const Security A popular USENET joke In C
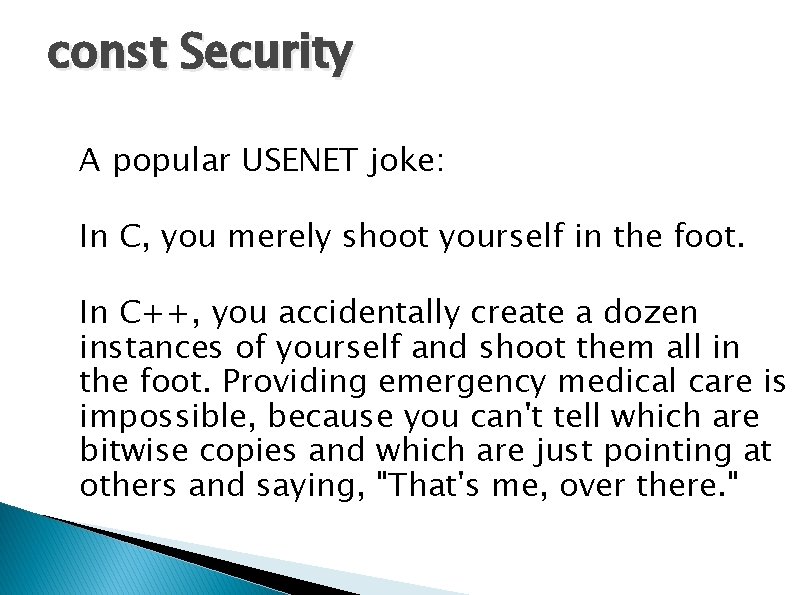
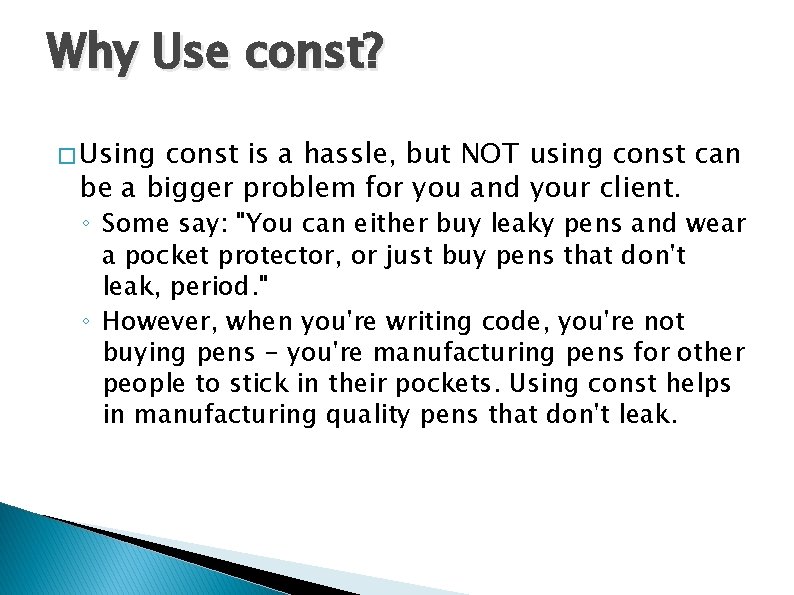
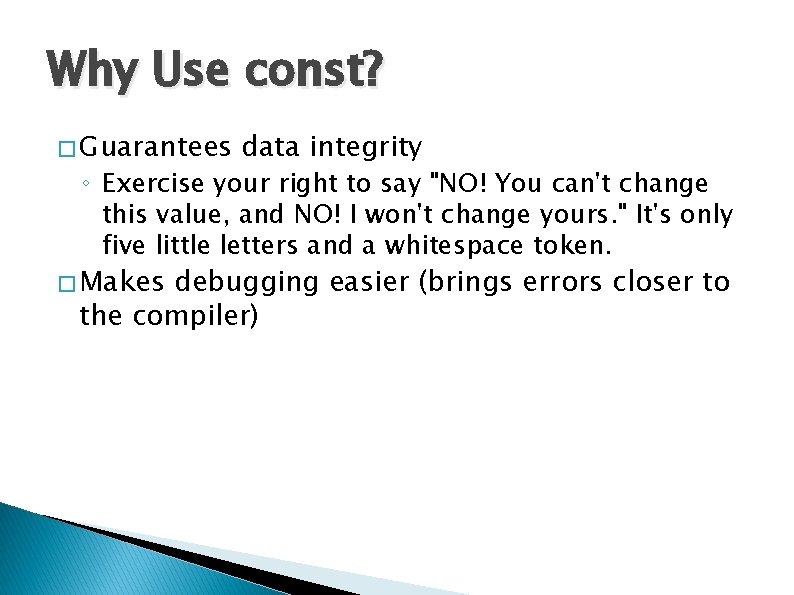
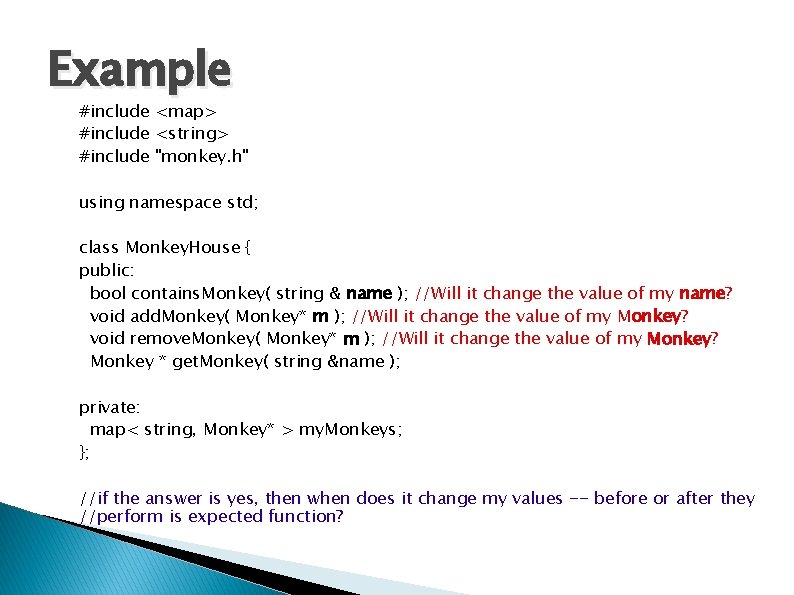
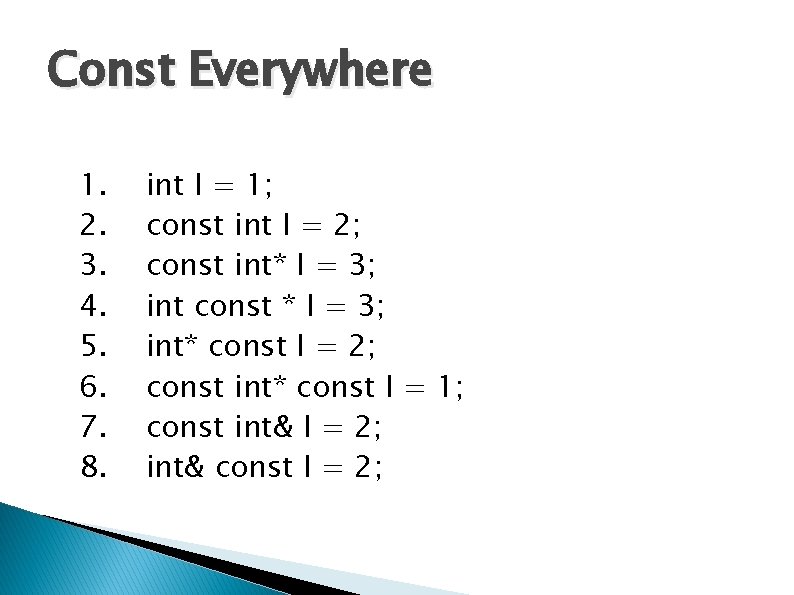
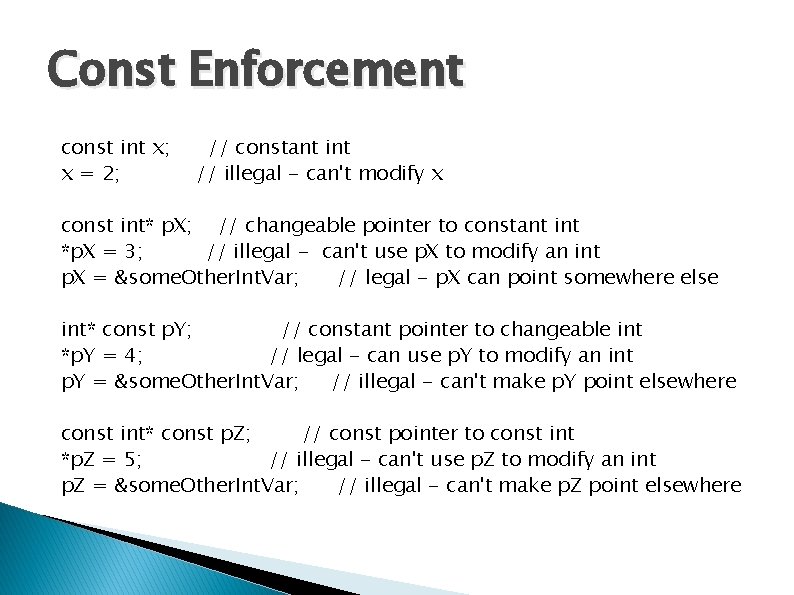
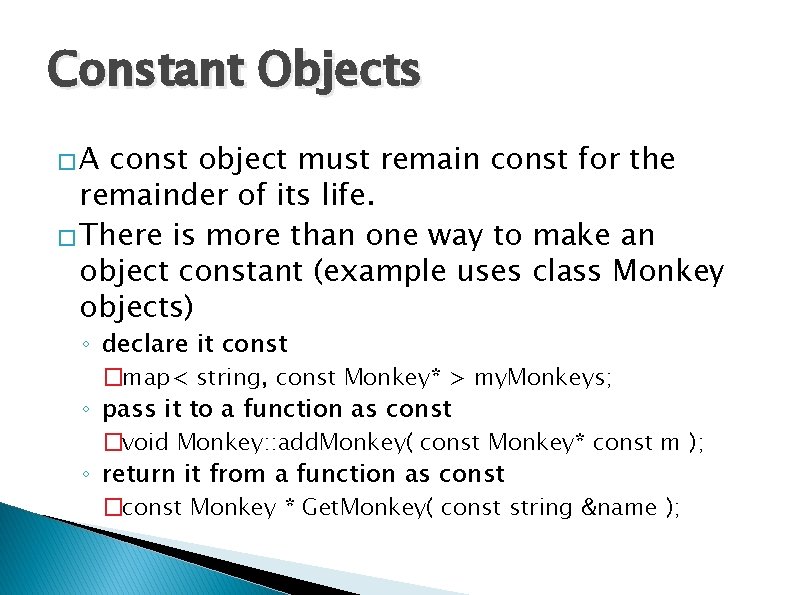
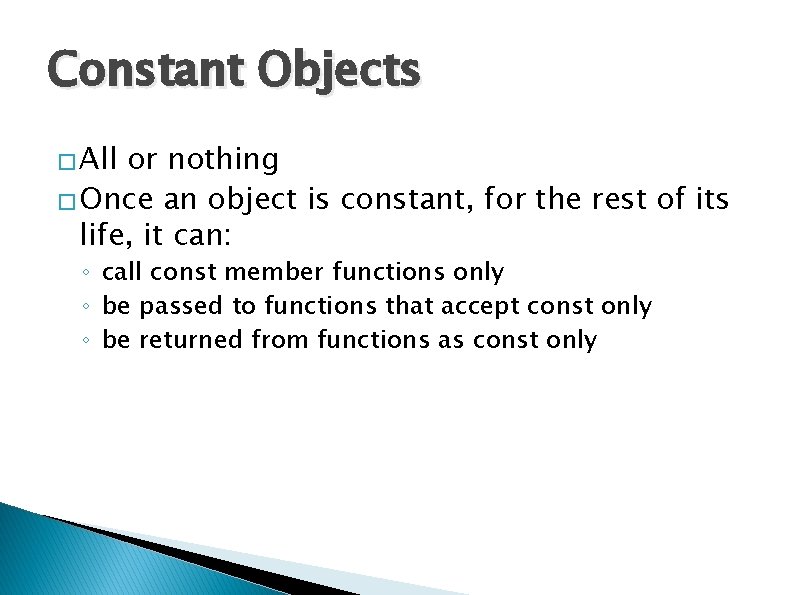
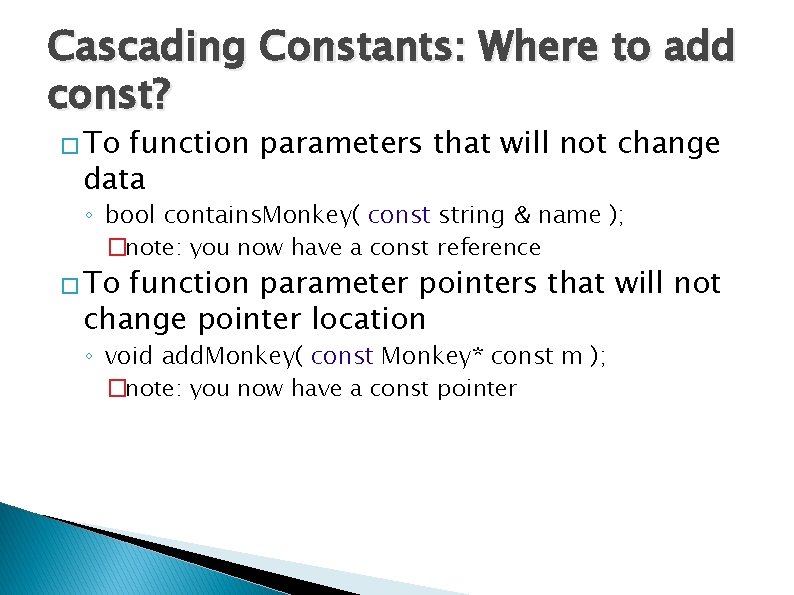
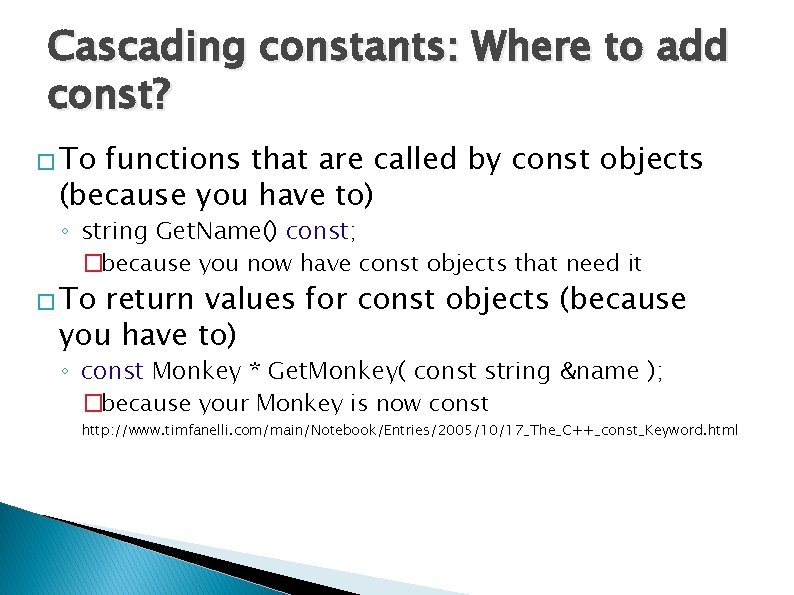
![Example class Person { public: Person(char* sz. New. Name) ~Person() { delete[] m_sz. Name; Example class Person { public: Person(char* sz. New. Name) ~Person() { delete[] m_sz. Name;](https://slidetodoc.com/presentation_image_h2/1457b0ba1a45376321ca1cea51dbc70d/image-11.jpg)
![Example class Person { public: Person(char* sz. New. Name) ~Person() { delete[] m_sz. Name; Example class Person { public: Person(char* sz. New. Name) ~Person() { delete[] m_sz. Name;](https://slidetodoc.com/presentation_image_h2/1457b0ba1a45376321ca1cea51dbc70d/image-12.jpg)
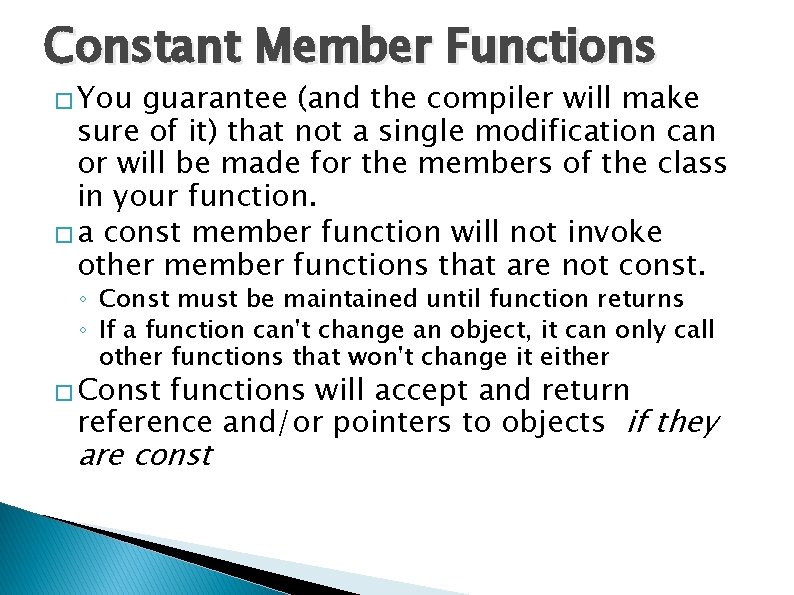
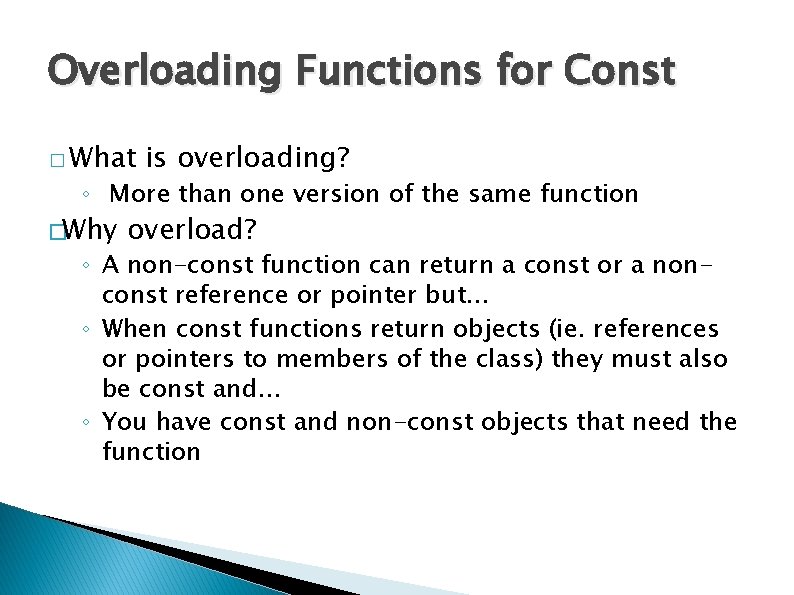
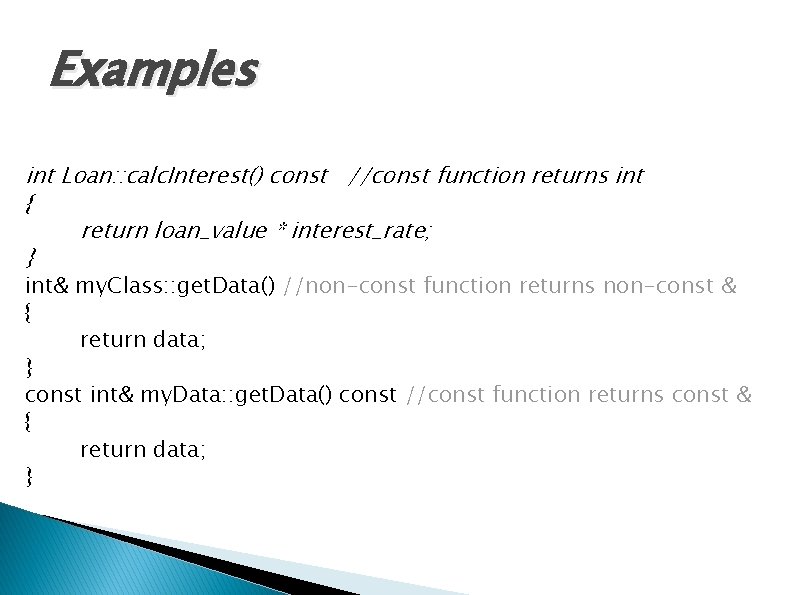
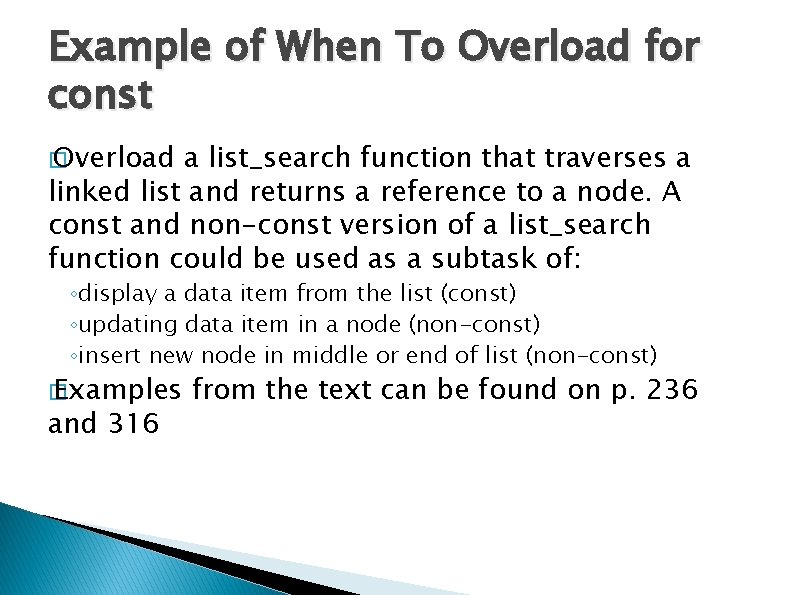
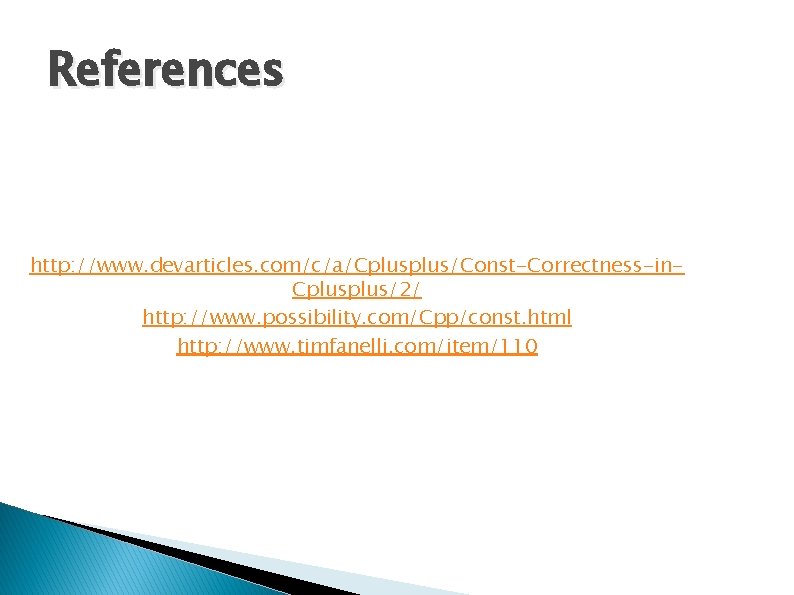
- Slides: 17
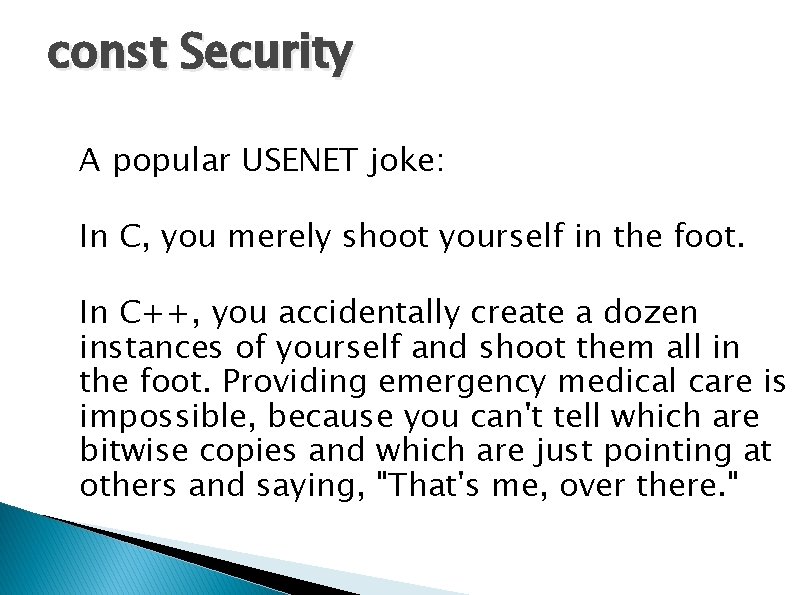
const Security A popular USENET joke: In C, you merely shoot yourself in the foot. In C++, you accidentally create a dozen instances of yourself and shoot them all in the foot. Providing emergency medical care is impossible, because you can't tell which are bitwise copies and which are just pointing at others and saying, "That's me, over there. "
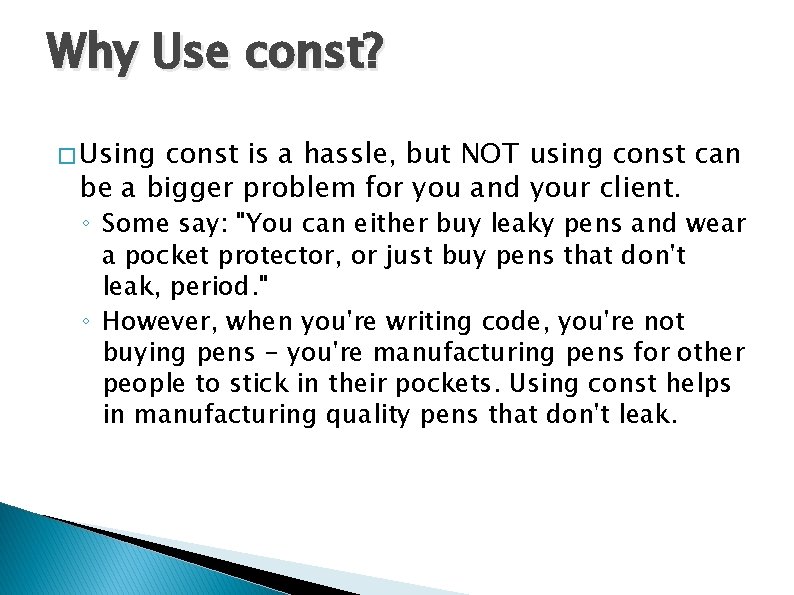
Why Use const? � Using const is a hassle, but NOT using const can be a bigger problem for you and your client. ◦ Some say: "You can either buy leaky pens and wear a pocket protector, or just buy pens that don't leak, period. " ◦ However, when you're writing code, you're not buying pens - you're manufacturing pens for other people to stick in their pockets. Using const helps in manufacturing quality pens that don't leak.
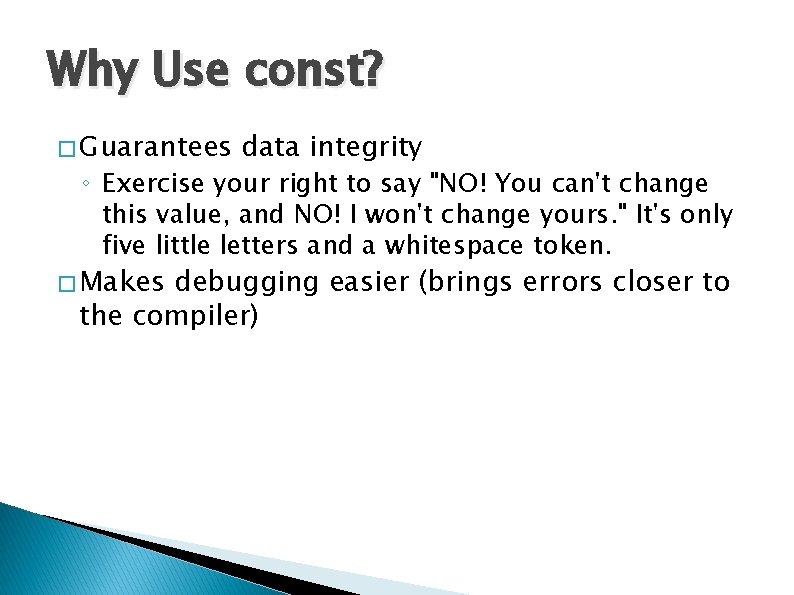
Why Use const? � Guarantees data integrity ◦ Exercise your right to say "NO! You can't change this value, and NO! I won't change yours. " It's only five little letters and a whitespace token. � Makes debugging easier (brings errors closer to the compiler)
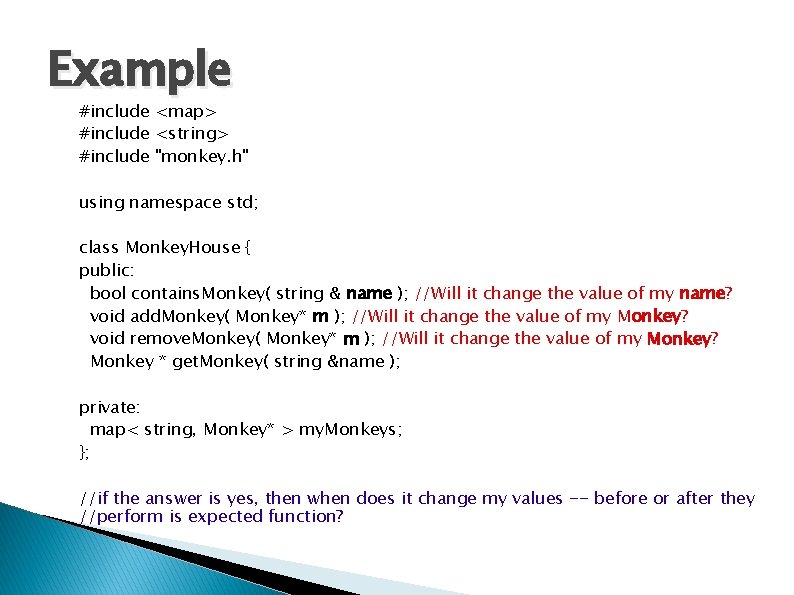
Example #include <map> #include <string> #include "monkey. h" using namespace std; class Monkey. House { public: bool contains. Monkey( string & name ); //Will it change the value of my name? void add. Monkey( Monkey* m ); //Will it change the value of my Monkey? void remove. Monkey( Monkey* m ); //Will it change the value of my Monkey? Monkey * get. Monkey( string &name ); private: map< string, Monkey* > my. Monkeys; }; //if the answer is yes, then when does it change my values -- before or after they //perform is expected function?
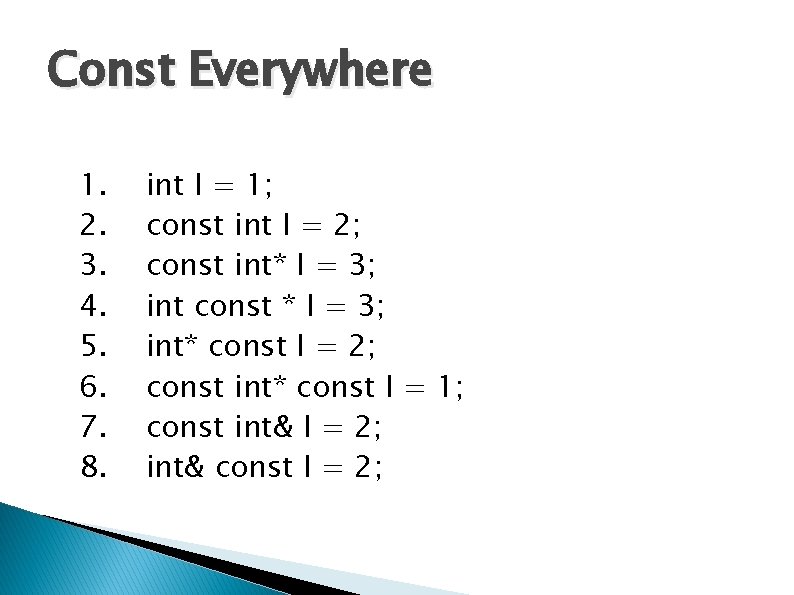
Const Everywhere 1. 2. 3. 4. 5. 6. 7. 8. int I = 1; const int I = 2; const int* I = 3; int const * I = 3; int* const I = 2; const int* const I = 1; const int& I = 2; int& const I = 2;
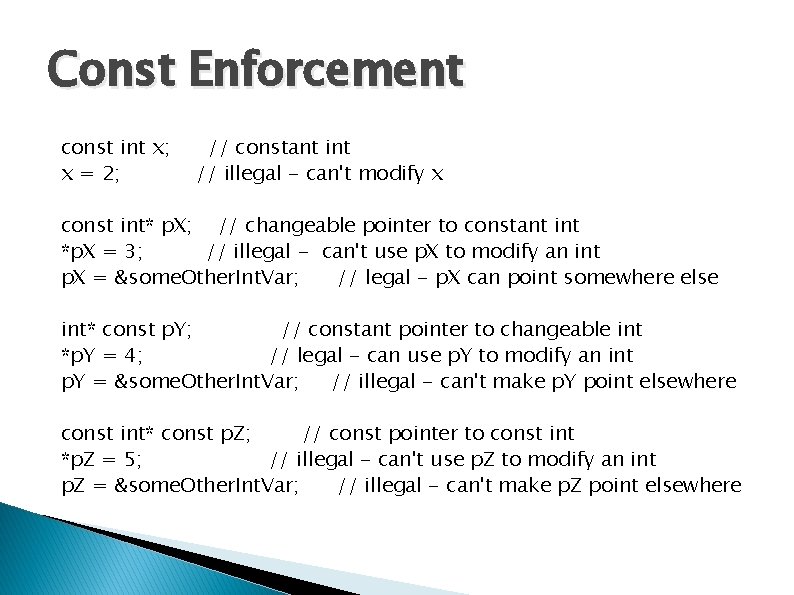
Const Enforcement const int x; x = 2; // constant int // illegal - can't modify x const int* p. X; // changeable pointer to constant int *p. X = 3; // illegal - can't use p. X to modify an int p. X = &some. Other. Int. Var; // legal - p. X can point somewhere else int* const p. Y; // constant pointer to changeable int *p. Y = 4; // legal - can use p. Y to modify an int p. Y = &some. Other. Int. Var; // illegal - can't make p. Y point elsewhere const int* const p. Z; // const pointer to const int *p. Z = 5; // illegal - can't use p. Z to modify an int p. Z = &some. Other. Int. Var; // illegal - can't make p. Z point elsewhere
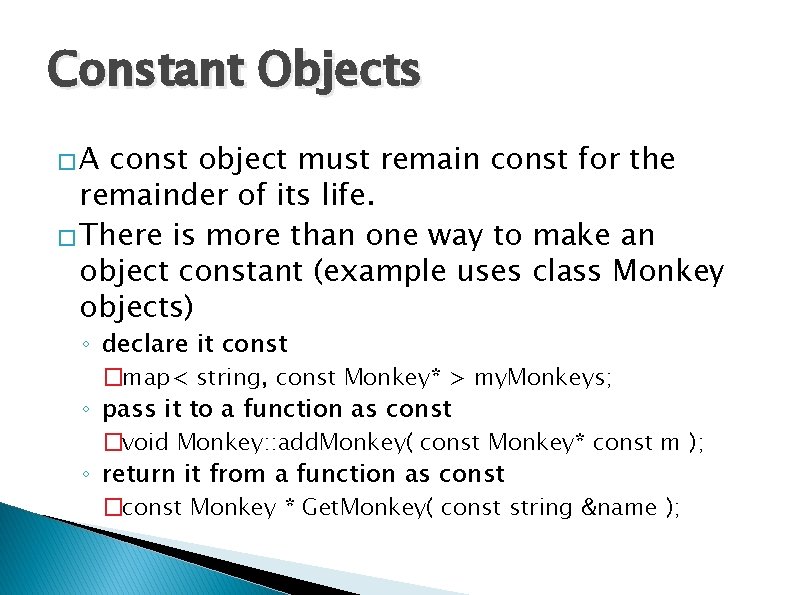
Constant Objects �A const object must remain const for the remainder of its life. � There is more than one way to make an object constant (example uses class Monkey objects) ◦ declare it const �map< string, const Monkey* > my. Monkeys; ◦ pass it to a function as const �void Monkey: : add. Monkey( const Monkey* const m ); ◦ return it from a function as const �const Monkey * Get. Monkey( const string &name );
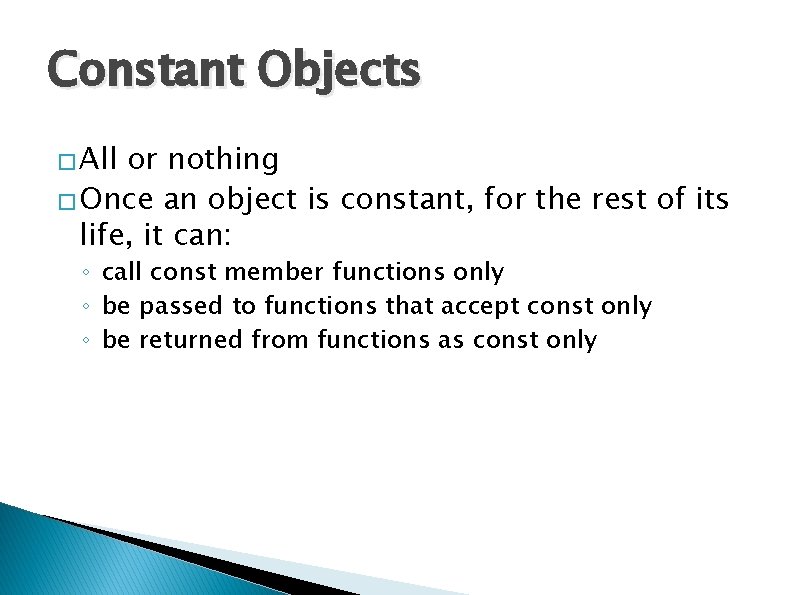
Constant Objects � All or nothing � Once an object is constant, for the rest of its life, it can: ◦ call const member functions only ◦ be passed to functions that accept const only ◦ be returned from functions as const only
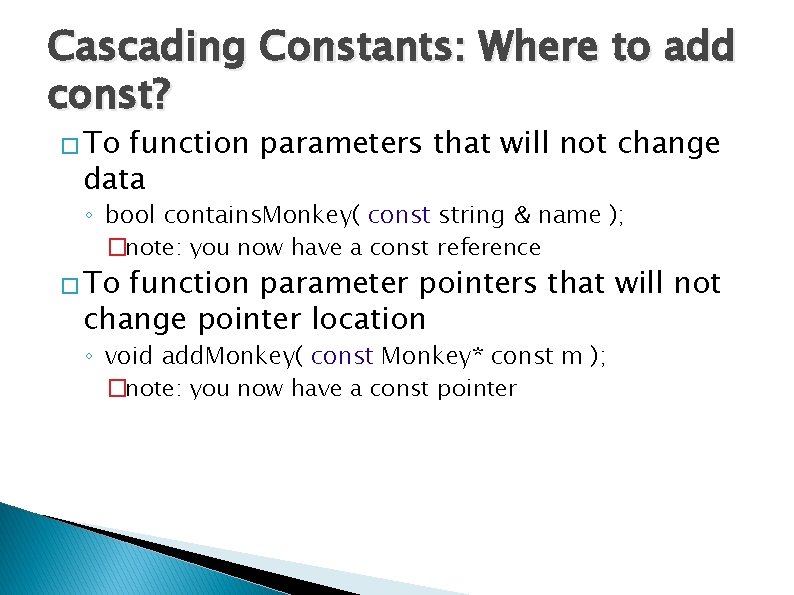
Cascading Constants: Where to add const? � To function parameters that will not change data ◦ bool contains. Monkey( const string & name ); �note: you now have a const reference � To function parameter pointers that will not change pointer location ◦ void add. Monkey( const Monkey* const m ); �note: you now have a const pointer
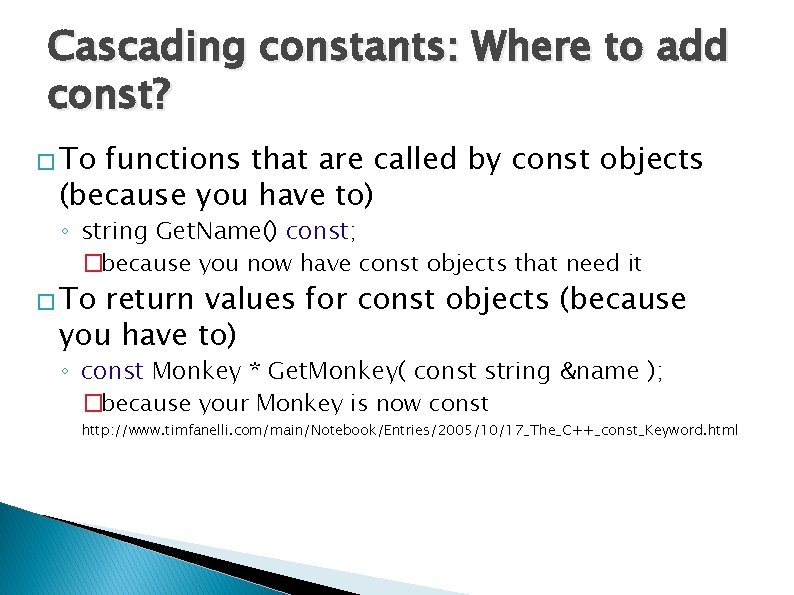
Cascading constants: Where to add const? � To functions that are called by const objects (because you have to) ◦ string Get. Name() const; �because you now have const objects that need it � To return values for const objects (because you have to) ◦ const Monkey * Get. Monkey( const string &name ); �because your Monkey is now const http: //www. timfanelli. com/main/Notebook/Entries/2005/10/17_The_C++_const_Keyword. html
![Example class Person public Personchar sz New Name Person delete msz Name Example class Person { public: Person(char* sz. New. Name) ~Person() { delete[] m_sz. Name;](https://slidetodoc.com/presentation_image_h2/1457b0ba1a45376321ca1cea51dbc70d/image-11.jpg)
Example class Person { public: Person(char* sz. New. Name) ~Person() { delete[] m_sz. Name; }; const char* const Get. Name() //return value is const, not the function { return m_sz. Name; }; private: char* m_sz. Name; }; //Non Member function implementation void Print. Person(const Person* const p. The. Person)//accepts a const object { // error - non-const member function called cout << p. The. Person->Get. Name() << endl; //const object's non-const member function }
![Example class Person public Personchar sz New Name Person delete msz Name Example class Person { public: Person(char* sz. New. Name) ~Person() { delete[] m_sz. Name;](https://slidetodoc.com/presentation_image_h2/1457b0ba1a45376321ca1cea51dbc70d/image-12.jpg)
Example class Person { public: Person(char* sz. New. Name) ~Person() { delete[] m_sz. Name; }; const char* const Get. Name() const }; private: char* m_sz. Name; //Non Member function implementation void Print. Person(const Person* const p. The. Person) //takes const object { // no error - const member function called cout << p. The. Person->Get. Name() << endl; //const object's const member function }
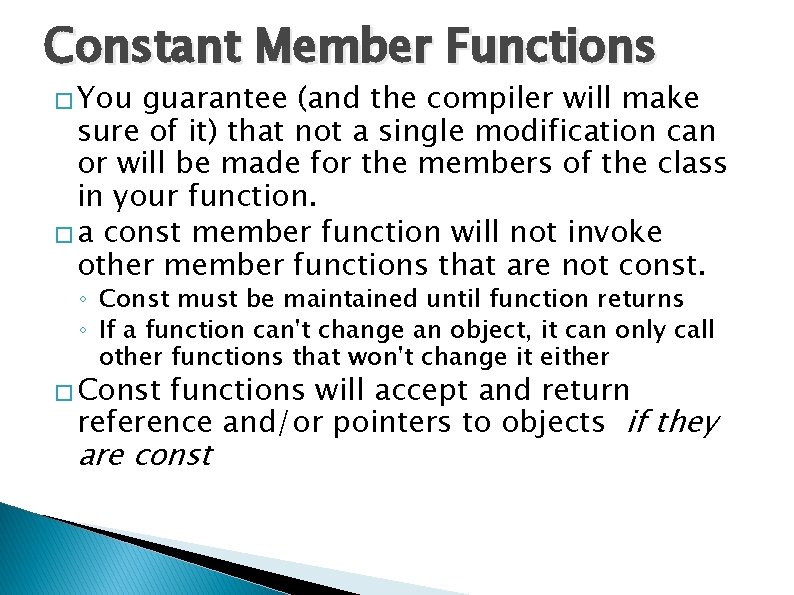
Constant Member Functions � You guarantee (and the compiler will make sure of it) that not a single modification can or will be made for the members of the class in your function. � a const member function will not invoke other member functions that are not const. ◦ Const must be maintained until function returns ◦ If a function can't change an object, it can only call other functions that won't change it either � Const functions will accept and return reference and/or pointers to objects if they are const
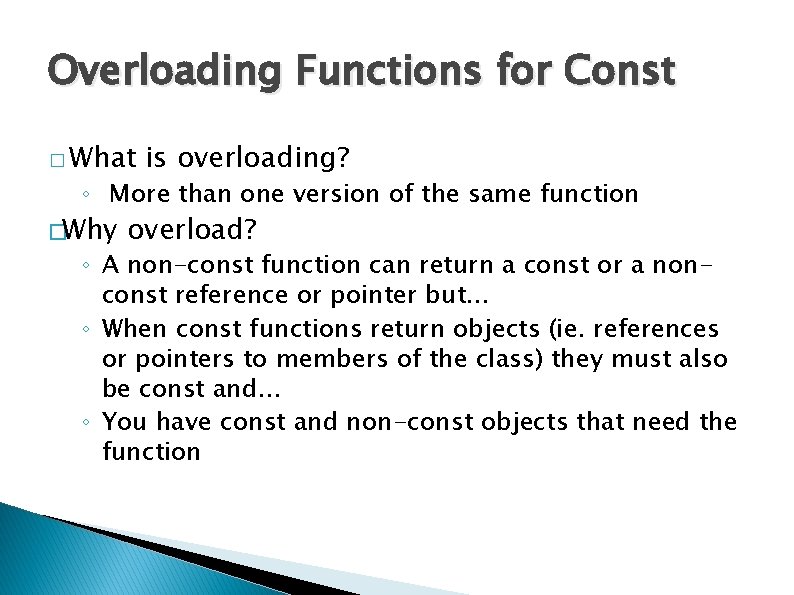
Overloading Functions for Const � What is overloading? ◦ More than one version of the same function �Why overload? ◦ A non-const function can return a const or a nonconst reference or pointer but. . . ◦ When const functions return objects (ie. references or pointers to members of the class) they must also be const and. . . ◦ You have const and non-const objects that need the function
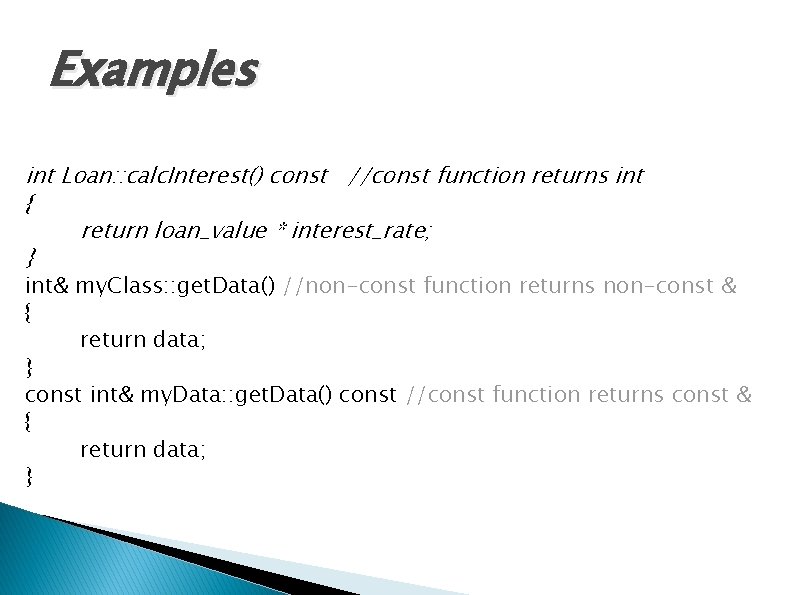
Examples int Loan: : calc. Interest() const //const function returns int { return loan_value * interest_rate; } int& my. Class: : get. Data() //non-const function returns non-const & { return data; } const int& my. Data: : get. Data() const //const function returns const & { return data; }
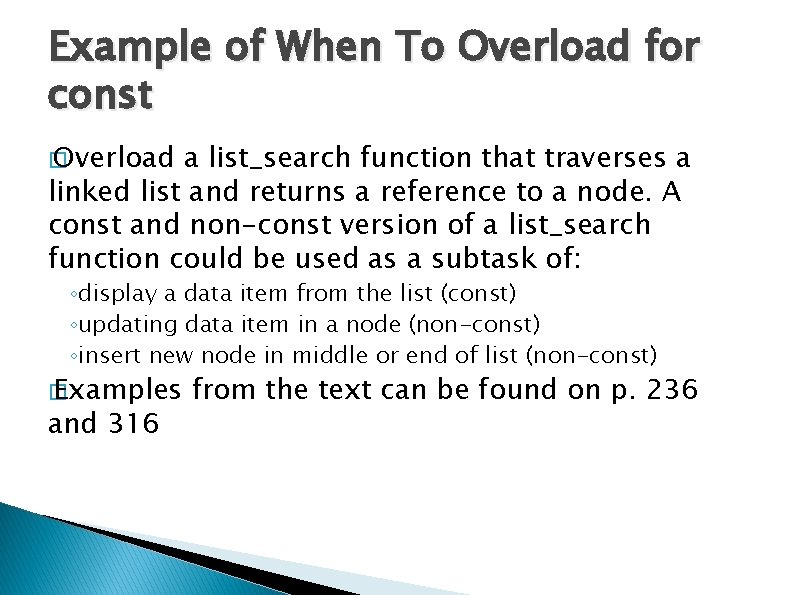
Example of When To Overload for const � Overload a list_search function that traverses a linked list and returns a reference to a node. A const and non-const version of a list_search function could be used as a subtask of: ◦display a data item from the list (const) ◦updating data item in a node (non-const) ◦insert new node in middle or end of list (non-const) � Examples and 316 from the text can be found on p. 236
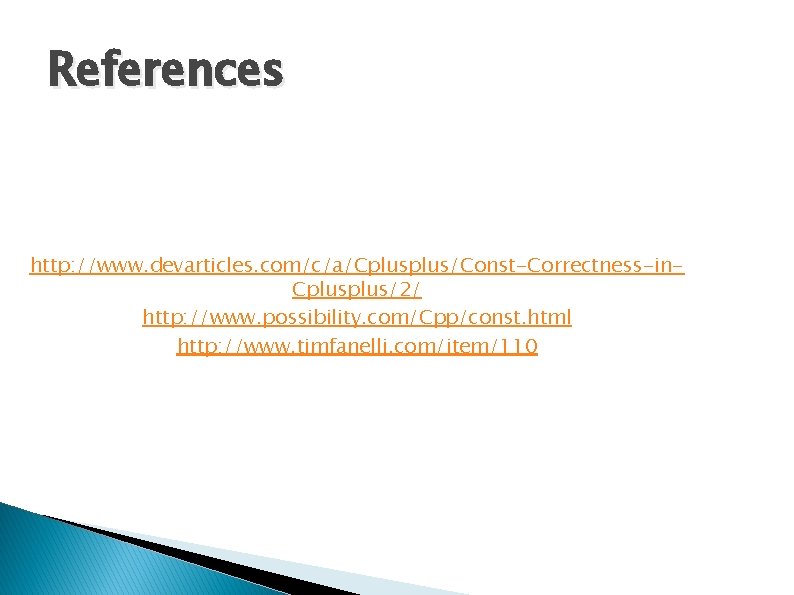
References http: //www. devarticles. com/c/a/Cplus/Const-Correctness-in. Cplus/2/ http: //www. possibility. com/Cpp/const. html http: //www. timfanelli. com/item/110