CS 206 A DATA STRUCTURE Spring 2003 KAIST
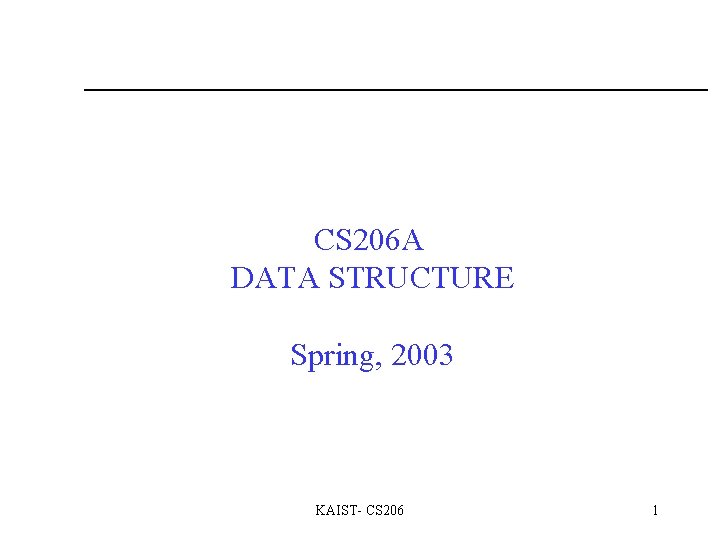
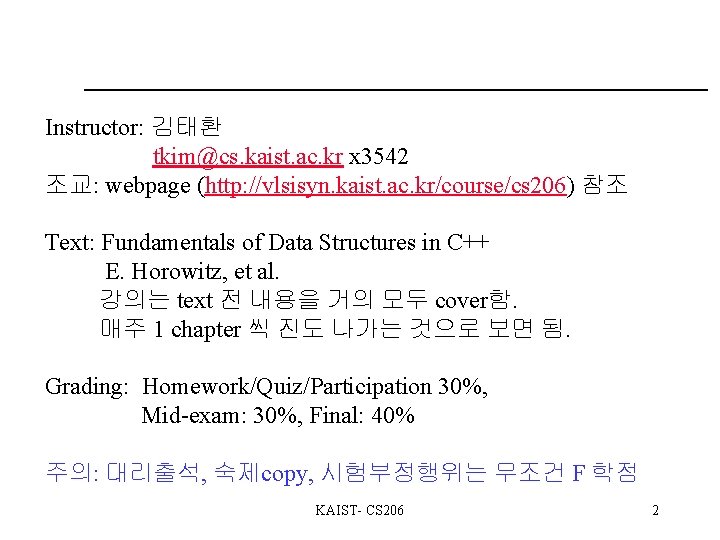
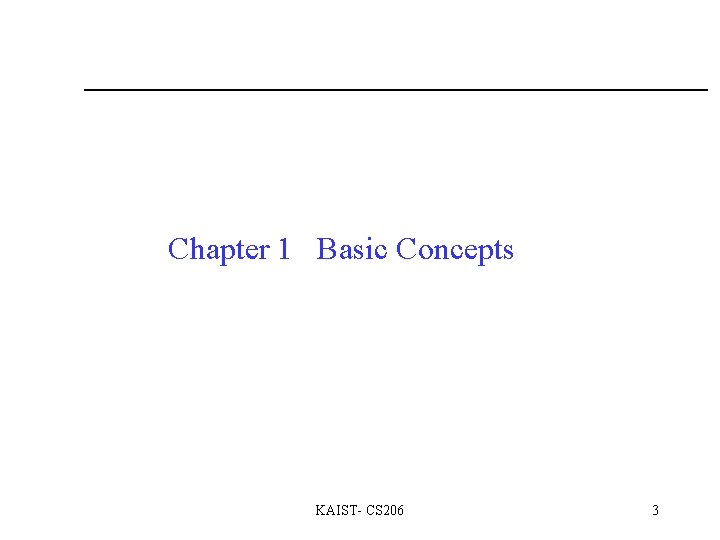
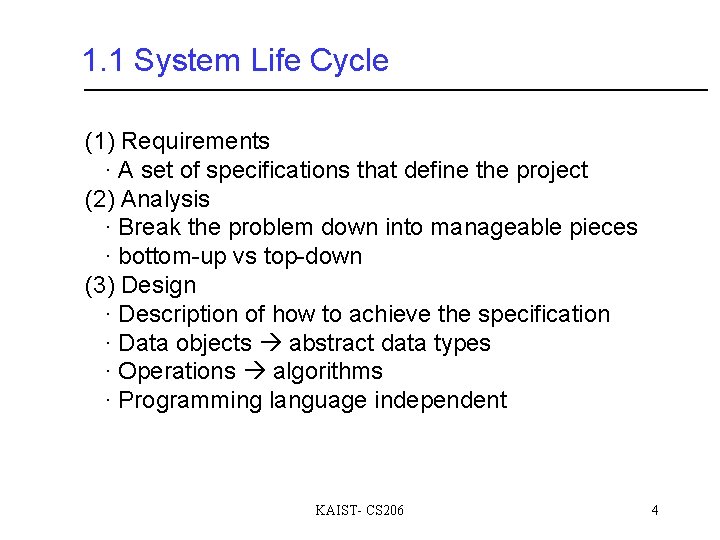
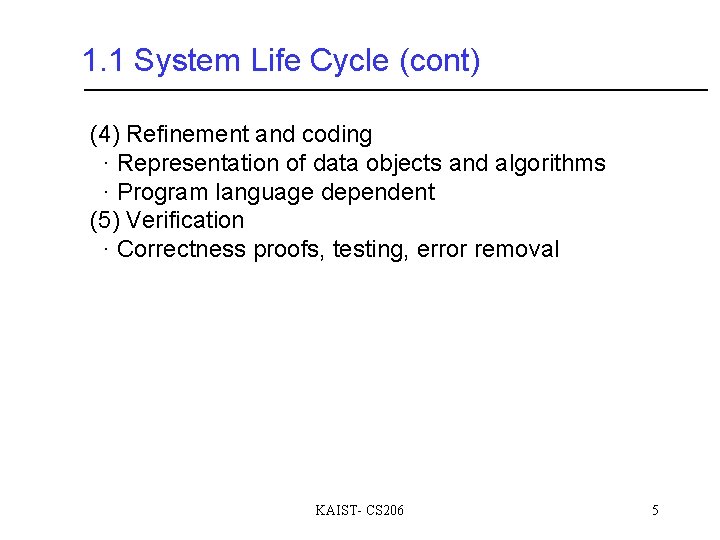
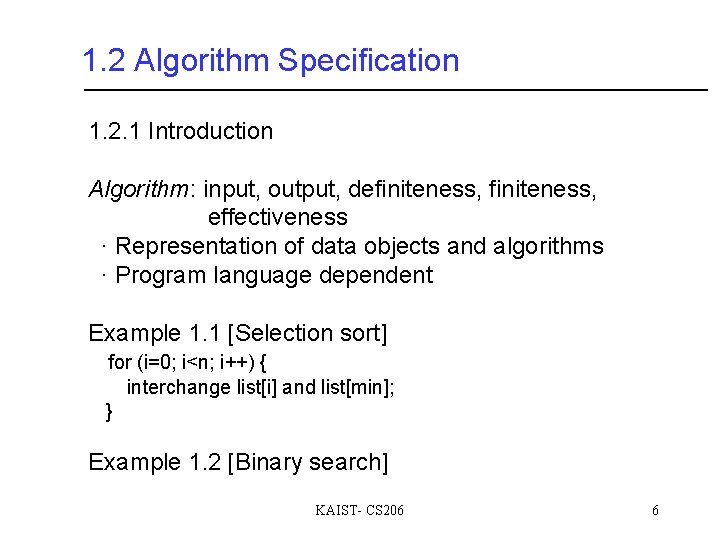
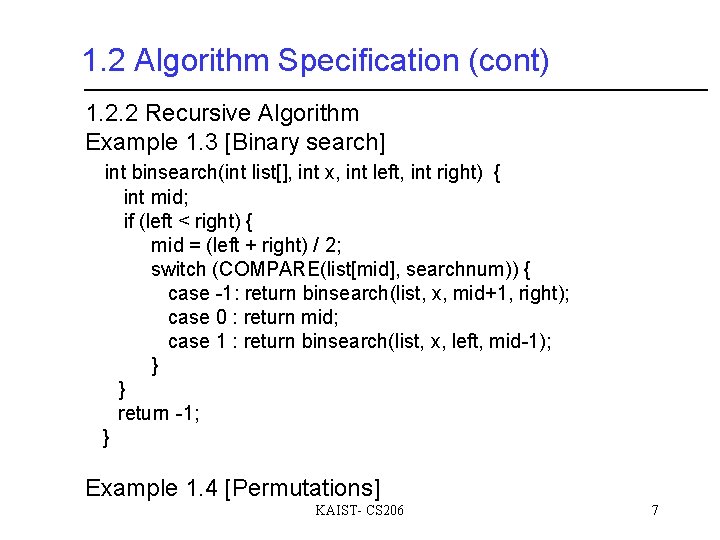
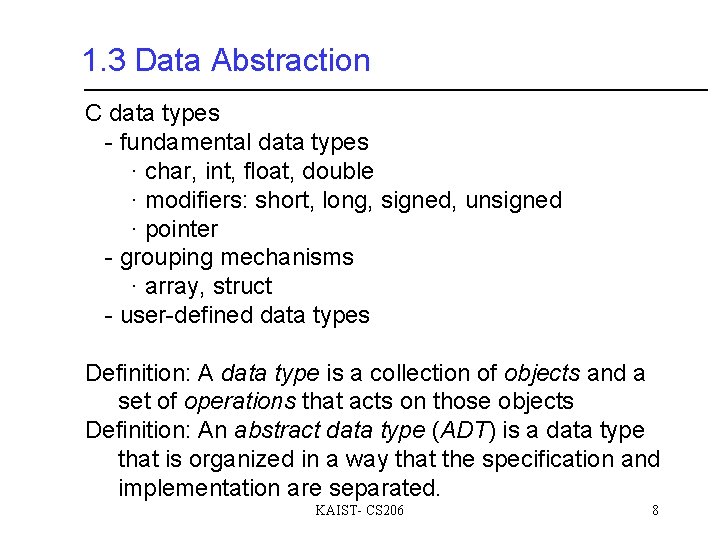
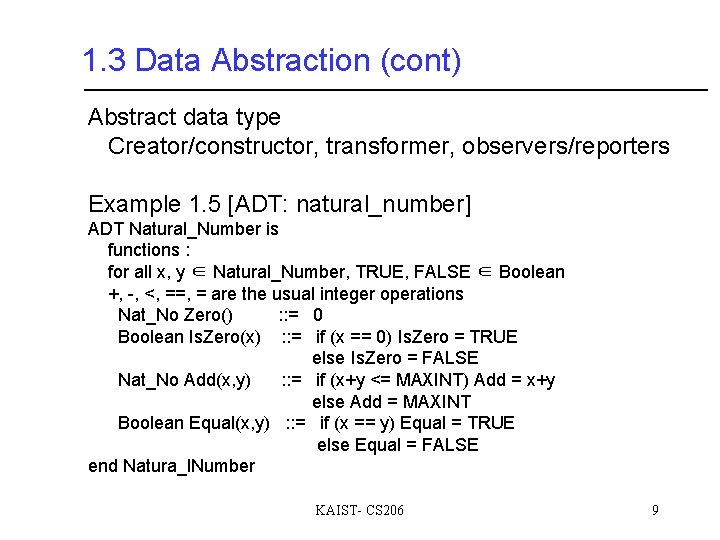
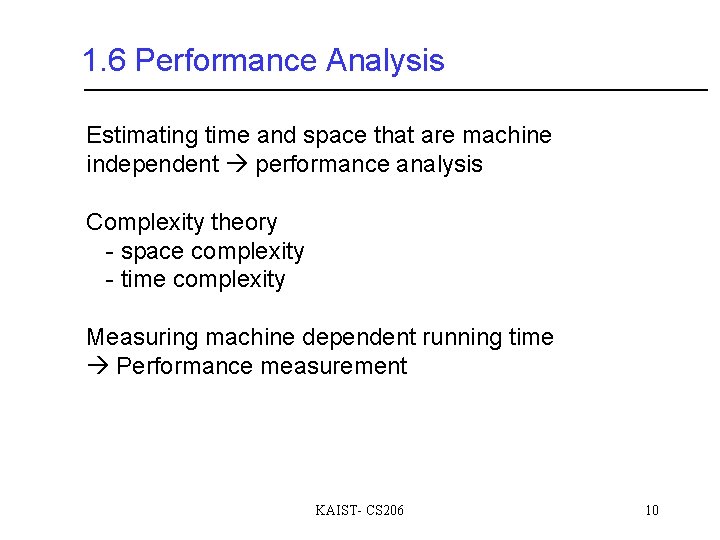
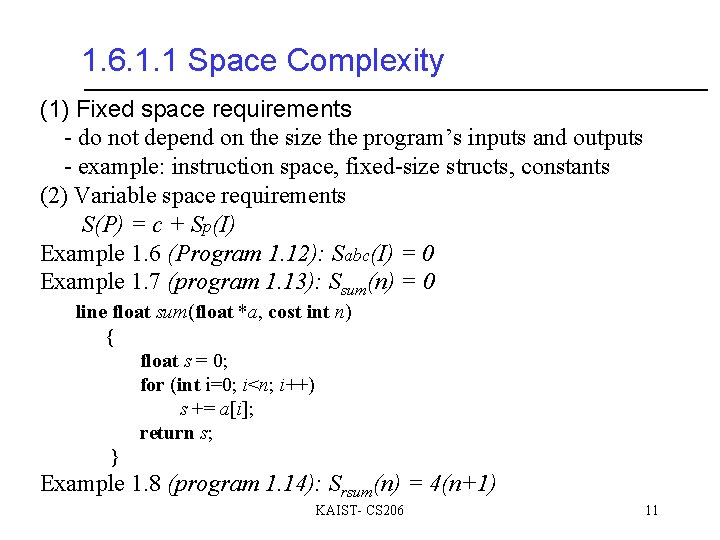
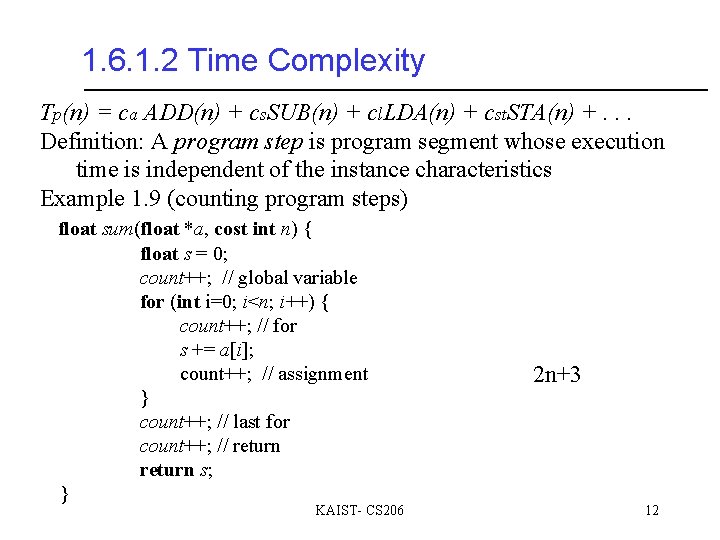
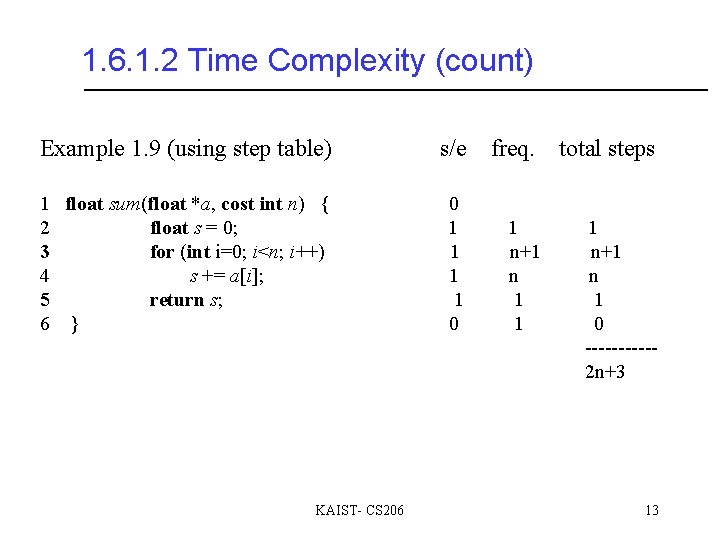
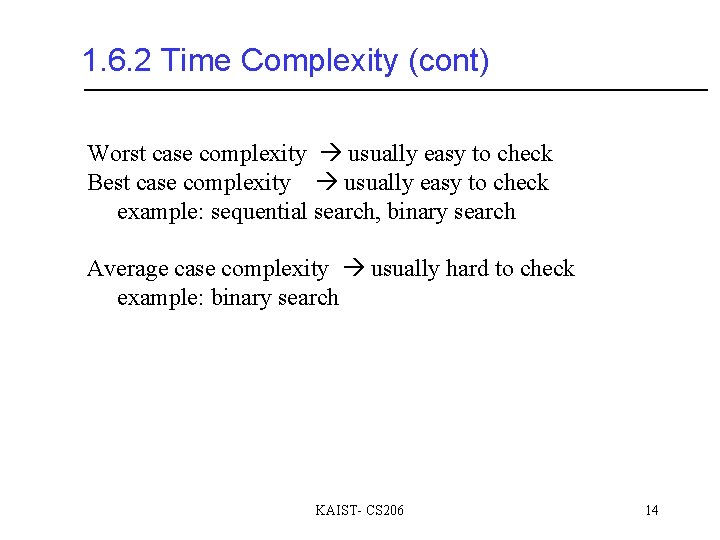
![1. 6. 1. 3 Asymptotic Notation Definition: [Big ‘’oh’’] f(n) = O(g(n)) Definition: [Omega] 1. 6. 1. 3 Asymptotic Notation Definition: [Big ‘’oh’’] f(n) = O(g(n)) Definition: [Omega]](https://slidetodoc.com/presentation_image_h2/36ec355b44c34e09ccd5092d7ad3b841/image-15.jpg)
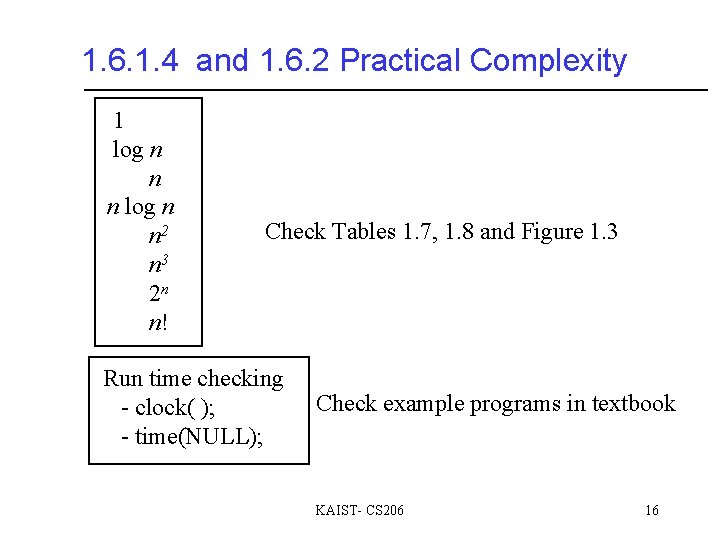
- Slides: 16
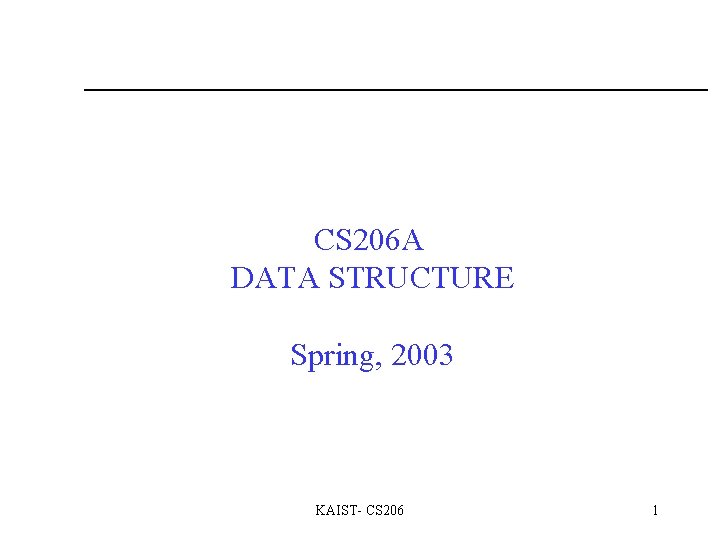
CS 206 A DATA STRUCTURE Spring, 2003 KAIST- CS 206 1
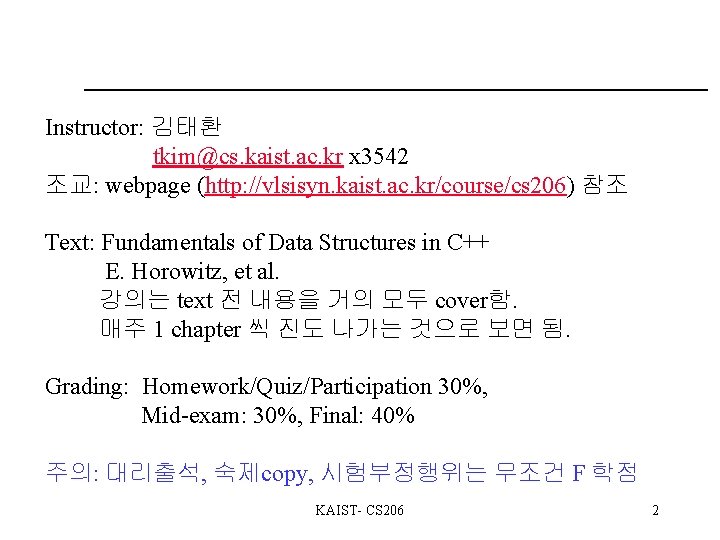
Instructor: 김태환 tkim@cs. kaist. ac. kr x 3542 조교: webpage (http: //vlsisyn. kaist. ac. kr/course/cs 206) 참조 Text: Fundamentals of Data Structures in C++ E. Horowitz, et al. 강의는 text 전 내용을 거의 모두 cover함. 매주 1 chapter 씩 진도 나가는 것으로 보면 됨. Grading: Homework/Quiz/Participation 30%, Mid-exam: 30%, Final: 40% 주의: 대리출석, 숙제copy, 시험부정행위는 무조건 F 학점 KAIST- CS 206 2
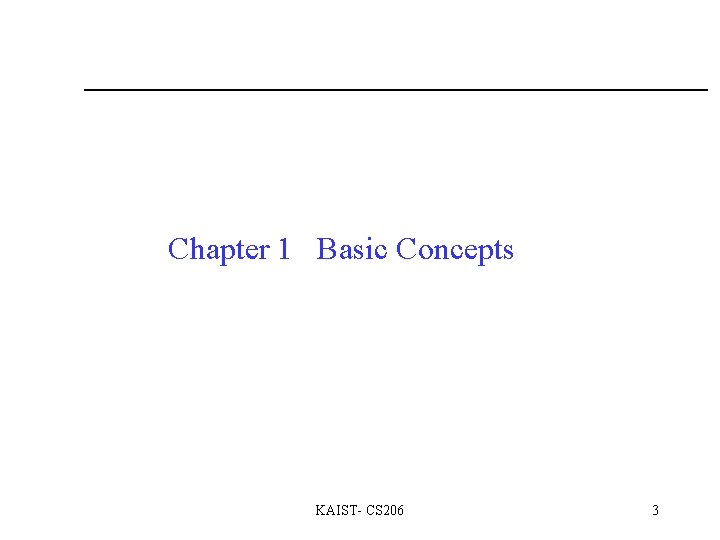
Chapter 1 Basic Concepts KAIST- CS 206 3
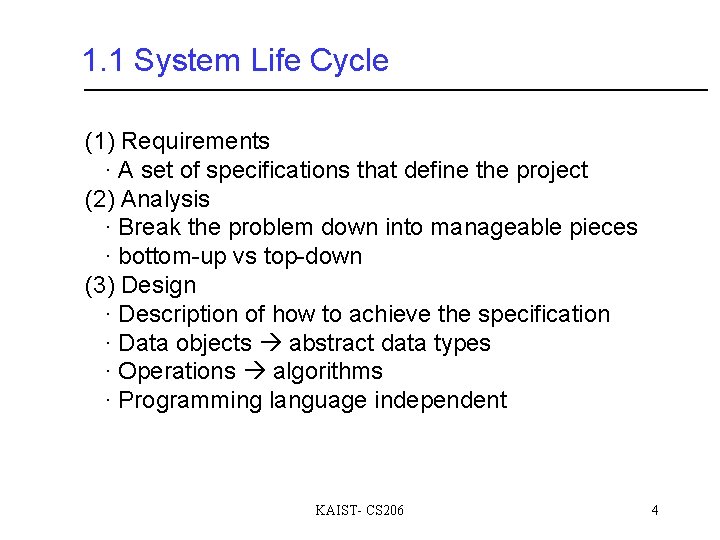
1. 1 System Life Cycle (1) Requirements ∙ A set of specifications that define the project (2) Analysis ∙ Break the problem down into manageable pieces ∙ bottom-up vs top-down (3) Design ∙ Description of how to achieve the specification ∙ Data objects abstract data types ∙ Operations algorithms ∙ Programming language independent KAIST- CS 206 4
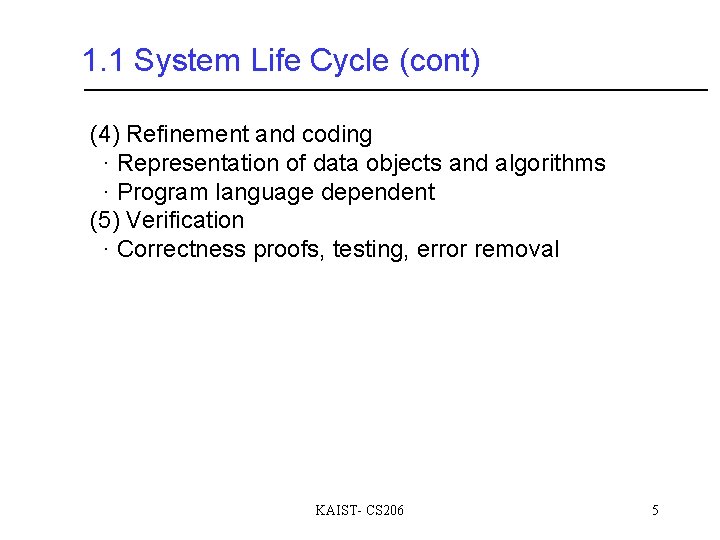
1. 1 System Life Cycle (cont) (4) Refinement and coding ∙ Representation of data objects and algorithms ∙ Program language dependent (5) Verification ∙ Correctness proofs, testing, error removal KAIST- CS 206 5
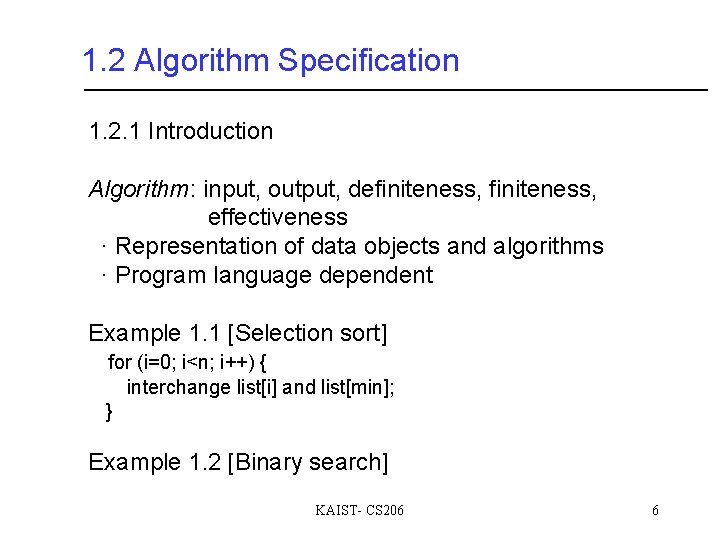
1. 2 Algorithm Specification 1. 2. 1 Introduction Algorithm: input, output, definiteness, effectiveness ∙ Representation of data objects and algorithms ∙ Program language dependent Example 1. 1 [Selection sort] for (i=0; i<n; i++) { interchange list[i] and list[min]; } Example 1. 2 [Binary search] KAIST- CS 206 6
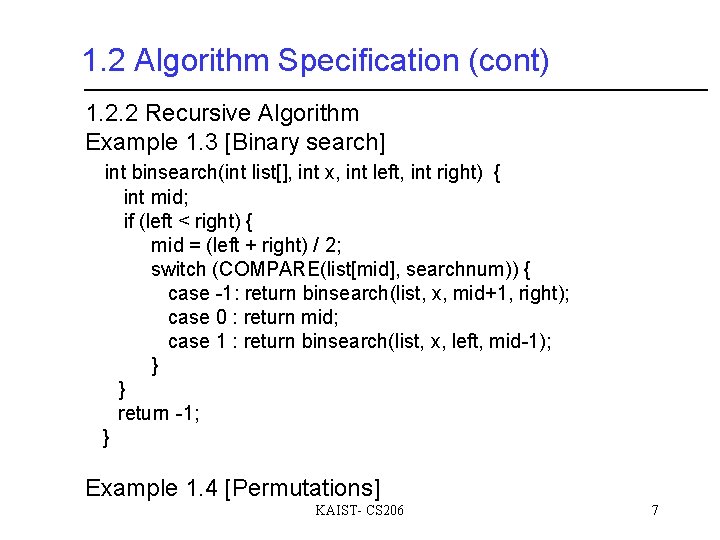
1. 2 Algorithm Specification (cont) 1. 2. 2 Recursive Algorithm Example 1. 3 [Binary search] int binsearch(int list[], int x, int left, int right) { int mid; if (left < right) { mid = (left + right) / 2; switch (COMPARE(list[mid], searchnum)) { case -1: return binsearch(list, x, mid+1, right); case 0 : return mid; case 1 : return binsearch(list, x, left, mid-1); } } return -1; } Example 1. 4 [Permutations] KAIST- CS 206 7
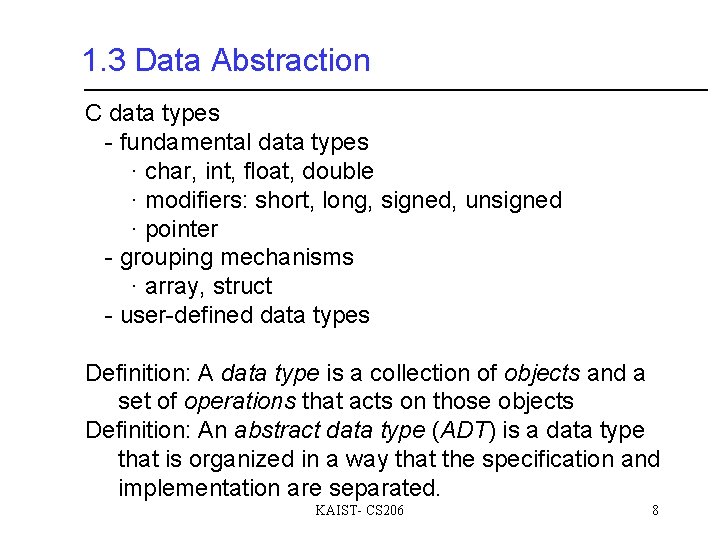
1. 3 Data Abstraction C data types - fundamental data types ∙ char, int, float, double ∙ modifiers: short, long, signed, unsigned ∙ pointer - grouping mechanisms ∙ array, struct - user-defined data types Definition: A data type is a collection of objects and a set of operations that acts on those objects Definition: An abstract data type (ADT) is a data type that is organized in a way that the specification and implementation are separated. KAIST- CS 206 8
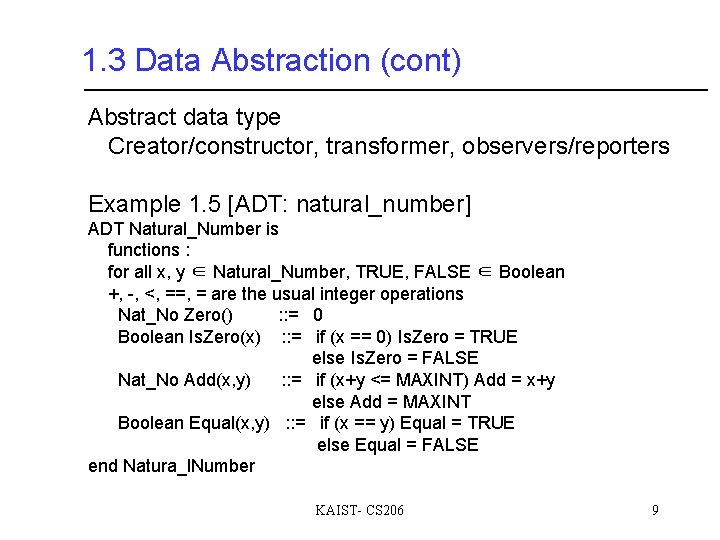
1. 3 Data Abstraction (cont) Abstract data type Creator/constructor, transformer, observers/reporters Example 1. 5 [ADT: natural_number] ADT Natural_Number is functions : for all x, y ∈ Natural_Number, TRUE, FALSE ∈ Boolean +, -, <, ==, = are the usual integer operations Nat_No Zero() : : = 0 Boolean Is. Zero(x) : : = if (x == 0) Is. Zero = TRUE else Is. Zero = FALSE Nat_No Add(x, y) : : = if (x+y <= MAXINT) Add = x+y else Add = MAXINT Boolean Equal(x, y) : : = if (x == y) Equal = TRUE else Equal = FALSE end Natura_l. Number KAIST- CS 206 9
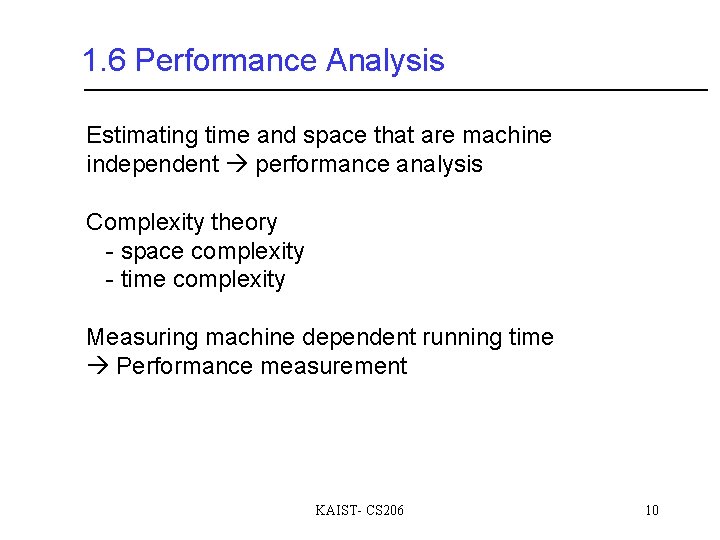
1. 6 Performance Analysis Estimating time and space that are machine independent performance analysis Complexity theory - space complexity - time complexity Measuring machine dependent running time Performance measurement KAIST- CS 206 10
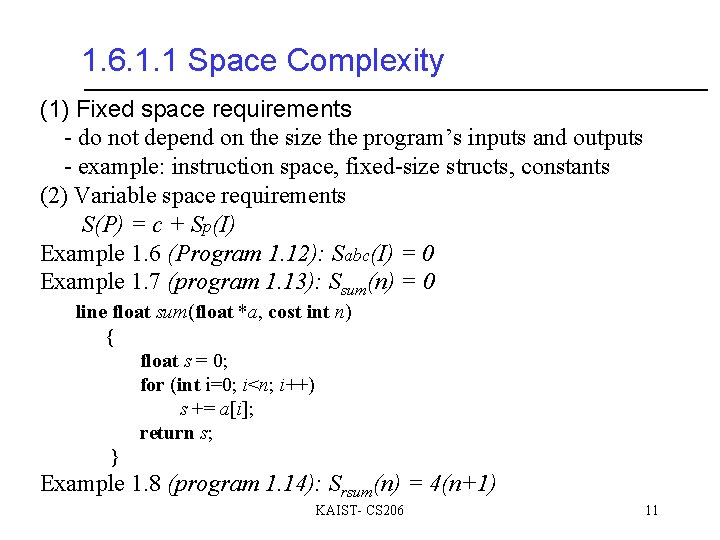
1. 6. 1. 1 Space Complexity (1) Fixed space requirements - do not depend on the size the program’s inputs and outputs - example: instruction space, fixed-size structs, constants (2) Variable space requirements S(P) = c + Sp(I) Example 1. 6 (Program 1. 12): Sabc(I) = 0 Example 1. 7 (program 1. 13): Ssum(n) = 0 line float sum(float *a, cost int n) { float s = 0; for (int i=0; i<n; i++) s += a[i]; return s; } Example 1. 8 (program 1. 14): Srsum(n) = 4(n+1) KAIST- CS 206 11
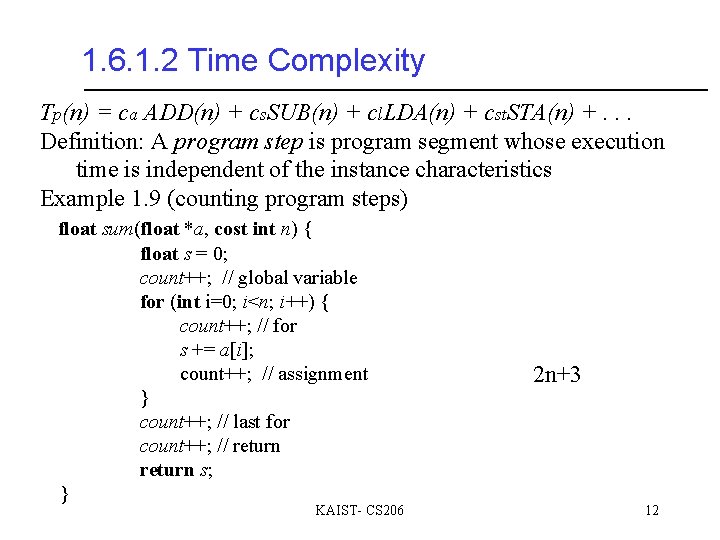
1. 6. 1. 2 Time Complexity Tp(n) = ca ADD(n) + cs. SUB(n) + cl. LDA(n) + cst. STA(n) +. . . Definition: A program step is program segment whose execution time is independent of the instance characteristics Example 1. 9 (counting program steps) float sum(float *a, cost int n) { float s = 0; count++; // global variable for (int i=0; i<n; i++) { count++; // for s += a[i]; count++; // assignment } count++; // last for count++; // return s; } KAIST- CS 206 2 n+3 12
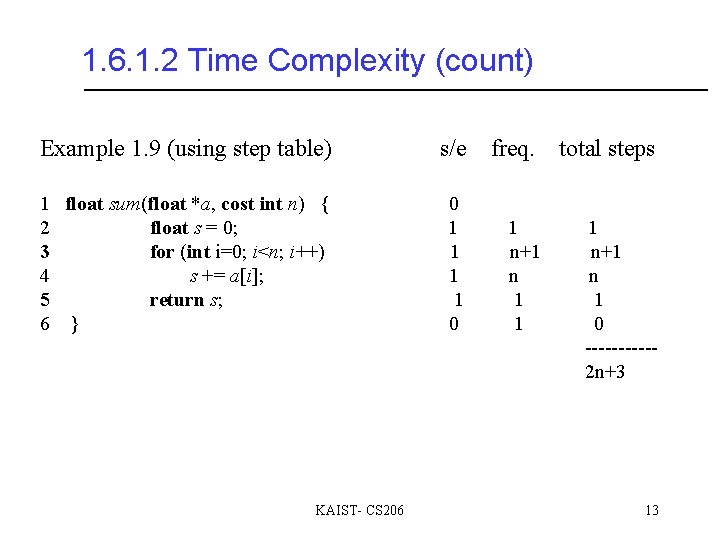
1. 6. 1. 2 Time Complexity (count) Example 1. 9 (using step table) s/e freq. total steps 1 float sum(float *a, cost int n) { 2 float s = 0; 3 for (int i=0; i<n; i++) 4 s += a[i]; 5 return s; 6 } 0 1 1 0 1 n+1 n 1 1 1 n+1 n 1 0 -----2 n+3 KAIST- CS 206 13
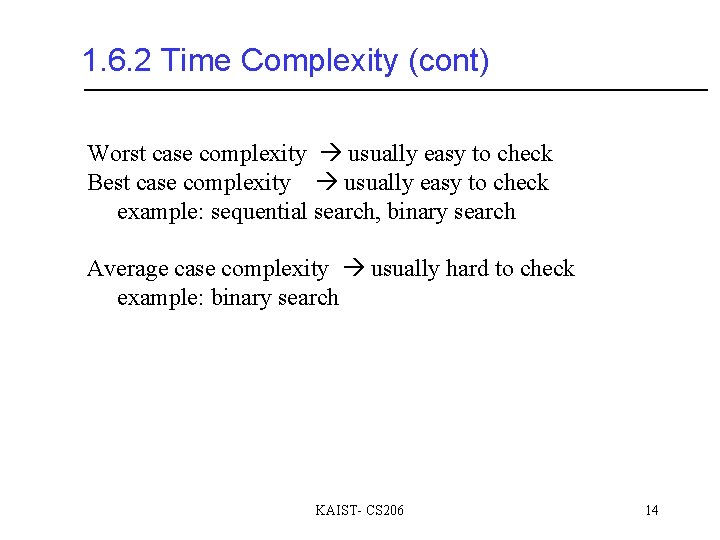
1. 6. 2 Time Complexity (cont) Worst case complexity usually easy to check Best case complexity usually easy to check example: sequential search, binary search Average case complexity usually hard to check example: binary search KAIST- CS 206 14
![1 6 1 3 Asymptotic Notation Definition Big oh fn Ogn Definition Omega 1. 6. 1. 3 Asymptotic Notation Definition: [Big ‘’oh’’] f(n) = O(g(n)) Definition: [Omega]](https://slidetodoc.com/presentation_image_h2/36ec355b44c34e09ccd5092d7ad3b841/image-15.jpg)
1. 6. 1. 3 Asymptotic Notation Definition: [Big ‘’oh’’] f(n) = O(g(n)) Definition: [Omega] f(n) = (g(n)) Definition: [Theta] f(n) = (g(n)) Example 1. 16 [Generating permutation] O(n!) for worst, best, average cases Example 1. 17 [Binary search] O(n log n) for worst case KAIST- CS 206 15
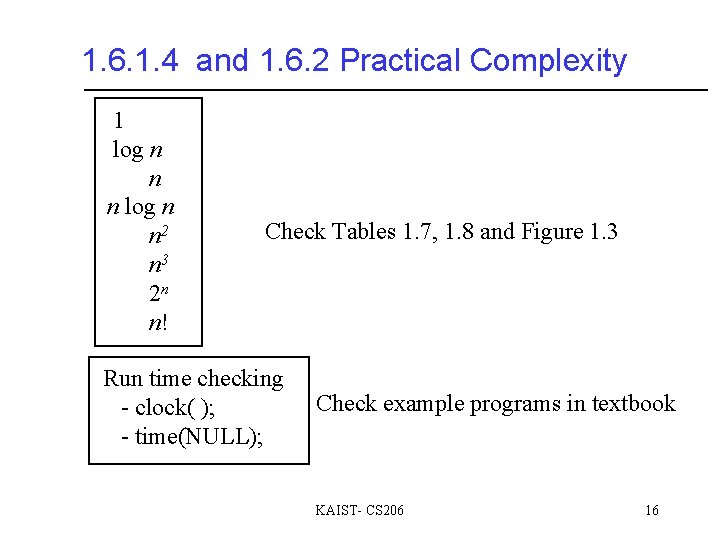
1. 6. 1. 4 and 1. 6. 2 Practical Complexity 1 log n n n log n n 2 n 3 2 n n! Check Tables 1. 7, 1. 8 and Figure 1. 3 Run time checking - clock( ); - time(NULL); Check example programs in textbook KAIST- CS 206 16