Course Contents 1 Sr Major and Detailed Coverage
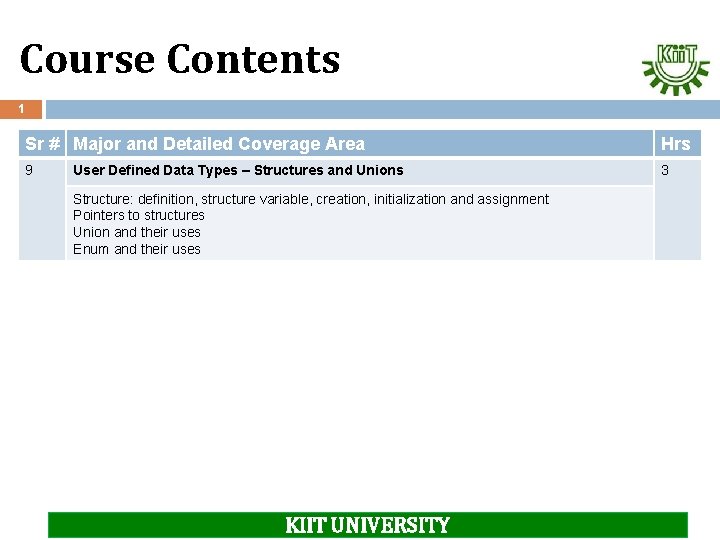
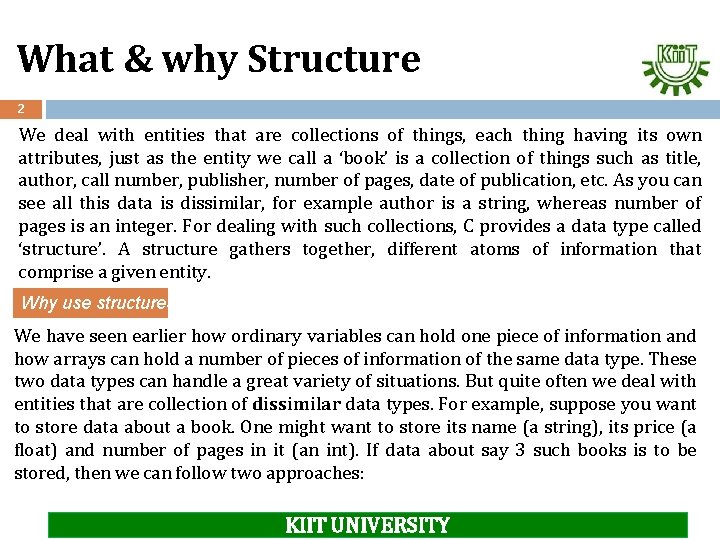
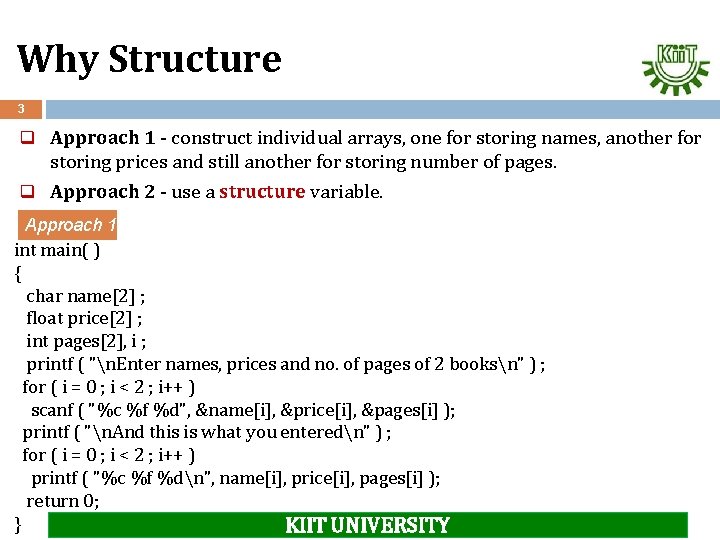
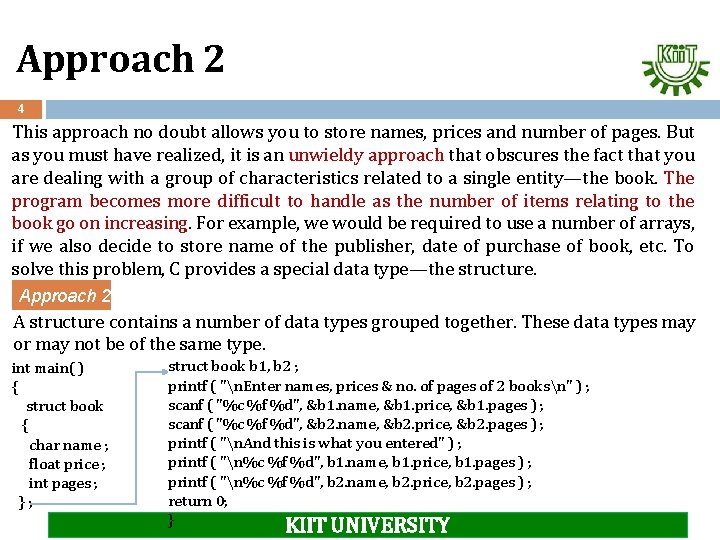
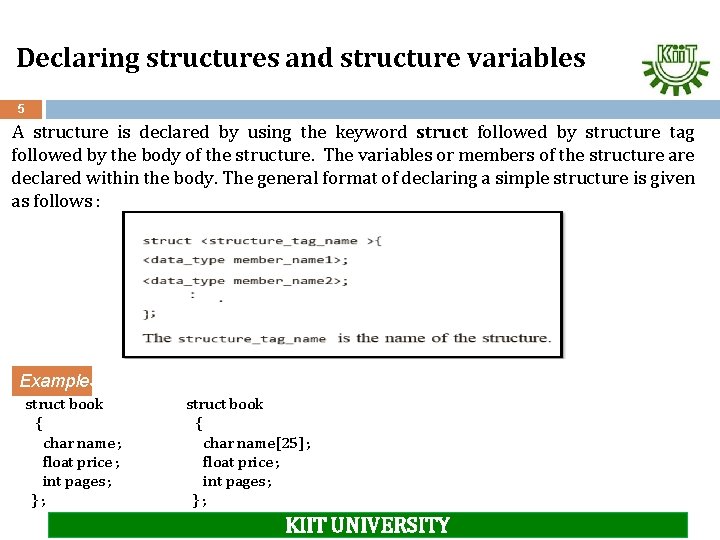
![Structures declaration type 6 struct book { char name[25] ; float price ; int Structures declaration type 6 struct book { char name[25] ; float price ; int](https://slidetodoc.com/presentation_image_h/aa273ab1efc1320e7663799f47bb4230/image-6.jpg)
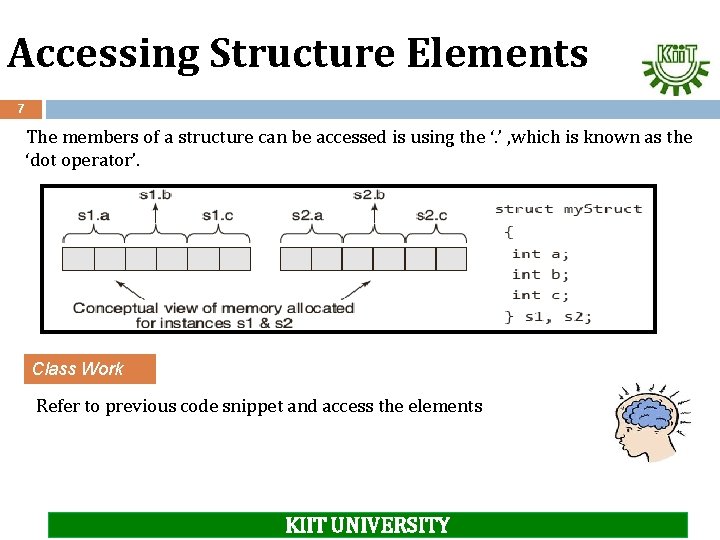
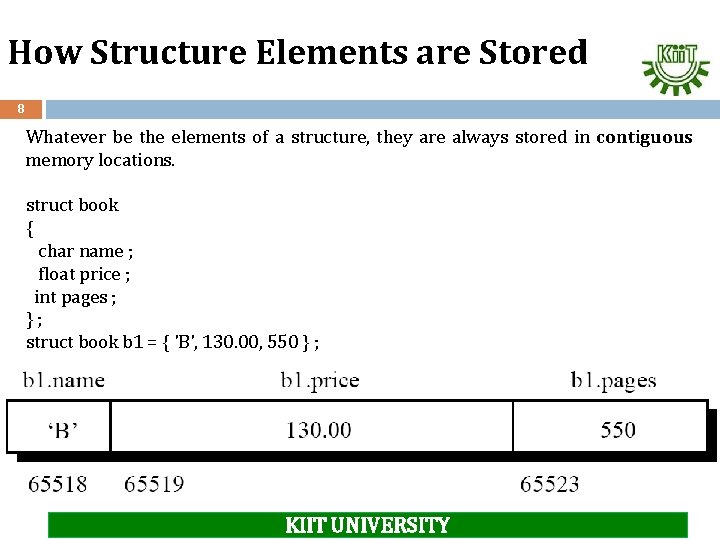
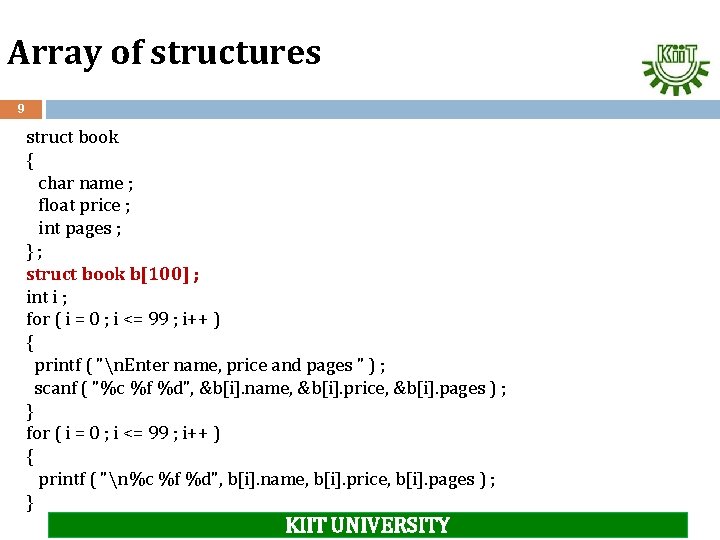
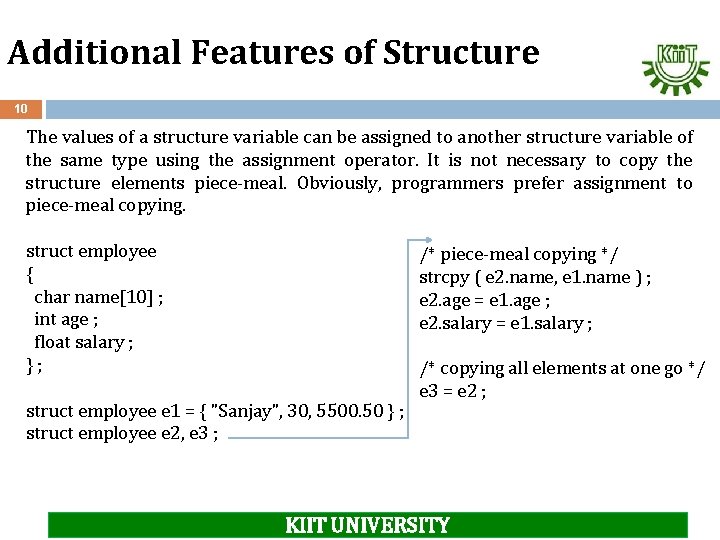
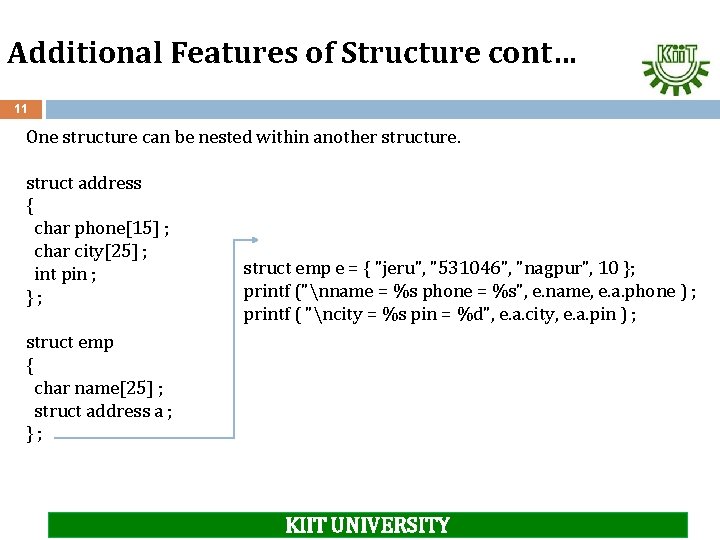
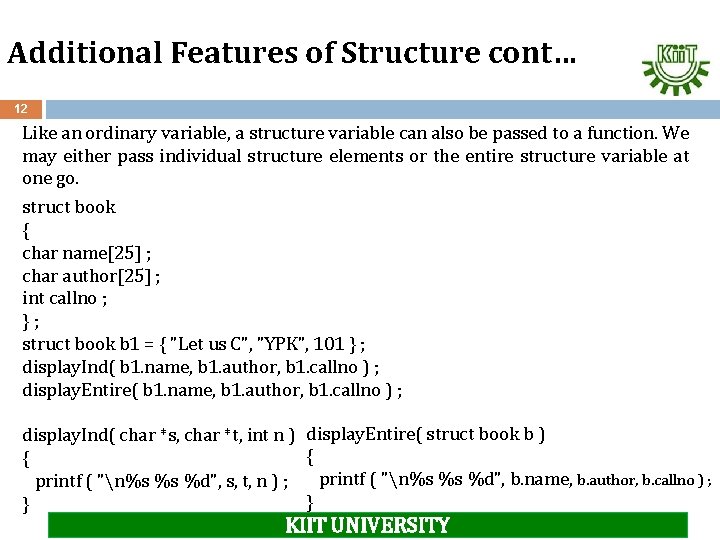
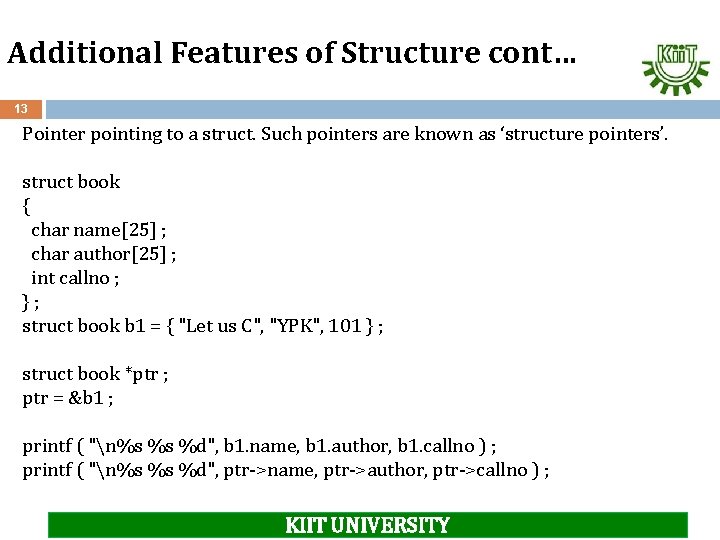
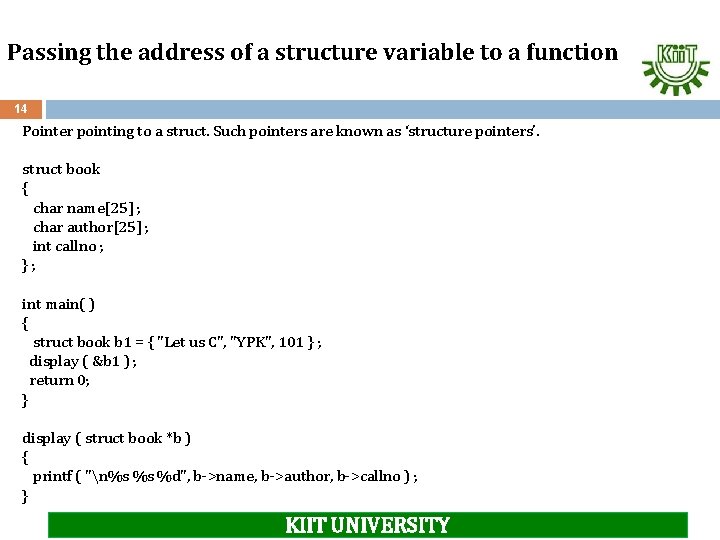
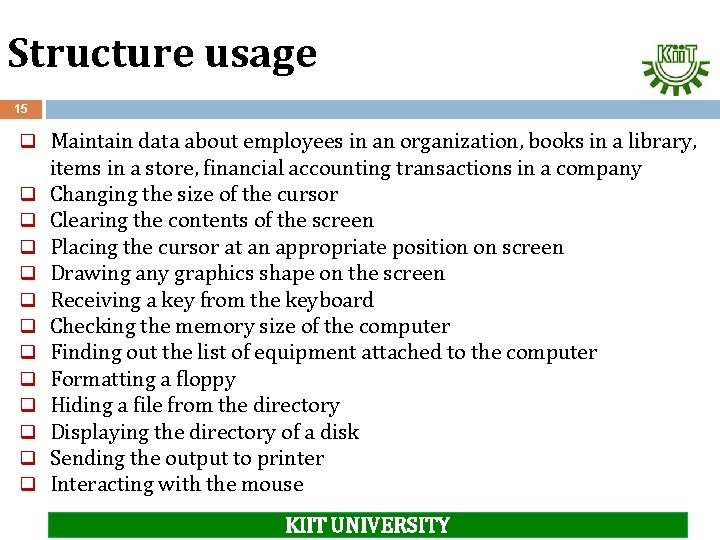
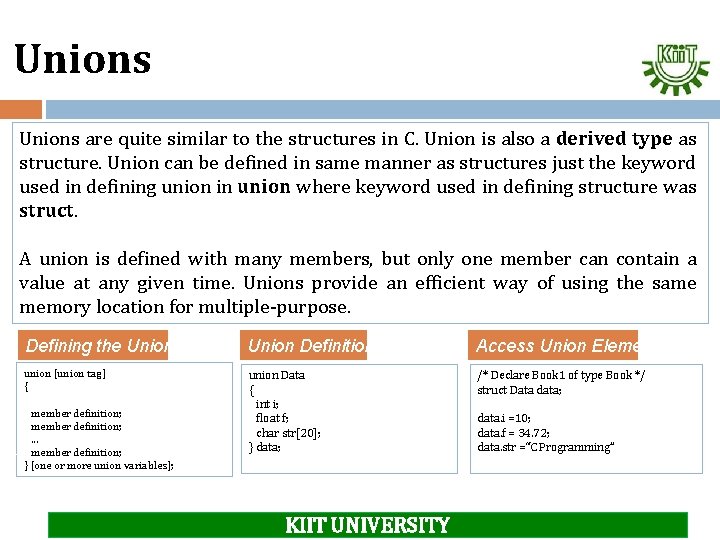
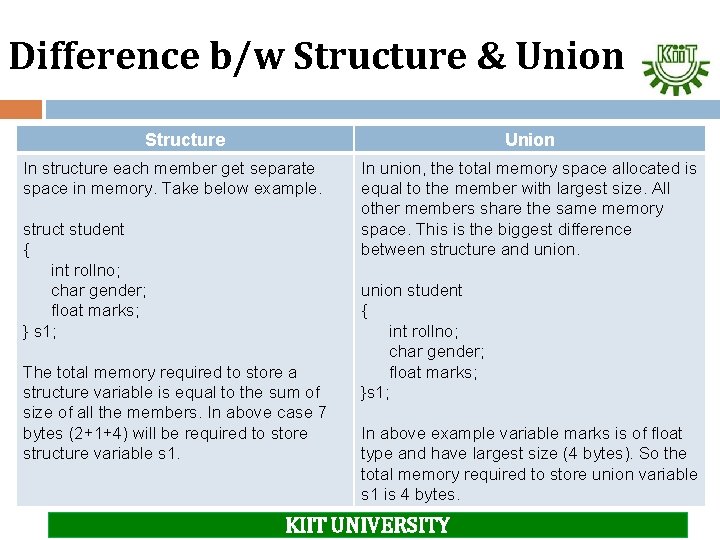
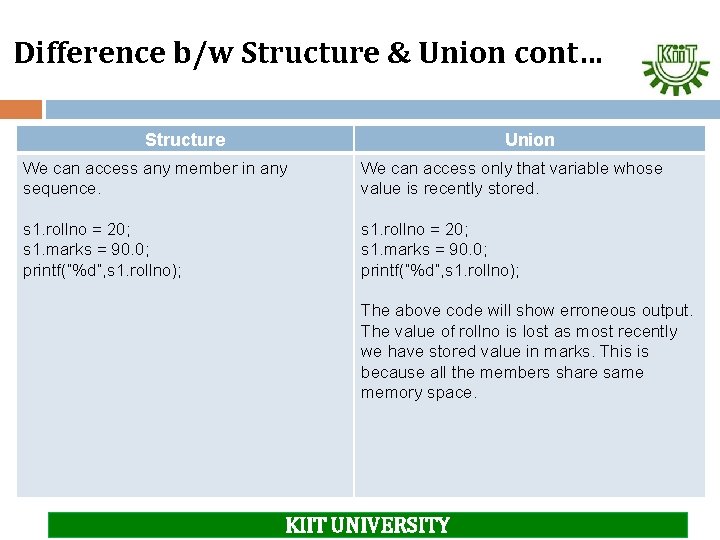
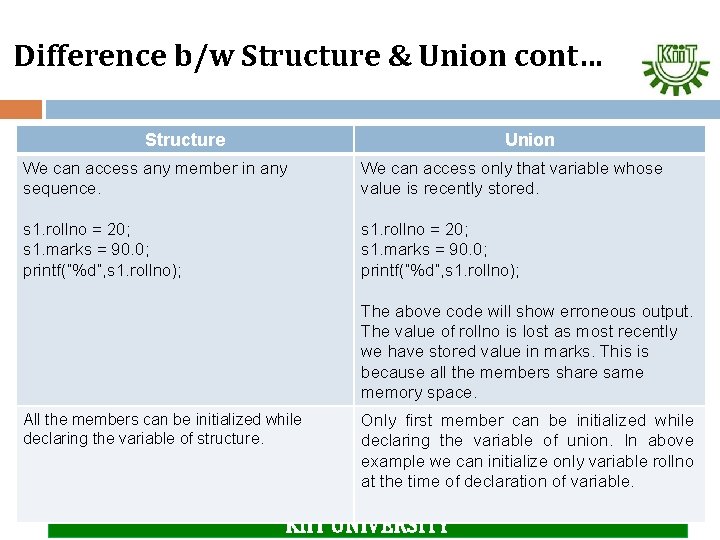
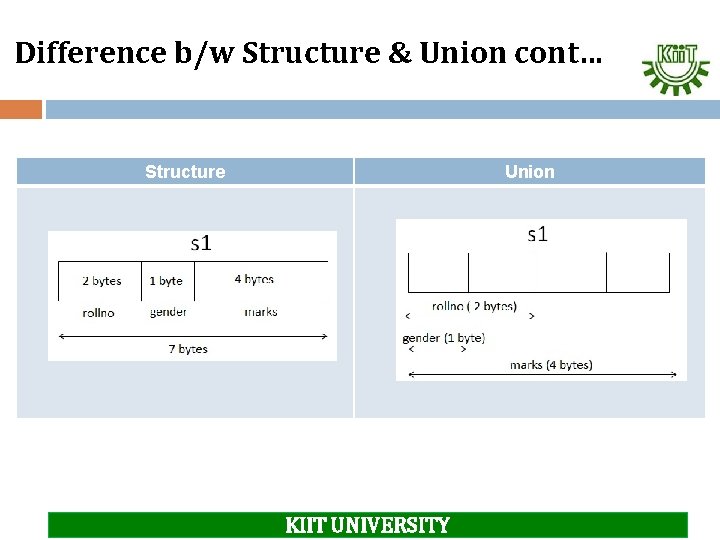
![Union of Structures struct a { int i; char c[2]; }; struct b { Union of Structures struct a { int i; char c[2]; }; struct b {](https://slidetodoc.com/presentation_image_h/aa273ab1efc1320e7663799f47bb4230/image-21.jpg)
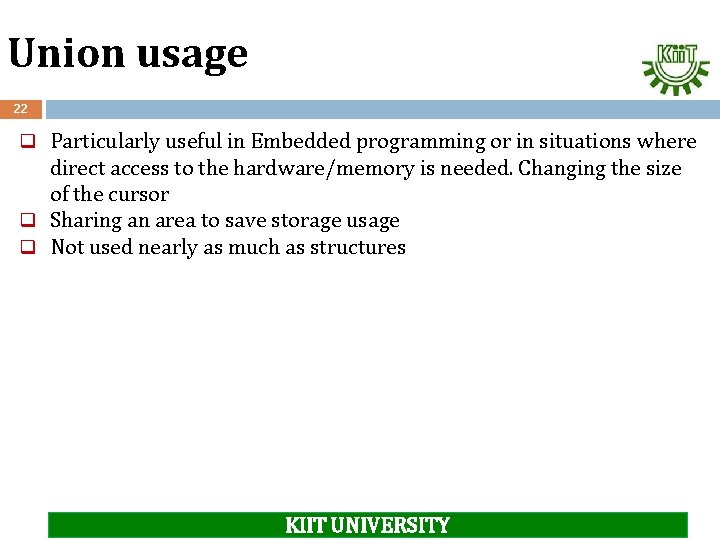
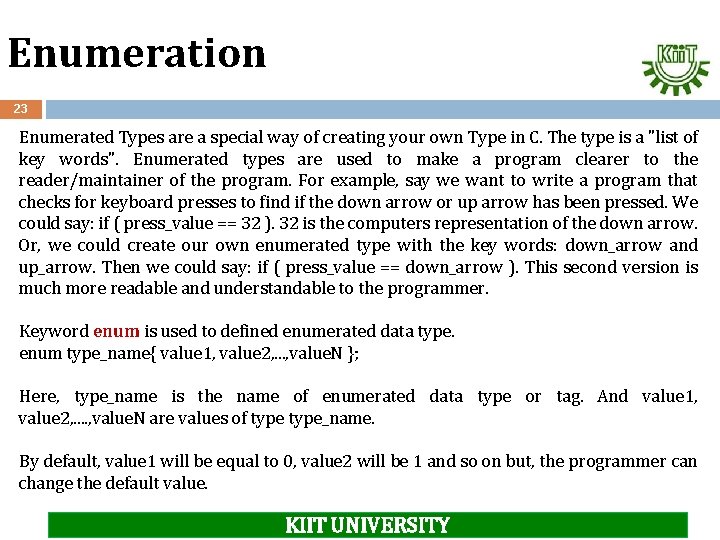
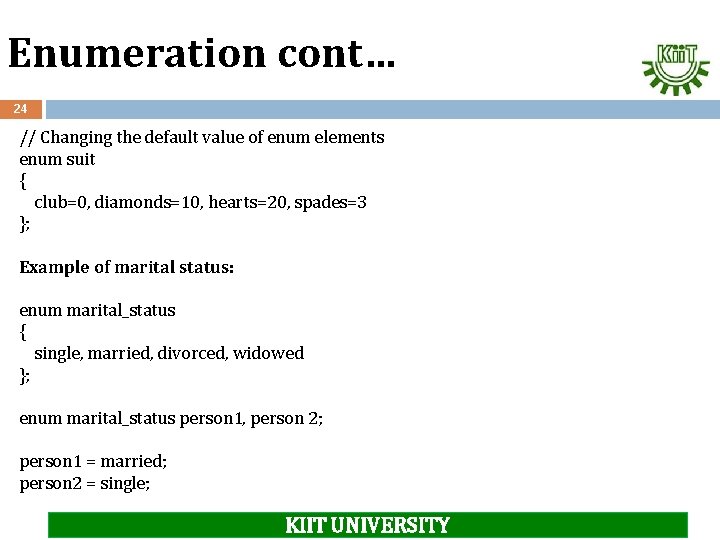
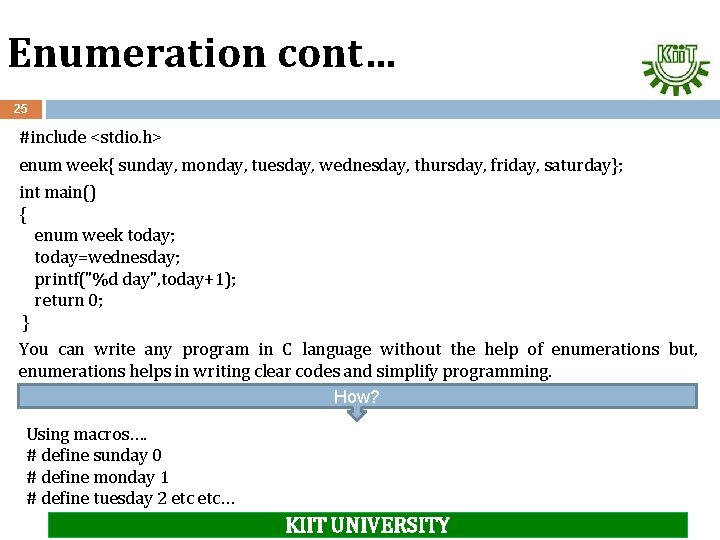
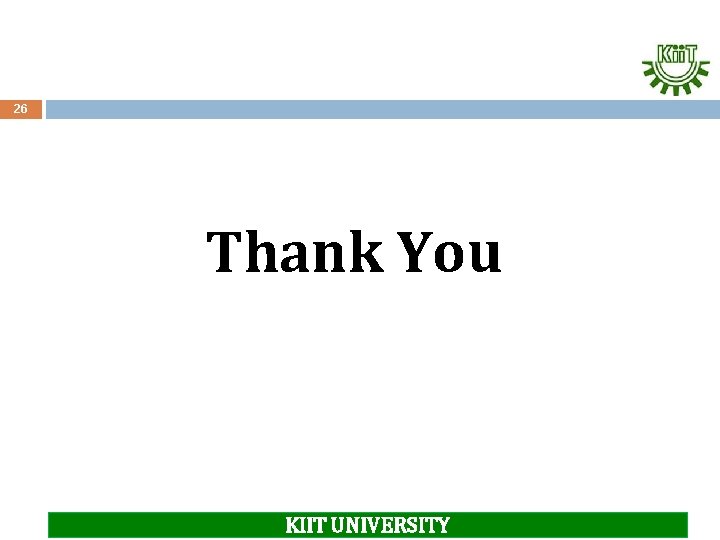
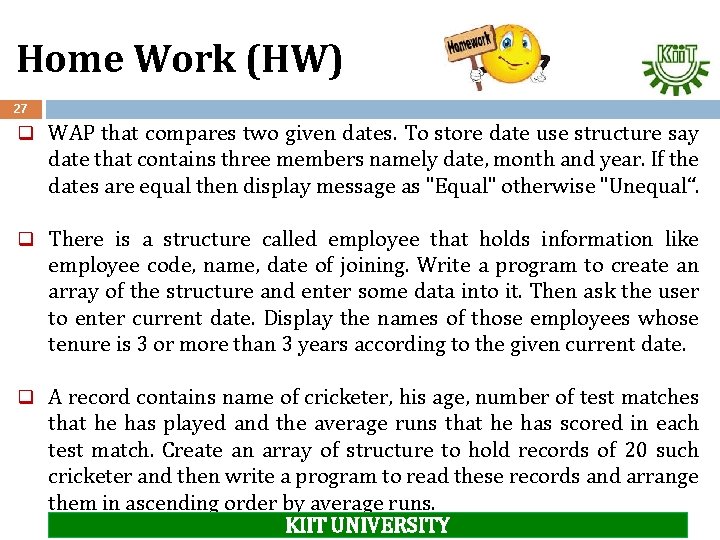
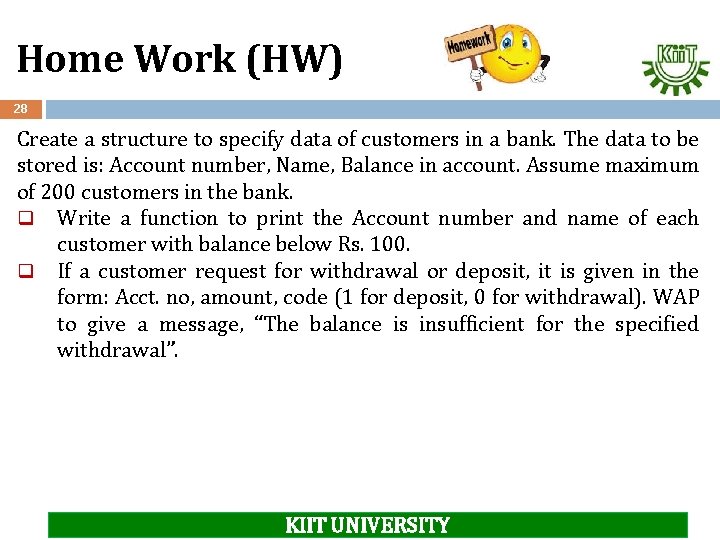
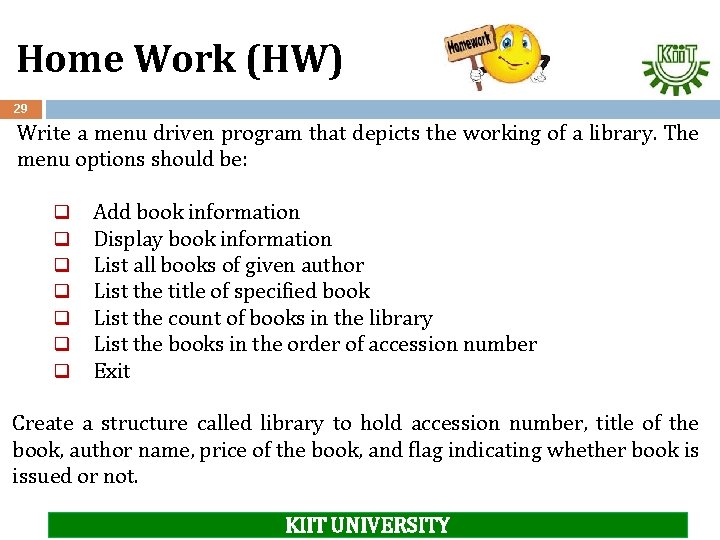
- Slides: 29
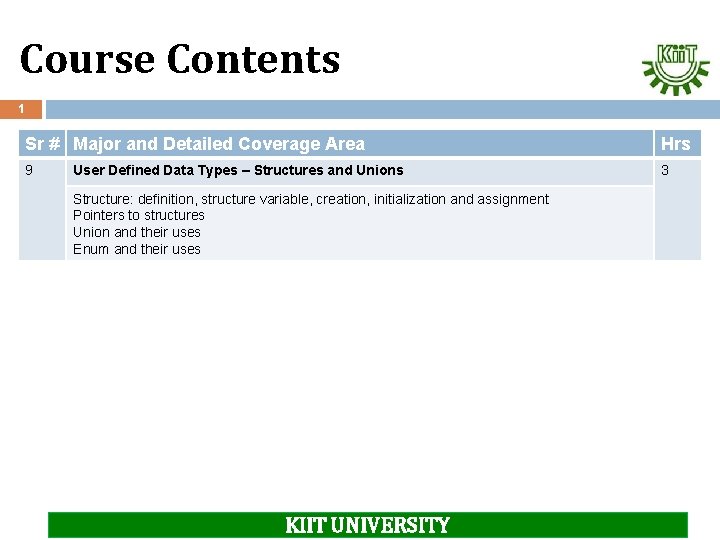
Course Contents 1 Sr # Major and Detailed Coverage Area Hrs 9 3 User Defined Data Types – Structures and Unions Structure: definition, structure variable, creation, initialization and assignment Pointers to structures Union and their uses Enum and their uses KIIT UNIVERSITY
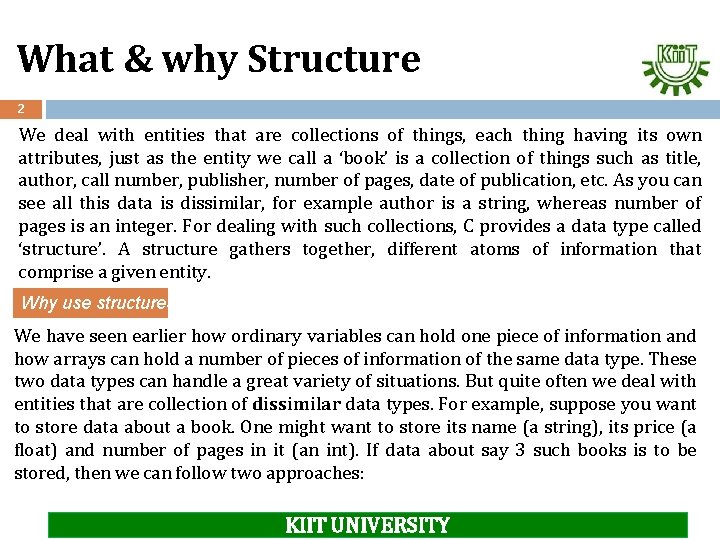
What & why Structure 2 We deal with entities that are collections of things, each thing having its own attributes, just as the entity we call a ‘book’ is a collection of things such as title, author, call number, publisher, number of pages, date of publication, etc. As you can see all this data is dissimilar, for example author is a string, whereas number of pages is an integer. For dealing with such collections, C provides a data type called ‘structure’. A structure gathers together, different atoms of information that comprise a given entity. Why use structures? We have seen earlier how ordinary variables can hold one piece of information and how arrays can hold a number of pieces of information of the same data type. These two data types can handle a great variety of situations. But quite often we deal with entities that are collection of dissimilar data types. For example, suppose you want to store data about a book. One might want to store its name (a string), its price (a float) and number of pages in it (an int). If data about say 3 such books is to be stored, then we can follow two approaches: KIIT UNIVERSITY
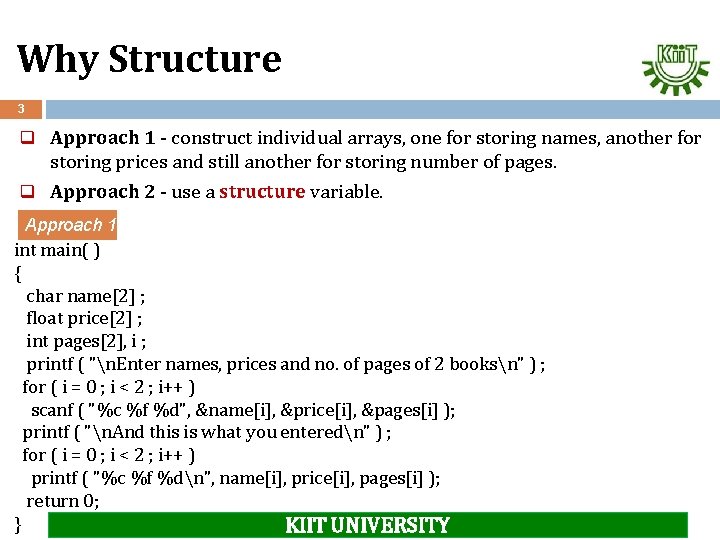
Why Structure 3 q Approach 1 - construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages. q Approach 2 - use a structure variable. Approach 1 int main( ) { char name[2] ; float price[2] ; int pages[2], i ; printf ( "n. Enter names, prices and no. of pages of 2 booksn" ) ; for ( i = 0 ; i < 2 ; i++ ) scanf ( "%c %f %d", &name[i], &price[i], &pages[i] ); printf ( "n. And this is what you enteredn" ) ; for ( i = 0 ; i < 2 ; i++ ) printf ( "%c %f %dn", name[i], price[i], pages[i] ); return 0; } KIIT UNIVERSITY
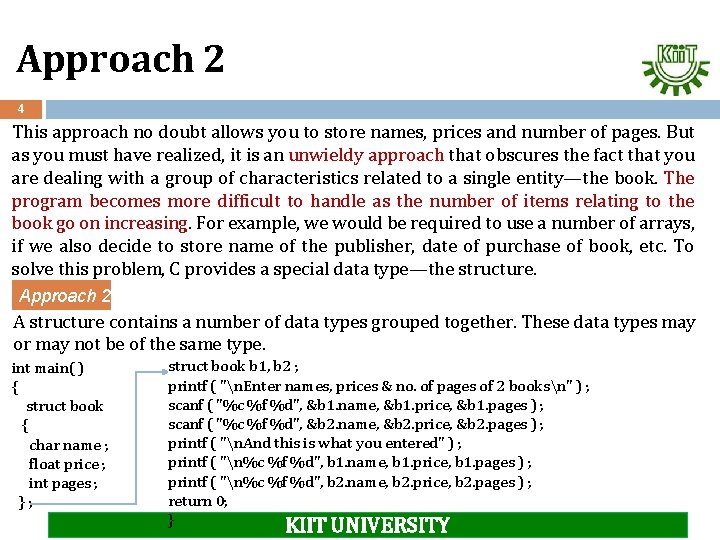
Approach 2 4 This approach no doubt allows you to store names, prices and number of pages. But as you must have realized, it is an unwieldy approach that obscures the fact that you are dealing with a group of characteristics related to a single entity—the book. The program becomes more difficult to handle as the number of items relating to the book go on increasing. For example, we would be required to use a number of arrays, if we also decide to store name of the publisher, date of purchase of book, etc. To solve this problem, C provides a special data type—the structure. Approach 2 A structure contains a number of data types grouped together. These data types may or may not be of the same type. int main( ) { struct book { char name ; float price ; int pages ; }; struct book b 1, b 2 ; printf ( "n. Enter names, prices & no. of pages of 2 booksn" ) ; scanf ( "%c %f %d", &b 1. name, &b 1. price, &b 1. pages ) ; scanf ( "%c %f %d", &b 2. name, &b 2. price, &b 2. pages ) ; printf ( "n. And this is what you entered" ) ; printf ( "n%c %f %d", b 1. name, b 1. price, b 1. pages ) ; printf ( "n%c %f %d", b 2. name, b 2. price, b 2. pages ) ; return 0; } KIIT UNIVERSITY
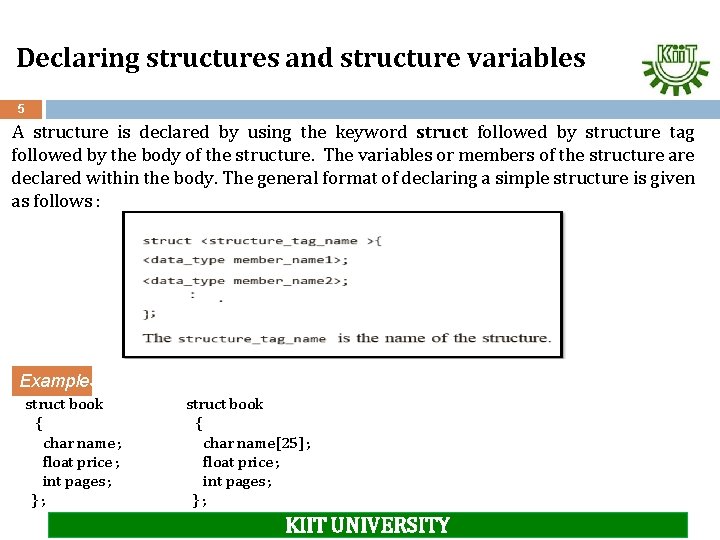
Declaring structures and structure variables 5 A structure is declared by using the keyword struct followed by structure tag followed by the body of the structure. The variables or members of the structure are declared within the body. The general format of declaring a simple structure is given as follows : Examples struct book { char name ; float price ; int pages ; }; struct book { char name[25] ; float price ; int pages ; }; KIIT UNIVERSITY
![Structures declaration type 6 struct book char name25 float price int Structures declaration type 6 struct book { char name[25] ; float price ; int](https://slidetodoc.com/presentation_image_h/aa273ab1efc1320e7663799f47bb4230/image-6.jpg)
Structures declaration type 6 struct book { char name[25] ; float price ; int pages ; }; struct book b 1, b 2, b 3 ; struct book { char name[25] ; float price ; int pages ; } b 1, b 2, b 3 ; struct { char name[25] ; float price ; int pages ; } b 1, b 2, b 3 ; Variable Initialization struct book b 1 = { "Basic", 130. 00, 550 } ; struct book b 2 = { "Physics", 150. 80, 800 } ; KIIT UNIVERSITY
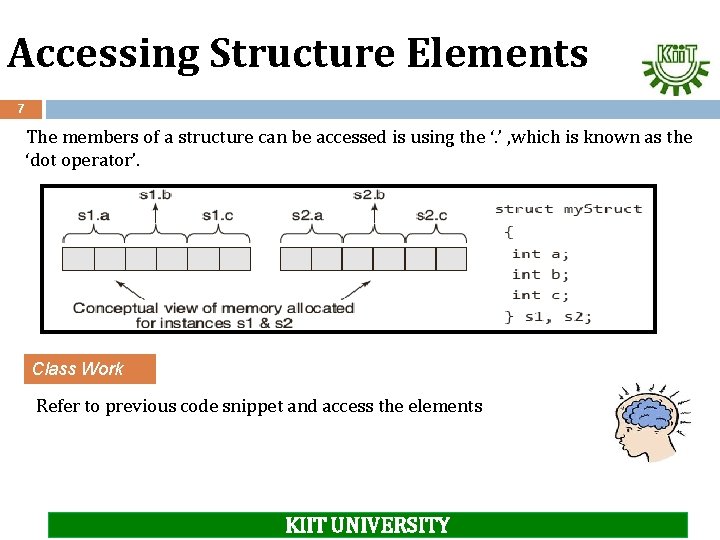
Accessing Structure Elements 7 The members of a structure can be accessed is using the ‘. ’ , which is known as the ‘dot operator’. Class Work Refer to previous code snippet and access the elements KIIT UNIVERSITY
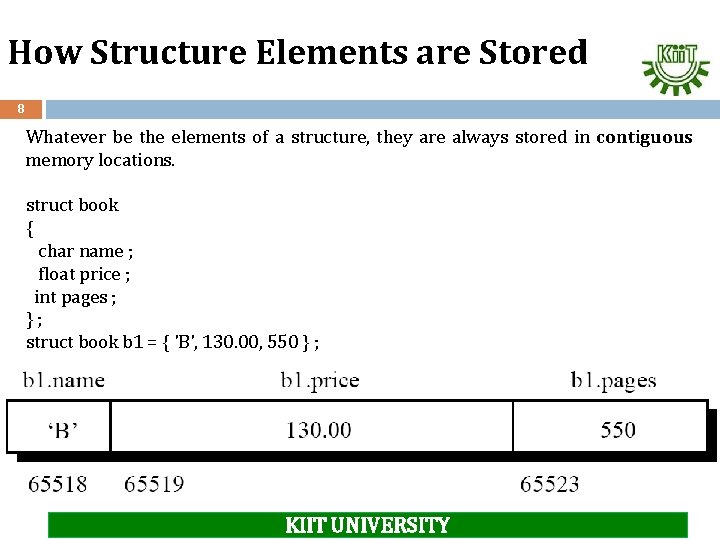
How Structure Elements are Stored 8 Whatever be the elements of a structure, they are always stored in contiguous memory locations. struct book { char name ; float price ; int pages ; }; struct book b 1 = { 'B', 130. 00, 550 } ; KIIT UNIVERSITY
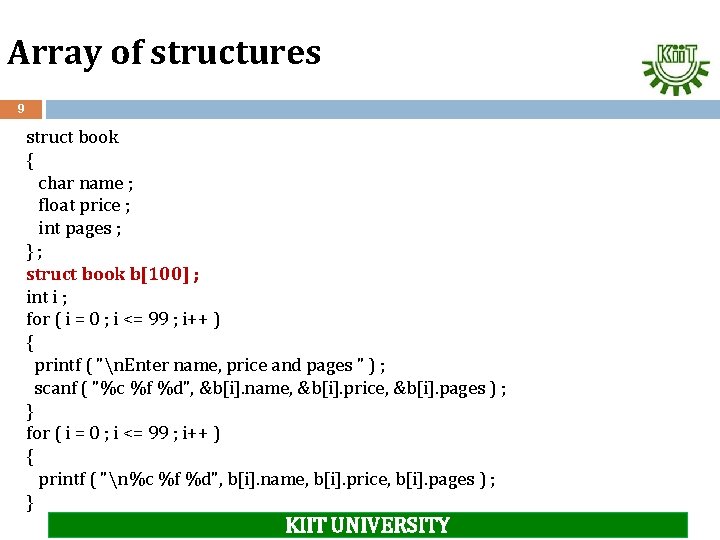
Array of structures 9 struct book { char name ; float price ; int pages ; }; struct book b[100] ; int i ; for ( i = 0 ; i <= 99 ; i++ ) { printf ( "n. Enter name, price and pages " ) ; scanf ( "%c %f %d", &b[i]. name, &b[i]. price, &b[i]. pages ) ; } for ( i = 0 ; i <= 99 ; i++ ) { printf ( "n%c %f %d", b[i]. name, b[i]. price, b[i]. pages ) ; } KIIT UNIVERSITY
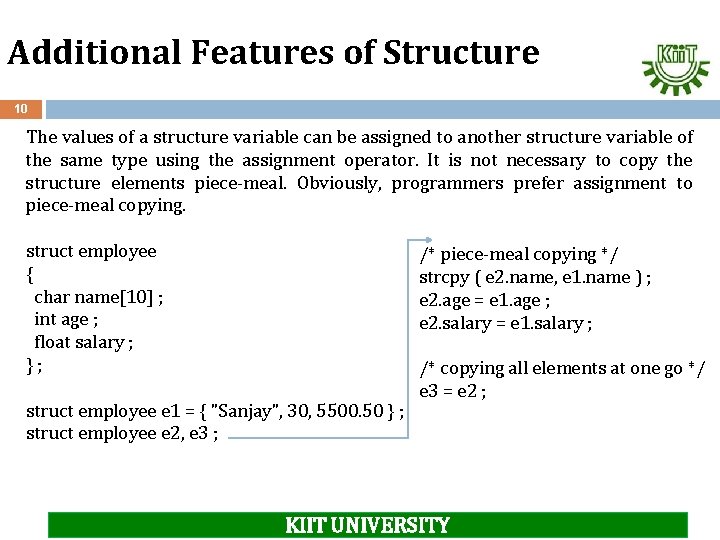
Additional Features of Structure 10 The values of a structure variable can be assigned to another structure variable of the same type using the assignment operator. It is not necessary to copy the structure elements piece-meal. Obviously, programmers prefer assignment to piece-meal copying. struct employee { char name[10] ; int age ; float salary ; }; /* piece-meal copying */ strcpy ( e 2. name, e 1. name ) ; e 2. age = e 1. age ; e 2. salary = e 1. salary ; struct employee e 1 = { "Sanjay", 30, 5500. 50 } ; struct employee e 2, e 3 ; /* copying all elements at one go */ e 3 = e 2 ; KIIT UNIVERSITY
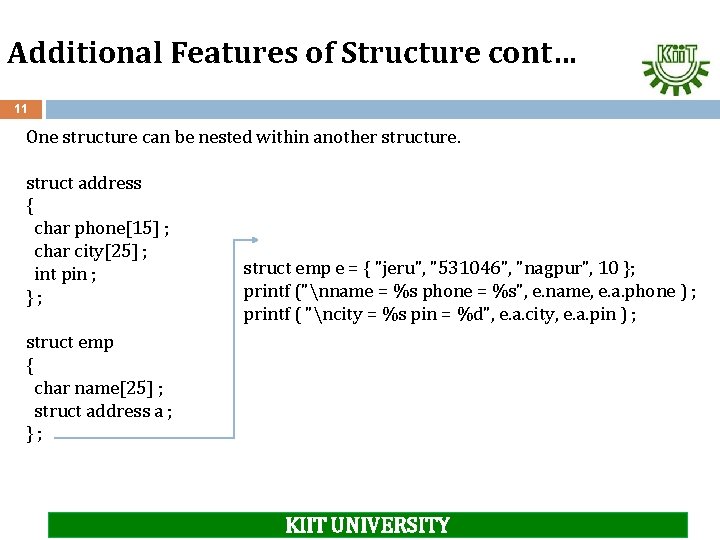
Additional Features of Structure cont… 11 One structure can be nested within another structure. struct address { char phone[15] ; char city[25] ; int pin ; }; struct emp e = { "jeru", "531046", "nagpur", 10 }; printf ("nname = %s phone = %s", e. name, e. a. phone ) ; printf ( "ncity = %s pin = %d", e. a. city, e. a. pin ) ; struct emp { char name[25] ; struct address a ; }; KIIT UNIVERSITY
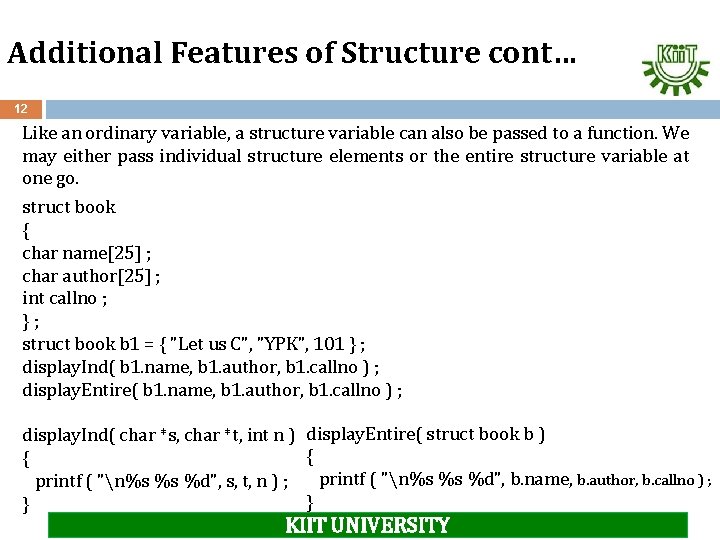
Additional Features of Structure cont… 12 Like an ordinary variable, a structure variable can also be passed to a function. We may either pass individual structure elements or the entire structure variable at one go. struct book { char name[25] ; char author[25] ; int callno ; }; struct book b 1 = { "Let us C", "YPK", 101 } ; display. Ind( b 1. name, b 1. author, b 1. callno ) ; display. Entire( b 1. name, b 1. author, b 1. callno ) ; display. Ind( char *s, char *t, int n ) display. Entire( struct book b ) { { printf ( "n%s %s %d", b. name, b. author, b. callno ) ; printf ( "n%s %s %d", s, t, n ) ; } } KIIT UNIVERSITY
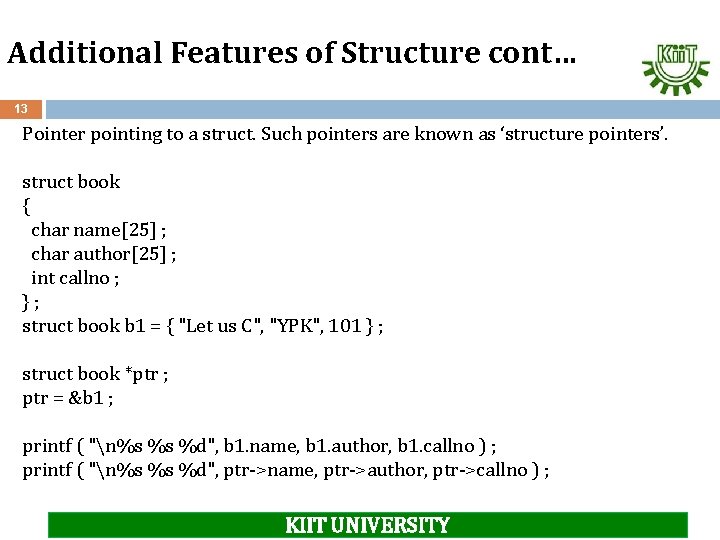
Additional Features of Structure cont… 13 Pointer pointing to a struct. Such pointers are known as ‘structure pointers’. struct book { char name[25] ; char author[25] ; int callno ; }; struct book b 1 = { "Let us C", "YPK", 101 } ; struct book *ptr ; ptr = &b 1 ; printf ( "n%s %s %d", b 1. name, b 1. author, b 1. callno ) ; printf ( "n%s %s %d", ptr->name, ptr->author, ptr->callno ) ; KIIT UNIVERSITY
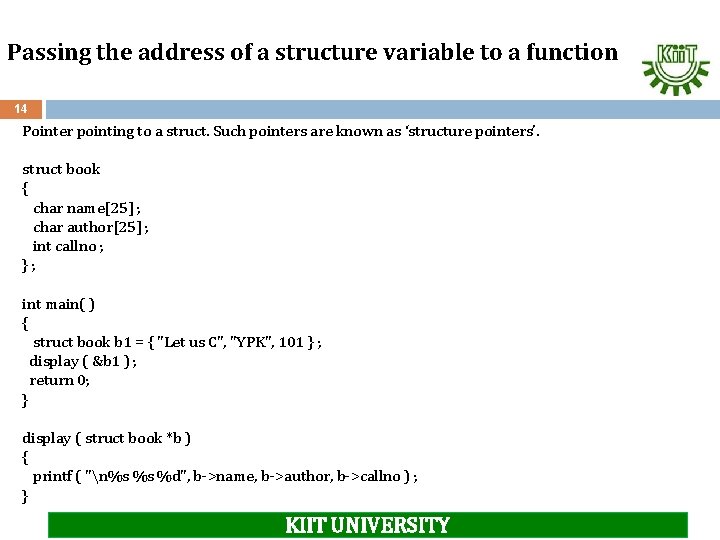
Passing the address of a structure variable to a function 14 Pointer pointing to a struct. Such pointers are known as ‘structure pointers’. struct book { char name[25] ; char author[25] ; int callno ; }; int main( ) { struct book b 1 = { "Let us C", "YPK", 101 } ; display ( &b 1 ) ; return 0; } display ( struct book *b ) { printf ( "n%s %s %d", b->name, b->author, b->callno ) ; } KIIT UNIVERSITY
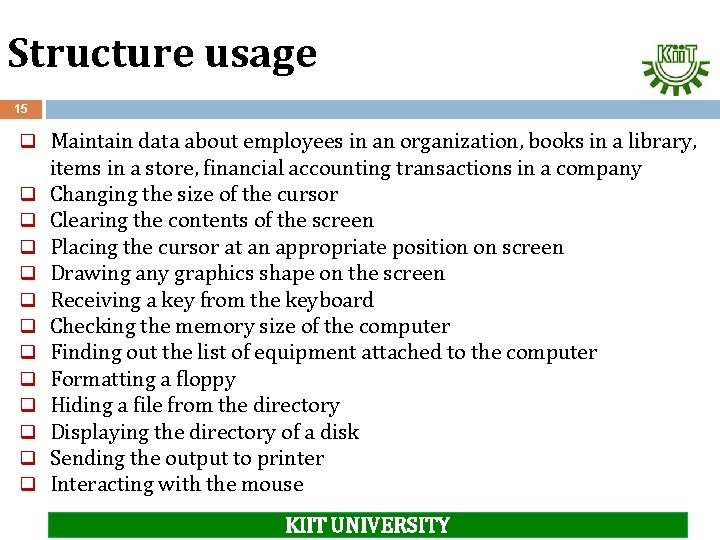
Structure usage 15 q Maintain data about employees in an organization, books in a library, q q q items in a store, financial accounting transactions in a company Changing the size of the cursor Clearing the contents of the screen Placing the cursor at an appropriate position on screen Drawing any graphics shape on the screen Receiving a key from the keyboard Checking the memory size of the computer Finding out the list of equipment attached to the computer Formatting a floppy Hiding a file from the directory Displaying the directory of a disk Sending the output to printer Interacting with the mouse KIIT UNIVERSITY
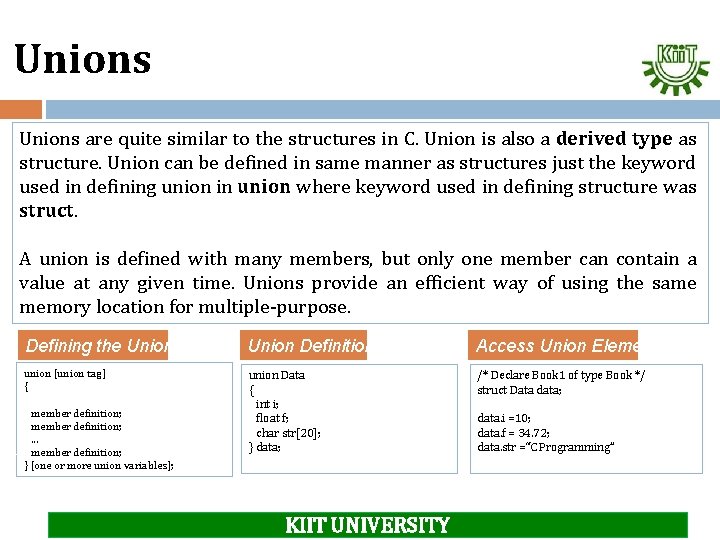
Unions are quite similar to the structures in C. Union is also a derived type as structure. Union can be defined in same manner as structures just the keyword used in defining union in union where keyword used in defining structure was struct. A union is defined with many members, but only one member can contain a value at any given time. Unions provide an efficient way of using the same memory location for multiple-purpose. Defining the Union: Union Definition Access Union Elements union [union tag] { union Data { int i; float f; char str[20]; } data; /* Declare Book 1 of type Book */ struct Data data; member definition; . . . member definition; } [one or more union variables]; KIIT UNIVERSITY data. i =10; data. f = 34. 72; data. str =“C Programming”
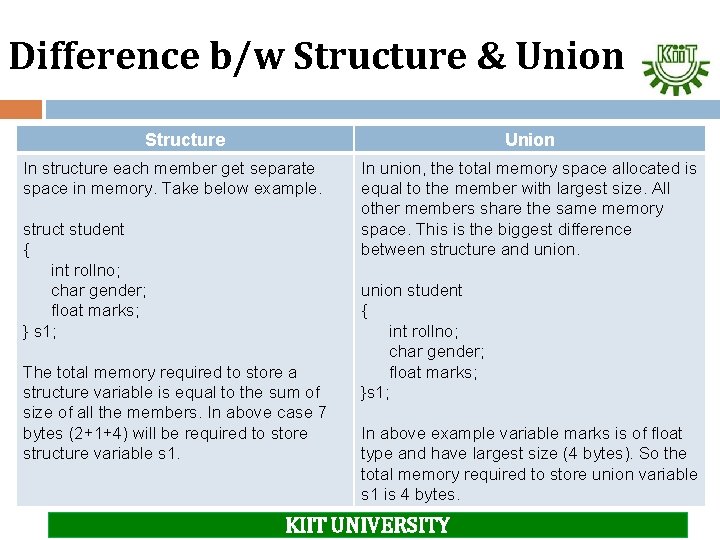
Difference b/w Structure & Union Structure Union In structure each member get separate space in memory. Take below example. struct student { int rollno; char gender; float marks; } s 1; The total memory required to store a structure variable is equal to the sum of size of all the members. In above case 7 bytes (2+1+4) will be required to store structure variable s 1. In union, the total memory space allocated is equal to the member with largest size. All other members share the same memory space. This is the biggest difference between structure and union student { int rollno; char gender; float marks; }s 1; In above example variable marks is of float type and have largest size (4 bytes). So the total memory required to store union variable s 1 is 4 bytes. KIIT UNIVERSITY
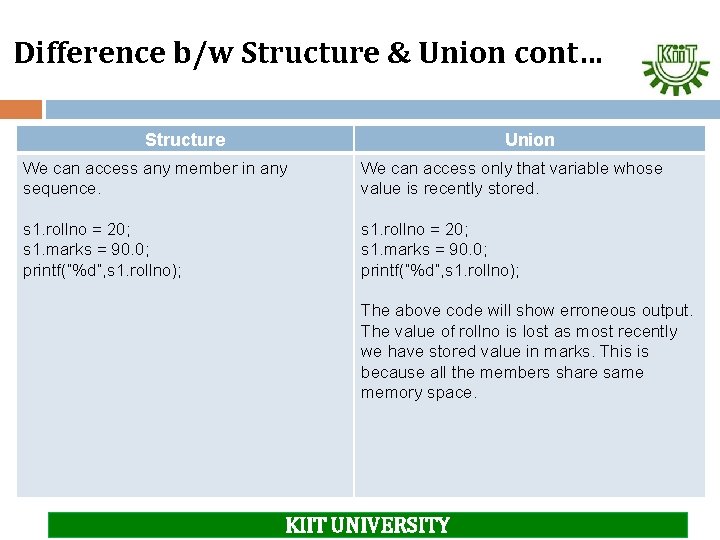
Difference b/w Structure & Union cont… Structure Union We can access any member in any sequence. We can access only that variable whose value is recently stored. s 1. rollno = 20; s 1. marks = 90. 0; printf(“%d”, s 1. rollno); The above code will show erroneous output. The value of rollno is lost as most recently we have stored value in marks. This is because all the members share same memory space. KIIT UNIVERSITY
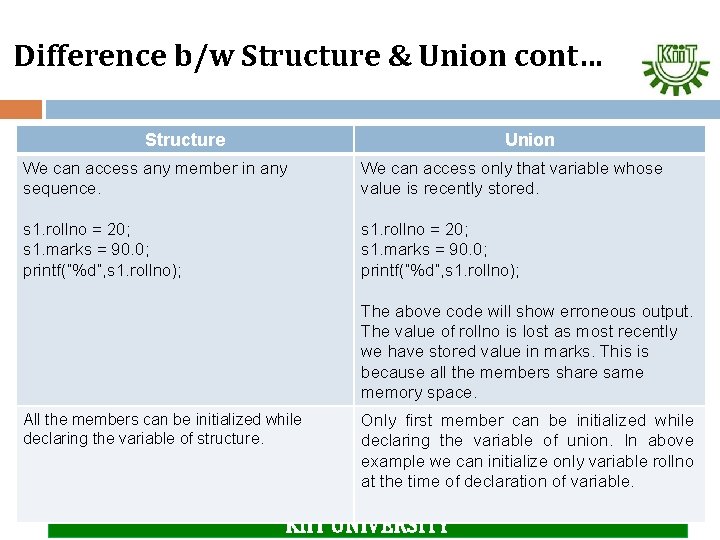
Difference b/w Structure & Union cont… Structure Union We can access any member in any sequence. We can access only that variable whose value is recently stored. s 1. rollno = 20; s 1. marks = 90. 0; printf(“%d”, s 1. rollno); The above code will show erroneous output. The value of rollno is lost as most recently we have stored value in marks. This is because all the members share same memory space. All the members can be initialized while declaring the variable of structure. Only first member can be initialized while declaring the variable of union. In above example we can initialize only variable rollno at the time of declaration of variable. KIIT UNIVERSITY
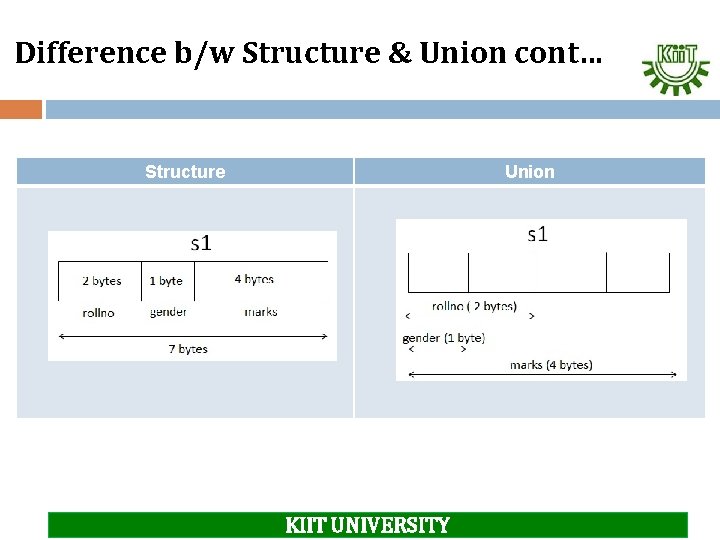
Difference b/w Structure & Union cont… Structure Union KIIT UNIVERSITY
![Union of Structures struct a int i char c2 struct b Union of Structures struct a { int i; char c[2]; }; struct b {](https://slidetodoc.com/presentation_image_h/aa273ab1efc1320e7663799f47bb4230/image-21.jpg)
Union of Structures struct a { int i; char c[2]; }; struct b { int j; char d[2]; }; union z { struct a key; struct b data; }; union z strange; strange. key. i = 512; strange. data. d[0] = 0; strange. data. d[1] = 32; KIIT UNIVERSITY
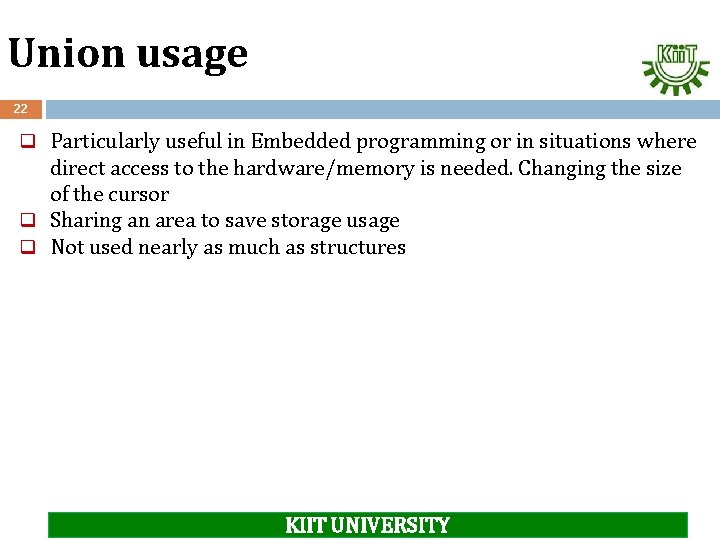
Union usage 22 q Particularly useful in Embedded programming or in situations where direct access to the hardware/memory is needed. Changing the size of the cursor q Sharing an area to save storage usage q Not used nearly as much as structures KIIT UNIVERSITY
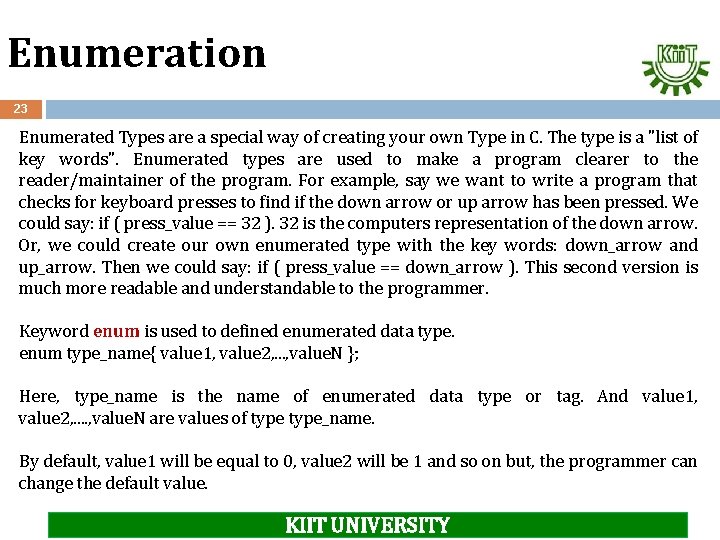
Enumeration 23 Enumerated Types are a special way of creating your own Type in C. The type is a "list of key words". Enumerated types are used to make a program clearer to the reader/maintainer of the program. For example, say we want to write a program that checks for keyboard presses to find if the down arrow or up arrow has been pressed. We could say: if ( press_value == 32 ). 32 is the computers representation of the down arrow. Or, we could create our own enumerated type with the key words: down_arrow and up_arrow. Then we could say: if ( press_value == down_arrow ). This second version is much more readable and understandable to the programmer. Keyword enum is used to defined enumerated data type. enum type_name{ value 1, value 2, . . . , value. N }; Here, type_name is the name of enumerated data type or tag. And value 1, value 2, . . , value. N are values of type_name. By default, value 1 will be equal to 0, value 2 will be 1 and so on but, the programmer can change the default value. KIIT UNIVERSITY
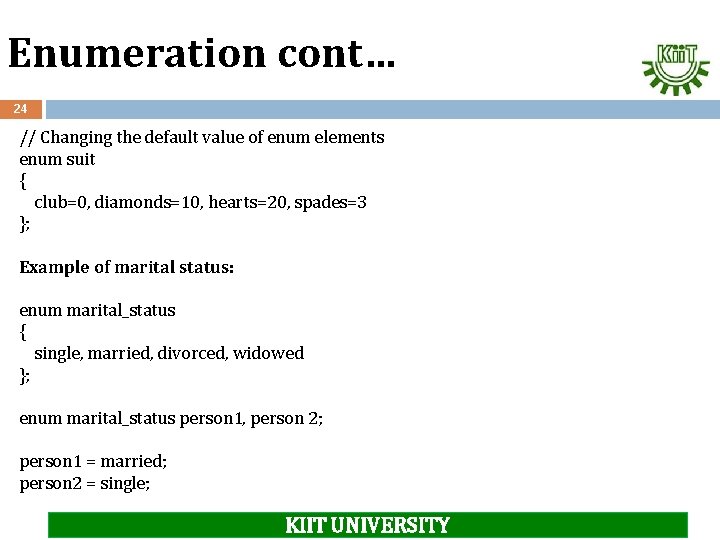
Enumeration cont… 24 // Changing the default value of enum elements enum suit { club=0, diamonds=10, hearts=20, spades=3 }; Example of marital status: enum marital_status { single, married, divorced, widowed }; enum marital_status person 1, person 2; person 1 = married; person 2 = single; KIIT UNIVERSITY
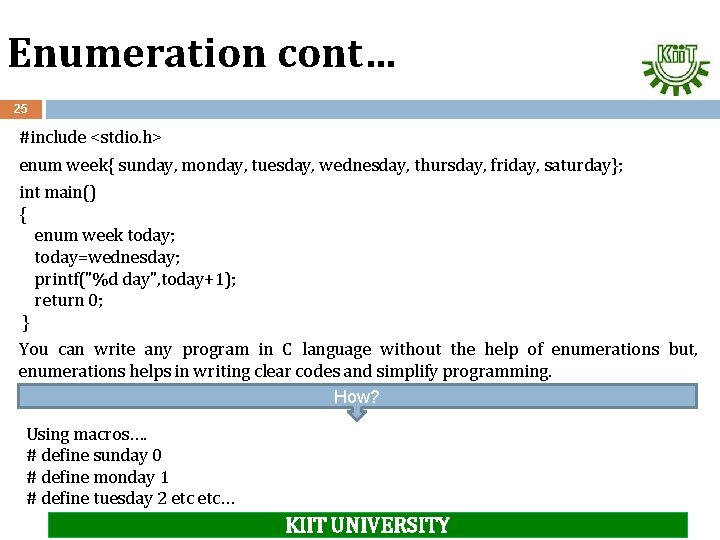
Enumeration cont… 25 #include <stdio. h> enum week{ sunday, monday, tuesday, wednesday, thursday, friday, saturday}; int main() { enum week today; today=wednesday; printf("%d day", today+1); return 0; } You can write any program in C language without the help of enumerations but, enumerations helps in writing clear codes and simplify programming. How? Using macros…. # define sunday 0 # define monday 1 # define tuesday 2 etc… KIIT UNIVERSITY
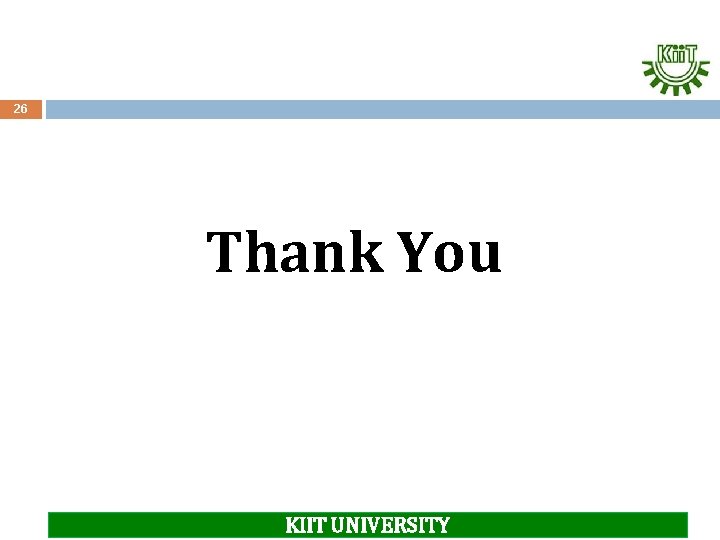
26 Thank You KIIT UNIVERSITY
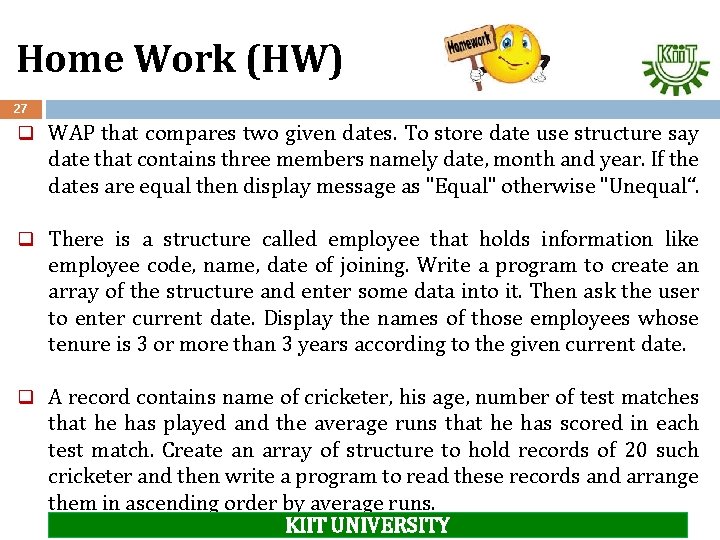
Home Work (HW) 27 q WAP that compares two given dates. To store date use structure say date that contains three members namely date, month and year. If the dates are equal then display message as "Equal" otherwise "Unequal“. q There is a structure called employee that holds information like employee code, name, date of joining. Write a program to create an array of the structure and enter some data into it. Then ask the user to enter current date. Display the names of those employees whose tenure is 3 or more than 3 years according to the given current date. q A record contains name of cricketer, his age, number of test matches that he has played and the average runs that he has scored in each test match. Create an array of structure to hold records of 20 such cricketer and then write a program to read these records and arrange them in ascending order by average runs. KIIT UNIVERSITY
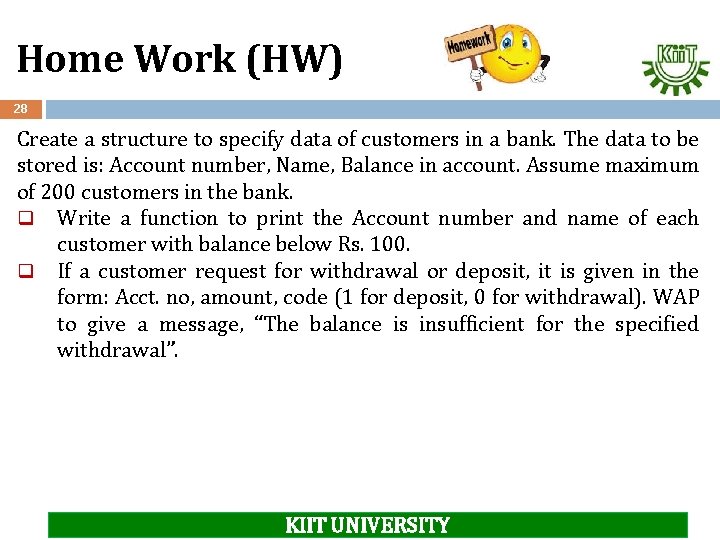
Home Work (HW) 28 Create a structure to specify data of customers in a bank. The data to be stored is: Account number, Name, Balance in account. Assume maximum of 200 customers in the bank. q Write a function to print the Account number and name of each customer with balance below Rs. 100. q If a customer request for withdrawal or deposit, it is given in the form: Acct. no, amount, code (1 for deposit, 0 for withdrawal). WAP to give a message, “The balance is insufficient for the specified withdrawal”. KIIT UNIVERSITY
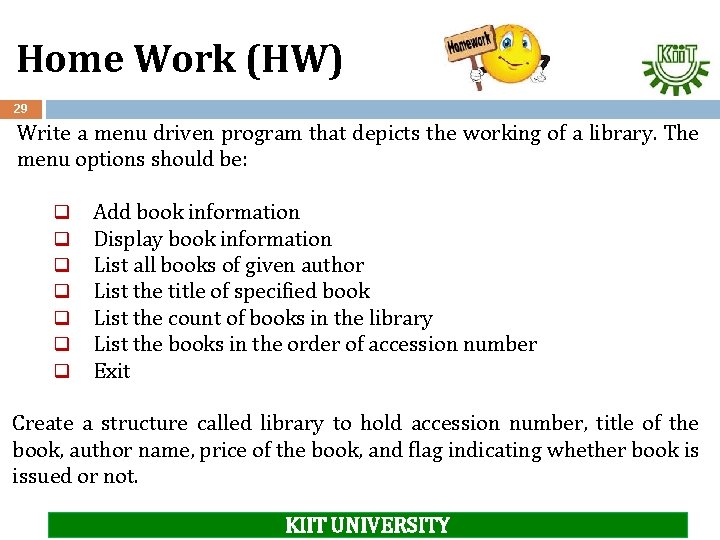
Home Work (HW) 29 Write a menu driven program that depicts the working of a library. The menu options should be: q q q q Add book information Display book information List all books of given author List the title of specified book List the count of books in the library List the books in the order of accession number Exit Create a structure called library to hold accession number, title of the book, author name, price of the book, and flag indicating whether book is issued or not. KIIT UNIVERSITY