CS 1301 Review Keith OHara keith oharagatech edu
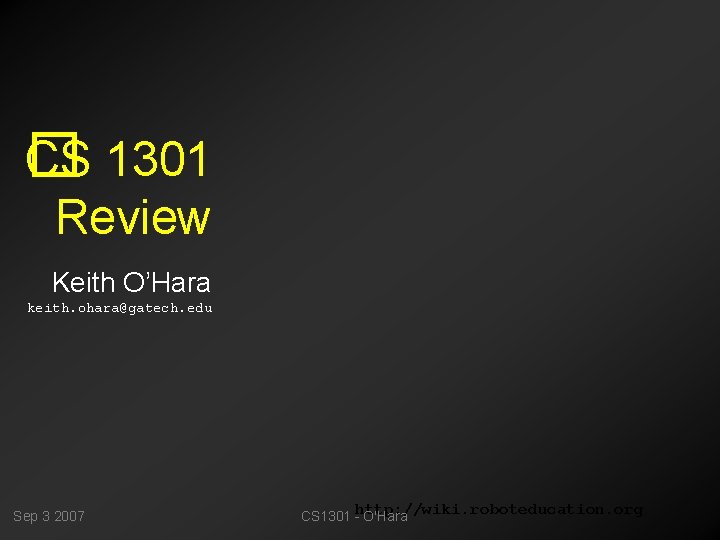
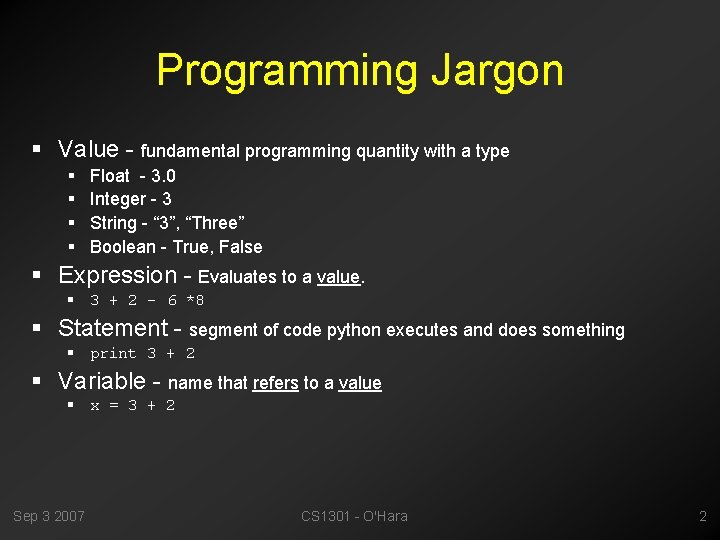
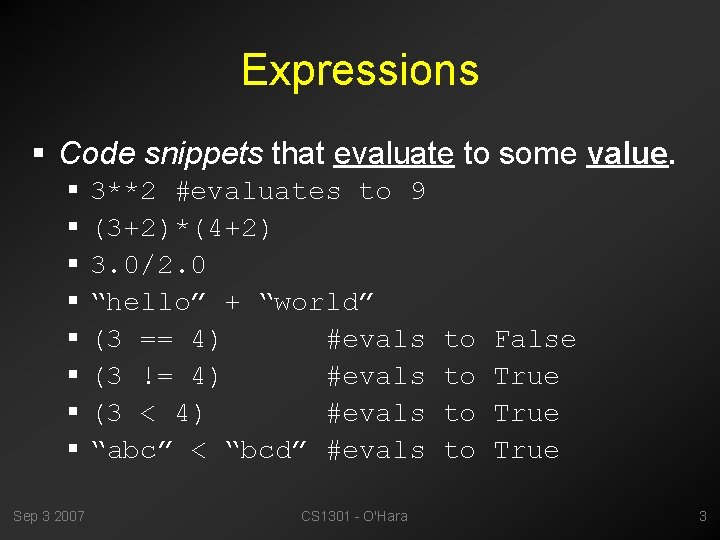
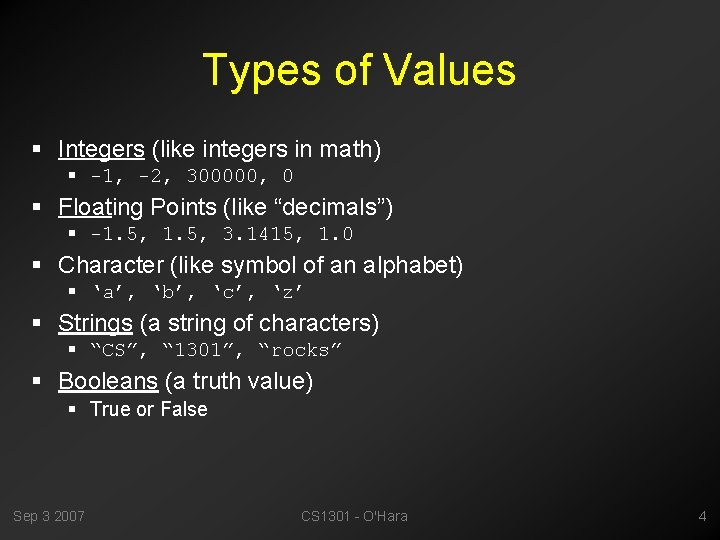
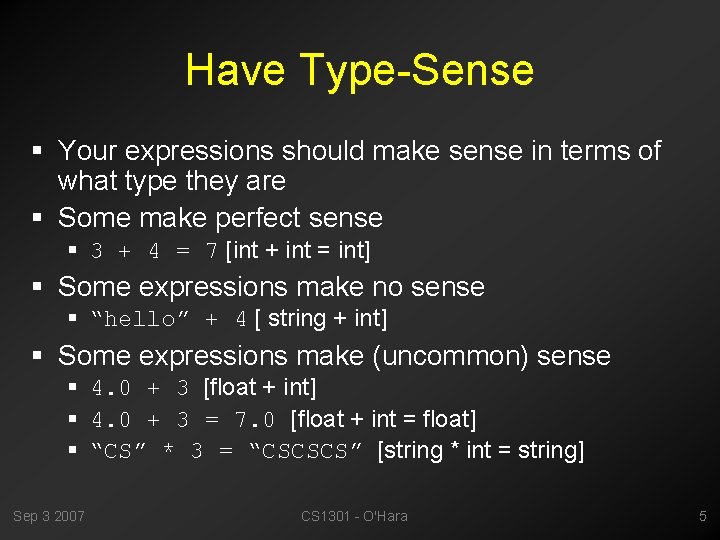
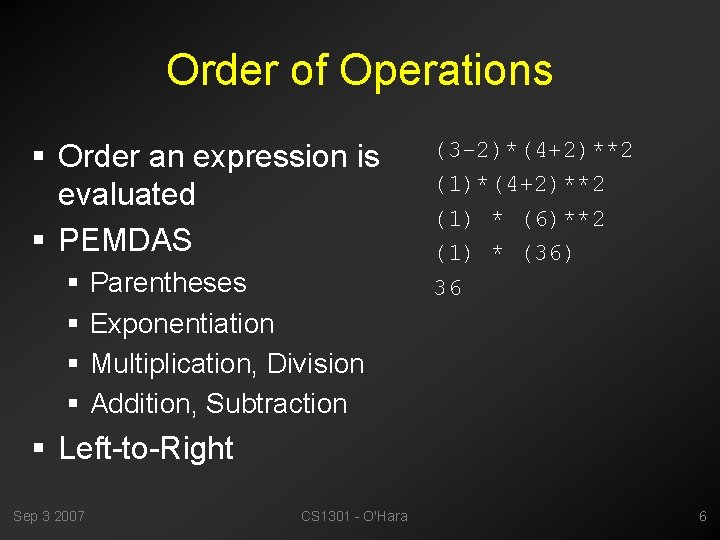
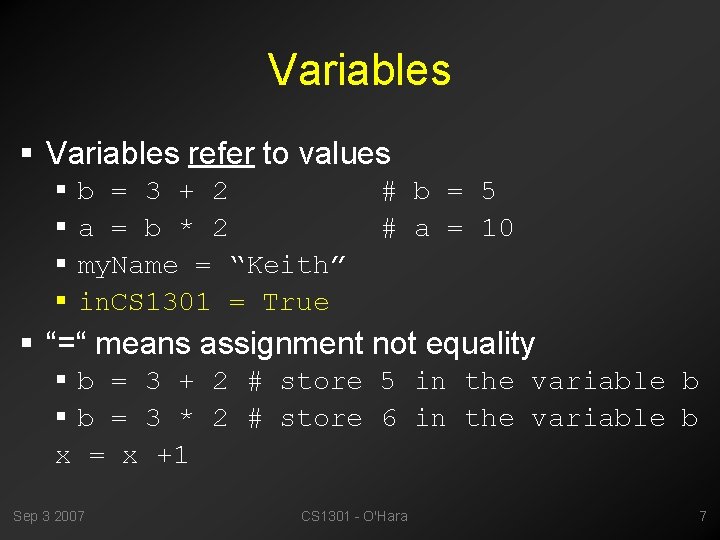
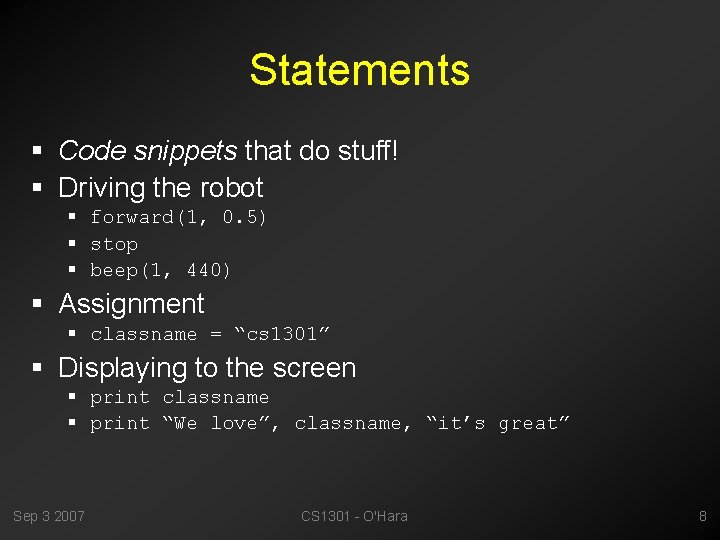
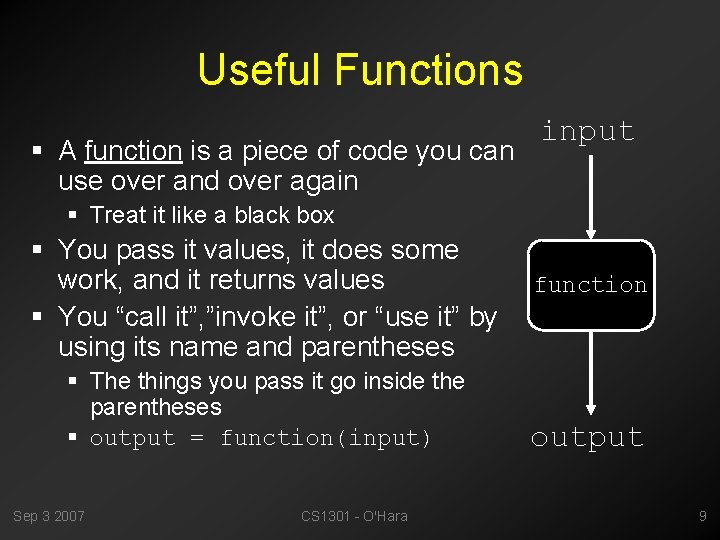
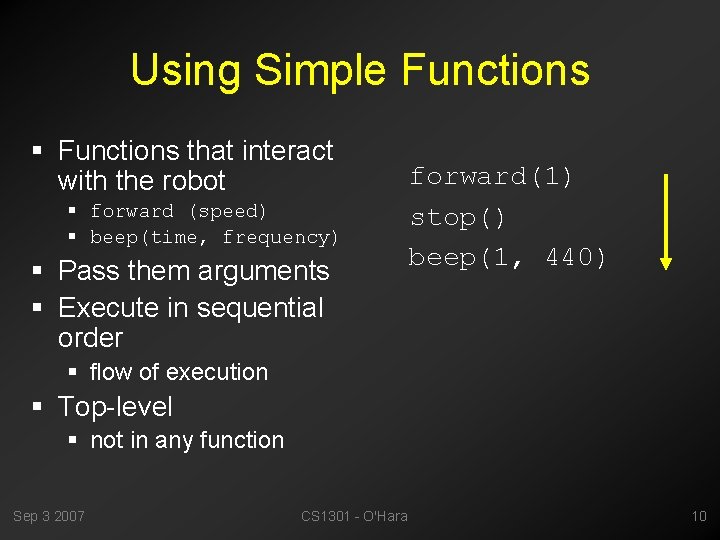
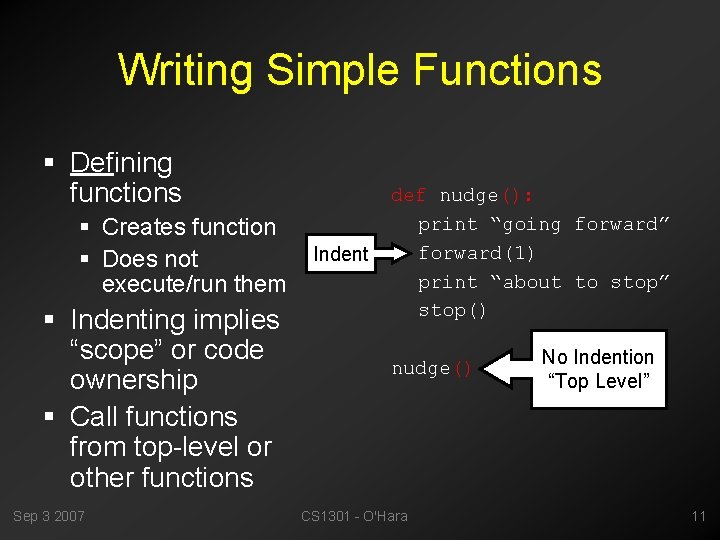
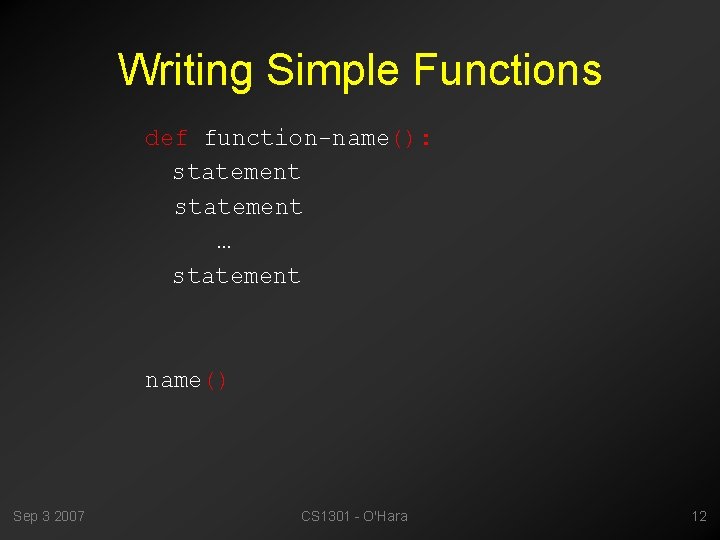
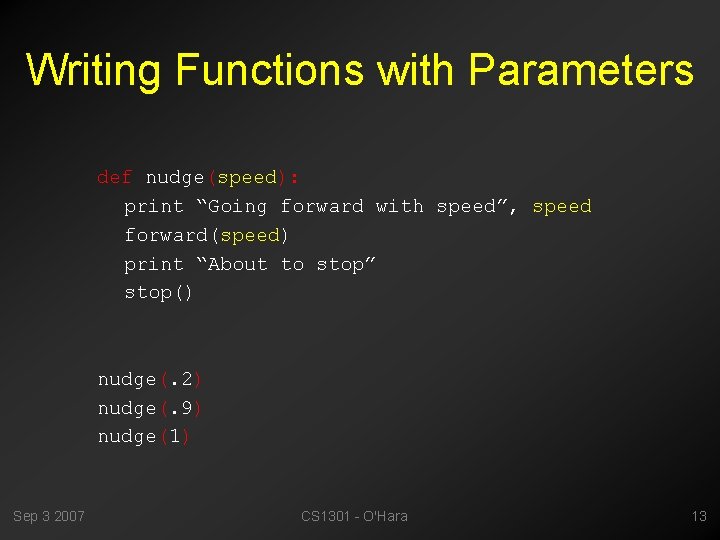
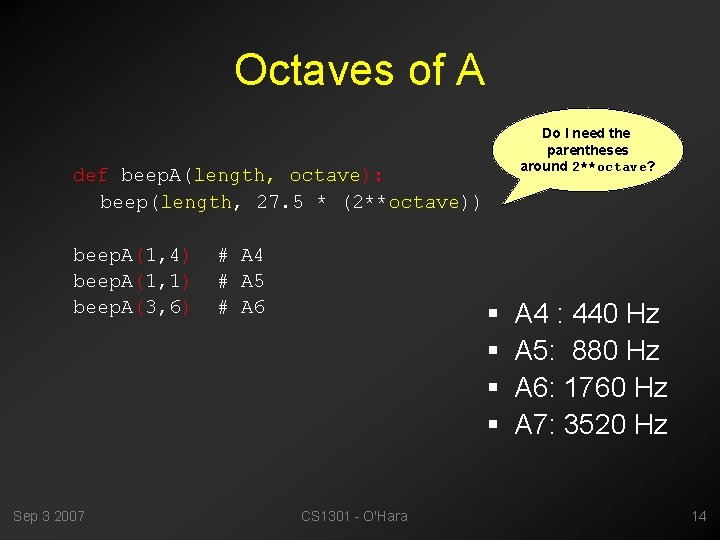
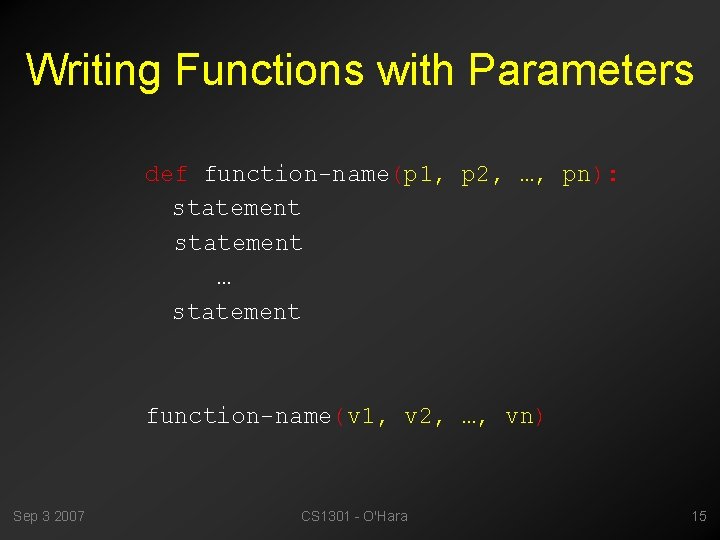
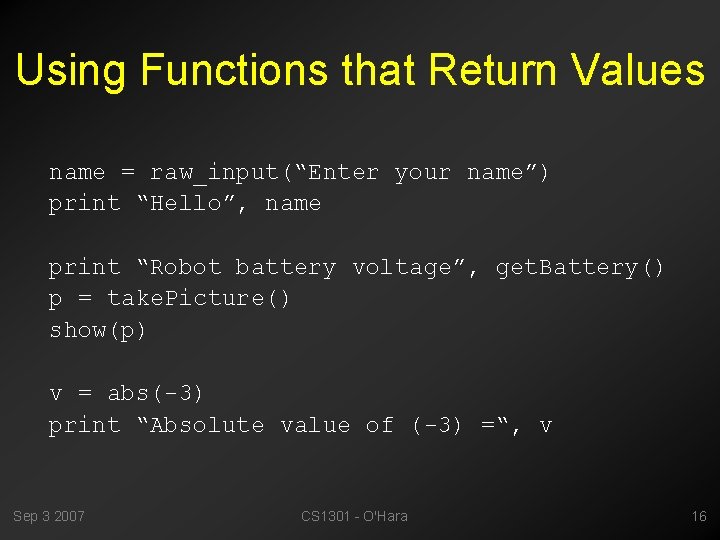
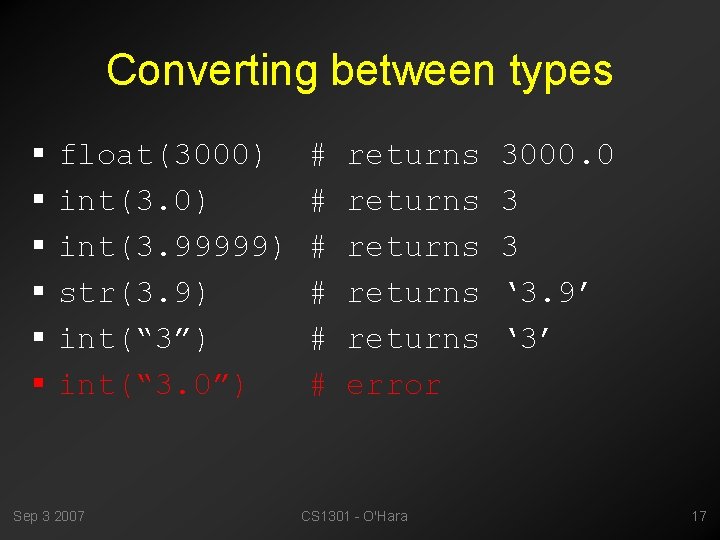
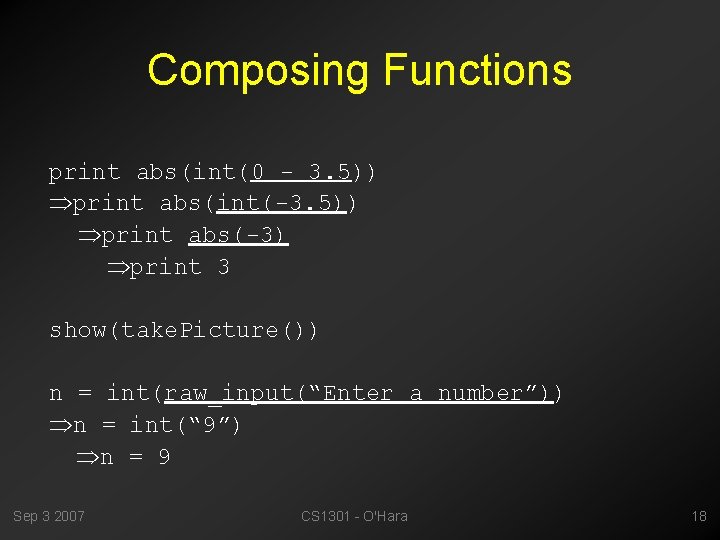
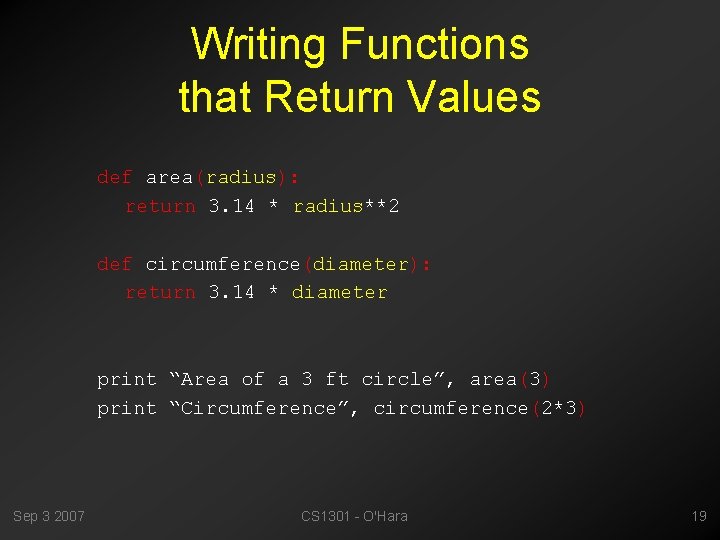
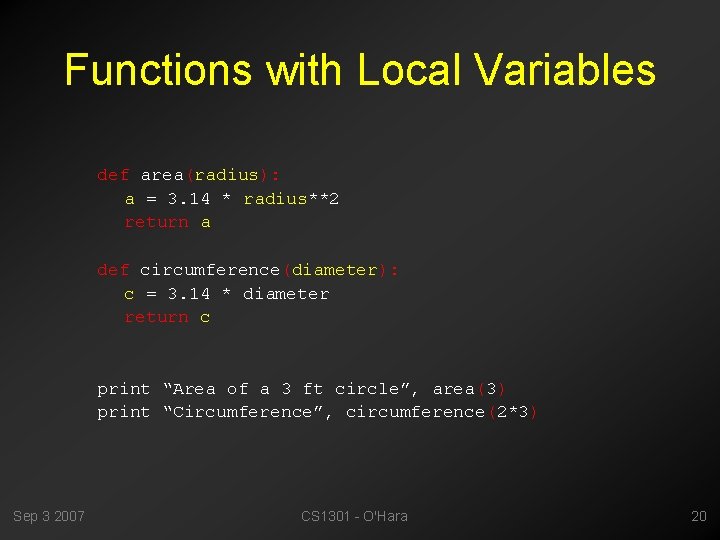
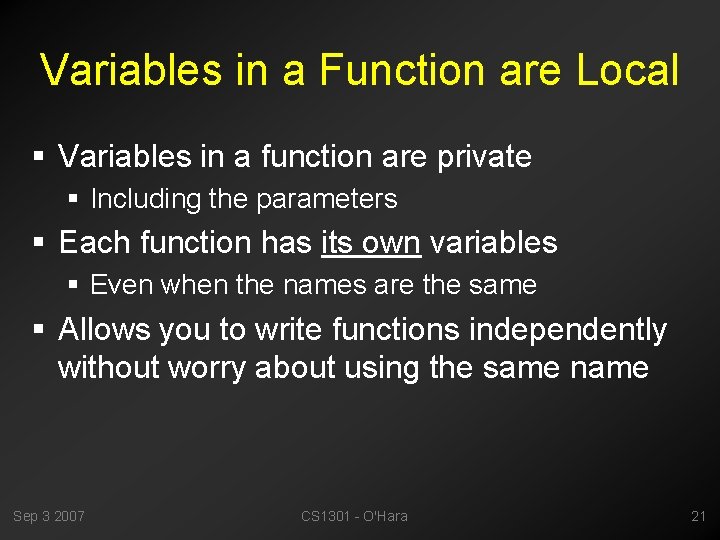
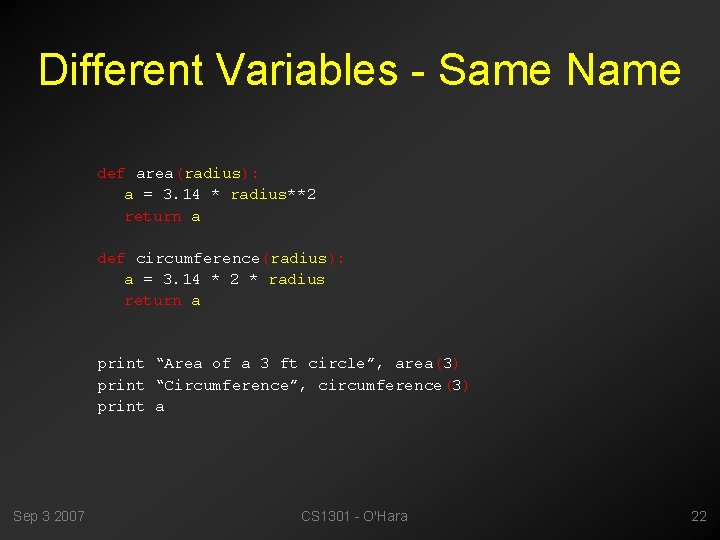
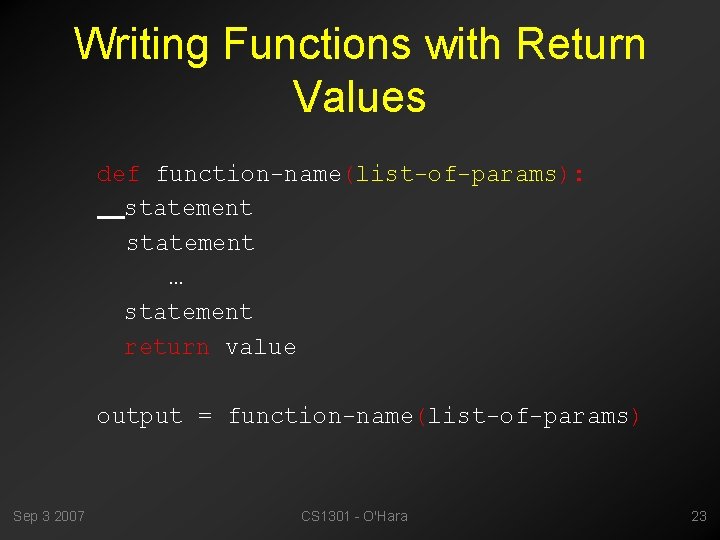
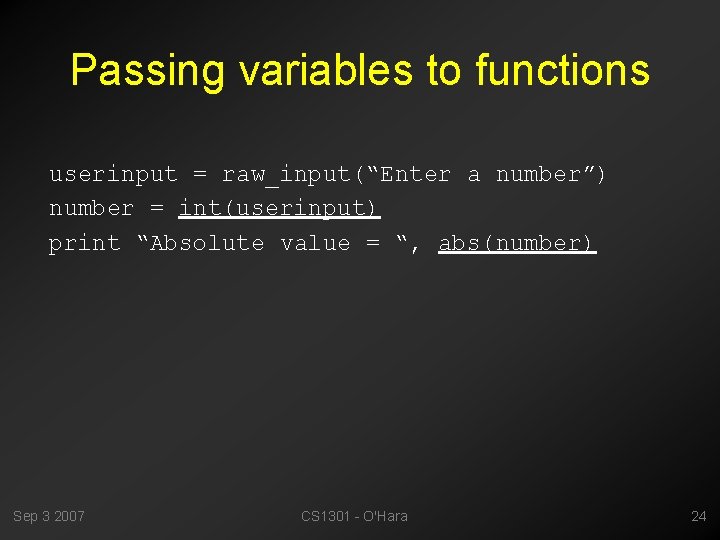
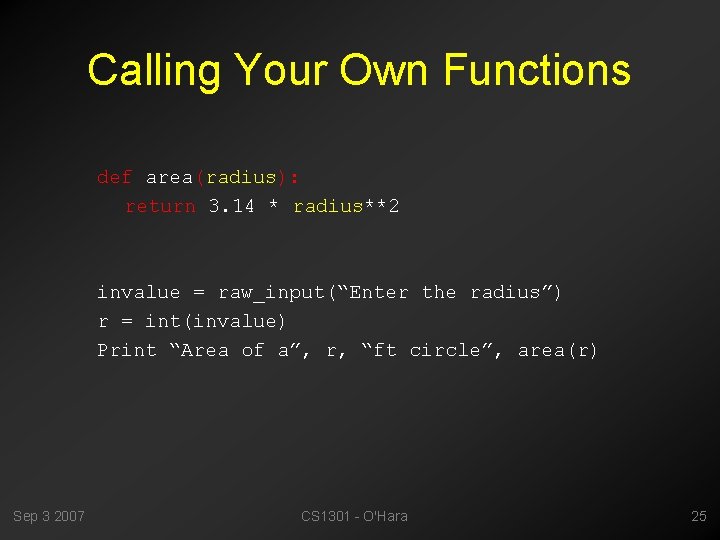
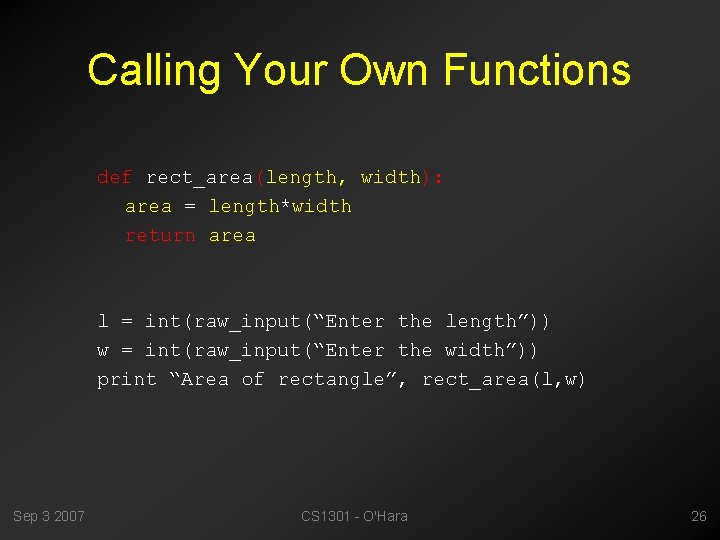
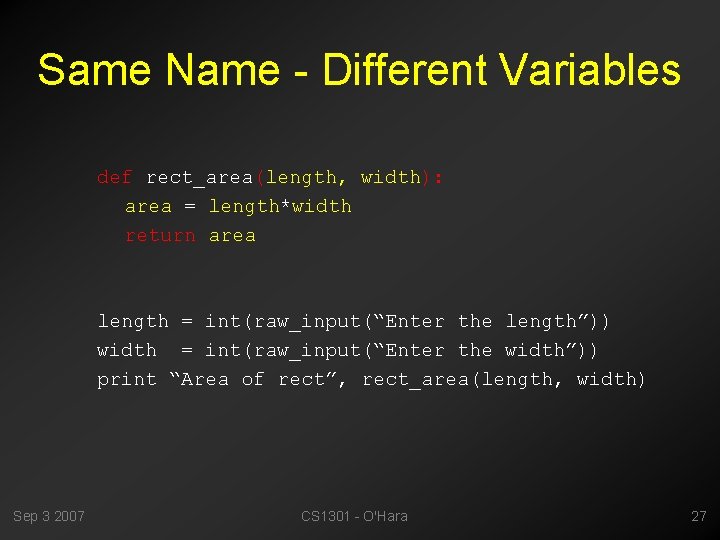
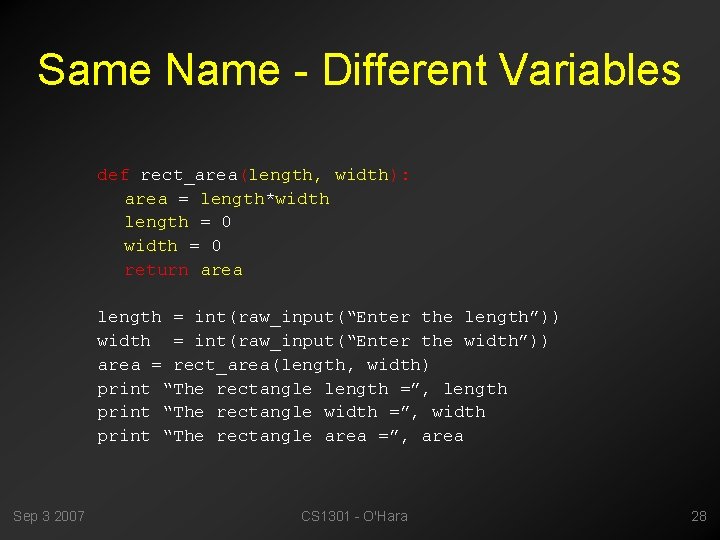
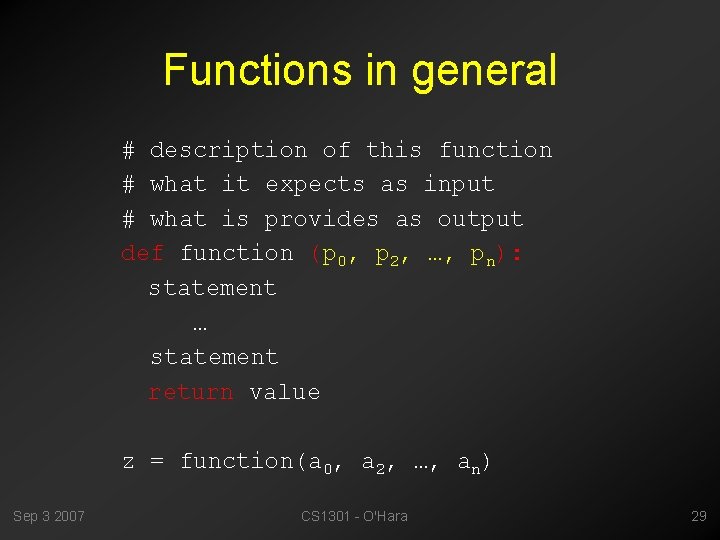
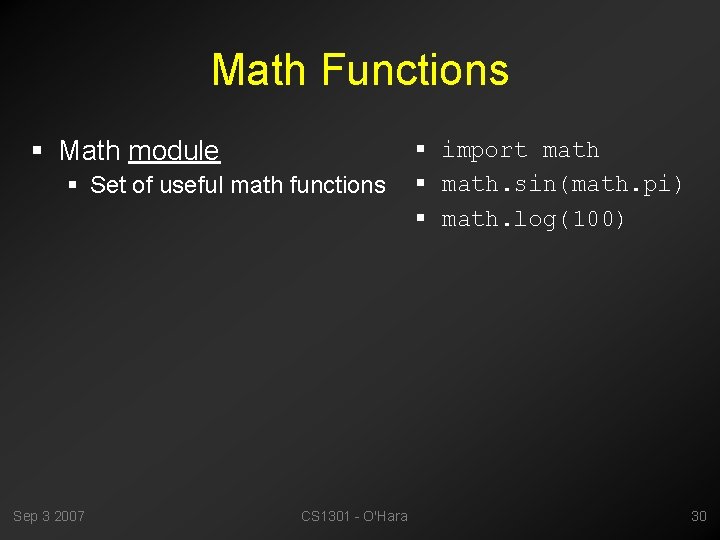
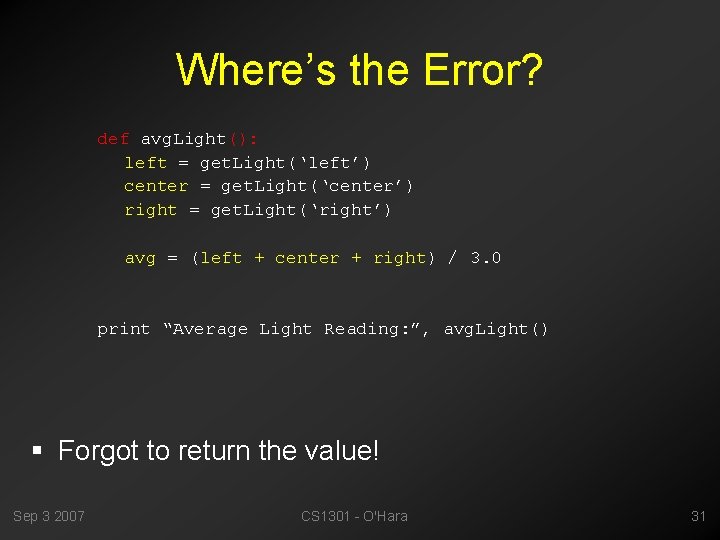
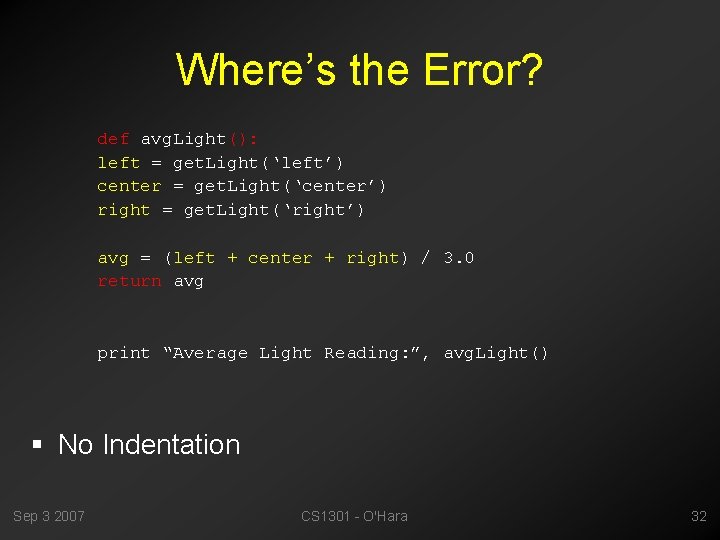
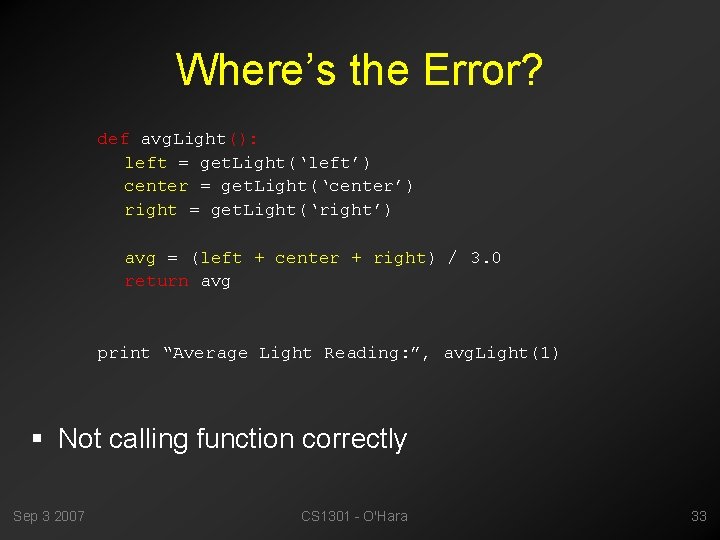
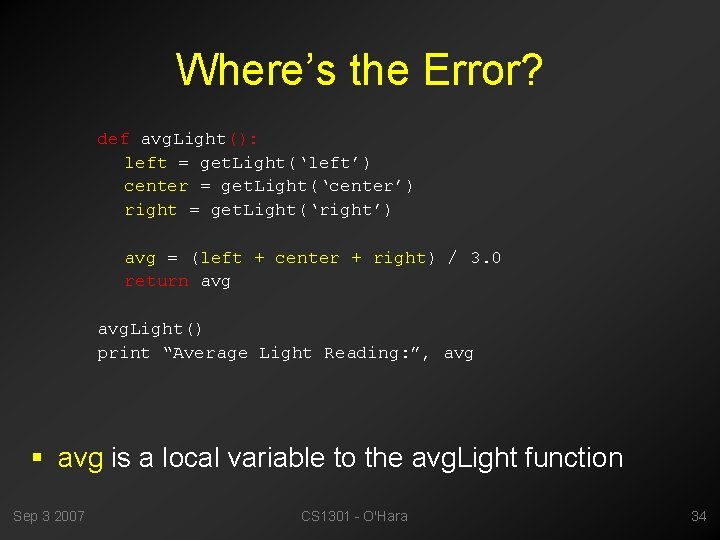
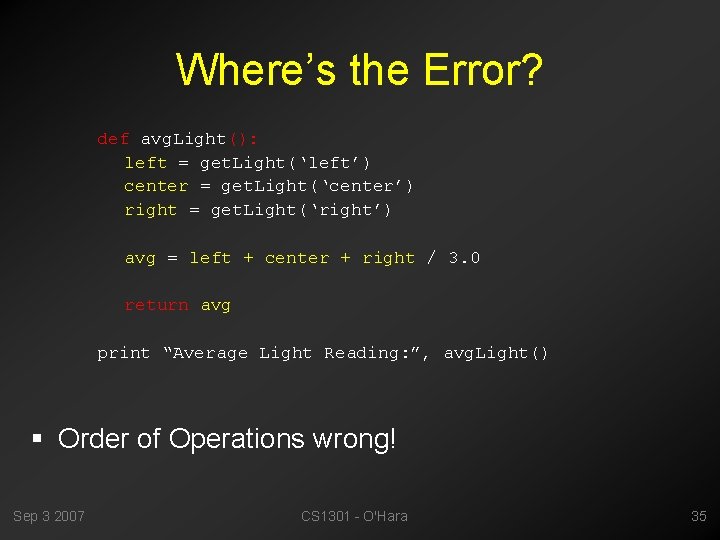
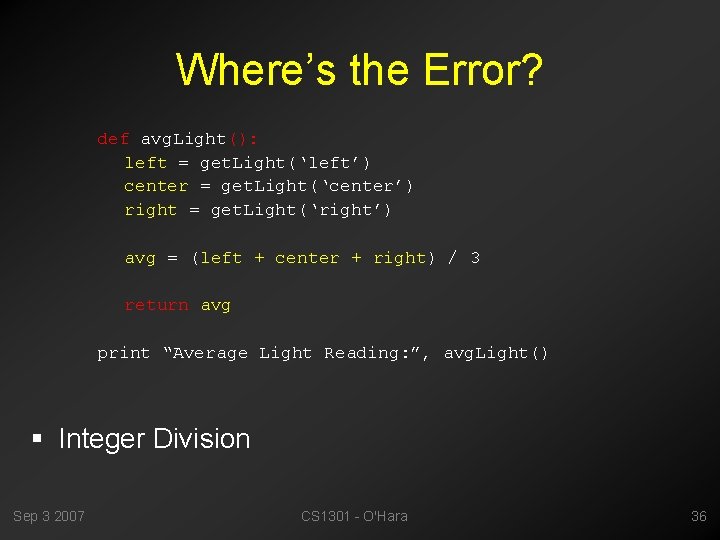
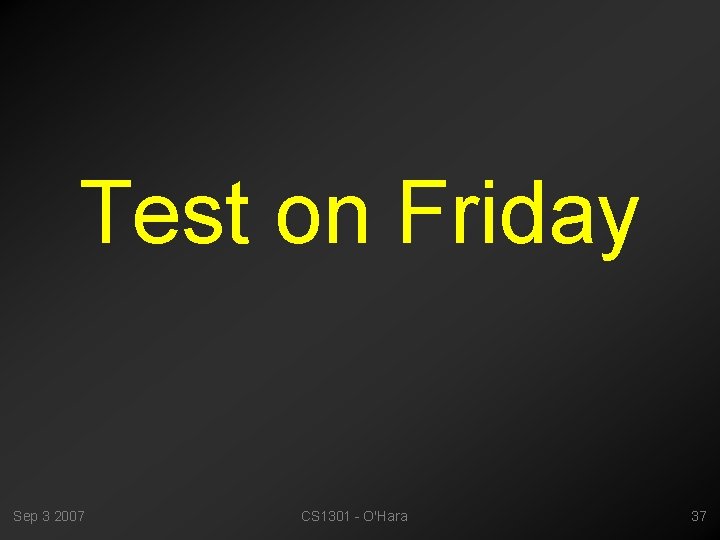
- Slides: 37
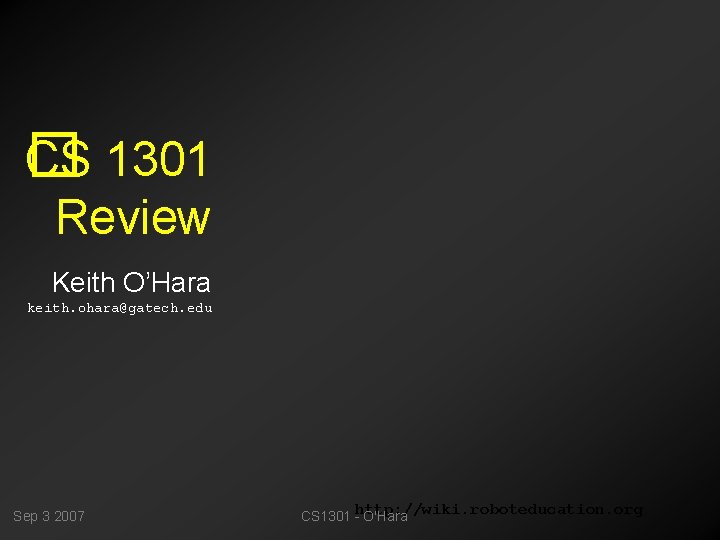
CS 1301 � Review Keith O’Hara keith. ohara@gatech. edu Sep 3 2007 CS 1301 -http: //wiki. roboteducation. org O'Hara
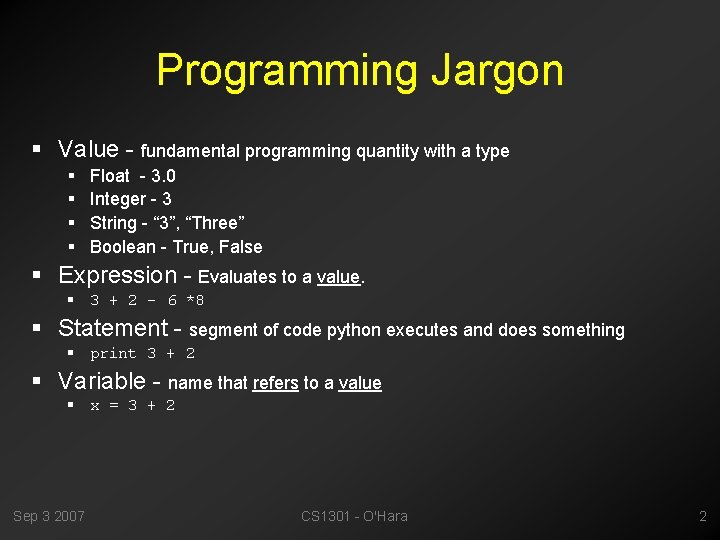
Programming Jargon § Value - fundamental programming quantity with a type § § Float - 3. 0 Integer - 3 String - “ 3”, “Three” Boolean - True, False § Expression - Evaluates to a value. § 3 + 2 - 6 *8 § Statement - segment of code python executes and does something § print 3 + 2 § Variable - name that refers to a value § x = 3 + 2 Sep 3 2007 CS 1301 - O'Hara 2
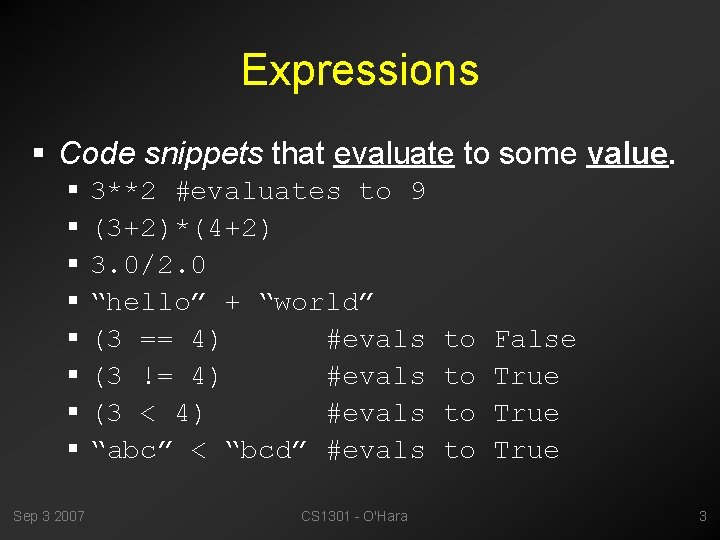
Expressions § Code snippets that evaluate to some value. § § § § Sep 3 2007 3**2 #evaluates to 9 (3+2)*(4+2) 3. 0/2. 0 “hello” + “world” (3 == 4) #evals (3 != 4) #evals (3 < 4) #evals “abc” < “bcd” #evals CS 1301 - O'Hara to to False True 3
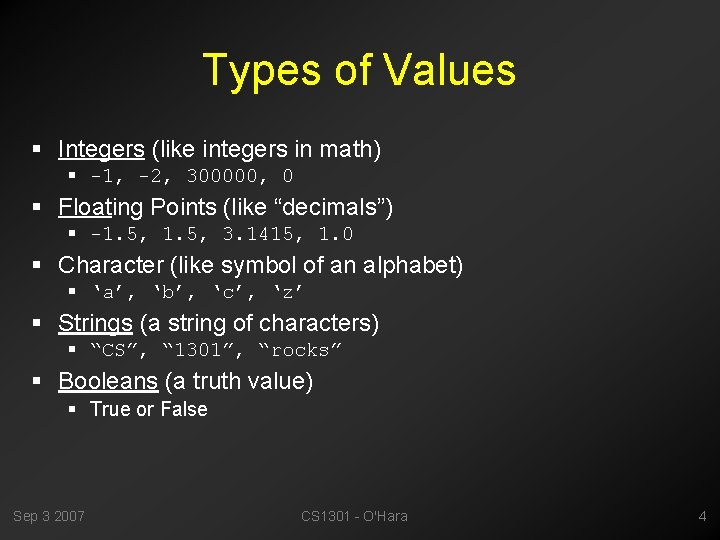
Types of Values § Integers (like integers in math) § -1, -2, 300000, 0 § Floating Points (like “decimals”) § -1. 5, 3. 1415, 1. 0 § Character (like symbol of an alphabet) § ‘a’, ‘b’, ‘c’, ‘z’ § Strings (a string of characters) § “CS”, “ 1301”, “rocks” § Booleans (a truth value) § True or False Sep 3 2007 CS 1301 - O'Hara 4
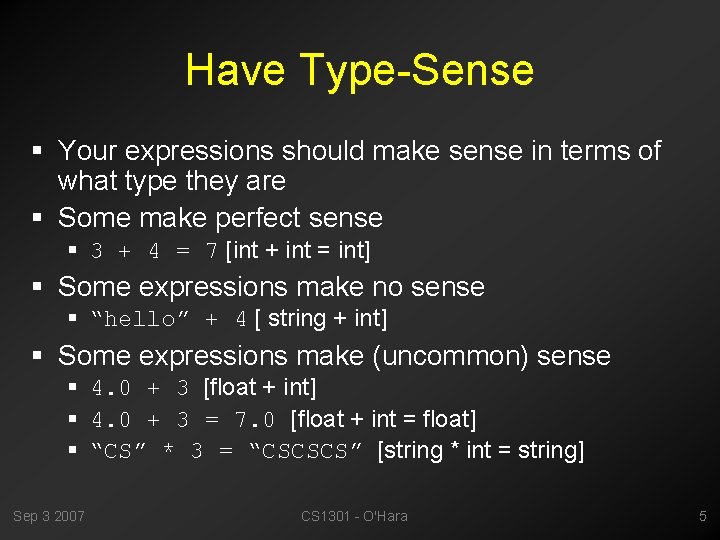
Have Type-Sense § Your expressions should make sense in terms of what type they are § Some make perfect sense § 3 + 4 = 7 [int + int = int] § Some expressions make no sense § “hello” + 4 [ string + int] § Some expressions make (uncommon) sense § 4. 0 + 3 [float + int] § 4. 0 + 3 = 7. 0 [float + int = float] § “CS” * 3 = “CSCSCS” [string * int = string] Sep 3 2007 CS 1301 - O'Hara 5
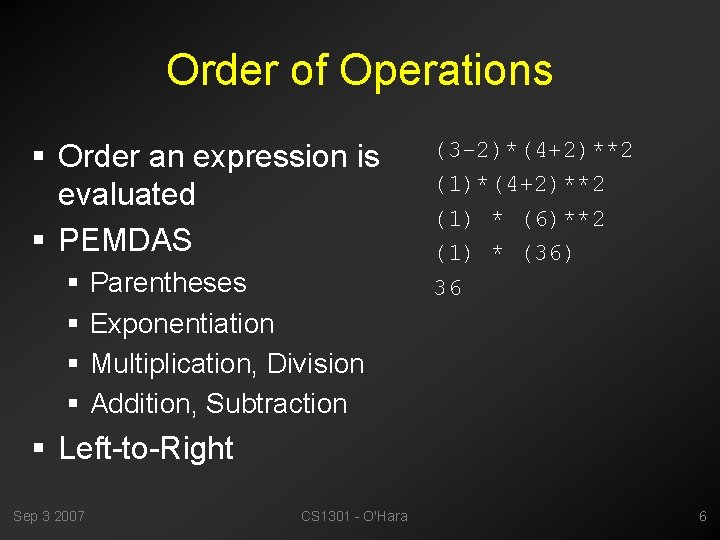
Order of Operations § Order an expression is evaluated § PEMDAS § § Parentheses Exponentiation Multiplication, Division Addition, Subtraction (3 -2)*(4+2)**2 (1) * (6)**2 (1) * (36) 36 § Left-to-Right Sep 3 2007 CS 1301 - O'Hara 6
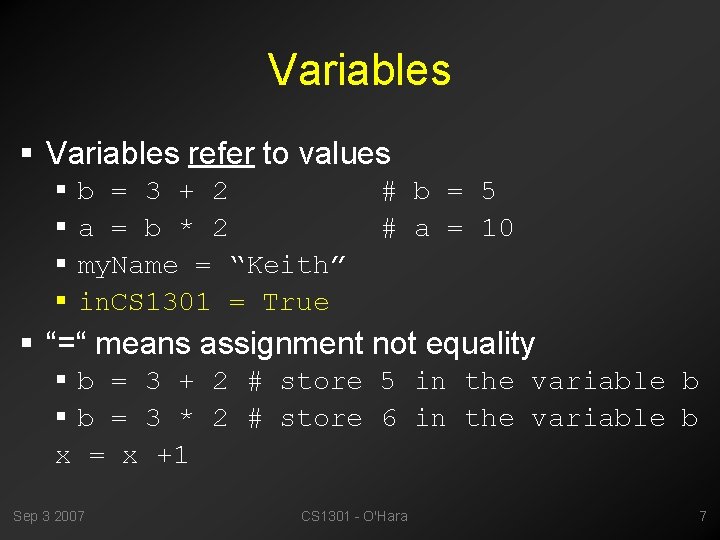
Variables § Variables refer to values § § b = 3 + 2 a = b * 2 my. Name = “Keith” in. CS 1301 = True # b = 5 # a = 10 § “=“ means assignment not equality § b = 3 + 2 # store 5 in the variable b § b = 3 * 2 # store 6 in the variable b x = x +1 Sep 3 2007 CS 1301 - O'Hara 7
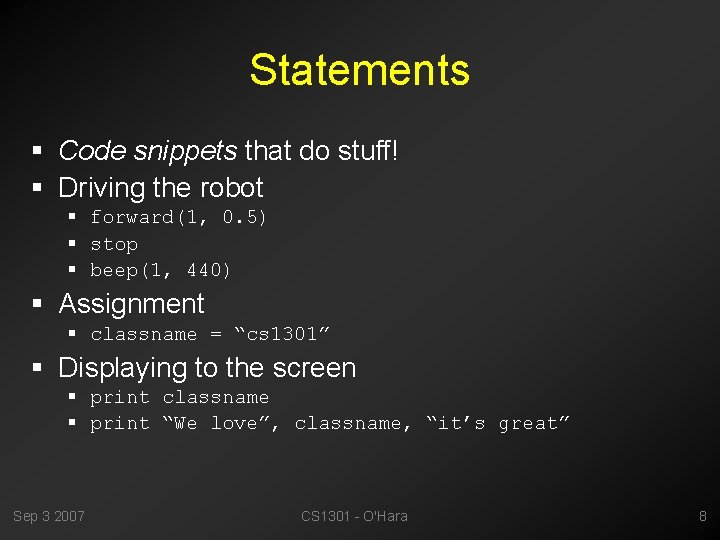
Statements § Code snippets that do stuff! § Driving the robot § forward(1, 0. 5) § stop § beep(1, 440) § Assignment § classname = “cs 1301” § Displaying to the screen § print classname § print “We love”, classname, “it’s great” Sep 3 2007 CS 1301 - O'Hara 8
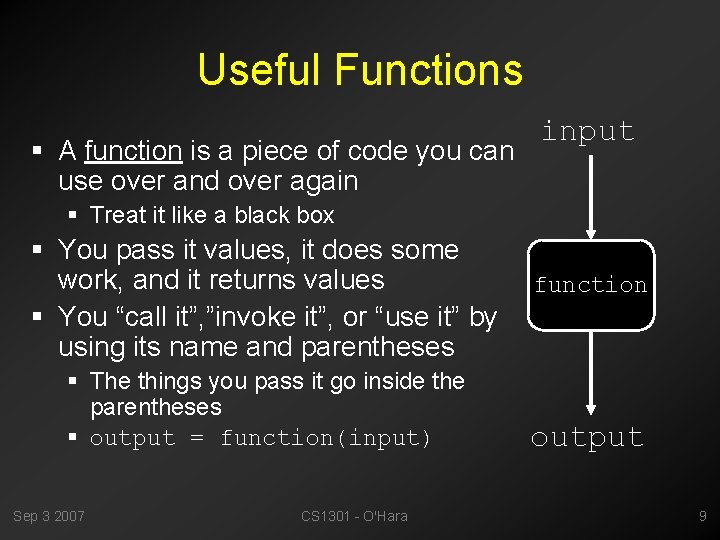
Useful Functions § A function is a piece of code you can use over and over again input § Treat it like a black box § You pass it values, it does some work, and it returns values § You “call it”, ”invoke it”, or “use it” by using its name and parentheses § The things you pass it go inside the parentheses § output = function(input) Sep 3 2007 CS 1301 - O'Hara function output 9
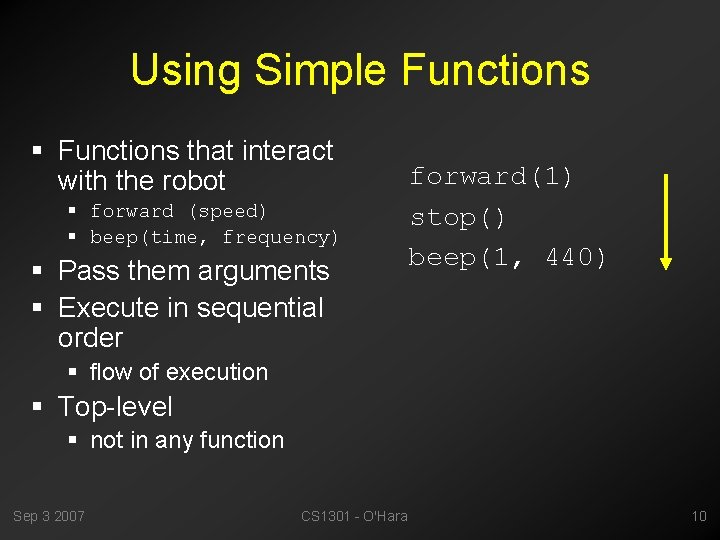
Using Simple Functions § Functions that interact with the robot § forward (speed) § beep(time, frequency) § Pass them arguments § Execute in sequential order forward(1) stop() beep(1, 440) § flow of execution § Top-level § not in any function Sep 3 2007 CS 1301 - O'Hara 10
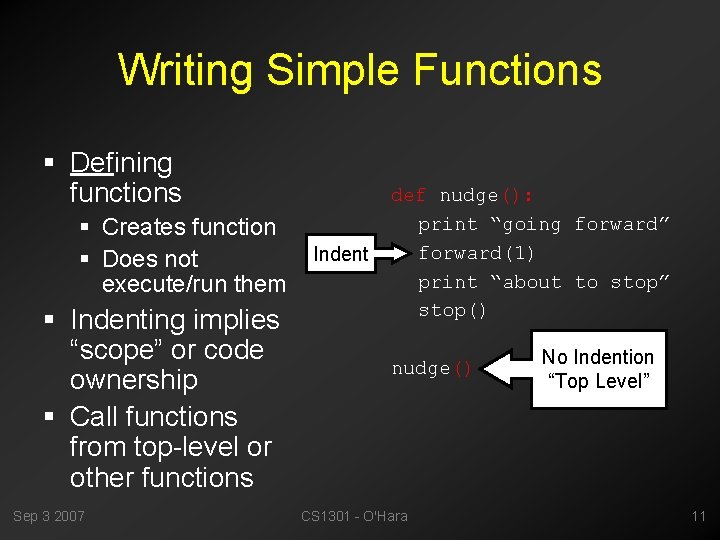
Writing Simple Functions § Defining functions § Creates function § Does not execute/run them § Indenting implies “scope” or code ownership § Call functions from top-level or other functions Sep 3 2007 Indent def nudge(): print “going forward” forward(1) print “about to stop” stop() nudge() CS 1301 - O'Hara No Indention “Top Level” 11
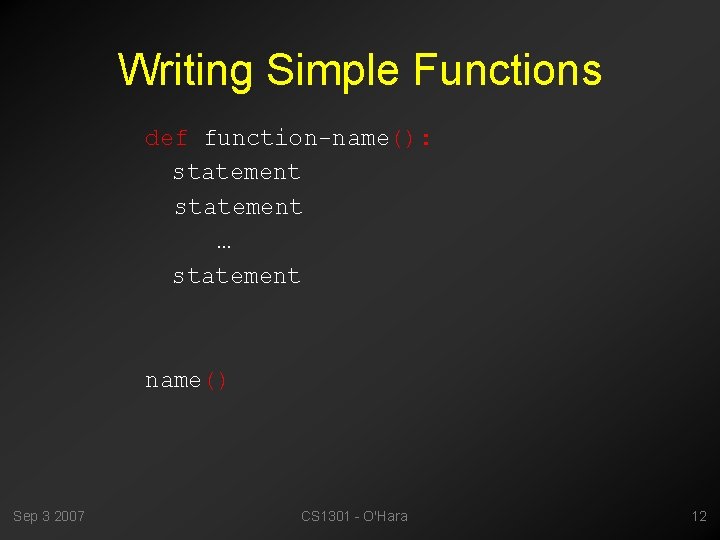
Writing Simple Functions def function-name(): statement … statement name() Sep 3 2007 CS 1301 - O'Hara 12
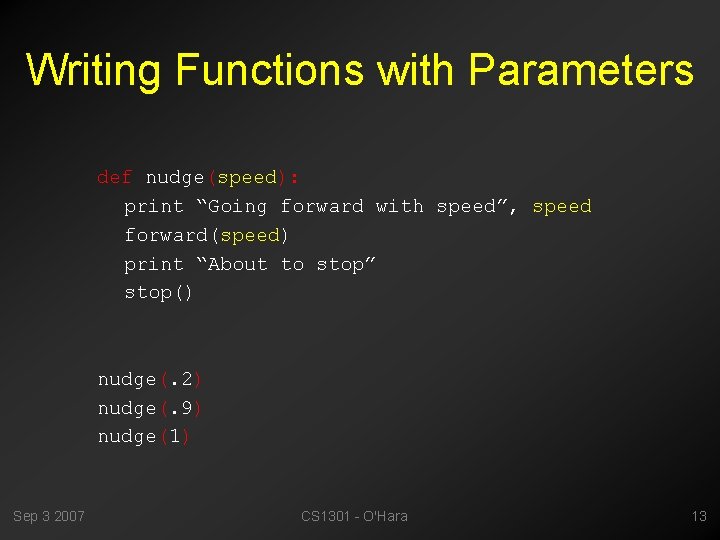
Writing Functions with Parameters def nudge(speed): print “Going forward with speed”, speed forward(speed) print “About to stop” stop() nudge(. 2) nudge(. 9) nudge(1) Sep 3 2007 CS 1301 - O'Hara 13
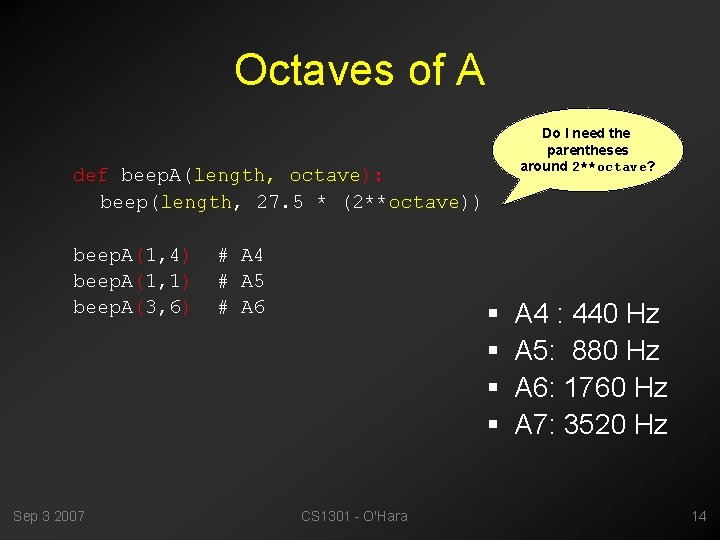
Octaves of A Do I need the parentheses around 2**octave? def beep. A(length, octave): beep(length, 27. 5 * (2**octave)) beep. A(1, 4) beep. A(1, 1) beep. A(3, 6) Sep 3 2007 # A 4 # A 5 # A 6 § § CS 1301 - O'Hara A 4 : 440 Hz A 5: 880 Hz A 6: 1760 Hz A 7: 3520 Hz 14
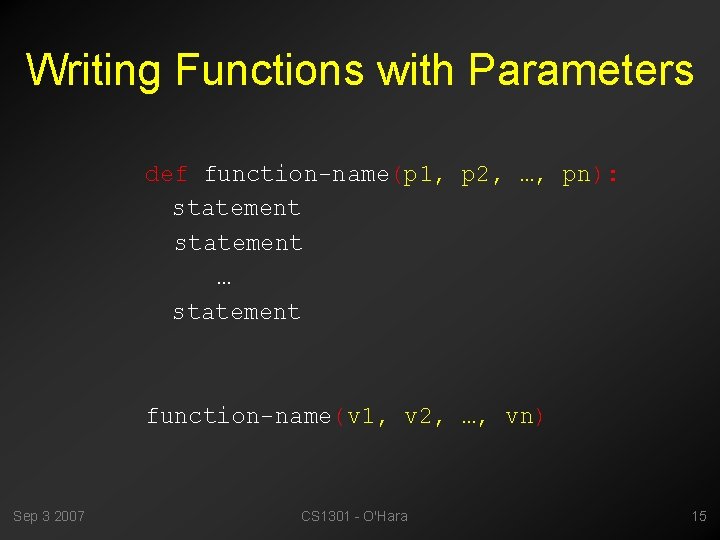
Writing Functions with Parameters def function-name(p 1, p 2, …, pn): statement … statement function-name(v 1, v 2, …, vn) Sep 3 2007 CS 1301 - O'Hara 15
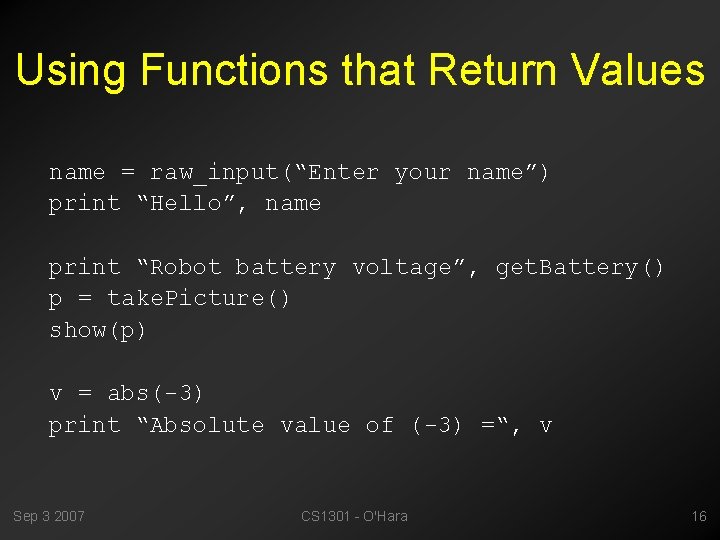
Using Functions that Return Values name = raw_input(“Enter your name”) print “Hello”, name print “Robot battery voltage”, get. Battery() p = take. Picture() show(p) v = abs(-3) print “Absolute value of (-3) =“, v Sep 3 2007 CS 1301 - O'Hara 16
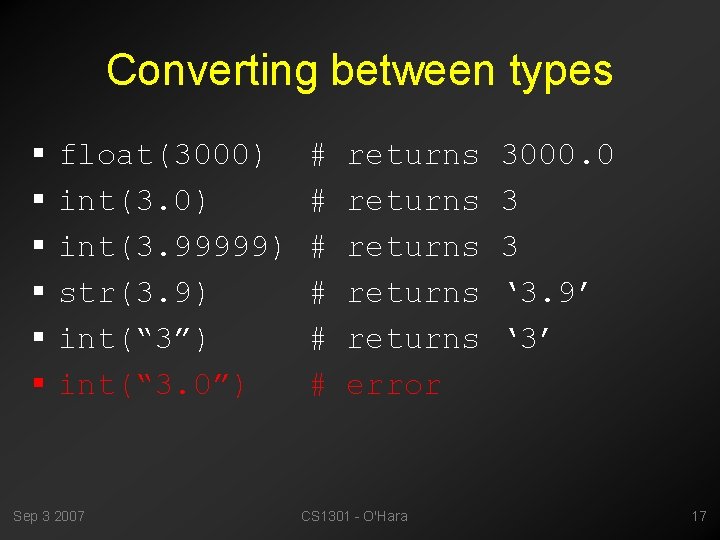
Converting between types § § § float(3000) int(3. 99999) str(3. 9) int(“ 3”) int(“ 3. 0”) Sep 3 2007 # # # returns returns error CS 1301 - O'Hara 3000. 0 3 3 ‘ 3. 9’ ‘ 3’ 17
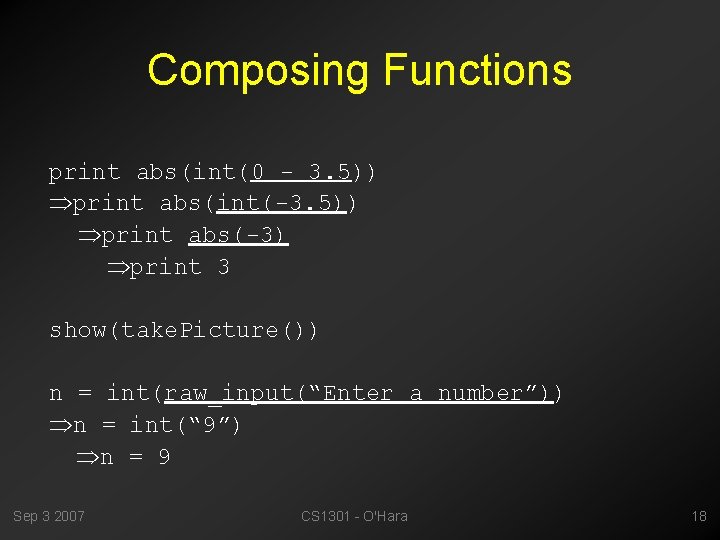
Composing Functions print abs(int(0 - 3. 5)) print abs(int(-3. 5)) print abs(-3) print 3 show(take. Picture()) n = int(raw_input(“Enter a number”)) n = int(“ 9”) n = 9 Sep 3 2007 CS 1301 - O'Hara 18
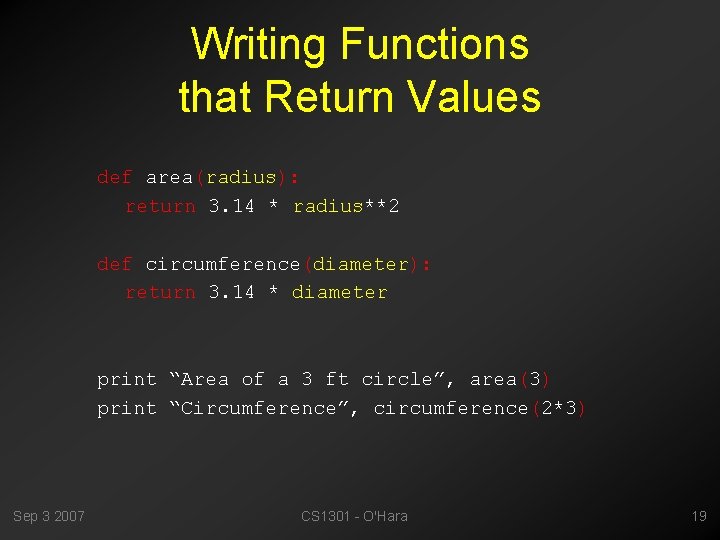
Writing Functions that Return Values def area(radius): return 3. 14 * radius**2 def circumference(diameter): return 3. 14 * diameter print “Area of a 3 ft circle”, area(3) print “Circumference”, circumference(2*3) Sep 3 2007 CS 1301 - O'Hara 19
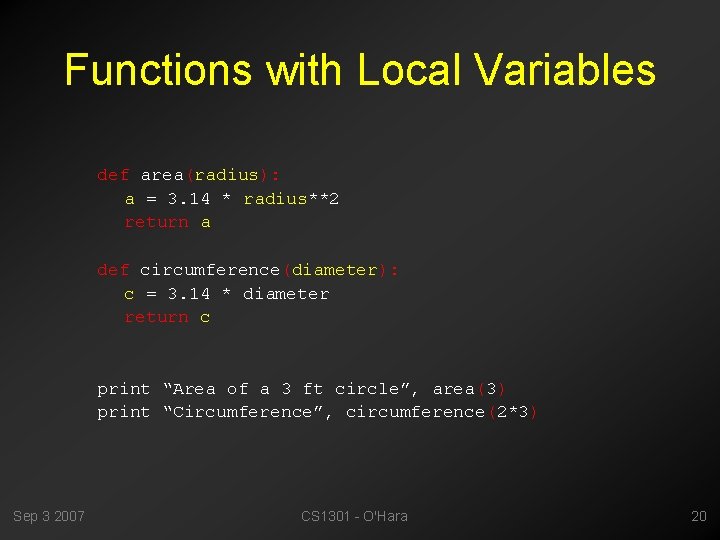
Functions with Local Variables def area(radius): a = 3. 14 * radius**2 return a def circumference(diameter): c = 3. 14 * diameter return c print “Area of a 3 ft circle”, area(3) print “Circumference”, circumference(2*3) Sep 3 2007 CS 1301 - O'Hara 20
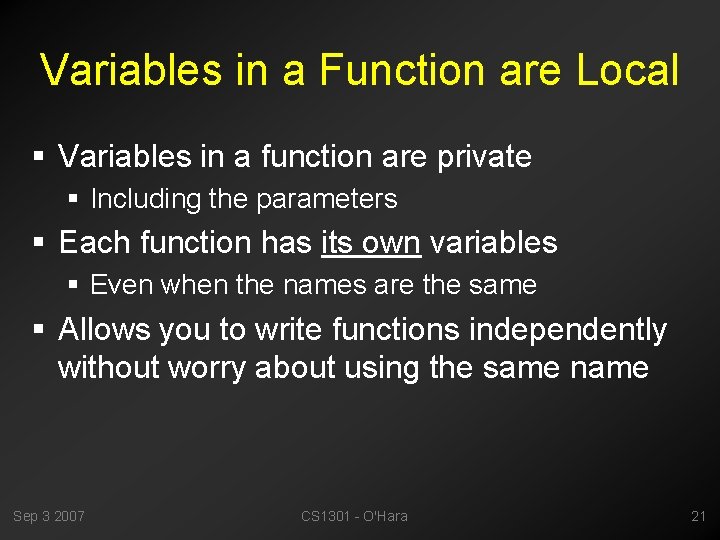
Variables in a Function are Local § Variables in a function are private § Including the parameters § Each function has its own variables § Even when the names are the same § Allows you to write functions independently without worry about using the same name Sep 3 2007 CS 1301 - O'Hara 21
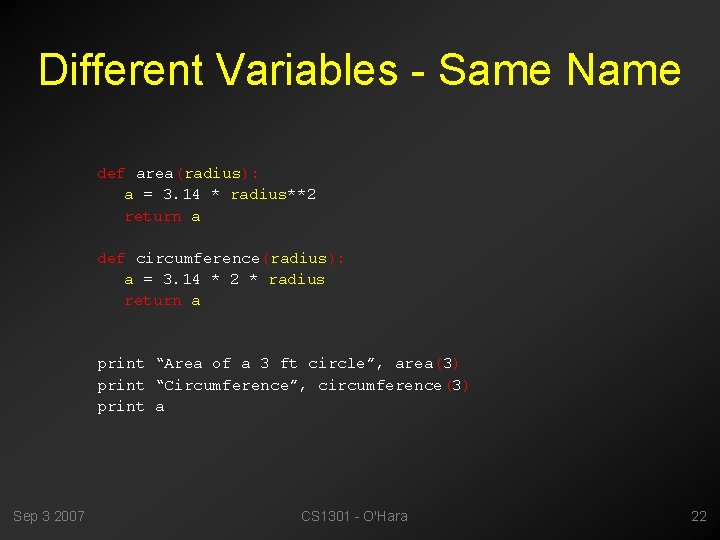
Different Variables - Same Name def area(radius): a = 3. 14 * radius**2 return a def circumference(radius): a = 3. 14 * 2 * radius return a print “Area of a 3 ft circle”, area(3) print “Circumference”, circumference(3) print a Sep 3 2007 CS 1301 - O'Hara 22
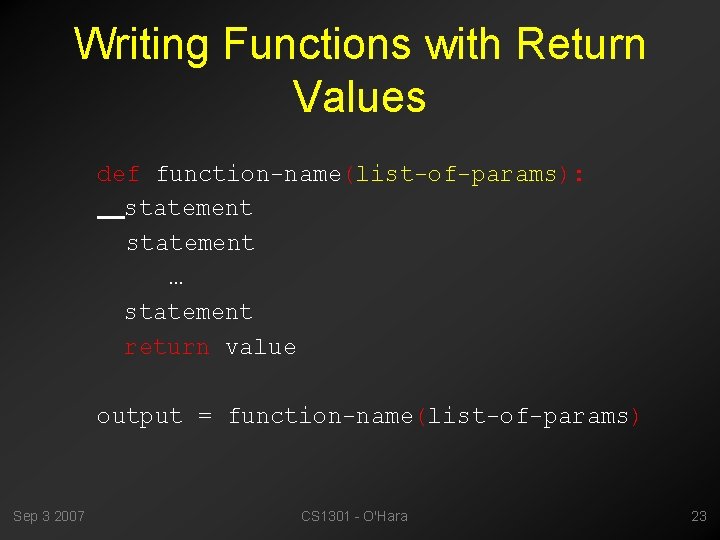
Writing Functions with Return Values def function-name(list-of-params): statement … statement return value output = function-name(list-of-params) Sep 3 2007 CS 1301 - O'Hara 23
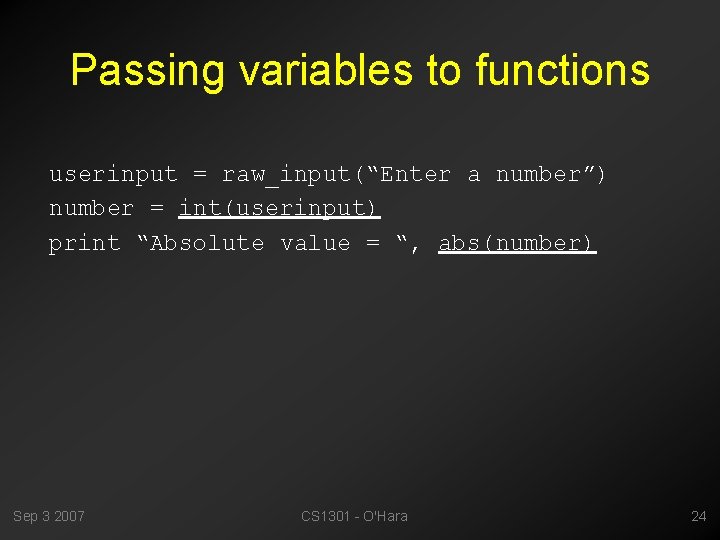
Passing variables to functions userinput = raw_input(“Enter a number”) number = int(userinput) print “Absolute value = “, abs(number) Sep 3 2007 CS 1301 - O'Hara 24
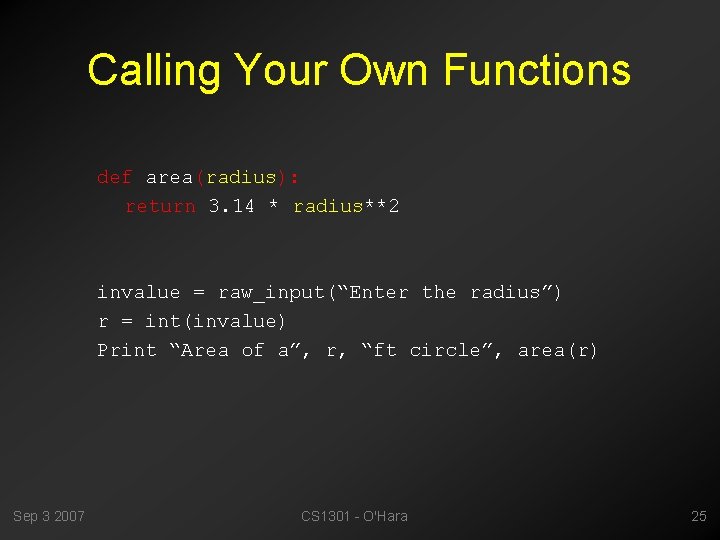
Calling Your Own Functions def area(radius): return 3. 14 * radius**2 invalue = raw_input(“Enter the radius”) r = int(invalue) Print “Area of a”, r, “ft circle”, area(r) Sep 3 2007 CS 1301 - O'Hara 25
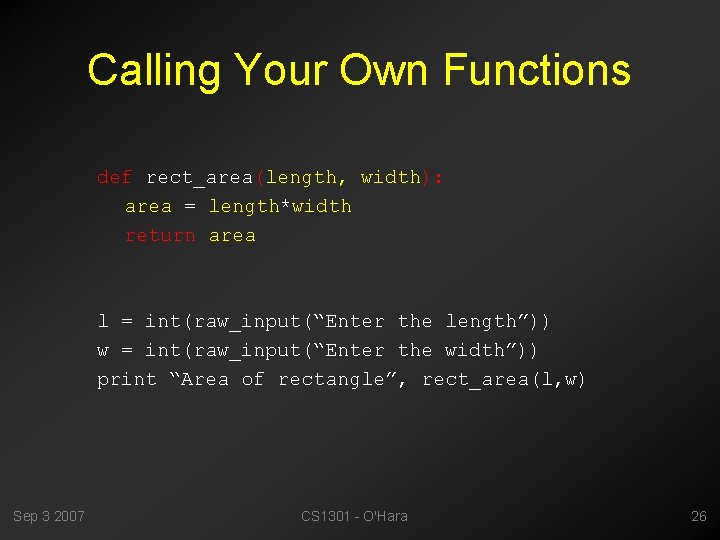
Calling Your Own Functions def rect_area(length, width): area = length*width return area l = int(raw_input(“Enter the length”)) w = int(raw_input(“Enter the width”)) print “Area of rectangle”, rect_area(l, w) Sep 3 2007 CS 1301 - O'Hara 26
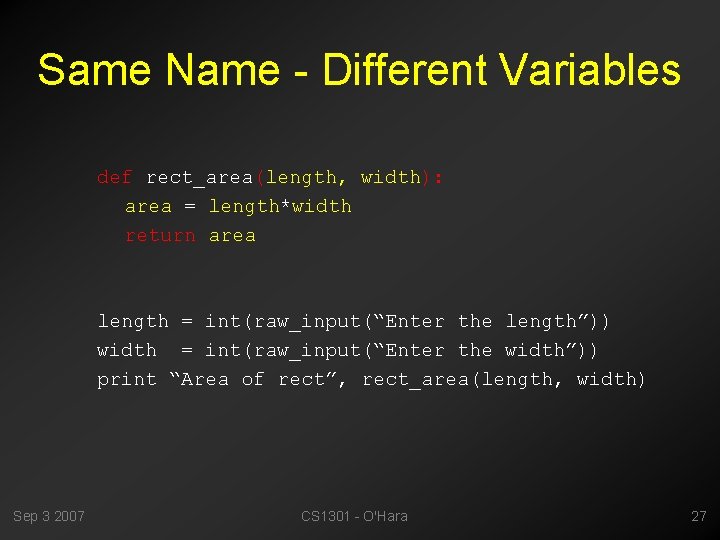
Same Name - Different Variables def rect_area(length, width): area = length*width return area length = int(raw_input(“Enter the length”)) width = int(raw_input(“Enter the width”)) print “Area of rect”, rect_area(length, width) Sep 3 2007 CS 1301 - O'Hara 27
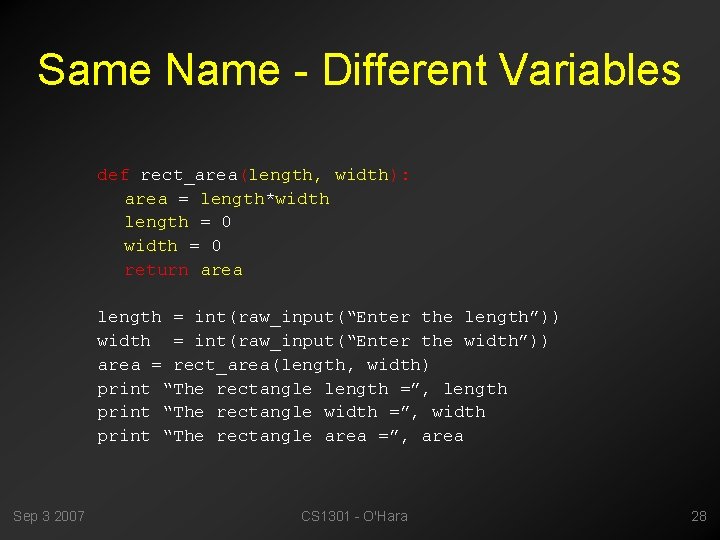
Same Name - Different Variables def rect_area(length, width): area = length*width length = 0 width = 0 return area length = int(raw_input(“Enter the length”)) width = int(raw_input(“Enter the width”)) area = rect_area(length, width) print “The rectangle length =”, length print “The rectangle width =”, width print “The rectangle area =”, area Sep 3 2007 CS 1301 - O'Hara 28
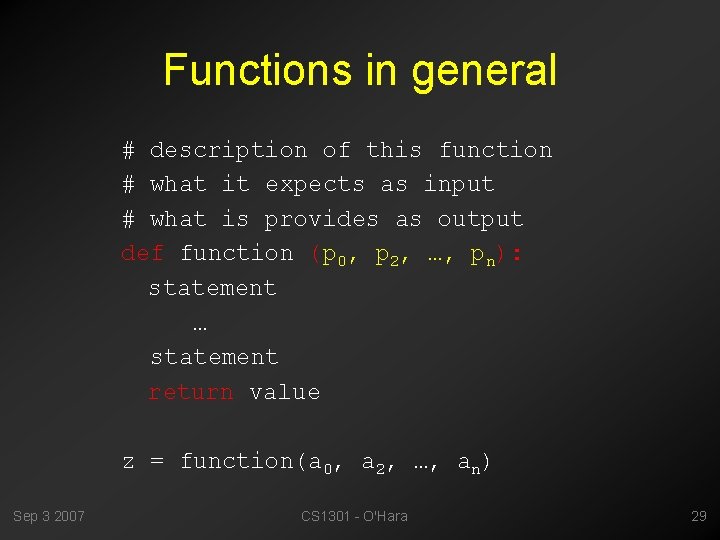
Functions in general # description of this function # what it expects as input # what is provides as output def function (p 0, p 2, …, pn): statement … statement return value z = function(a 0, a 2, …, an) Sep 3 2007 CS 1301 - O'Hara 29
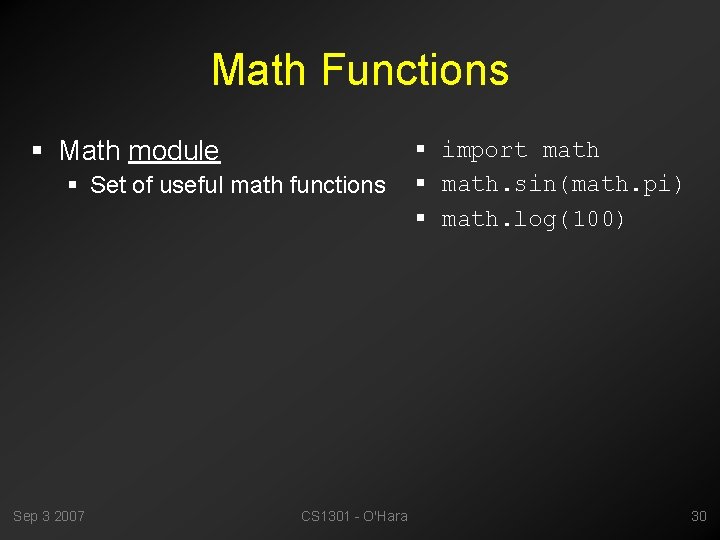
Math Functions § Math module § Set of useful math functions Sep 3 2007 CS 1301 - O'Hara § import math § math. sin(math. pi) § math. log(100) 30
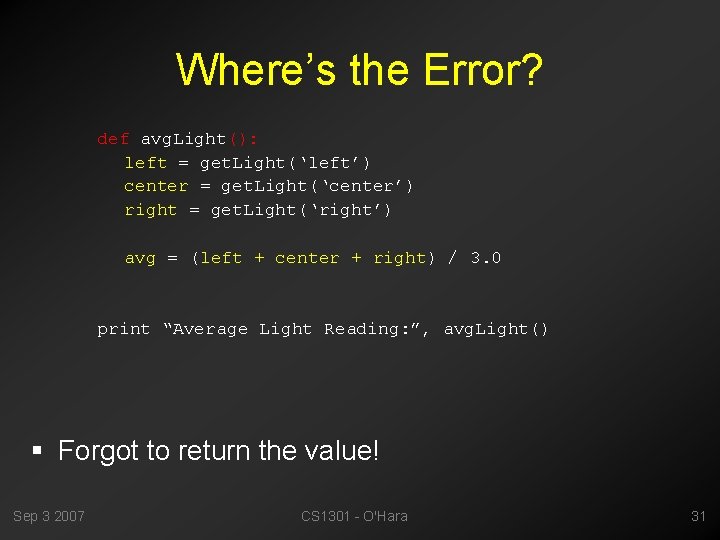
Where’s the Error? def avg. Light(): left = get. Light(‘left’) center = get. Light(‘center’) right = get. Light(‘right’) avg = (left + center + right) / 3. 0 print “Average Light Reading: ”, avg. Light() § Forgot to return the value! Sep 3 2007 CS 1301 - O'Hara 31
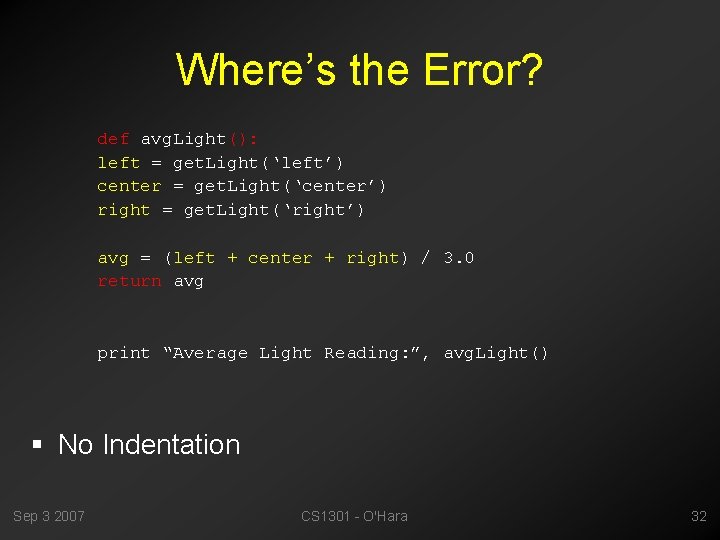
Where’s the Error? def avg. Light(): left = get. Light(‘left’) center = get. Light(‘center’) right = get. Light(‘right’) avg = (left + center + right) / 3. 0 return avg print “Average Light Reading: ”, avg. Light() § No Indentation Sep 3 2007 CS 1301 - O'Hara 32
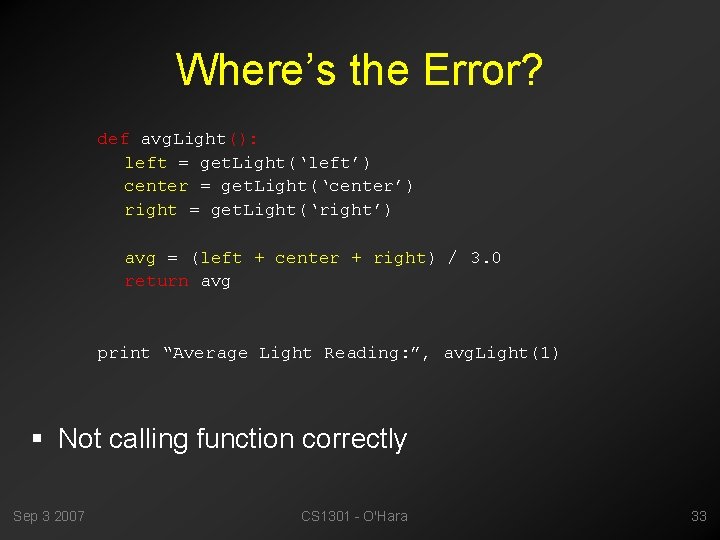
Where’s the Error? def avg. Light(): left = get. Light(‘left’) center = get. Light(‘center’) right = get. Light(‘right’) avg = (left + center + right) / 3. 0 return avg print “Average Light Reading: ”, avg. Light(1) § Not calling function correctly Sep 3 2007 CS 1301 - O'Hara 33
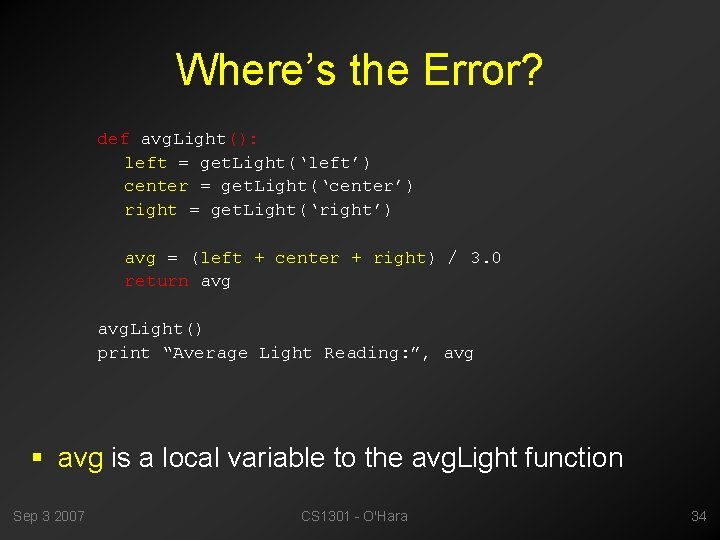
Where’s the Error? def avg. Light(): left = get. Light(‘left’) center = get. Light(‘center’) right = get. Light(‘right’) avg = (left + center + right) / 3. 0 return avg. Light() print “Average Light Reading: ”, avg § avg is a local variable to the avg. Light function Sep 3 2007 CS 1301 - O'Hara 34
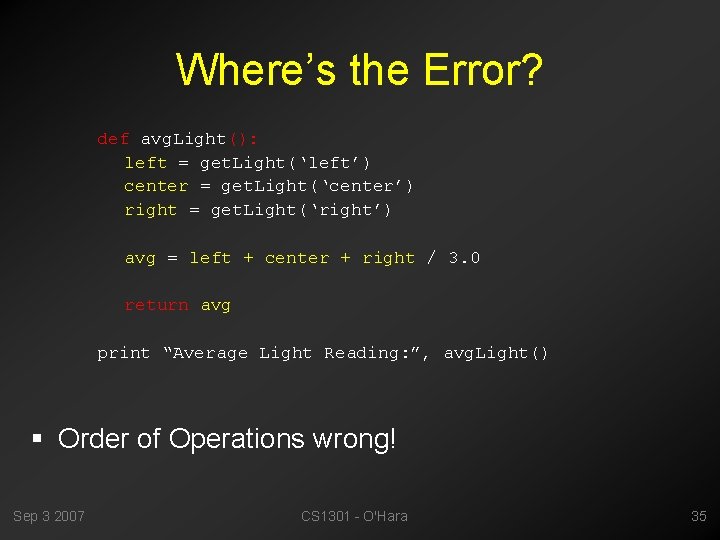
Where’s the Error? def avg. Light(): left = get. Light(‘left’) center = get. Light(‘center’) right = get. Light(‘right’) avg = left + center + right / 3. 0 return avg print “Average Light Reading: ”, avg. Light() § Order of Operations wrong! Sep 3 2007 CS 1301 - O'Hara 35
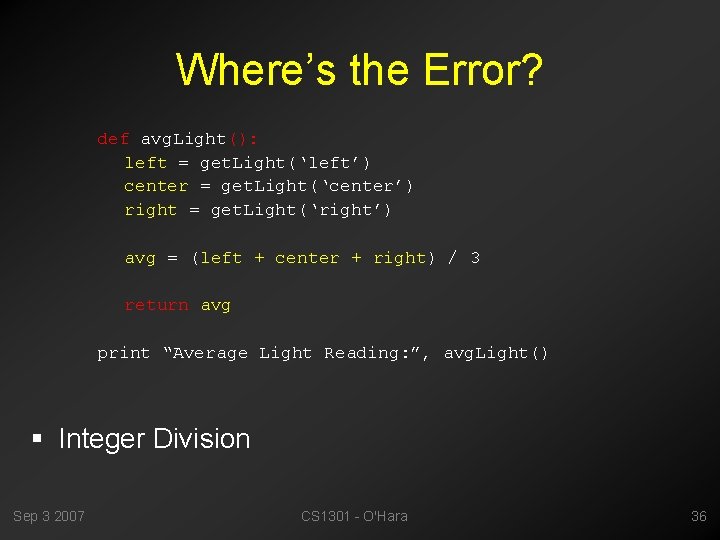
Where’s the Error? def avg. Light(): left = get. Light(‘left’) center = get. Light(‘center’) right = get. Light(‘right’) avg = (left + center + right) / 3 return avg print “Average Light Reading: ”, avg. Light() § Integer Division Sep 3 2007 CS 1301 - O'Hara 36
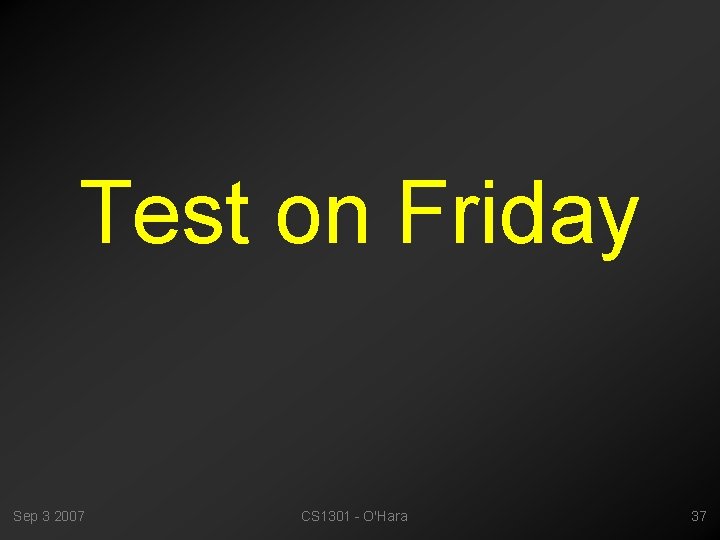
Test on Friday Sep 3 2007 CS 1301 - O'Hara 37
La 1301
La1301
General education movie
Cs 1301
Envr 1301
Ee 1301
Arts 1301
Chickens never wear shoes movie genre
Edu.sharif.edu
Chapter review motion part a vocabulary review answer key
Writ of certiorari ap gov example
Nader amin-salehi
Inclusion criteria examples
Narrative review vs systematic review
Keith mukami
George keith and john shuttleworth
Keith blakely md
Keith wagner classification
Keith legoy
Keith foord
Keith bryant
Keith shubert
Moses and the burning bush keith haring
Charles and keith swot analysis
Keith and wagner classification
Keith hohn
Keith palmer pastor
Keith bjorge
Keith haring characteristics
Erin keith unr
Fred korematsu
Keith burke ucc
Nonrestrictive clauses
Keith witney
Which numbered section contains correct punctuation?
Keith harris flavours
Keith tooley
Keith palmer pastor