CS 476 Programming Language Design William Mansky LowLevel
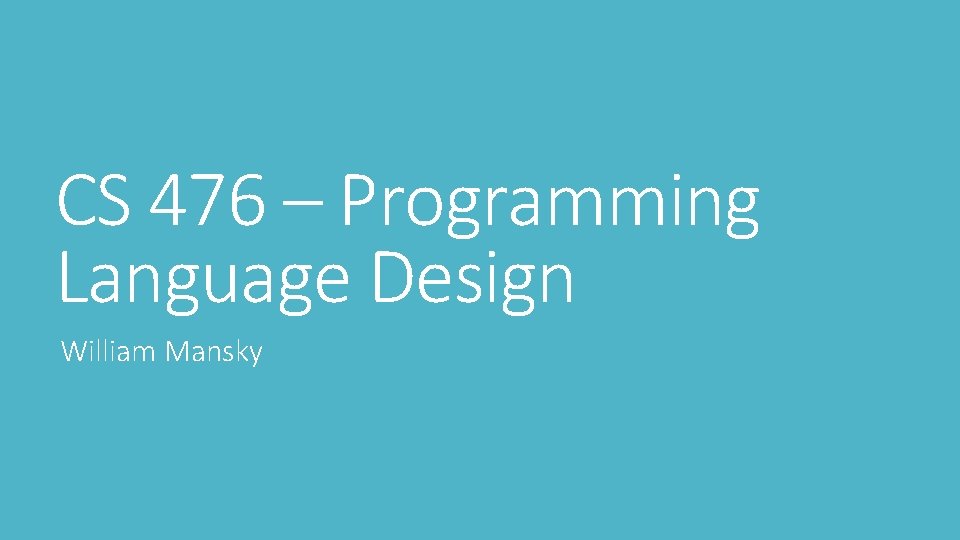
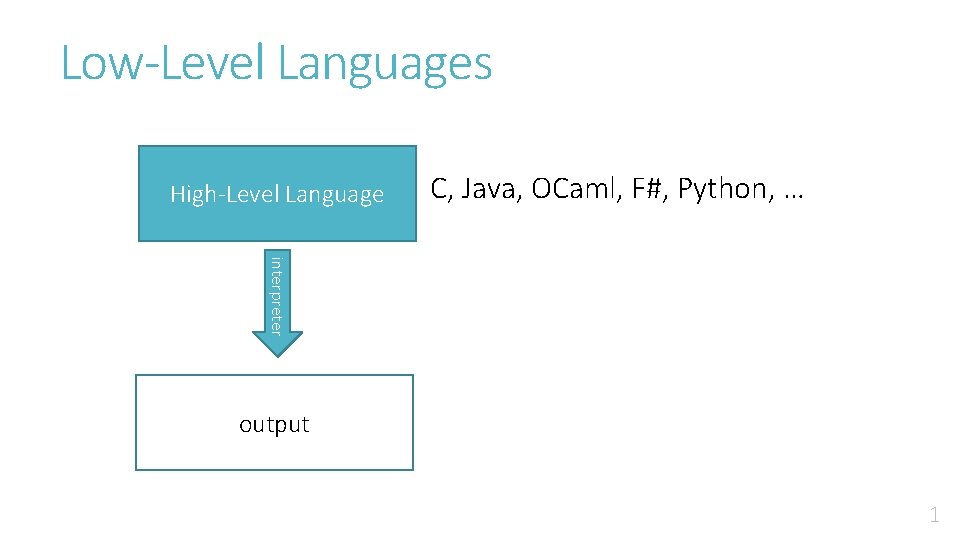
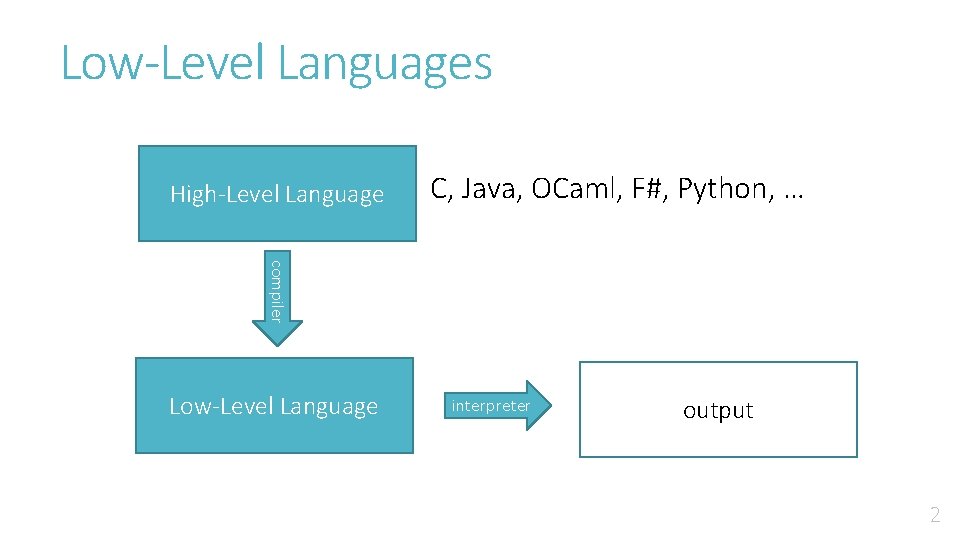
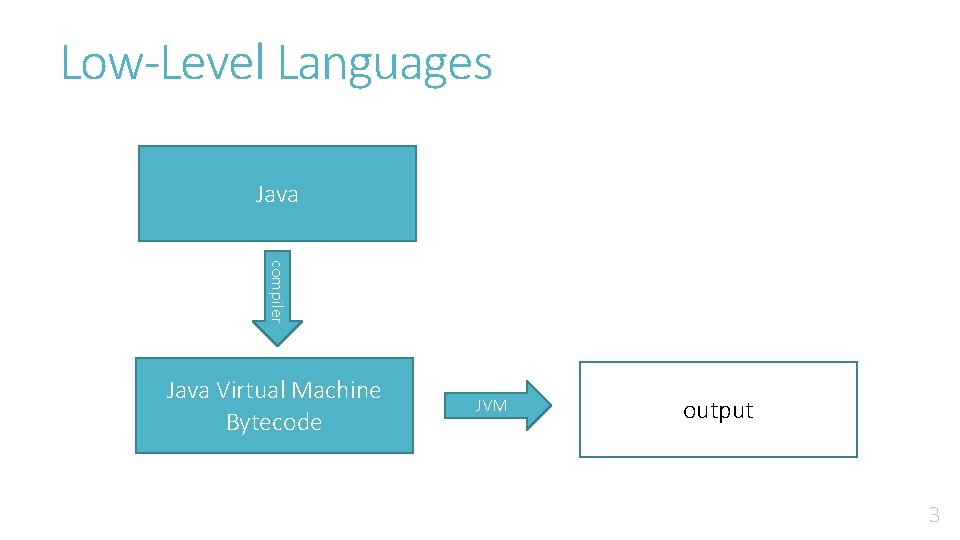
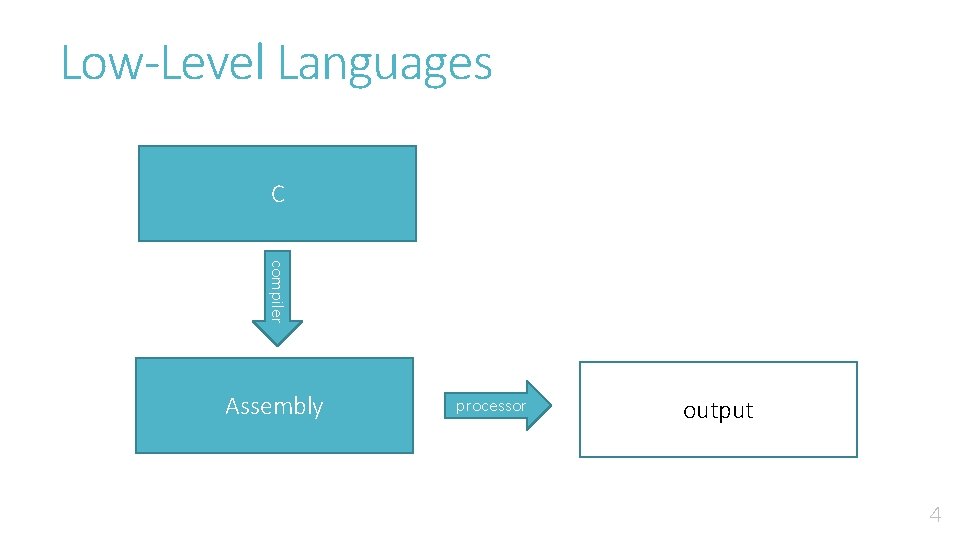
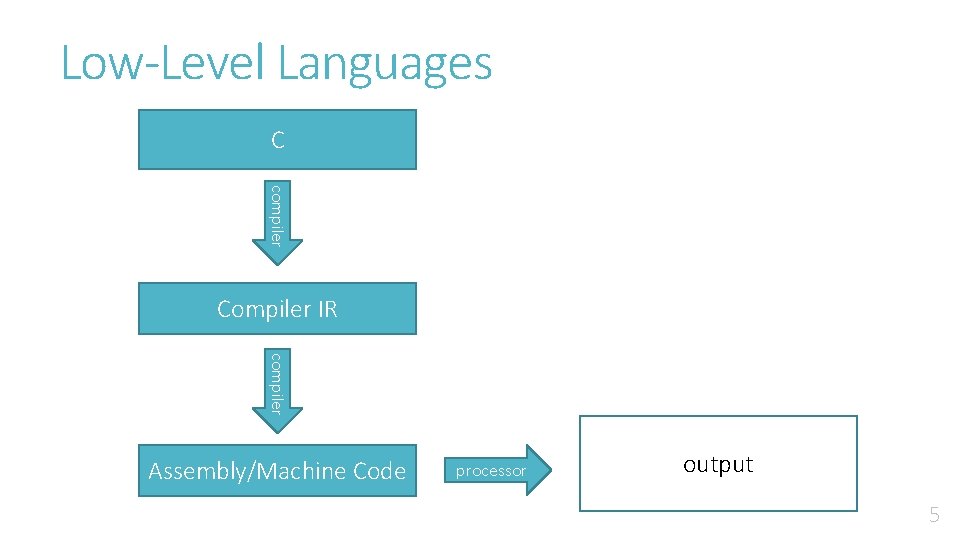
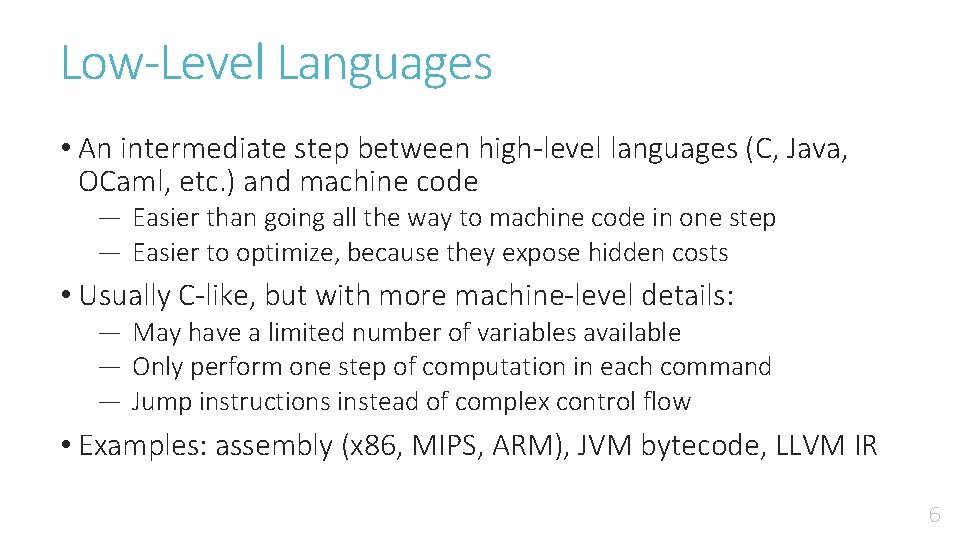
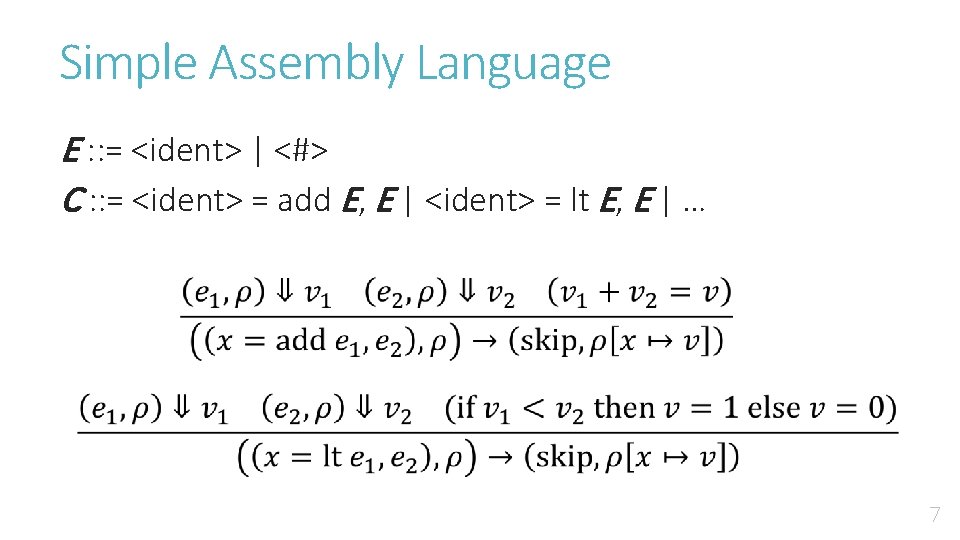
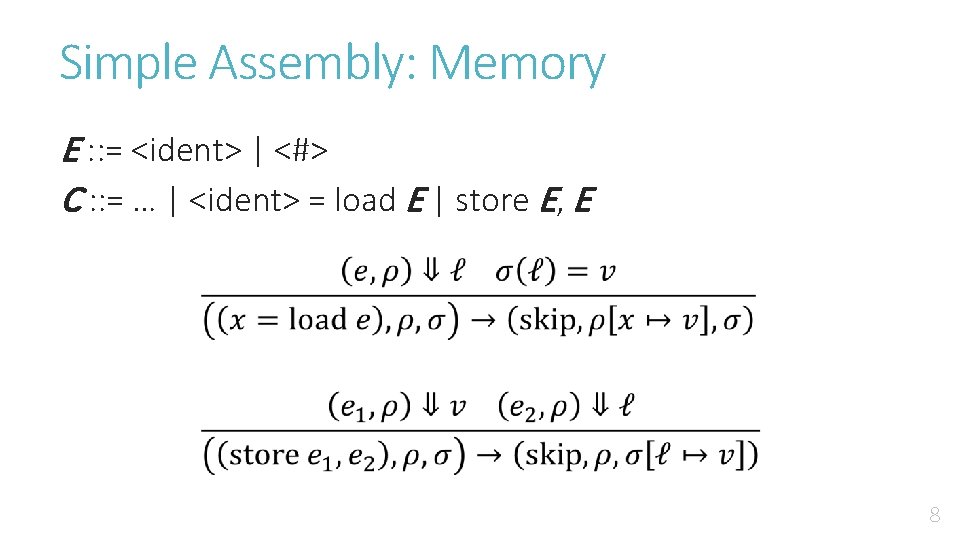
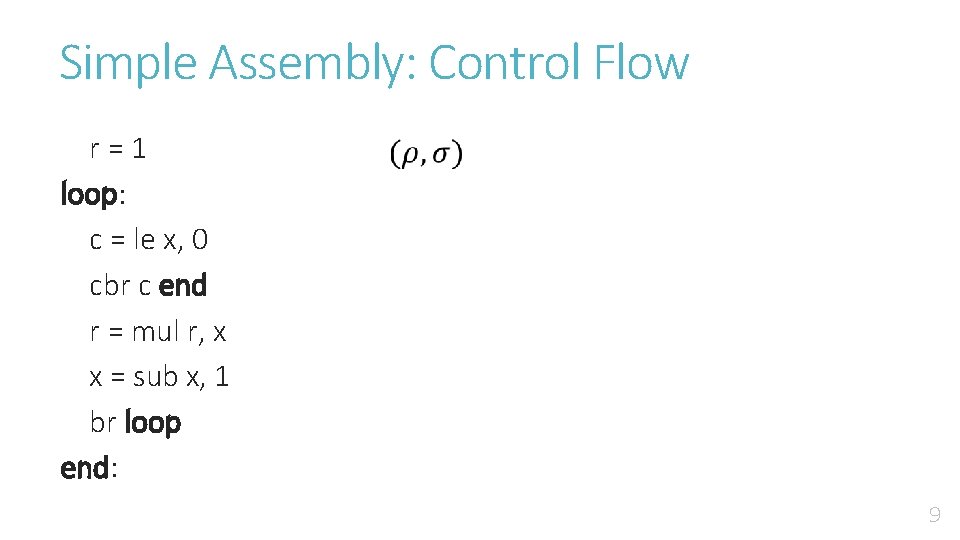
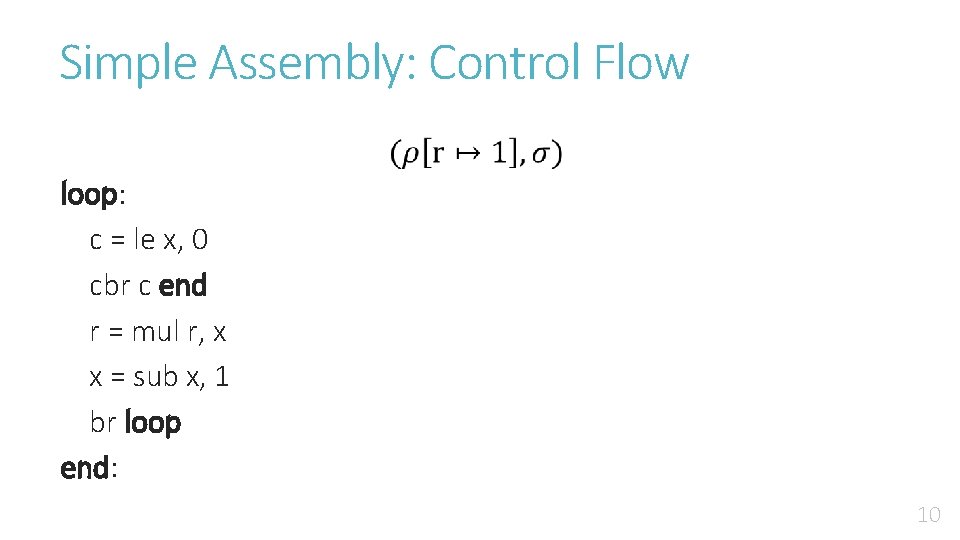
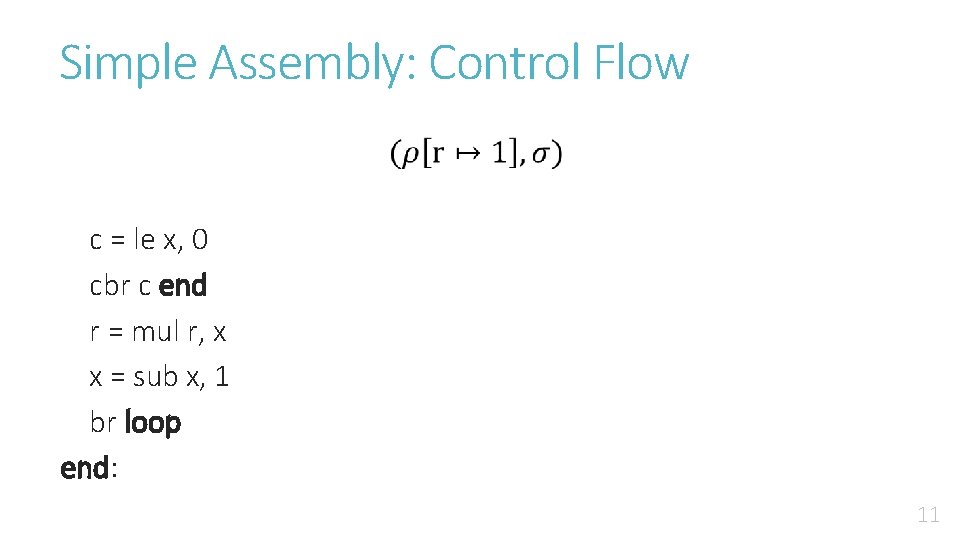
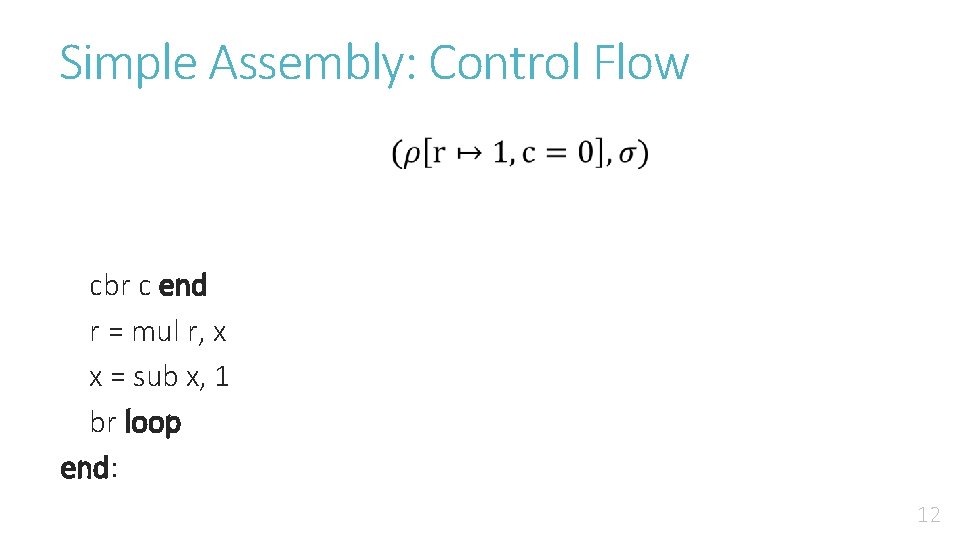
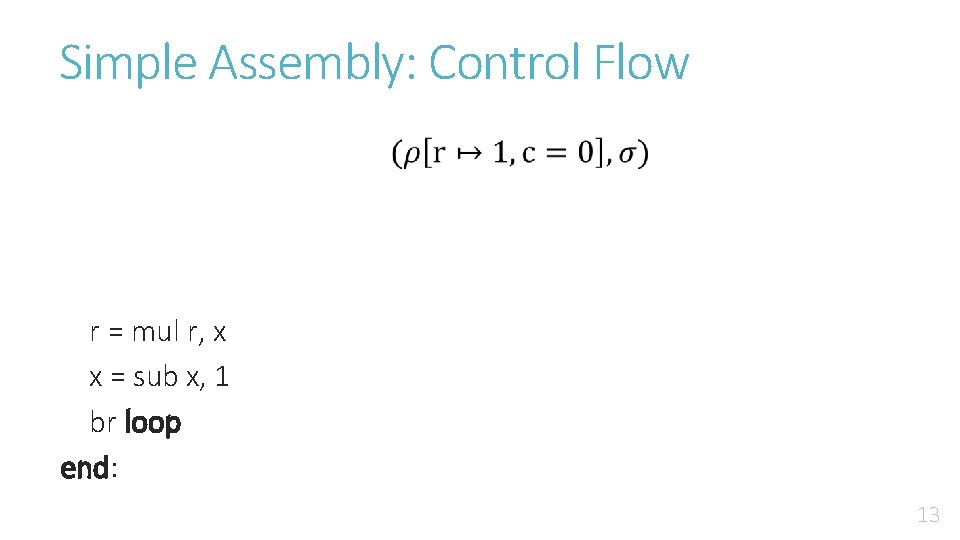
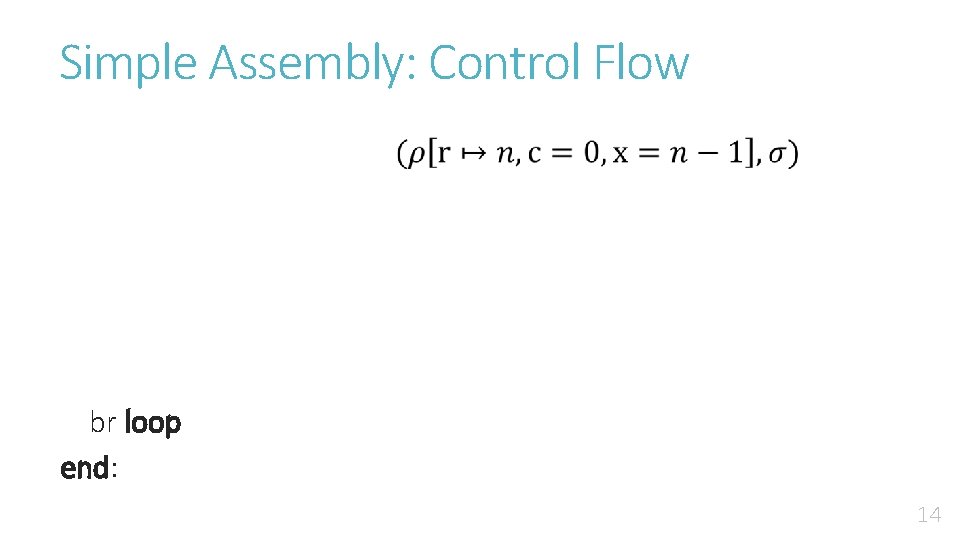
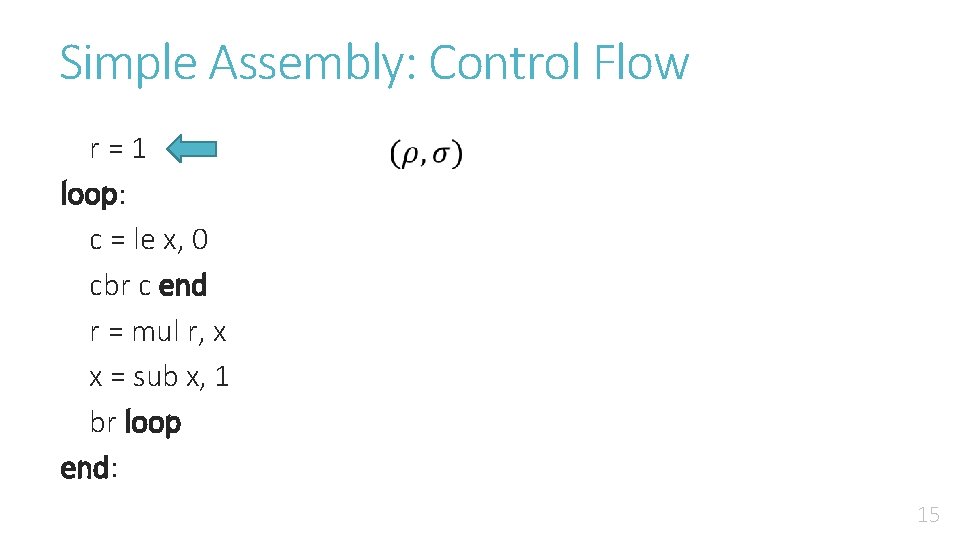
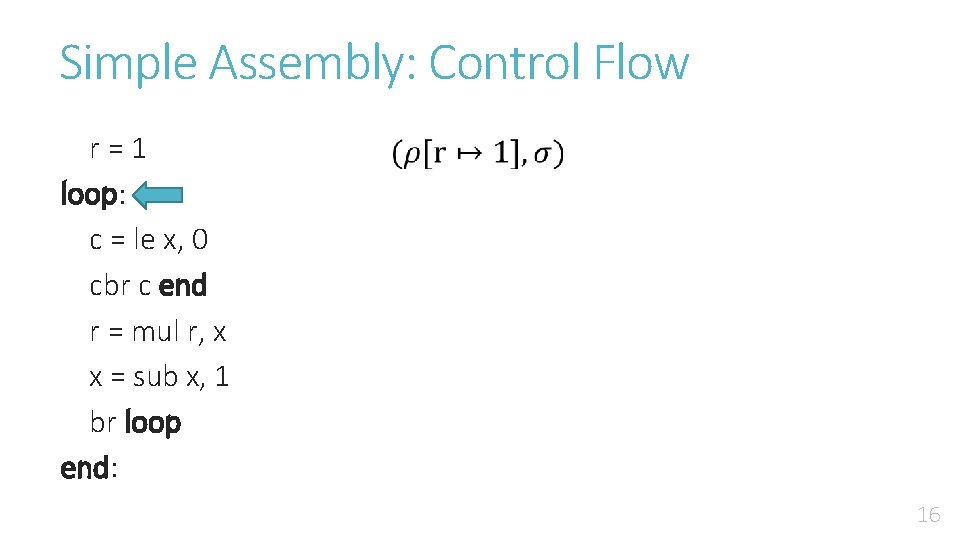
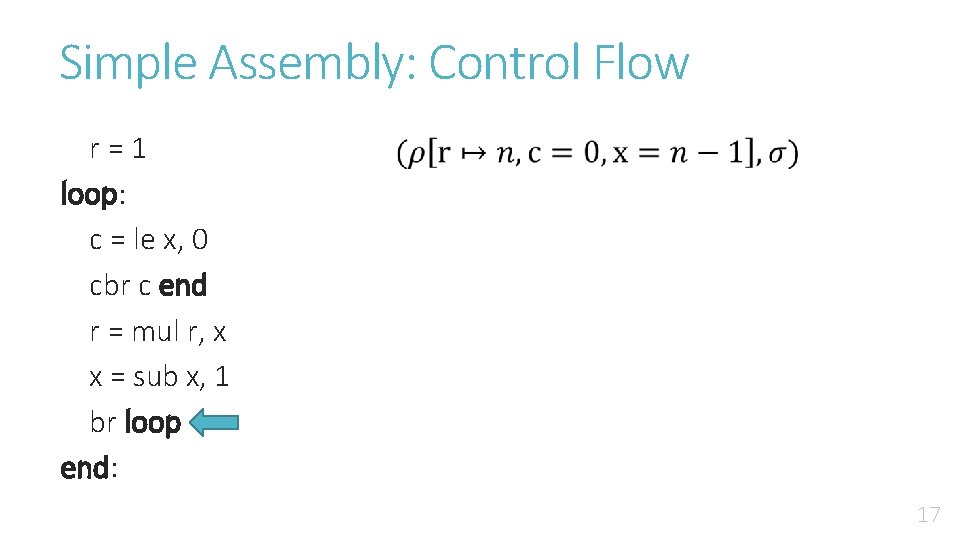
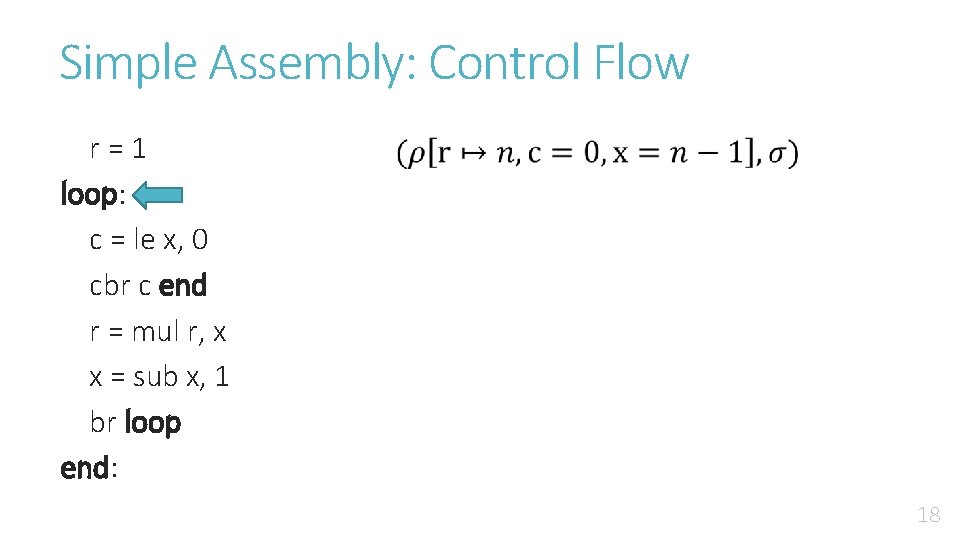
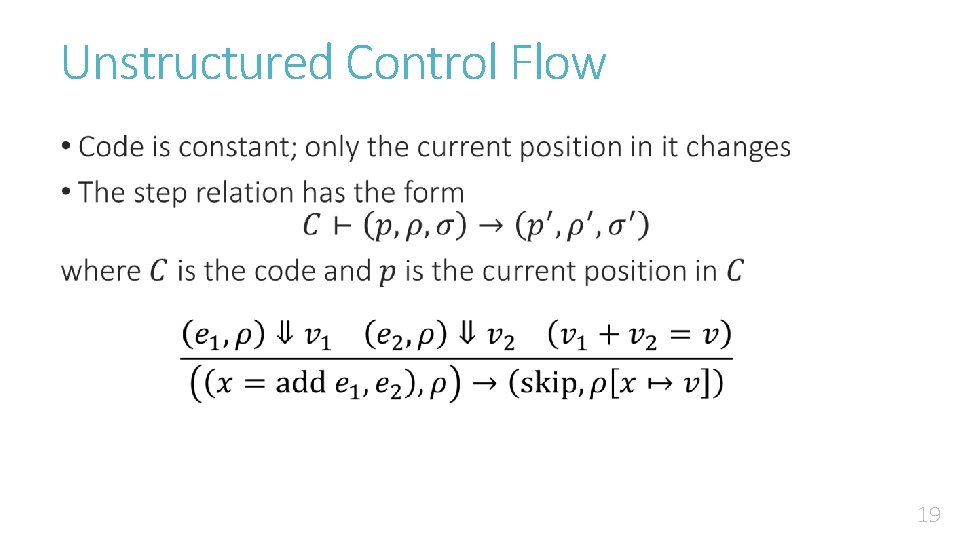
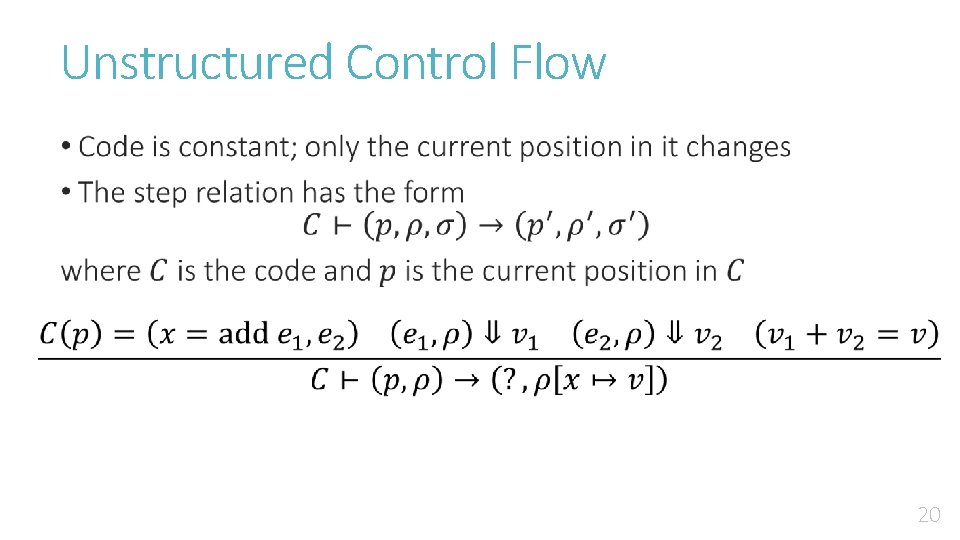
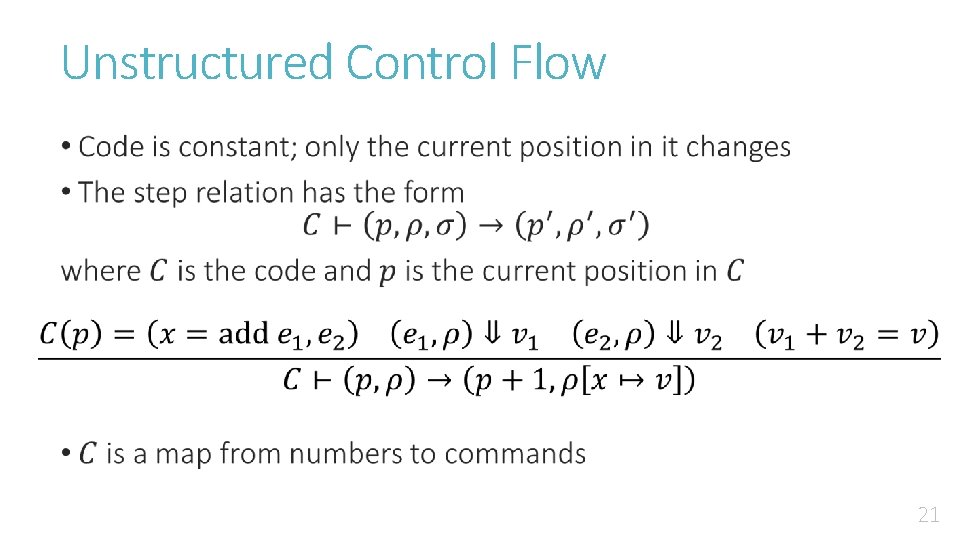
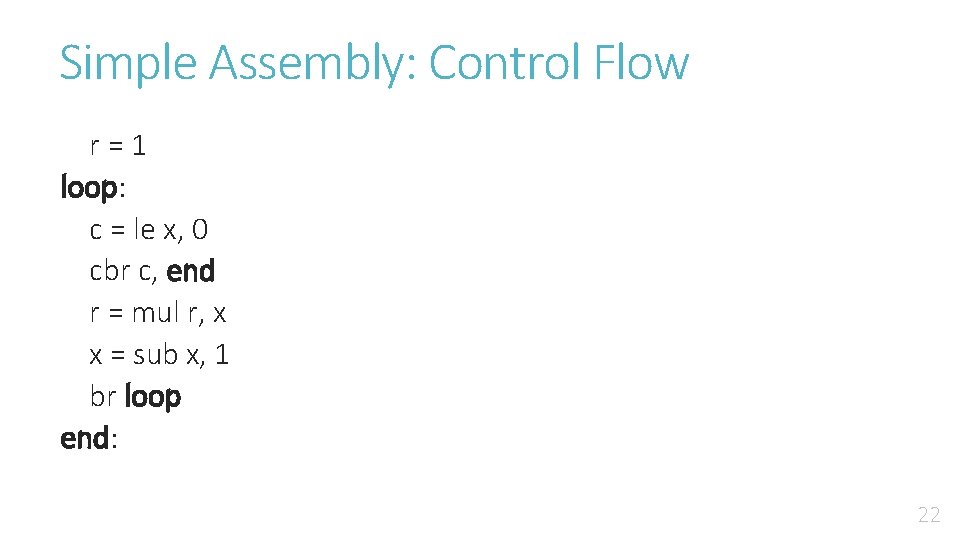
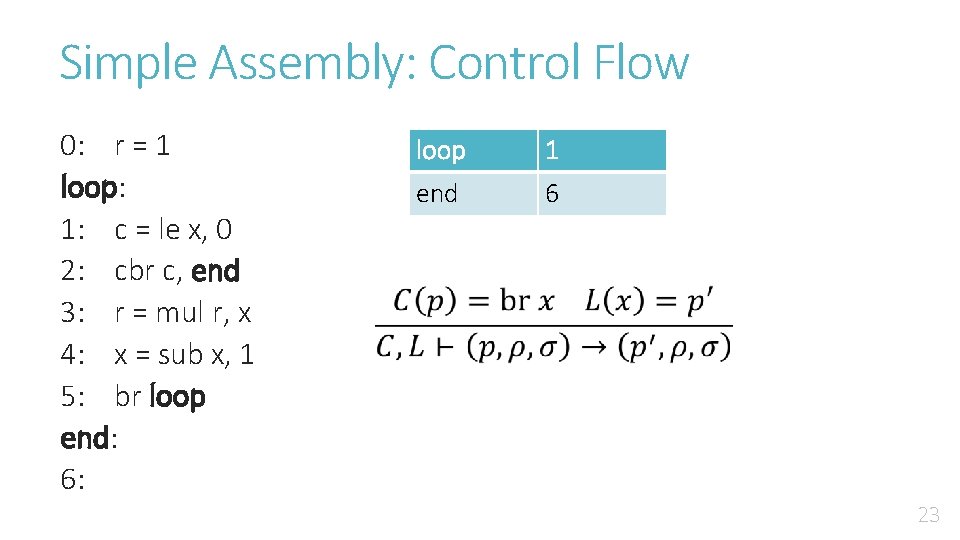
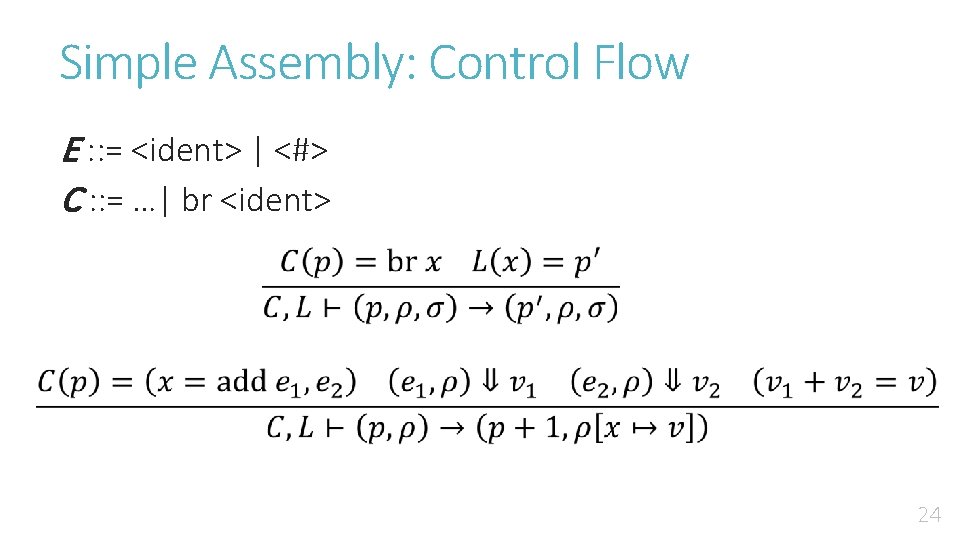
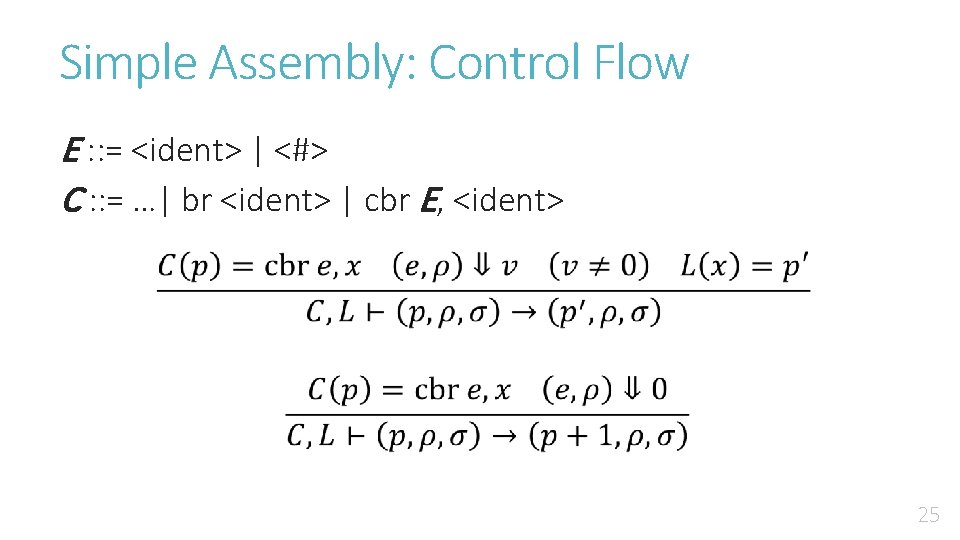
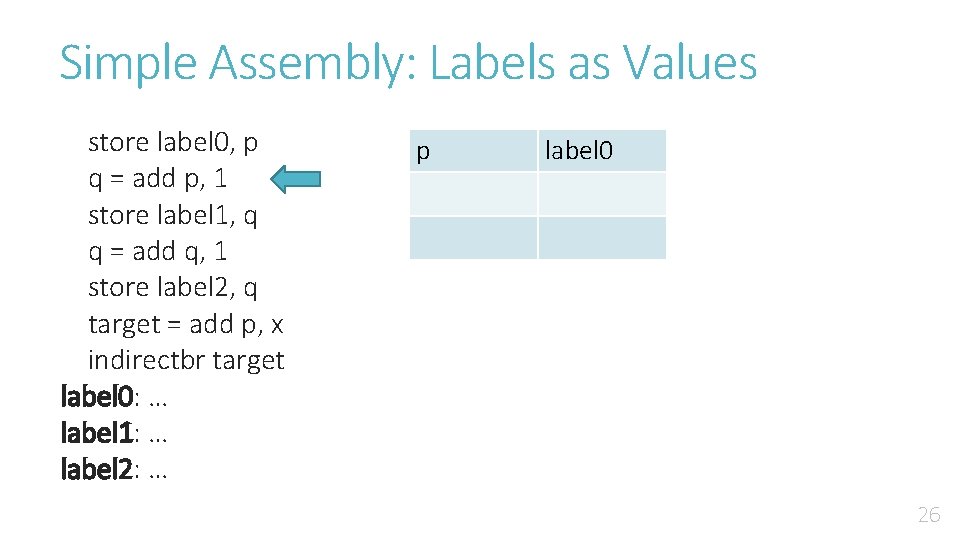
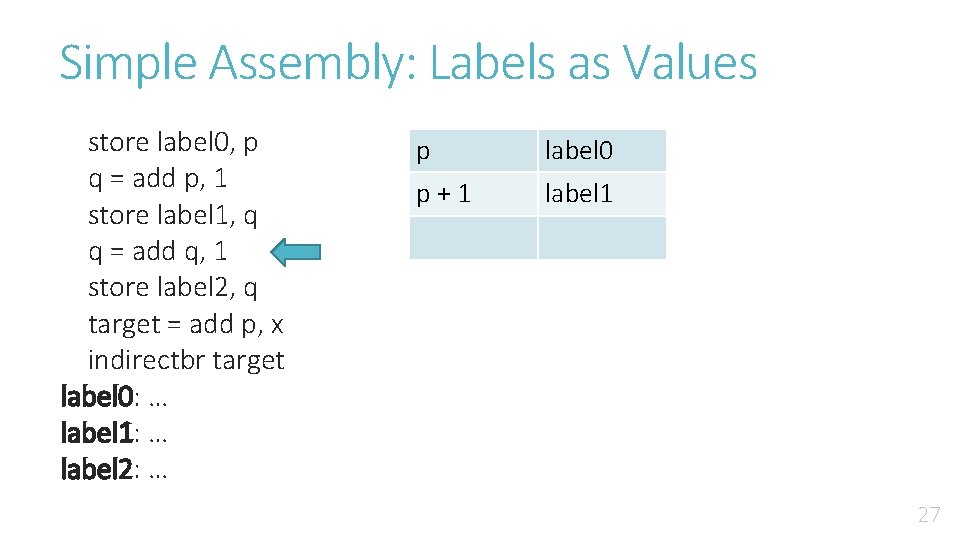
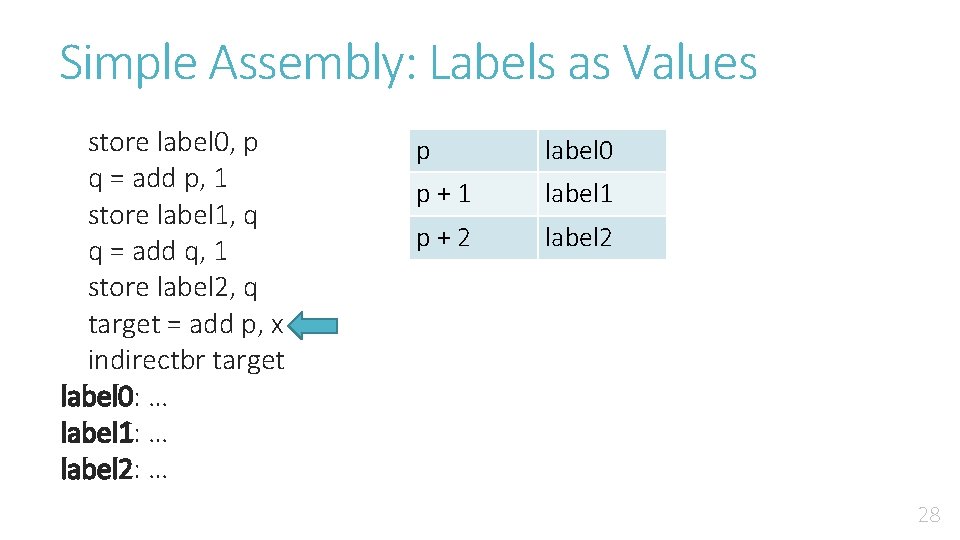
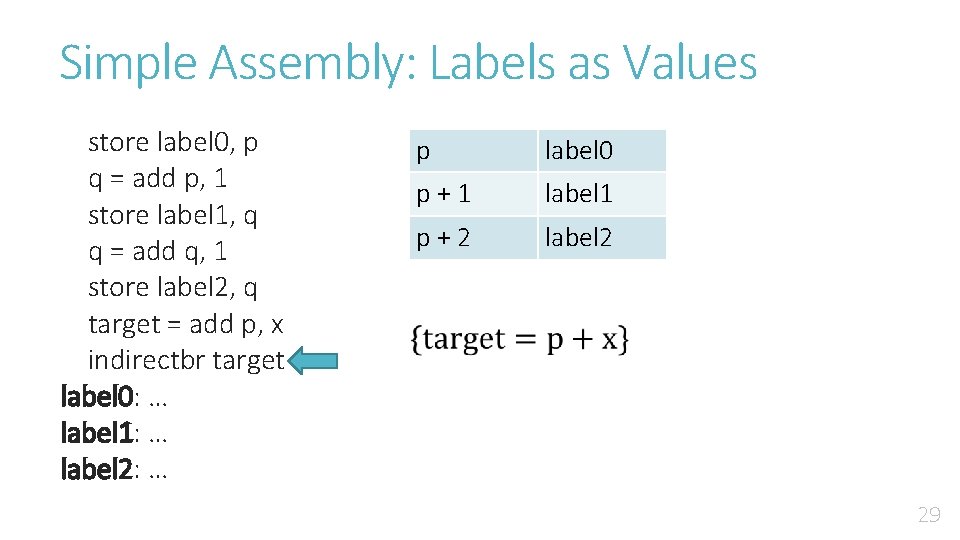
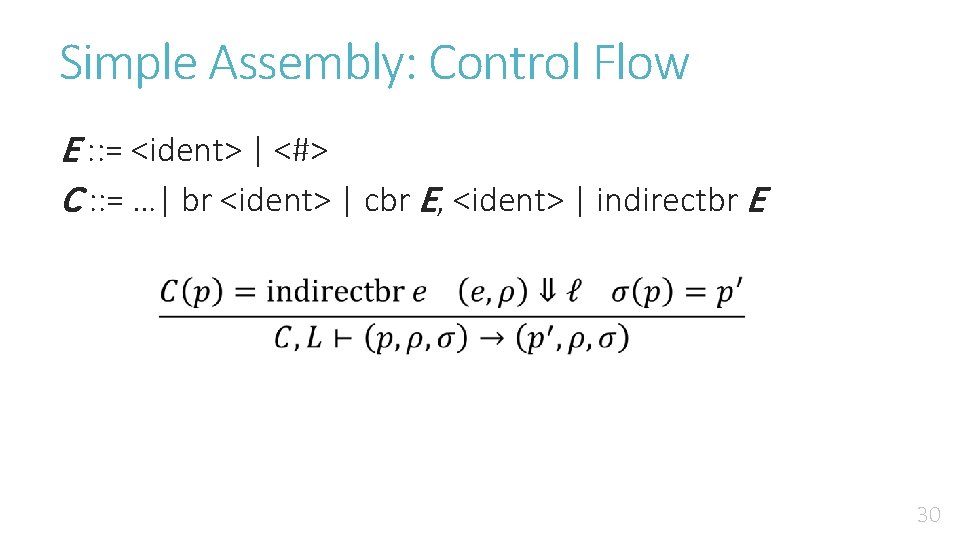
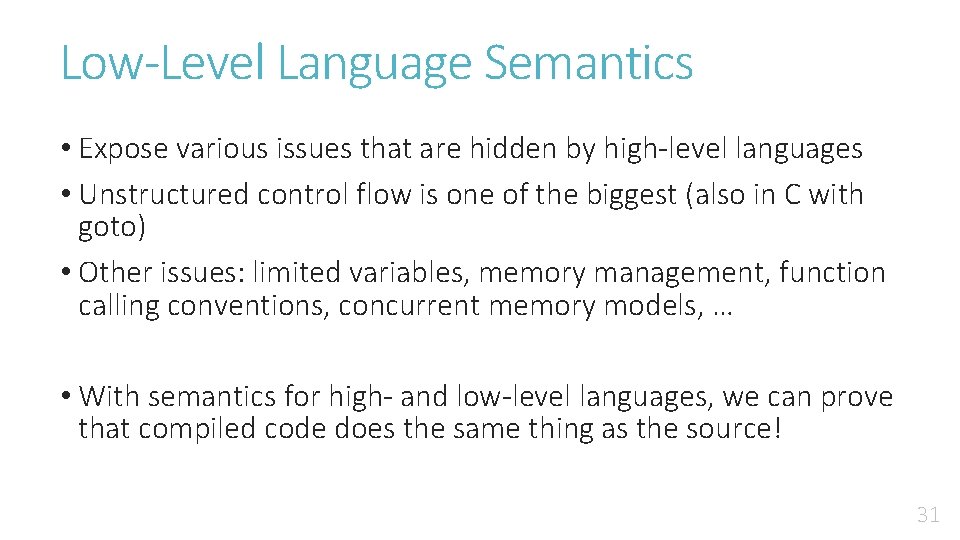
- Slides: 32
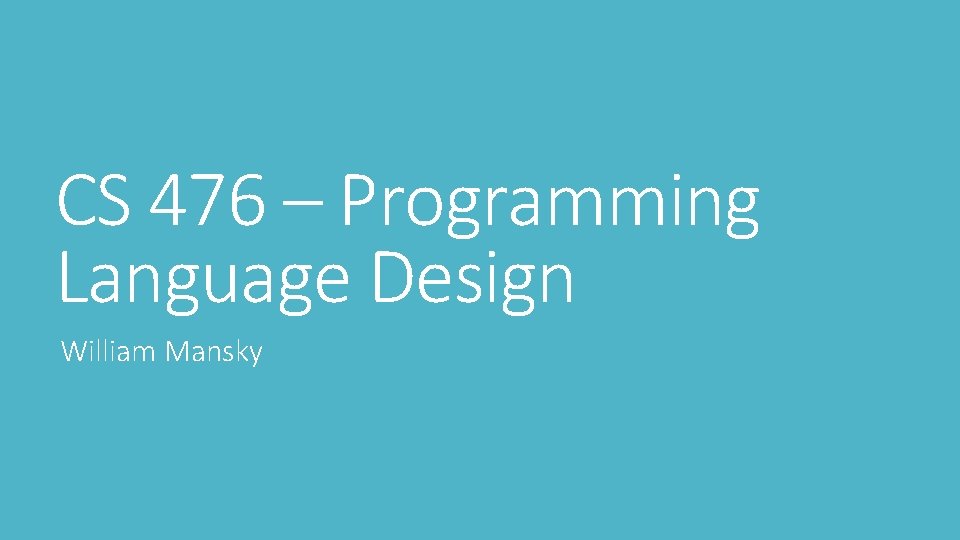
CS 476 – Programming Language Design William Mansky
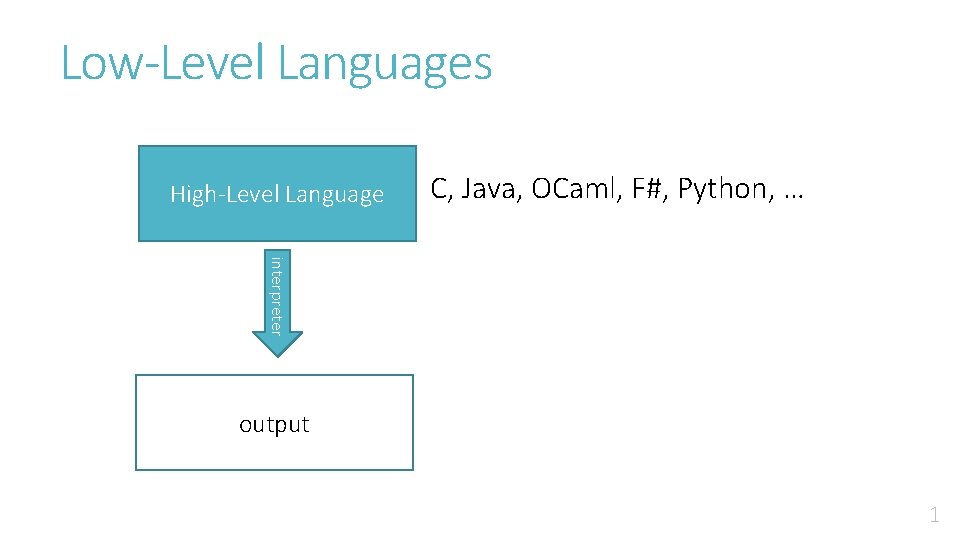
Low-Level Languages High-Level Language C, Java, OCaml, F#, Python, … interpreter output 1
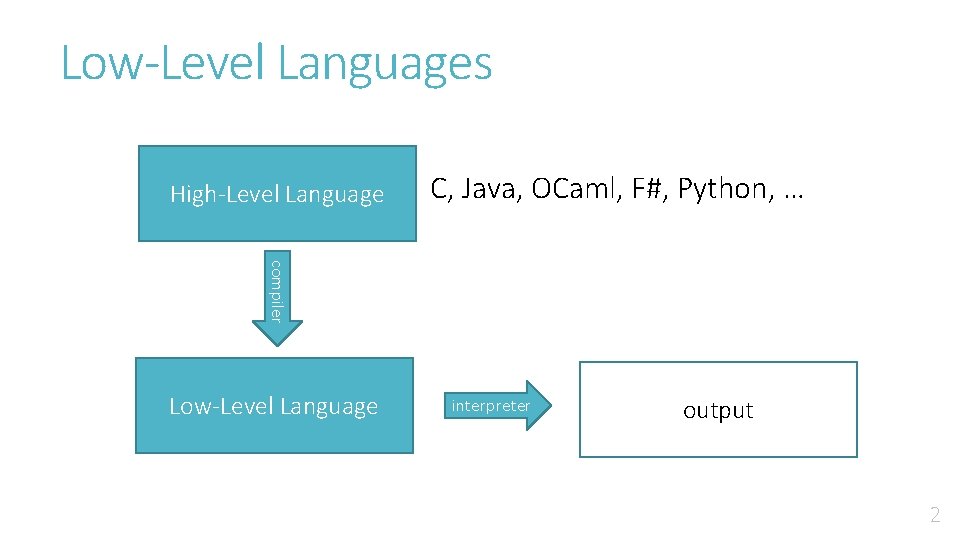
Low-Level Languages High-Level Language C, Java, OCaml, F#, Python, … compiler Low-Level Language interpreter output 2
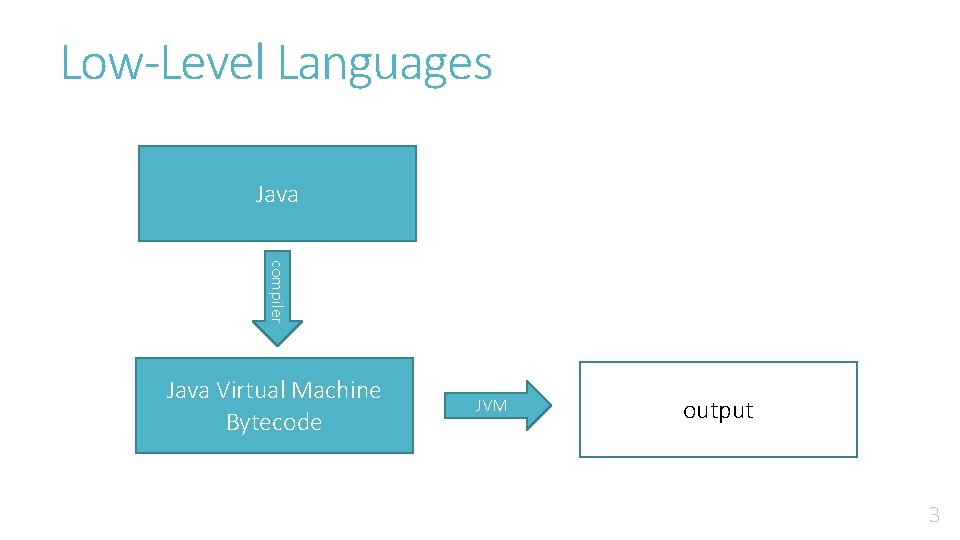
Low-Level Languages Java compiler Java Virtual Machine Bytecode JVM output 3
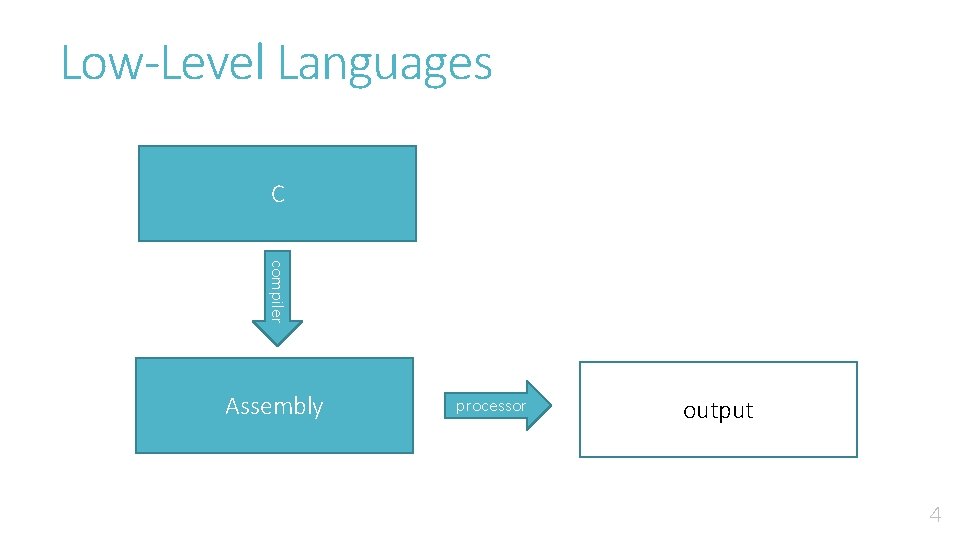
Low-Level Languages C compiler Assembly processor output 4
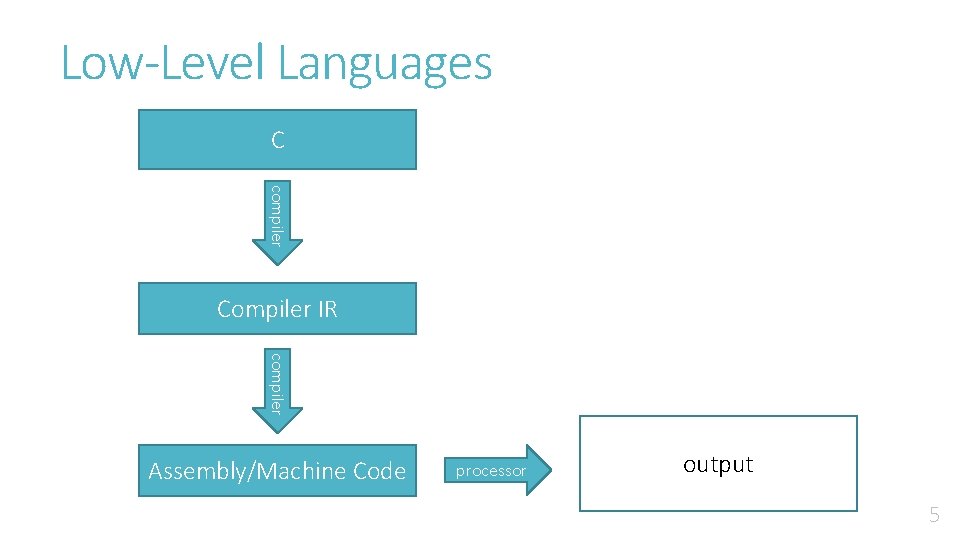
Low-Level Languages C compiler Compiler IR compiler Assembly/Machine Code processor output 5
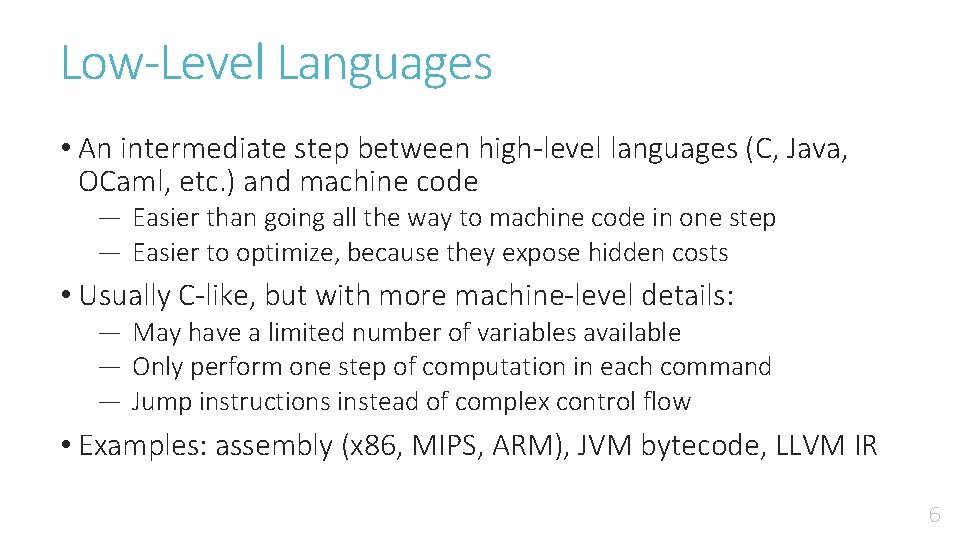
Low-Level Languages • An intermediate step between high-level languages (C, Java, OCaml, etc. ) and machine code ― Easier than going all the way to machine code in one step ― Easier to optimize, because they expose hidden costs • Usually C-like, but with more machine-level details: ― May have a limited number of variables available ― Only perform one step of computation in each command ― Jump instructions instead of complex control flow • Examples: assembly (x 86, MIPS, ARM), JVM bytecode, LLVM IR 6
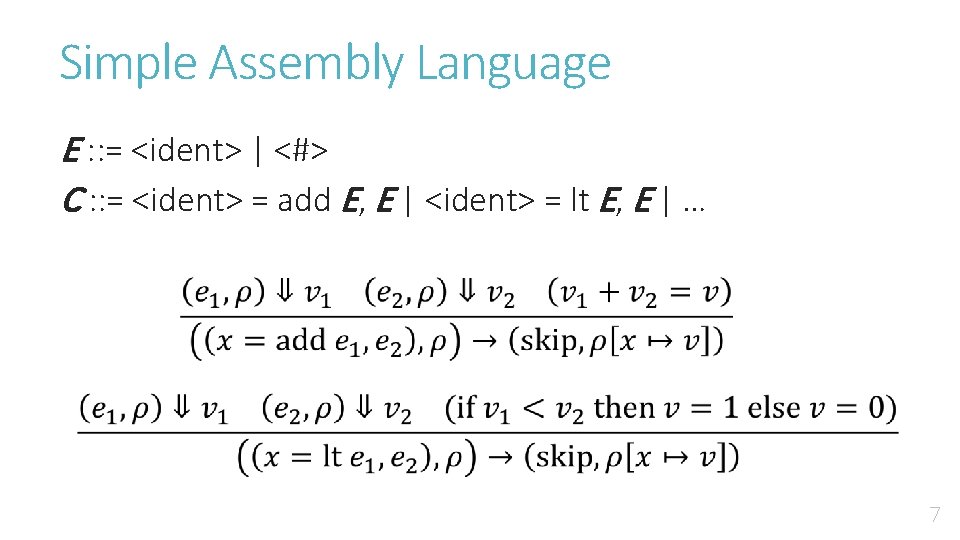
Simple Assembly Language E : : = <ident> | <#> C : : = <ident> = add E, E | <ident> = lt E, E | … 7
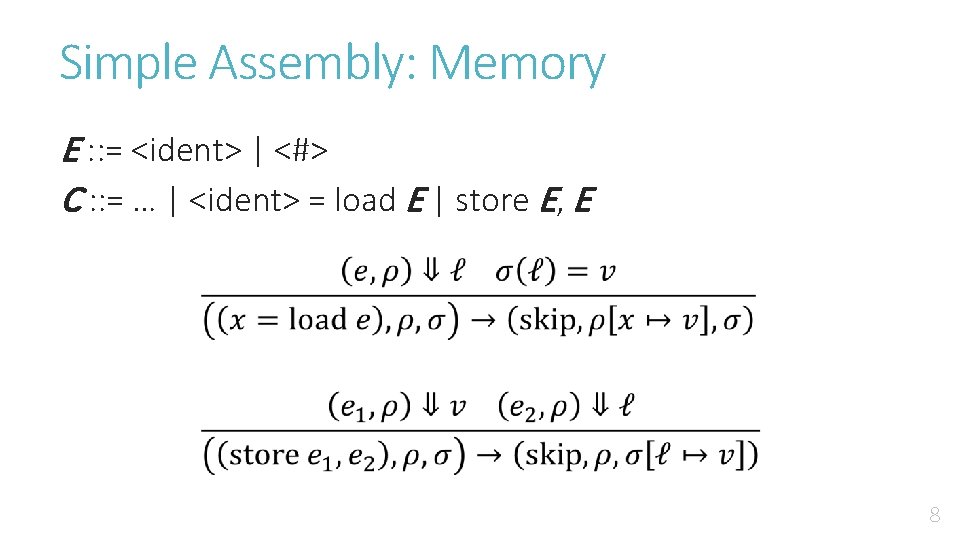
Simple Assembly: Memory E : : = <ident> | <#> C : : = … | <ident> = load E | store E, E 8
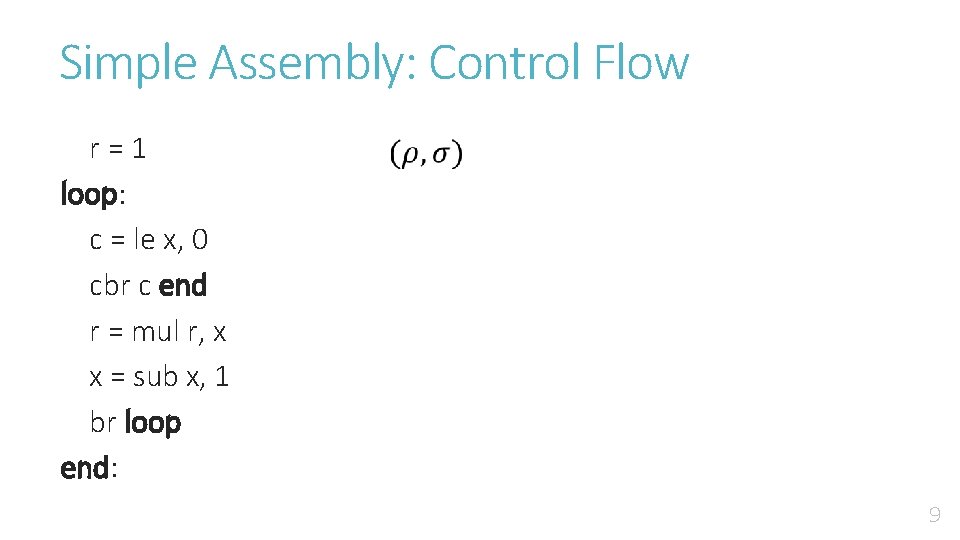
Simple Assembly: Control Flow r = 1 loop: c = le x, 0 cbr c end r = mul r, x x = sub x, 1 br loop end: 9
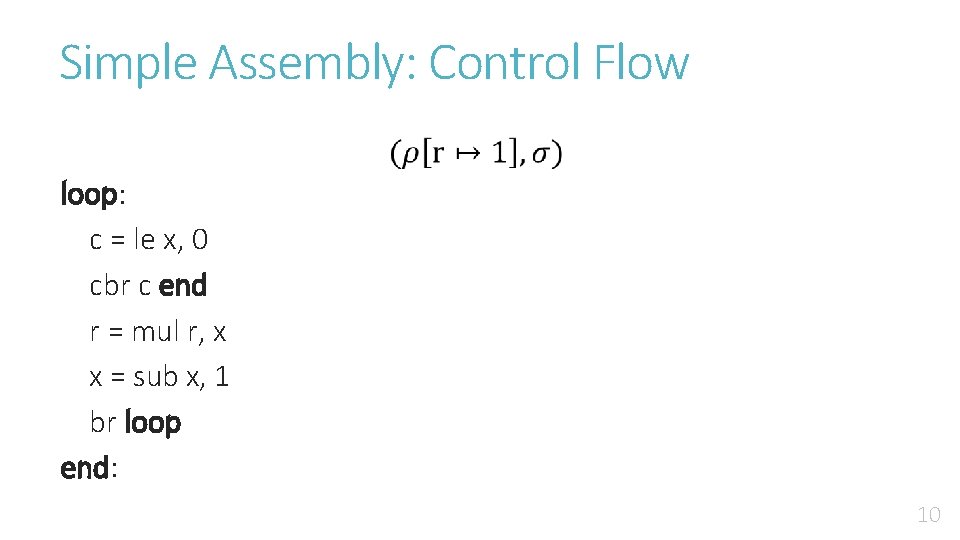
Simple Assembly: Control Flow loop: c = le x, 0 cbr c end r = mul r, x x = sub x, 1 br loop end: 10
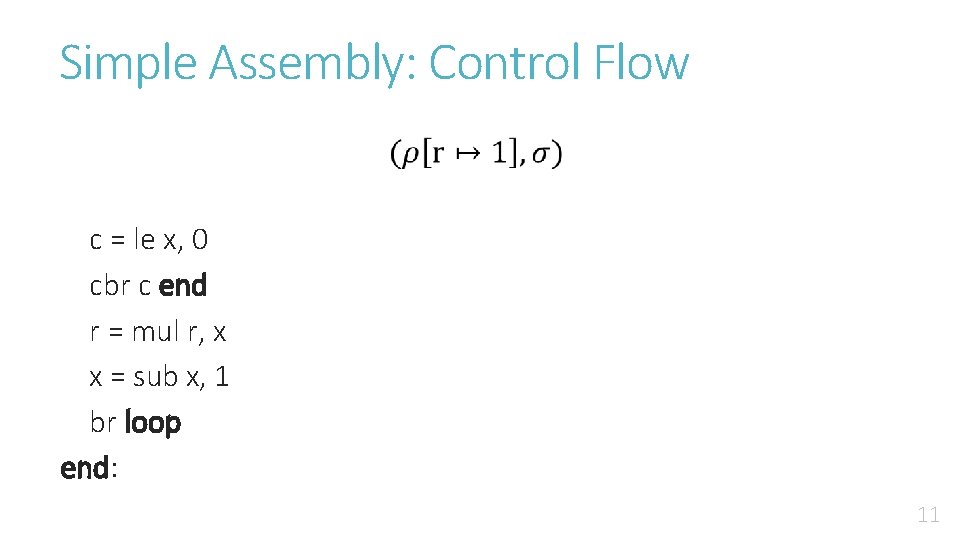
Simple Assembly: Control Flow c = le x, 0 cbr c end r = mul r, x x = sub x, 1 br loop end: 11
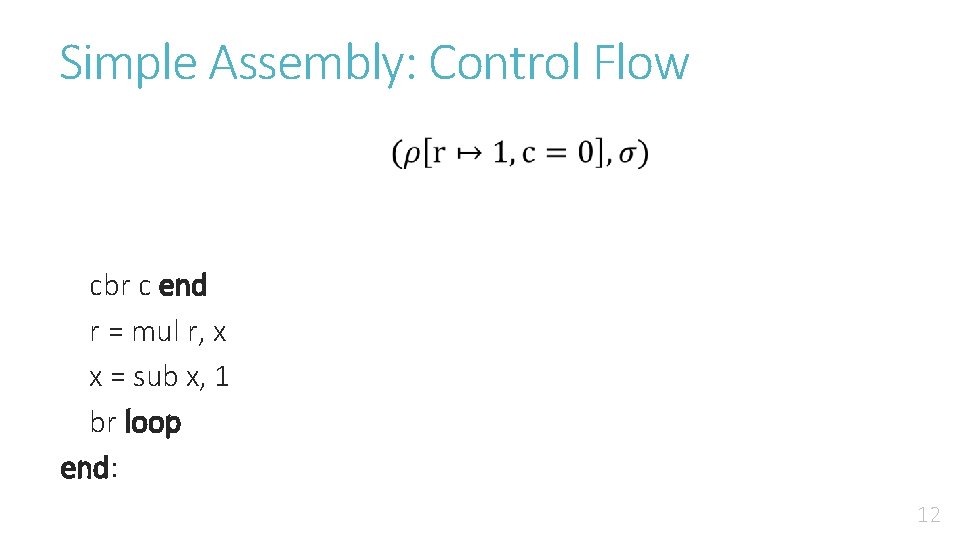
Simple Assembly: Control Flow cbr c end r = mul r, x x = sub x, 1 br loop end: 12
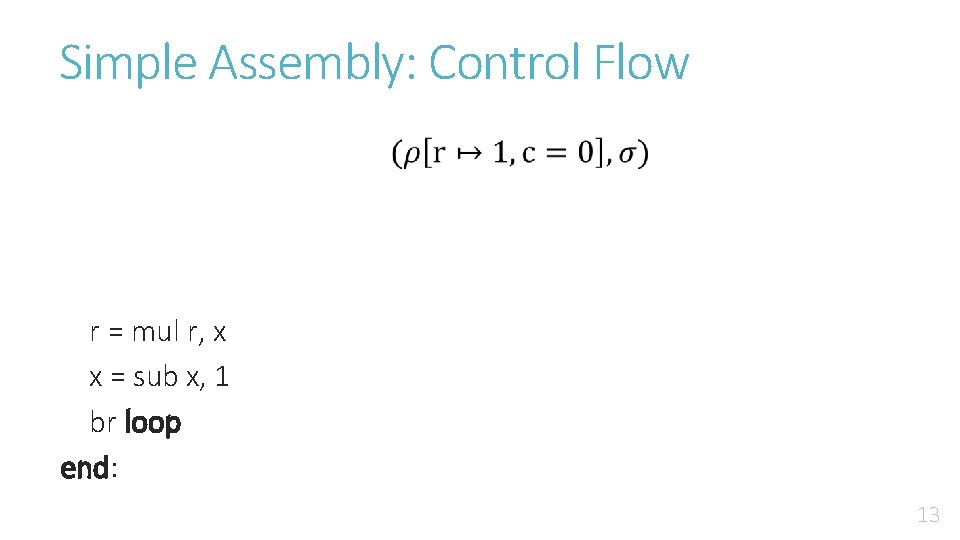
Simple Assembly: Control Flow r = mul r, x x = sub x, 1 br loop end: 13
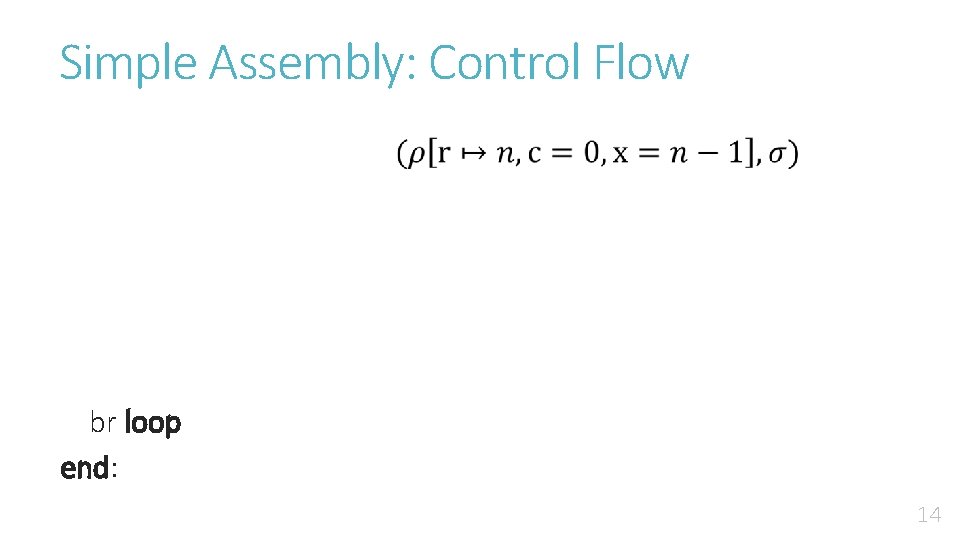
Simple Assembly: Control Flow br loop end: 14
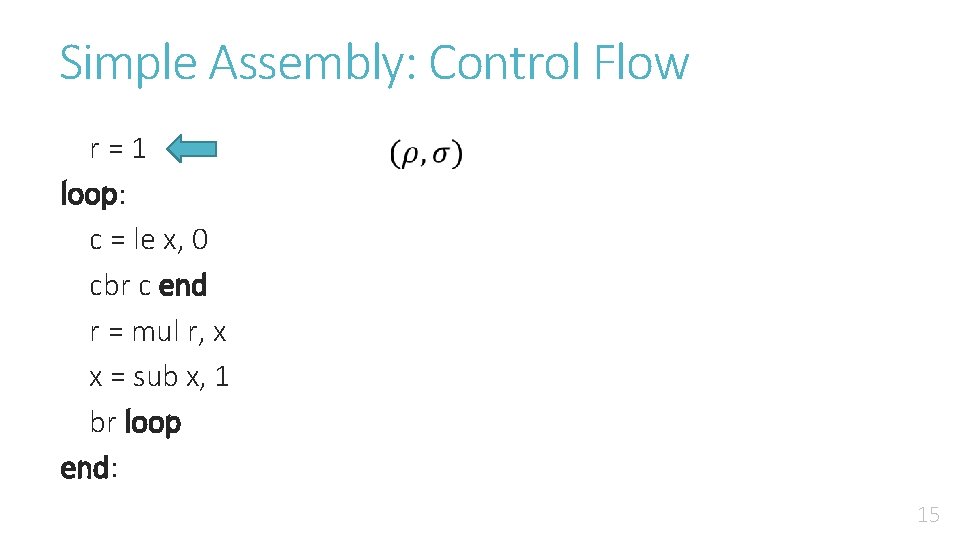
Simple Assembly: Control Flow r = 1 loop: c = le x, 0 cbr c end r = mul r, x x = sub x, 1 br loop end: 15
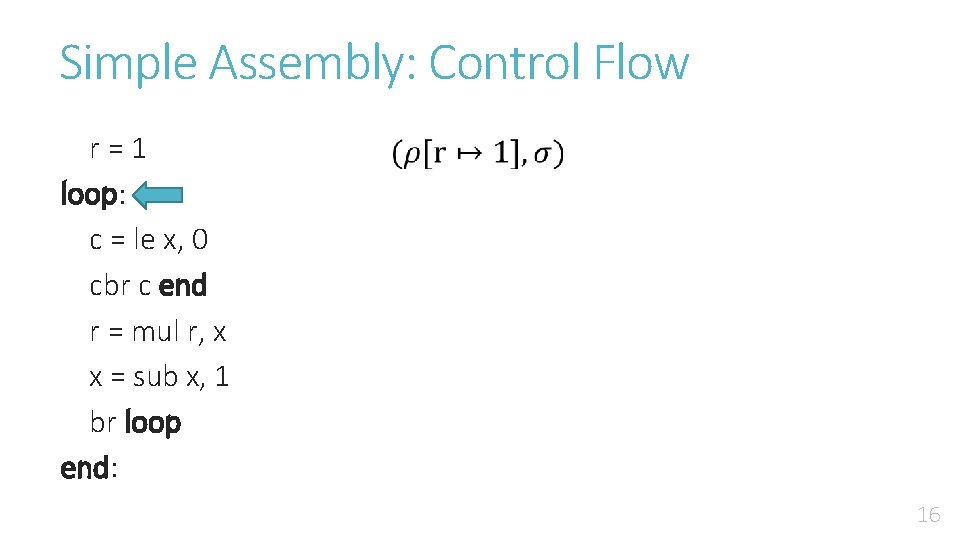
Simple Assembly: Control Flow r = 1 loop: c = le x, 0 cbr c end r = mul r, x x = sub x, 1 br loop end: 16
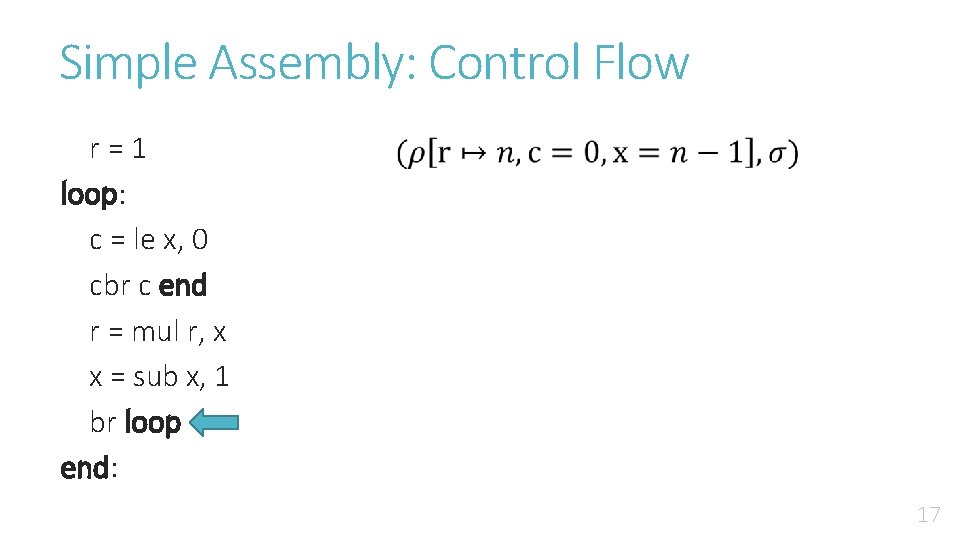
Simple Assembly: Control Flow r = 1 loop: c = le x, 0 cbr c end r = mul r, x x = sub x, 1 br loop end: 17
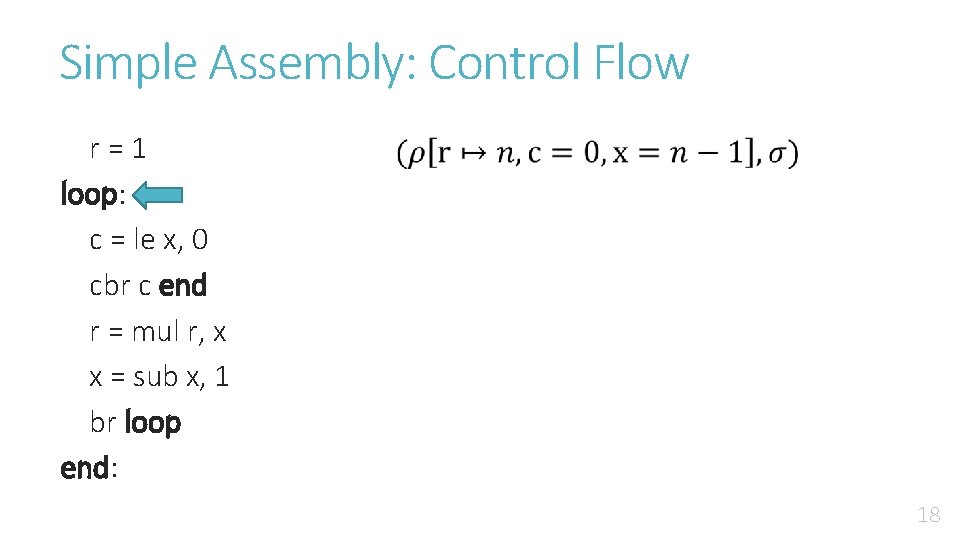
Simple Assembly: Control Flow r = 1 loop: c = le x, 0 cbr c end r = mul r, x x = sub x, 1 br loop end: 18
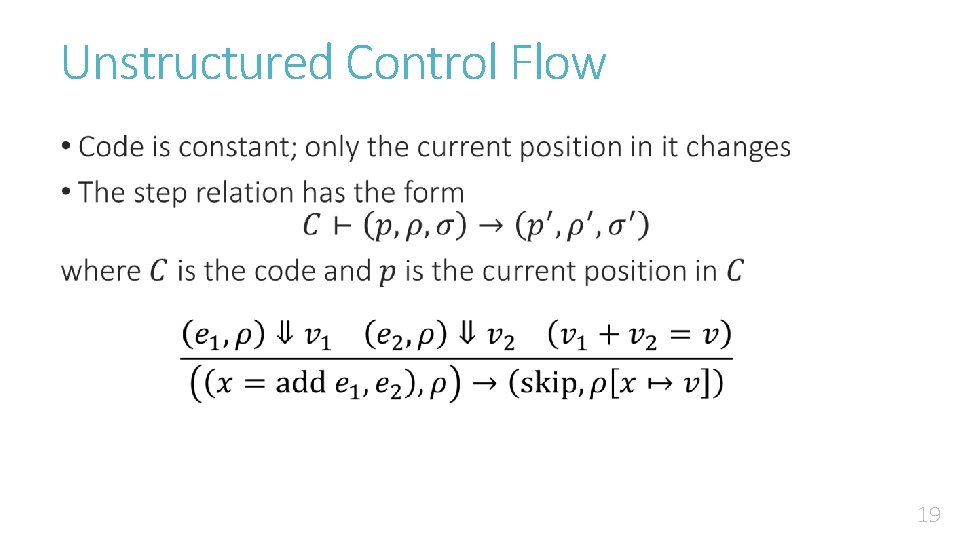
Unstructured Control Flow • 19
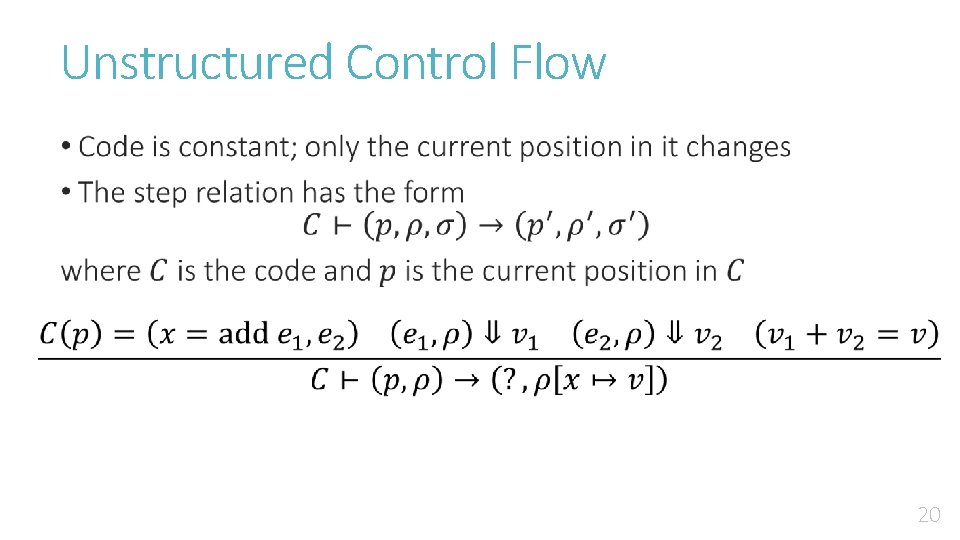
Unstructured Control Flow • 20
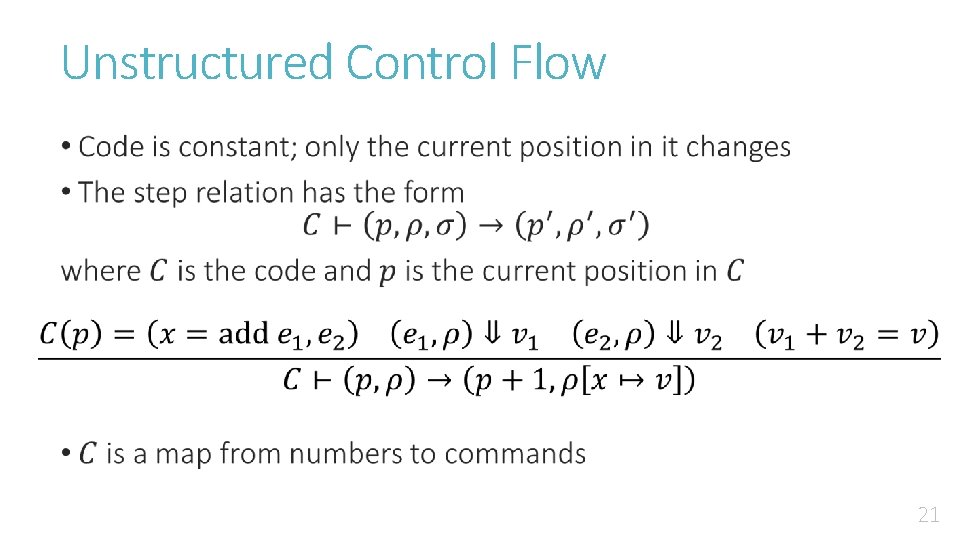
Unstructured Control Flow • 21
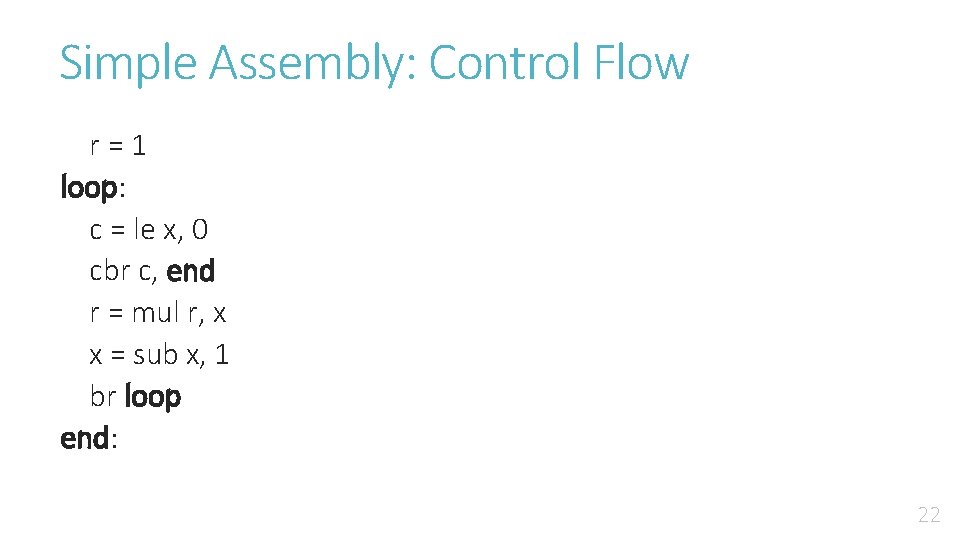
Simple Assembly: Control Flow r = 1 loop: c = le x, 0 cbr c, end r = mul r, x x = sub x, 1 br loop end: 22
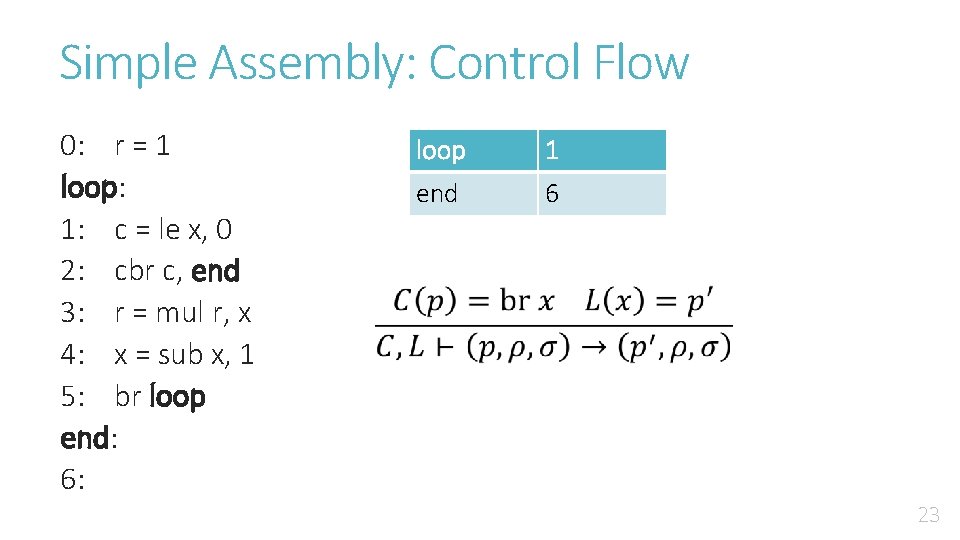
Simple Assembly: Control Flow 0: r = 1 loop: 1: c = le x, 0 2: cbr c, end 3: r = mul r, x 4: x = sub x, 1 5: br loop end: 6: loop 1 end 6 23
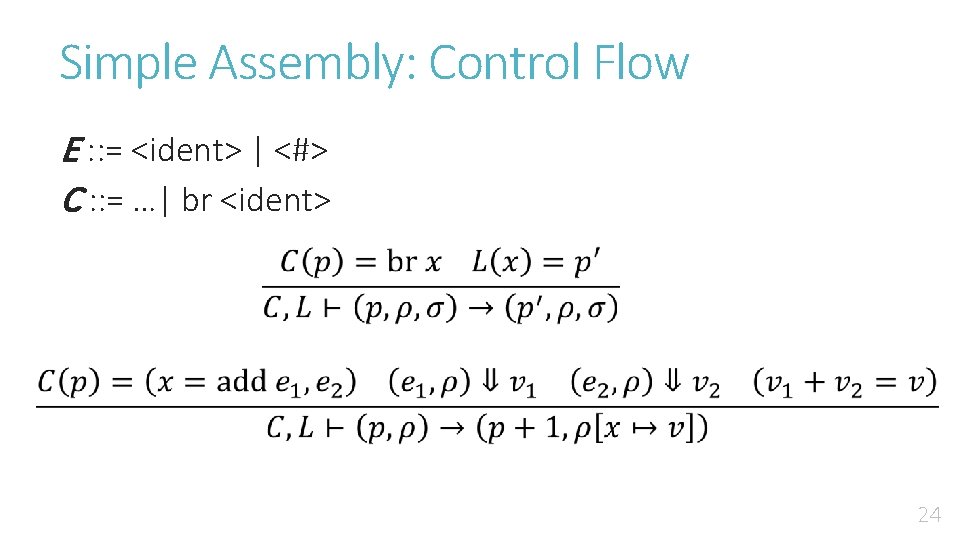
Simple Assembly: Control Flow E : : = <ident> | <#> C : : = …| br <ident> 24
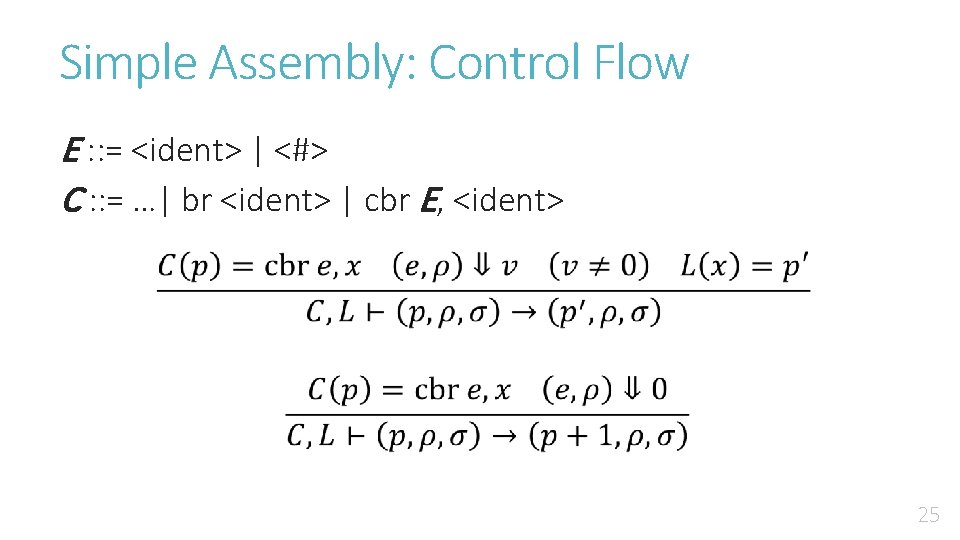
Simple Assembly: Control Flow E : : = <ident> | <#> C : : = …| br <ident> | cbr E, <ident> 25
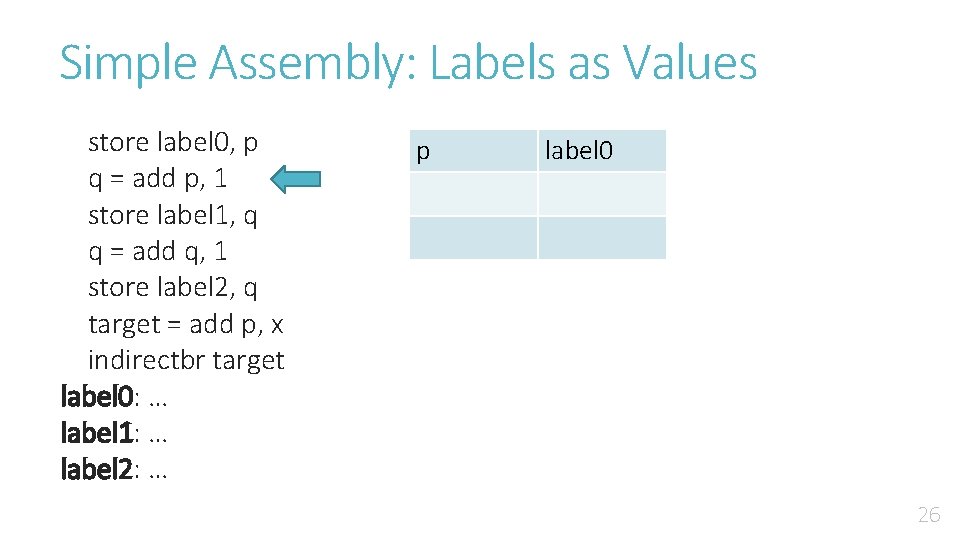
Simple Assembly: Labels as Values store label 0, p q = add p, 1 store label 1, q q = add q, 1 store label 2, q target = add p, x indirectbr target label 0: … label 1: … label 2: … p label 0 26
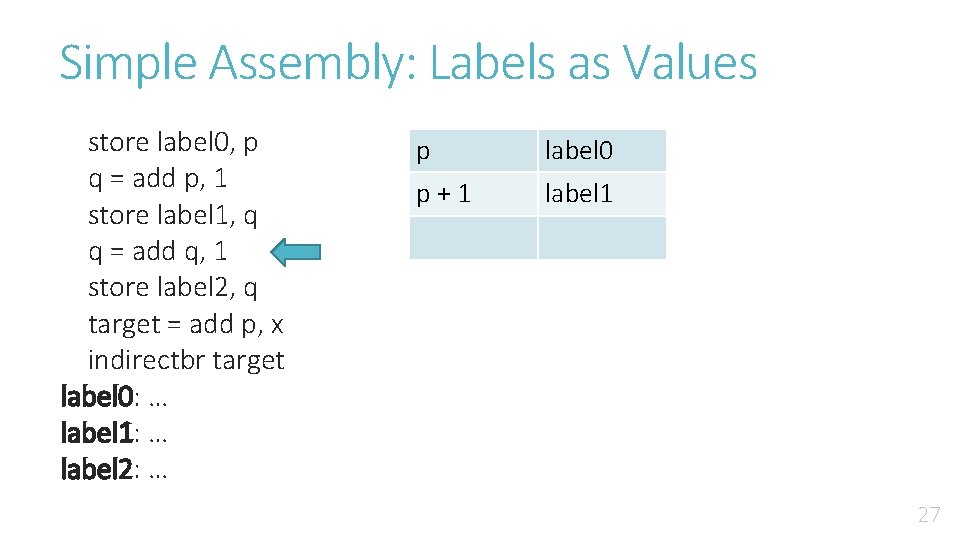
Simple Assembly: Labels as Values store label 0, p q = add p, 1 store label 1, q q = add q, 1 store label 2, q target = add p, x indirectbr target label 0: … label 1: … label 2: … p label 0 p + 1 label 1 27
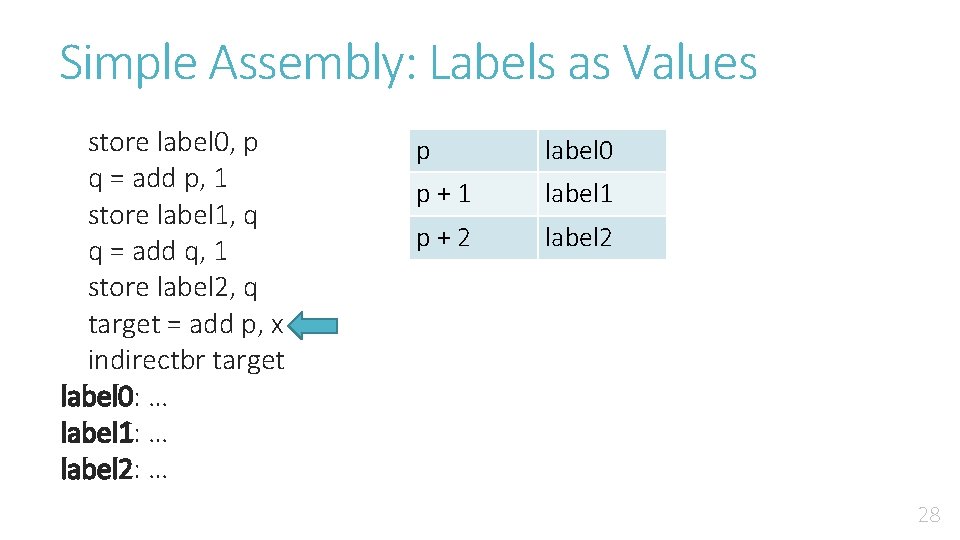
Simple Assembly: Labels as Values store label 0, p q = add p, 1 store label 1, q q = add q, 1 store label 2, q target = add p, x indirectbr target label 0: … label 1: … label 2: … p label 0 p + 1 label 1 p + 2 label 2 28
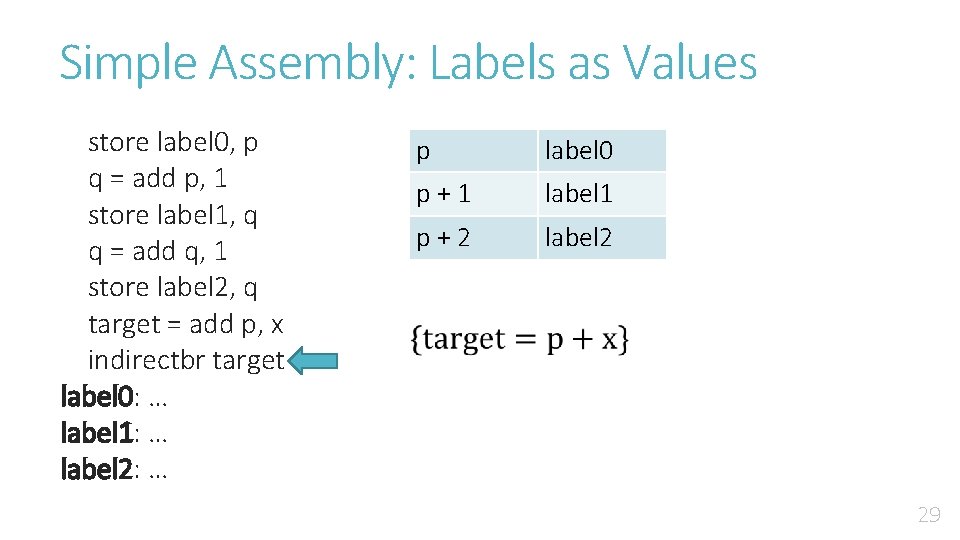
Simple Assembly: Labels as Values store label 0, p q = add p, 1 store label 1, q q = add q, 1 store label 2, q target = add p, x indirectbr target label 0: … label 1: … label 2: … p label 0 p + 1 label 1 p + 2 label 2 29
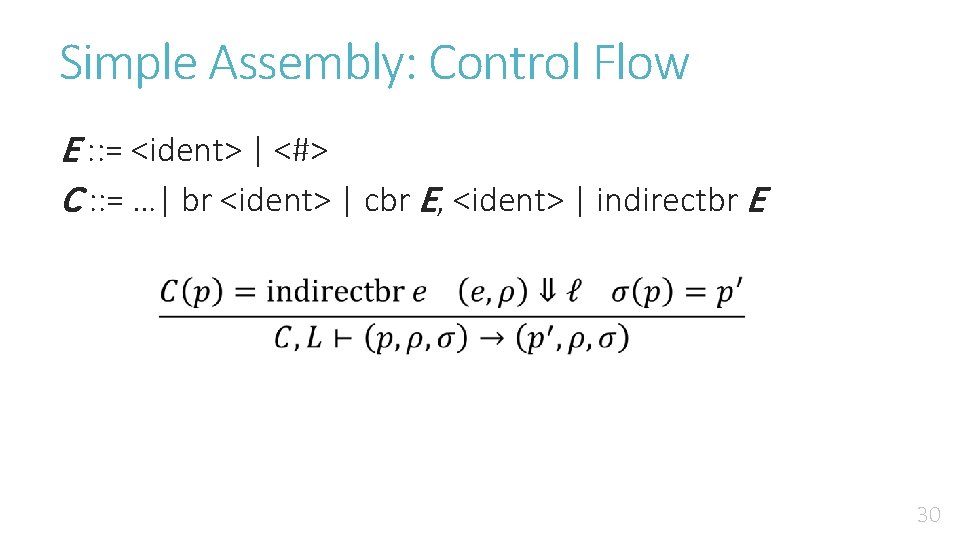
Simple Assembly: Control Flow E : : = <ident> | <#> C : : = …| br <ident> | cbr E, <ident> | indirectbr E 30
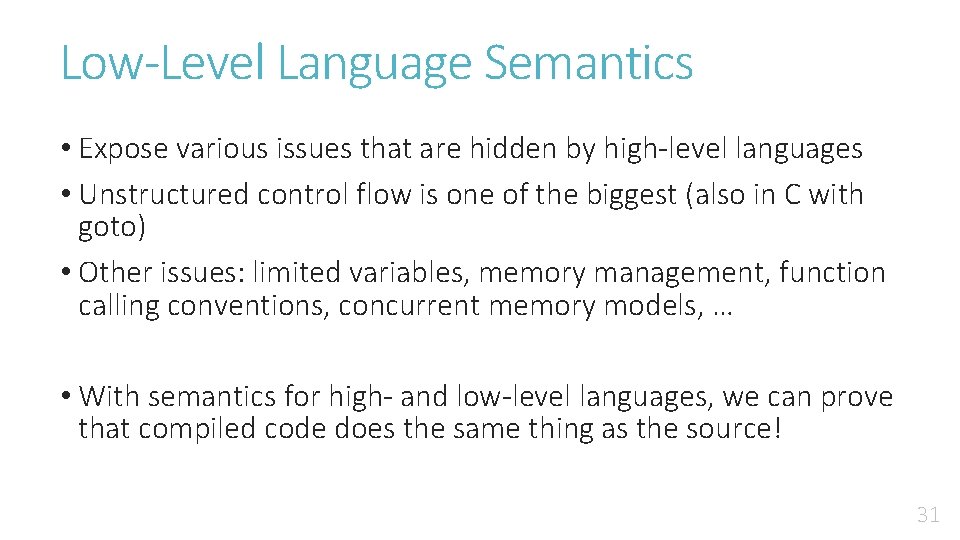
Low-Level Language Semantics • Expose various issues that are hidden by high-level languages • Unstructured control flow is one of the biggest (also in C with goto) • Other issues: limited variables, memory management, function calling conventions, concurrent memory models, … • With semantics for high- and low-level languages, we can prove that compiled code does the same thing as the source! 31
William mansky
William mansky
William mansky
500 - 476
Cs 476
What are compatible numbers
476 a 1453
Renascimento urbano
Symmetrical components in power system pdf
Paminklas
476 a 1453
That "rome fell in a.d. 476" means
12 tables of roman law
476 fighter group
Bistogram cikajang
476 bus times
Ece 476
Ece 476
Dhol chantier
Stipta
Ntp 476
I.sz. 476
Resolve
Ece 476
476 ce
Ece 476
22power dot com
Ece 476
Ece 476
Ece 476
What is hdl hardware description language
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming