Course Overview Mooly Sagiv msagivpost tau ac il
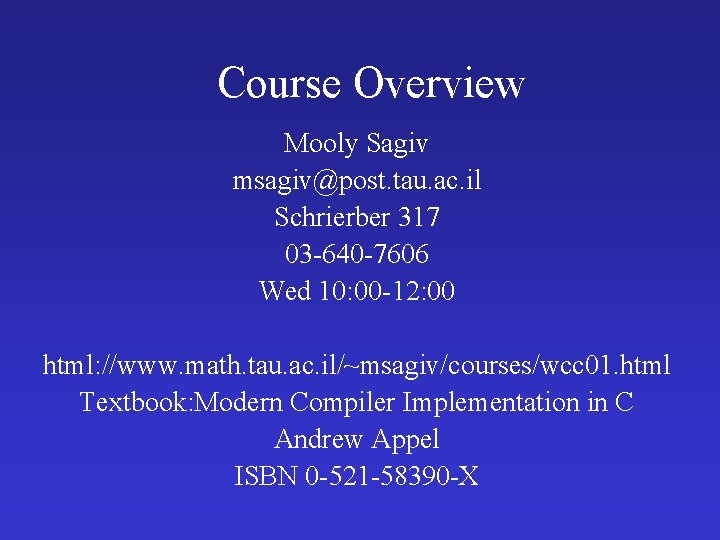
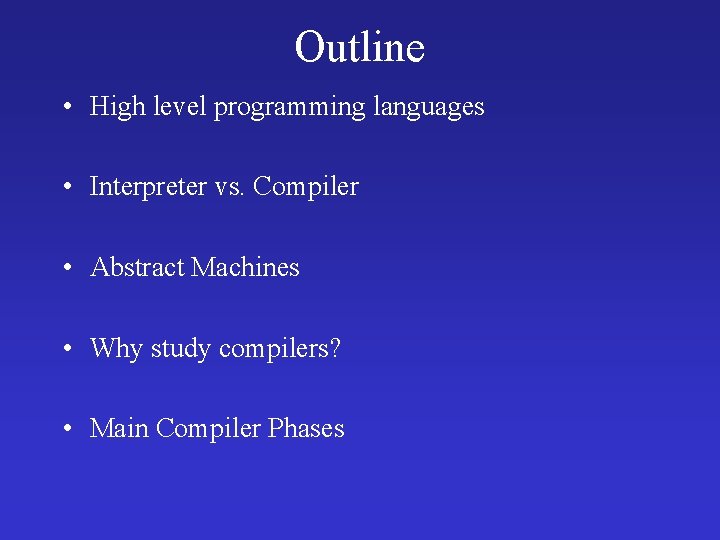
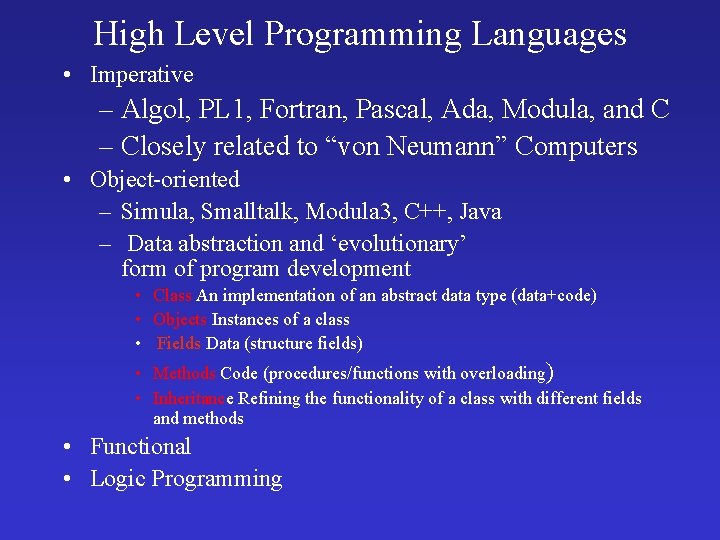
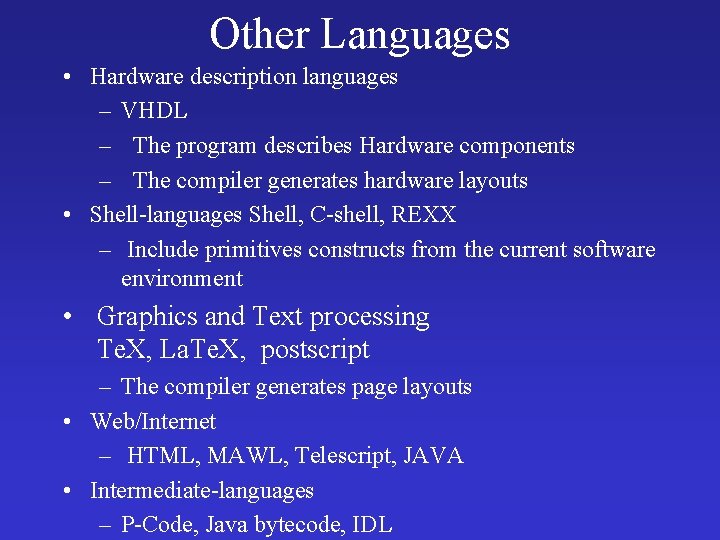
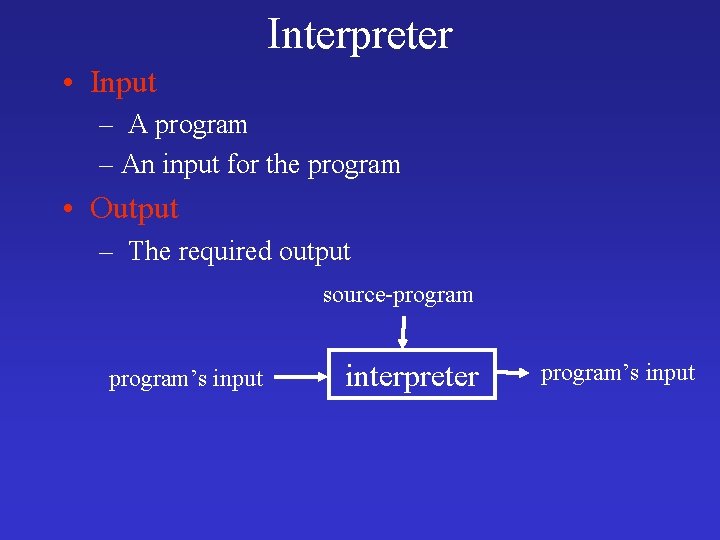
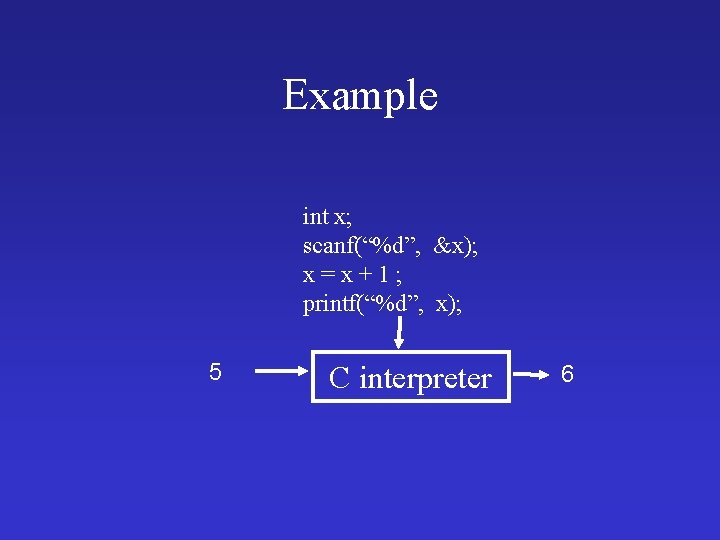
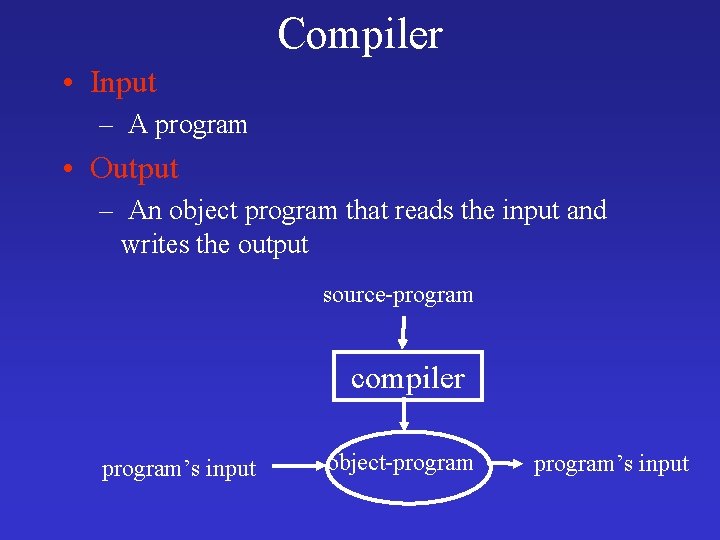
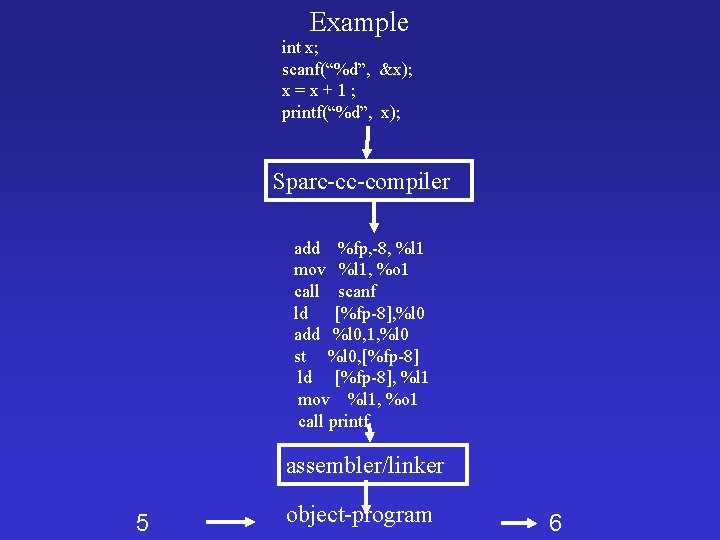
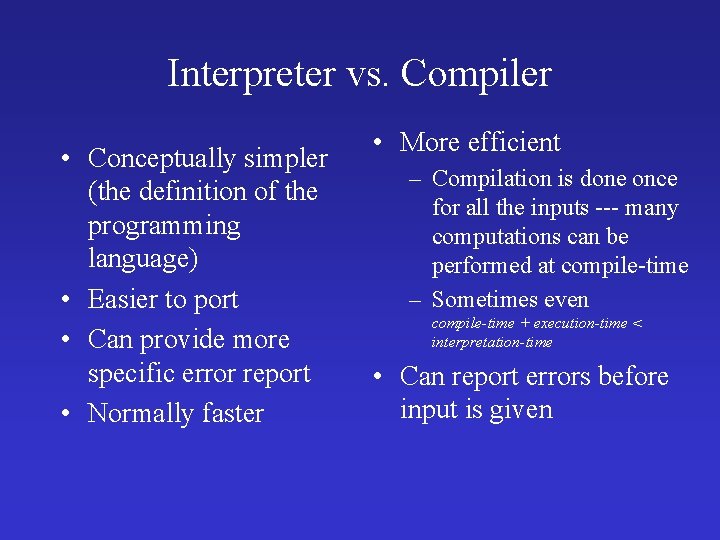
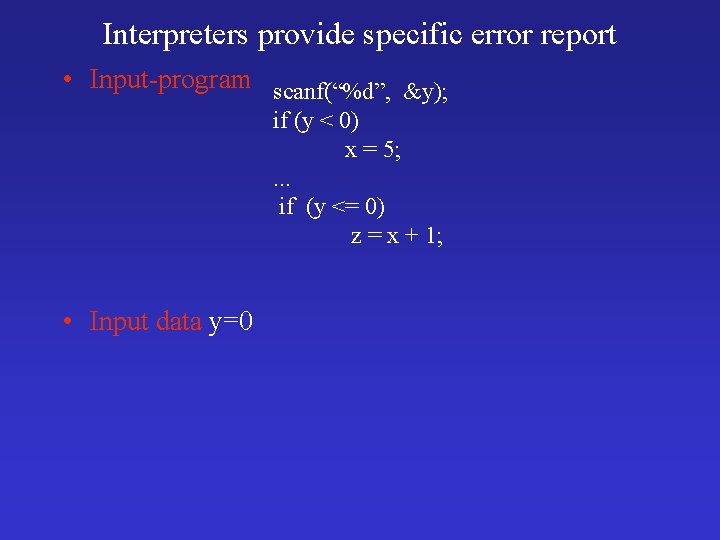
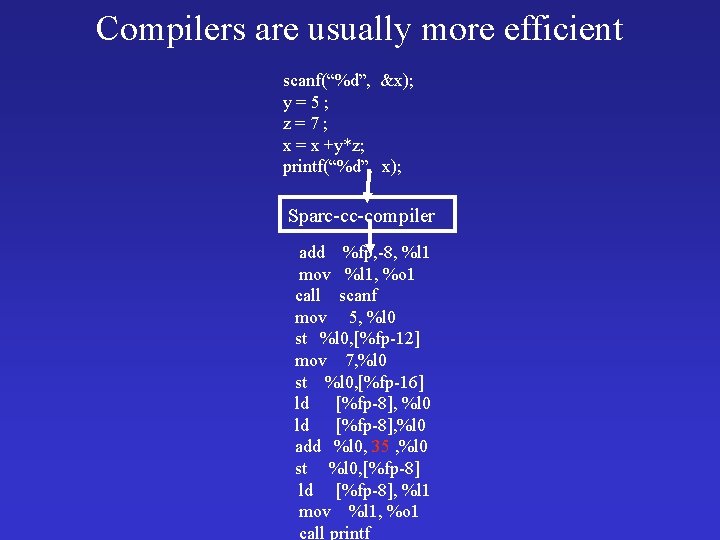
![Compilers can provide errors before actual input is given • Input-program int a[100], x, Compilers can provide errors before actual input is given • Input-program int a[100], x,](https://slidetodoc.com/presentation_image_h2/07c03d780158146791aba728087057a1/image-12.jpg)
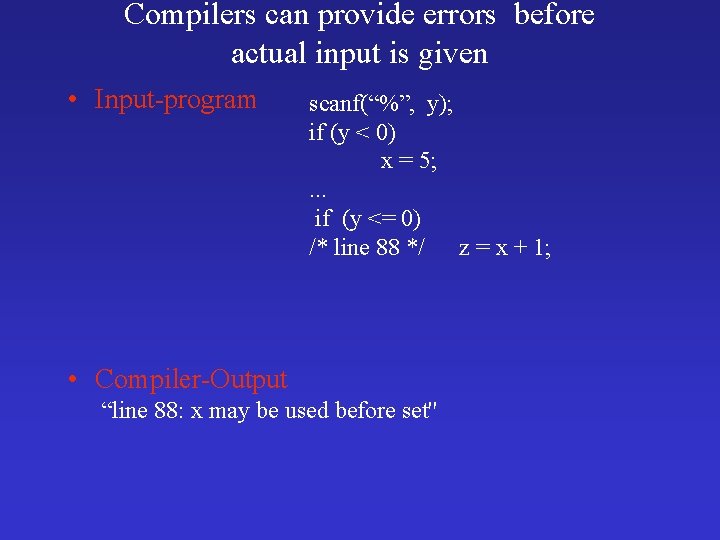
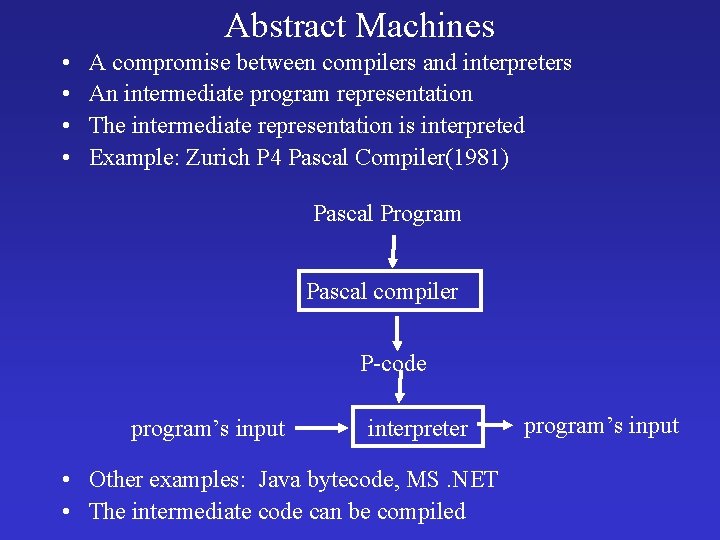
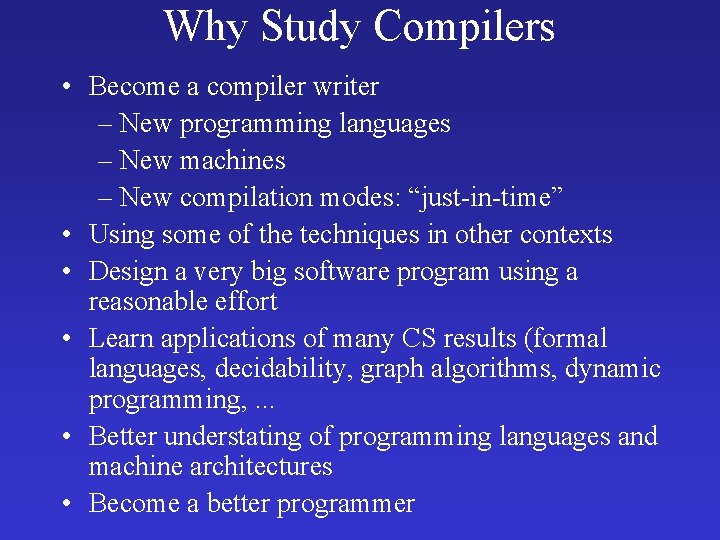
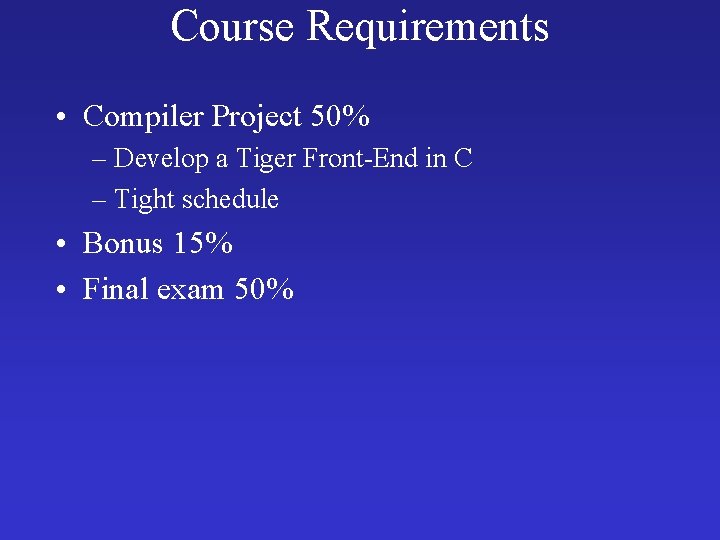
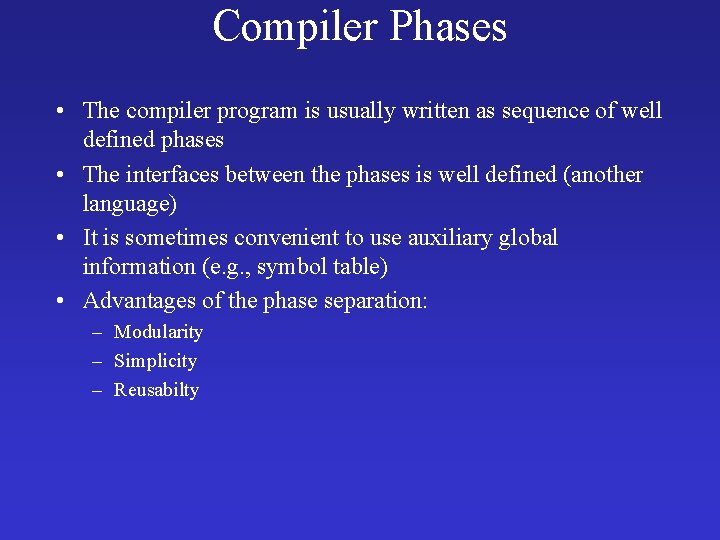
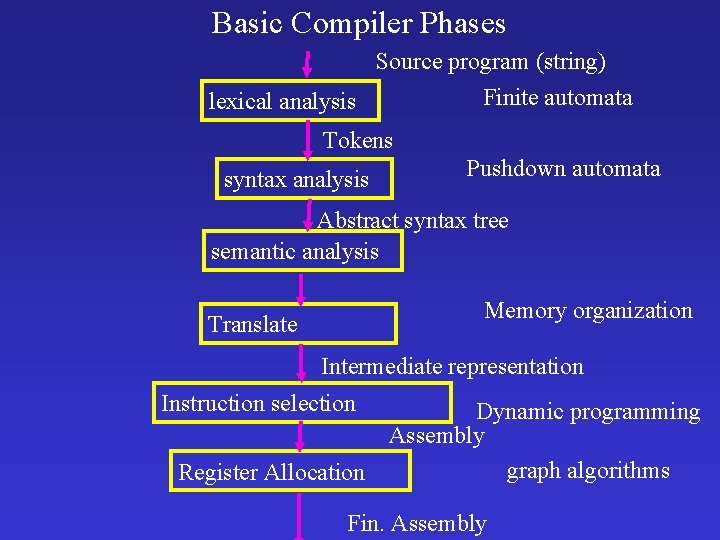
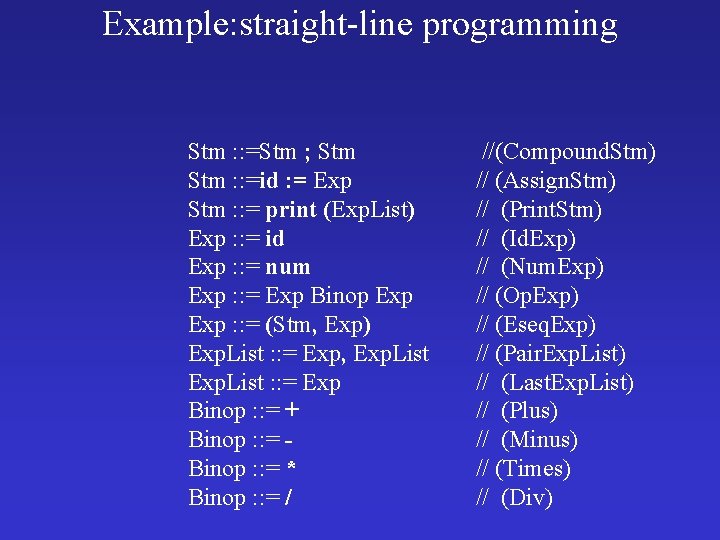
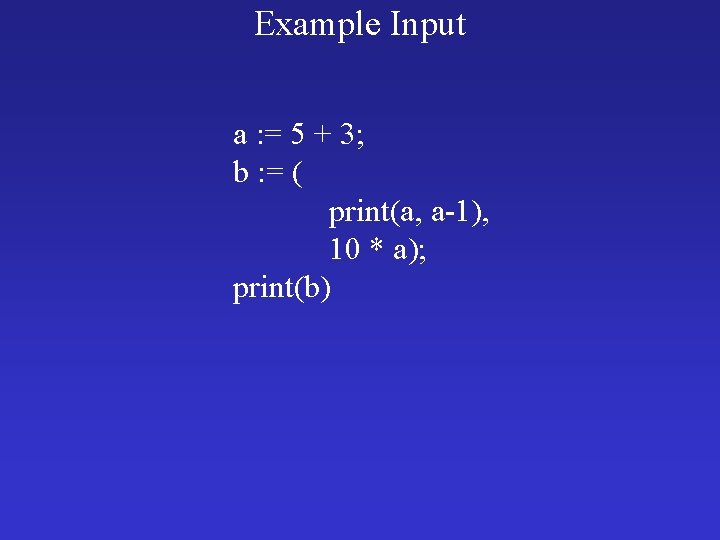
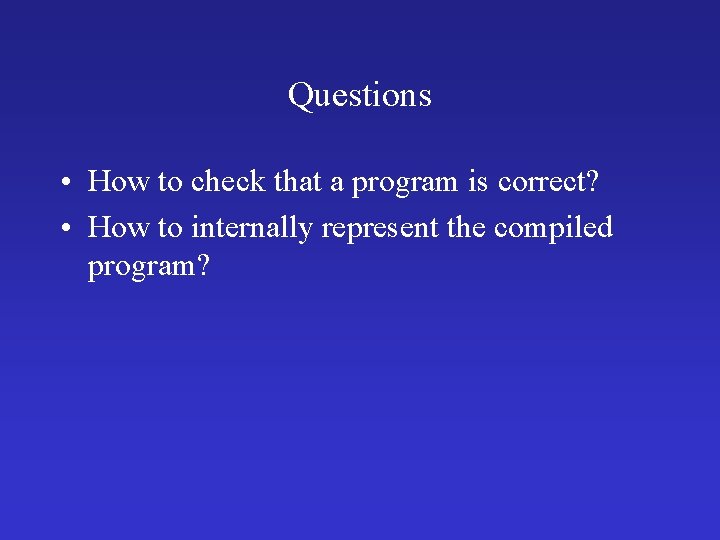
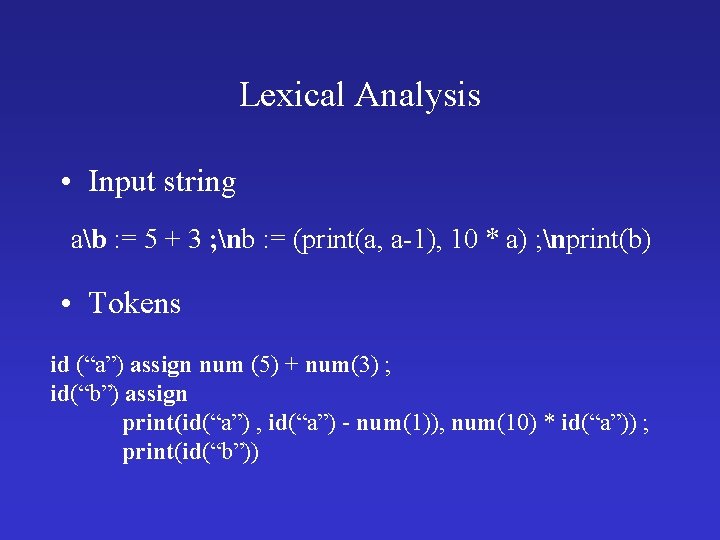
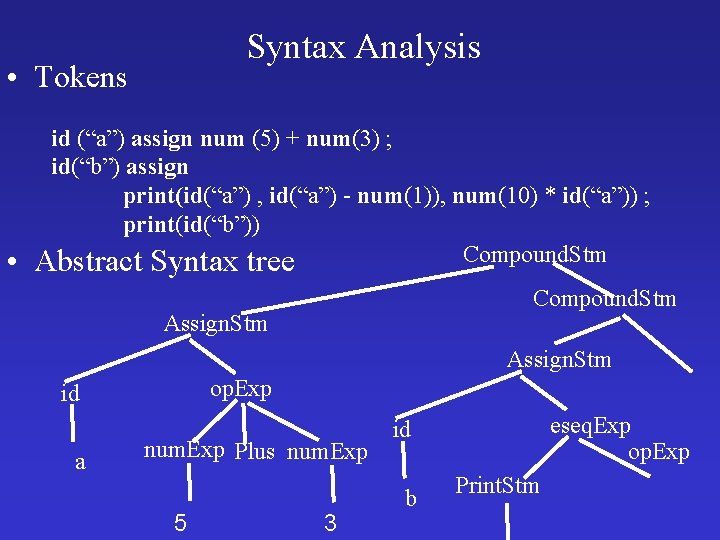
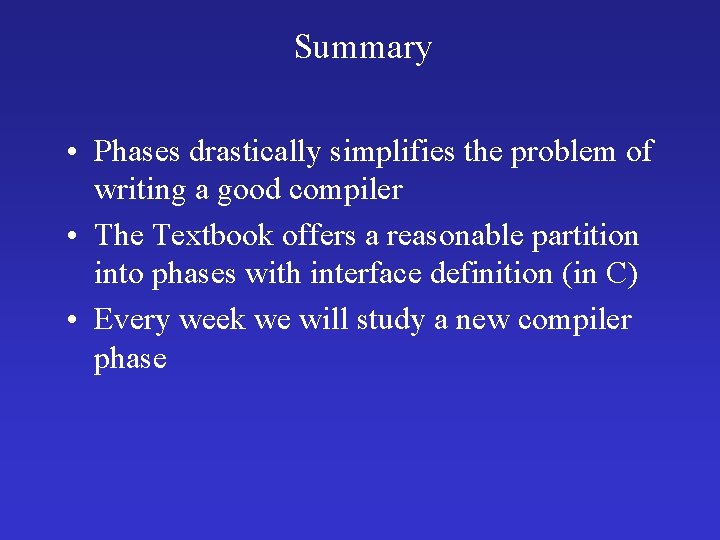
- Slides: 24
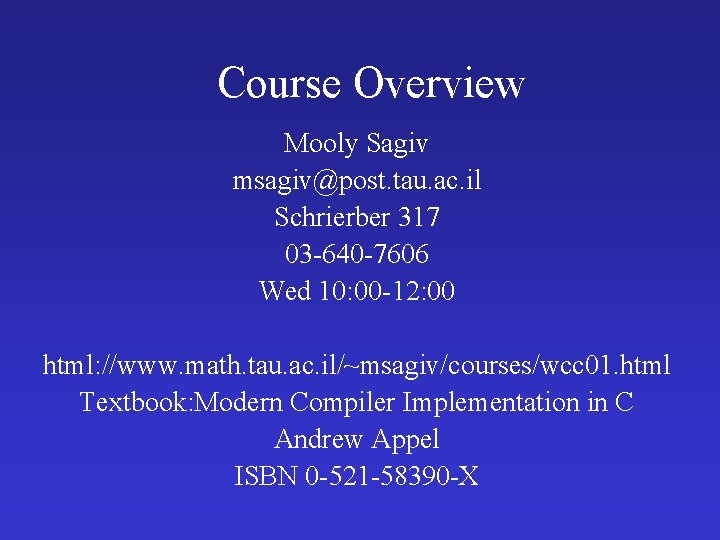
Course Overview Mooly Sagiv msagiv@post. tau. ac. il Schrierber 317 03 -640 -7606 Wed 10: 00 -12: 00 html: //www. math. tau. ac. il/~msagiv/courses/wcc 01. html Textbook: Modern Compiler Implementation in C Andrew Appel ISBN 0 -521 -58390 -X
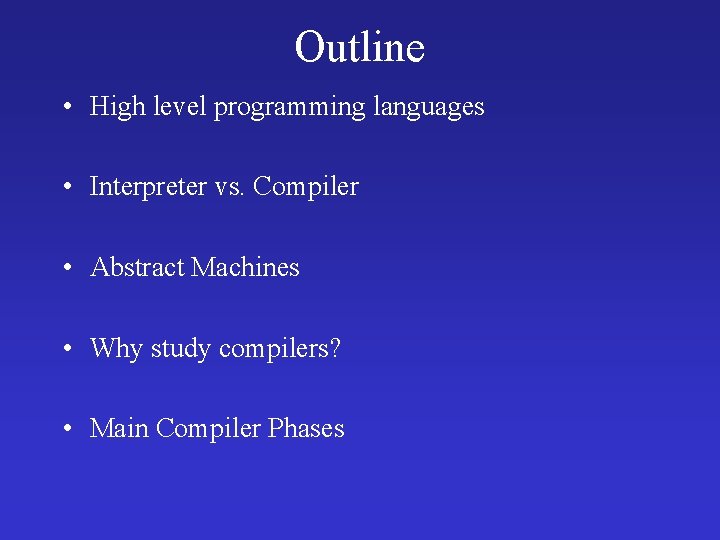
Outline • High level programming languages • Interpreter vs. Compiler • Abstract Machines • Why study compilers? • Main Compiler Phases
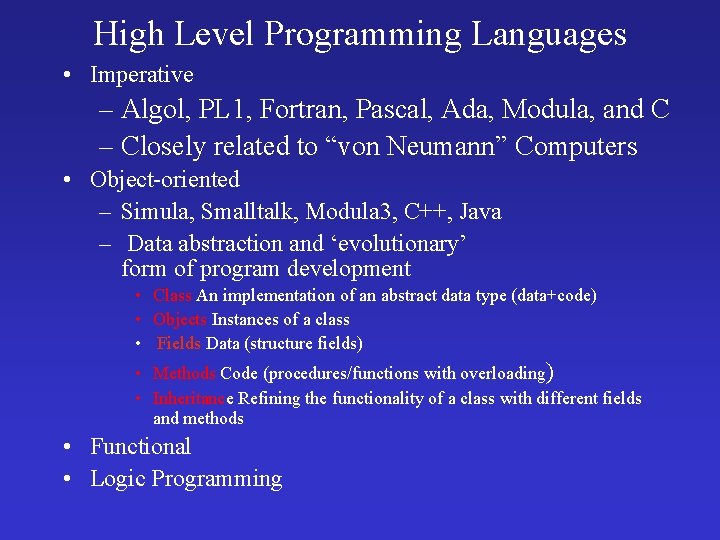
High Level Programming Languages • Imperative – Algol, PL 1, Fortran, Pascal, Ada, Modula, and C – Closely related to “von Neumann” Computers • Object-oriented – Simula, Smalltalk, Modula 3, C++, Java – Data abstraction and ‘evolutionary’ form of program development • Class An implementation of an abstract data type (data+code) • Objects Instances of a class • Fields Data (structure fields) • Methods Code (procedures/functions with overloading) • Inheritance Refining the functionality of a class with different fields and methods • Functional • Logic Programming
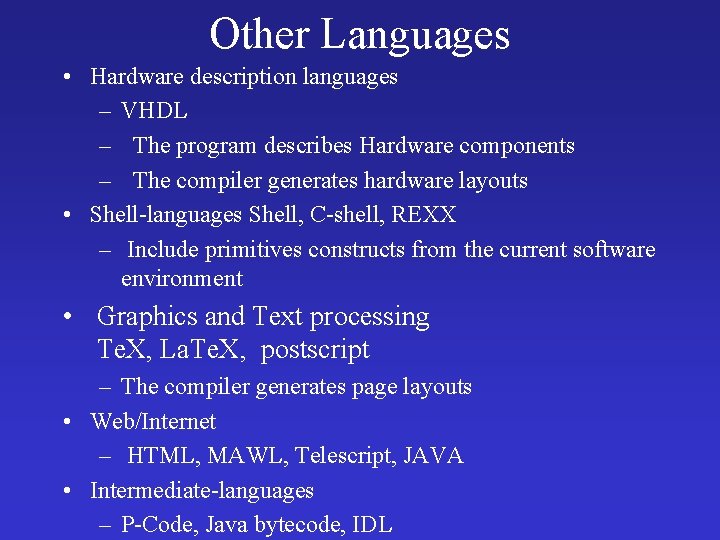
Other Languages • Hardware description languages – VHDL – The program describes Hardware components – The compiler generates hardware layouts • Shell-languages Shell, C-shell, REXX – Include primitives constructs from the current software environment • Graphics and Text processing Te. X, La. Te. X, postscript – The compiler generates page layouts • Web/Internet – HTML, MAWL, Telescript, JAVA • Intermediate-languages – P-Code, Java bytecode, IDL
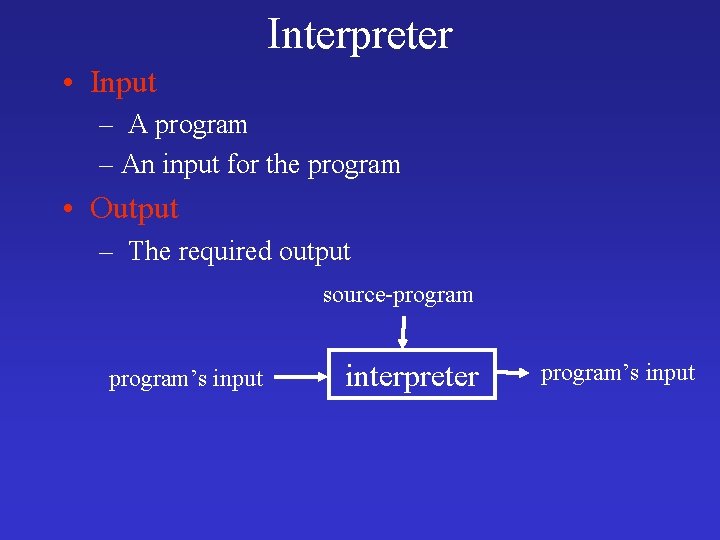
Interpreter • Input – A program – An input for the program • Output – The required output source-program’s input interpreter program’s input
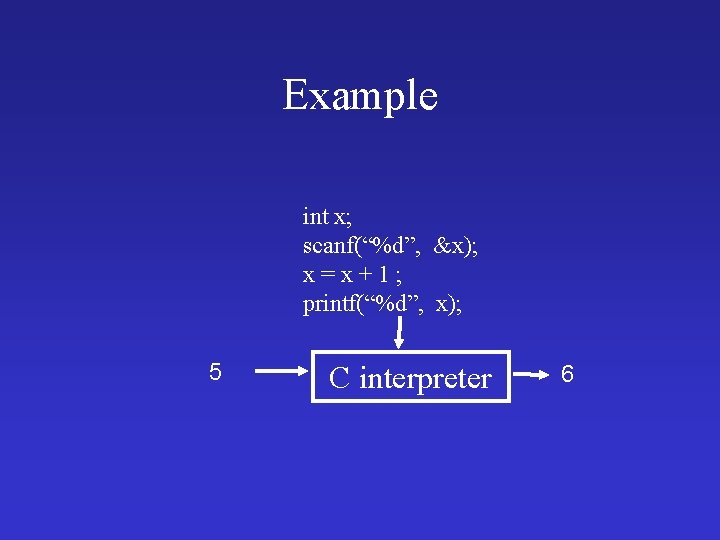
Example int x; scanf(“%d”, &x); x=x+1; printf(“%d”, x); 5 C interpreter 6
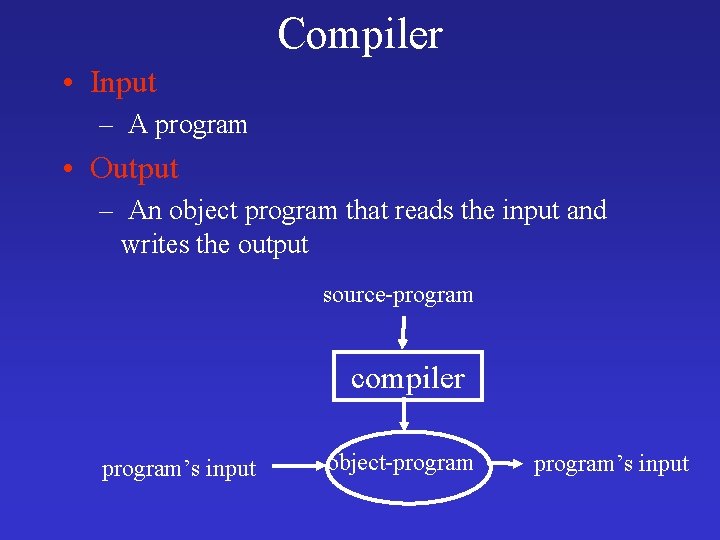
Compiler • Input – A program • Output – An object program that reads the input and writes the output source-program compiler program’s input object-program’s input
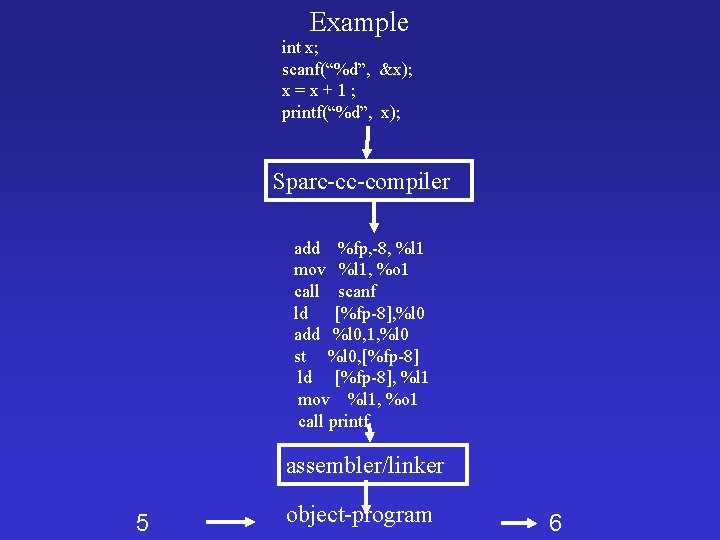
Example int x; scanf(“%d”, &x); x=x+1; printf(“%d”, x); Sparc-cc-compiler add %fp, -8, %l 1 mov %l 1, %o 1 call scanf ld [%fp-8], %l 0 add %l 0, 1, %l 0 st %l 0, [%fp-8] ld [%fp-8], %l 1 mov %l 1, %o 1 call printf assembler/linker 5 object-program 6
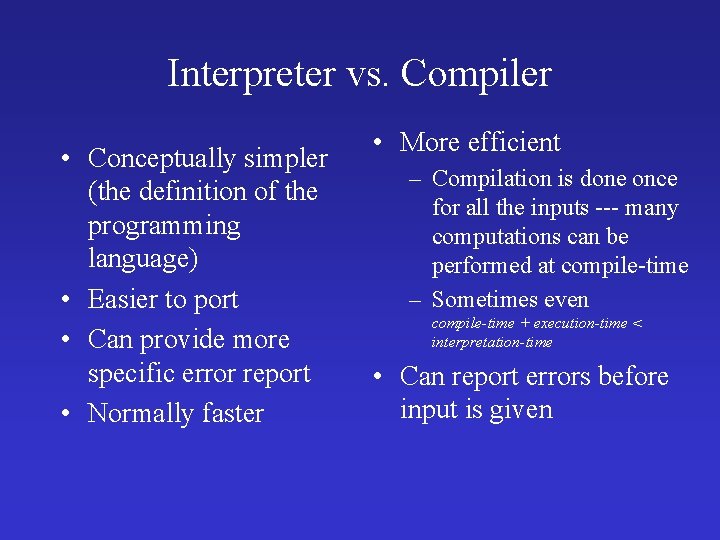
Interpreter vs. Compiler • Conceptually simpler (the definition of the programming language) • Easier to port • Can provide more specific error report • Normally faster • More efficient – Compilation is done once for all the inputs --- many computations can be performed at compile-time – Sometimes even compile-time + execution-time < interpretation-time • Can report errors before input is given
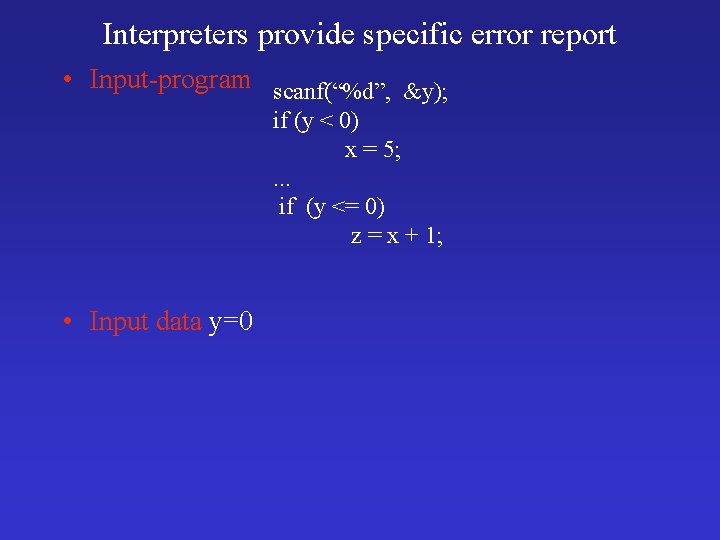
Interpreters provide specific error report • Input-program scanf(“%d”, &y); if (y < 0) x = 5; . . . if (y <= 0) z = x + 1; • Input data y=0
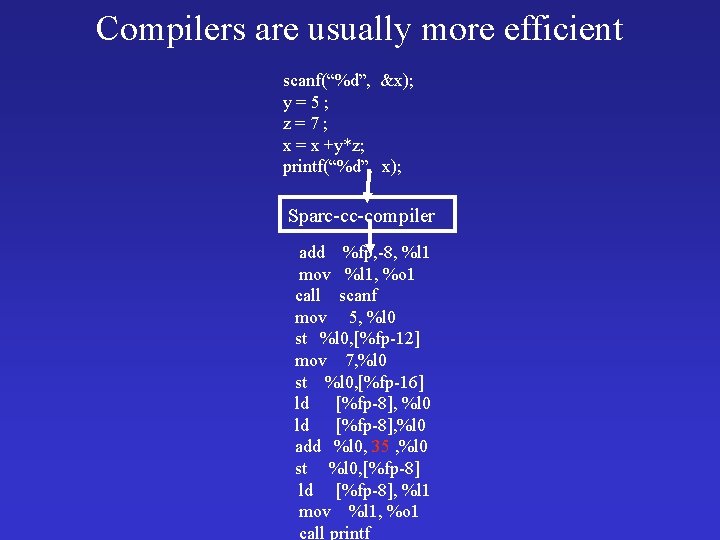
Compilers are usually more efficient scanf(“%d”, &x); y=5; z=7; x = x +y*z; printf(“%d”, x); Sparc-cc-compiler add %fp, -8, %l 1 mov %l 1, %o 1 call scanf mov 5, %l 0 st %l 0, [%fp-12] mov 7, %l 0 st %l 0, [%fp-16] ld [%fp-8], %l 0 add %l 0, 35 , %l 0 st %l 0, [%fp-8] ld [%fp-8], %l 1 mov %l 1, %o 1 call printf
![Compilers can provide errors before actual input is given Inputprogram int a100 x Compilers can provide errors before actual input is given • Input-program int a[100], x,](https://slidetodoc.com/presentation_image_h2/07c03d780158146791aba728087057a1/image-12.jpg)
Compilers can provide errors before actual input is given • Input-program int a[100], x, y ; scanf(“%d”, y) ; if (y < 0) /* line 4*/ y=a; • Compiler-Output “line 4: improper pointer/integer combination: op =''
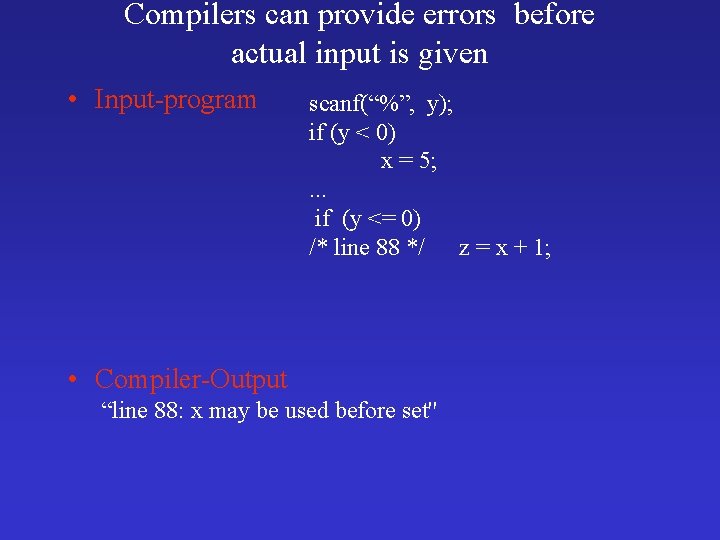
Compilers can provide errors before actual input is given • Input-program scanf(“%”, y); if (y < 0) x = 5; . . . if (y <= 0) /* line 88 */ z = x + 1; • Compiler-Output “line 88: x may be used before set''
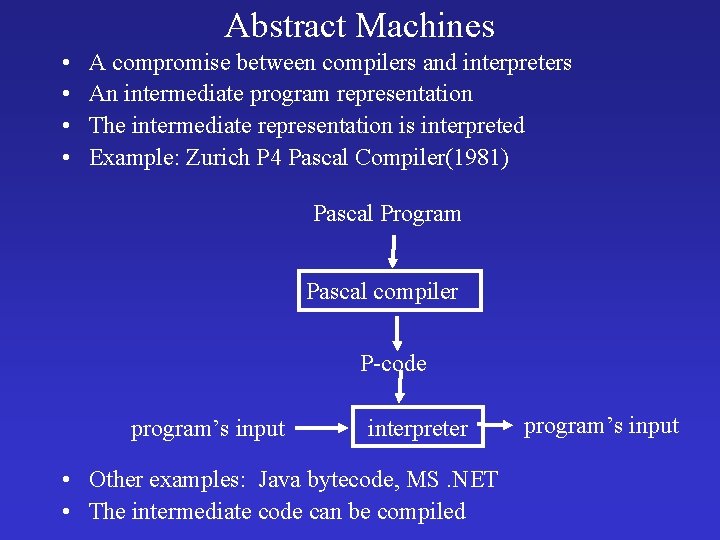
Abstract Machines • • A compromise between compilers and interpreters An intermediate program representation The intermediate representation is interpreted Example: Zurich P 4 Pascal Compiler(1981) Pascal Program Pascal compiler P-code program’s input interpreter • Other examples: Java bytecode, MS. NET • The intermediate code can be compiled program’s input
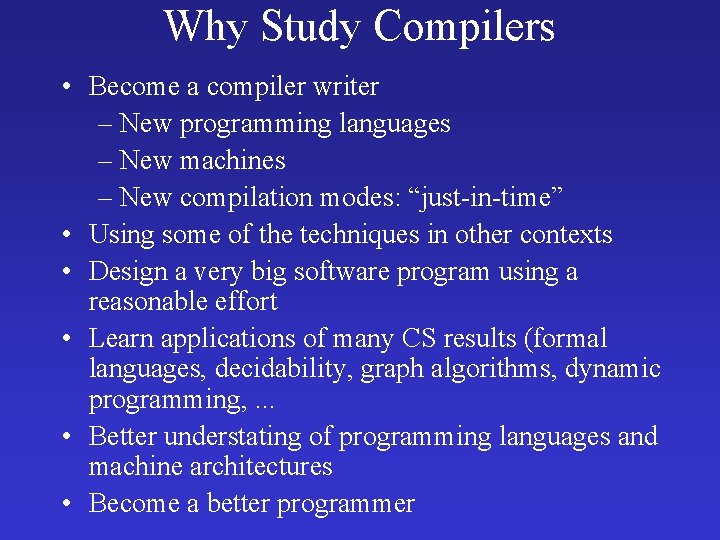
Why Study Compilers • Become a compiler writer – New programming languages – New machines – New compilation modes: “just-in-time” • Using some of the techniques in other contexts • Design a very big software program using a reasonable effort • Learn applications of many CS results (formal languages, decidability, graph algorithms, dynamic programming, . . . • Better understating of programming languages and machine architectures • Become a better programmer
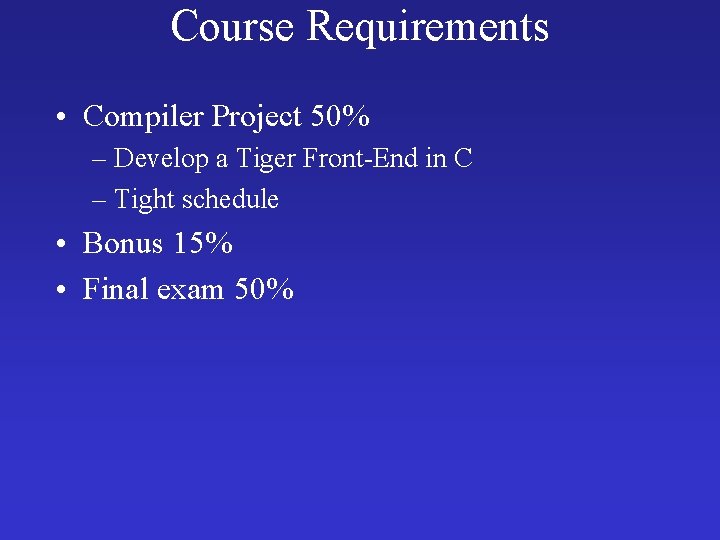
Course Requirements • Compiler Project 50% – Develop a Tiger Front-End in C – Tight schedule • Bonus 15% • Final exam 50%
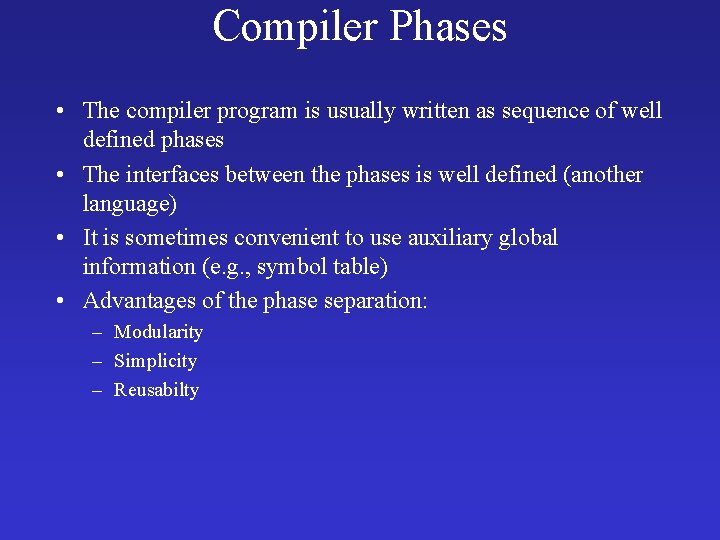
Compiler Phases • The compiler program is usually written as sequence of well defined phases • The interfaces between the phases is well defined (another language) • It is sometimes convenient to use auxiliary global information (e. g. , symbol table) • Advantages of the phase separation: – Modularity – Simplicity – Reusabilty
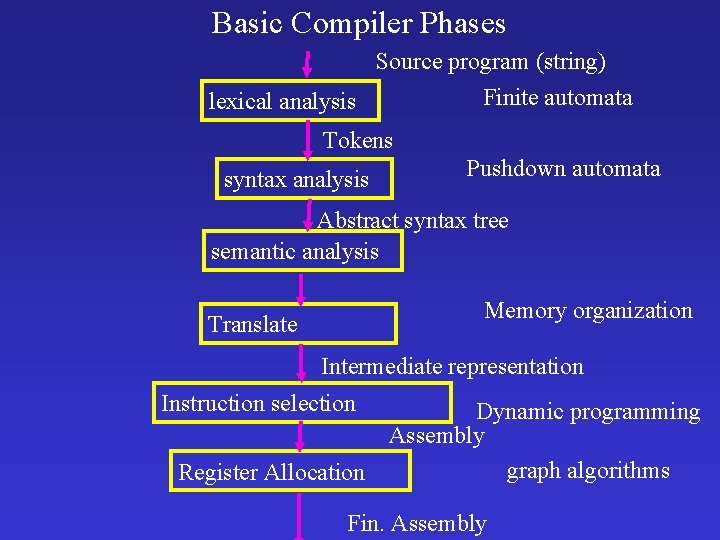
Basic Compiler Phases Source program (string) Finite automata lexical analysis Tokens syntax analysis Pushdown automata Abstract syntax tree semantic analysis Memory organization Translate Intermediate representation Instruction selection Dynamic programming Assembly graph algorithms Register Allocation Fin. Assembly
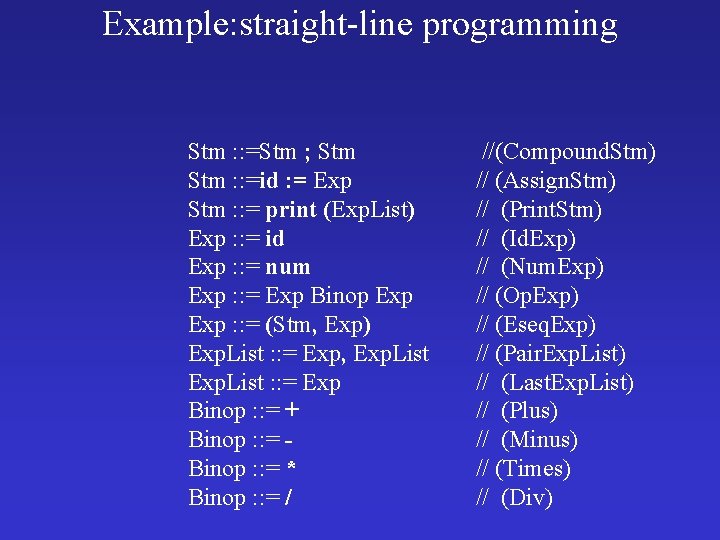
Example: straight-line programming Stm : : =Stm ; Stm : : =id : = Exp Stm : : = print (Exp. List) Exp : : = id Exp : : = num Exp : : = Exp Binop Exp : : = (Stm, Exp) Exp. List : : = Exp, Exp. List : : = Exp Binop : : = + Binop : : = * Binop : : = / //(Compound. Stm) // (Assign. Stm) // (Print. Stm) // (Id. Exp) // (Num. Exp) // (Op. Exp) // (Eseq. Exp) // (Pair. Exp. List) // (Last. Exp. List) // (Plus) // (Minus) // (Times) // (Div)
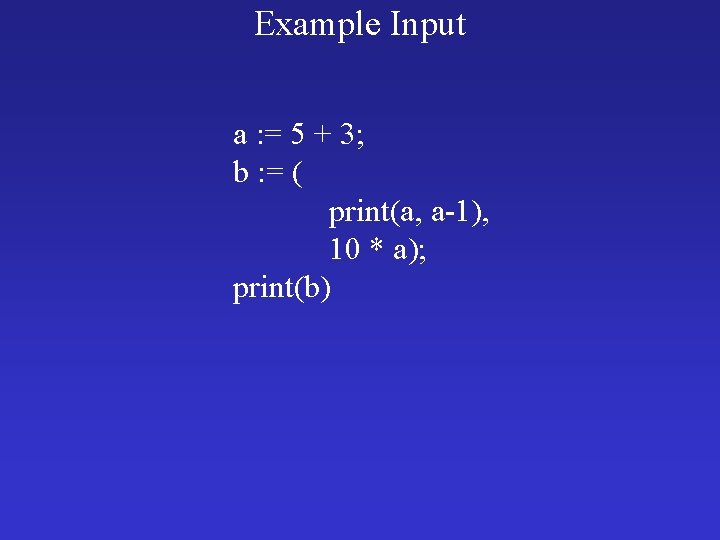
Example Input a : = 5 + 3; b : = ( print(a, a-1), 10 * a); print(b)
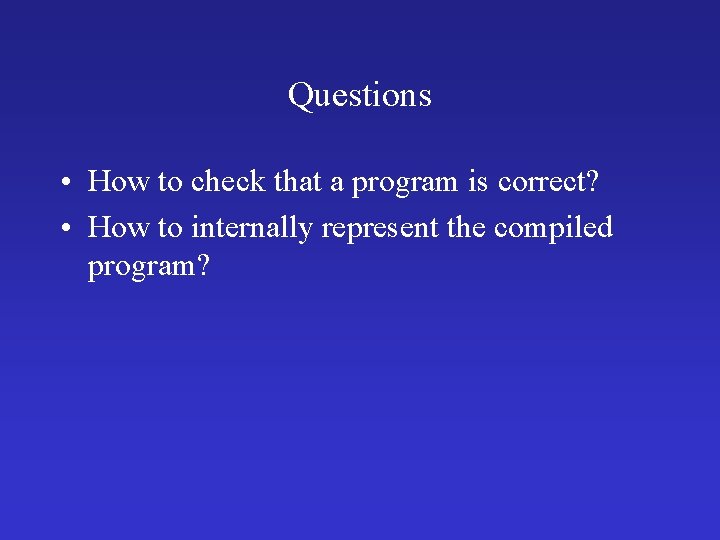
Questions • How to check that a program is correct? • How to internally represent the compiled program?
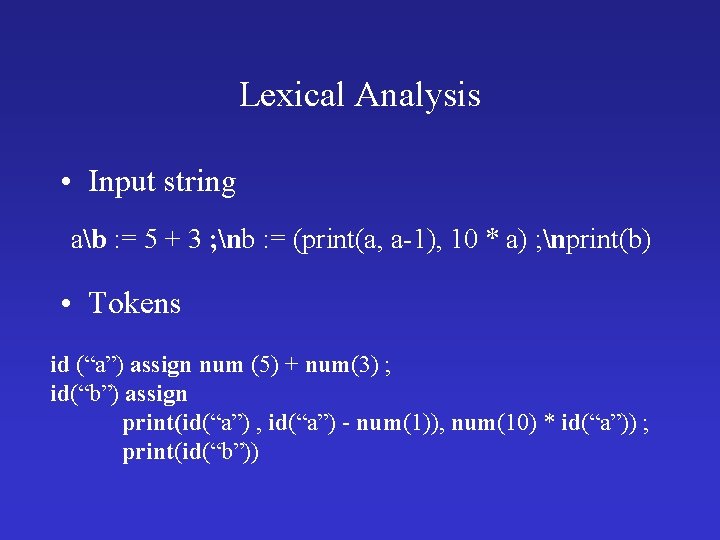
Lexical Analysis • Input string ab : = 5 + 3 ; nb : = (print(a, a-1), 10 * a) ; nprint(b) • Tokens id (“a”) assign num (5) + num(3) ; id(“b”) assign print(id(“a”) , id(“a”) - num(1)), num(10) * id(“a”)) ; print(id(“b”))
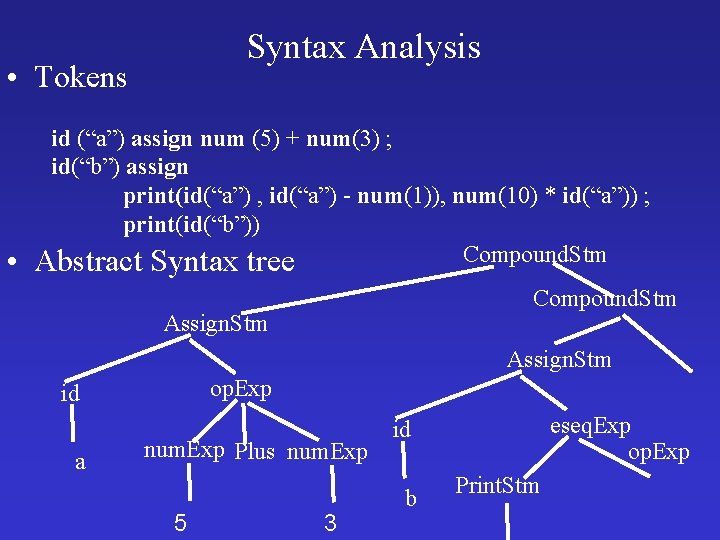
Syntax Analysis • Tokens • id (“a”) assign num (5) + num(3) ; id(“b”) assign print(id(“a”) , id(“a”) - num(1)), num(10) * id(“a”)) ; print(id(“b”)) Compound. Stm Abstract Syntax tree Compound. Stm Assign. Stm op. Exp id a num. Exp Plus num. Exp 5 3 eseq. Exp op. Exp id b Print. Stm
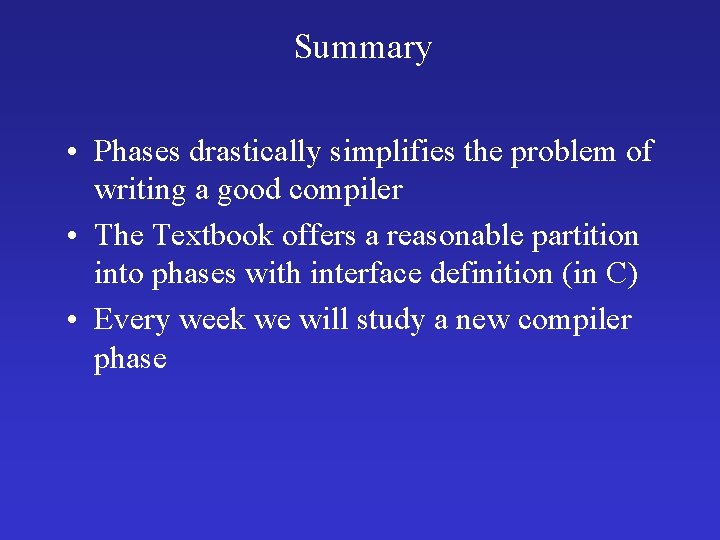
Summary • Phases drastically simplifies the problem of writing a good compiler • The Textbook offers a reasonable partition into phases with interface definition (in C) • Every week we will study a new compiler phase
Mooly sagiv
Higgs to tau tau
Course title and course number
Course interne course externe
Flemish bond t junction
Tau ceti distance to earth
Signo franciscano
Gấp tàu thủy hai ống khói
Karakia whakamutunga kia tau
Letra tav significado
Proxy tau
Mombe'upy lago ypacarai
Tetrathlon olympics
Upper ngau tau kok estate
Tali ui sepitema
Punktbiseriale korrelation beispiel
Desain topologi jaringan
Mama mamyte as tave myliu
Sen tau
Elastisk tau biltema
Delta tau alpha honor society
Tau vs titans
Tau dem
Karakia for strength
Que es una runa y para que sirve