CS 146 Data Structures and Algorithms June 30
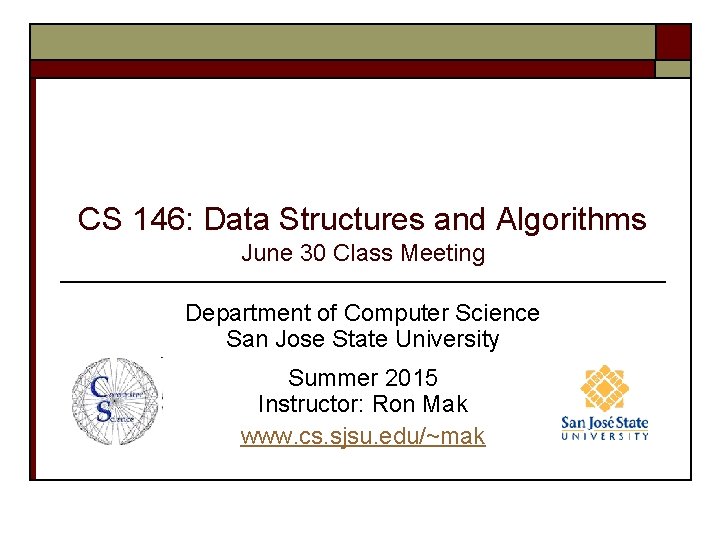
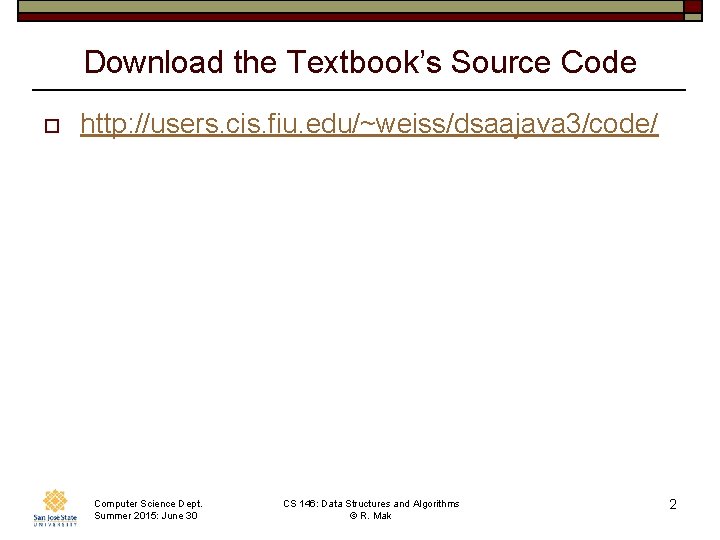
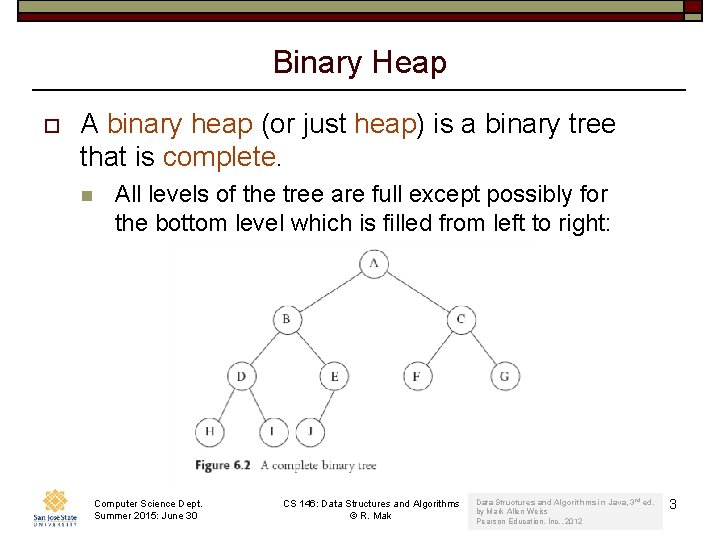
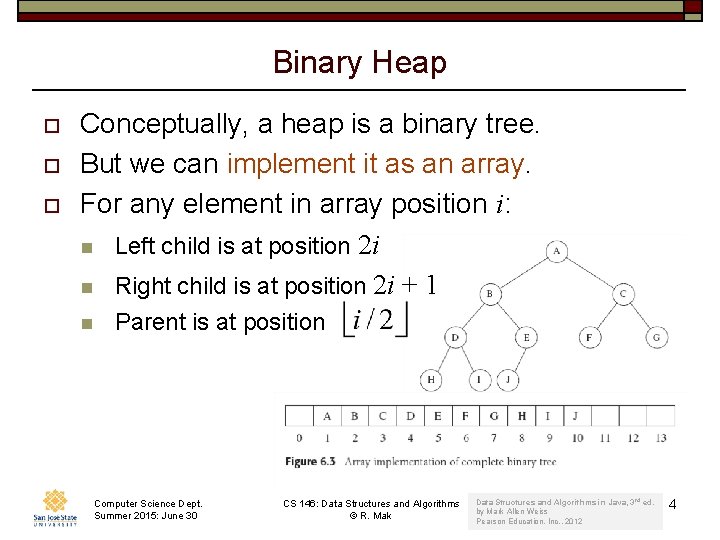
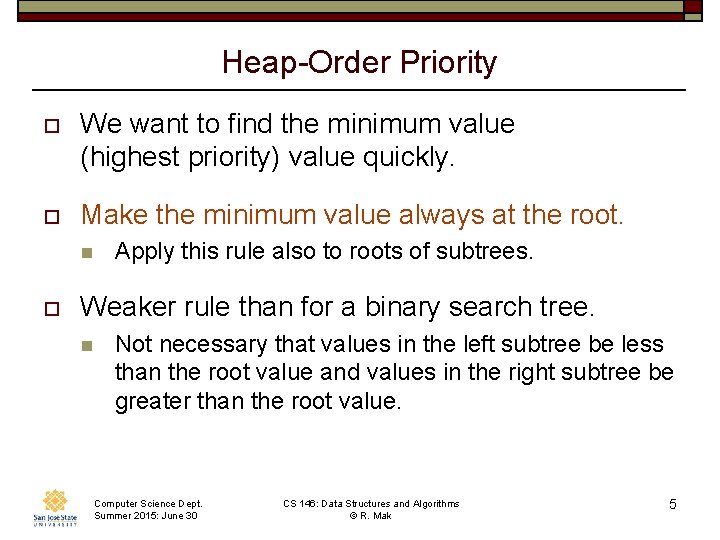
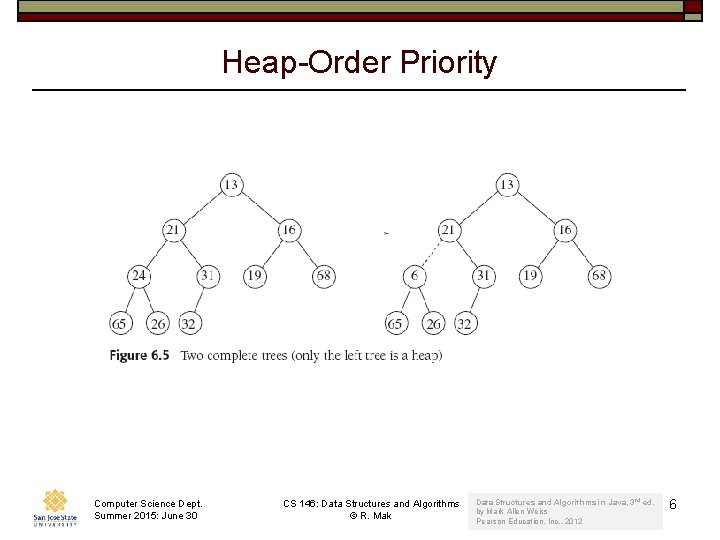
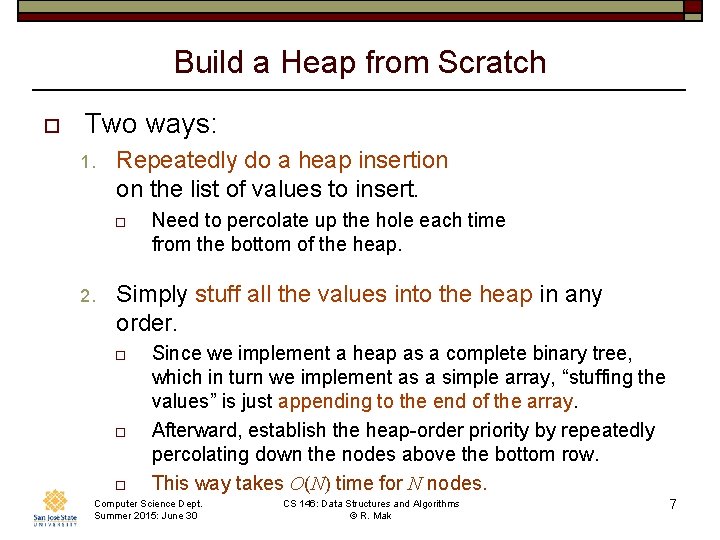
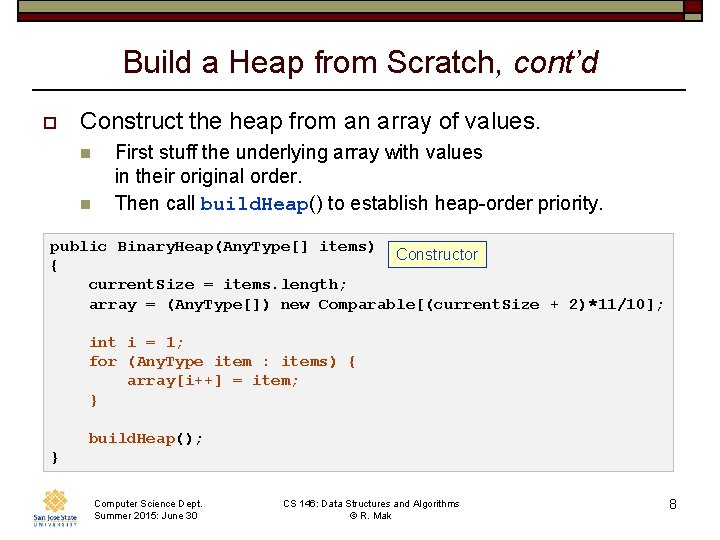
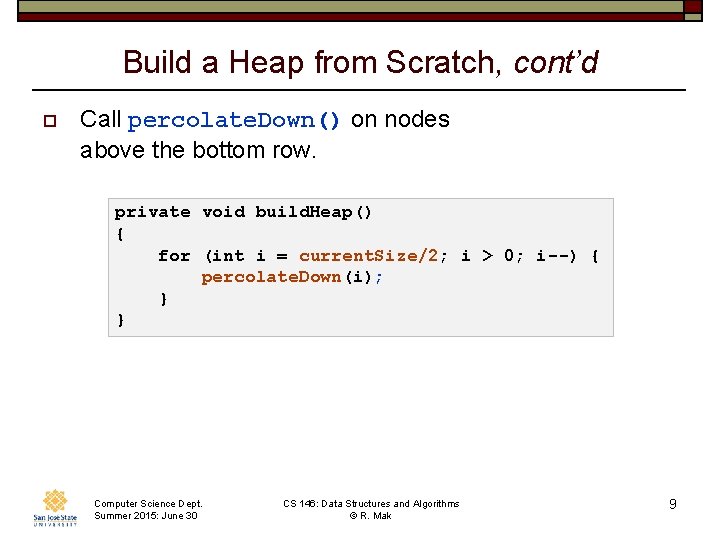
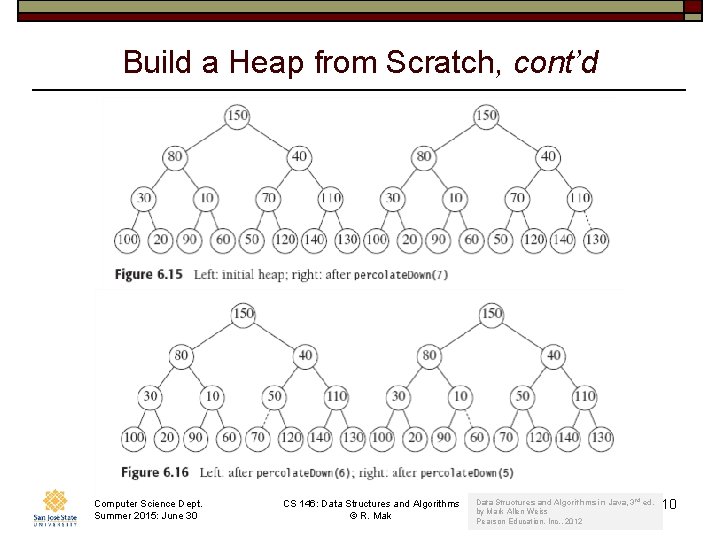
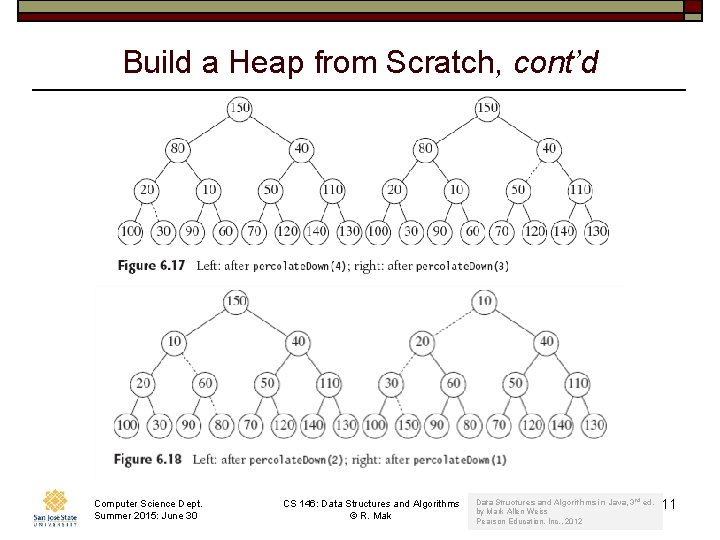
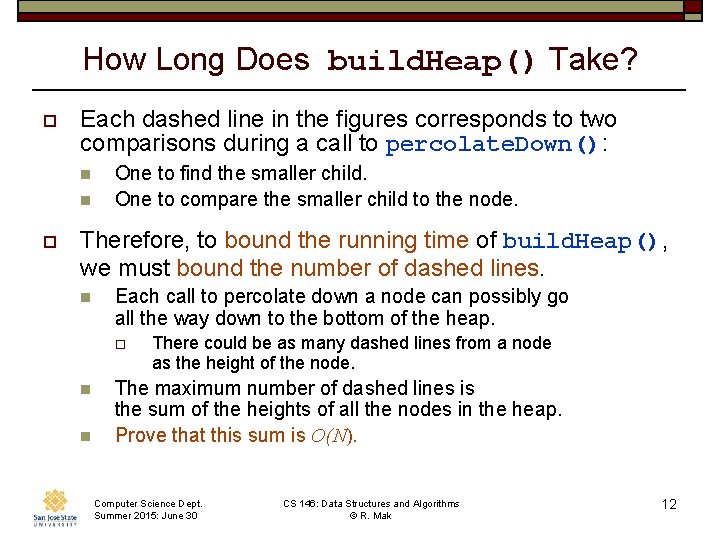
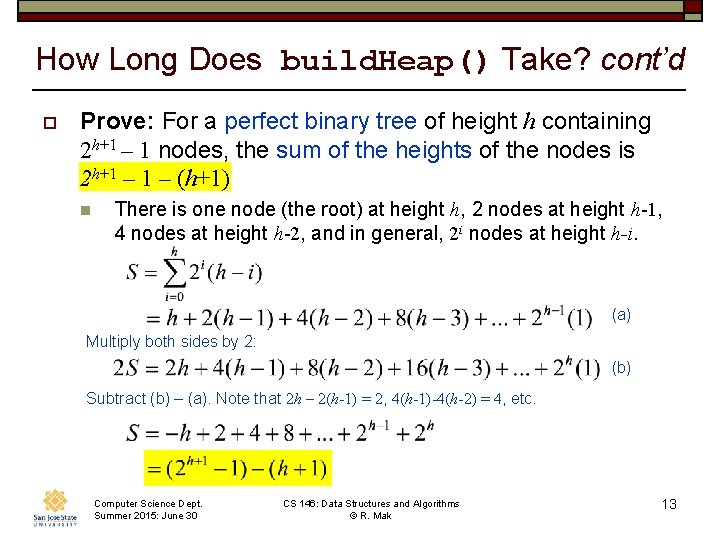
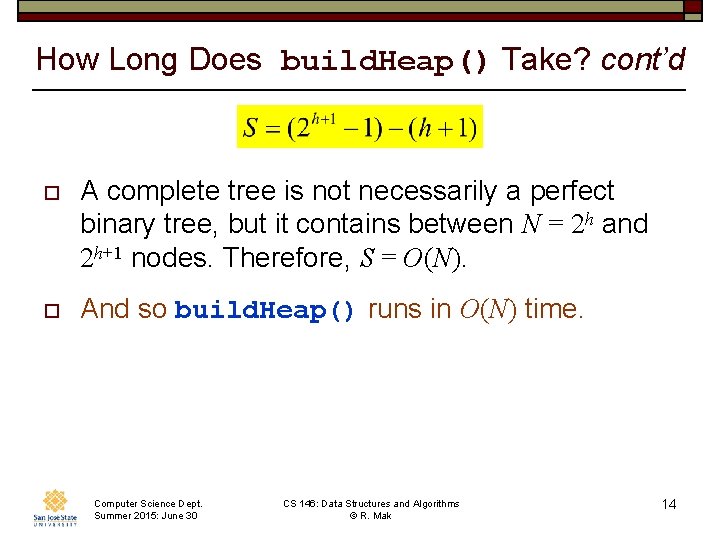
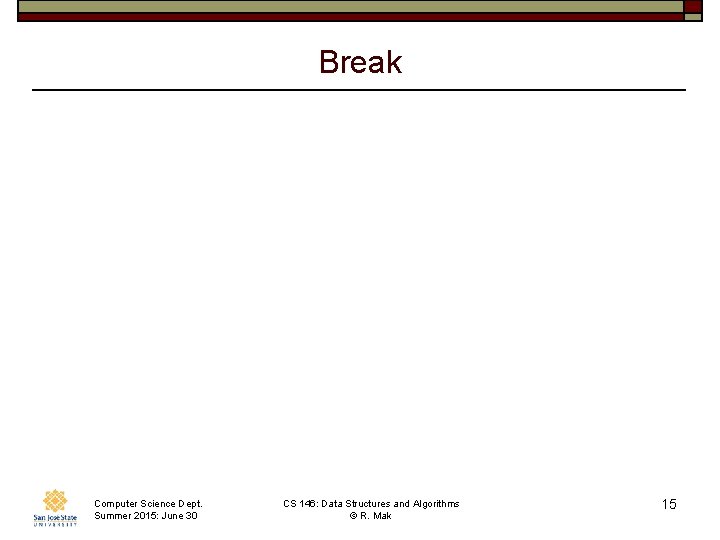
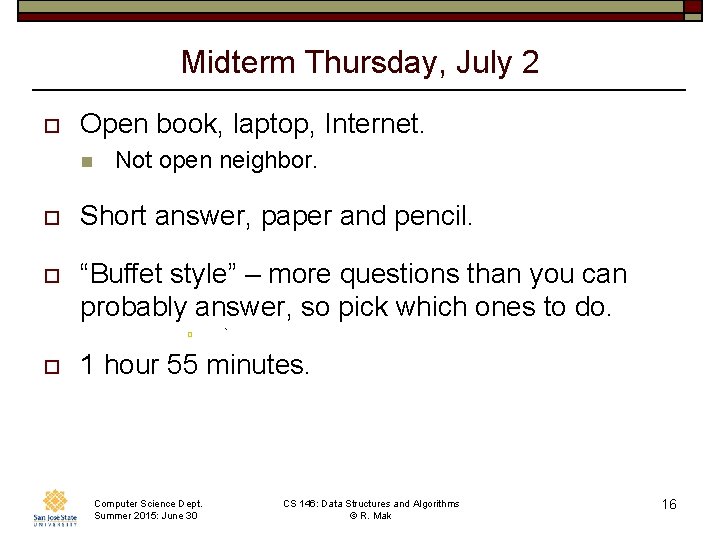
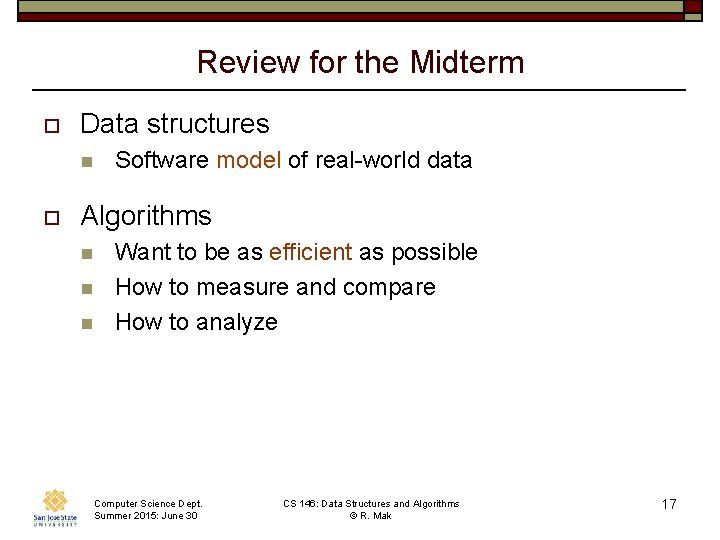
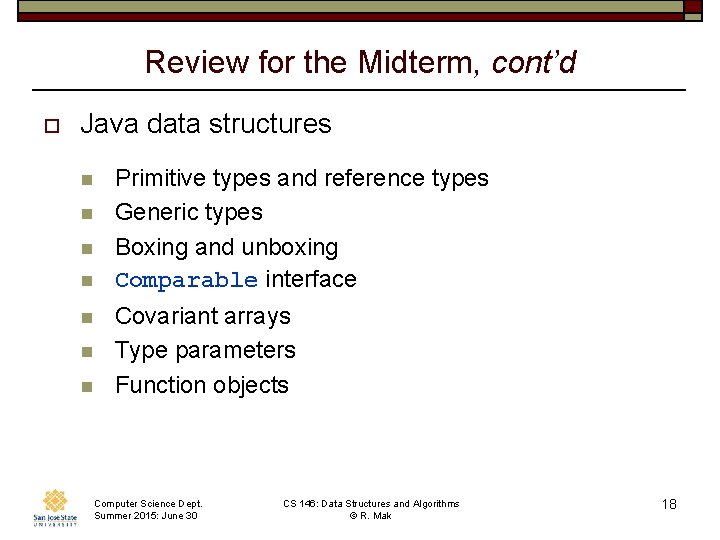
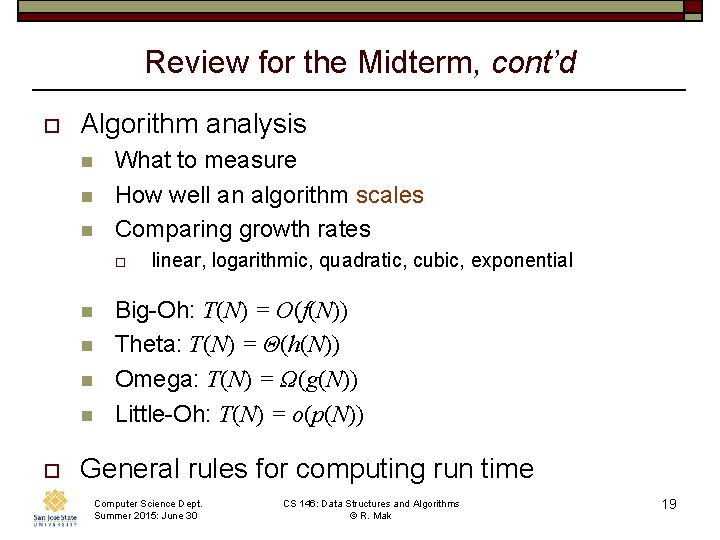
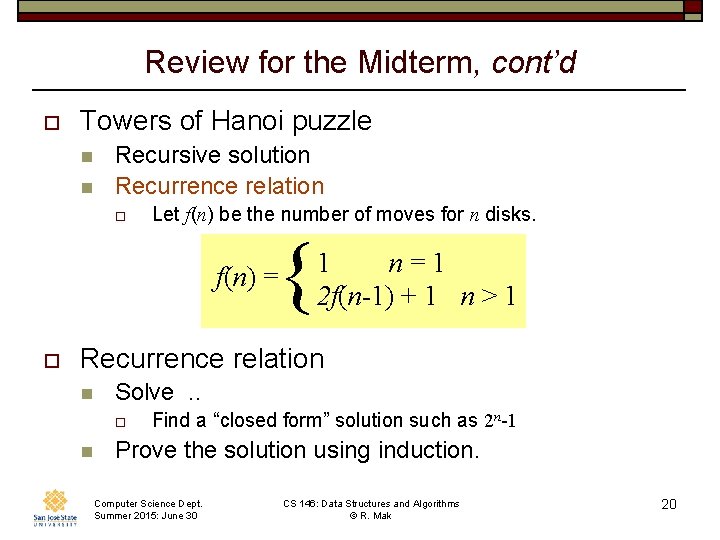
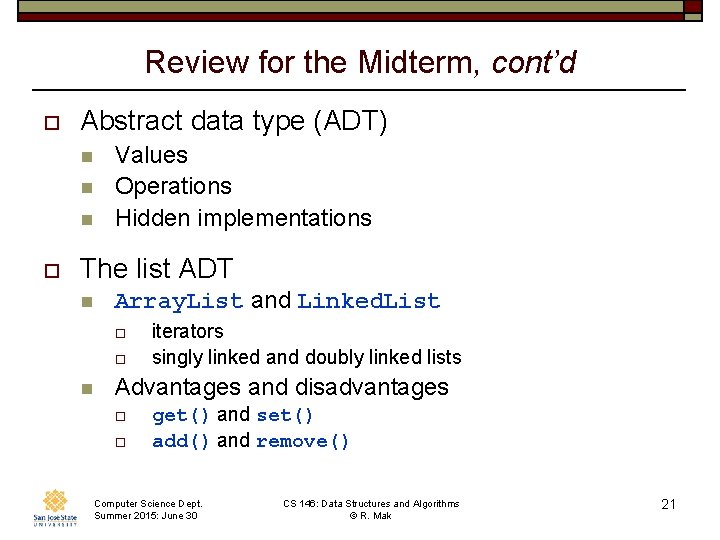
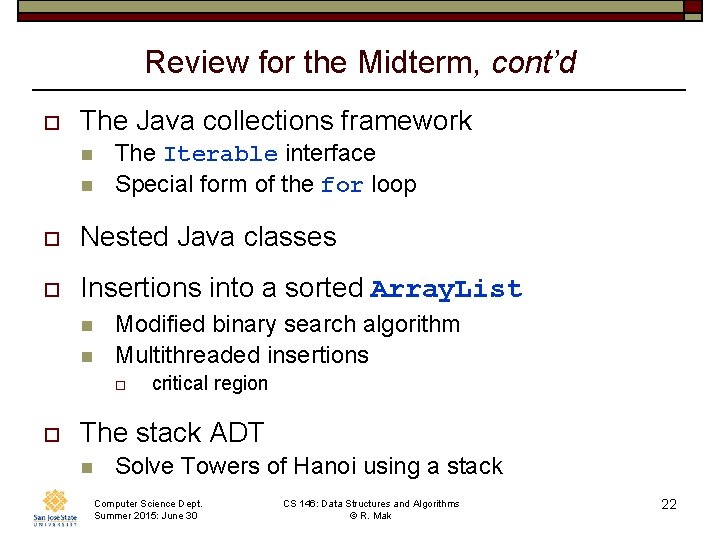
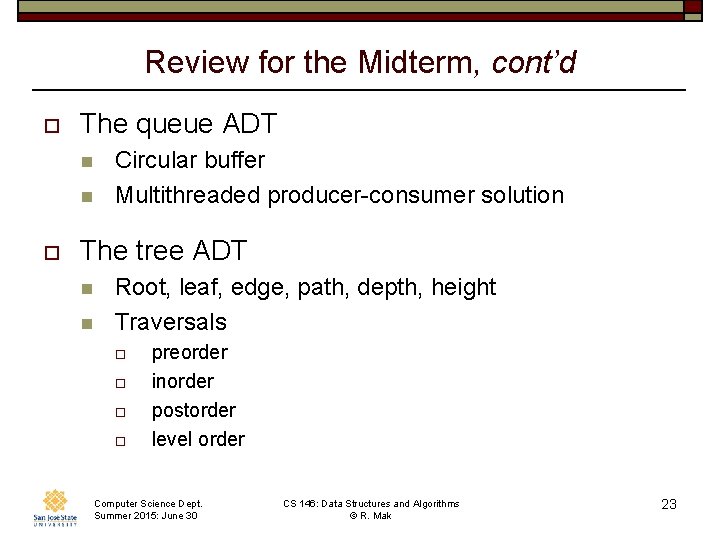
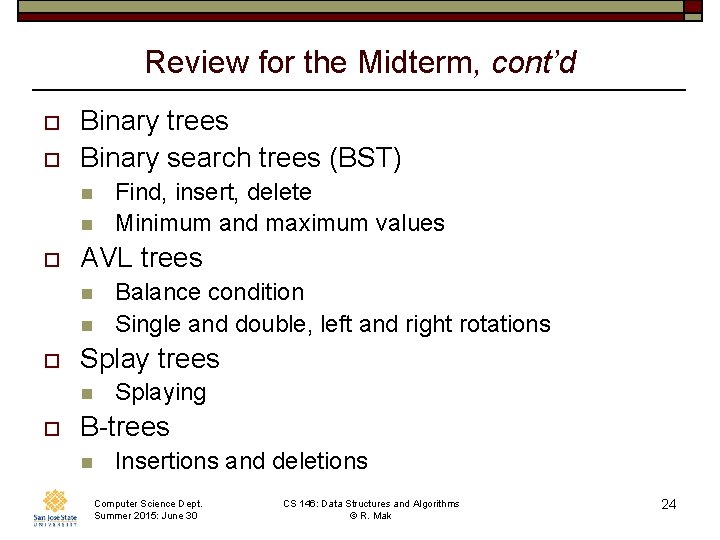
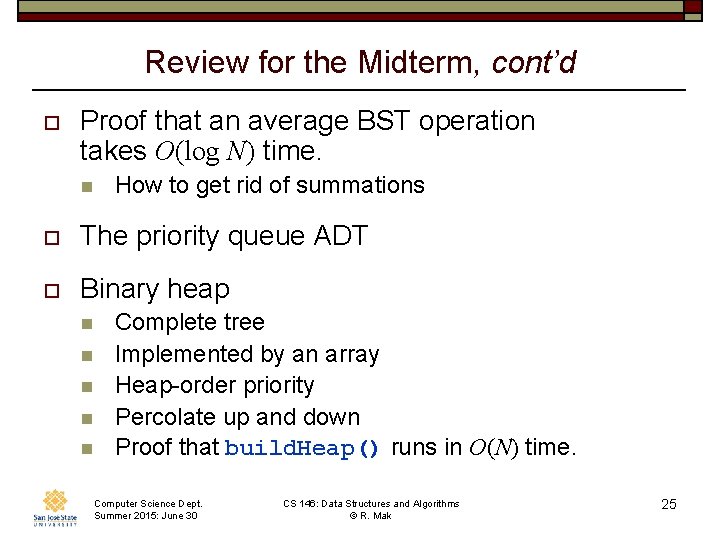
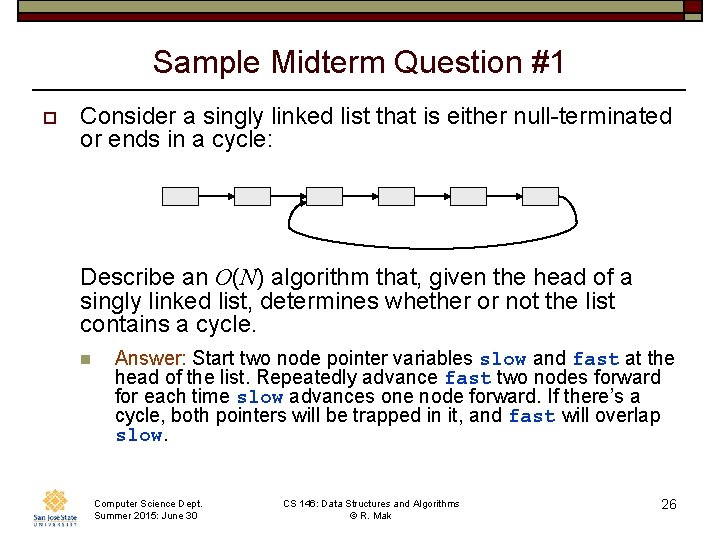
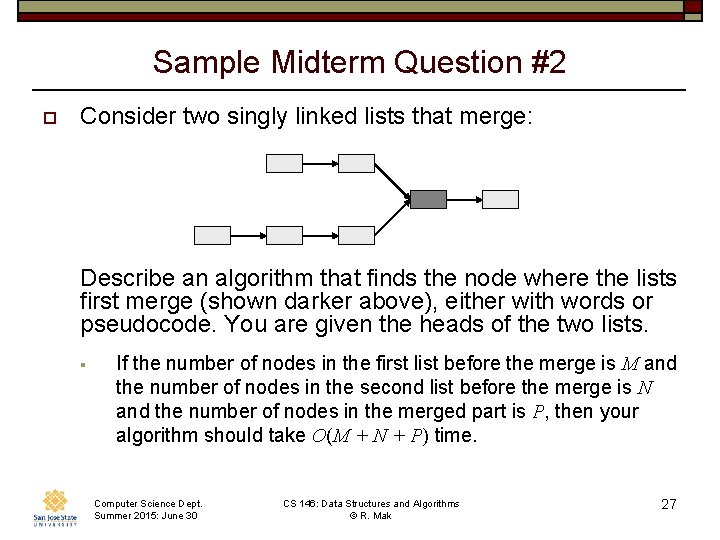
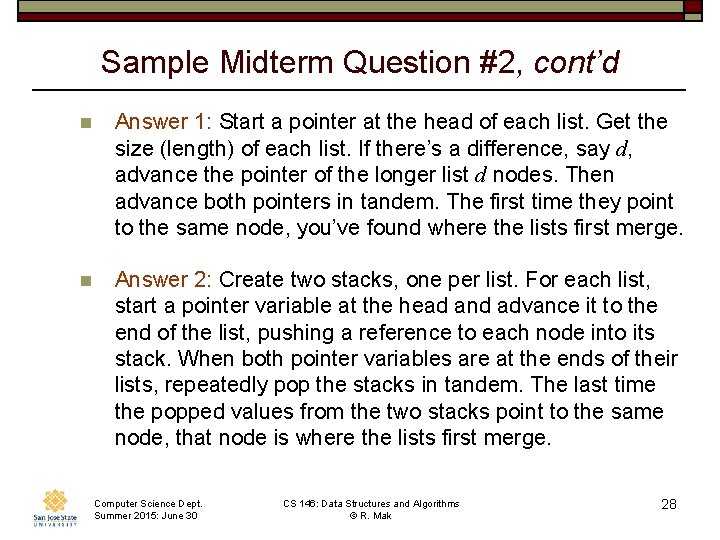
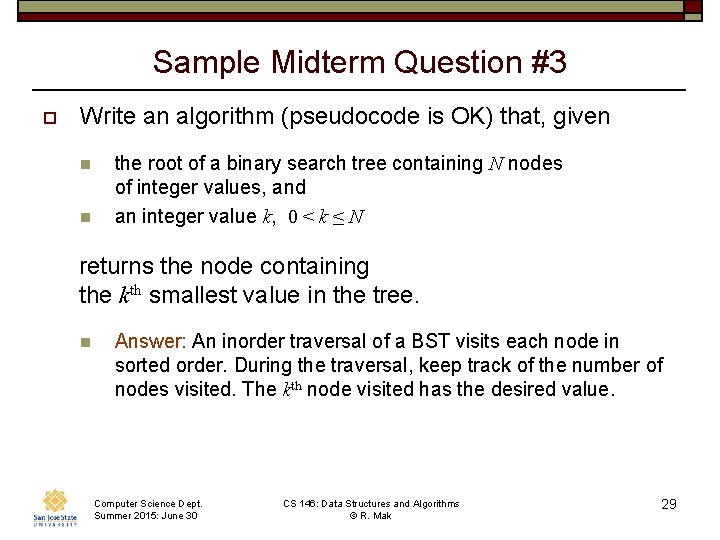
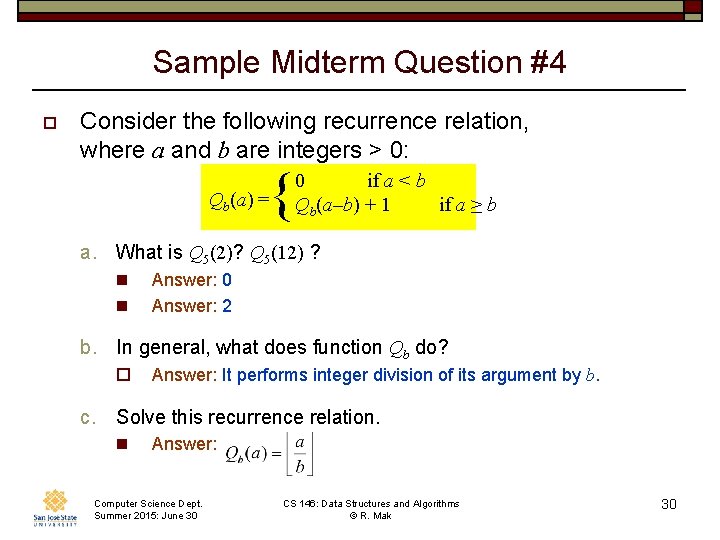
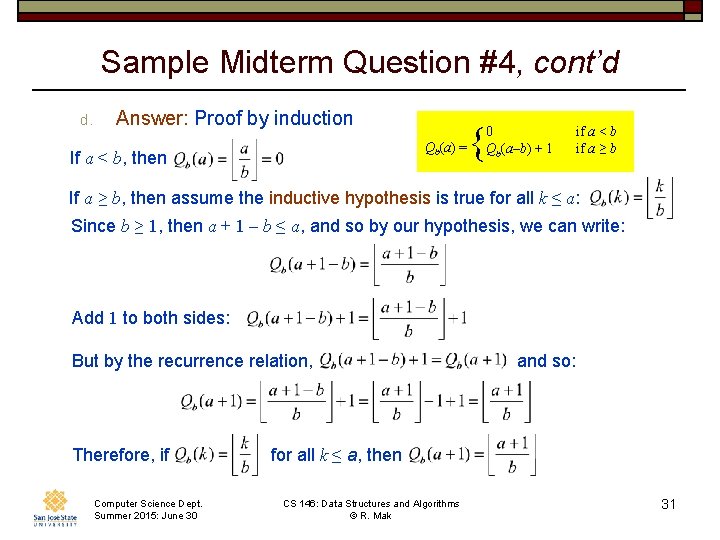
- Slides: 31
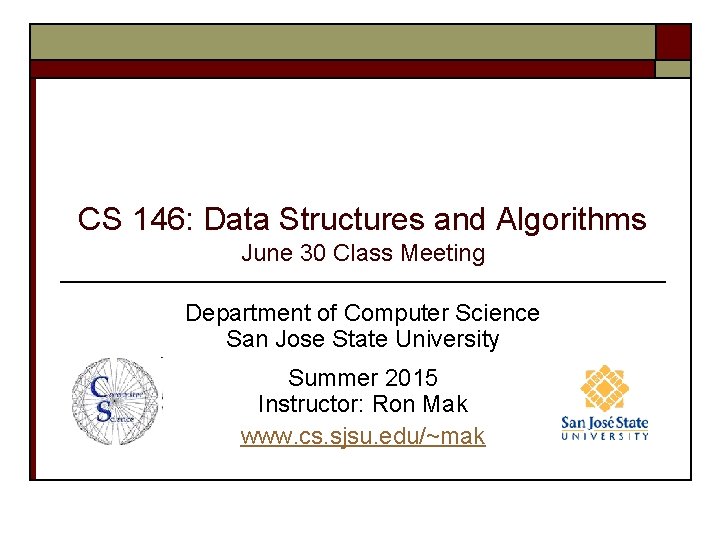
CS 146: Data Structures and Algorithms June 30 Class Meeting Department of Computer Science San Jose State University Summer 2015 Instructor: Ron Mak www. cs. sjsu. edu/~mak
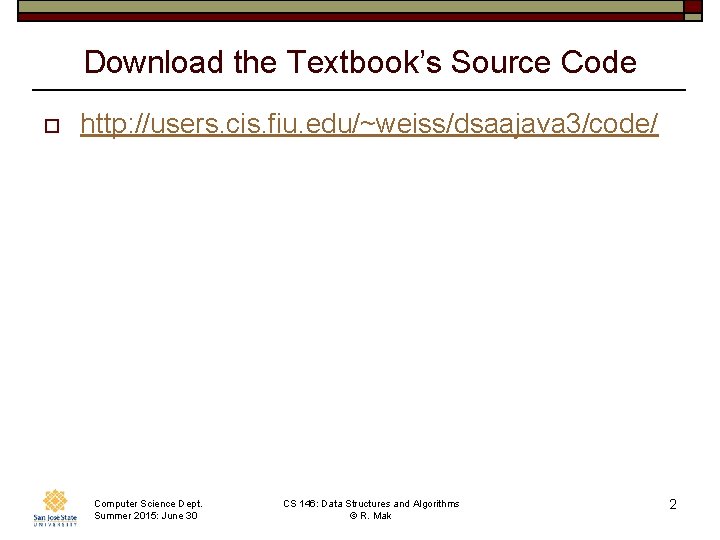
Download the Textbook’s Source Code o http: //users. cis. fiu. edu/~weiss/dsaajava 3/code/ Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 2
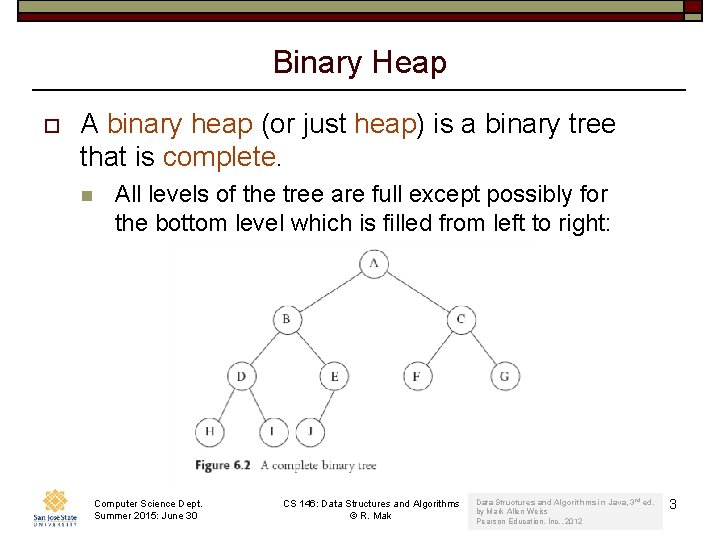
Binary Heap o A binary heap (or just heap) is a binary tree that is complete. n All levels of the tree are full except possibly for the bottom level which is filled from left to right: Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak Data Structures and Algorithms in Java, 3 rd ed. by Mark Allen Weiss Pearson Education, Inc. , 2012 3
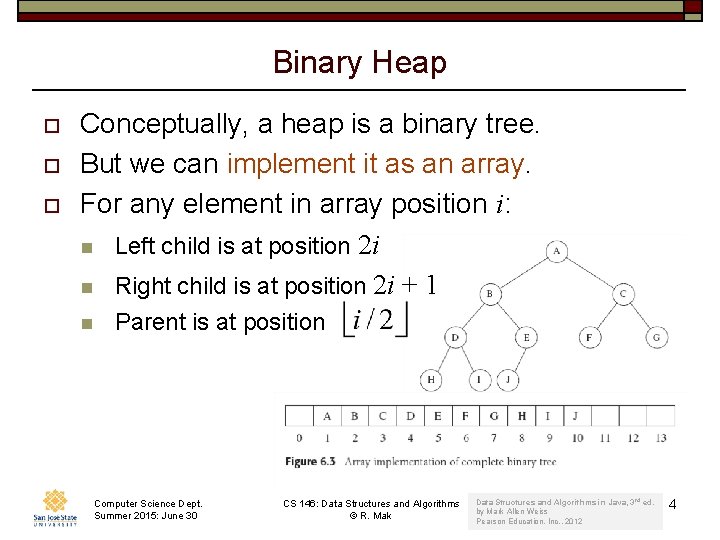
Binary Heap o o o Conceptually, a heap is a binary tree. But we can implement it as an array. For any element in array position i: n Left child is at position 2 i n Right child is at position 2 i + 1 n Parent is at position Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak Data Structures and Algorithms in Java, 3 rd ed. by Mark Allen Weiss Pearson Education, Inc. , 2012 4
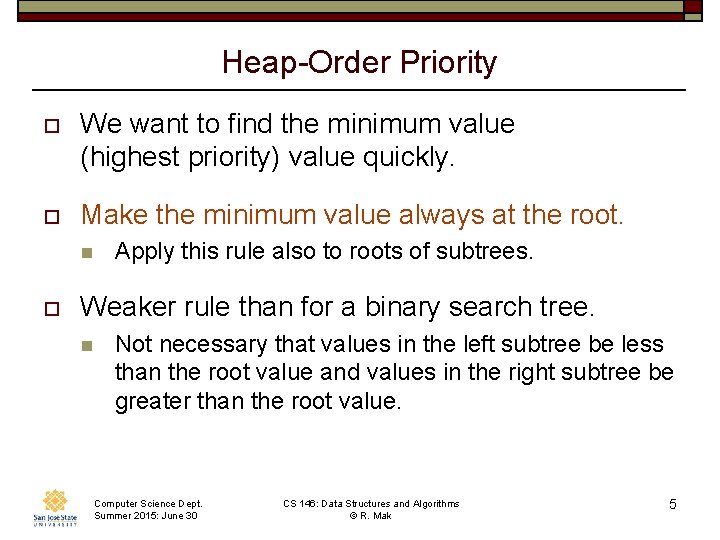
Heap-Order Priority o We want to find the minimum value (highest priority) value quickly. o Make the minimum value always at the root. n o Apply this rule also to roots of subtrees. Weaker rule than for a binary search tree. n Not necessary that values in the left subtree be less than the root value and values in the right subtree be greater than the root value. Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 5
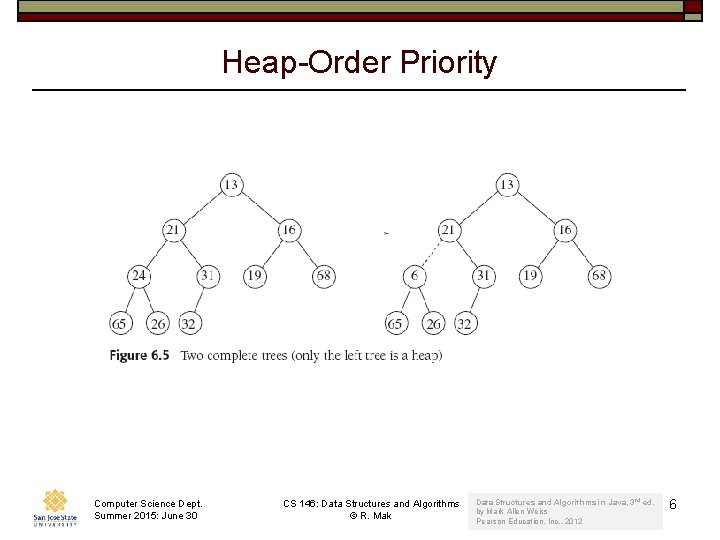
Heap-Order Priority Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak Data Structures and Algorithms in Java, 3 rd ed. by Mark Allen Weiss Pearson Education, Inc. , 2012 6
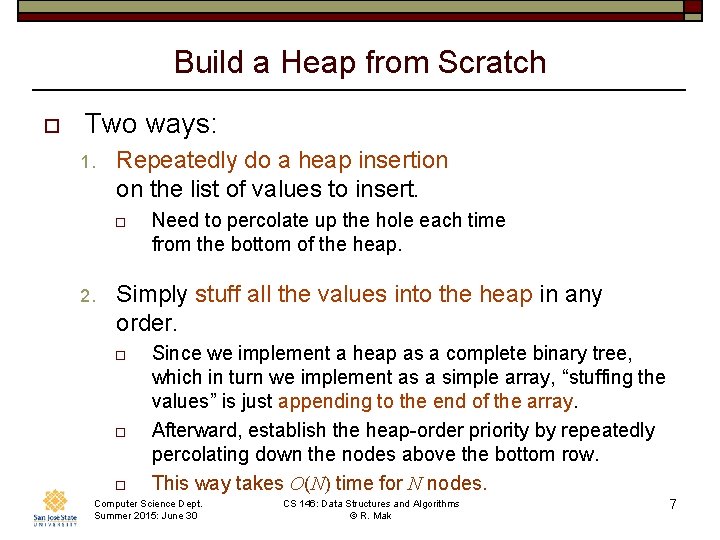
Build a Heap from Scratch o Two ways: 1. Repeatedly do a heap insertion on the list of values to insert. o 2. Need to percolate up the hole each time from the bottom of the heap. Simply stuff all the values into the heap in any order. o o o Since we implement a heap as a complete binary tree, which in turn we implement as a simple array, “stuffing the values” is just appending to the end of the array. Afterward, establish the heap-order priority by repeatedly percolating down the nodes above the bottom row. This way takes O(N) time for N nodes. Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 7
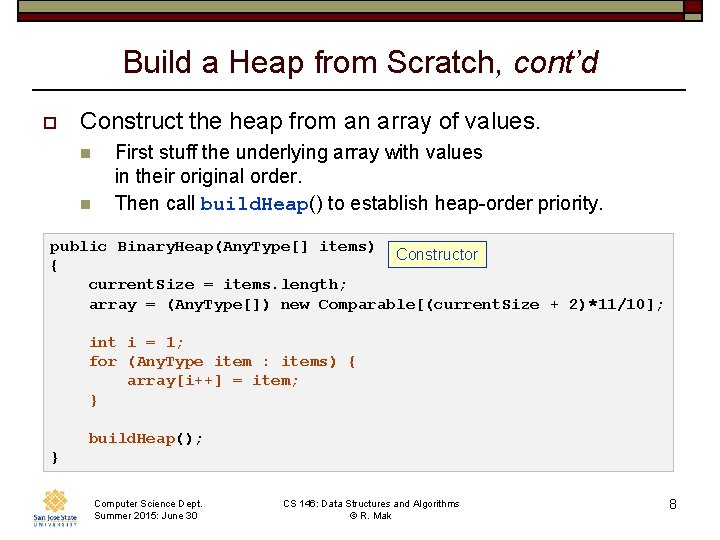
Build a Heap from Scratch, cont’d o Construct the heap from an array of values. n n First stuff the underlying array with values in their original order. Then call build. Heap() to establish heap-order priority. public Binary. Heap(Any. Type[] items) Constructor { current. Size = items. length; array = (Any. Type[]) new Comparable[(current. Size + 2)*11/10]; int i = 1; for (Any. Type item : items) { array[i++] = item; } build. Heap(); } Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 8
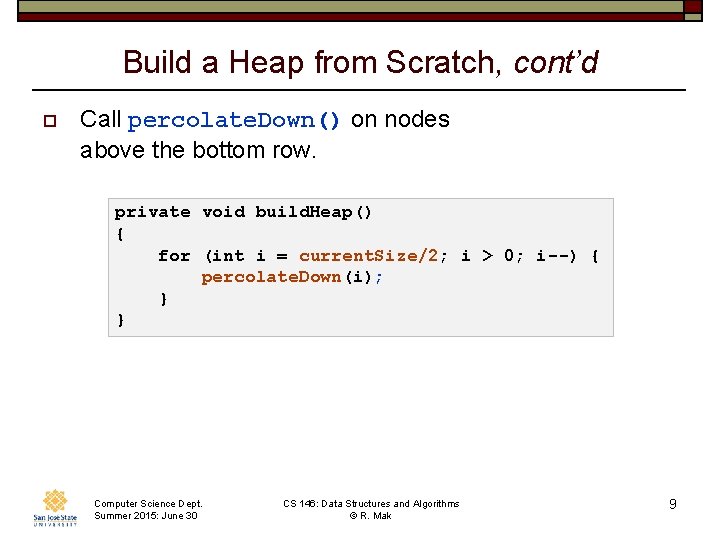
Build a Heap from Scratch, cont’d o Call percolate. Down() on nodes above the bottom row. private void build. Heap() { for (int i = current. Size/2; i > 0; i--) { percolate. Down(i); } } Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 9
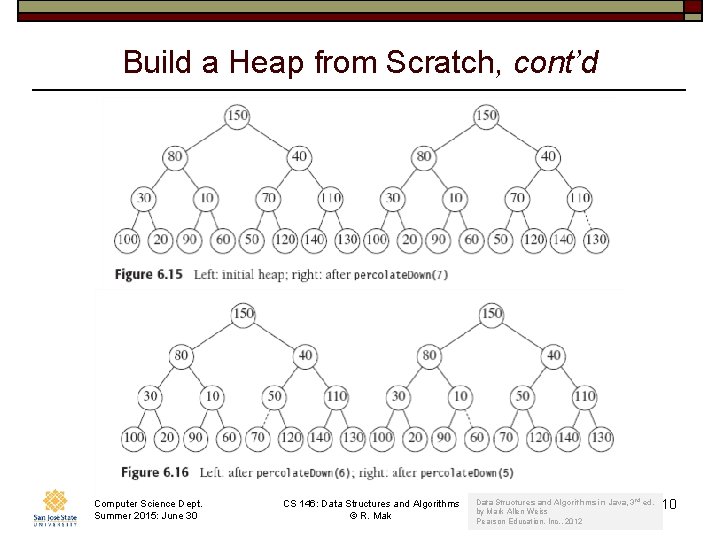
Build a Heap from Scratch, cont’d Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak Data Structures and Algorithms in Java, 3 rd ed. by Mark Allen Weiss Pearson Education, Inc. , 2012 10
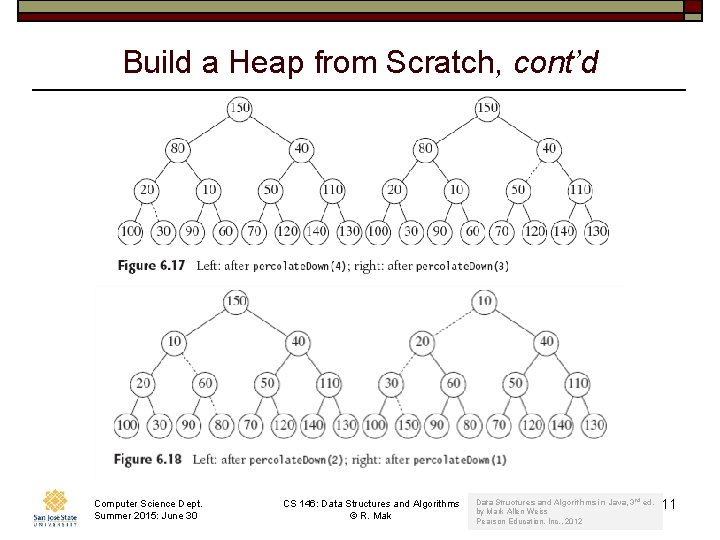
Build a Heap from Scratch, cont’d Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak Data Structures and Algorithms in Java, 3 rd ed. by Mark Allen Weiss Pearson Education, Inc. , 2012 11
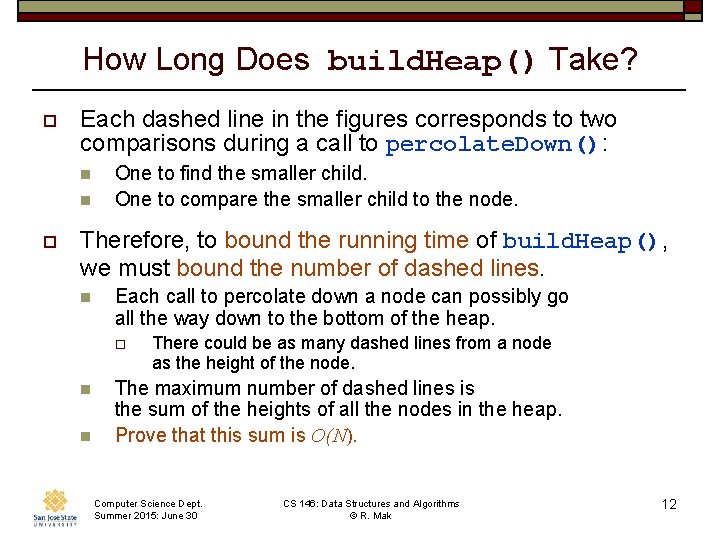
How Long Does build. Heap() Take? o Each dashed line in the figures corresponds to two comparisons during a call to percolate. Down(): n n o One to find the smaller child. One to compare the smaller child to the node. Therefore, to bound the running time of build. Heap(), we must bound the number of dashed lines. n Each call to percolate down a node can possibly go all the way down to the bottom of the heap. o n n There could be as many dashed lines from a node as the height of the node. The maximum number of dashed lines is the sum of the heights of all the nodes in the heap. Prove that this sum is O(N). Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 12
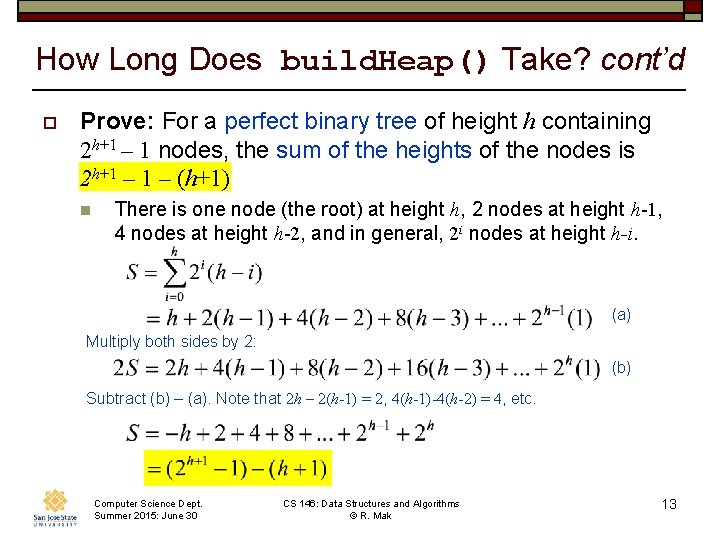
How Long Does build. Heap() Take? cont’d o Prove: For a perfect binary tree of height h containing 2 h+1 – 1 nodes, the sum of the heights of the nodes is 2 h+1 – (h+1) n There is one node (the root) at height h, 2 nodes at height h-1, 4 nodes at height h-2, and in general, 2 i nodes at height h-i. (a) Multiply both sides by 2: (b) Subtract (b) – (a). Note that 2 h – 2(h-1) = 2, 4(h-1)-4(h-2) = 4, etc. Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 13
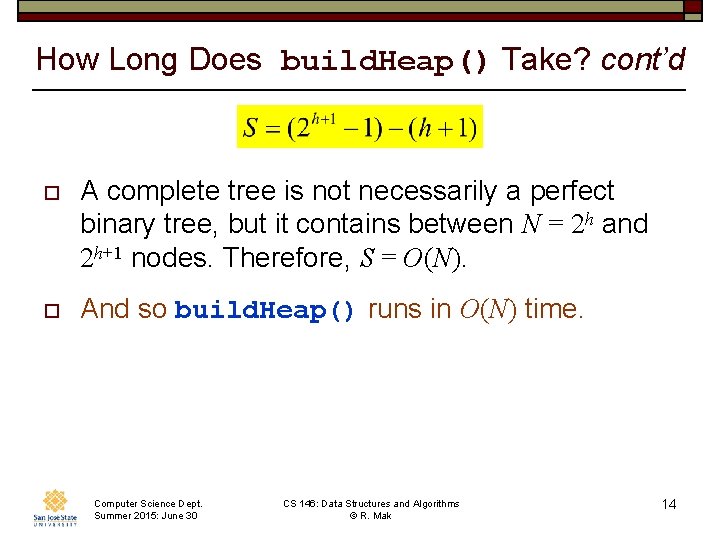
How Long Does build. Heap() Take? cont’d o A complete tree is not necessarily a perfect binary tree, but it contains between N = 2 h and 2 h+1 nodes. Therefore, S = O(N). o And so build. Heap() runs in O(N) time. Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 14
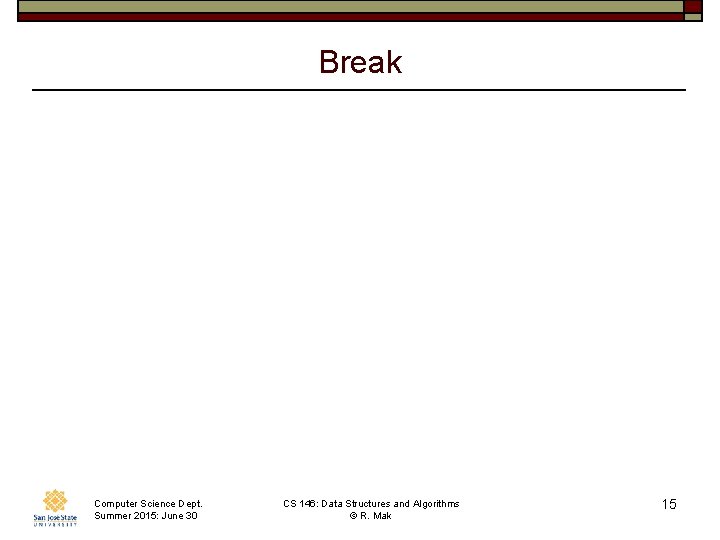
Break Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 15
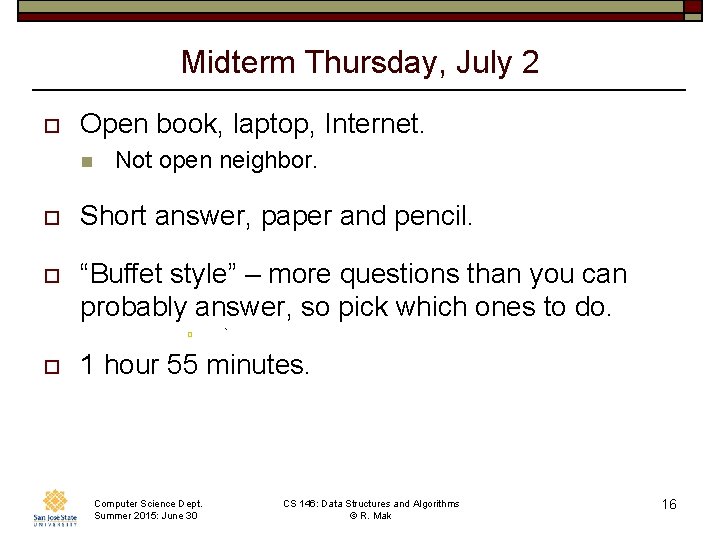
Midterm Thursday, July 2 o Open book, laptop, Internet. n Not open neighbor. o Short answer, paper and pencil. o “Buffet style” – more questions than you can probably answer, so pick which ones to do. o o ` 1 hour 55 minutes. Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 16
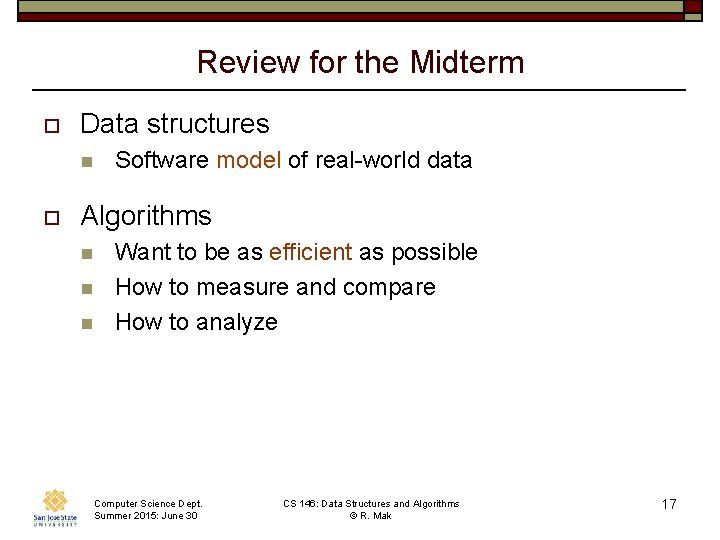
Review for the Midterm o Data structures n o Software model of real-world data Algorithms n n n Want to be as efficient as possible How to measure and compare How to analyze Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 17
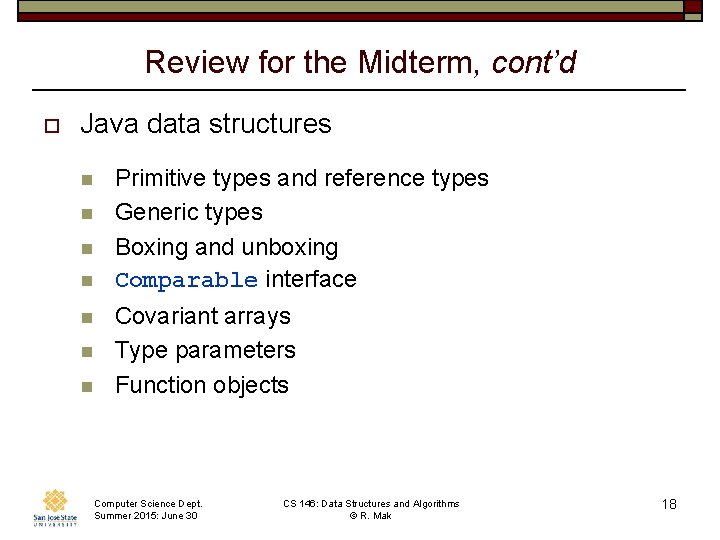
Review for the Midterm, cont’d o Java data structures n n n n Primitive types and reference types Generic types Boxing and unboxing Comparable interface Covariant arrays Type parameters Function objects Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 18
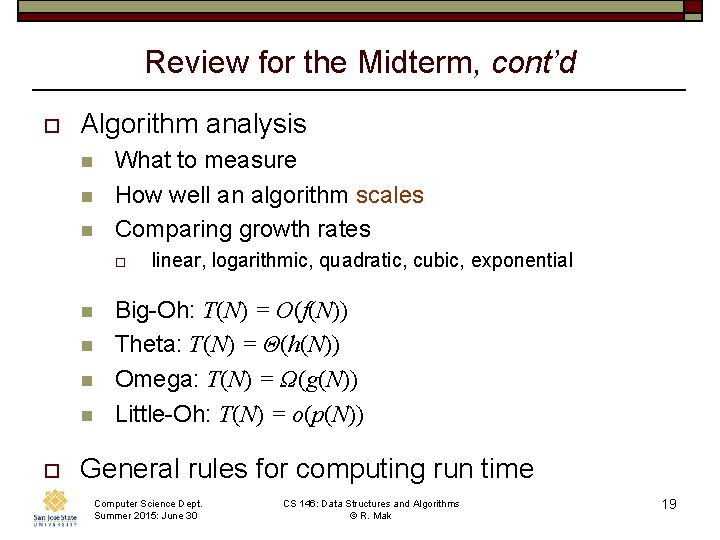
Review for the Midterm, cont’d o Algorithm analysis n n n What to measure How well an algorithm scales Comparing growth rates o n n o linear, logarithmic, quadratic, cubic, exponential Big-Oh: T(N) = O(f(N)) Theta: T(N) = Θ(h(N)) Omega: T(N) = Ω(g(N)) Little-Oh: T(N) = o(p(N)) General rules for computing run time Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 19
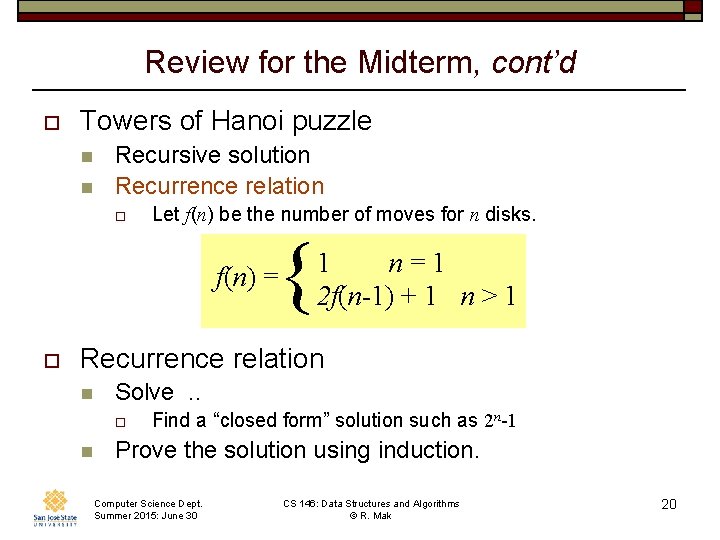
Review for the Midterm, cont’d o Towers of Hanoi puzzle n n Recursive solution Recurrence relation o Let f(n) be the number of moves for n disks. { f(n) = o 1 n=1 2 f(n-1) + 1 n > 1 Recurrence relation n Solve. . o n Find a “closed form” solution such as 2 n-1 Prove the solution using induction. Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 20
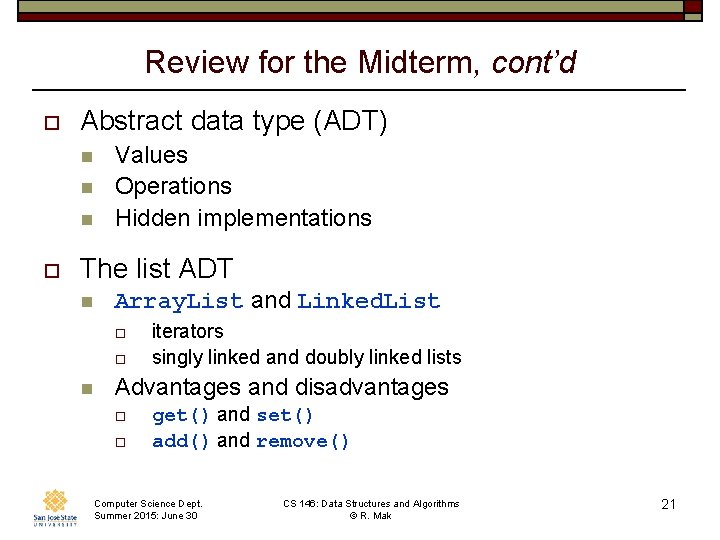
Review for the Midterm, cont’d o Abstract data type (ADT) n n n o Values Operations Hidden implementations The list ADT n Array. List and Linked. List o o n iterators singly linked and doubly linked lists Advantages and disadvantages o o get() and set() add() and remove() Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 21
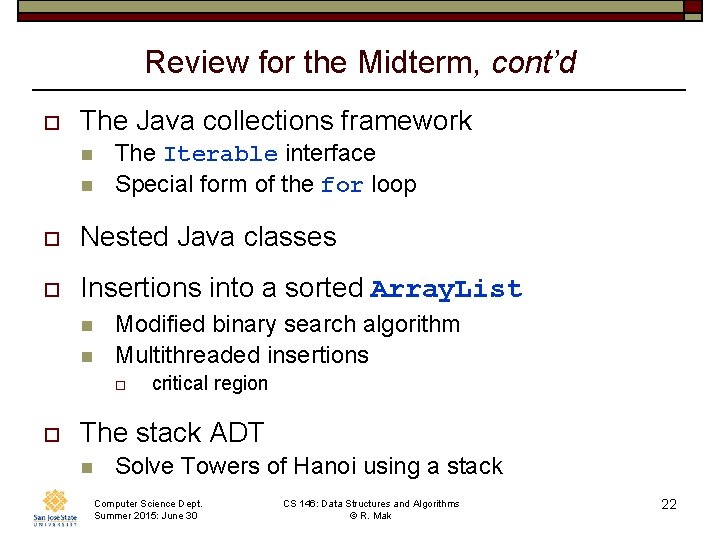
Review for the Midterm, cont’d o The Java collections framework n n The Iterable interface Special form of the for loop o Nested Java classes o Insertions into a sorted Array. List n n Modified binary search algorithm Multithreaded insertions o o critical region The stack ADT n Solve Towers of Hanoi using a stack Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 22
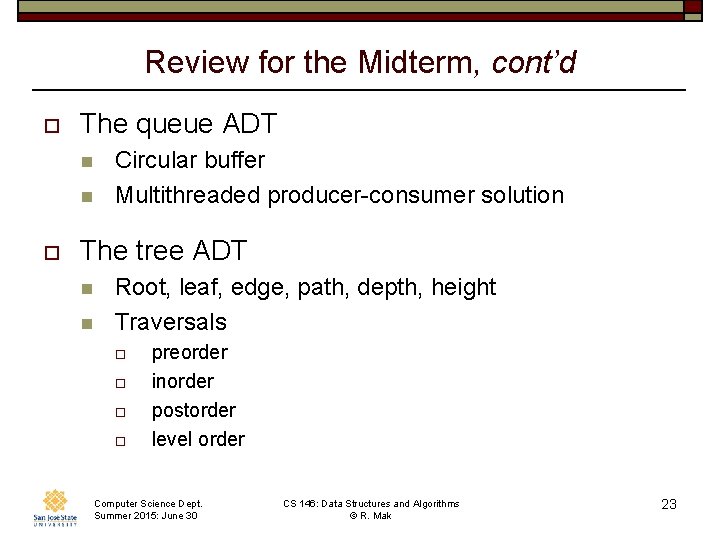
Review for the Midterm, cont’d o The queue ADT n n o Circular buffer Multithreaded producer-consumer solution The tree ADT n n Root, leaf, edge, path, depth, height Traversals o o preorder inorder postorder level order Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 23
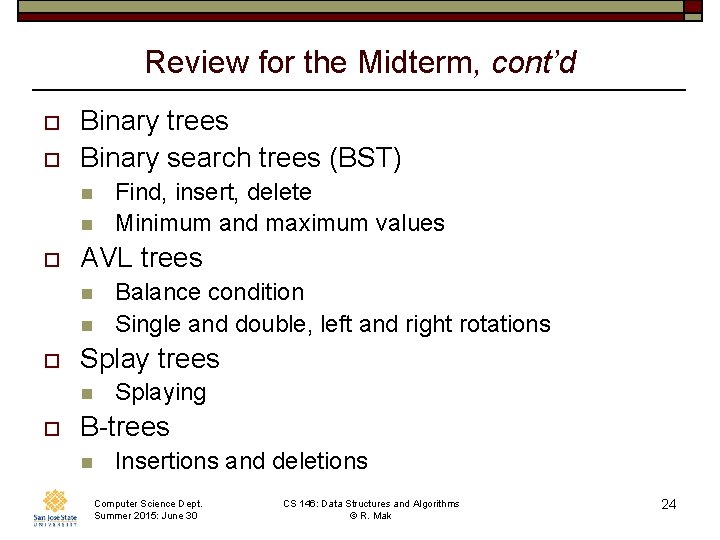
Review for the Midterm, cont’d o o Binary trees Binary search trees (BST) n n o AVL trees n n o Balance condition Single and double, left and right rotations Splay trees n o Find, insert, delete Minimum and maximum values Splaying B-trees n Insertions and deletions Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 24
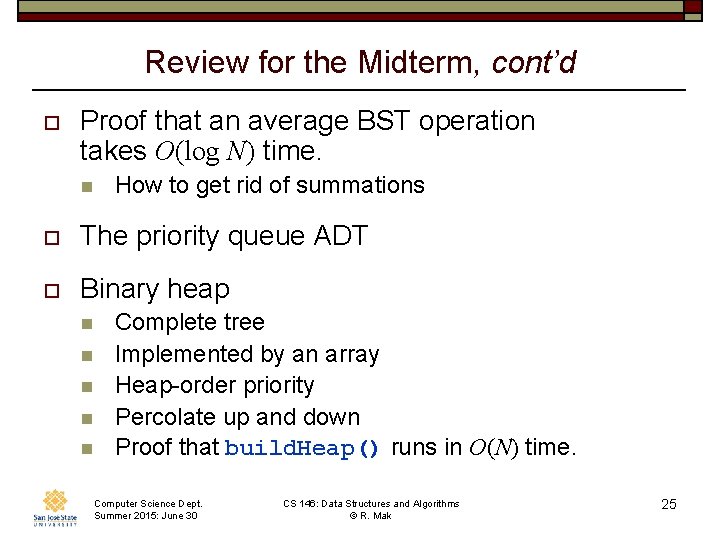
Review for the Midterm, cont’d o Proof that an average BST operation takes O(log N) time. n How to get rid of summations o The priority queue ADT o Binary heap n n n Complete tree Implemented by an array Heap-order priority Percolate up and down Proof that build. Heap() runs in O(N) time. Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 25
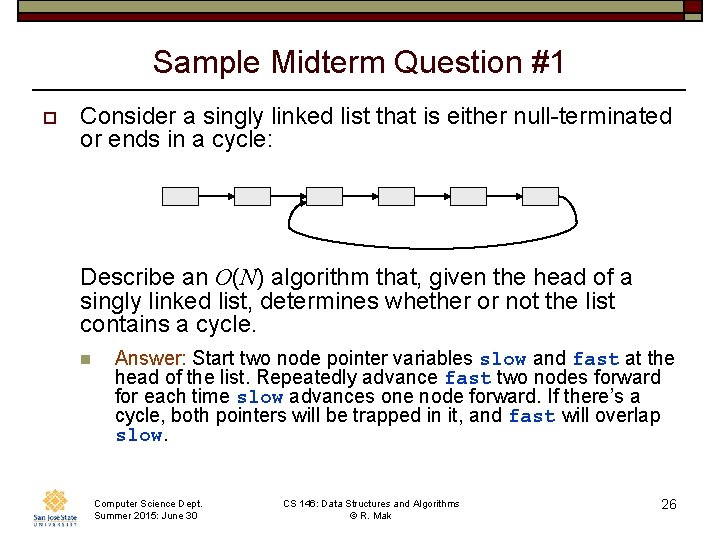
Sample Midterm Question #1 o Consider a singly linked list that is either null-terminated or ends in a cycle: Describe an O(N) algorithm that, given the head of a singly linked list, determines whether or not the list contains a cycle. n Answer: Start two node pointer variables slow and fast at the head of the list. Repeatedly advance fast two nodes forward for each time slow advances one node forward. If there’s a cycle, both pointers will be trapped in it, and fast will overlap slow. Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 26
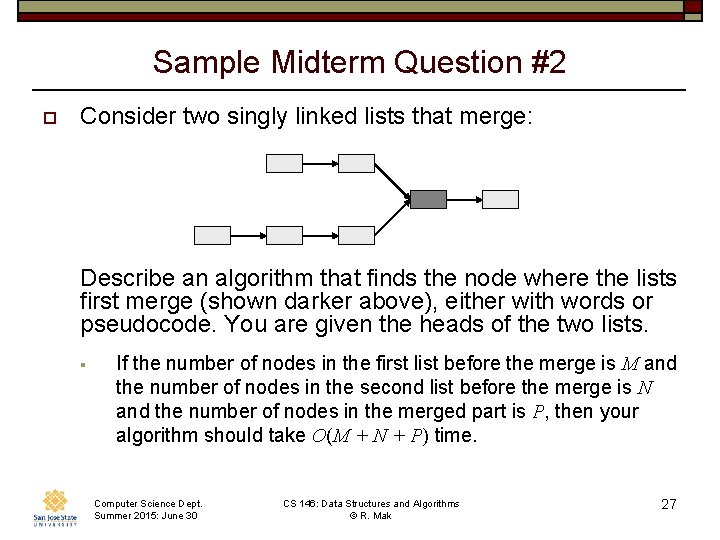
Sample Midterm Question #2 o Consider two singly linked lists that merge: Describe an algorithm that finds the node where the lists first merge (shown darker above), either with words or pseudocode. You are given the heads of the two lists. § If the number of nodes in the first list before the merge is M and the number of nodes in the second list before the merge is N and the number of nodes in the merged part is P, then your algorithm should take O(M + N + P) time. Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 27
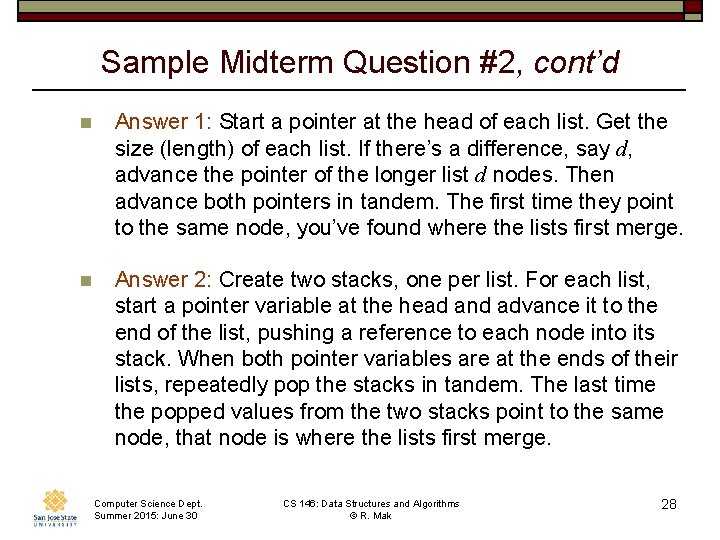
Sample Midterm Question #2, cont’d n Answer 1: Start a pointer at the head of each list. Get the size (length) of each list. If there’s a difference, say d, advance the pointer of the longer list d nodes. Then advance both pointers in tandem. The first time they point to the same node, you’ve found where the lists first merge. n Answer 2: Create two stacks, one per list. For each list, start a pointer variable at the head and advance it to the end of the list, pushing a reference to each node into its stack. When both pointer variables are at the ends of their lists, repeatedly pop the stacks in tandem. The last time the popped values from the two stacks point to the same node, that node is where the lists first merge. Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 28
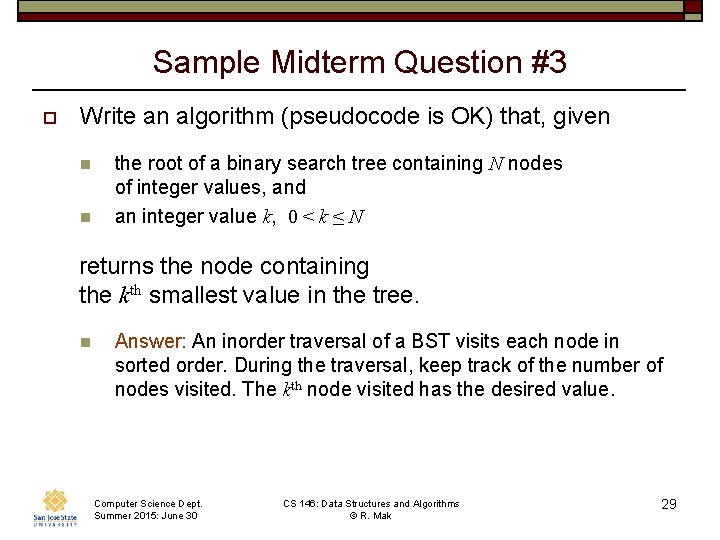
Sample Midterm Question #3 o Write an algorithm (pseudocode is OK) that, given n n the root of a binary search tree containing N nodes of integer values, and an integer value k, 0 < k ≤ N returns the node containing the kth smallest value in the tree. n Answer: An inorder traversal of a BST visits each node in sorted order. During the traversal, keep track of the number of nodes visited. The kth node visited has the desired value. Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 29
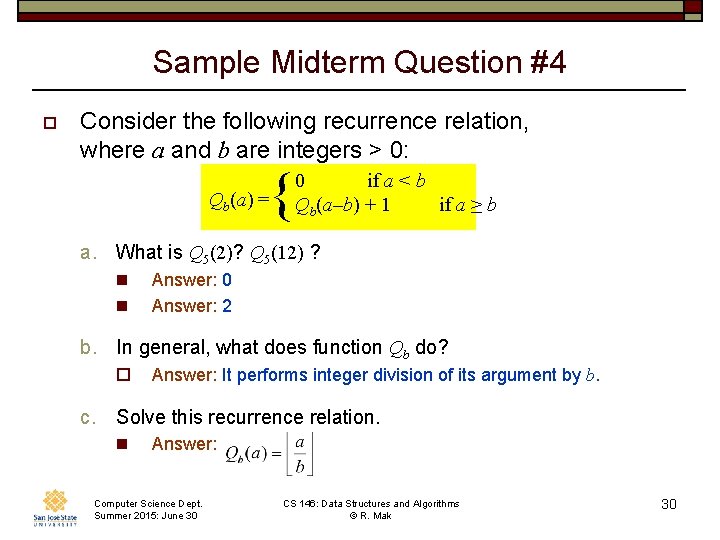
Sample Midterm Question #4 o Consider the following recurrence relation, where a and b are integers > 0: { Qb(a) = 0 if a < b Qb(a–b) + 1 if a ≥ b a. What is Q 5(2)? Q 5(12) ? n n Answer: 0 Answer: 2 b. In general, what does function Qb do? o Answer: It performs integer division of its argument by b. c. Solve this recurrence relation. n Answer: Computer Science Dept. Summer 2015: June 30 CS 146: Data Structures and Algorithms © R. Mak 30
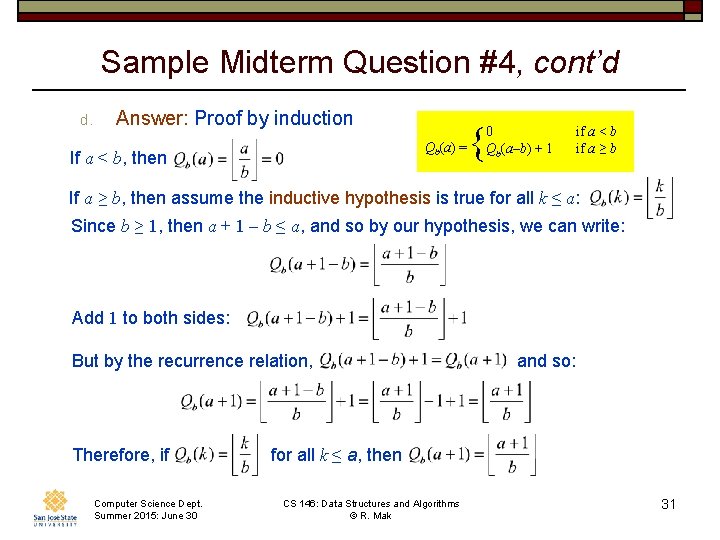
Sample Midterm Question #4, cont’d d. Answer: Proof by induction Qb(a) = If a < b, then { 0 Qb(a–b) + 1 if a < b if a ≥ b If a ≥ b, then assume the inductive hypothesis is true for all k ≤ a: Since b ≥ 1, then a + 1 – b ≤ a, and so by our hypothesis, we can write: Add 1 to both sides: But by the recurrence relation, Therefore, if Computer Science Dept. Summer 2015: June 30 and so: for all k ≤ a, then CS 146: Data Structures and Algorithms © R. Mak 31
Data structures and algorithms iit bombay
Cos423
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Ajit diwan
Data structures and algorithms
Data structures and algorithms
Ian munro waterloo
Signature file structure in information retrieval system
Data structures and algorithms
Algorithms + data structures = programs
Examples of homologous
Salmo 146
Pkj bawa persembahanmu
Afi 48-146
Contoh rpph model webbed paud
Sjsu cs 146
Osha 1910 confined space
1910 146
Confined space 1910
1910-146
A 63 kg astronaut is on a spacewalk
1910-146
264 - 146
Siapakah dia
Cs 146
Route 146 drive in
Lagu mazmur 146
Uss kawishiwi
146/79 blood pressure
Hino 146 letra