CSC 141 Computer Science I Zhen Jiang Dept
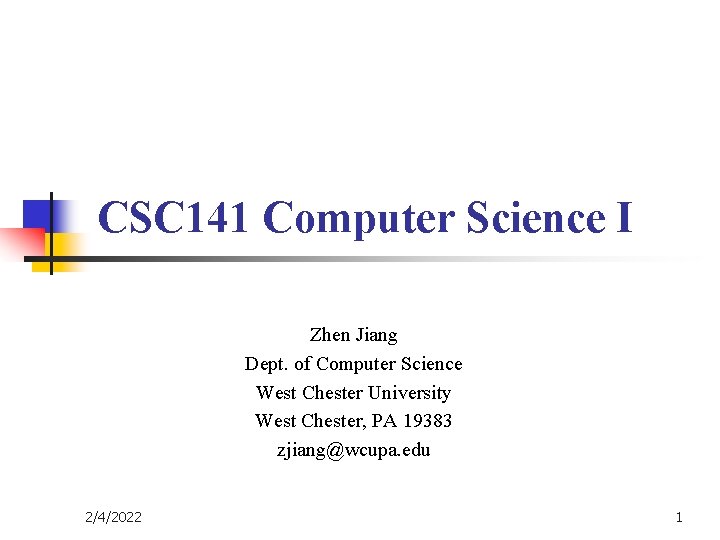
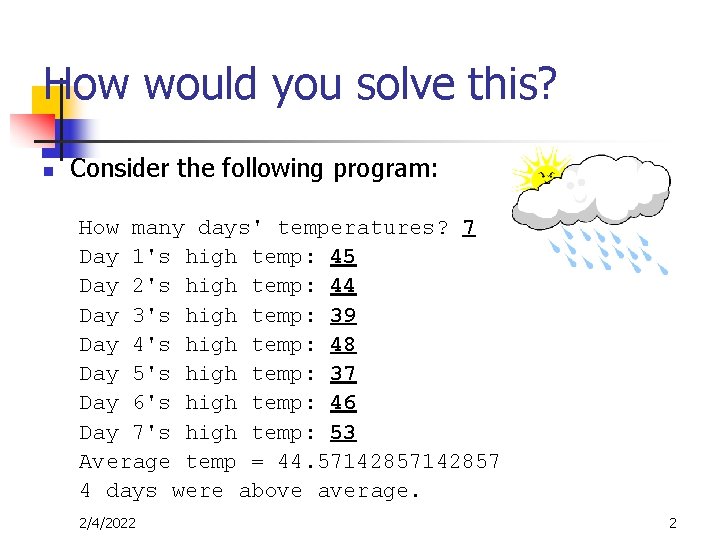
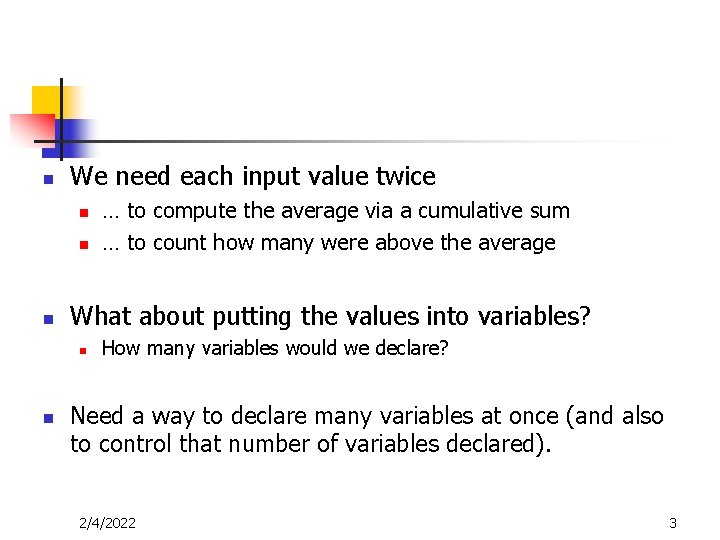
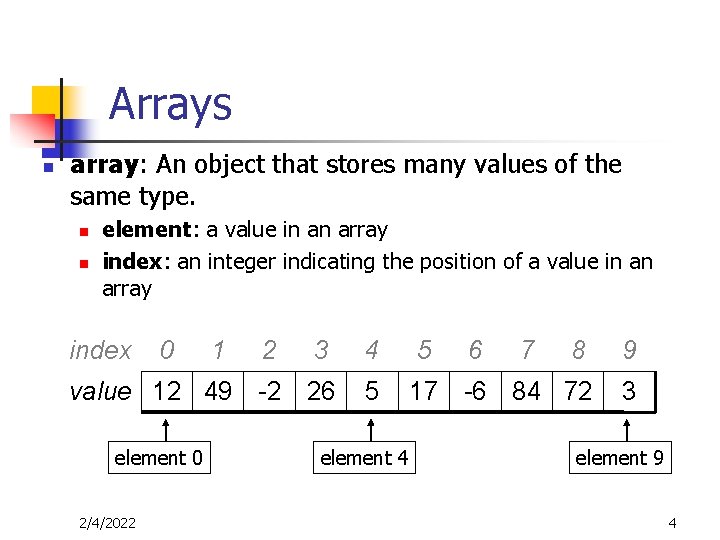
![n n Declaring/initializing an array: <type>[] <name> = new <type>[<length>]; Example: int[] numbers = n n Declaring/initializing an array: <type>[] <name> = new <type>[<length>]; Example: int[] numbers =](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-5.jpg)
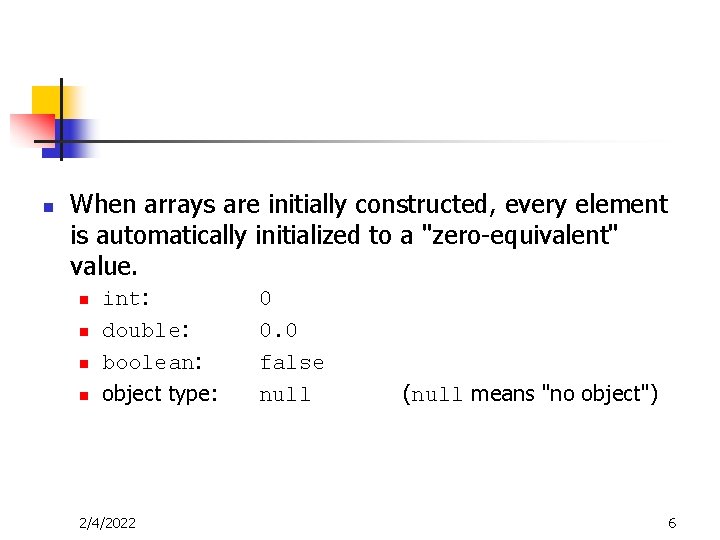
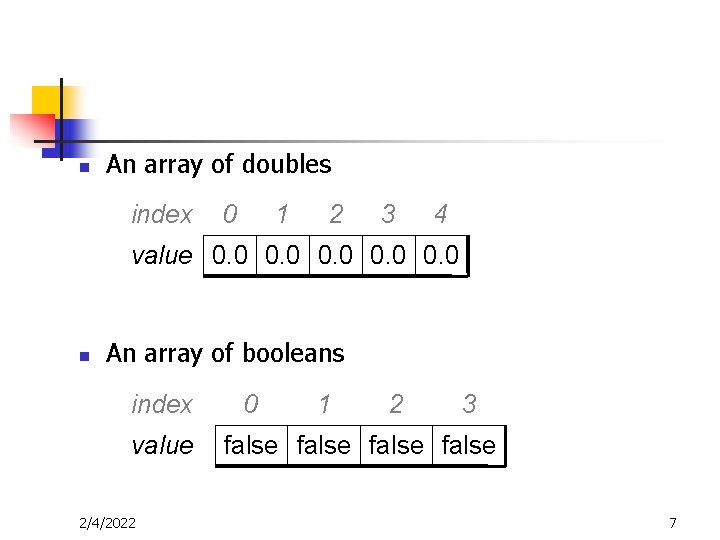
![n Assigning a value to an array element: <array name>[<index>] = <value>; n Example: n Assigning a value to an array element: <array name>[<index>] = <value>; n Example:](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-8.jpg)
![n Using an array element's value in an expression: <array name>[<index>] n Example: System. n Using an array element's value in an expression: <array name>[<index>] n Example: System.](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-9.jpg)
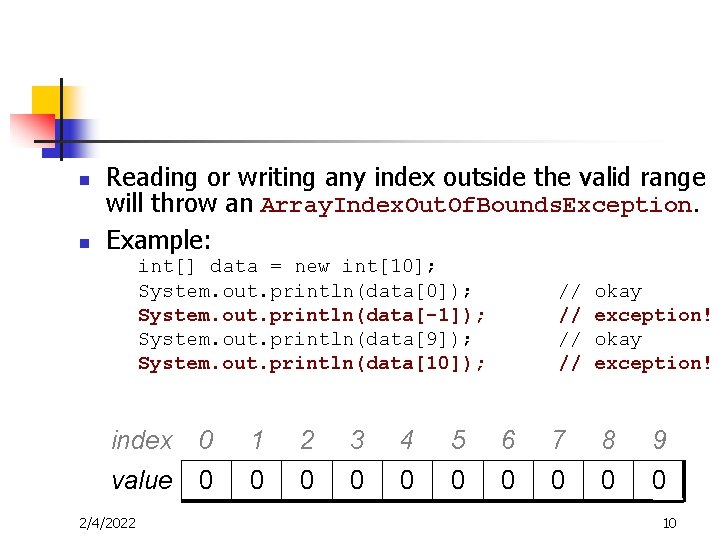
![int[] numbers = new int[8]; numbers[1] = 4; numbers[4] = 99; numbers[7] = 2; int[] numbers = new int[8]; numbers[1] = 4; numbers[4] = 99; numbers[7] = 2;](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-11.jpg)
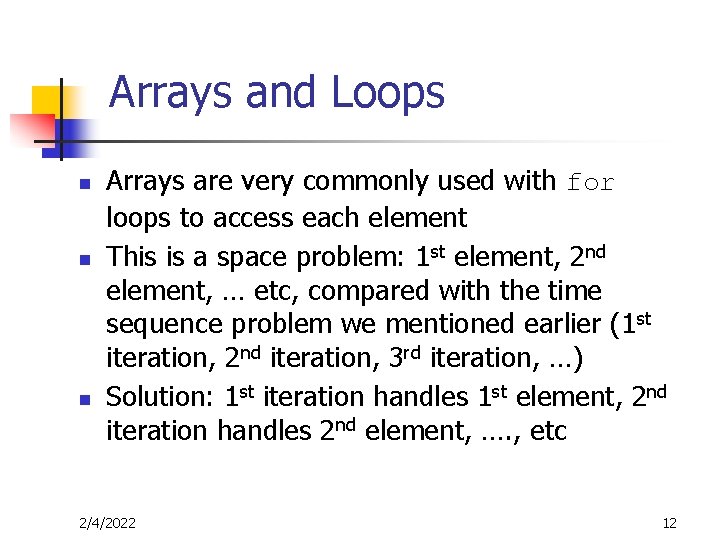
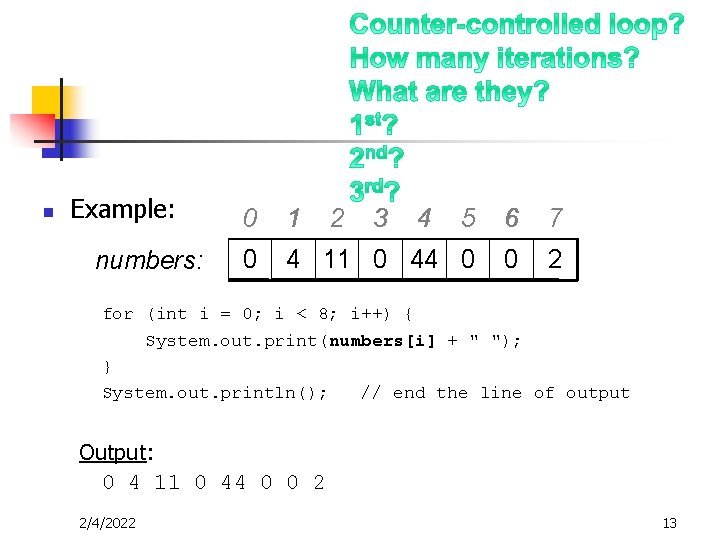
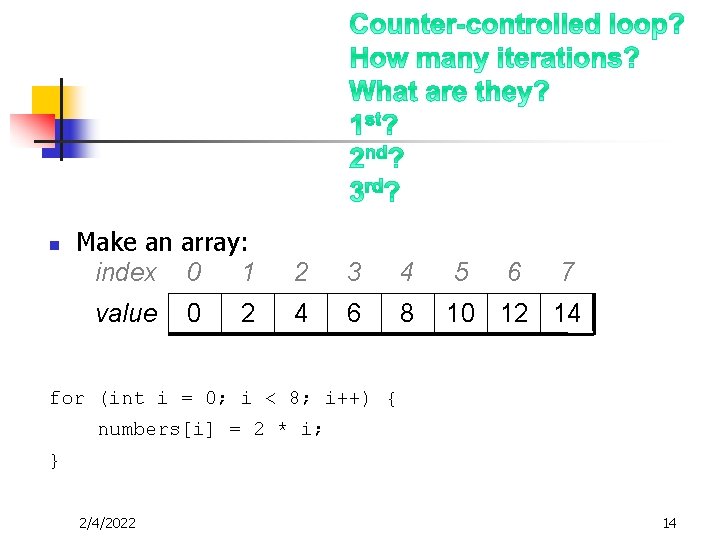
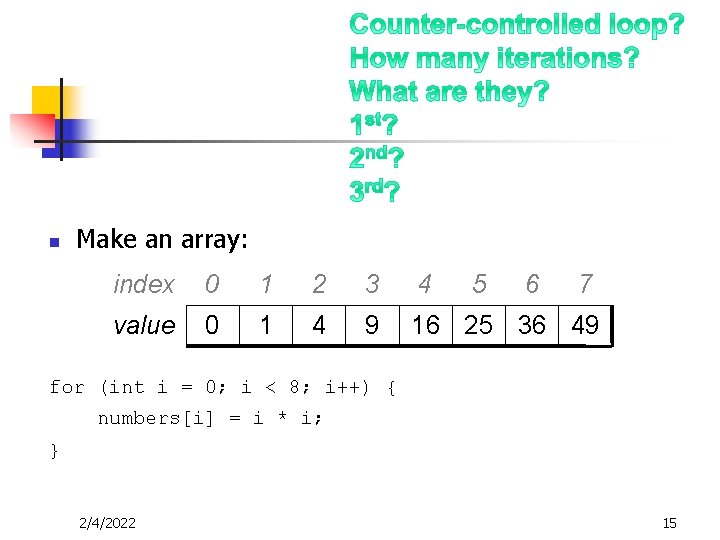
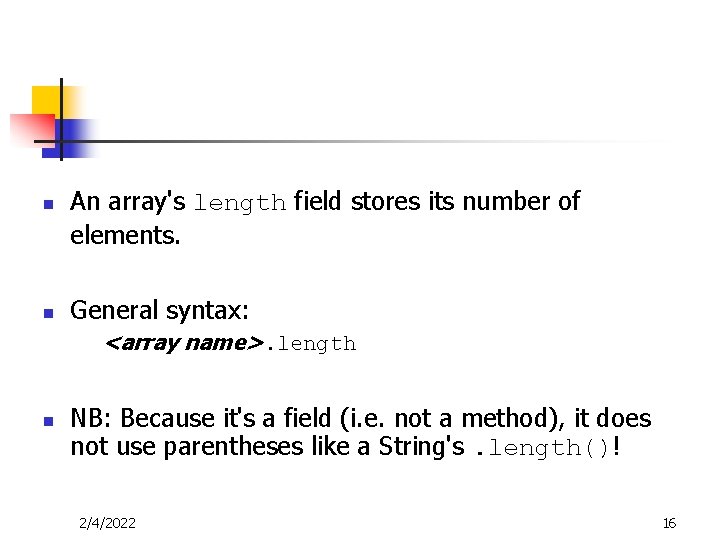
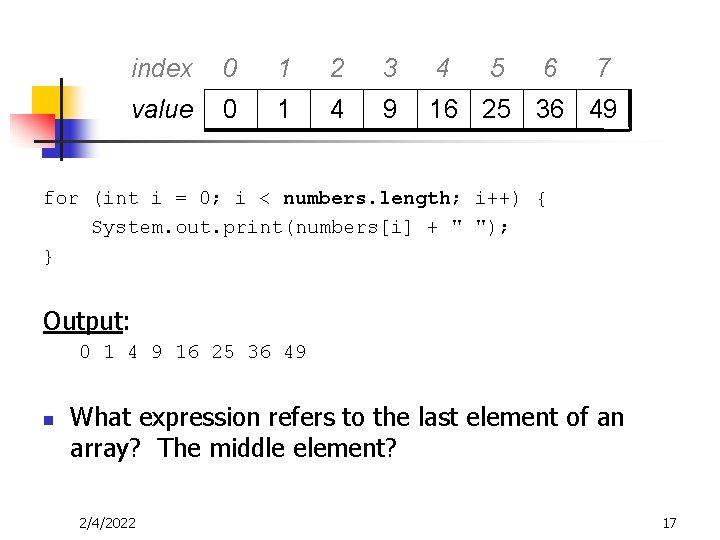
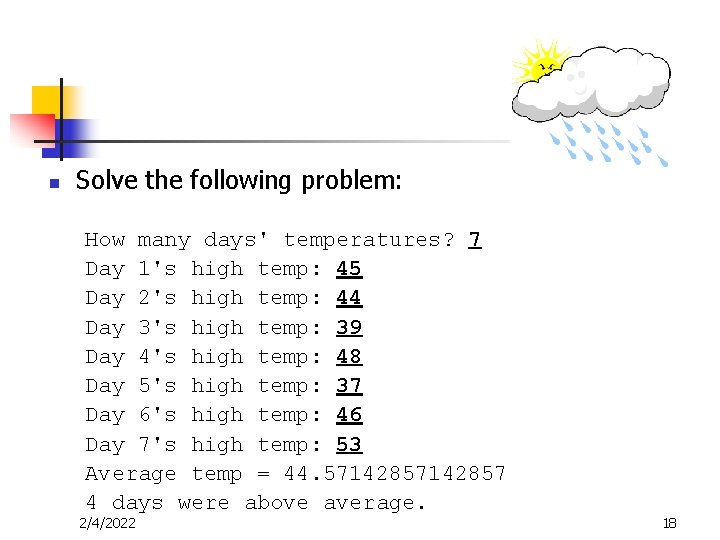
![import java. util. *; public class Weather { public static void main(String[] args) { import java. util. *; public class Weather { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-19.jpg)
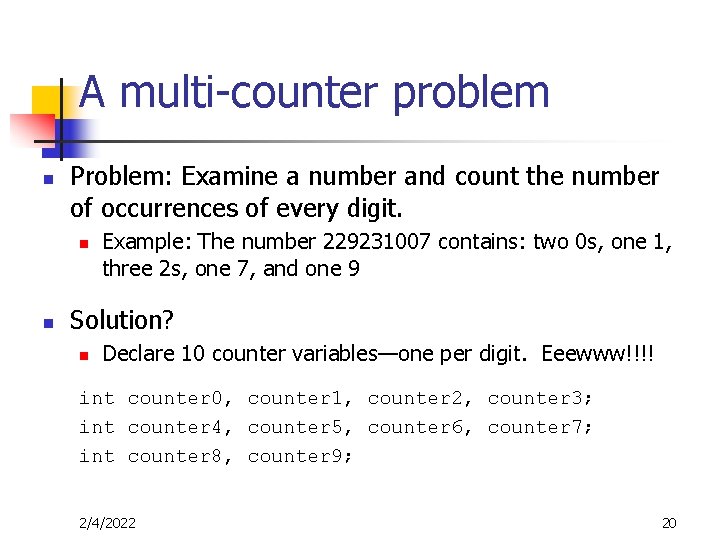
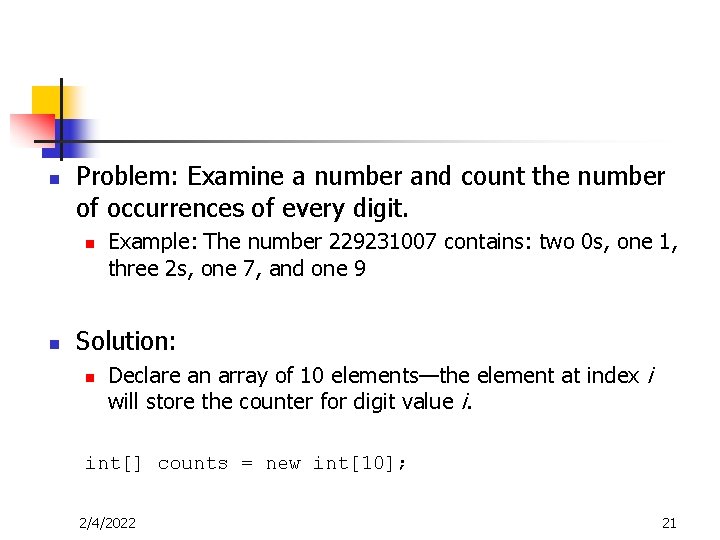
![int num = 229231007; //ensure num > 0 int[] counts = new int[10]; while int num = 229231007; //ensure num > 0 int[] counts = new int[10]; while](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-22.jpg)
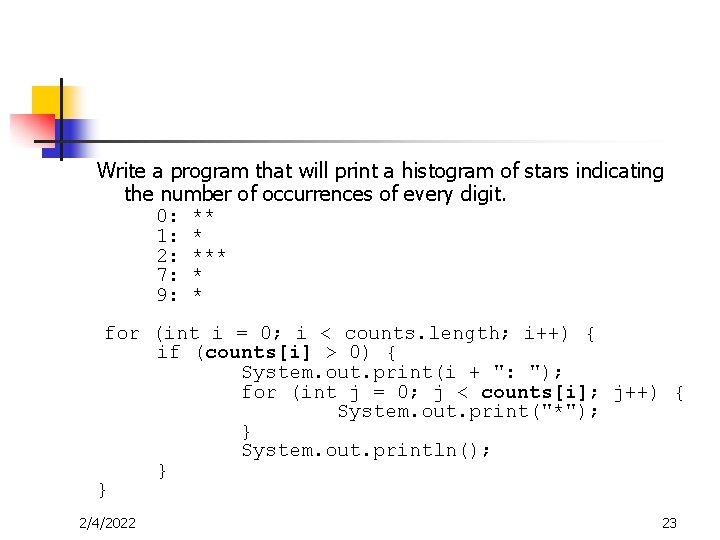
![n Quick array initialization, general syntax: <type>[] <name> = {<value>, . . . , n Quick array initialization, general syntax: <type>[] <name> = {<value>, . . . ,](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-24.jpg)
![int[] a = { 2, 5, 1, 6, 14, 7, 9 }; for (int int[] a = { 2, 5, 1, 6, 14, 7, 9 }; for (int](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-25.jpg)
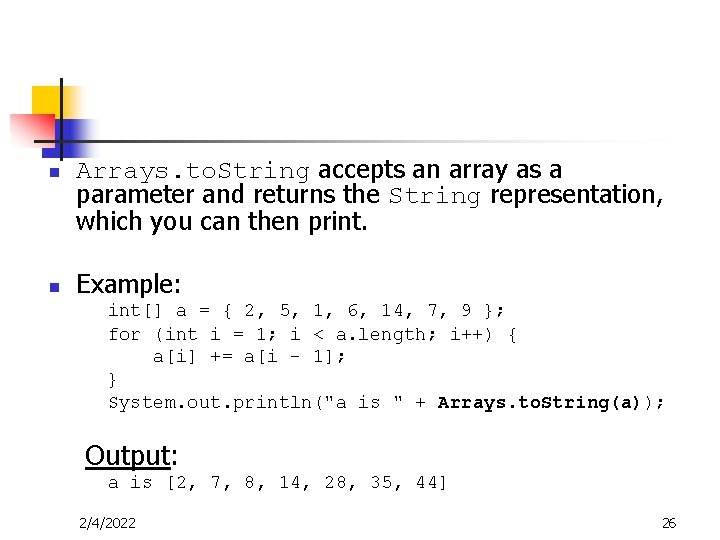
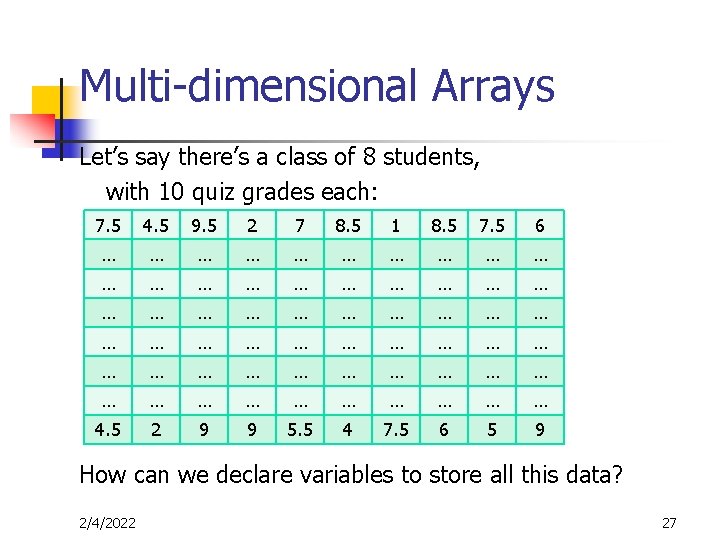
![double [] student 1 = new double[10]; . . . double [] student 8 double [] student 1 = new double[10]; . . . double [] student 8](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-28.jpg)
![double [] quiz 1 = new double[8]; . . . double [] quiz 10 double [] quiz 1 = new double[8]; . . . double [] quiz 10](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-29.jpg)
![double [][] quiz. Scores = new double[8][10]; quiz. Scores[0][6] quiz. Scores: 7. 5 4. double [][] quiz. Scores = new double[8][10]; quiz. Scores[0][6] quiz. Scores: 7. 5 4.](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-30.jpg)
![n A 2 -D array is really an array of arrays! double [][] quiz. n A 2 -D array is really an array of arrays! double [][] quiz.](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-31.jpg)
![double [][] quiz. Scores = new double[4][]; quiz. Scores[0] = new double[3]; quiz. Scores[2] double [][] quiz. Scores = new double[4][]; quiz. Scores[0] = new double[3]; quiz. Scores[2]](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-32.jpg)
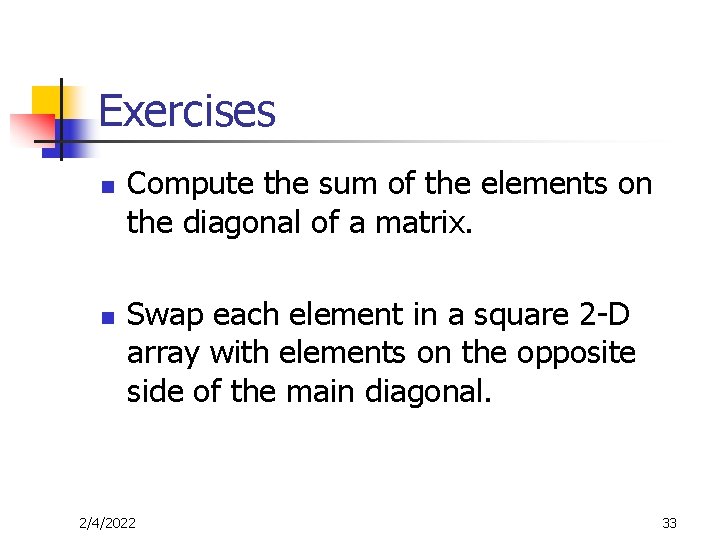
- Slides: 33
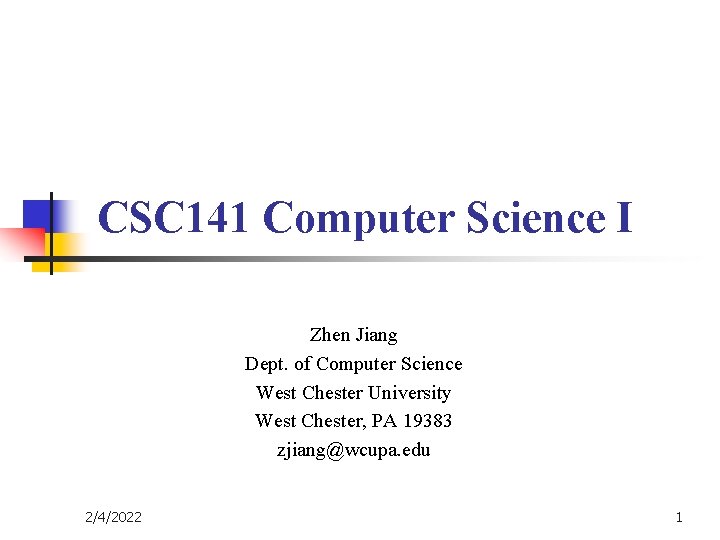
CSC 141 Computer Science I Zhen Jiang Dept. of Computer Science West Chester University West Chester, PA 19383 zjiang@wcupa. edu 2/4/2022 1
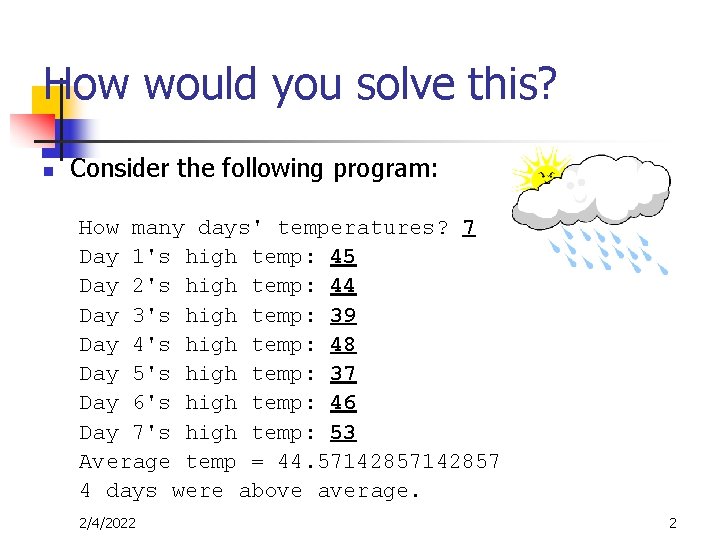
How would you solve this? n Consider the following program: How many days' temperatures? 7 Day 1's high temp: 45 Day 2's high temp: 44 Day 3's high temp: 39 Day 4's high temp: 48 Day 5's high temp: 37 Day 6's high temp: 46 Day 7's high temp: 53 Average temp = 44. 57142857 4 days were above average. 2/4/2022 2
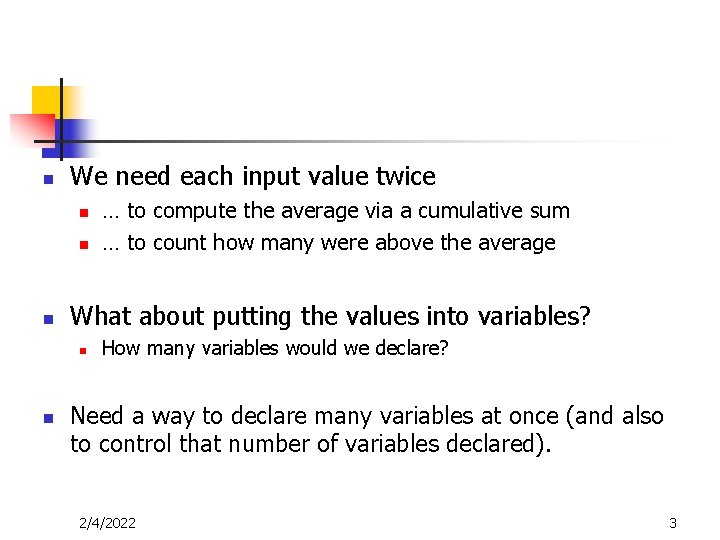
n We need each input value twice n n n What about putting the values into variables? n n … to compute the average via a cumulative sum … to count how many were above the average How many variables would we declare? Need a way to declare many variables at once (and also to control that number of variables declared). 2/4/2022 3
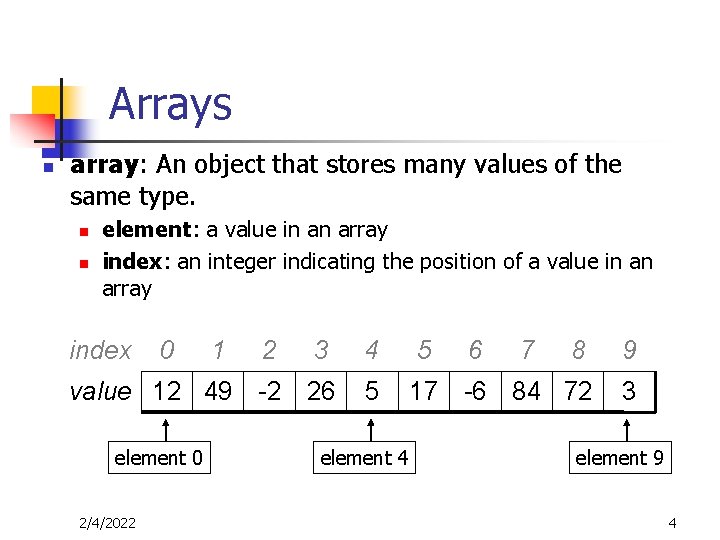
Arrays n array: An object that stores many values of the same type. n n element: a value in an array index: an integer indicating the position of a value in an array index 0 3 4 5 8 9 value 12 49 -2 26 5 17 -6 84 72 3 element 0 2/4/2022 1 2 element 4 6 7 element 9 4
![n n Declaringinitializing an array type name new typelength Example int numbers n n Declaring/initializing an array: <type>[] <name> = new <type>[<length>]; Example: int[] numbers =](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-5.jpg)
n n Declaring/initializing an array: <type>[] <name> = new <type>[<length>]; Example: int[] numbers = new int[10]; index value n 0 0 1 0 2 0 3 0 4 0 5 0 6 0 7 0 8 0 9 0 The length can be any integer expression: int x = 2 * 3 + 1; int[] data = new int[x % 5 + 2]; 2/4/2022 5
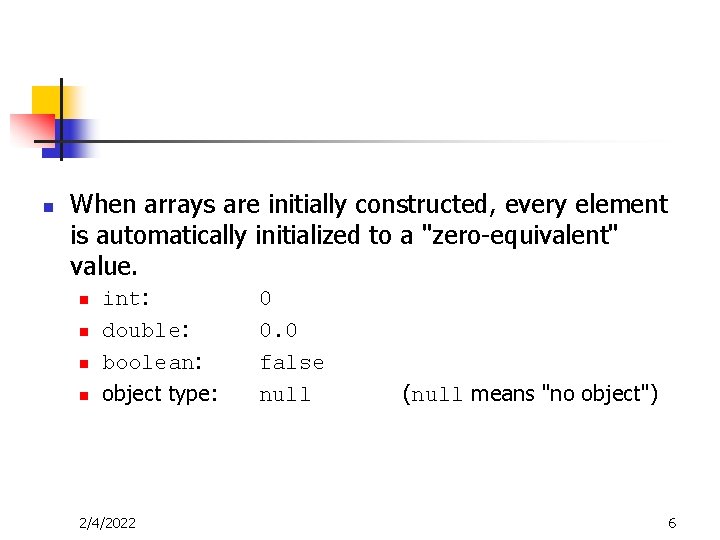
n When arrays are initially constructed, every element is automatically initialized to a "zero-equivalent" value. n n int: double: boolean: object type: 2/4/2022 0 0. 0 false null (null means "no object") 6
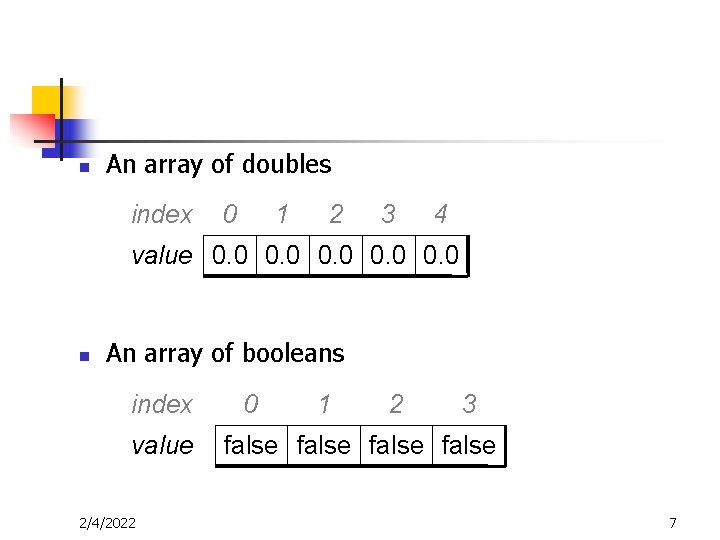
n An array of doubles index 0 1 2 3 4 value 0. 0 0. 0 n An array of booleans index value 2/4/2022 0 1 2 3 false 7
![n Assigning a value to an array element array nameindex value n Example n Assigning a value to an array element: <array name>[<index>] = <value>; n Example:](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-8.jpg)
n Assigning a value to an array element: <array name>[<index>] = <value>; n Example: numbers[0] = 27; numbers[3] = -6; index 0 1 2 3 4 5 6 7 8 9 value 27 0 0 -6 0 0 0 2/4/2022 8
![n Using an array elements value in an expression array nameindex n Example System n Using an array element's value in an expression: <array name>[<index>] n Example: System.](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-9.jpg)
n Using an array element's value in an expression: <array name>[<index>] n Example: System. out. println(numbers[0]); if (numbers[3] < 0) { System. out. println("Element 3 is negative. "); } index 0 1 2 3 4 5 6 7 8 9 value 27 0 0 -6 0 0 0 2/4/2022 9
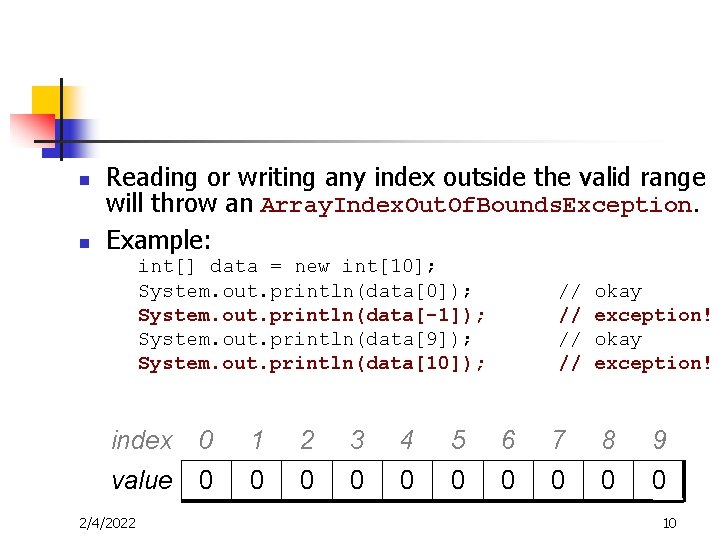
n n Reading or writing any index outside the valid range will throw an Array. Index. Out. Of. Bounds. Exception. Example: int[] data = new int[10]; System. out. println(data[0]); System. out. println(data[-1]); System. out. println(data[9]); System. out. println(data[10]); // // okay exception! index 0 1 2 3 4 5 6 7 8 9 value 0 0 0 0 0 2/4/2022 10
![int numbers new int8 numbers1 4 numbers4 99 numbers7 2 int[] numbers = new int[8]; numbers[1] = 4; numbers[4] = 99; numbers[7] = 2;](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-11.jpg)
int[] numbers = new int[8]; numbers[1] = 4; numbers[4] = 99; numbers[7] = 2; x: 4 int x = numbers[1]; numbers[x] = 44; numbers[7]] = 11; // use numbers[7] as index! numbers: 2/4/2022 0 1 2 3 4 5 6 7 0 0 0 44 99 4 0 11 0 0 0 2 0 11
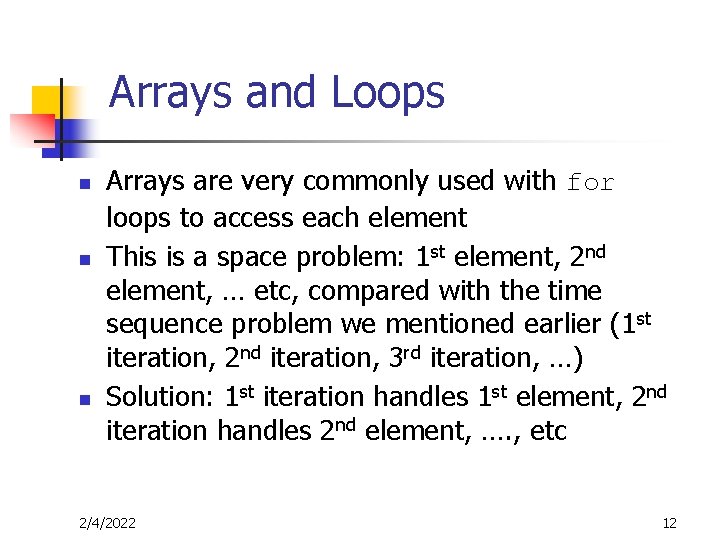
Arrays and Loops n n n Arrays are very commonly used with for loops to access each element This is a space problem: 1 st element, 2 nd element, … etc, compared with the time sequence problem we mentioned earlier (1 st iteration, 2 nd iteration, 3 rd iteration, …) Solution: 1 st iteration handles 1 st element, 2 nd iteration handles 2 nd element, …. , etc 2/4/2022 12
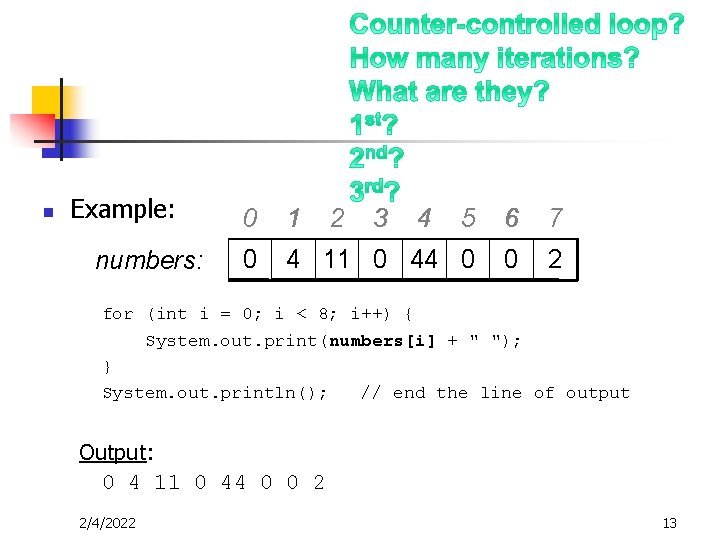
n Example: numbers: 0 1 2 3 4 5 6 7 0 0 0 44 99 4 0 11 0 0 0 2 0 for (int i = 0; i < 8; i++) { System. out. print(numbers[i] + " "); } System. out. println(); // end the line of output Output: 0 4 11 0 44 0 0 2 2/4/2022 13
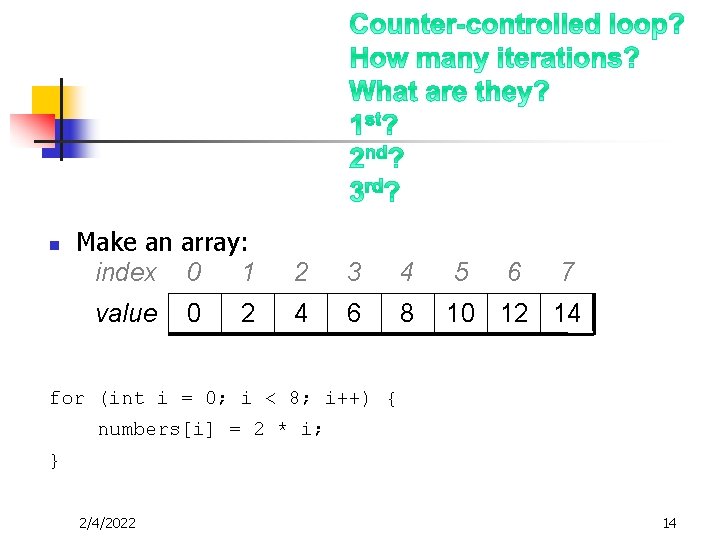
n Make an array: index 0 1 value 0 2 2 3 4 5 6 7 4 6 8 10 12 14 for (int i = 0; i < 8; i++) { numbers[i] = 2 * i; } 2/4/2022 14
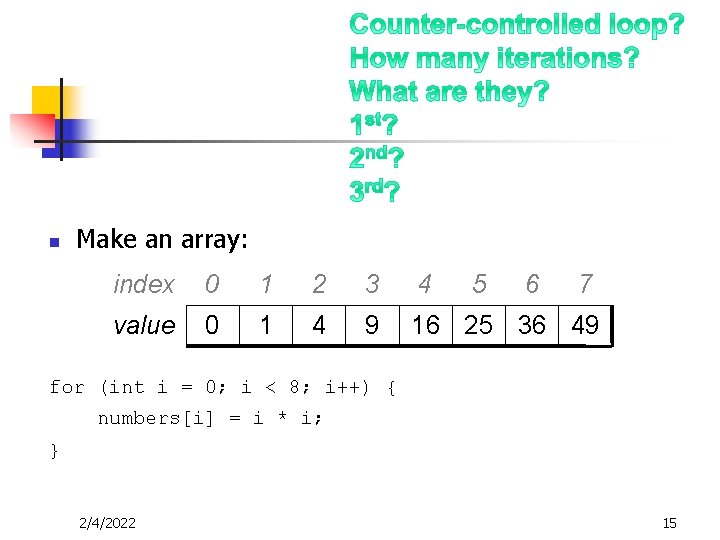
n Make an array: index 0 1 2 3 4 5 6 7 value 0 1 4 9 16 25 36 49 for (int i = 0; i < 8; i++) { numbers[i] = i * i; } 2/4/2022 15
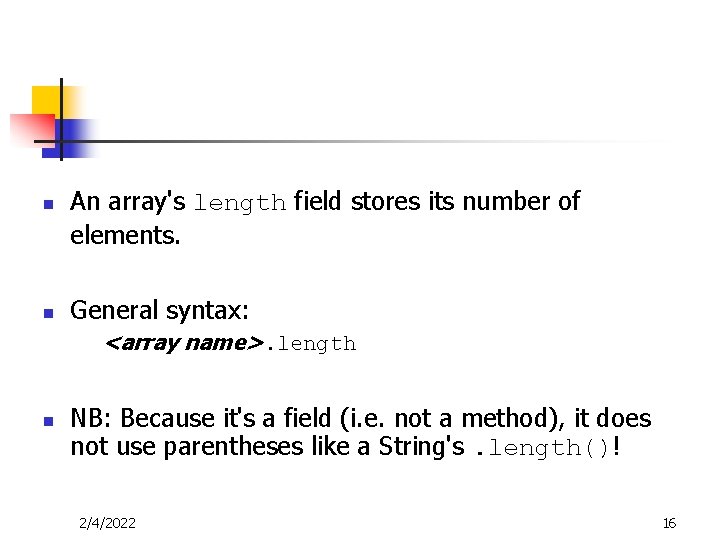
n n An array's length field stores its number of elements. General syntax: <array name>. length n NB: Because it's a field (i. e. not a method), it does not use parentheses like a String's. length()! 2/4/2022 16
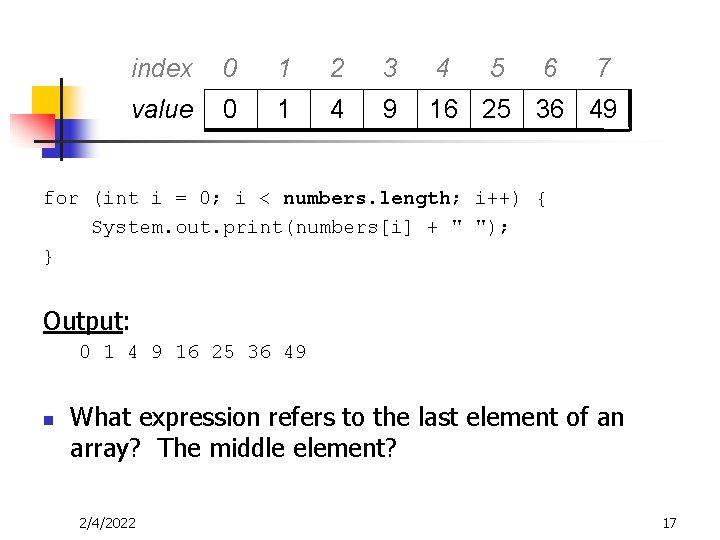
index 0 1 2 3 4 5 6 7 value 0 1 4 9 16 25 36 49 for (int i = 0; i < numbers. length; i++) { System. out. print(numbers[i] + " "); } Output: 0 1 4 9 16 25 36 49 n What expression refers to the last element of an array? The middle element? 2/4/2022 17
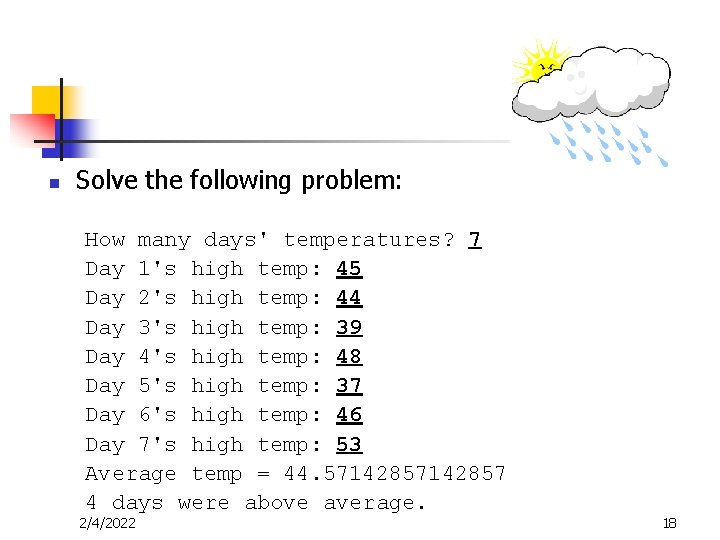
n Solve the following problem: How many days' temperatures? 7 Day 1's high temp: 45 Day 2's high temp: 44 Day 3's high temp: 39 Day 4's high temp: 48 Day 5's high temp: 37 Day 6's high temp: 46 Day 7's high temp: 53 Average temp = 44. 57142857 4 days were above average. 2/4/2022 18
![import java util public class Weather public static void mainString args import java. util. *; public class Weather { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-19.jpg)
import java. util. *; public class Weather { public static void main(String[] args) { Scanner console = new Scanner(System. in); System. out. print("How many days' temperatures? "); int days = console. next. Int(); int[] temperatures = new int[days]; int sum = 0; // to store temperatu for (int i = 0; i < days; i++) { // process each day System. out. print("Day " + (i + 1) + "'s high temp: "); temperatures[i] = console. next. Int(); sum += temperatures[i]; } double average = (double) sum / days; int count = 0; // see if above average for (int i = 0; i < days; i++) { if (temperatures[i] > average) { count++; } } // report results System. out. println("Average temp = " + average); System. out. println(count + " days above average"); 2/4/2022 19
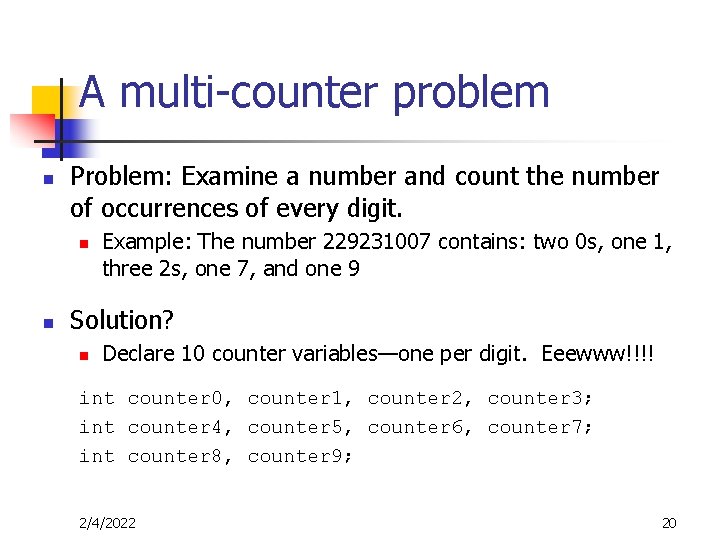
A multi-counter problem n Problem: Examine a number and count the number of occurrences of every digit. n n Example: The number 229231007 contains: two 0 s, one 1, three 2 s, one 7, and one 9 Solution? n Declare 10 counter variables—one per digit. Eeewww!!!! int counter 0, counter 1, counter 2, counter 3; int counter 4, counter 5, counter 6, counter 7; int counter 8, counter 9; 2/4/2022 20
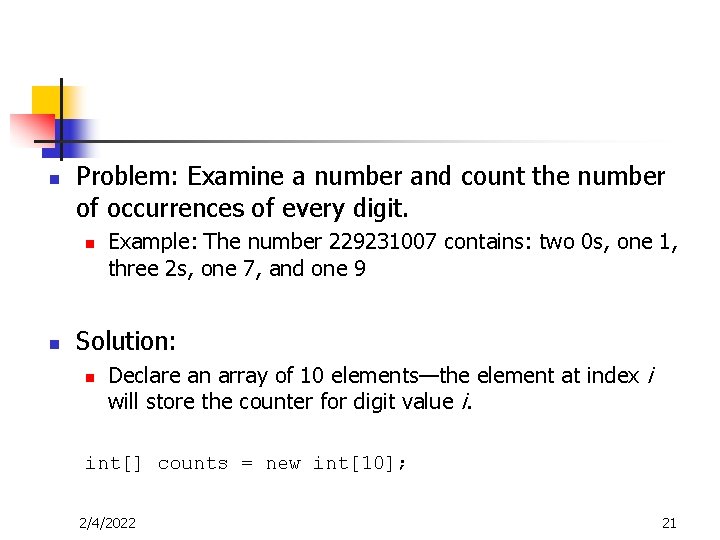
n Problem: Examine a number and count the number of occurrences of every digit. n n Example: The number 229231007 contains: two 0 s, one 1, three 2 s, one 7, and one 9 Solution: n Declare an array of 10 elements—the element at index i will store the counter for digit value i. int[] counts = new int[10]; 2/4/2022 21
![int num 229231007 ensure num 0 int counts new int10 while int num = 229231007; //ensure num > 0 int[] counts = new int[10]; while](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-22.jpg)
int num = 229231007; //ensure num > 0 int[] counts = new int[10]; while (num > 0) { int digit = num % 10; counts[digit]++; num = num / 10; } index 0 1 2 3 4 5 6 7 8 9 value 2 1 3 0 0 1 0 1 2/4/2022 22
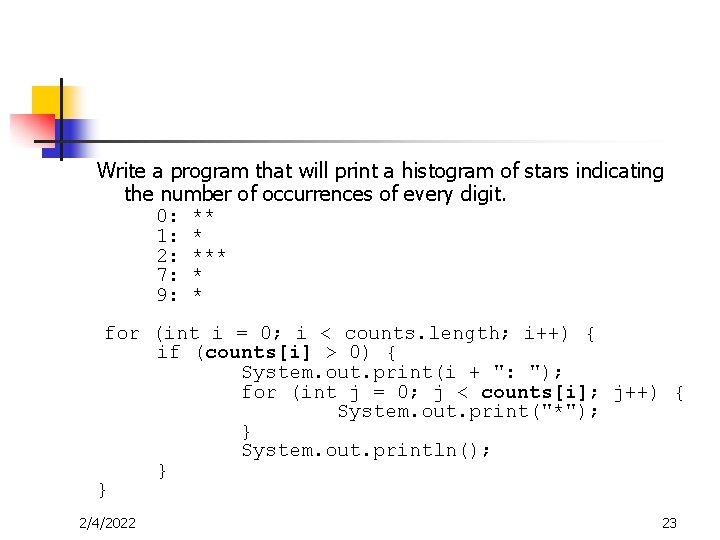
Write a program that will print a histogram of stars indicating the number of occurrences of every digit. 0: ** 1: * 2: *** 7: * 9: * for (int i = 0; i < counts. length; i++) { if (counts[i] > 0) { System. out. print(i + ": "); for (int j = 0; j < counts[i]; j++) { System. out. print("*"); } System. out. println(); } } 2/4/2022 23
![n Quick array initialization general syntax type name value n Quick array initialization, general syntax: <type>[] <name> = {<value>, . . . ,](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-24.jpg)
n Quick array initialization, general syntax: <type>[] <name> = {<value>, . . . , <value>}; n Example: int[] numbers = { 12, 49, -2, 26, 5, 17, -6 }; index n 0 1 2 3 4 5 6 value 12 49 -2 26 5 17 -6 Useful when you know in advance what the array's element values will be. 2/4/2022 24
![int a 2 5 1 6 14 7 9 for int int[] a = { 2, 5, 1, 6, 14, 7, 9 }; for (int](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-25.jpg)
int[] a = { 2, 5, 1, 6, 14, 7, 9 }; for (int i = 1; i < a. length; i++) { a[i] += a[i - 1]; } n What’s in the array? 2/4/2022 index 0 1 2 3 4 5 6 value 2 5 7 1 8 14 6 28 14 35 7 44 9 25
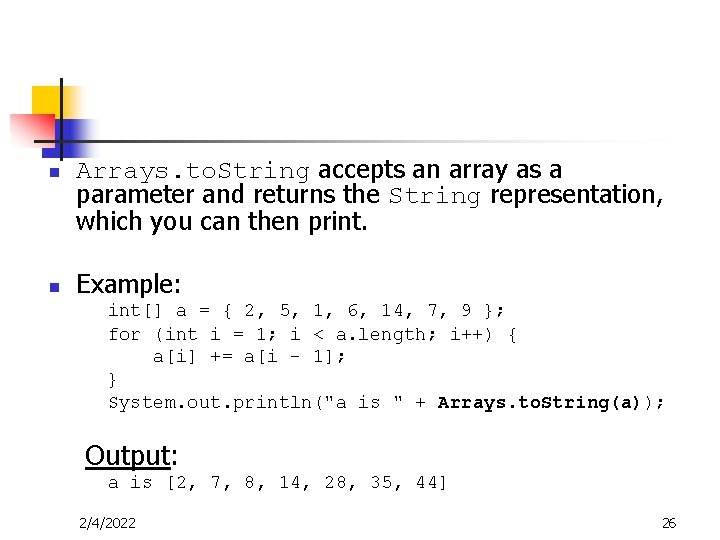
n n Arrays. to. String accepts an array as a parameter and returns the String representation, which you can then print. Example: int[] a = { 2, 5, 1, 6, 14, 7, 9 }; for (int i = 1; i < a. length; i++) { a[i] += a[i - 1]; } System. out. println("a is " + Arrays. to. String(a)); Output: a is [2, 7, 8, 14, 28, 35, 44] 2/4/2022 26
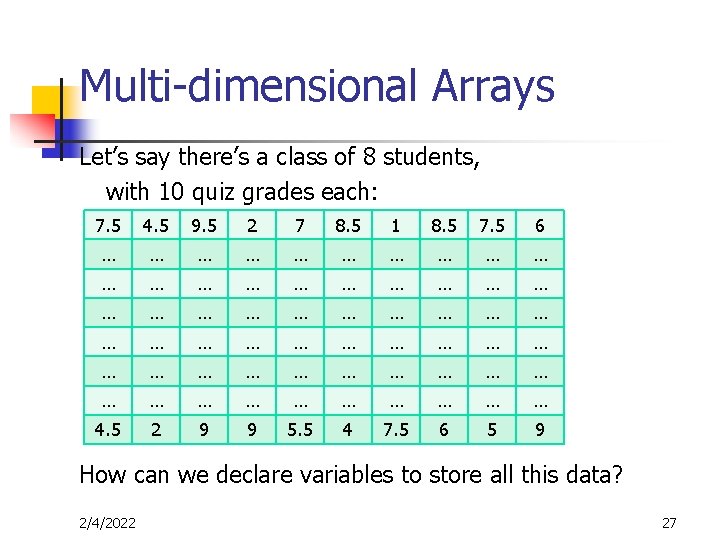
Multi-dimensional Arrays Let’s say there’s a class of 8 students, with 10 quiz grades each: 7. 5 4. 5 9. 5 2 7 8. 5 1 8. 5 7. 5 6 … … … … … … … … … … … … … … … 4. 5 2 9 9 5. 5 4 7. 5 6 5 9 How can we declare variables to store all this data? 2/4/2022 27
![double student 1 new double10 double student 8 double [] student 1 = new double[10]; . . . double [] student 8](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-28.jpg)
double [] student 1 = new double[10]; . . . double [] student 8 = new double[10]; student 1: . . . student 8: 7. 5 4. 5 9. 5 2 7 8. 5 1 8. 5 7. 5 6 4. 5 2 9 9 5. 5 4 7. 5 6 5 9 Solution 1: An array per student. Tedious! 2/4/2022 28
![double quiz 1 new double8 double quiz 10 double [] quiz 1 = new double[8]; . . . double [] quiz 10](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-29.jpg)
double [] quiz 1 = new double[8]; . . . double [] quiz 10 = new double[8]; quiz 1: . . . quiz 10: 7. 5 … … … 4. 5 6 … … … 9 Solution 2: An array per quiz. Same problem! 2/4/2022 29
![double quiz Scores new double810 quiz Scores06 quiz Scores 7 5 4 double [][] quiz. Scores = new double[8][10]; quiz. Scores[0][6] quiz. Scores: 7. 5 4.](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-30.jpg)
double [][] quiz. Scores = new double[8][10]; quiz. Scores[0][6] quiz. Scores: 7. 5 4. 5 9. 5 2 7 8. 5 1 8. 5 7. 5 6 … … … … … … … … … … … … … … … 4. 5 2 9 9 5. 5 4 7. 5 6 5 9 quiz. Scores[7][9] 2/4/2022 30
![n A 2 D array is really an array of arrays double quiz n A 2 -D array is really an array of arrays! double [][] quiz.](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-31.jpg)
n A 2 -D array is really an array of arrays! double [][] quiz. Scores = new double[4][3]; quiz. Scores[0][0] quiz. Scores[0][1] quiz. Scores[0][2] quiz. Scores[0] quiz. Scores[1] quiz. Scores[2] quiz. Scores[3] 2/4/2022 31
![double quiz Scores new double4 quiz Scores0 new double3 quiz Scores2 double [][] quiz. Scores = new double[4][]; quiz. Scores[0] = new double[3]; quiz. Scores[2]](https://slidetodoc.com/presentation_image_h2/a092f289b689b3ccdcf8d1ffb12da68e/image-32.jpg)
double [][] quiz. Scores = new double[4][]; quiz. Scores[0] = new double[3]; quiz. Scores[2] = new double[5]; quiz. Scores[0][0] quiz. Scores[0][1] quiz. Scores[0][2] quiz. Scores[0] quiz. Scores[1] quiz. Scores[2] quiz. Scores[3] 2/4/2022 32
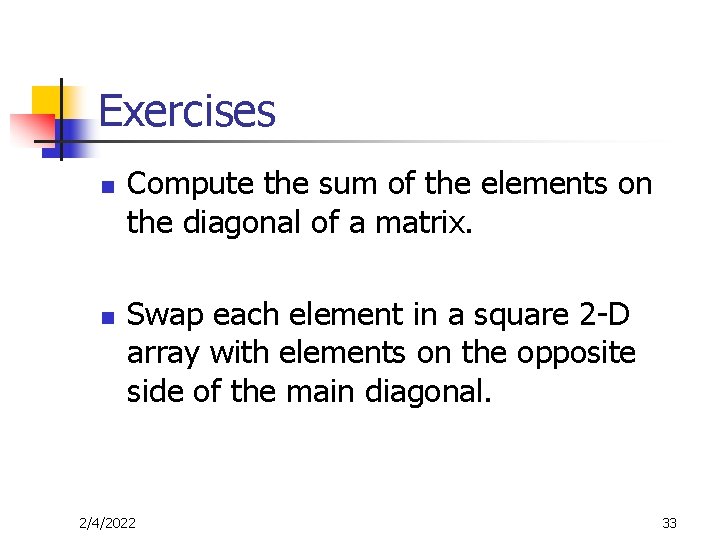
Exercises n n Compute the sum of the elements on the diagonal of a matrix. Swap each element in a square 2 -D array with elements on the opposite side of the main diagonal. 2/4/2022 33
Zhen jiang actor
Zhen gu
Zhen dong berkeley
Cis 1068
Zhen._.nnnn
My favorite subject is english because
Feifei jiang
Cs zhe jiang
Dr shan jiang
Xi river map
Tianxiao jiang
Roland jiang
Roland jiang
Qiang jiang
Qiang jiang
Vodstvo azie
Dr yung chen
Raymond jiang
"jiepu jiang"
Min jiang uncc
Jiang jeishi
Zheng jiang history
Jiang jeishi
Rbac 141
141 ir
Cse 141
Cse 141
Berger code checker
Buy bremelanotide australia
Art 141 cod fiscal
Upn 141
Asc 805 business combinations
How many months is 141 days
Ee 141