CSC 270 Survey of Programming Languages C Lecture
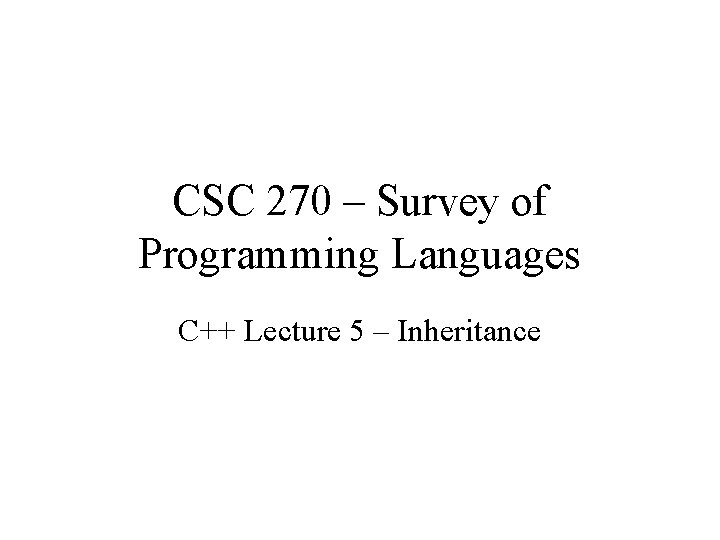
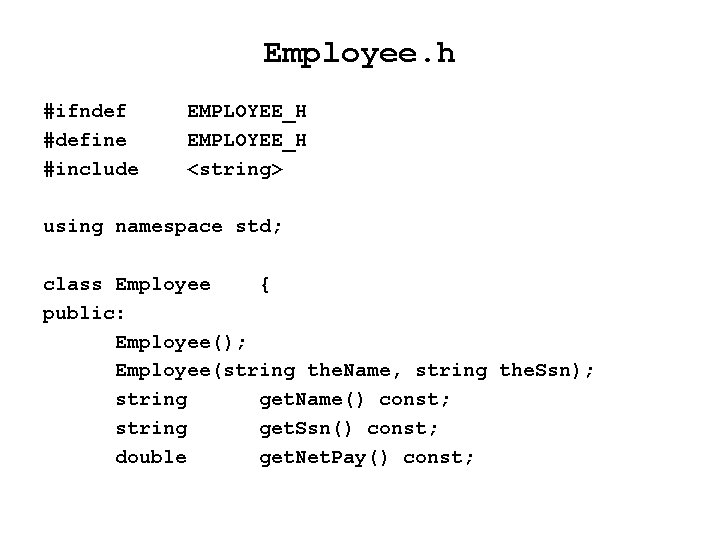
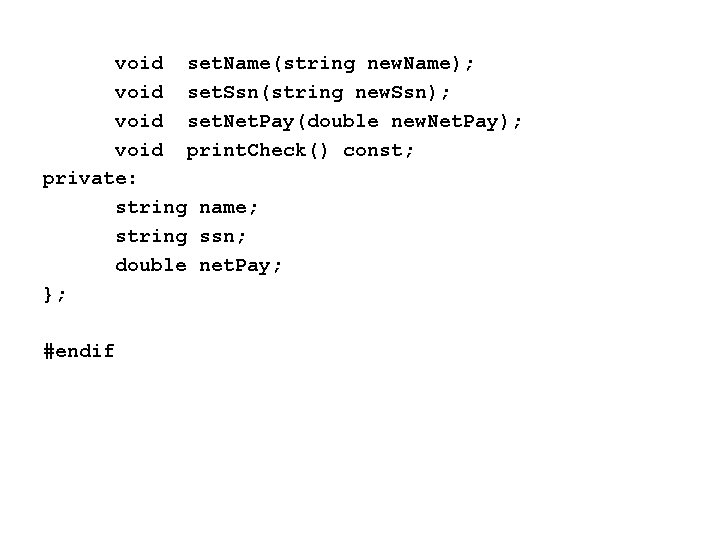
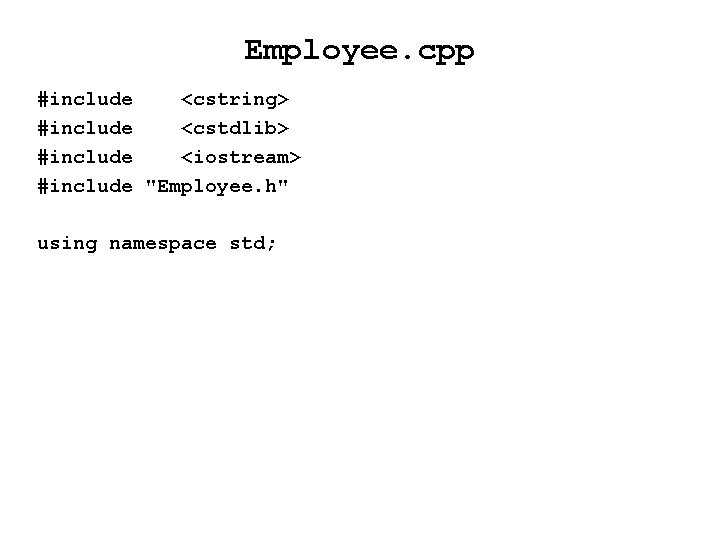
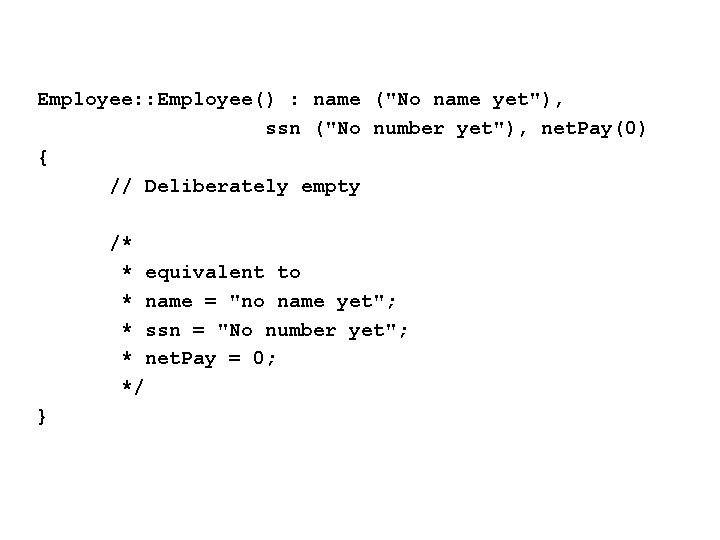
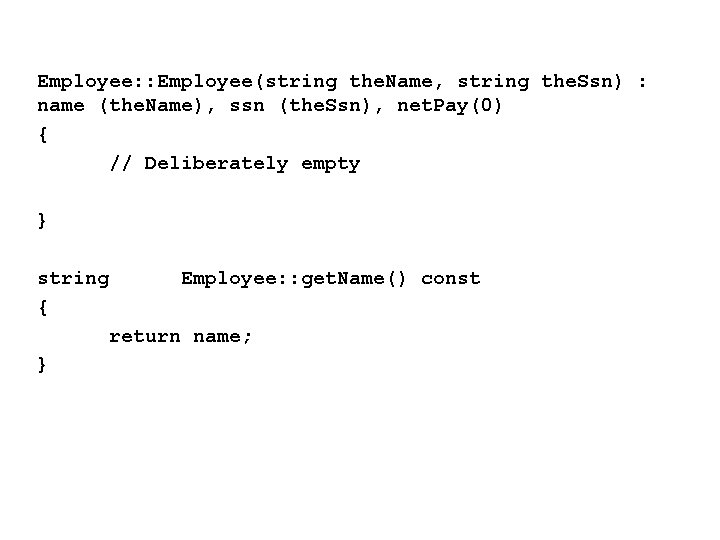
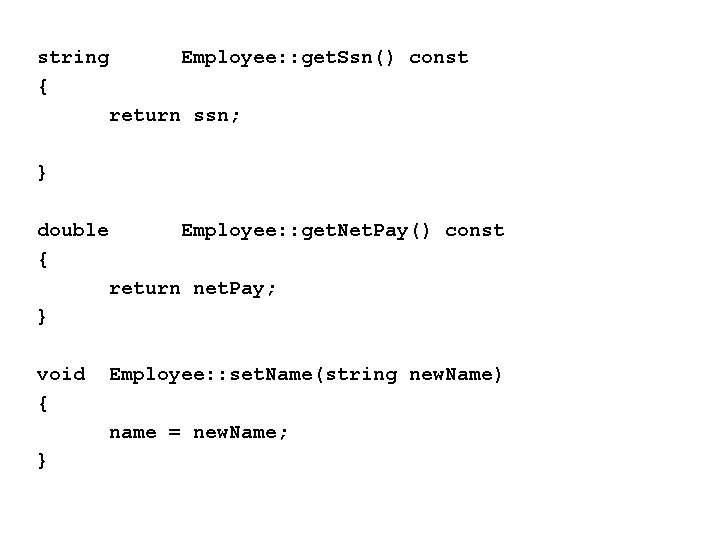
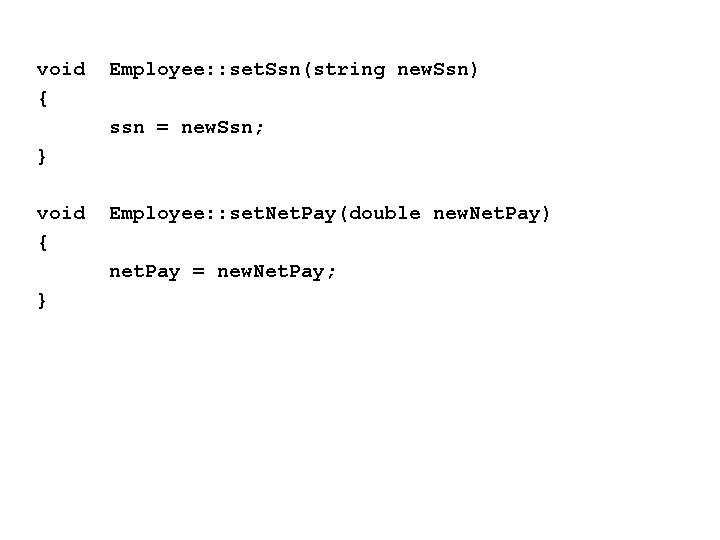
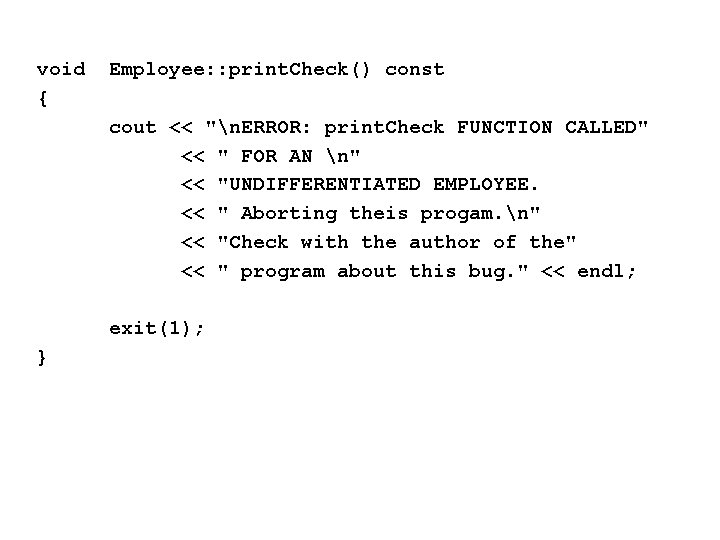
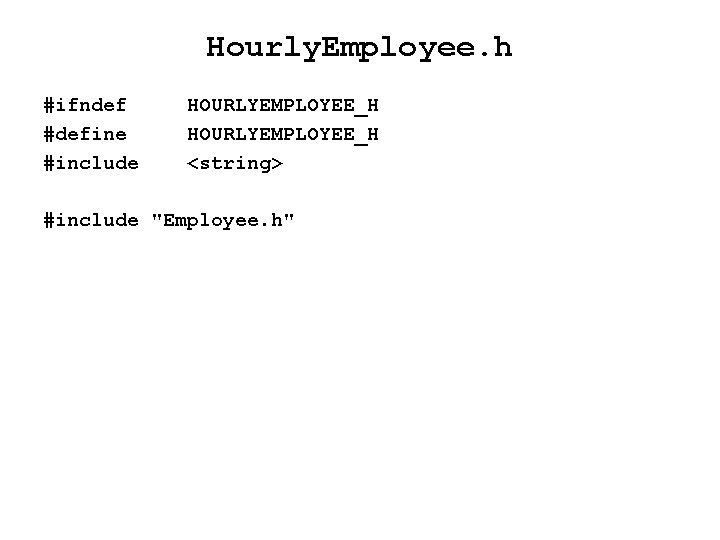
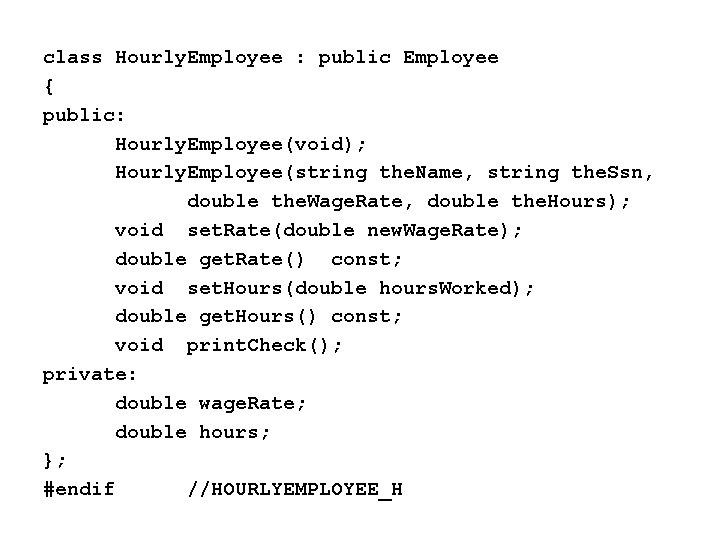
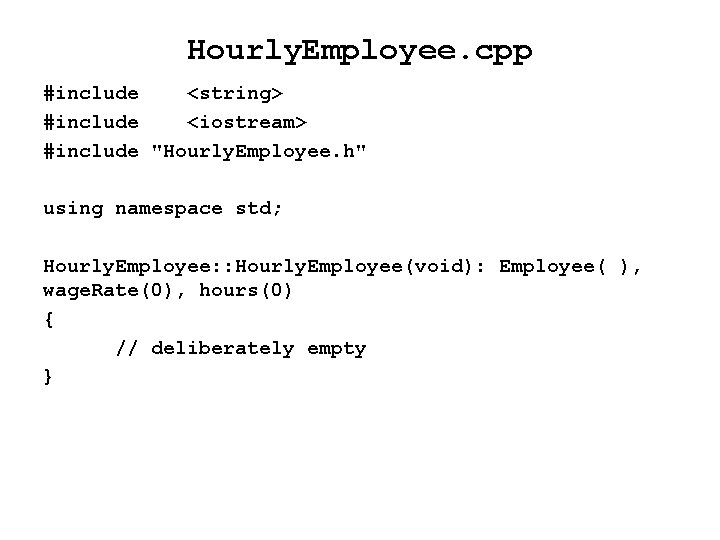
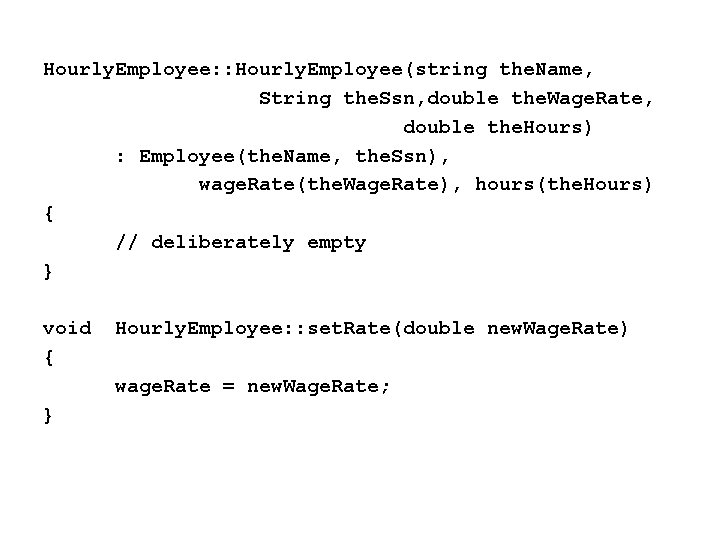
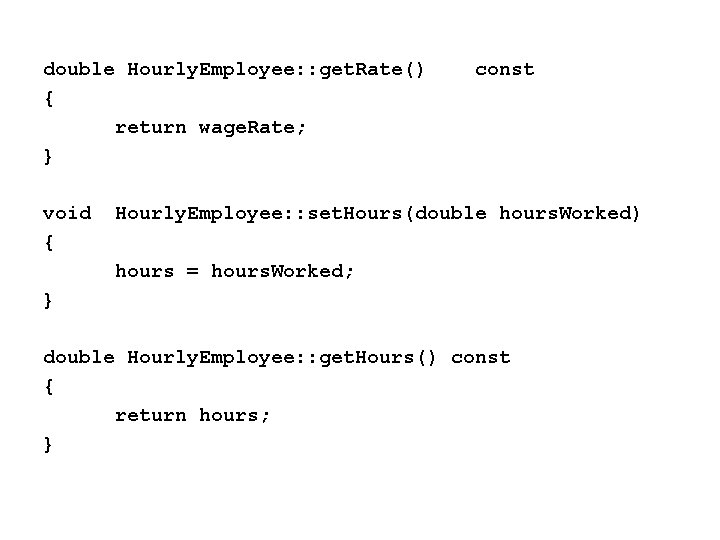
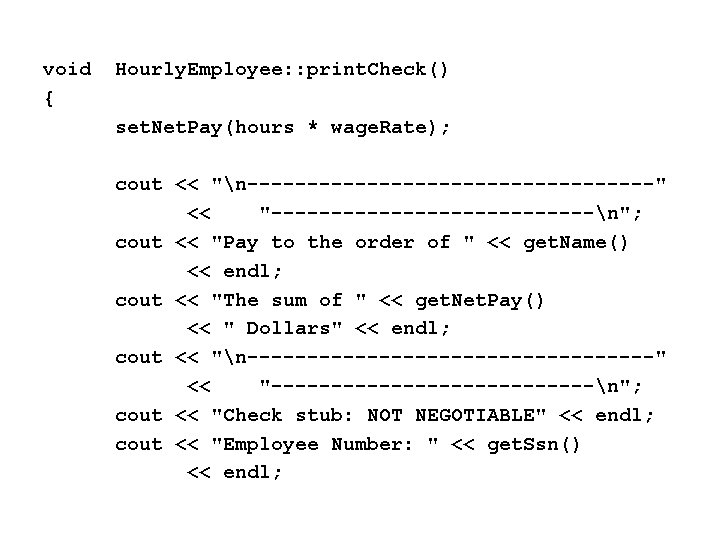
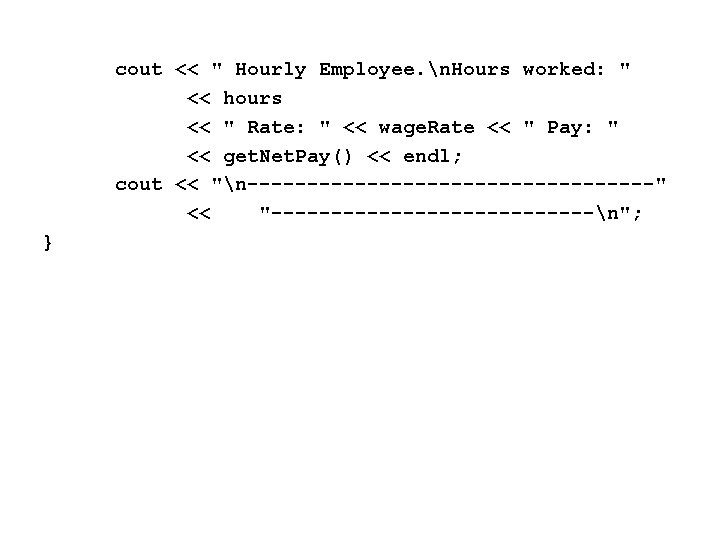
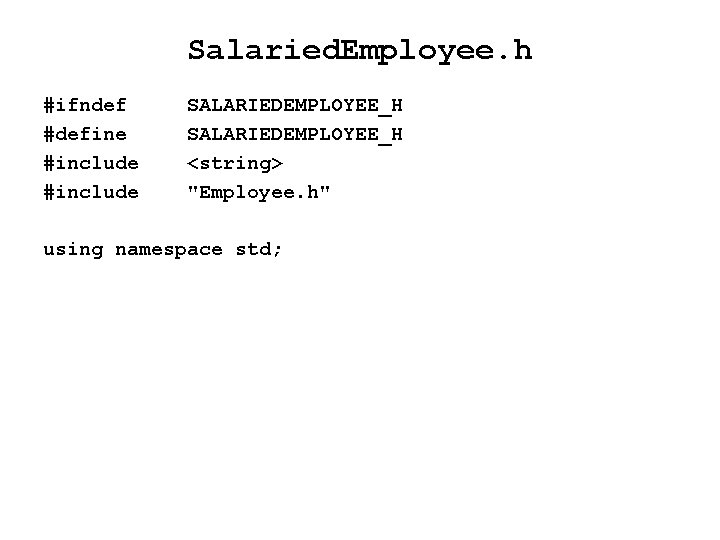
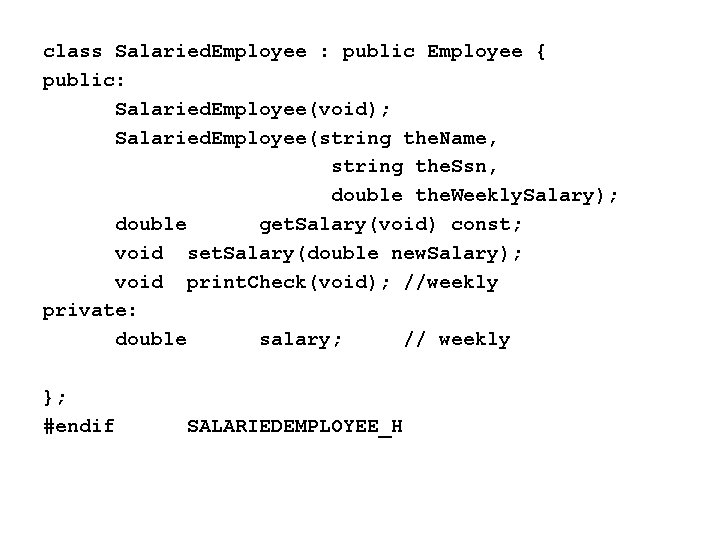
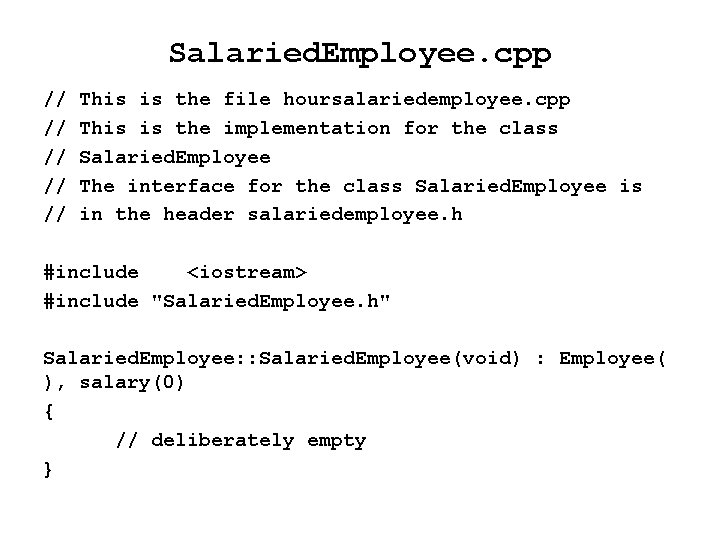
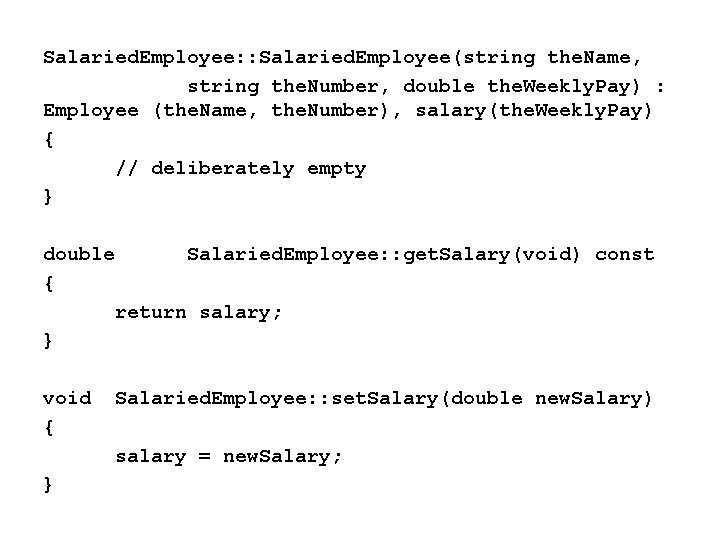
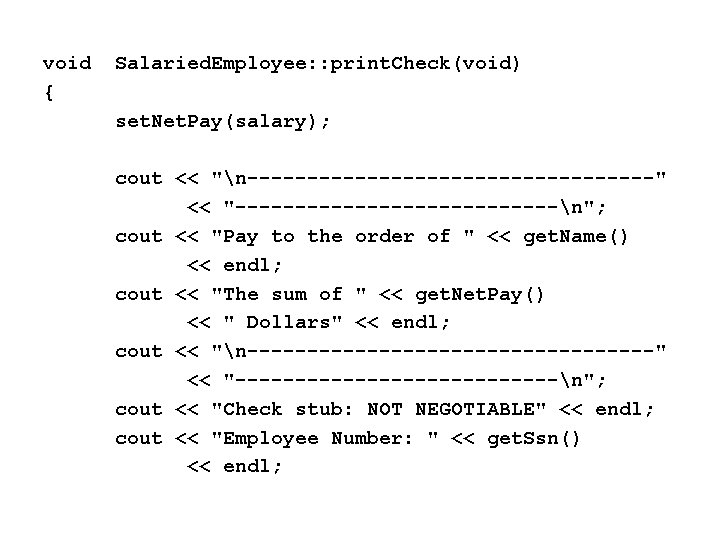
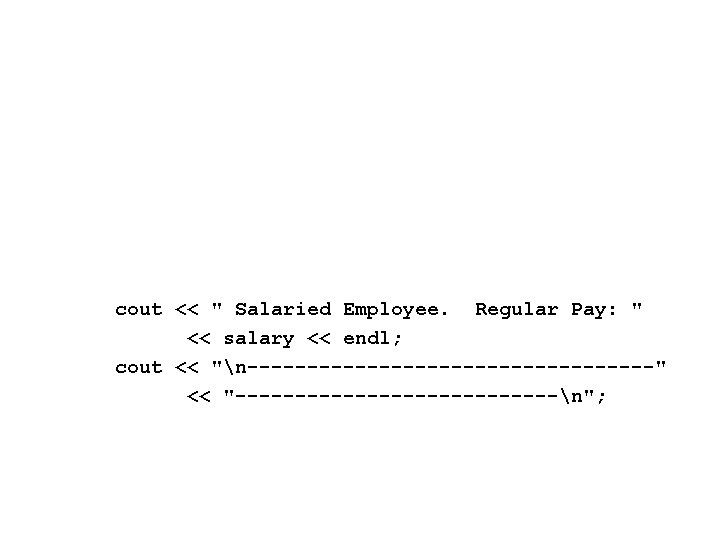
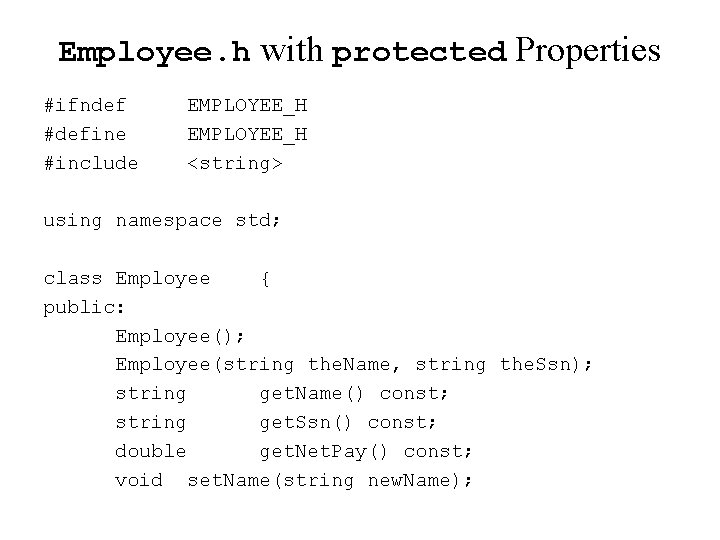
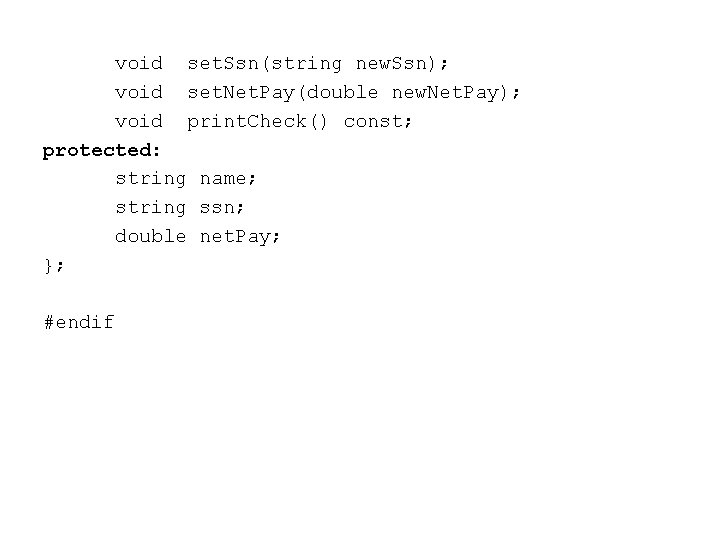
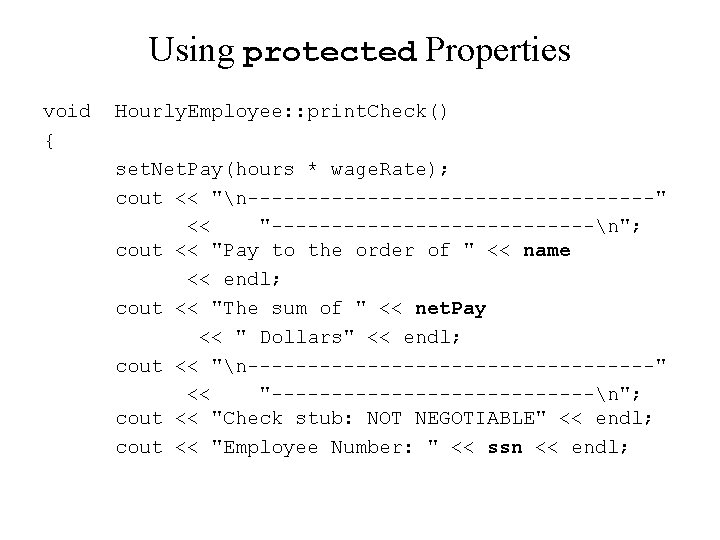
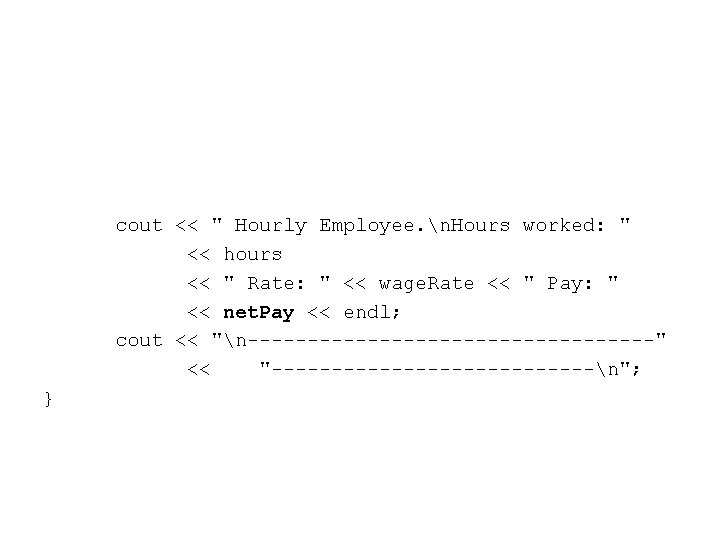
- Slides: 26
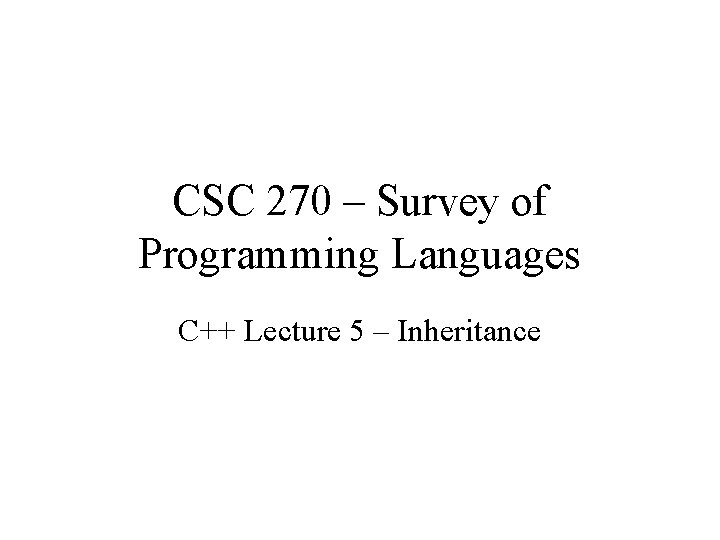
CSC 270 – Survey of Programming Languages C++ Lecture 5 – Inheritance
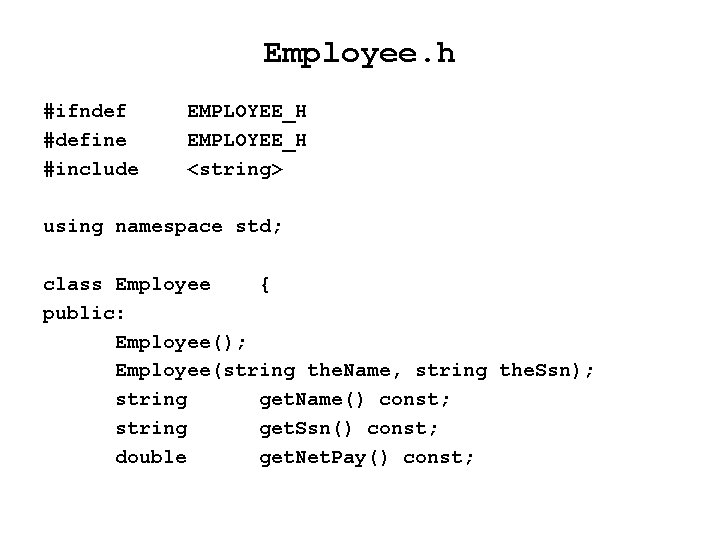
Employee. h #ifndef #define #include EMPLOYEE_H <string> using namespace std; class Employee { public: Employee(); Employee(string the. Name, string the. Ssn); string get. Name() const; string get. Ssn() const; double get. Net. Pay() const;
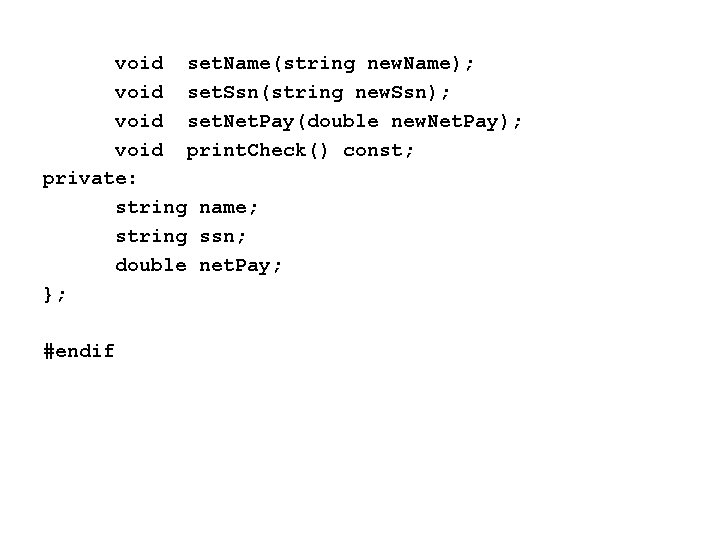
void set. Name(string new. Name); void set. Ssn(string new. Ssn); void set. Net. Pay(double new. Net. Pay); void print. Check() const; private: string name; string ssn; double net. Pay; }; #endif
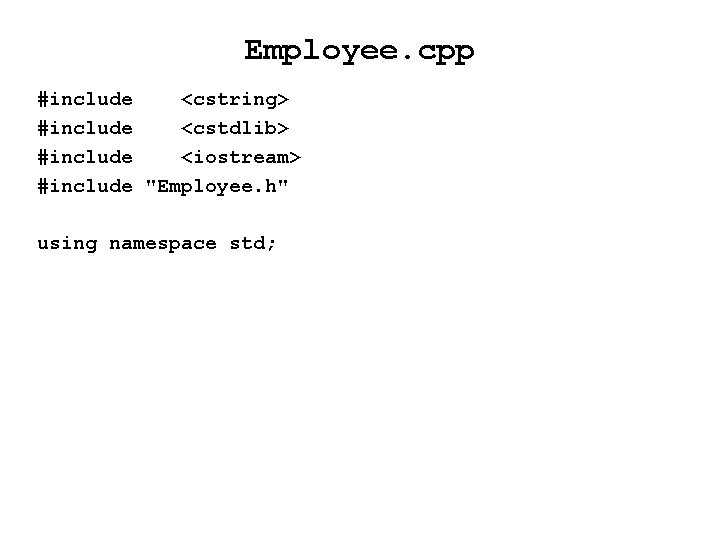
Employee. cpp #include <cstring> #include <cstdlib> #include <iostream> #include "Employee. h" using namespace std;
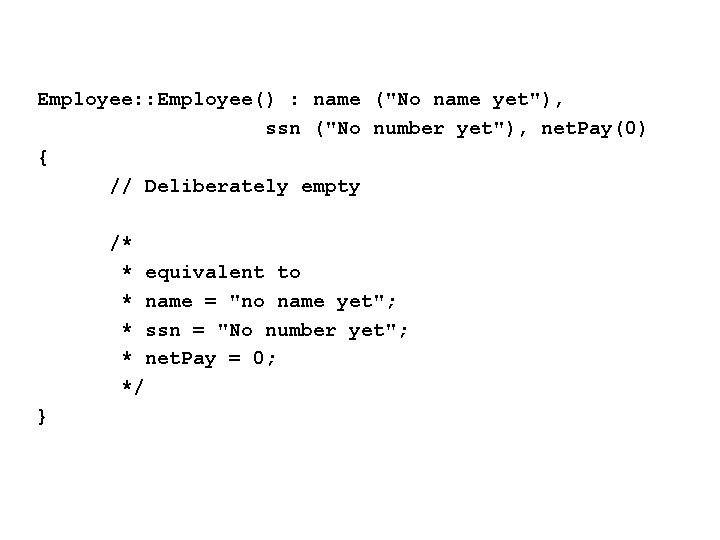
Employee: : Employee() : name ("No name yet"), ssn ("No number yet"), net. Pay(0) { // Deliberately empty /* * equivalent to * name = "no name yet"; * ssn = "No number yet"; * net. Pay = 0; */ }
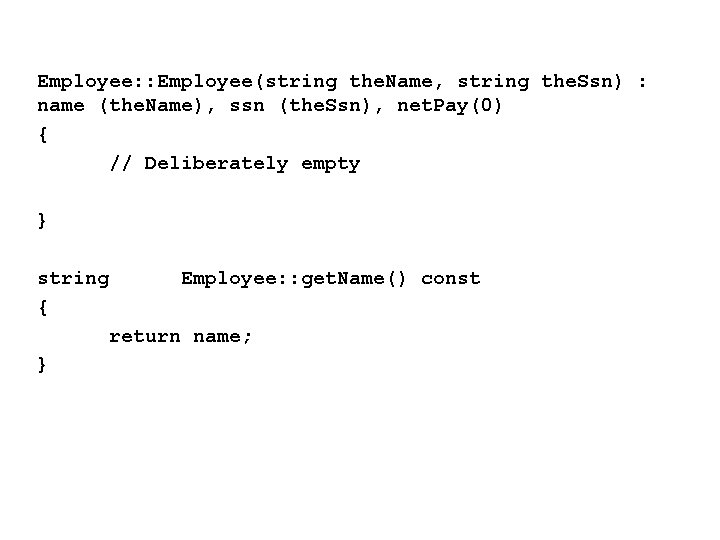
Employee: : Employee(string the. Name, string the. Ssn) : name (the. Name), ssn (the. Ssn), net. Pay(0) { // Deliberately empty } string { Employee: : get. Name() const return name; }
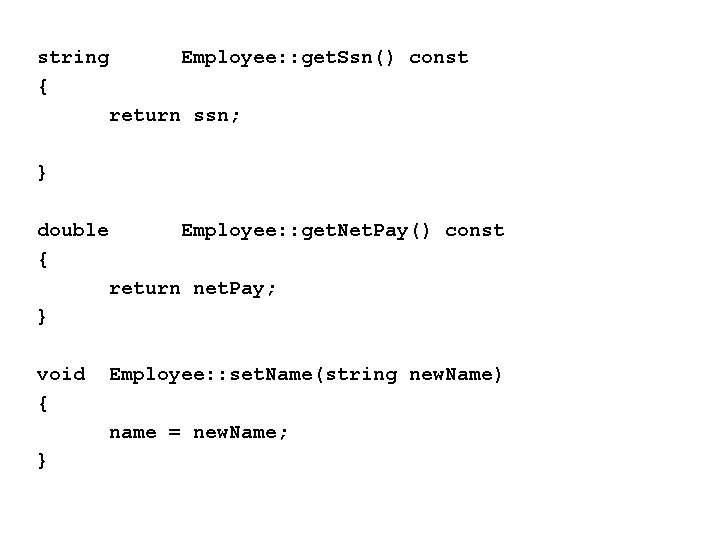
string { Employee: : get. Ssn() const return ssn; } double { Employee: : get. Net. Pay() const return net. Pay; } void { Employee: : set. Name(string new. Name) name = new. Name; }
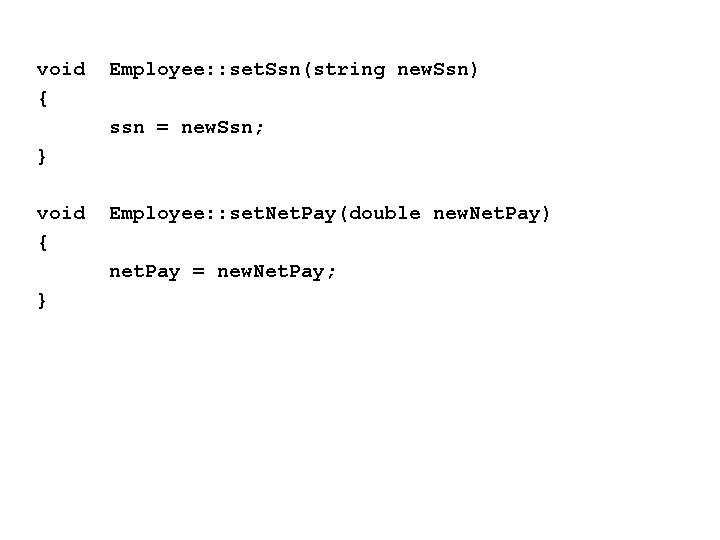
void { Employee: : set. Ssn(string new. Ssn) ssn = new. Ssn; } void { Employee: : set. Net. Pay(double new. Net. Pay) net. Pay = new. Net. Pay; }
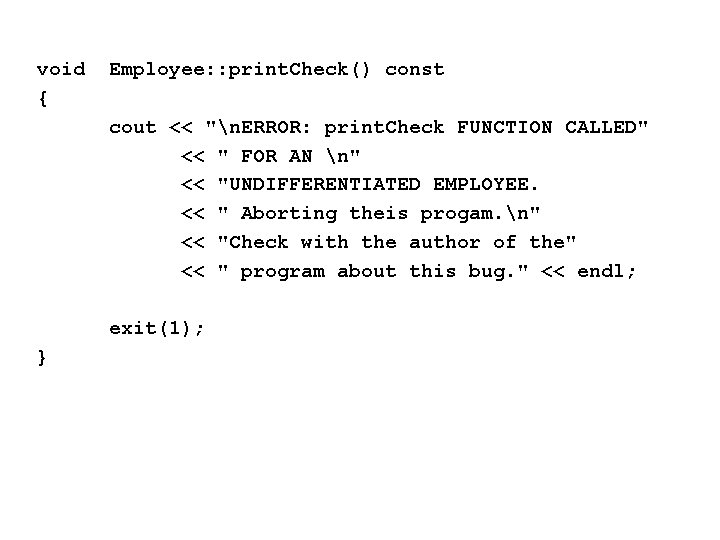
void { Employee: : print. Check() const cout << "n. ERROR: print. Check FUNCTION CALLED" << " FOR AN n" << "UNDIFFERENTIATED EMPLOYEE. << " Aborting theis progam. n" << "Check with the author of the" << " program about this bug. " << endl; exit(1); }
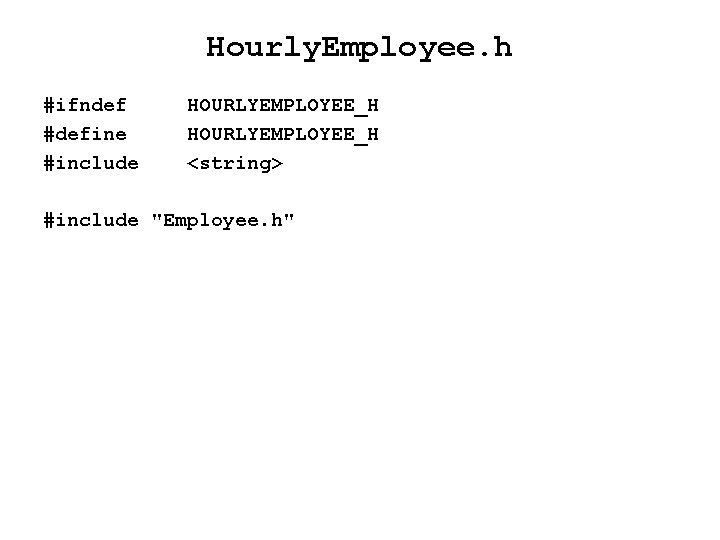
Hourly. Employee. h #ifndef #define #include HOURLYEMPLOYEE_H <string> #include "Employee. h"
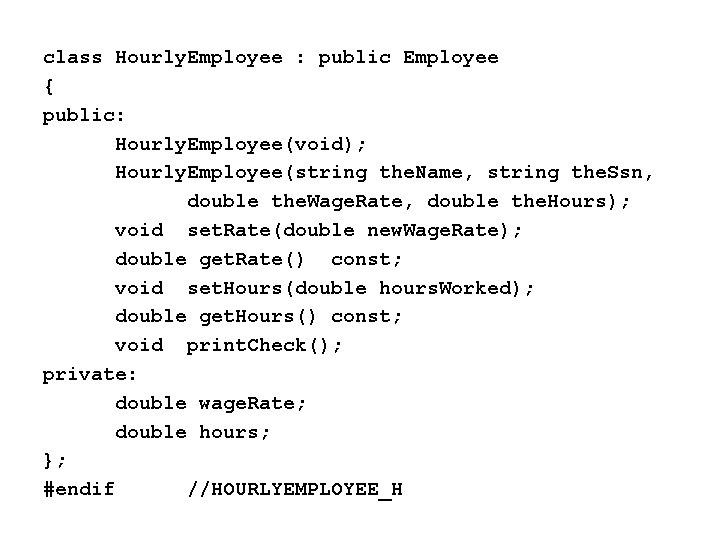
class Hourly. Employee : public Employee { public: Hourly. Employee(void); Hourly. Employee(string the. Name, string the. Ssn, double the. Wage. Rate, double the. Hours); void set. Rate(double new. Wage. Rate); double get. Rate() const; void set. Hours(double hours. Worked); double get. Hours() const; void print. Check(); private: double wage. Rate; double hours; }; #endif //HOURLYEMPLOYEE_H
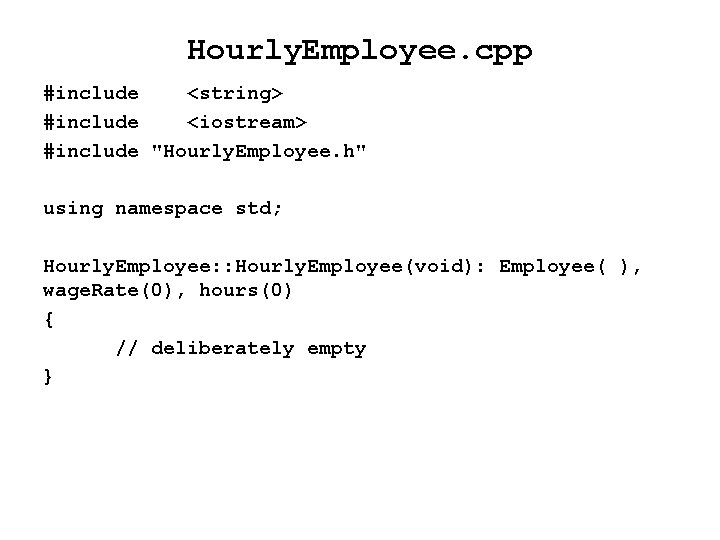
Hourly. Employee. cpp #include <string> #include <iostream> #include "Hourly. Employee. h" using namespace std; Hourly. Employee: : Hourly. Employee(void): Employee( ), wage. Rate(0), hours(0) { // deliberately empty }
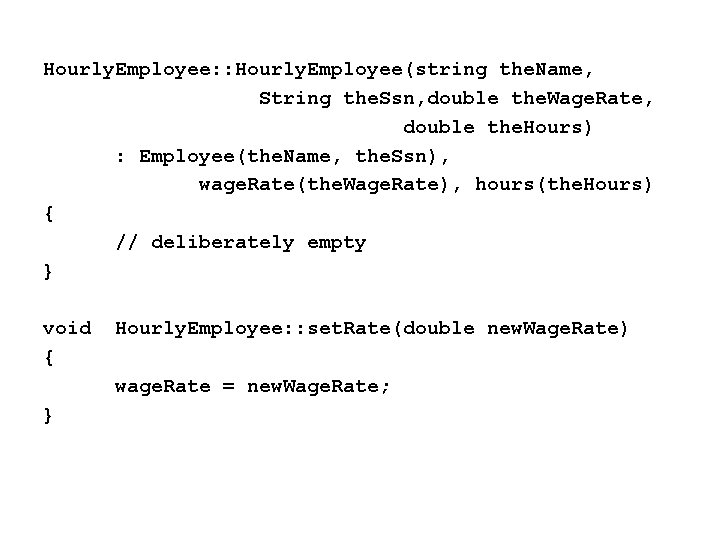
Hourly. Employee: : Hourly. Employee(string the. Name, String the. Ssn, double the. Wage. Rate, double the. Hours) : Employee(the. Name, the. Ssn), wage. Rate(the. Wage. Rate), hours(the. Hours) { // deliberately empty } void { Hourly. Employee: : set. Rate(double new. Wage. Rate) wage. Rate = new. Wage. Rate; }
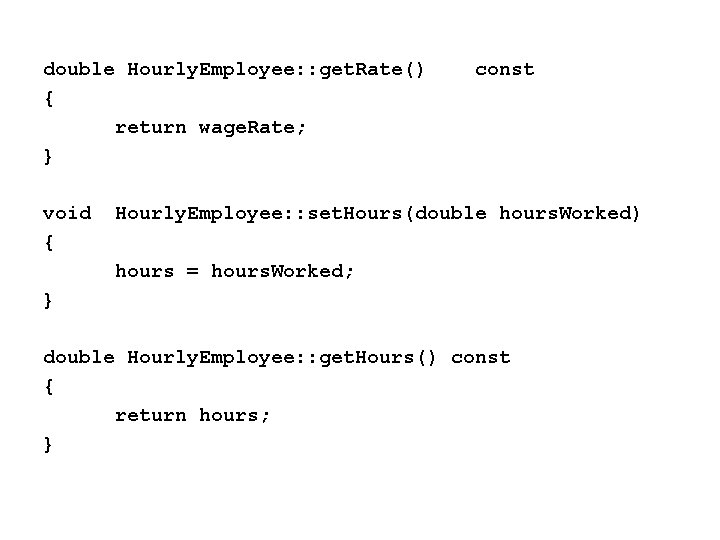
double Hourly. Employee: : get. Rate() { return wage. Rate; } void { const Hourly. Employee: : set. Hours(double hours. Worked) hours = hours. Worked; } double Hourly. Employee: : get. Hours() const { return hours; }
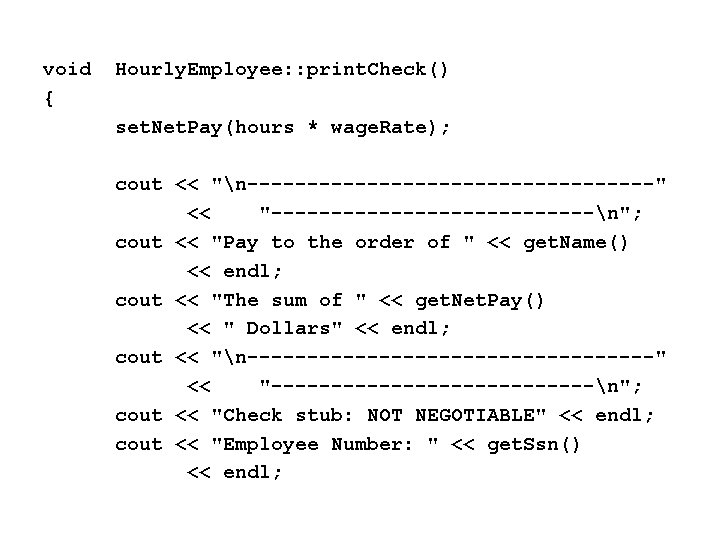
void { Hourly. Employee: : print. Check() set. Net. Pay(hours * wage. Rate); cout << "n-----------------" << "--------------n"; cout << "Pay to the order of " << get. Name() << endl; cout << "The sum of " << get. Net. Pay() << " Dollars" << endl; cout << "n-----------------" << "--------------n"; cout << "Check stub: NOT NEGOTIABLE" << endl; cout << "Employee Number: " << get. Ssn() << endl;
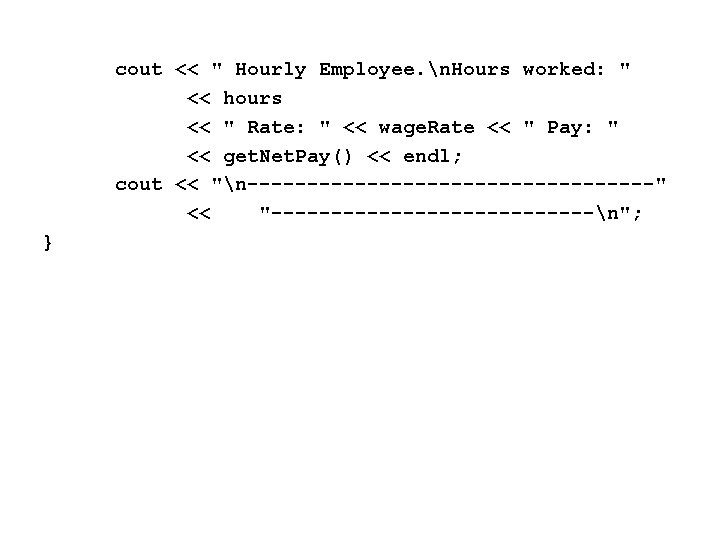
cout << " Hourly Employee. n. Hours worked: " << hours << " Rate: " << wage. Rate << " Pay: " << get. Net. Pay() << endl; cout << "n-----------------" << "--------------n"; }
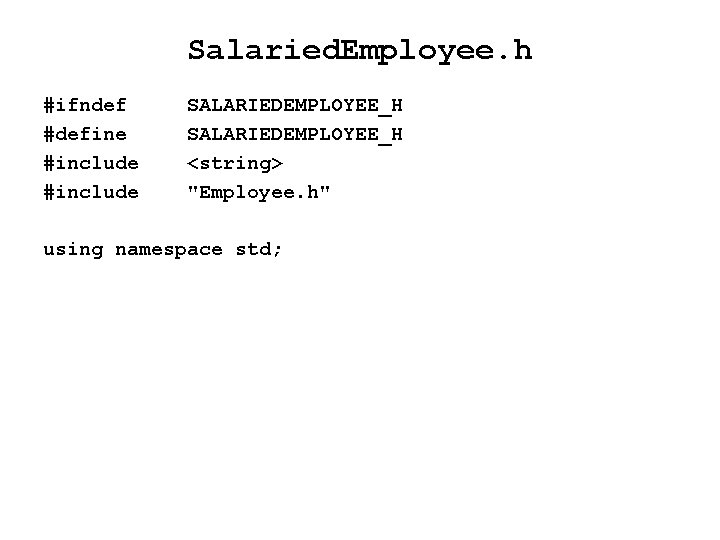
Salaried. Employee. h #ifndef #define #include SALARIEDEMPLOYEE_H <string> "Employee. h" using namespace std;
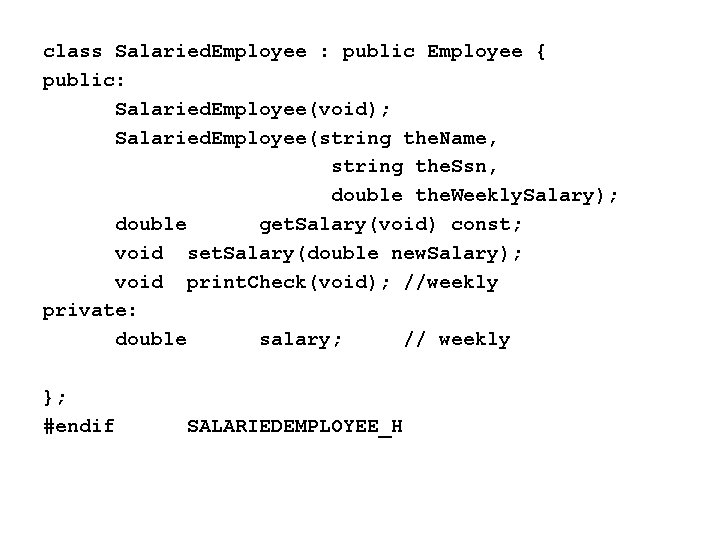
class Salaried. Employee : public Employee { public: Salaried. Employee(void); Salaried. Employee(string the. Name, string the. Ssn, double the. Weekly. Salary); double get. Salary(void) const; void set. Salary(double new. Salary); void print. Check(void); //weekly private: double salary; // weekly }; #endif SALARIEDEMPLOYEE_H
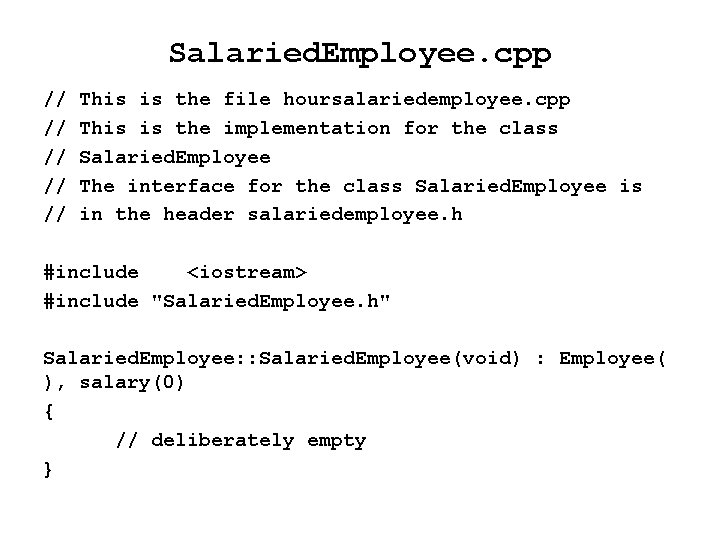
Salaried. Employee. cpp // // // This is the file hoursalariedemployee. cpp This is the implementation for the class Salaried. Employee The interface for the class Salaried. Employee is in the header salariedemployee. h #include <iostream> #include "Salaried. Employee. h" Salaried. Employee: : Salaried. Employee(void) : Employee( ), salary(0) { // deliberately empty }
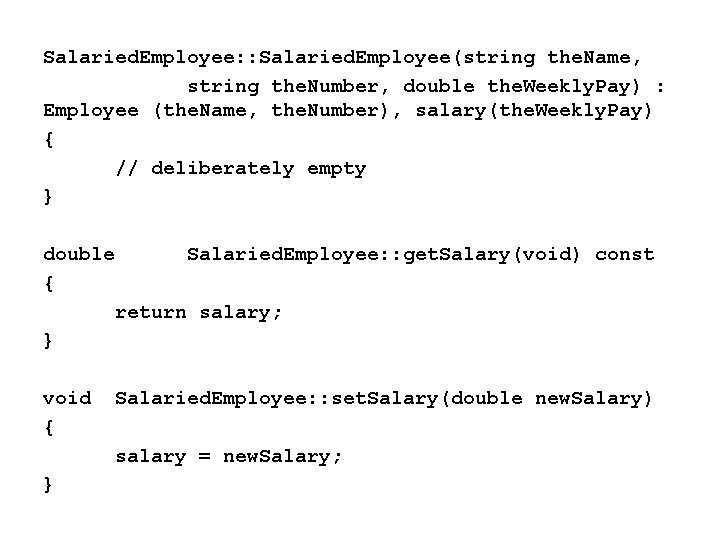
Salaried. Employee: : Salaried. Employee(string the. Name, string the. Number, double the. Weekly. Pay) : Employee (the. Name, the. Number), salary(the. Weekly. Pay) { // deliberately empty } double { Salaried. Employee: : get. Salary(void) const return salary; } void { Salaried. Employee: : set. Salary(double new. Salary) salary = new. Salary; }
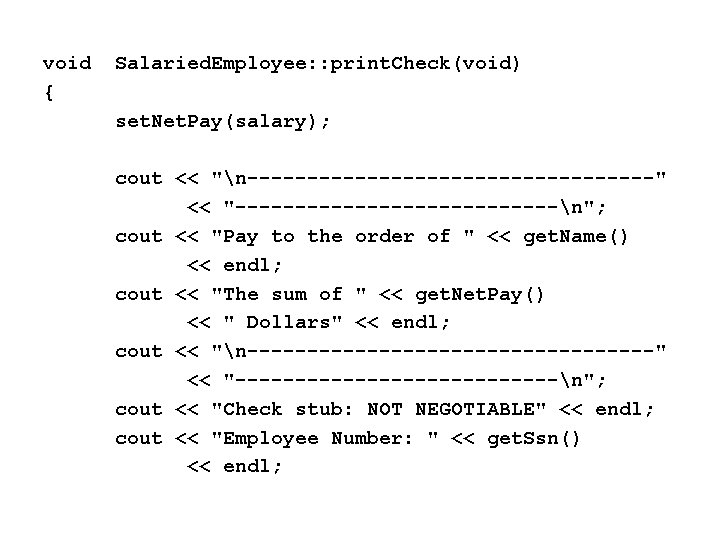
void { Salaried. Employee: : print. Check(void) set. Net. Pay(salary); cout << "n-----------------" << "--------------n"; cout << "Pay to the order of " << get. Name() << endl; cout << "The sum of " << get. Net. Pay() << " Dollars" << endl; cout << "n-----------------" << "--------------n"; cout << "Check stub: NOT NEGOTIABLE" << endl; cout << "Employee Number: " << get. Ssn() << endl;
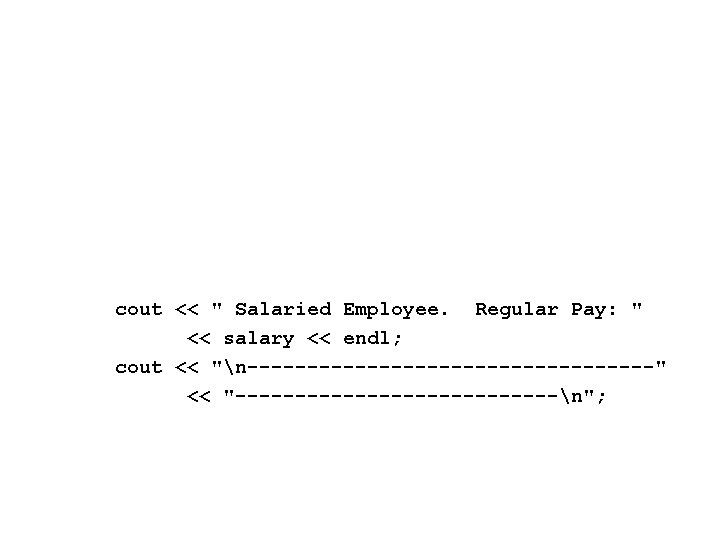
cout << " Salaried Employee. Regular Pay: " << salary << endl; cout << "n-----------------" << "--------------n";
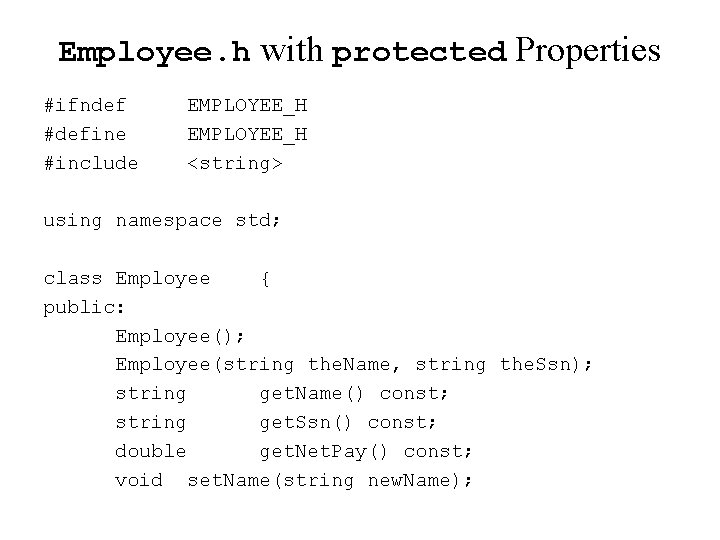
Employee. h with protected Properties #ifndef #define #include EMPLOYEE_H <string> using namespace std; class Employee { public: Employee(); Employee(string the. Name, string the. Ssn); string get. Name() const; string get. Ssn() const; double get. Net. Pay() const; void set. Name(string new. Name);
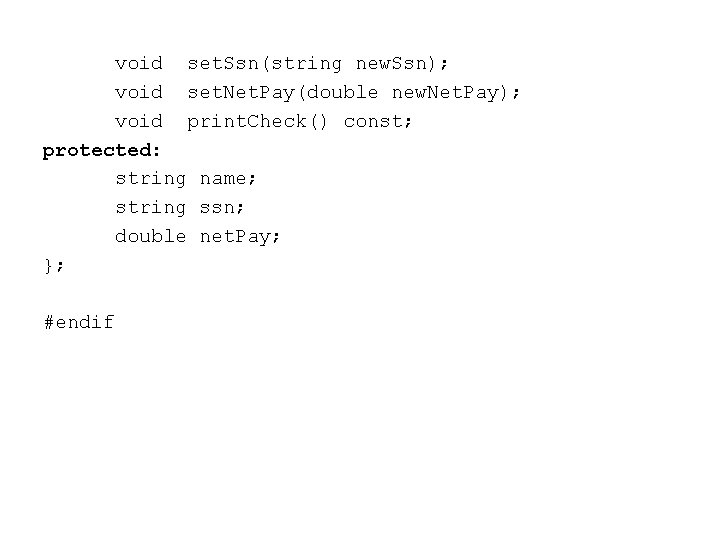
void set. Ssn(string new. Ssn); void set. Net. Pay(double new. Net. Pay); void print. Check() const; protected: string name; string ssn; double net. Pay; }; #endif
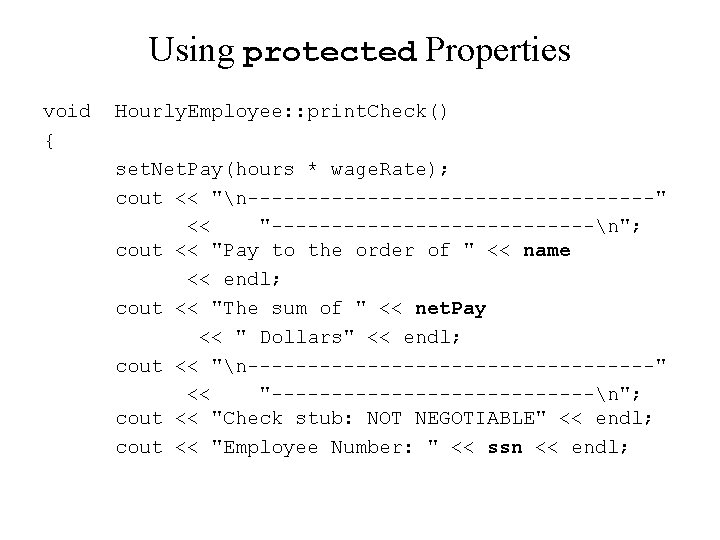
Using protected Properties void { Hourly. Employee: : print. Check() set. Net. Pay(hours * wage. Rate); cout << "n-----------------" << "--------------n"; cout << "Pay to the order of " << name << endl; cout << "The sum of " << net. Pay << " Dollars" << endl; cout << "n-----------------" << "--------------n"; cout << "Check stub: NOT NEGOTIABLE" << endl; cout << "Employee Number: " << ssn << endl;
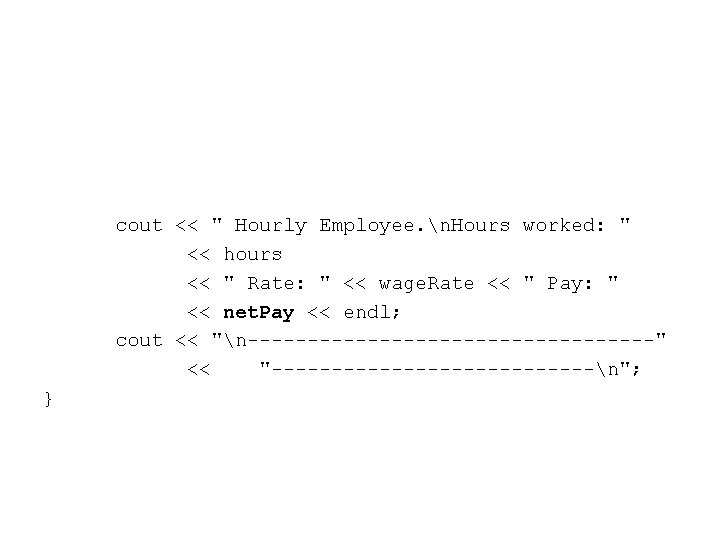
cout << " Hourly Employee. n. Hours worked: " << hours << " Rate: " << wage. Rate << " Pay: " << net. Pay << endl; cout << "n-----------------" << "--------------n"; }