CS 297 Graphics with Java and Open GL
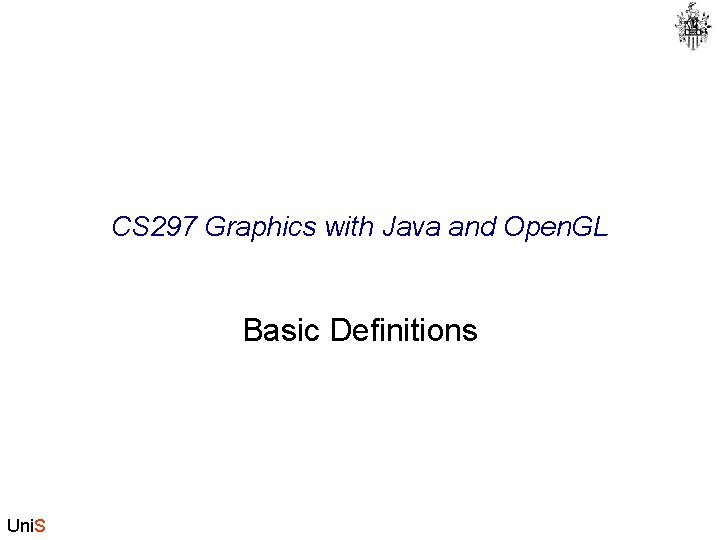
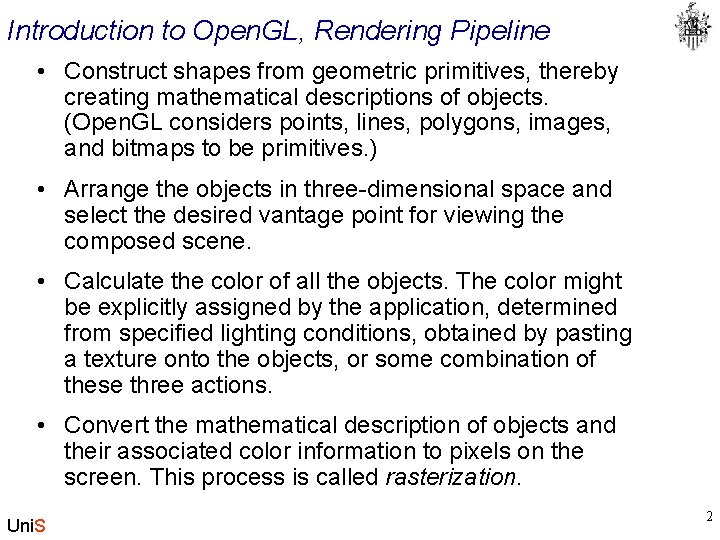
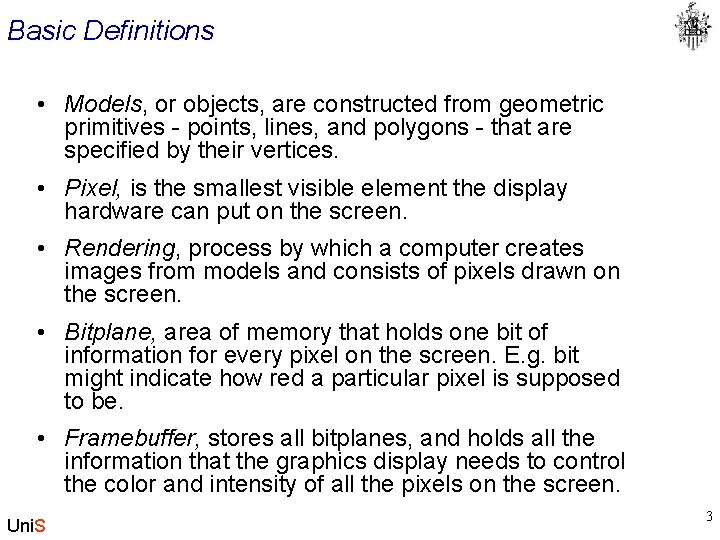
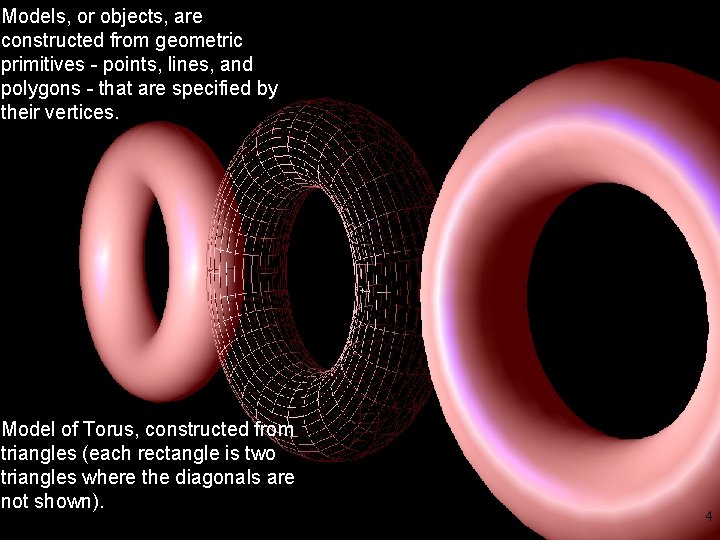
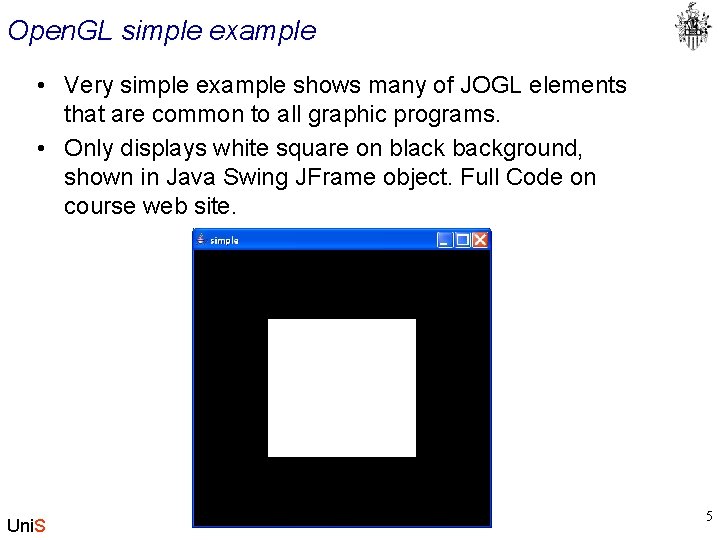
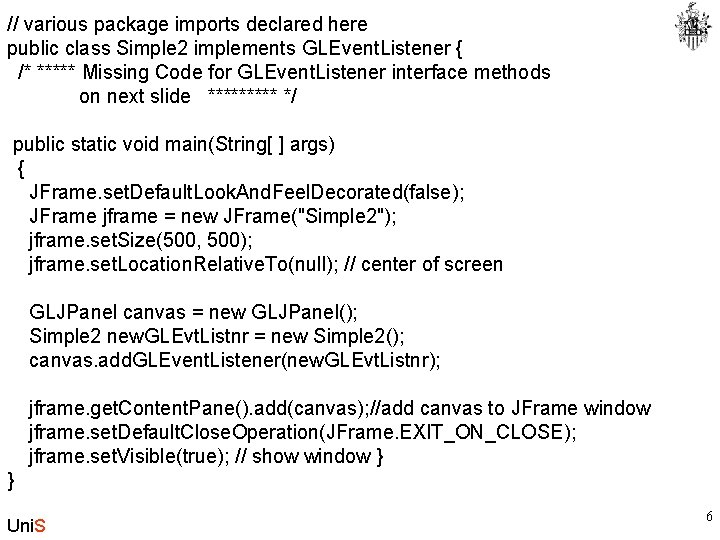
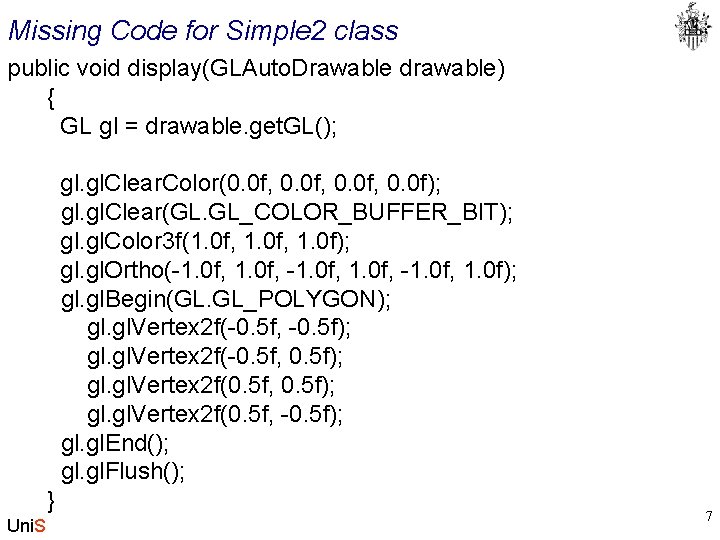
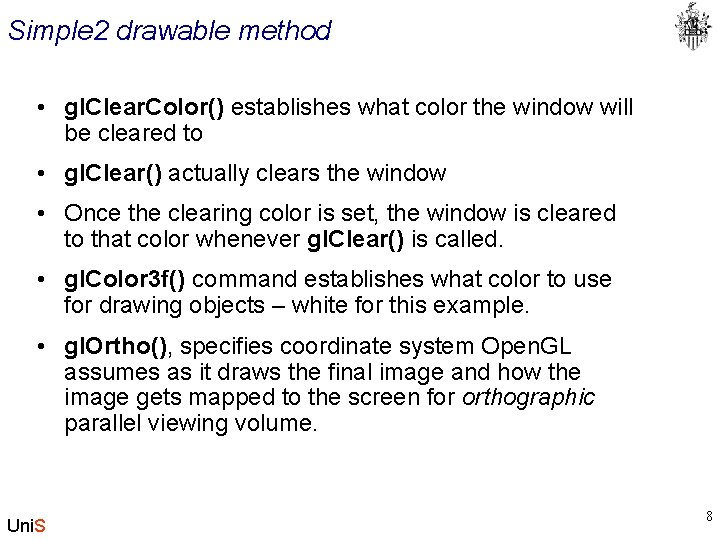
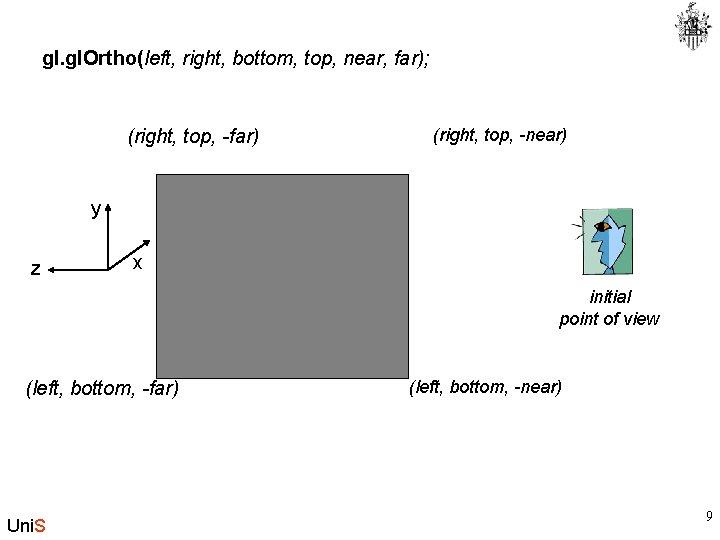
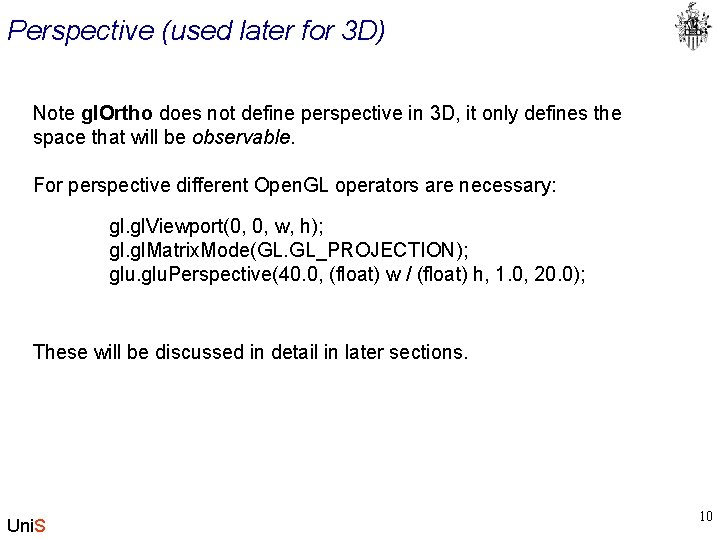
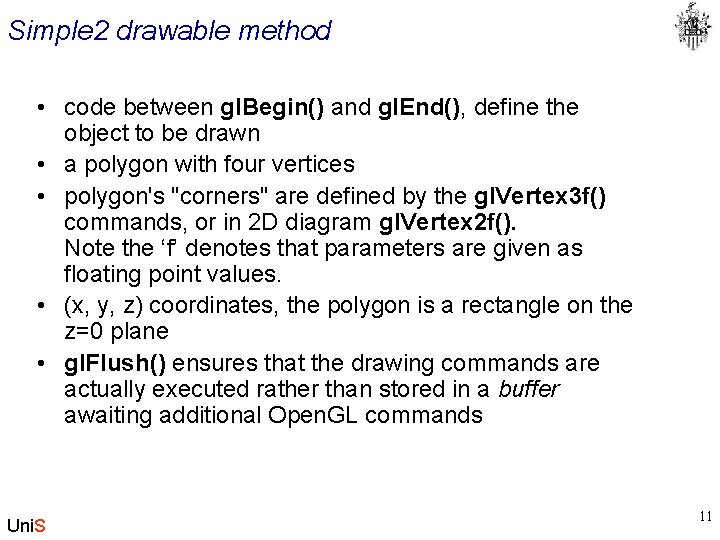
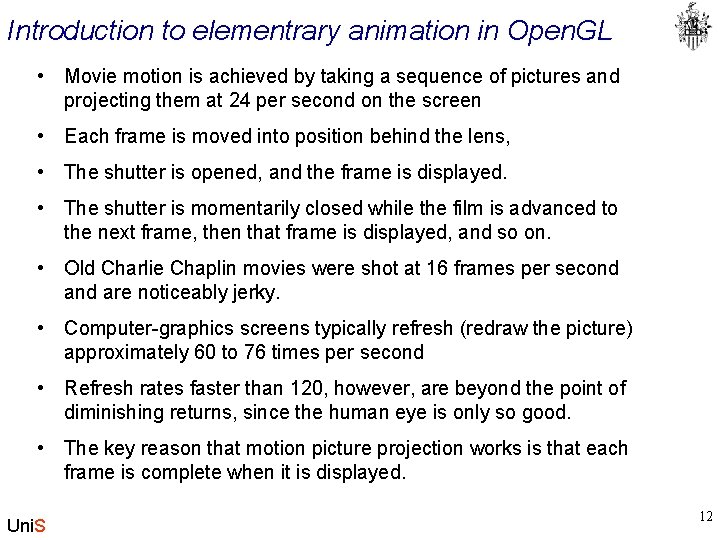
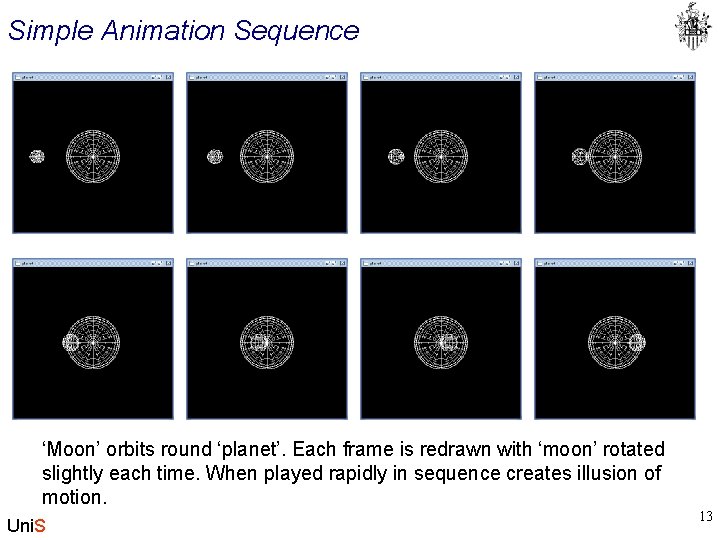
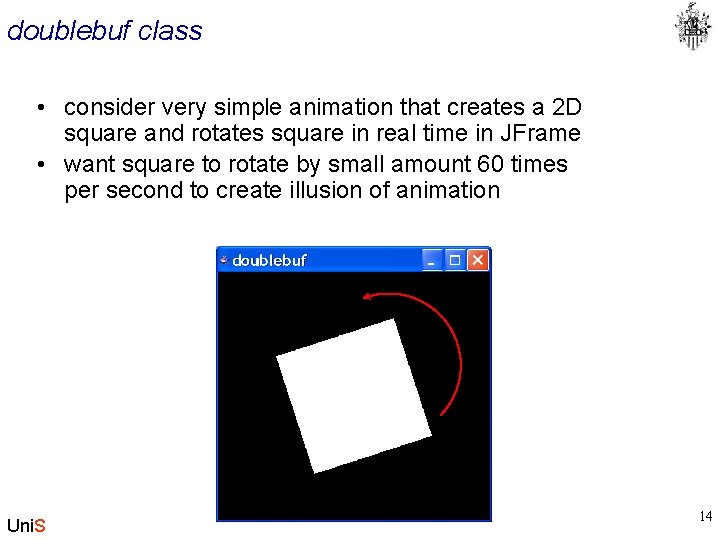
![doublebuf class example, using animator thread public static void main(String[ ] args) { /* doublebuf class example, using animator thread public static void main(String[ ] args) { /*](https://slidetodoc.com/presentation_image/6681466515049c223ecaeb5aed876c81/image-15.jpg)
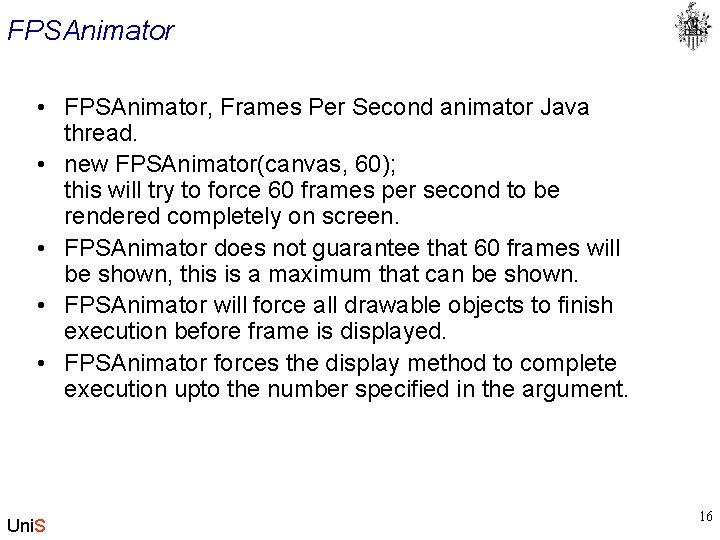
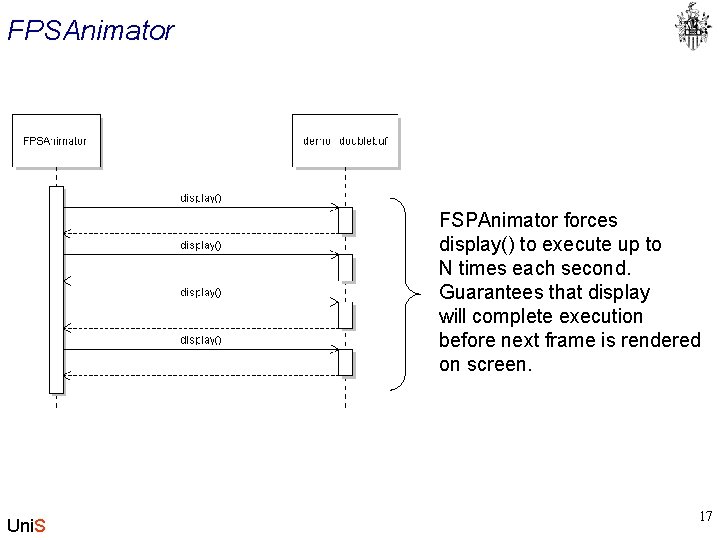
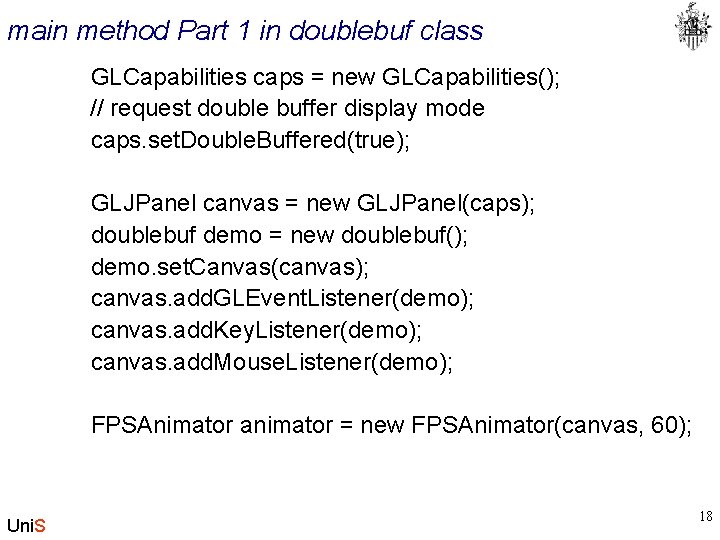
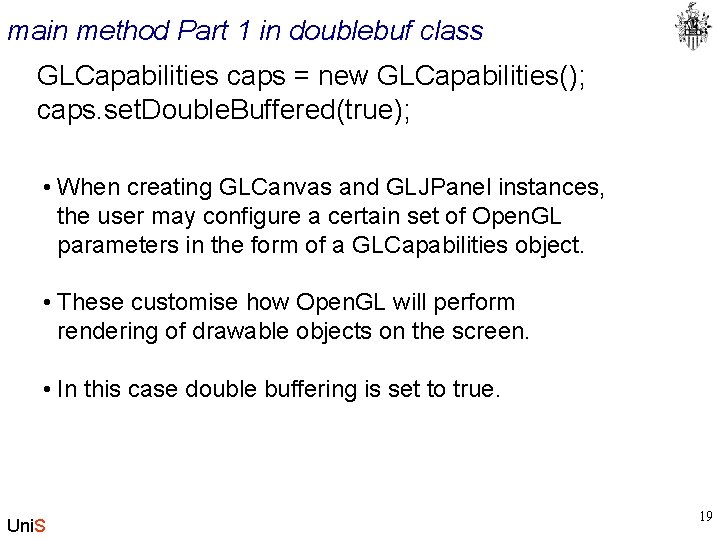
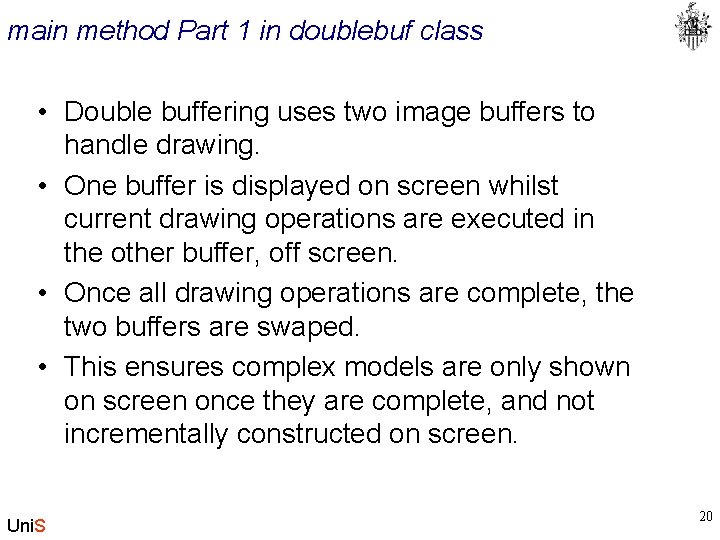
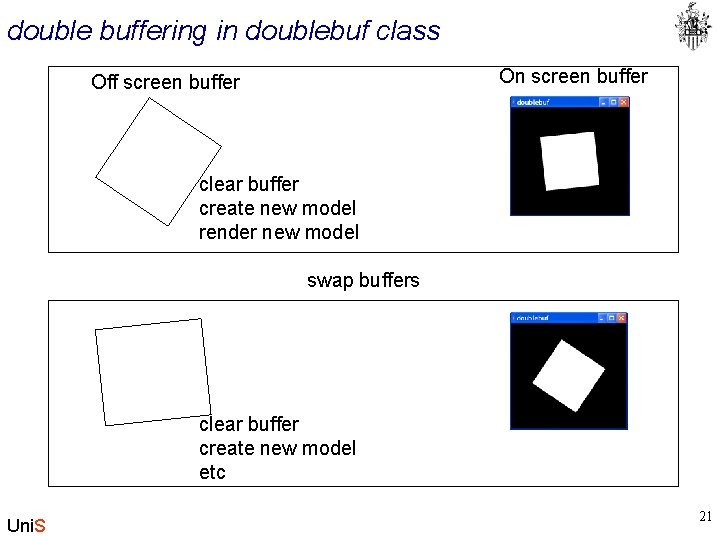
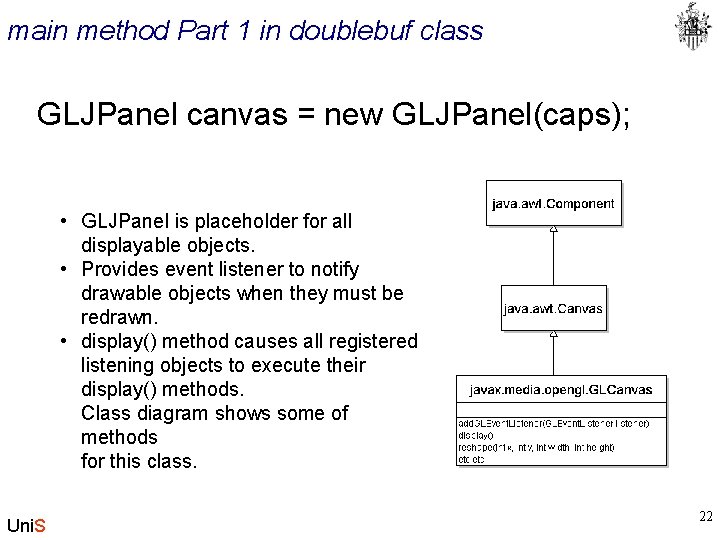
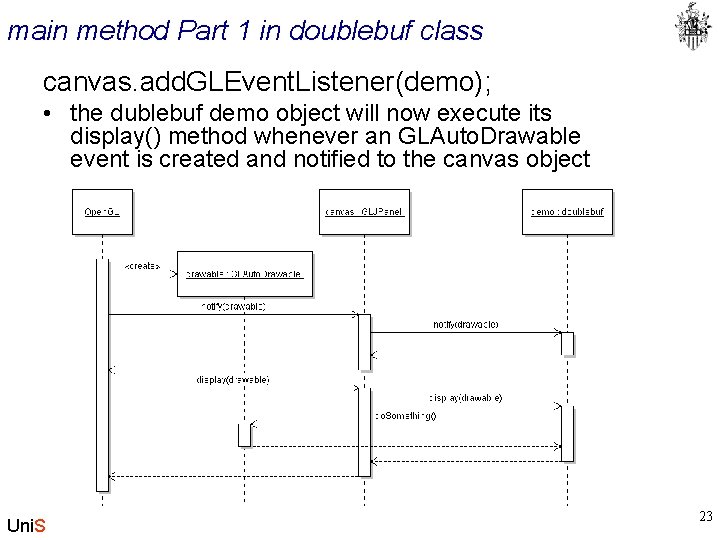
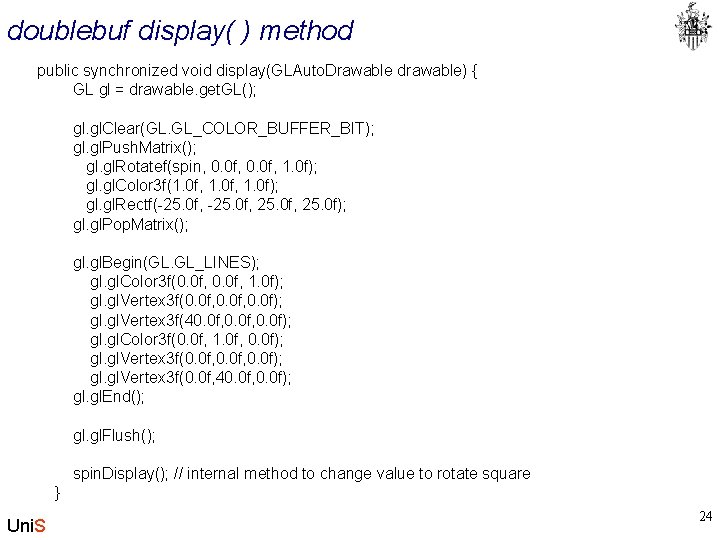
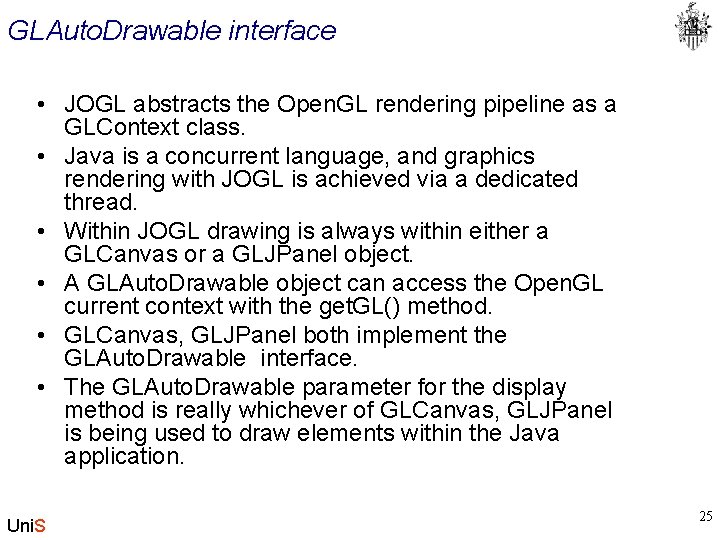
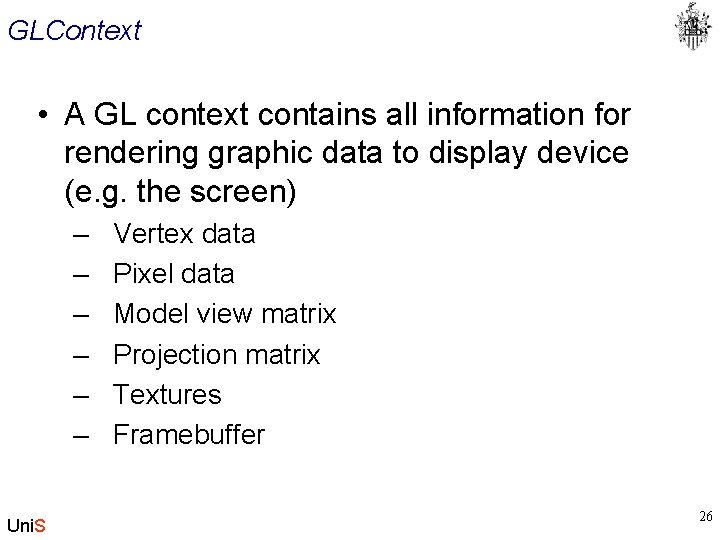
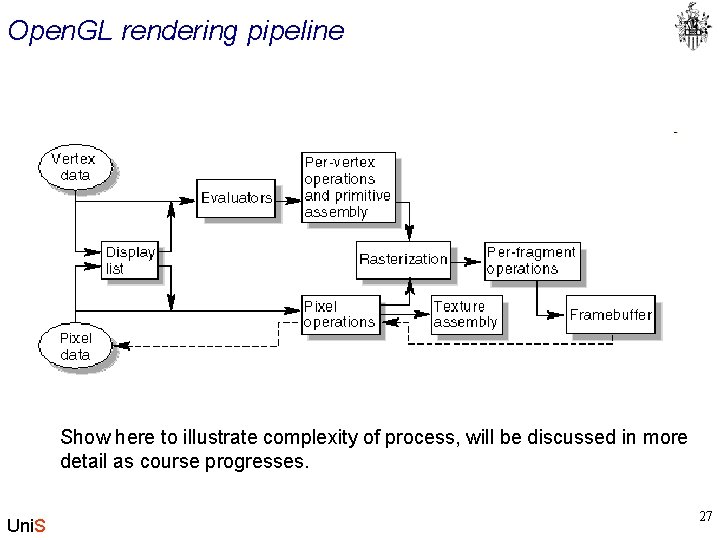
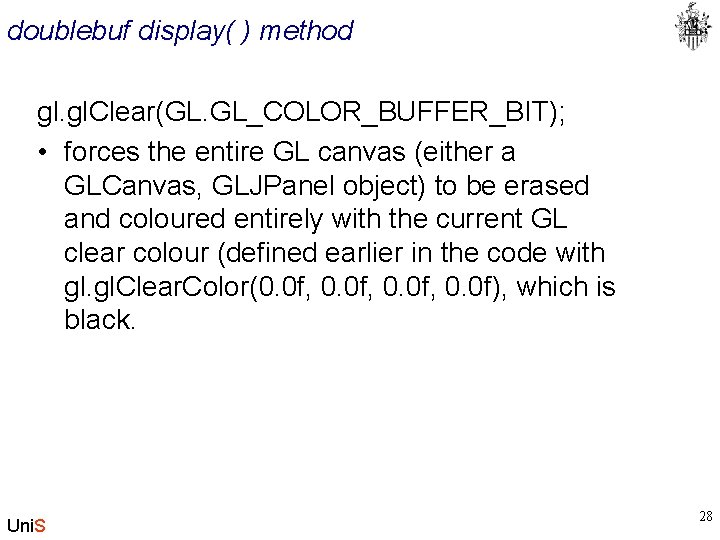
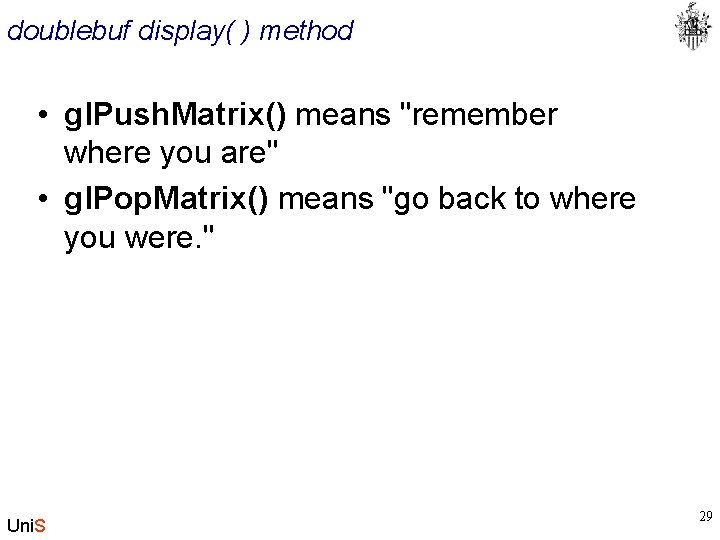
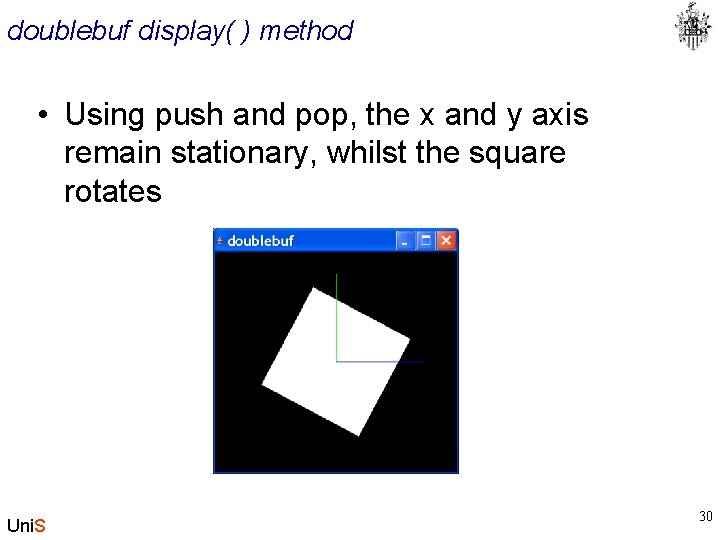
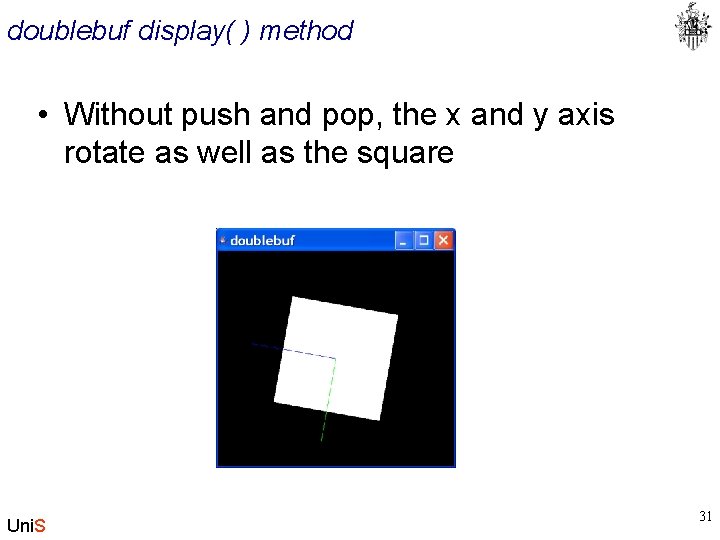
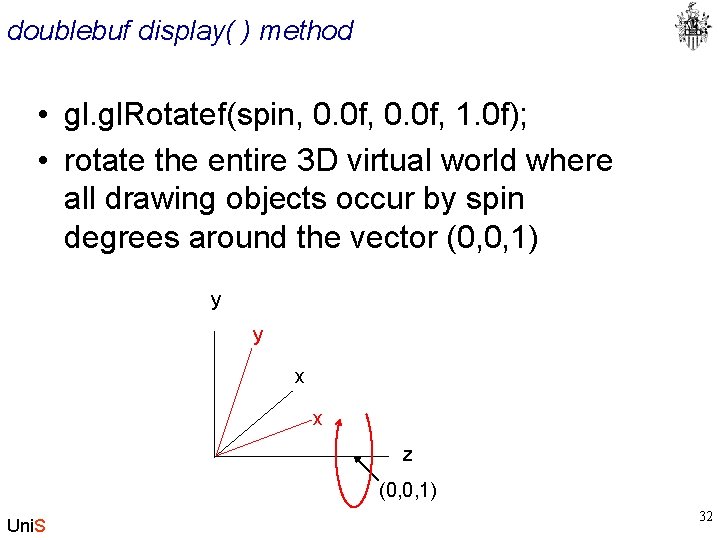
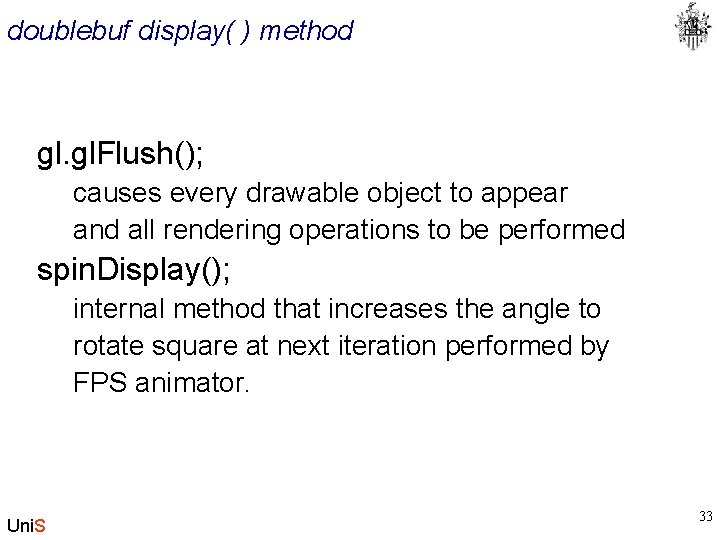
- Slides: 33
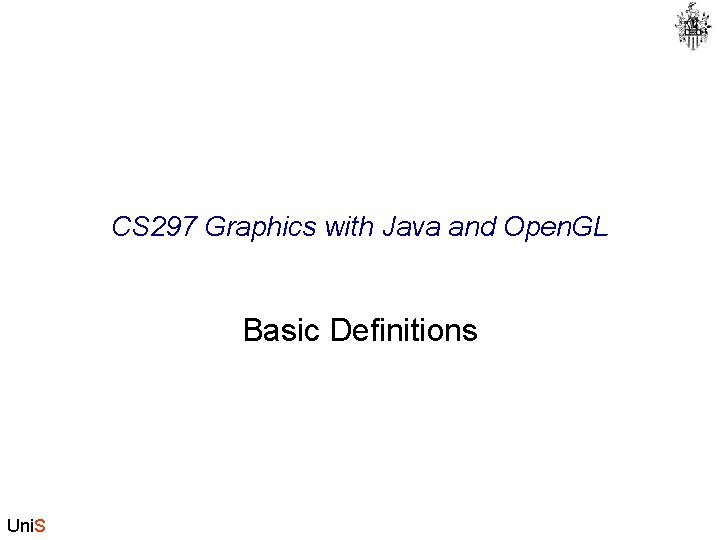
CS 297 Graphics with Java and Open. GL Basic Definitions Uni. S
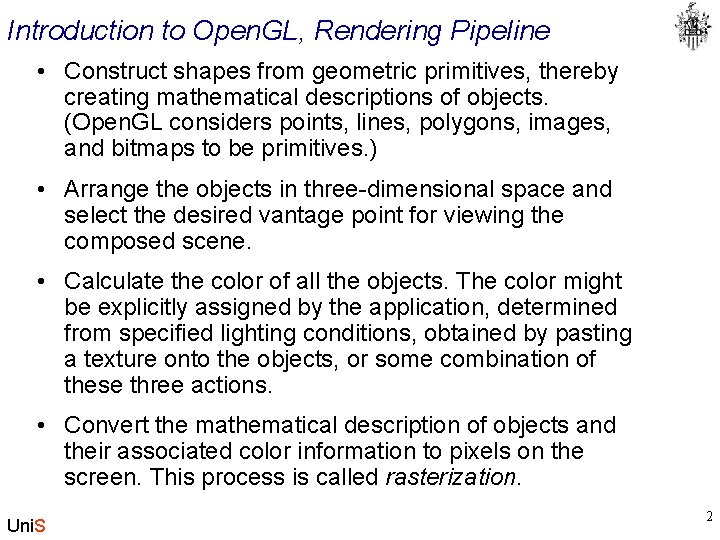
Introduction to Open. GL, Rendering Pipeline • Construct shapes from geometric primitives, thereby creating mathematical descriptions of objects. (Open. GL considers points, lines, polygons, images, and bitmaps to be primitives. ) • Arrange the objects in three-dimensional space and select the desired vantage point for viewing the composed scene. • Calculate the color of all the objects. The color might be explicitly assigned by the application, determined from specified lighting conditions, obtained by pasting a texture onto the objects, or some combination of these three actions. • Convert the mathematical description of objects and their associated color information to pixels on the screen. This process is called rasterization. Uni. S 2
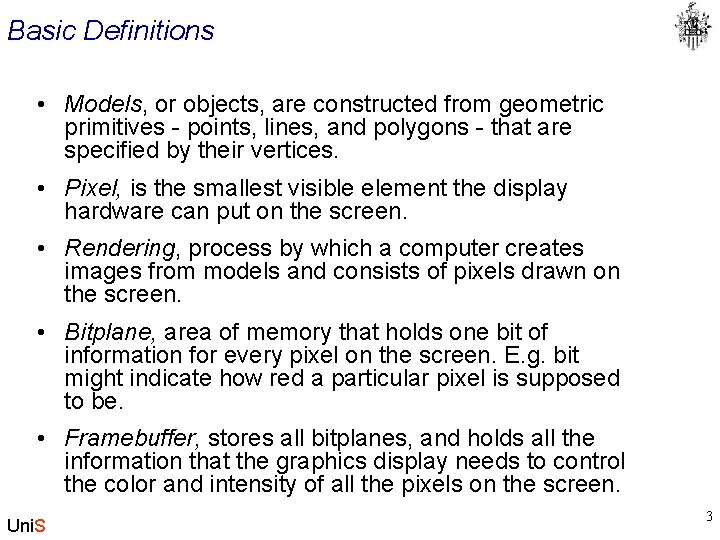
Basic Definitions • Models, or objects, are constructed from geometric primitives - points, lines, and polygons - that are specified by their vertices. • Pixel, is the smallest visible element the display hardware can put on the screen. • Rendering, process by which a computer creates images from models and consists of pixels drawn on the screen. • Bitplane, area of memory that holds one bit of information for every pixel on the screen. E. g. bit might indicate how red a particular pixel is supposed to be. • Framebuffer, stores all bitplanes, and holds all the information that the graphics display needs to control the color and intensity of all the pixels on the screen. Uni. S 3
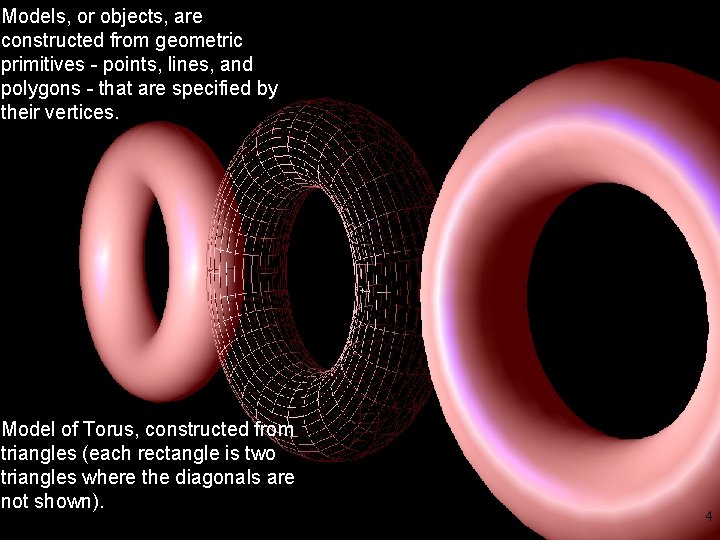
Models, or objects, are constructed from geometric primitives - points, lines, and polygons - that are specified by their vertices. Model of Torus, constructed from triangles (each rectangle is two triangles where the diagonals are not shown). Uni. S 4
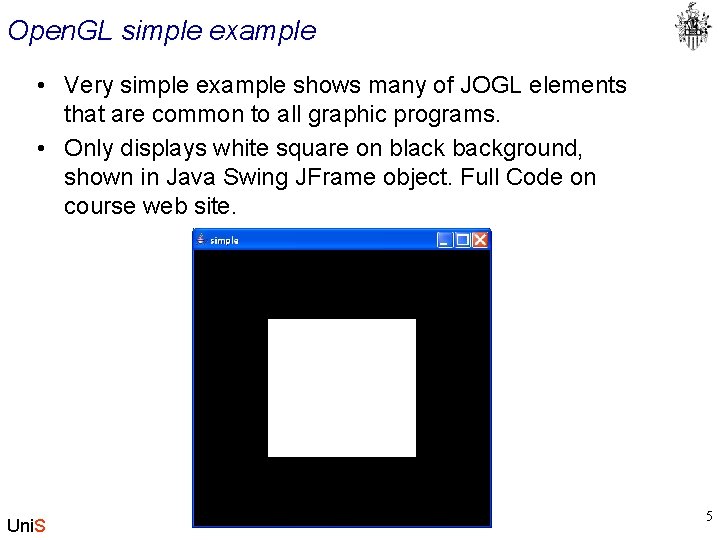
Open. GL simple example • Very simple example shows many of JOGL elements that are common to all graphic programs. • Only displays white square on black background, shown in Java Swing JFrame object. Full Code on course web site. Uni. S 5
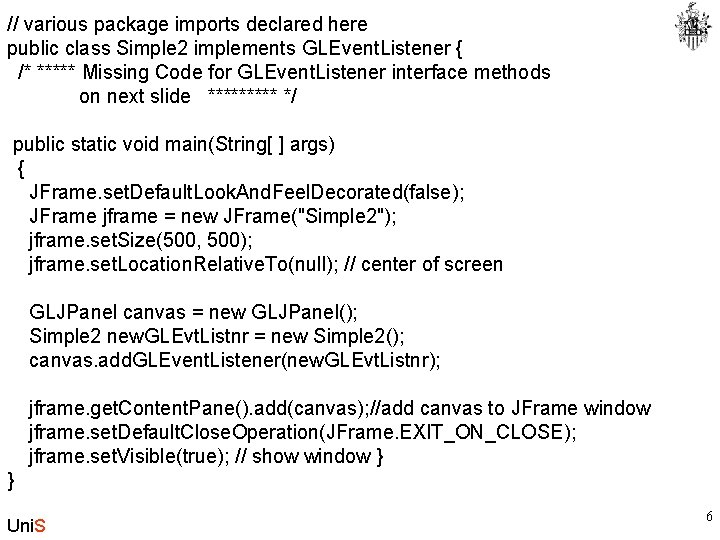
// various package imports declared here public class Simple 2 implements GLEvent. Listener { /* ***** Missing Code for GLEvent. Listener interface methods on next slide ***** */ public static void main(String[ ] args) { JFrame. set. Default. Look. And. Feel. Decorated(false); JFrame jframe = new JFrame("Simple 2"); jframe. set. Size(500, 500); jframe. set. Location. Relative. To(null); // center of screen GLJPanel canvas = new GLJPanel(); Simple 2 new. GLEvt. Listnr = new Simple 2(); canvas. add. GLEvent. Listener(new. GLEvt. Listnr); jframe. get. Content. Pane(). add(canvas); //add canvas to JFrame window jframe. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); jframe. set. Visible(true); // show window } } Uni. S 6
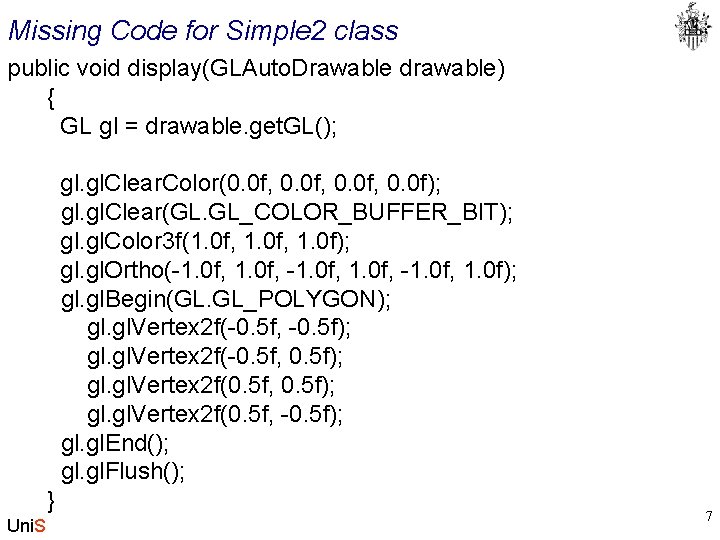
Missing Code for Simple 2 class public void display(GLAuto. Drawable drawable) { GL gl = drawable. get. GL(); gl. Clear. Color(0. 0 f, 0. 0 f); gl. Clear(GL. GL_COLOR_BUFFER_BIT); gl. Color 3 f(1. 0 f, 1. 0 f); gl. Ortho(-1. 0 f, -1. 0 f, 1. 0 f); gl. Begin(GL. GL_POLYGON); gl. Vertex 2 f(-0. 5 f, -0. 5 f); gl. Vertex 2 f(-0. 5 f, 0. 5 f); gl. gl. Vertex 2 f(0. 5 f, -0. 5 f); gl. End(); gl. Flush(); } Uni. S 7
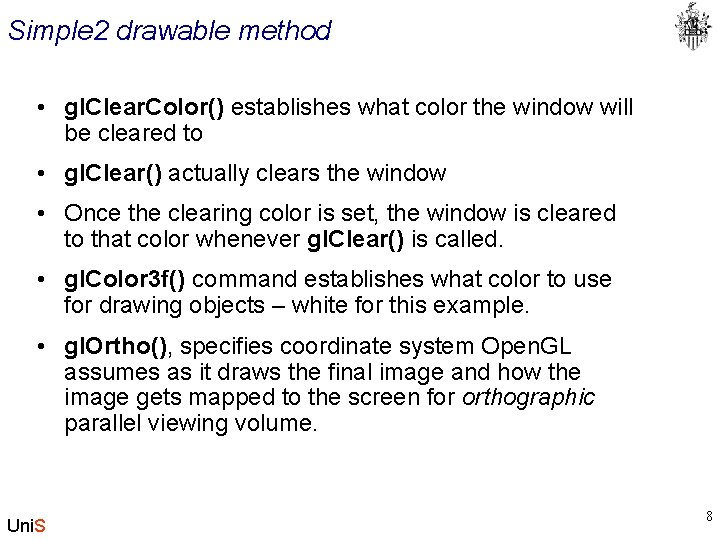
Simple 2 drawable method • gl. Clear. Color() establishes what color the window will be cleared to • gl. Clear() actually clears the window • Once the clearing color is set, the window is cleared to that color whenever gl. Clear() is called. • gl. Color 3 f() command establishes what color to use for drawing objects – white for this example. • gl. Ortho(), specifies coordinate system Open. GL assumes as it draws the final image and how the image gets mapped to the screen for orthographic parallel viewing volume. Uni. S 8
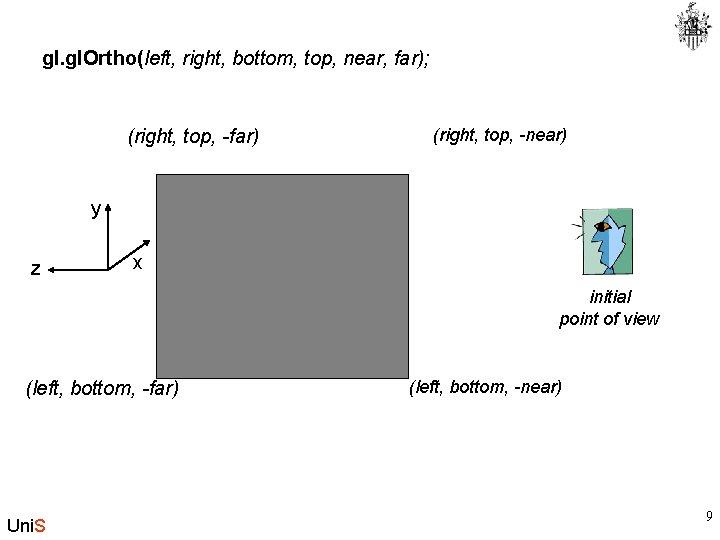
gl. Ortho(left, right, bottom, top, near, far); (right, top, -far) (right, top, -near) y z x initial point of view (left, bottom, -far) Uni. S (left, bottom, -near) 9
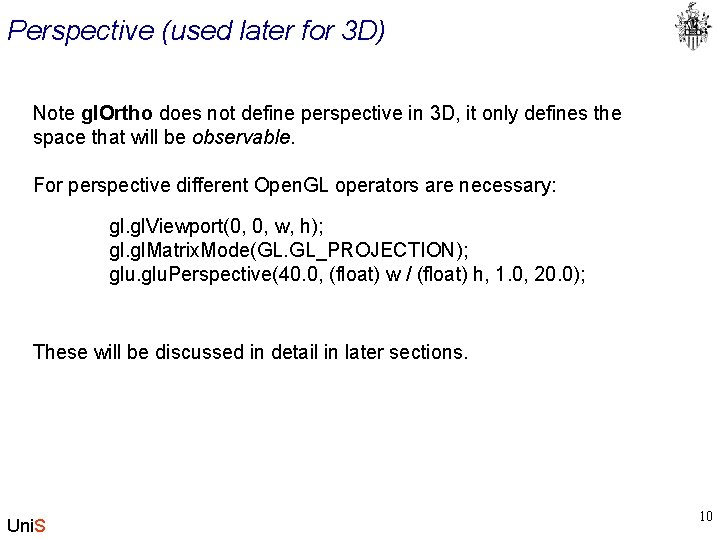
Perspective (used later for 3 D) Note gl. Ortho does not define perspective in 3 D, it only defines the space that will be observable. For perspective different Open. GL operators are necessary: gl. Viewport(0, 0, w, h); gl. Matrix. Mode(GL. GL_PROJECTION); glu. Perspective(40. 0, (float) w / (float) h, 1. 0, 20. 0); These will be discussed in detail in later sections. Uni. S 10
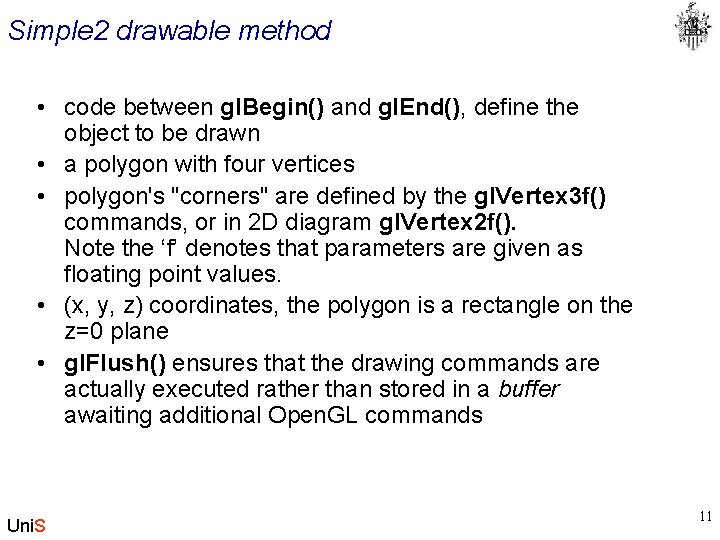
Simple 2 drawable method • code between gl. Begin() and gl. End(), define the object to be drawn • a polygon with four vertices • polygon's "corners" are defined by the gl. Vertex 3 f() commands, or in 2 D diagram gl. Vertex 2 f(). Note the ‘f’ denotes that parameters are given as floating point values. • (x, y, z) coordinates, the polygon is a rectangle on the z=0 plane • gl. Flush() ensures that the drawing commands are actually executed rather than stored in a buffer awaiting additional Open. GL commands Uni. S 11
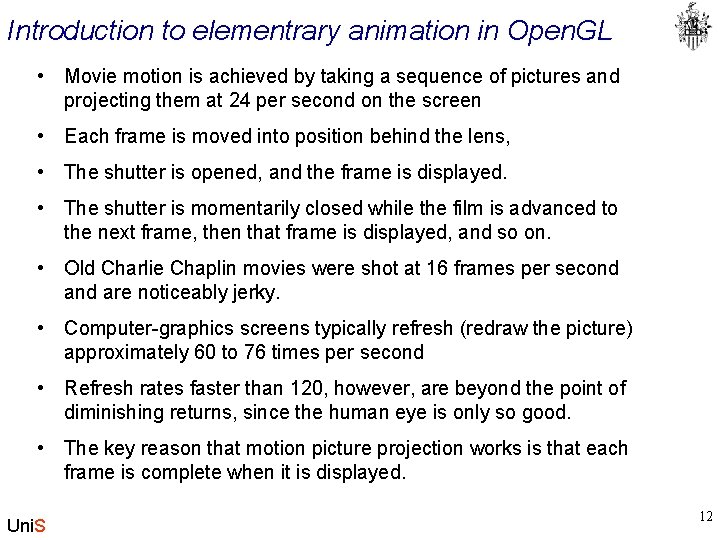
Introduction to elementrary animation in Open. GL • Movie motion is achieved by taking a sequence of pictures and projecting them at 24 per second on the screen • Each frame is moved into position behind the lens, • The shutter is opened, and the frame is displayed. • The shutter is momentarily closed while the film is advanced to the next frame, then that frame is displayed, and so on. • Old Charlie Chaplin movies were shot at 16 frames per second are noticeably jerky. • Computer-graphics screens typically refresh (redraw the picture) approximately 60 to 76 times per second • Refresh rates faster than 120, however, are beyond the point of diminishing returns, since the human eye is only so good. • The key reason that motion picture projection works is that each frame is complete when it is displayed. Uni. S 12
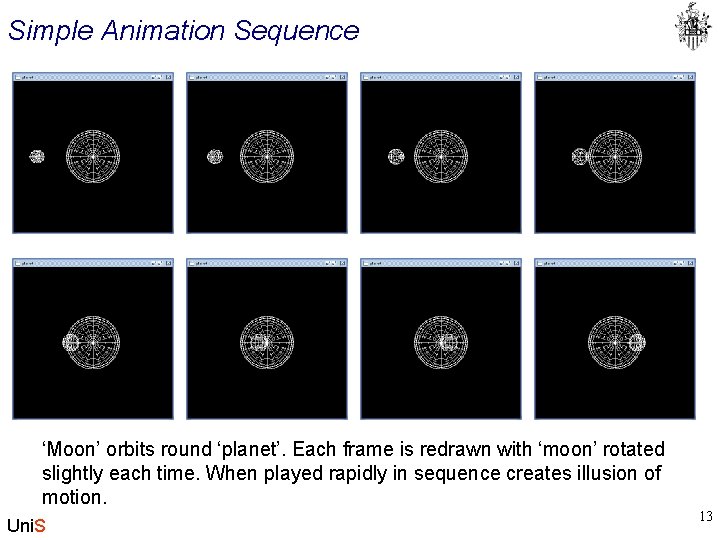
Simple Animation Sequence ‘Moon’ orbits round ‘planet’. Each frame is redrawn with ‘moon’ rotated slightly each time. When played rapidly in sequence creates illusion of motion. Uni. S 13
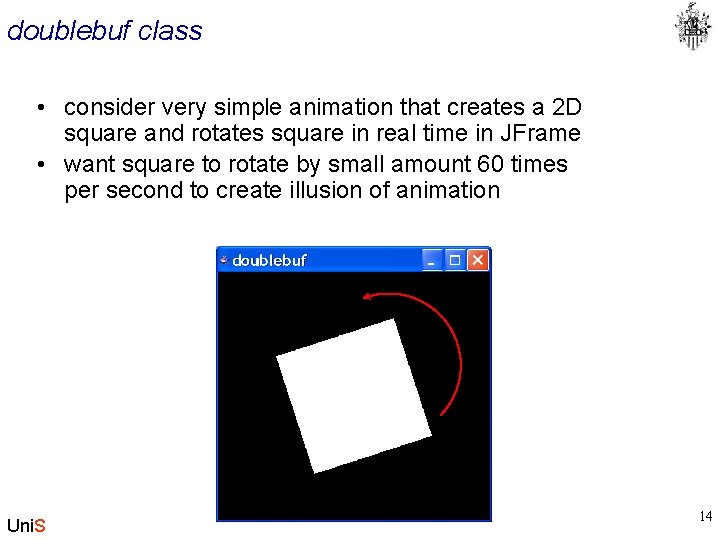
doublebuf class • consider very simple animation that creates a 2 D square and rotates square in real time in JFrame • want square to rotate by small amount 60 times per second to create illusion of animation Uni. S 14
![doublebuf class example using animator thread public static void mainString args doublebuf class example, using animator thread public static void main(String[ ] args) { /*](https://slidetodoc.com/presentation_image/6681466515049c223ecaeb5aed876c81/image-15.jpg)
doublebuf class example, using animator thread public static void main(String[ ] args) { /* Part 1: create new Java application object demo with double buffering here Set up Open. GL canvas and Java Swing JFrame and define drawing elements here. */ FPSAnimator animator = new FPSAnimator(canvas, 60); demo. set. Animator(animator); /* Part 2: initialise frame look and feel and attach drawing canvas here */ animator. start(); } Uni. S 15
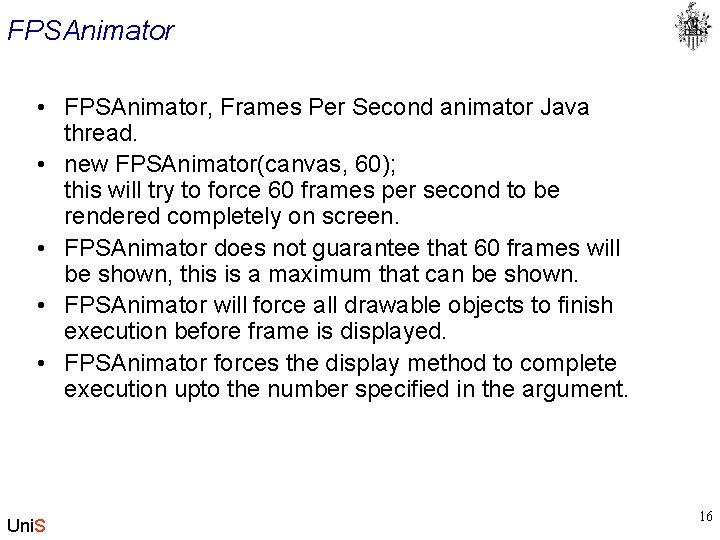
FPSAnimator • FPSAnimator, Frames Per Second animator Java thread. • new FPSAnimator(canvas, 60); this will try to force 60 frames per second to be rendered completely on screen. • FPSAnimator does not guarantee that 60 frames will be shown, this is a maximum that can be shown. • FPSAnimator will force all drawable objects to finish execution before frame is displayed. • FPSAnimator forces the display method to complete execution upto the number specified in the argument. Uni. S 16
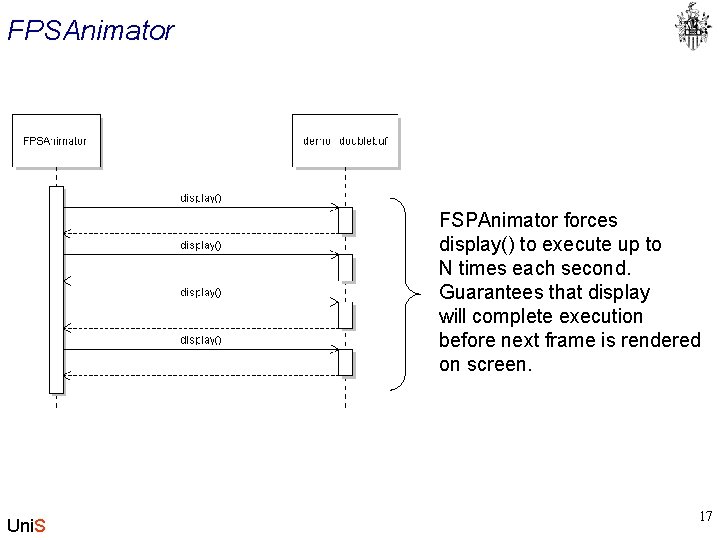
FPSAnimator FSPAnimator forces display() to execute up to N times each second. Guarantees that display will complete execution before next frame is rendered on screen. Uni. S 17
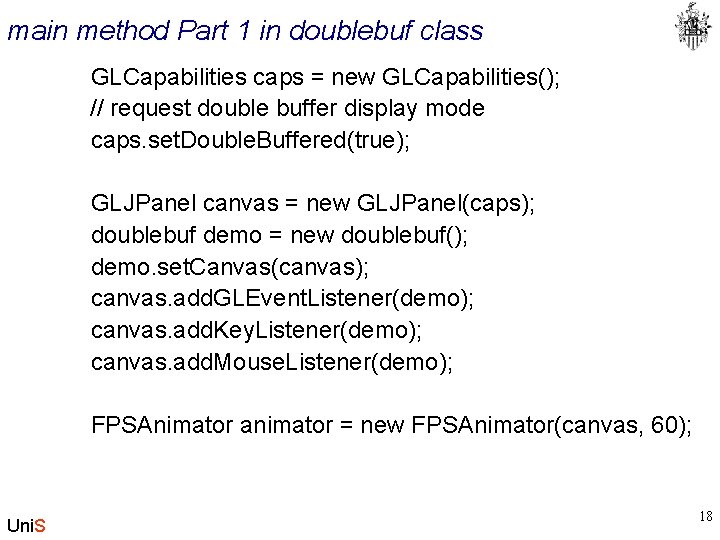
main method Part 1 in doublebuf class GLCapabilities caps = new GLCapabilities(); // request double buffer display mode caps. set. Double. Buffered(true); GLJPanel canvas = new GLJPanel(caps); doublebuf demo = new doublebuf(); demo. set. Canvas(canvas); canvas. add. GLEvent. Listener(demo); canvas. add. Key. Listener(demo); canvas. add. Mouse. Listener(demo); FPSAnimator animator = new FPSAnimator(canvas, 60); Uni. S 18
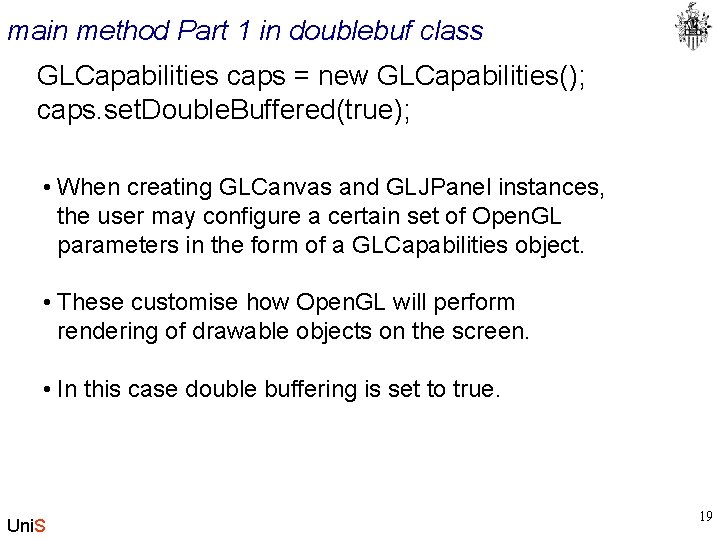
main method Part 1 in doublebuf class GLCapabilities caps = new GLCapabilities(); caps. set. Double. Buffered(true); • When creating GLCanvas and GLJPanel instances, the user may configure a certain set of Open. GL parameters in the form of a GLCapabilities object. • These customise how Open. GL will perform rendering of drawable objects on the screen. • In this case double buffering is set to true. Uni. S 19
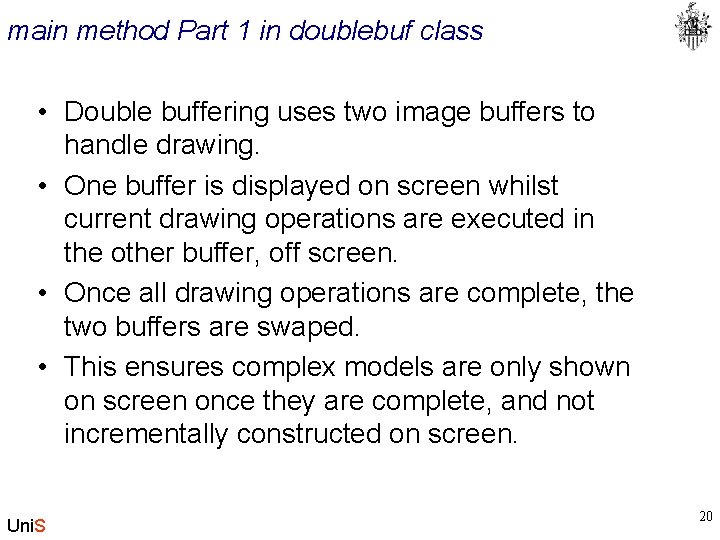
main method Part 1 in doublebuf class • Double buffering uses two image buffers to handle drawing. • One buffer is displayed on screen whilst current drawing operations are executed in the other buffer, off screen. • Once all drawing operations are complete, the two buffers are swaped. • This ensures complex models are only shown on screen once they are complete, and not incrementally constructed on screen. Uni. S 20
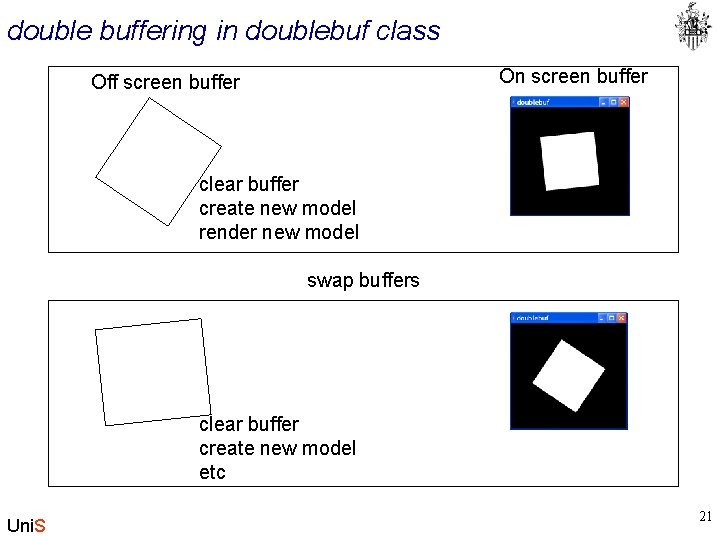
double buffering in doublebuf class On screen buffer Off screen buffer clear buffer create new model render new model swap buffers clear buffer create new model etc Uni. S 21
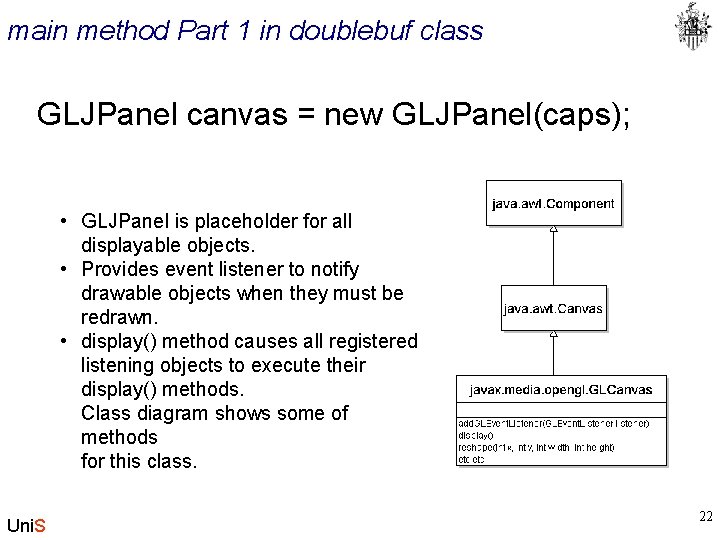
main method Part 1 in doublebuf class GLJPanel canvas = new GLJPanel(caps); • GLJPanel is placeholder for all displayable objects. • Provides event listener to notify drawable objects when they must be redrawn. • display() method causes all registered listening objects to execute their display() methods. Class diagram shows some of methods for this class. Uni. S 22
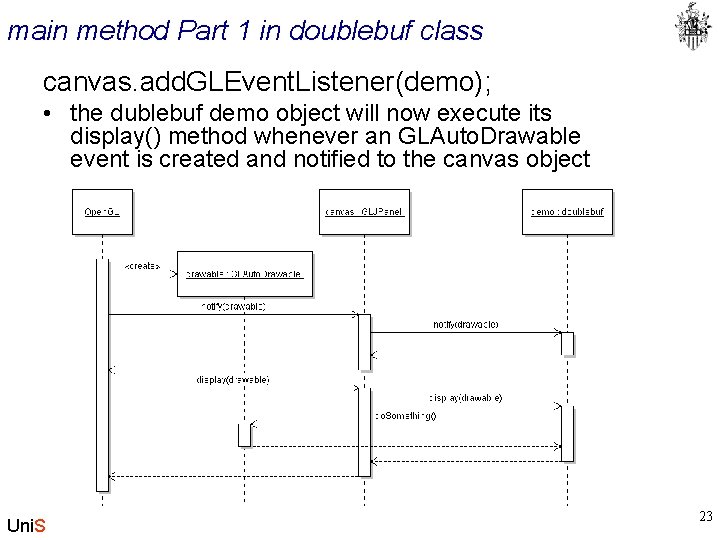
main method Part 1 in doublebuf class canvas. add. GLEvent. Listener(demo); • the dublebuf demo object will now execute its display() method whenever an GLAuto. Drawable event is created and notified to the canvas object Uni. S 23
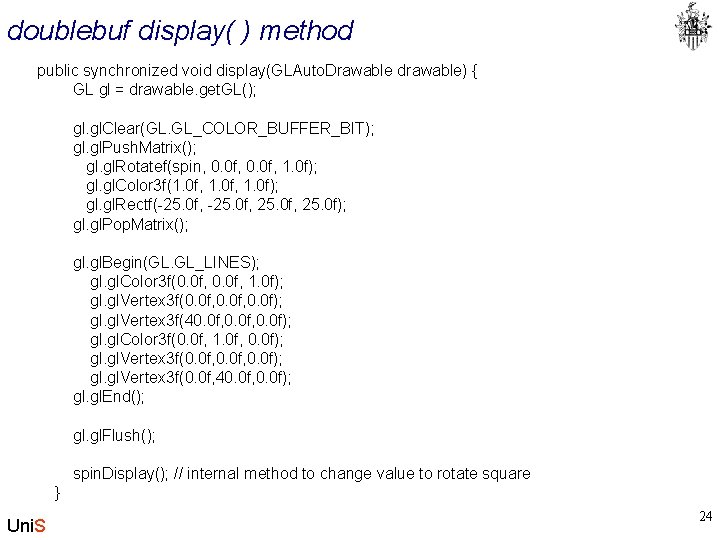
doublebuf display( ) method public synchronized void display(GLAuto. Drawable drawable) { GL gl = drawable. get. GL(); gl. Clear(GL. GL_COLOR_BUFFER_BIT); gl. Push. Matrix(); gl. Rotatef(spin, 0. 0 f, 1. 0 f); gl. Color 3 f(1. 0 f, 1. 0 f); gl. Rectf(-25. 0 f, 25. 0 f); gl. Pop. Matrix(); gl. Begin(GL. GL_LINES); gl. Color 3 f(0. 0 f, 1. 0 f); gl. Vertex 3 f(0. 0 f, 0. 0 f); gl. Vertex 3 f(40. 0 f, 0. 0 f); gl. Color 3 f(0. 0 f, 1. 0 f, 0. 0 f); gl. Vertex 3 f(0. 0 f, 40. 0 f, 0. 0 f); gl. End(); gl. Flush(); spin. Display(); // internal method to change value to rotate square } Uni. S 24
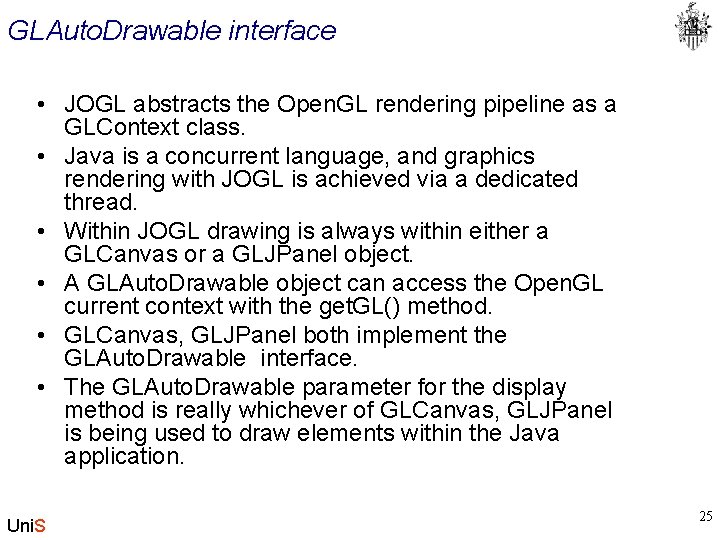
GLAuto. Drawable interface • JOGL abstracts the Open. GL rendering pipeline as a GLContext class. • Java is a concurrent language, and graphics rendering with JOGL is achieved via a dedicated thread. • Within JOGL drawing is always within either a GLCanvas or a GLJPanel object. • A GLAuto. Drawable object can access the Open. GL current context with the get. GL() method. • GLCanvas, GLJPanel both implement the GLAuto. Drawable interface. • The GLAuto. Drawable parameter for the display method is really whichever of GLCanvas, GLJPanel is being used to draw elements within the Java application. Uni. S 25
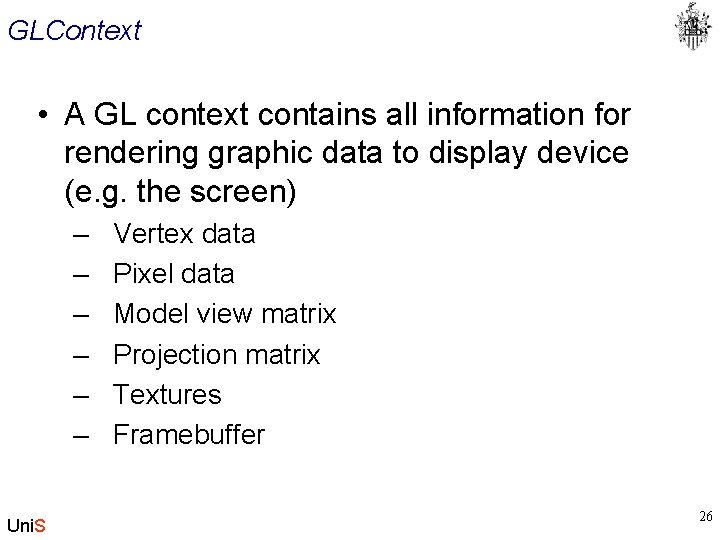
GLContext • A GL context contains all information for rendering graphic data to display device (e. g. the screen) – – – Uni. S Vertex data Pixel data Model view matrix Projection matrix Textures Framebuffer 26
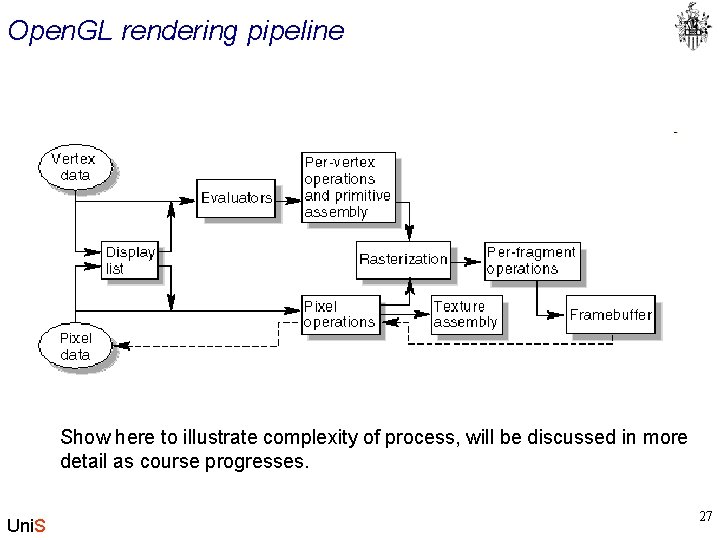
Open. GL rendering pipeline Show here to illustrate complexity of process, will be discussed in more detail as course progresses. Uni. S 27
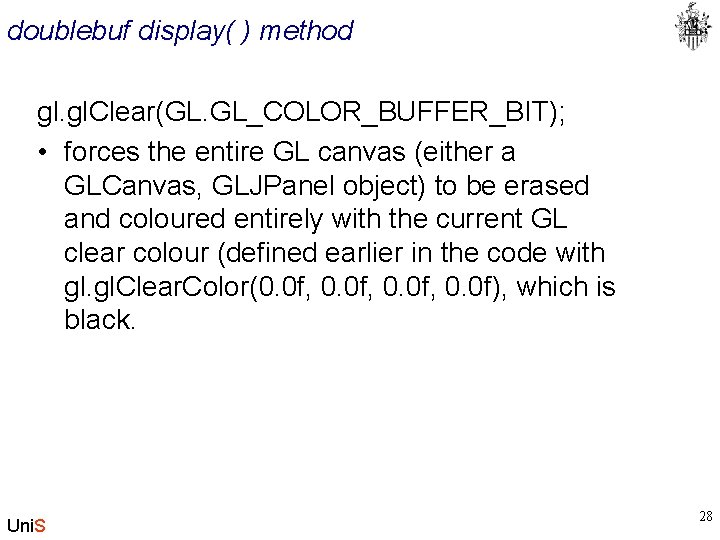
doublebuf display( ) method gl. Clear(GL. GL_COLOR_BUFFER_BIT); • forces the entire GL canvas (either a GLCanvas, GLJPanel object) to be erased and coloured entirely with the current GL clear colour (defined earlier in the code with gl. Clear. Color(0. 0 f, 0. 0 f), which is black. Uni. S 28
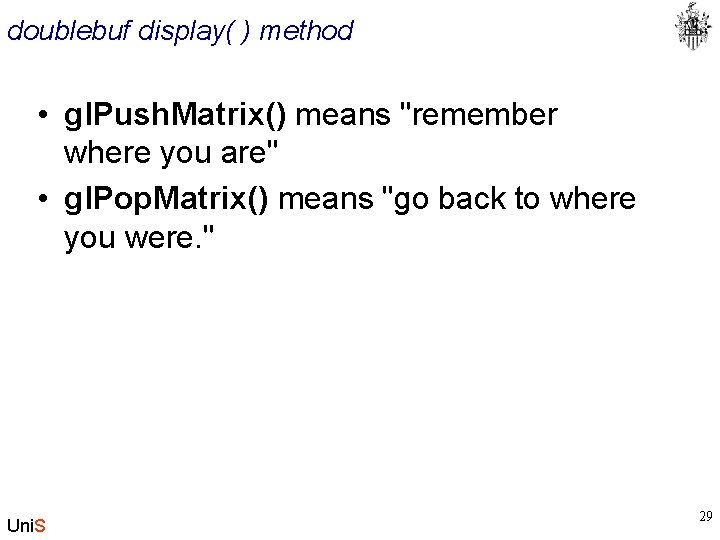
doublebuf display( ) method • gl. Push. Matrix() means "remember where you are" • gl. Pop. Matrix() means "go back to where you were. " Uni. S 29
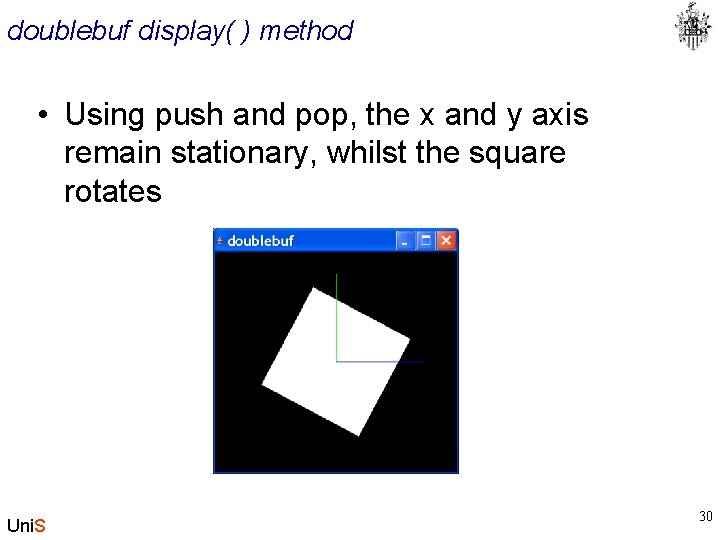
doublebuf display( ) method • Using push and pop, the x and y axis remain stationary, whilst the square rotates Uni. S 30
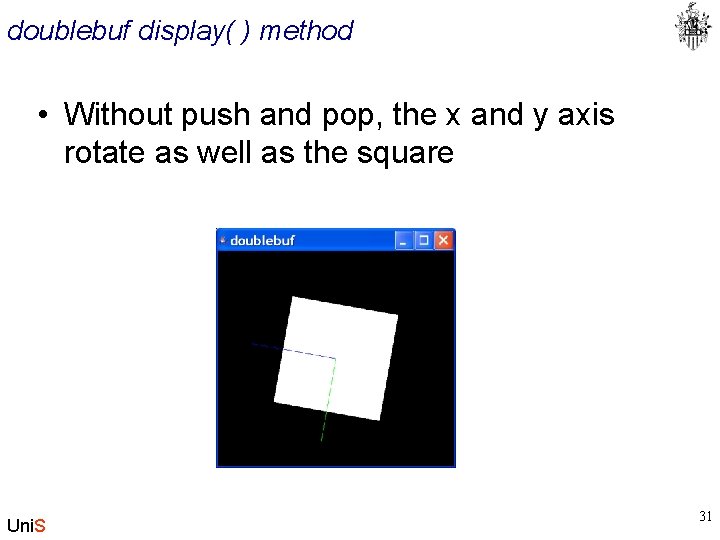
doublebuf display( ) method • Without push and pop, the x and y axis rotate as well as the square Uni. S 31
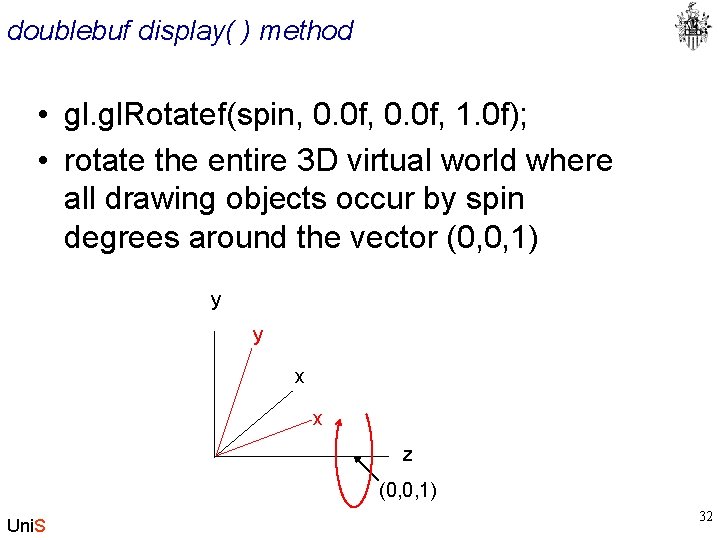
doublebuf display( ) method • gl. Rotatef(spin, 0. 0 f, 1. 0 f); • rotate the entire 3 D virtual world where all drawing objects occur by spin degrees around the vector (0, 0, 1) y y x x z (0, 0, 1) Uni. S 32
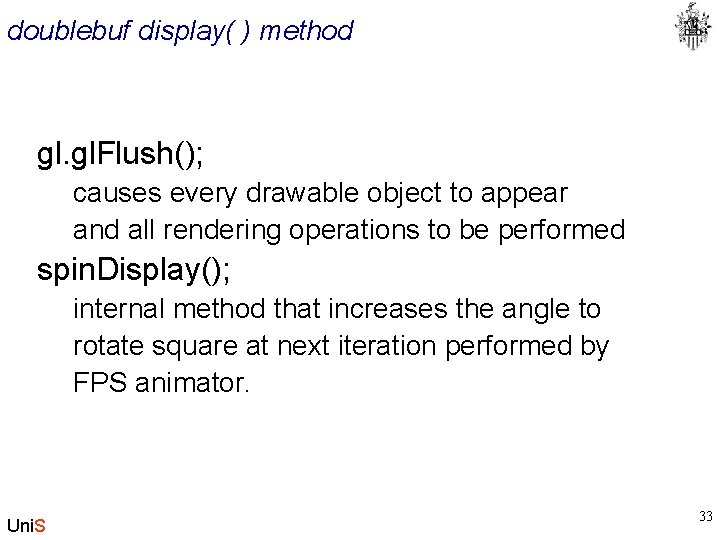
doublebuf display( ) method gl. Flush(); causes every drawable object to appear and all rendering operations to be performed spin. Display(); internal method that increases the angle to rotate square at next iteration performed by FPS animator. Uni. S 33