COSC 1306 COMPUTER SCIENCE AND PROGRAMMING JehanFranois Pris
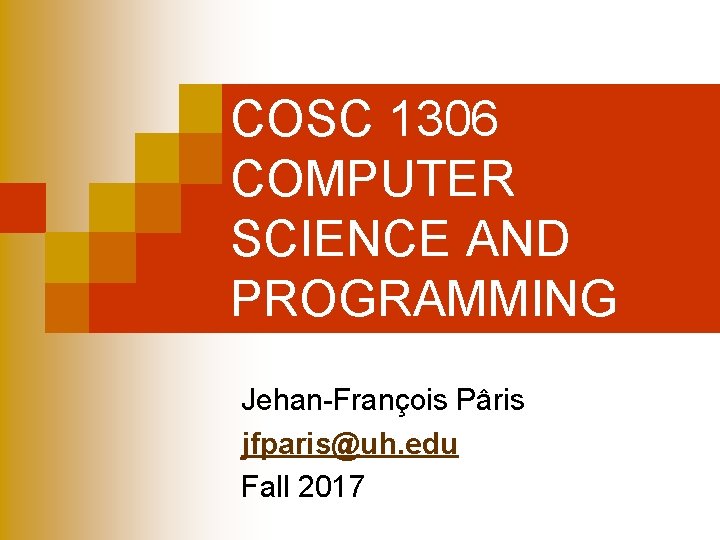
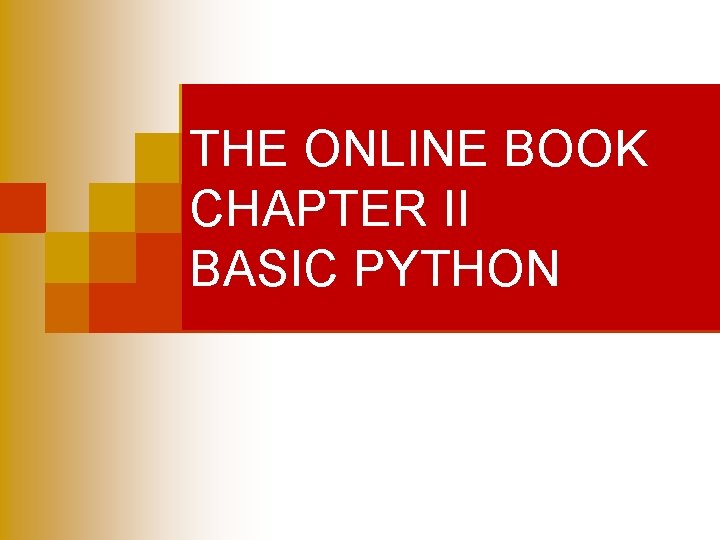
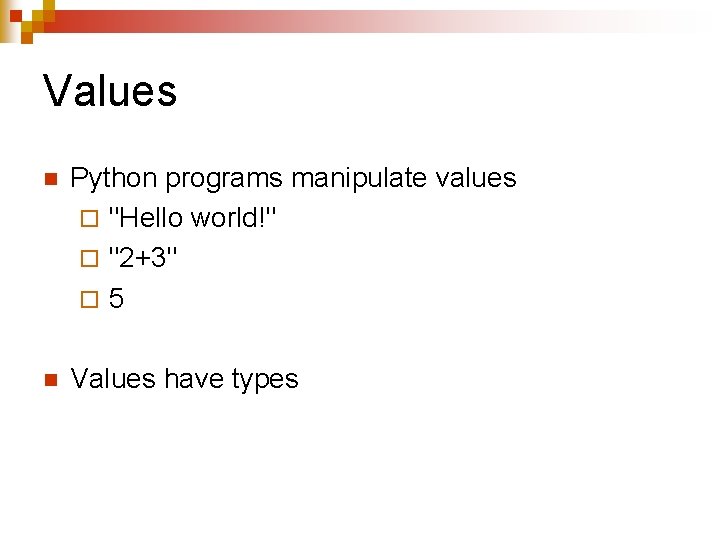
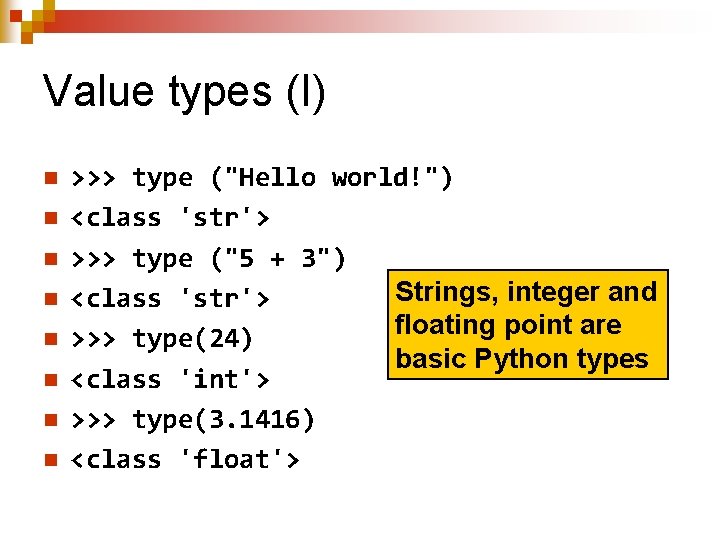
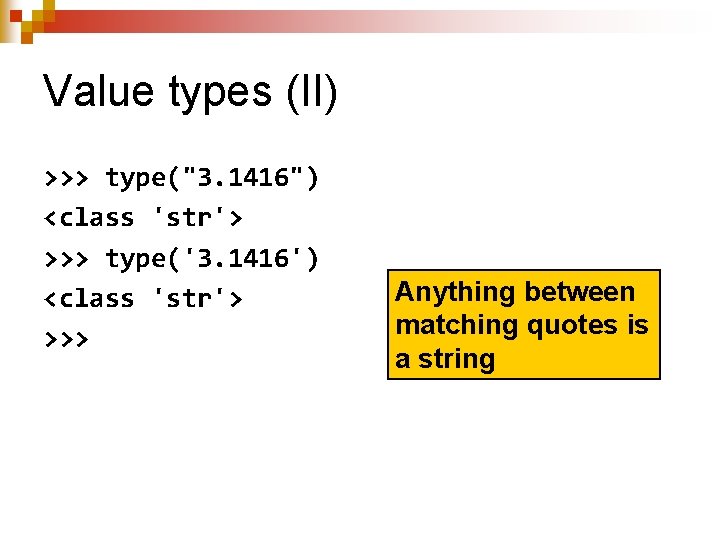
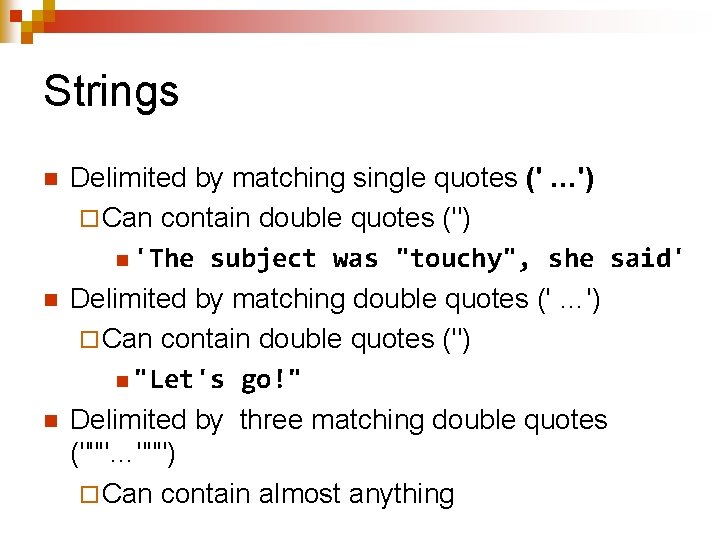
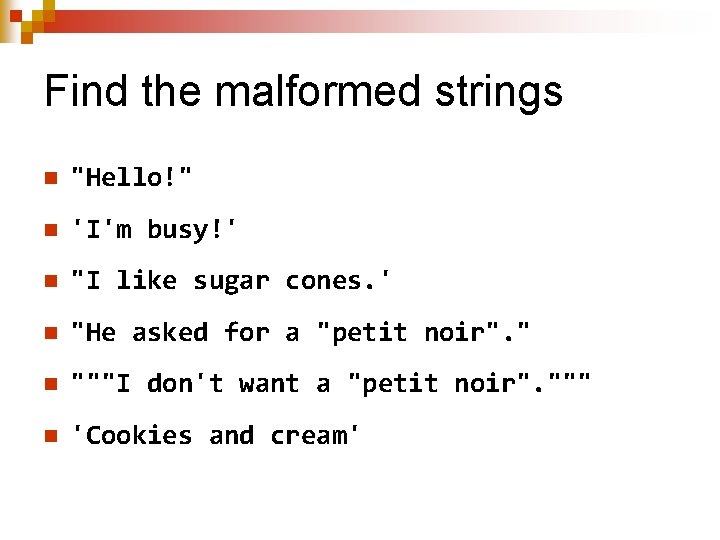
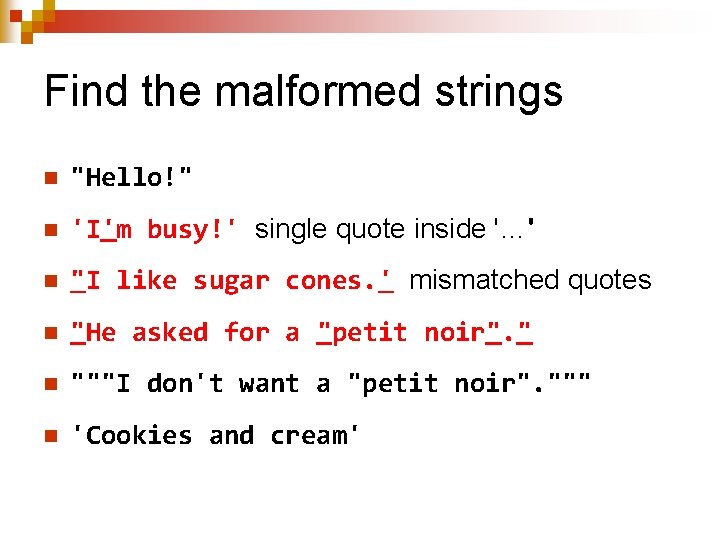
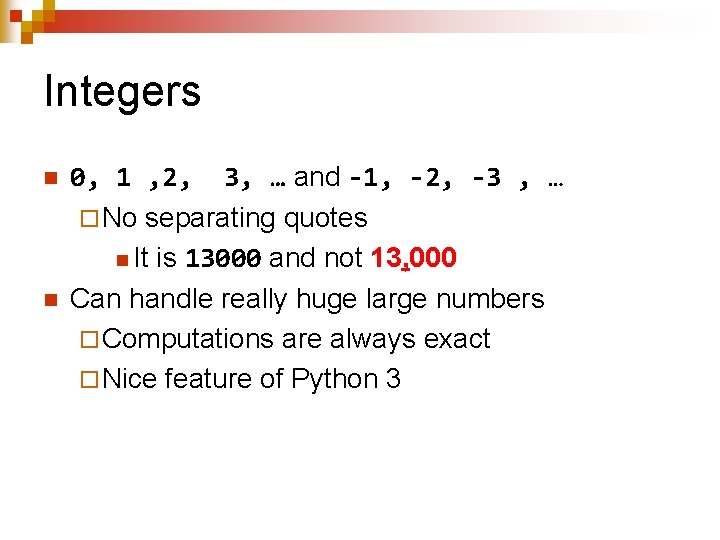
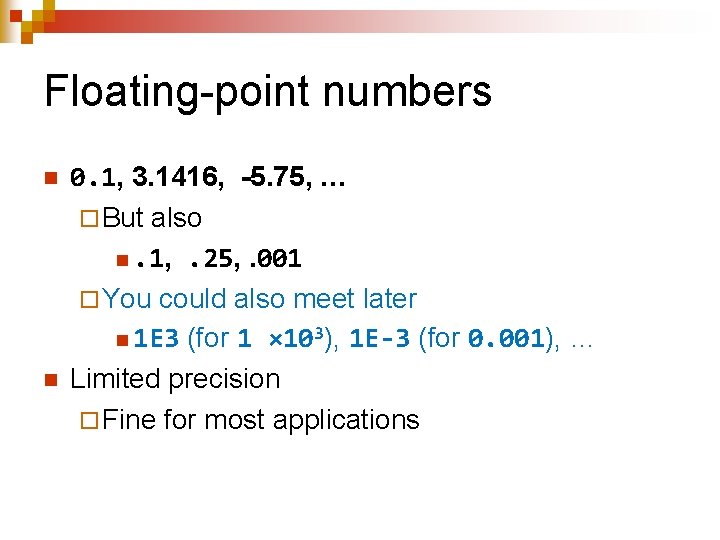
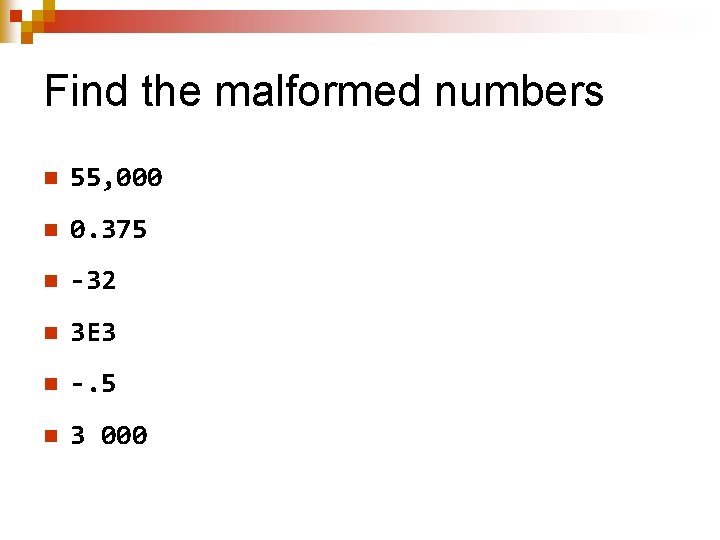
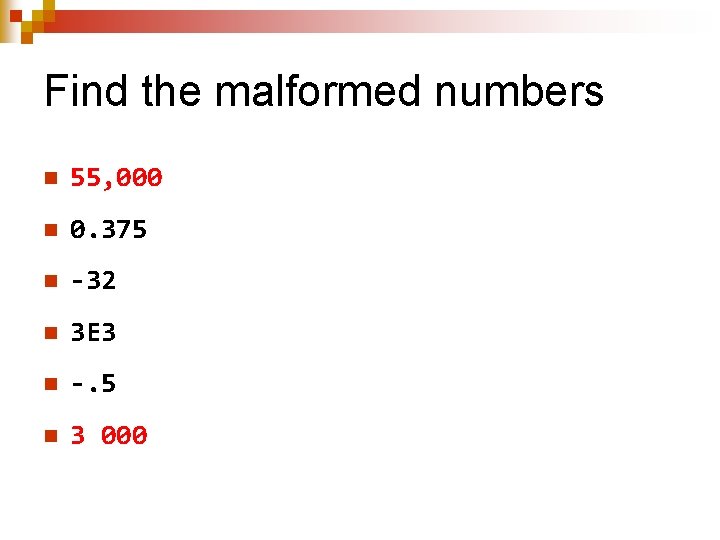
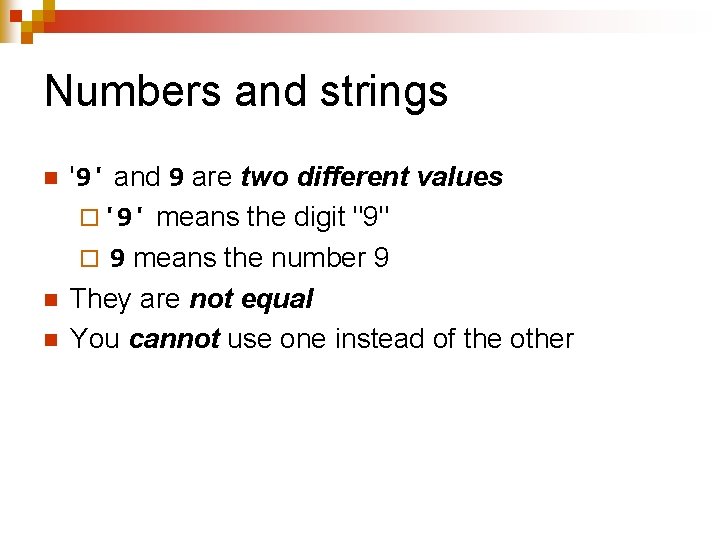
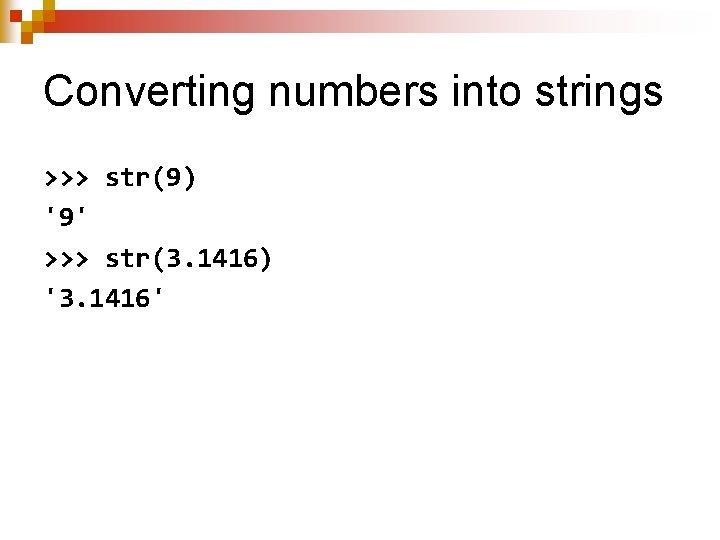
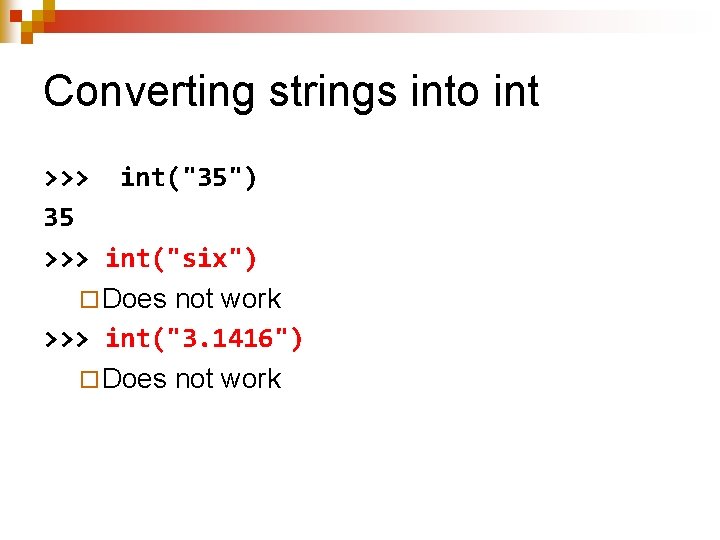
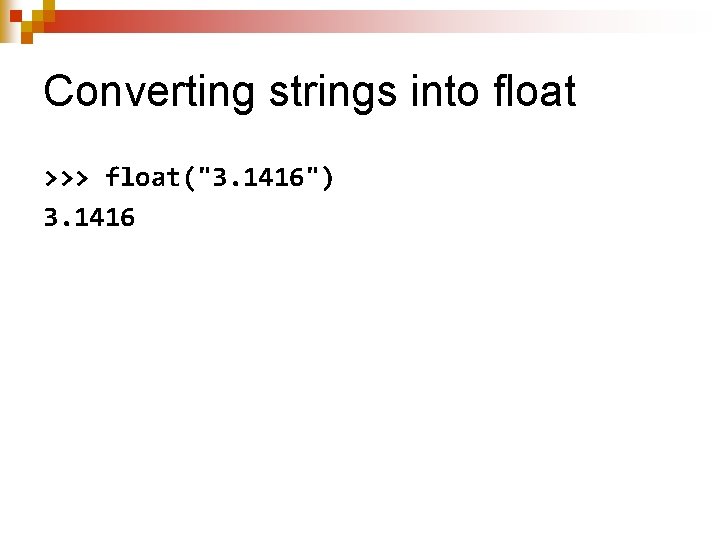
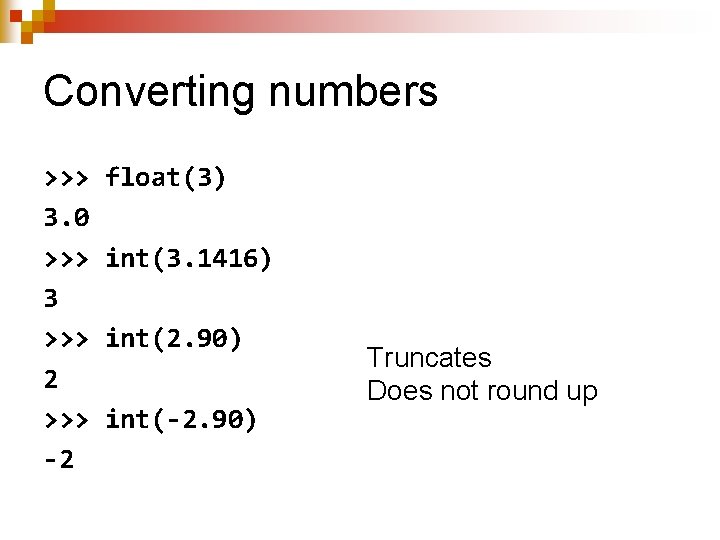
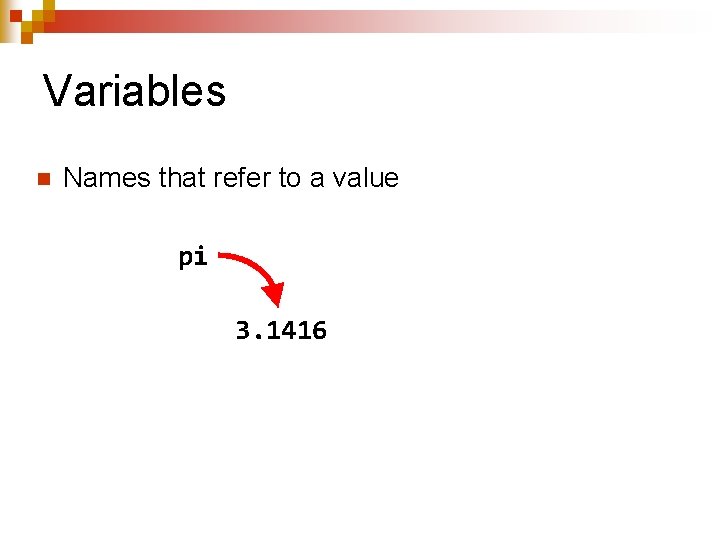
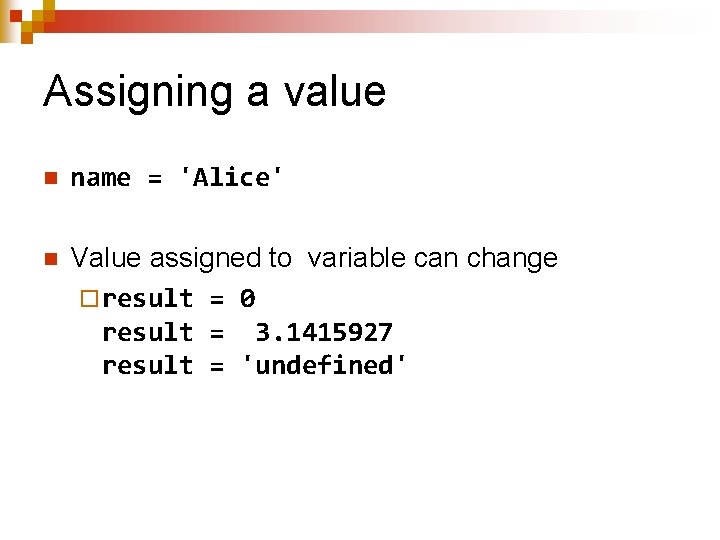
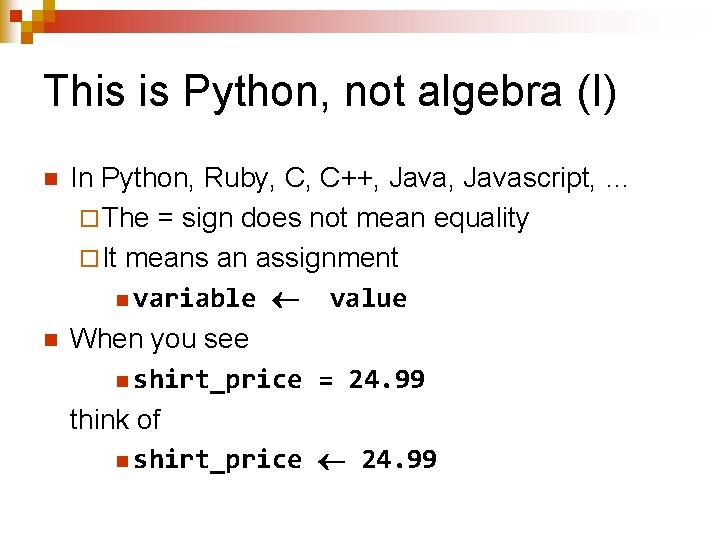
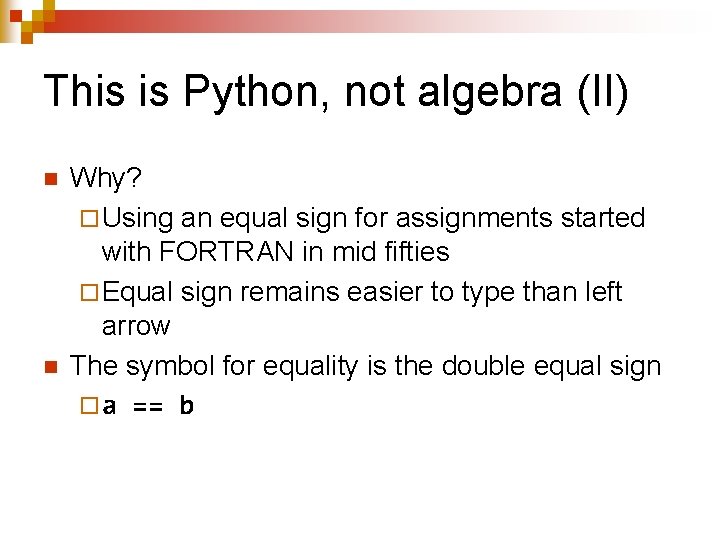
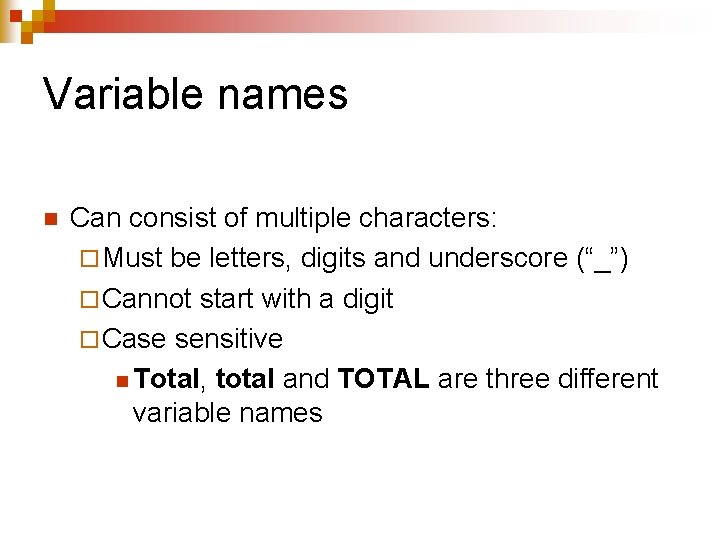
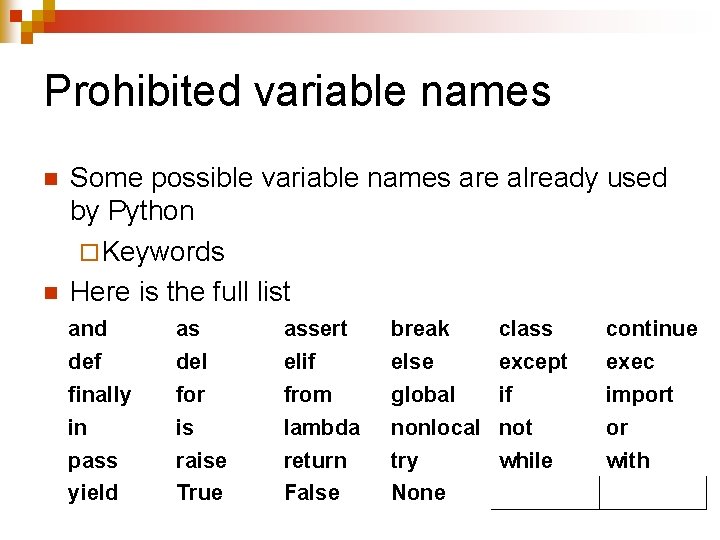
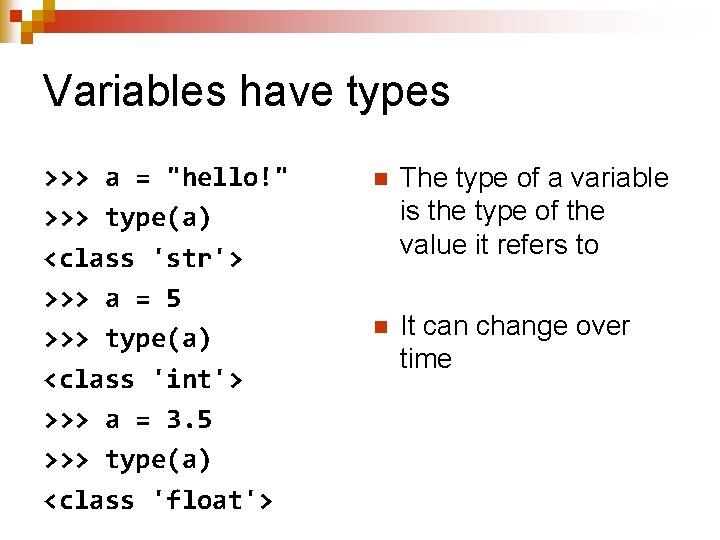
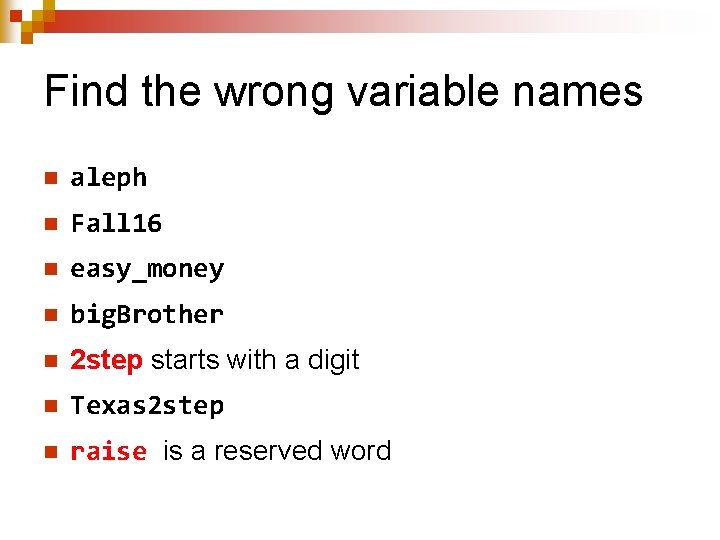
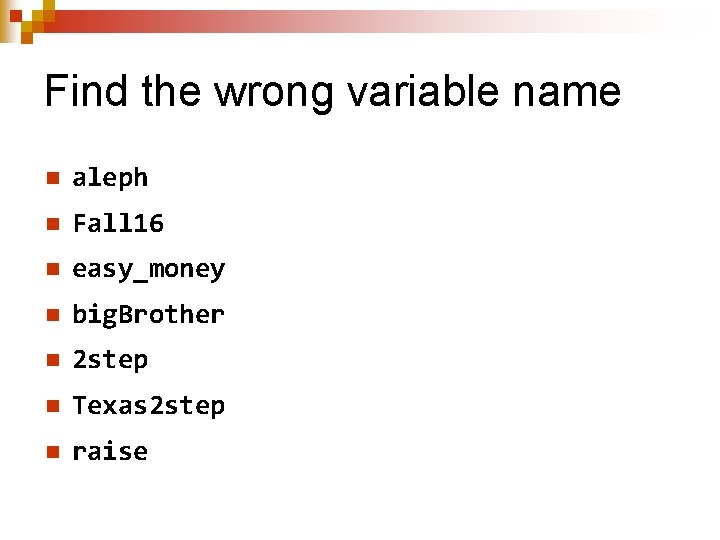
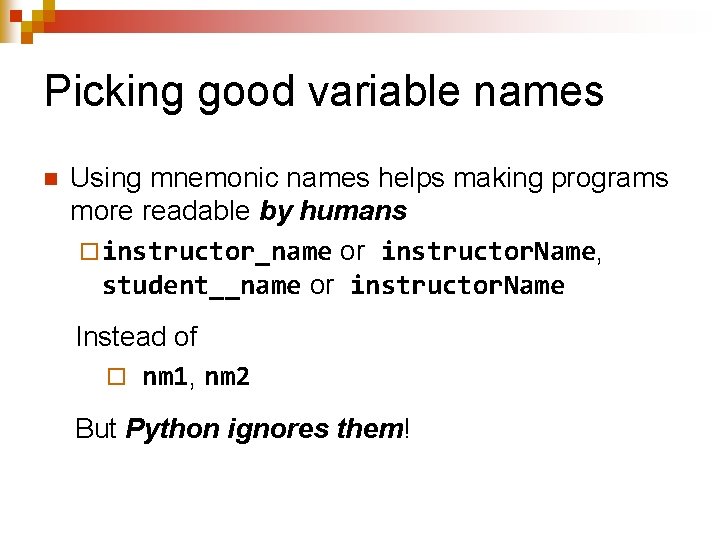
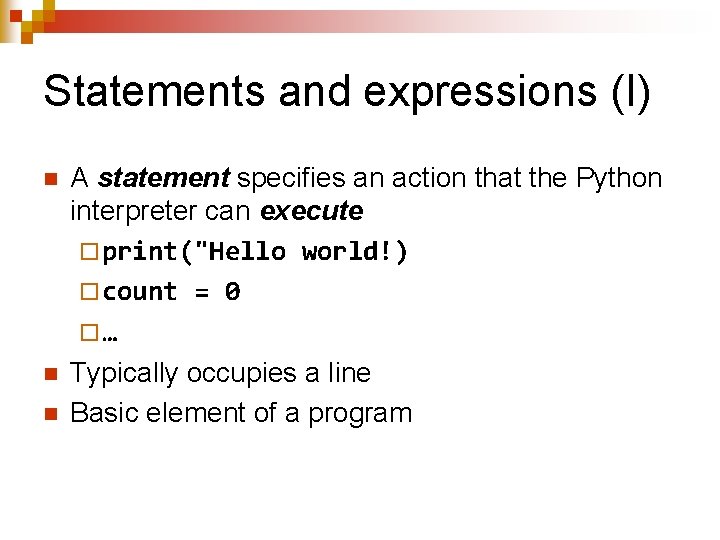
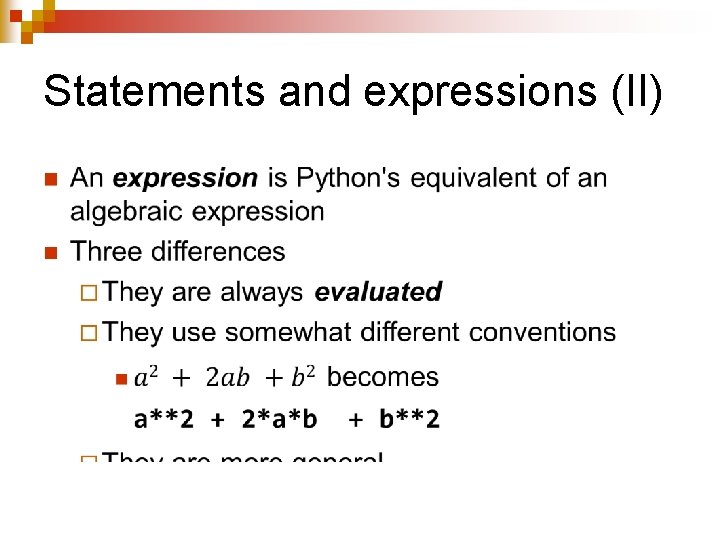
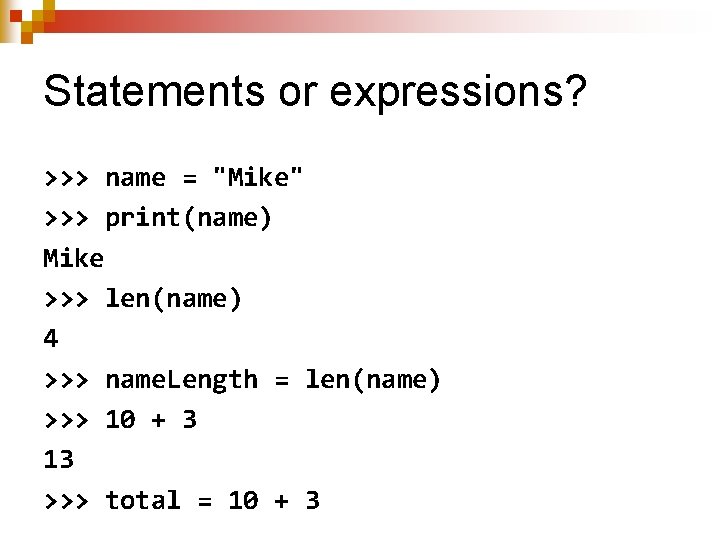
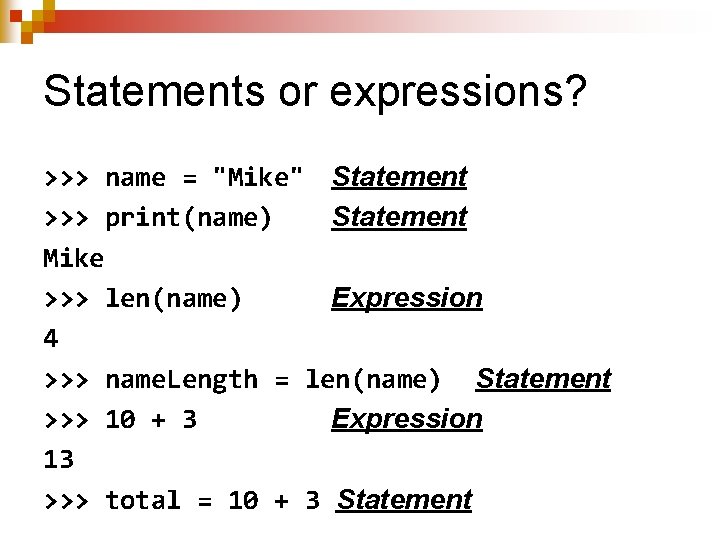
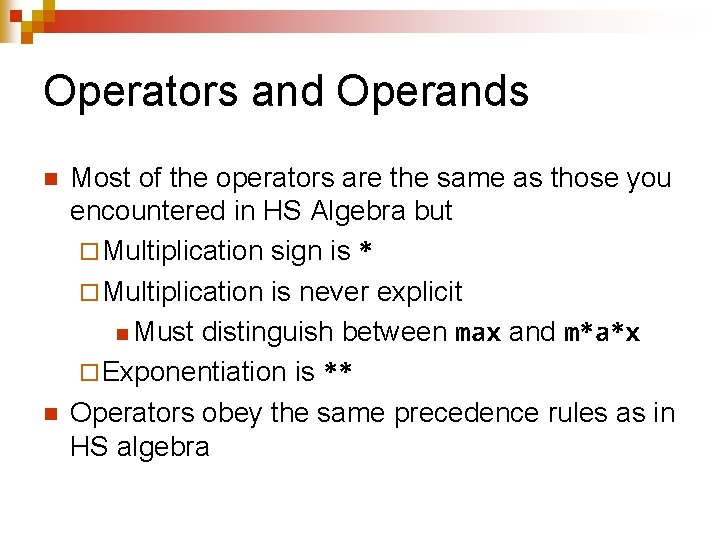
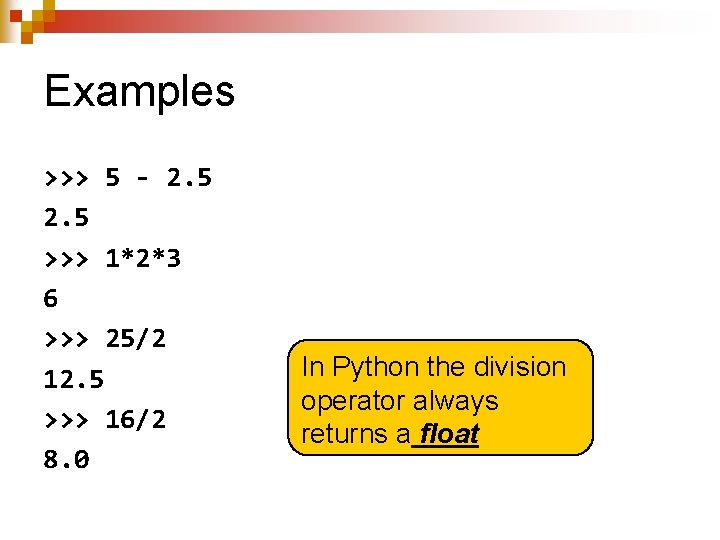
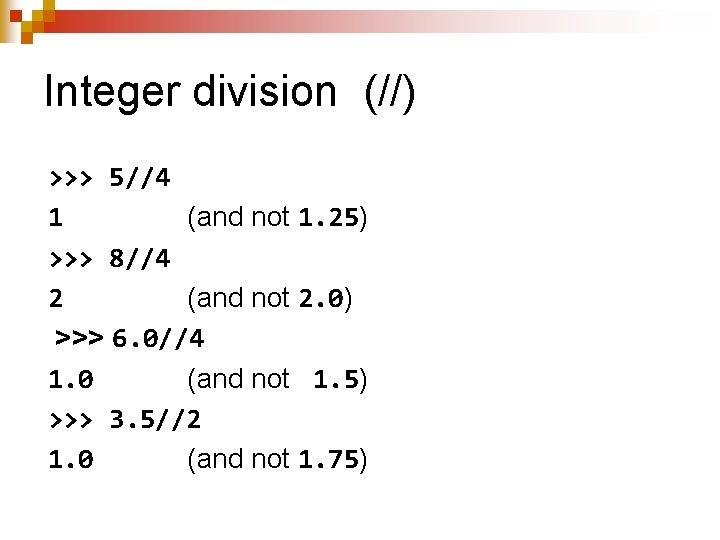
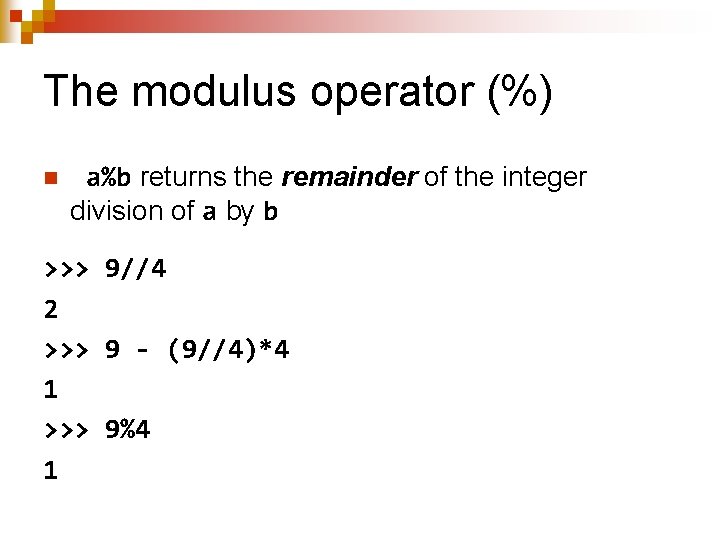
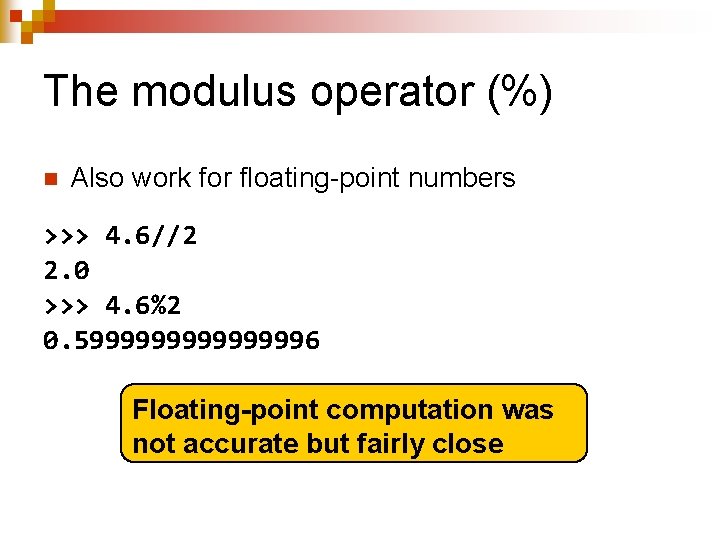
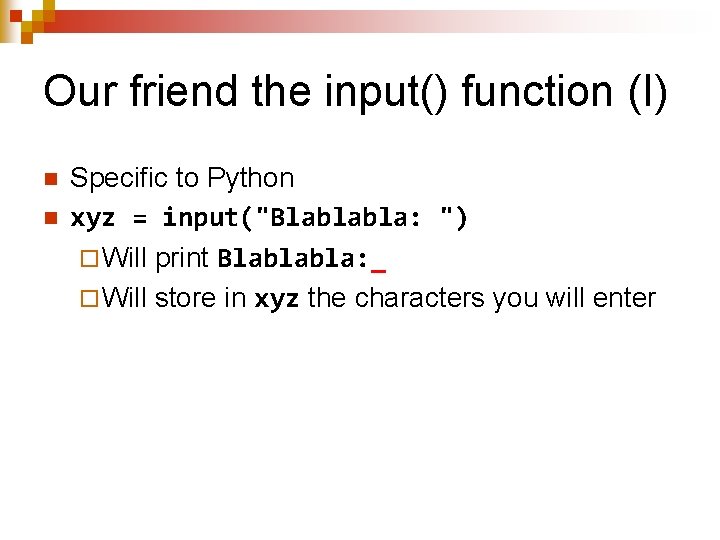
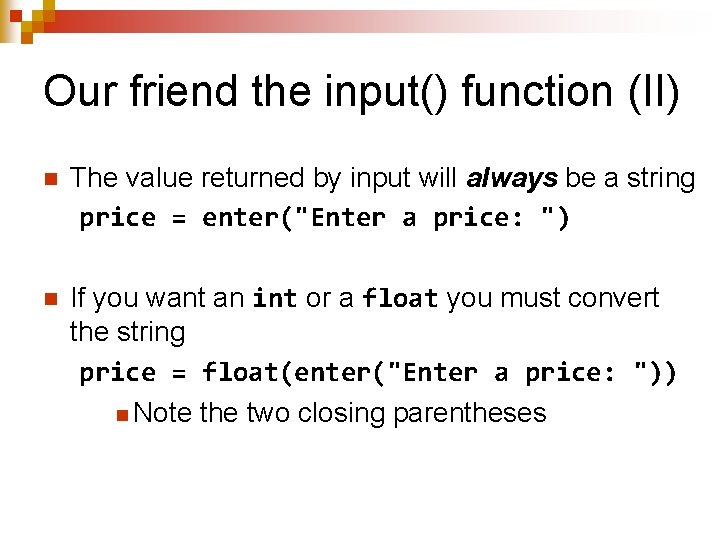
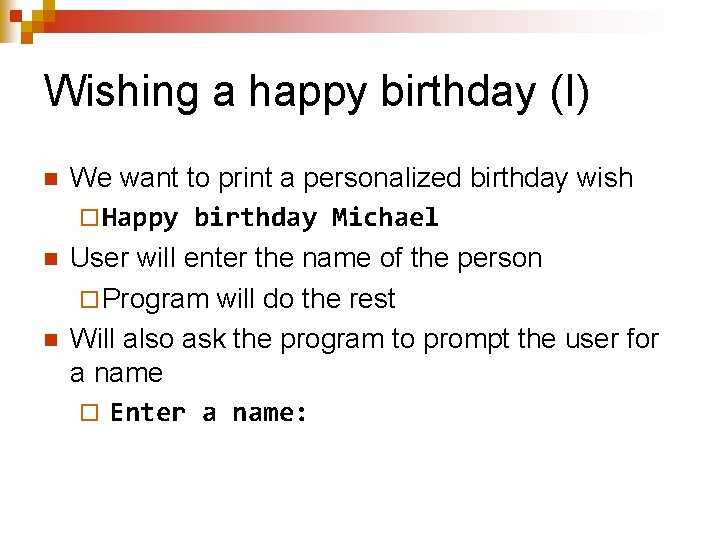
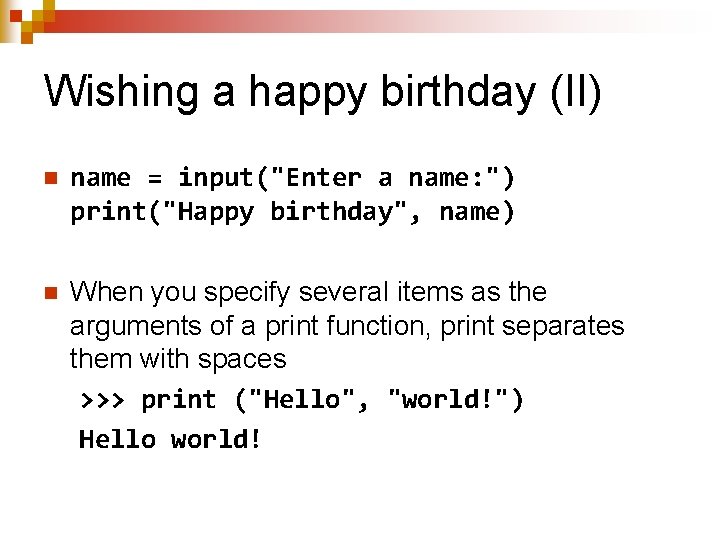
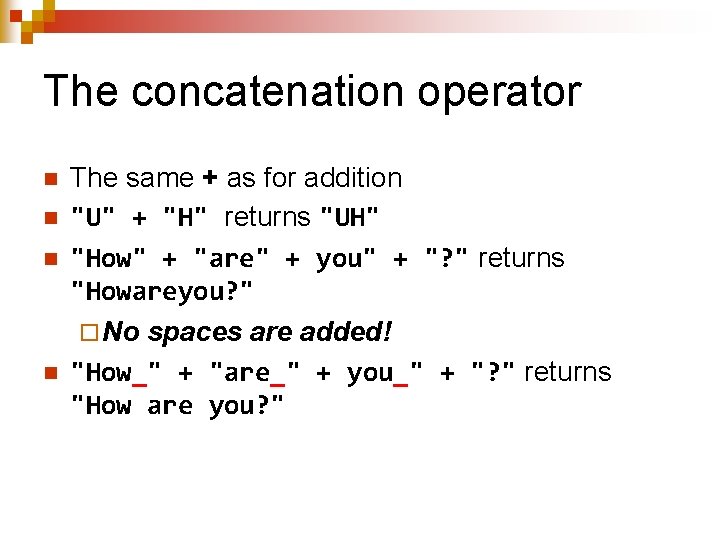
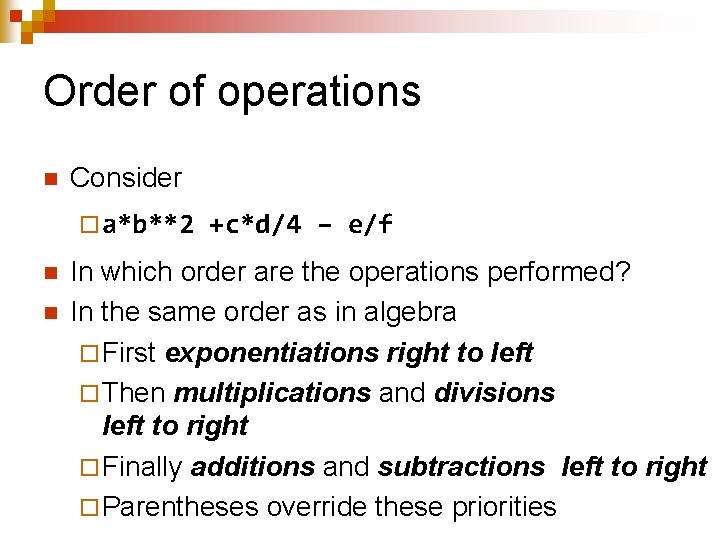
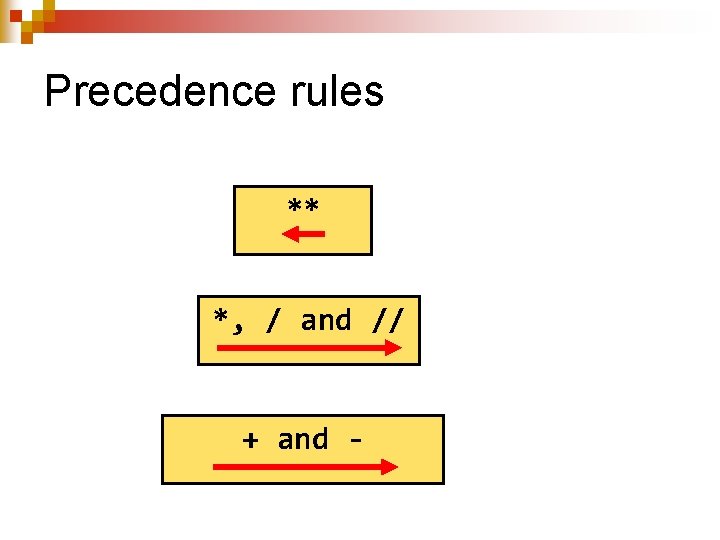
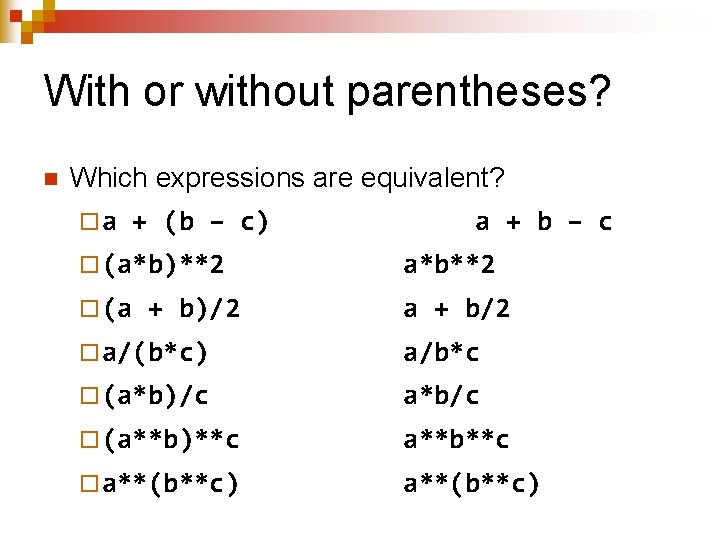
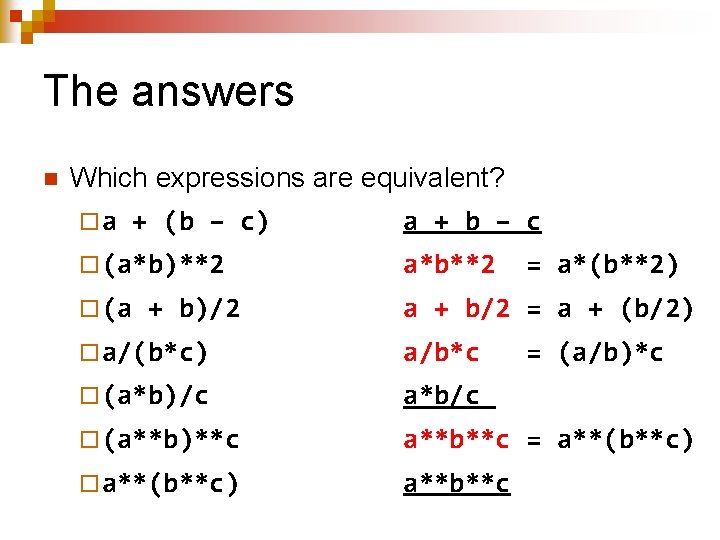
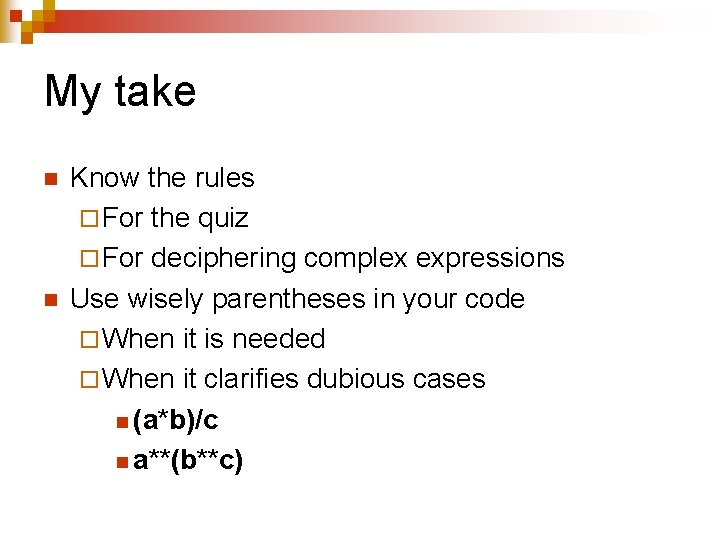
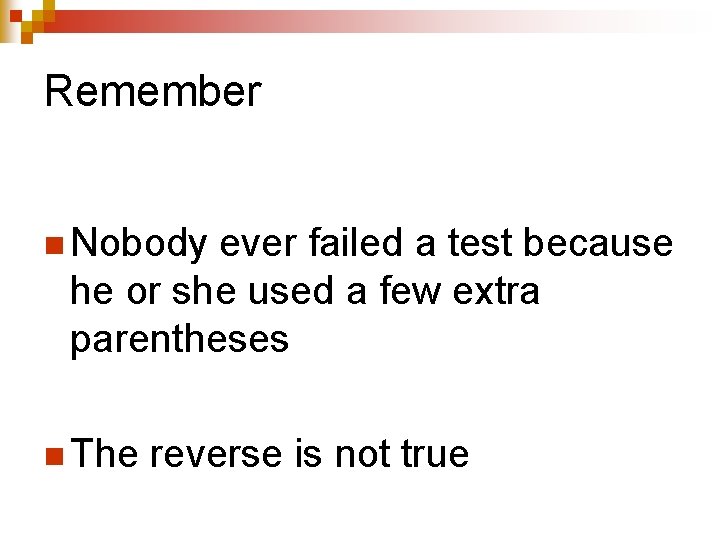
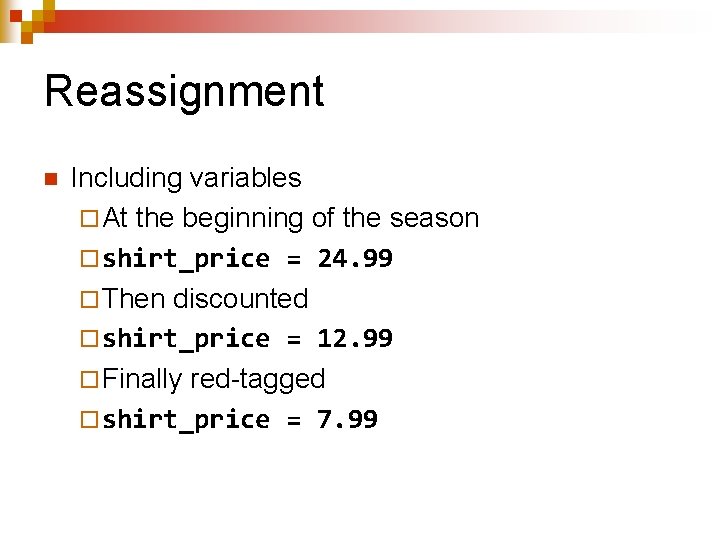
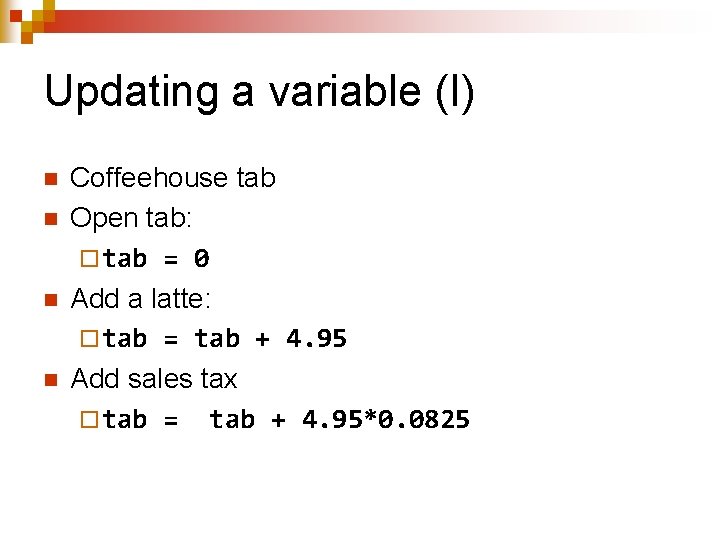
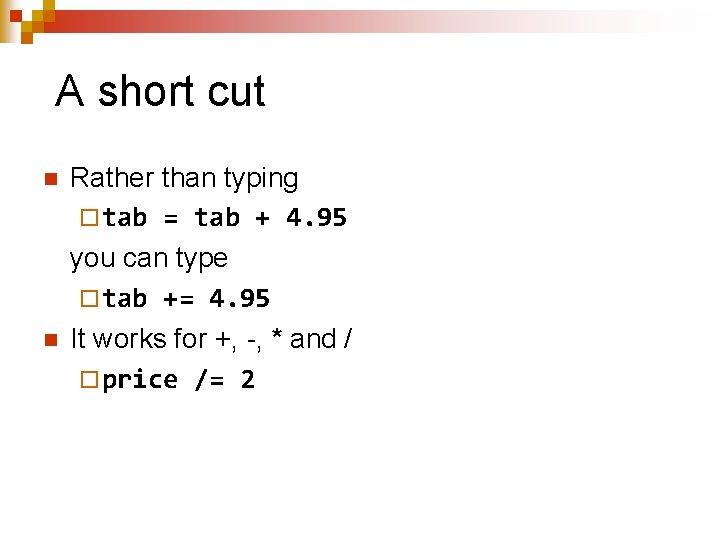
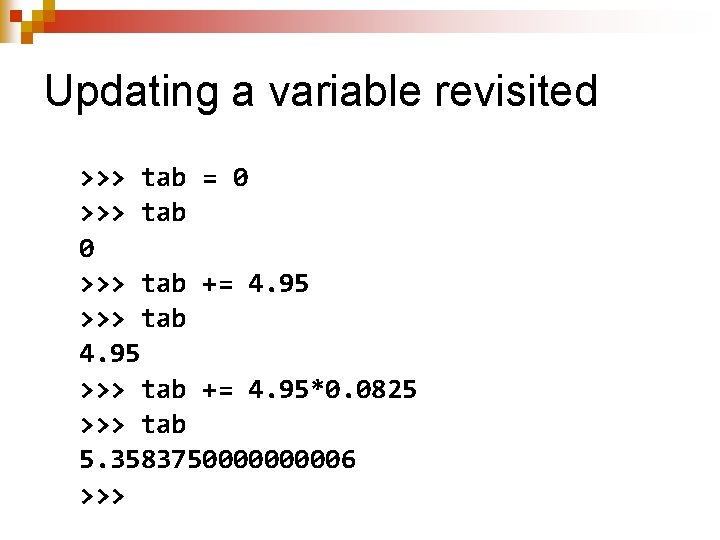
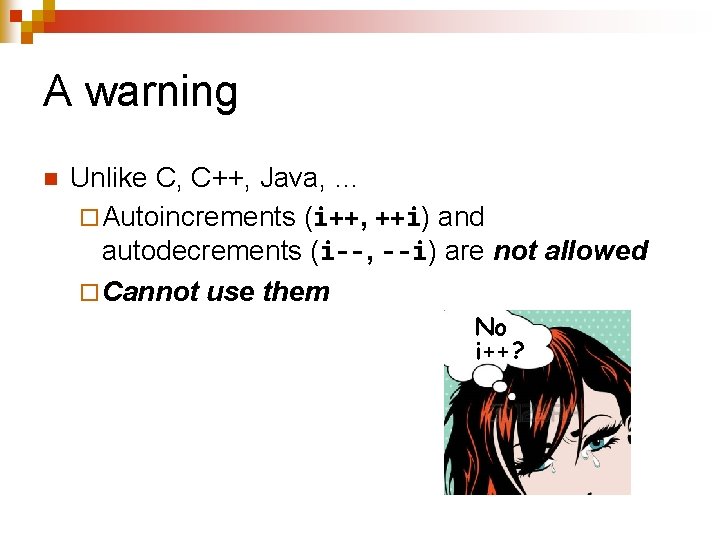
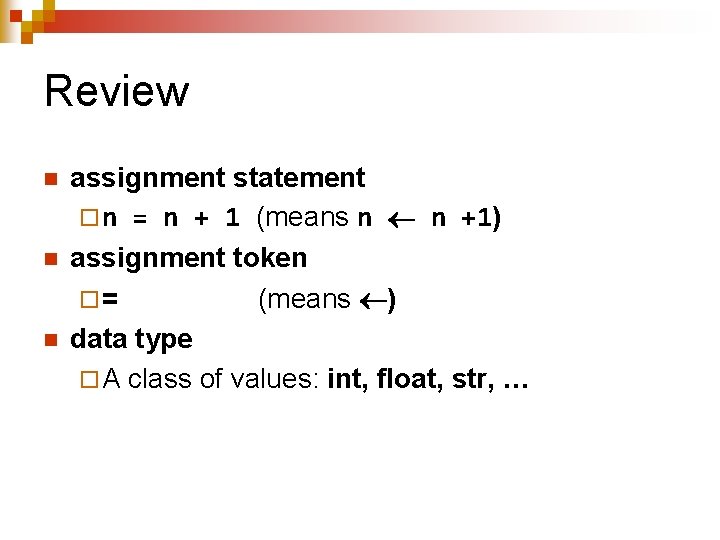
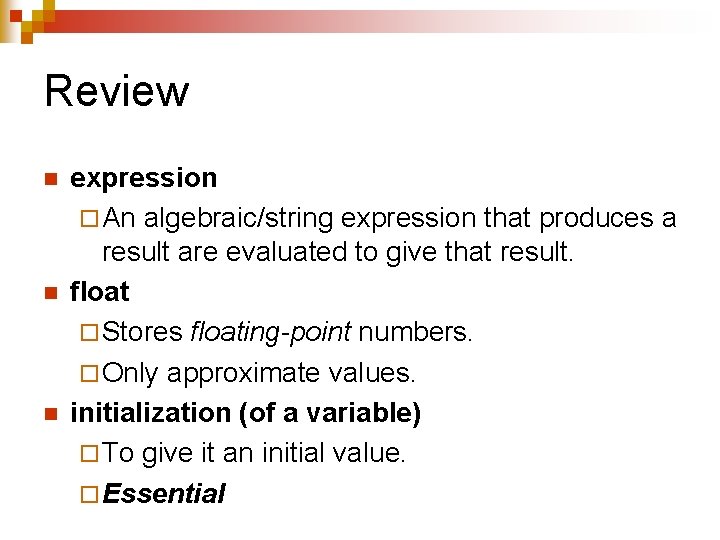
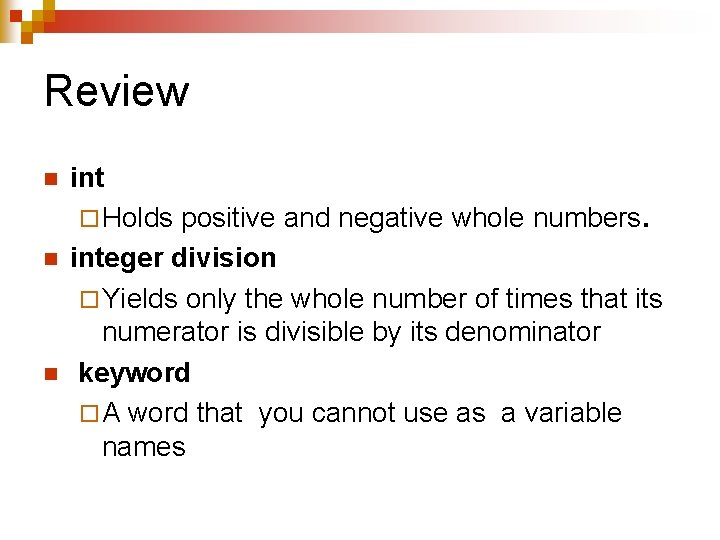
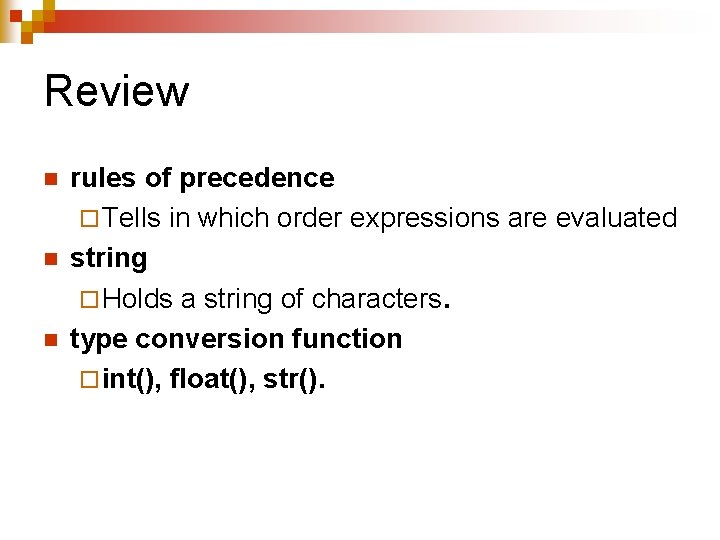
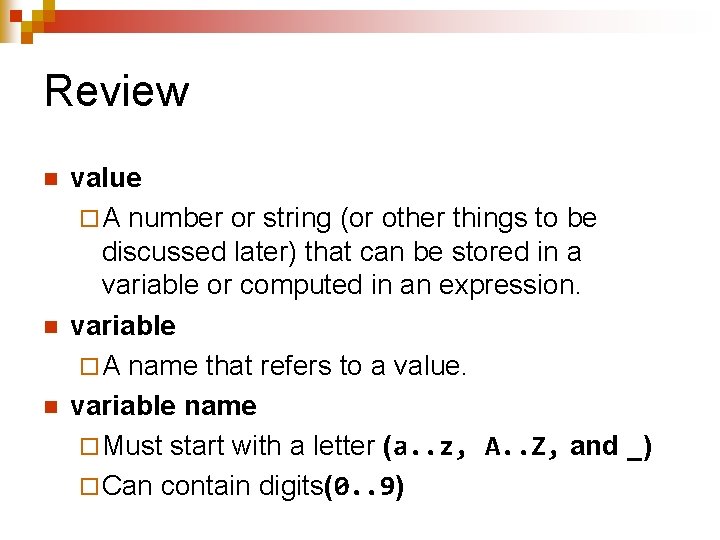
- Slides: 57
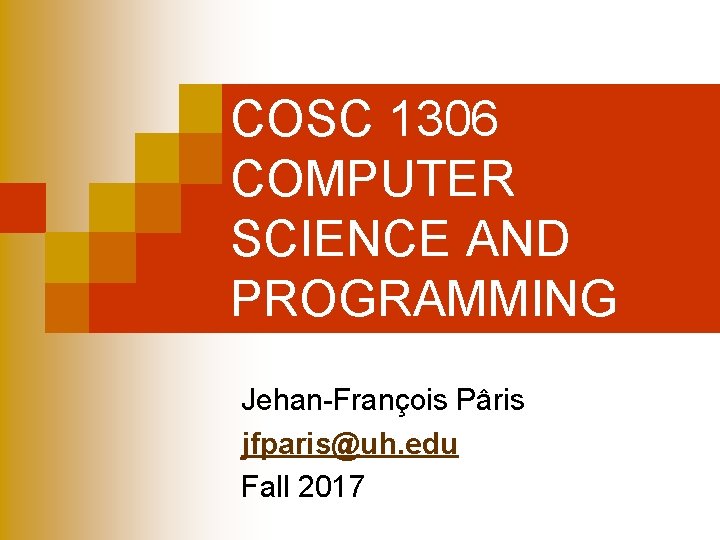
COSC 1306 COMPUTER SCIENCE AND PROGRAMMING Jehan-François Pâris jfparis@uh. edu Fall 2017
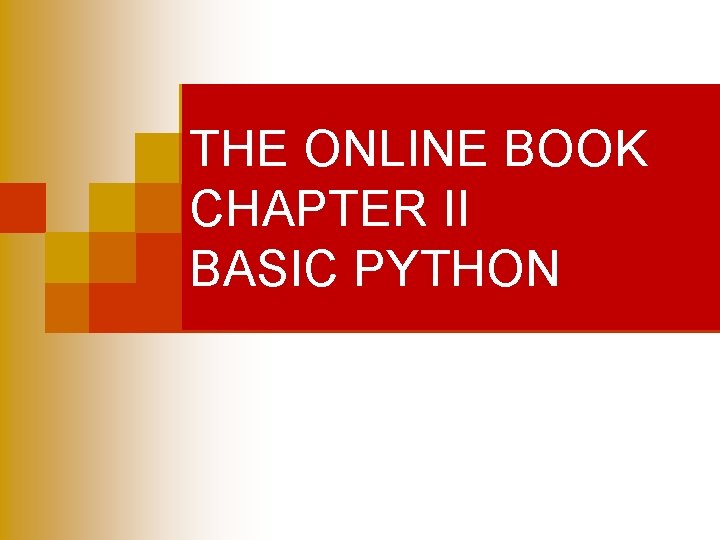
THE ONLINE BOOK CHAPTER II BASIC PYTHON
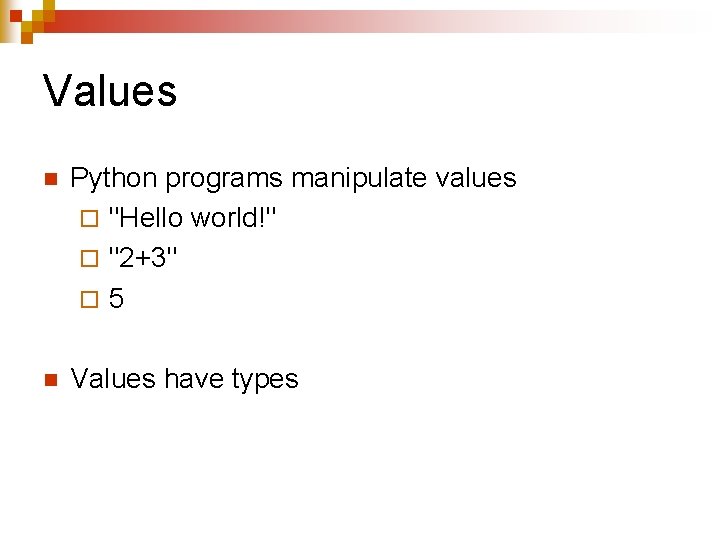
Values n Python programs manipulate values ¨ "Hello world!" ¨ "2+3" ¨ 5 n Values have types
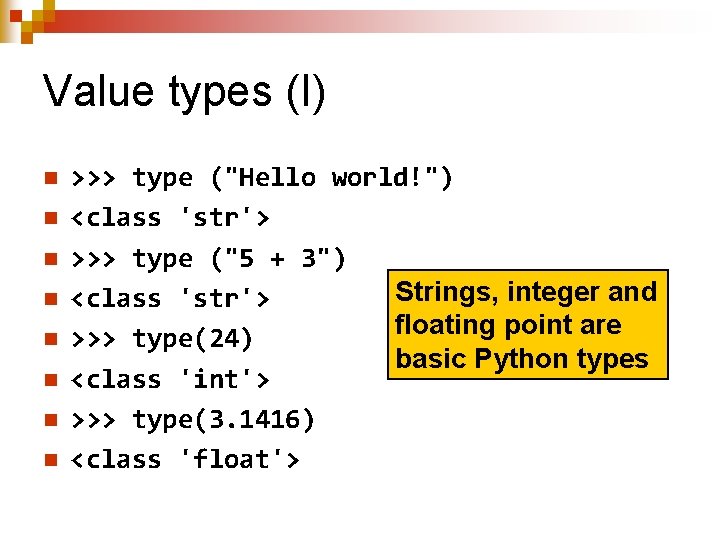
Value types (I) n n n n >>> type ("Hello world!") <class 'str'> >>> type ("5 + 3") Strings, integer and <class 'str'> floating point are >>> type(24) basic Python types <class 'int'> >>> type(3. 1416) <class 'float'>
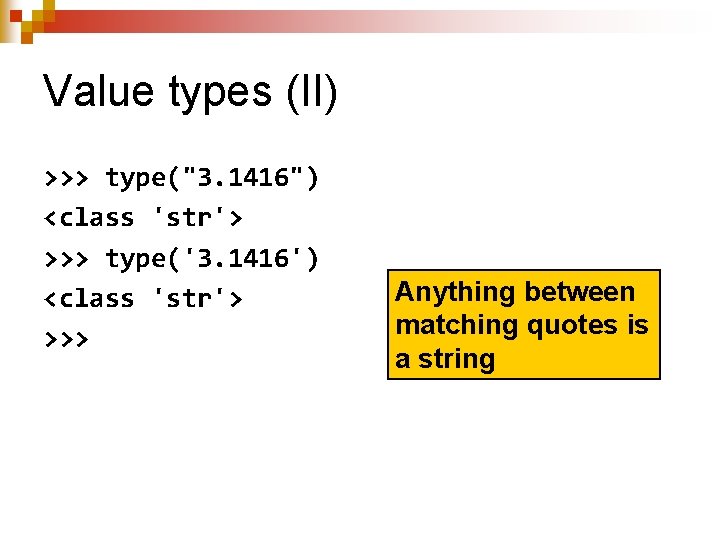
Value types (II) >>> type("3. 1416") <class 'str'> >>> type('3. 1416') <class 'str'> >>> Anything between matching quotes is a string
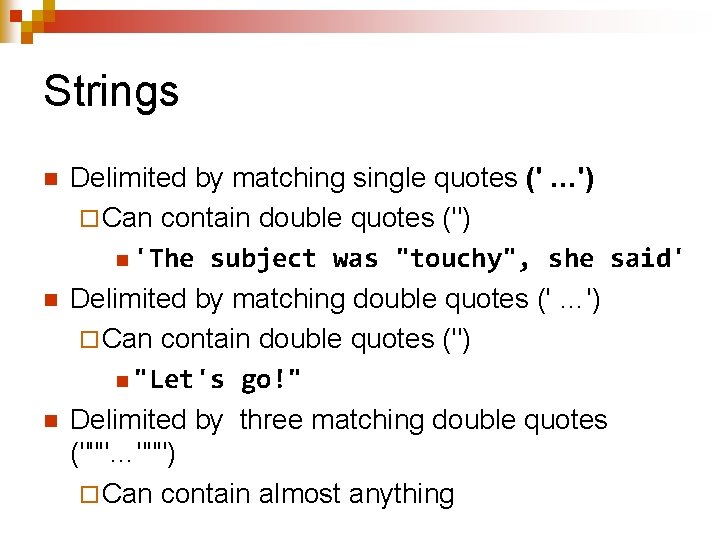
Strings n n n Delimited by matching single quotes (' …') ¨ Can contain double quotes (") n 'The subject was "touchy", she said' Delimited by matching double quotes (' …') ¨ Can contain double quotes (") n "Let's go!" Delimited by three matching double quotes ("""…""") ¨ Can contain almost anything
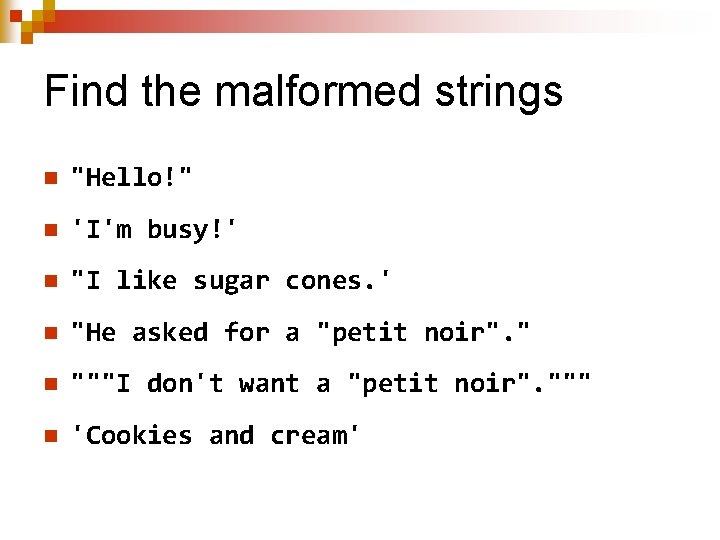
Find the malformed strings n "Hello!" n 'I'm busy!' n "I like sugar cones. ' n "He asked for a "petit noir". " n """I don't want a "petit noir". """ n 'Cookies and cream'
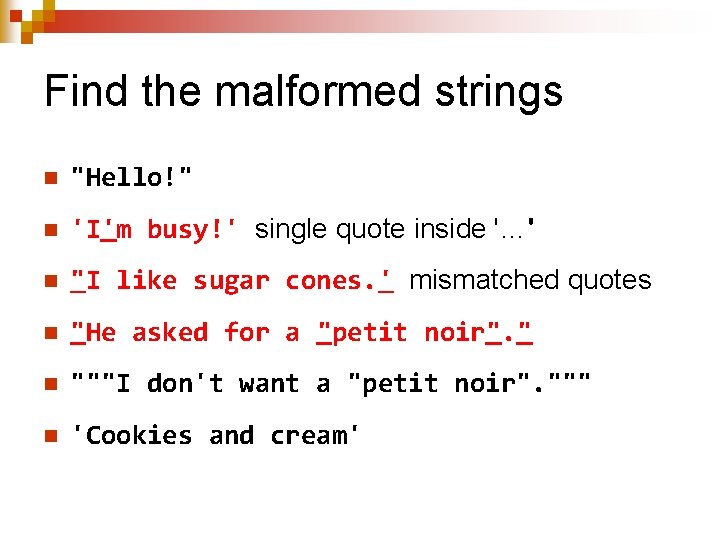
Find the malformed strings n "Hello!" n 'I'm busy!' single quote inside '…' n "I like sugar cones. ' mismatched quotes n "He asked for a "petit noir". " n """I don't want a "petit noir". """ n 'Cookies and cream'
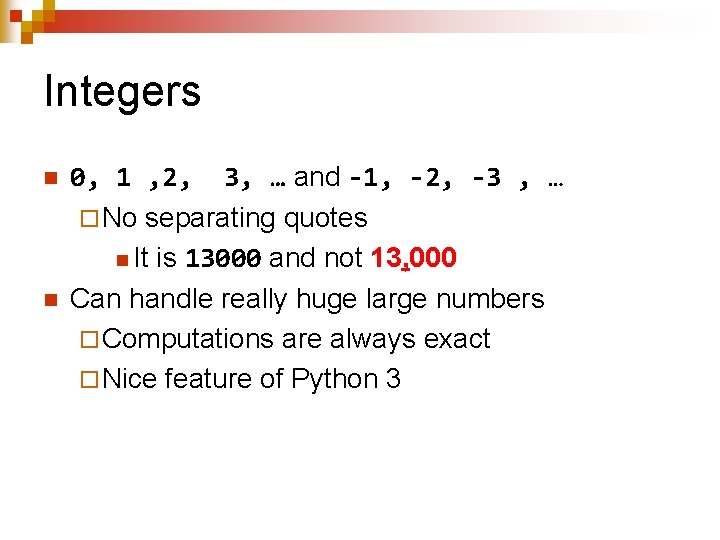
Integers n n 0, 1 , 2, 3, … and -1, -2, -3 , … ¨ No separating quotes n It is 13000 and not 13, 000 Can handle really huge large numbers ¨ Computations are always exact ¨ Nice feature of Python 3
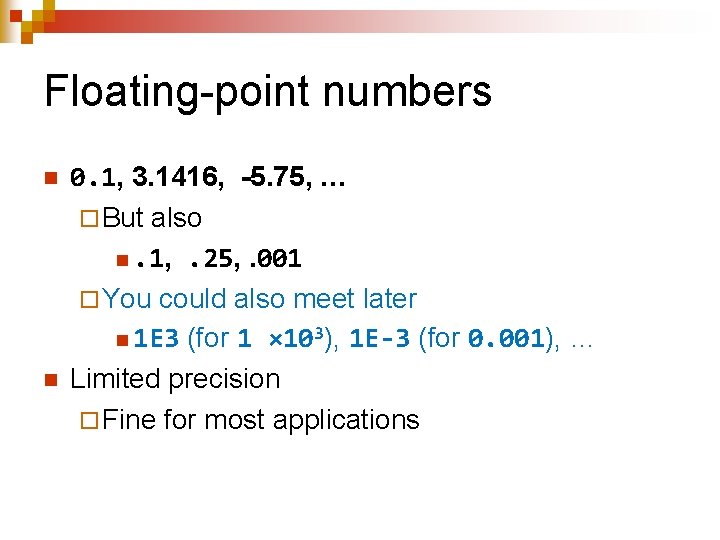
Floating-point numbers n n 0. 1, 3. 1416, -5. 75, … ¨ But also n. 1, . 25, . 001 ¨ You could also meet later n 1 E 3 (for 1 × 103), 1 E-3 (for 0. 001), … Limited precision ¨ Fine for most applications
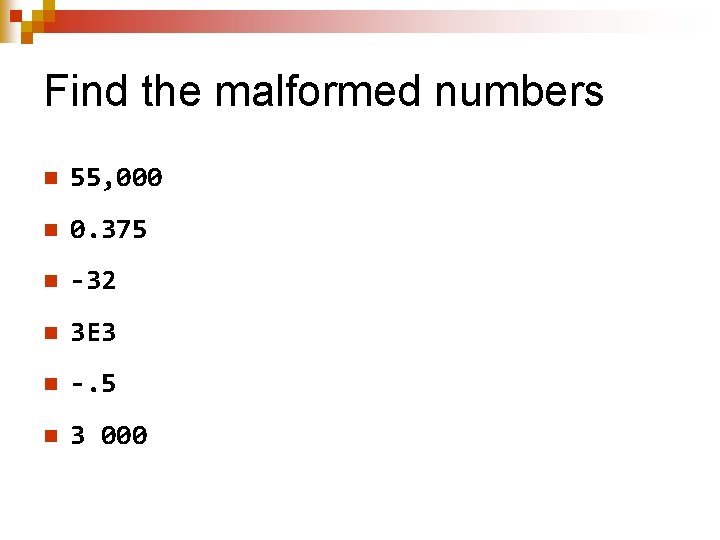
Find the malformed numbers n 55, 000 n 0. 375 n -32 n 3 E 3 n -. 5 n 3 000
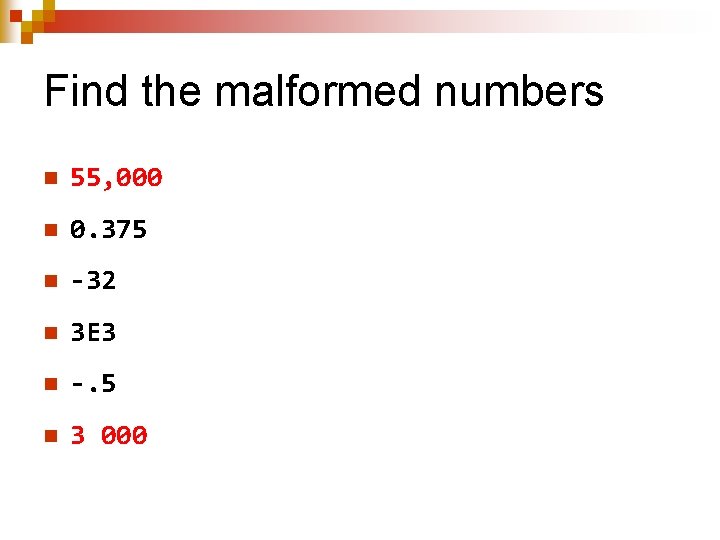
Find the malformed numbers n 55, 000 n 0. 375 n -32 n 3 E 3 n -. 5 n 3 000
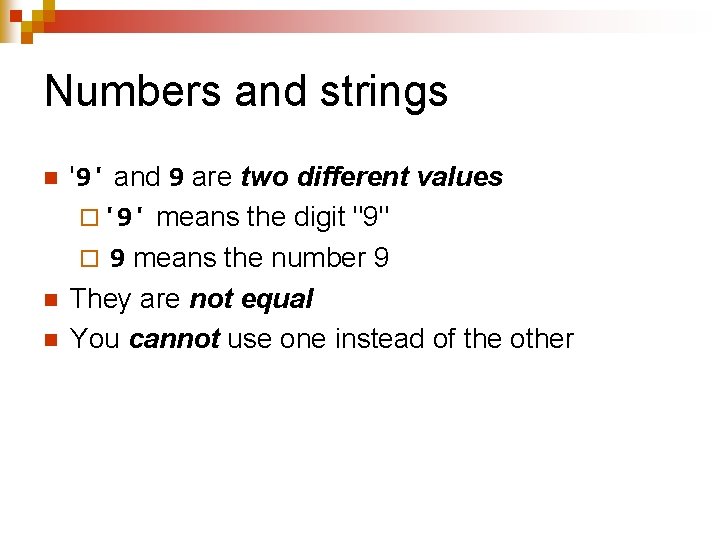
Numbers and strings n n n '9' and 9 are two different values ¨ '9' means the digit "9" ¨ 9 means the number 9 They are not equal You cannot use one instead of the other
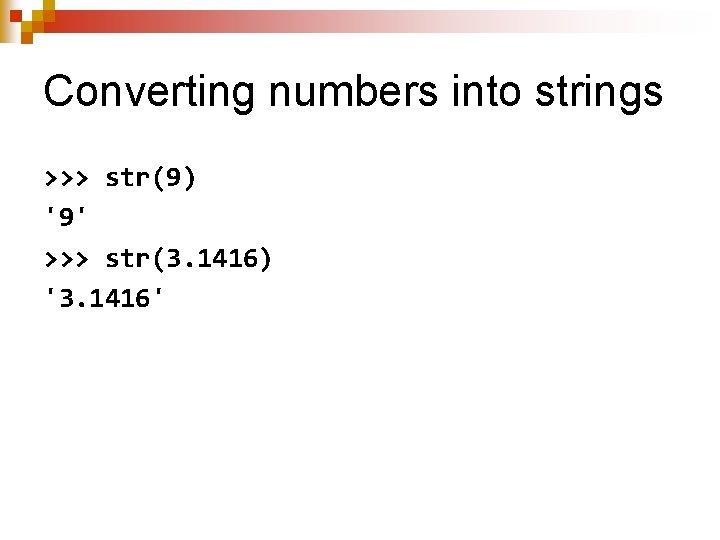
Converting numbers into strings >>> str(9) '9' >>> str(3. 1416) '3. 1416'
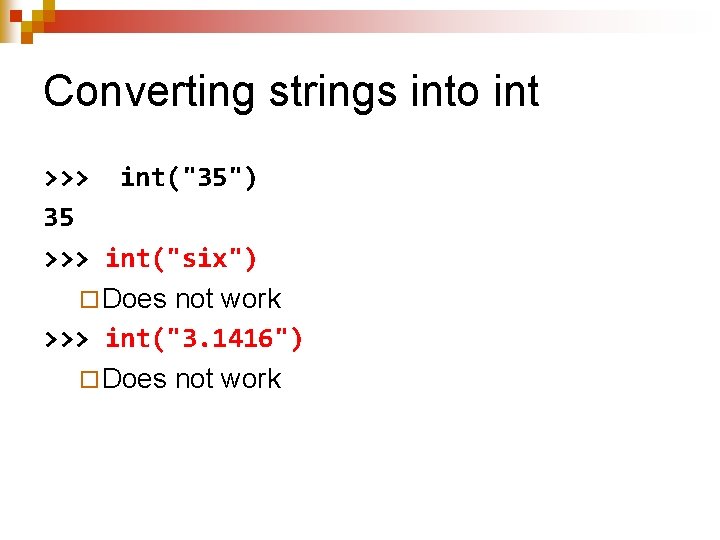
Converting strings into int >>> int("35") 35 >>> int("six") ¨ Does not work >>> int("3. 1416") ¨ Does not work
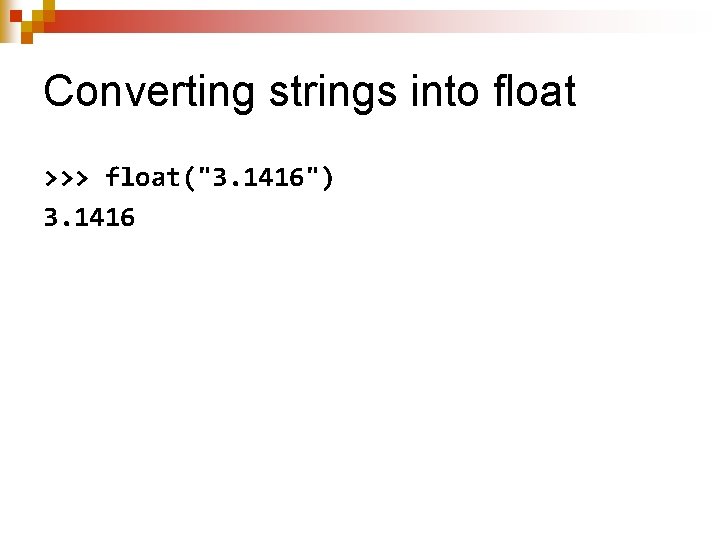
Converting strings into float >>> float("3. 1416") 3. 1416
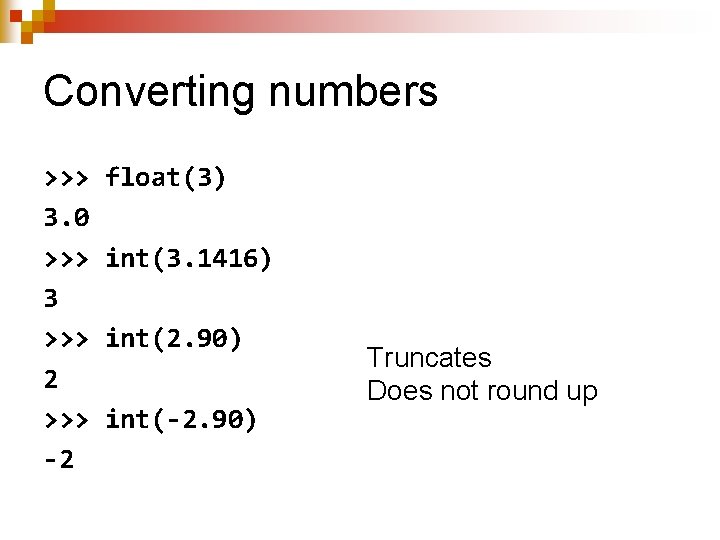
Converting numbers >>> 3. 0 >>> 3 >>> 2 >>> -2 float(3) int(3. 1416) int(2. 90) int(-2. 90) Truncates Does not round up
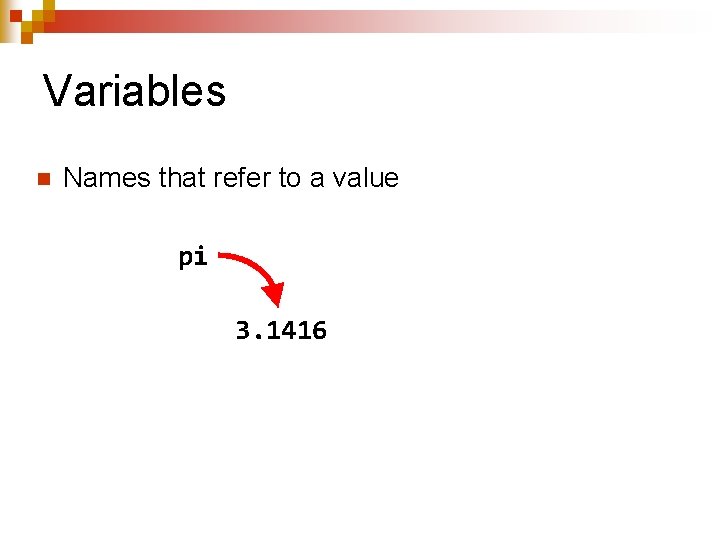
Variables n Names that refer to a value pi 3. 1416
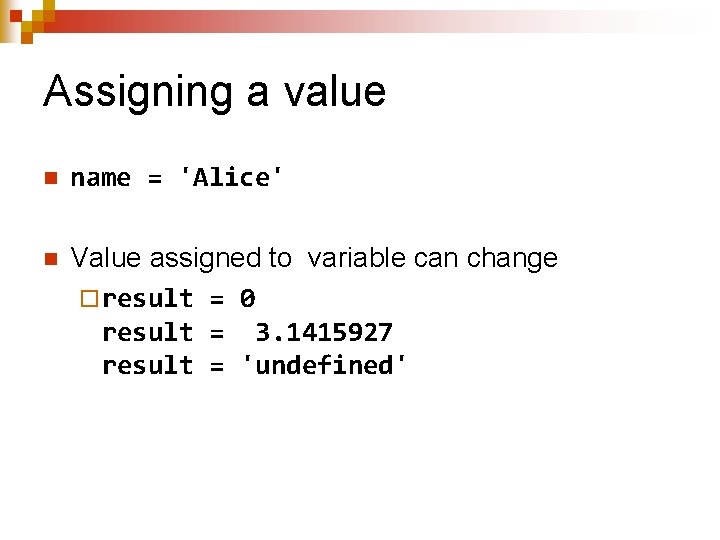
Assigning a value n name = 'Alice' n Value assigned to variable can change ¨ result = 0 result = 3. 1415927 result = 'undefined'
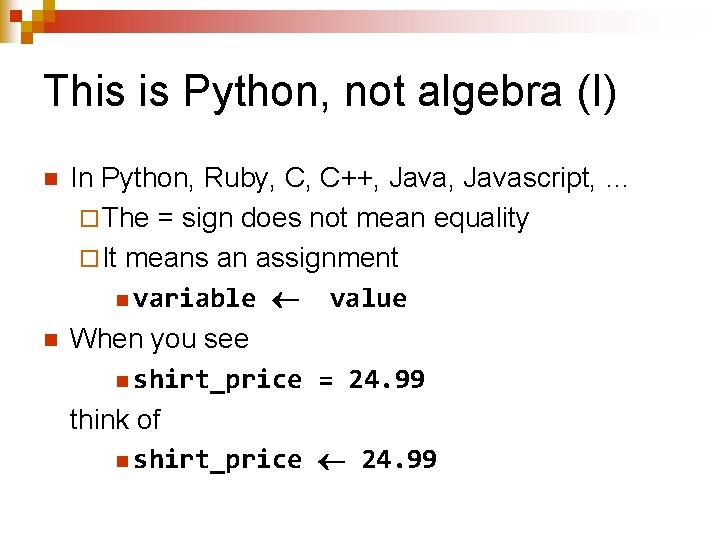
This is Python, not algebra (I) n n In Python, Ruby, C, C++, Javascript, … ¨ The = sign does not mean equality ¨ It means an assignment n variable value When you see n shirt_price = 24. 99 think of n shirt_price 24. 99
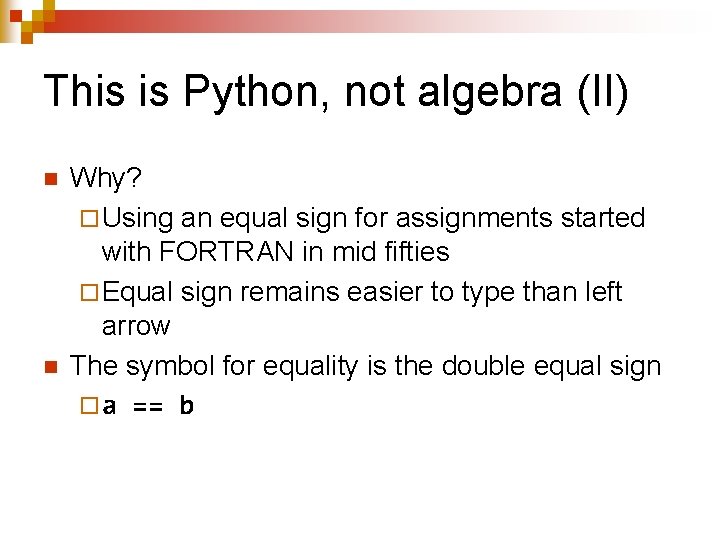
This is Python, not algebra (II) n n Why? ¨ Using an equal sign for assignments started with FORTRAN in mid fifties ¨ Equal sign remains easier to type than left arrow The symbol for equality is the double equal sign ¨ a == b
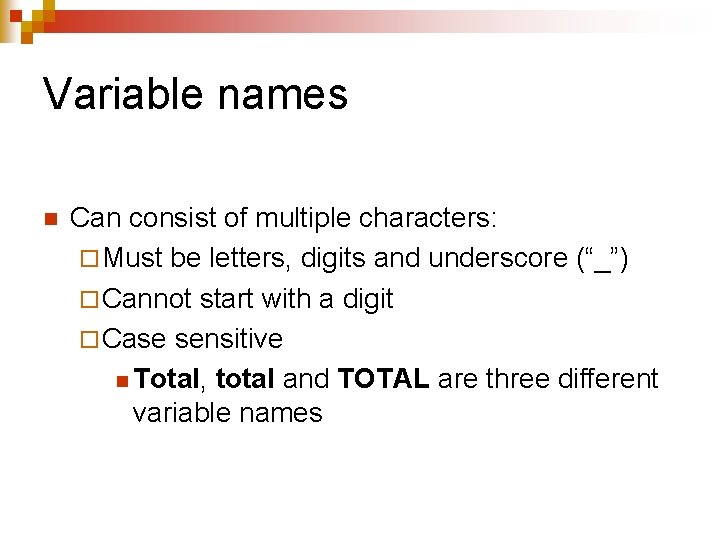
Variable names n Can consist of multiple characters: ¨ Must be letters, digits and underscore (“_”) ¨ Cannot start with a digit ¨ Case sensitive n Total, total and TOTAL are three different variable names
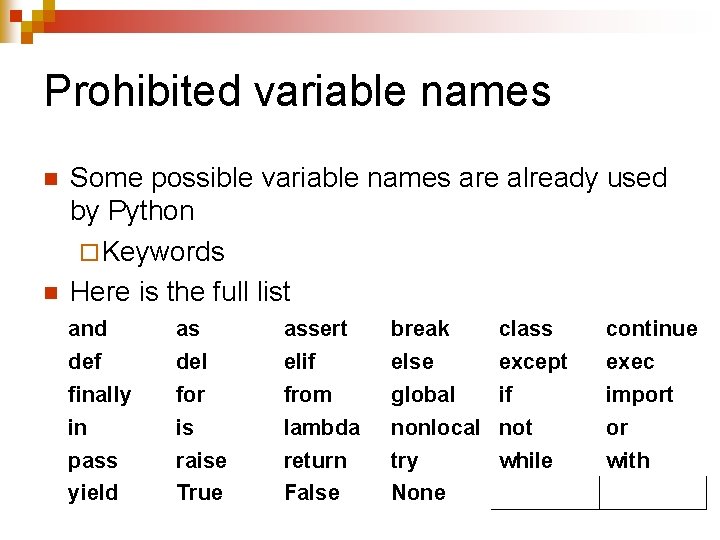
Prohibited variable names n n Some possible variable names are already used by Python ¨ Keywords Here is the full list and def finally in pass yield as del for is raise True assert elif from lambda return False break else global nonlocal try None class except if not while continue exec import or with
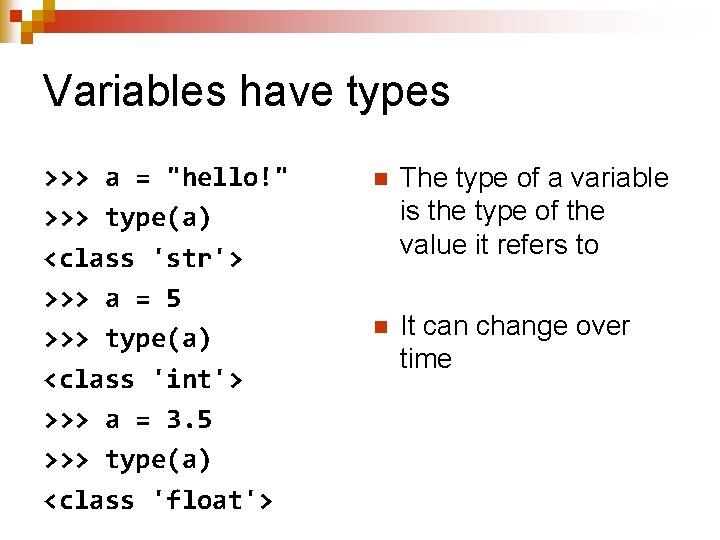
Variables have types >>> a = "hello!" >>> type(a) <class 'str'> >>> a = 5 >>> type(a) <class 'int'> >>> a = 3. 5 >>> type(a) <class 'float'> n The type of a variable is the type of the value it refers to n It can change over time
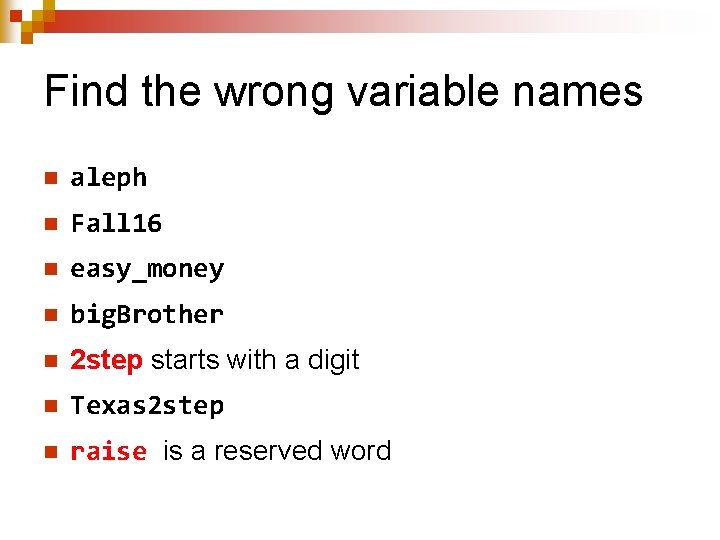
Find the wrong variable names n aleph n Fall 16 n easy_money n big. Brother n 2 step starts with a digit n Texas 2 step n raise is a reserved word
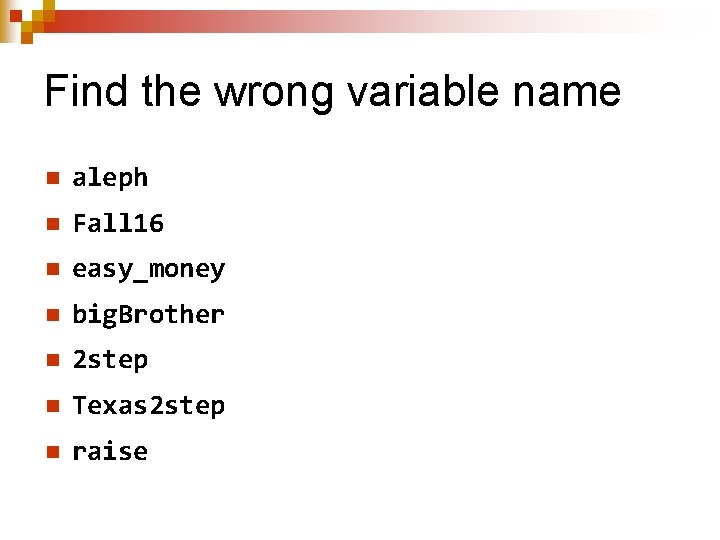
Find the wrong variable name n aleph n Fall 16 n easy_money n big. Brother n 2 step n Texas 2 step n raise
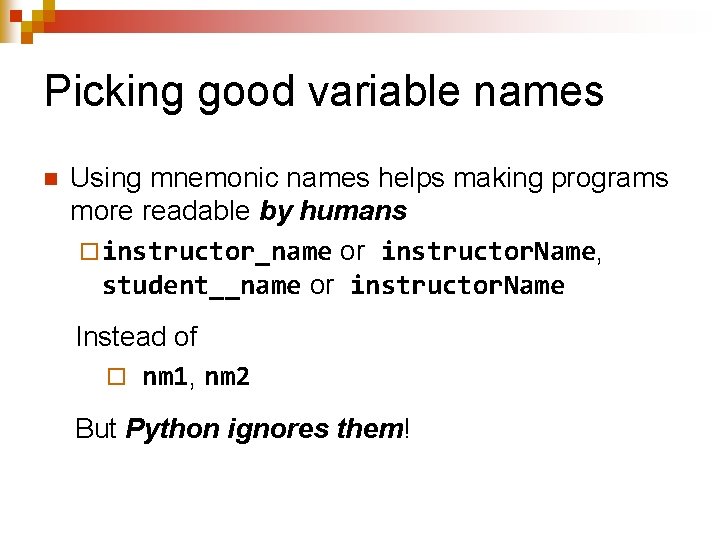
Picking good variable names n Using mnemonic names helps making programs more readable by humans ¨ instructor_name or instructor. Name, student__name or instructor. Name Instead of ¨ nm 1, nm 2 But Python ignores them!
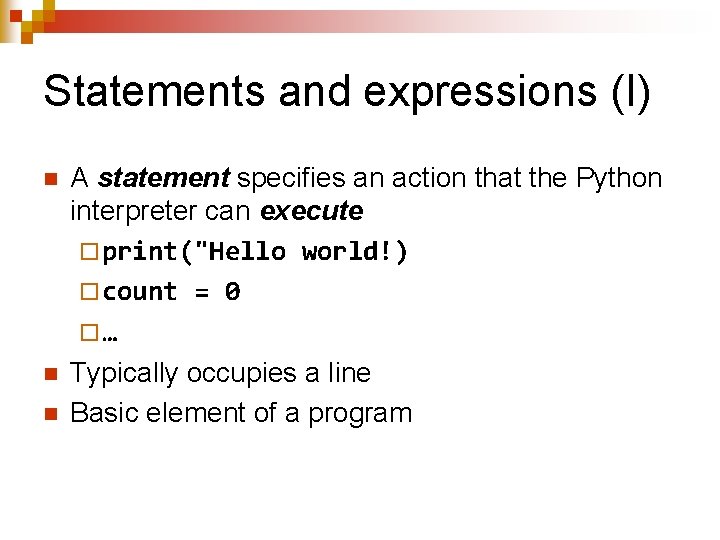
Statements and expressions (I) n n n A statement specifies an action that the Python interpreter can execute ¨ print("Hello world!) ¨ count = 0 ¨… Typically occupies a line Basic element of a program
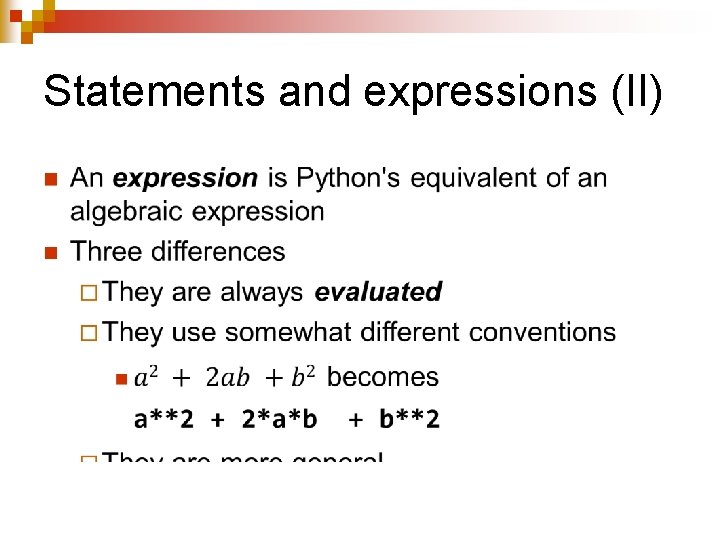
Statements and expressions (II) n
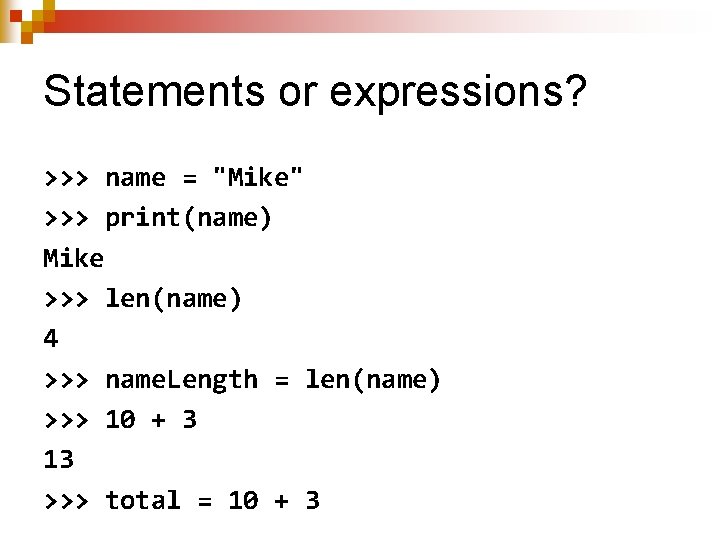
Statements or expressions? >>> name = "Mike" >>> print(name) Mike >>> len(name) 4 >>> name. Length = len(name) >>> 10 + 3 13 >>> total = 10 + 3
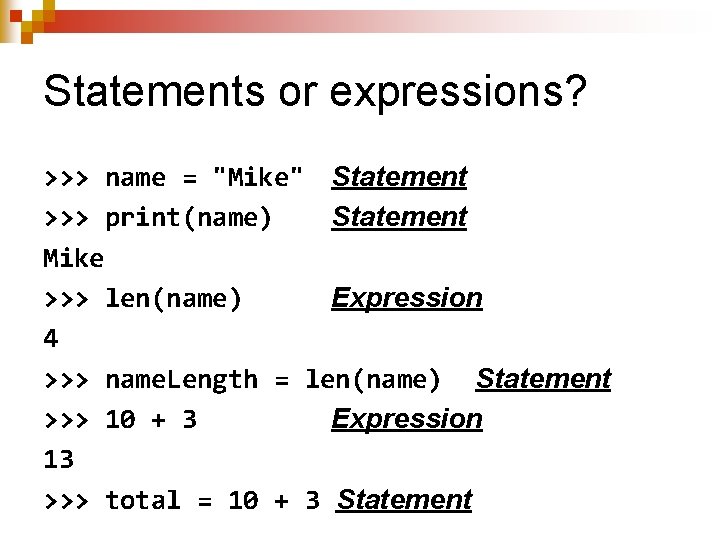
Statements or expressions? >>> name = "Mike" Statement >>> print(name) Statement Mike >>> len(name) Expression 4 >>> name. Length = len(name) Statement >>> 10 + 3 Expression 13 >>> total = 10 + 3 Statement
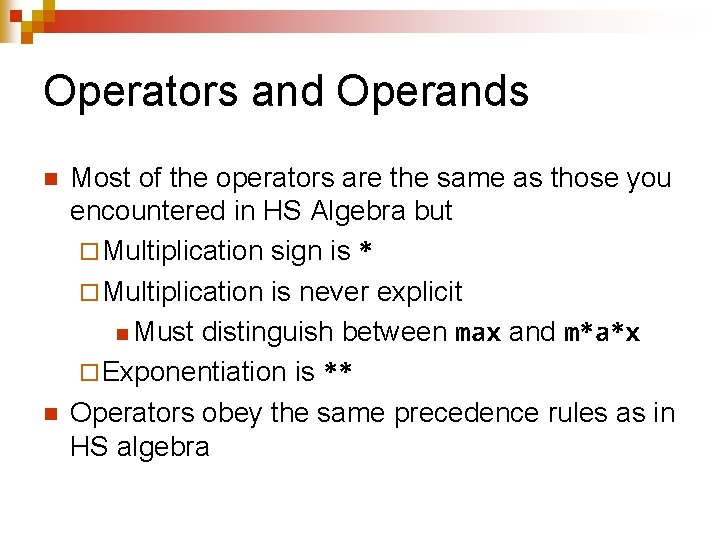
Operators and Operands n n Most of the operators are the same as those you encountered in HS Algebra but ¨ Multiplication sign is * ¨ Multiplication is never explicit n Must distinguish between max and m*a*x ¨ Exponentiation is ** Operators obey the same precedence rules as in HS algebra
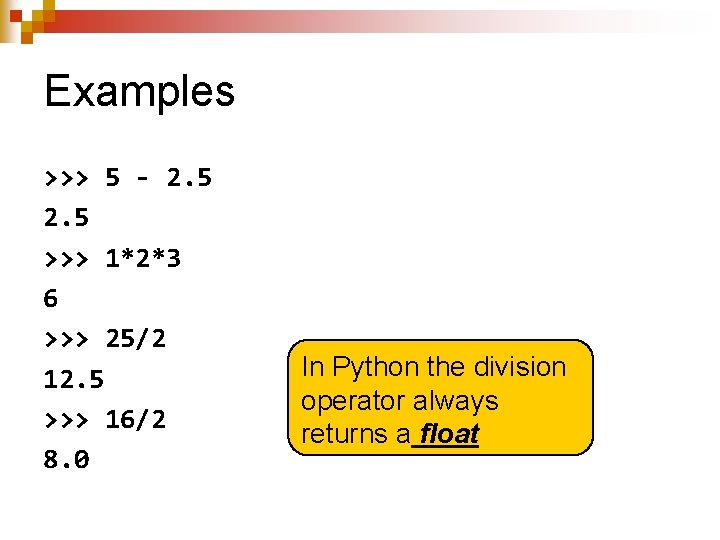
Examples >>> 5 - 2. 5 >>> 1*2*3 6 >>> 25/2 12. 5 >>> 16/2 8. 0 In Python the division operator always returns a float
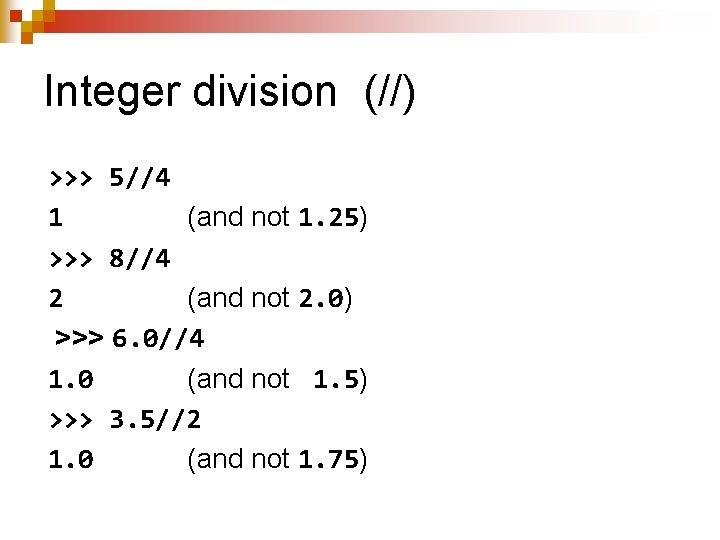
Integer division (//) >>> 5//4 1 (and not 1. 25) >>> 8//4 2 (and not 2. 0) >>> 6. 0//4 1. 0 (and not 1. 5) >>> 3. 5//2 1. 0 (and not 1. 75)
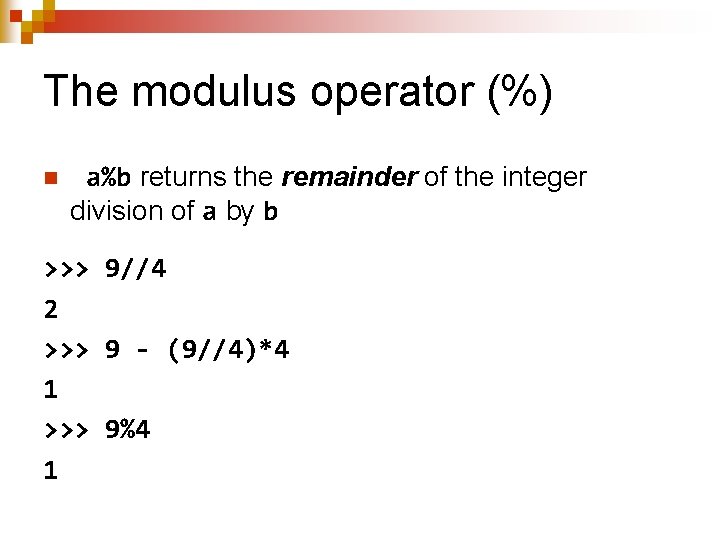
The modulus operator (%) n a%b returns the remainder of the integer division of a by b >>> 9//4 2 >>> 9 - (9//4)*4 1 >>> 9%4 1
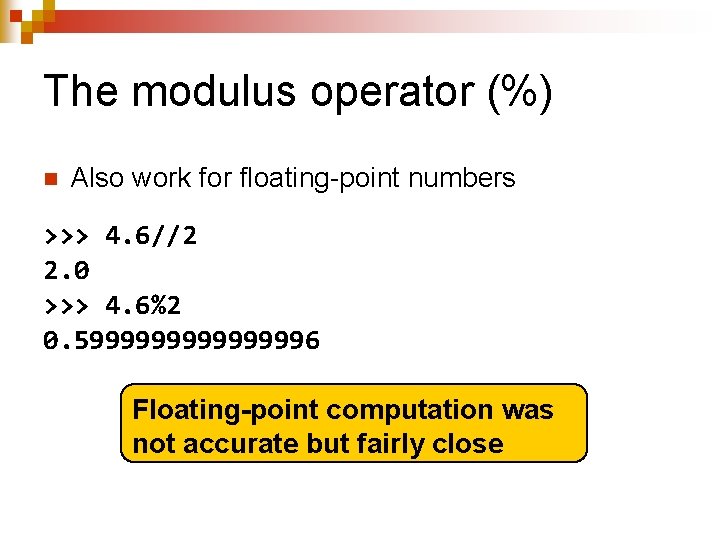
The modulus operator (%) n Also work for floating-point numbers >>> 4. 6//2 2. 0 >>> 4. 6%2 0. 599999996 Floating-point computation was not accurate but fairly close
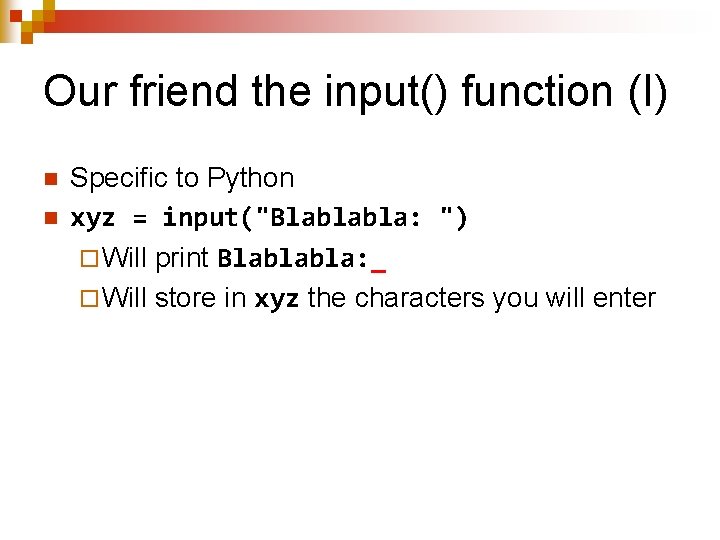
Our friend the input() function (I) n n Specific to Python xyz = input("Blablabla: ") ¨ Will print Blablabla: _ ¨ Will store in xyz the characters you will enter
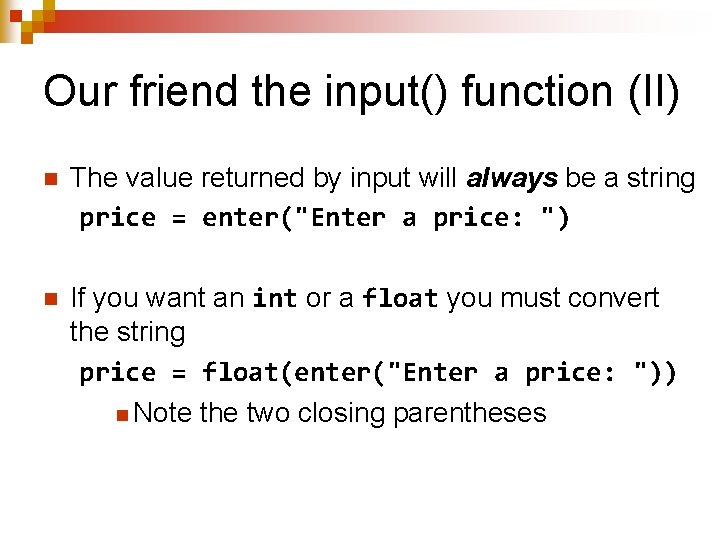
Our friend the input() function (II) n The value returned by input will always be a string price = enter("Enter a price: ") n If you want an int or a float you must convert the string price = float(enter("Enter a price: ")) n Note the two closing parentheses
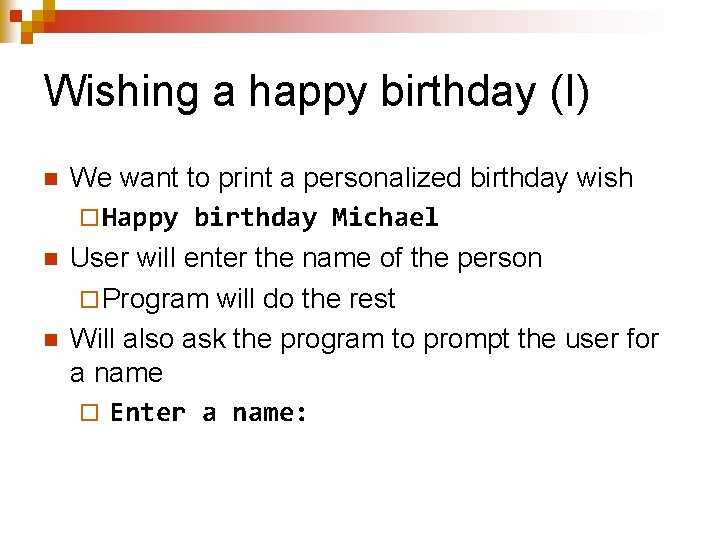
Wishing a happy birthday (I) n n n We want to print a personalized birthday wish ¨ Happy birthday Michael User will enter the name of the person ¨ Program will do the rest Will also ask the program to prompt the user for a name ¨ Enter a name:
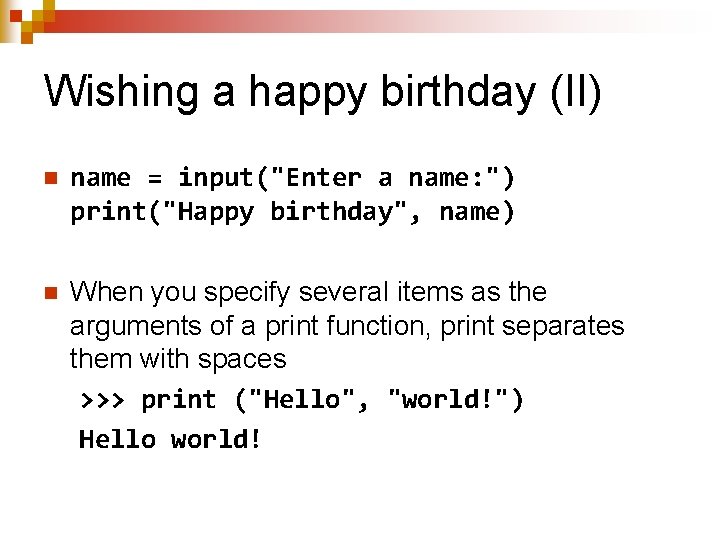
Wishing a happy birthday (II) n name = input("Enter a name: ") print("Happy birthday", name) n When you specify several items as the arguments of a print function, print separates them with spaces >>> print ("Hello", "world!") Hello world!
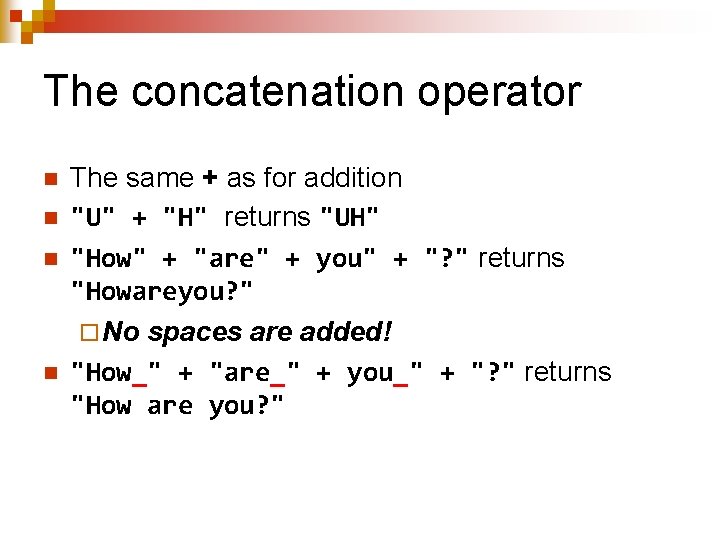
The concatenation operator n n The same + as for addition "U" + "H" returns "UH" "How" + "are" + you" + "? " returns "Howareyou? " ¨ No spaces are added! "How_" + "are_" + you_" + "? " returns "How are you? "
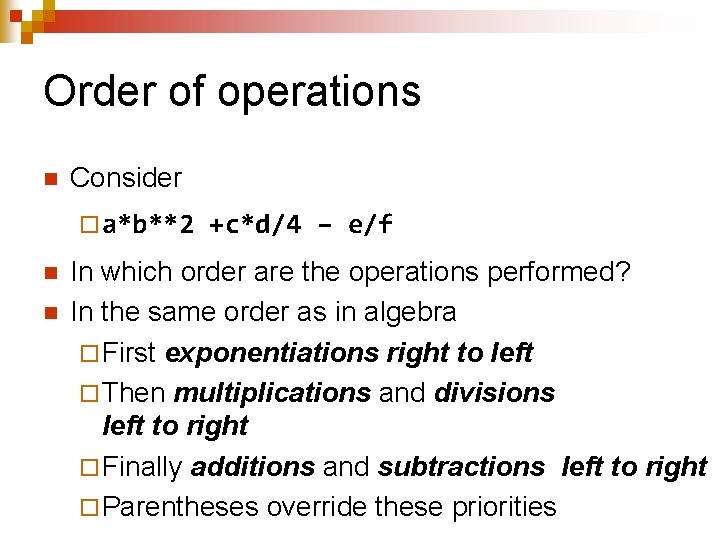
Order of operations n Consider ¨ a*b**2 n n +c*d/4 – e/f In which order are the operations performed? In the same order as in algebra ¨ First exponentiations right to left ¨ Then multiplications and divisions left to right ¨ Finally additions and subtractions left to right ¨ Parentheses override these priorities
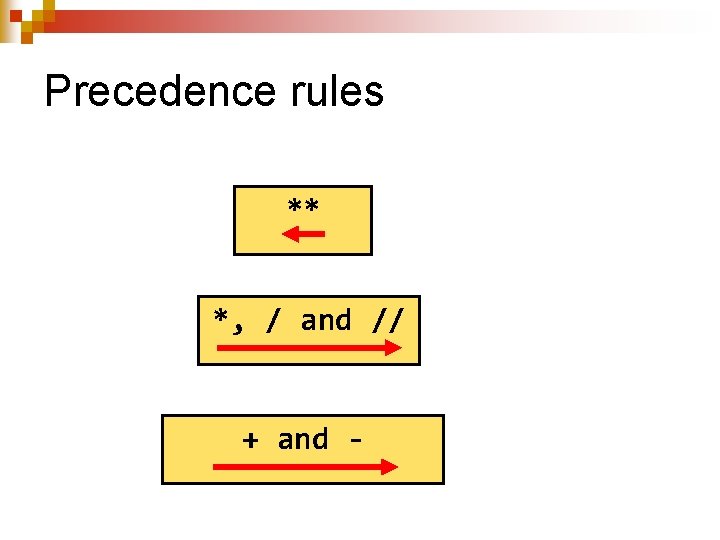
Precedence rules ** *, / and // + and -
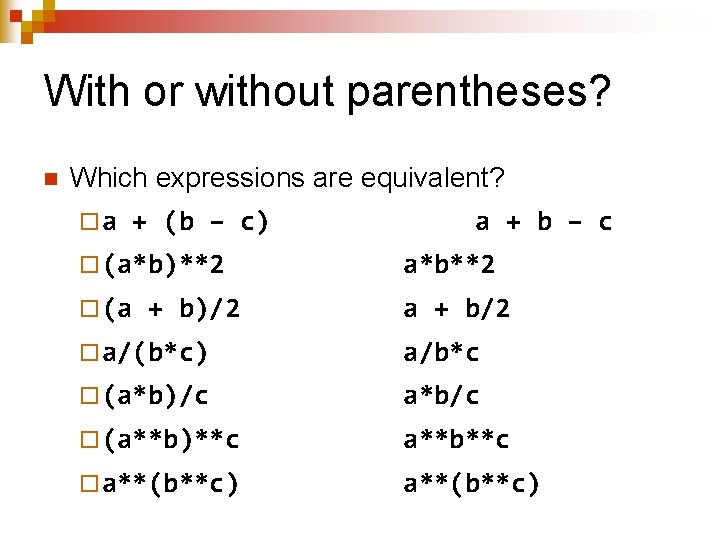
With or without parentheses? n Which expressions are equivalent? ¨a + (b – c) a + b – c ¨ (a*b)**2 a*b**2 ¨ (a a + b/2 + b)/2 ¨ a/(b*c) a/b*c ¨ (a*b)/c a*b/c ¨ (a**b)**c a**b**c ¨ a**(b**c)
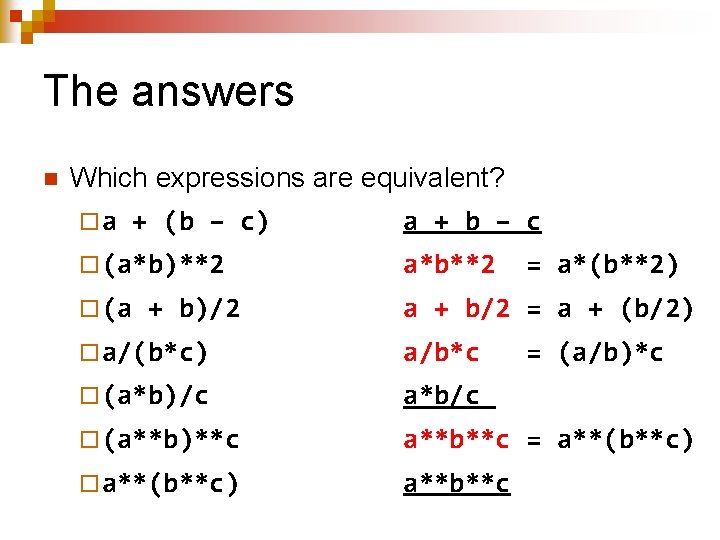
The answers n Which expressions are equivalent? ¨a + (b – c) a + b – c ¨ (a*b)**2 a*b**2 ¨ (a a + b/2 = a + (b/2) + b)/2 = a*(b**2) ¨ a/(b*c) a/b*c = (a/b)*c ¨ (a*b)/c a*b/c ¨ (a**b)**c a**b**c = a**(b**c) ¨ a**(b**c) a**b**c
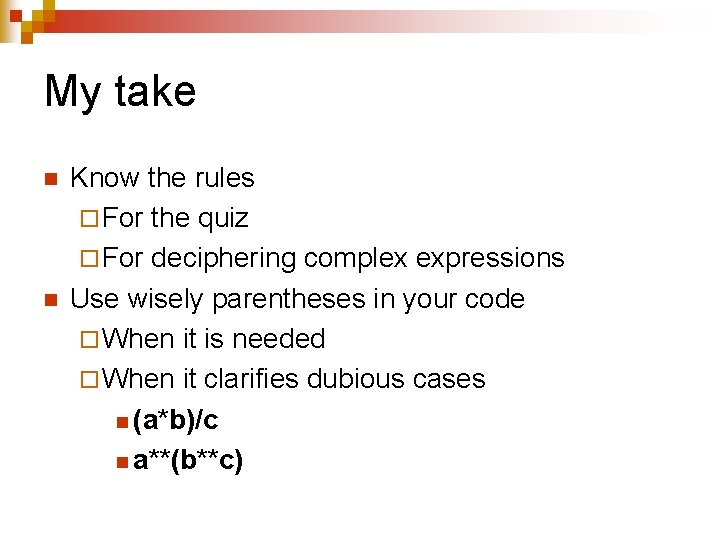
My take n n Know the rules ¨ For the quiz ¨ For deciphering complex expressions Use wisely parentheses in your code ¨ When it is needed ¨ When it clarifies dubious cases n (a*b)/c n a**(b**c)
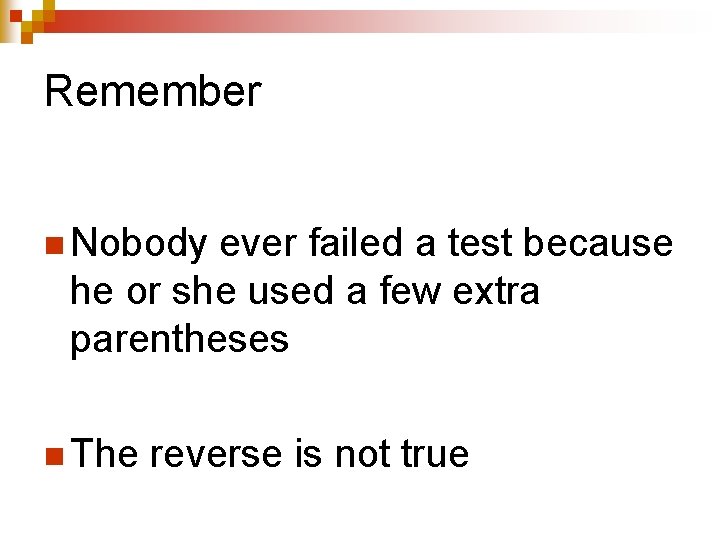
Remember n Nobody ever failed a test because he or she used a few extra parentheses n The reverse is not true
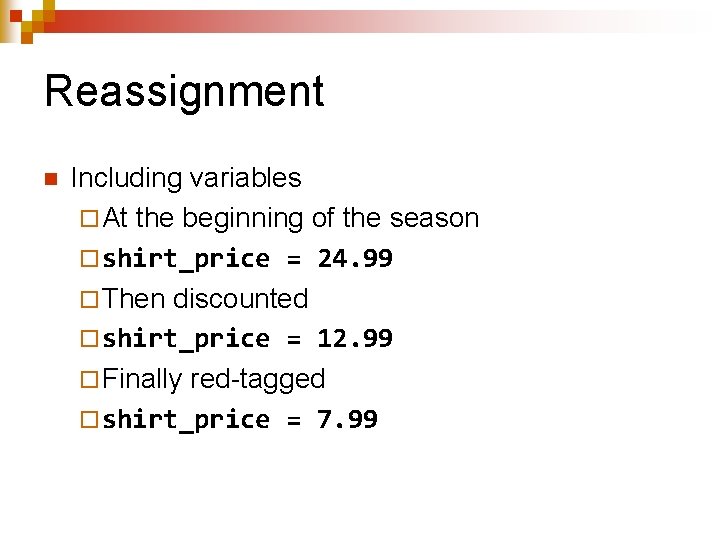
Reassignment n Including variables ¨ At the beginning of the season ¨ shirt_price = 24. 99 ¨ Then discounted ¨ shirt_price = 12. 99 ¨ Finally red-tagged ¨ shirt_price = 7. 99
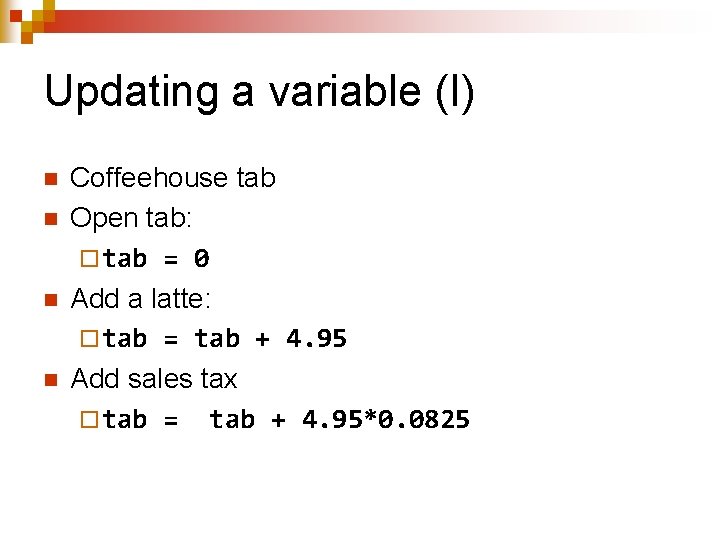
Updating a variable (I) n n Coffeehouse tab Open tab: ¨ tab = 0 Add a latte: ¨ tab = tab + 4. 95 Add sales tax ¨ tab = tab + 4. 95*0. 0825
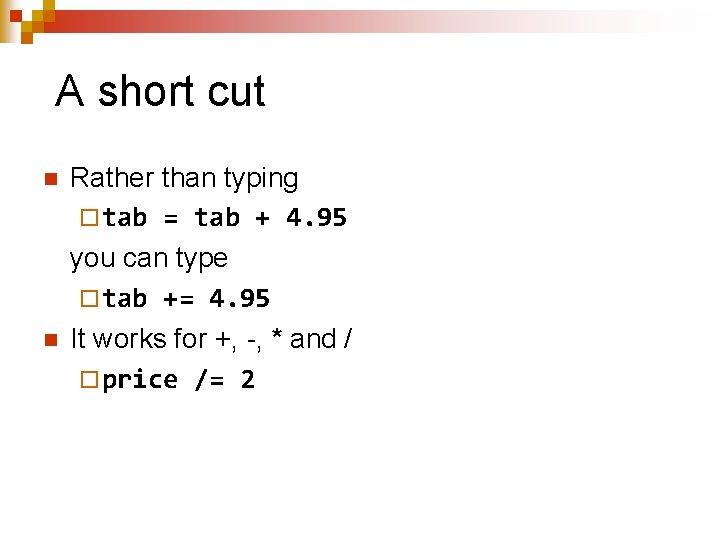
A short cut n n Rather than typing ¨ tab = tab + 4. 95 you can type ¨ tab += 4. 95 It works for +, -, * and / ¨ price /= 2
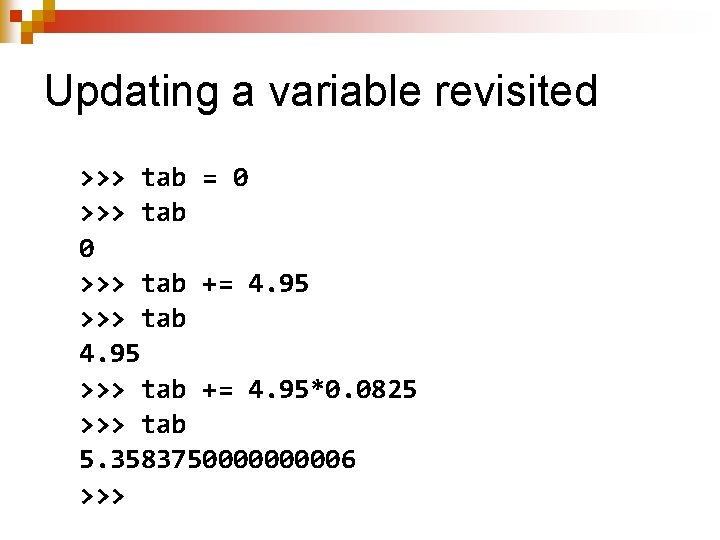
Updating a variable revisited >>> tab = 0 >>> tab += 4. 95*0. 0825 >>> tab 5. 358375000006 >>>
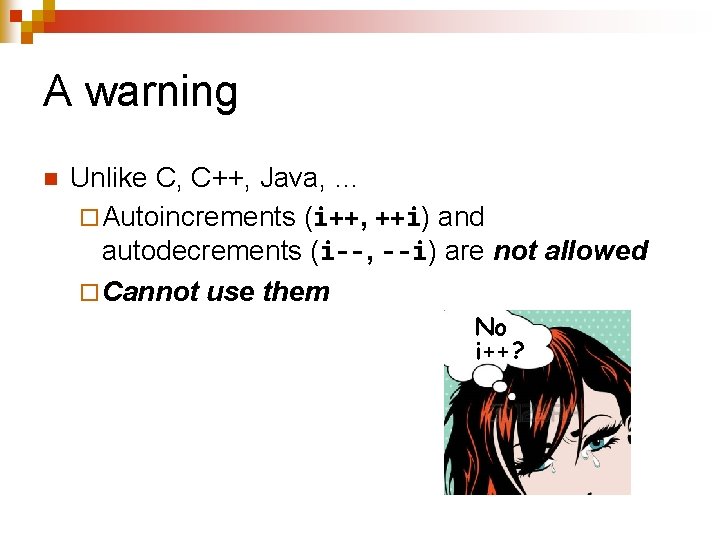
A warning n Unlike C, C++, Java, … ¨ Autoincrements (i++, ++i) and autodecrements (i--, --i) are not allowed ¨ Cannot use them No i++?
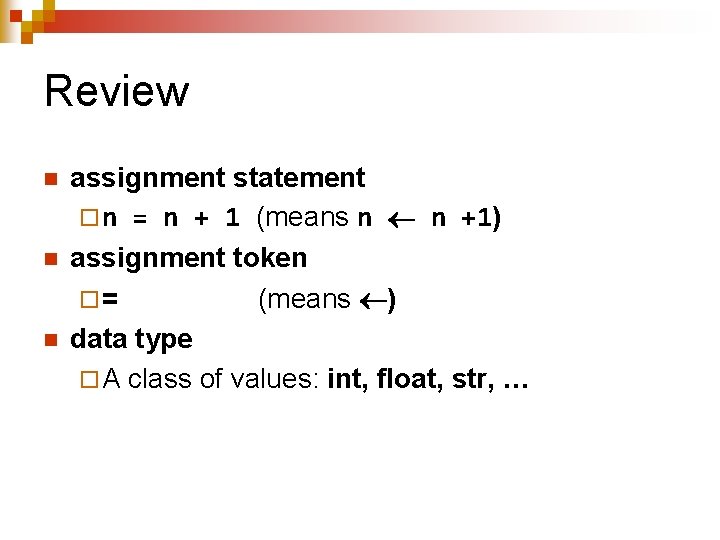
Review n n n assignment statement ¨ n = n + 1 (means n n +1) assignment token ¨= (means ) data type ¨ A class of values: int, float, str, …
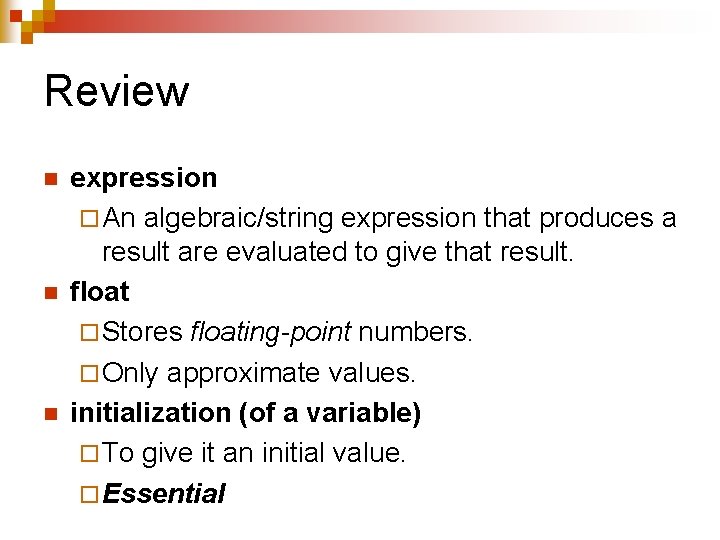
Review n n n expression ¨ An algebraic/string expression that produces a result are evaluated to give that result. float ¨ Stores floating-point numbers. ¨ Only approximate values. initialization (of a variable) ¨ To give it an initial value. ¨ Essential
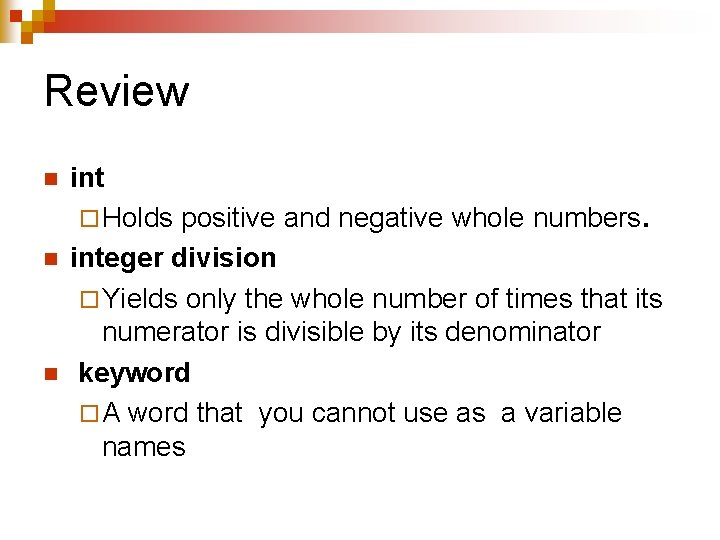
Review n n n int ¨ Holds positive and negative whole numbers. integer division ¨ Yields only the whole number of times that its numerator is divisible by its denominator keyword ¨ A word that you cannot use as a variable names
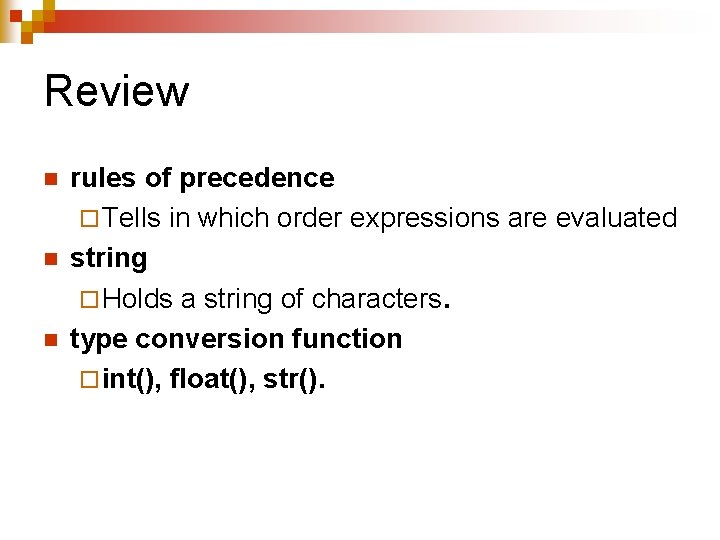
Review n n n rules of precedence ¨ Tells in which order expressions are evaluated string ¨ Holds a string of characters. type conversion function ¨ int(), float(), str().
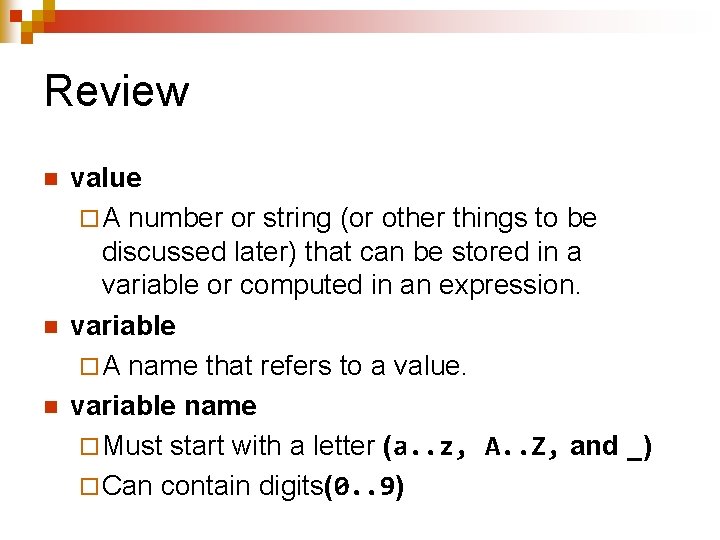
Review n n n value ¨ A number or string (or other things to be discussed later) that can be stored in a variable or computed in an expression. variable ¨ A name that refers to a value. variable name ¨ Must start with a letter (a. . z, A. . Z, and _) ¨ Can contain digits(0. . 9)