CSCICMPE 4341 Topic Programming in Python Chapter 6
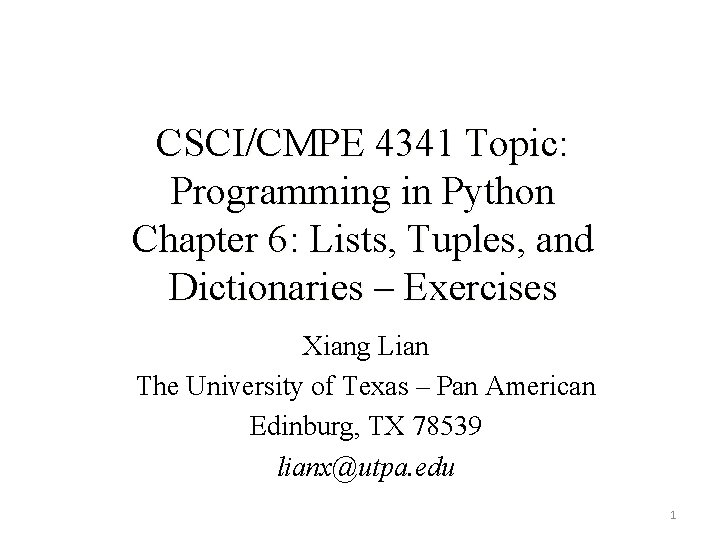
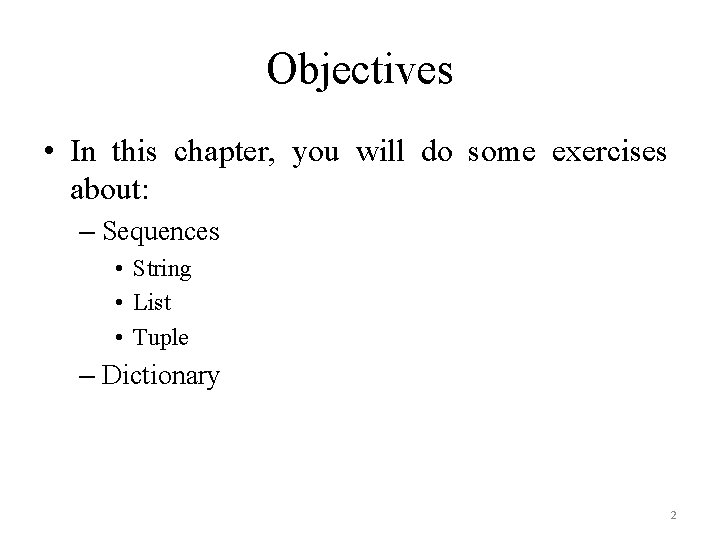
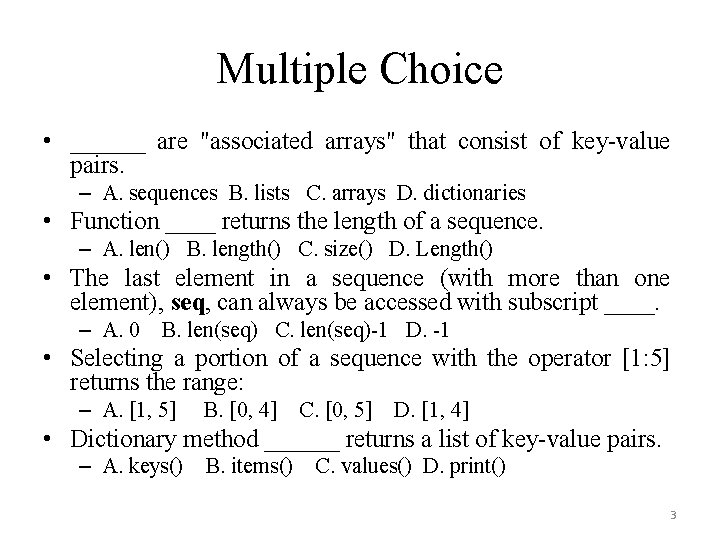
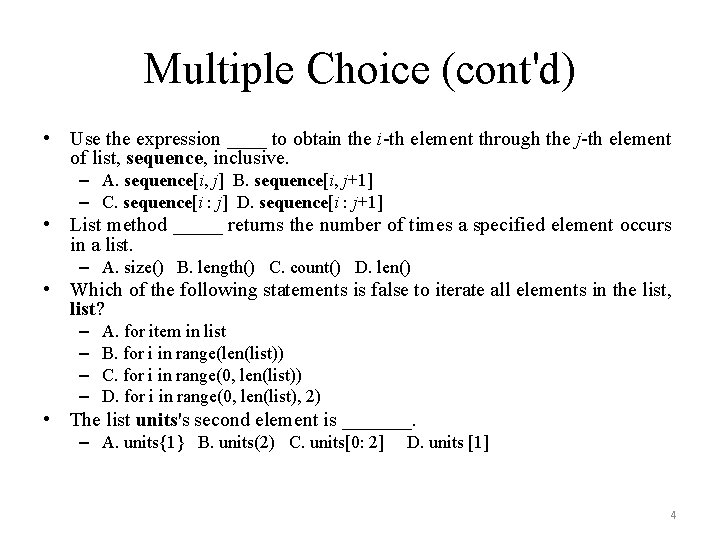
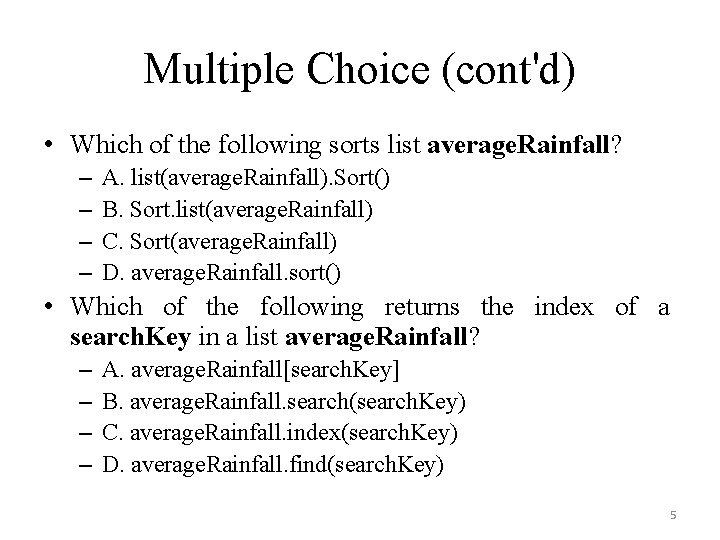
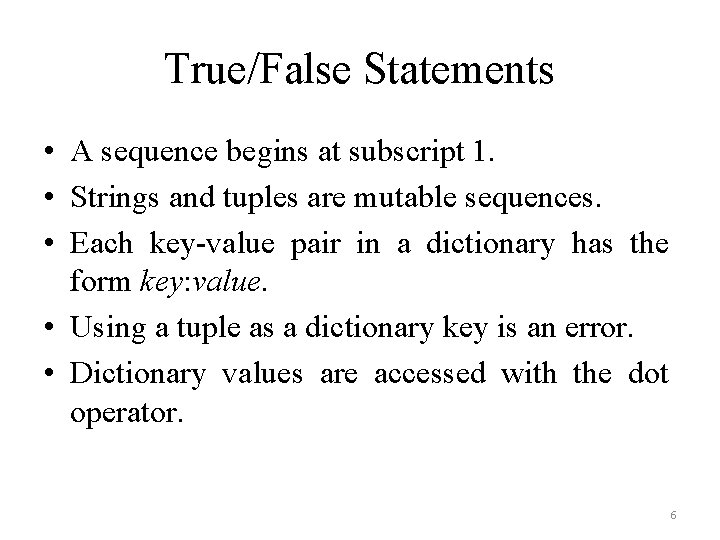
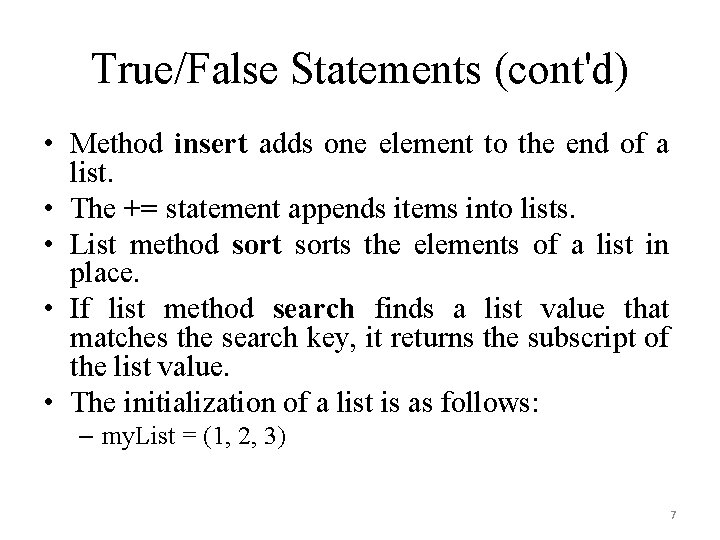
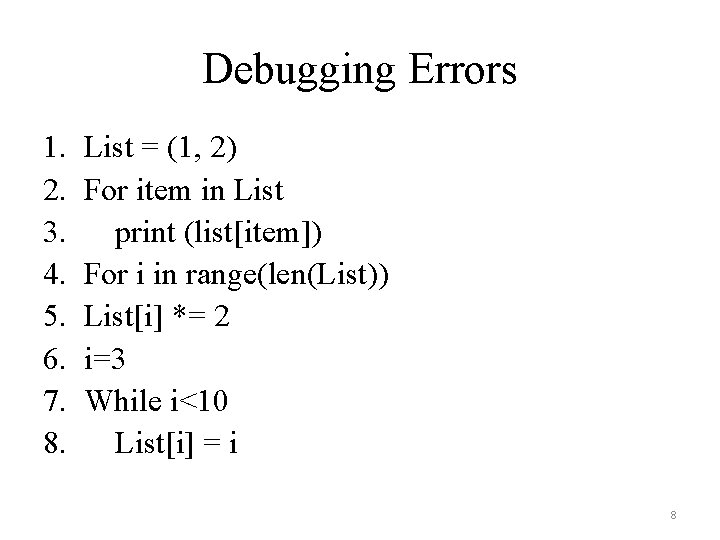
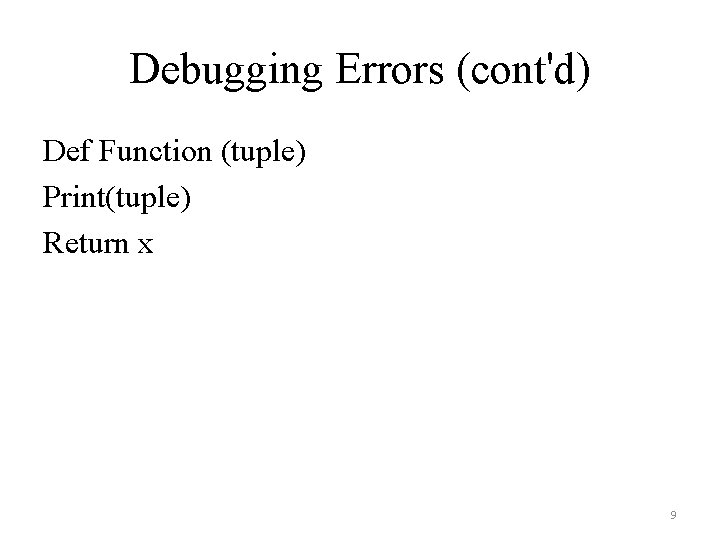
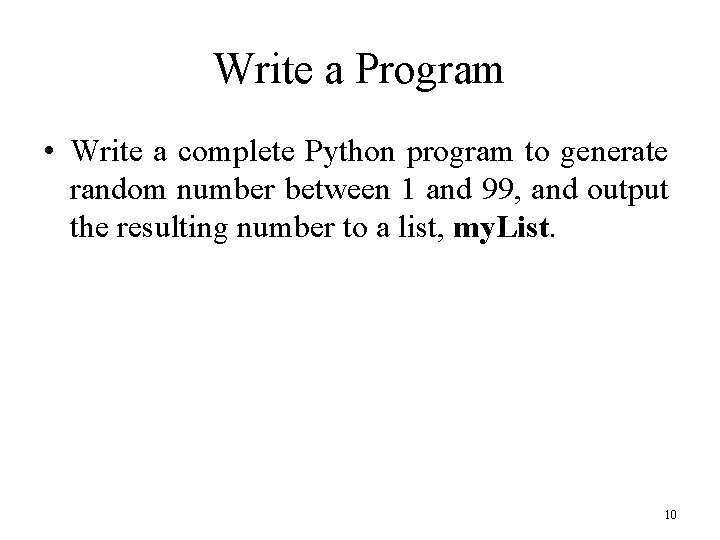
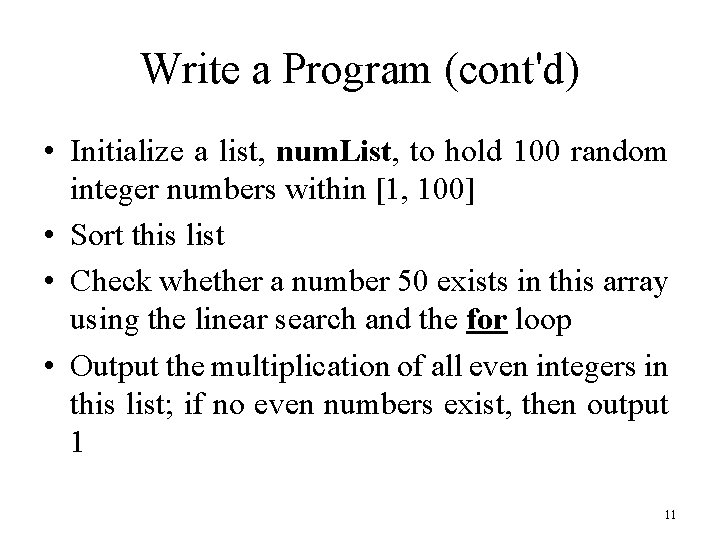
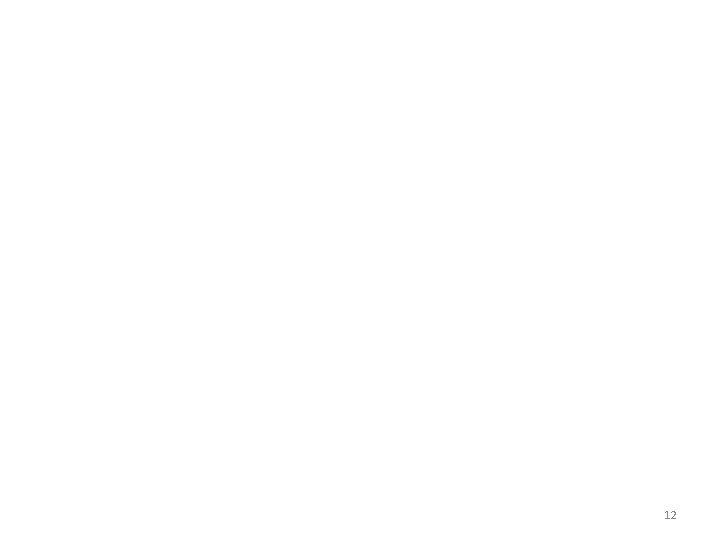
- Slides: 12
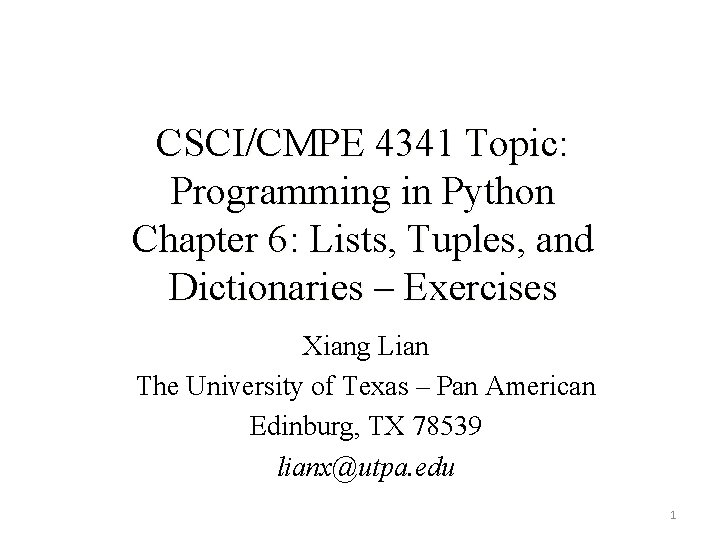
CSCI/CMPE 4341 Topic: Programming in Python Chapter 6: Lists, Tuples, and Dictionaries – Exercises Xiang Lian The University of Texas – Pan American Edinburg, TX 78539 lianx@utpa. edu 1
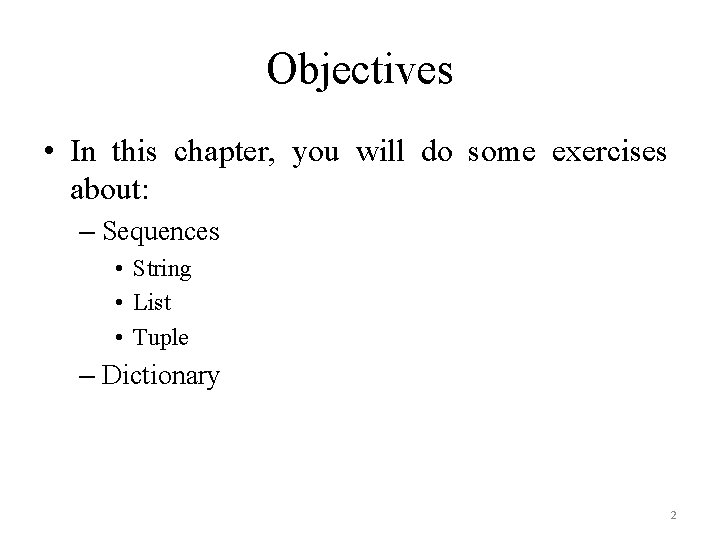
Objectives • In this chapter, you will do some exercises about: – Sequences • String • List • Tuple – Dictionary 2
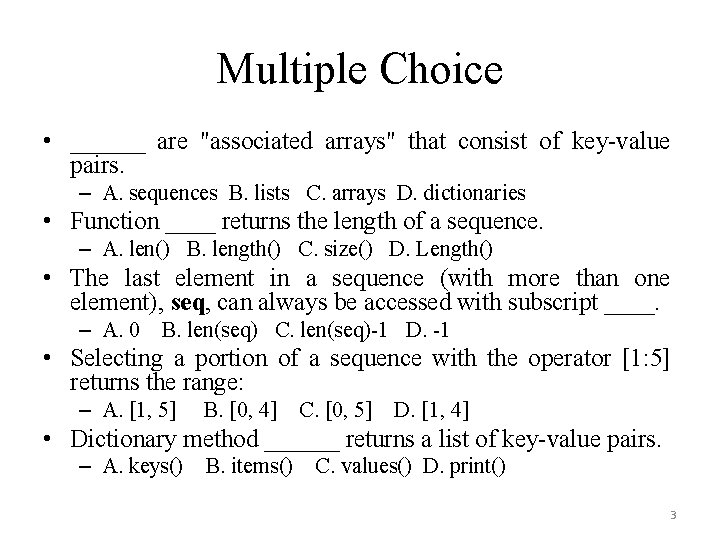
Multiple Choice • ______ are "associated arrays" that consist of key-value pairs. – A. sequences B. lists C. arrays D. dictionaries • Function ____ returns the length of a sequence. – A. len() B. length() C. size() D. Length() • The last element in a sequence (with more than one element), seq, can always be accessed with subscript ____. – A. 0 B. len(seq) C. len(seq)-1 D. -1 • Selecting a portion of a sequence with the operator [1: 5] returns the range: – A. [1, 5] B. [0, 4] C. [0, 5] D. [1, 4] • Dictionary method ______ returns a list of key-value pairs. – A. keys() B. items() C. values() D. print() 3
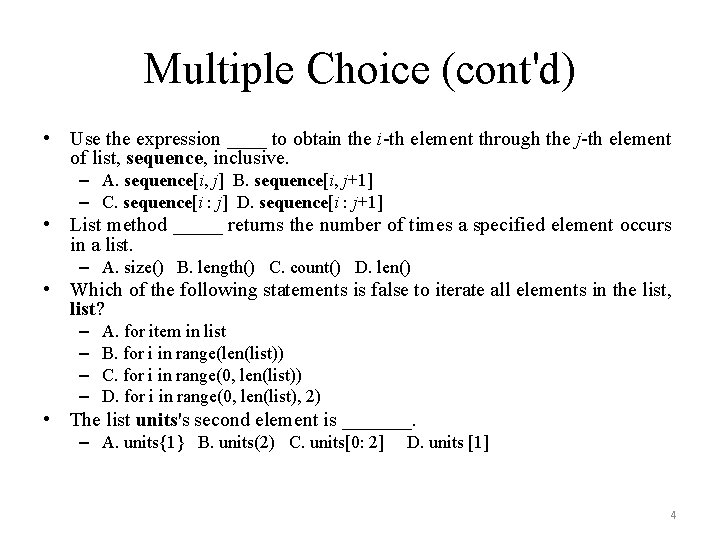
Multiple Choice (cont'd) • Use the expression ____ to obtain the i-th element through the j-th element of list, sequence, inclusive. – A. sequence[i, j] B. sequence[i, j+1] – C. sequence[i : j] D. sequence[i : j+1] • List method _____ returns the number of times a specified element occurs in a list. – A. size() B. length() C. count() D. len() • Which of the following statements is false to iterate all elements in the list, list? – – A. for item in list B. for i in range(len(list)) C. for i in range(0, len(list)) D. for i in range(0, len(list), 2) • The list units's second element is _______. – A. units{1} B. units(2) C. units[0: 2] D. units [1] 4
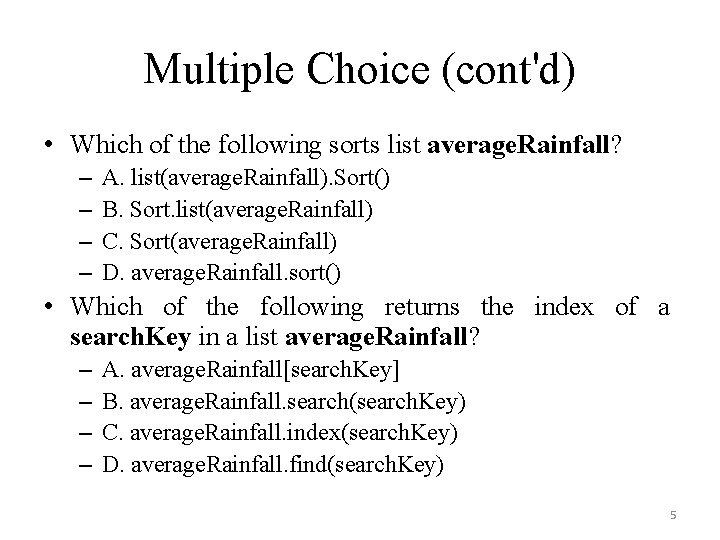
Multiple Choice (cont'd) • Which of the following sorts list average. Rainfall? – – A. list(average. Rainfall). Sort() B. Sort. list(average. Rainfall) C. Sort(average. Rainfall) D. average. Rainfall. sort() • Which of the following returns the index of a search. Key in a list average. Rainfall? – – A. average. Rainfall[search. Key] B. average. Rainfall. search(search. Key) C. average. Rainfall. index(search. Key) D. average. Rainfall. find(search. Key) 5
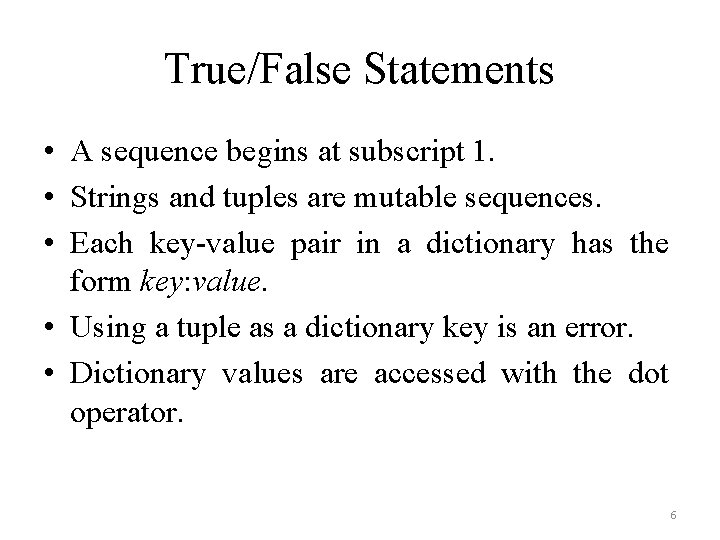
True/False Statements • A sequence begins at subscript 1. • Strings and tuples are mutable sequences. • Each key-value pair in a dictionary has the form key: value. • Using a tuple as a dictionary key is an error. • Dictionary values are accessed with the dot operator. 6
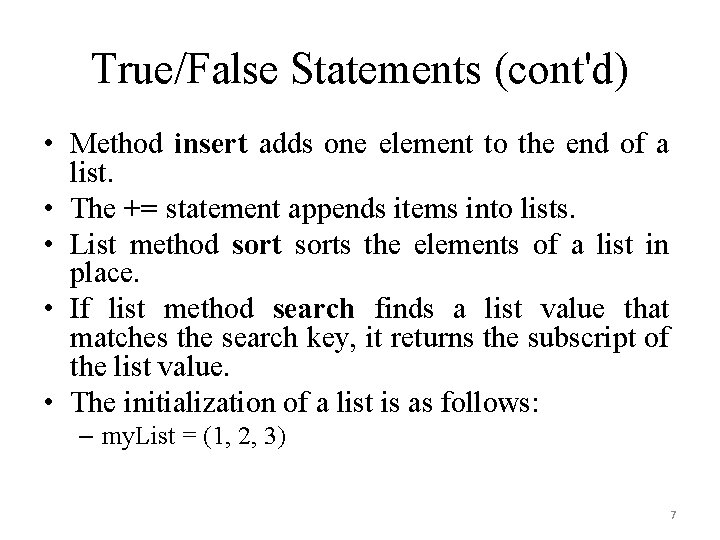
True/False Statements (cont'd) • Method insert adds one element to the end of a list. • The += statement appends items into lists. • List method sorts the elements of a list in place. • If list method search finds a list value that matches the search key, it returns the subscript of the list value. • The initialization of a list is as follows: – my. List = (1, 2, 3) 7
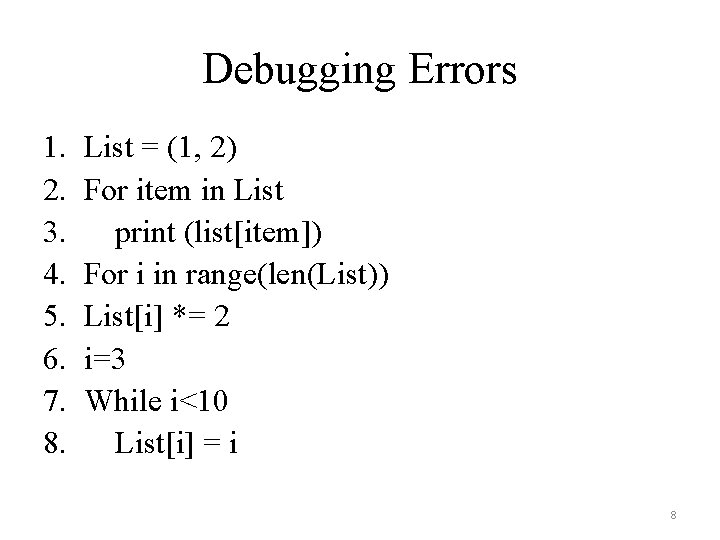
Debugging Errors 1. 2. 3. 4. 5. 6. 7. 8. List = (1, 2) For item in List print (list[item]) For i in range(len(List)) List[i] *= 2 i=3 While i<10 List[i] = i 8
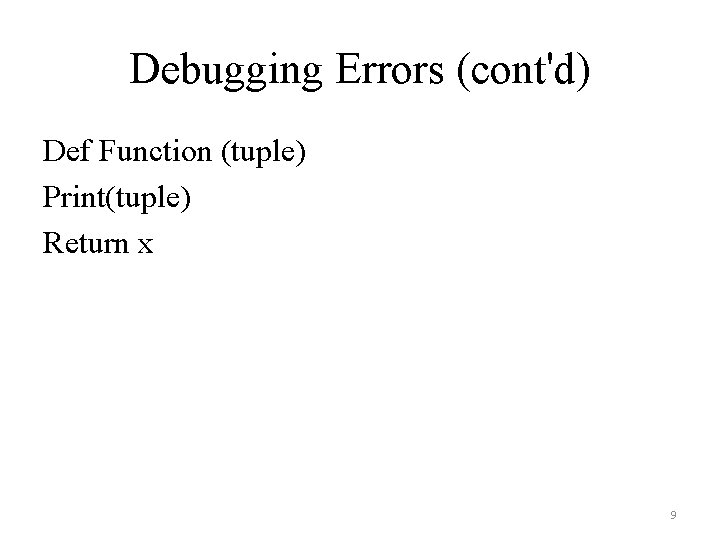
Debugging Errors (cont'd) Def Function (tuple) Print(tuple) Return x 9
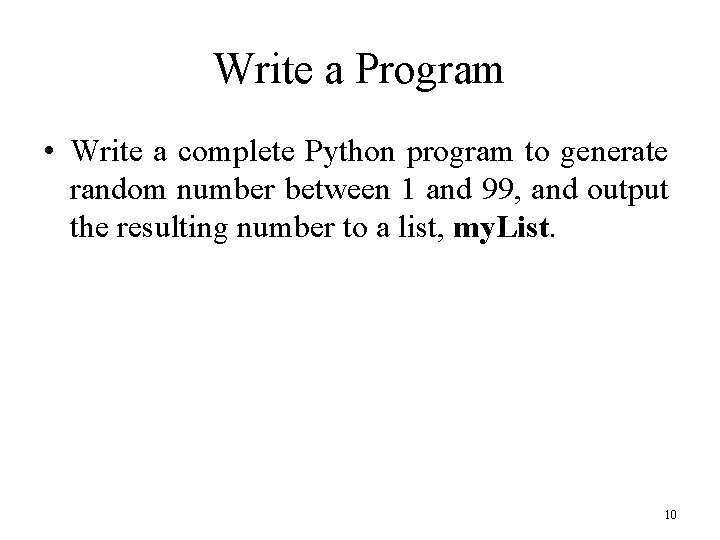
Write a Program • Write a complete Python program to generate random number between 1 and 99, and output the resulting number to a list, my. List. 10
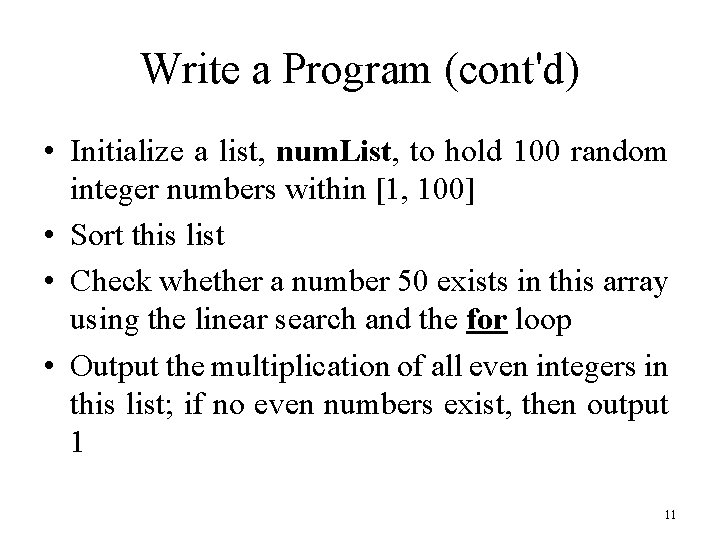
Write a Program (cont'd) • Initialize a list, num. List, to hold 100 random integer numbers within [1, 100] • Sort this list • Check whether a number 50 exists in this array using the linear search and the for loop • Output the multiplication of all even integers in this list; if no even numbers exist, then output 1 11
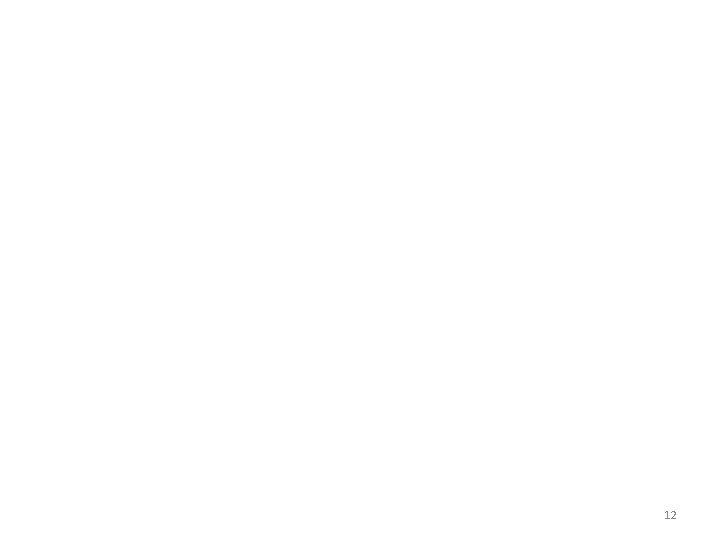
12