CSC 115 Introduction to Computer Programming Zhen Jiang
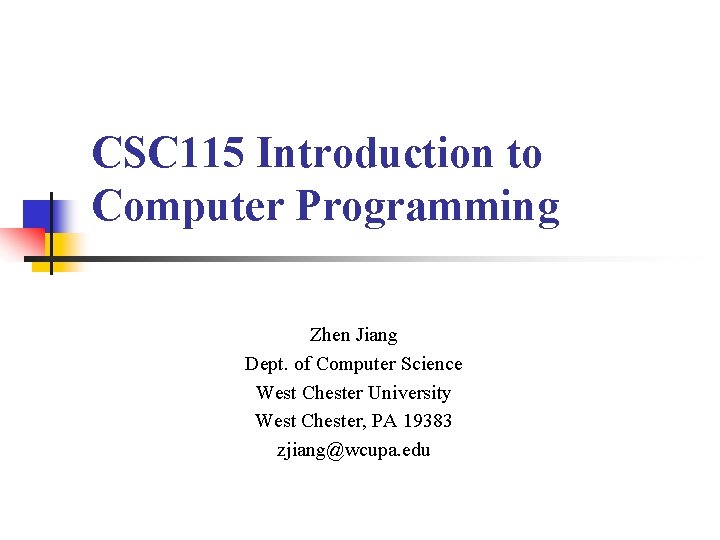
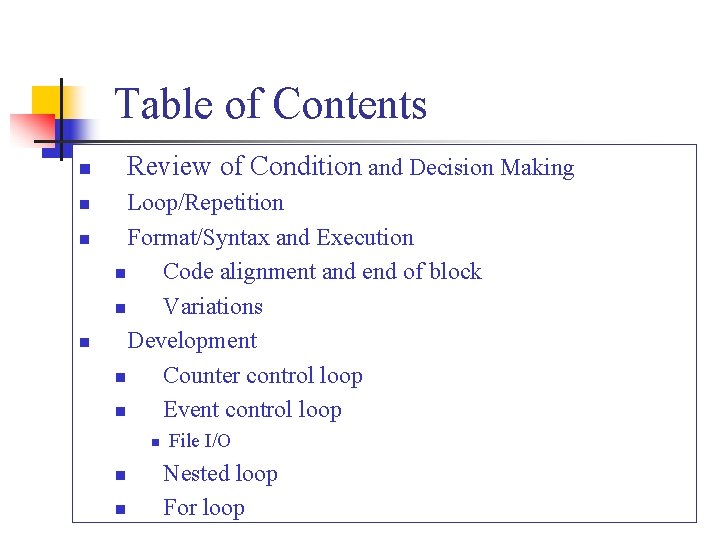
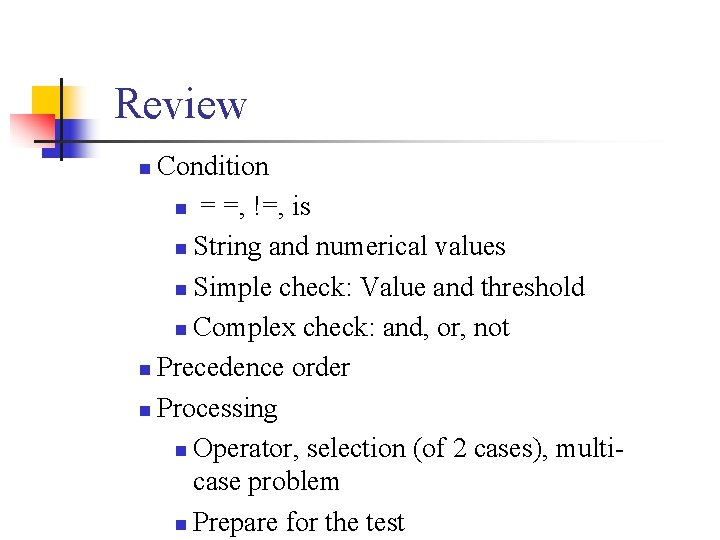
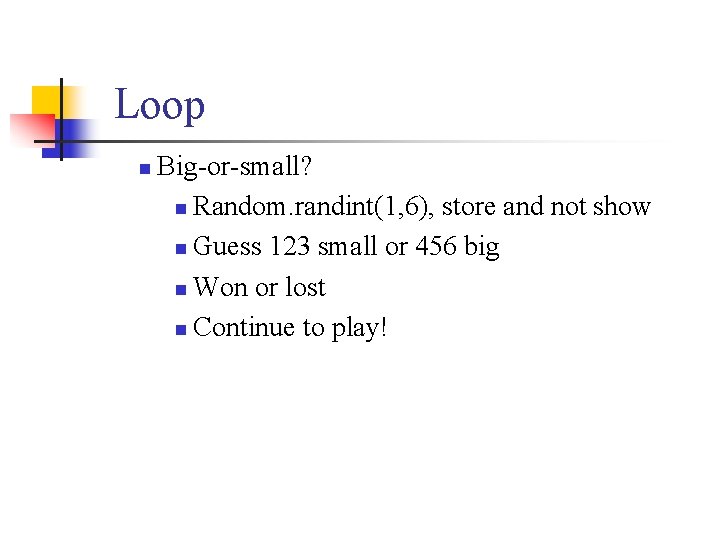
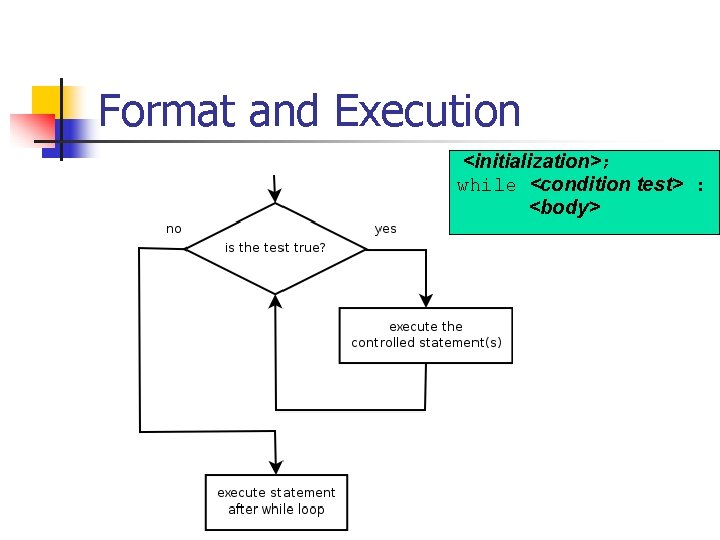
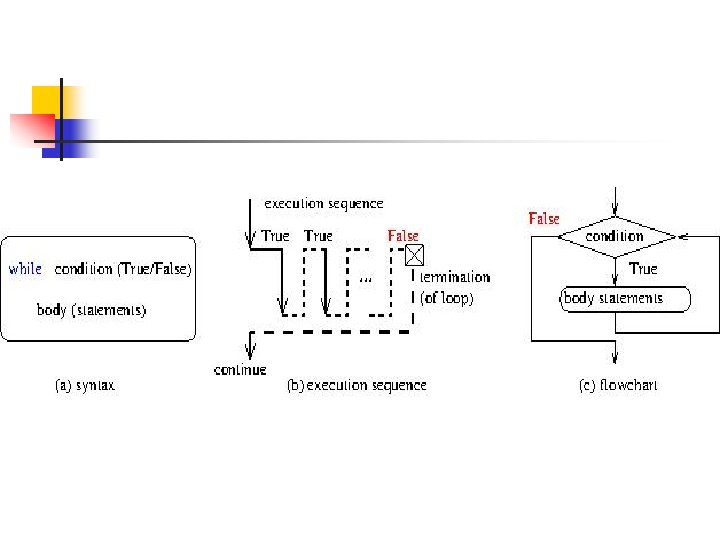
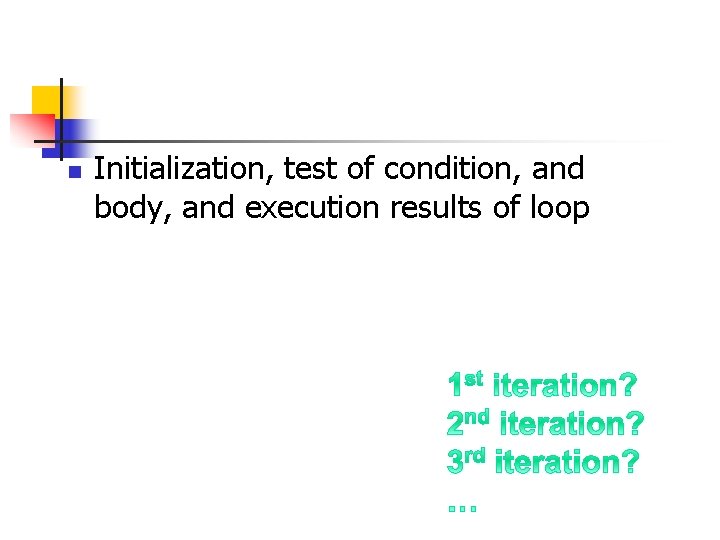
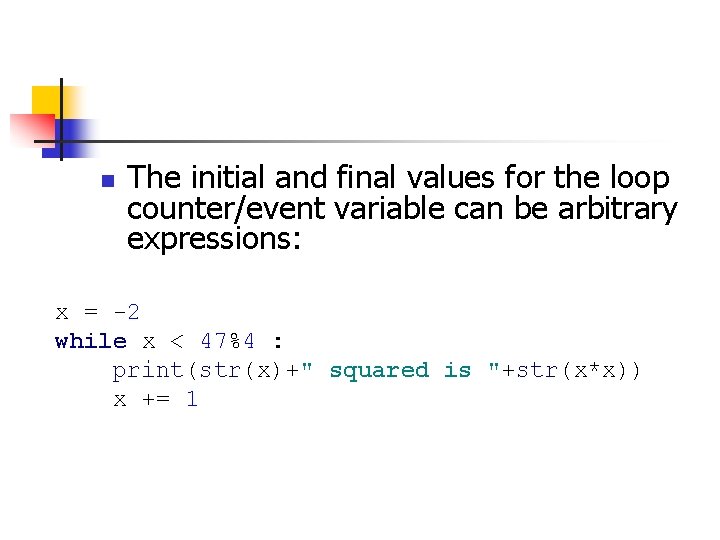
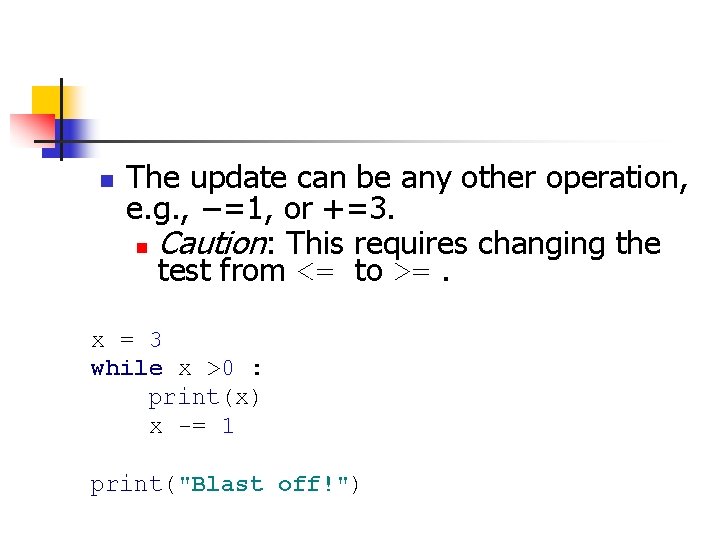
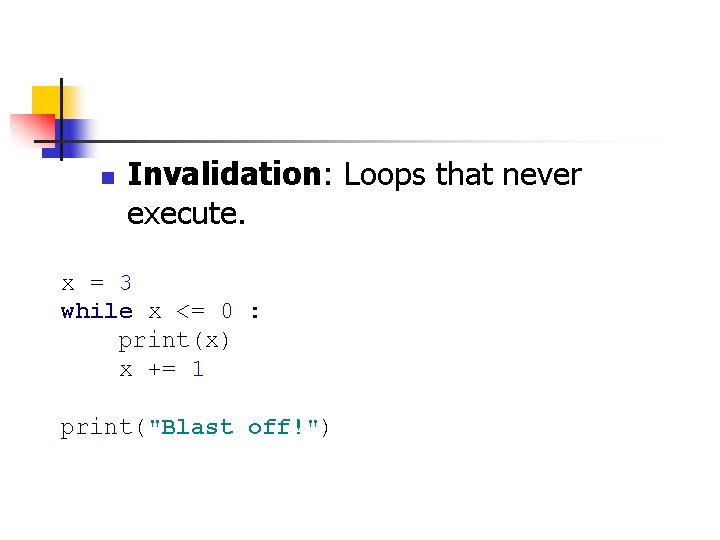
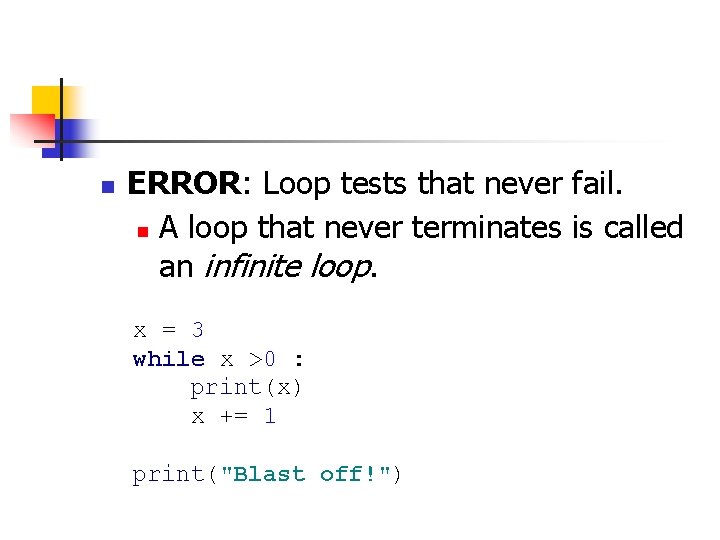
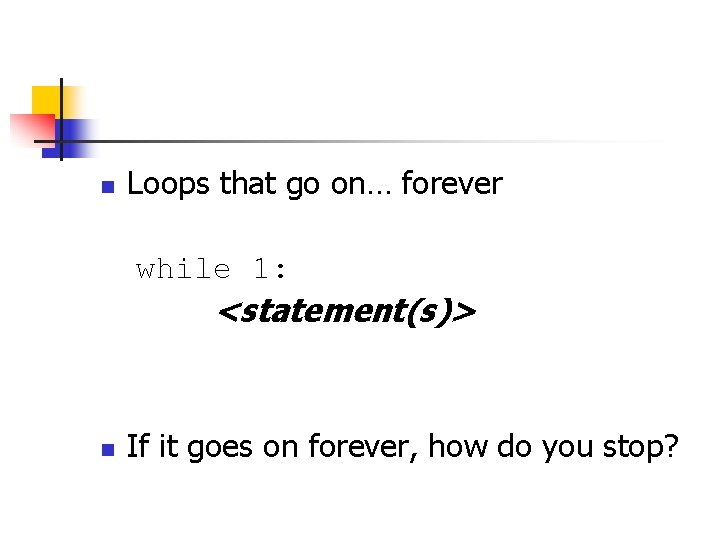
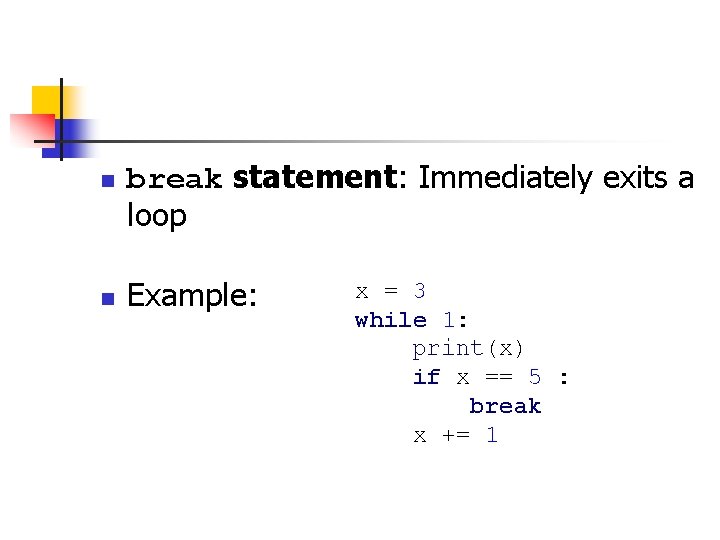
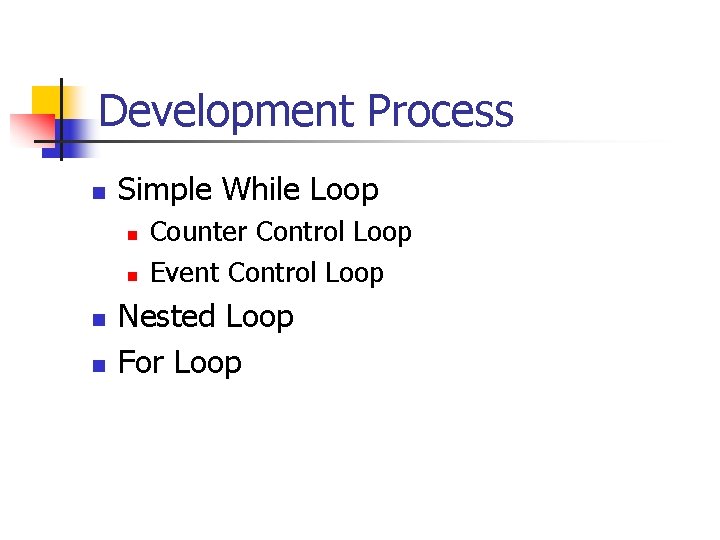
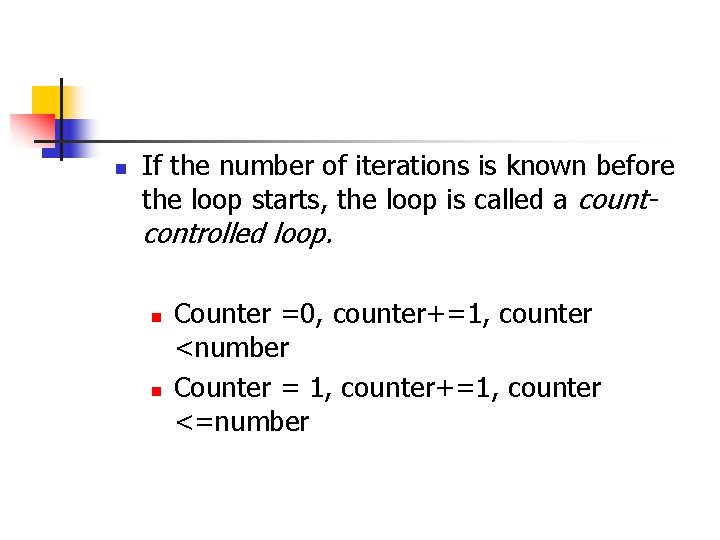
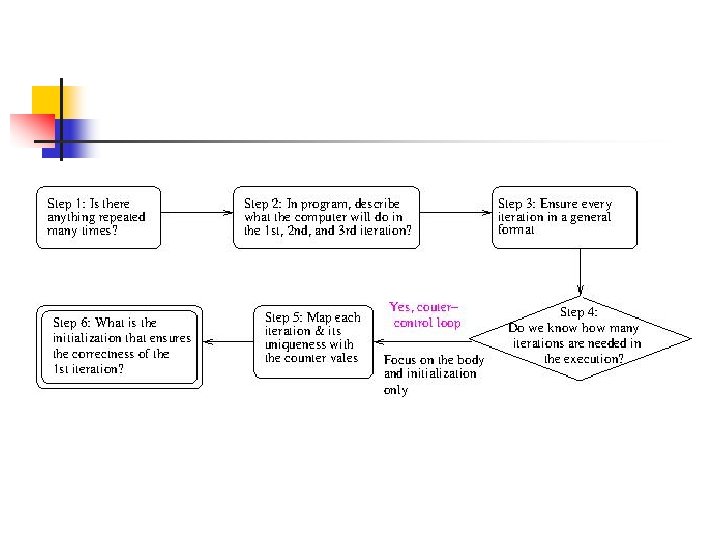
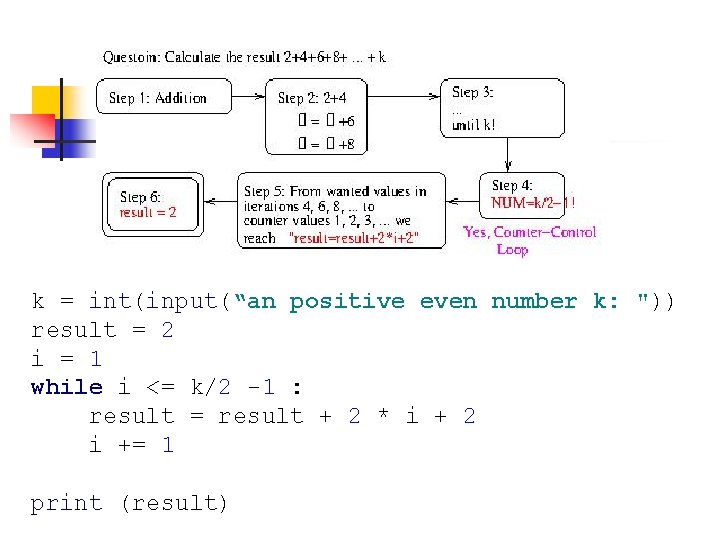
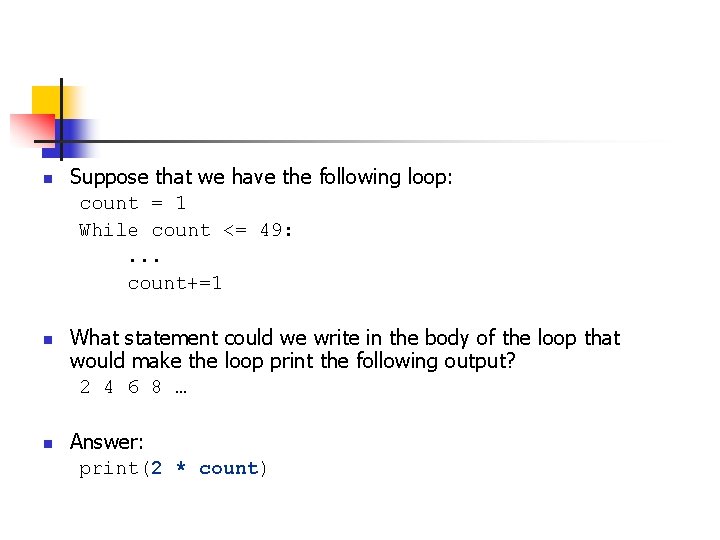
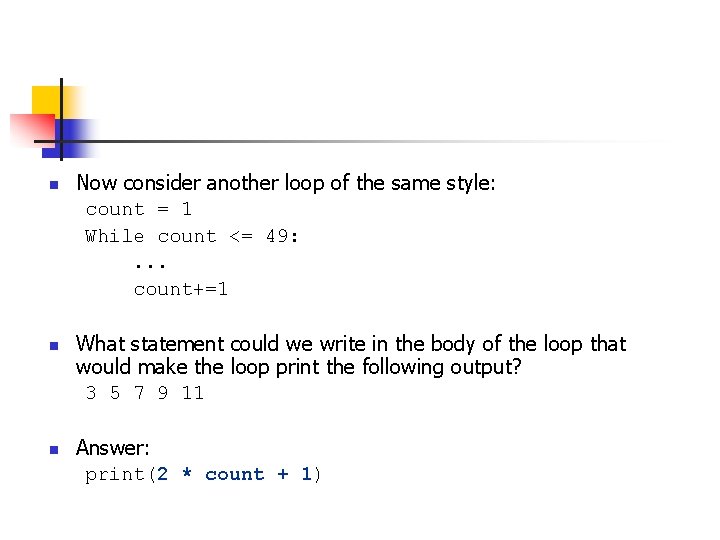
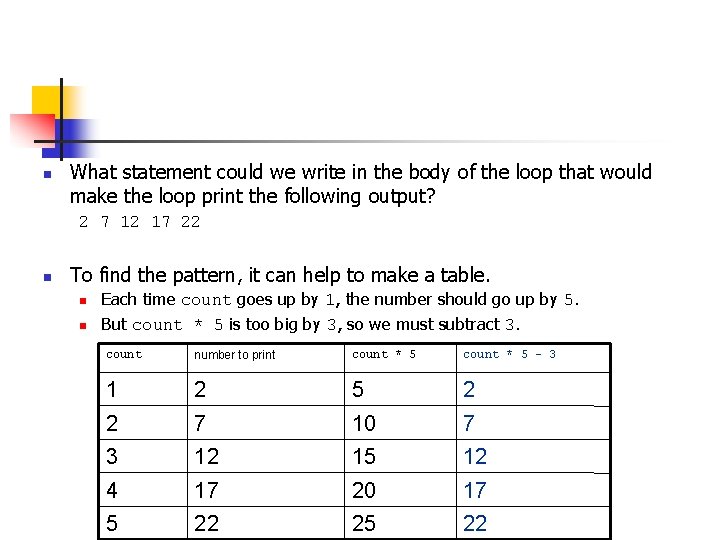
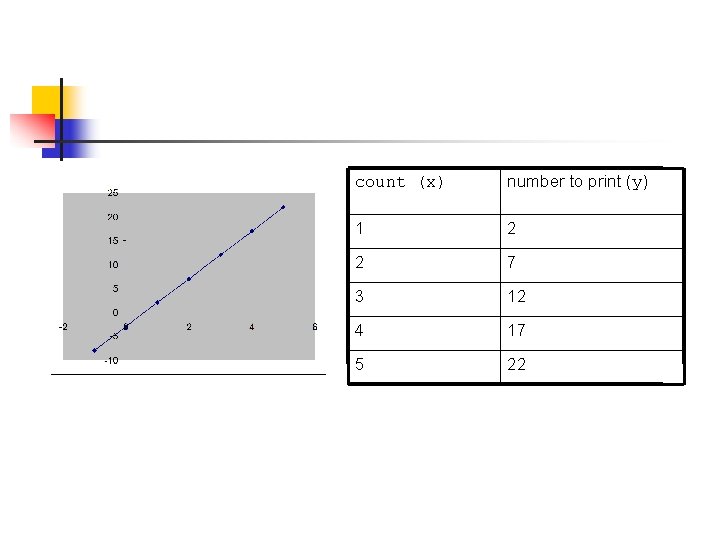
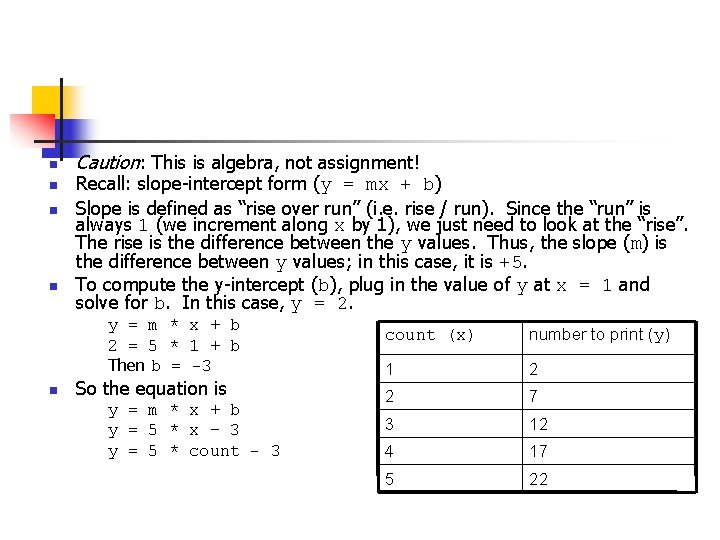
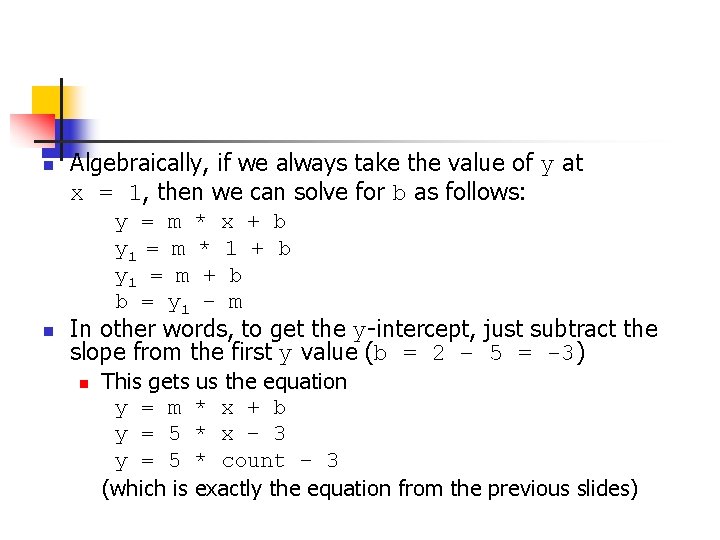
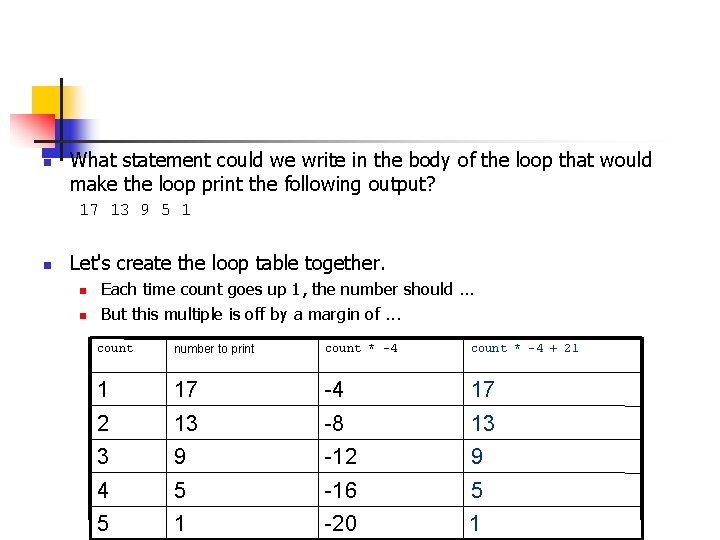
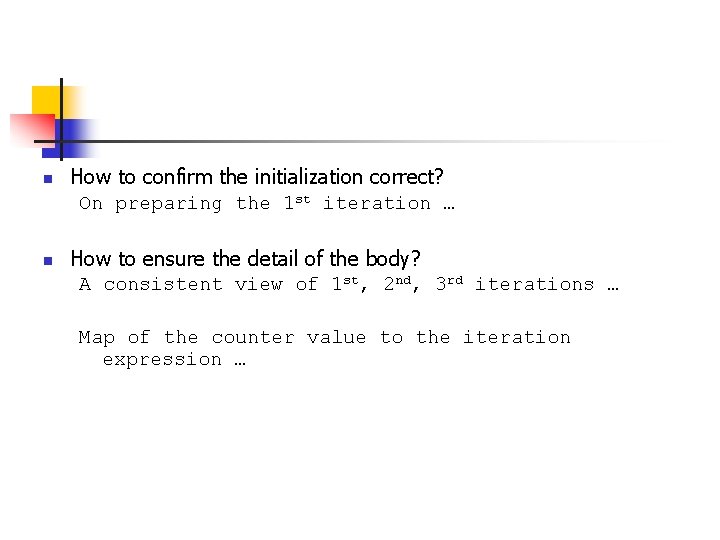
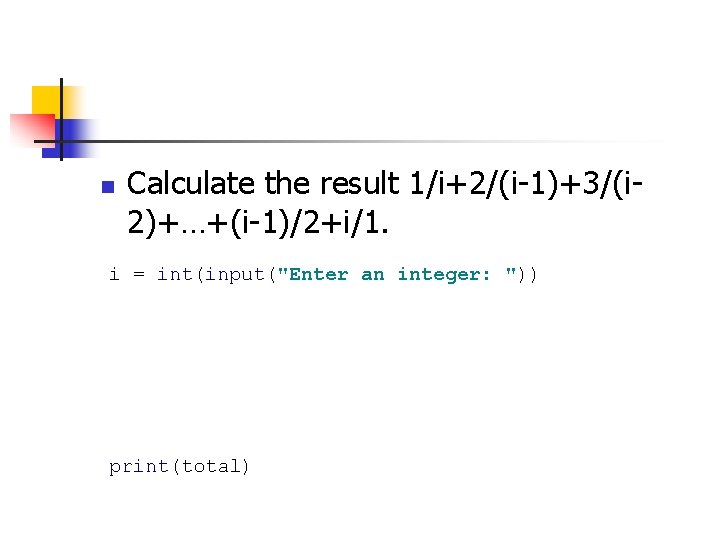
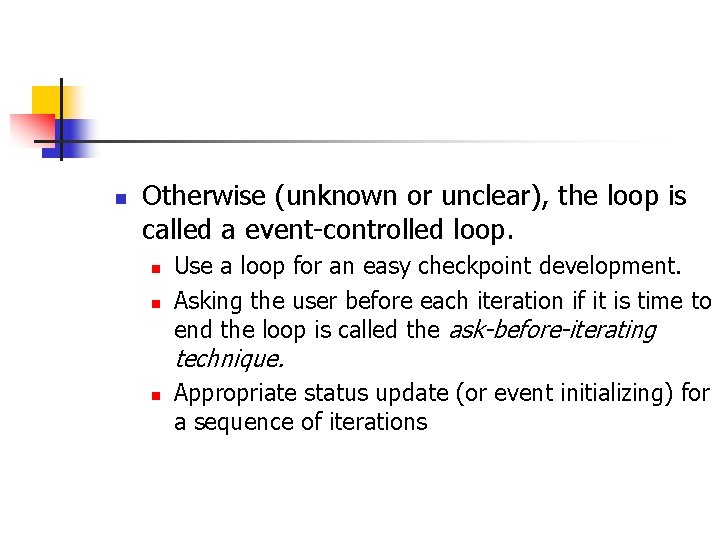
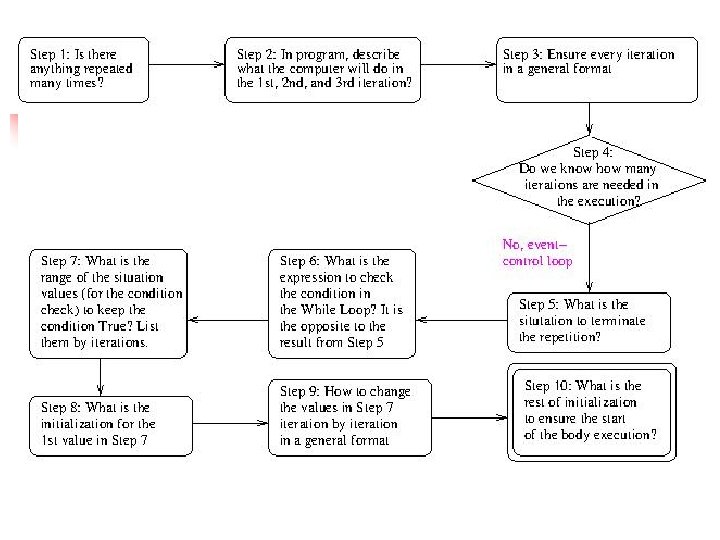
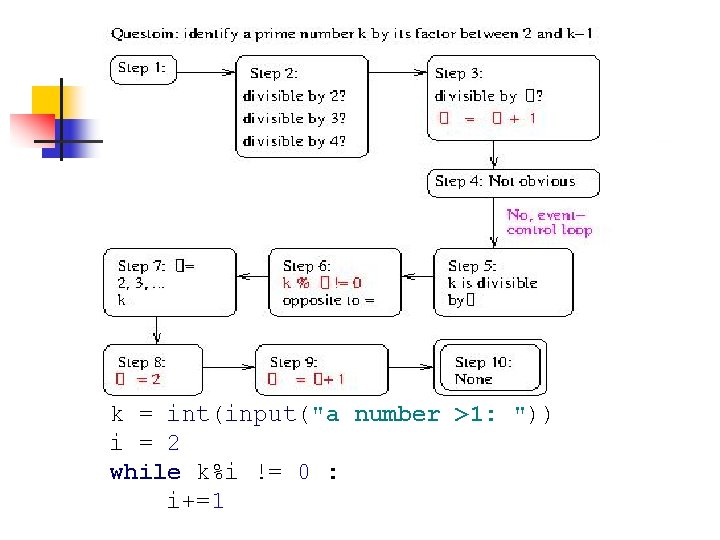
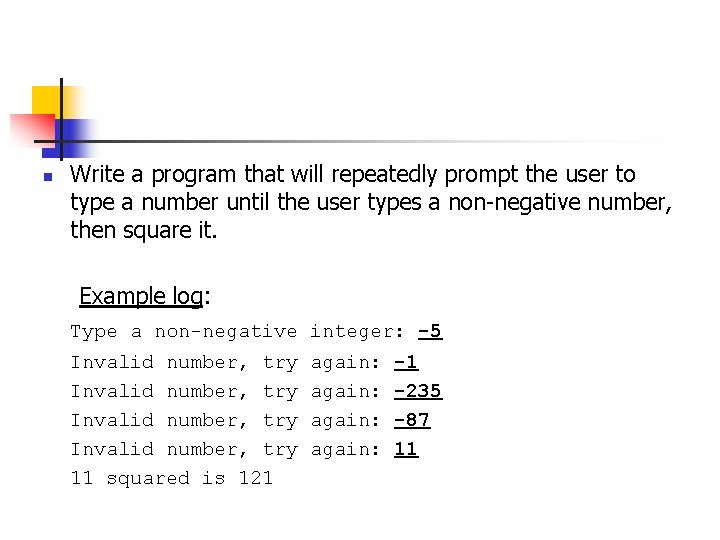
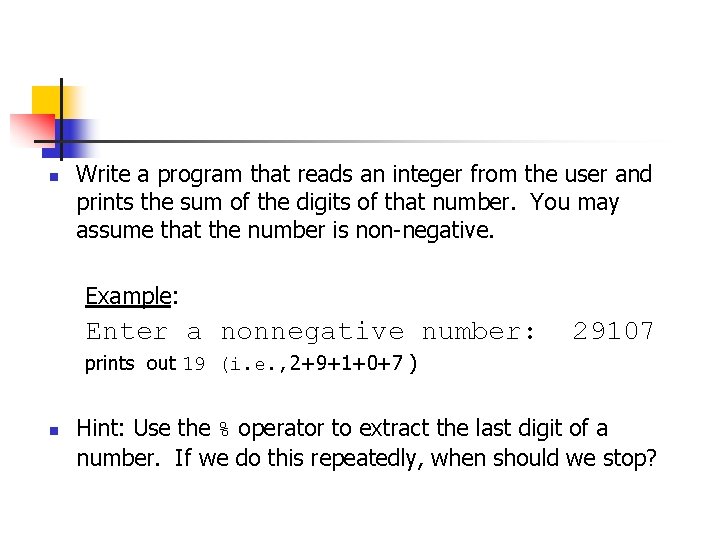
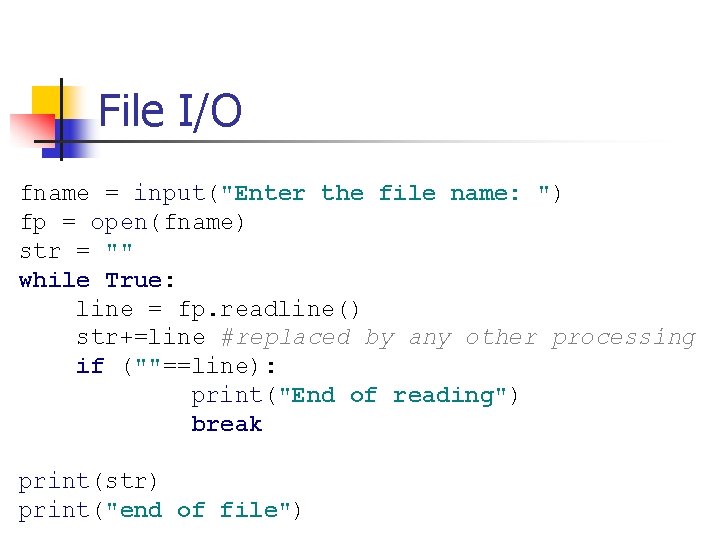
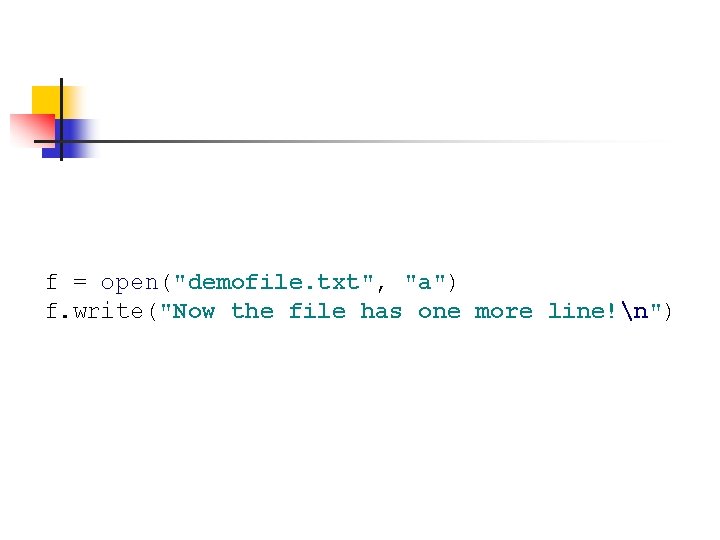
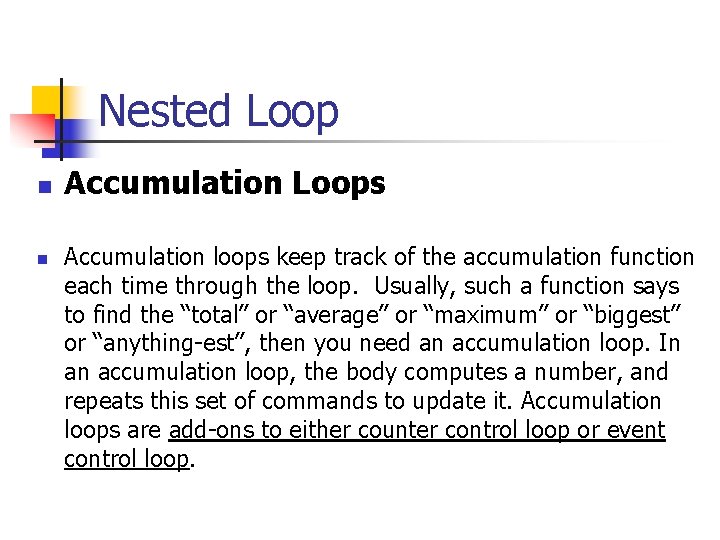
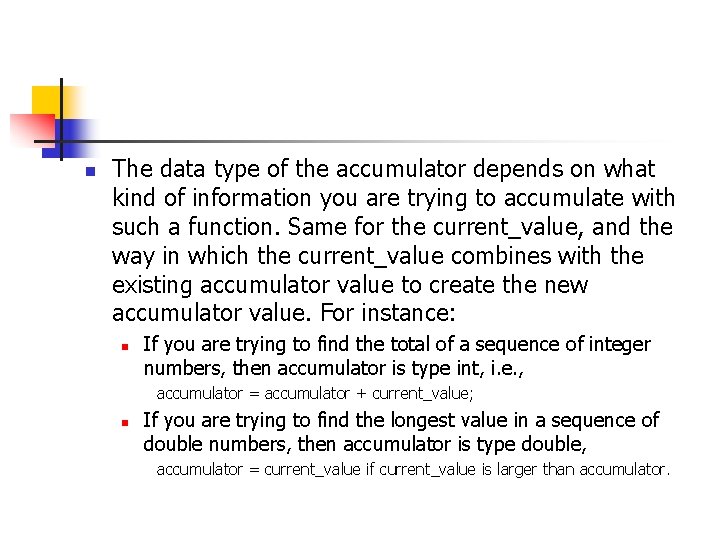
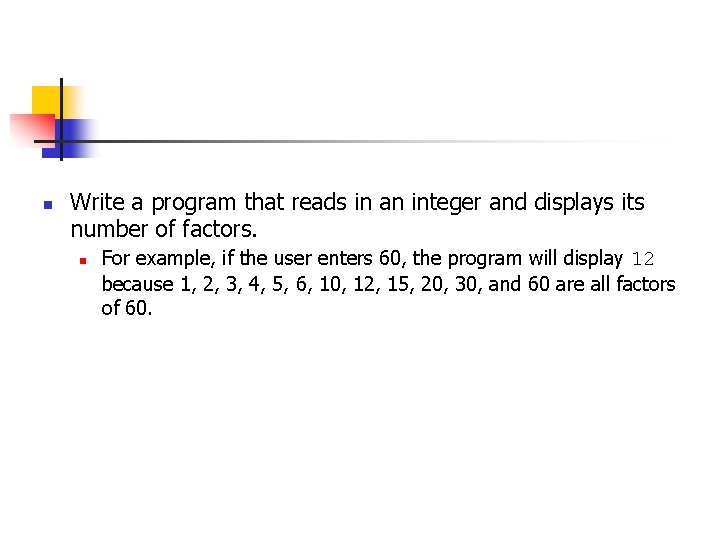
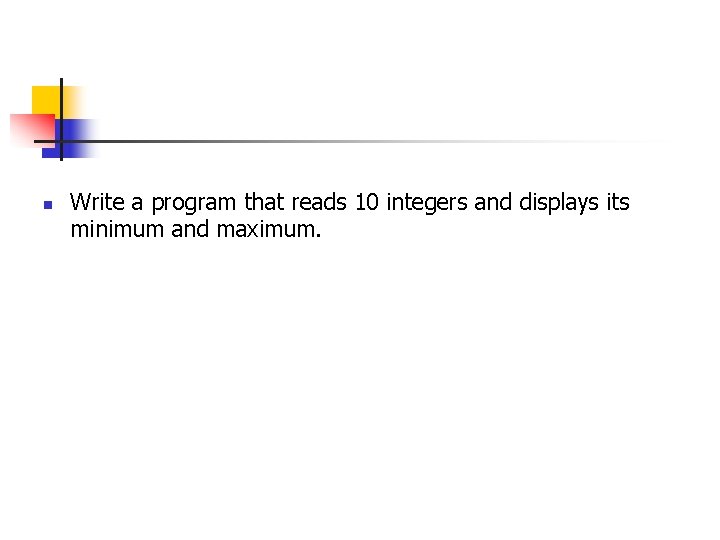
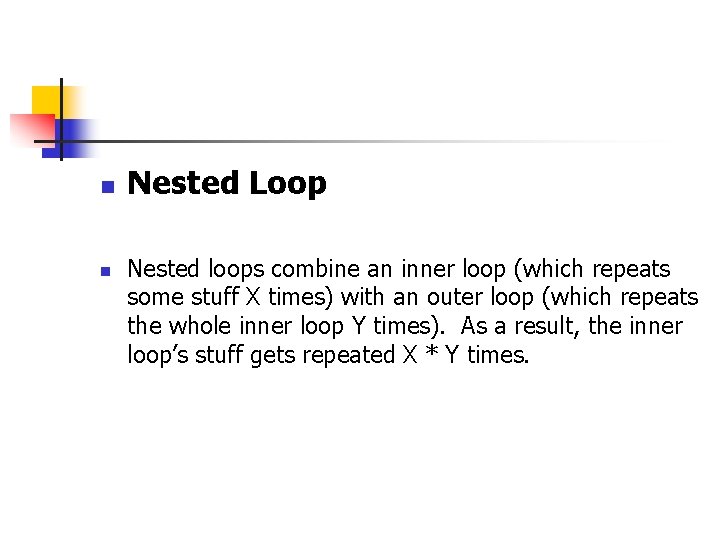
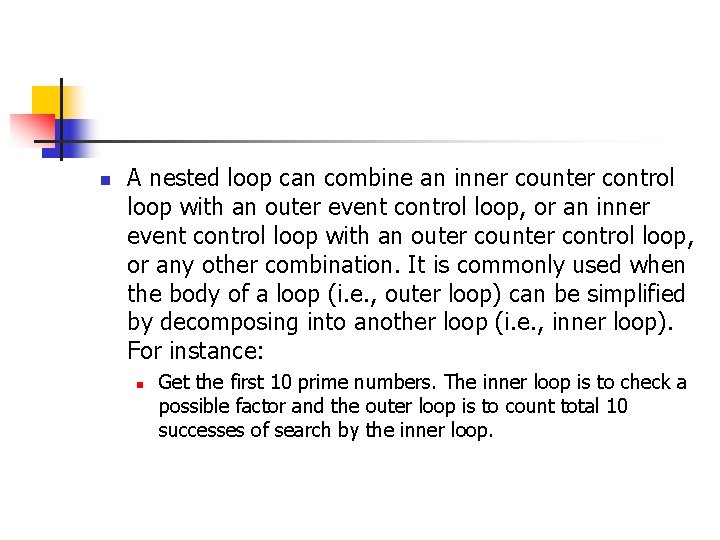
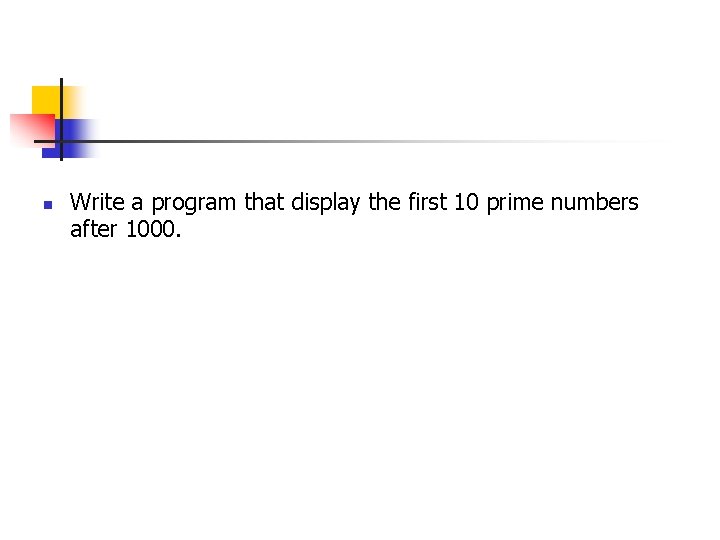
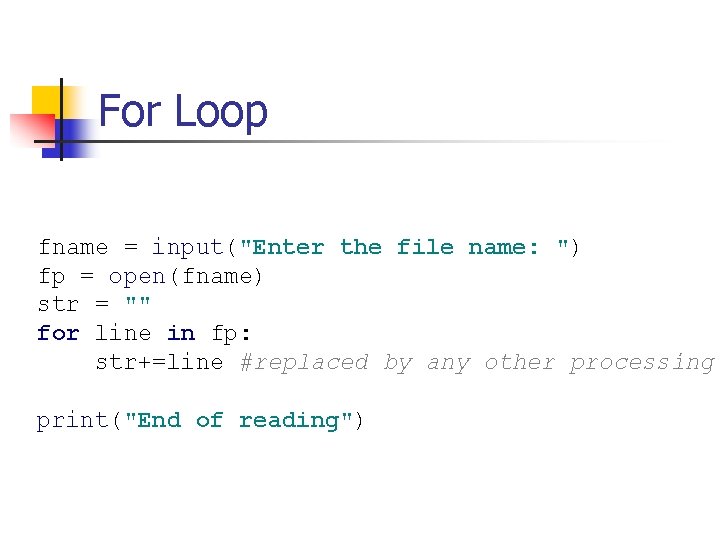
![fruits = ["apple", "banana", "cherry"] for x in fruits: print(x) fruits = ["apple", "banana", "cherry"] for x in fruits: print(x)](https://slidetodoc.com/presentation_image_h/ca95f41373ce6ea331cc5d7eea4dc7ad/image-42.jpg)
- Slides: 42
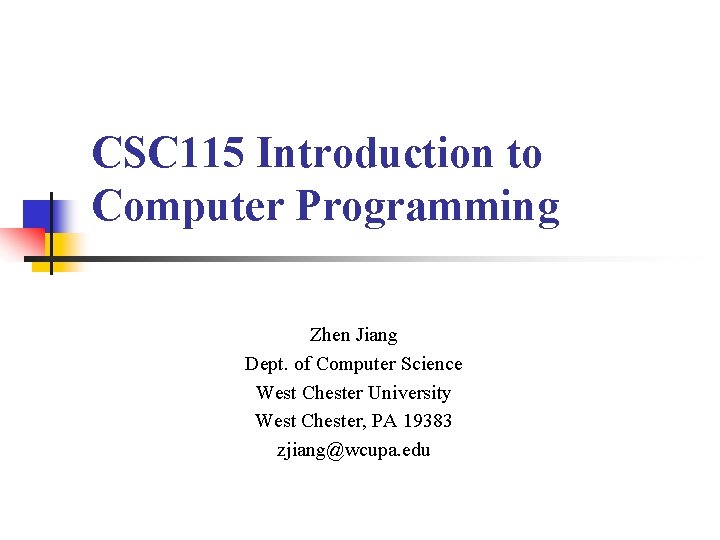
CSC 115 Introduction to Computer Programming Zhen Jiang Dept. of Computer Science West Chester University West Chester, PA 19383 zjiang@wcupa. edu
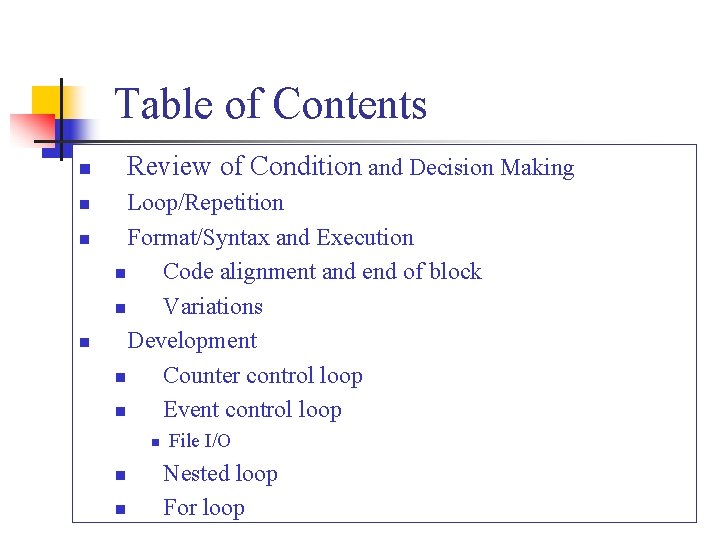
Table of Contents Review of Condition and Decision Making n n Loop/Repetition Format/Syntax and Execution n Code alignment and end of block n Variations Development n Counter control loop n Event control loop n n n File I/O Nested loop For loop
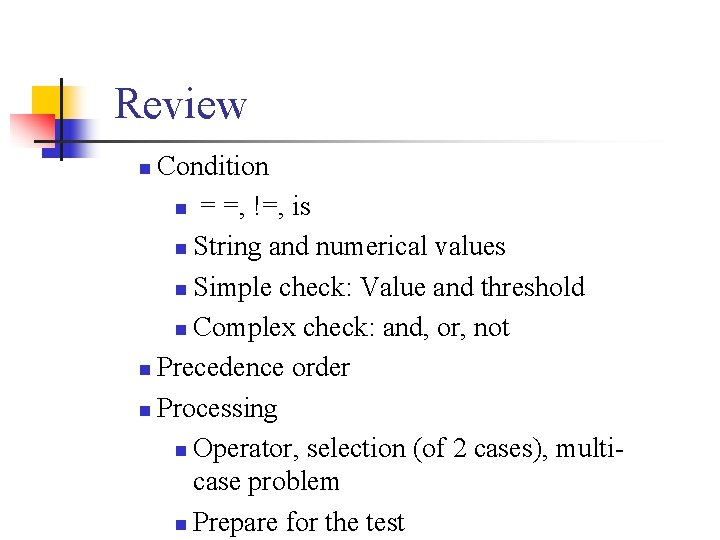
Review Condition n = =, !=, is n String and numerical values n Simple check: Value and threshold n Complex check: and, or, not n Precedence order n Processing n Operator, selection (of 2 cases), multicase problem n Prepare for the test n
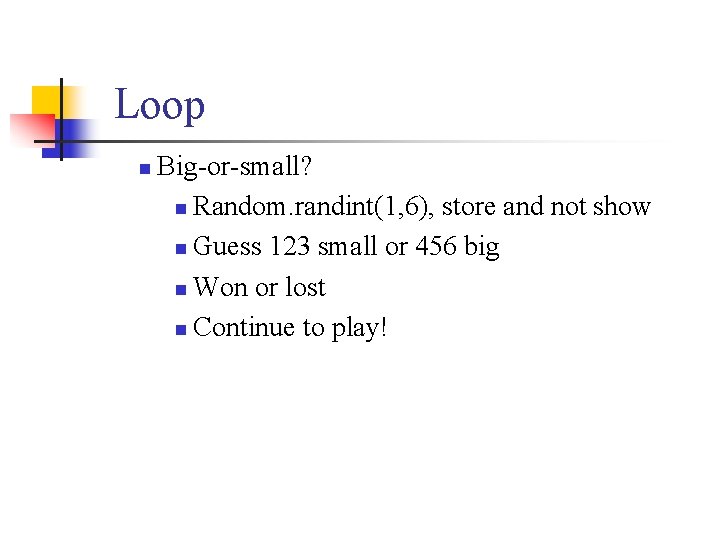
Loop n Big-or-small? n Random. randint(1, 6), store and not show n Guess 123 small or 456 big n Won or lost n Continue to play!
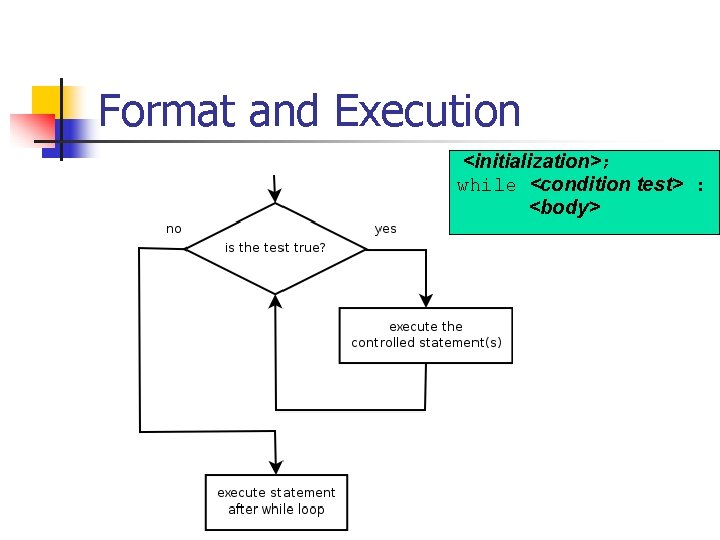
Format and Execution <initialization>; while <condition test> : <body>
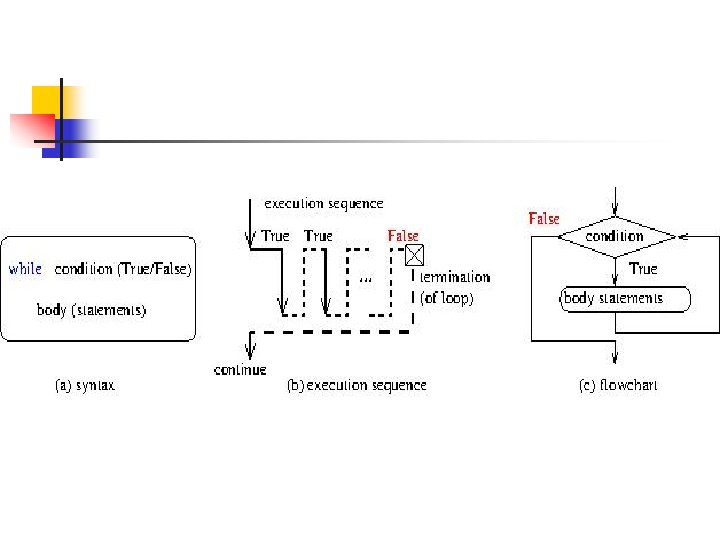
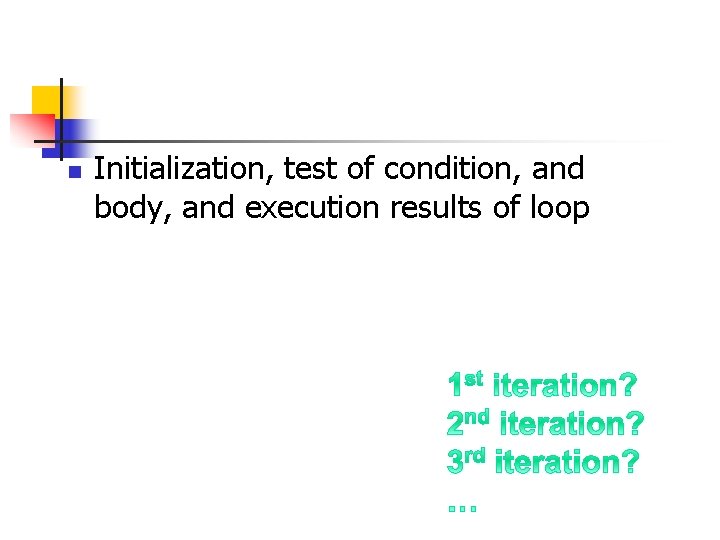
n Initialization, test of condition, and body, and execution results of loop
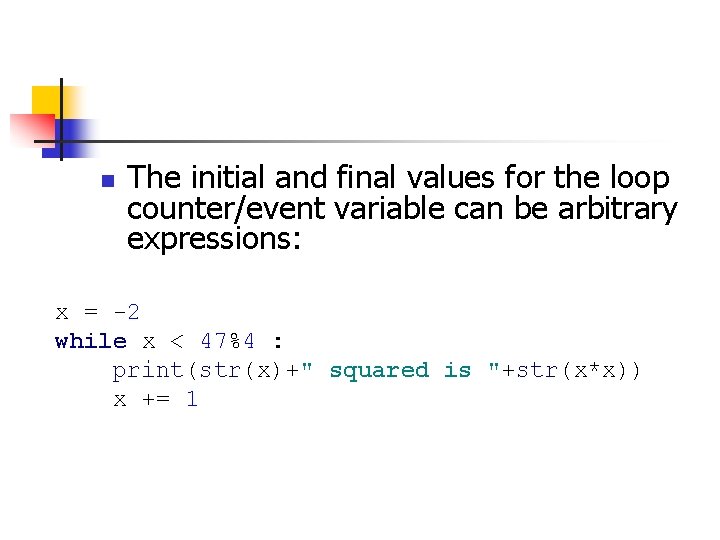
n The initial and final values for the loop counter/event variable can be arbitrary expressions: x = -2 while x < 47%4 : print(str(x)+" squared is "+str(x*x)) x += 1
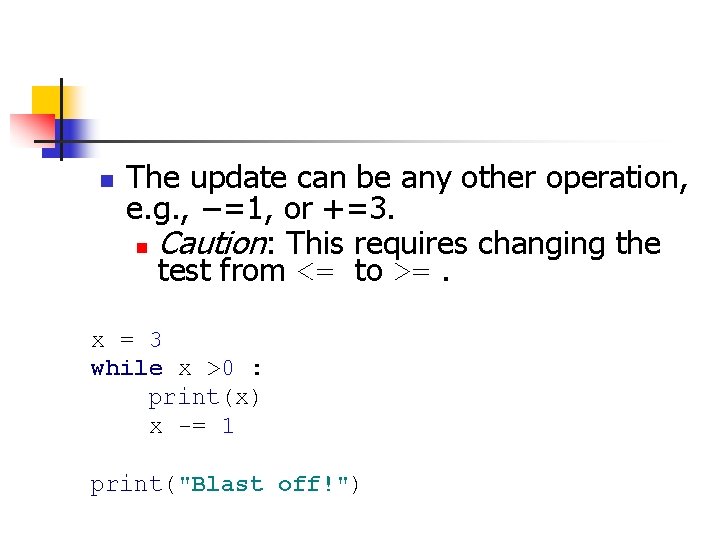
n The update can be any other operation, e. g. , −=1, or +=3. n Caution: This requires changing the test from <= to >=. x = 3 while x >0 : print(x) x -= 1 print("Blast off!")
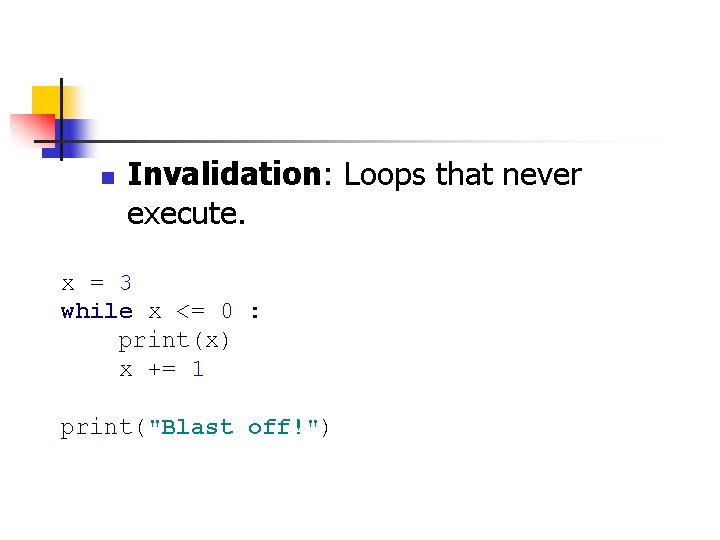
n Invalidation: Loops that never execute. x = 3 while x <= 0 : print(x) x += 1 print("Blast off!")
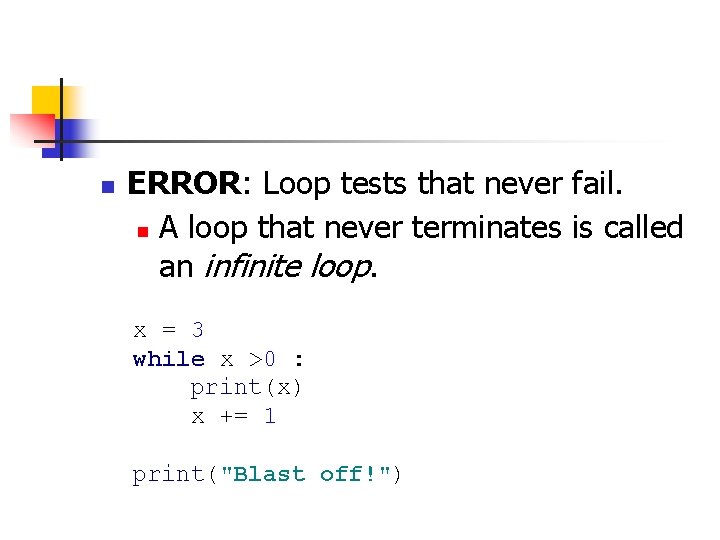
n ERROR: Loop tests that never fail. n A loop that never terminates is called an infinite loop. x = 3 while x >0 : print(x) x += 1 print("Blast off!")
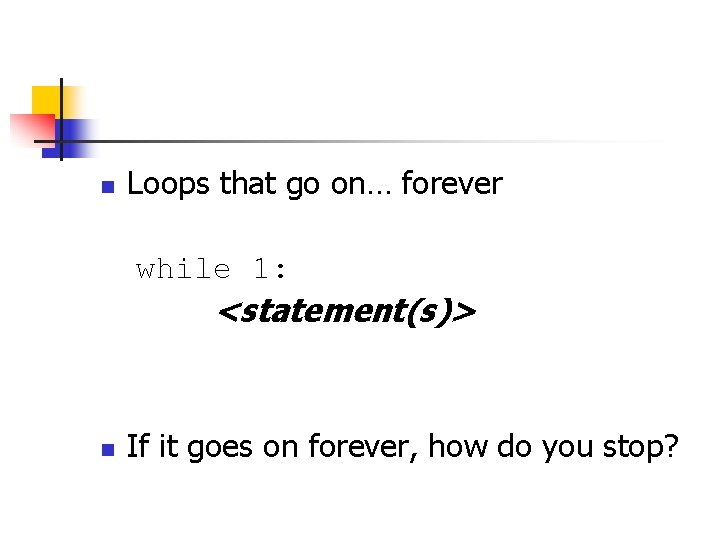
n Loops that go on… forever while 1: <statement(s)> n If it goes on forever, how do you stop?
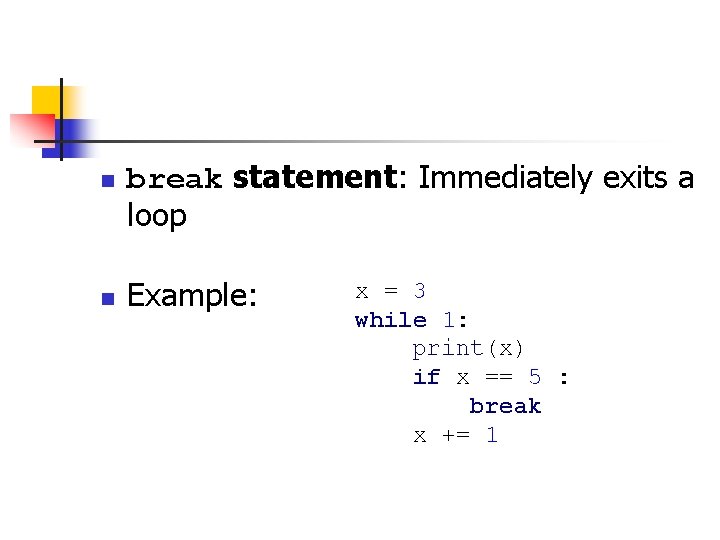
n n break statement: Immediately exits a loop Example: x = 3 while 1: print(x) if x == 5 : break x += 1
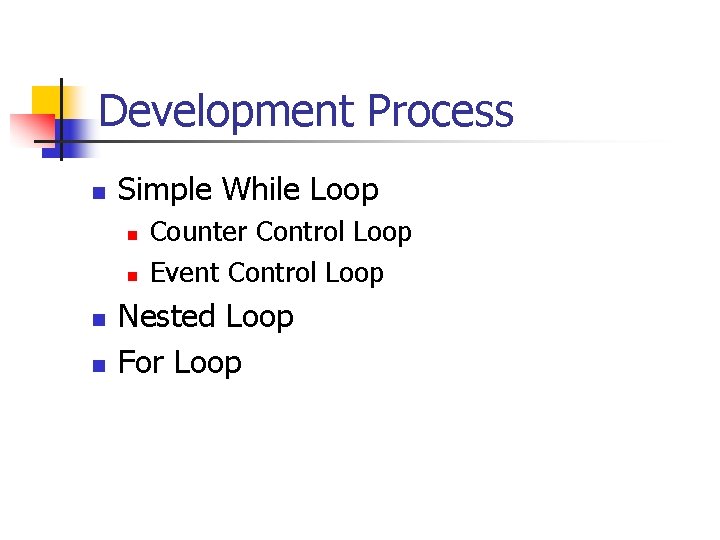
Development Process n Simple While Loop n n Counter Control Loop Event Control Loop Nested Loop For Loop
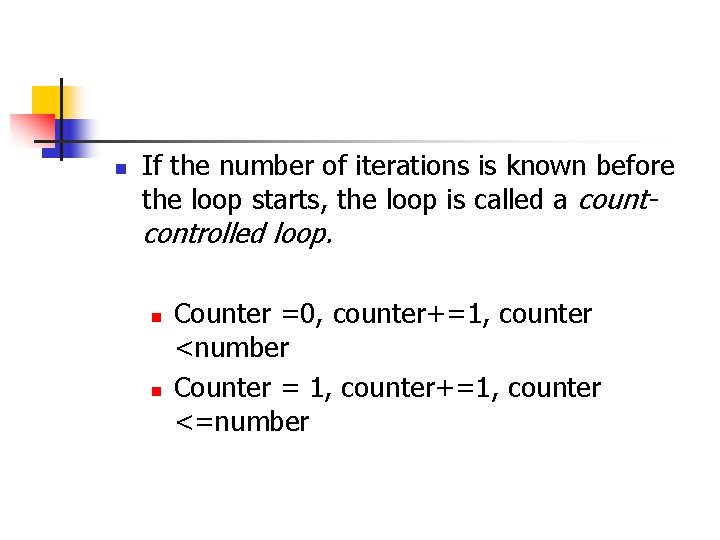
n If the number of iterations is known before the loop starts, the loop is called a count- controlled loop. n n Counter =0, counter+=1, counter <number Counter = 1, counter+=1, counter <=number
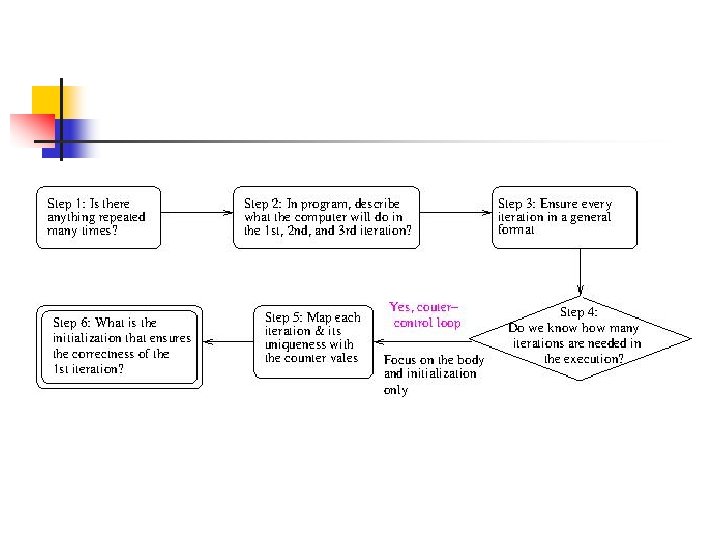
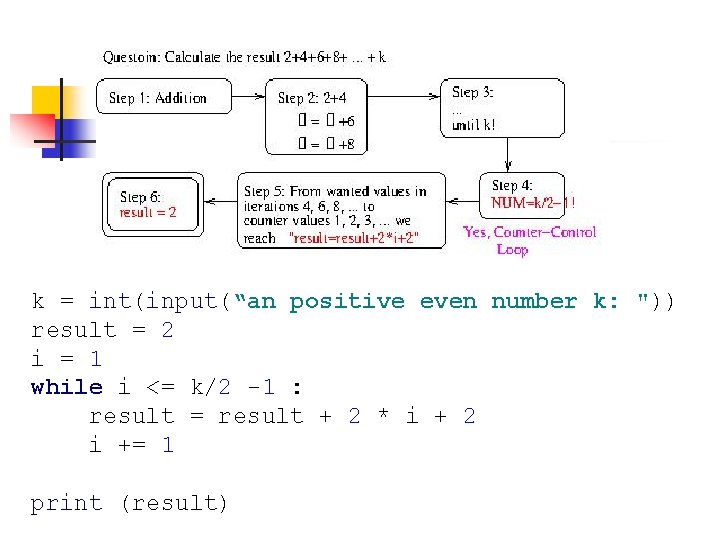
k = int(input(“an positive even number k: ")) result = 2 i = 1 while i <= k/2 -1 : result = result + 2 * i + 2 i += 1 print (result)
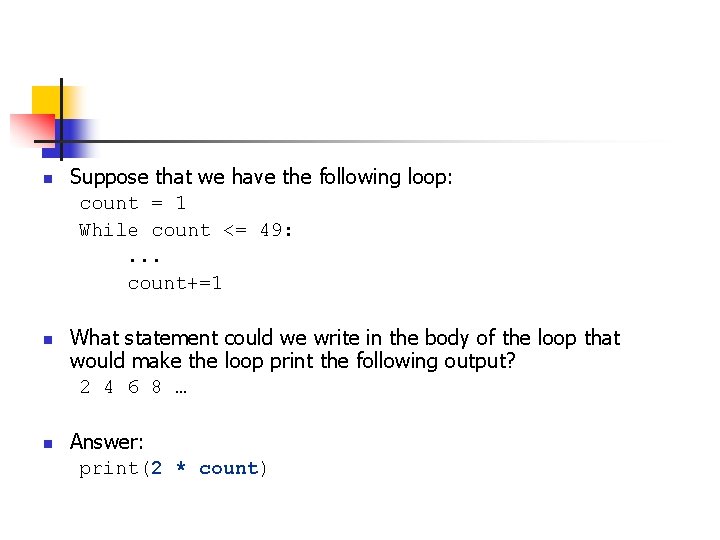
n n n Suppose that we have the following loop: count = 1 While count <= 49: . . . count+=1 What statement could we write in the body of the loop that would make the loop print the following output? 2 4 6 8 … Answer: print(2 * count)
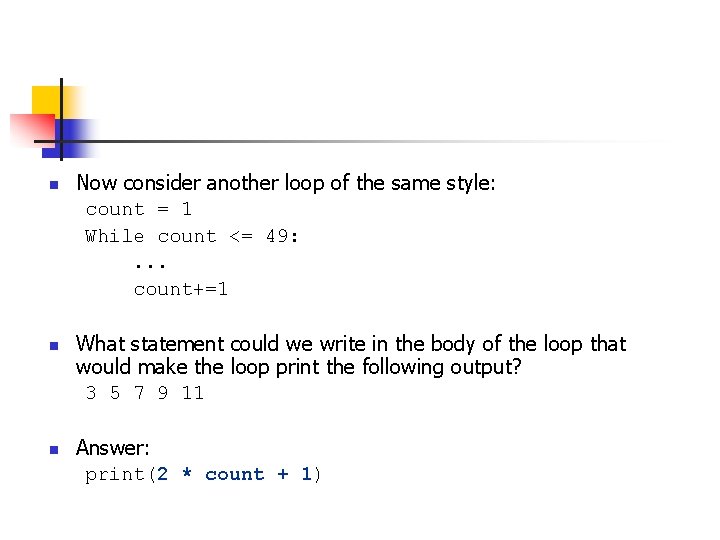
n n n Now consider another loop of the same style: count = 1 While count <= 49: . . . count+=1 What statement could we write in the body of the loop that would make the loop print the following output? 3 5 7 9 11 Answer: print(2 * count + 1)
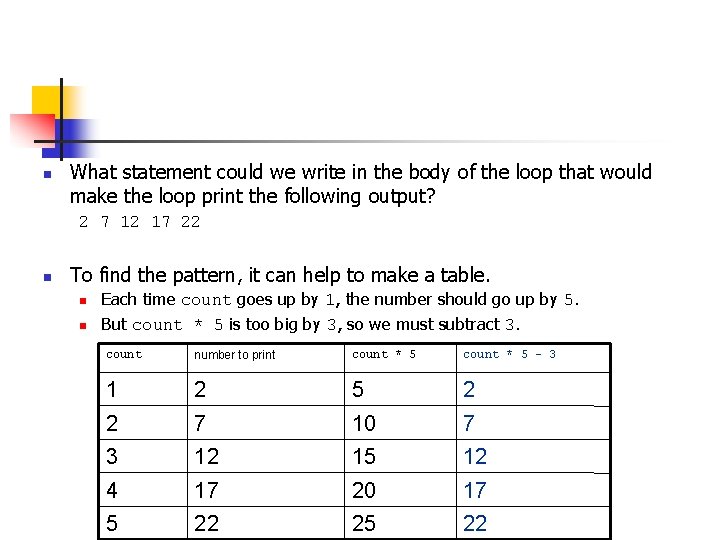
n What statement could we write in the body of the loop that would make the loop print the following output? 2 7 12 17 22 n To find the pattern, it can help to make a table. n n Each time count goes up by 1, the number should go up by 5. But count * 5 is too big by 3, so we must subtract 3. count number to print count * 5 - 3 1 2 5 2 2 7 10 7 3 12 15 12 4 17 20 17 5 22 25 22
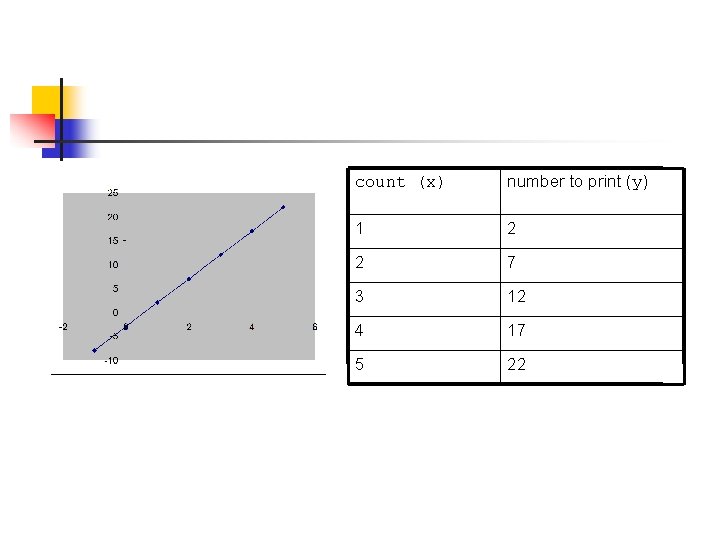
count (x) number to print (y) 1 2 2 7 3 12 4 17 5 22
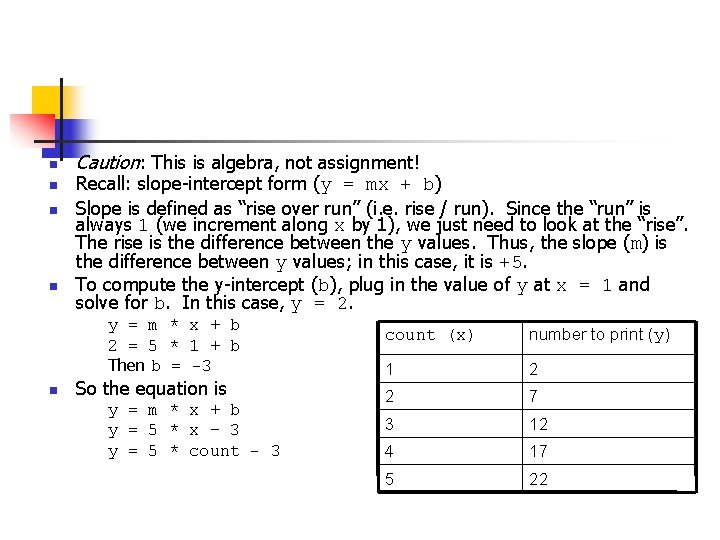
n n Caution: This is algebra, not assignment! Recall: slope-intercept form (y = mx + b) Slope is defined as “rise over run” (i. e. rise / run). Since the “run” is always 1 (we increment along x by 1), we just need to look at the “rise”. The rise is the difference between the y values. Thus, the slope (m) is the difference between y values; in this case, it is +5. To compute the y-intercept (b), plug in the value of y at x = 1 and solve for b. In this case, y = 2. y = m * x + b 2 = 5 * 1 + b Then b = -3 n So the equation is y = m * x + b y = 5 * x – 3 y = 5 * count - 3 count (x) number to print (y) 1 2 2 7 3 12 4 17 5 22
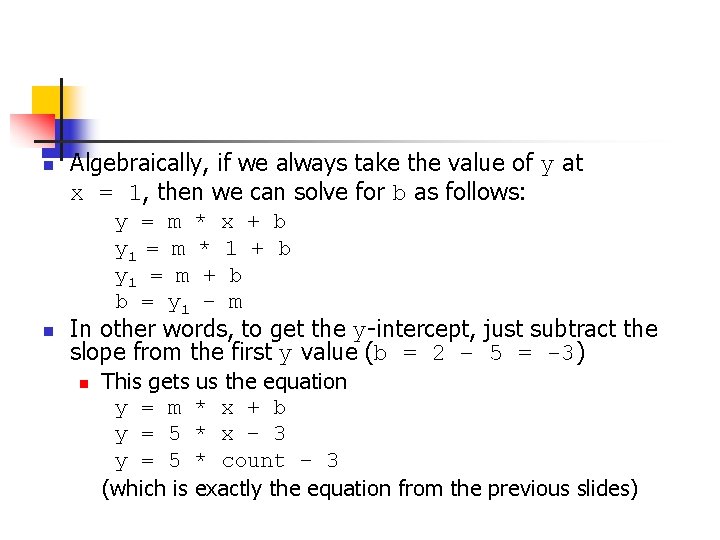
n Algebraically, if we always take the value of y at x = 1, then we can solve for b as follows: y = m * x + b y 1 = m * 1 + b y 1 = m + b b = y 1 – m n In other words, to get the y-intercept, just subtract the slope from the first y value (b = 2 – 5 = -3) n This gets us the equation y = m * x + b y = 5 * x – 3 y = 5 * count – 3 (which is exactly the equation from the previous slides)
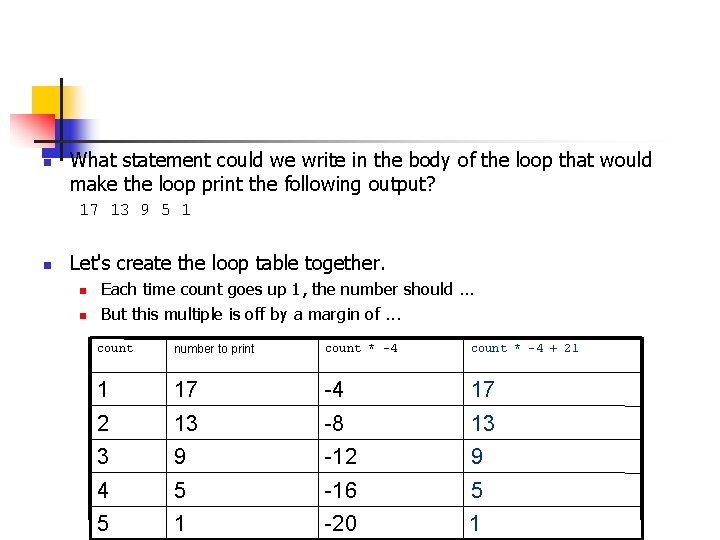
n What statement could we write in the body of the loop that would make the loop print the following output? 17 13 9 5 1 n Let's create the loop table together. n n Each time count goes up 1, the number should. . . But this multiple is off by a margin of. . . count number to print count * -4 + 21 1 17 -4 17 2 13 -8 13 3 9 -12 9 4 5 -16 5 5 1 -20 1
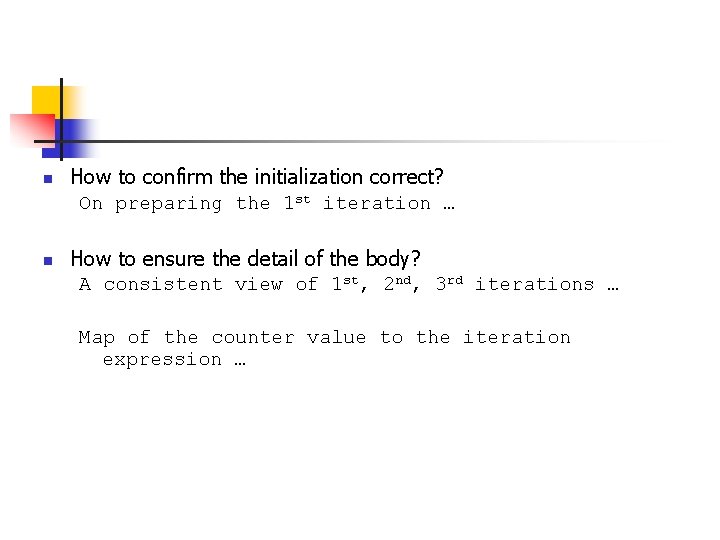
n n How to confirm the initialization correct? On preparing the 1 st iteration … How to ensure the detail of the body? A consistent view of 1 st, 2 nd, 3 rd iterations … Map of the counter value to the iteration expression …
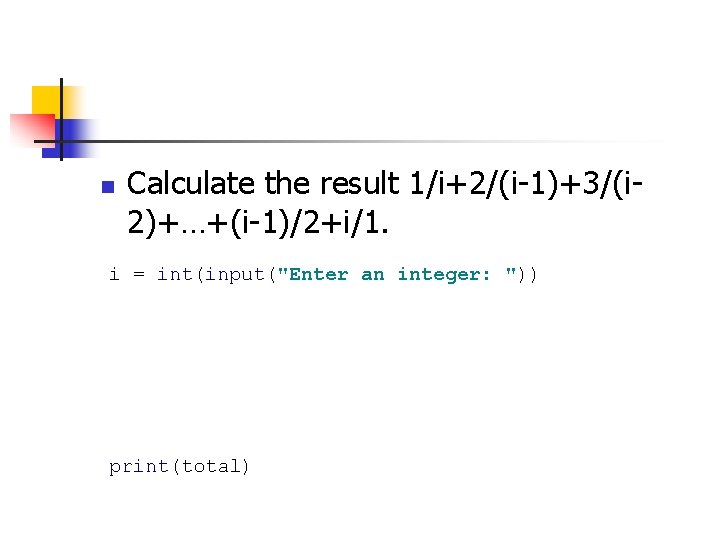
n Calculate the result 1/i+2/(i-1)+3/(i 2)+…+(i-1)/2+i/1. i = int(input("Enter an integer: ")) print(total)
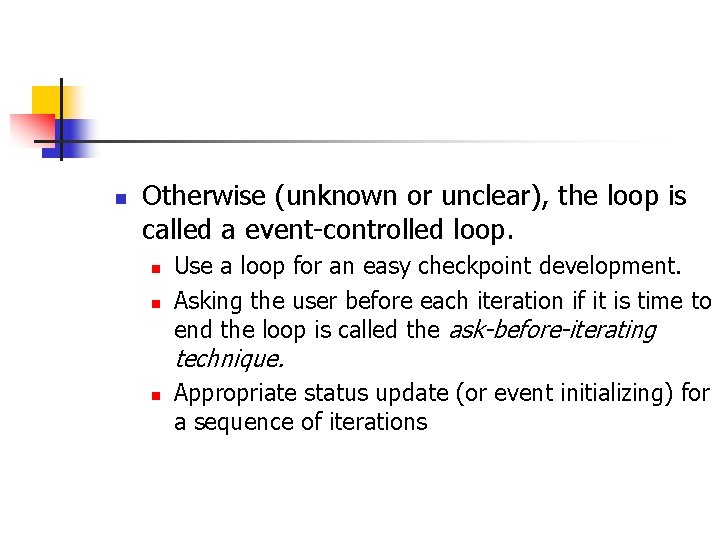
n Otherwise (unknown or unclear), the loop is called a event-controlled loop. n n Use a loop for an easy checkpoint development. Asking the user before each iteration if it is time to end the loop is called the ask-before-iterating technique. n Appropriate status update (or event initializing) for a sequence of iterations
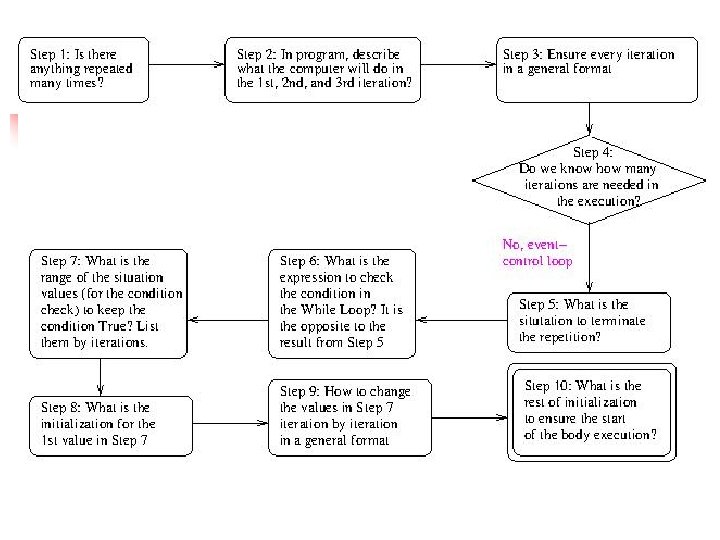
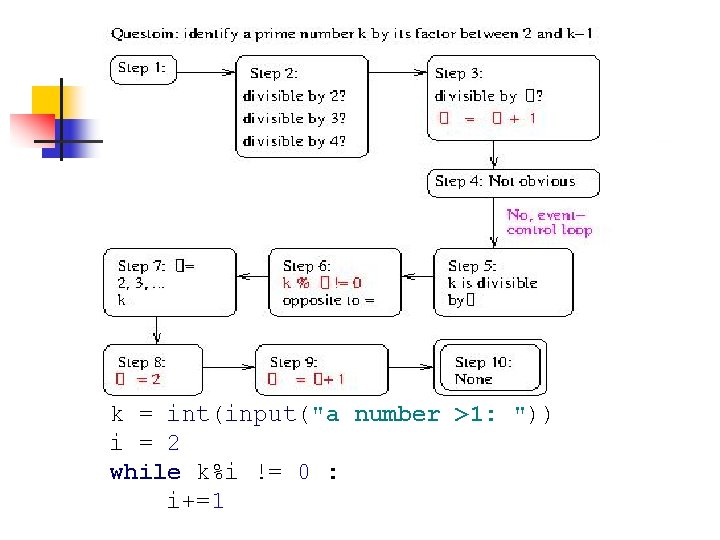
k = int(input("a number >1: ")) i = 2 while k%i != 0 : i+=1
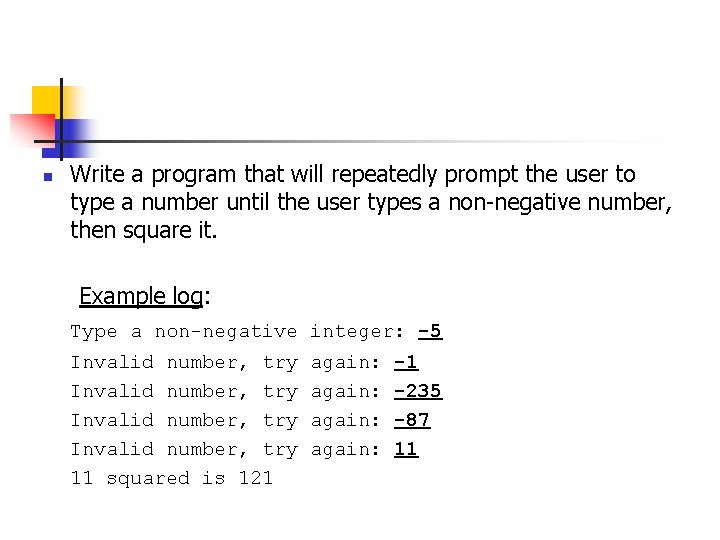
n Write a program that will repeatedly prompt the user to type a number until the user types a non-negative number, then square it. Example log: Type a non-negative integer: -5 Invalid number, try 11 squared is 121 again: -1 -235 -87 11
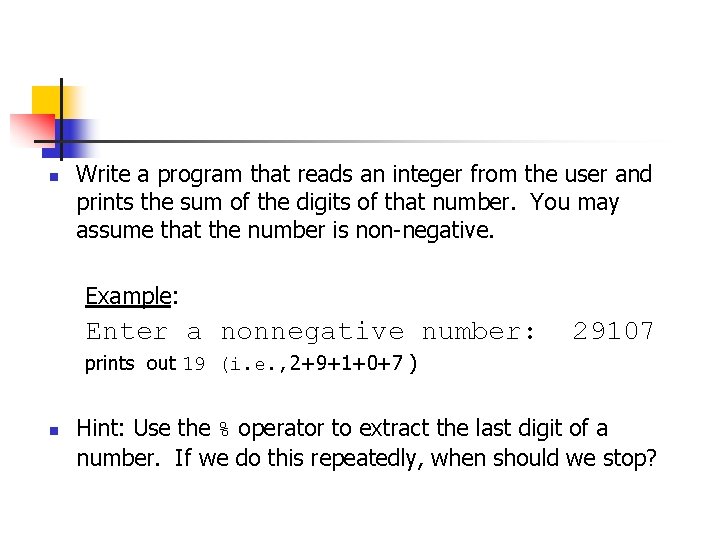
n Write a program that reads an integer from the user and prints the sum of the digits of that number. You may assume that the number is non-negative. Example: Enter a nonnegative number: 29107 prints out 19 (i. e. , 2+9+1+0+7 ) n Hint: Use the % operator to extract the last digit of a number. If we do this repeatedly, when should we stop?
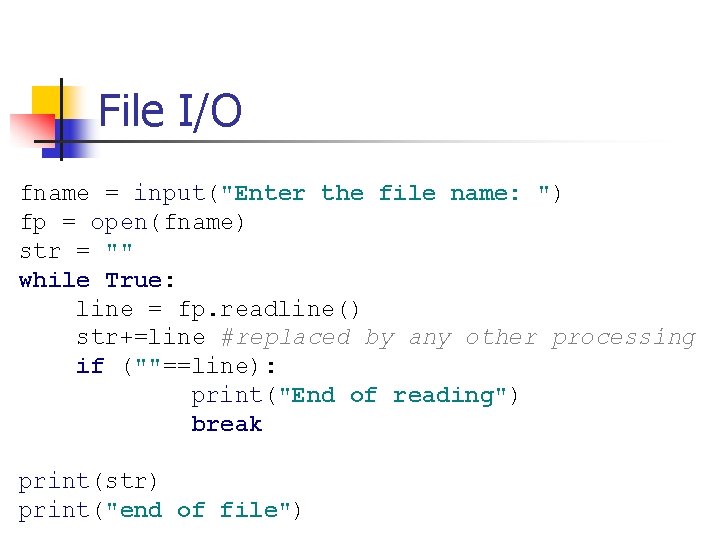
File I/O fname = input("Enter the file name: ") fp = open(fname) str = "" while True: line = fp. readline() str+=line #replaced by any other processing if (""==line): print("End of reading") break print(str) print("end of file")
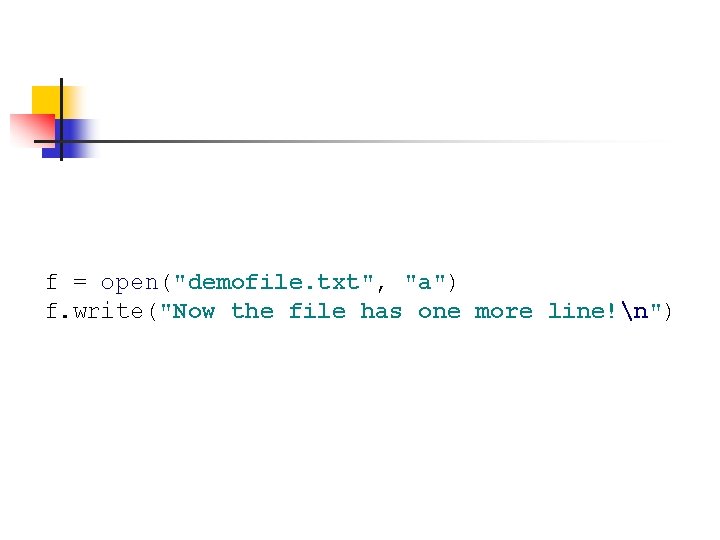
f = open("demofile. txt", "a") f. write("Now the file has one more line!n")
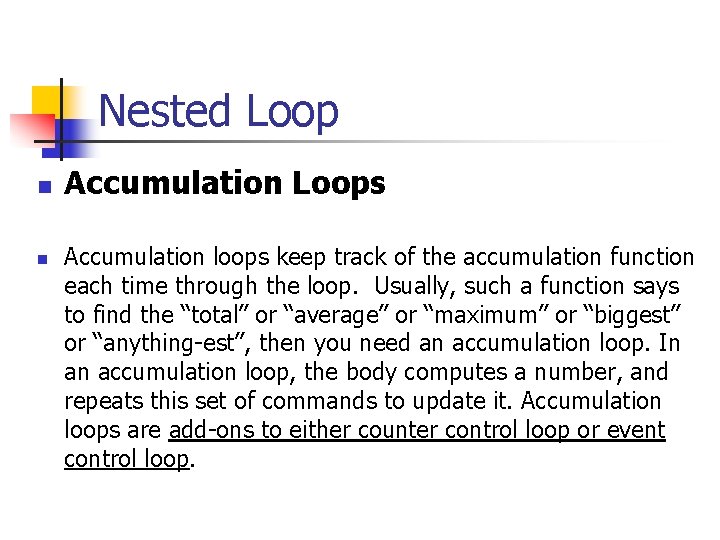
Nested Loop n n Accumulation Loops Accumulation loops keep track of the accumulation function each time through the loop. Usually, such a function says to find the “total” or “average” or “maximum” or “biggest” or “anything-est”, then you need an accumulation loop. In an accumulation loop, the body computes a number, and repeats this set of commands to update it. Accumulation loops are add-ons to either counter control loop or event control loop.
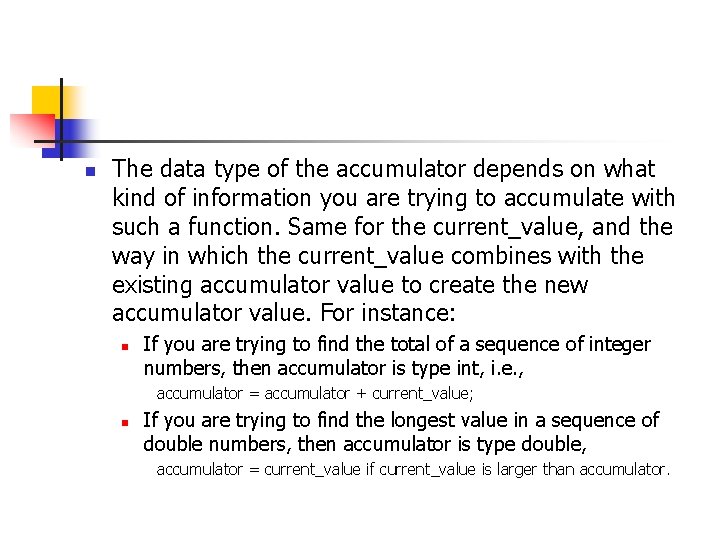
n The data type of the accumulator depends on what kind of information you are trying to accumulate with such a function. Same for the current_value, and the way in which the current_value combines with the existing accumulator value to create the new accumulator value. For instance: n If you are trying to find the total of a sequence of integer numbers, then accumulator is type int, i. e. , accumulator = accumulator + current_value; n If you are trying to find the longest value in a sequence of double numbers, then accumulator is type double, accumulator = current_value if current_value is larger than accumulator.
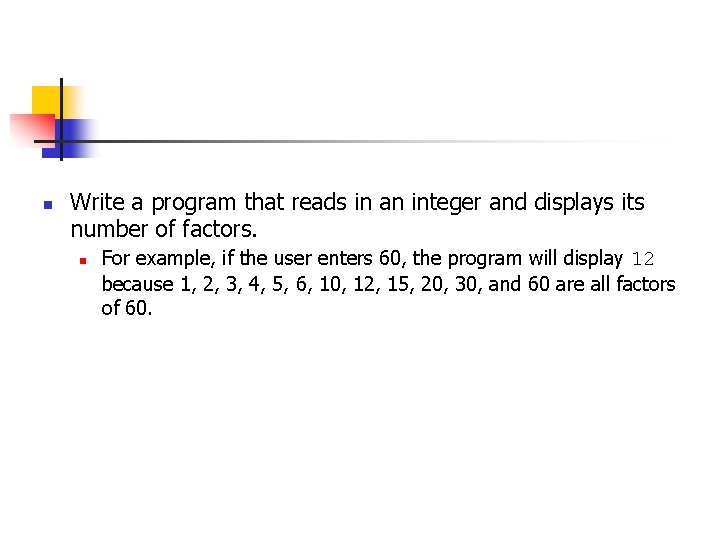
n Write a program that reads in an integer and displays its number of factors. n For example, if the user enters 60, the program will display 12 because 1, 2, 3, 4, 5, 6, 10, 12, 15, 20, 30, and 60 are all factors of 60.
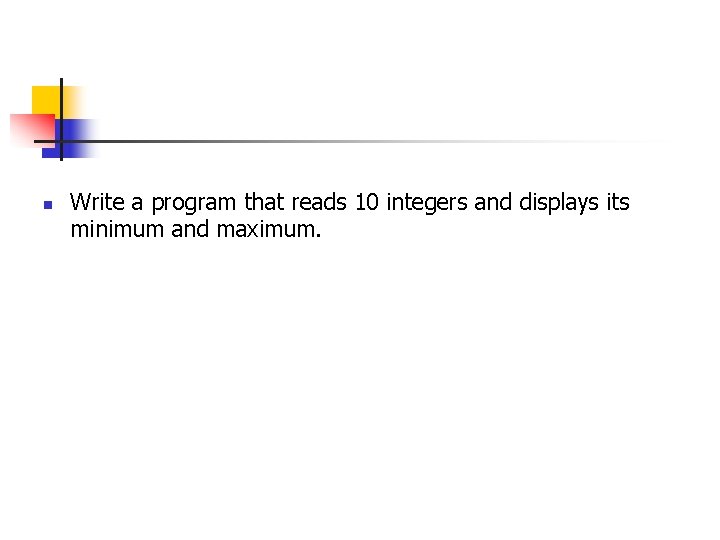
n Write a program that reads 10 integers and displays its minimum and maximum.
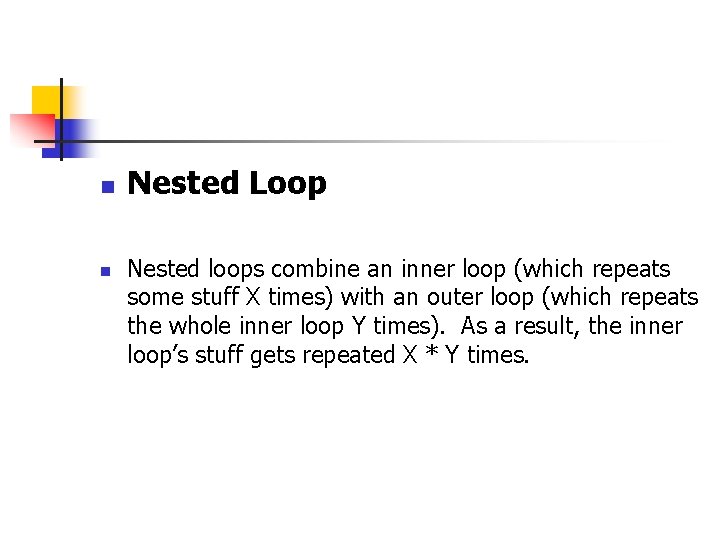
n n Nested Loop Nested loops combine an inner loop (which repeats some stuff X times) with an outer loop (which repeats the whole inner loop Y times). As a result, the inner loop’s stuff gets repeated X * Y times.
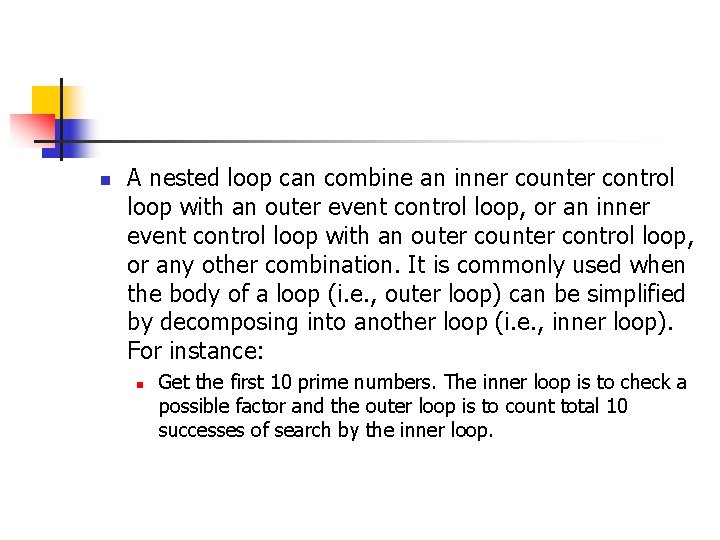
n A nested loop can combine an inner counter control loop with an outer event control loop, or an inner event control loop with an outer counter control loop, or any other combination. It is commonly used when the body of a loop (i. e. , outer loop) can be simplified by decomposing into another loop (i. e. , inner loop). For instance: n Get the first 10 prime numbers. The inner loop is to check a possible factor and the outer loop is to count total 10 successes of search by the inner loop.
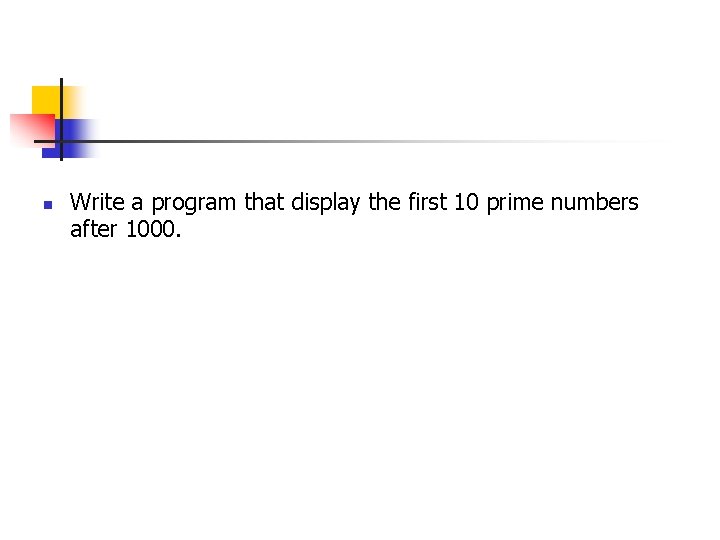
n Write a program that display the first 10 prime numbers after 1000.
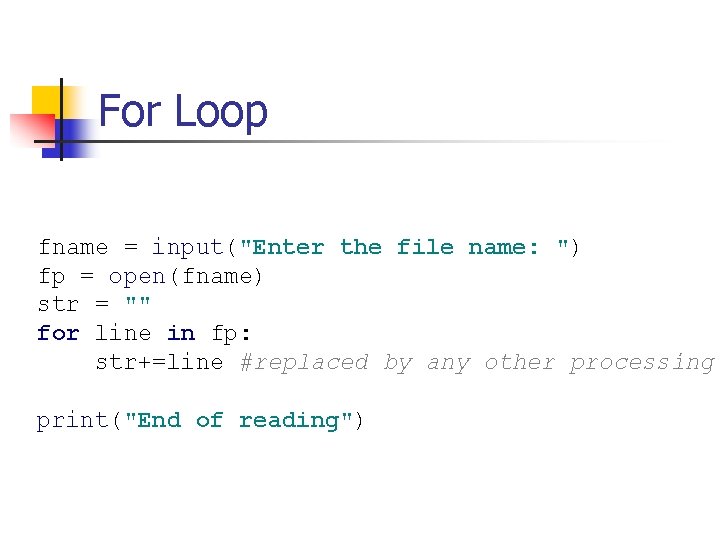
For Loop fname = input("Enter the file name: ") fp = open(fname) str = "" for line in fp: str+=line #replaced by any other processing print("End of reading")
![fruits apple banana cherry for x in fruits printx fruits = ["apple", "banana", "cherry"] for x in fruits: print(x)](https://slidetodoc.com/presentation_image_h/ca95f41373ce6ea331cc5d7eea4dc7ad/image-42.jpg)
fruits = ["apple", "banana", "cherry"] for x in fruits: print(x)