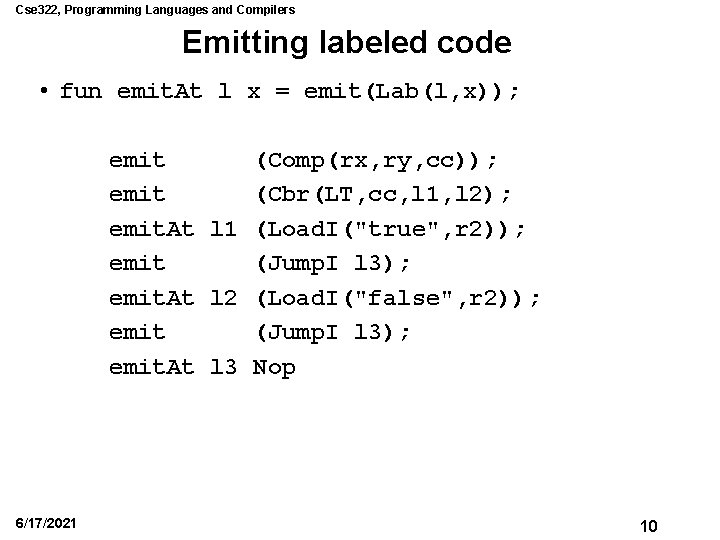
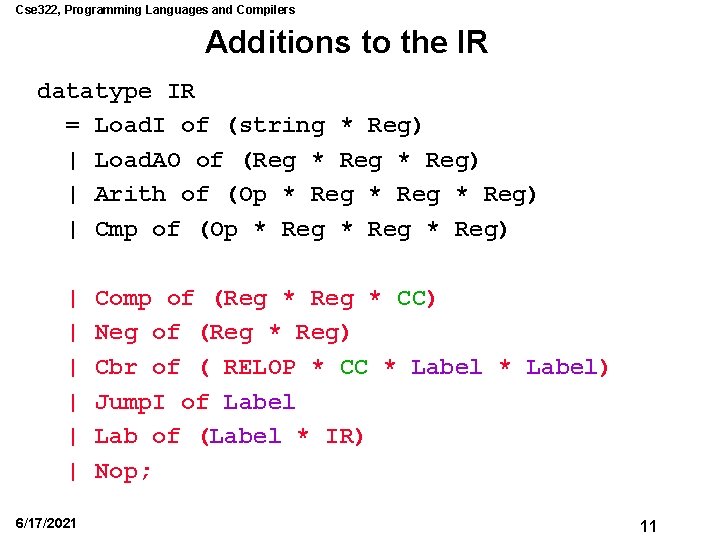
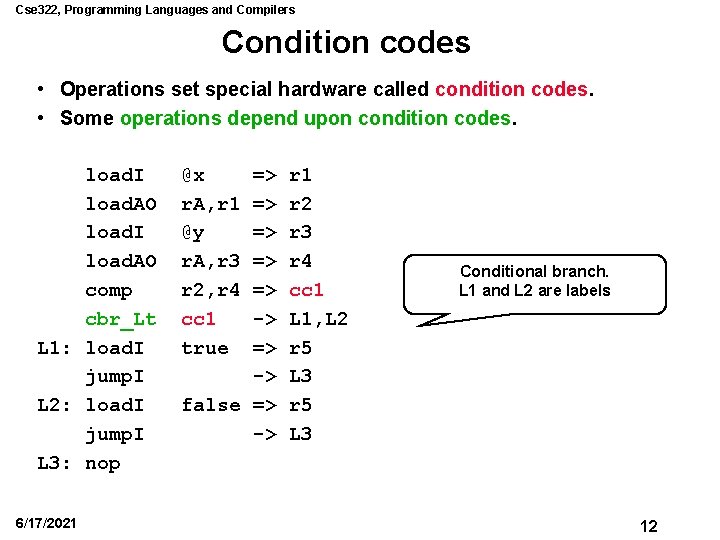
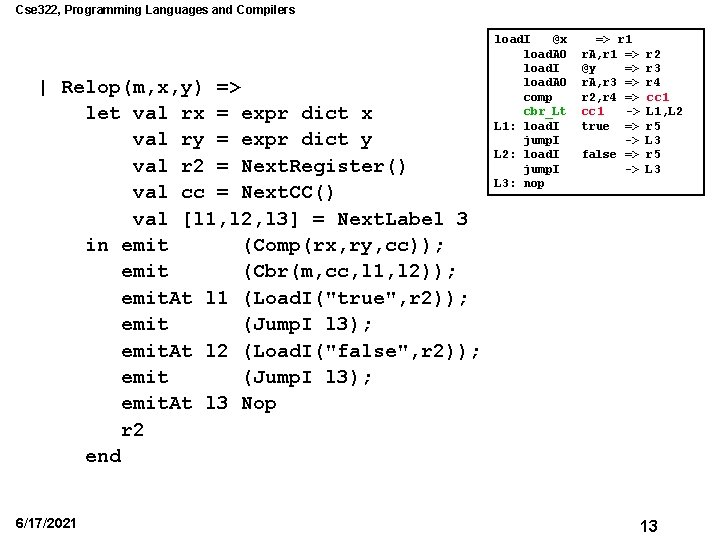
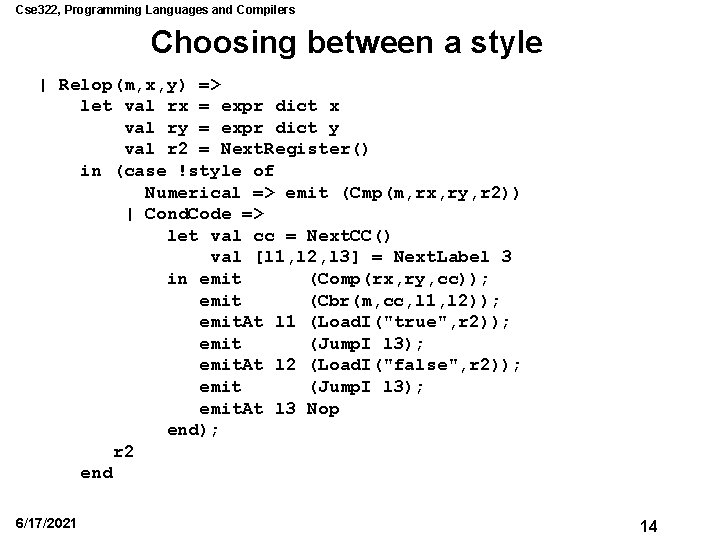
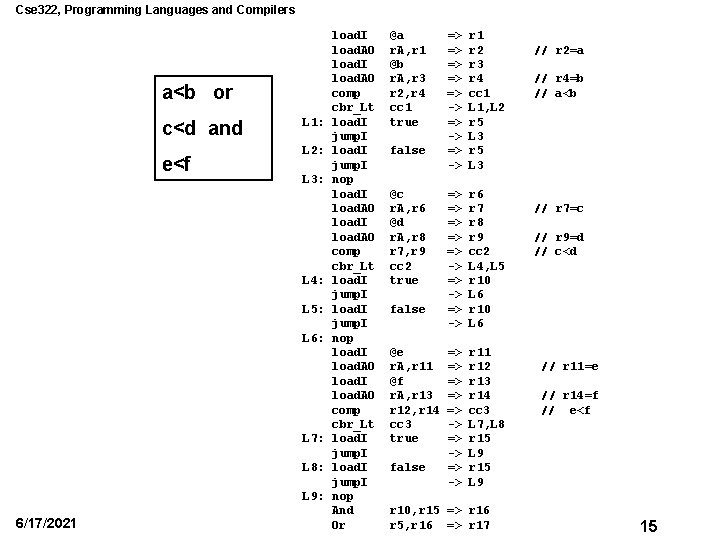
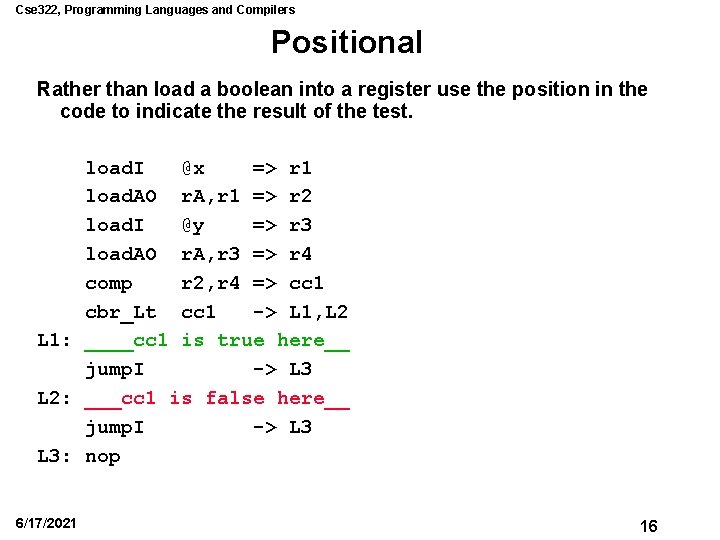
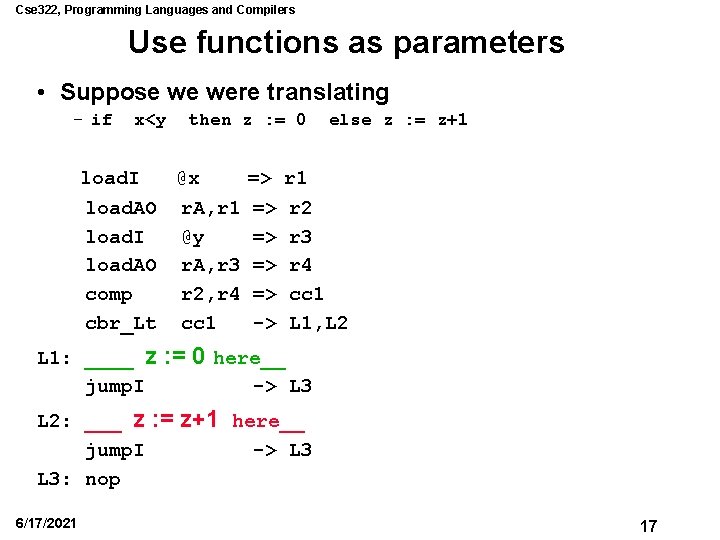
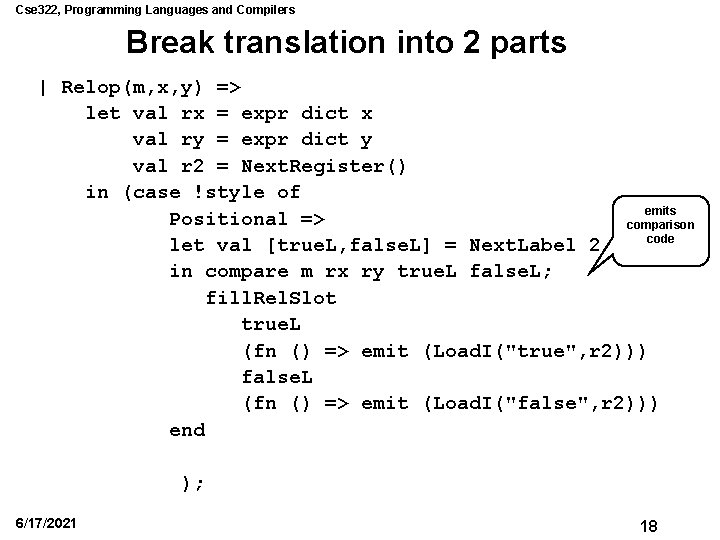
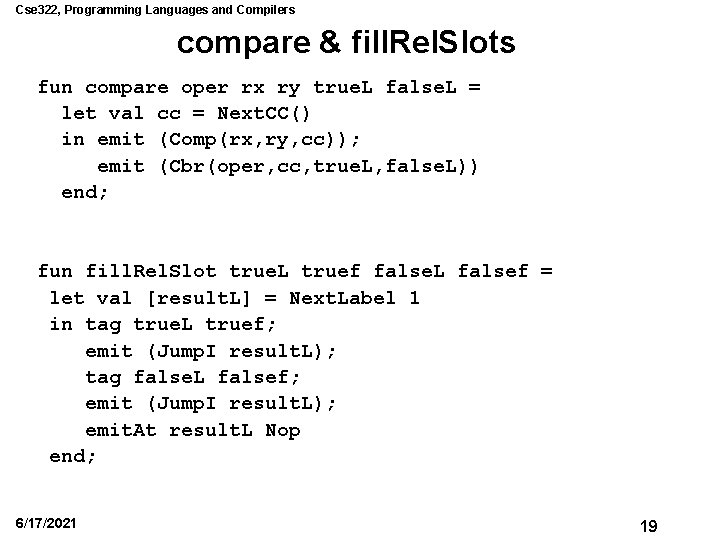
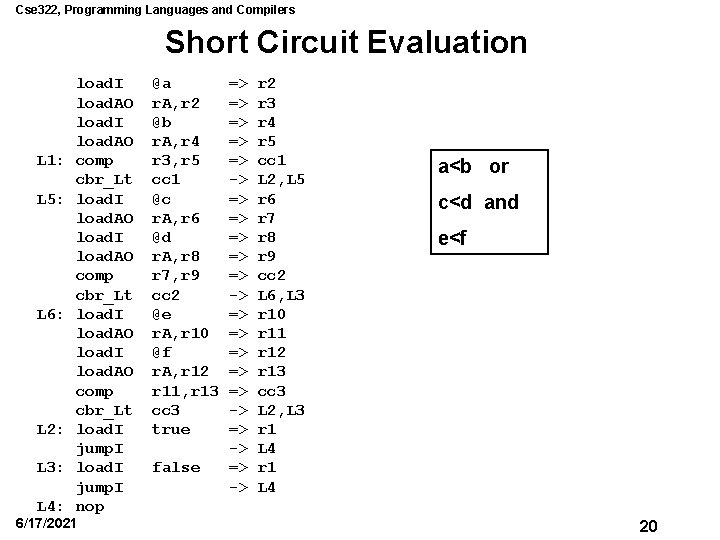
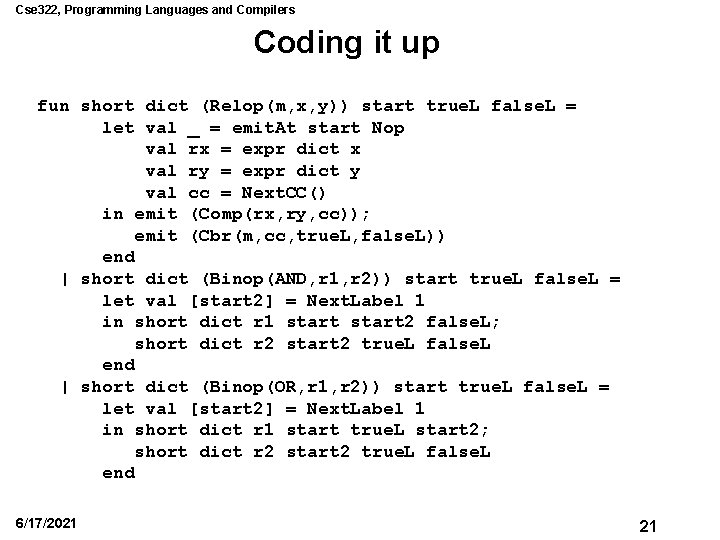
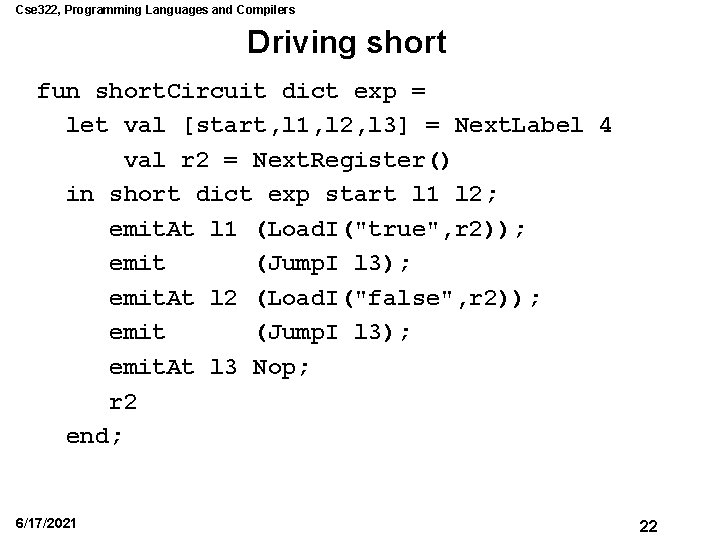
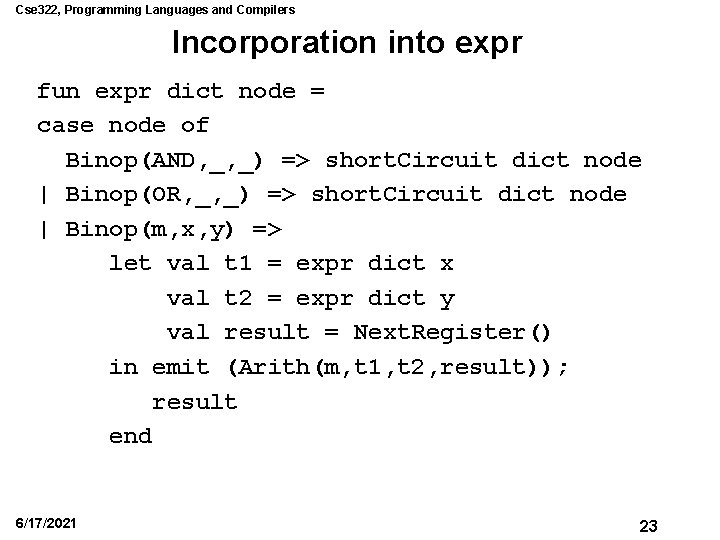
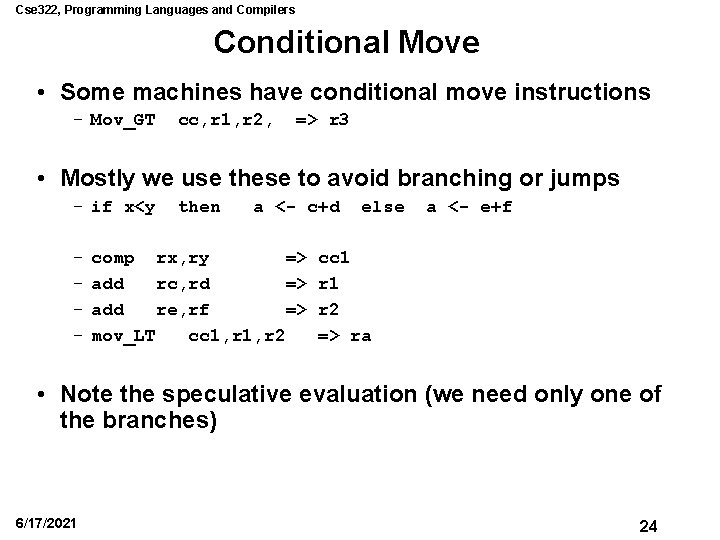
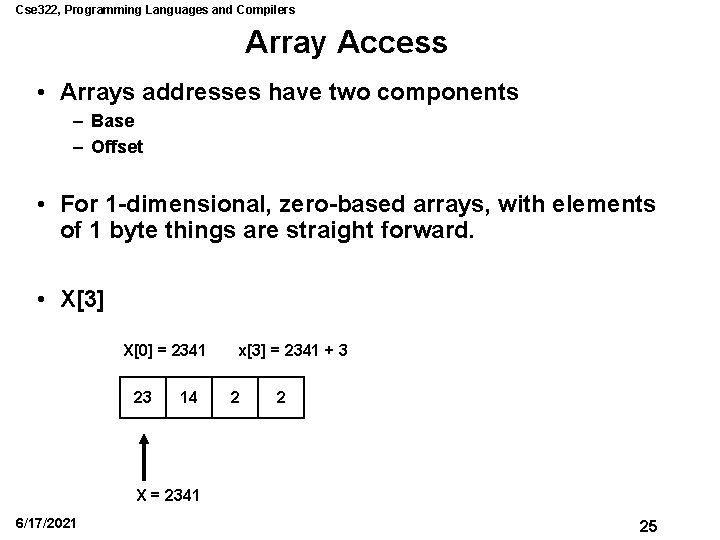
![Cse 322, Programming Languages and Compilers 1 dimensional address calculation • X[y] • Address Cse 322, Programming Languages and Compilers 1 dimensional address calculation • X[y] • Address](https://slidetodoc.com/presentation_image_h2/7c09833967e13293494dec4e4c277793/image-26.jpg)
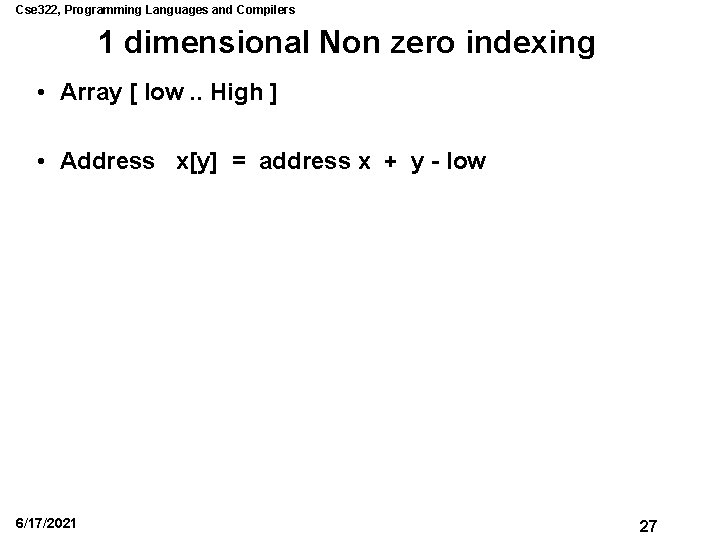
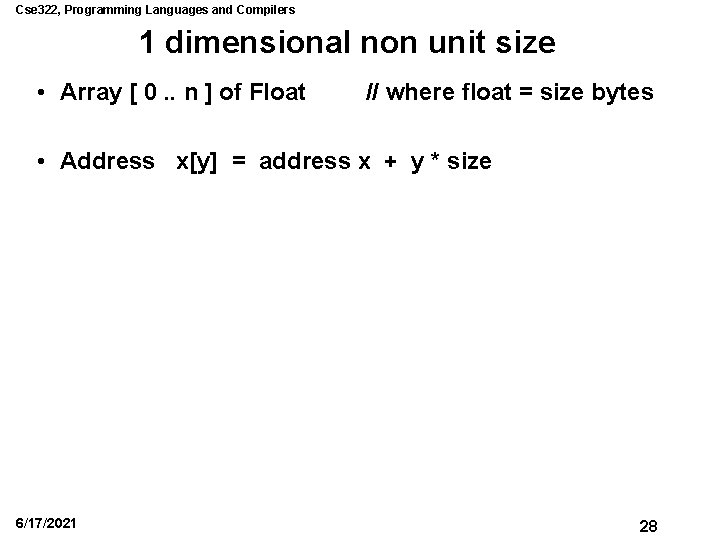
![Cse 322, Programming Languages and Compilers Combined • Array [ low. . High ] Cse 322, Programming Languages and Compilers Combined • Array [ low. . High ]](https://slidetodoc.com/presentation_image_h2/7c09833967e13293494dec4e4c277793/image-29.jpg)
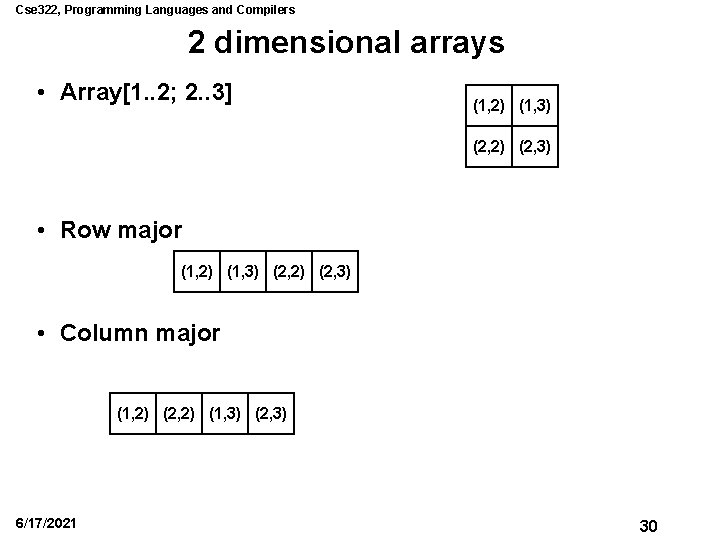
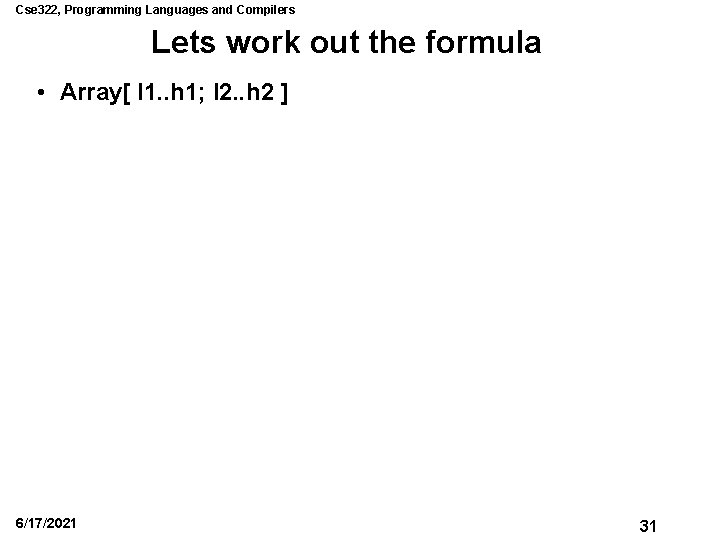
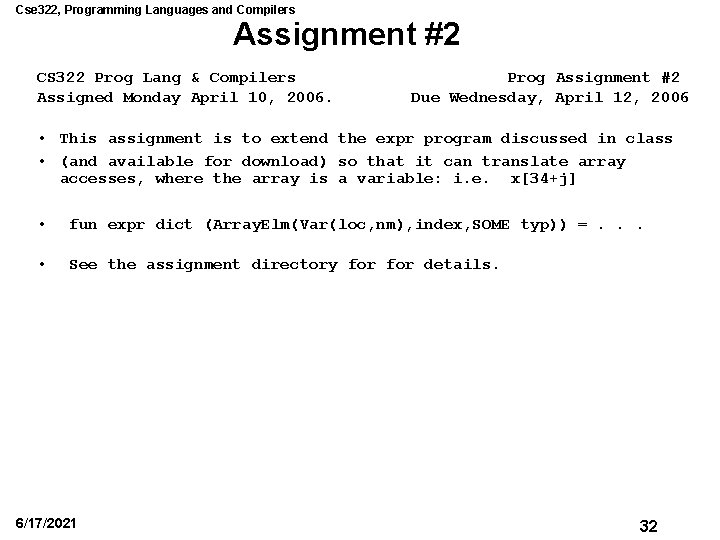
- Slides: 32
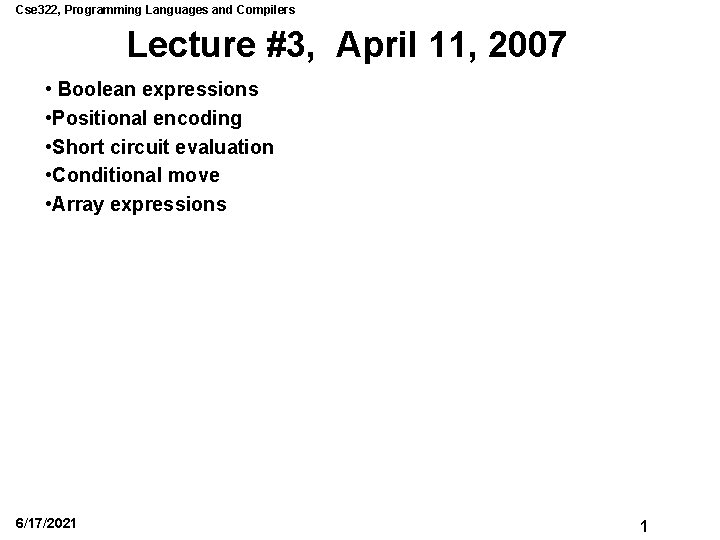
Cse 322, Programming Languages and Compilers Lecture #3, April 11, 2007 • Boolean expressions • Positional encoding • Short circuit evaluation • Conditional move • Array expressions 6/17/2021 1
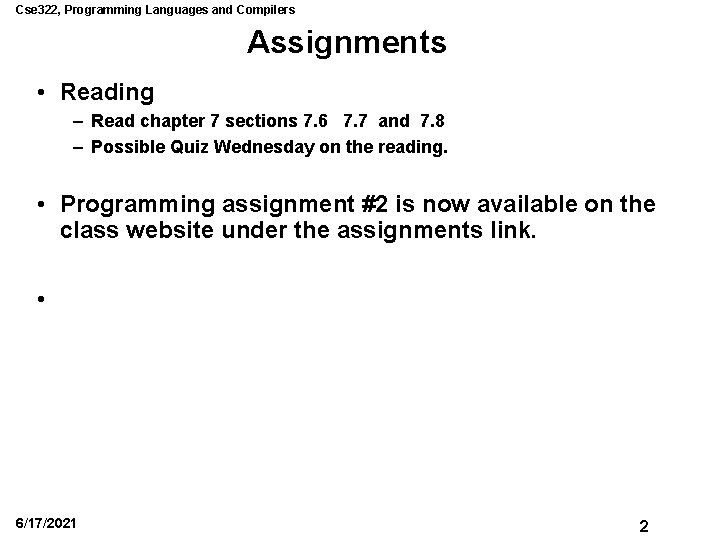
Cse 322, Programming Languages and Compilers Assignments • Reading – Read chapter 7 sections 7. 6 7. 7 and 7. 8 – Possible Quiz Wednesday on the reading. • Programming assignment #2 is now available on the class website under the assignments link. • 6/17/2021 2
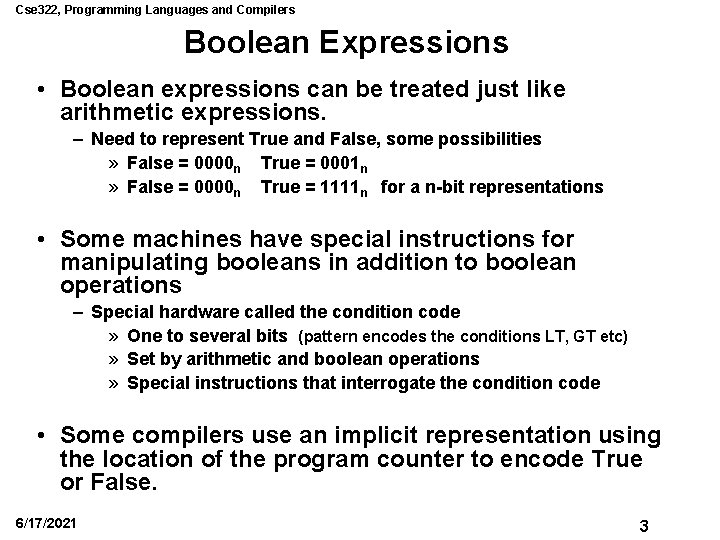
Cse 322, Programming Languages and Compilers Boolean Expressions • Boolean expressions can be treated just like arithmetic expressions. – Need to represent True and False, some possibilities » False = 0000 n True = 0001 n » False = 0000 n True = 1111 n for a n-bit representations • Some machines have special instructions for manipulating booleans in addition to boolean operations – Special hardware called the condition code » One to several bits (pattern encodes the conditions LT, GT etc) » Set by arithmetic and boolean operations » Special instructions that interrogate the condition code • Some compilers use an implicit representation using the location of the program counter to encode True or False. 6/17/2021 3
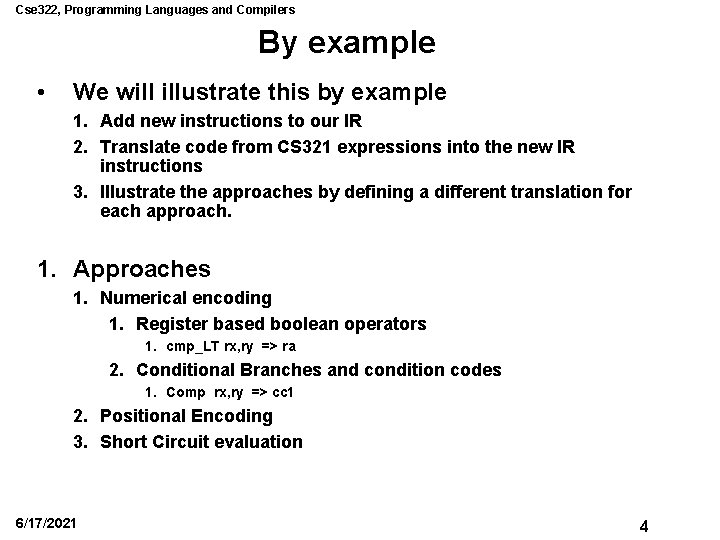
Cse 322, Programming Languages and Compilers By example • We will illustrate this by example 1. Add new instructions to our IR 2. Translate code from CS 321 expressions into the new IR instructions 3. Illustrate the approaches by defining a different translation for each approach. 1. Approaches 1. Numerical encoding 1. Register based boolean operators 1. cmp_LT rx, ry => ra 2. Conditional Branches and condition codes 1. Comp rx, ry => cc 1 2. Positional Encoding 3. Short Circuit evaluation 6/17/2021 4
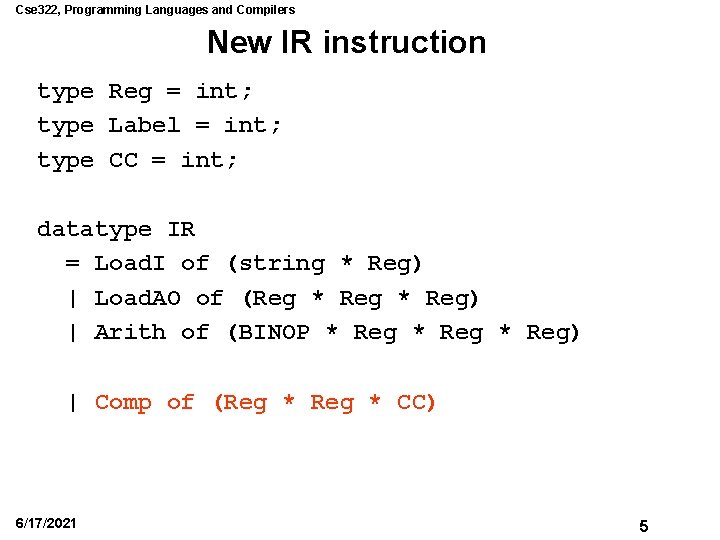
Cse 322, Programming Languages and Compilers New IR instruction type Reg = int; type Label = int; type CC = int; datatype IR = Load. I of (string * Reg) | Load. AO of (Reg * Reg) | Arith of (BINOP * Reg) | Comp of (Reg * CC) 6/17/2021 5
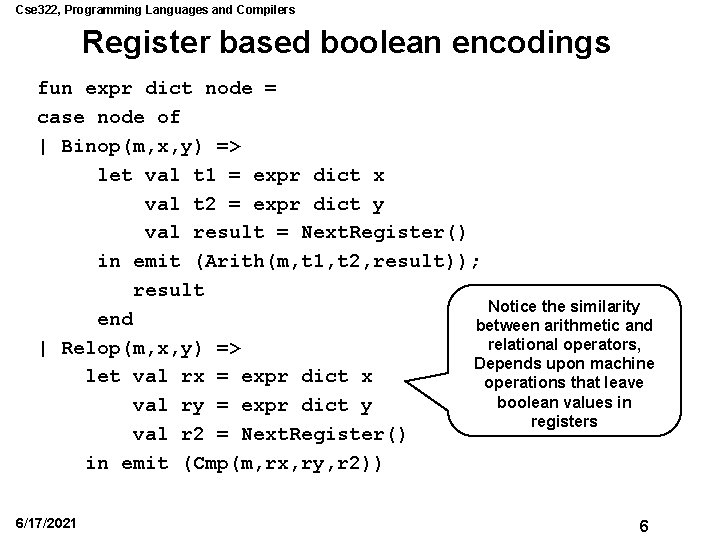
Cse 322, Programming Languages and Compilers Register based boolean encodings fun expr dict node = case node of | Binop(m, x, y) => let val t 1 = expr dict x val t 2 = expr dict y val result = Next. Register() in emit (Arith(m, t 1, t 2, result)); result Notice the similarity end between arithmetic and relational operators, | Relop(m, x, y) => Depends upon machine let val rx = expr dict x operations that leave boolean values in val ry = expr dict y registers val r 2 = Next. Register() in emit (Cmp(m, rx, ry, r 2)) 6/17/2021 6
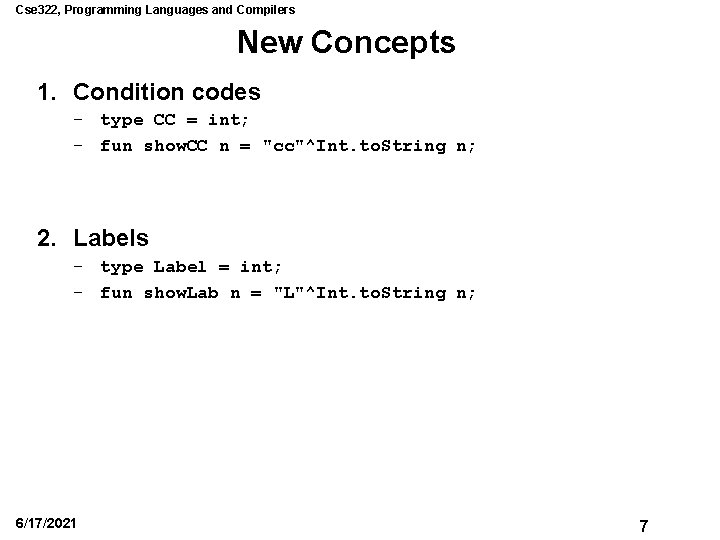
Cse 322, Programming Languages and Compilers New Concepts 1. Condition codes – type CC = int; – fun show. CC n = "cc"^Int. to. String n; 2. Labels – type Label = int; – fun show. Lab n = "L"^Int. to. String n; 6/17/2021 7
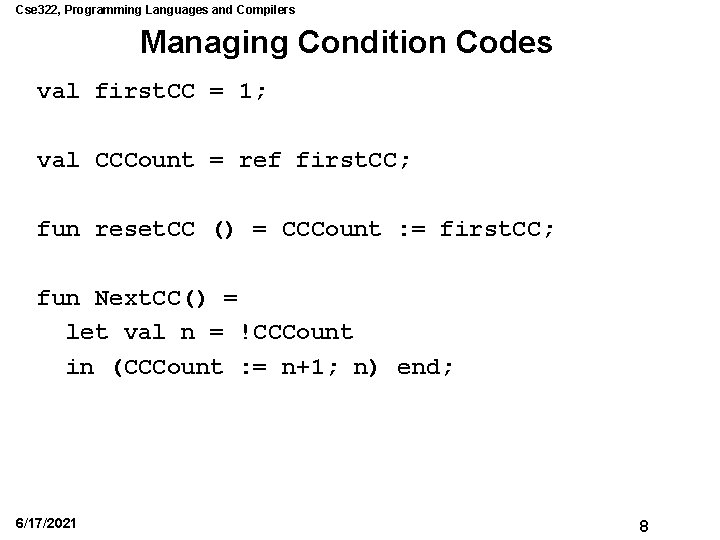
Cse 322, Programming Languages and Compilers Managing Condition Codes val first. CC = 1; val CCCount = ref first. CC; fun reset. CC () = CCCount : = first. CC; fun Next. CC() = let val n = !CCCount in (CCCount : = n+1; n) end; 6/17/2021 8
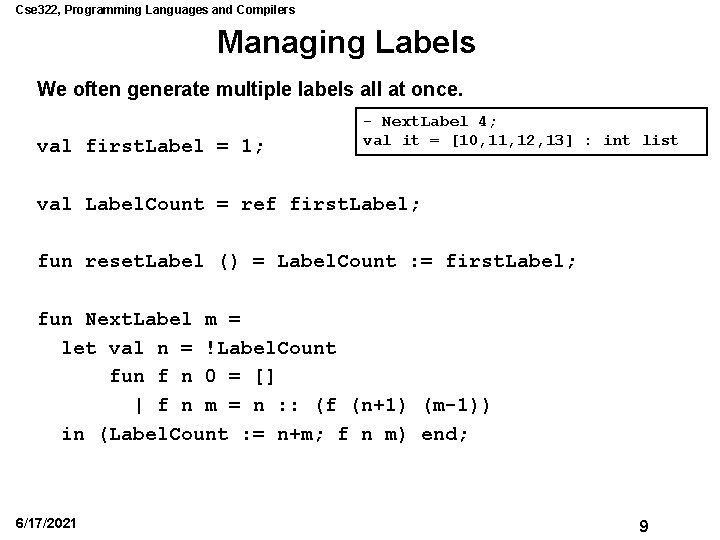
Cse 322, Programming Languages and Compilers Managing Labels We often generate multiple labels all at once. val first. Label = 1; - Next. Label 4; val it = [10, 11, 12, 13] : int list val Label. Count = ref first. Label; fun reset. Label () = Label. Count : = first. Label; fun Next. Label m = let val n = !Label. Count fun f n 0 = [] | f n m = n : : (f (n+1) (m-1)) in (Label. Count : = n+m; f n m) end; 6/17/2021 9
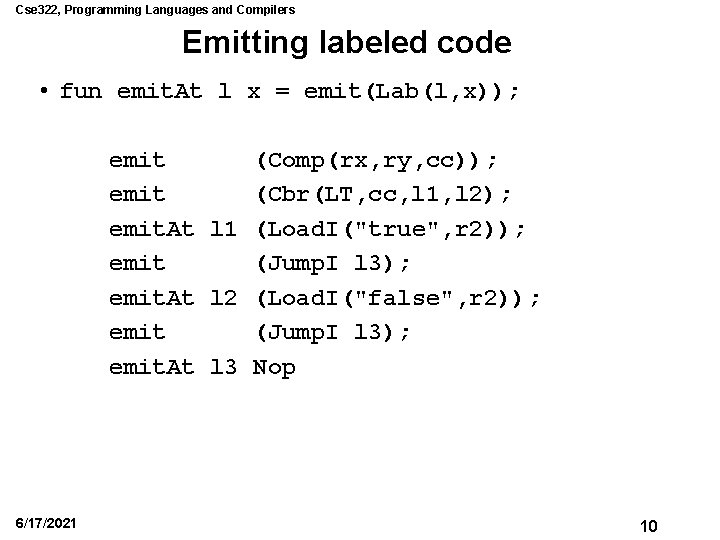
Cse 322, Programming Languages and Compilers Emitting labeled code • fun emit. At l x = emit(Lab(l, x)); emit (Comp(rx, ry, cc)); emit (Cbr(LT, cc, l 1, l 2); emit. At l 1 (Load. I("true", r 2)); emit (Jump. I l 3); emit. At l 2 (Load. I("false", r 2)); emit (Jump. I l 3); emit. At l 3 Nop 6/17/2021 10
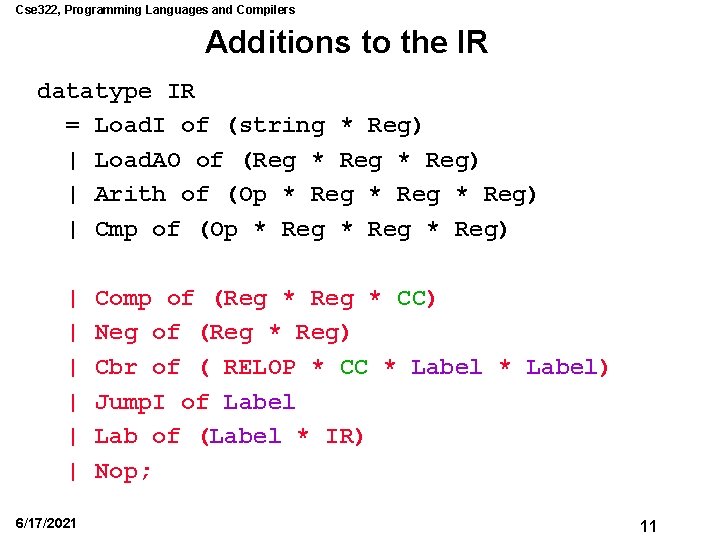
Cse 322, Programming Languages and Compilers Additions to the IR datatype IR = Load. I of (string * Reg) | Load. AO of (Reg * Reg) | Arith of (Op * Reg) | Cmp of (Op * Reg) | | | 6/17/2021 Comp of (Reg * CC) Neg of (Reg * Reg) Cbr of ( RELOP * CC * Label) Jump. I of Label Lab of (Label * IR) Nop; 11
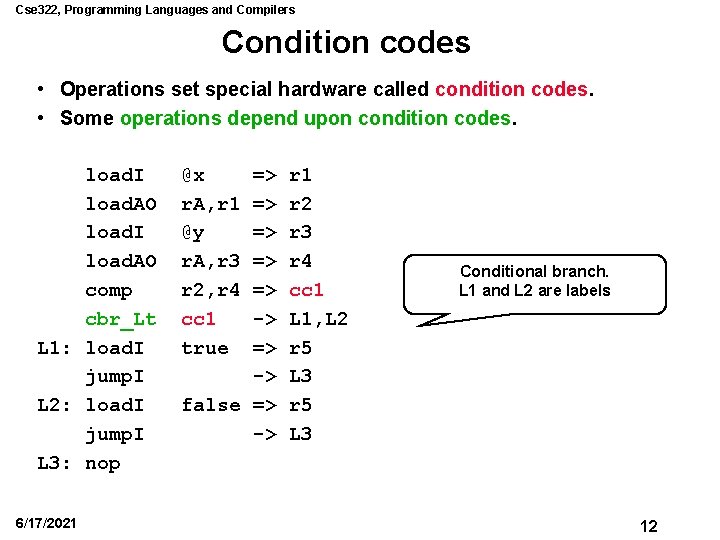
Cse 322, Programming Languages and Compilers Condition codes • Operations set special hardware called condition codes. • Some operations depend upon condition codes. load. I load. AO comp cbr_Lt L 1: load. I jump. I L 2: load. I jump. I L 3: nop 6/17/2021 @x r. A, r 1 @y r. A, r 3 r 2, r 4 cc 1 true => => => -> false => -> r 1 r 2 r 3 r 4 cc 1 L 1, L 2 r 5 L 3 Conditional branch. L 1 and L 2 are labels 12
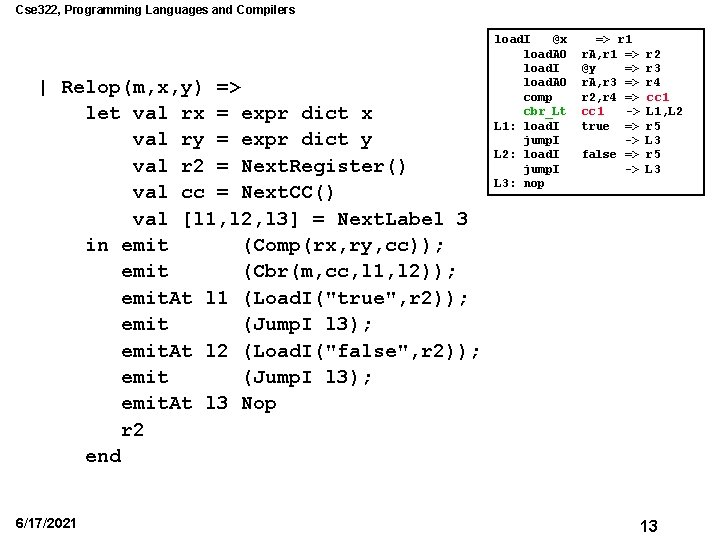
Cse 322, Programming Languages and Compilers | Relop(m, x, y) => let val rx = expr dict x val ry = expr dict y val r 2 = Next. Register() val cc = Next. CC() val [l 1, l 2, l 3] = Next. Label 3 in emit (Comp(rx, ry, cc)); emit (Cbr(m, cc, l 1, l 2)); emit. At l 1 (Load. I("true", r 2)); emit (Jump. I l 3); emit. At l 2 (Load. I("false", r 2)); emit (Jump. I l 3); emit. At l 3 Nop r 2 end 6/17/2021 load. I @x load. AO load. I load. AO comp cbr_Lt L 1: load. I jump. I L 2: load. I jump. I L 3: nop => r 1 r. A, r 1 => @y => r. A, r 3 => r 2, r 4 => cc 1 -> true => -> false => -> r 2 r 3 r 4 cc 1 L 1, L 2 r 5 L 3 13
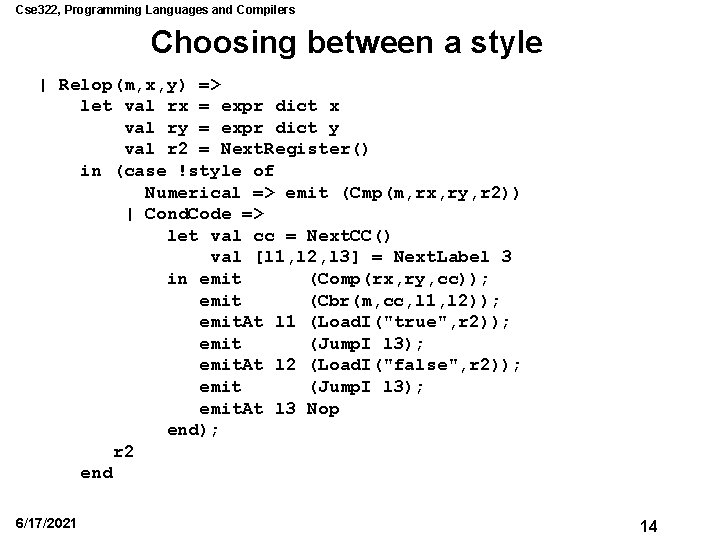
Cse 322, Programming Languages and Compilers Choosing between a style | Relop(m, x, y) => let val rx = expr dict x val ry = expr dict y val r 2 = Next. Register() in (case !style of Numerical => emit (Cmp(m, rx, ry, r 2)) | Cond. Code => let val cc = Next. CC() val [l 1, l 2, l 3] = Next. Label 3 in emit (Comp(rx, ry, cc)); emit (Cbr(m, cc, l 1, l 2)); emit. At l 1 (Load. I("true", r 2)); emit (Jump. I l 3); emit. At l 2 (Load. I("false", r 2)); emit (Jump. I l 3); emit. At l 3 Nop end); r 2 end 6/17/2021 14
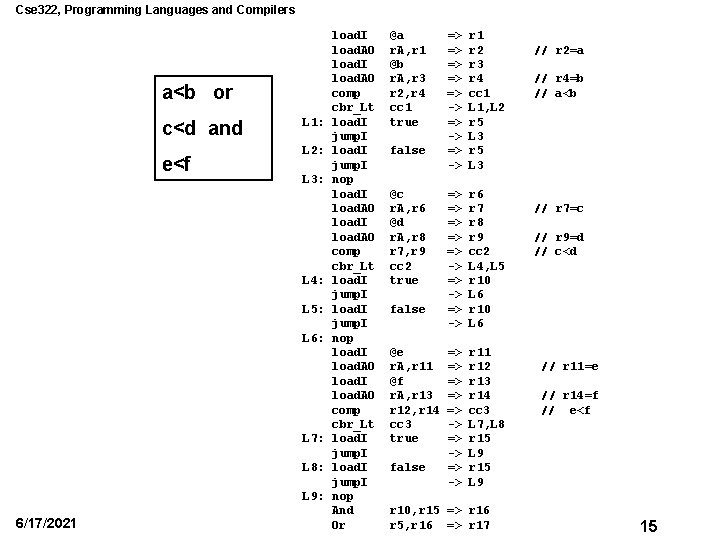
Cse 322, Programming Languages and Compilers a<b or c<d and e<f L 1: L 2: L 3: L 4: L 5: L 6: L 7: L 8: L 9: 6/17/2021 load. I load. AO comp cbr_Lt load. I jump. I nop load. I load. AO comp cbr_Lt load. I jump. I nop And Or @a r. A, r 1 @b r. A, r 3 r 2, r 4 cc 1 true false @c r. A, r 6 @d r. A, r 8 r 7, r 9 cc 2 true false @e r. A, r 11 @f r. A, r 13 r 12, r 14 cc 3 true false => => => -> r 1 r 2 r 3 r 4 cc 1 L 1, L 2 r 5 L 3 => => => -> r 6 r 7 r 8 r 9 cc 2 L 4, L 5 r 10 L 6 => => => -> r 11 r 12 r 13 r 14 cc 3 L 7, L 8 r 15 L 9 r 10, r 15 => r 16 r 5, r 16 => r 17 // r 2=a // r 4=b // a<b // r 7=c // r 9=d // c<d // r 11=e // r 14=f // e<f 15
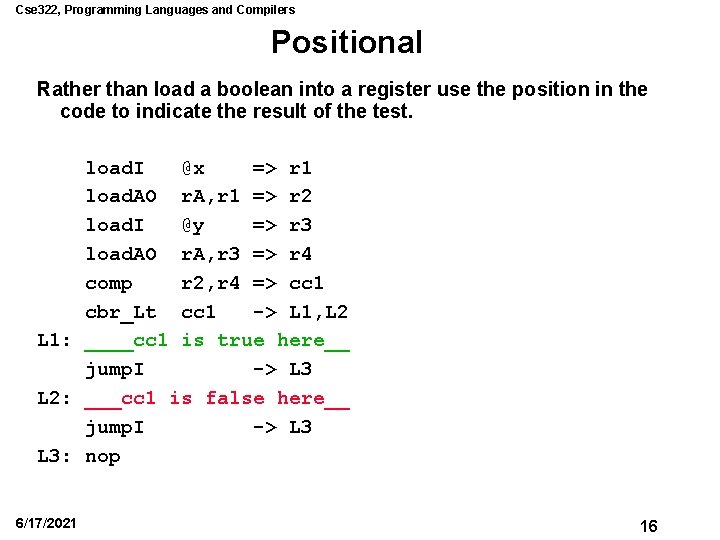
Cse 322, Programming Languages and Compilers Positional Rather than load a boolean into a register use the position in the code to indicate the result of the test. load. I @x => r 1 load. AO r. A, r 1 => r 2 load. I @y => r 3 load. AO r. A, r 3 => r 4 comp r 2, r 4 => cc 1 cbr_Lt cc 1 -> L 1, L 2 L 1: ____cc 1 is true here__ jump. I -> L 3 L 2: ___cc 1 is false here__ jump. I -> L 3: nop 6/17/2021 16
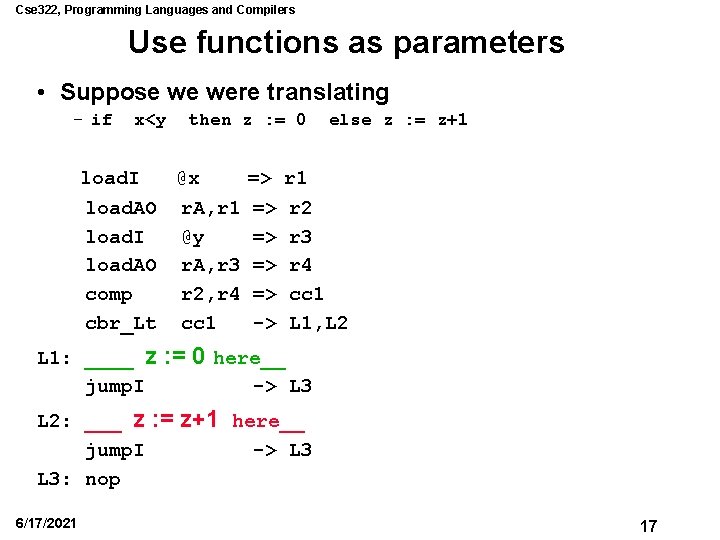
Cse 322, Programming Languages and Compilers Use functions as parameters • Suppose we were translating – if x<y then z : = 0 load. I @x load. AO r. A, r 1 load. I @y load. AO r. A, r 3 comp r 2, r 4 cbr_Lt cc 1 L 1: ____ z jump. I L 2: ___ z jump. I L 3: nop 6/17/2021 => => => -> else z : = z+1 r 2 r 3 r 4 cc 1 L 1, L 2 : = 0 here__ -> L 3 : = z+1 here__ -> L 3 17
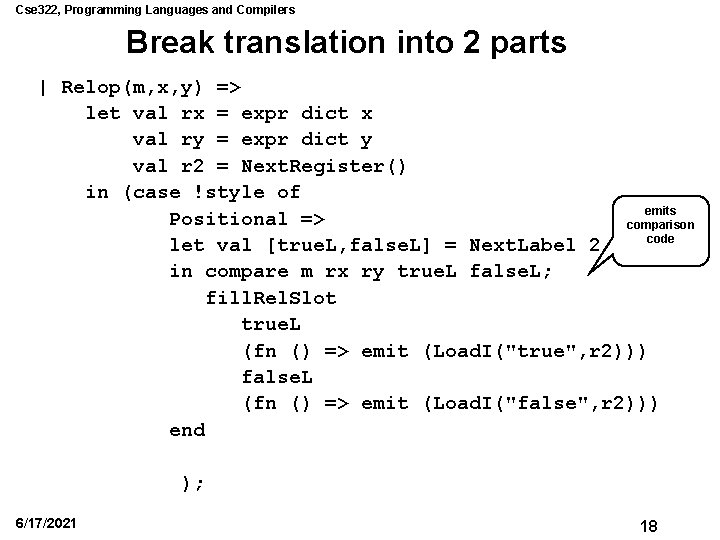
Cse 322, Programming Languages and Compilers Break translation into 2 parts | Relop(m, x, y) => let val rx = expr dict x val ry = expr dict y val r 2 = Next. Register() in (case !style of emits Positional => comparison code let val [true. L, false. L] = Next. Label 2 in compare m rx ry true. L false. L; fill. Rel. Slot true. L (fn () => emit (Load. I("true", r 2))) false. L (fn () => emit (Load. I("false", r 2))) end ); 6/17/2021 18
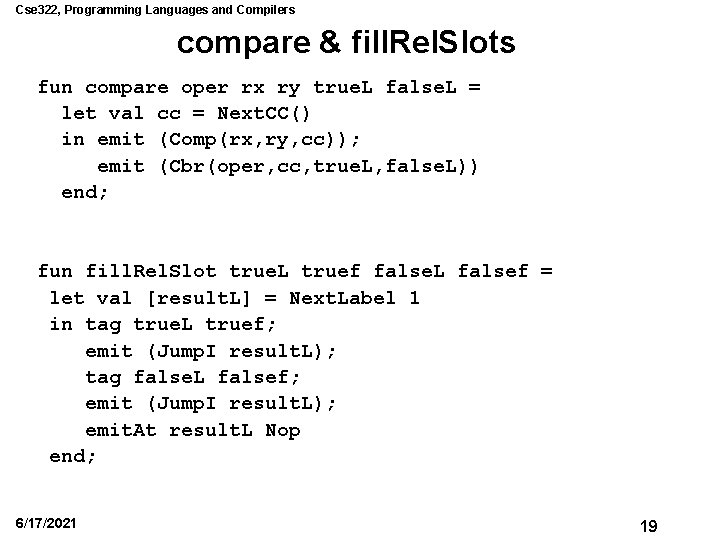
Cse 322, Programming Languages and Compilers compare & fill. Rel. Slots fun compare oper rx ry true. L false. L = let val cc = Next. CC() in emit (Comp(rx, ry, cc)); emit (Cbr(oper, cc, true. L, false. L)) end; fun fill. Rel. Slot true. L truef false. L falsef = let val [result. L] = Next. Label 1 in tag true. L truef; emit (Jump. I result. L); tag false. L falsef; emit (Jump. I result. L); emit. At result. L Nop end; 6/17/2021 19
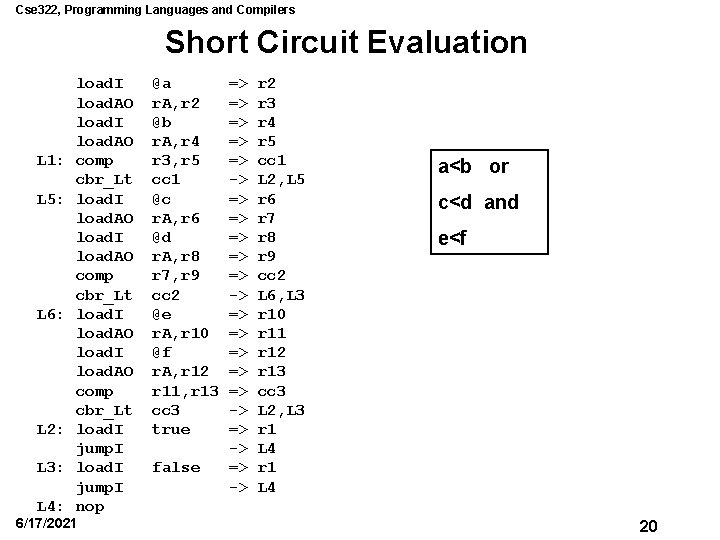
Cse 322, Programming Languages and Compilers Short Circuit Evaluation L 1: L 5: L 6: L 2: L 3: L 4: load. I load. AO comp cbr_Lt load. I jump. I nop 6/17/2021 @a r. A, r 2 @b r. A, r 4 r 3, r 5 cc 1 @c r. A, r 6 @d r. A, r 8 r 7, r 9 cc 2 @e r. A, r 10 @f r. A, r 12 r 11, r 13 cc 3 true false => => => -> => => => -> r 2 r 3 r 4 r 5 cc 1 L 2, L 5 r 6 r 7 r 8 r 9 cc 2 L 6, L 3 r 10 r 11 r 12 r 13 cc 3 L 2, L 3 r 1 L 4 a<b or c<d and e<f 20
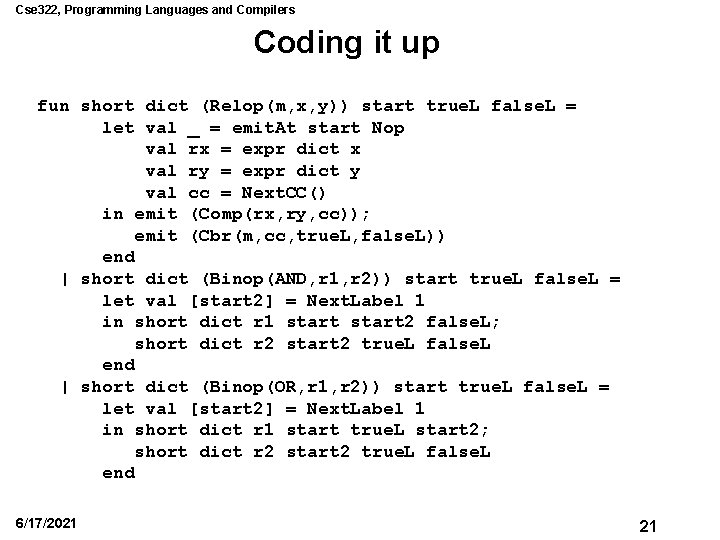
Cse 322, Programming Languages and Compilers Coding it up fun short dict (Relop(m, x, y)) start true. L false. L = let val _ = emit. At start Nop val rx = expr dict x val ry = expr dict y val cc = Next. CC() in emit (Comp(rx, ry, cc)); emit (Cbr(m, cc, true. L, false. L)) end | short dict (Binop(AND, r 1, r 2)) start true. L false. L = let val [start 2] = Next. Label 1 in short dict r 1 start 2 false. L; short dict r 2 start 2 true. L false. L end | short dict (Binop(OR, r 1, r 2)) start true. L false. L = let val [start 2] = Next. Label 1 in short dict r 1 start true. L start 2; short dict r 2 start 2 true. L false. L end 6/17/2021 21
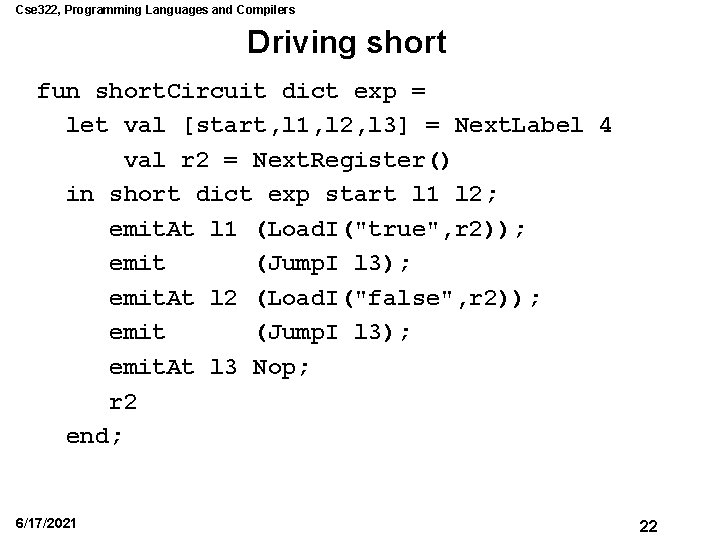
Cse 322, Programming Languages and Compilers Driving short fun short. Circuit dict exp = let val [start, l 1, l 2, l 3] = Next. Label 4 val r 2 = Next. Register() in short dict exp start l 1 l 2; emit. At l 1 (Load. I("true", r 2)); emit (Jump. I l 3); emit. At l 2 (Load. I("false", r 2)); emit (Jump. I l 3); emit. At l 3 Nop; r 2 end; 6/17/2021 22
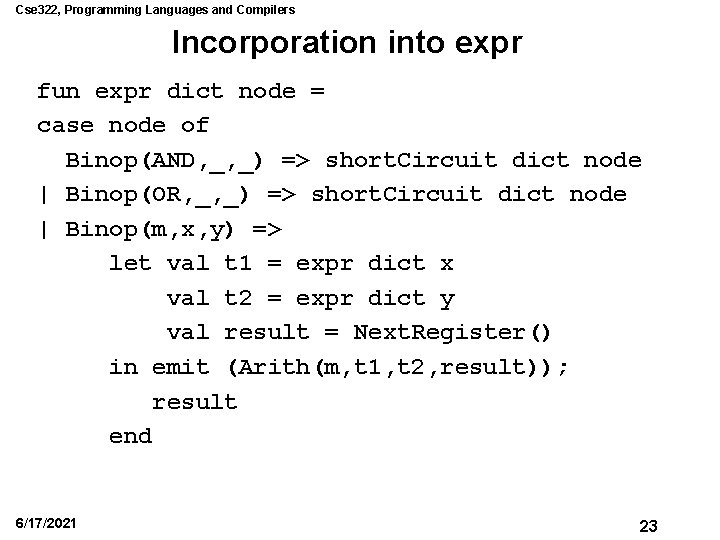
Cse 322, Programming Languages and Compilers Incorporation into expr fun expr dict node = case node of Binop(AND, _, _) => short. Circuit dict node | Binop(OR, _, _) => short. Circuit dict node | Binop(m, x, y) => let val t 1 = expr dict x val t 2 = expr dict y val result = Next. Register() in emit (Arith(m, t 1, t 2, result)); result end 6/17/2021 23
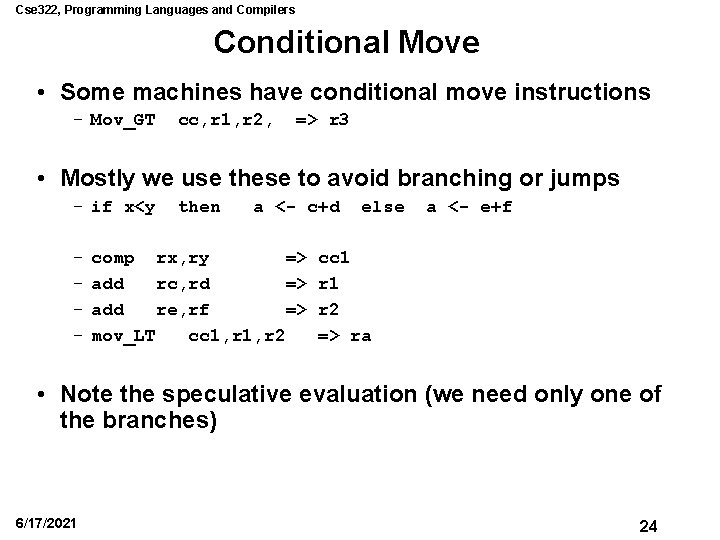
Cse 322, Programming Languages and Compilers Conditional Move • Some machines have conditional move instructions – Mov_GT cc, r 1, r 2, => r 3 • Mostly we use these to avoid branching or jumps – if x<y – – then a <- c+d comp rx, ry => add rc, rd => add re, rf => mov_LT cc 1, r 2 else a <- e+f cc 1 r 2 => ra • Note the speculative evaluation (we need only one of the branches) 6/17/2021 24
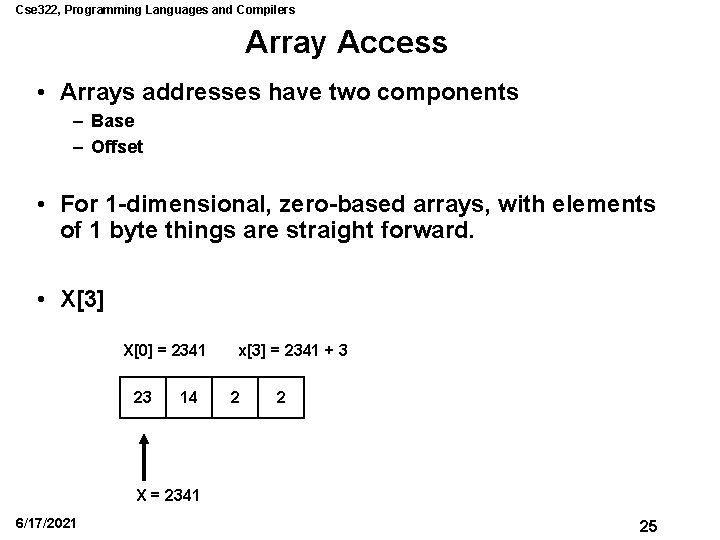
Cse 322, Programming Languages and Compilers Array Access • Arrays addresses have two components – Base – Offset • For 1 -dimensional, zero-based arrays, with elements of 1 byte things are straight forward. • X[3] X[0] = 2341 23 14 x[3] = 2341 + 3 2 2 X = 2341 6/17/2021 25
![Cse 322 Programming Languages and Compilers 1 dimensional address calculation Xy Address Cse 322, Programming Languages and Compilers 1 dimensional address calculation • X[y] • Address](https://slidetodoc.com/presentation_image_h2/7c09833967e13293494dec4e4c277793/image-26.jpg)
Cse 322, Programming Languages and Compilers 1 dimensional address calculation • X[y] • Address of x[y] = address of x + y load. I load. A 0 load. I add load 6/17/2021 @y ra, r 1 @x r 1, r 2 r 3 => => => r 1 r 2 r 3 r 4 26
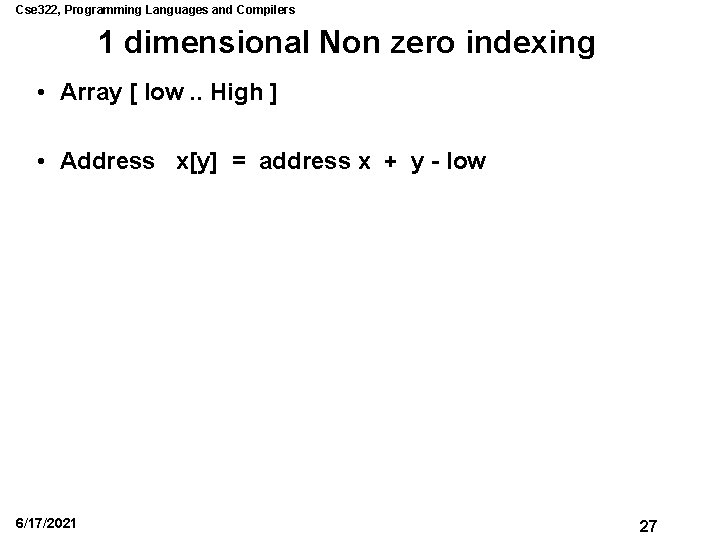
Cse 322, Programming Languages and Compilers 1 dimensional Non zero indexing • Array [ low. . High ] • Address x[y] = address x + y - low 6/17/2021 27
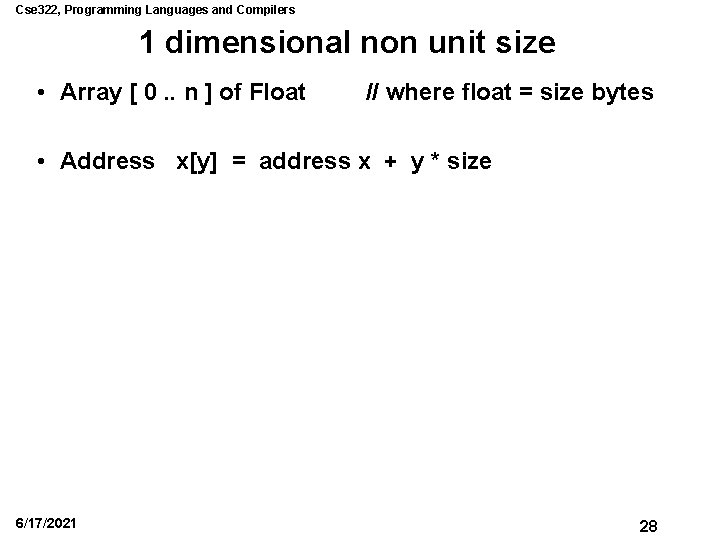
Cse 322, Programming Languages and Compilers 1 dimensional non unit size • Array [ 0. . n ] of Float // where float = size bytes • Address x[y] = address x + y * size 6/17/2021 28
![Cse 322 Programming Languages and Compilers Combined Array low High Cse 322, Programming Languages and Compilers Combined • Array [ low. . High ]](https://slidetodoc.com/presentation_image_h2/7c09833967e13293494dec4e4c277793/image-29.jpg)
Cse 322, Programming Languages and Compilers Combined • Array [ low. . High ] of Float bytes // where float = size • Address x[y] = address x + (y – low) * size • Optimization • Address x[y] = address x + (y * size) – (low * size) • Address x[y] = address x – (low * size) + (y * size) Perhaps this is known at compile-time? 6/17/2021 Performed with a shift if size is a power of 2 29
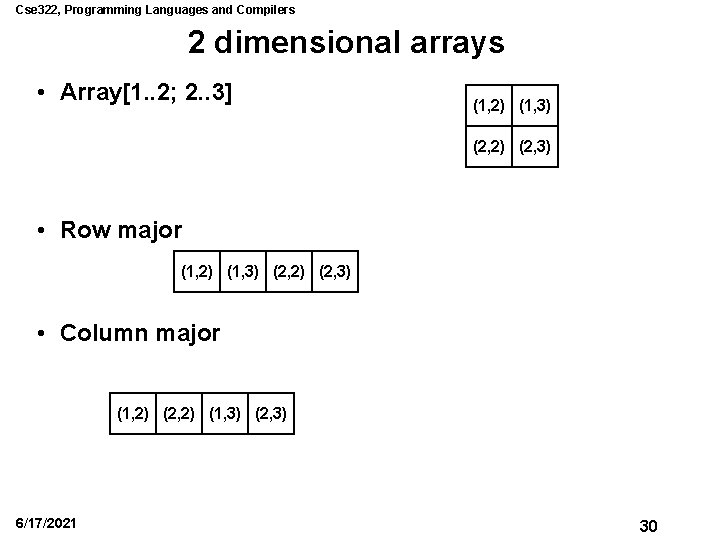
Cse 322, Programming Languages and Compilers 2 dimensional arrays • Array[1. . 2; 2. . 3] (1, 2) (1, 3) (2, 2) (2, 3) • Row major (1, 2) (1, 3) (2, 2) (2, 3) • Column major (1, 2) (2, 2) (1, 3) (2, 3) 6/17/2021 30
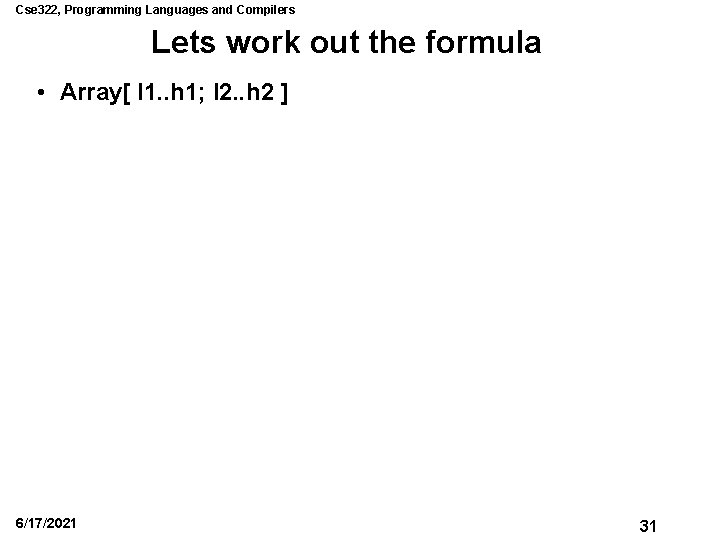
Cse 322, Programming Languages and Compilers Lets work out the formula • Array[ l 1. . h 1; l 2. . h 2 ] 6/17/2021 31
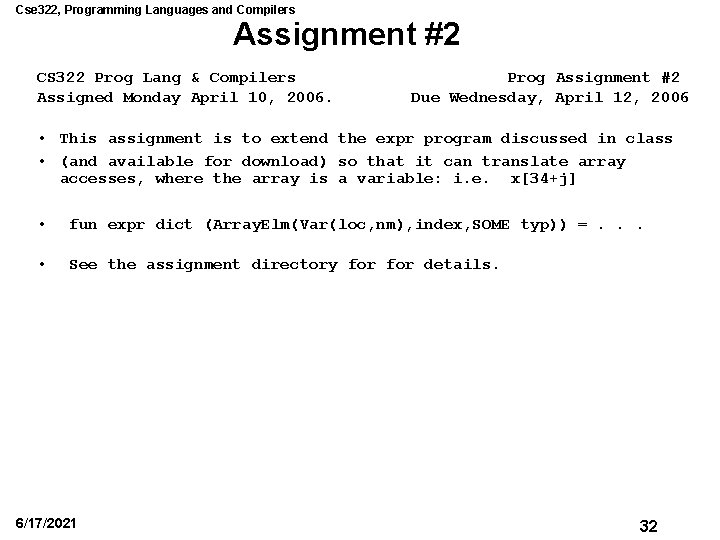
Cse 322, Programming Languages and Compilers Assignment #2 CS 322 Prog Lang & Compilers Assigned Monday April 10, 2006. Prog Assignment #2 Due Wednesday, April 12, 2006 • This assignment is to extend the expr program discussed in class • (and available for download) so that it can translate array accesses, where the array is a variable: i. e. x[34+j] • fun expr dict (Array. Elm(Var(loc, nm), index, SOME typ)) =. . . • See the assignment directory for details. 6/17/2021 32
Cs 421 uiuc
Cs 421 programming languages and compilers
Cse 340 principles of programming languages
Vineeth kashyap
What is an interpreter
Finding and understanding bugs in c compilers
Yacc symbol table
If an error occurs, what interpreter do
Real-time systems and programming languages
Advantages of high level language
Real time programming language
Binarymove compilers
Cross compilers
Crafting a compiler with c
Basic compiler functions
Front end and back end compiler
01:640:244 lecture notes - lecture 15: plat, idah, farad
Multithreading program in java
Cxc it
Introduction to programming languages
Plc coding language
Joey paquet
Comparative programming languages
Alternative programming languages
Strongly typed vs weakly typed
Transmission programming languages
Integral data type in c
Xenia programming languages
Mainstream programming languages
Programming languages
Programming languages
Programming languages
Programming languages