CS1001 Structured Programming Approach Pointers in C programming
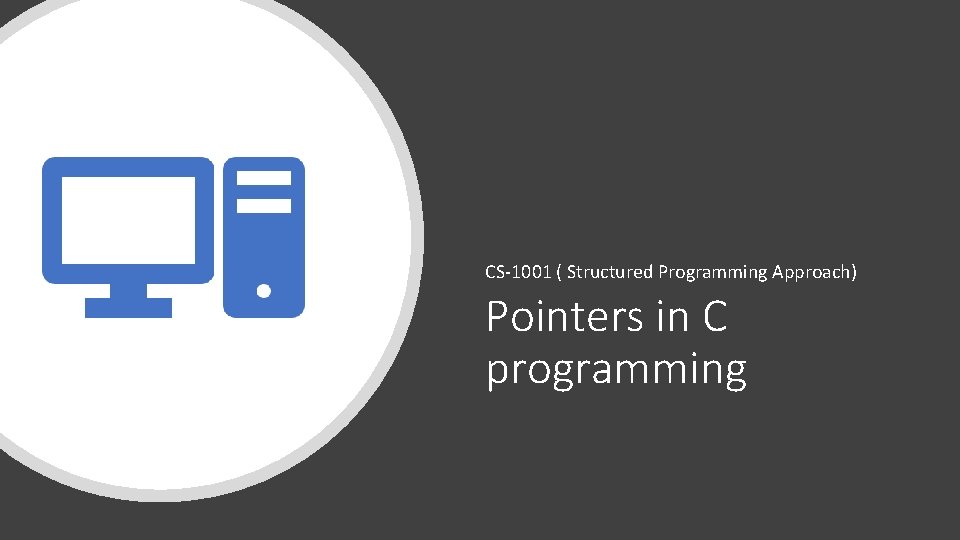
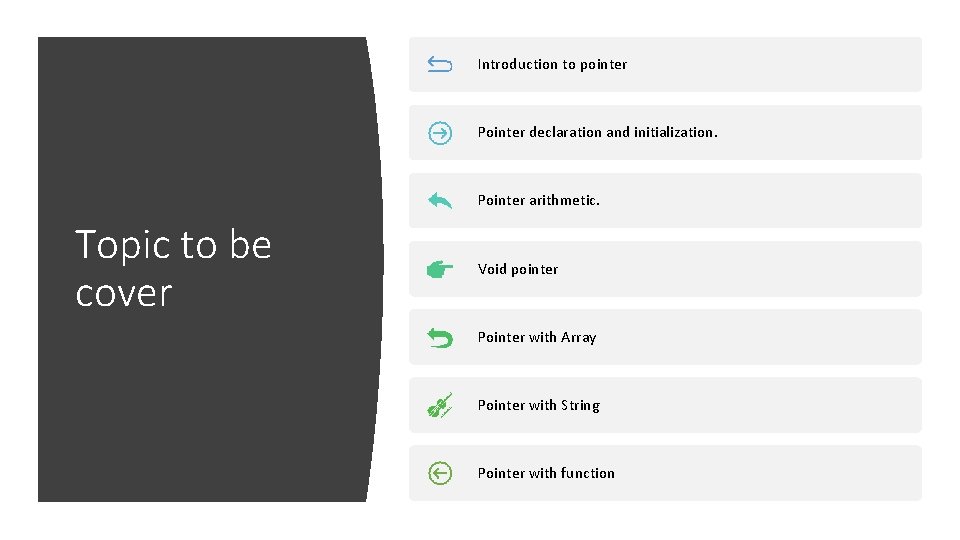
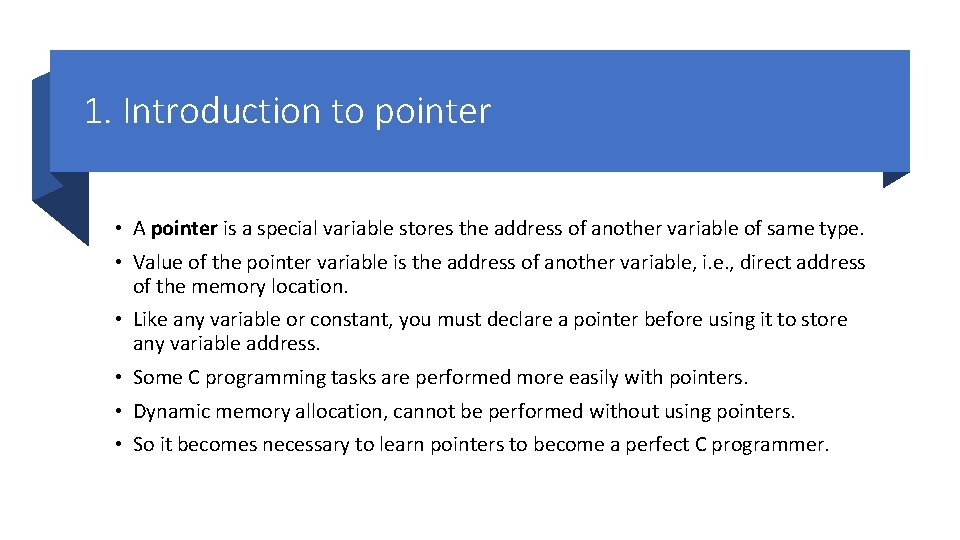
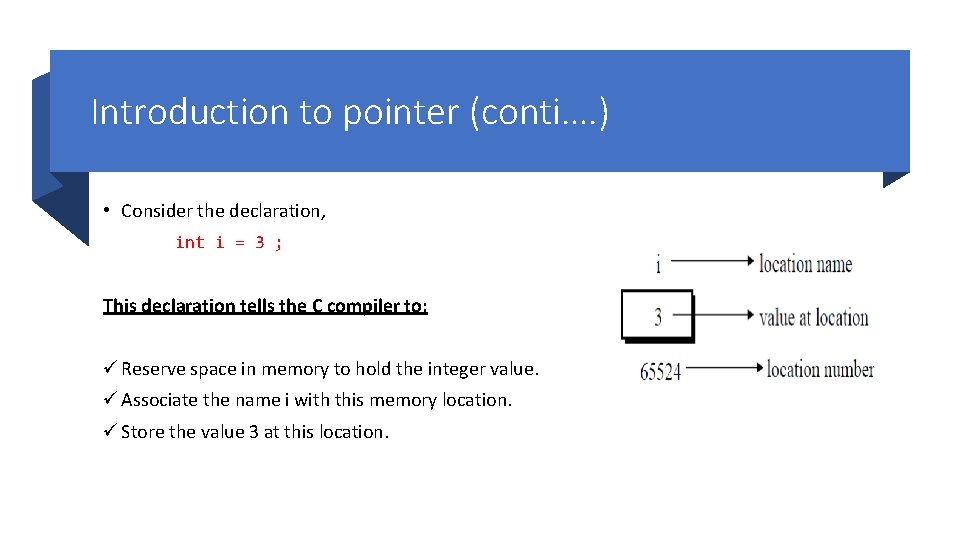
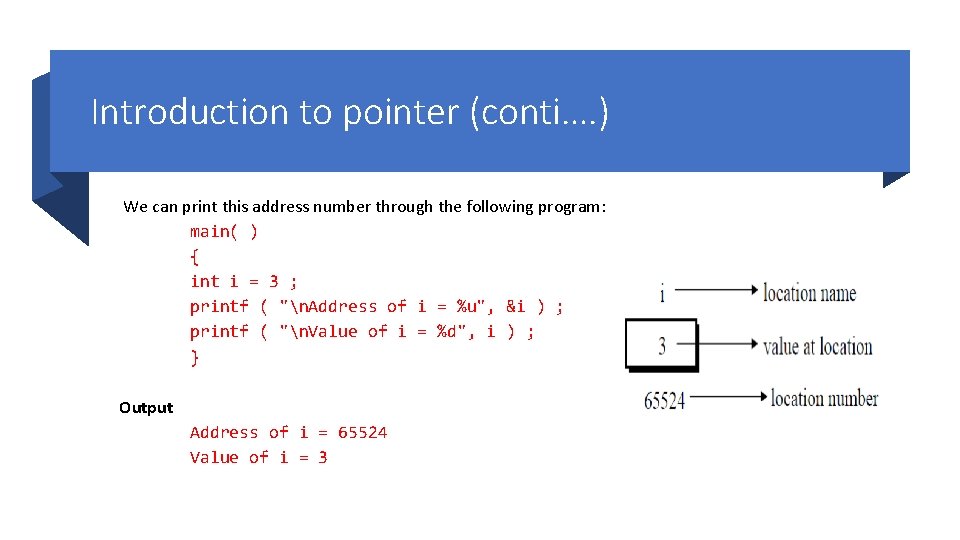
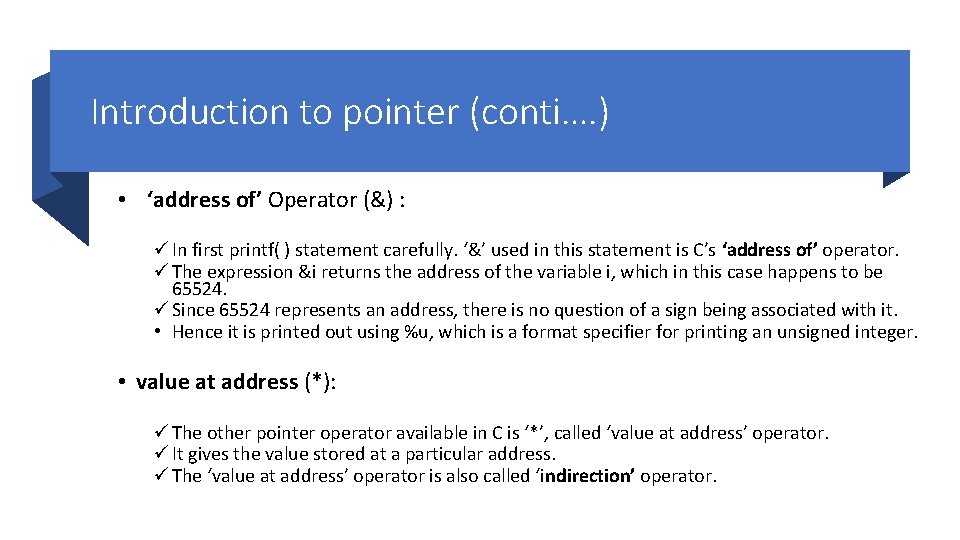
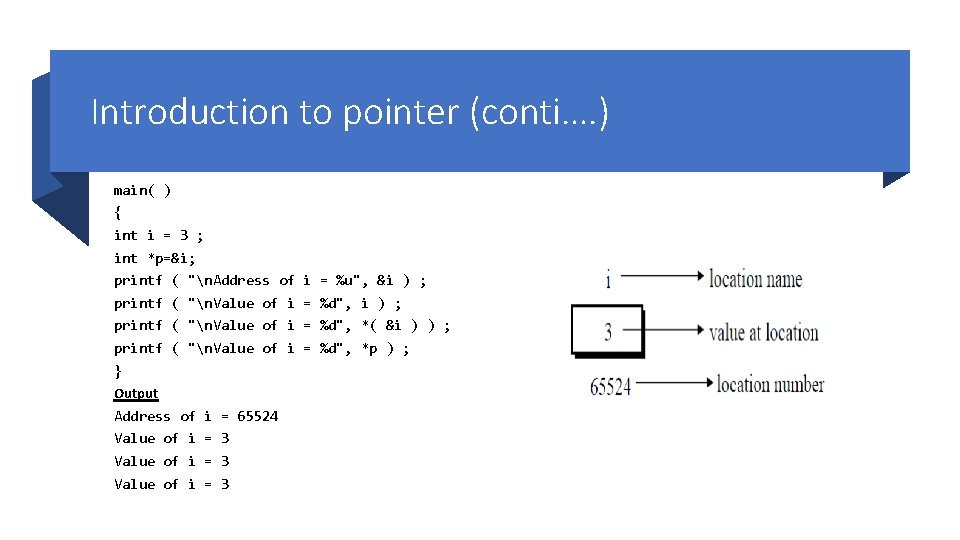
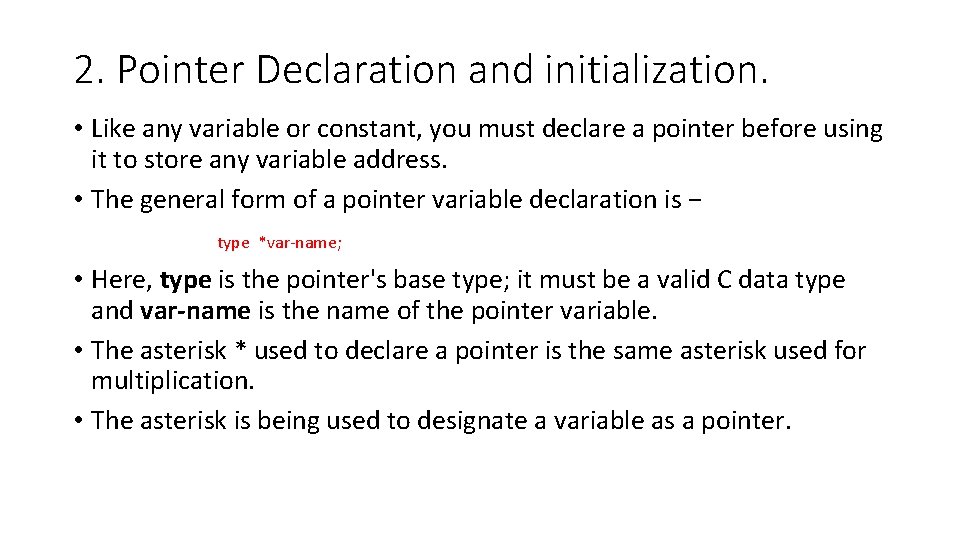
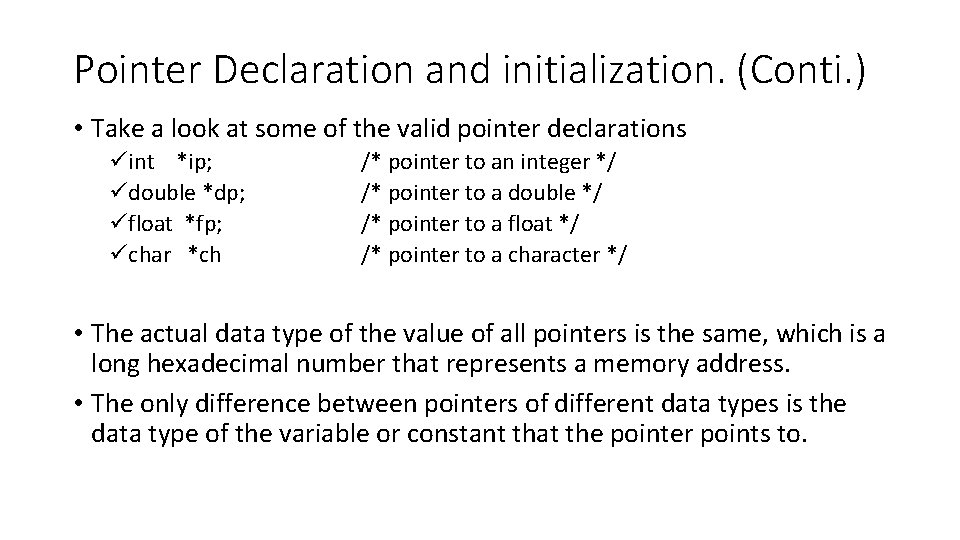
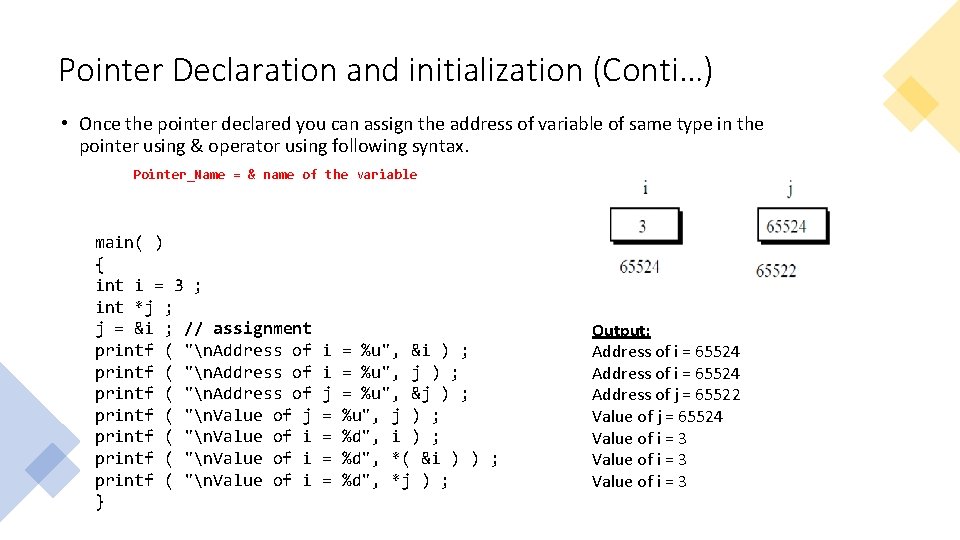
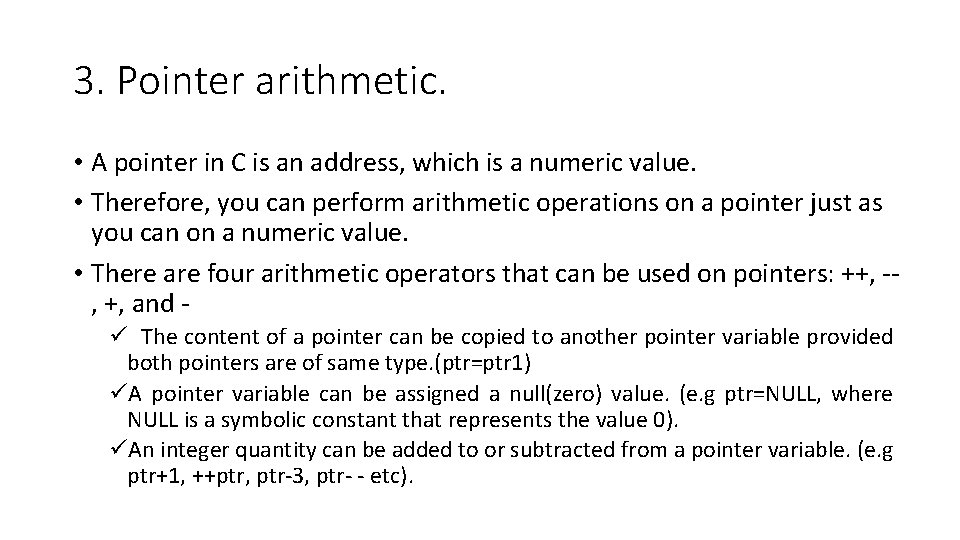
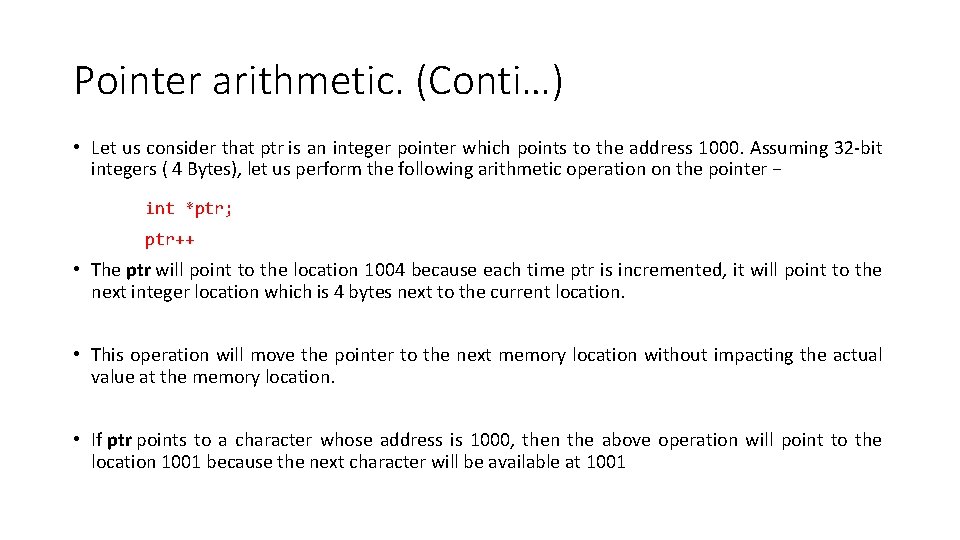
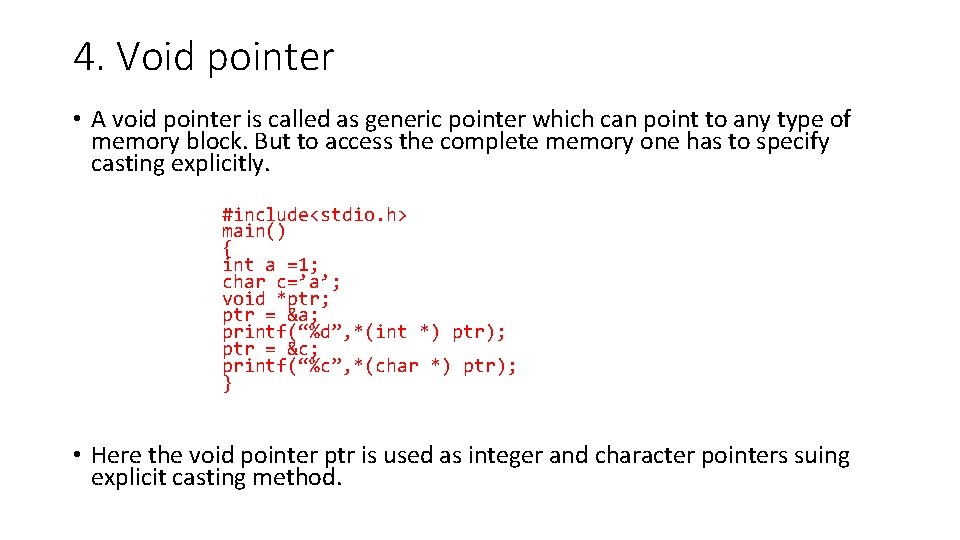
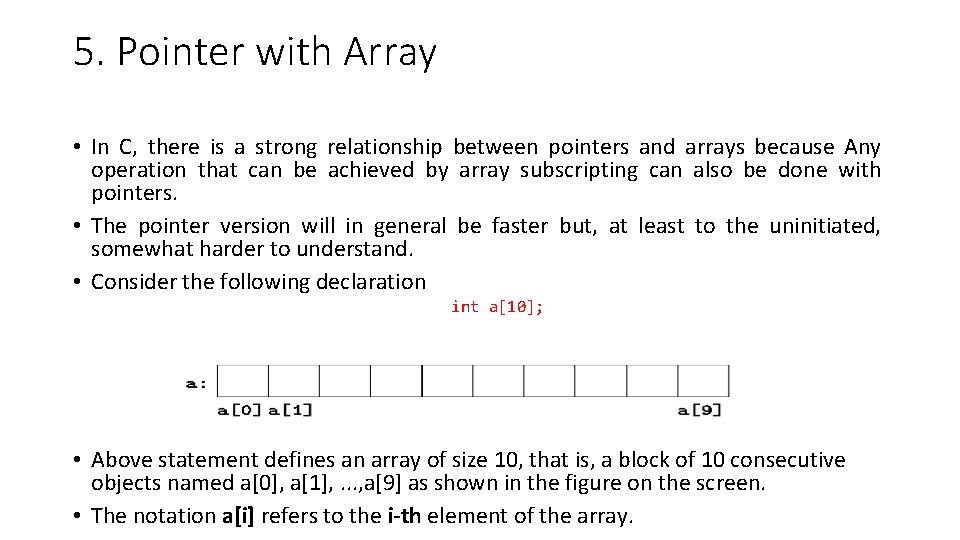
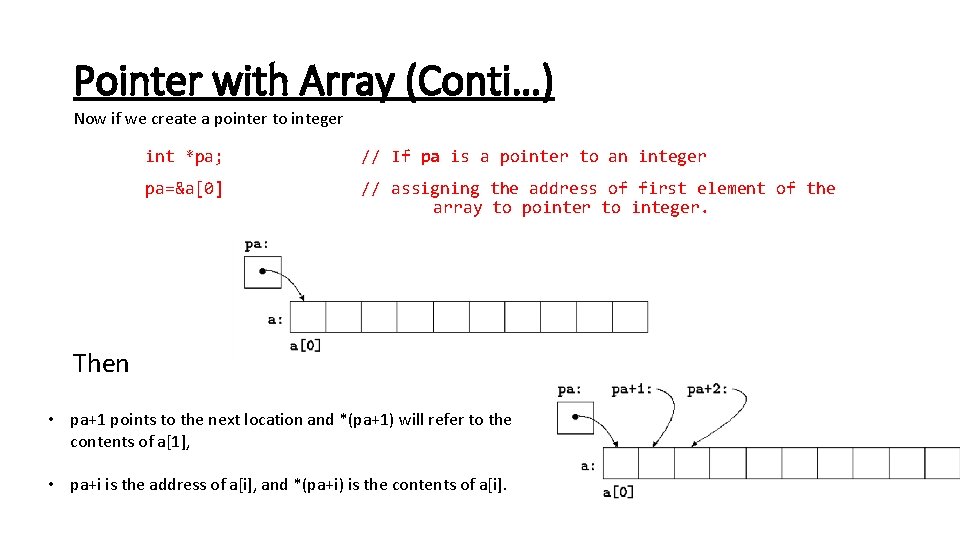
![Pointer with Array (Conti…) Program #include <stdio. h> int main() { int x[5] = Pointer with Array (Conti…) Program #include <stdio. h> int main() { int x[5] =](https://slidetodoc.com/presentation_image_h2/5dbe86eebfd1717cf3d7cc9009b78f2b/image-16.jpg)
![6. Pointer with String #include <stdio. h> int main(void) { char str[6] = "Hello"; 6. Pointer with String #include <stdio. h> int main(void) { char str[6] = "Hello";](https://slidetodoc.com/presentation_image_h2/5dbe86eebfd1717cf3d7cc9009b78f2b/image-17.jpg)
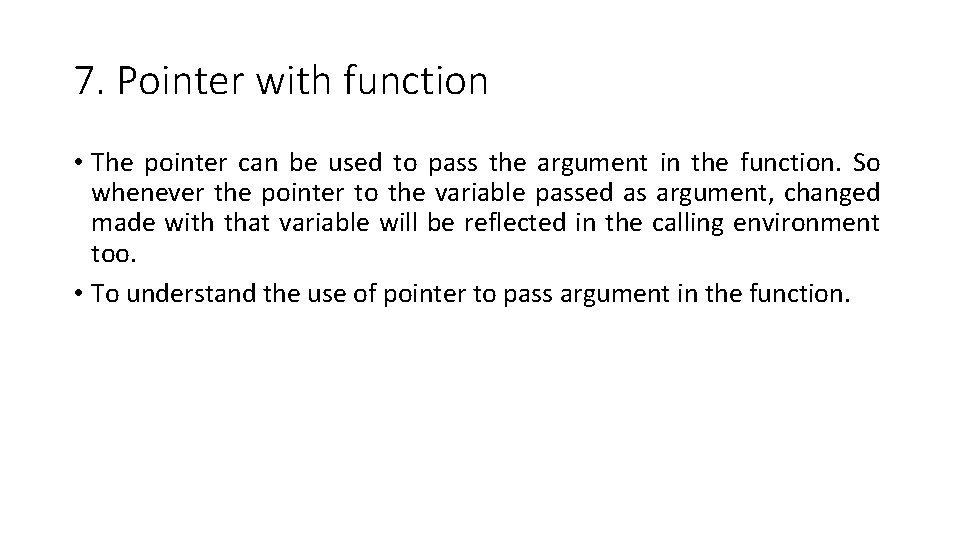
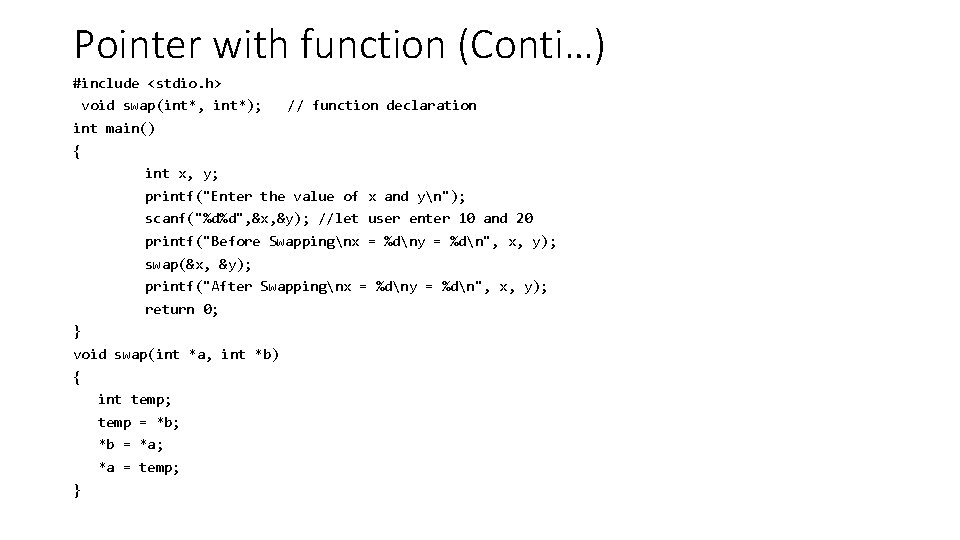
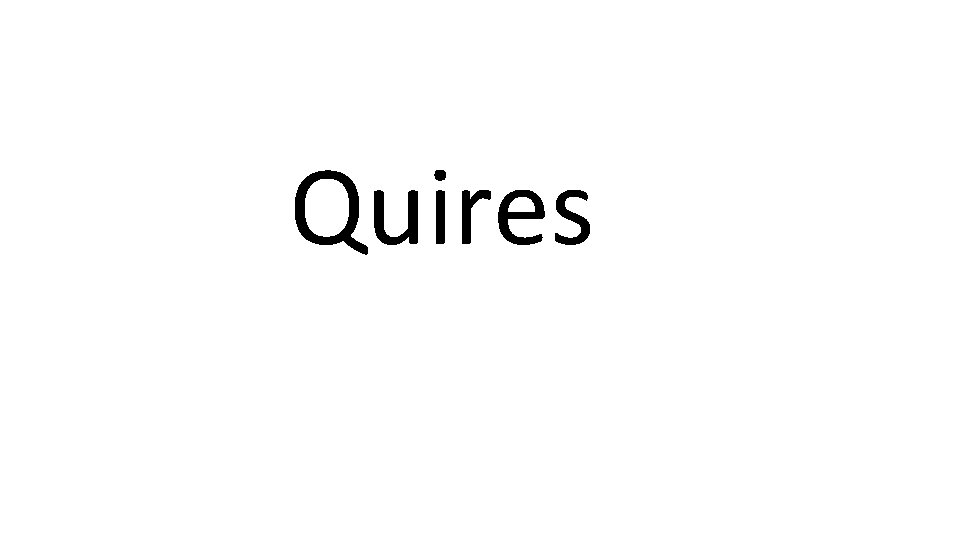
- Slides: 20
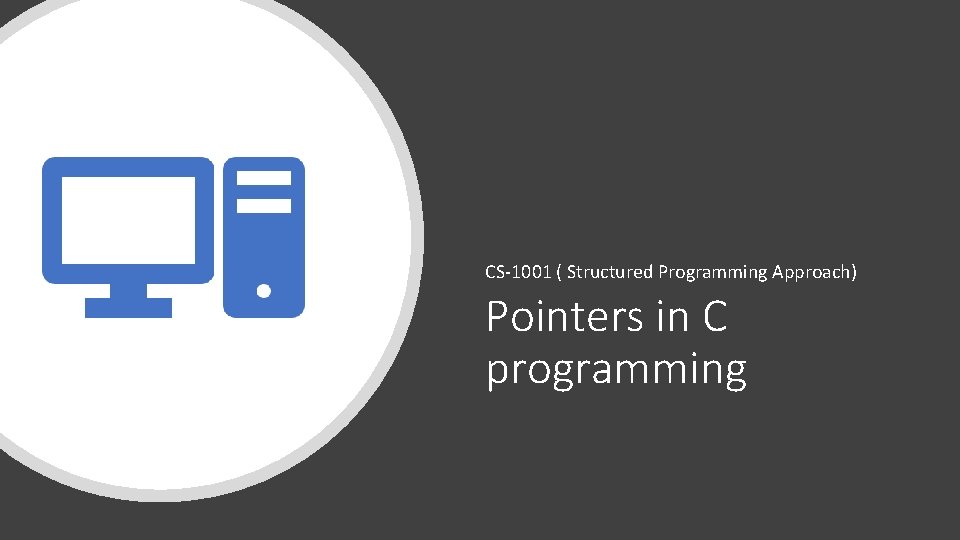
CS-1001 ( Structured Programming Approach) Pointers in C programming
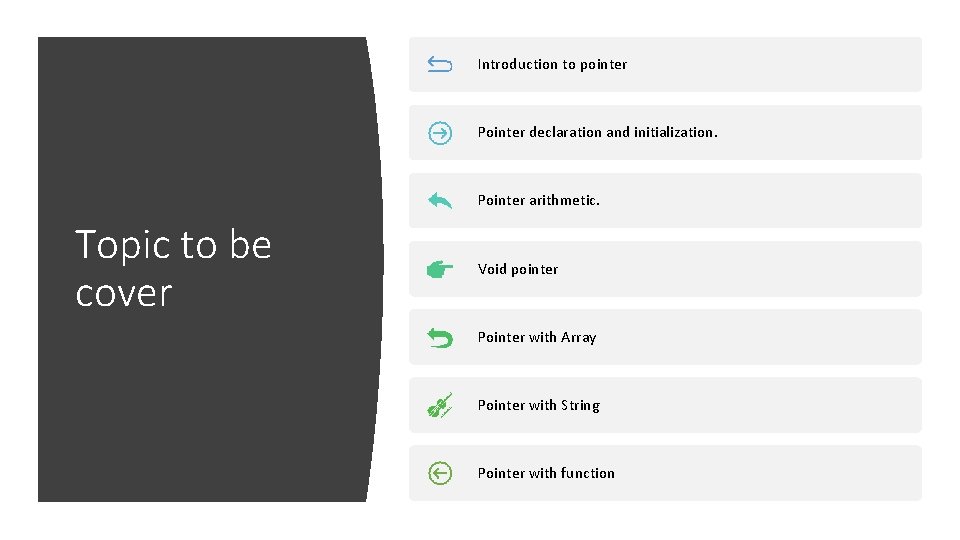
Introduction to pointer Pointer declaration and initialization. Pointer arithmetic. Topic to be cover Void pointer Pointer with Array Pointer with String Pointer with function
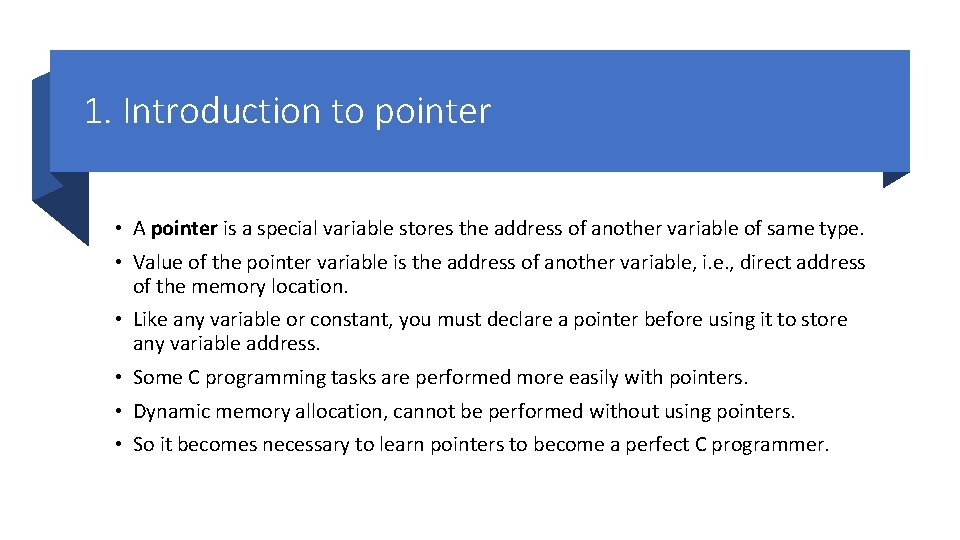
1. Introduction to pointer • A pointer is a special variable stores the address of another variable of same type. • Value of the pointer variable is the address of another variable, i. e. , direct address of the memory location. • Like any variable or constant, you must declare a pointer before using it to store any variable address. • Some C programming tasks are performed more easily with pointers. • Dynamic memory allocation, cannot be performed without using pointers. • So it becomes necessary to learn pointers to become a perfect C programmer.
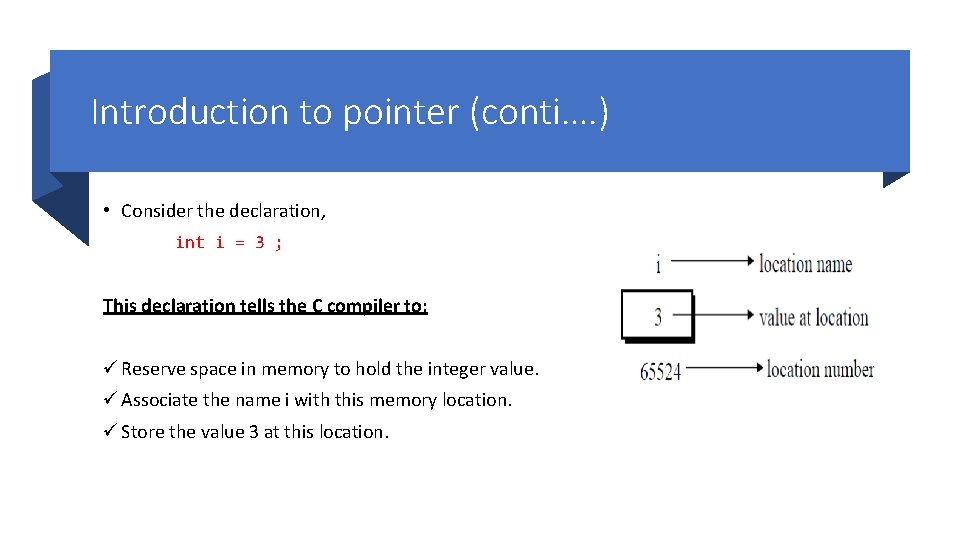
Introduction to pointer (conti…. ) • Consider the declaration, int i = 3 ; This declaration tells the C compiler to: ü Reserve space in memory to hold the integer value. ü Associate the name i with this memory location. ü Store the value 3 at this location.
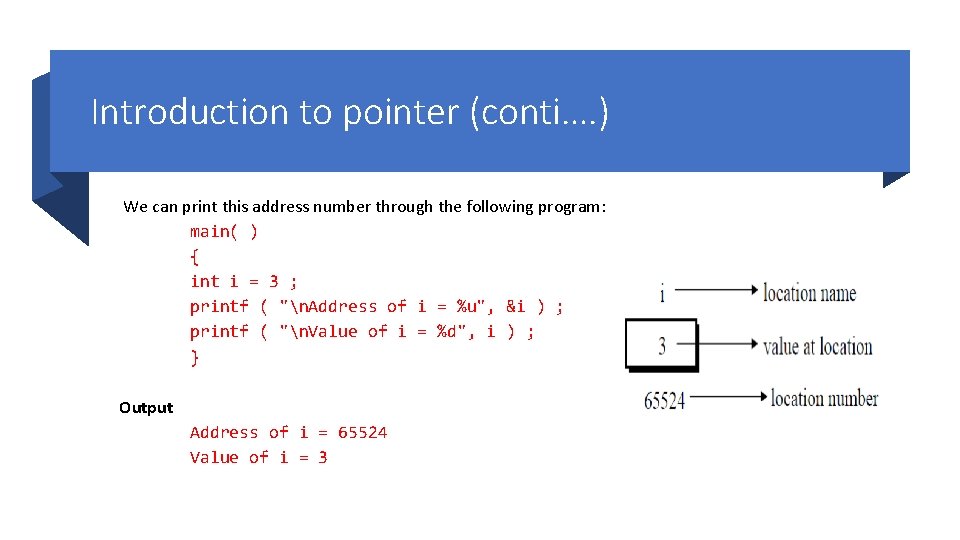
Introduction to pointer (conti…. ) We can print this address number through the following program: main( ) { int i = 3 ; printf ( "n. Address of i = %u", &i ) ; printf ( "n. Value of i = %d", i ) ; } Output Address of i = 65524 Value of i = 3
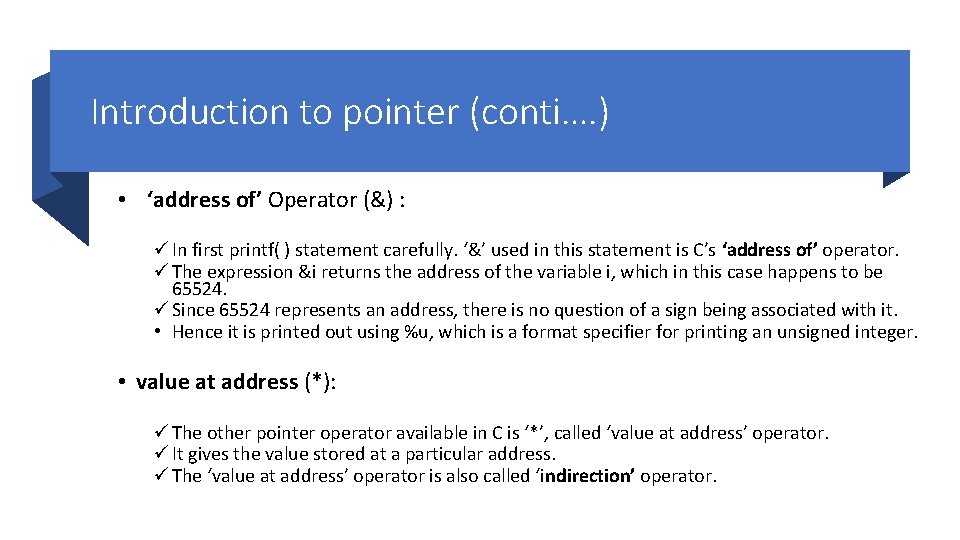
Introduction to pointer (conti…. ) • ‘address of’ Operator (&) : ü In first printf( ) statement carefully. ‘&’ used in this statement is C’s ‘address of’ operator. ü The expression &i returns the address of the variable i, which in this case happens to be 65524. ü Since 65524 represents an address, there is no question of a sign being associated with it. • Hence it is printed out using %u, which is a format specifier for printing an unsigned integer. • value at address (*): ü The other pointer operator available in C is ‘*’, called ‘value at address’ operator. ü It gives the value stored at a particular address. ü The ‘value at address’ operator is also called ‘indirection’ operator.
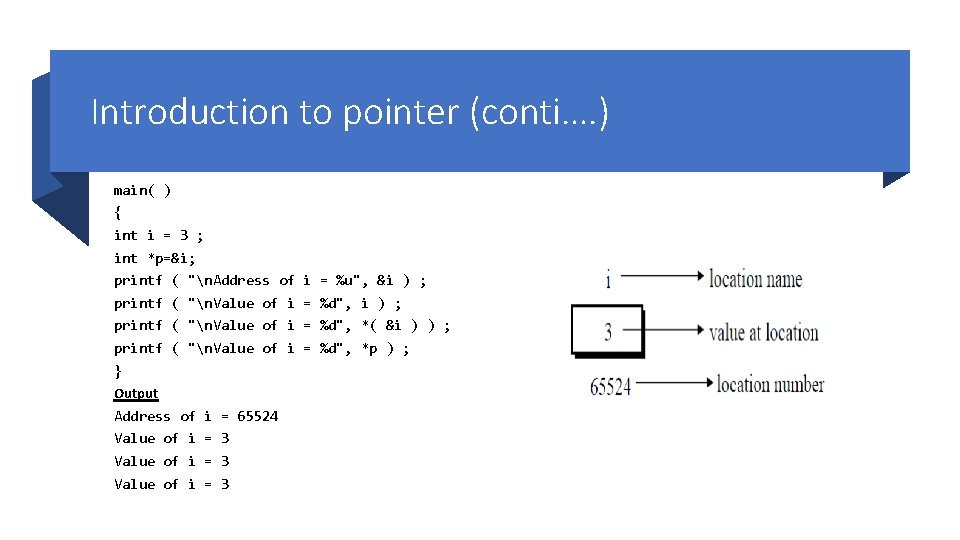
Introduction to pointer (conti…. ) main( ) { int i = 3 ; int *p=&i; printf ( "n. Address of i = %u", &i ) ; printf ( "n. Value of i = %d", *( &i ) ) ; printf ( "n. Value of i = %d", *p ) ; } Output Address of i = 65524 Value of i = 3
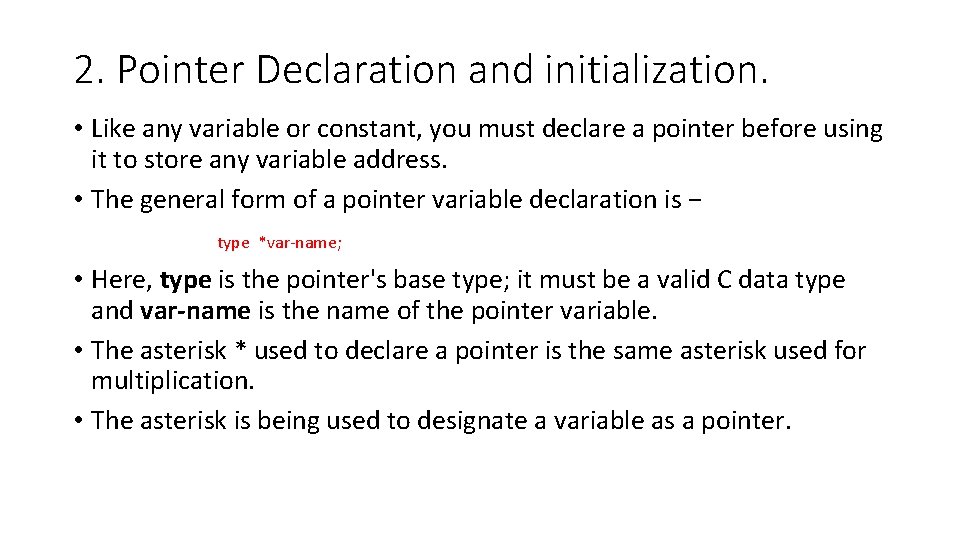
2. Pointer Declaration and initialization. • Like any variable or constant, you must declare a pointer before using it to store any variable address. • The general form of a pointer variable declaration is − type *var-name; • Here, type is the pointer's base type; it must be a valid C data type and var-name is the name of the pointer variable. • The asterisk * used to declare a pointer is the same asterisk used for multiplication. • The asterisk is being used to designate a variable as a pointer.
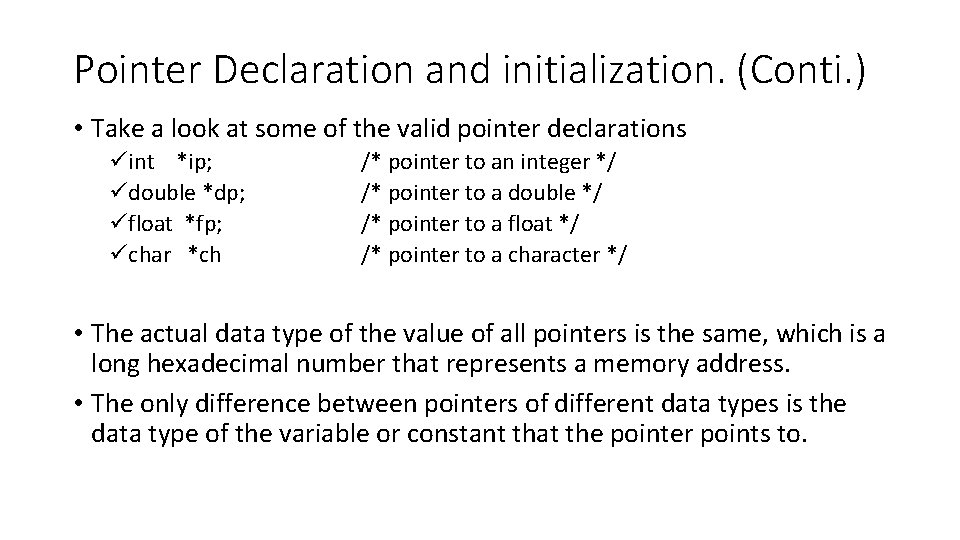
Pointer Declaration and initialization. (Conti. ) • Take a look at some of the valid pointer declarations üint *ip; üdouble *dp; üfloat *fp; üchar *ch /* pointer to an integer */ /* pointer to a double */ /* pointer to a float */ /* pointer to a character */ • The actual data type of the value of all pointers is the same, which is a long hexadecimal number that represents a memory address. • The only difference between pointers of different data types is the data type of the variable or constant that the pointer points to.
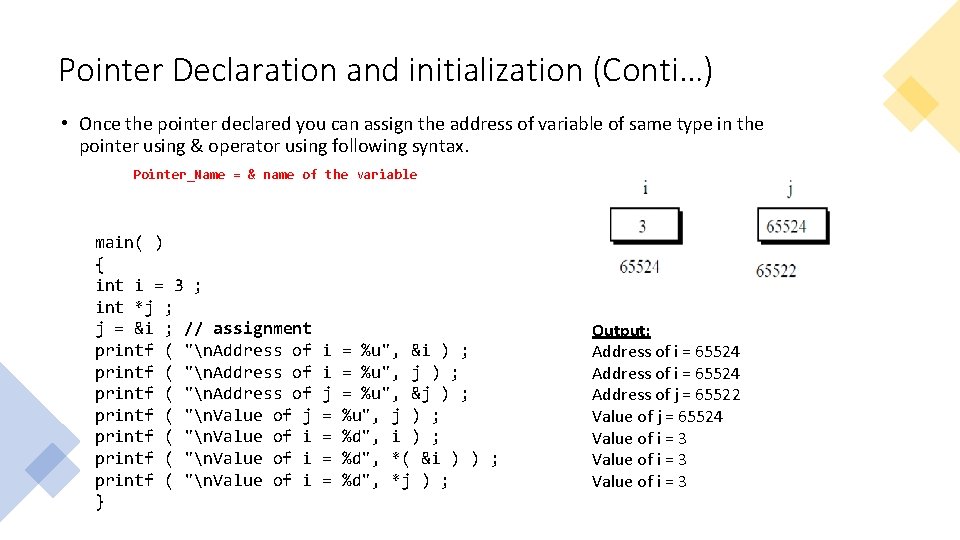
Pointer Declaration and initialization (Conti…) • Once the pointer declared you can assign the address of variable of same type in the pointer using & operator using following syntax. Pointer_Name = & name of the variable main( ) { int i = 3 ; int *j ; j = &i ; // assignment printf ( "n. Address of printf ( "n. Value of j printf ( "n. Value of i } i i j = = = %u", &i ) ; = %u", j ) ; = %u", &j ) ; %u", j ) ; %d", i ) ; %d", *( &i ) ) ; %d", *j ) ; Output: Address of i = 65524 Address of j = 65522 Value of j = 65524 Value of i = 3
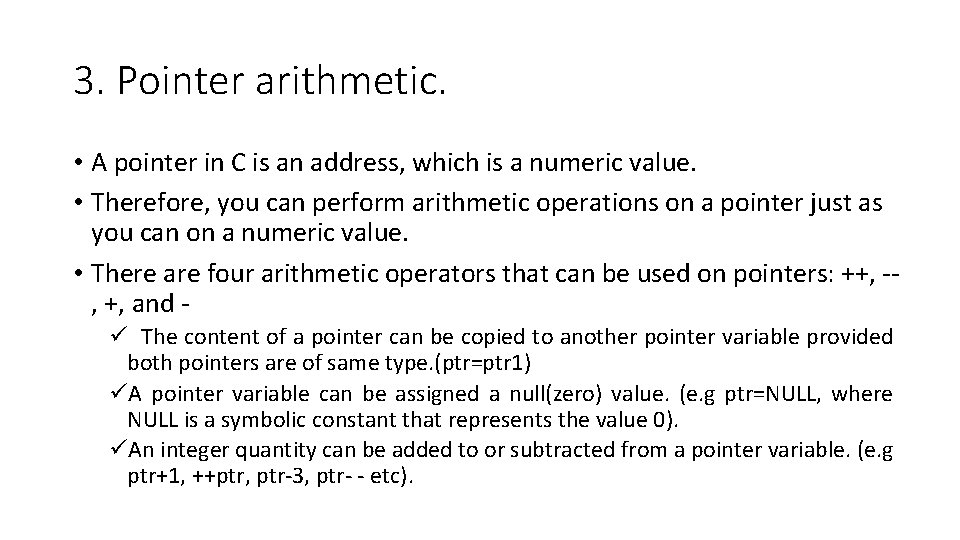
3. Pointer arithmetic. • A pointer in C is an address, which is a numeric value. • Therefore, you can perform arithmetic operations on a pointer just as you can on a numeric value. • There are four arithmetic operators that can be used on pointers: ++, -, +, and ü The content of a pointer can be copied to another pointer variable provided both pointers are of same type. (ptr=ptr 1) üA pointer variable can be assigned a null(zero) value. (e. g ptr=NULL, where NULL is a symbolic constant that represents the value 0). üAn integer quantity can be added to or subtracted from a pointer variable. (e. g ptr+1, ++ptr, ptr-3, ptr- - etc).
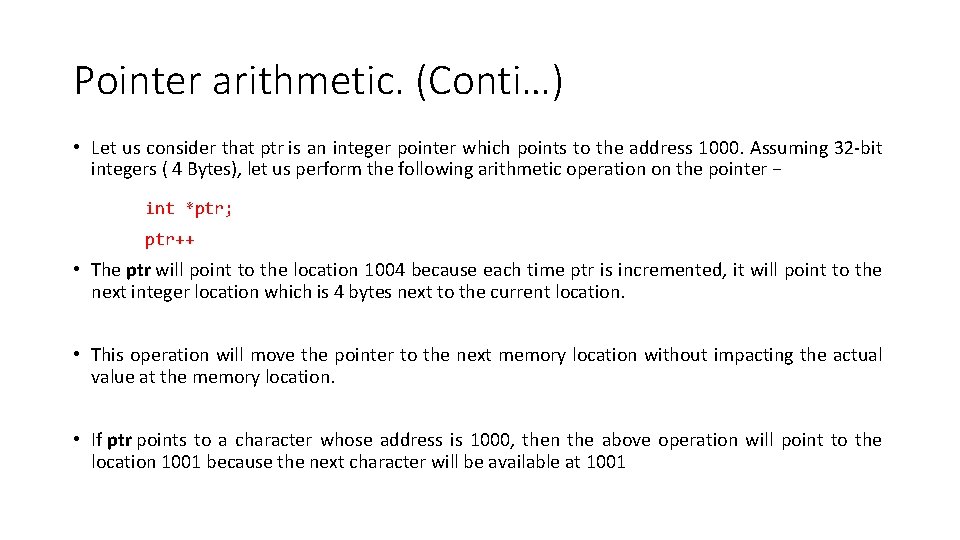
Pointer arithmetic. (Conti…) • Let us consider that ptr is an integer pointer which points to the address 1000. Assuming 32 -bit integers ( 4 Bytes), let us perform the following arithmetic operation on the pointer − int *ptr; ptr++ • The ptr will point to the location 1004 because each time ptr is incremented, it will point to the next integer location which is 4 bytes next to the current location. • This operation will move the pointer to the next memory location without impacting the actual value at the memory location. • If ptr points to a character whose address is 1000, then the above operation will point to the location 1001 because the next character will be available at 1001
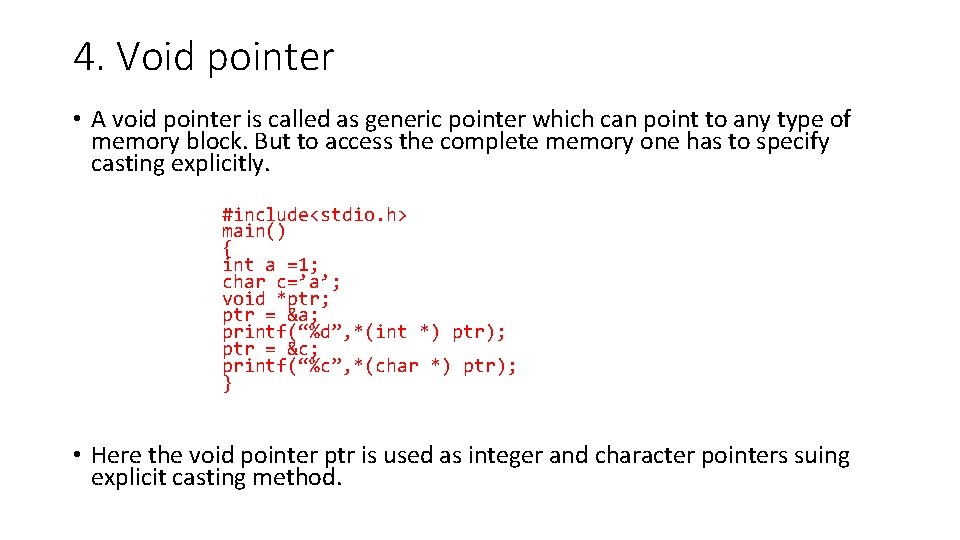
4. Void pointer • A void pointer is called as generic pointer which can point to any type of memory block. But to access the complete memory one has to specify casting explicitly. #include<stdio. h> main() { int a =1; char c=’a’; void *ptr; ptr = &a; printf(“%d”, *(int *) ptr); ptr = &c; printf(“%c”, *(char *) ptr); } • Here the void pointer ptr is used as integer and character pointers suing explicit casting method.
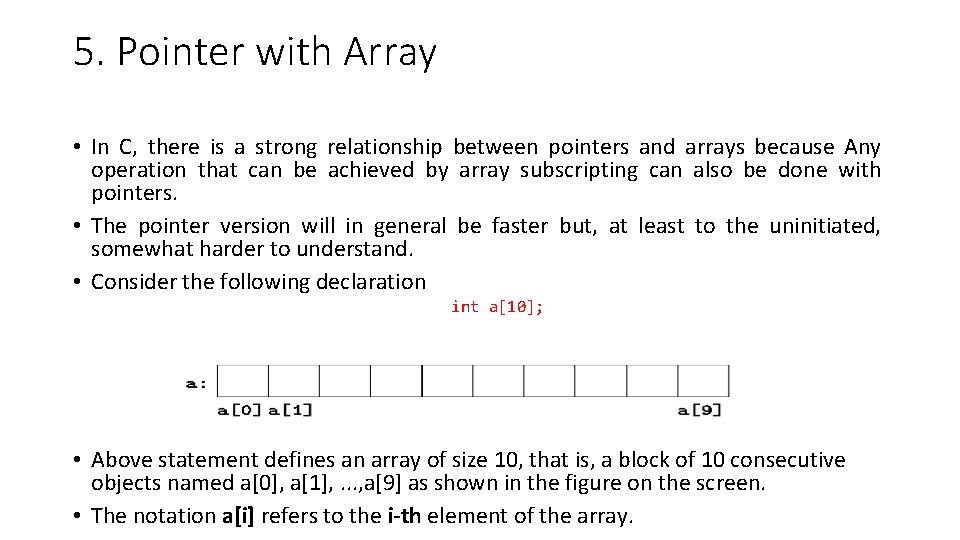
5. Pointer with Array • In C, there is a strong relationship between pointers and arrays because Any operation that can be achieved by array subscripting can also be done with pointers. • The pointer version will in general be faster but, at least to the uninitiated, somewhat harder to understand. • Consider the following declaration int a[10]; • Above statement defines an array of size 10, that is, a block of 10 consecutive objects named a[0], a[1], . . . , a[9] as shown in the figure on the screen. • The notation a[i] refers to the i-th element of the array.
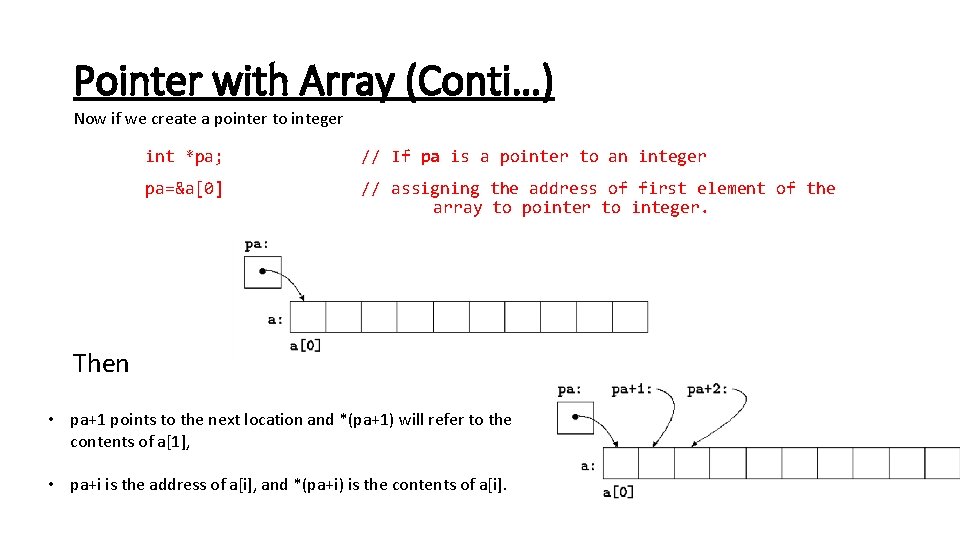
Pointer with Array (Conti…) Now if we create a pointer to integer int *pa; // If pa is a pointer to an integer pa=&a[0] // assigning the address of first element of the array to pointer to integer. Then • pa+1 points to the next location and *(pa+1) will refer to the contents of a[1], • pa+i is the address of a[i], and *(pa+i) is the contents of a[i].
![Pointer with Array Conti Program include stdio h int main int x5 Pointer with Array (Conti…) Program #include <stdio. h> int main() { int x[5] =](https://slidetodoc.com/presentation_image_h2/5dbe86eebfd1717cf3d7cc9009b78f2b/image-16.jpg)
Pointer with Array (Conti…) Program #include <stdio. h> int main() { int x[5] = {1, 2, 3, 4, 5}; int* ptr; // ptr is assigned the address of the third element ptr = &x[2]; printf("*ptr = %d n", *ptr); // 3 printf("*(ptr+1) = %d n", *(ptr+1)); // 4 printf("*(ptr-1) = %d", *(ptr-1)); // 2 return 0; }
![6 Pointer with String include stdio h int mainvoid char str6 Hello 6. Pointer with String #include <stdio. h> int main(void) { char str[6] = "Hello";](https://slidetodoc.com/presentation_image_h2/5dbe86eebfd1717cf3d7cc9009b78f2b/image-17.jpg)
6. Pointer with String #include <stdio. h> int main(void) { char str[6] = "Hello"; char *ptr = str; // print the string while(*ptr != '