CS 201 Repetition loops Types Of Loops n
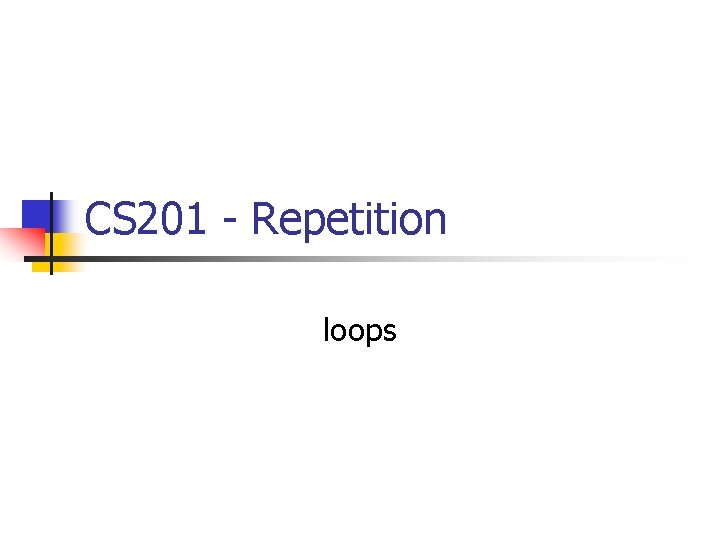
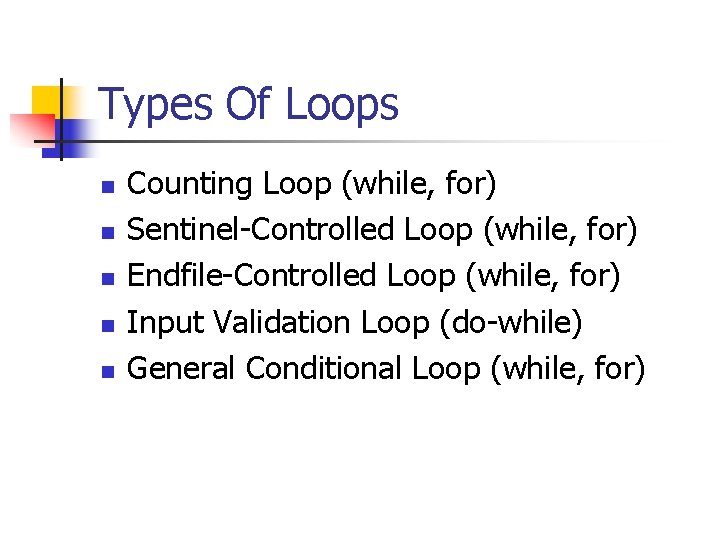
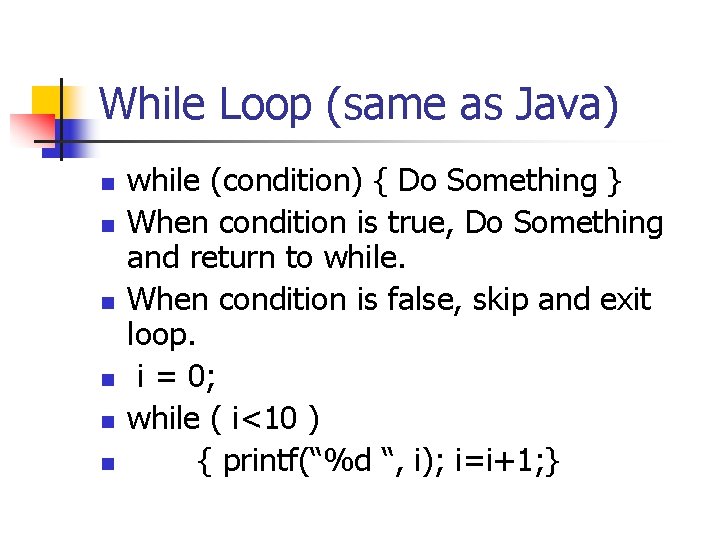
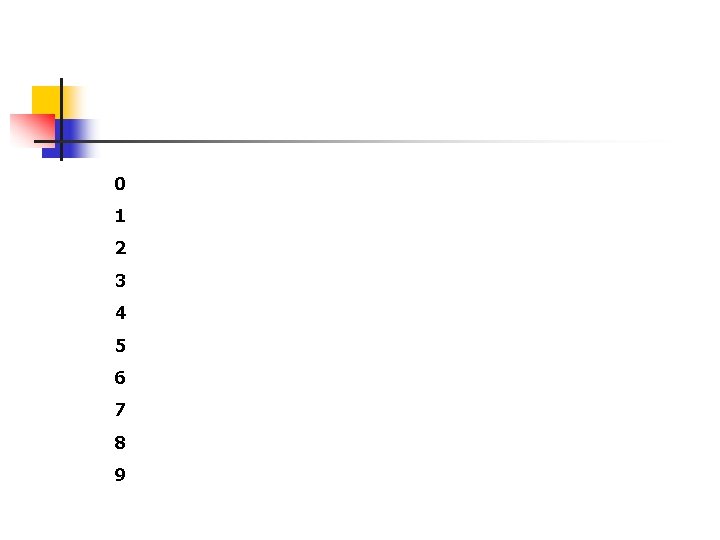
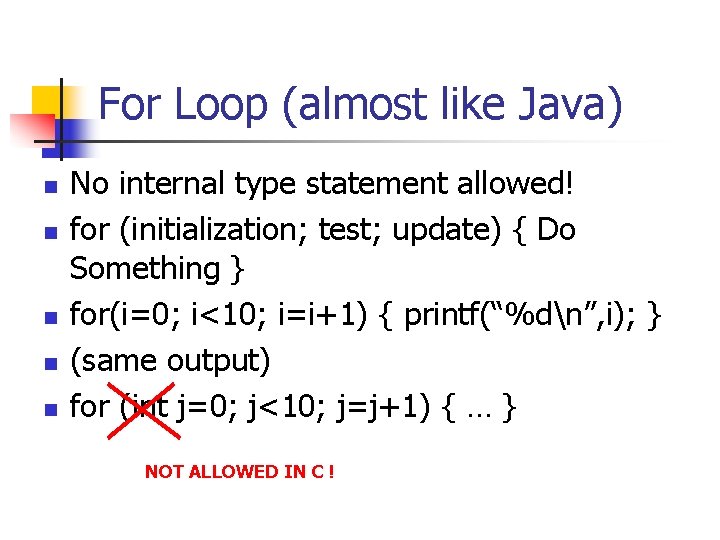
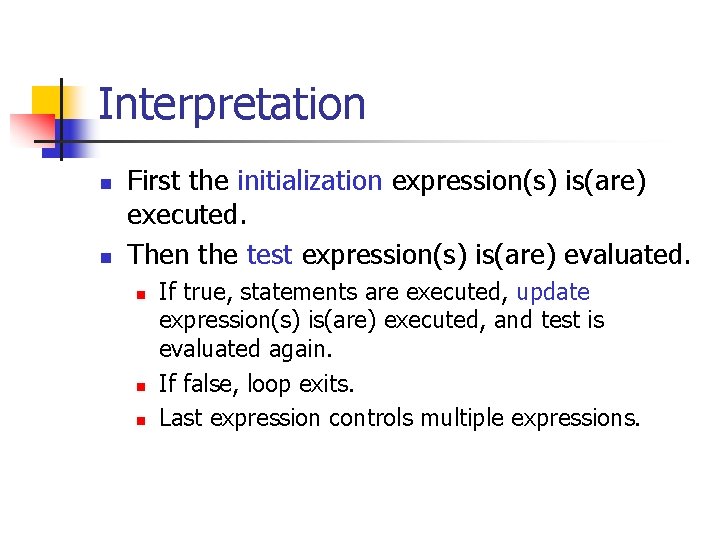
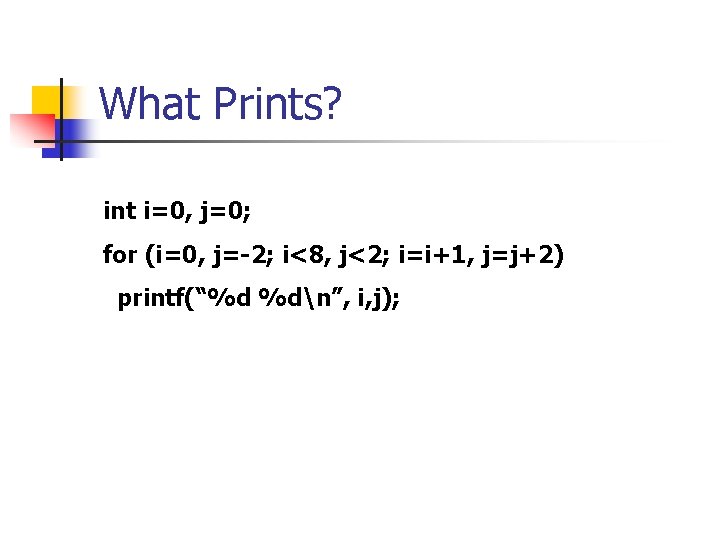
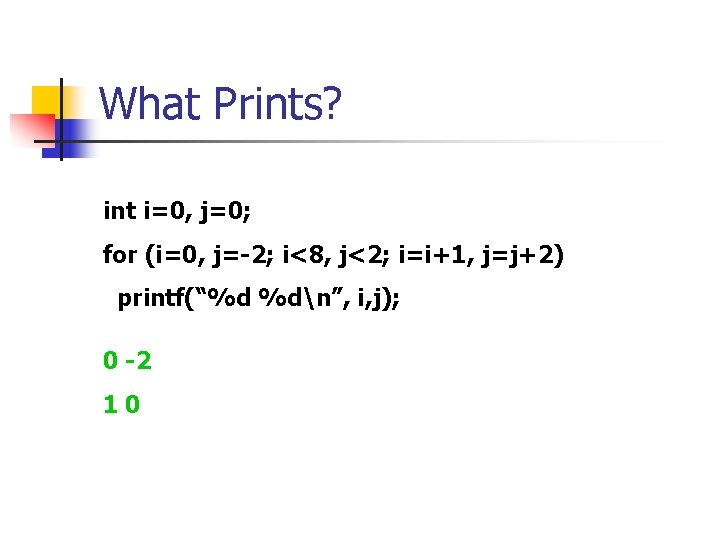
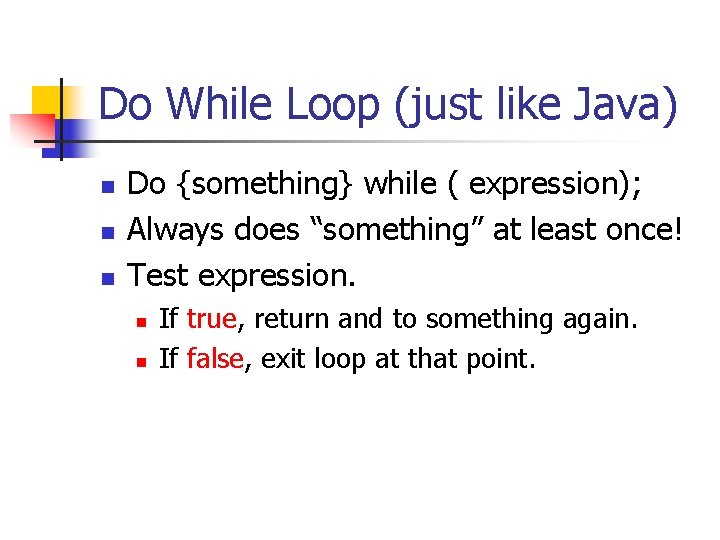
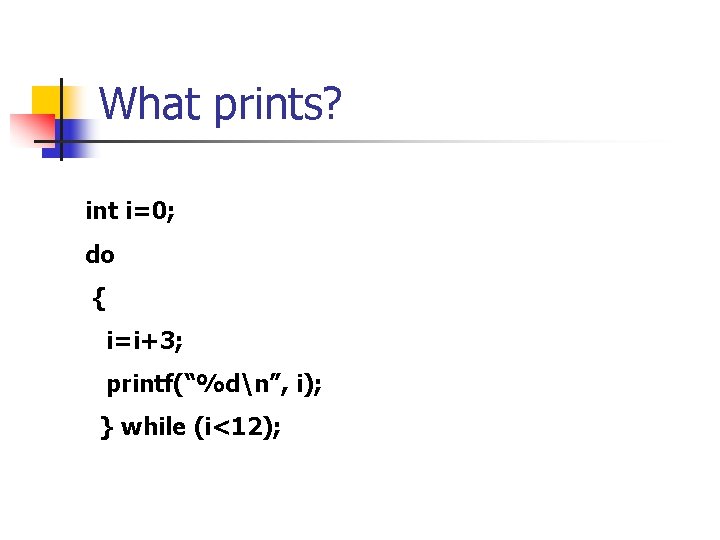
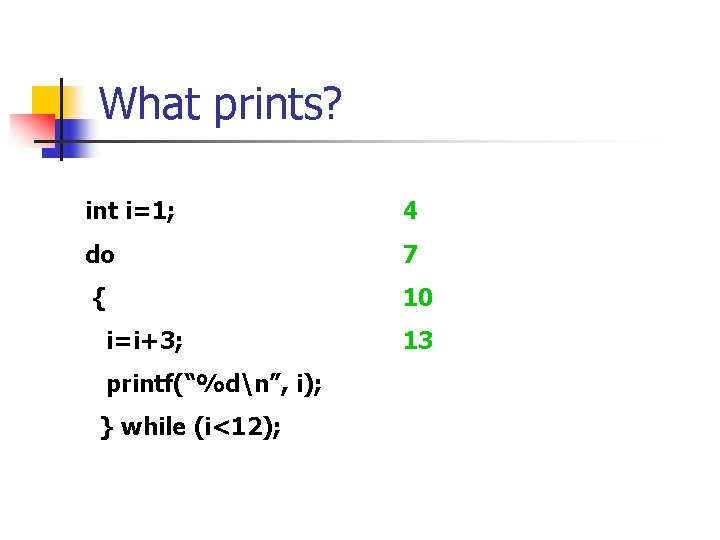
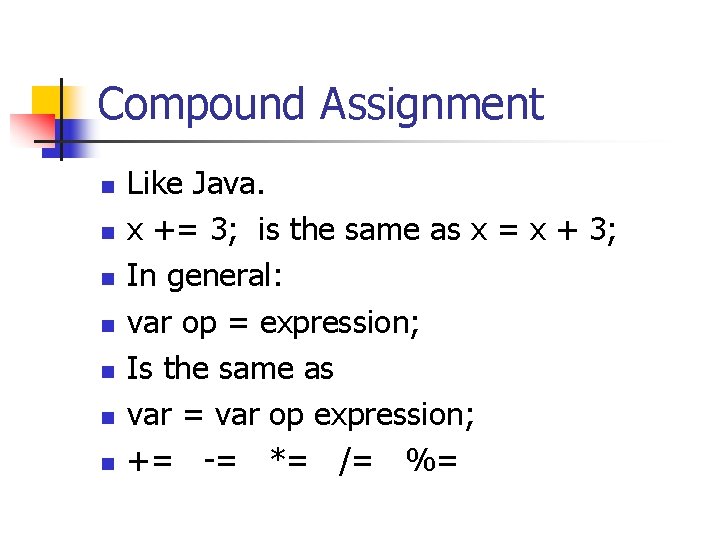
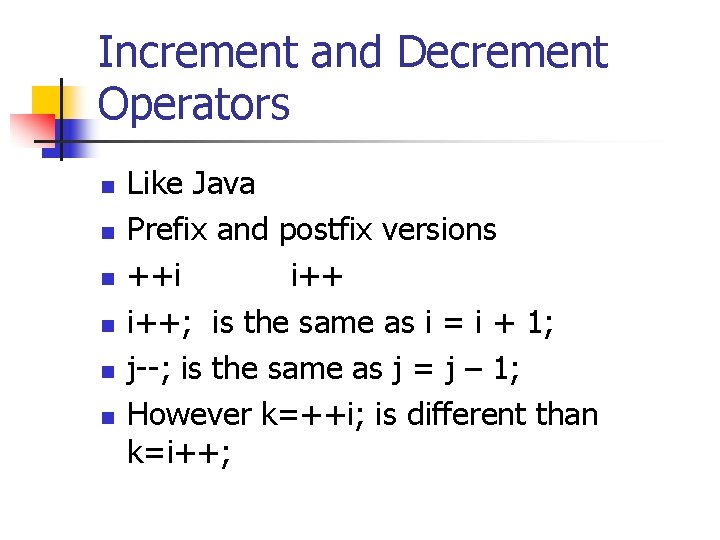
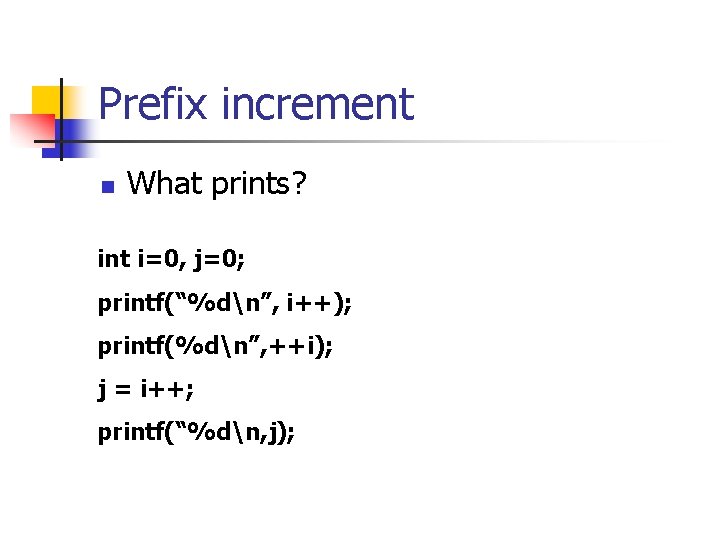
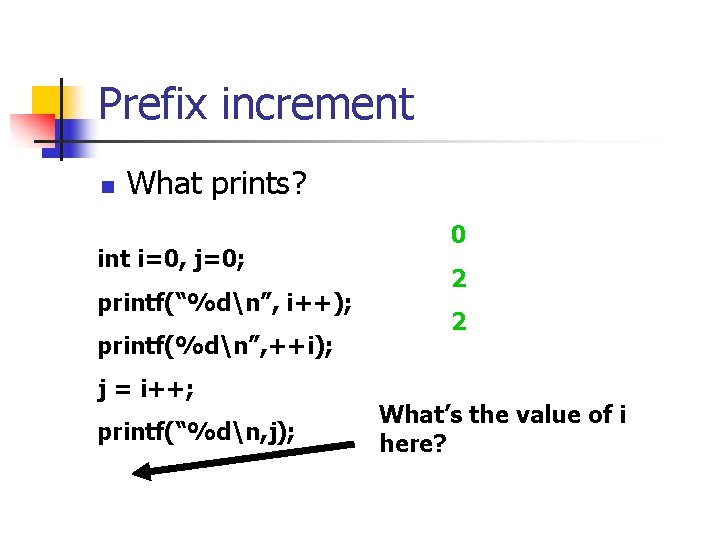
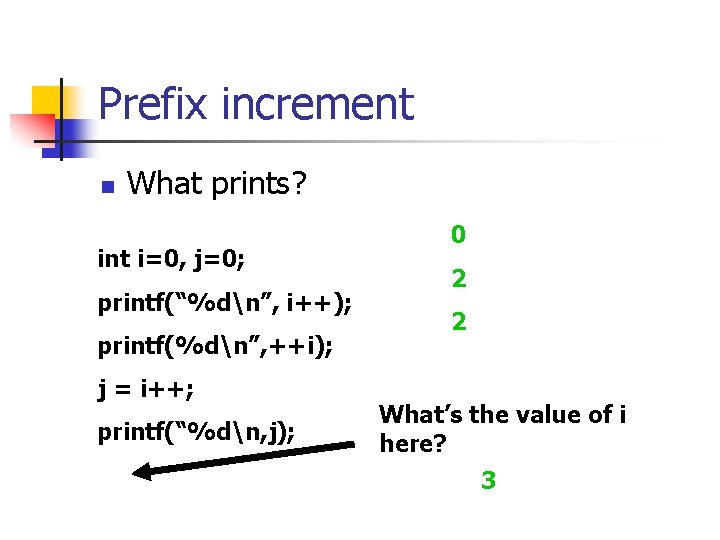
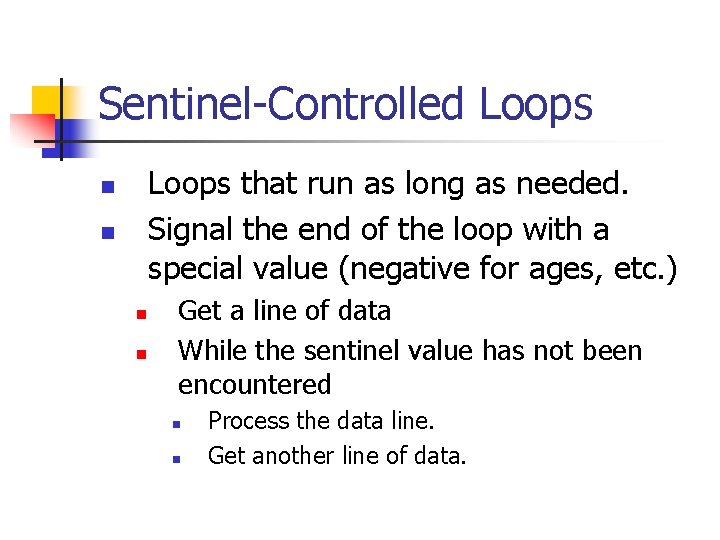
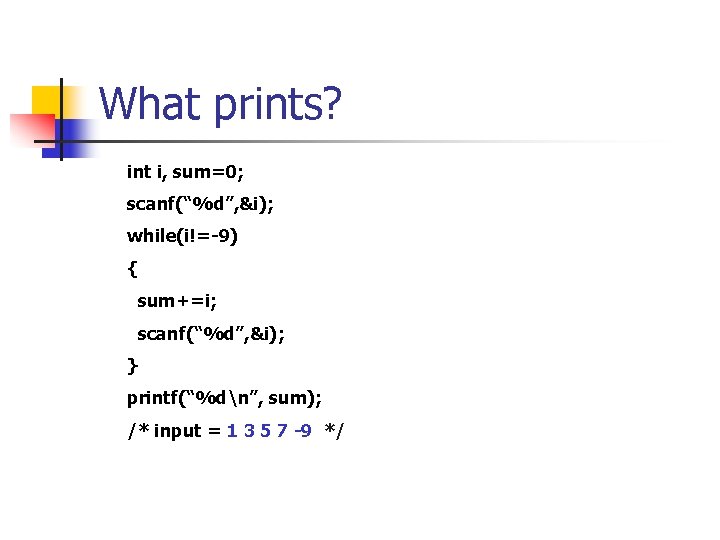
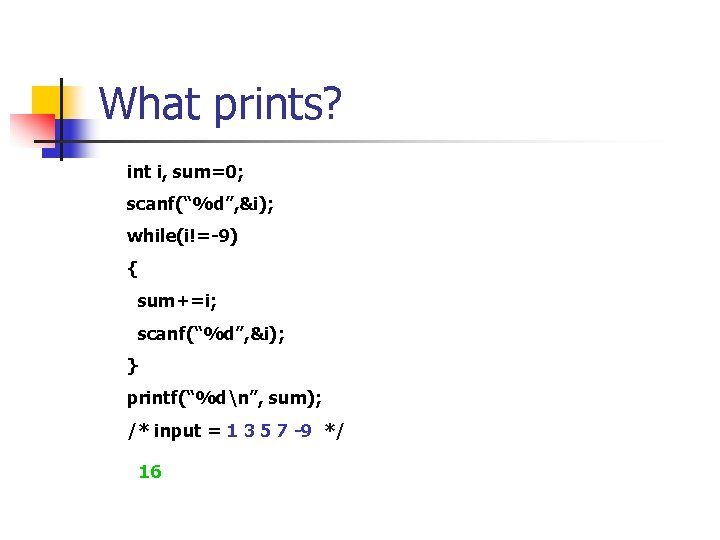
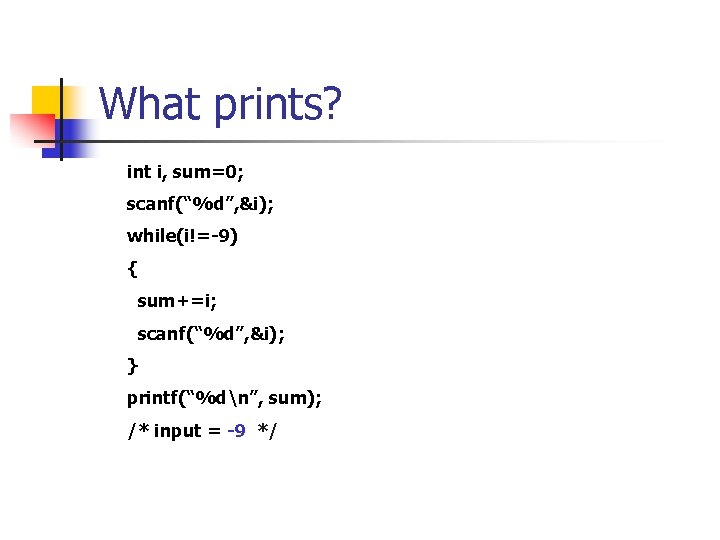
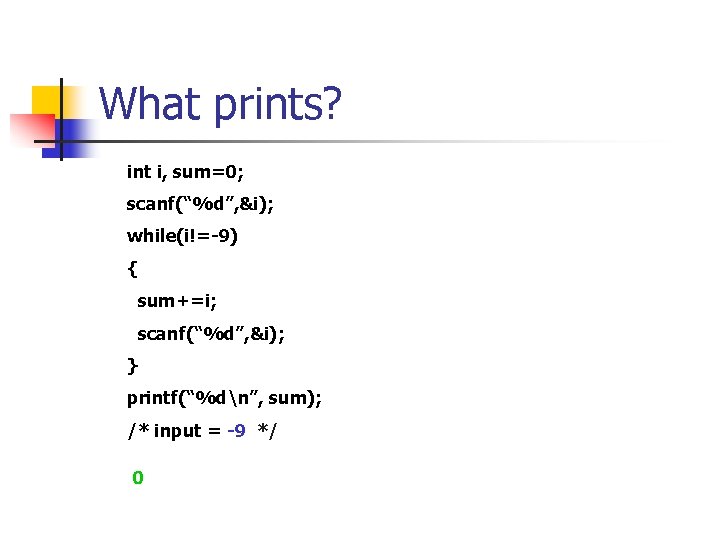
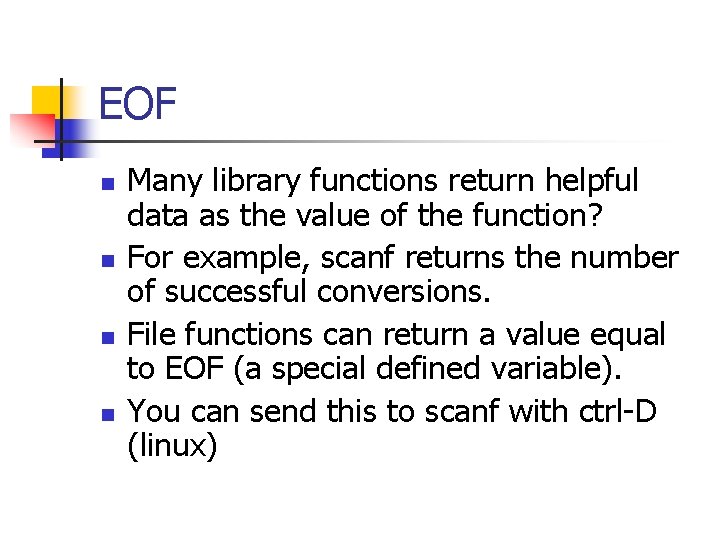
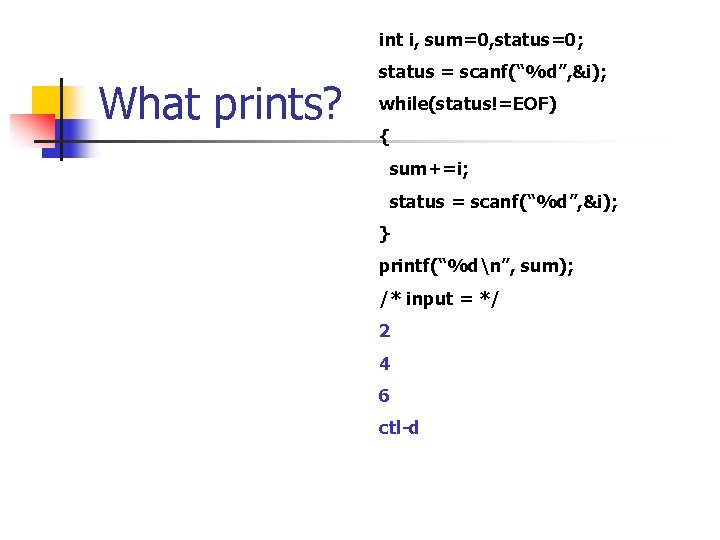
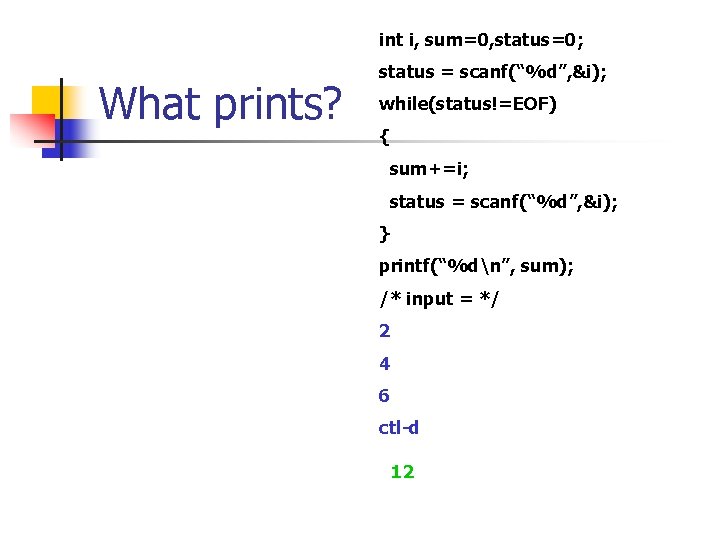
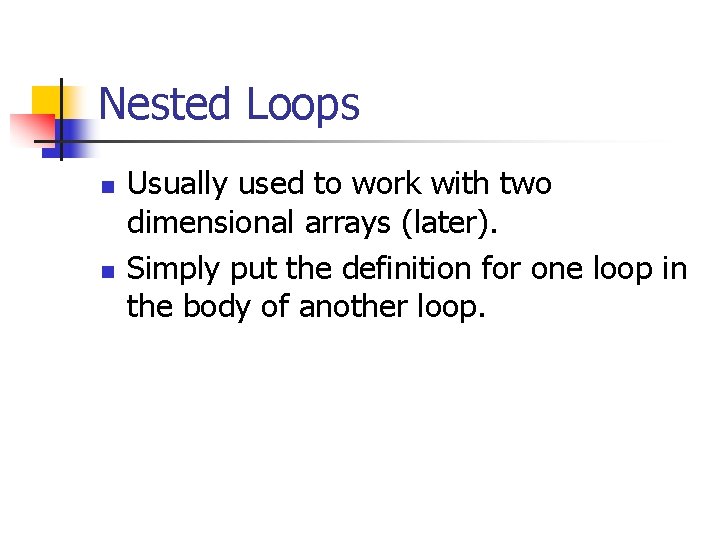
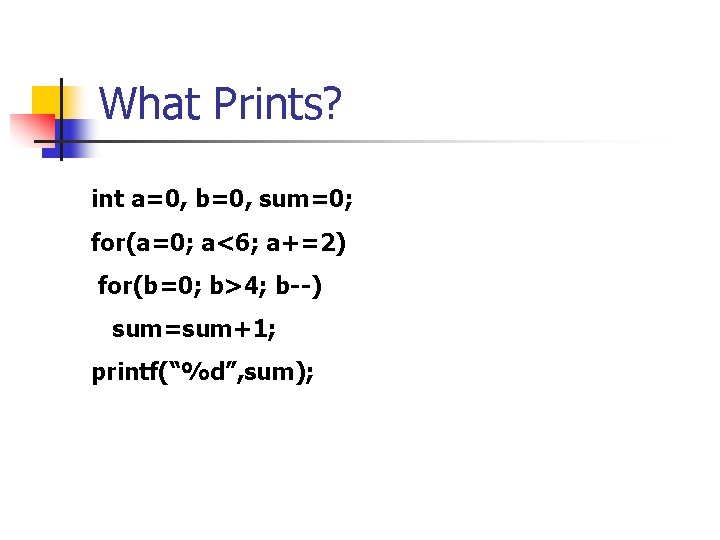
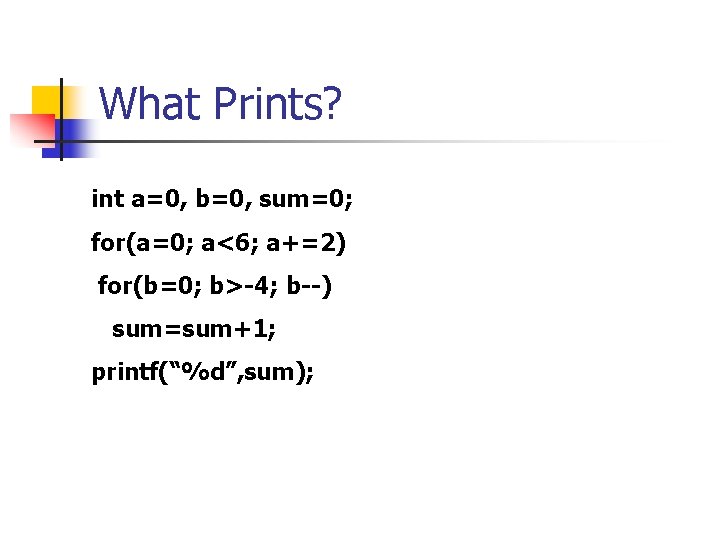
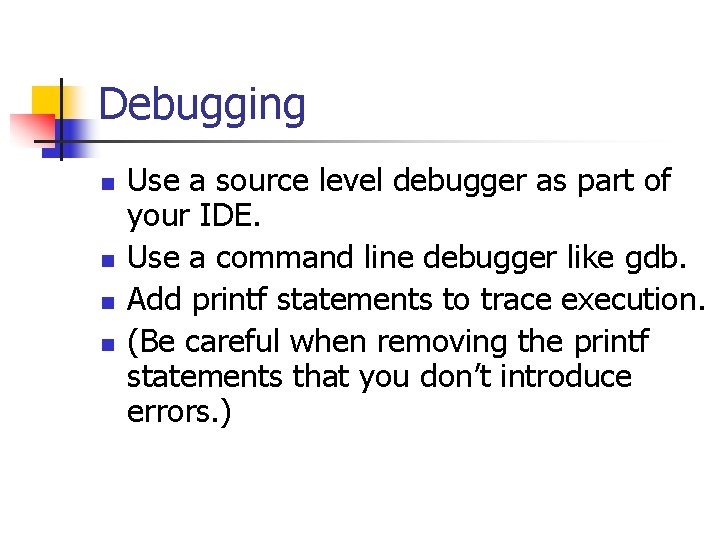
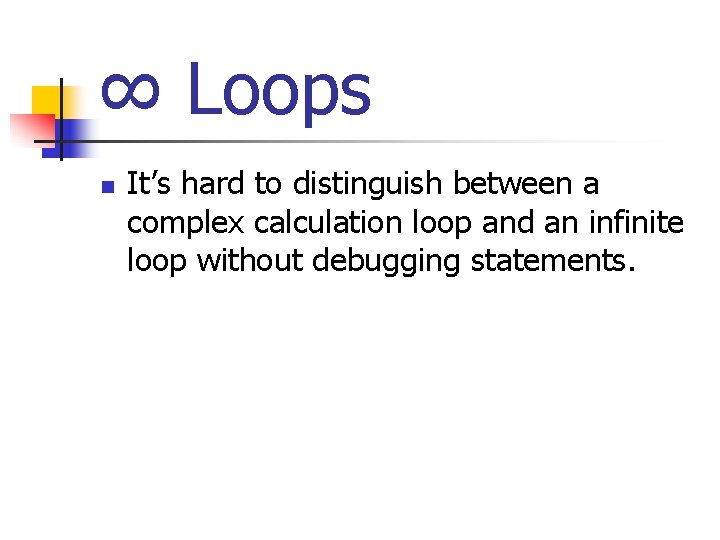
- Slides: 29
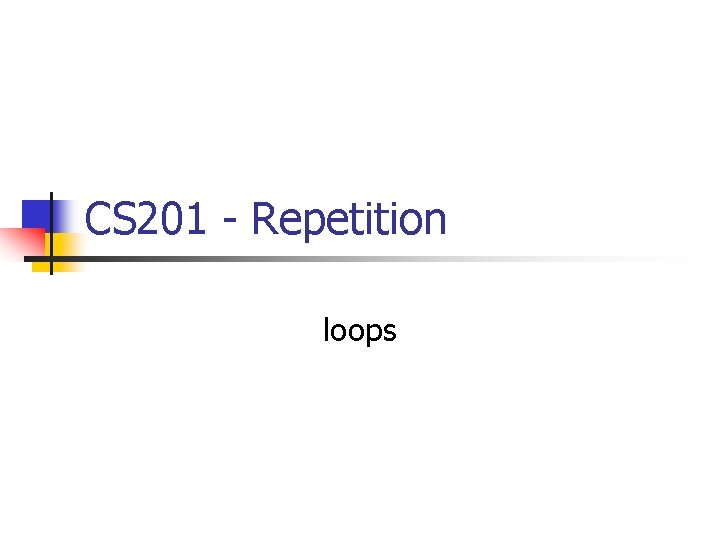
CS 201 - Repetition loops
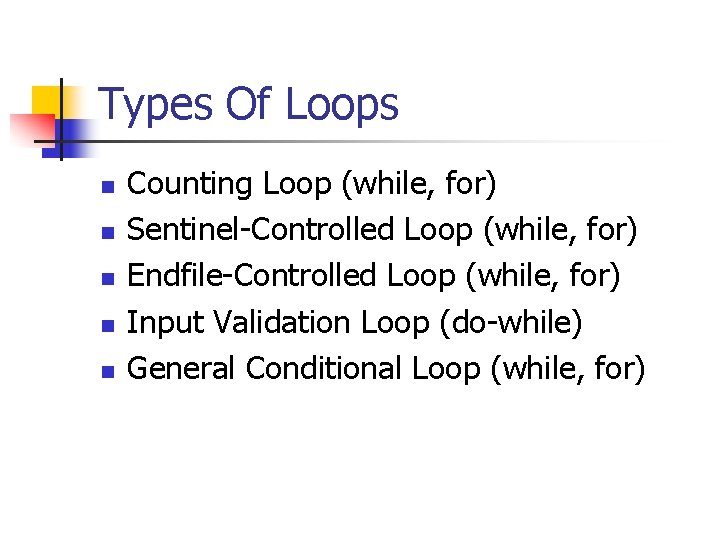
Types Of Loops n n n Counting Loop (while, for) Sentinel-Controlled Loop (while, for) Endfile-Controlled Loop (while, for) Input Validation Loop (do-while) General Conditional Loop (while, for)
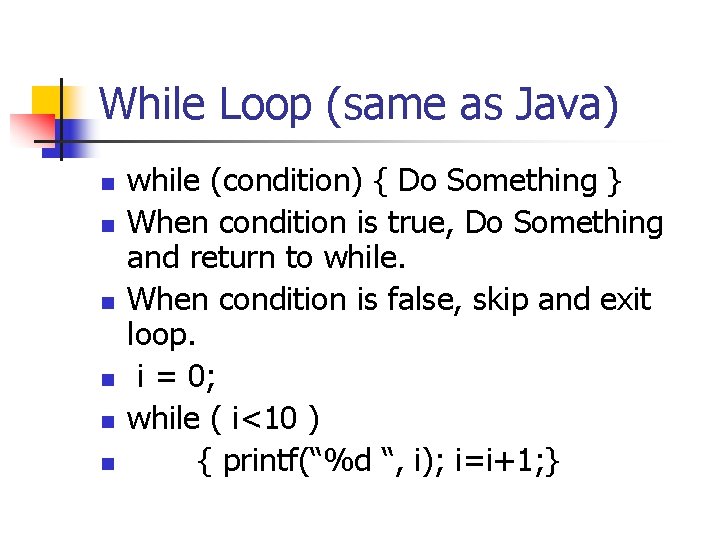
While Loop (same as Java) n n n while (condition) { Do Something } When condition is true, Do Something and return to while. When condition is false, skip and exit loop. i = 0; while ( i<10 ) { printf(“%d “, i); i=i+1; }
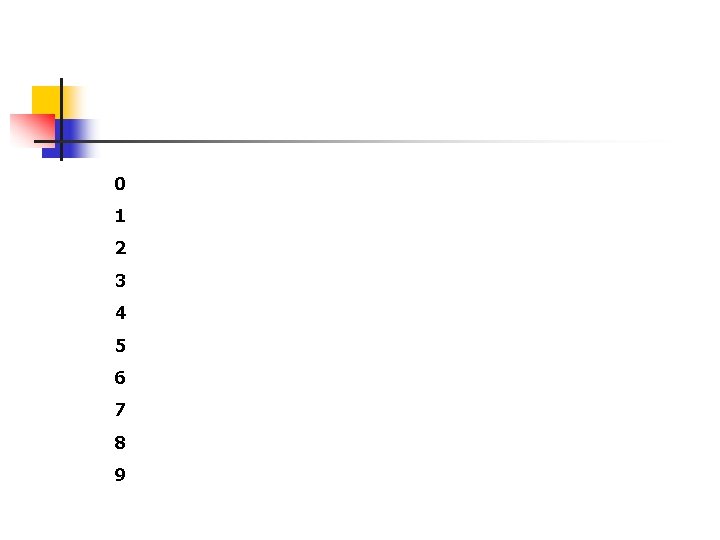
0 1 2 3 4 5 6 7 8 9
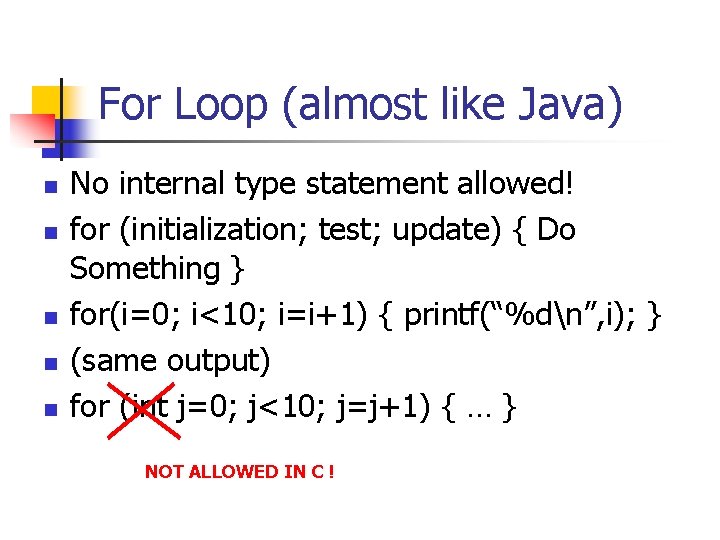
For Loop (almost like Java) n n n No internal type statement allowed! for (initialization; test; update) { Do Something } for(i=0; i<10; i=i+1) { printf(“%dn”, i); } (same output) for (int j=0; j<10; j=j+1) { … } NOT ALLOWED IN C !
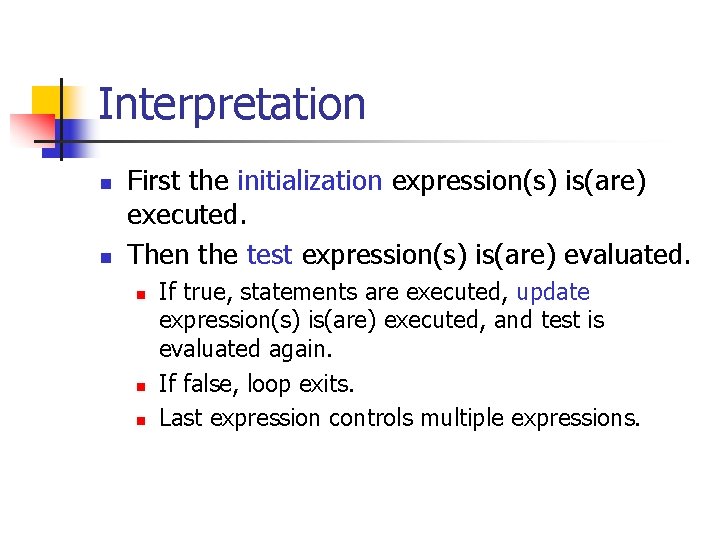
Interpretation n n First the initialization expression(s) is(are) executed. Then the test expression(s) is(are) evaluated. n n n If true, statements are executed, update expression(s) is(are) executed, and test is evaluated again. If false, loop exits. Last expression controls multiple expressions.
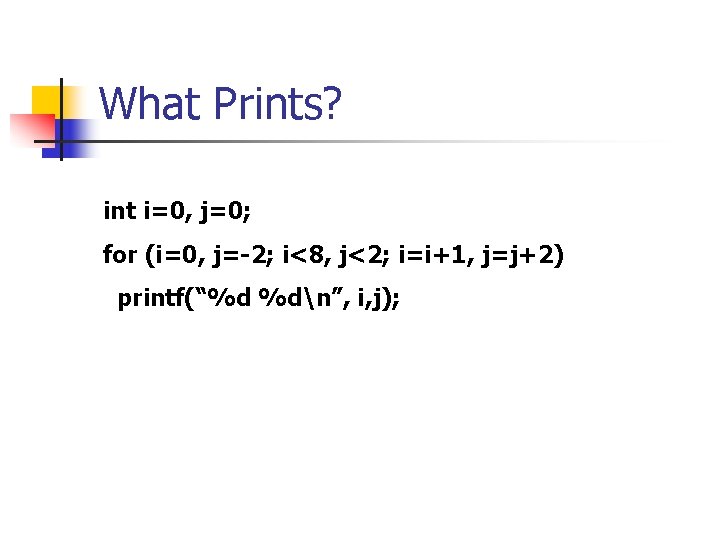
What Prints? int i=0, j=0; for (i=0, j=-2; i<8, j<2; i=i+1, j=j+2) printf(“%d %dn”, i, j);
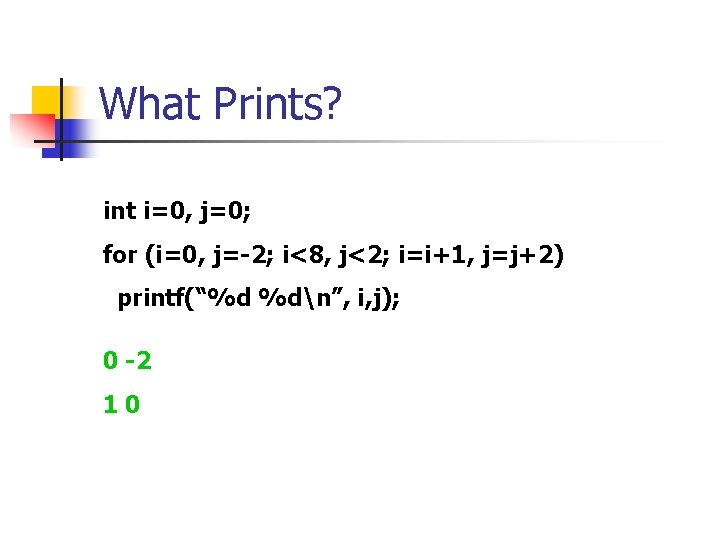
What Prints? int i=0, j=0; for (i=0, j=-2; i<8, j<2; i=i+1, j=j+2) printf(“%d %dn”, i, j); 0 -2 10
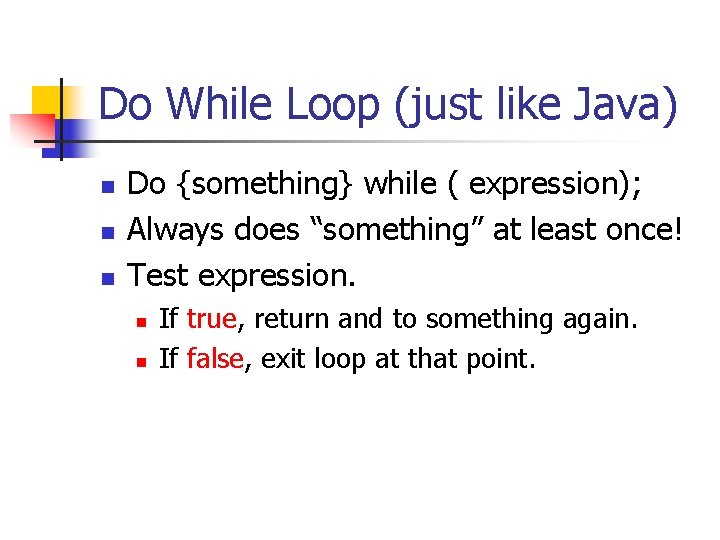
Do While Loop (just like Java) n n n Do {something} while ( expression); Always does “something” at least once! Test expression. n n If true, return and to something again. If false, exit loop at that point.
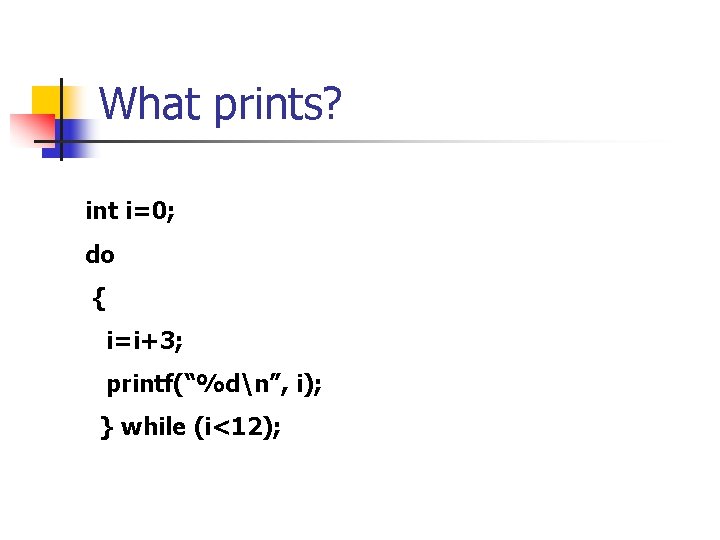
What prints? int i=0; do { i=i+3; printf(“%dn”, i); } while (i<12);
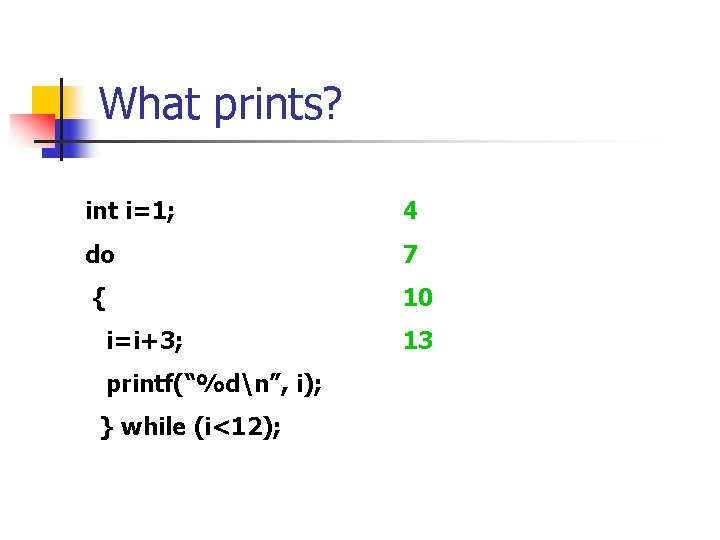
What prints? int i=1; 4 do 7 { 10 i=i+3; printf(“%dn”, i); } while (i<12); 13
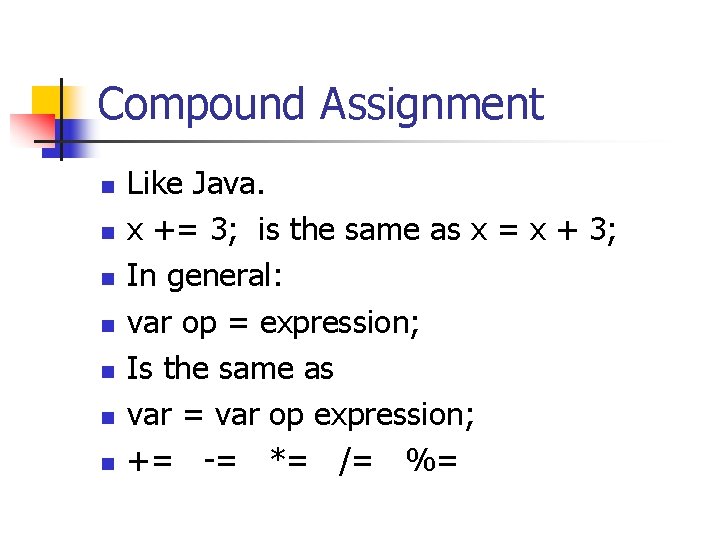
Compound Assignment n n n n Like Java. x += 3; is the same as x = x + 3; In general: var op = expression; Is the same as var = var op expression; += -= *= /= %=
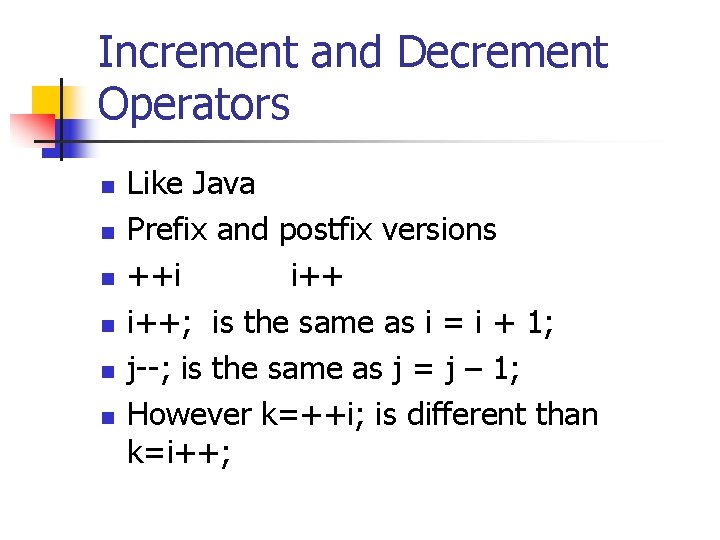
Increment and Decrement Operators n n n Like Java Prefix and postfix versions ++i i++; is the same as i = i + 1; j--; is the same as j = j – 1; However k=++i; is different than k=i++;
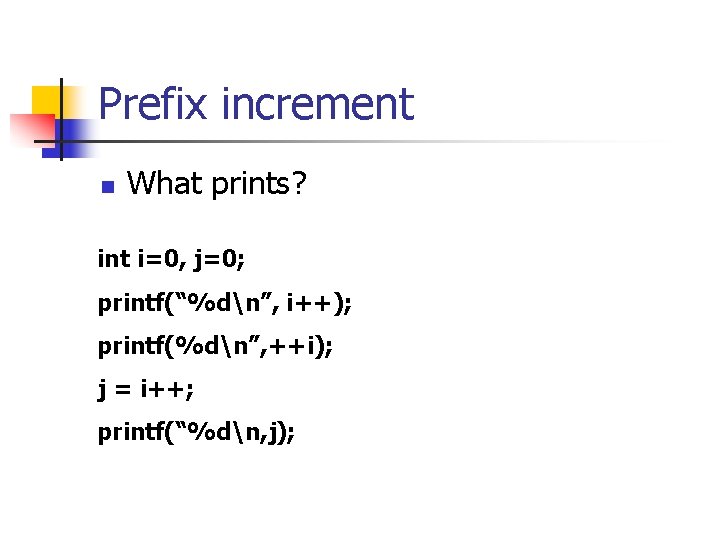
Prefix increment n What prints? int i=0, j=0; printf(“%dn”, i++); printf(%dn”, ++i); j = i++; printf(“%dn, j);
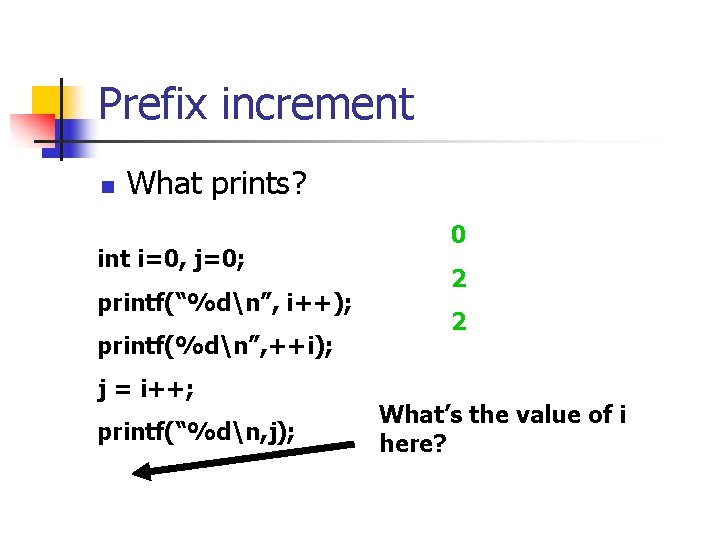
Prefix increment n What prints? int i=0, j=0; printf(“%dn”, i++); printf(%dn”, ++i); j = i++; printf(“%dn, j); 0 2 2 What’s the value of i here?
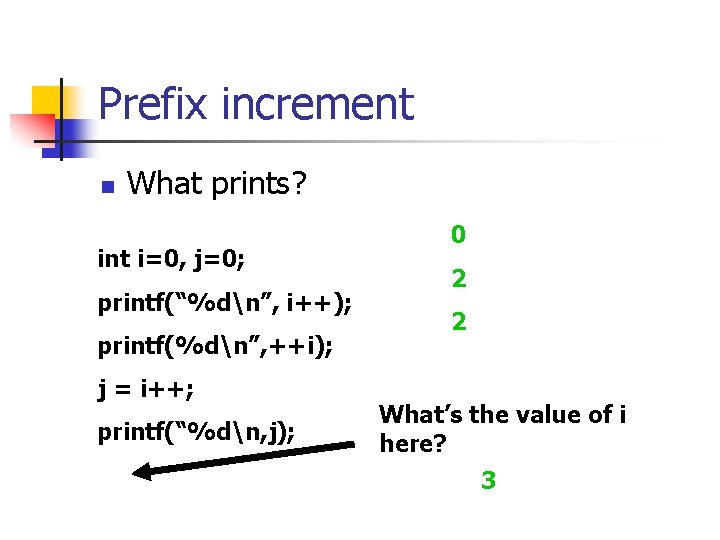
Prefix increment n What prints? int i=0, j=0; printf(“%dn”, i++); printf(%dn”, ++i); j = i++; printf(“%dn, j); 0 2 2 What’s the value of i here? 3
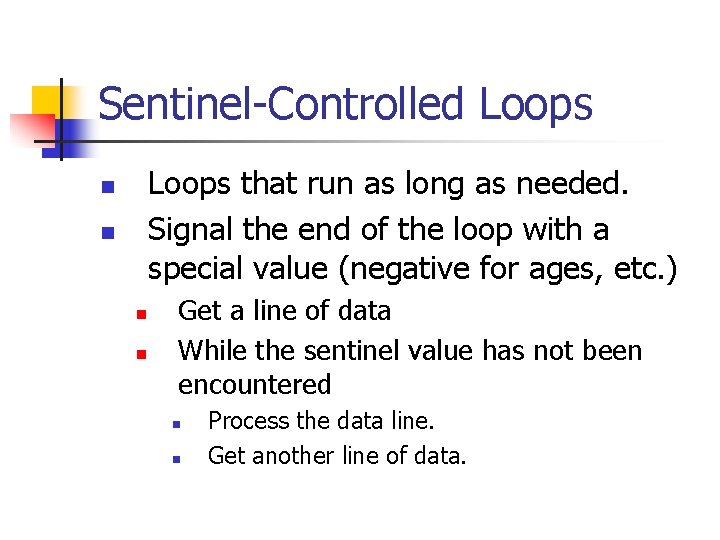
Sentinel-Controlled Loops that run as long as needed. Signal the end of the loop with a special value (negative for ages, etc. ) n n Get a line of data While the sentinel value has not been encountered n n Process the data line. Get another line of data.
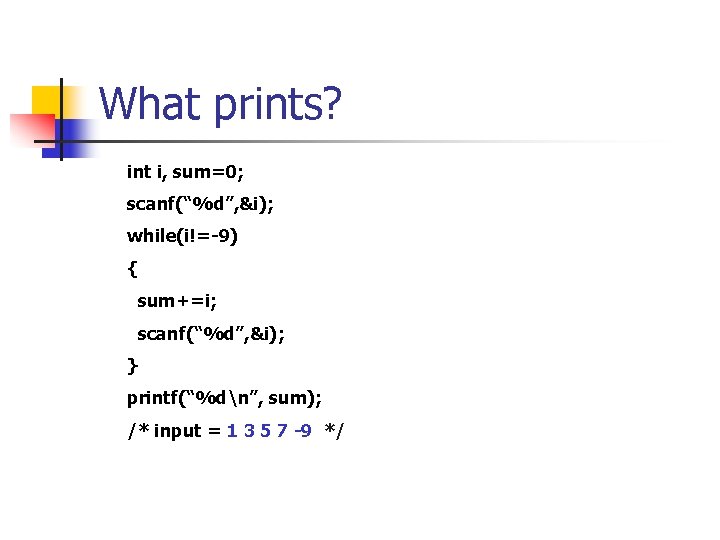
What prints? int i, sum=0; scanf(“%d”, &i); while(i!=-9) { sum+=i; scanf(“%d”, &i); } printf(“%dn”, sum); /* input = 1 3 5 7 -9 */
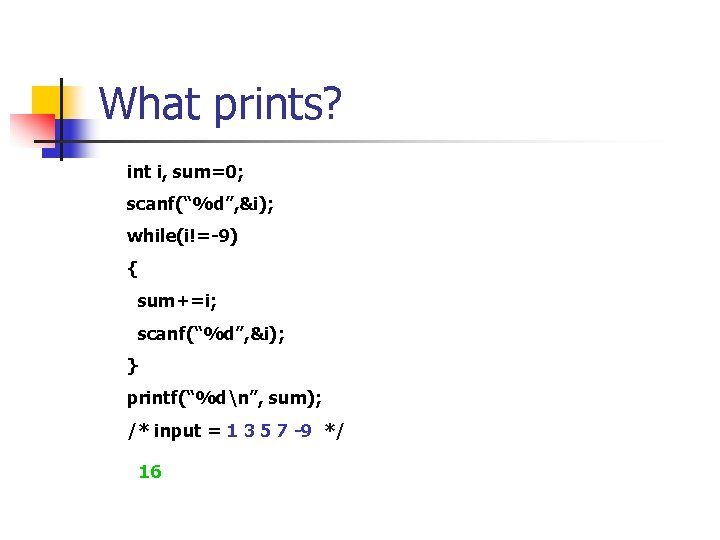
What prints? int i, sum=0; scanf(“%d”, &i); while(i!=-9) { sum+=i; scanf(“%d”, &i); } printf(“%dn”, sum); /* input = 1 3 5 7 -9 */ 16
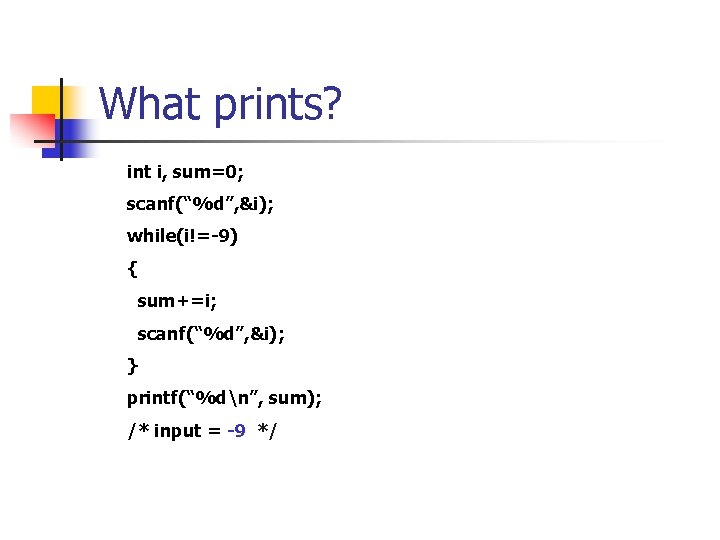
What prints? int i, sum=0; scanf(“%d”, &i); while(i!=-9) { sum+=i; scanf(“%d”, &i); } printf(“%dn”, sum); /* input = -9 */
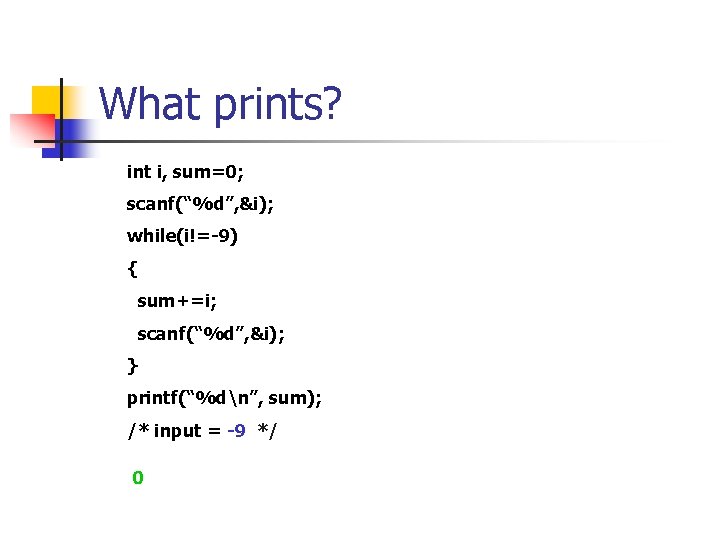
What prints? int i, sum=0; scanf(“%d”, &i); while(i!=-9) { sum+=i; scanf(“%d”, &i); } printf(“%dn”, sum); /* input = -9 */ 0
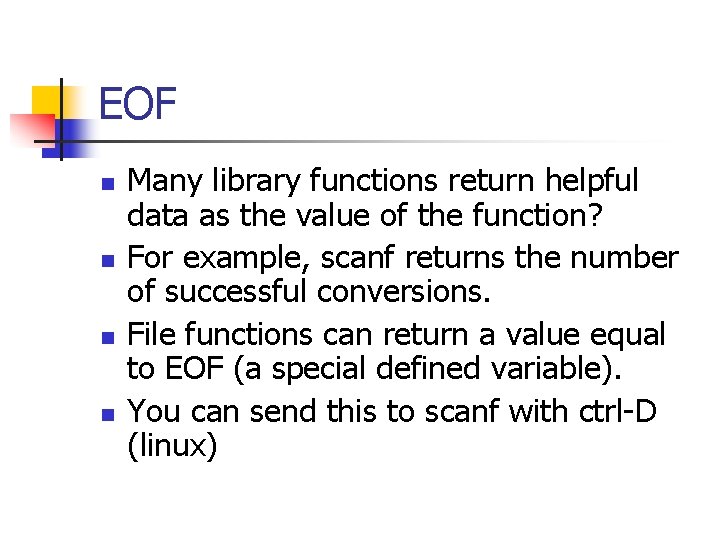
EOF n n Many library functions return helpful data as the value of the function? For example, scanf returns the number of successful conversions. File functions can return a value equal to EOF (a special defined variable). You can send this to scanf with ctrl-D (linux)
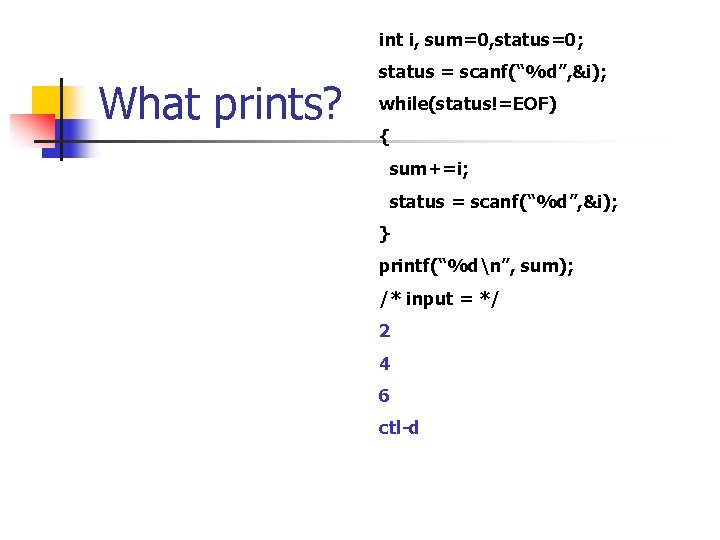
int i, sum=0, status=0; What prints? status = scanf(“%d”, &i); while(status!=EOF) { sum+=i; status = scanf(“%d”, &i); } printf(“%dn”, sum); /* input = */ 2 4 6 ctl-d
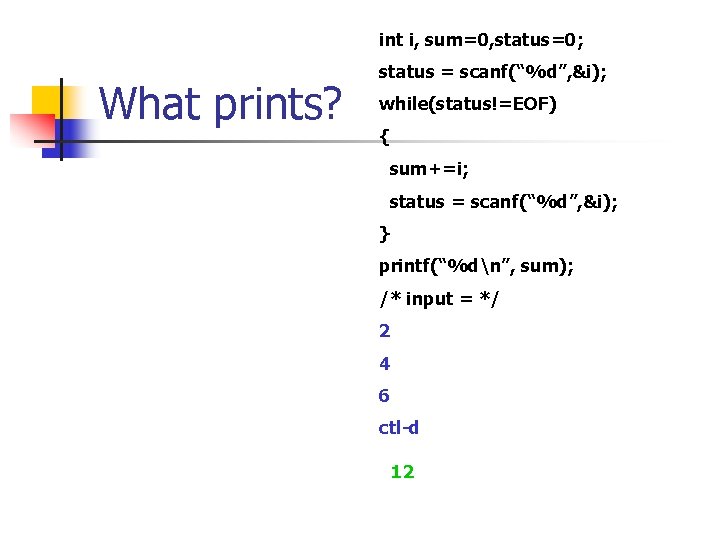
int i, sum=0, status=0; What prints? status = scanf(“%d”, &i); while(status!=EOF) { sum+=i; status = scanf(“%d”, &i); } printf(“%dn”, sum); /* input = */ 2 4 6 ctl-d 12
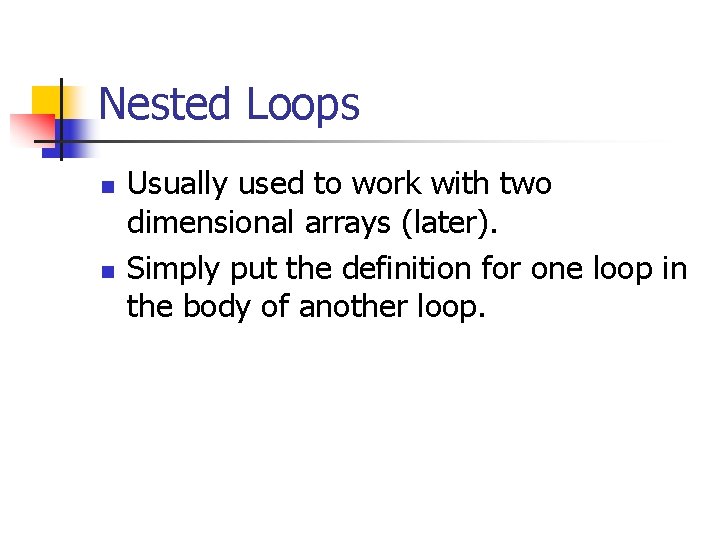
Nested Loops n n Usually used to work with two dimensional arrays (later). Simply put the definition for one loop in the body of another loop.
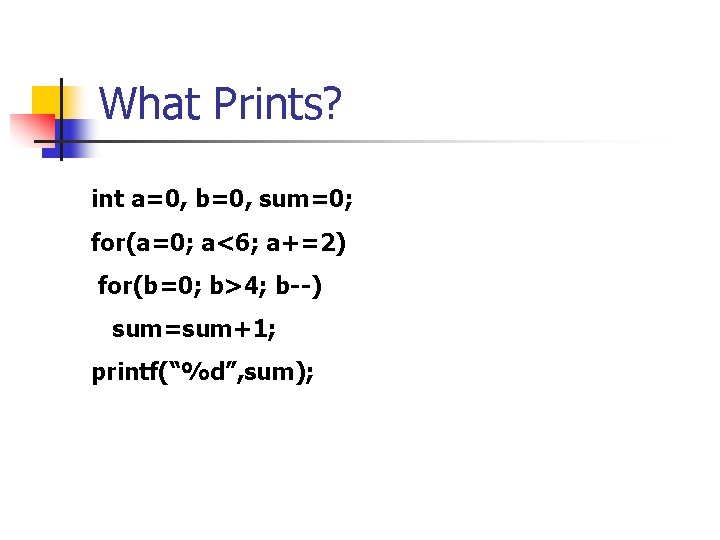
What Prints? int a=0, b=0, sum=0; for(a=0; a<6; a+=2) for(b=0; b>4; b--) sum=sum+1; printf(“%d”, sum);
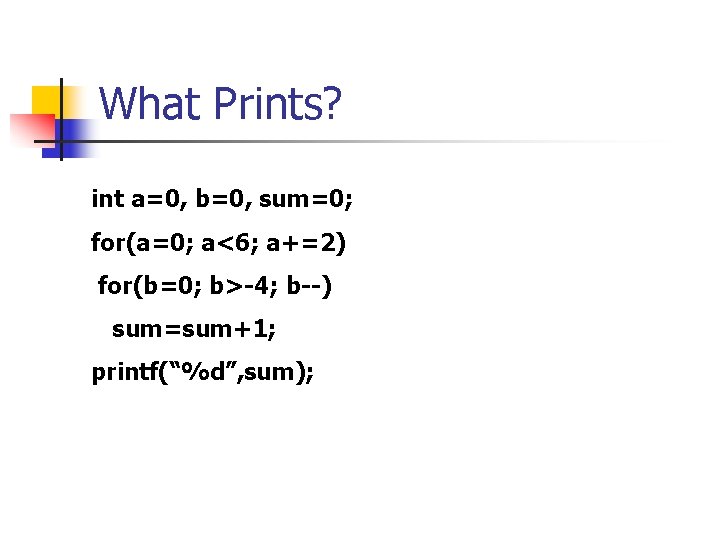
What Prints? int a=0, b=0, sum=0; for(a=0; a<6; a+=2) for(b=0; b>-4; b--) sum=sum+1; printf(“%d”, sum);
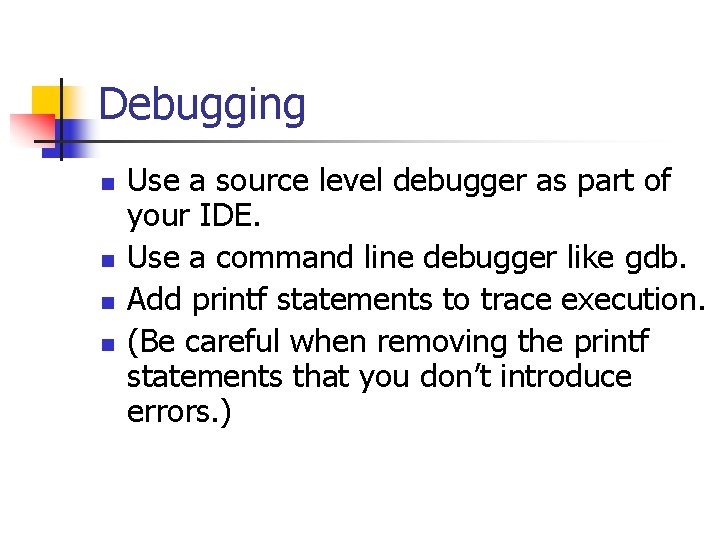
Debugging n n Use a source level debugger as part of your IDE. Use a command line debugger like gdb. Add printf statements to trace execution. (Be careful when removing the printf statements that you don’t introduce errors. )
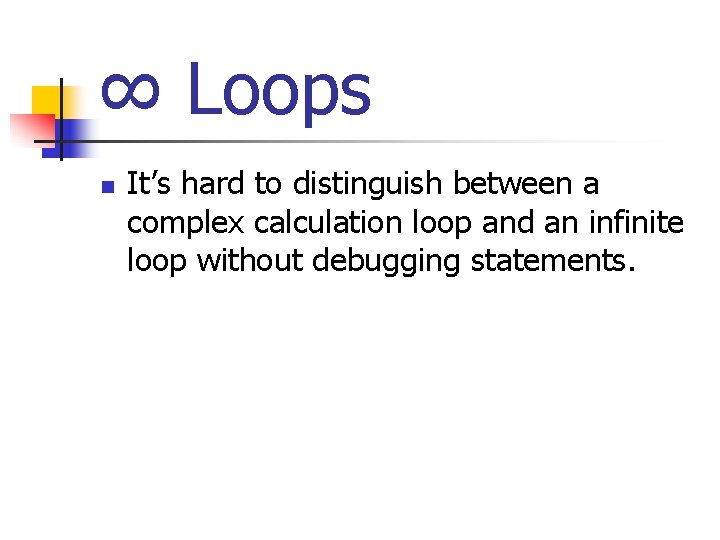
∞ Loops n It’s hard to distinguish between a complex calculation loop and an infinite loop without debugging statements.
What is bandwagon advertisement
Types of loops in matlab
Types of repetition
Types of repetition
Types of repetition
Types of repetition
While loops and if-else structures
Flow volume loop obstructive
Reddish loops of gas
Break statement in matlab
Programming puzzle
Perulangan looping
Underground heat exchanger
Matlab for loop
Cakewalk loop construction
Featureless loops of wangensteen
Nodes branches and loops formula
Adobe audition loops
Nested loops python
While loop in matlab
Python sentinel loop
Pseudocode nested if
Looping bersarang
Gas-filled bowel loops in abdomen meaning
Turtle small basic
Reddish loops of gas that link parts of sunspot regions
Meconium ileus
Non touching loops in control system
Control roadmap
Loops o repeticiones