Control Structures Part 2 Outline Introduction Essentials of
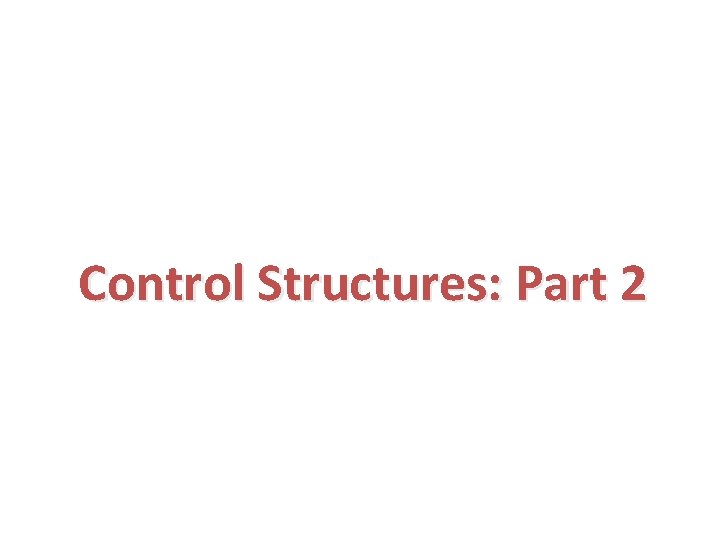
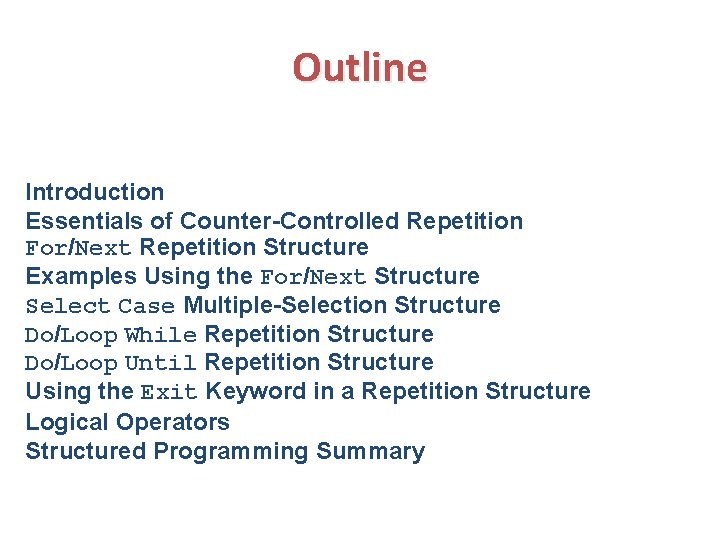
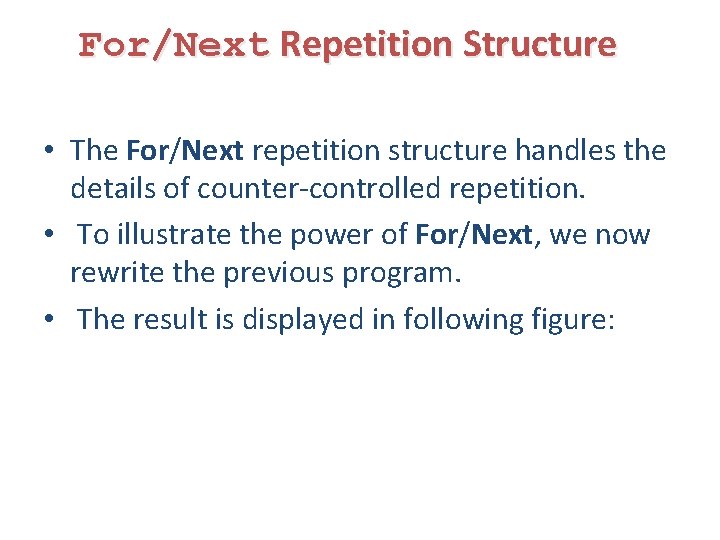
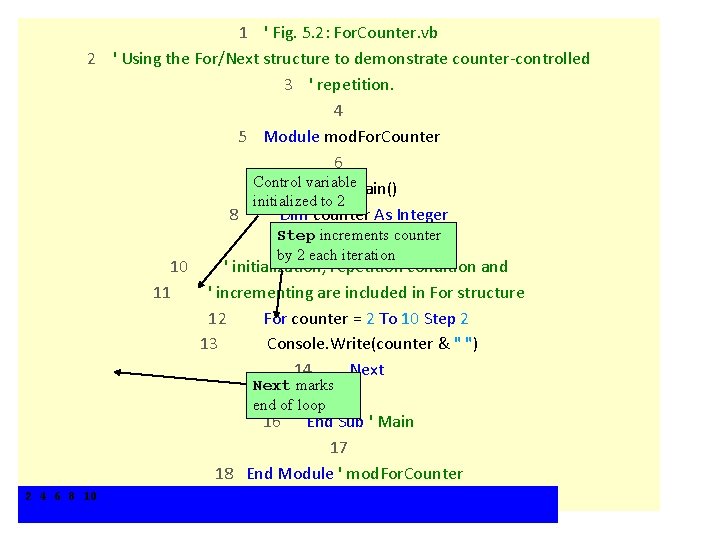
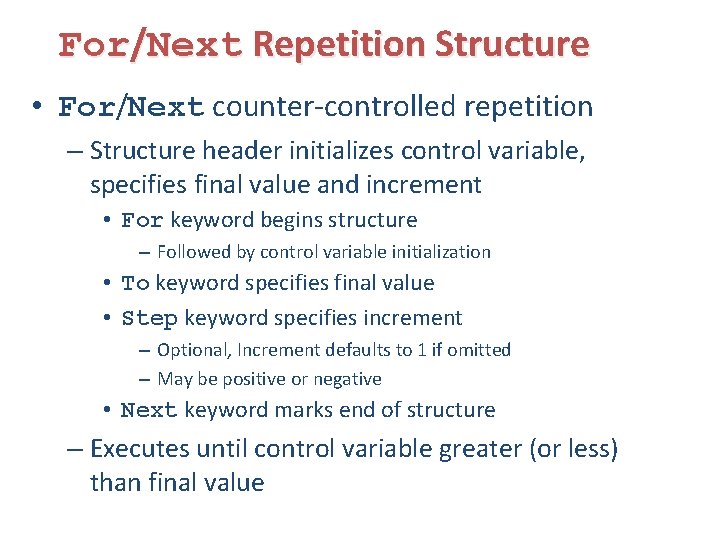
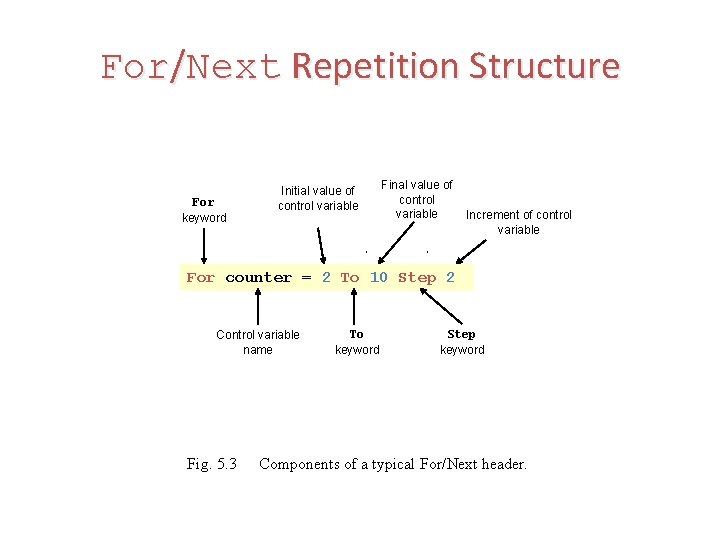
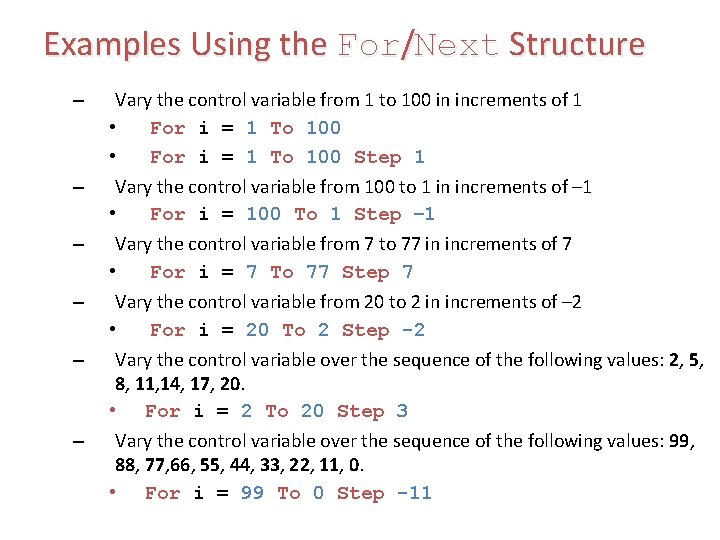
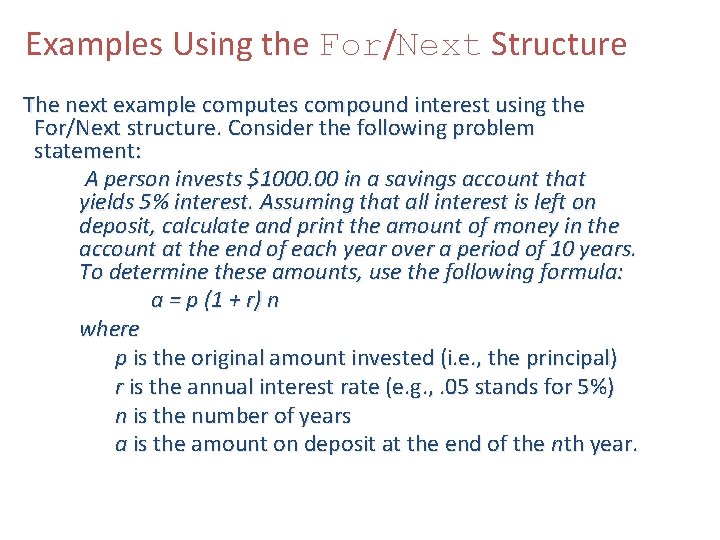
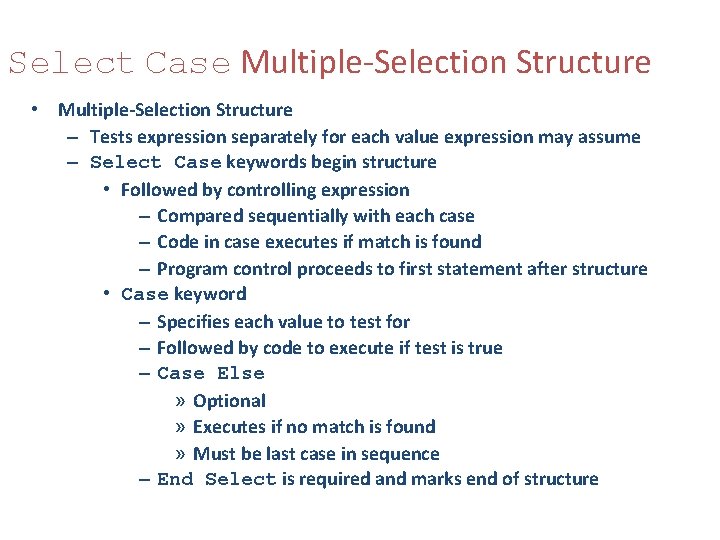
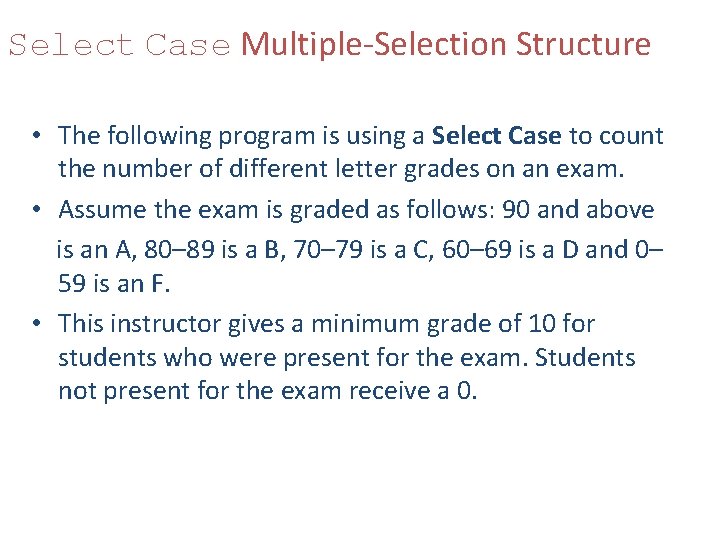
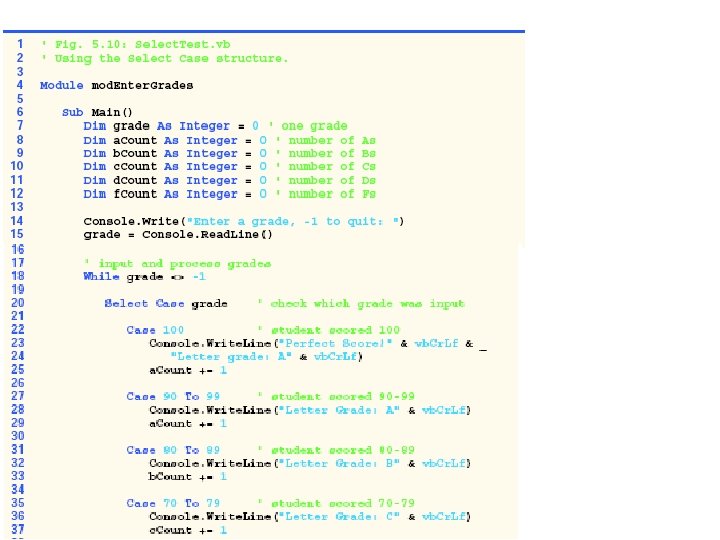
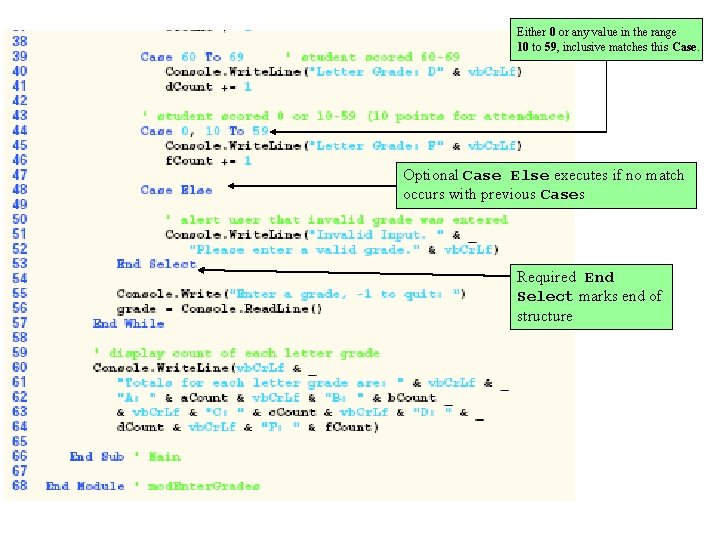
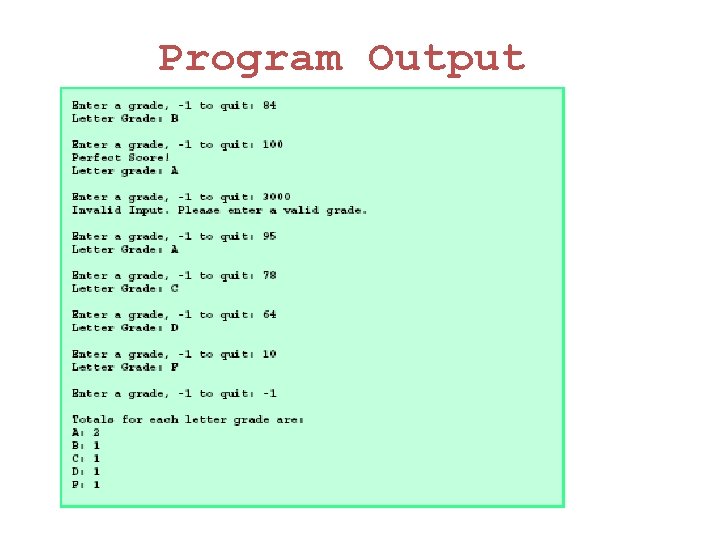
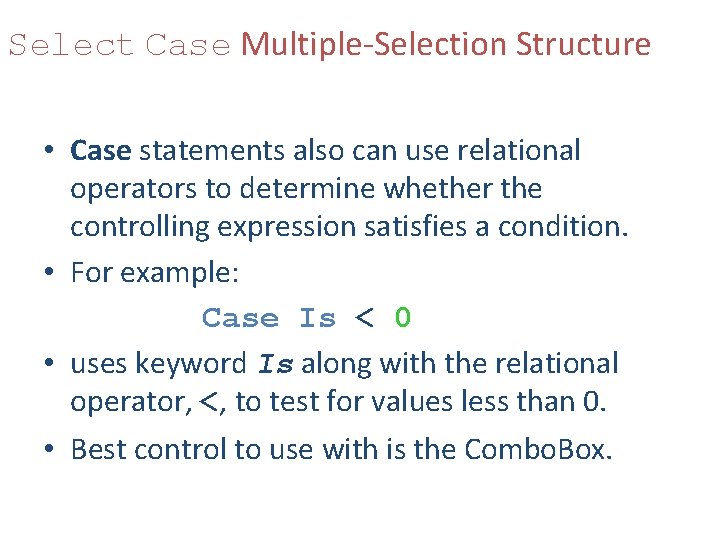
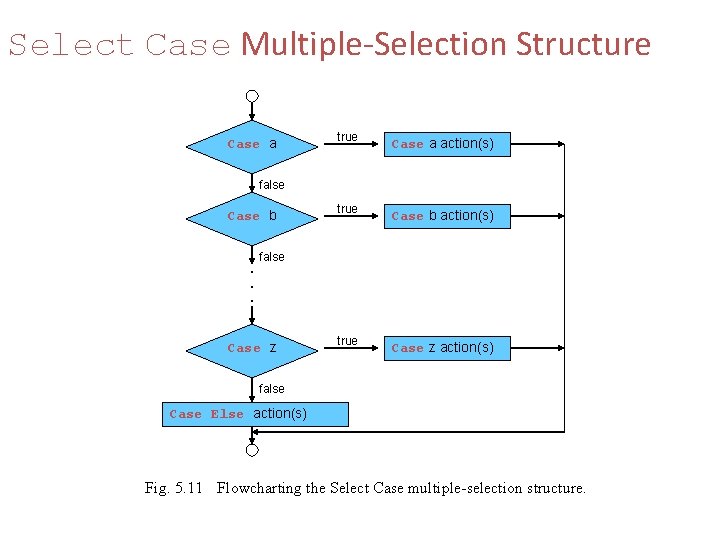
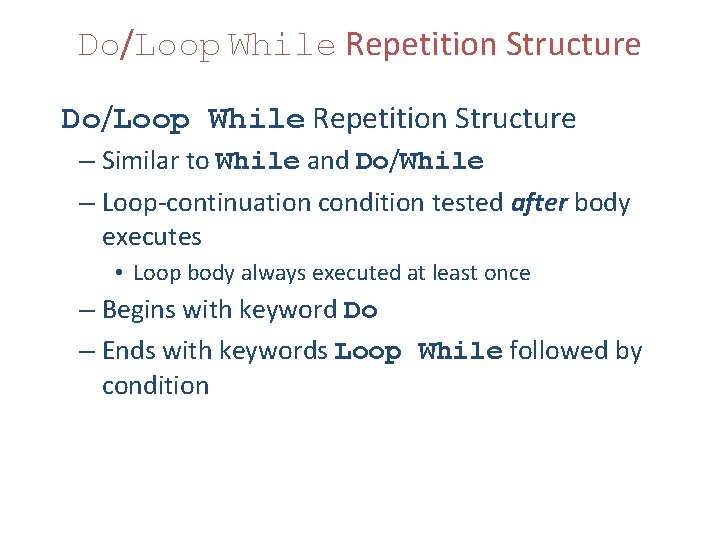
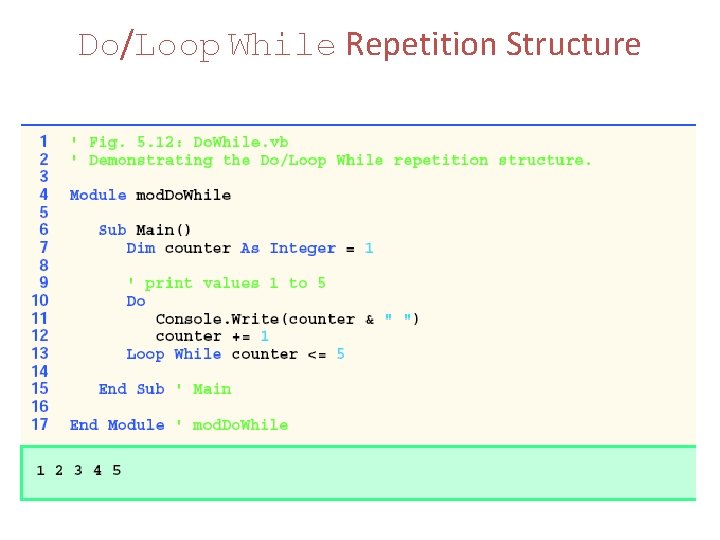
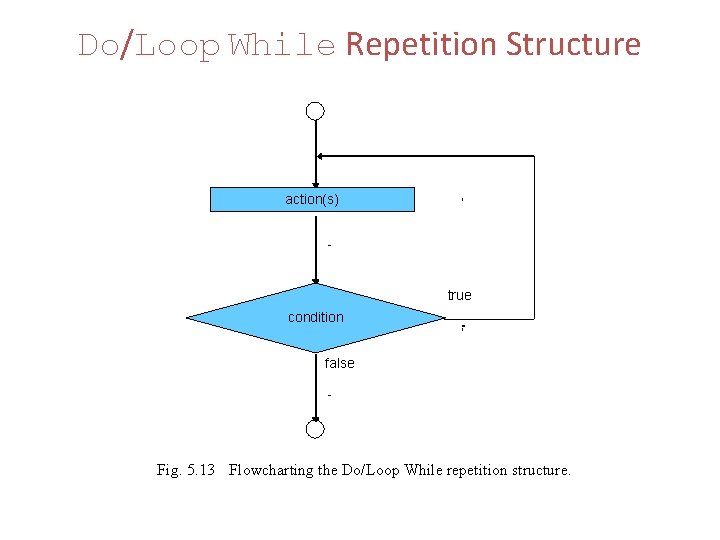
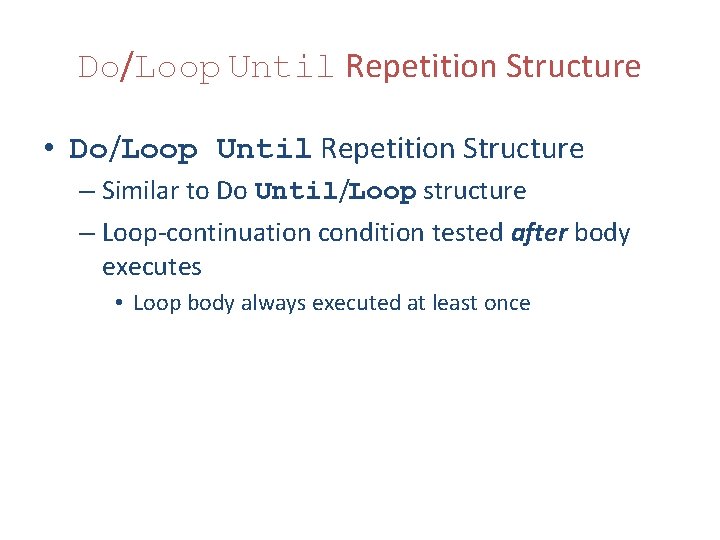
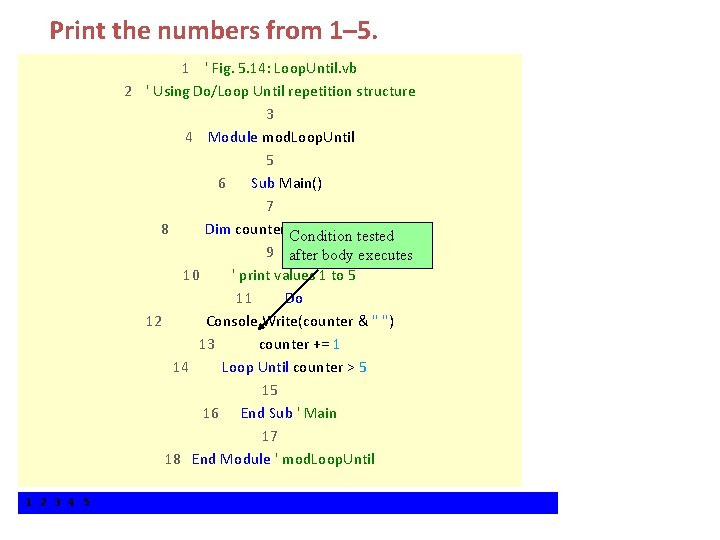
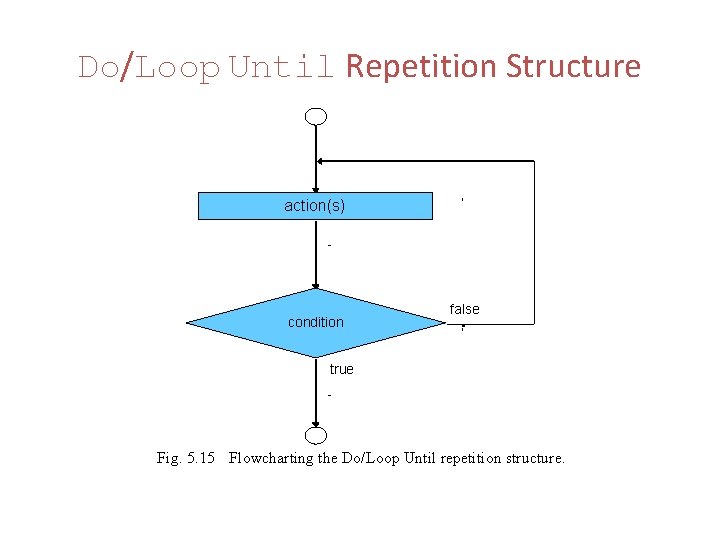
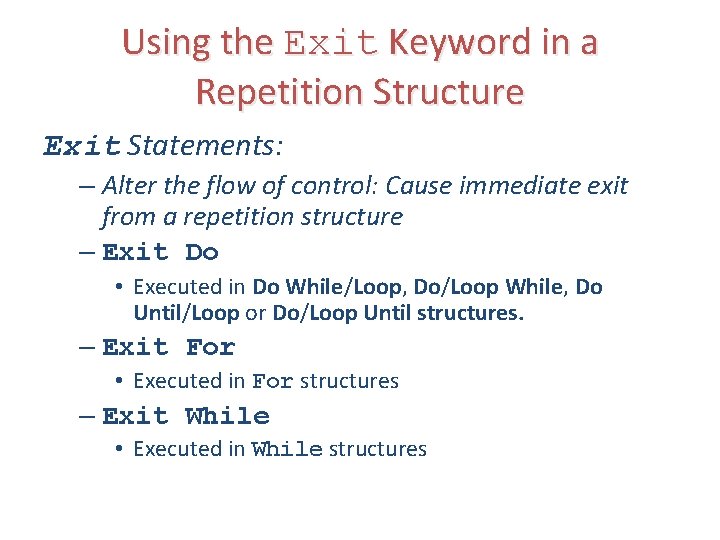
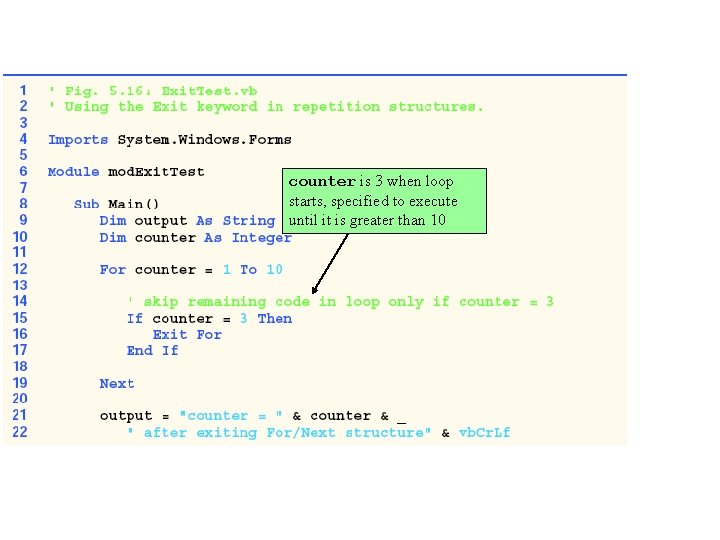
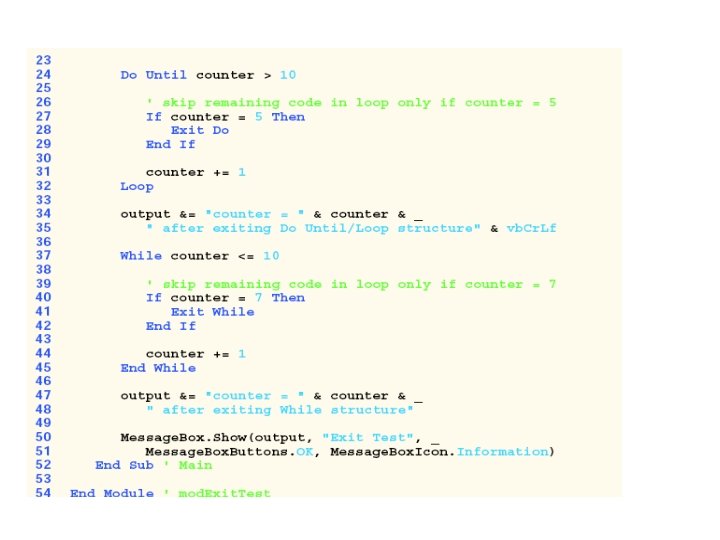
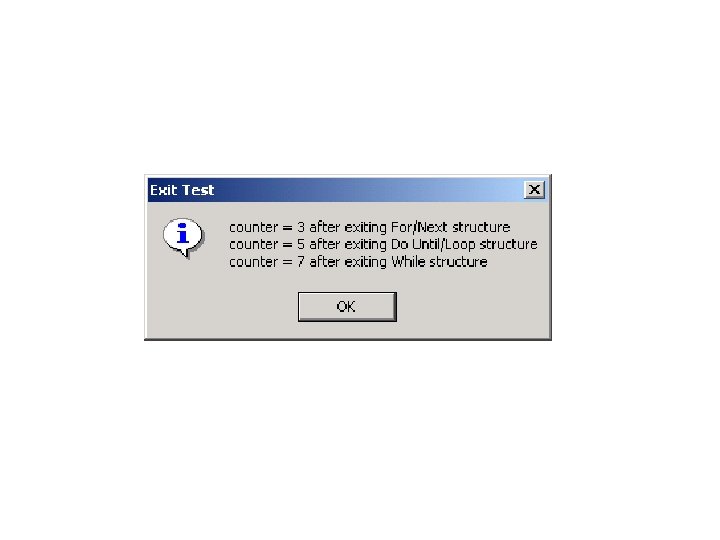
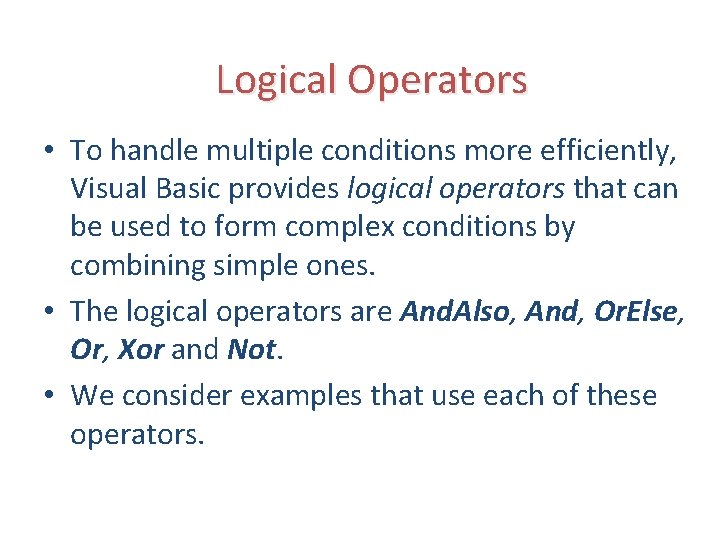
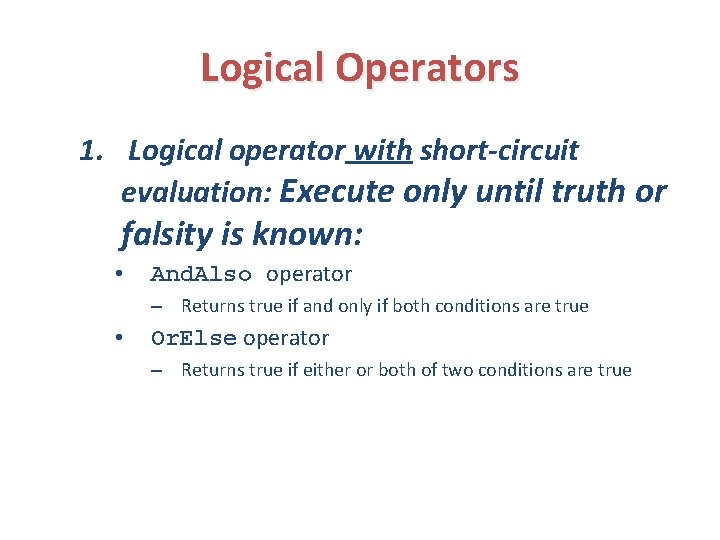
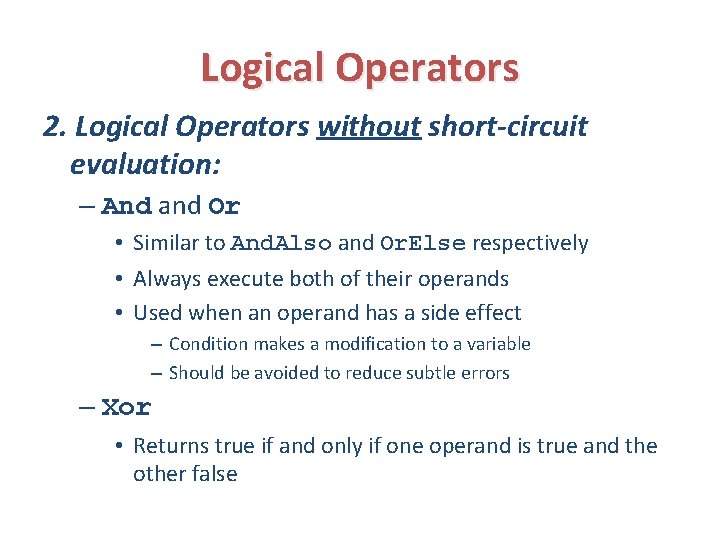
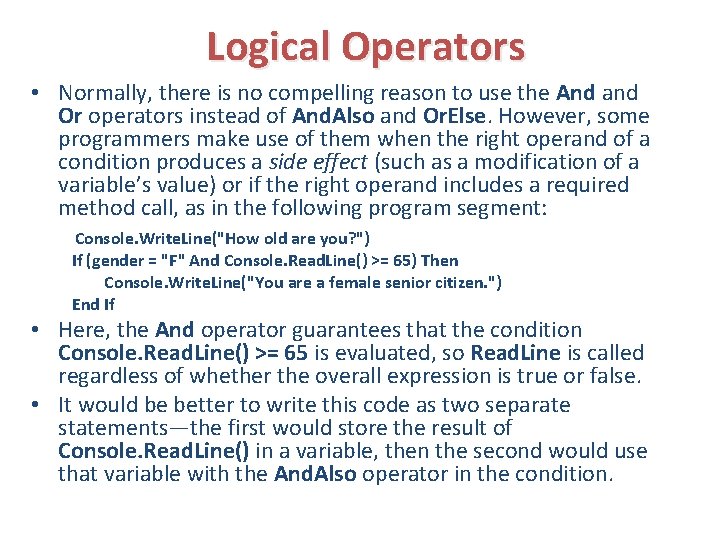
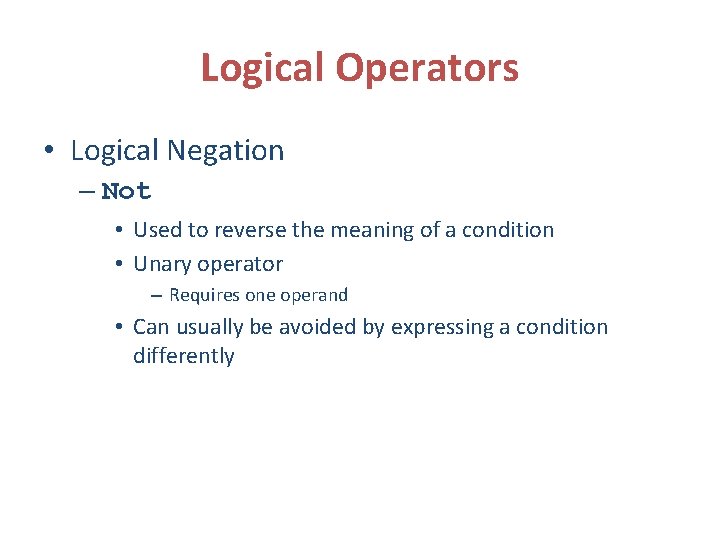
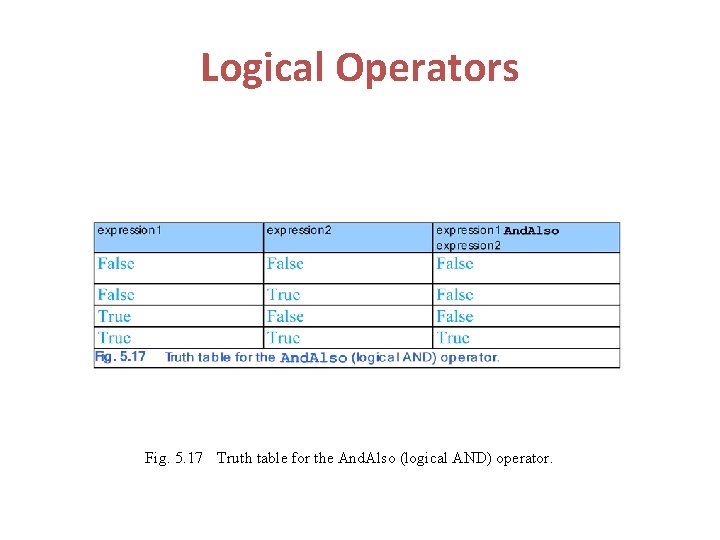
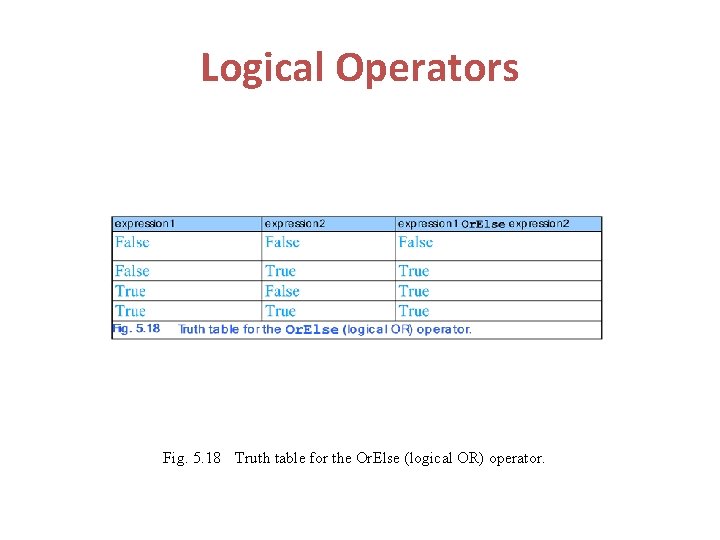
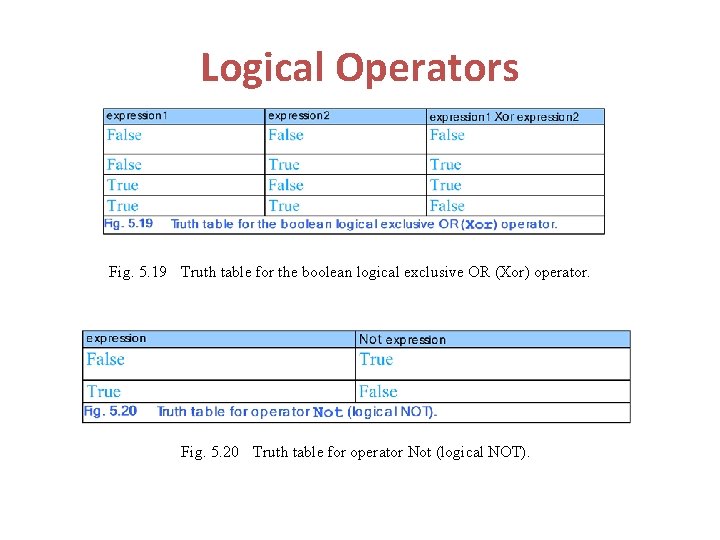
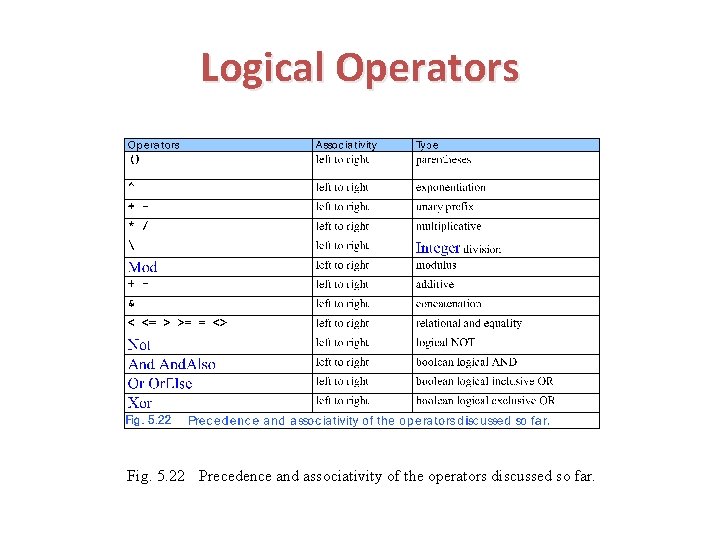
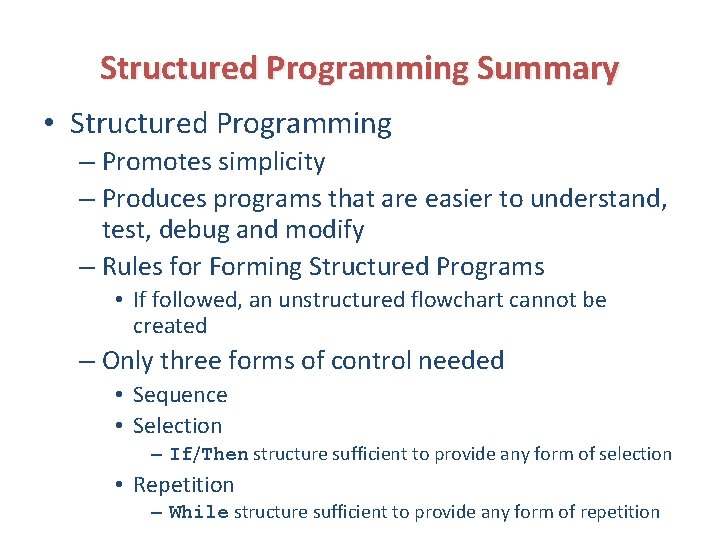
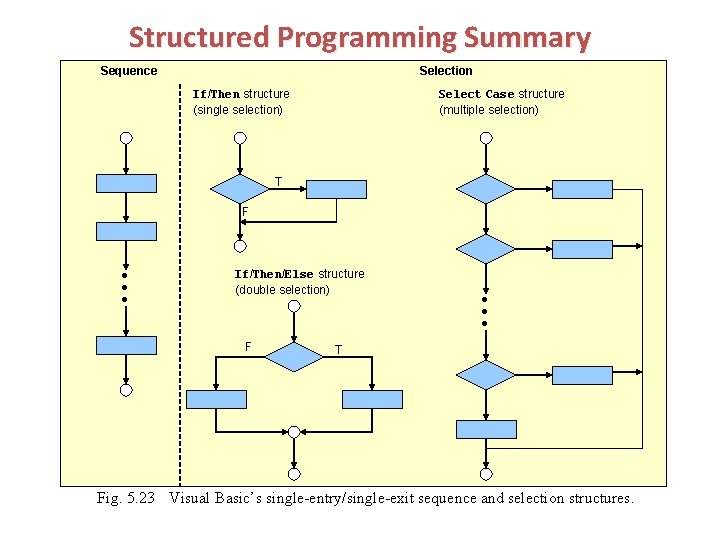
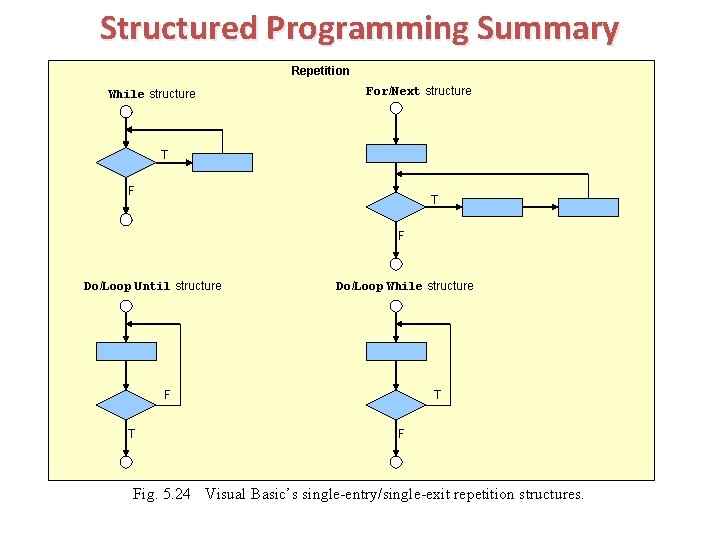
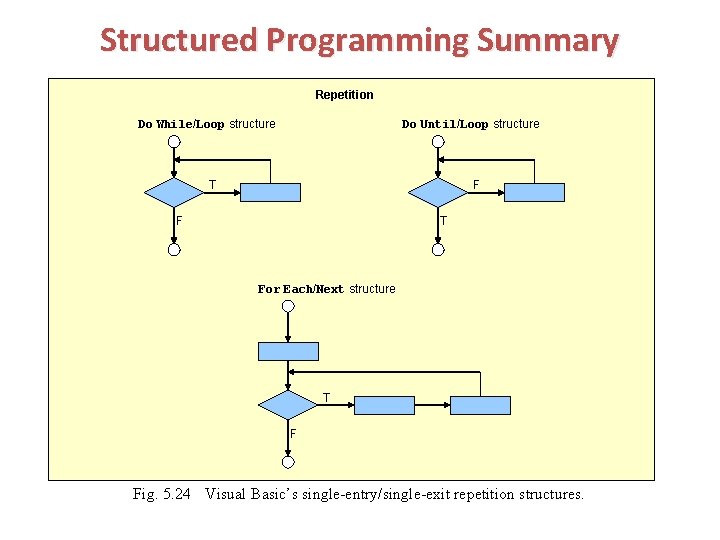
- Slides: 38
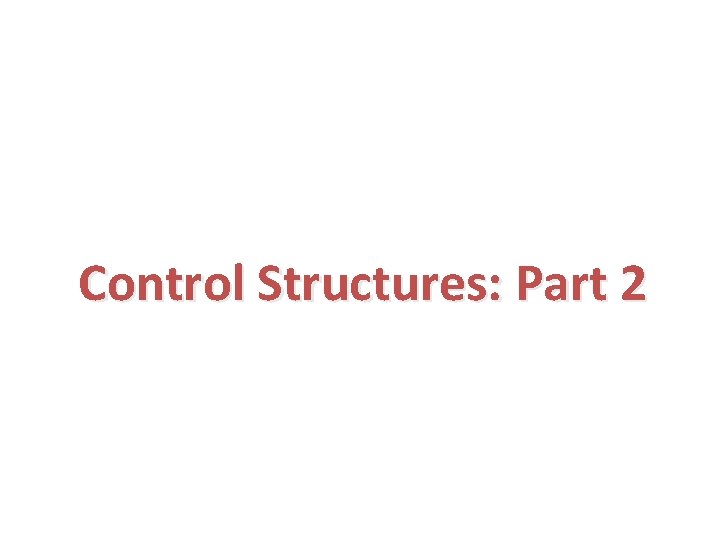
Control Structures: Part 2
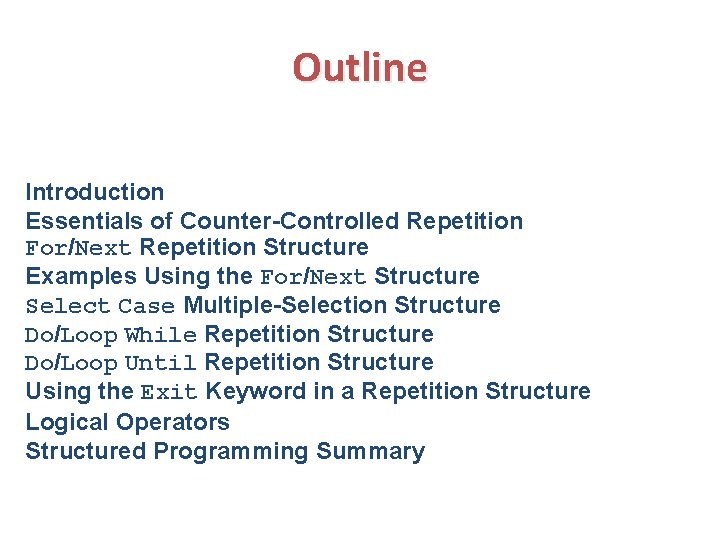
Outline Introduction Essentials of Counter-Controlled Repetition For/Next Repetition Structure Examples Using the For/Next Structure Select Case Multiple-Selection Structure Do/Loop While Repetition Structure Do/Loop Until Repetition Structure Using the Exit Keyword in a Repetition Structure Logical Operators Structured Programming Summary
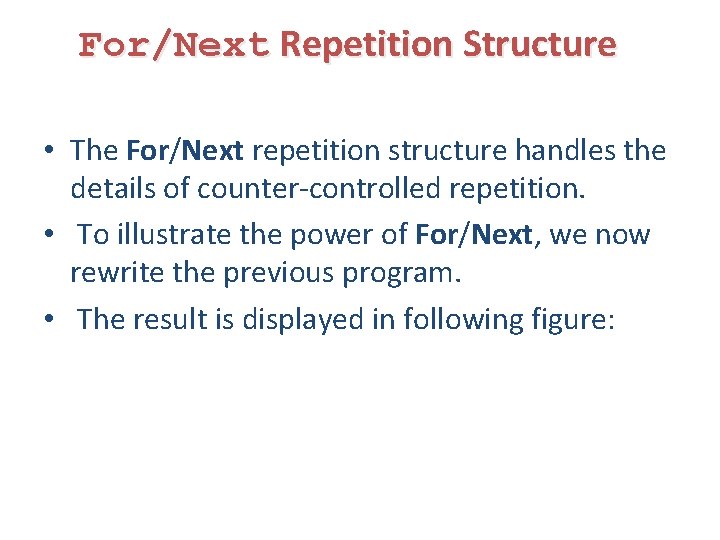
For/Next Repetition Structure • The For/Next repetition structure handles the details of counter-controlled repetition. • To illustrate the power of For/Next, we now rewrite the previous program. • The result is displayed in following figure:
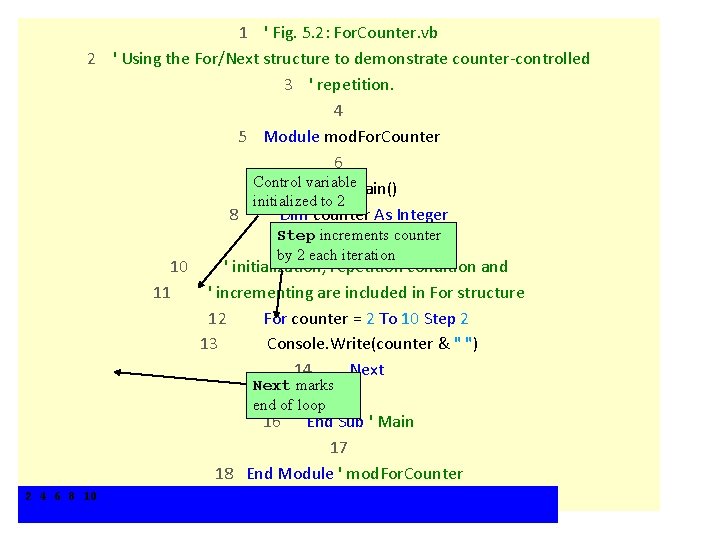
1 ' Fig. 5. 2: For. Counter. vb 2 ' Using the For/Next structure to demonstrate counter-controlled 3 ' repetition. 4 5 Module mod. For. Counter 6 Control 7 variable Sub Main() initialized to 2 8 Dim counter As Integer Step increments counter 9 by 2 each iteration 10 ' initialization, repetition condition and 11 ' incrementing are included in For structure 12 For counter = 2 To 10 Step 2 13 Console. Write(counter & " ") 14 Next marks 15 end of loop 16 End Sub ' Main 17 18 End Module ' mod. For. Counter 2 4 6 8 10
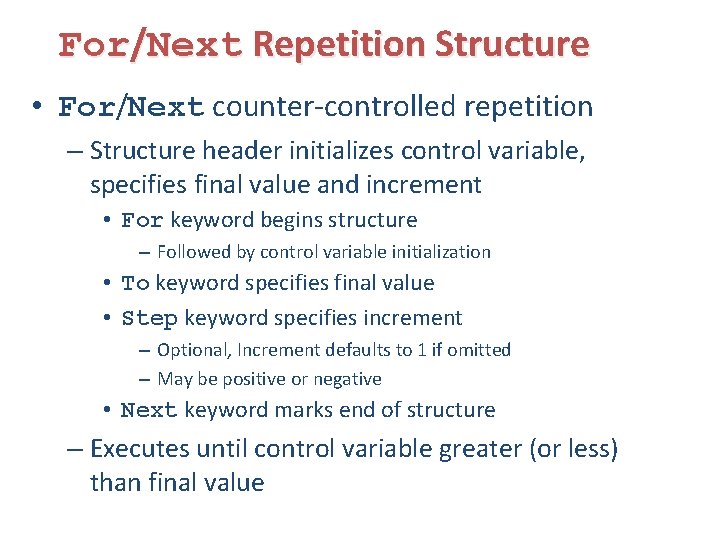
For/Next Repetition Structure • For/Next counter-controlled repetition – Structure header initializes control variable, specifies final value and increment • For keyword begins structure – Followed by control variable initialization • To keyword specifies final value • Step keyword specifies increment – Optional, Increment defaults to 1 if omitted – May be positive or negative • Next keyword marks end of structure – Executes until control variable greater (or less) than final value
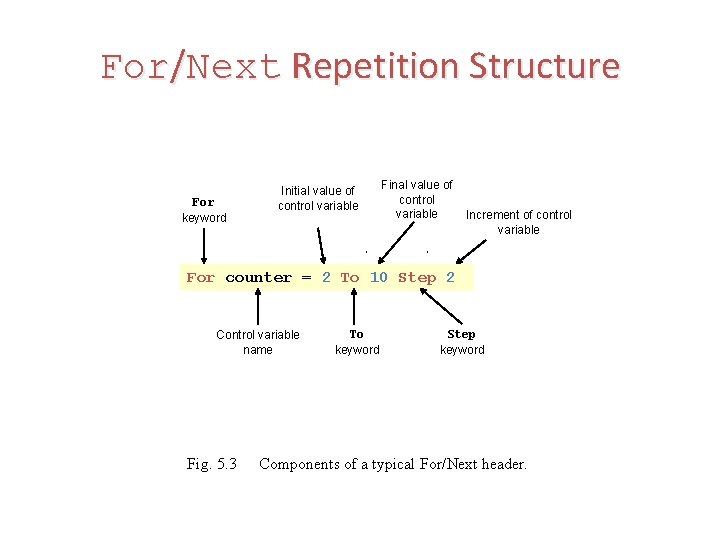
For/Next Repetition Structure For keyword Initial value of control variable Final value of control variable Increment of control variable For counter = 2 To 10 Step 2 Control variable name Fig. 5. 3 To keyword Step keyword Components of a typical For/Next header.
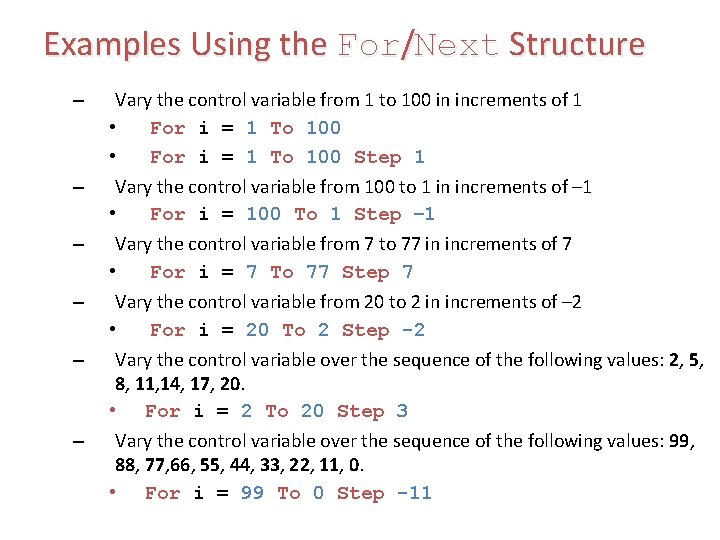
Examples Using the For/Next Structure – – – Vary the control variable from 1 to 100 in increments of 1 • For i = 1 To 100 Step 1 Vary the control variable from 100 to 1 in increments of – 1 • For i = 100 To 1 Step – 1 Vary the control variable from 7 to 77 in increments of 7 • For i = 7 To 77 Step 7 Vary the control variable from 20 to 2 in increments of – 2 • For i = 20 To 2 Step -2 Vary the control variable over the sequence of the following values: 2, 5, 8, 11, 14, 17, 20. • For i = 2 To 20 Step 3 Vary the control variable over the sequence of the following values: 99, 88, 77, 66, 55, 44, 33, 22, 11, 0. • For i = 99 To 0 Step -11
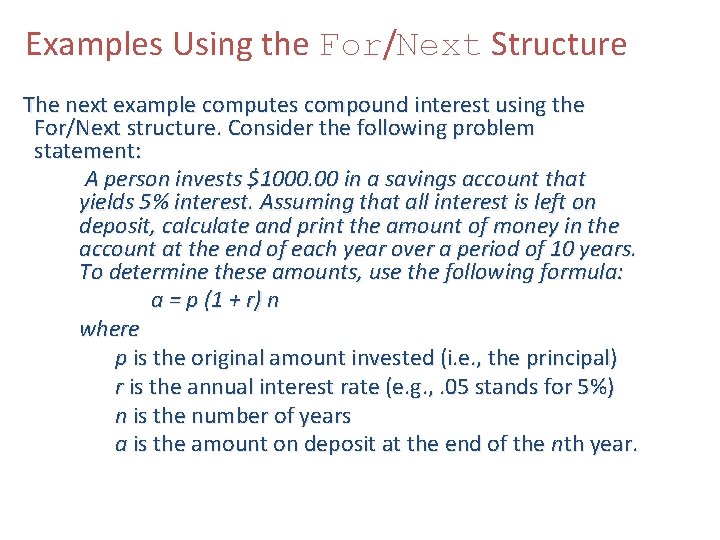
Examples Using the For/Next Structure The next example computes compound interest using the For/Next structure. Consider the following problem statement: A person invests $1000. 00 in a savings account that yields 5% interest. Assuming that all interest is left on deposit, calculate and print the amount of money in the account at the end of each year over a period of 10 years. To determine these amounts, use the following formula: a = p (1 + r) n where p is the original amount invested (i. e. , the principal) r is the annual interest rate (e. g. , . 05 stands for 5%) n is the number of years a is the amount on deposit at the end of the nth year.
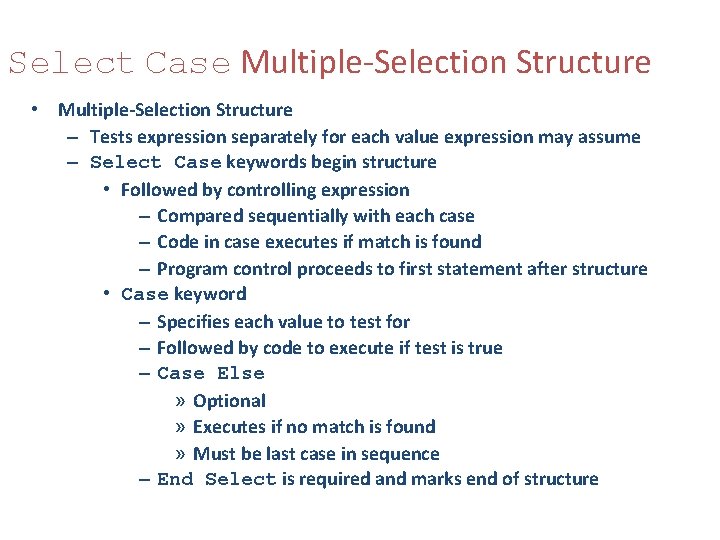
Select Case Multiple-Selection Structure • Multiple-Selection Structure – Tests expression separately for each value expression may assume – Select Case keywords begin structure • Followed by controlling expression – Compared sequentially with each case – Code in case executes if match is found – Program control proceeds to first statement after structure • Case keyword – Specifies each value to test for – Followed by code to execute if test is true – Case Else » Optional » Executes if no match is found » Must be last case in sequence – End Select is required and marks end of structure
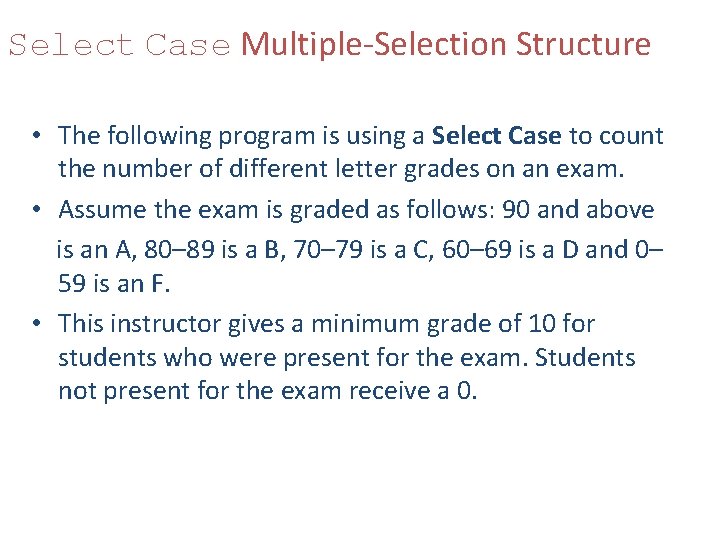
Select Case Multiple-Selection Structure • The following program is using a Select Case to count the number of different letter grades on an exam. • Assume the exam is graded as follows: 90 and above is an A, 80– 89 is a B, 70– 79 is a C, 60– 69 is a D and 0– 59 is an F. • This instructor gives a minimum grade of 10 for students who were present for the exam. Students not present for the exam receive a 0.
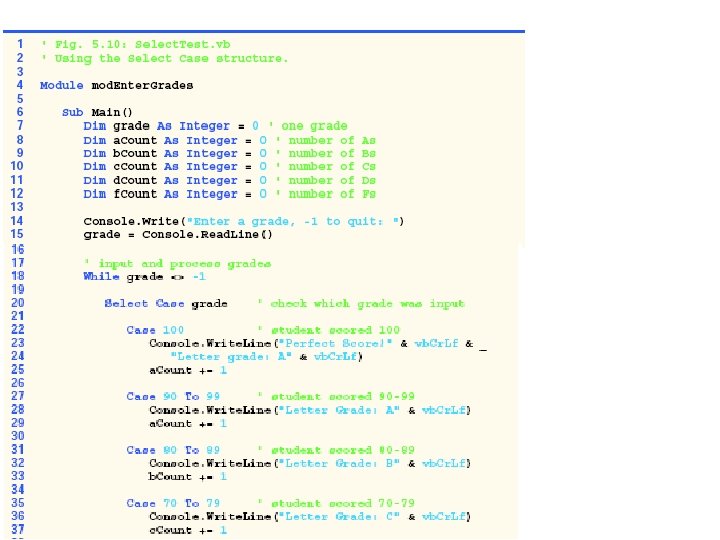
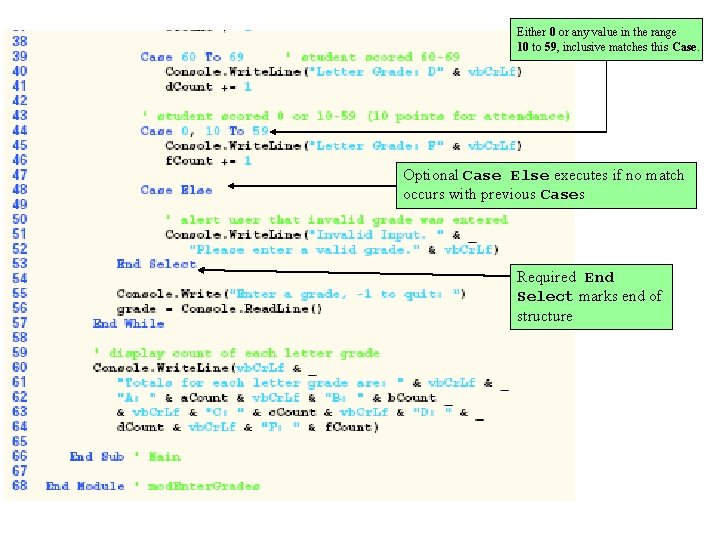
Either 0 or any value in the range 10 to 59, inclusive matches this Case. Optional Case Else executes if no match occurs with previous Cases Required End Select marks end of structure
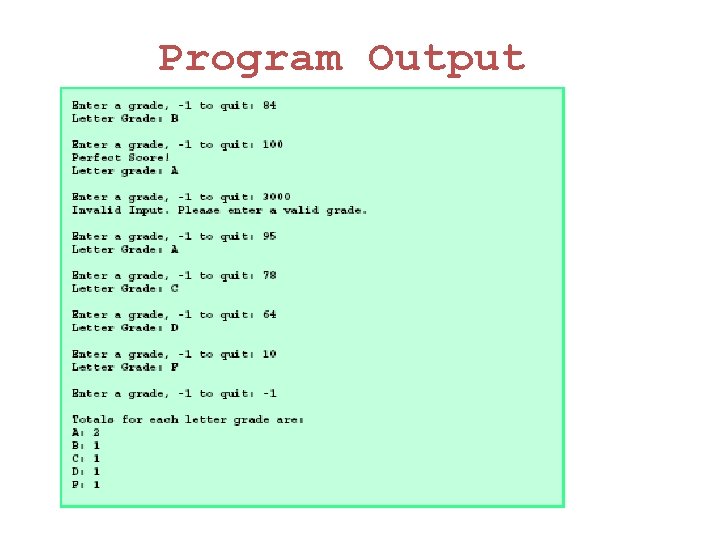
Program Output
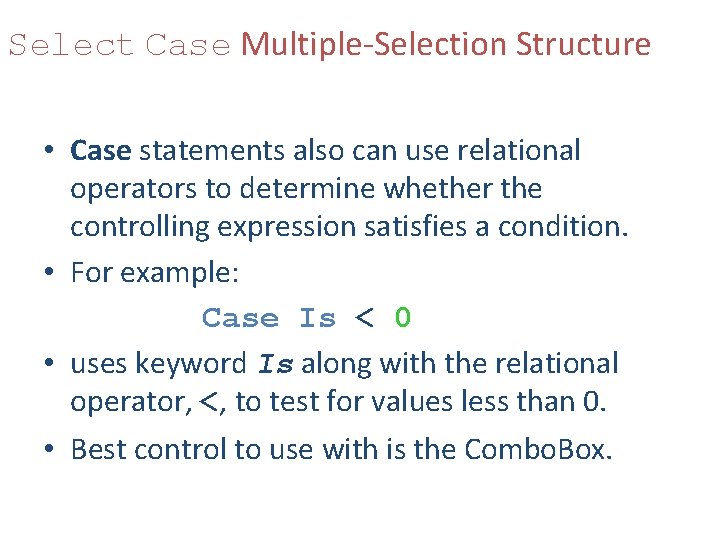
Select Case Multiple-Selection Structure • Case statements also can use relational operators to determine whether the controlling expression satisfies a condition. • For example: Case Is < 0 • uses keyword Is along with the relational operator, <, to test for values less than 0. • Best control to use with is the Combo. Box.
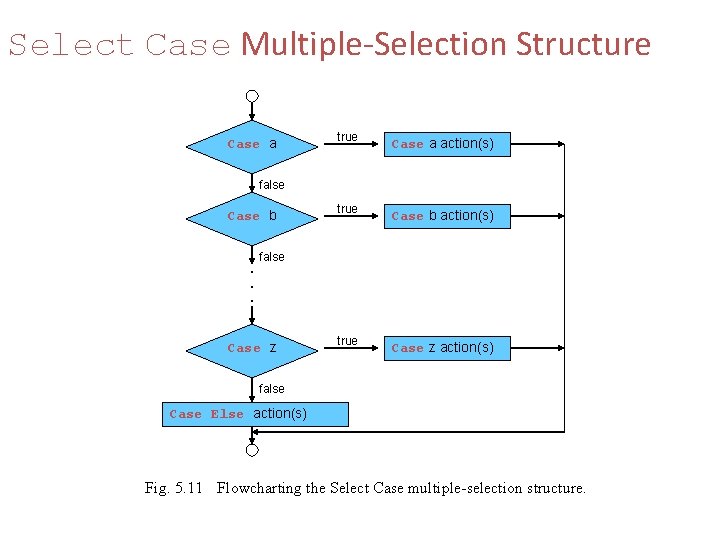
Select Case Multiple-Selection Structure Case a true Case a action(s) true Case b action(s) true Case z action(s) false Case b . . . false Case z false Case Else action(s) Fig. 5. 11 Flowcharting the Select Case multiple-selection structure.
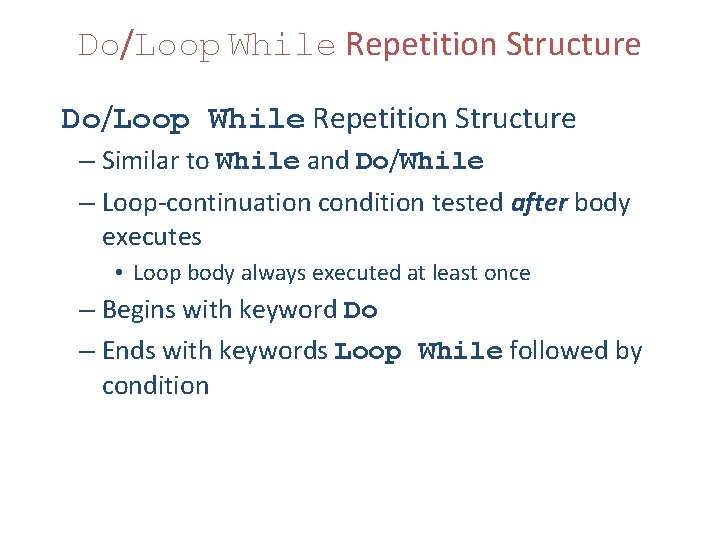
Do/Loop While Repetition Structure – Similar to While and Do/While – Loop-continuation condition tested after body executes • Loop body always executed at least once – Begins with keyword Do – Ends with keywords Loop While followed by condition
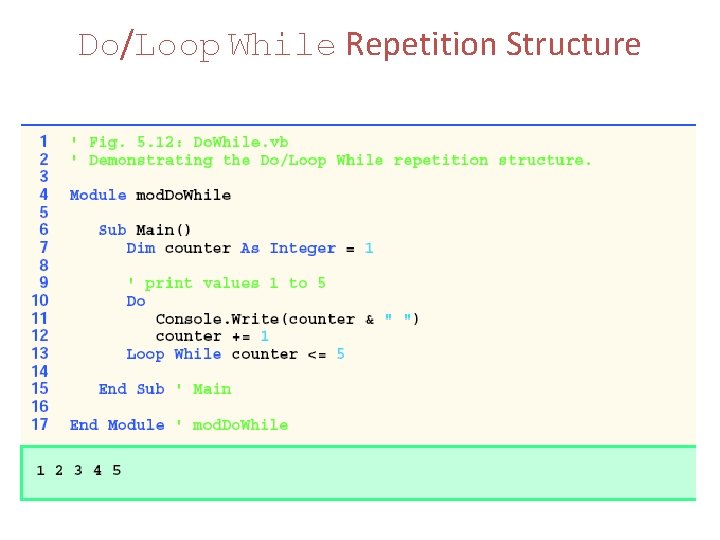
Do/Loop While Repetition Structure
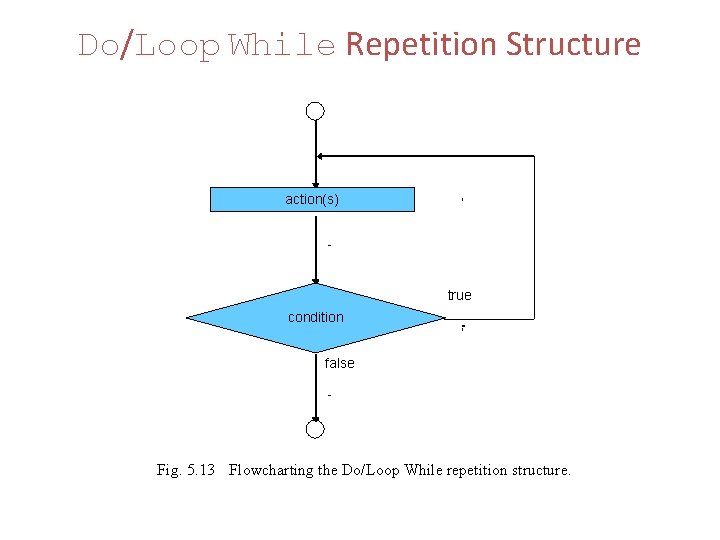
Do/Loop While Repetition Structure action(s) true condition false Fig. 5. 13 Flowcharting the Do/Loop While repetition structure.
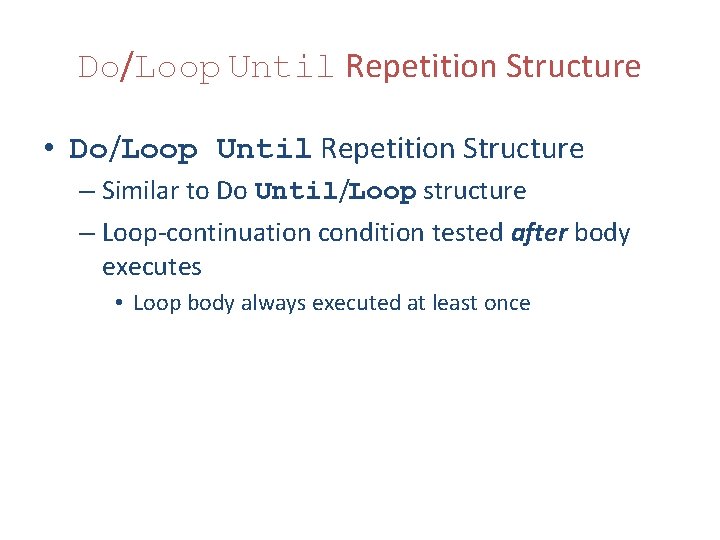
Do/Loop Until Repetition Structure • Do/Loop Until Repetition Structure – Similar to Do Until/Loop structure – Loop-continuation condition tested after body executes • Loop body always executed at least once
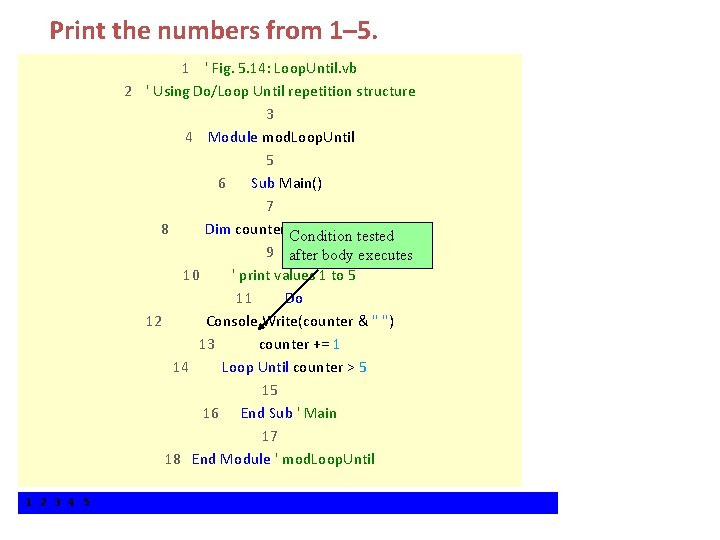
Print the numbers from 1– 5. 1 ' Fig. 5. 14: Loop. Until. vb 2 ' Using Do/Loop Until repetition structure 3 4 Module mod. Loop. Until 5 6 Sub Main() 7 8 Dim counter Condition As Integer tested =1 9 after body executes 10 ' print values 1 to 5 11 Do 12 Console. Write(counter & " ") 13 counter += 1 14 Loop Until counter > 5 15 16 End Sub ' Main 17 18 End Module ' mod. Loop. Until 1 2 3 4 5
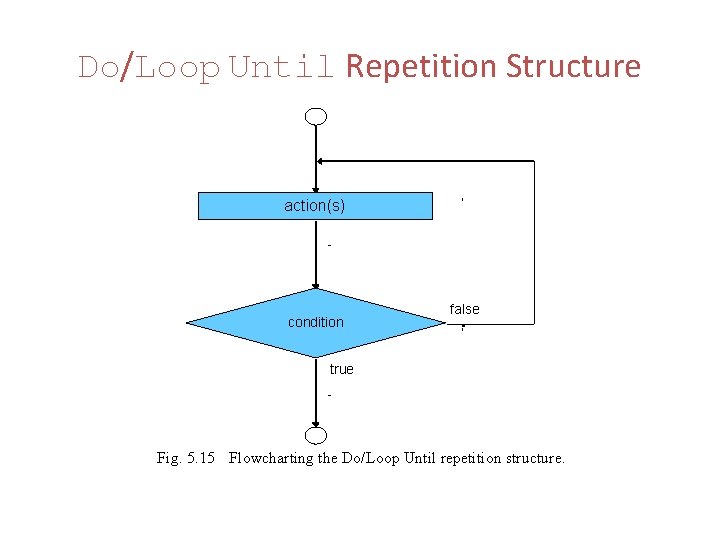
Do/Loop Until Repetition Structure action(s) condition false true Fig. 5. 15 Flowcharting the Do/Loop Until repetition structure.
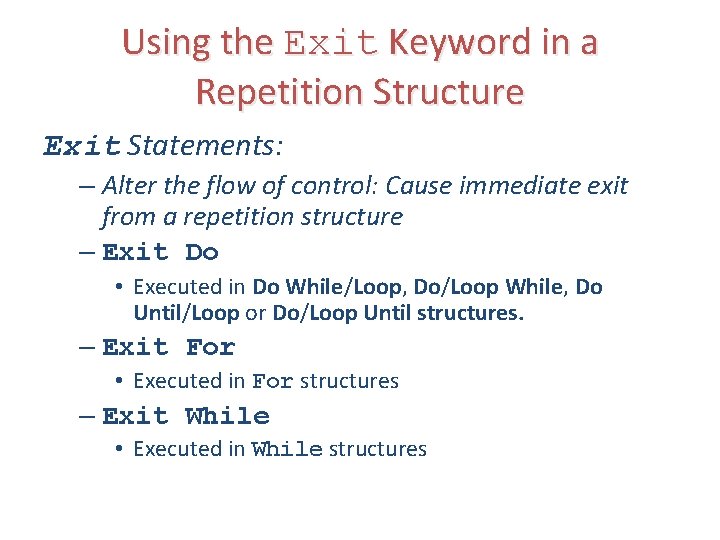
Using the Exit Keyword in a Repetition Structure Exit Statements: – Alter the flow of control: Cause immediate exit from a repetition structure – Exit Do • Executed in Do While/Loop, Do/Loop While, Do Until/Loop or Do/Loop Until structures. – Exit For • Executed in For structures – Exit While • Executed in While structures
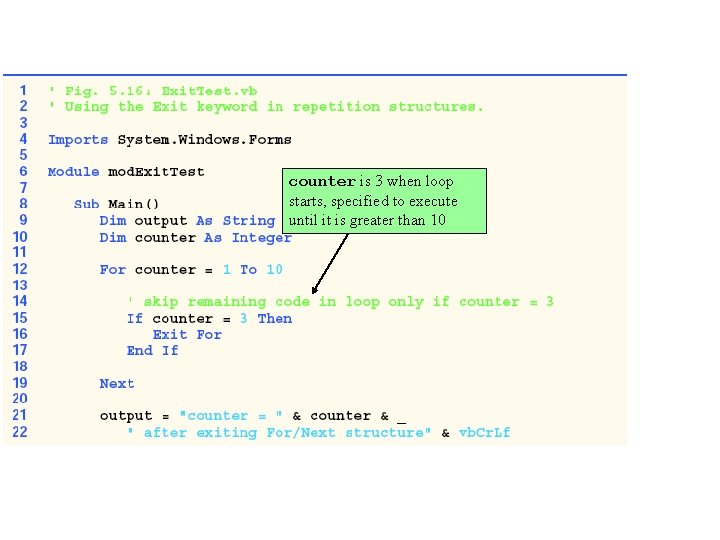
counter is 3 when loop starts, specified to execute until it is greater than 10
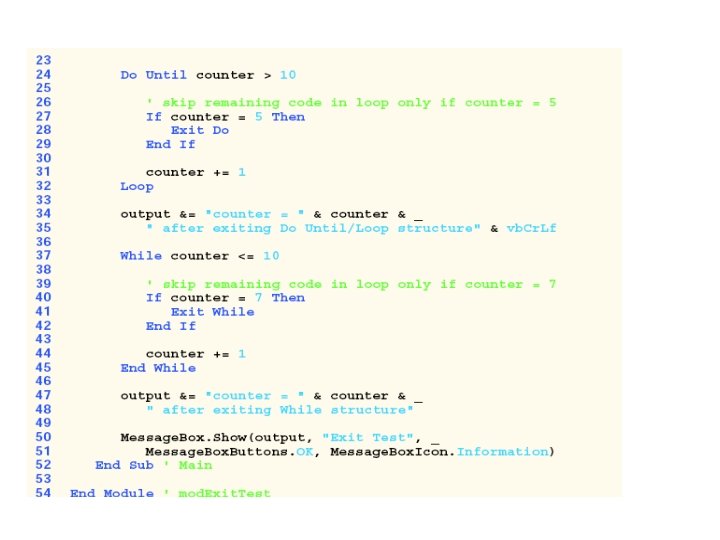
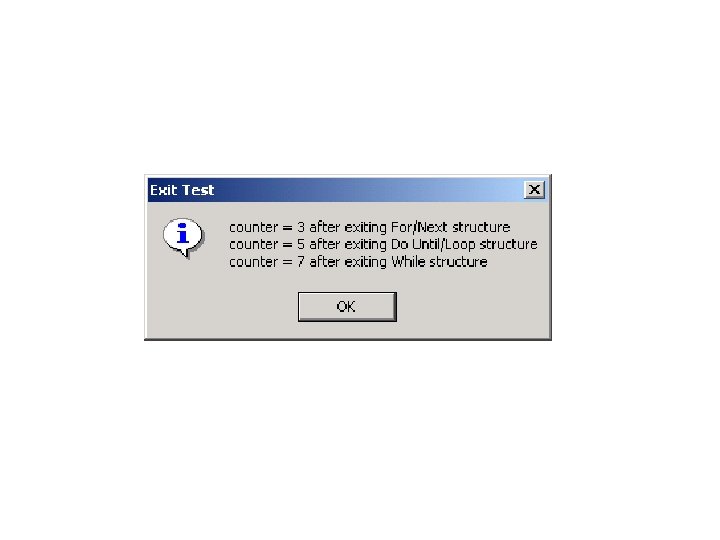
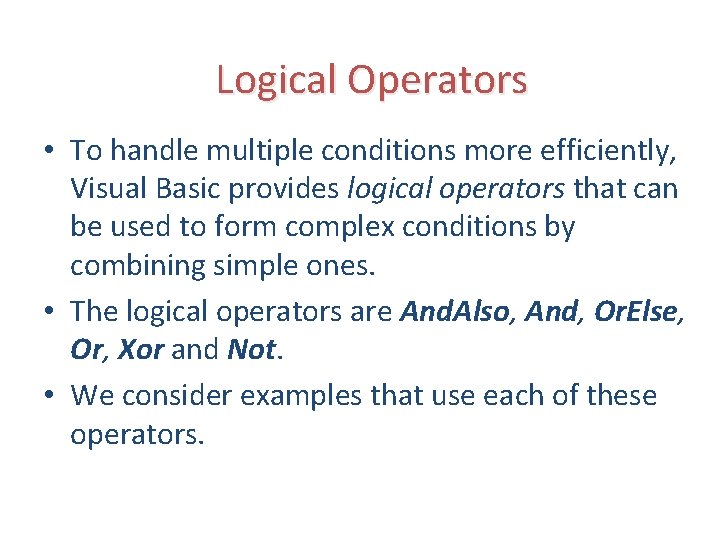
Logical Operators • To handle multiple conditions more efficiently, Visual Basic provides logical operators that can be used to form complex conditions by combining simple ones. • The logical operators are And. Also, And, Or. Else, Or, Xor and Not. • We consider examples that use each of these operators.
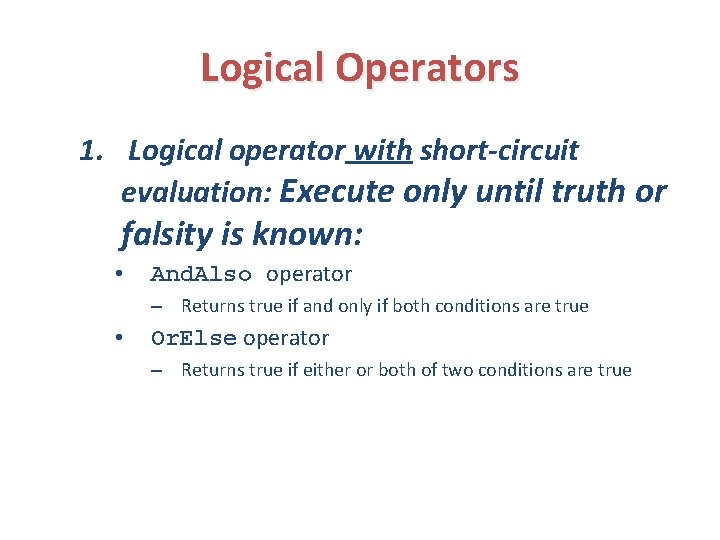
Logical Operators 1. Logical operator with short-circuit evaluation: Execute only until truth or falsity is known: • And. Also operator – Returns true if and only if both conditions are true • Or. Else operator – Returns true if either or both of two conditions are true
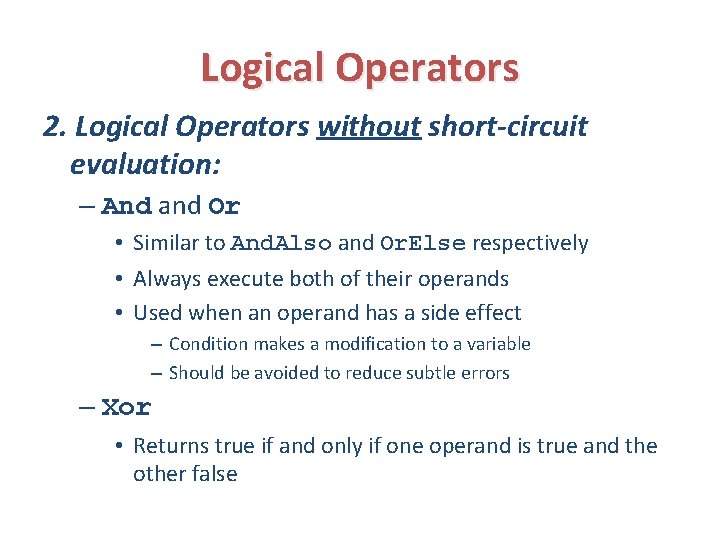
Logical Operators 2. Logical Operators without short-circuit evaluation: – And and Or • Similar to And. Also and Or. Else respectively • Always execute both of their operands • Used when an operand has a side effect – Condition makes a modification to a variable – Should be avoided to reduce subtle errors – Xor • Returns true if and only if one operand is true and the other false
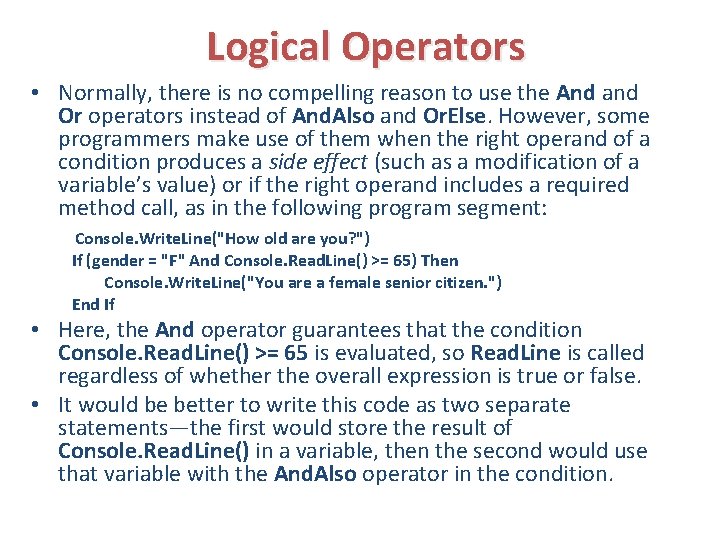
Logical Operators • Normally, there is no compelling reason to use the And and Or operators instead of And. Also and Or. Else. However, some programmers make use of them when the right operand of a condition produces a side effect (such as a modification of a variable’s value) or if the right operand includes a required method call, as in the following program segment: Console. Write. Line("How old are you? ") If (gender = "F" And Console. Read. Line() >= 65) Then Console. Write. Line("You are a female senior citizen. ") End If • Here, the And operator guarantees that the condition Console. Read. Line() >= 65 is evaluated, so Read. Line is called regardless of whether the overall expression is true or false. • It would be better to write this code as two separate statements—the first would store the result of Console. Read. Line() in a variable, then the second would use that variable with the And. Also operator in the condition.
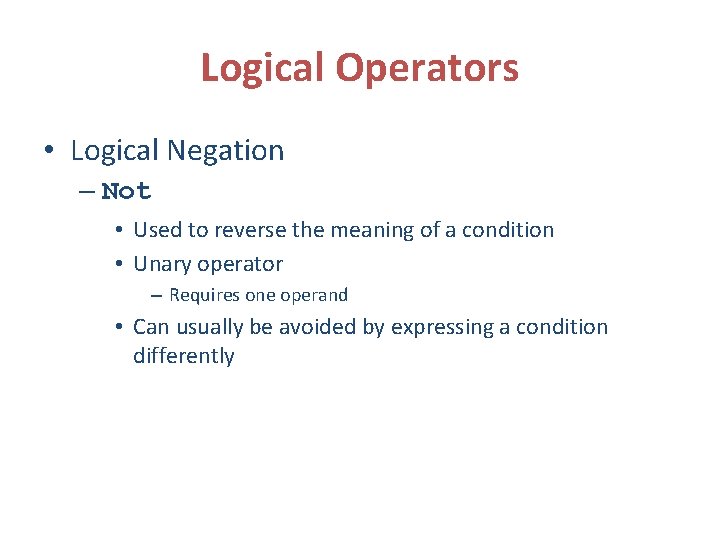
Logical Operators • Logical Negation – Not • Used to reverse the meaning of a condition • Unary operator – Requires one operand • Can usually be avoided by expressing a condition differently
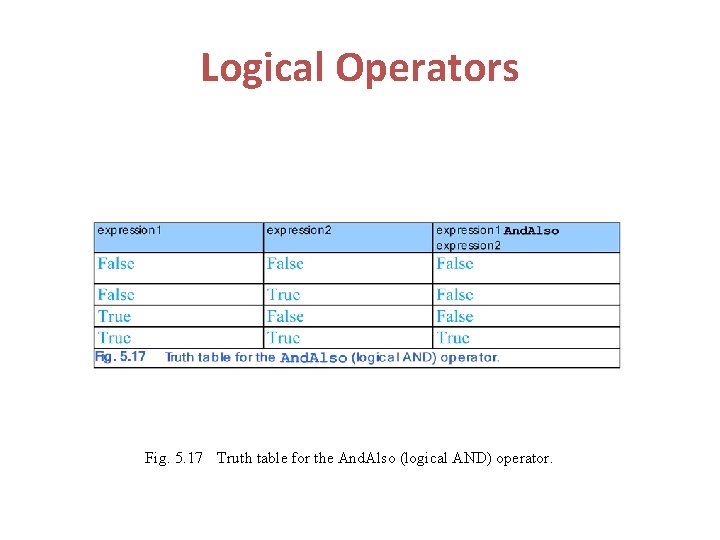
Logical Operators Fig. 5. 17 Truth table for the And. Also (logical AND) operator.
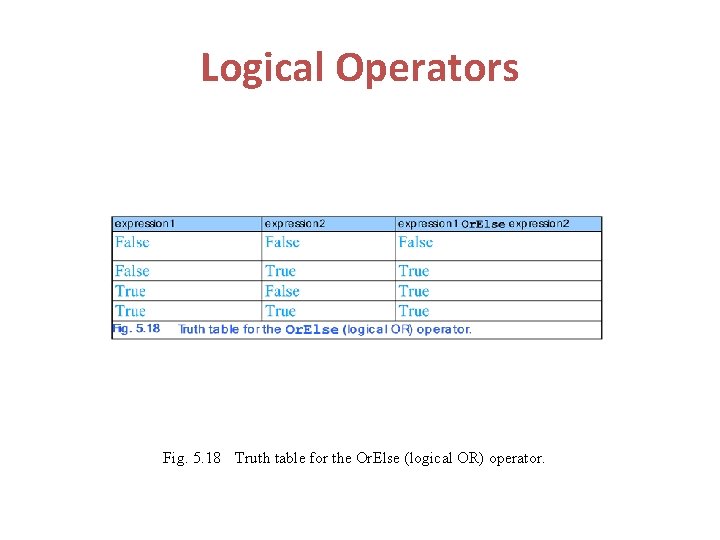
Logical Operators Fig. 5. 18 Truth table for the Or. Else (logical OR) operator.
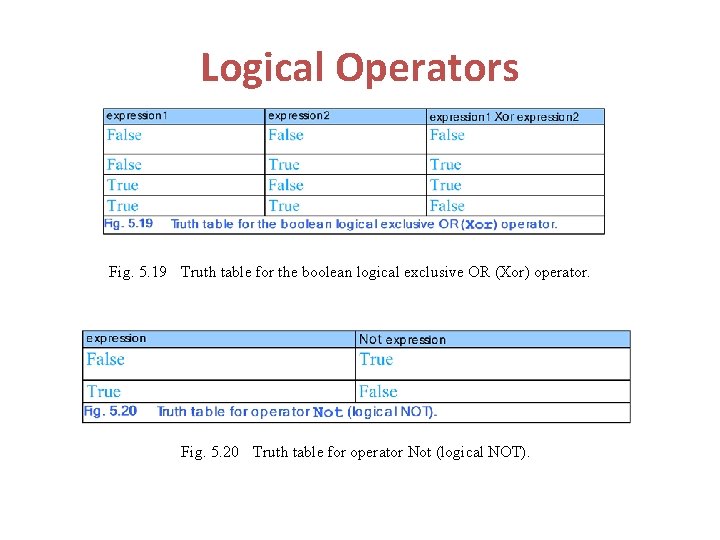
Logical Operators Fig. 5. 19 Truth table for the boolean logical exclusive OR (Xor) operator. Fig. 5. 20 Truth table for operator Not (logical NOT).
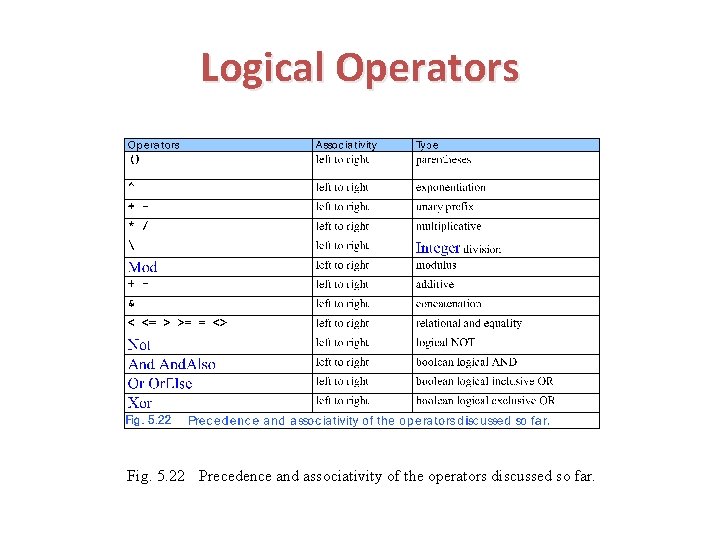
Logical Operators Fig. 5. 22 Precedence and associativity of the operators discussed so far.
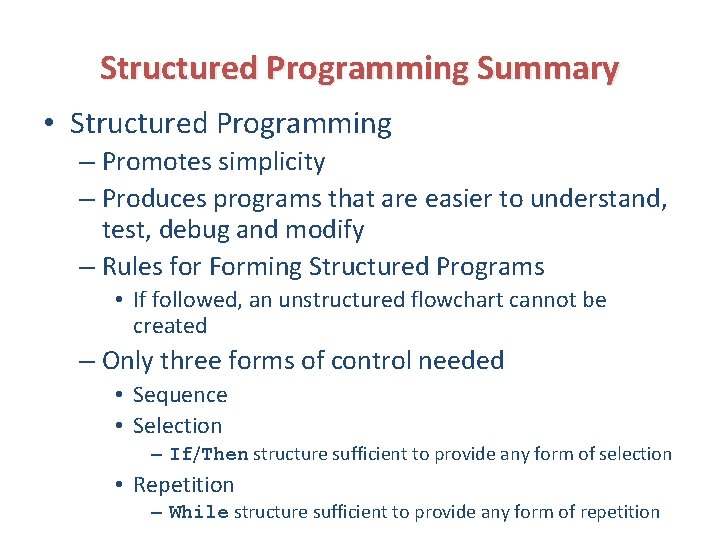
Structured Programming Summary • Structured Programming – Promotes simplicity – Produces programs that are easier to understand, test, debug and modify – Rules for Forming Structured Programs • If followed, an unstructured flowchart cannot be created – Only three forms of control needed • Sequence • Selection – If/Then structure sufficient to provide any form of selection • Repetition – While structure sufficient to provide any form of repetition
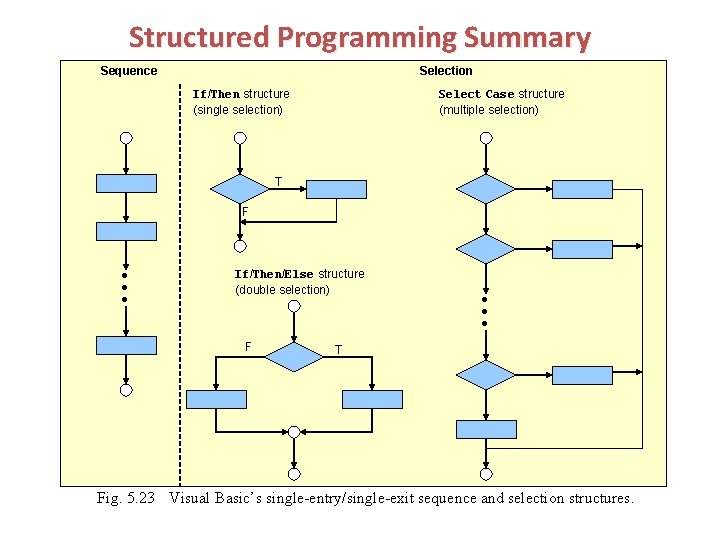
Structured Programming Summary Sequence Selection If/Then structure (single selection) Select Case structure (multiple selection) T F . . . If/Then/Else structure (double selection) F . . . T Fig. 5. 23 Visual Basic’s single-entry/single-exit sequence and selection structures.
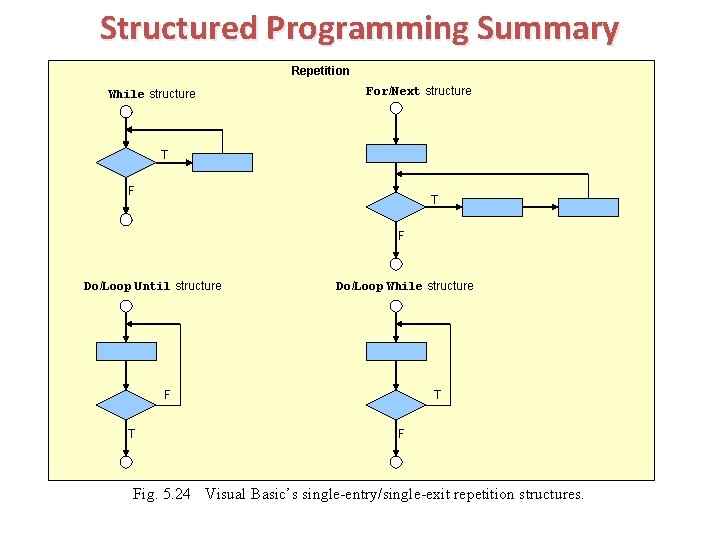
Structured Programming Summary Repetition While structure For/Next structure T F Do/Loop Until structure Do/Loop While structure F T T F Fig. 5. 24 Visual Basic’s single-entry/single-exit repetition structures.
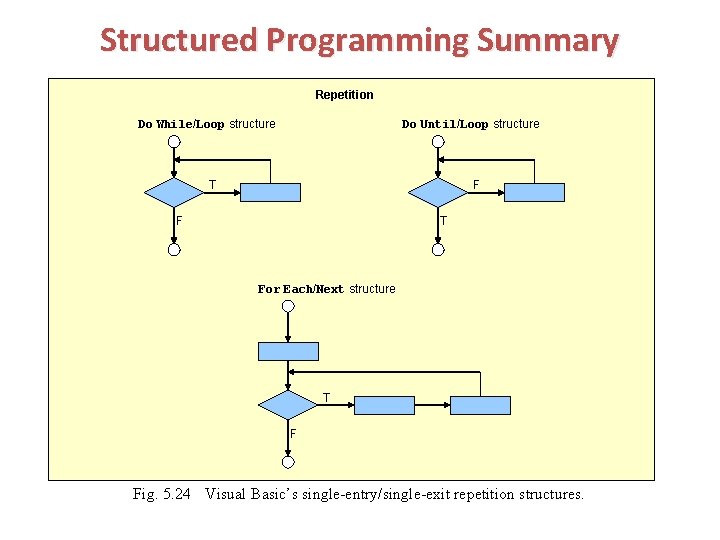
Structured Programming Summary Repetition Do While/Loop structure Do Until/Loop structure T F F T For Each/Next structure T F Fig. 5. 24 Visual Basic’s single-entry/single-exit repetition structures.