CSC 270 Survey of Programming Languages C Lecture
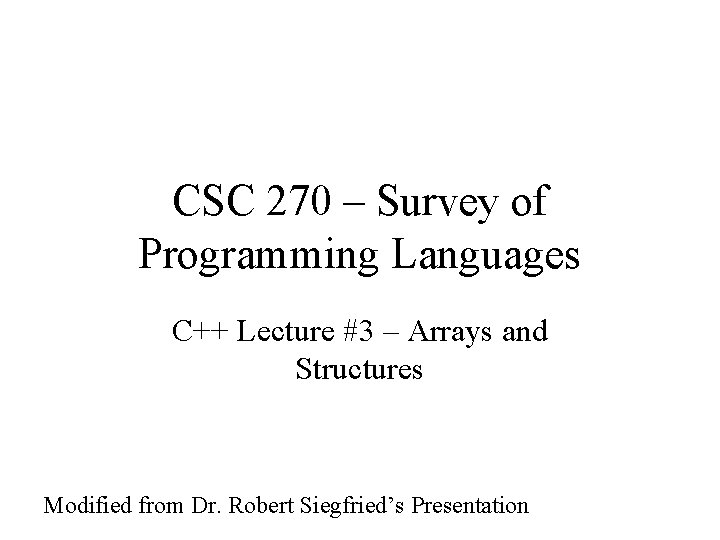
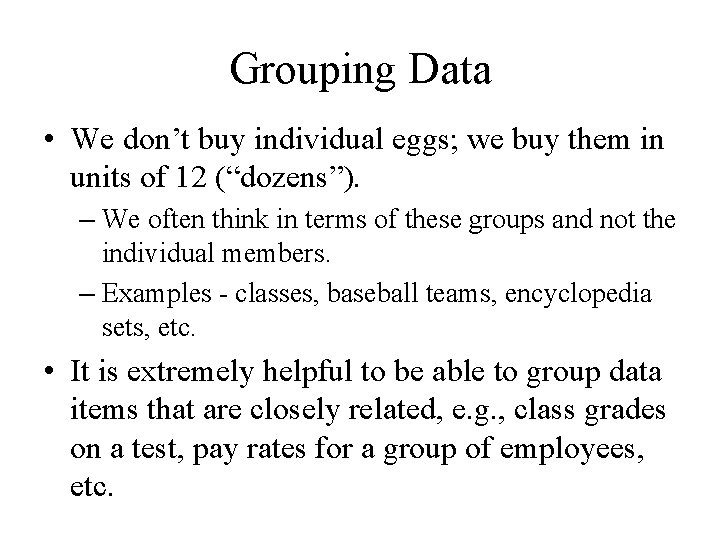
![Declaring Arrays • Instead of writing: int x; • we can write: int x[10]; Declaring Arrays • Instead of writing: int x; • we can write: int x[10];](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-3.jpg)
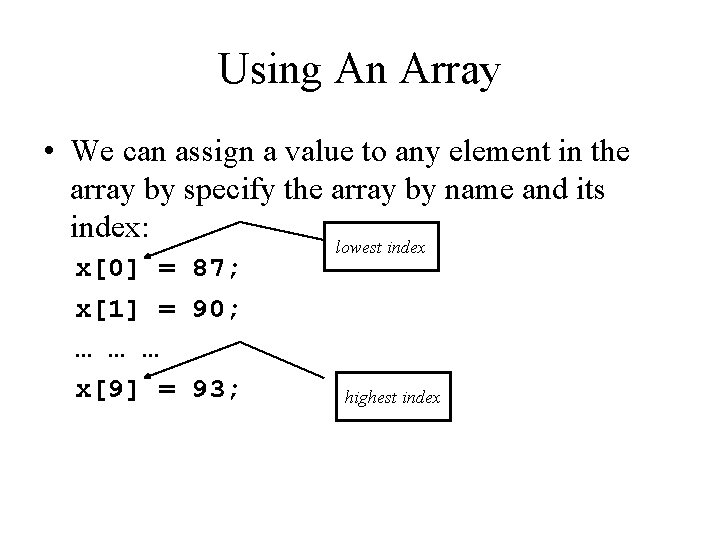
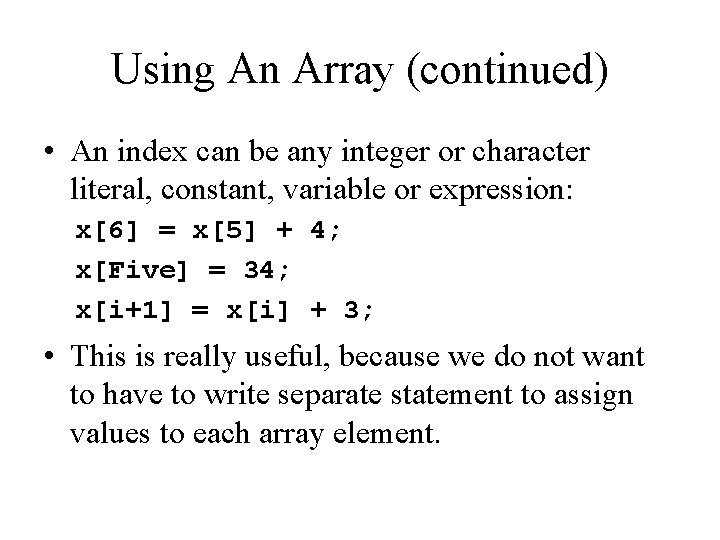
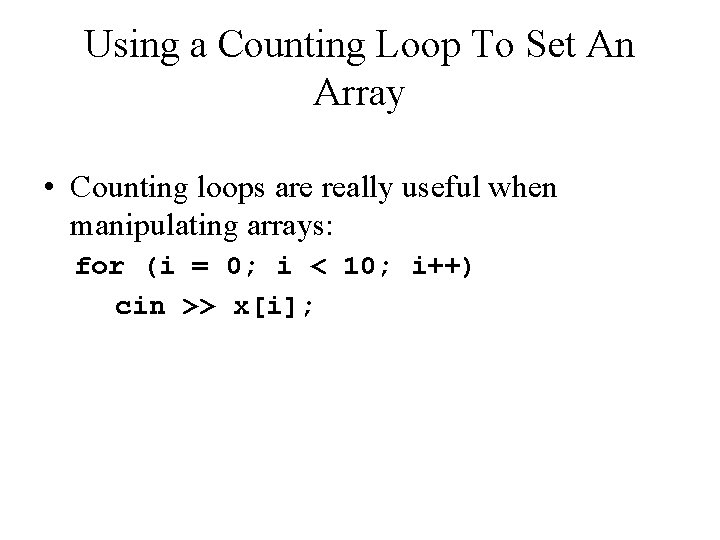
![A Program To Find Class Average #include <iostream> using namespace std; void getgrades(int grades[]); A Program To Find Class Average #include <iostream> using namespace std; void getgrades(int grades[]);](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-7.jpg)
![int main(void) { int grades[numgrades], average; getgrades(grades); average = calcaverage(grades); printresults(grades, average); return(0); } int main(void) { int grades[numgrades], average; getgrades(grades); average = calcaverage(grades); printresults(grades, average); return(0); }](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-8.jpg)
![void getgrades(int grades[]) { int count; for (count = 0; count < numgrades; count++) void getgrades(int grades[]) { int count; for (count = 0; count < numgrades; count++)](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-9.jpg)
![void printresults(int grades[], int mean) { int i; cout << "The grades are: " void printresults(int grades[], int mean) { int i; cout << "The grades are: "](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-10.jpg)
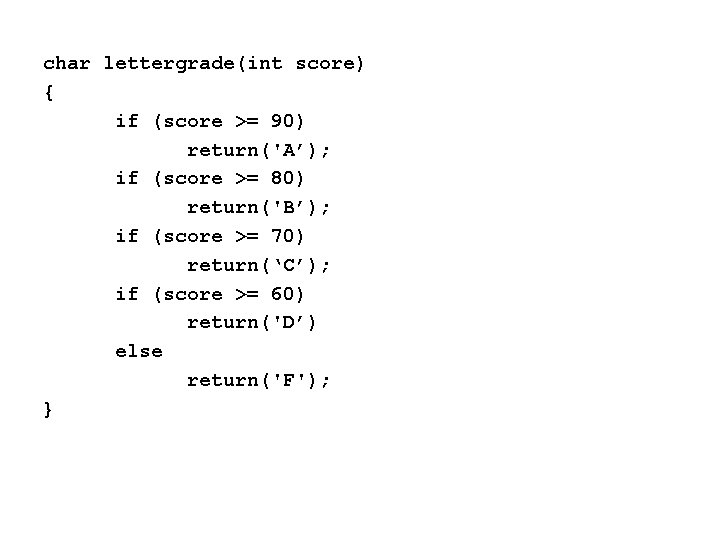
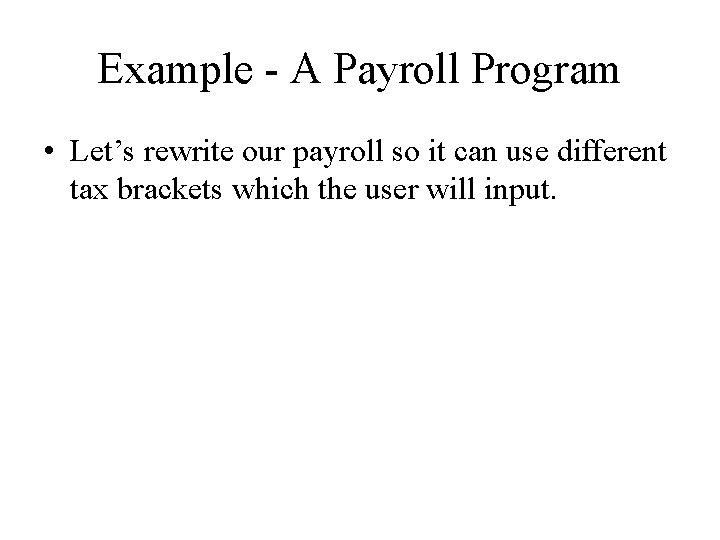
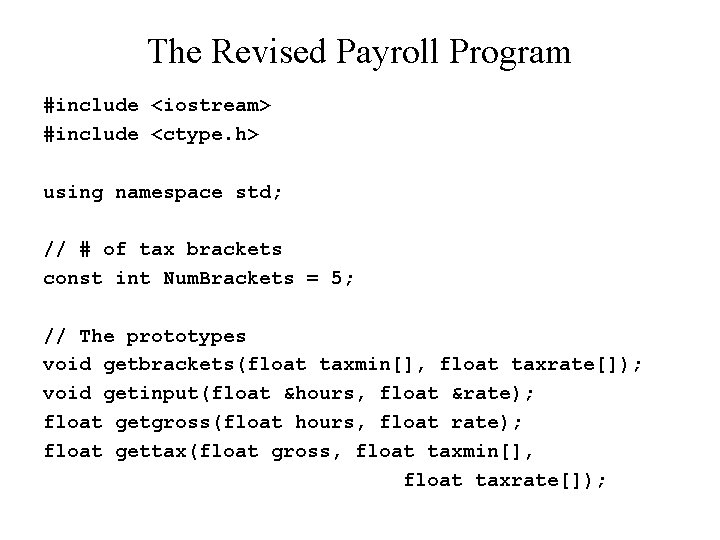
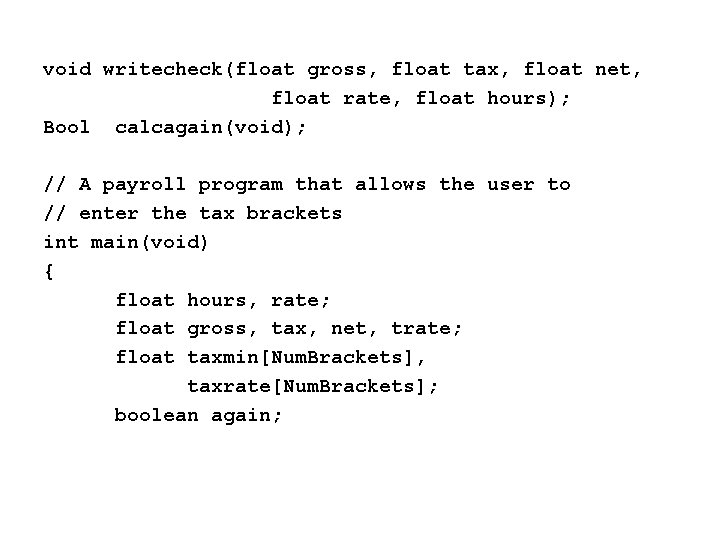
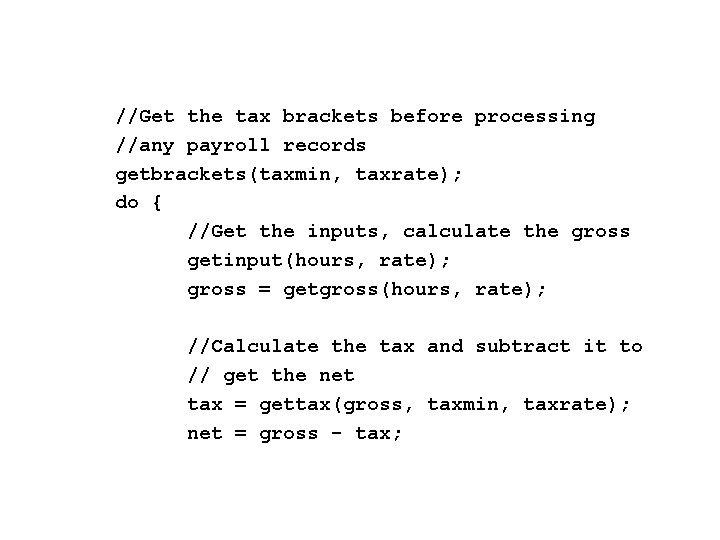
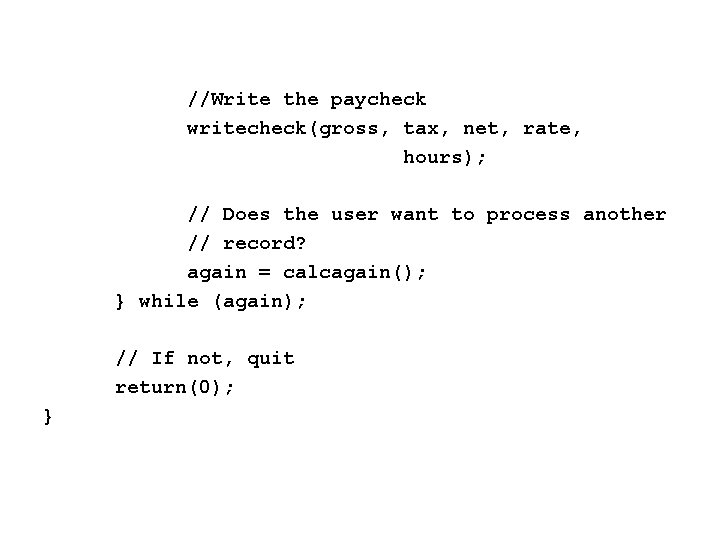
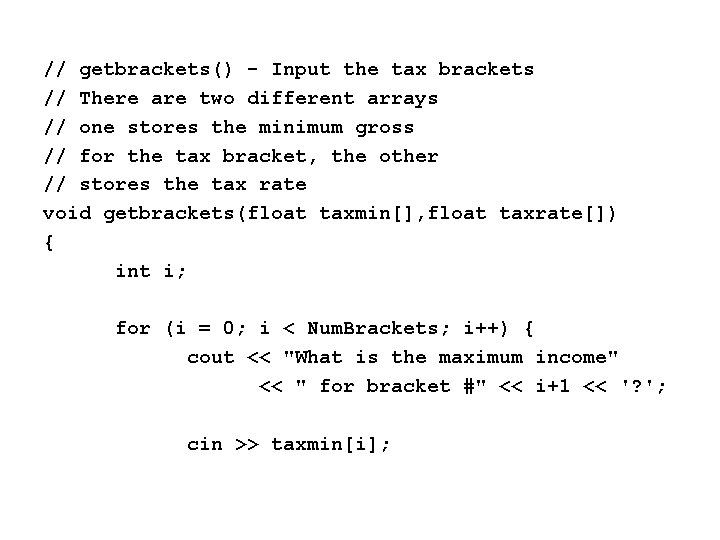
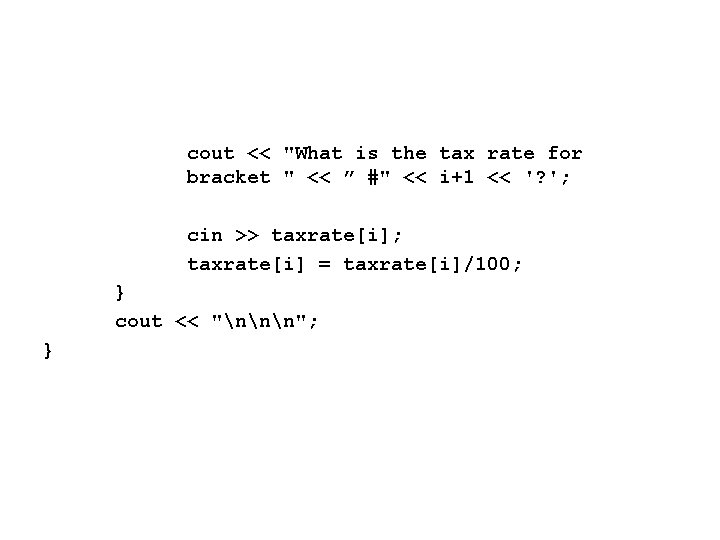
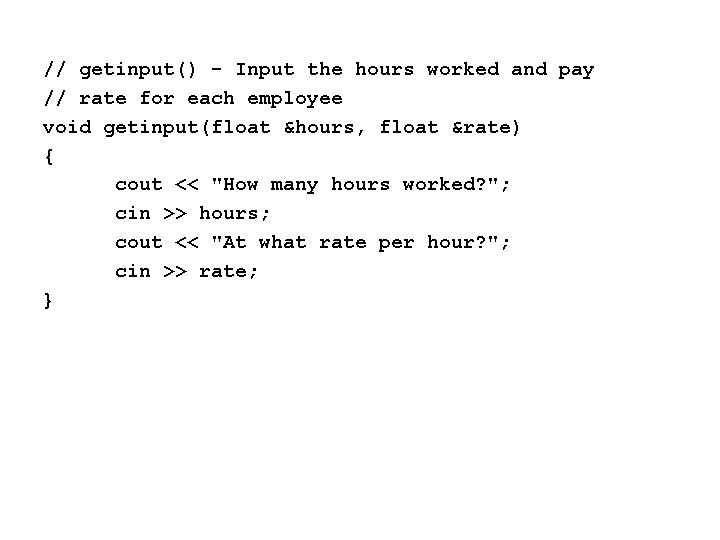
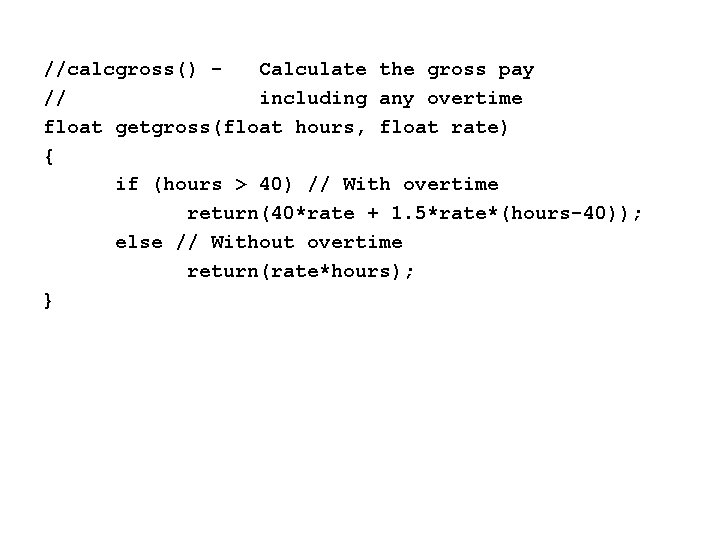
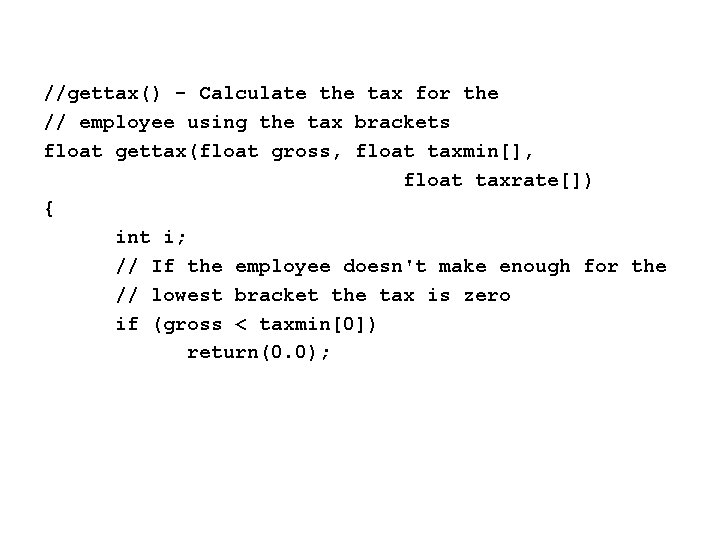
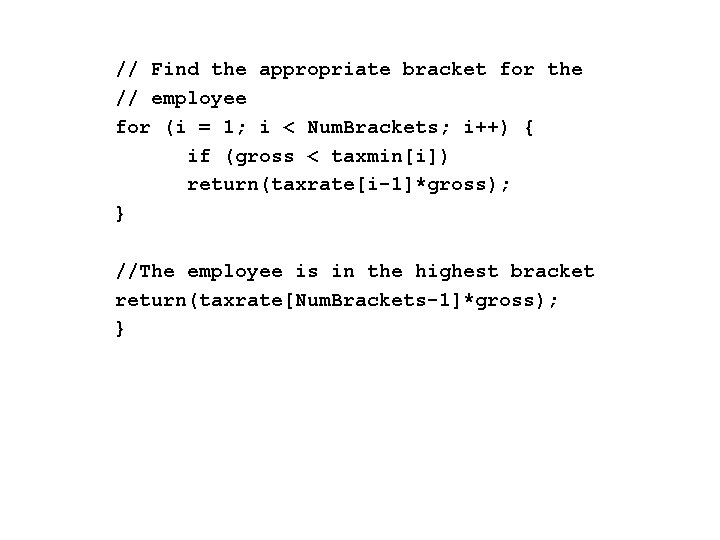
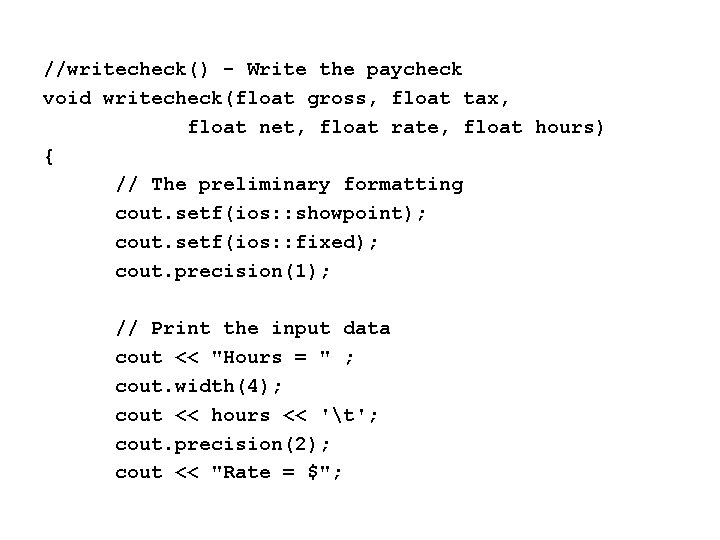
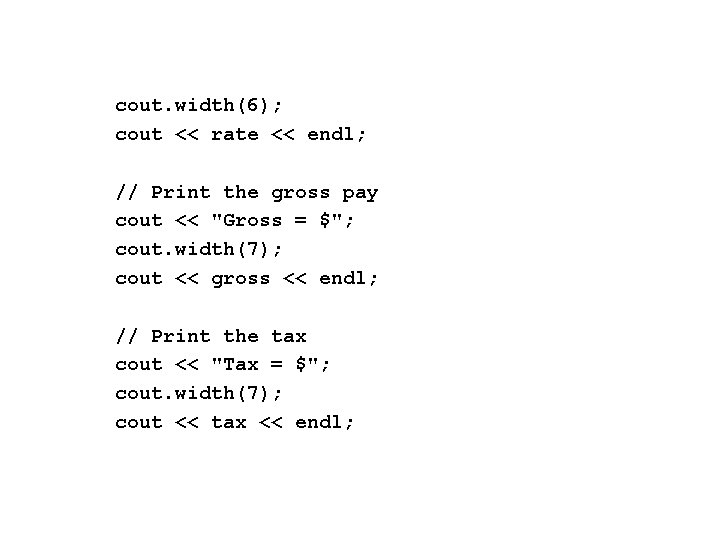
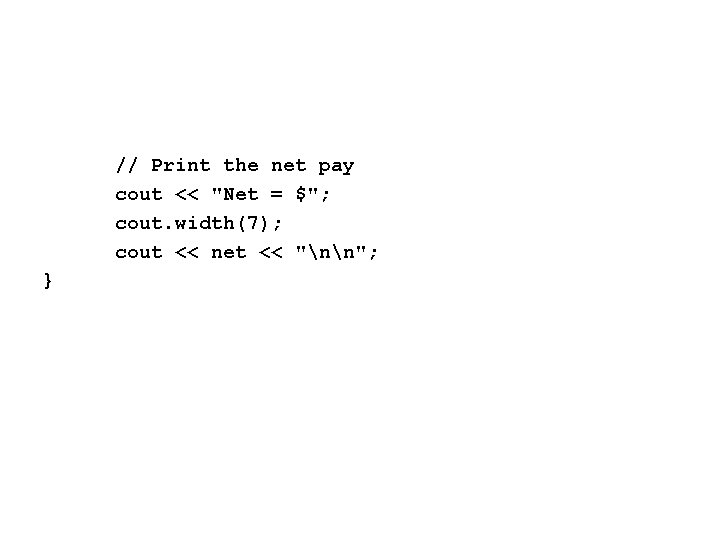
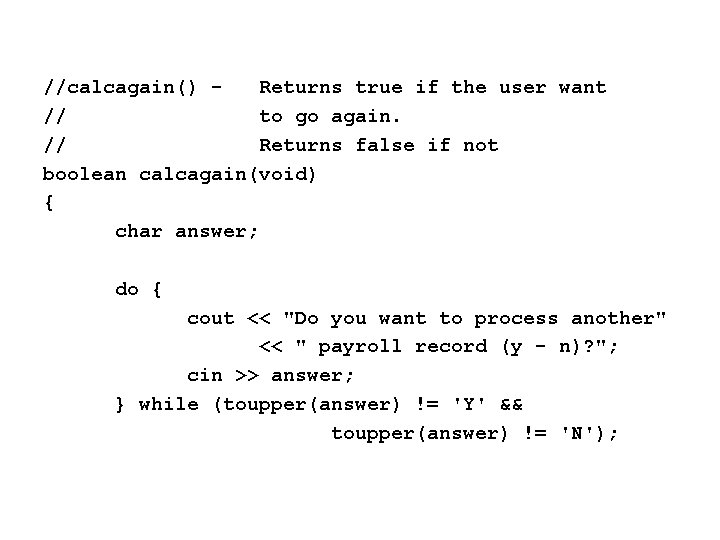
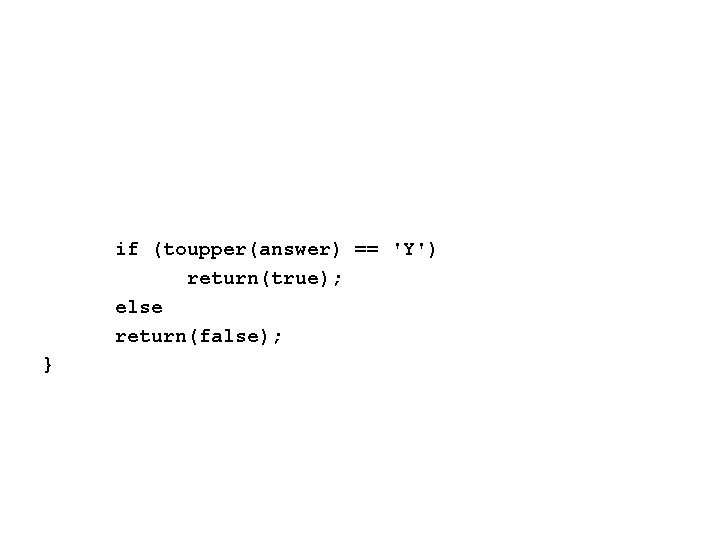
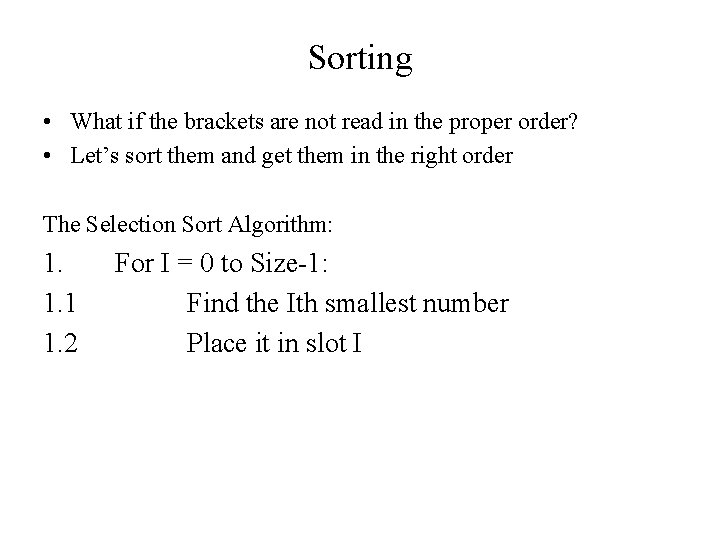
![Selection Sorting #include <iostream. h> const int Size = 5; void sort(int x[]); int Selection Sorting #include <iostream. h> const int Size = 5; void sort(int x[]); int](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-29.jpg)
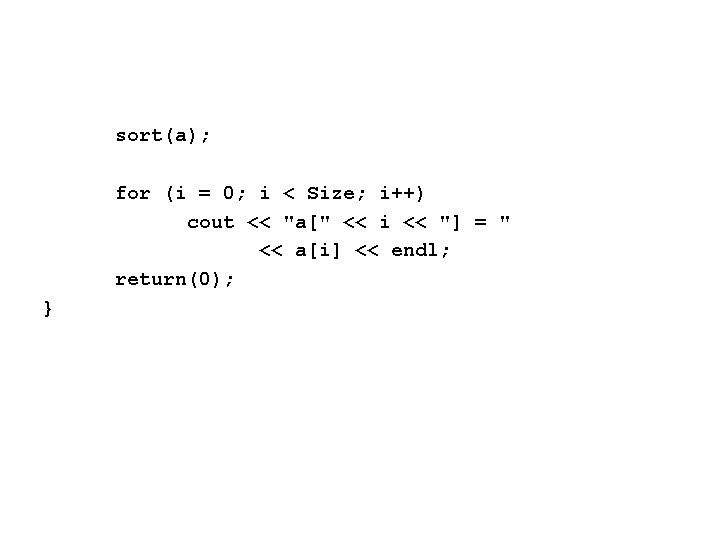
![// sort() - Sort an array of numbers void sort(int x[]) { int i, // sort() - Sort an array of numbers void sort(int x[]) { int i,](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-31.jpg)
![for (j = i; j < Size; j++) if (x[j] <small) { small = for (j = i; j < Size; j++) if (x[j] <small) { small =](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-32.jpg)
![Multidimensional Arrays • You can declare a two-dimensional array by writing: int x[Num. Rows][Num. Multidimensional Arrays • You can declare a two-dimensional array by writing: int x[Num. Rows][Num.](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-33.jpg)
![Implementing Multidimensional Arrays x[0][0] x[0][1] x[0][2] x[0][3] x[0][4] x[0][5] x[1][0] x[1][1] x[1][2] x[1][3] x[1][4] Implementing Multidimensional Arrays x[0][0] x[0][1] x[0][2] x[0][3] x[0][4] x[0][5] x[1][0] x[1][1] x[1][2] x[1][3] x[1][4]](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-34.jpg)
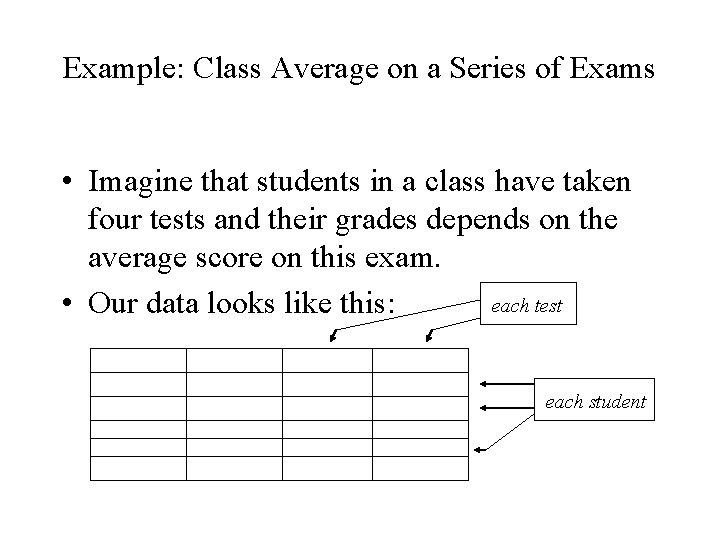
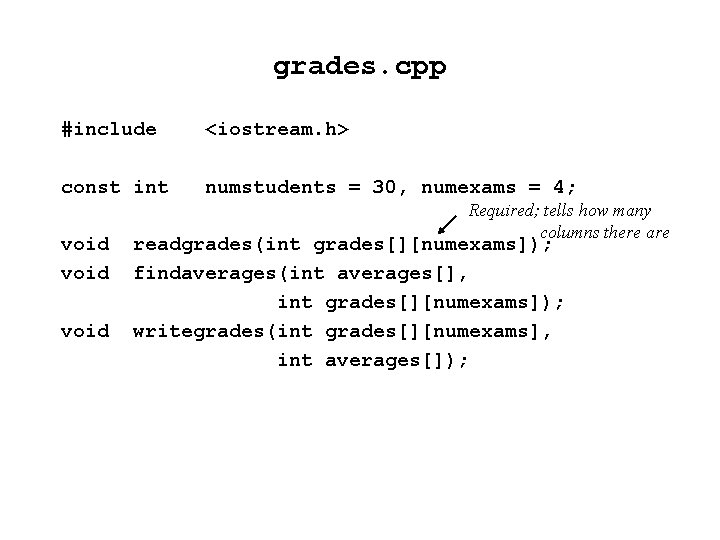
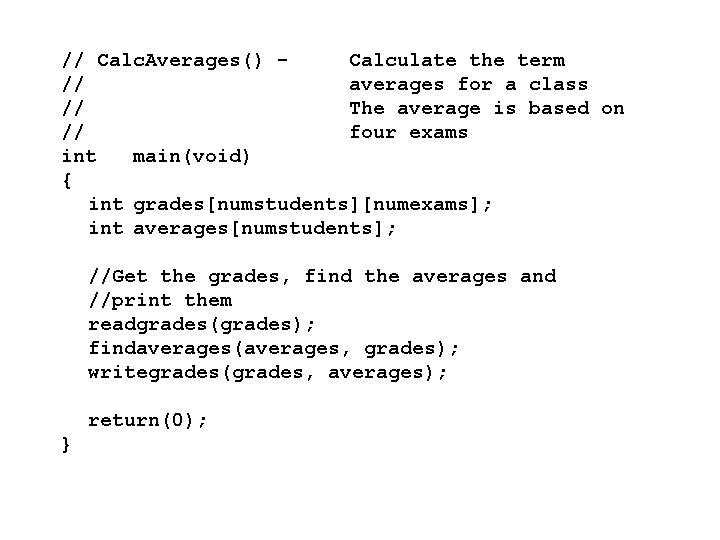
![// readgrades() - Read the complete set of grades void readgrades(int grades[][numexams]) { int // readgrades() - Read the complete set of grades void readgrades(int grades[][numexams]) { int](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-38.jpg)
![// Find. Averages() Find the average for each // student void findaverages(int averages[], int // Find. Averages() Find the average for each // student void findaverages(int averages[], int](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-39.jpg)
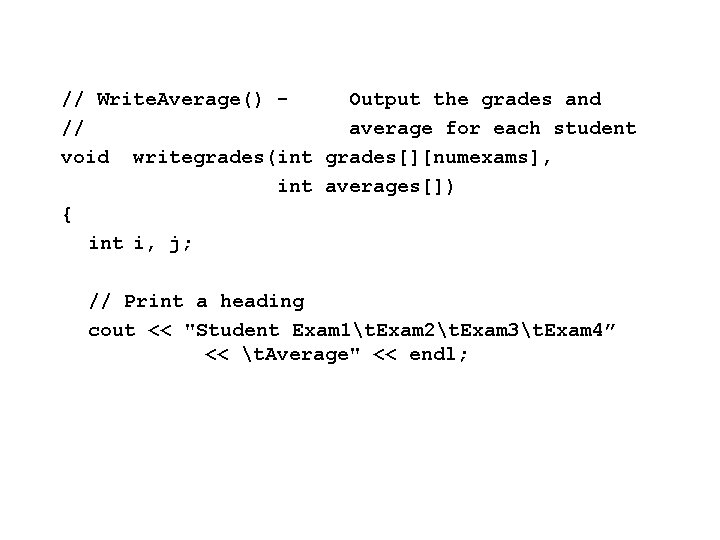
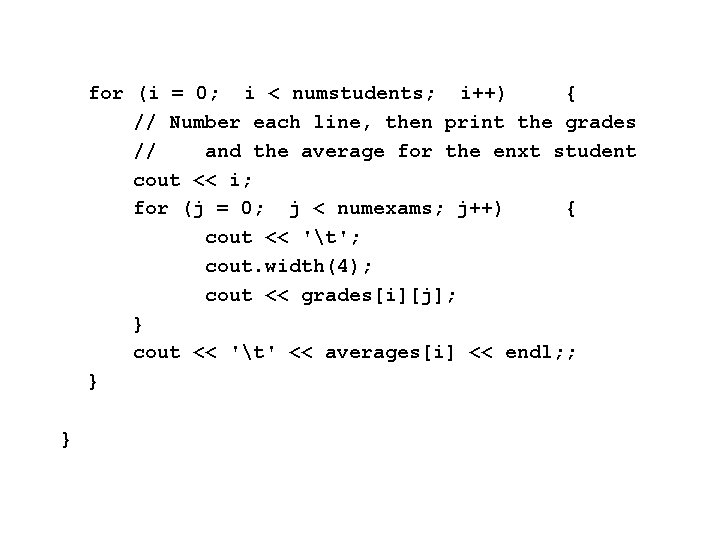
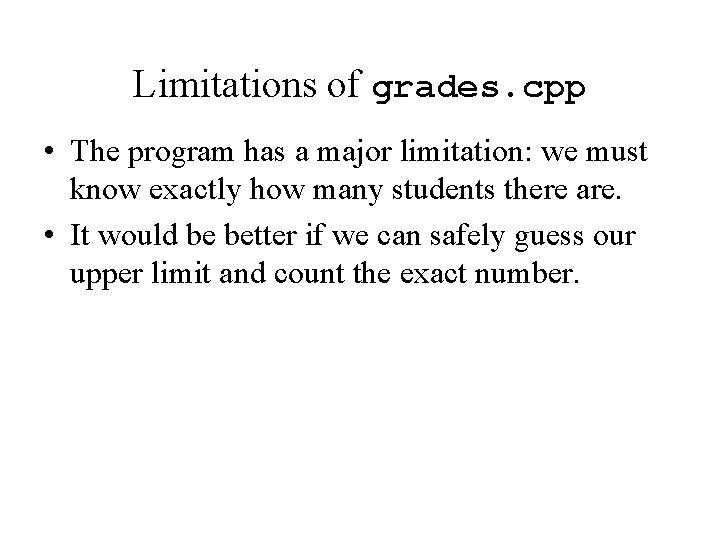
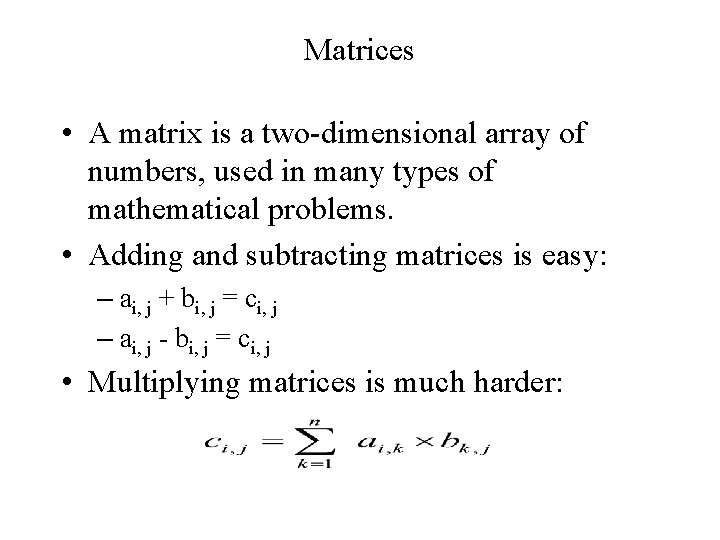
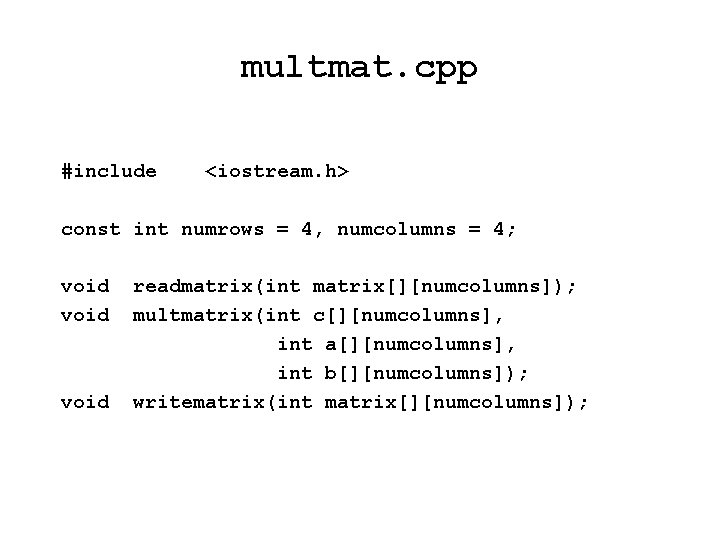
![// multmat() – Read and multiply two matrices int main(void) { int a[numrows][numcolumns], b[numrows][numcolumns], // multmat() – Read and multiply two matrices int main(void) { int a[numrows][numcolumns], b[numrows][numcolumns],](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-45.jpg)
![//readmatrix() - Read in a matrix void readmatrix(int matrix[][numcolumns]) { int i, j; for //readmatrix() - Read in a matrix void readmatrix(int matrix[][numcolumns]) { int i, j; for](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-46.jpg)
![// multmatrix() – Multiply a x b to get c void multmatrix(int c[][numcolumns], int // multmatrix() – Multiply a x b to get c void multmatrix(int c[][numcolumns], int](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-47.jpg)
![// writematrix() – Write an i x j matrix void writematrix(int matrix[][numcolumns]) { int // writematrix() – Write an i x j matrix void writematrix(int matrix[][numcolumns]) { int](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-48.jpg)
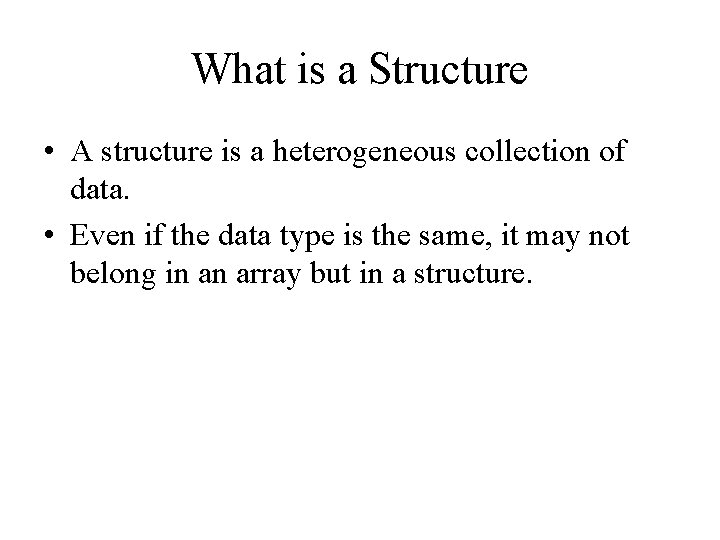
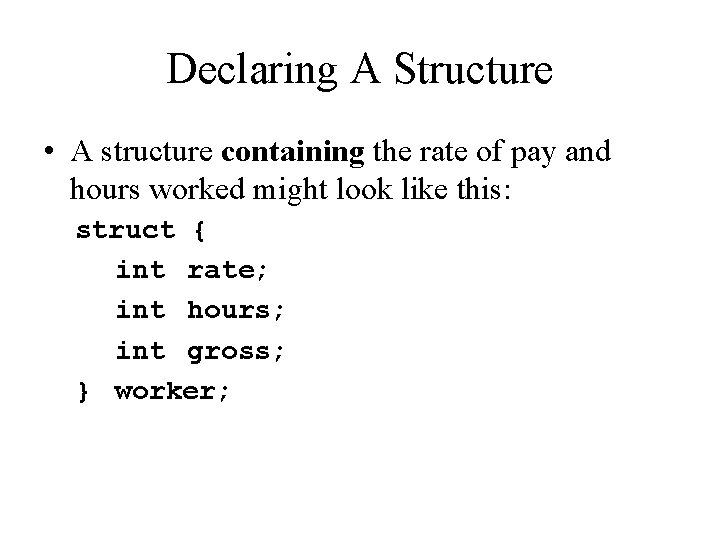
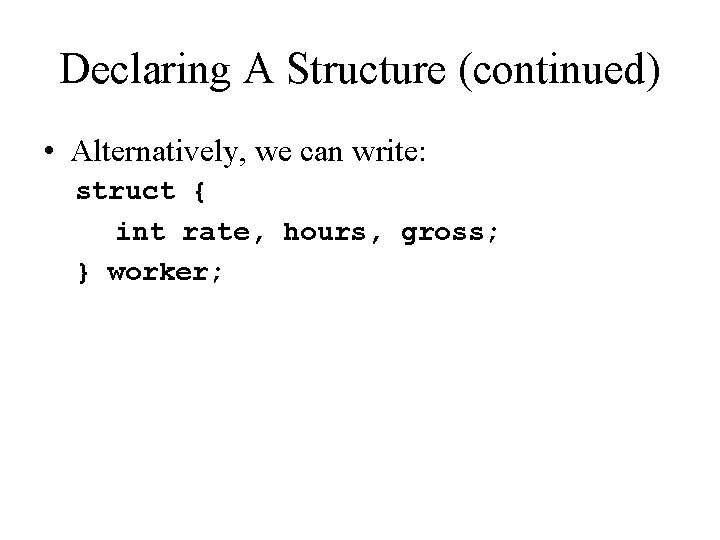
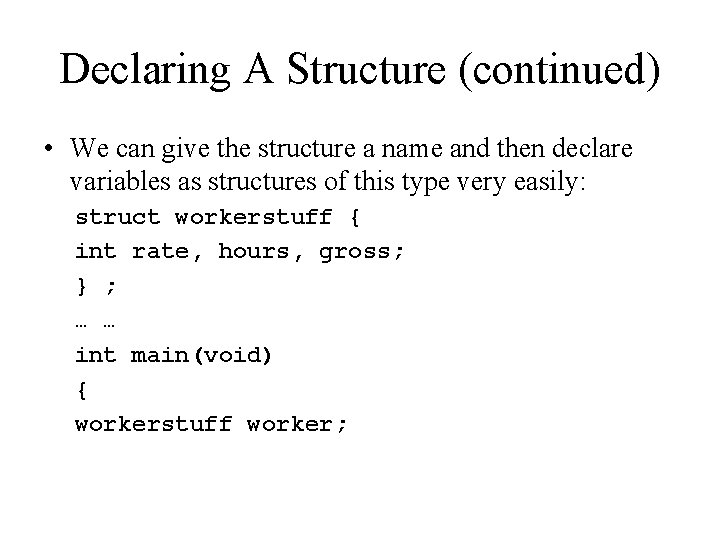
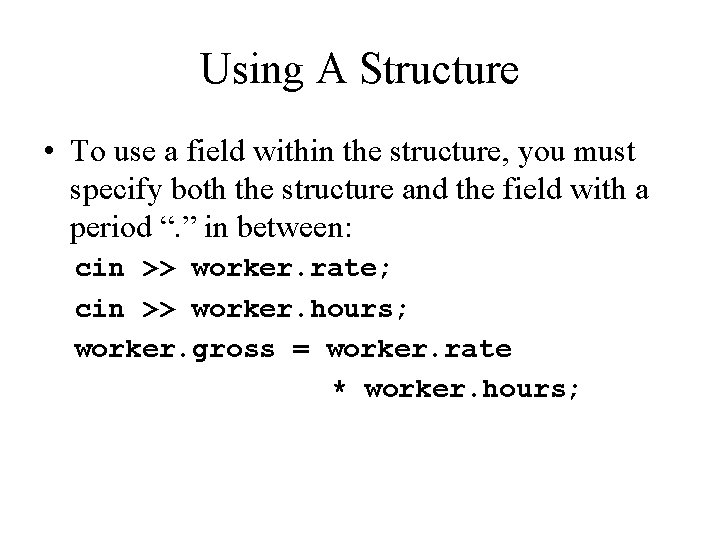
![A simple payroll program #include <iostream. h> struct workerstuff { char name[20]; float rate; A simple payroll program #include <iostream. h> struct workerstuff { char name[20]; float rate;](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-54.jpg)
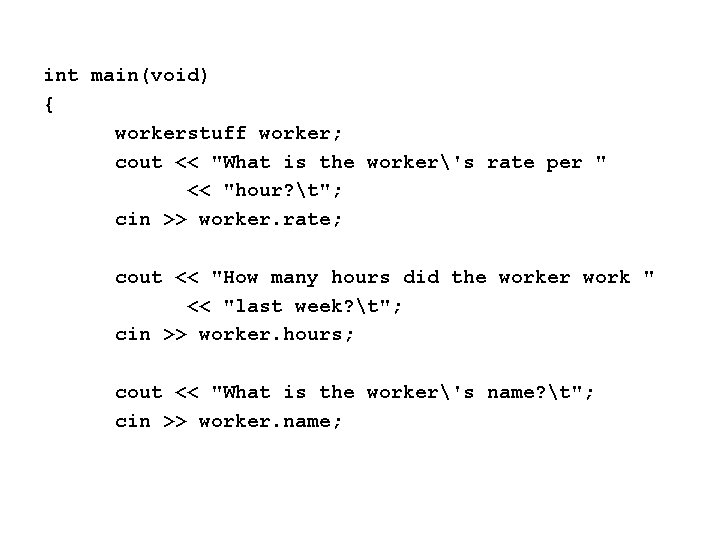
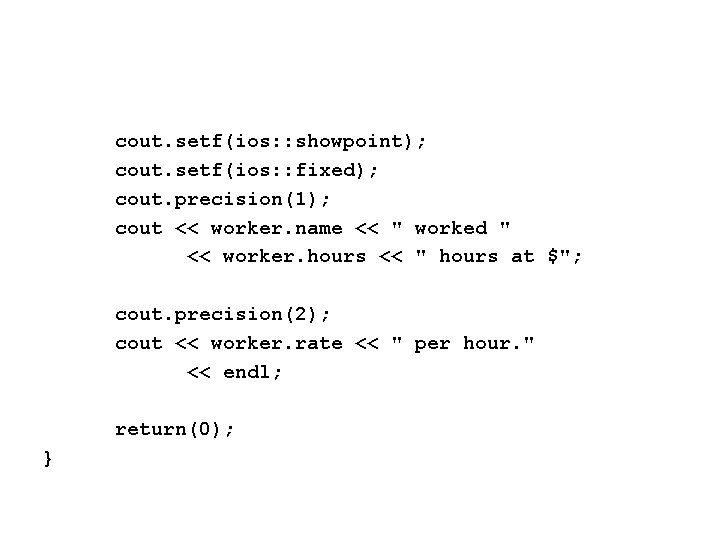
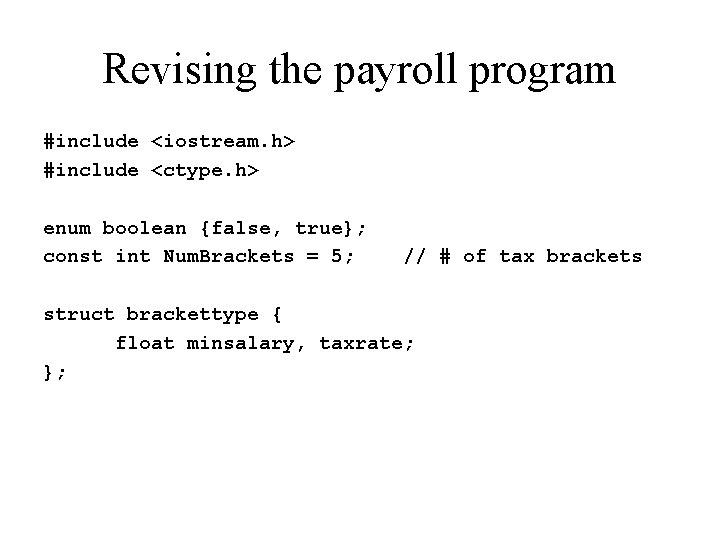
![// The prototypes void getbrackets(brackettype brackets[]); void getinput(float &hours, float &rate); float getgross(float hours, // The prototypes void getbrackets(brackettype brackets[]); void getinput(float &hours, float &rate); float getgross(float hours,](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-58.jpg)
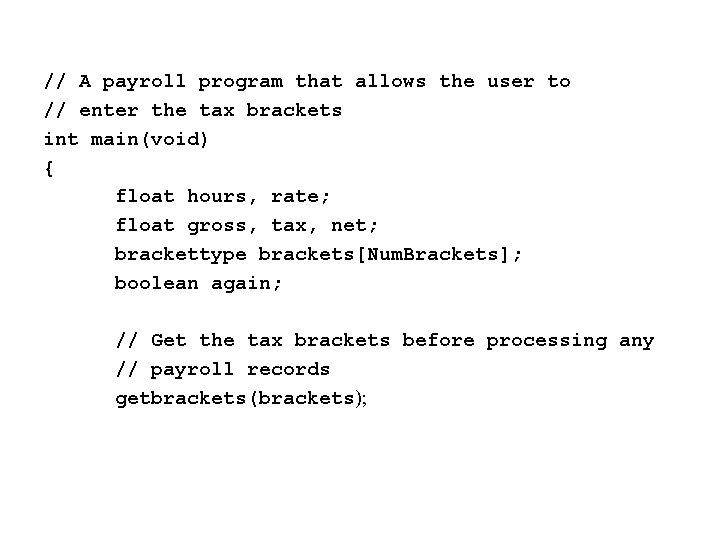
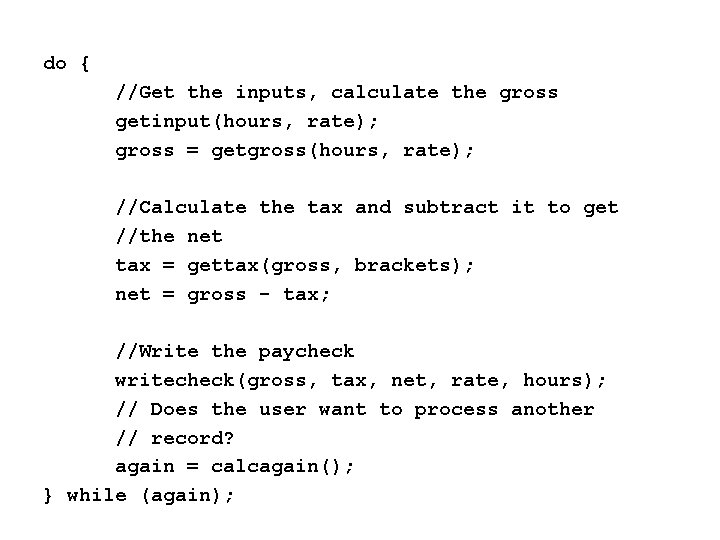
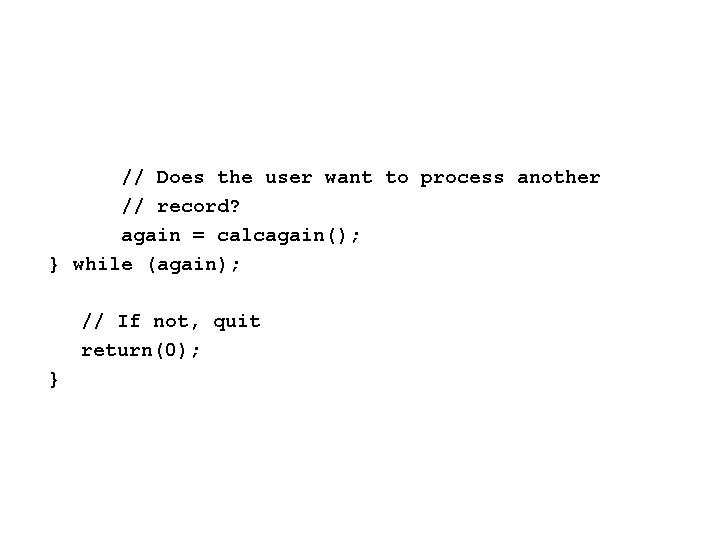
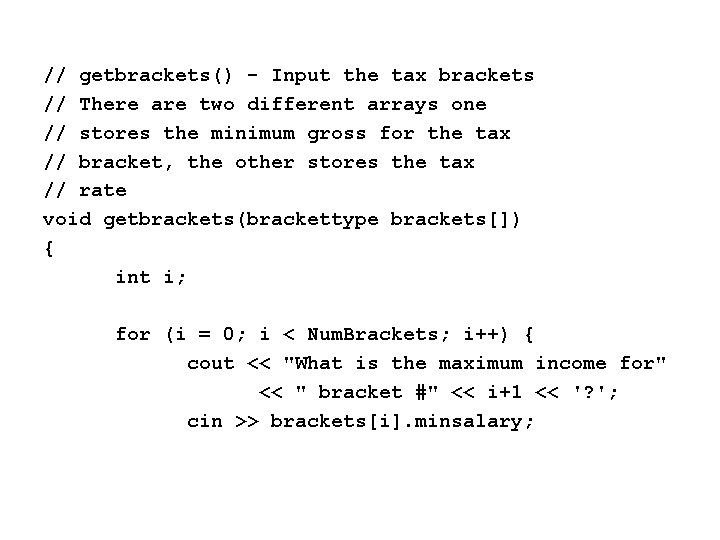
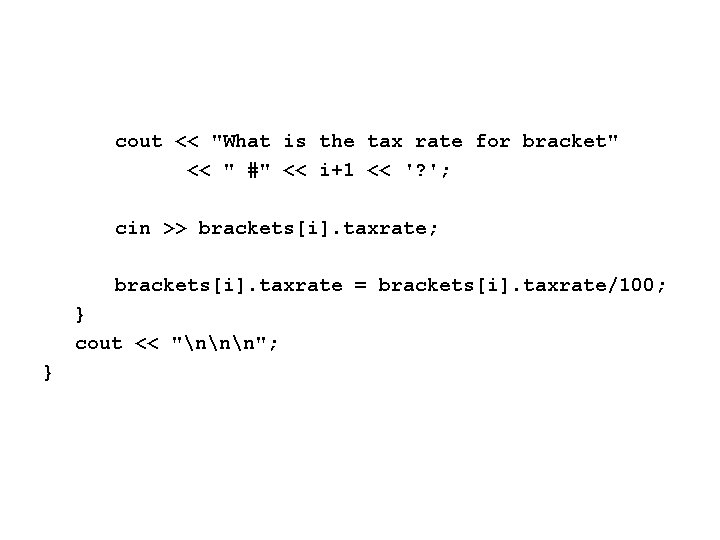
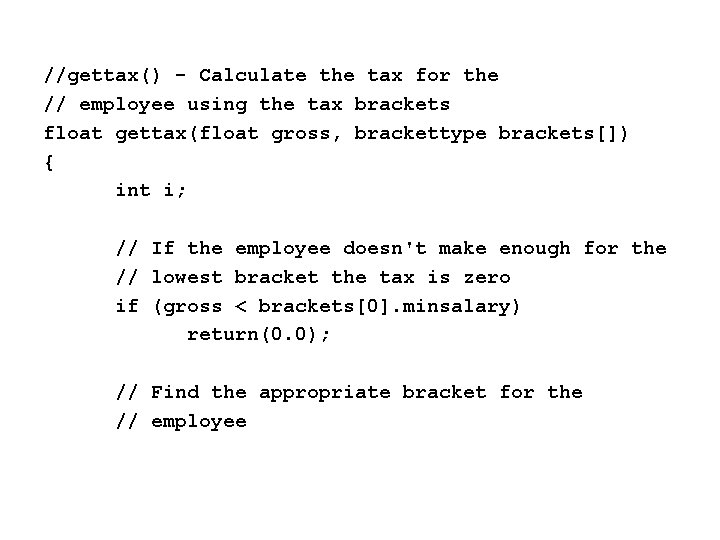
![for (i = 1; i < Num. Brackets; i++) { if (gross < brackets[i]. for (i = 1; i < Num. Brackets; i++) { if (gross < brackets[i].](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-65.jpg)
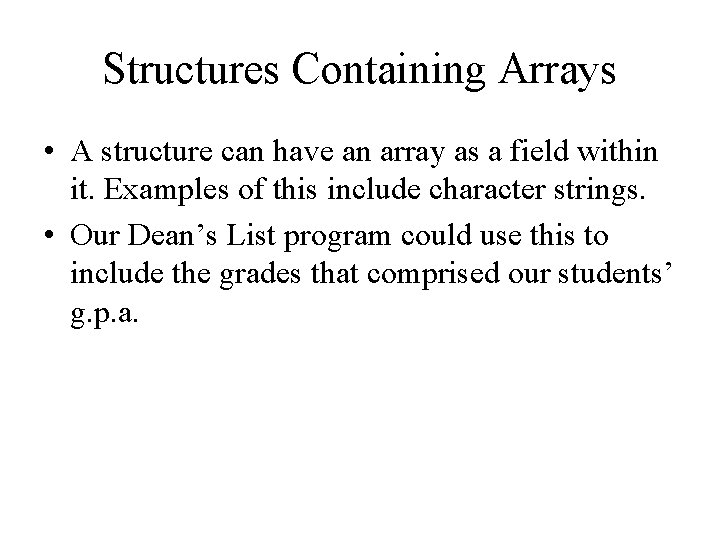
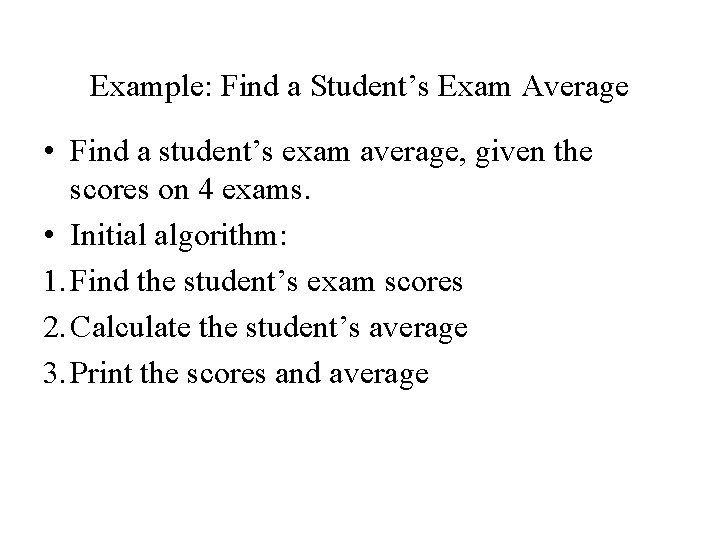
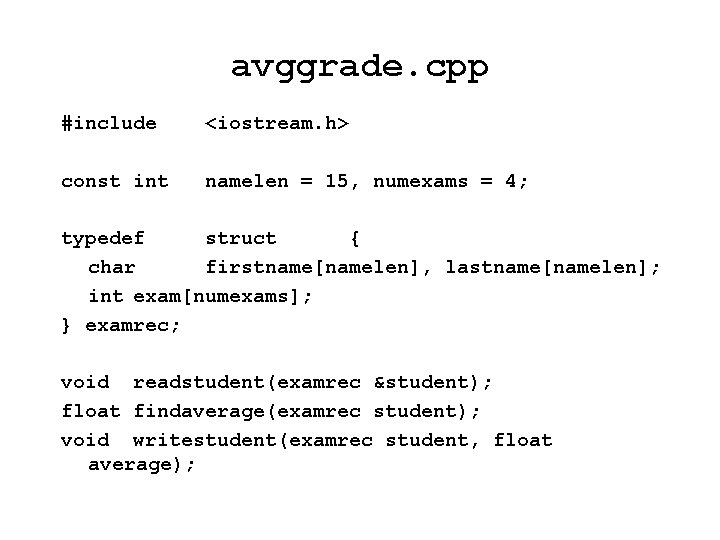
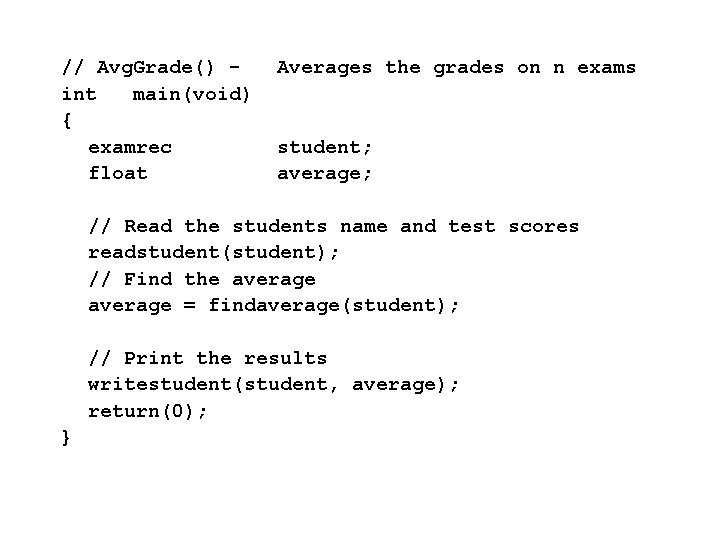
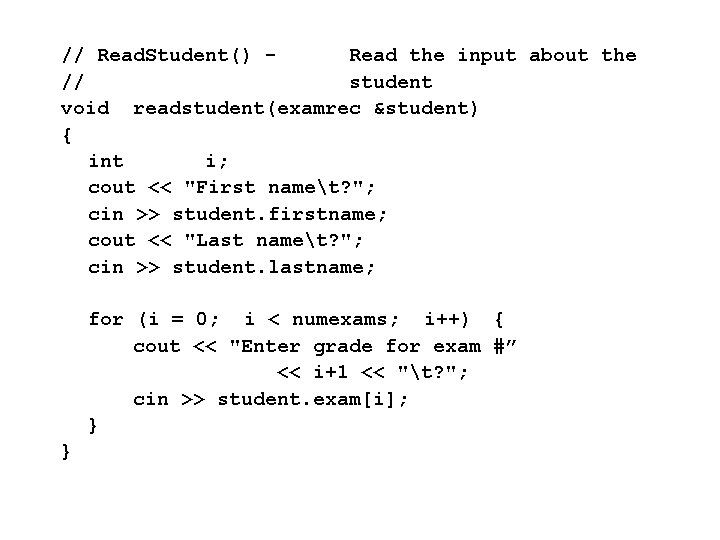
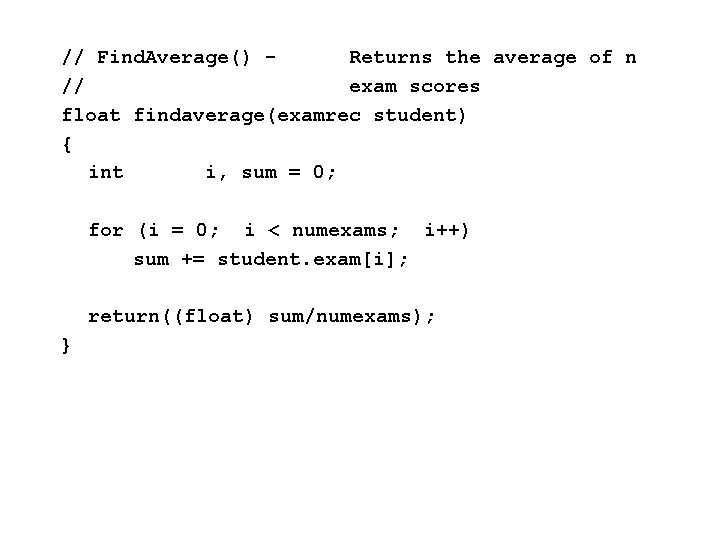
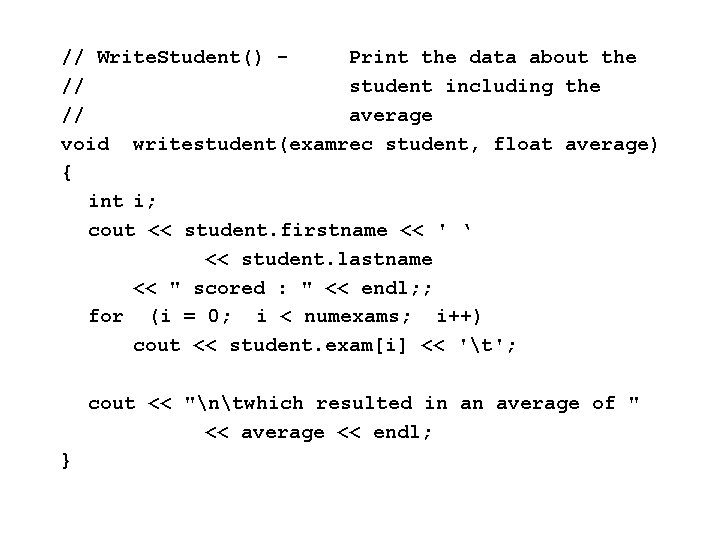
- Slides: 72
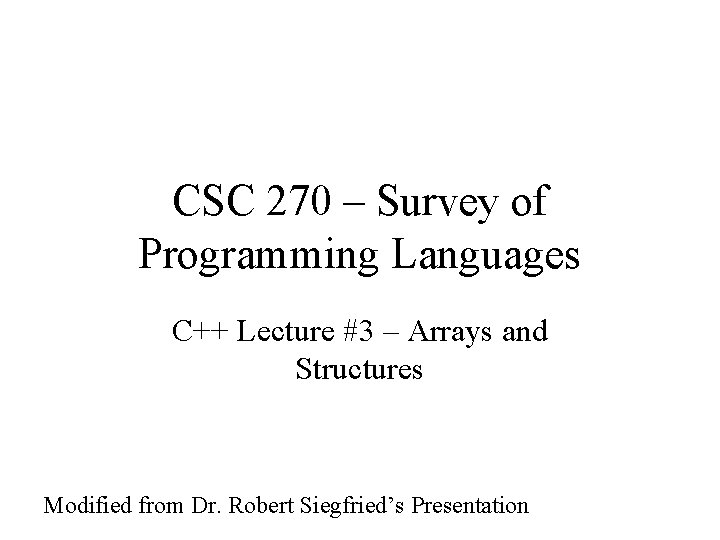
CSC 270 – Survey of Programming Languages C++ Lecture #3 – Arrays and Structures Modified from Dr. Robert Siegfried’s Presentation
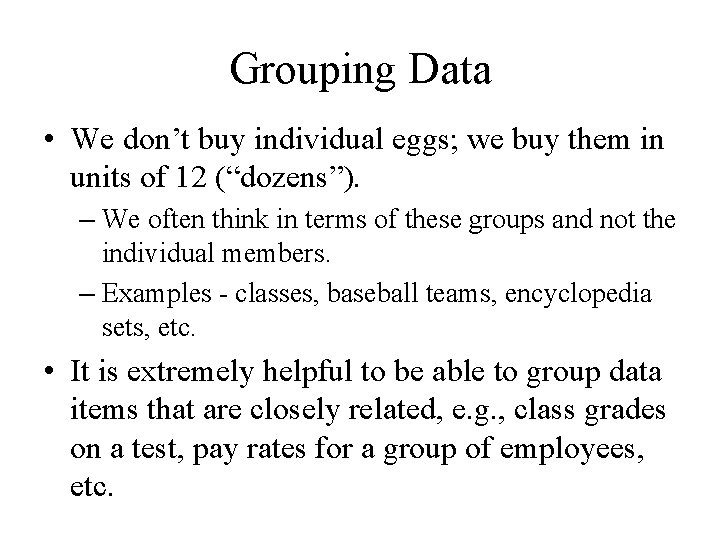
Grouping Data • We don’t buy individual eggs; we buy them in units of 12 (“dozens”). – We often think in terms of these groups and not the individual members. – Examples - classes, baseball teams, encyclopedia sets, etc. • It is extremely helpful to be able to group data items that are closely related, e. g. , class grades on a test, pay rates for a group of employees, etc.
![Declaring Arrays Instead of writing int x we can write int x10 Declaring Arrays • Instead of writing: int x; • we can write: int x[10];](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-3.jpg)
Declaring Arrays • Instead of writing: int x; • we can write: int x[10]; • the name x refers to the collection (or array) of integer values, which can contain up to 10 values.
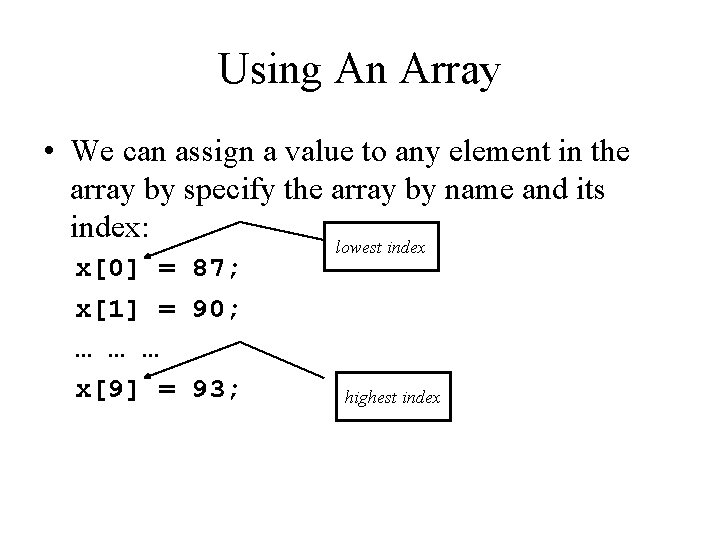
Using An Array • We can assign a value to any element in the array by specify the array by name and its index: lowest index x[0] = 87; x[1] = 90; … … … x[9] = 93; highest index
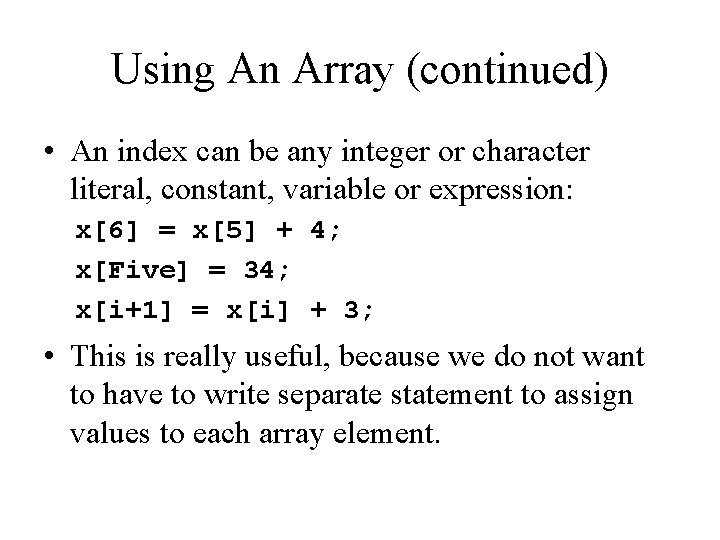
Using An Array (continued) • An index can be any integer or character literal, constant, variable or expression: x[6] = x[5] + 4; x[Five] = 34; x[i+1] = x[i] + 3; • This is really useful, because we do not want to have to write separate statement to assign values to each array element.
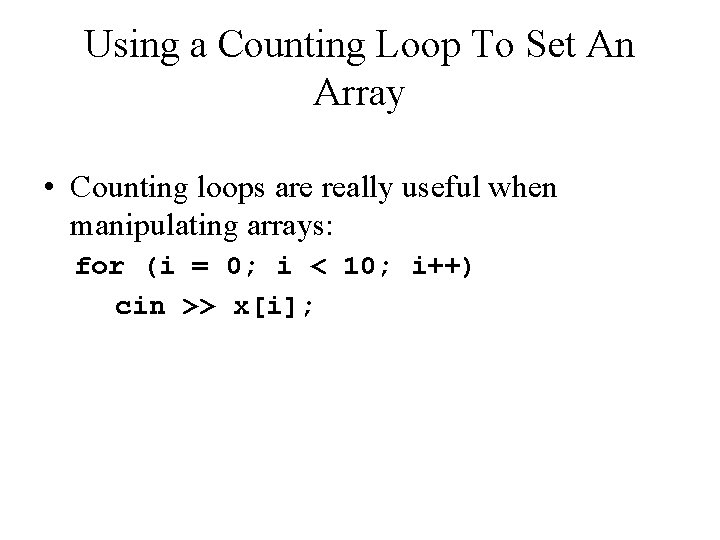
Using a Counting Loop To Set An Array • Counting loops are really useful when manipulating arrays: for (i = 0; i < 10; i++) cin >> x[i];
![A Program To Find Class Average include iostream using namespace std void getgradesint grades A Program To Find Class Average #include <iostream> using namespace std; void getgrades(int grades[]);](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-7.jpg)
A Program To Find Class Average #include <iostream> using namespace std; void getgrades(int grades[]); int calcaverage(int grades[]); void printresults(int grades[], int mean); char lettergrade(int score); const int numgrades = 10;
![int mainvoid int gradesnumgrades average getgradesgrades average calcaveragegrades printresultsgrades average return0 int main(void) { int grades[numgrades], average; getgrades(grades); average = calcaverage(grades); printresults(grades, average); return(0); }](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-8.jpg)
int main(void) { int grades[numgrades], average; getgrades(grades); average = calcaverage(grades); printresults(grades, average); return(0); }
![void getgradesint grades int count for count 0 count numgrades count void getgrades(int grades[]) { int count; for (count = 0; count < numgrades; count++)](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-9.jpg)
void getgrades(int grades[]) { int count; for (count = 0; count < numgrades; count++) { cout << "Enter a grade "; cin >> grades[count]; } } int calcaverage(int grades[]) { int count, sum = 0; for (count = 0; count < numgrades; count++) sum = sum + grades[count]; return(sum/numgrades); }
![void printresultsint grades int mean int i cout The grades are void printresults(int grades[], int mean) { int i; cout << "The grades are: "](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-10.jpg)
void printresults(int grades[], int mean) { int i; cout << "The grades are: " << endl; for (i = 0; i < 10; i++) cout << grades[i] << endl; cout << "The average is " << mean; cout << " corresponding to a grade of “ << lettergrade(mean) << endl; }
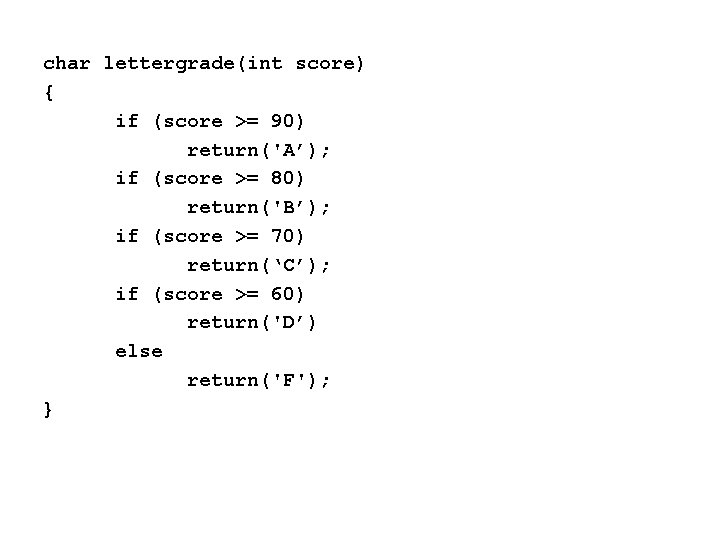
char lettergrade(int score) { if (score >= 90) return('A’); if (score >= 80) return('B’); if (score >= 70) return(‘C’); if (score >= 60) return('D’) else return('F'); }
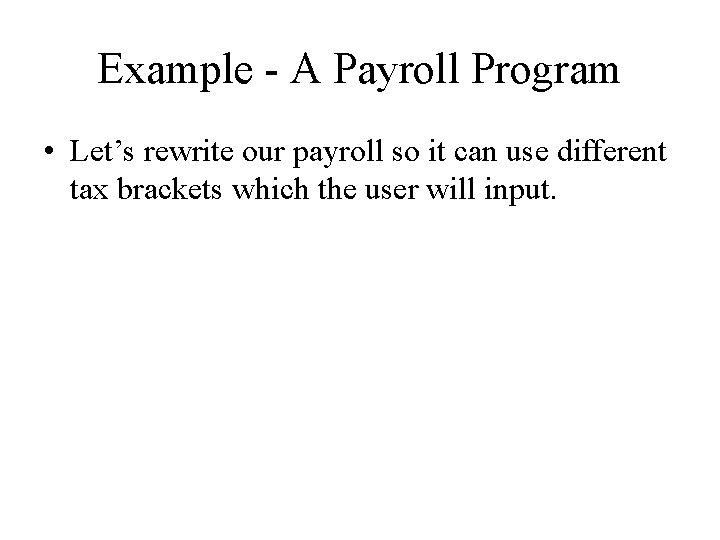
Example - A Payroll Program • Let’s rewrite our payroll so it can use different tax brackets which the user will input.
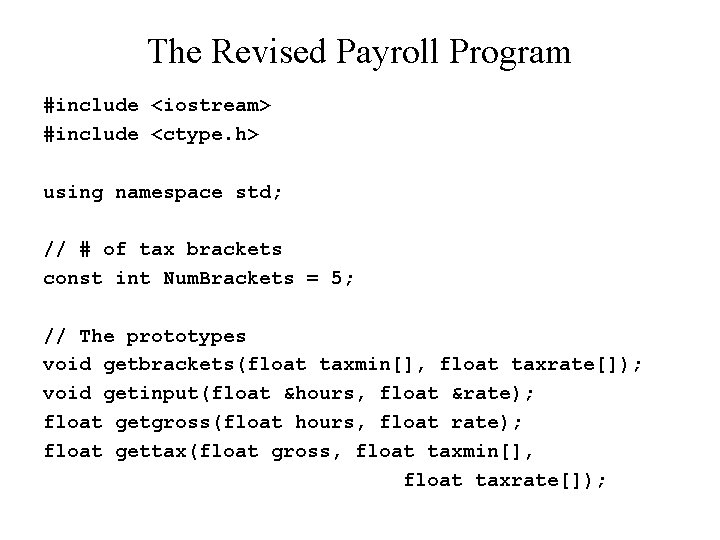
The Revised Payroll Program #include <iostream> #include <ctype. h> using namespace std; // # of tax brackets const int Num. Brackets = 5; // The prototypes void getbrackets(float taxmin[], float taxrate[]); void getinput(float &hours, float &rate); float getgross(float hours, float rate); float gettax(float gross, float taxmin[], float taxrate[]);
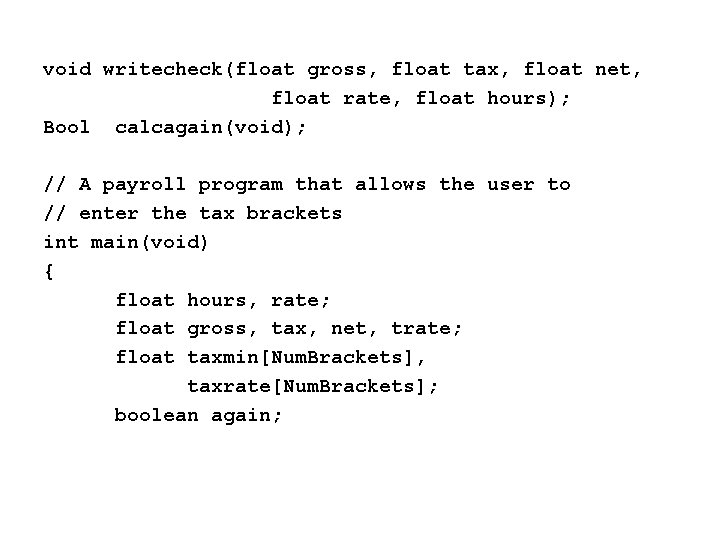
void writecheck(float gross, float tax, float net, float rate, float hours); Bool calcagain(void); // A payroll program that allows the user to // enter the tax brackets int main(void) { float hours, rate; float gross, tax, net, trate; float taxmin[Num. Brackets], taxrate[Num. Brackets]; boolean again;
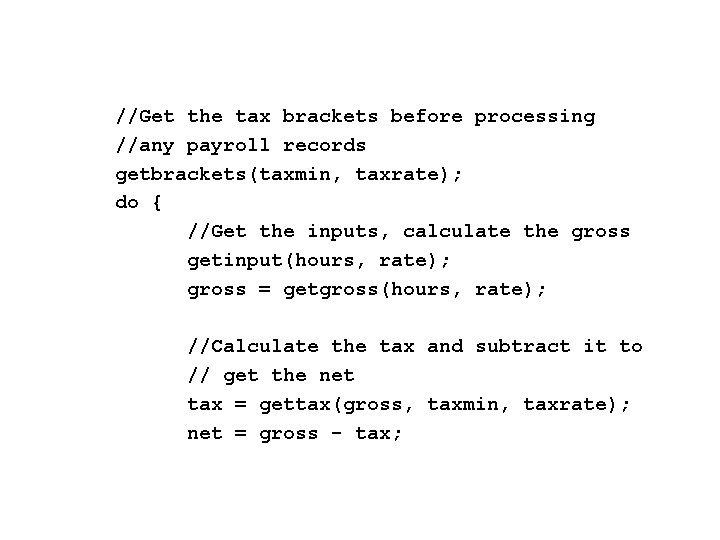
//Get the tax brackets before processing //any payroll records getbrackets(taxmin, taxrate); do { //Get the inputs, calculate the gross getinput(hours, rate); gross = getgross(hours, rate); //Calculate the tax and subtract it to // get the net tax = gettax(gross, taxmin, taxrate); net = gross - tax;
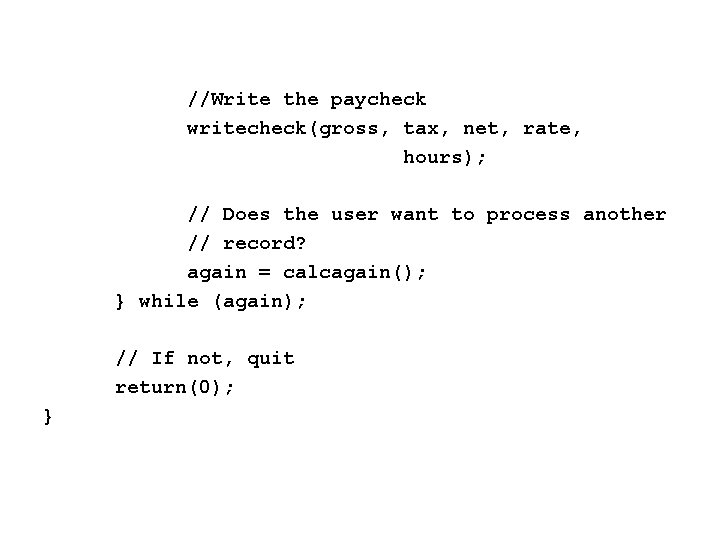
//Write the paycheck writecheck(gross, tax, net, rate, hours); // Does the user want to process another // record? again = calcagain(); } while (again); // If not, quit return(0); }
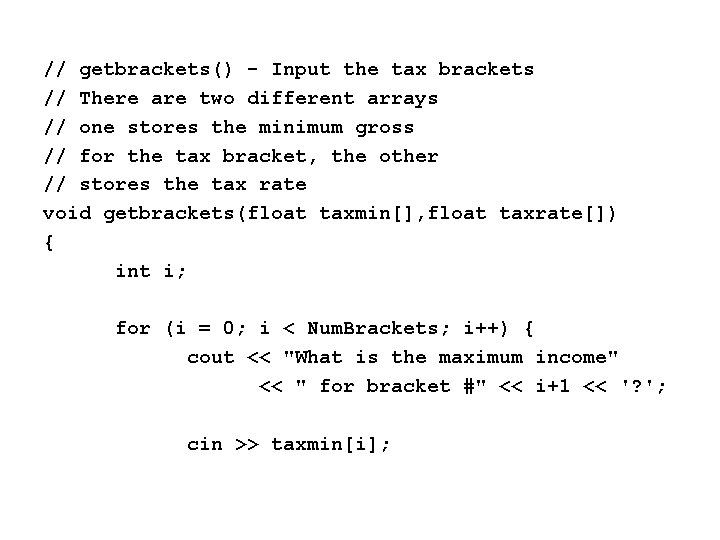
// getbrackets() - Input the tax brackets // There are two different arrays // one stores the minimum gross // for the tax bracket, the other // stores the tax rate void getbrackets(float taxmin[], float taxrate[]) { int i; for (i = 0; i < Num. Brackets; i++) { cout << "What is the maximum income" << " for bracket #" << i+1 << '? '; cin >> taxmin[i];
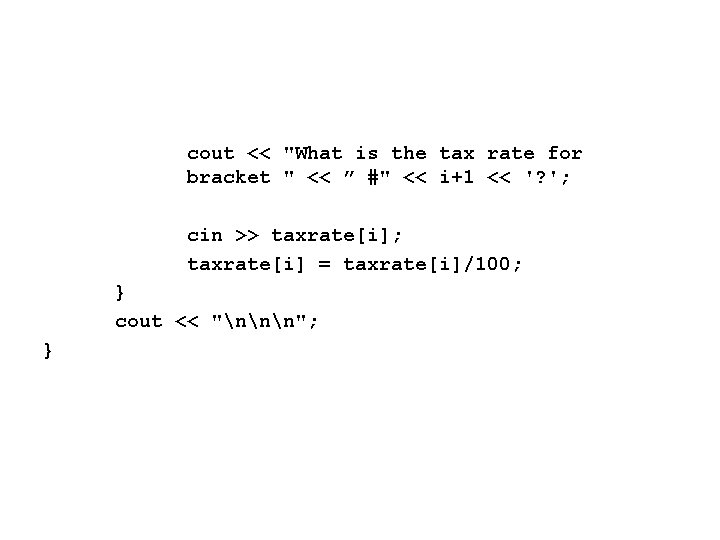
cout << "What is the tax rate for bracket " << ” #" << i+1 << '? '; cin >> taxrate[i]; taxrate[i] = taxrate[i]/100; } cout << "nnn"; }
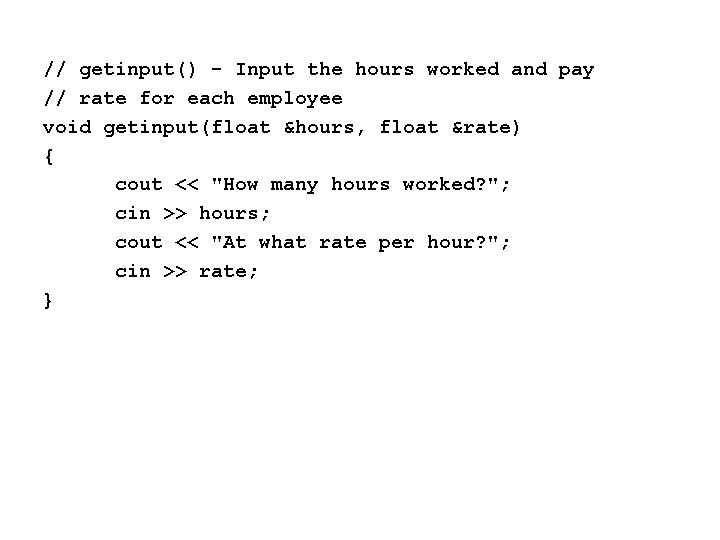
// getinput() - Input the hours worked and pay // rate for each employee void getinput(float &hours, float &rate) { cout << "How many hours worked? "; cin >> hours; cout << "At what rate per hour? "; cin >> rate; }
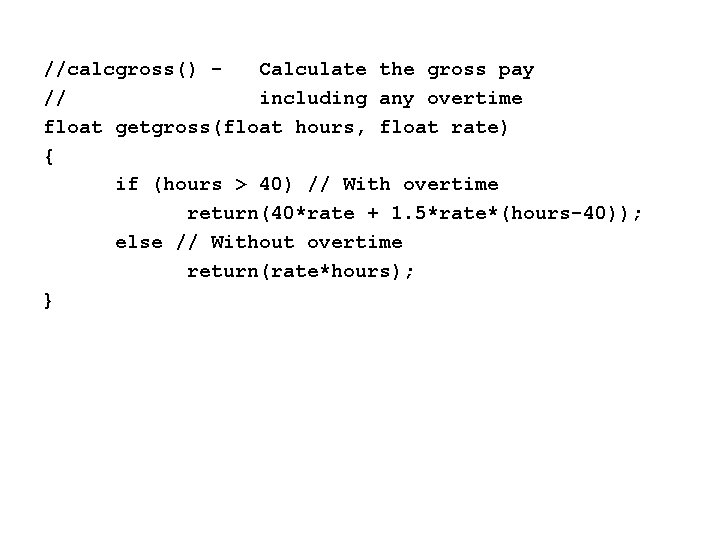
//calcgross() Calculate the gross pay // including any overtime float getgross(float hours, float rate) { if (hours > 40) // With overtime return(40*rate + 1. 5*rate*(hours-40)); else // Without overtime return(rate*hours); }
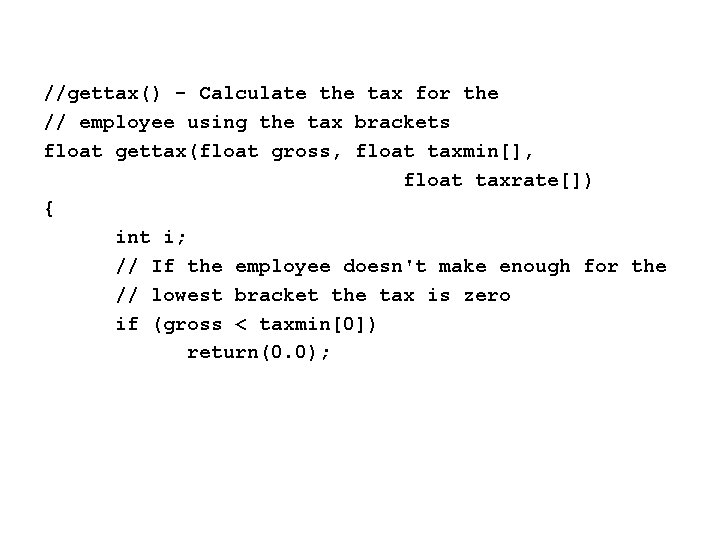
//gettax() - Calculate the tax for the // employee using the tax brackets float gettax(float gross, float taxmin[], float taxrate[]) { int i; // If the employee doesn't make enough for the // lowest bracket the tax is zero if (gross < taxmin[0]) return(0. 0);
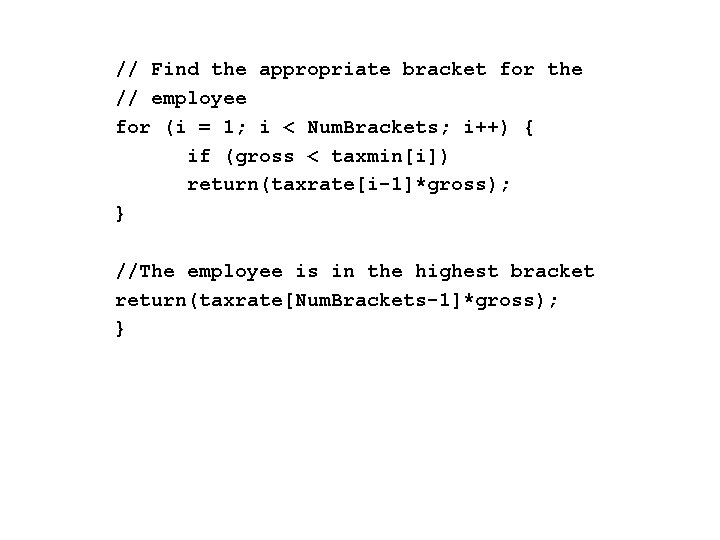
// Find the appropriate bracket for the // employee for (i = 1; i < Num. Brackets; i++) { if (gross < taxmin[i]) return(taxrate[i-1]*gross); } //The employee is in the highest bracket return(taxrate[Num. Brackets-1]*gross); }
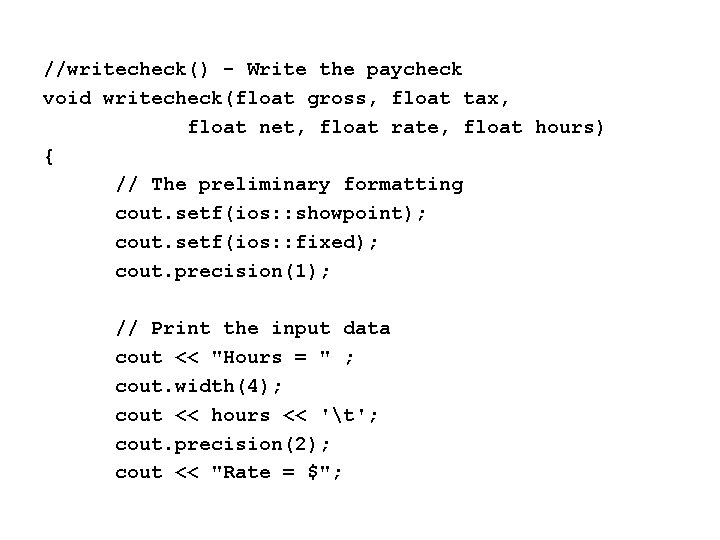
//writecheck() - Write the paycheck void writecheck(float gross, float tax, float net, float rate, float hours) { // The preliminary formatting cout. setf(ios: : showpoint); cout. setf(ios: : fixed); cout. precision(1); // Print the input data cout << "Hours = " ; cout. width(4); cout << hours << 't'; cout. precision(2); cout << "Rate = $";
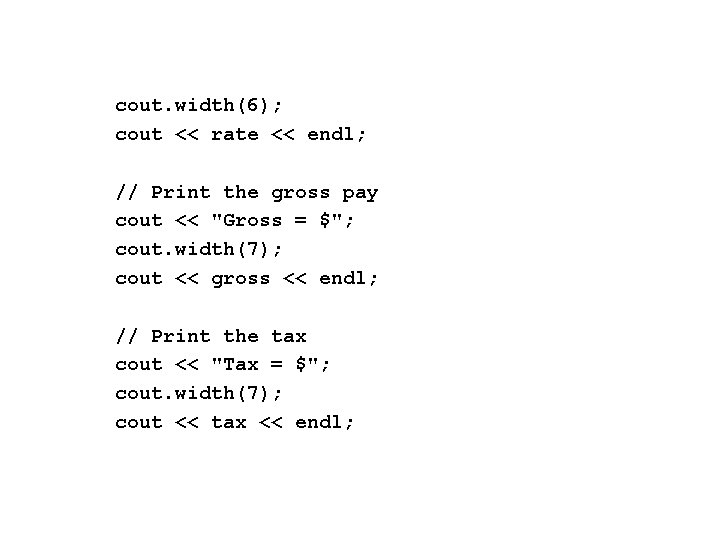
cout. width(6); cout << rate << endl; // Print the gross pay cout << "Gross = $"; cout. width(7); cout << gross << endl; // Print the tax cout << "Tax = $"; cout. width(7); cout << tax << endl;
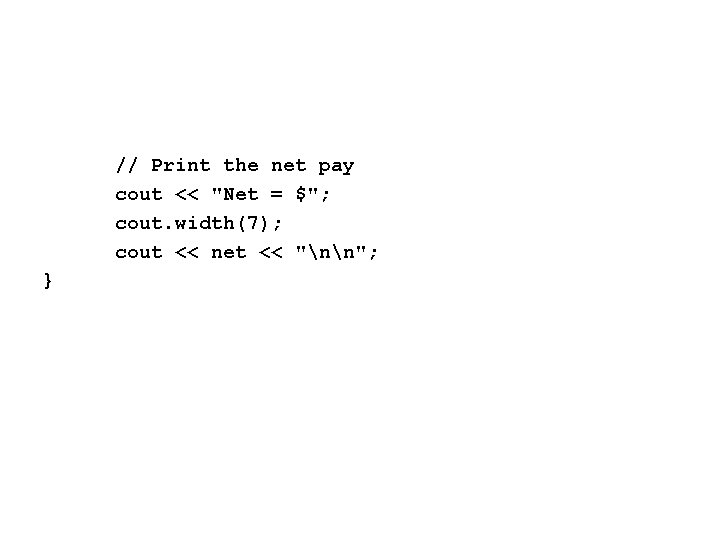
// Print the net pay cout << "Net = $"; cout. width(7); cout << net << "nn"; }
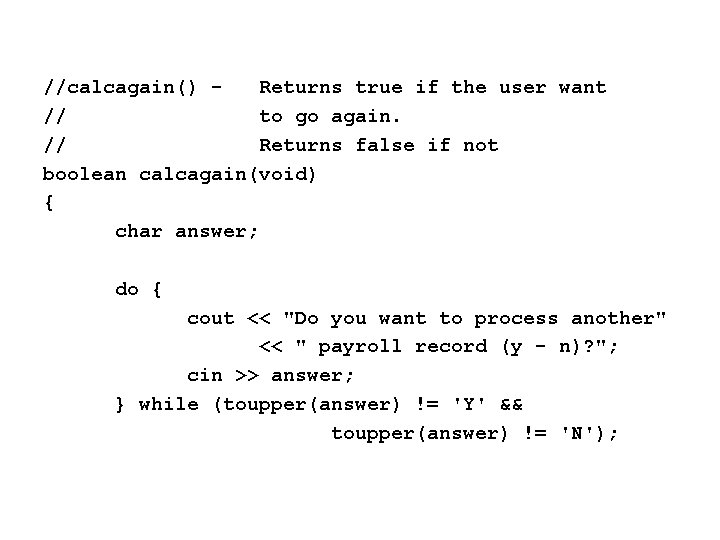
//calcagain() Returns true if the user want // to go again. // Returns false if not boolean calcagain(void) { char answer; do { cout << "Do you want to process another" << " payroll record (y - n)? "; cin >> answer; } while (toupper(answer) != 'Y' && toupper(answer) != 'N');
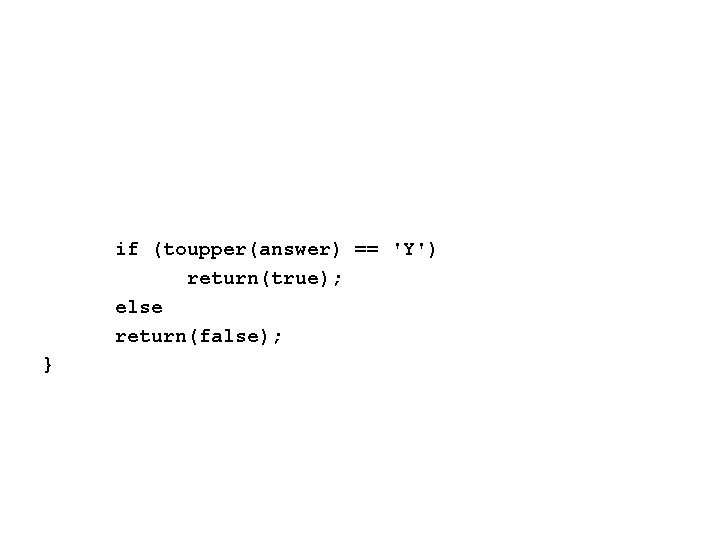
if (toupper(answer) == 'Y') return(true); else return(false); }
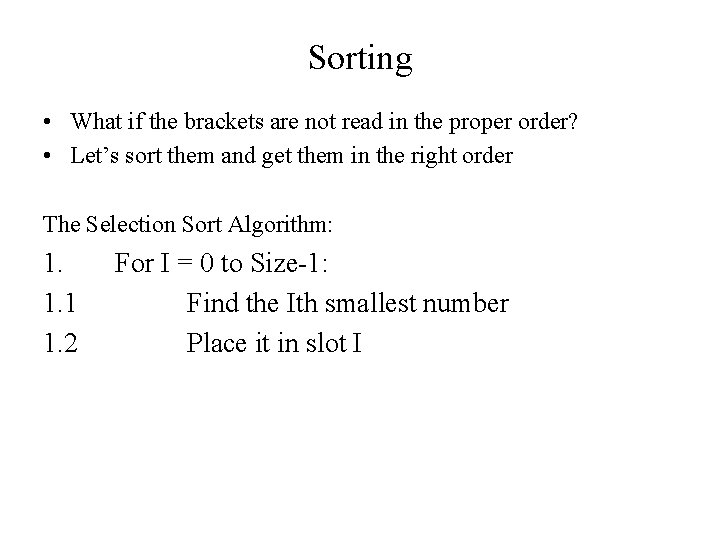
Sorting • What if the brackets are not read in the proper order? • Let’s sort them and get them in the right order The Selection Sort Algorithm: 1. 1. 1 1. 2 For I = 0 to Size-1: Find the Ith smallest number Place it in slot I
![Selection Sorting include iostream h const int Size 5 void sortint x int Selection Sorting #include <iostream. h> const int Size = 5; void sort(int x[]); int](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-29.jpg)
Selection Sorting #include <iostream. h> const int Size = 5; void sort(int x[]); int main(void) { int i, a[Size]; for (i = 0; i < Size; i++) { cout << "Enter a[" << i << "]t? "; cin >> a[i]; }
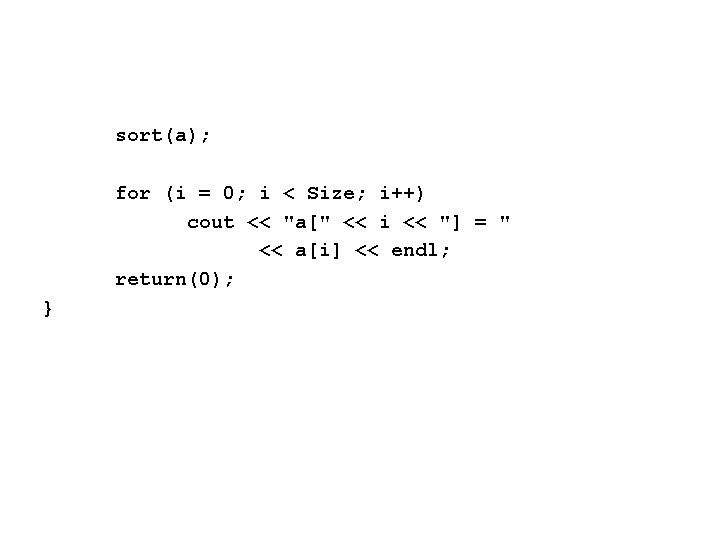
sort(a); for (i = 0; i < Size; i++) cout << "a[" << i << "] = " << a[i] << endl; return(0); }
![sort Sort an array of numbers void sortint x int i // sort() - Sort an array of numbers void sort(int x[]) { int i,](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-31.jpg)
// sort() - Sort an array of numbers void sort(int x[]) { int i, j, small, index, temp; // Place the smallest number in the first // position // Place the second smallest in the second // position and so on. for (i = 0; i < Size -1; i++) { small = 32767; index = -1; // Compare each number that is not in // its proper place to the smallest so // far
![for j i j Size j if xj small small for (j = i; j < Size; j++) if (x[j] <small) { small =](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-32.jpg)
for (j = i; j < Size; j++) if (x[j] <small) { small = x[j]; index = j; } // Swap the ith smallest number into its // proper place temp = x[i]; x[i] = x[index]; x[index] = temp; } }
![Multidimensional Arrays You can declare a twodimensional array by writing int xNum RowsNum Multidimensional Arrays • You can declare a two-dimensional array by writing: int x[Num. Rows][Num.](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-33.jpg)
Multidimensional Arrays • You can declare a two-dimensional array by writing: int x[Num. Rows][Num. Columns]; • E. g. , int x[3][6] x[0][0] x[0][1] x[0][2] x[0][3] x[0][4] x[0][5] x[1][0] x[1][1] x[1][2] x[1][3] x[1][4] x[1][5] x[2][0] x[2][1] x[2][2] x[2][3] x[2][4] x[2][5]
![Implementing Multidimensional Arrays x00 x01 x02 x03 x04 x05 x10 x11 x12 x13 x14 Implementing Multidimensional Arrays x[0][0] x[0][1] x[0][2] x[0][3] x[0][4] x[0][5] x[1][0] x[1][1] x[1][2] x[1][3] x[1][4]](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-34.jpg)
Implementing Multidimensional Arrays x[0][0] x[0][1] x[0][2] x[0][3] x[0][4] x[0][5] x[1][0] x[1][1] x[1][2] x[1][3] x[1][4] x[1][5] x[2][0] Two-dimensional arrays are stored row-major, which means that the entire row is stored in consecutive memory addresses, with subsequent rows following it.
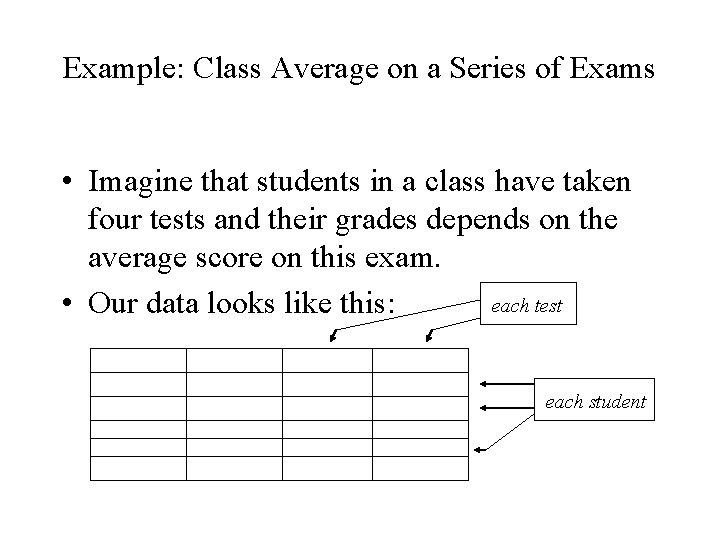
Example: Class Average on a Series of Exams • Imagine that students in a class have taken four tests and their grades depends on the average score on this exam. each test • Our data looks like this: each student
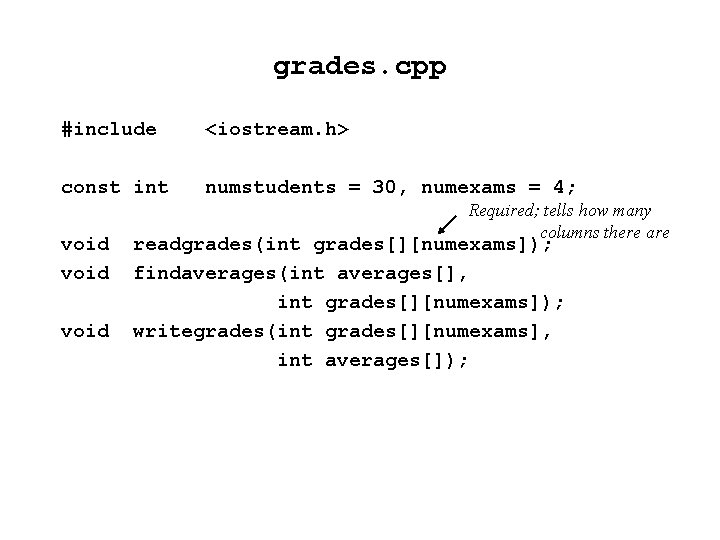
grades. cpp #include <iostream. h> const int numstudents = 30, numexams = 4; void Required; tells how many columns there are readgrades(int grades[][numexams]); findaverages(int averages[], int grades[][numexams]); writegrades(int grades[][numexams], int averages[]);
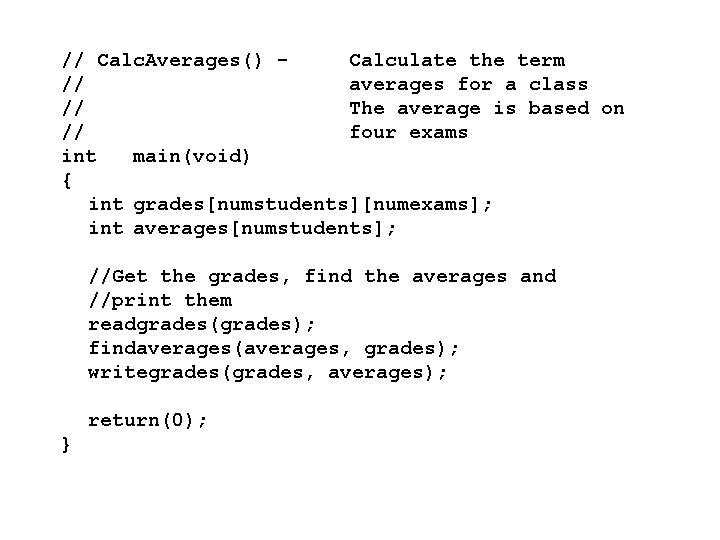
// Calc. Averages() Calculate the term // averages for a class // The average is based on // four exams int main(void) { int grades[numstudents][numexams]; int averages[numstudents]; //Get the grades, find the averages and //print them readgrades(grades); findaverages(averages, grades); writegrades(grades, averages); return(0); }
![readgrades Read the complete set of grades void readgradesint gradesnumexams int // readgrades() - Read the complete set of grades void readgrades(int grades[][numexams]) { int](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-38.jpg)
// readgrades() - Read the complete set of grades void readgrades(int grades[][numexams]) { int i, j; // Get each students grade for (i = 0; i < numstudents; i++) { //Get the next grade for this student for (j = 0; j < numexams; j++) { cout << "Grade on test #" << j << " for student # " << i << "t? "; cin >> grades[i][j]; } //Skip one line for clarity cout << 'n'; } }
![Find Averages Find the average for each student void findaveragesint averages int // Find. Averages() Find the average for each // student void findaverages(int averages[], int](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-39.jpg)
// Find. Averages() Find the average for each // student void findaverages(int averages[], int grades[][numexams]) { int i, j, sum; for (i = 0; i < numstudents; i++) sum = 0; for (j = 0; j < numexams; j++) sum += grades[i][j]; averages[i] = sum/numexams; } } {
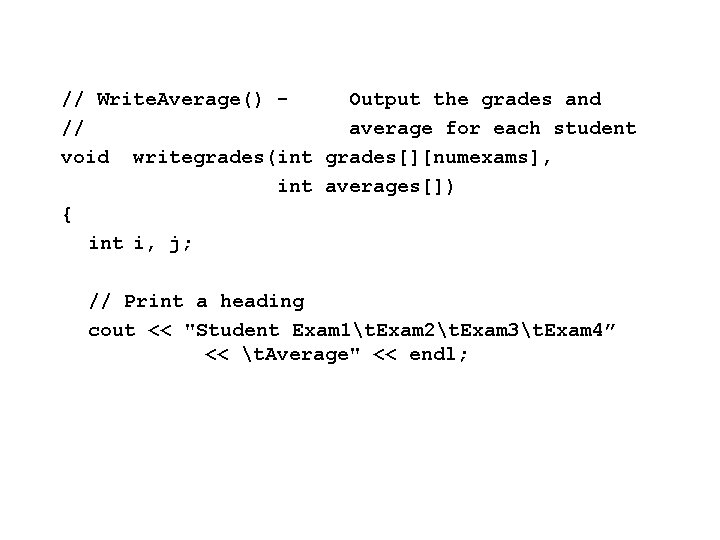
// Write. Average() Output the grades and // average for each student void writegrades(int grades[][numexams], int averages[]) { int i, j; // Print a heading cout << "Student Exam 1t. Exam 2t. Exam 3t. Exam 4” << t. Average" << endl;
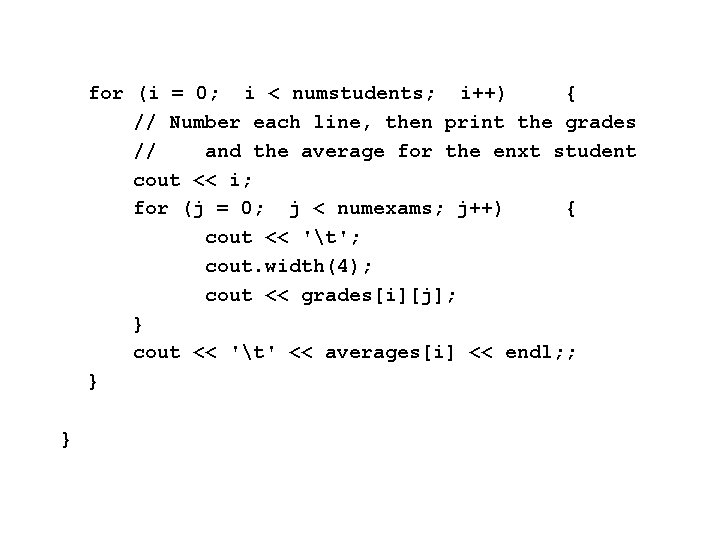
for (i = 0; i < numstudents; i++) { // Number each line, then print the grades // and the average for the enxt student cout << i; for (j = 0; j < numexams; j++) { cout << 't'; cout. width(4); cout << grades[i][j]; } cout << 't' << averages[i] << endl; ; } }
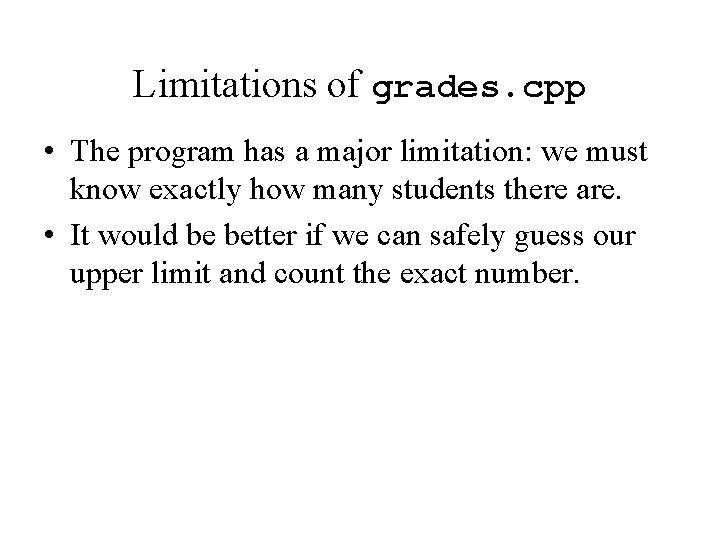
Limitations of grades. cpp • The program has a major limitation: we must know exactly how many students there are. • It would be better if we can safely guess our upper limit and count the exact number.
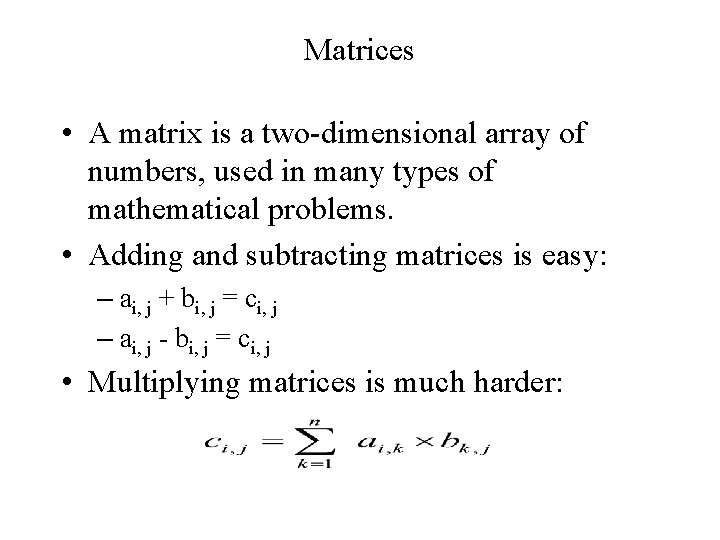
Matrices • A matrix is a two-dimensional array of numbers, used in many types of mathematical problems. • Adding and subtracting matrices is easy: – ai, j + bi, j = ci, j – ai, j - bi, j = ci, j • Multiplying matrices is much harder:
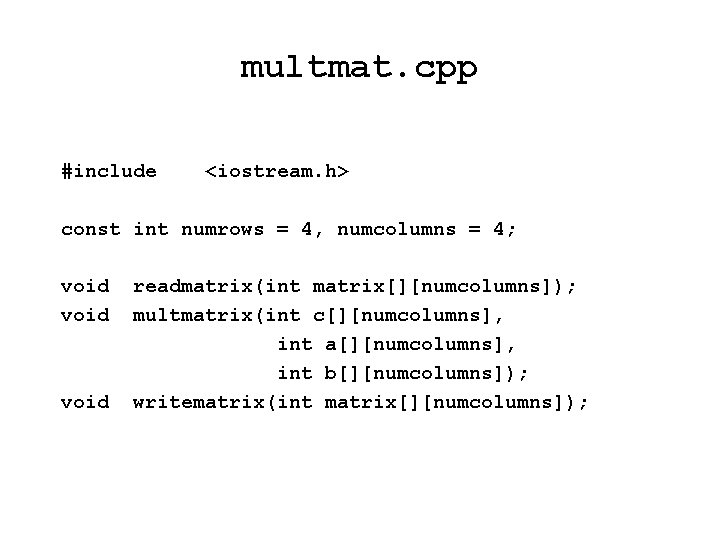
multmat. cpp #include <iostream. h> const int numrows = 4, numcolumns = 4; void readmatrix(int matrix[][numcolumns]); multmatrix(int c[][numcolumns], int a[][numcolumns], int b[][numcolumns]); writematrix(int matrix[][numcolumns]);
![multmat Read and multiply two matrices int mainvoid int anumrowsnumcolumns bnumrowsnumcolumns // multmat() – Read and multiply two matrices int main(void) { int a[numrows][numcolumns], b[numrows][numcolumns],](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-45.jpg)
// multmat() – Read and multiply two matrices int main(void) { int a[numrows][numcolumns], b[numrows][numcolumns], c[numrows][numcolumns]; cout << "Enter matrix a" << endl; readmatrix(a); cout << "Enter matrix b" << endl; readmatrix(b); multmatrix(c, a, b); cout << "The product is: " << endl; writematrix(c); return(0); }
![readmatrix Read in a matrix void readmatrixint matrixnumcolumns int i j for //readmatrix() - Read in a matrix void readmatrix(int matrix[][numcolumns]) { int i, j; for](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-46.jpg)
//readmatrix() - Read in a matrix void readmatrix(int matrix[][numcolumns]) { int i, j; for (i = 0; i < numrows; i++) { cout << "Enter row #" << i+1 << "t? "; for (j = 0; j < numcolumns; j++) cin >> matrix[i][j]; } }
![multmatrix Multiply a x b to get c void multmatrixint cnumcolumns int // multmatrix() – Multiply a x b to get c void multmatrix(int c[][numcolumns], int](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-47.jpg)
// multmatrix() – Multiply a x b to get c void multmatrix(int c[][numcolumns], int a[][numcolumns], int b[][numcolumns]) { int i, j, k; for (i = 0; i < numrows; i++) for (j = 0; j < numcolumns; j++) { c[i][j] = 0; for (k = 0; k < numrows; k++) c[i][j] += a[i][k]*b[k][j]; } }
![writematrix Write an i x j matrix void writematrixint matrixnumcolumns int // writematrix() – Write an i x j matrix void writematrix(int matrix[][numcolumns]) { int](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-48.jpg)
// writematrix() – Write an i x j matrix void writematrix(int matrix[][numcolumns]) { int i, j; for (i = 0; i < numrows; i++) { for (j = 0; j < numcolumns; j++) cout << 't' << matrix[i][j]; cout << 'n'; } }
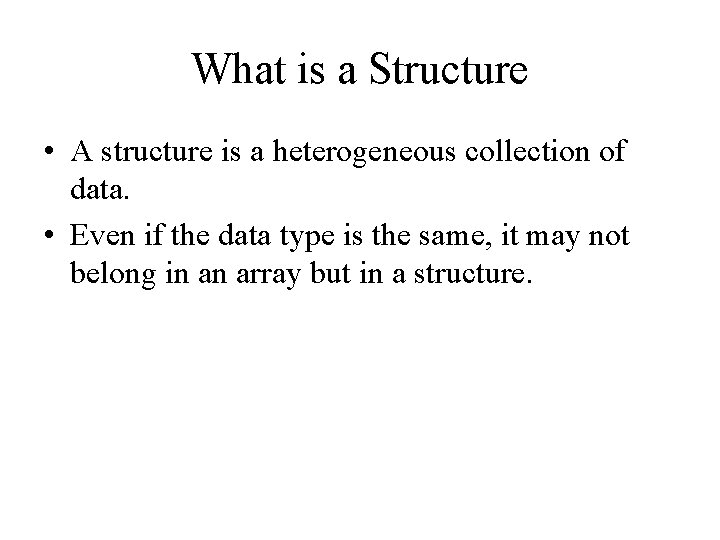
What is a Structure • A structure is a heterogeneous collection of data. • Even if the data type is the same, it may not belong in an array but in a structure.
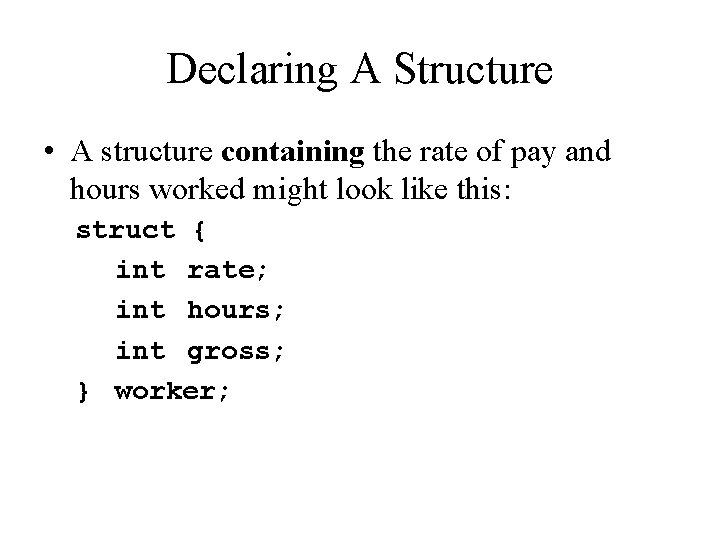
Declaring A Structure • A structure containing the rate of pay and hours worked might look like this: struct { int rate; int hours; int gross; } worker;
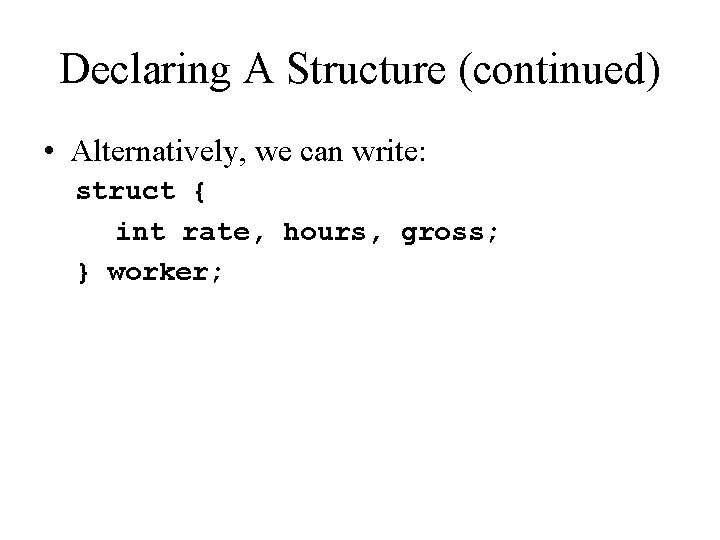
Declaring A Structure (continued) • Alternatively, we can write: struct { int rate, hours, gross; } worker;
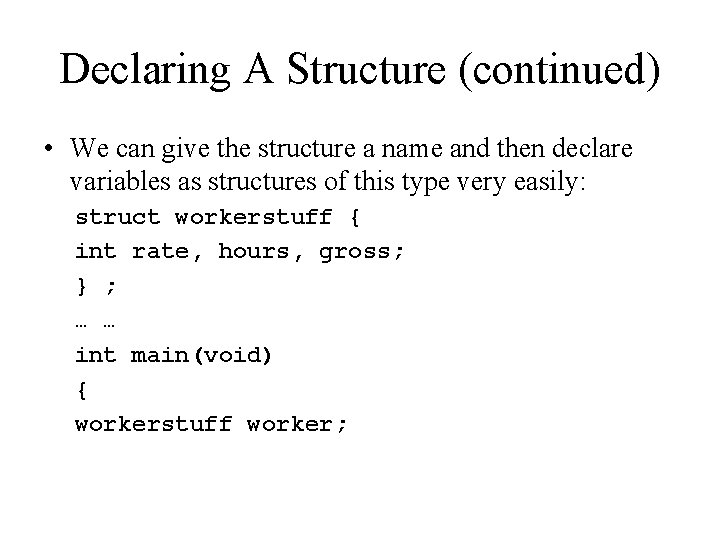
Declaring A Structure (continued) • We can give the structure a name and then declare variables as structures of this type very easily: struct workerstuff { int rate, hours, gross; } ; … … int main(void) { workerstuff worker;
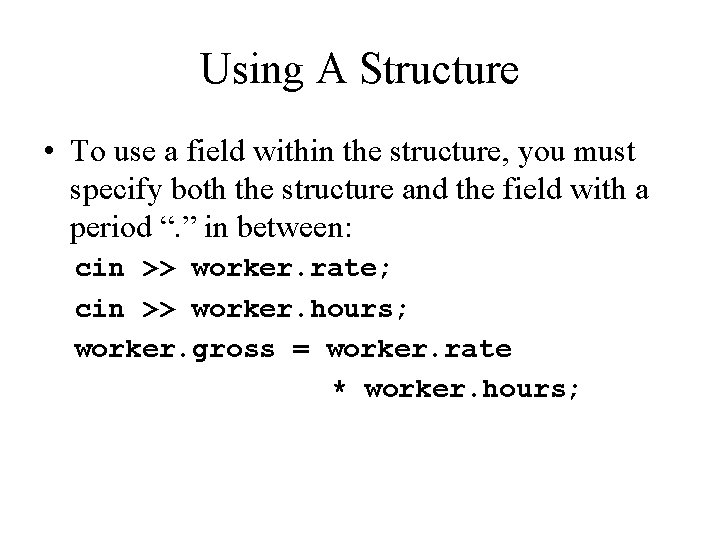
Using A Structure • To use a field within the structure, you must specify both the structure and the field with a period “. ” in between: cin >> worker. rate; cin >> worker. hours; worker. gross = worker. rate * worker. hours;
![A simple payroll program include iostream h struct workerstuff char name20 float rate A simple payroll program #include <iostream. h> struct workerstuff { char name[20]; float rate;](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-54.jpg)
A simple payroll program #include <iostream. h> struct workerstuff { char name[20]; float rate; float hours; float gross; };
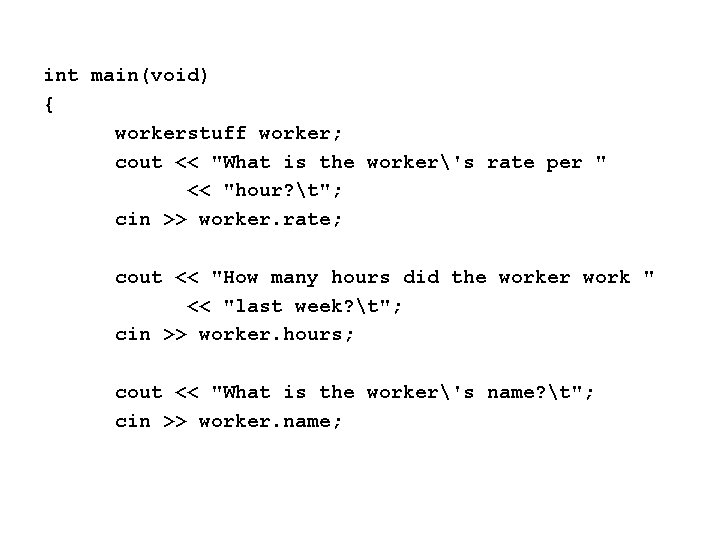
int main(void) { workerstuff worker; cout << "What is the worker's rate per " << "hour? t"; cin >> worker. rate; cout << "How many hours did the worker work " << "last week? t"; cin >> worker. hours; cout << "What is the worker's name? t"; cin >> worker. name;
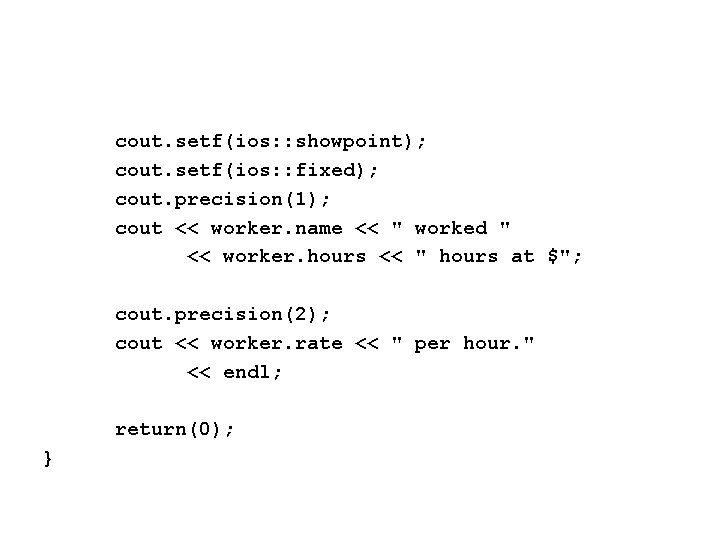
cout. setf(ios: : showpoint); cout. setf(ios: : fixed); cout. precision(1); cout << worker. name << " worked " << worker. hours << " hours at $"; cout. precision(2); cout << worker. rate << " per hour. " << endl; return(0); }
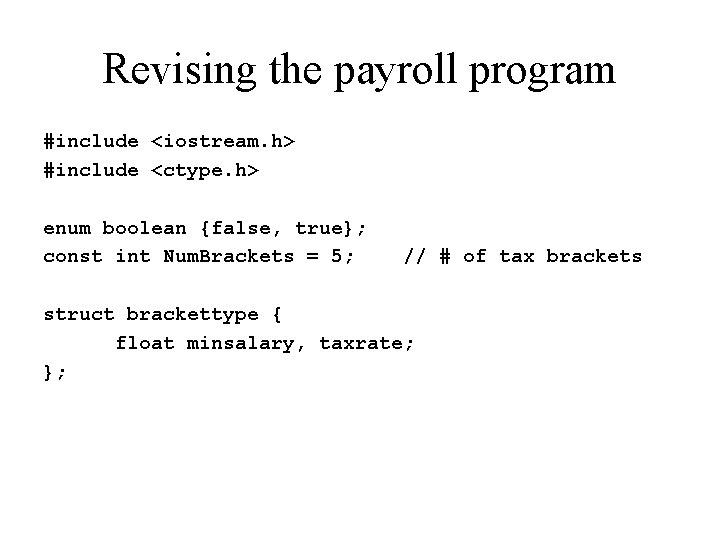
Revising the payroll program #include <iostream. h> #include <ctype. h> enum boolean {false, true}; const int Num. Brackets = 5; // # of tax brackets struct brackettype { float minsalary, taxrate; };
![The prototypes void getbracketsbrackettype brackets void getinputfloat hours float rate float getgrossfloat hours // The prototypes void getbrackets(brackettype brackets[]); void getinput(float &hours, float &rate); float getgross(float hours,](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-58.jpg)
// The prototypes void getbrackets(brackettype brackets[]); void getinput(float &hours, float &rate); float getgross(float hours, float rate); float gettax(float gross, brackettype brackets[]); void writecheck(float gross, float tax, float net, float rate, float hours); boolean calcagain(void);
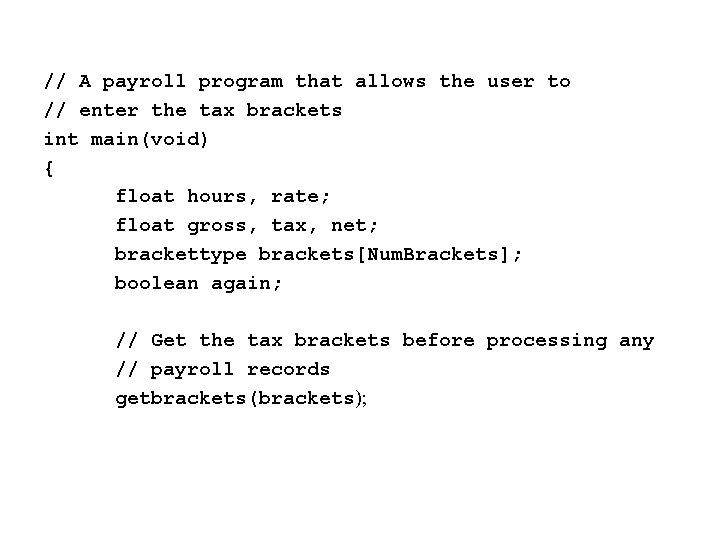
// A payroll program that allows the user to // enter the tax brackets int main(void) { float hours, rate; float gross, tax, net; brackettype brackets[Num. Brackets]; boolean again; // Get the tax brackets before processing any // payroll records getbrackets(brackets);
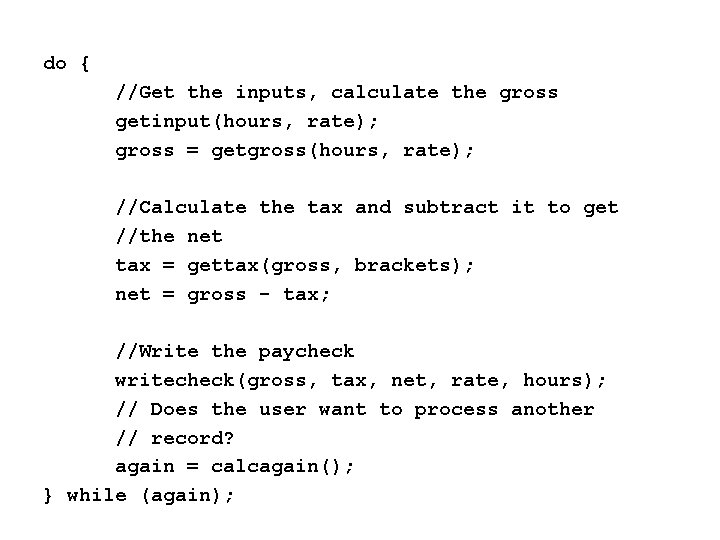
do { //Get the inputs, calculate the gross getinput(hours, rate); gross = getgross(hours, rate); //Calculate the tax and subtract it to get //the net tax = gettax(gross, brackets); net = gross - tax; //Write the paycheck writecheck(gross, tax, net, rate, hours); // Does the user want to process another // record? again = calcagain(); } while (again);
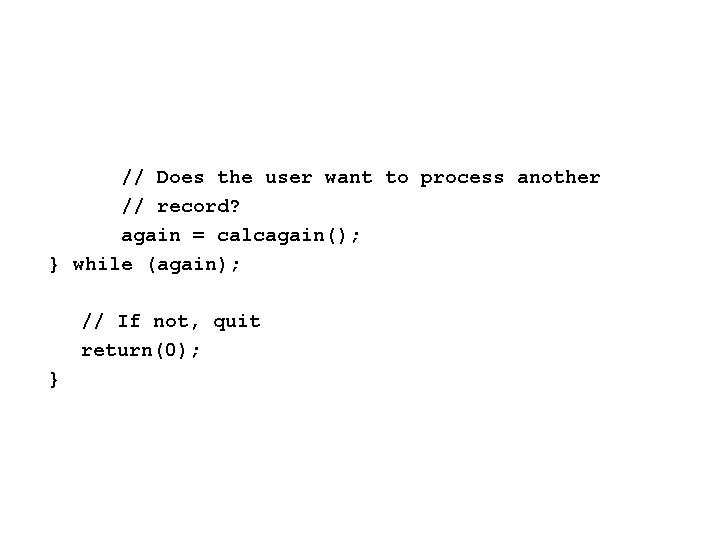
// Does the user want to process another // record? again = calcagain(); } while (again); // If not, quit return(0); }
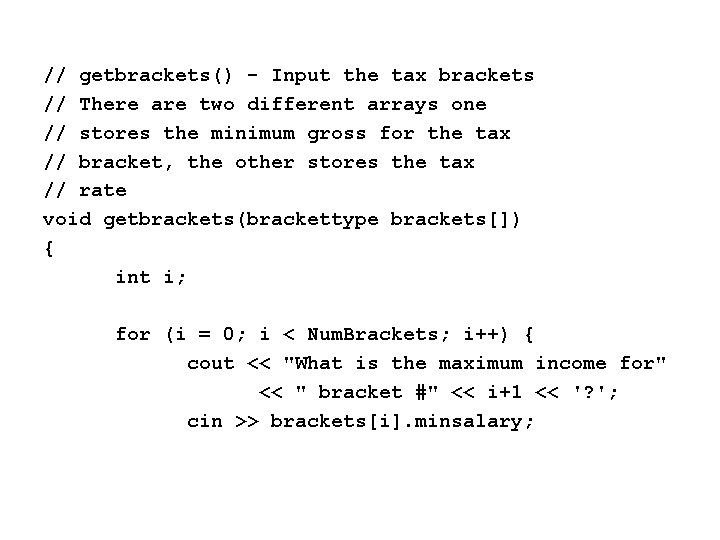
// getbrackets() - Input the tax brackets // There are two different arrays one // stores the minimum gross for the tax // bracket, the other stores the tax // rate void getbrackets(brackettype brackets[]) { int i; for (i = 0; i < Num. Brackets; i++) { cout << "What is the maximum income for" << " bracket #" << i+1 << '? '; cin >> brackets[i]. minsalary;
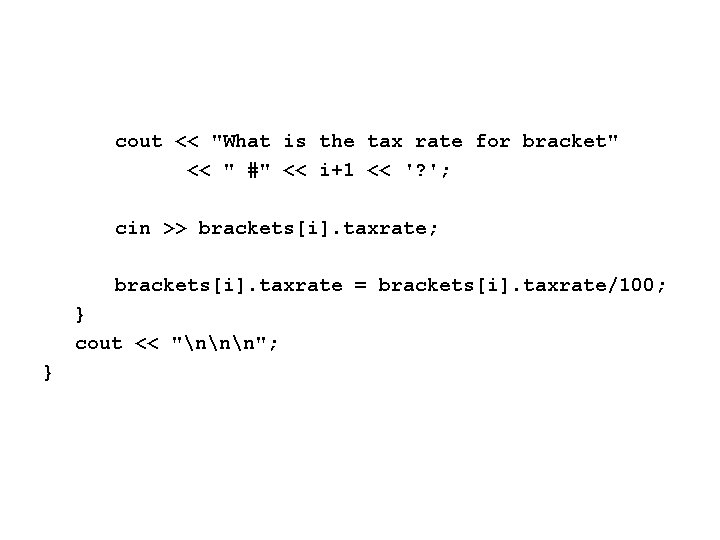
cout << "What is the tax rate for bracket" << " #" << i+1 << '? '; cin >> brackets[i]. taxrate; brackets[i]. taxrate = brackets[i]. taxrate/100; } cout << "nnn"; }
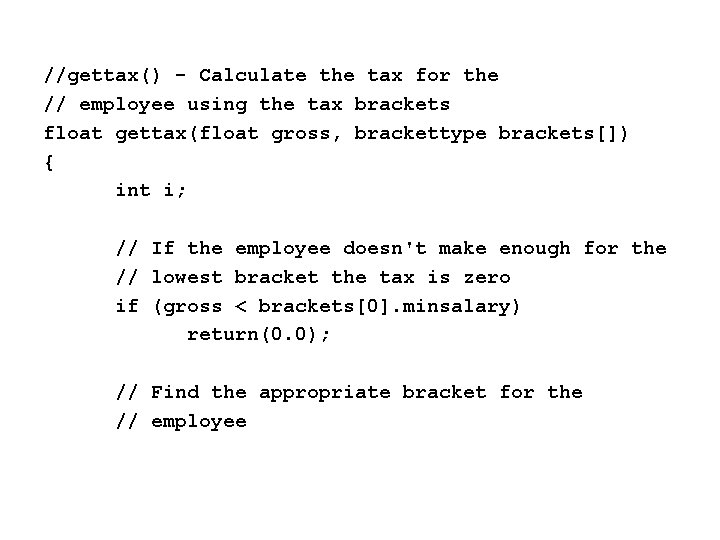
//gettax() - Calculate the tax for the // employee using the tax brackets float gettax(float gross, brackettype brackets[]) { int i; // If the employee doesn't make enough for the // lowest bracket the tax is zero if (gross < brackets[0]. minsalary) return(0. 0); // Find the appropriate bracket for the // employee
![for i 1 i Num Brackets i if gross bracketsi for (i = 1; i < Num. Brackets; i++) { if (gross < brackets[i].](https://slidetodoc.com/presentation_image_h2/90c448eb61e6fed9025599794aff9aba/image-65.jpg)
for (i = 1; i < Num. Brackets; i++) { if (gross < brackets[i]. minsalary) return(brackets[i-1]. taxrate*gross); } //The employee is in the highest bracket return(brackets[Num. Brackets-1]. taxrate*gross); }
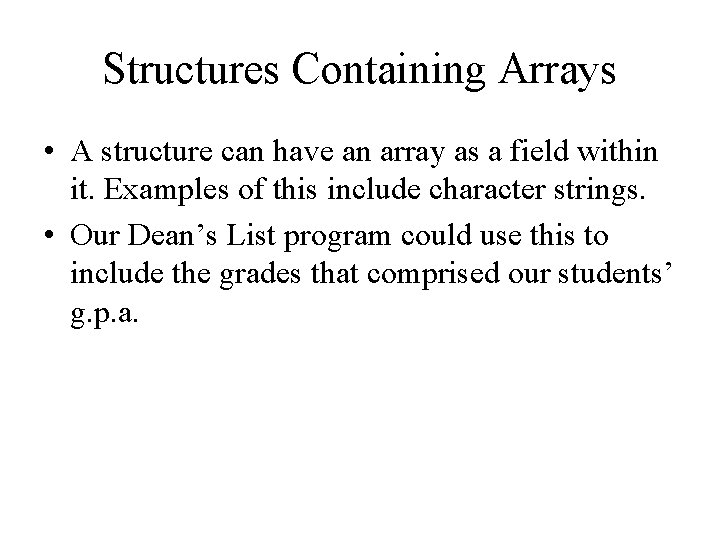
Structures Containing Arrays • A structure can have an array as a field within it. Examples of this include character strings. • Our Dean’s List program could use this to include the grades that comprised our students’ g. p. a.
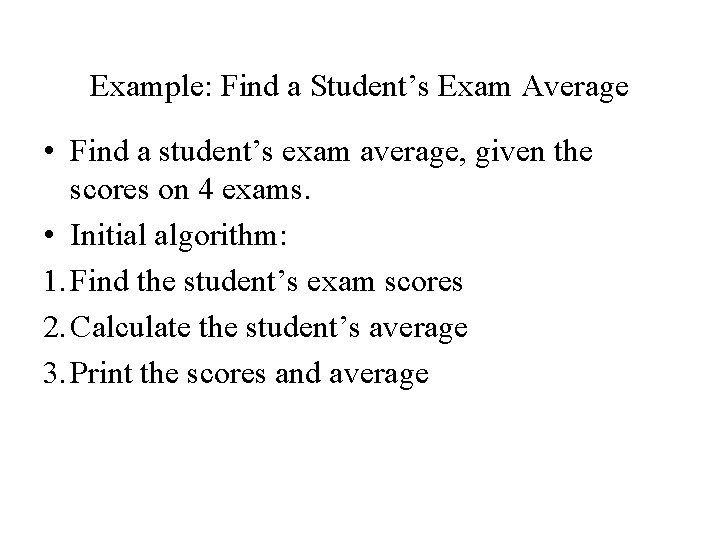
Example: Find a Student’s Exam Average • Find a student’s exam average, given the scores on 4 exams. • Initial algorithm: 1. Find the student’s exam scores 2. Calculate the student’s average 3. Print the scores and average
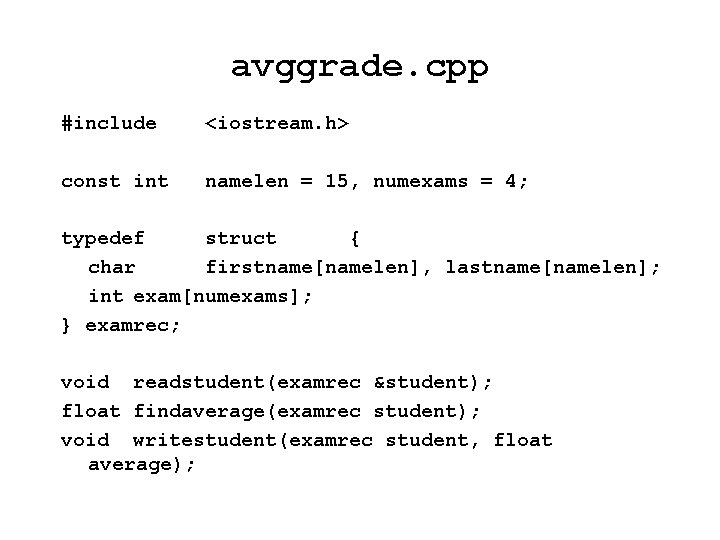
avggrade. cpp #include <iostream. h> const int namelen = 15, numexams = 4; typedef struct { char firstname[namelen], lastname[namelen]; int exam[numexams]; } examrec; void readstudent(examrec &student); float findaverage(examrec student); void writestudent(examrec student, float average);
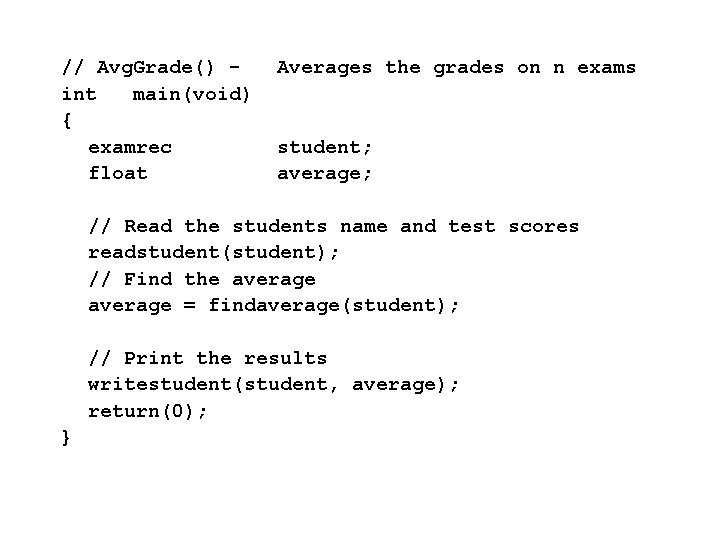
// Avg. Grade() int main(void) { examrec float Averages the grades on n exams student; average; // Read the students name and test scores readstudent(student); // Find the average = findaverage(student); // Print the results writestudent(student, average); return(0); }
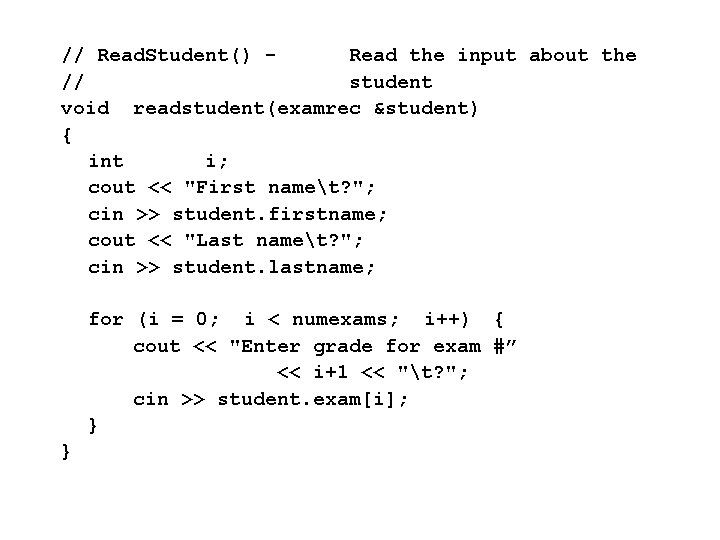
// Read. Student() Read the input about the // student void readstudent(examrec &student) { int i; cout << "First namet? "; cin >> student. firstname; cout << "Last namet? "; cin >> student. lastname; for (i = 0; i < numexams; i++) { cout << "Enter grade for exam #” << i+1 << "t? "; cin >> student. exam[i]; } }
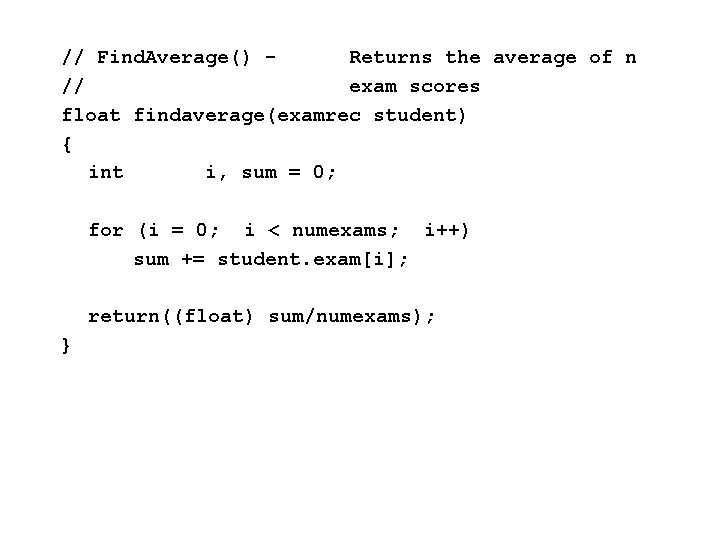
// Find. Average() Returns the average of n // exam scores float findaverage(examrec student) { int i, sum = 0; for (i = 0; i < numexams; i++) sum += student. exam[i]; return((float) sum/numexams); }
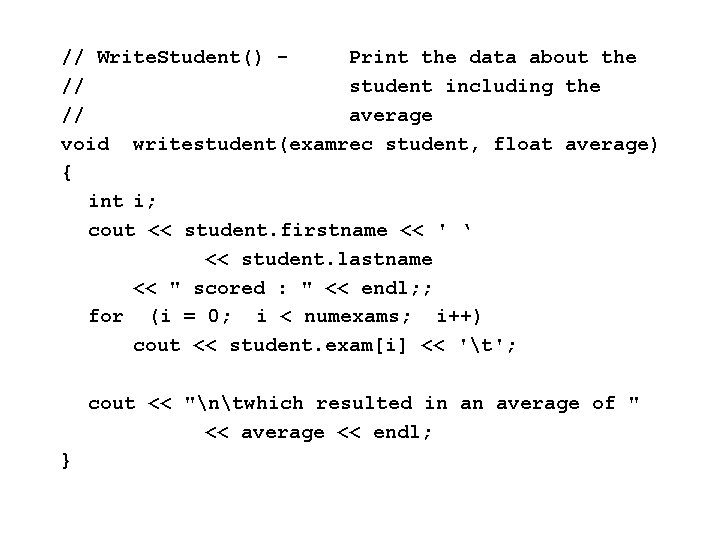
// Write. Student() Print the data about the // student including the // average void writestudent(examrec student, float average) { int i; cout << student. firstname << ' ‘ << student. lastname << " scored : " << endl; ; for (i = 0; i < numexams; i++) cout << student. exam[i] << 't'; cout << "ntwhich resulted in an average of " << average << endl; }