CPSC 441 UDP Socket Programming Department of Computer
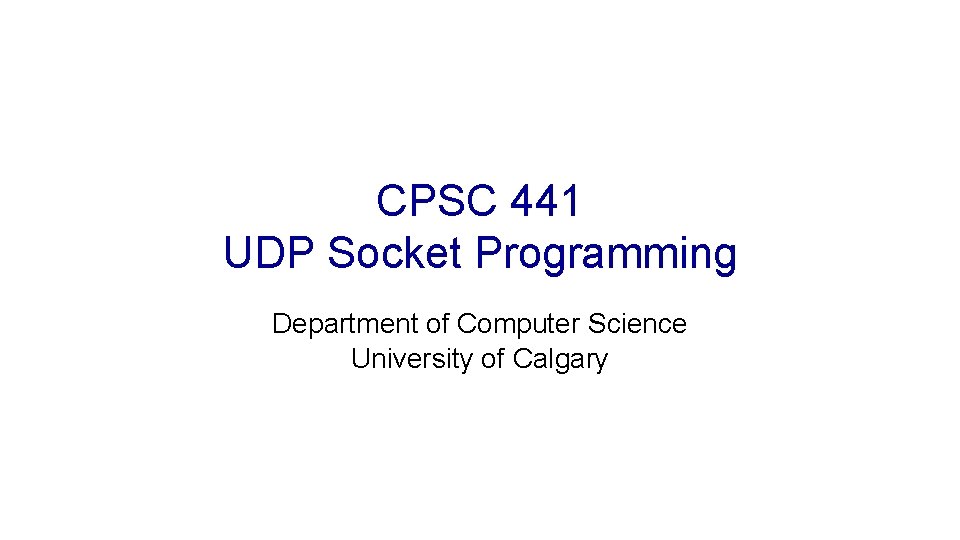
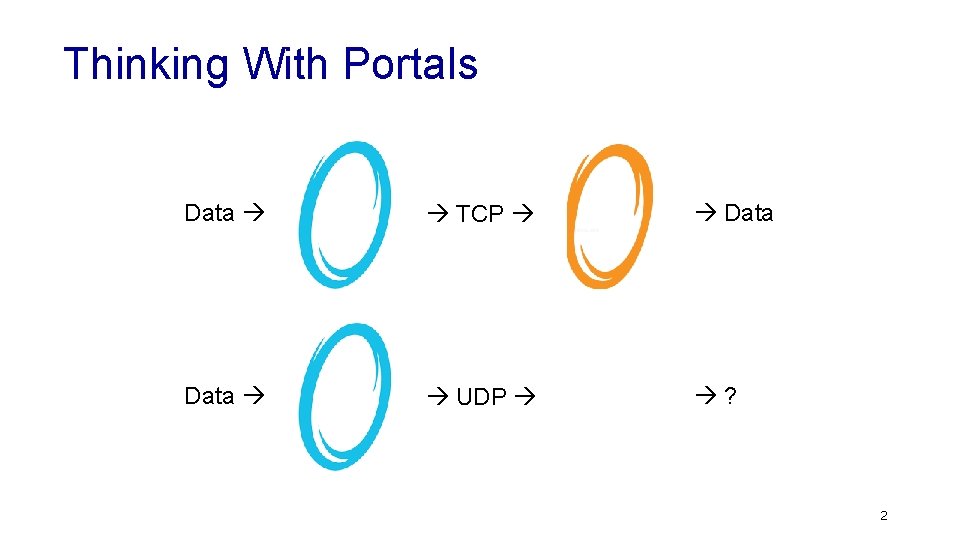
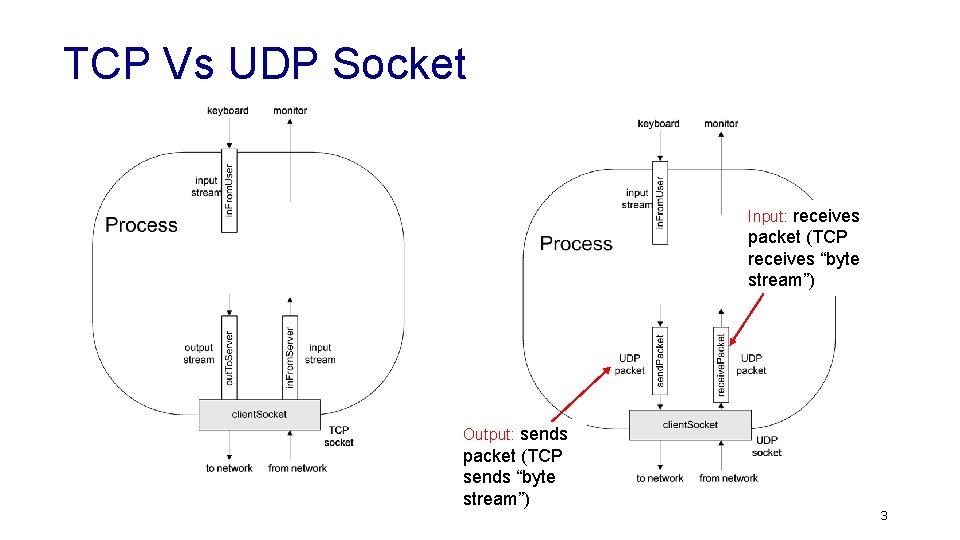
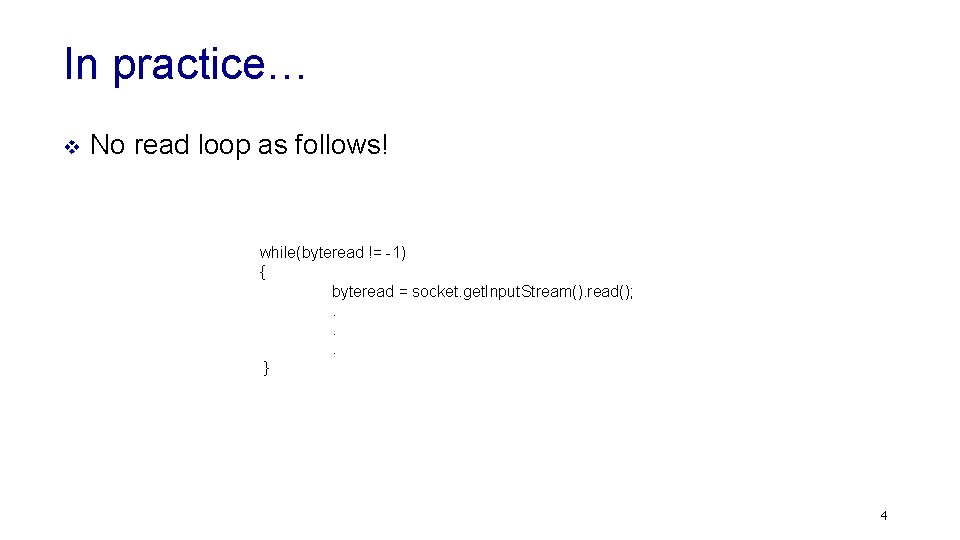
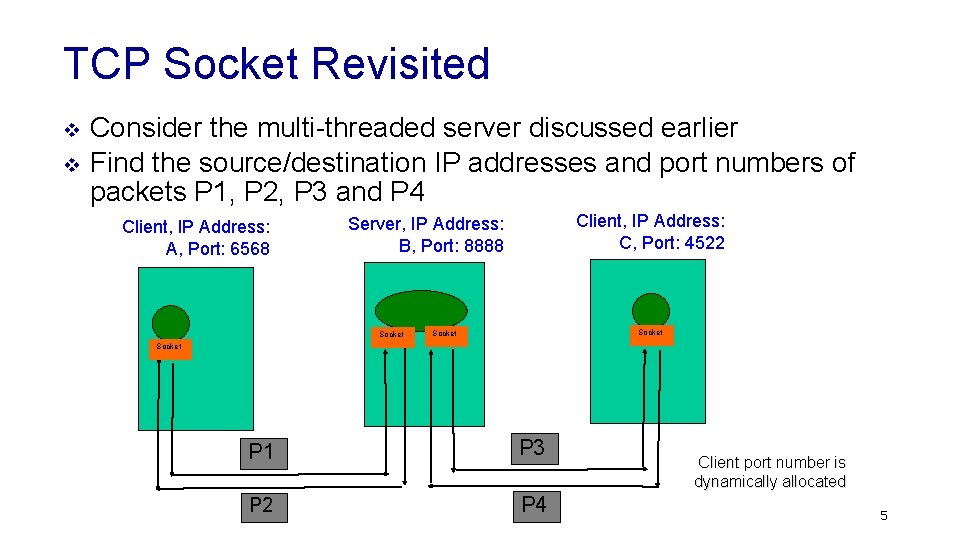
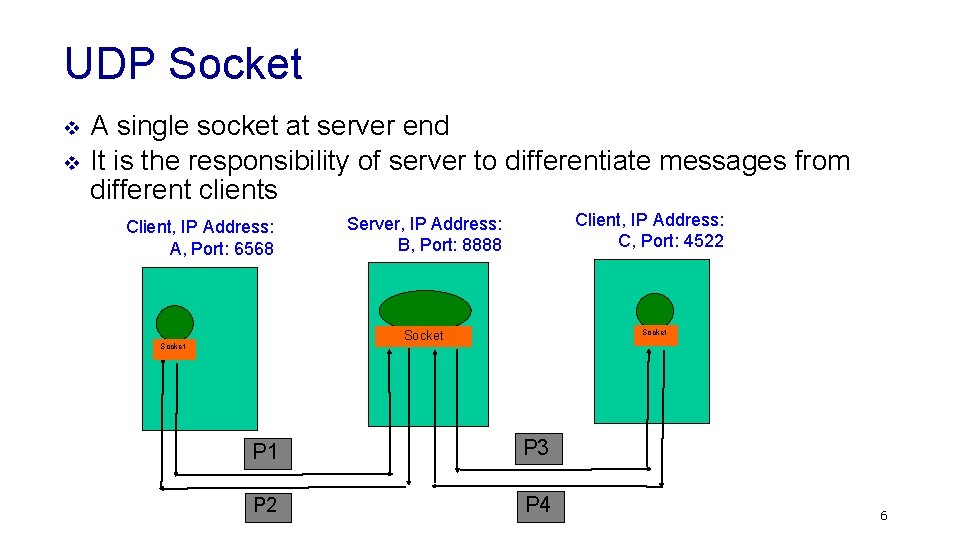
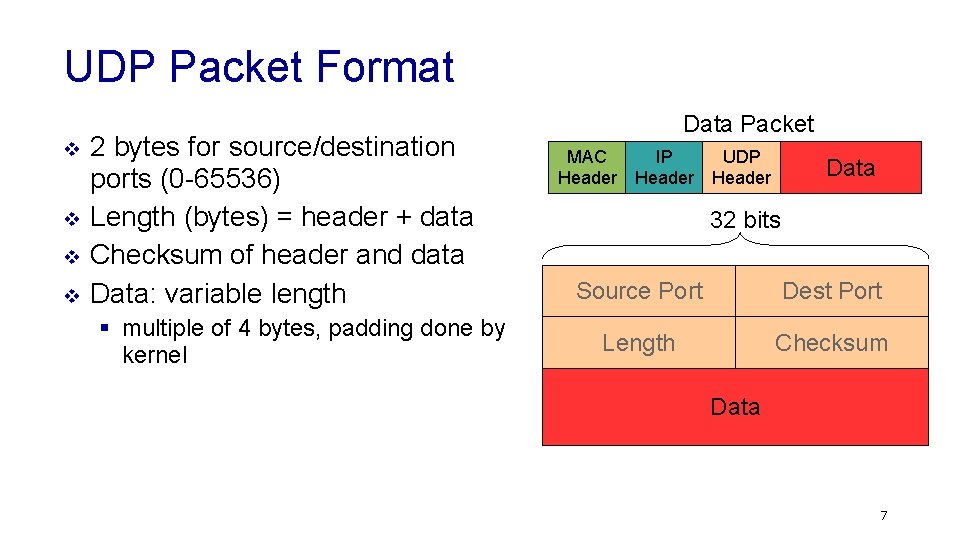
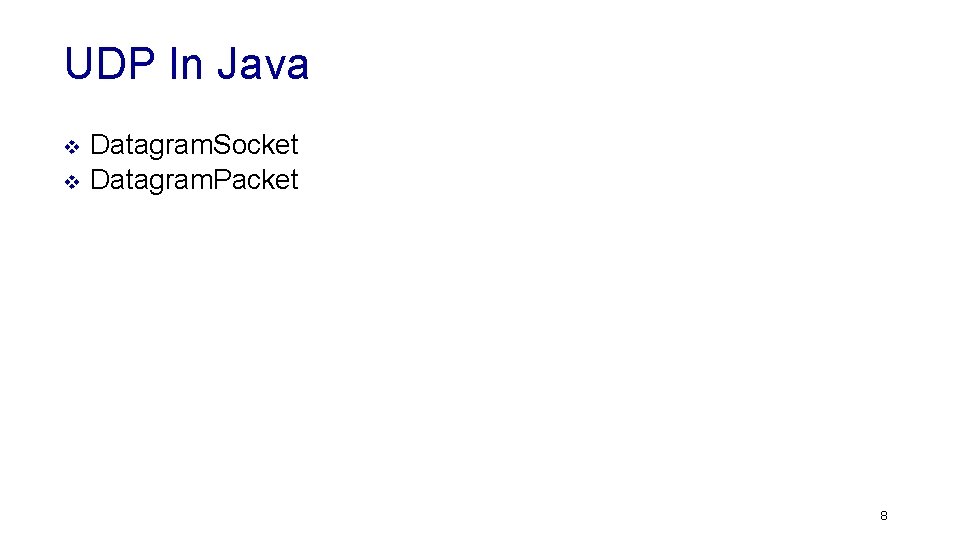
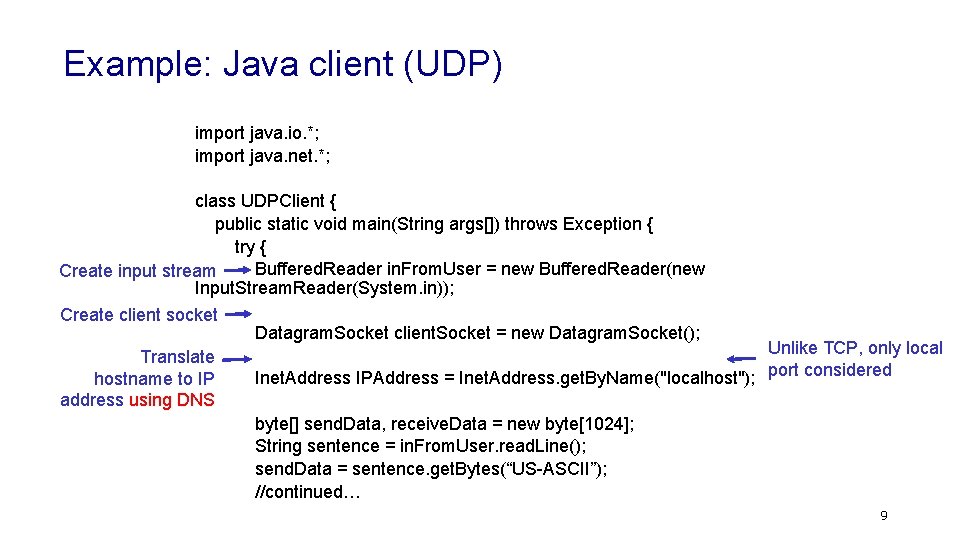
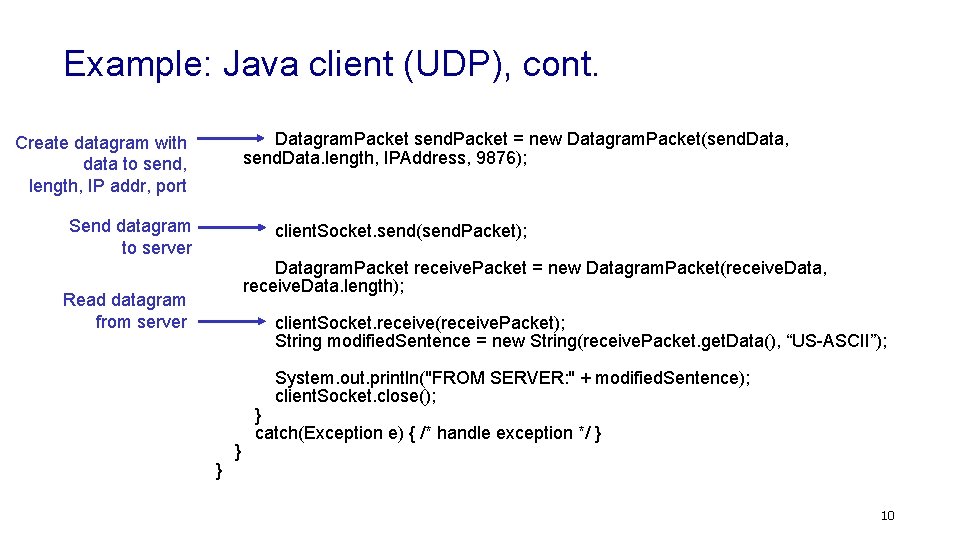
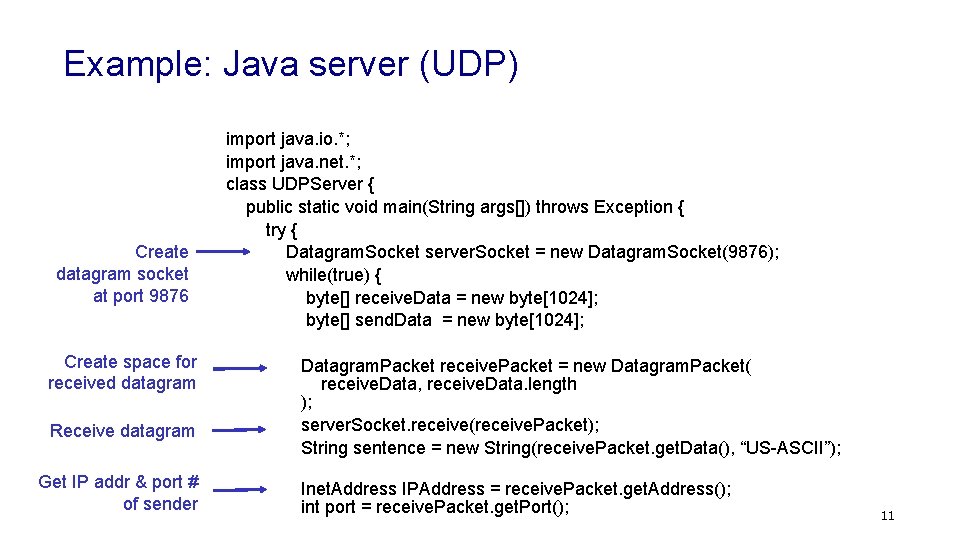
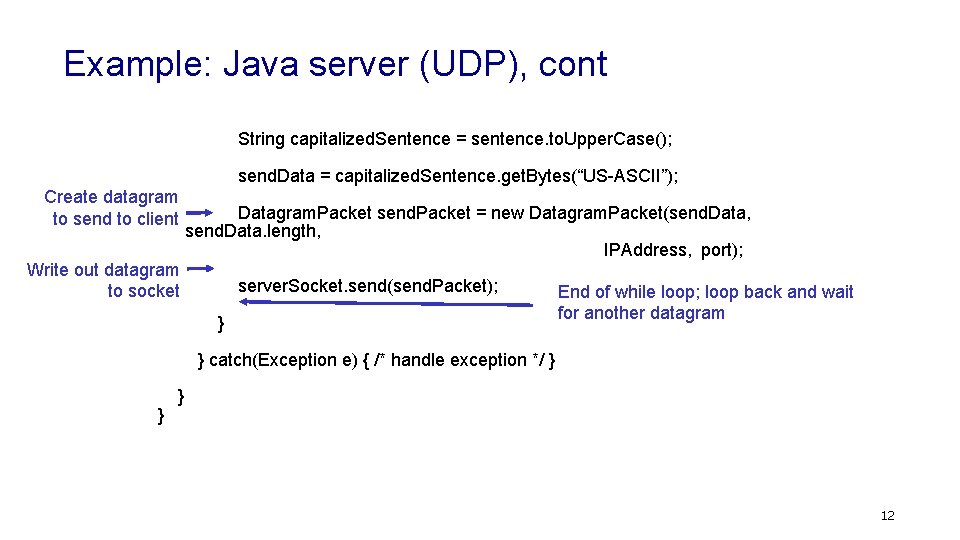
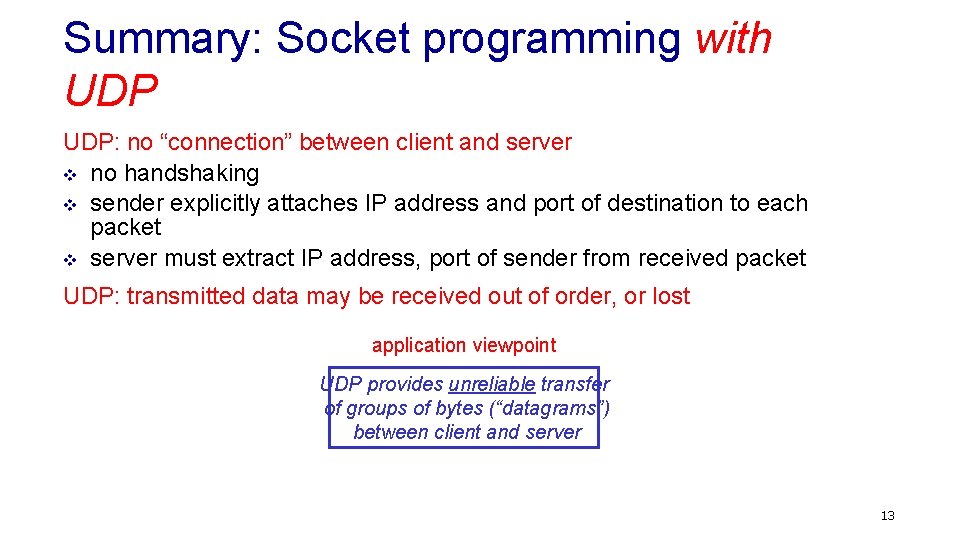
- Slides: 13
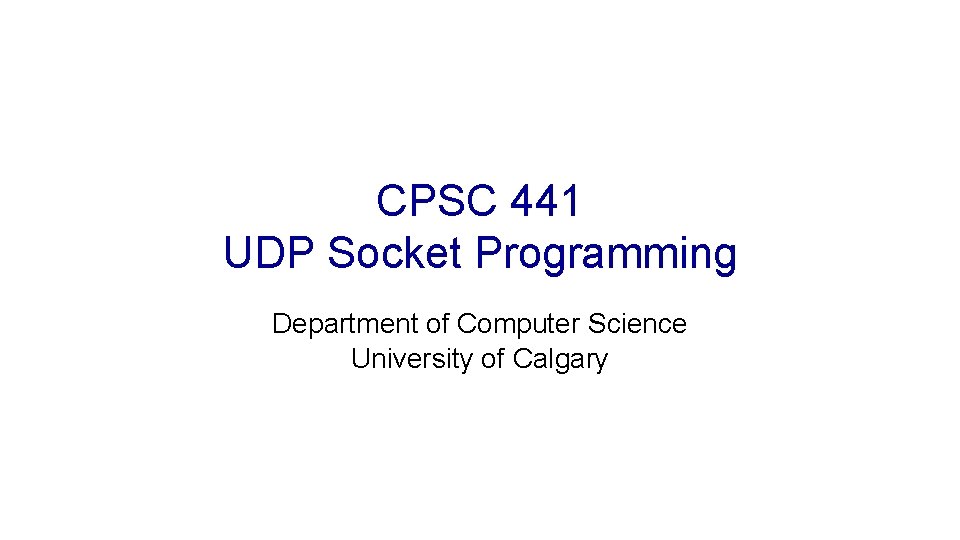
CPSC 441 UDP Socket Programming Department of Computer Science University of Calgary
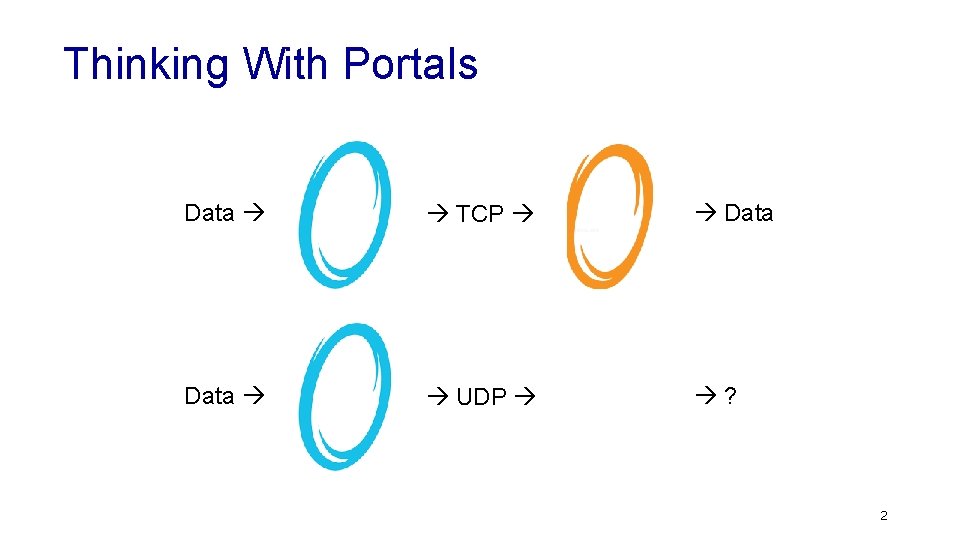
Thinking With Portals Data TCP Data UDP ? 2
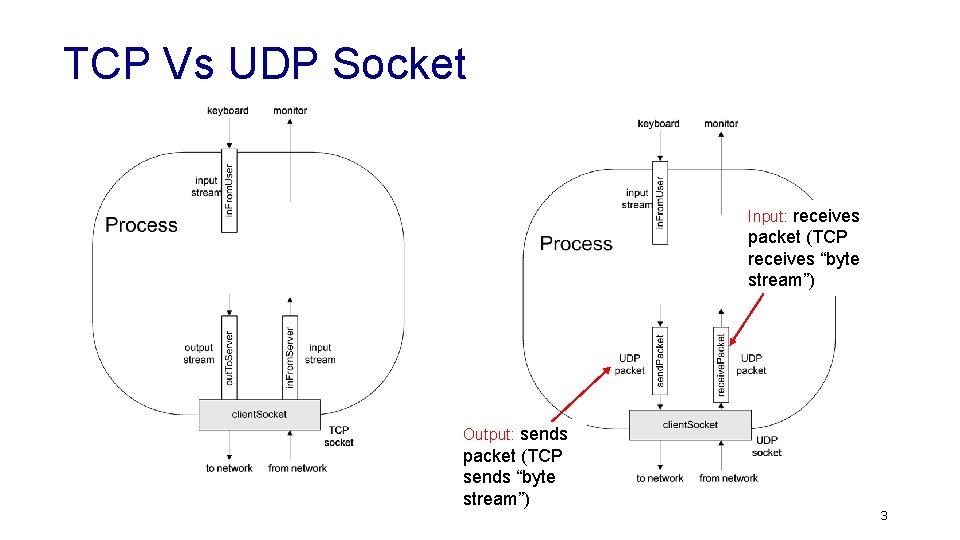
TCP Vs UDP Socket Input: receives packet (TCP receives “byte stream”) Output: sends packet (TCP sends “byte stream”) 3
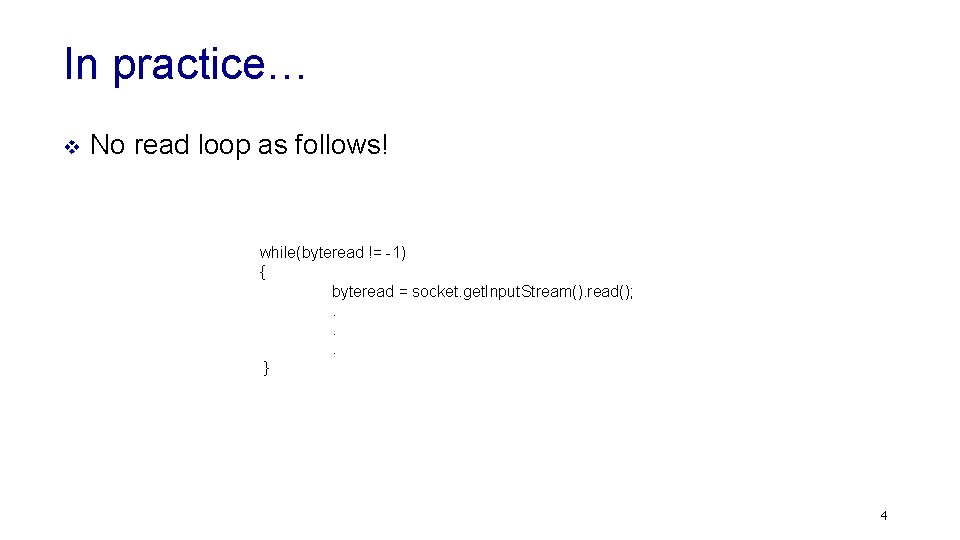
In practice… v No read loop as follows! while(byteread != -1) { byteread = socket. get. Input. Stream(). read(); . . . } 4
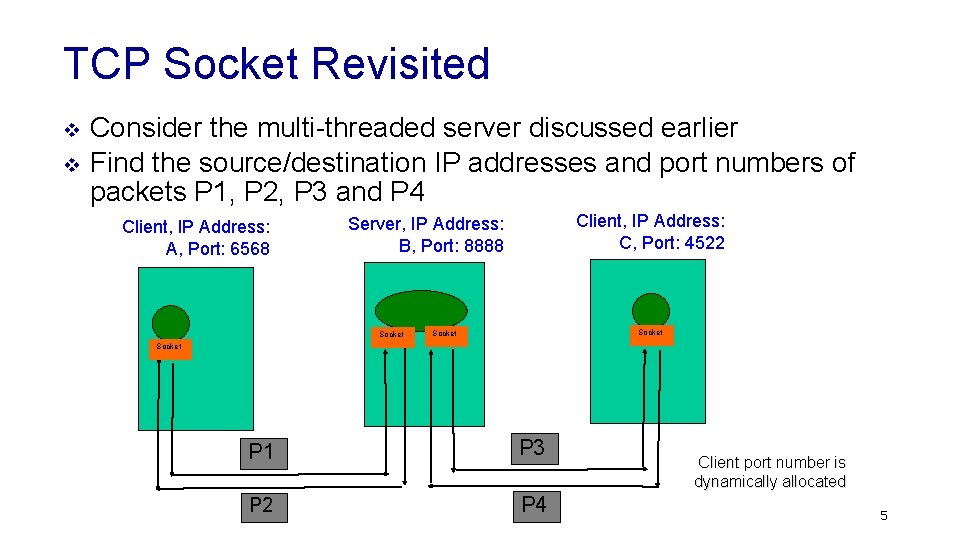
TCP Socket Revisited v v Consider the multi-threaded server discussed earlier Find the source/destination IP addresses and port numbers of packets P 1, P 2, P 3 and P 4 Client, IP Address: A, Port: 6568 Client, IP Address: C, Port: 4522 Server, IP Address: B, Port: 8888 Socket P 1 P 3 P 2 P 4 Client port number is dynamically allocated 5
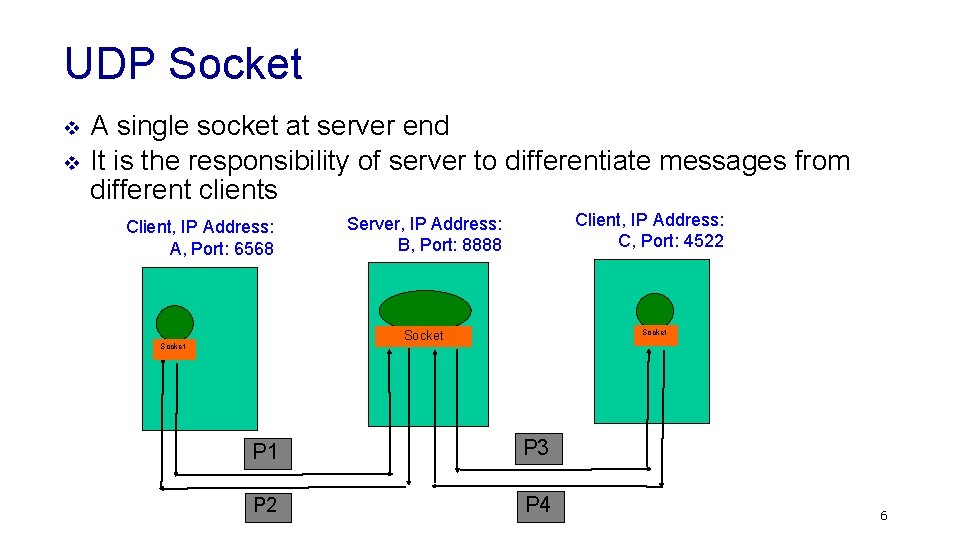
UDP Socket v v A single socket at server end It is the responsibility of server to differentiate messages from different clients Client, IP Address: A, Port: 6568 Client, IP Address: C, Port: 4522 Server, IP Address: B, Port: 8888 Socket P 1 P 3 P 2 P 4 6
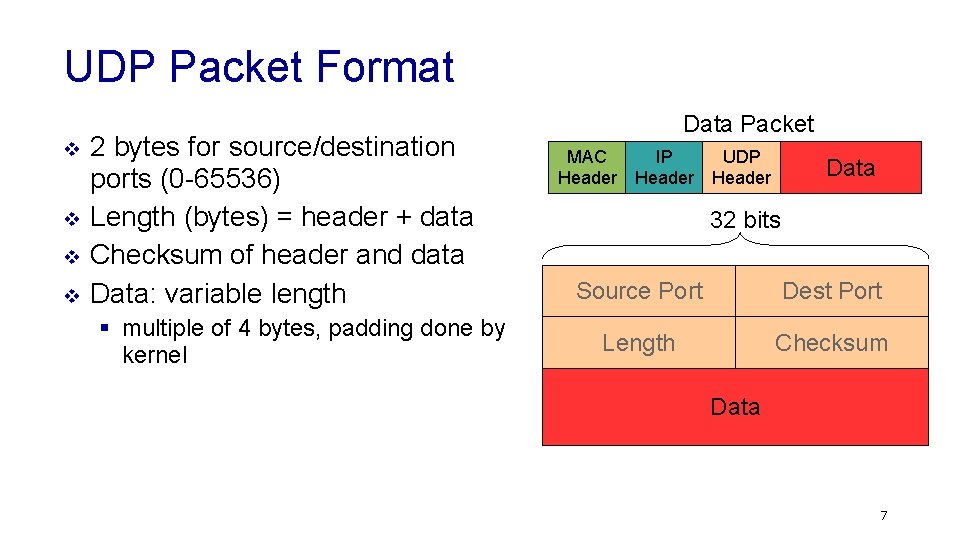
UDP Packet Format v v 2 bytes for source/destination ports (0 -65536) Length (bytes) = header + data Checksum of header and data Data: variable length § multiple of 4 bytes, padding done by kernel Data Packet MAC Header IP Header UDP Header Data 32 bits Source Port Dest Port Length Checksum Data 7
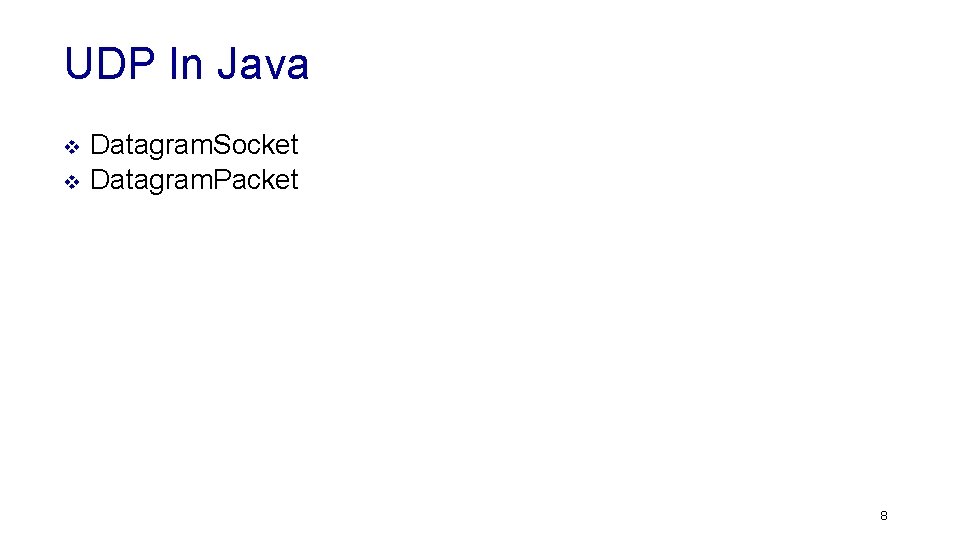
UDP In Java v v Datagram. Socket Datagram. Packet 8
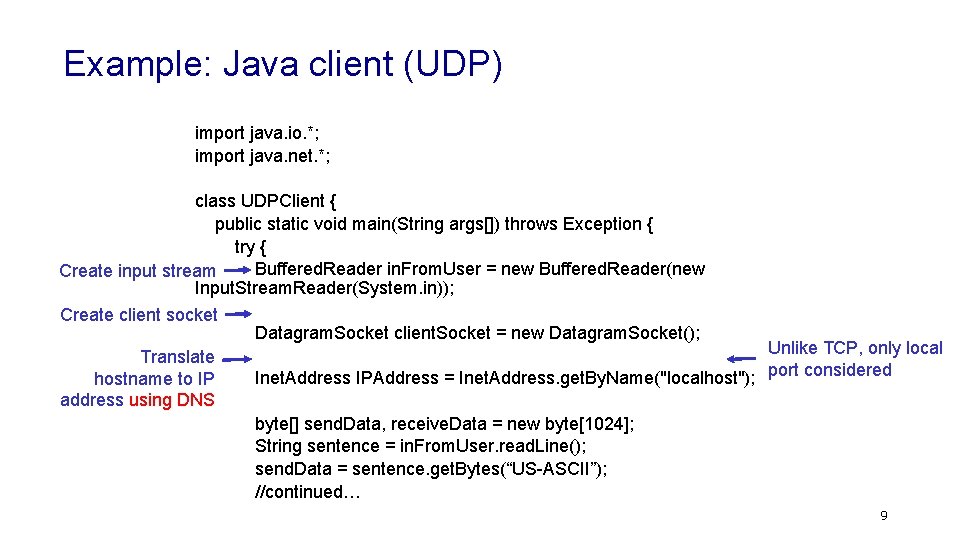
Example: Java client (UDP) import java. io. *; import java. net. *; class UDPClient { public static void main(String args[]) throws Exception { try { Buffered. Reader in. From. User = new Buffered. Reader(new Create input stream Input. Stream. Reader(System. in)); Create client socket Translate hostname to IP address using DNS Datagram. Socket client. Socket = new Datagram. Socket(); Unlike TCP, only local Inet. Address IPAddress = Inet. Address. get. By. Name("localhost"); port considered byte[] send. Data, receive. Data = new byte[1024]; String sentence = in. From. User. read. Line(); send. Data = sentence. get. Bytes(“US-ASCII”); //continued… 9
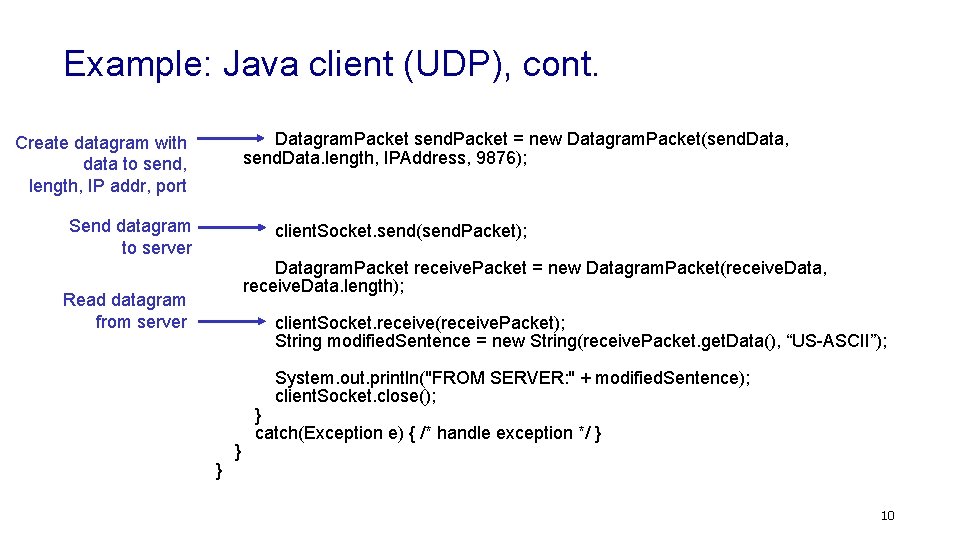
Example: Java client (UDP), cont. Datagram. Packet send. Packet = new Datagram. Packet(send. Data, send. Data. length, IPAddress, 9876); Create datagram with data to send, length, IP addr, port Send datagram to server client. Socket. send(send. Packet); Datagram. Packet receive. Packet = new Datagram. Packet(receive. Data, receive. Data. length); Read datagram from server client. Socket. receive(receive. Packet); String modified. Sentence = new String(receive. Packet. get. Data(), “US-ASCII”); System. out. println("FROM SERVER: " + modified. Sentence); client. Socket. close(); } } } catch(Exception e) { /* handle exception */ } 10
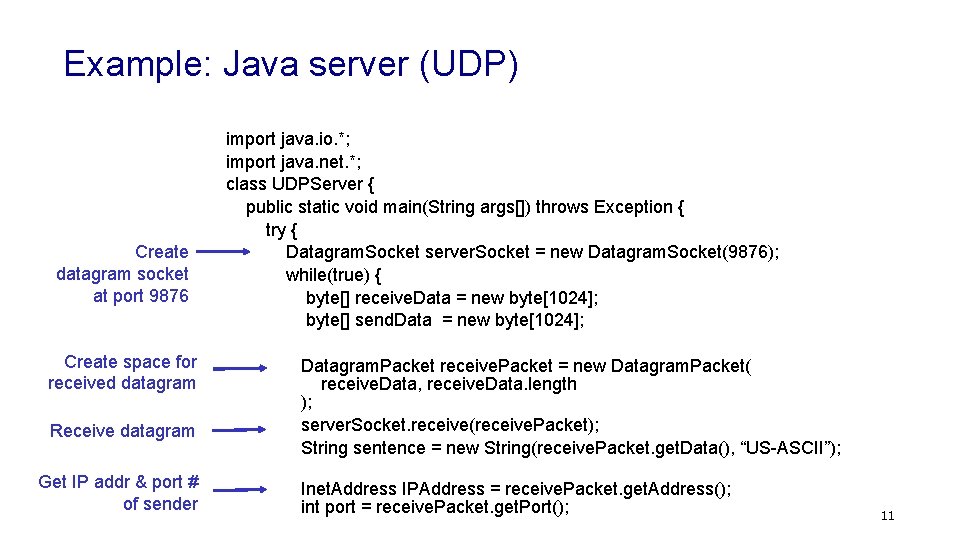
Example: Java server (UDP) Create datagram socket at port 9876 Create space for received datagram Receive datagram Get IP addr & port # of sender import java. io. *; import java. net. *; class UDPServer { public static void main(String args[]) throws Exception { try { Datagram. Socket server. Socket = new Datagram. Socket(9876); while(true) { byte[] receive. Data = new byte[1024]; byte[] send. Data = new byte[1024]; Datagram. Packet receive. Packet = new Datagram. Packet( receive. Data, receive. Data. length ); server. Socket. receive(receive. Packet); String sentence = new String(receive. Packet. get. Data(), “US-ASCII”); Inet. Address IPAddress = receive. Packet. get. Address(); int port = receive. Packet. get. Port(); 11
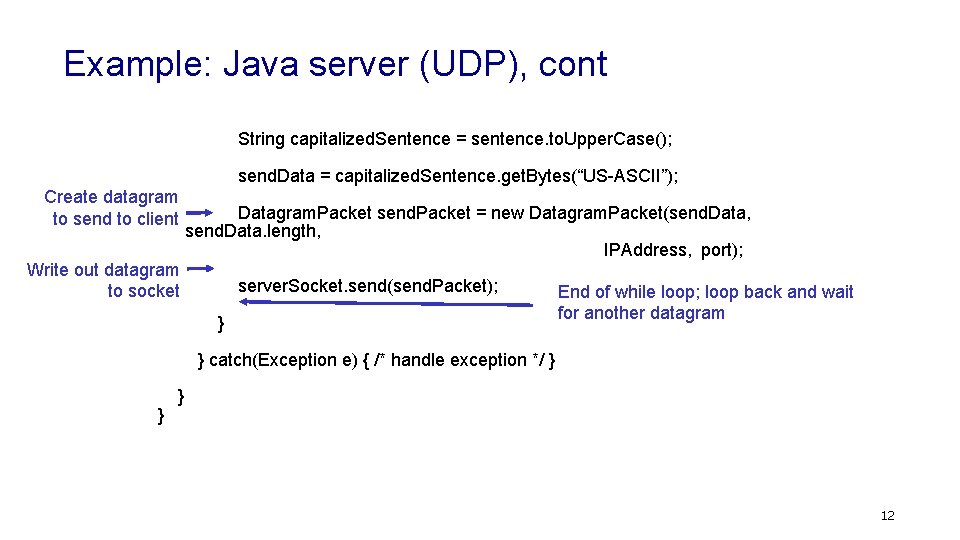
Example: Java server (UDP), cont String capitalized. Sentence = sentence. to. Upper. Case(); send. Data = capitalized. Sentence. get. Bytes(“US-ASCII”); Create datagram to send to client Datagram. Packet send. Packet = new Datagram. Packet(send. Data, send. Data. length, IPAddress, port); Write out datagram to socket server. Socket. send(send. Packet); } End of while loop; loop back and wait for another datagram } catch(Exception e) { /* handle exception */ } } } 12
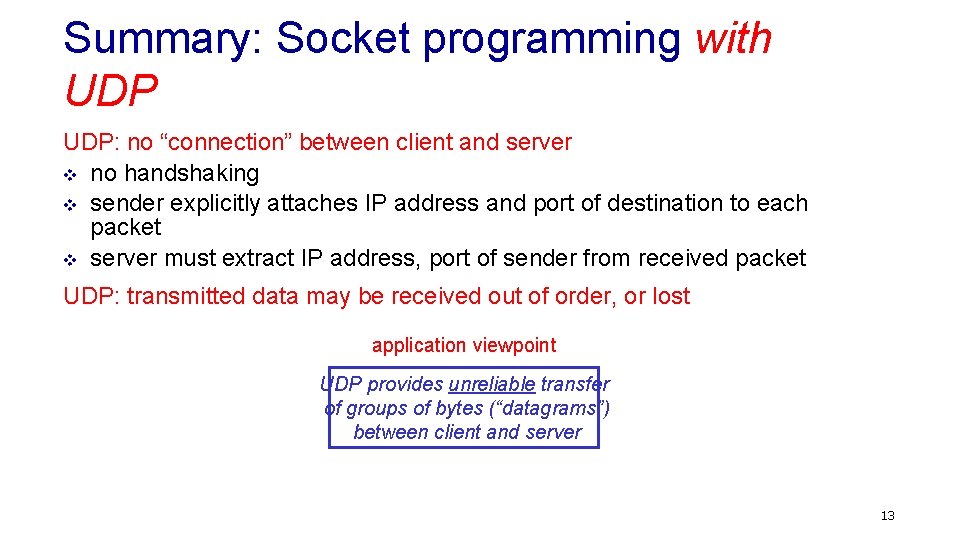
Summary: Socket programming with UDP: no “connection” between client and server v no handshaking v sender explicitly attaches IP address and port of destination to each packet v server must extract IP address, port of sender from received packet UDP: transmitted data may be received out of order, or lost application viewpoint UDP provides unreliable transfer of groups of bytes (“datagrams”) between client and server 13