CS 314 Section 5 Recitation 7 Long Zhao
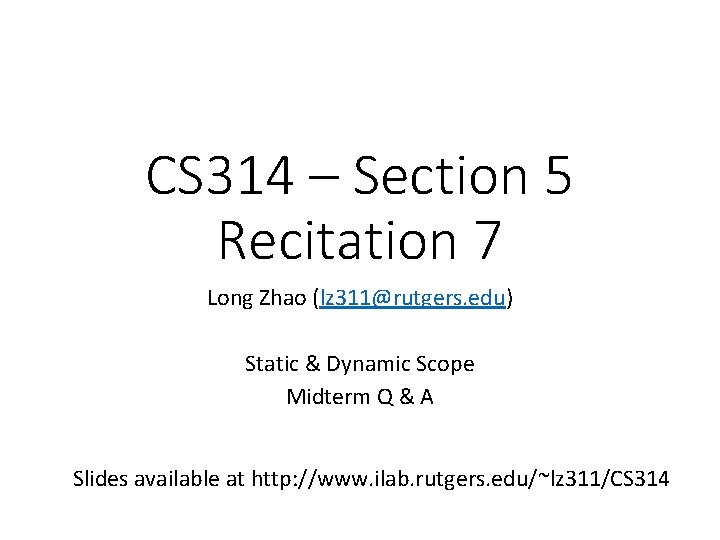
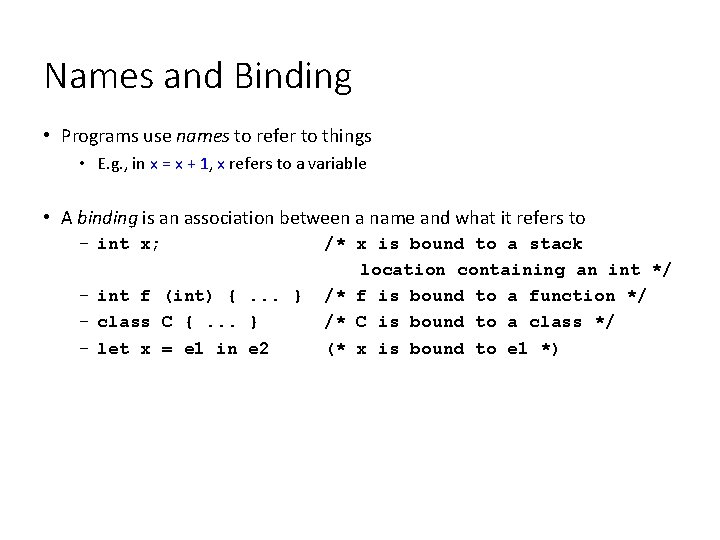
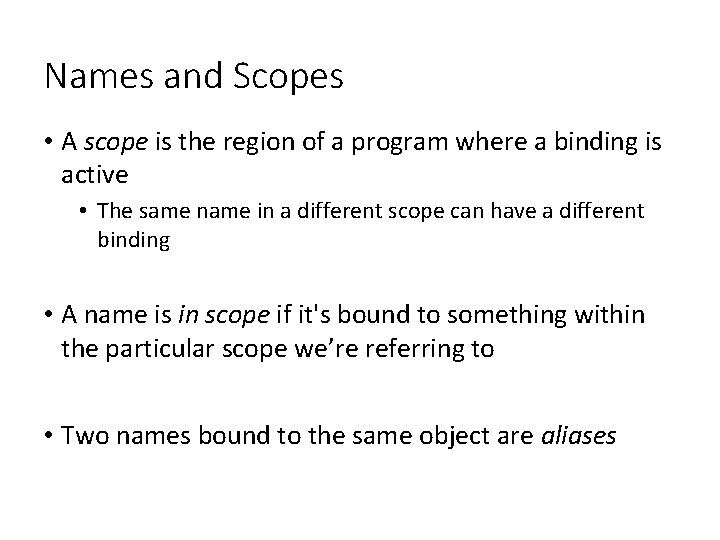
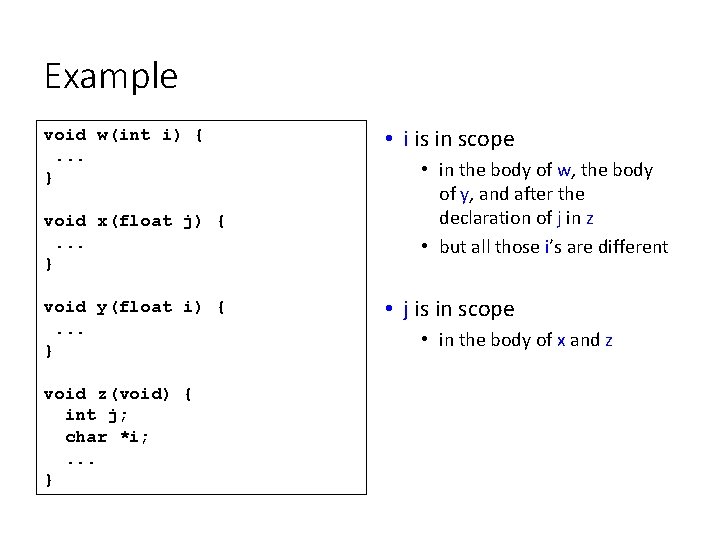
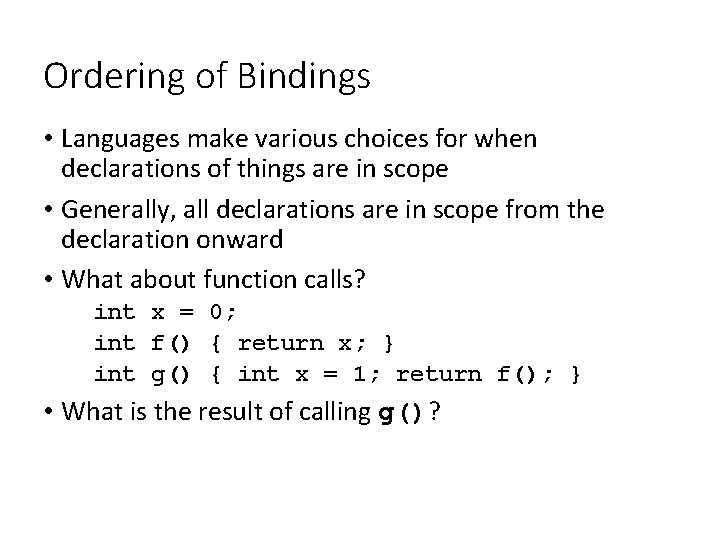
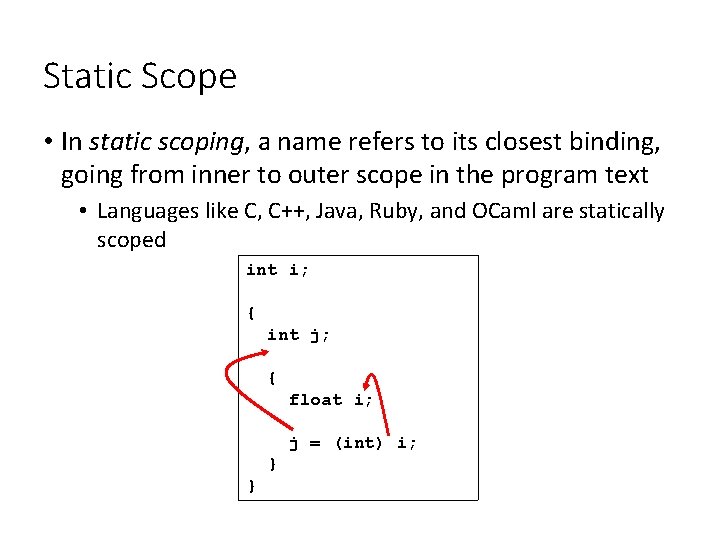
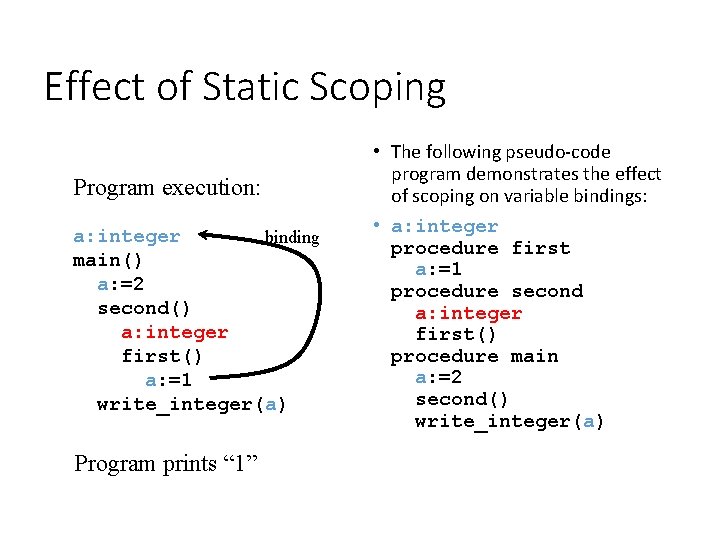
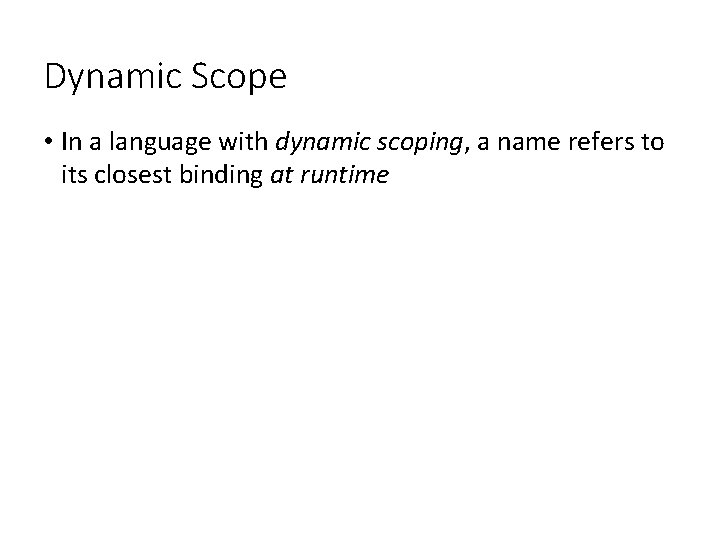
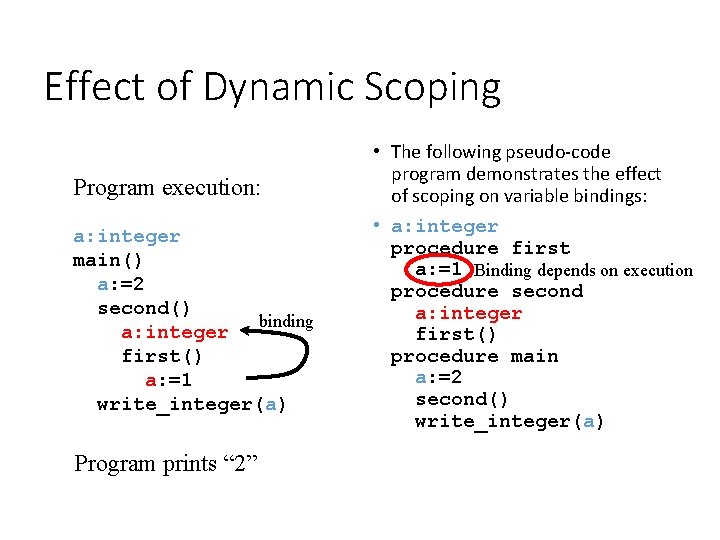
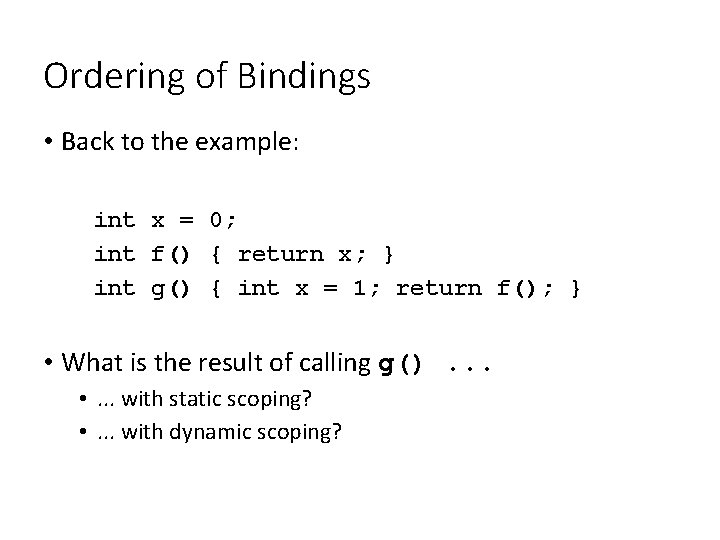
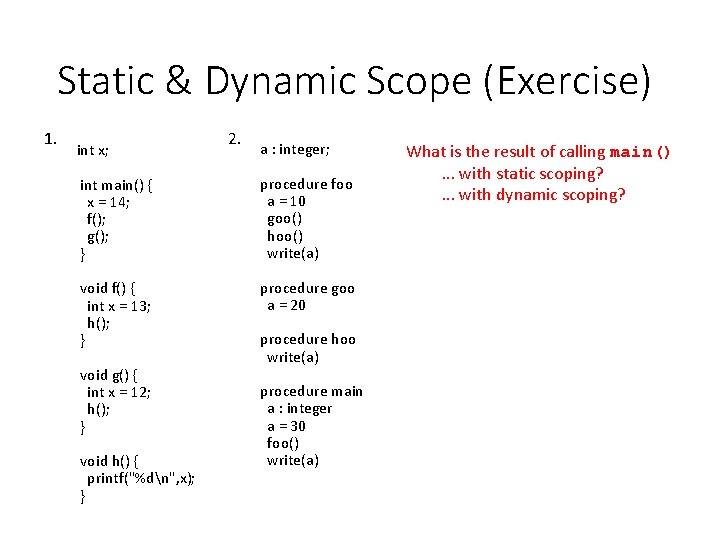
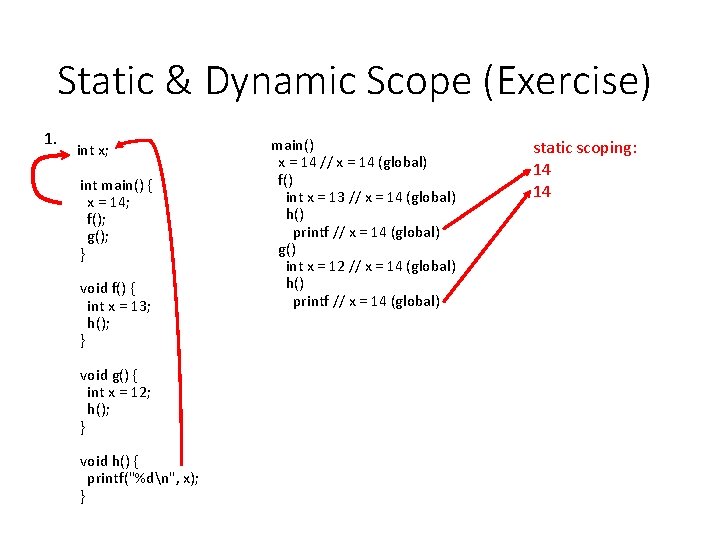
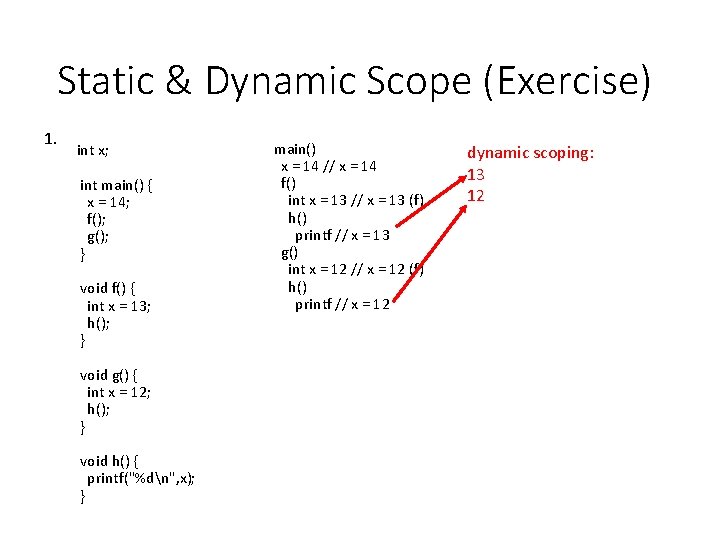
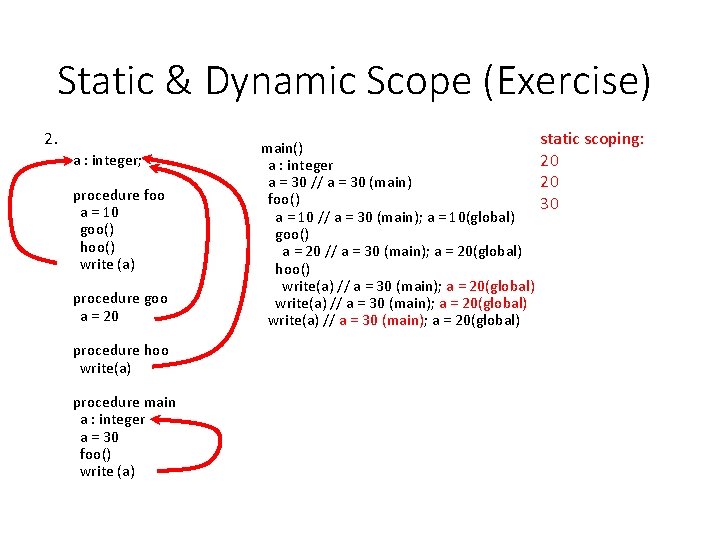
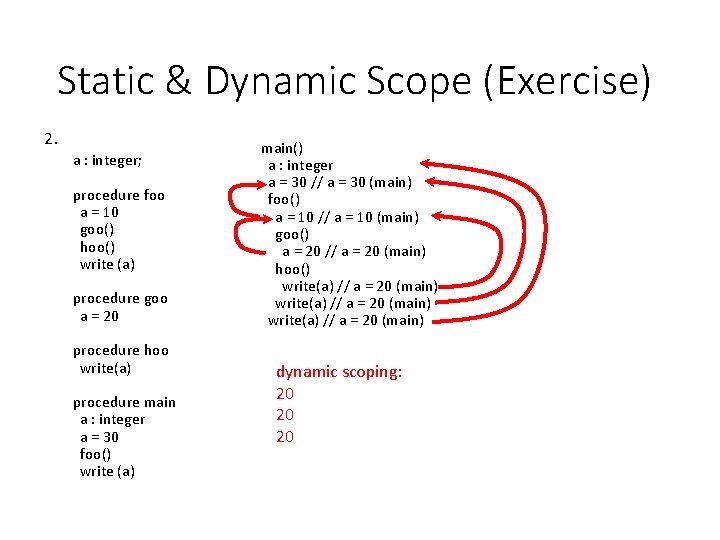
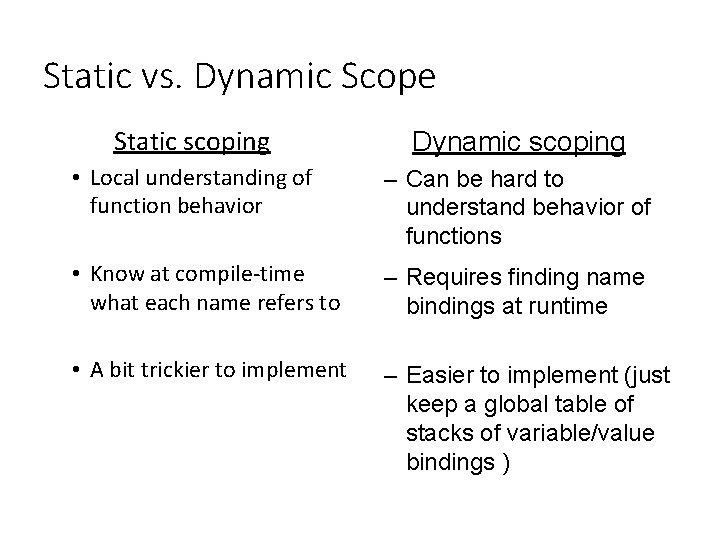
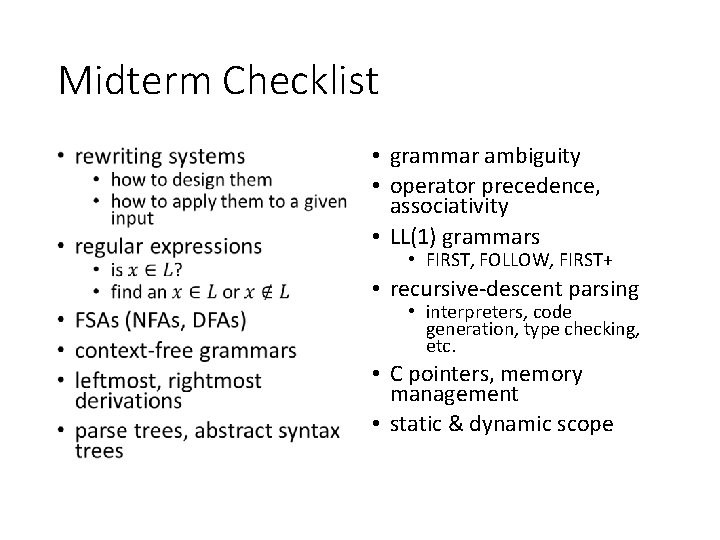
- Slides: 17
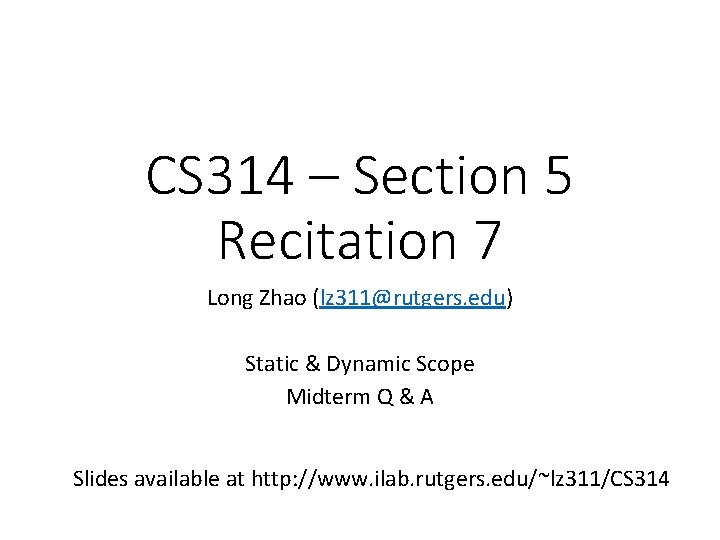
CS 314 – Section 5 Recitation 7 Long Zhao (lz 311@rutgers. edu) Static & Dynamic Scope Midterm Q & A Slides available at http: //www. ilab. rutgers. edu/~lz 311/CS 314
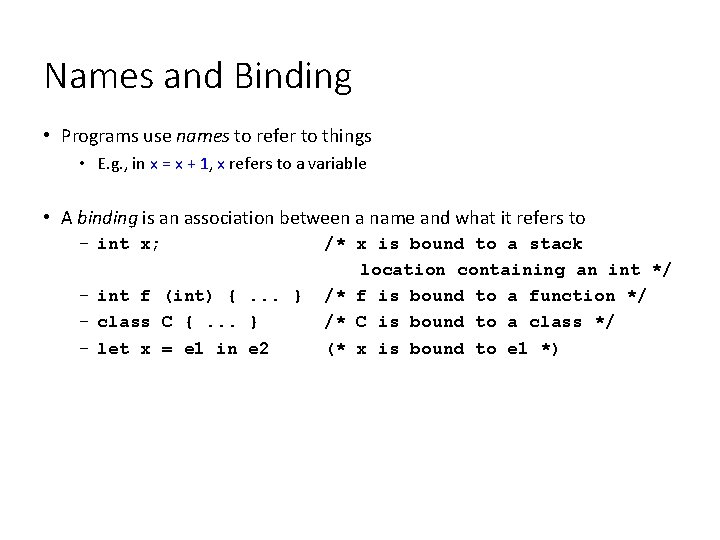
Names and Binding • Programs use names to refer to things • E. g. , in x = x + 1, x refers to a variable • A binding is an association between a name and what it refers to – int x; /* x is bound to a stack location containing an int */ – int f (int) {. . . } /* f is bound to a function */ – class C {. . . } /* C is bound to a class */ – let x = e 1 in e 2 (* x is bound to e 1 *)
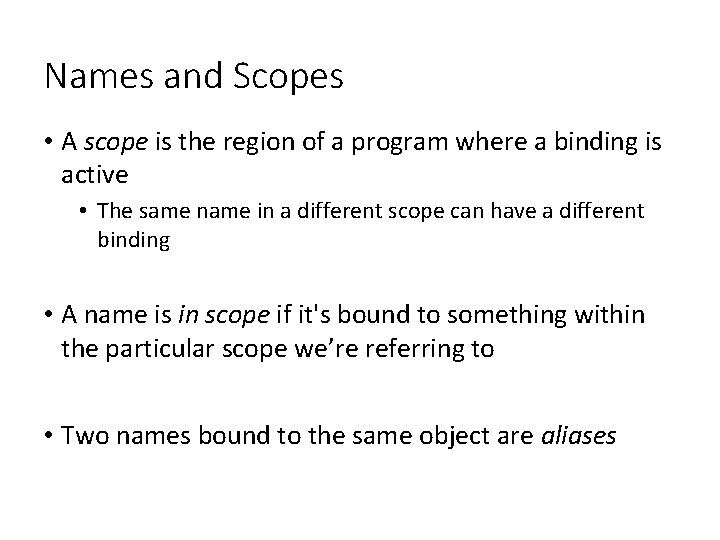
Names and Scopes • A scope is the region of a program where a binding is active • The same name in a different scope can have a different binding • A name is in scope if it's bound to something within the particular scope we’re referring to • Two names bound to the same object are aliases
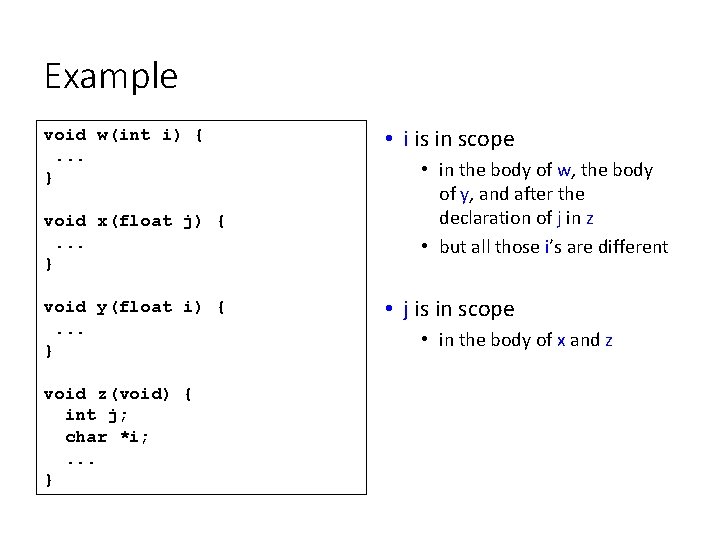
Example void w(int i) { . . . } void x(float j) { . . . } void y(float i) { . . . } void z(void) { int j; char *i; . . . } • i is in scope • in the body of w, the body of y, and after the declaration of j in z • but all those i’s are different • j is in scope • in the body of x and z
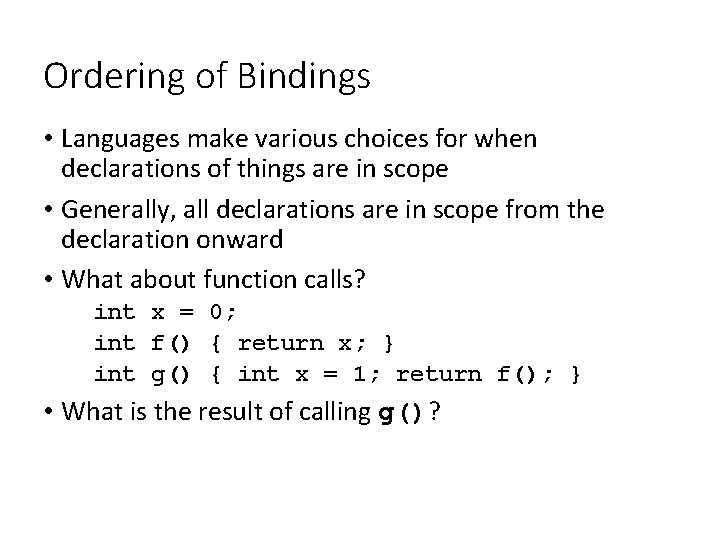
Ordering of Bindings • Languages make various choices for when declarations of things are in scope • Generally, all declarations are in scope from the declaration onward • What about function calls? int x = 0; int f() { return x; } int g() { int x = 1; return f(); } • What is the result of calling g()?
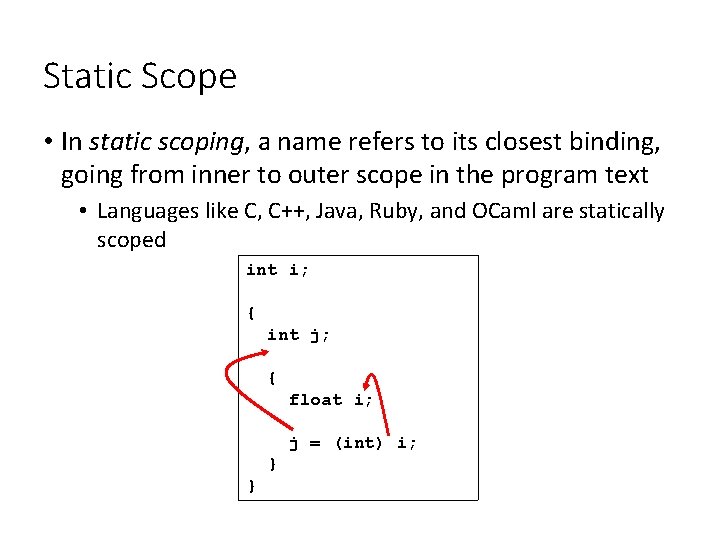
Static Scope • In static scoping, a name refers to its closest binding, going from inner to outer scope in the program text • Languages like C, C++, Java, Ruby, and OCaml are statically scoped int i; { int j; { float i; j = (int) i; } }
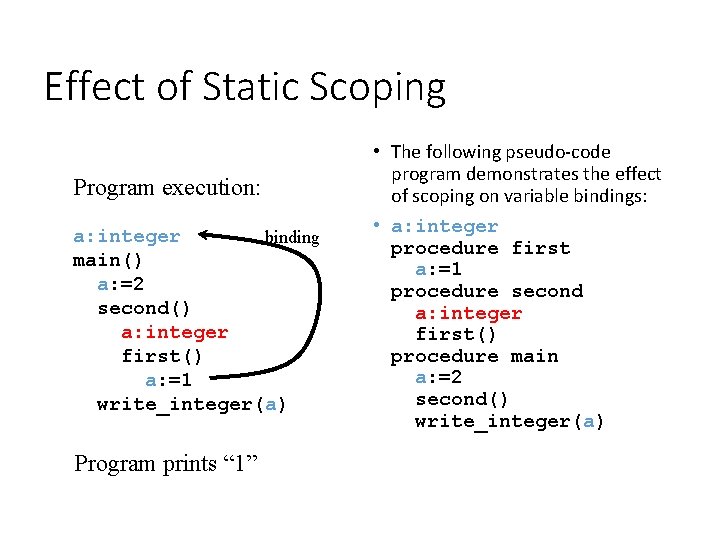
Effect of Static Scoping Program execution: a: integer binding main() a: =2 second() a: integer first() a: =1 write_integer(a) Program prints “ 1” • The following pseudo-code program demonstrates the effect of scoping on variable bindings: • a: integer procedure first a: =1 procedure second a: integer first() procedure main a: =2 second() write_integer(a)
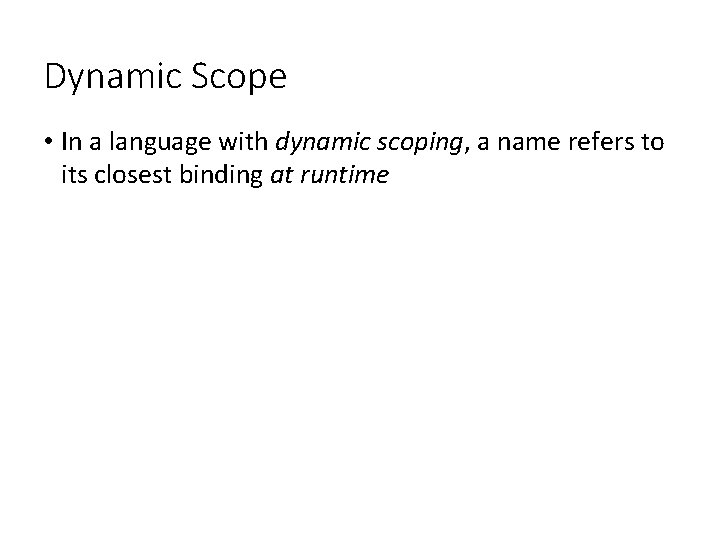
Dynamic Scope • In a language with dynamic scoping, a name refers to its closest binding at runtime
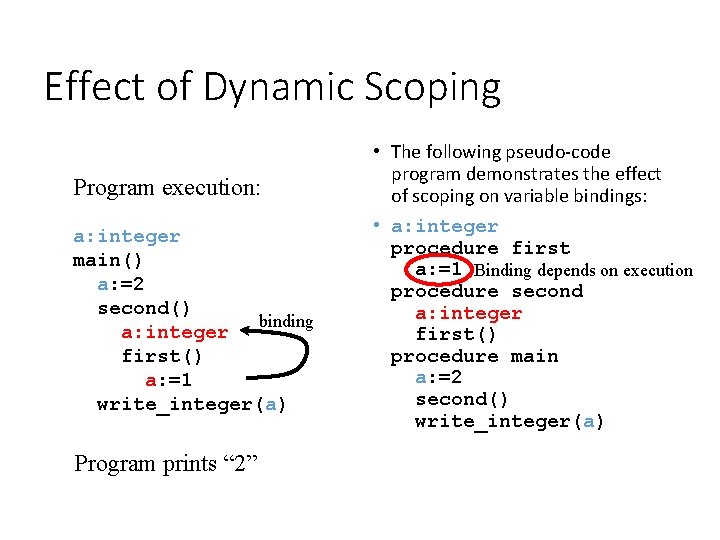
Effect of Dynamic Scoping Program execution: a: integer main() a: =2 second() binding a: integer first() a: =1 write_integer(a) Program prints “ 2” • The following pseudo-code program demonstrates the effect of scoping on variable bindings: • a: integer procedure first a: =1 Binding depends on execution procedure second a: integer first() procedure main a: =2 second() write_integer(a)
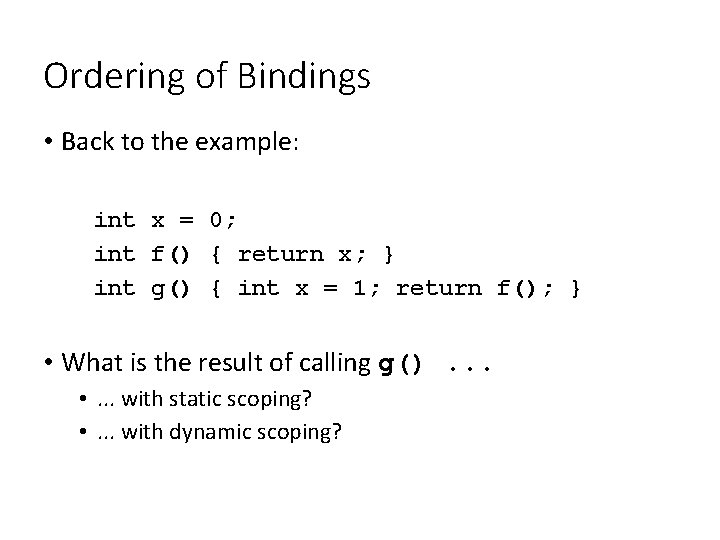
Ordering of Bindings • Back to the example: int x = 0; int f() { return x; } int g() { int x = 1; return f(); } • What is the result of calling g(). . . • . . . with static scoping? • . . . with dynamic scoping?
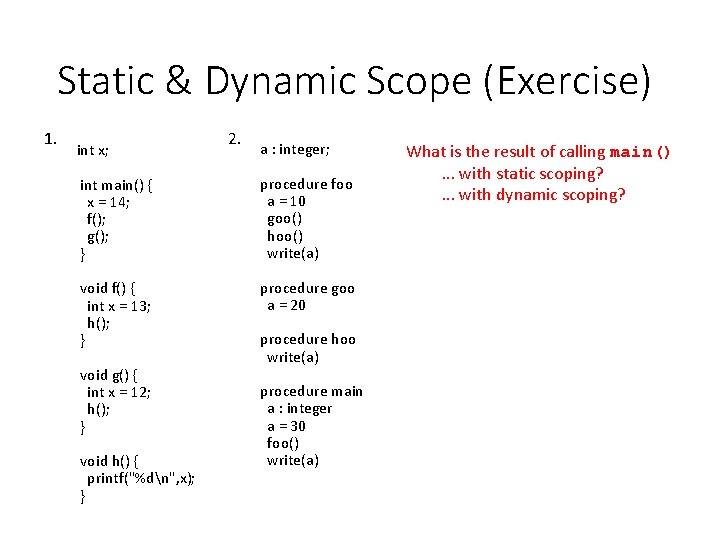
Static & Dynamic Scope (Exercise) 1. int x; 2. a : integer; int main() { x = 14; f(); g(); } procedure foo a = 10 goo() hoo() write(a) void f() { int x = 13; h(); } procedure goo a = 20 void g() { int x = 12; h(); } void h() { printf("%dn", x); } procedure hoo write(a) procedure main a : integer a = 30 foo() write(a) What is the result of calling main(). . . with static scoping? . . . with dynamic scoping?
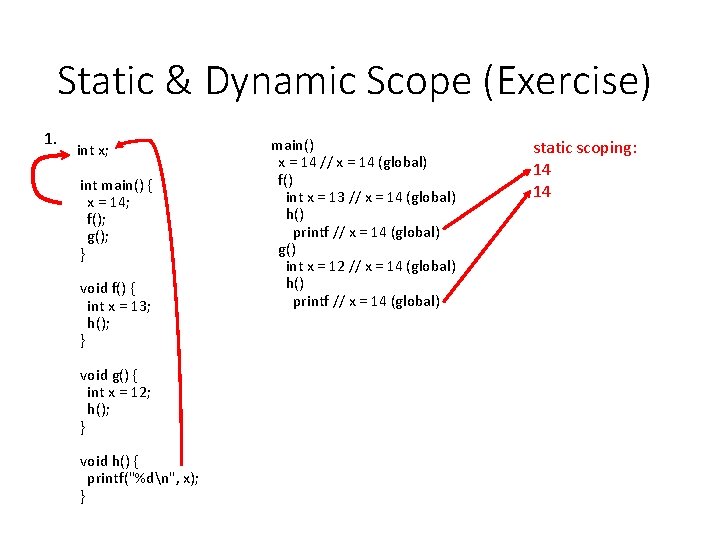
Static & Dynamic Scope (Exercise) 1. int x; int main() { x = 14; f(); g(); } void f() { int x = 13; h(); } void g() { int x = 12; h(); } void h() { printf("%dn", x); } main() x = 14 // x = 14 (global) f() int x = 13 // x = 14 (global) h() printf // x = 14 (global) g() int x = 12 // x = 14 (global) h() printf // x = 14 (global) static scoping: 14 14
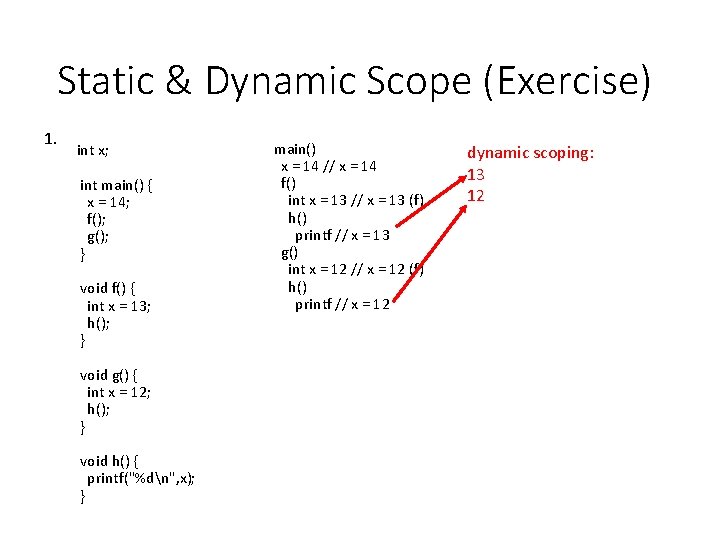
Static & Dynamic Scope (Exercise) 1. int x; int main() { x = 14; f(); g(); } void f() { int x = 13; h(); } void g() { int x = 12; h(); } void h() { printf("%dn", x); } main() x = 14 // x = 14 f() int x = 13 // x = 13 (f) h() printf // x = 13 g() int x = 12 // x = 12 (f) h() printf // x = 12 dynamic scoping: 13 12
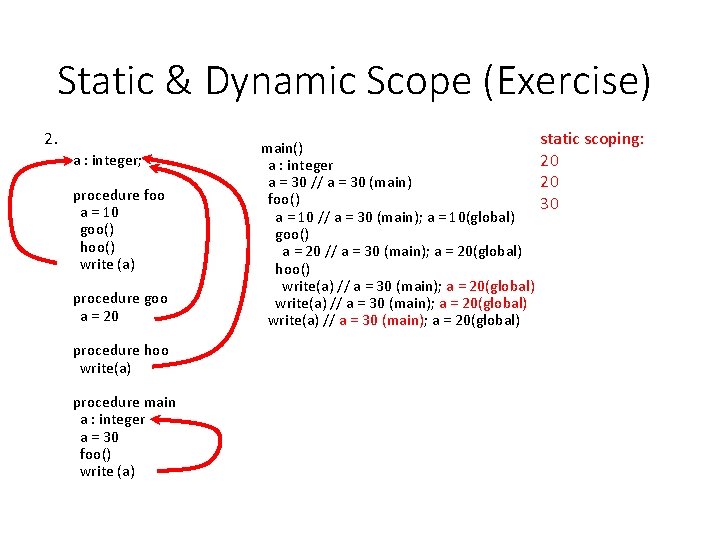
Static & Dynamic Scope (Exercise) 2. static scoping: a : integer; procedure foo a = 10 goo() hoo() write (a) procedure goo a = 20 procedure hoo write(a) procedure main a : integer a = 30 foo() write (a) main() 20 a : integer 20 a = 30 // a = 30 (main) foo() 30 a = 10 // a = 30 (main); a = 10(global) goo() a = 20 // a = 30 (main); a = 20(global) hoo() write(a) // a = 30 (main); a = 20(global)
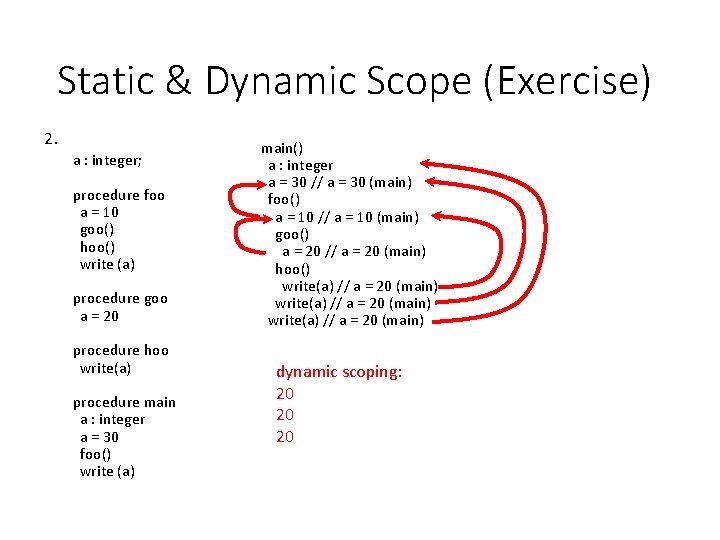
Static & Dynamic Scope (Exercise) 2. a : integer; procedure foo a = 10 goo() hoo() write (a) procedure goo a = 20 procedure hoo write(a) procedure main a : integer a = 30 foo() write (a) main() a : integer a = 30 // a = 30 (main) foo() a = 10 // a = 10 (main) goo() a = 20 // a = 20 (main) hoo() write(a) // a = 20 (main) dynamic scoping: 20 20 20
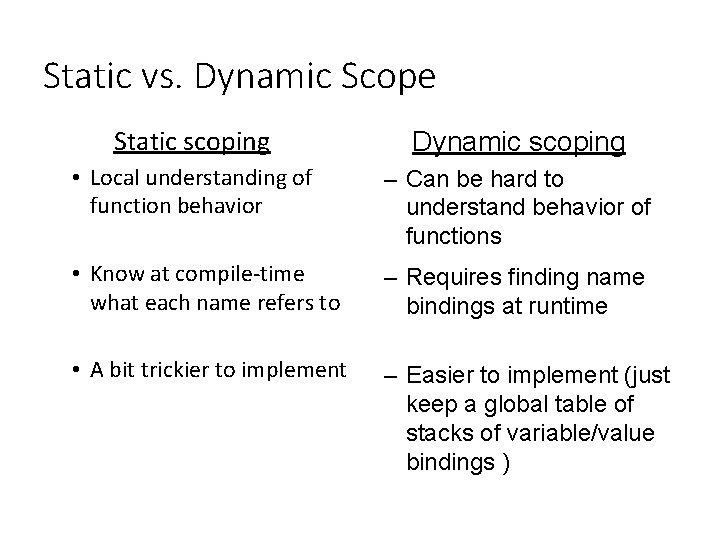
Static vs. Dynamic Scope Static scoping Dynamic scoping • Local understanding of function behavior – Can be hard to understand behavior of functions • Know at compile-time what each name refers to – Requires finding name bindings at runtime • A bit trickier to implement – Easier to implement (just keep a global table of stacks of variable/value bindings )
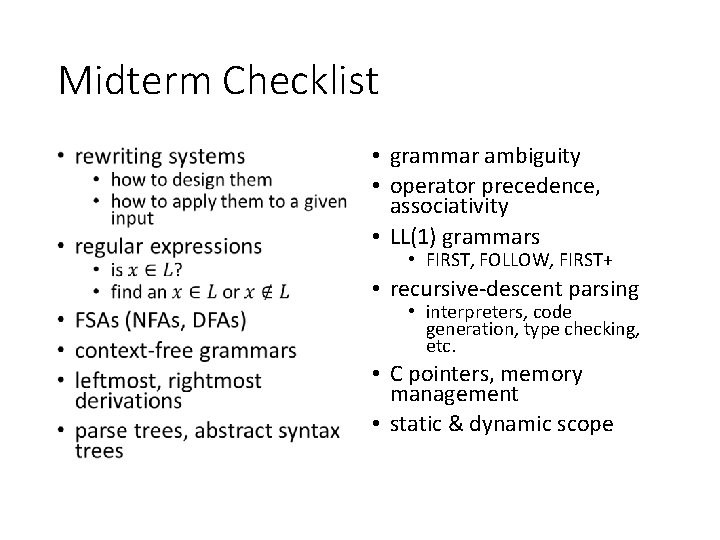
Midterm Checklist • • grammar ambiguity • operator precedence, associativity • LL(1) grammars • FIRST, FOLLOW, FIRST+ • recursive-descent parsing • interpreters, code generation, type checking, etc. • C pointers, memory management • static & dynamic scope
Tall + short h
Once upon a time a little man
Passive recitation
Quood posture 8
Impromptu speaking business
Poem recitation objectives
Expressive poems for recitation
What is meant by etiquette of recitation of the holy quran
Xvvvxv
Design a museum exhibit holistic rubric
Récitation les hiboux
Hui zhao unlv
Lilac zihui zhao
Guttman effect
Zhao chun
Zhao yuqi
Irene zhao
Jeremy zhao