CS 1050 Understanding and Constructing Proofs Spring 2006
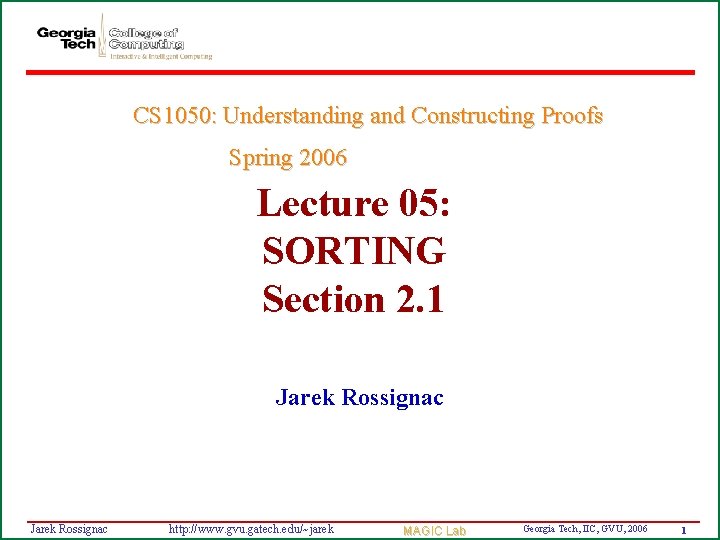
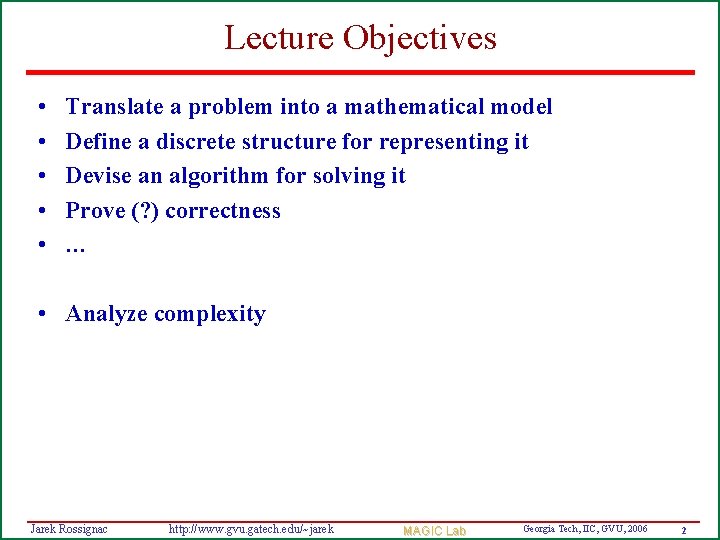
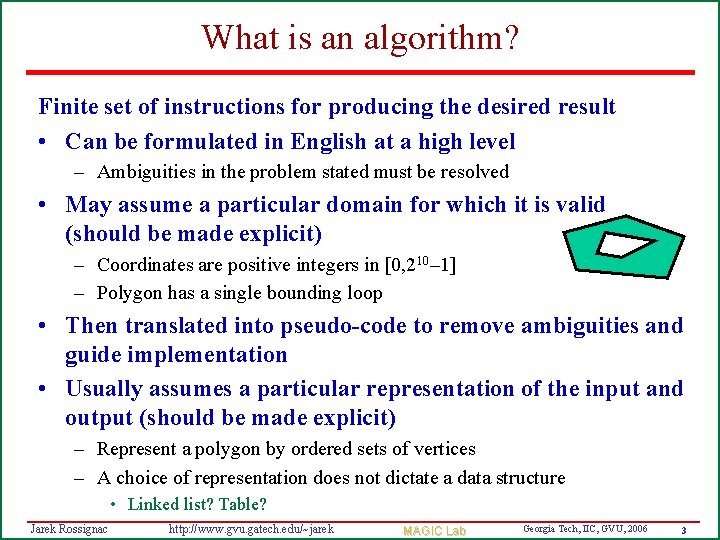
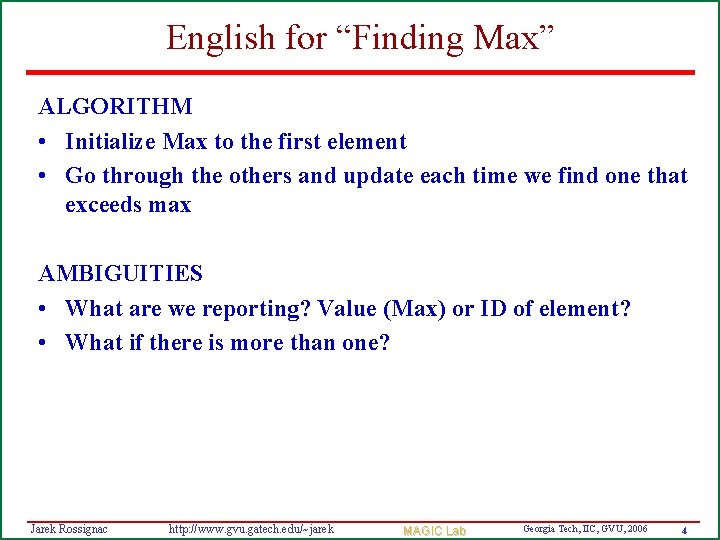
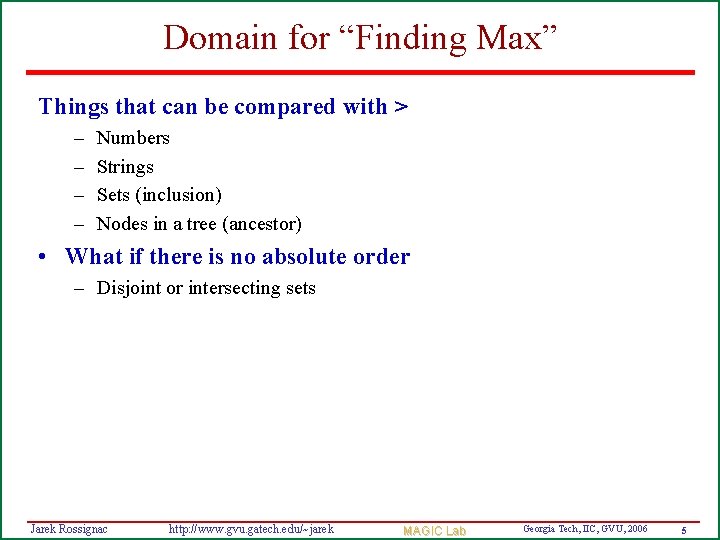
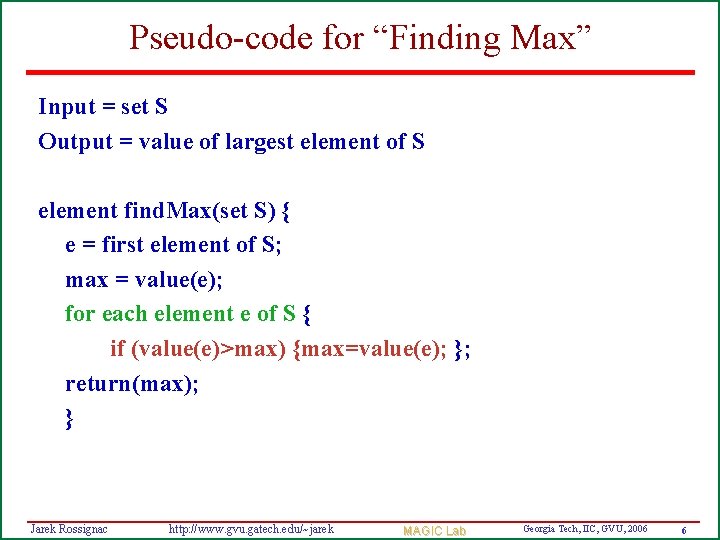
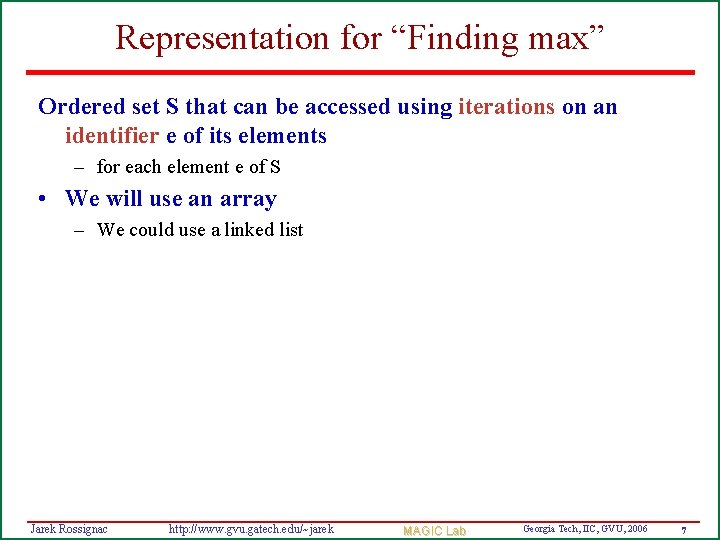
![Processing code for “Finding Max” int[] T = new int [6]; T[0]=4; T[1]=2; T[2]=5; Processing code for “Finding Max” int[] T = new int [6]; T[0]=4; T[1]=2; T[2]=5;](https://slidetodoc.com/presentation_image_h2/b3cb6ee1d0eeb4fab3a39a5a9ee18a66/image-8.jpg)
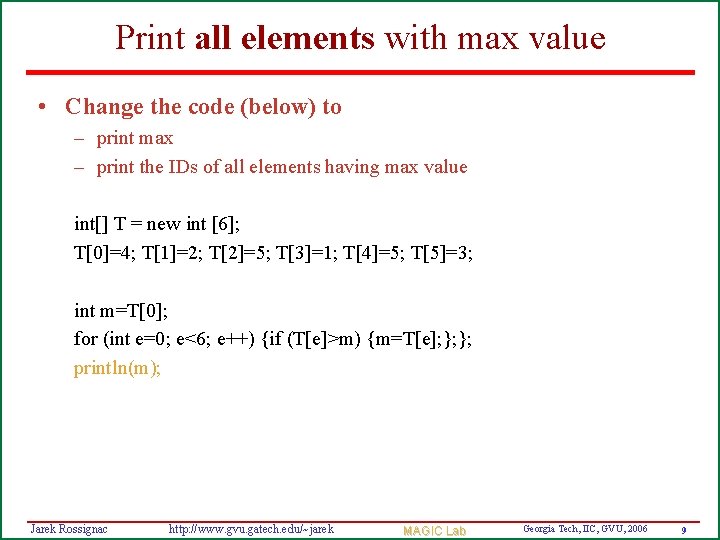
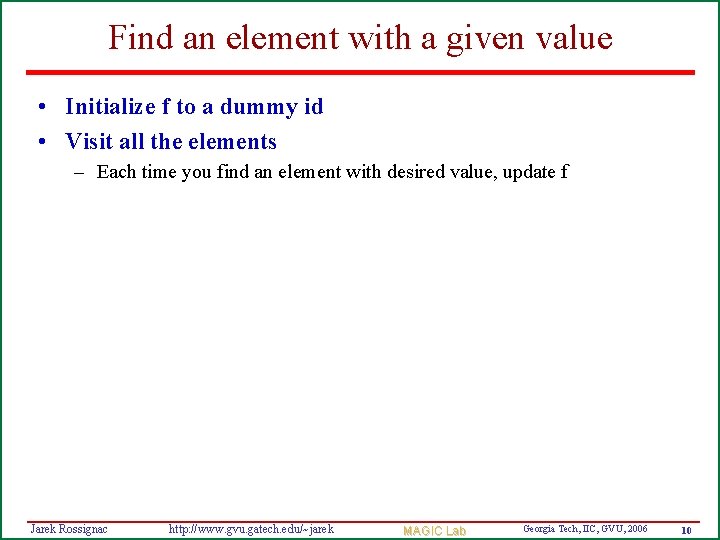
![Linear search (what does it report? ) float[] T = new float [6]; float Linear search (what does it report? ) float[] T = new float [6]; float](https://slidetodoc.com/presentation_image_h2/b3cb6ee1d0eeb4fab3a39a5a9ee18a66/image-11.jpg)
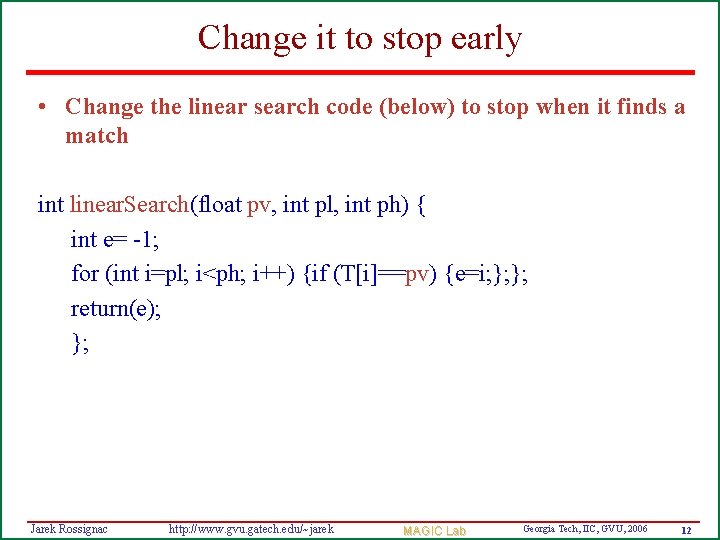
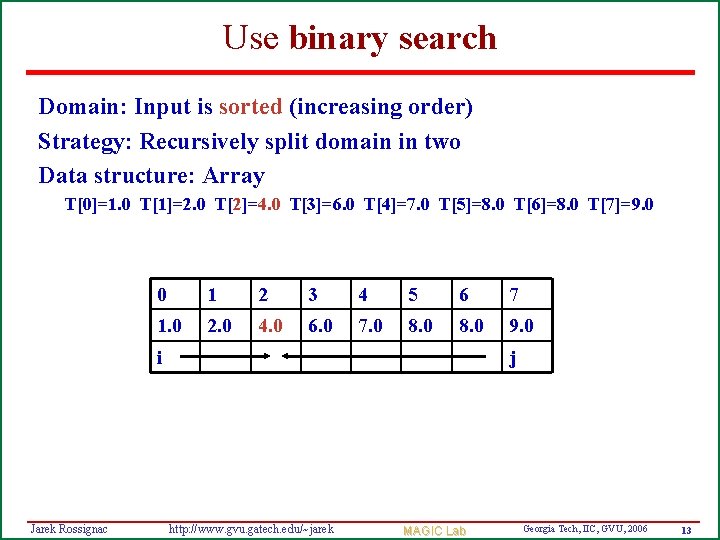
![Example: Binary search for v=4. 0 while (i<j) { m=(i+j)/2; if (v>T[m]) {i=m+1; } Example: Binary search for v=4. 0 while (i<j) { m=(i+j)/2; if (v>T[m]) {i=m+1; }](https://slidetodoc.com/presentation_image_h2/b3cb6ee1d0eeb4fab3a39a5a9ee18a66/image-14.jpg)
![Write the code for Binary search ! float[] T = new float [6]; float Write the code for Binary search ! float[] T = new float [6]; float](https://slidetodoc.com/presentation_image_h2/b3cb6ee1d0eeb4fab3a39a5a9ee18a66/image-15.jpg)
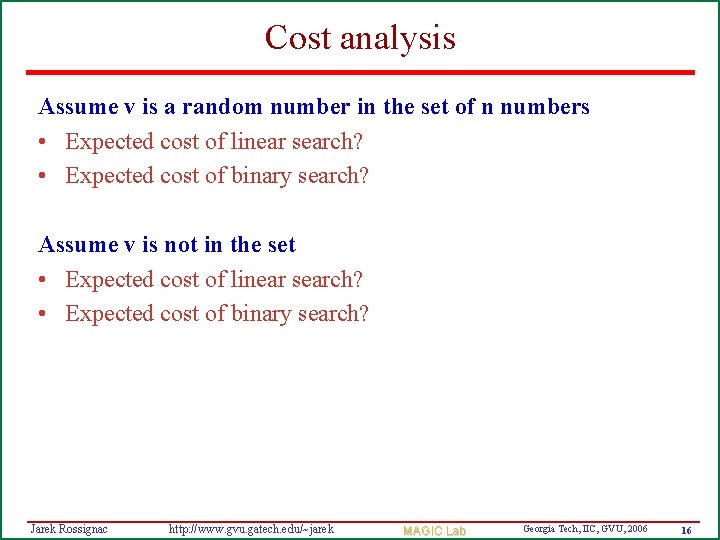
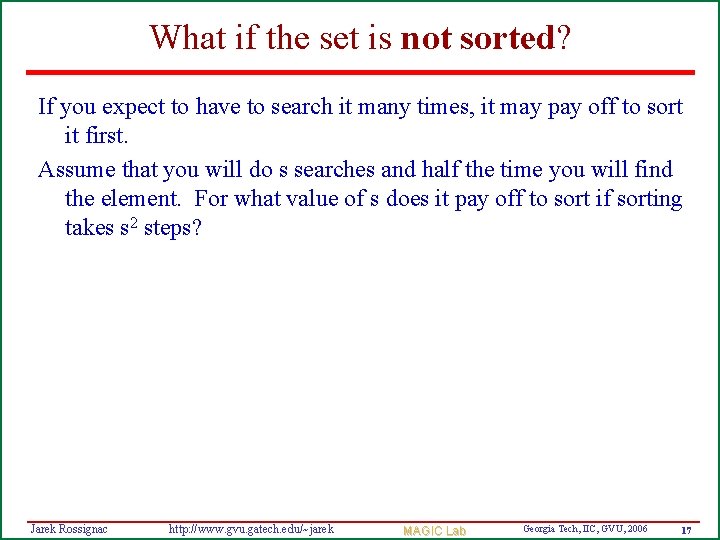
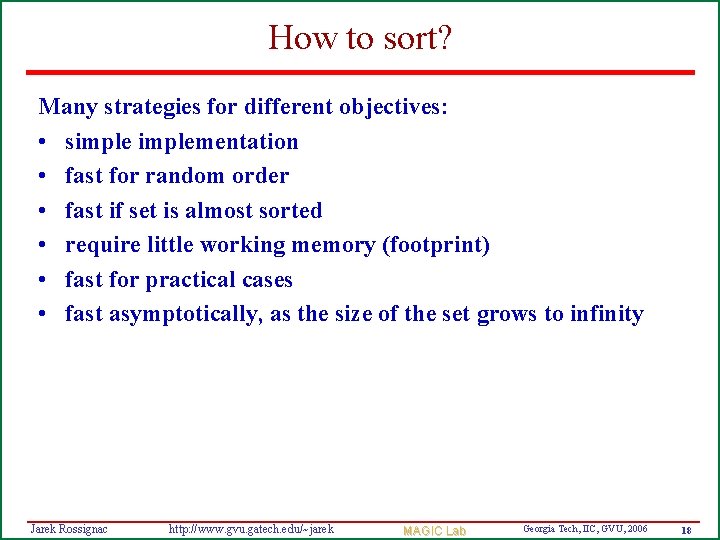
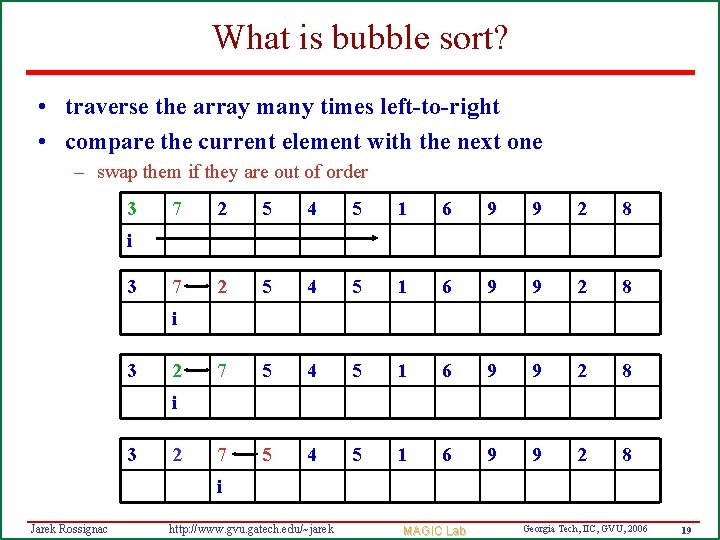
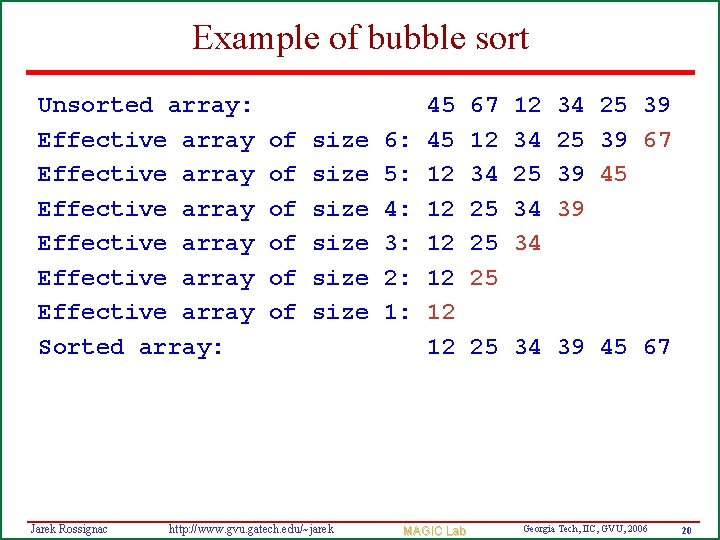
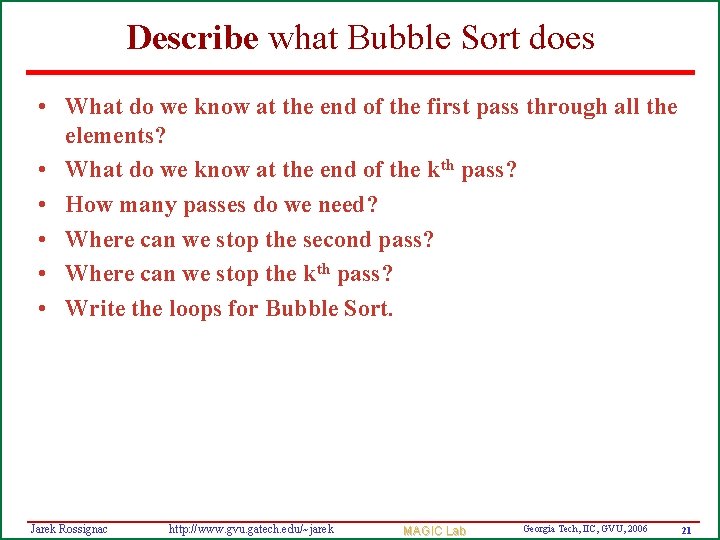
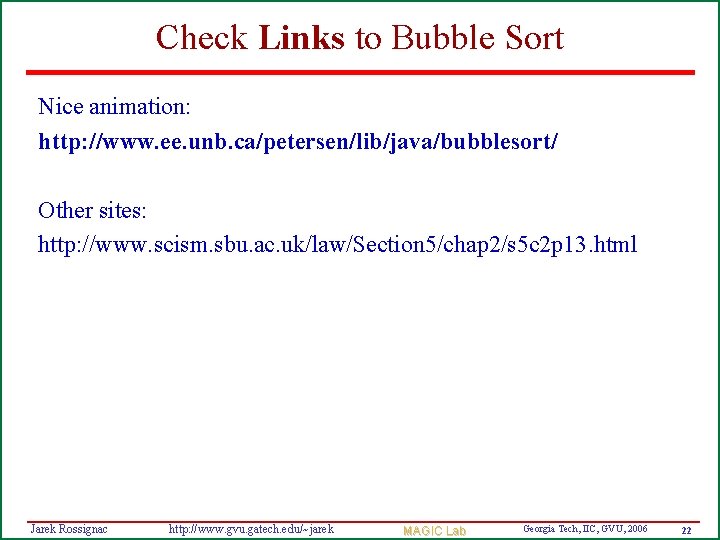
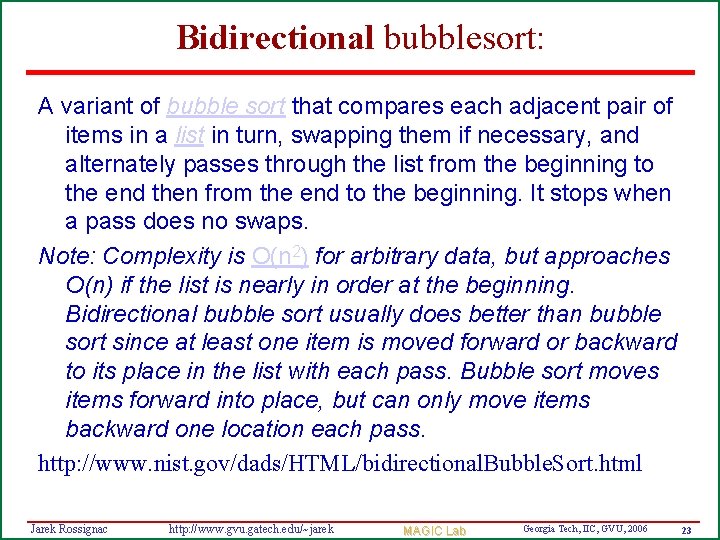
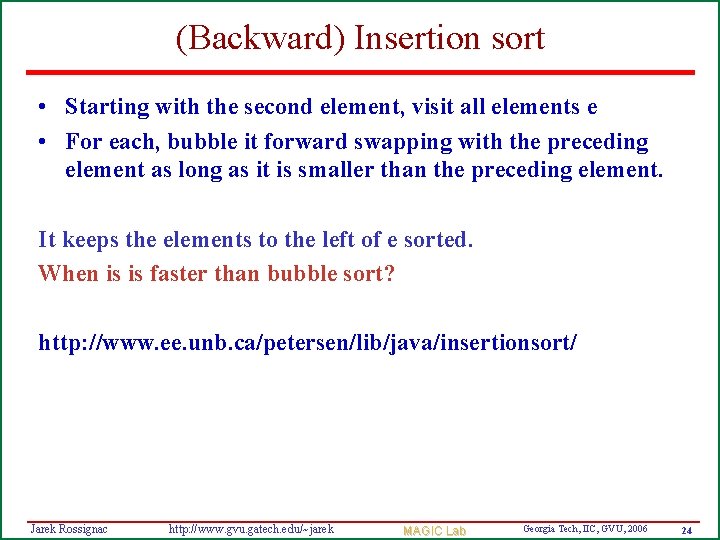
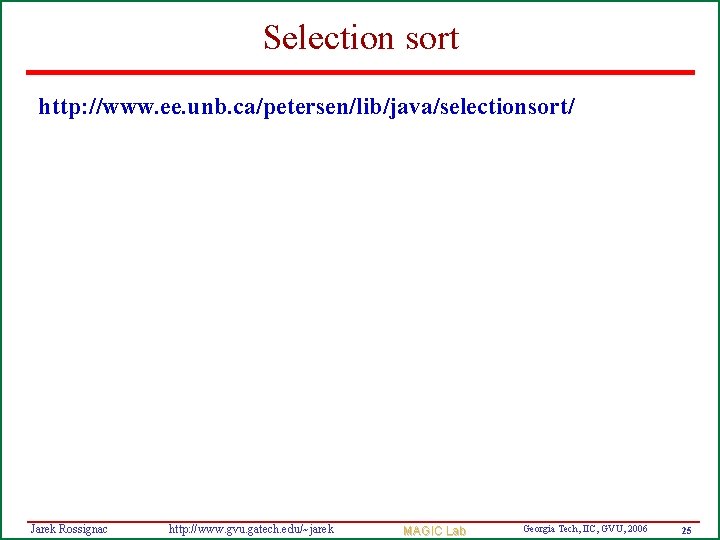
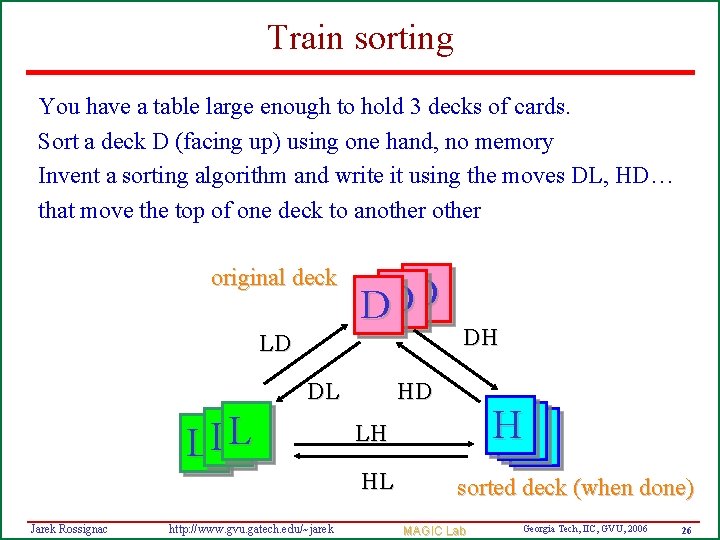
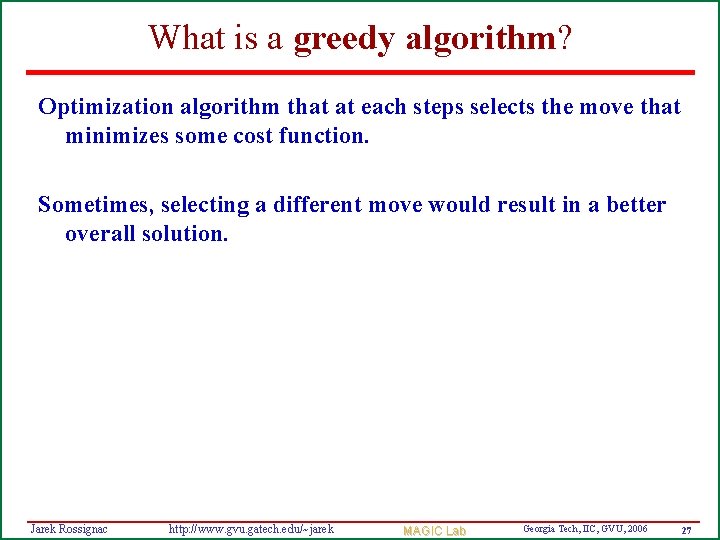
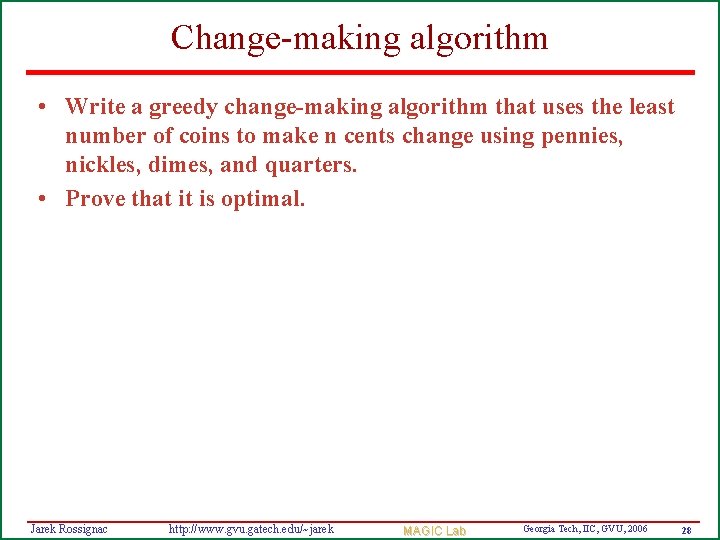
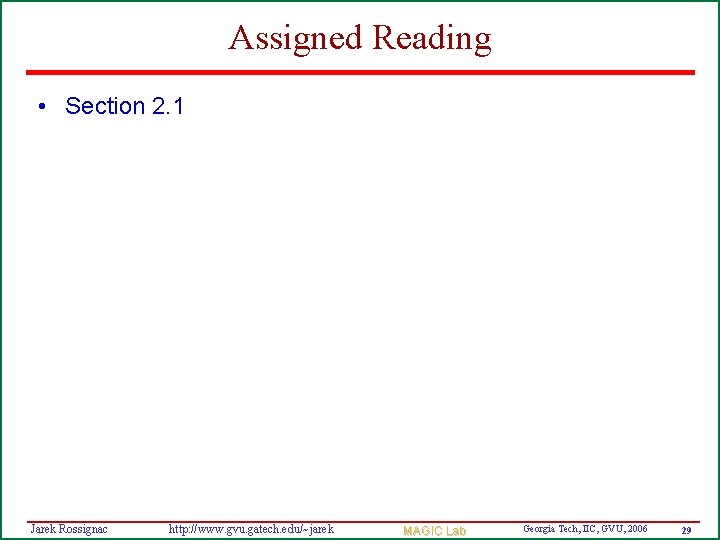
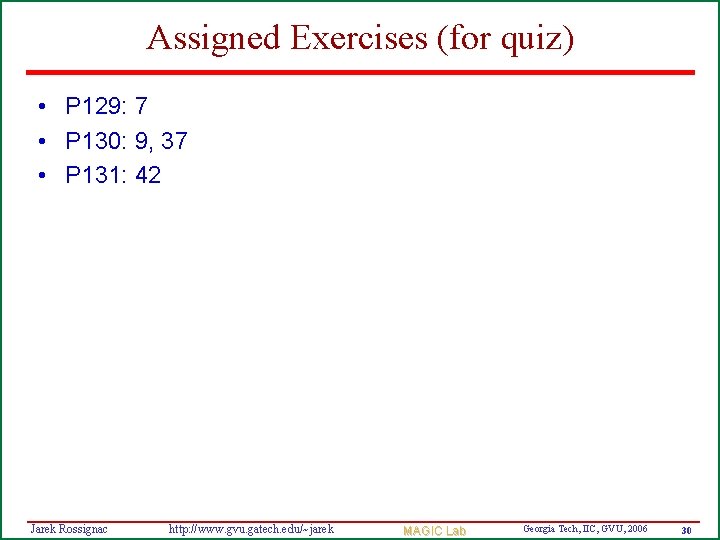
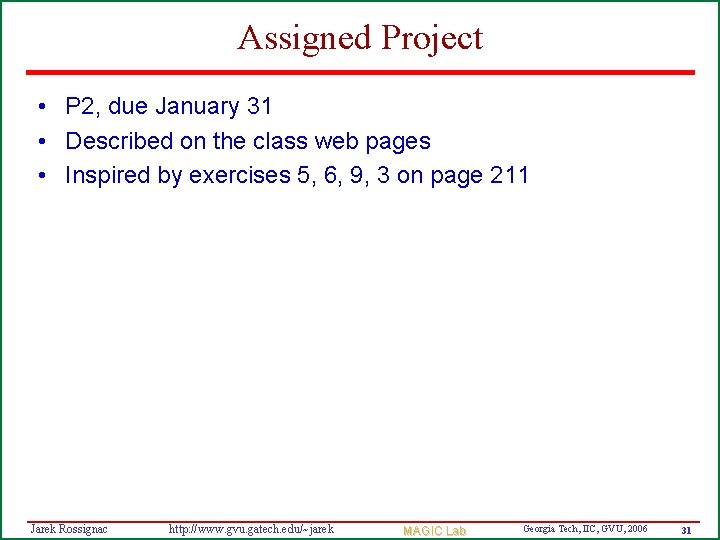
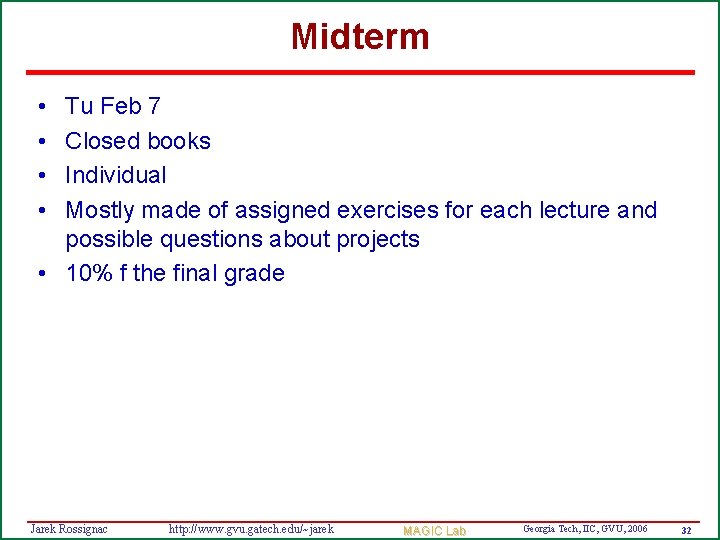
- Slides: 32
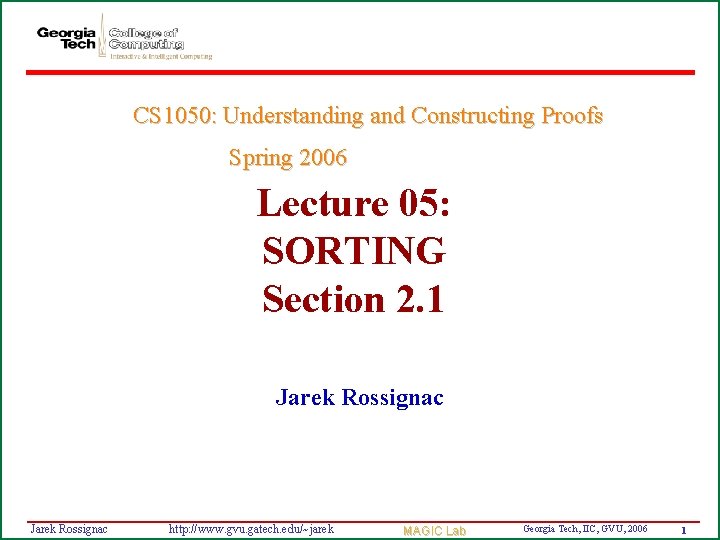
CS 1050: Understanding and Constructing Proofs Spring 2006 Lecture 05: SORTING Section 2. 1 Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 1
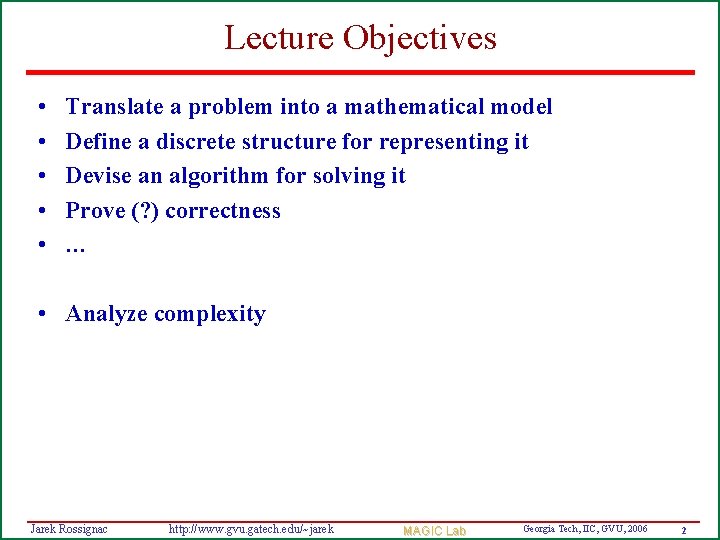
Lecture Objectives • • • Translate a problem into a mathematical model Define a discrete structure for representing it Devise an algorithm for solving it Prove (? ) correctness … • Analyze complexity Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 2
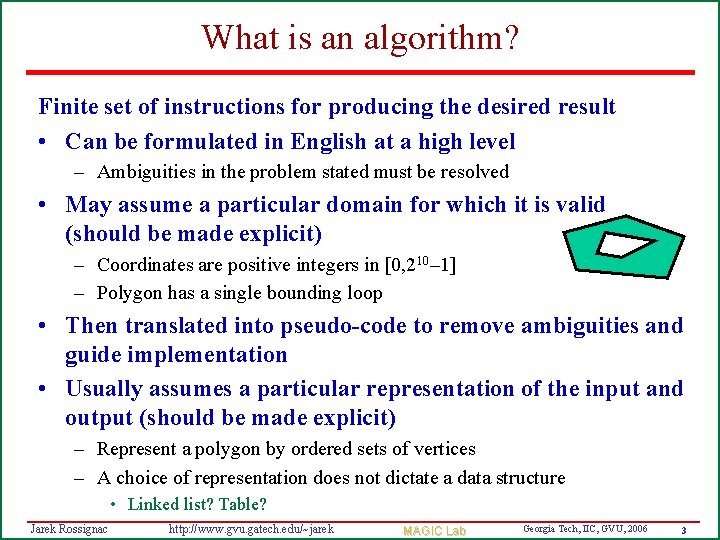
What is an algorithm? Finite set of instructions for producing the desired result • Can be formulated in English at a high level – Ambiguities in the problem stated must be resolved • May assume a particular domain for which it is valid (should be made explicit) – Coordinates are positive integers in [0, 210– 1] – Polygon has a single bounding loop • Then translated into pseudo-code to remove ambiguities and guide implementation • Usually assumes a particular representation of the input and output (should be made explicit) – Represent a polygon by ordered sets of vertices – A choice of representation does not dictate a data structure • Linked list? Table? Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 3
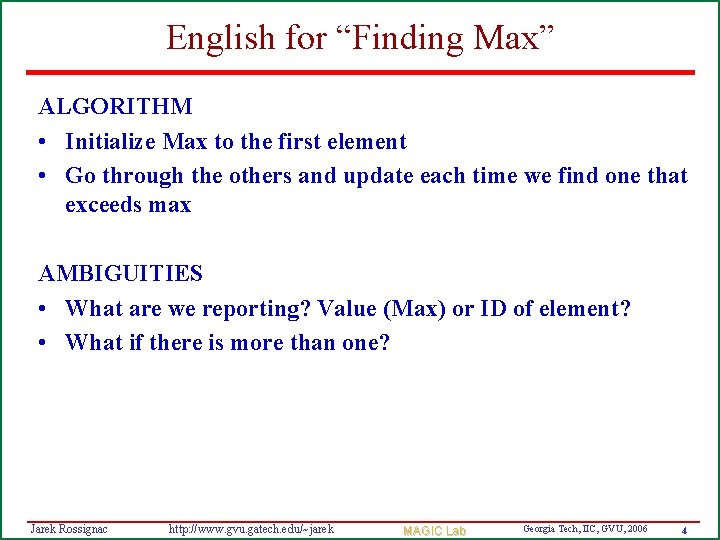
English for “Finding Max” ALGORITHM • Initialize Max to the first element • Go through the others and update each time we find one that exceeds max AMBIGUITIES • What are we reporting? Value (Max) or ID of element? • What if there is more than one? Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 4
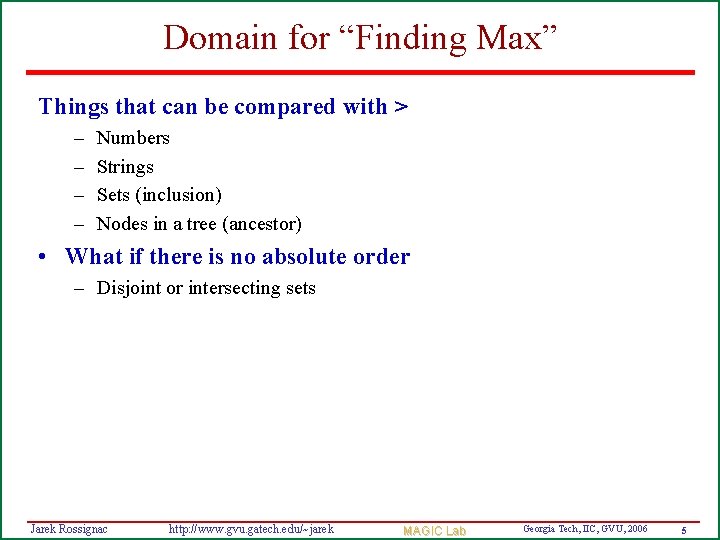
Domain for “Finding Max” Things that can be compared with > – – Numbers Strings Sets (inclusion) Nodes in a tree (ancestor) • What if there is no absolute order – Disjoint or intersecting sets Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 5
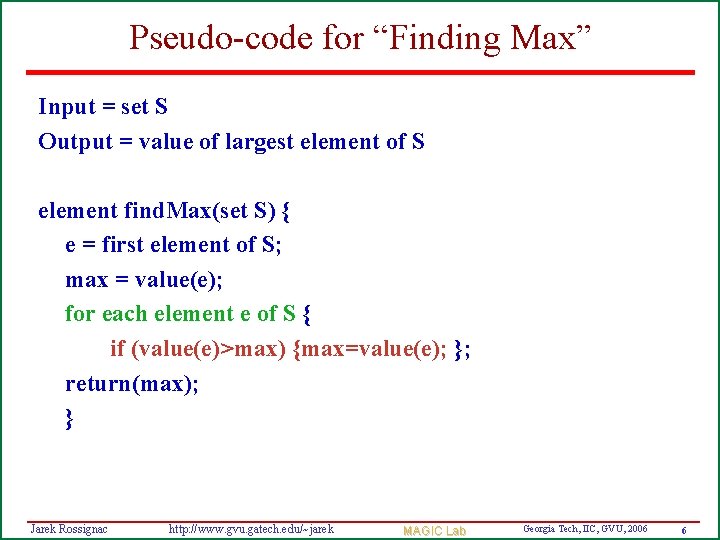
Pseudo-code for “Finding Max” Input = set S Output = value of largest element of S element find. Max(set S) { e = first element of S; max = value(e); for each element e of S { if (value(e)>max) {max=value(e); }; return(max); } Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 6
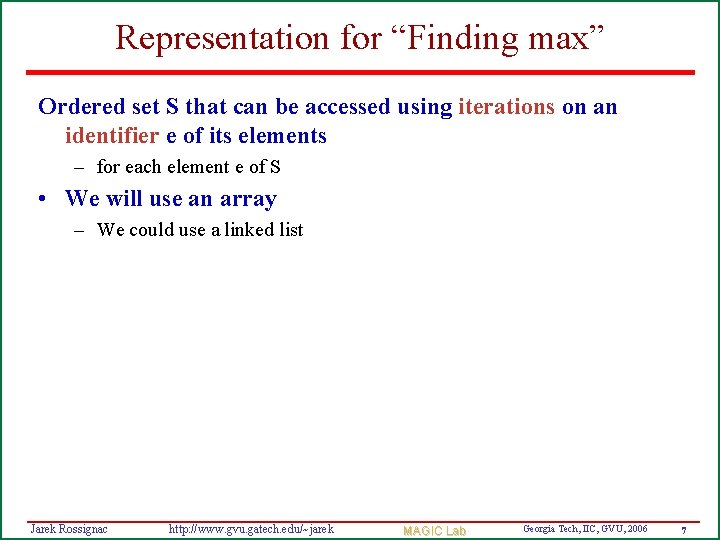
Representation for “Finding max” Ordered set S that can be accessed using iterations on an identifier e of its elements – for each element e of S • We will use an array – We could use a linked list Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 7
![Processing code for Finding Max int T new int 6 T04 T12 T25 Processing code for “Finding Max” int[] T = new int [6]; T[0]=4; T[1]=2; T[2]=5;](https://slidetodoc.com/presentation_image_h2/b3cb6ee1d0eeb4fab3a39a5a9ee18a66/image-8.jpg)
Processing code for “Finding Max” int[] T = new int [6]; T[0]=4; T[1]=2; T[2]=5; T[3]=1; T[4]=5; T[5]=3; int m=T[0]; for (int e=0; e<6; e++) { if (T[e]>m) {m=T[e]; }; }; println(m); Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 8
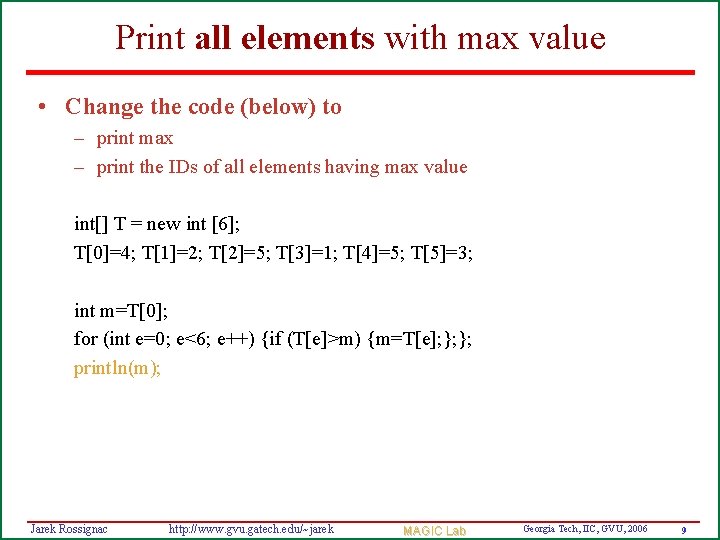
Print all elements with max value • Change the code (below) to – print max – print the IDs of all elements having max value int[] T = new int [6]; T[0]=4; T[1]=2; T[2]=5; T[3]=1; T[4]=5; T[5]=3; int m=T[0]; for (int e=0; e<6; e++) {if (T[e]>m) {m=T[e]; }; }; println(m); Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 9
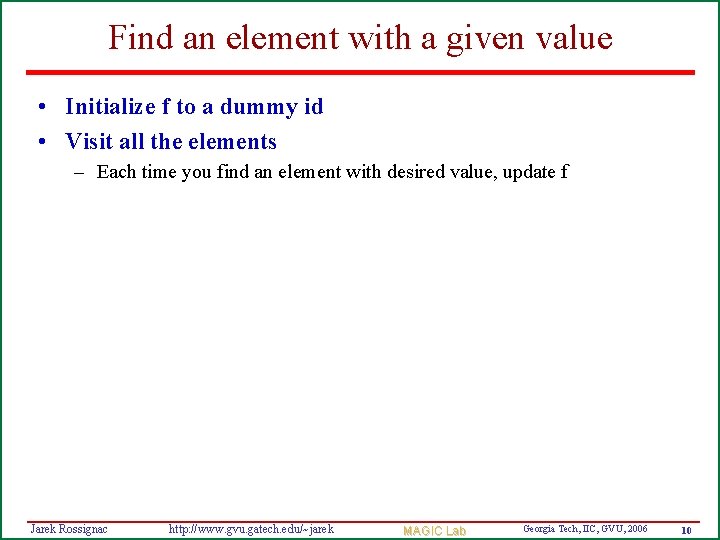
Find an element with a given value • Initialize f to a dummy id • Visit all the elements – Each time you find an element with desired value, update f Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 10
![Linear search what does it report float T new float 6 float Linear search (what does it report? ) float[] T = new float [6]; float](https://slidetodoc.com/presentation_image_h2/b3cb6ee1d0eeb4fab3a39a5a9ee18a66/image-11.jpg)
Linear search (what does it report? ) float[] T = new float [6]; float v=5; void setup() { size(300, 300); T[0]=1. 0; T[1]=2. 0; T[2]=4. 0; T[3]=4. 0; T[4]=5. 0; T[5]=6. 0; }; void draw(){}; void mouse. Pressed() { int f = linear. Search(v, 0, 6); if (f==-1) {println("No match"); } else {println("found "+v+" in T["+f+"]"); }; no. Loop(); }; int linear. Search(float pv, int pl, int ph) { int e= -1; for (int i=pl; i<ph; i++) {if (T[i]==pv) {e=i; }; }; return(e); }; Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 11
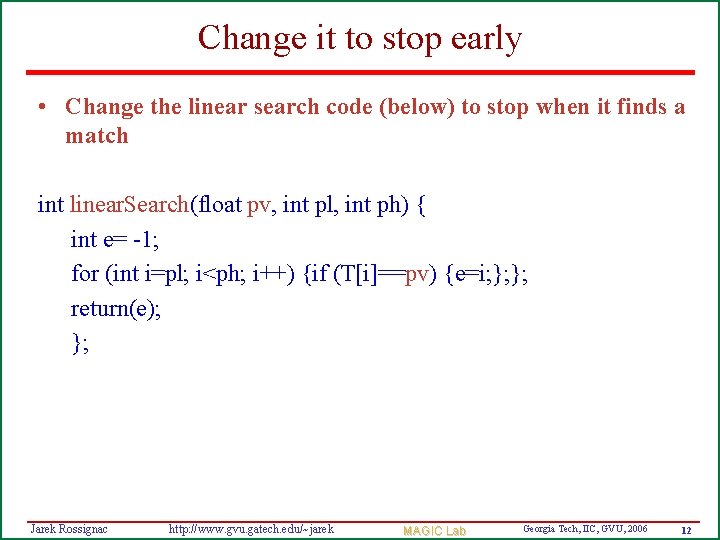
Change it to stop early • Change the linear search code (below) to stop when it finds a match int linear. Search(float pv, int pl, int ph) { int e= -1; for (int i=pl; i<ph; i++) {if (T[i]==pv) {e=i; }; }; return(e); }; Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 12
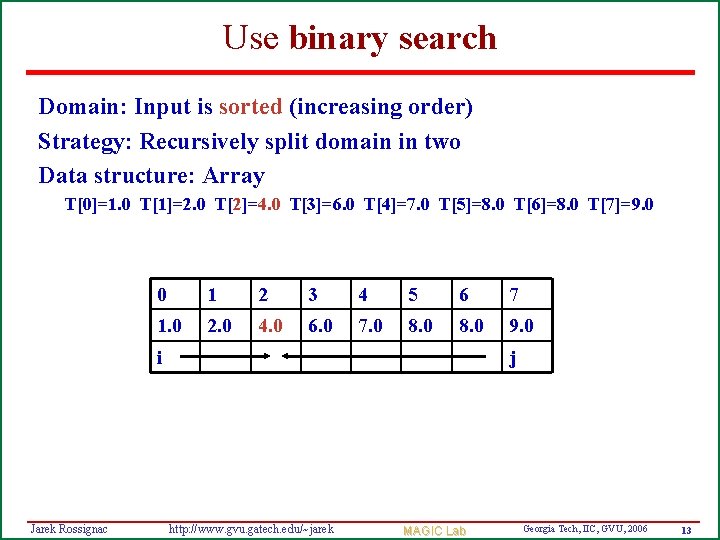
Use binary search Domain: Input is sorted (increasing order) Strategy: Recursively split domain in two Data structure: Array T[0]=1. 0 T[1]=2. 0 T[2]=4. 0 T[3]=6. 0 T[4]=7. 0 T[5]=8. 0 T[6]=8. 0 T[7]=9. 0 0 1 2 3 4 5 6 7 1. 0 2. 0 4. 0 6. 0 7. 0 8. 0 9. 0 i Jarek Rossignac j http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 13
![Example Binary search for v4 0 while ij mij2 if vTm im1 Example: Binary search for v=4. 0 while (i<j) { m=(i+j)/2; if (v>T[m]) {i=m+1; }](https://slidetodoc.com/presentation_image_h2/b3cb6ee1d0eeb4fab3a39a5a9ee18a66/image-14.jpg)
Example: Binary search for v=4. 0 while (i<j) { m=(i+j)/2; if (v>T[m]) {i=m+1; } else { j=m; } if (T[i]==v) { return(i); } else { return(-1); }; 0 1 2 3 4 5 6 7 1. 0 2. 0 4. 0 6. 0 7. 0 8. 0 9. 0 i m j 0 1 2 3 4 5 6 7 1. 0 2. 0 4. 0 6. 0 7. 0 8. 0 9. 0 i m 0 1 2 3 4 5 6 7 1. 0 2. 0 4. 0 6. 0 7. 0 8. 0 9. 0 i j j 0 1 2 3 4 5 6 7 1. 0 2. 0 4. 0 6. 0 7. 0 8. 0 9. 0 i=j Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 14
![Write the code for Binary search float T new float 6 float Write the code for Binary search ! float[] T = new float [6]; float](https://slidetodoc.com/presentation_image_h2/b3cb6ee1d0eeb4fab3a39a5a9ee18a66/image-15.jpg)
Write the code for Binary search ! float[] T = new float [6]; float v=4. 0; void setup() { size(300, 300); T[0]=1. 0; T[1]=2. 0; T[2]=4. 0; T[3]=6. 0; T[4]=7. 0; T[5]=9. 0; }; void draw(){}; void mouse. Pressed() { int f = binary. Search(v, 0, 6); if (f==-1) {println(" No match"); } else {println("found "+v+" in T["+f+"]"); }; no. Loop(); }; int binary. Search(float pv, int pl, int ph) { int f= -1; ? ? ? return(f); }; Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 15
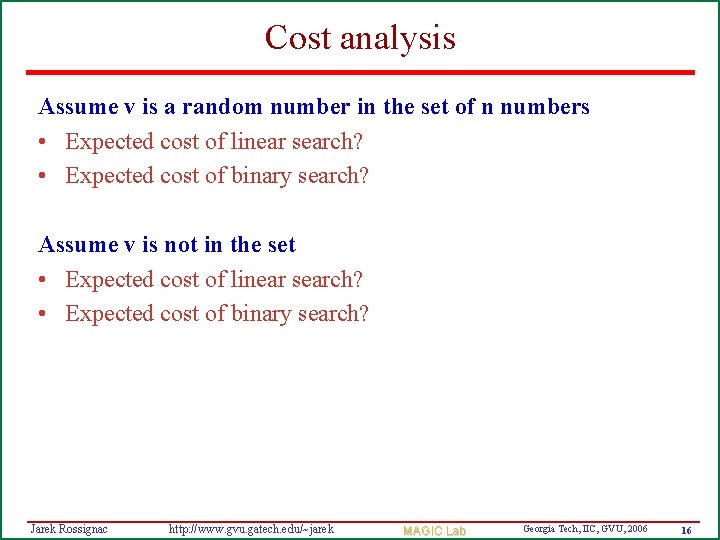
Cost analysis Assume v is a random number in the set of n numbers • Expected cost of linear search? • Expected cost of binary search? Assume v is not in the set • Expected cost of linear search? • Expected cost of binary search? Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 16
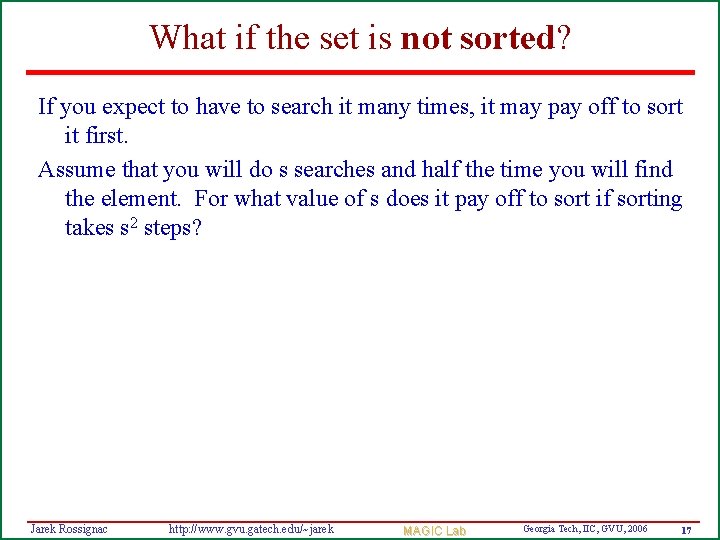
What if the set is not sorted? If you expect to have to search it many times, it may pay off to sort it first. Assume that you will do s searches and half the time you will find the element. For what value of s does it pay off to sort if sorting takes s 2 steps? Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 17
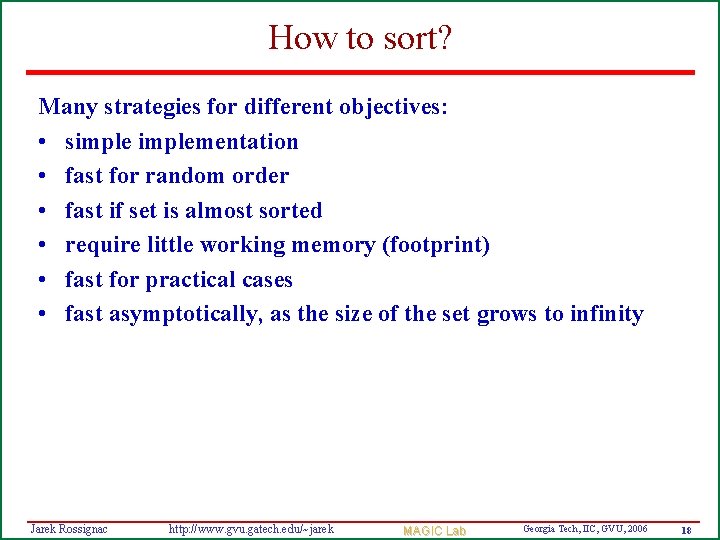
How to sort? Many strategies for different objectives: • simplementation • fast for random order • fast if set is almost sorted • require little working memory (footprint) • fast for practical cases • fast asymptotically, as the size of the set grows to infinity Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 18
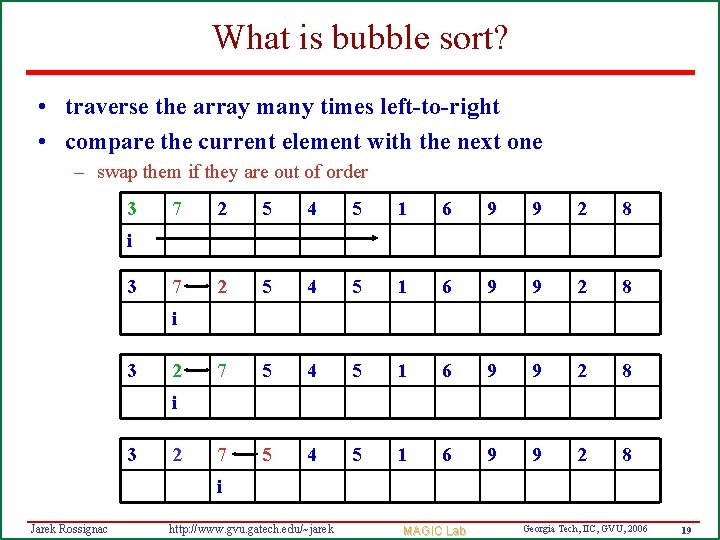
What is bubble sort? • traverse the array many times left-to-right • compare the current element with the next one – swap them if they are out of order 3 7 2 5 4 5 1 6 9 9 2 8 7 5 4 5 1 6 9 9 2 8 i 3 2 i Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 19
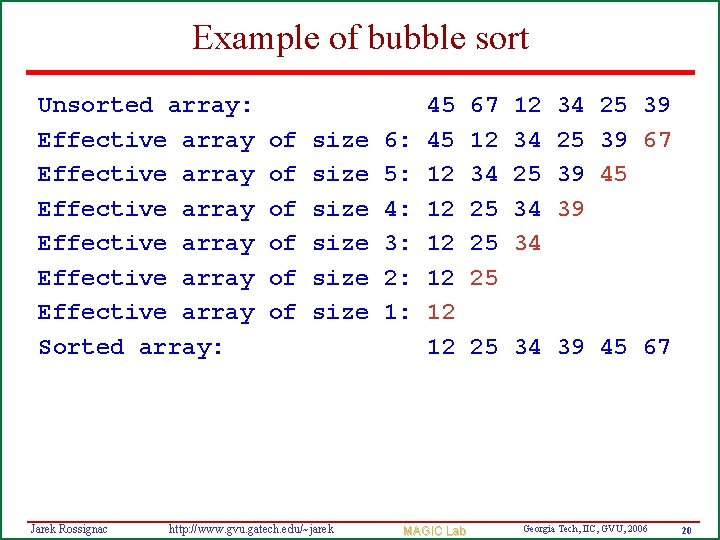
Example of bubble sort Unsorted array: Effective array Effective array Sorted array: Jarek Rossignac of of of size size http: //www. gvu. gatech. edu/~jarek 6: 5: 4: 3: 2: 1: 45 45 12 12 12 MAGIC Lab 67 12 34 25 25 25 12 34 25 34 34 34 25 39 67 39 45 39 25 34 39 45 67 Georgia Tech, IIC, GVU, 2006 20
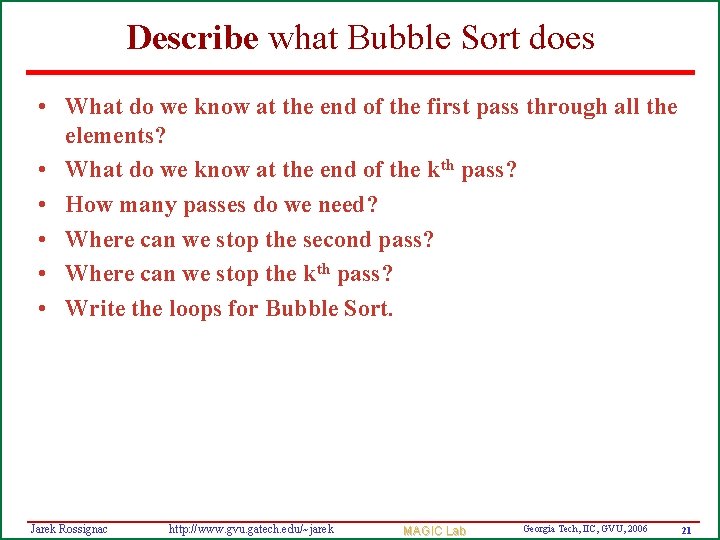
Describe what Bubble Sort does • What do we know at the end of the first pass through all the elements? • What do we know at the end of the kth pass? • How many passes do we need? • Where can we stop the second pass? • Where can we stop the kth pass? • Write the loops for Bubble Sort. Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 21
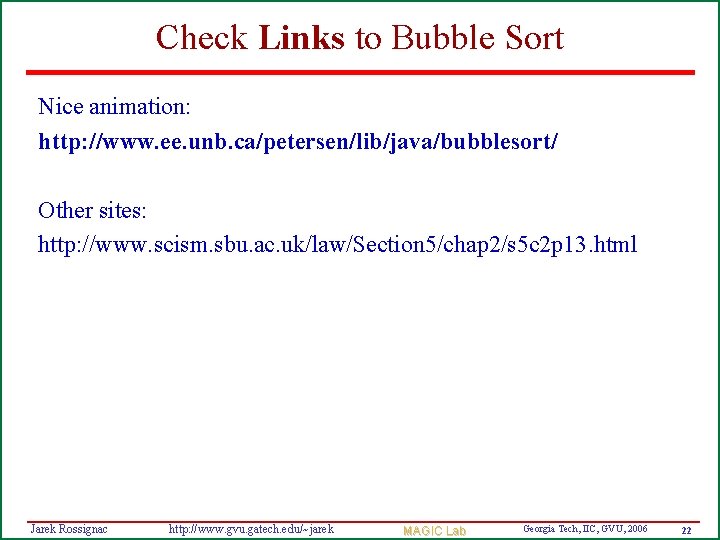
Check Links to Bubble Sort Nice animation: http: //www. ee. unb. ca/petersen/lib/java/bubblesort/ Other sites: http: //www. scism. sbu. ac. uk/law/Section 5/chap 2/s 5 c 2 p 13. html Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 22
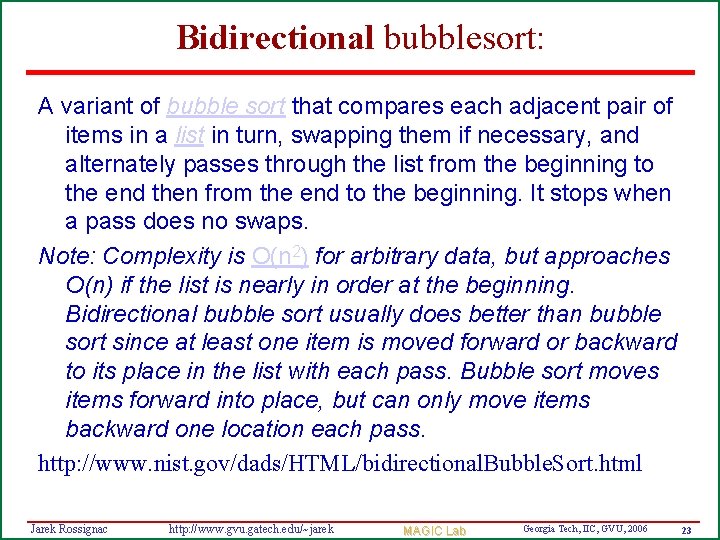
Bidirectional bubblesort: A variant of bubble sort that compares each adjacent pair of items in a list in turn, swapping them if necessary, and alternately passes through the list from the beginning to the end then from the end to the beginning. It stops when a pass does no swaps. Note: Complexity is O(n 2) for arbitrary data, but approaches O(n) if the list is nearly in order at the beginning. Bidirectional bubble sort usually does better than bubble sort since at least one item is moved forward or backward to its place in the list with each pass. Bubble sort moves items forward into place, but can only move items backward one location each pass. http: //www. nist. gov/dads/HTML/bidirectional. Bubble. Sort. html Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 23
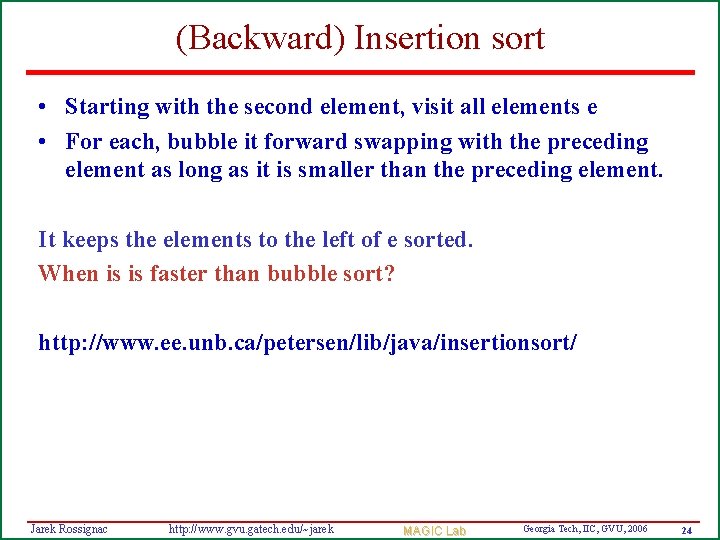
(Backward) Insertion sort • Starting with the second element, visit all elements e • For each, bubble it forward swapping with the preceding element as long as it is smaller than the preceding element. It keeps the elements to the left of e sorted. When is is faster than bubble sort? http: //www. ee. unb. ca/petersen/lib/java/insertionsort/ Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 24
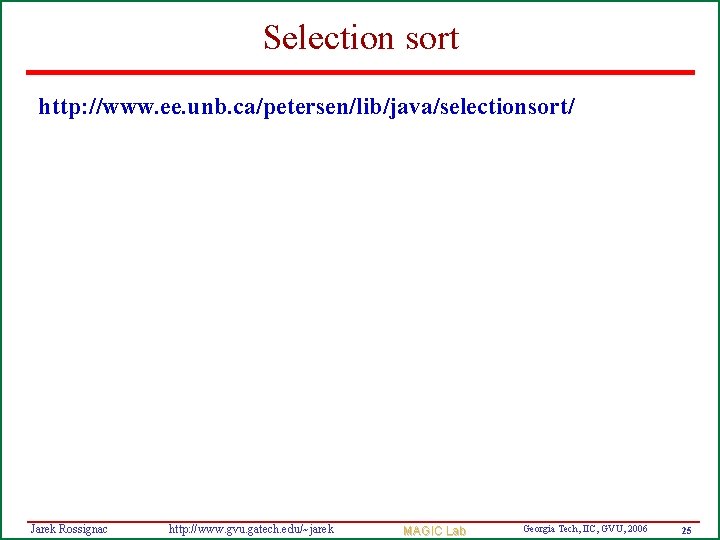
Selection sort http: //www. ee. unb. ca/petersen/lib/java/selectionsort/ Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 25
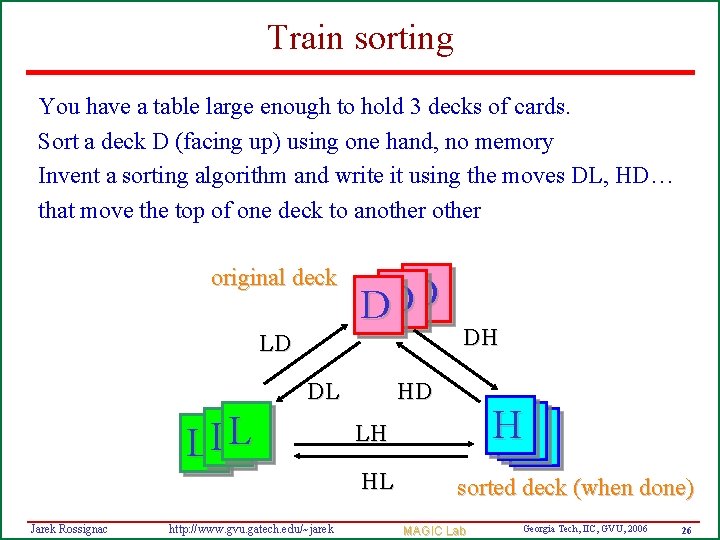
Train sorting You have a table large enough to hold 3 decks of cards. Sort a deck D (facing up) using one hand, no memory Invent a sorting algorithm and write it using the moves DL, HD… that move the top of one deck to another original deck DDD LD DL LLL HD http: //www. gvu. gatech. edu/~jarek HHH LH HL Jarek Rossignac DH sorted deck (when done) MAGIC Lab Georgia Tech, IIC, GVU, 2006 26
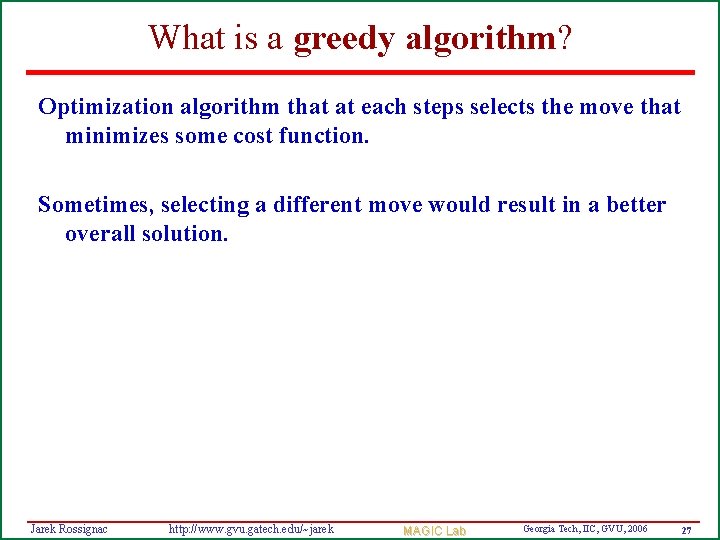
What is a greedy algorithm? Optimization algorithm that at each steps selects the move that minimizes some cost function. Sometimes, selecting a different move would result in a better overall solution. Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 27
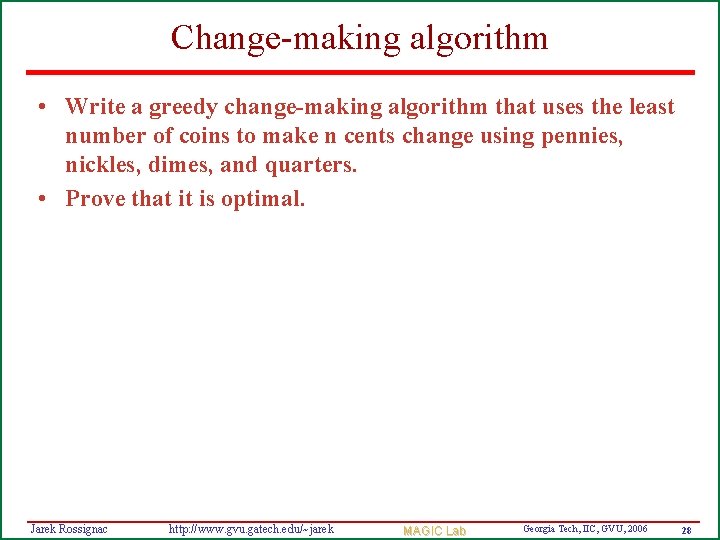
Change-making algorithm • Write a greedy change-making algorithm that uses the least number of coins to make n cents change using pennies, nickles, dimes, and quarters. • Prove that it is optimal. Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 28
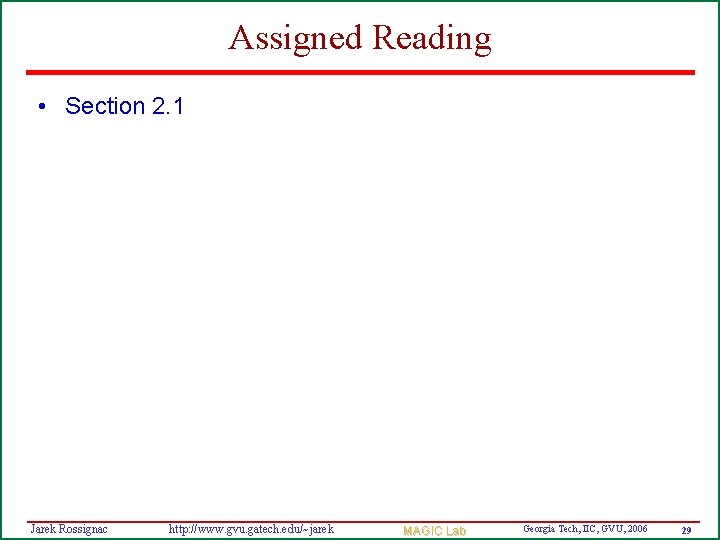
Assigned Reading • Section 2. 1 Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 29
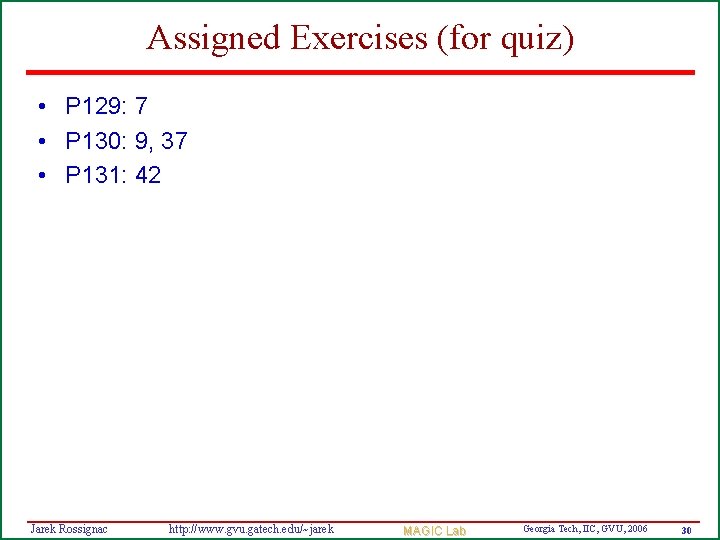
Assigned Exercises (for quiz) • P 129: 7 • P 130: 9, 37 • P 131: 42 Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 30
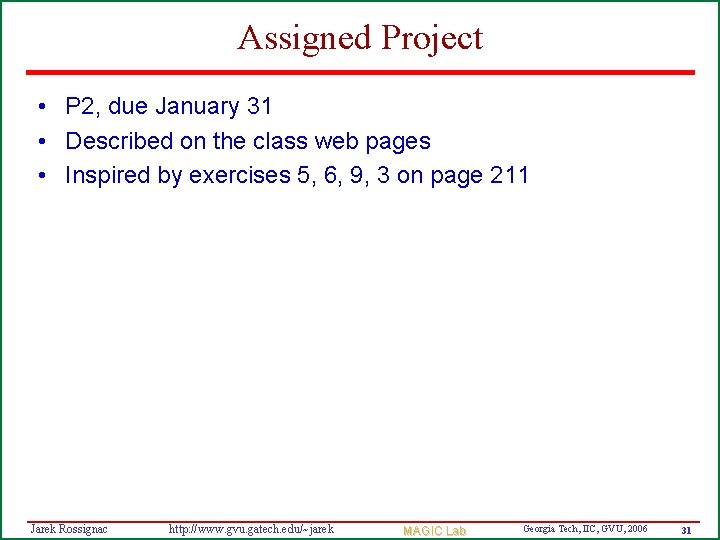
Assigned Project • P 2, due January 31 • Described on the class web pages • Inspired by exercises 5, 6, 9, 3 on page 211 Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 31
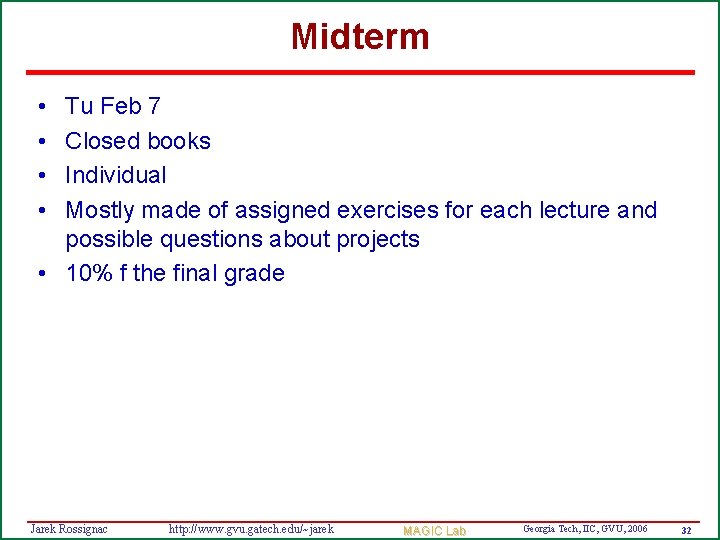
Midterm • • Tu Feb 7 Closed books Individual Mostly made of assigned exercises for each lecture and possible questions about projects • 10% f the final grade Jarek Rossignac http: //www. gvu. gatech. edu/~jarek MAGIC Lab Georgia Tech, IIC, GVU, 2006 32