Concurrency Mutual Exclusion and Synchronization Chapter 5 Part
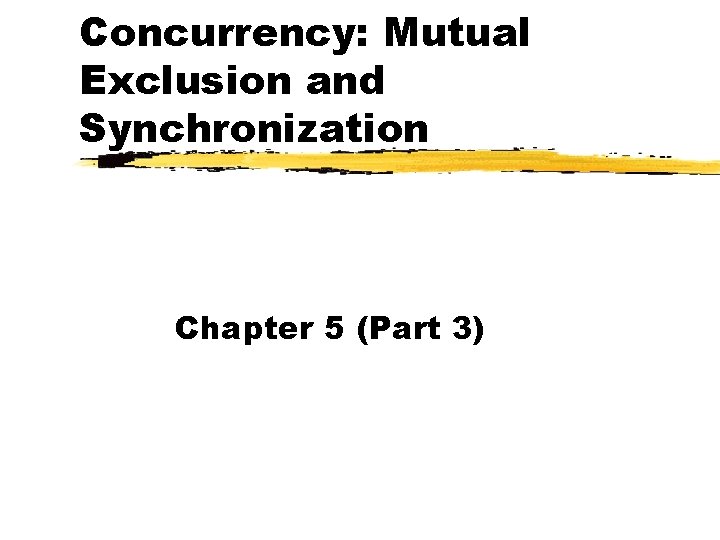
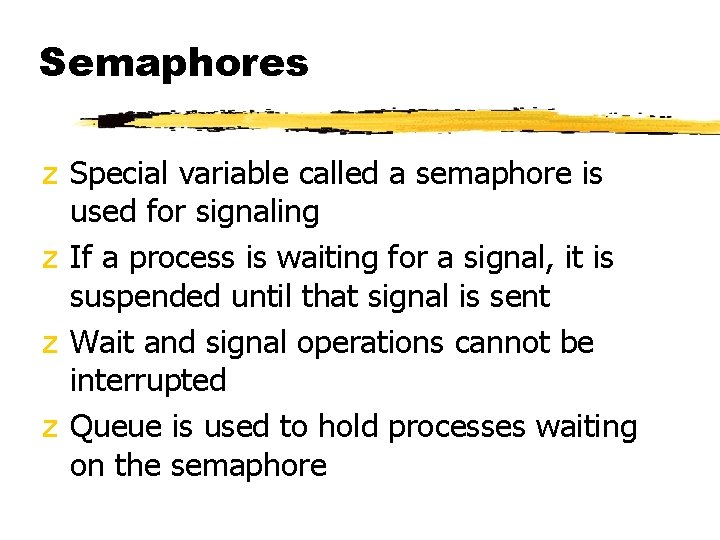
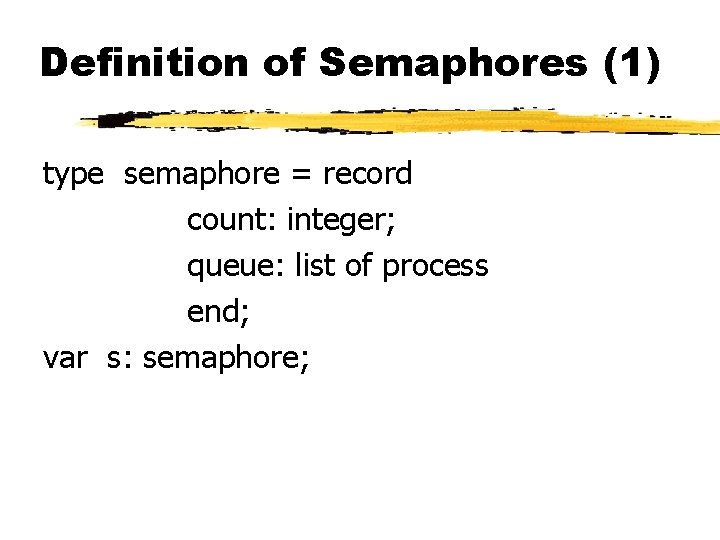
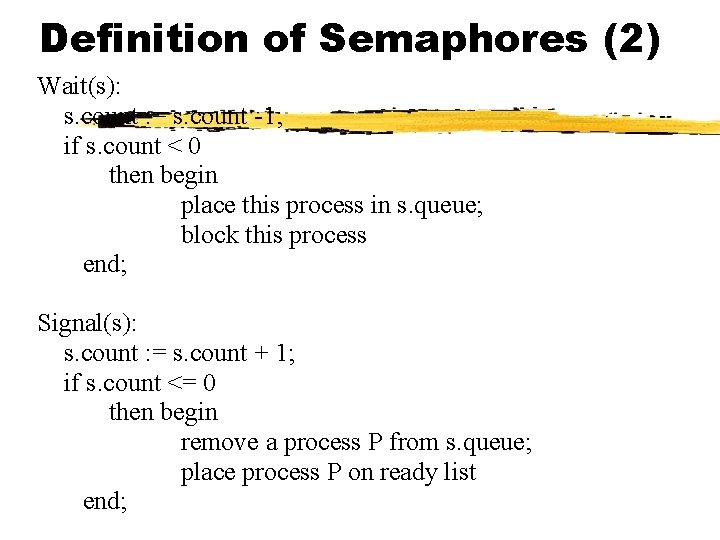
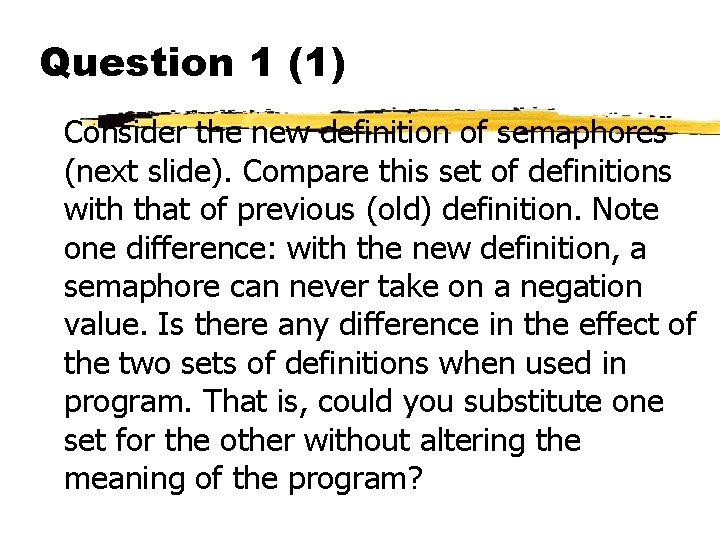
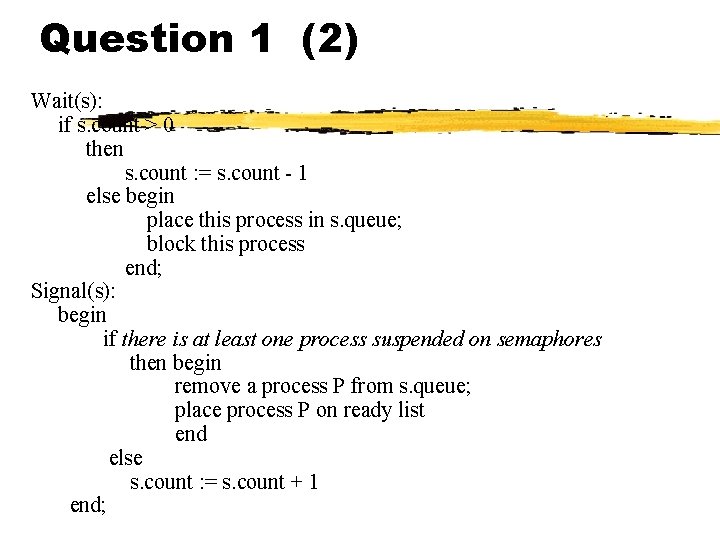
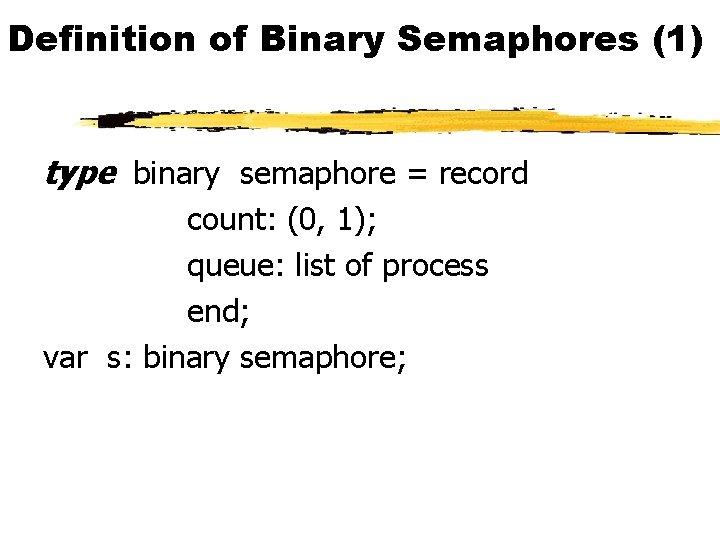
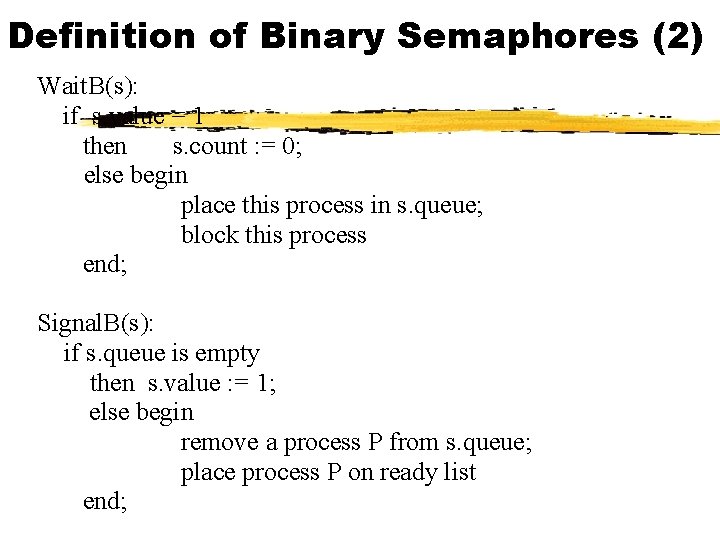
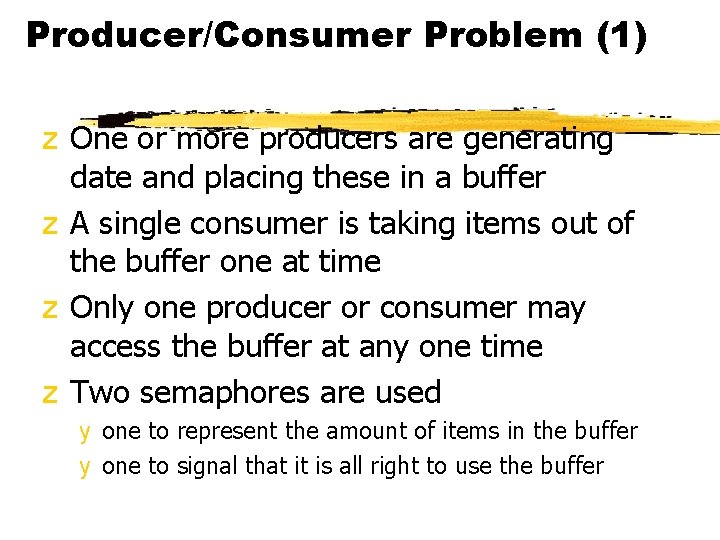
![Producer Function - Producer/Consumer Problem (2) producer: repeat produce item v; b[in] : = Producer Function - Producer/Consumer Problem (2) producer: repeat produce item v; b[in] : =](https://slidetodoc.com/presentation_image_h/c333faf5d760e5dbed2423dc0bfbe9d3/image-10.jpg)
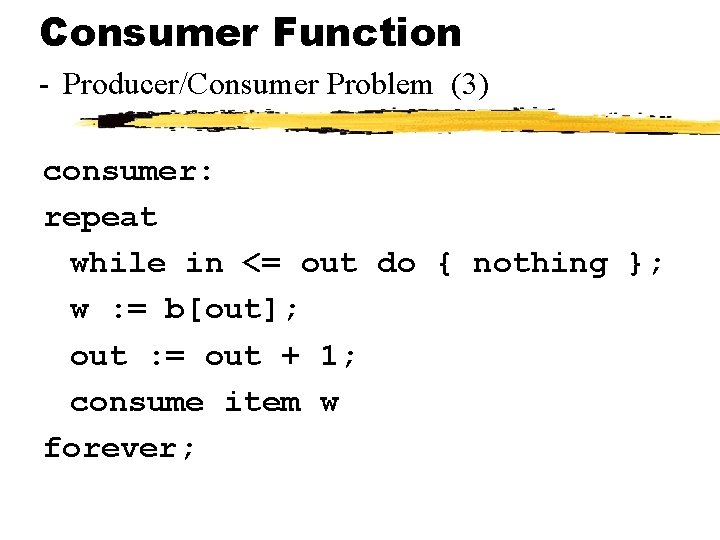
![Infinite Buffer for Producer/Consumer Problem - Producer/Consumer Problem (4) b[1] b[2] b[3] b[4] b[5] Infinite Buffer for Producer/Consumer Problem - Producer/Consumer Problem (4) b[1] b[2] b[3] b[4] b[5]](https://slidetodoc.com/presentation_image_h/c333faf5d760e5dbed2423dc0bfbe9d3/image-12.jpg)
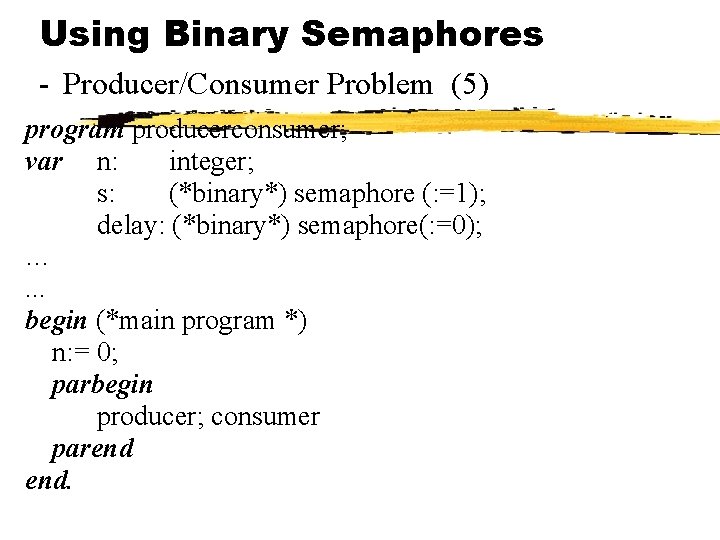
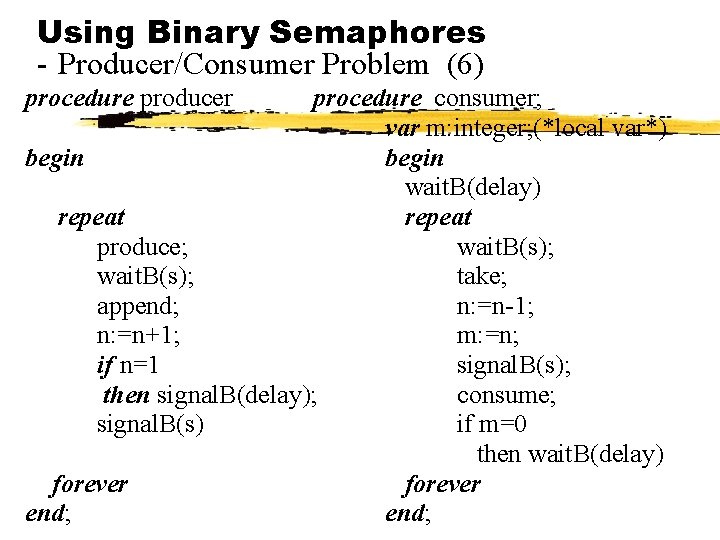
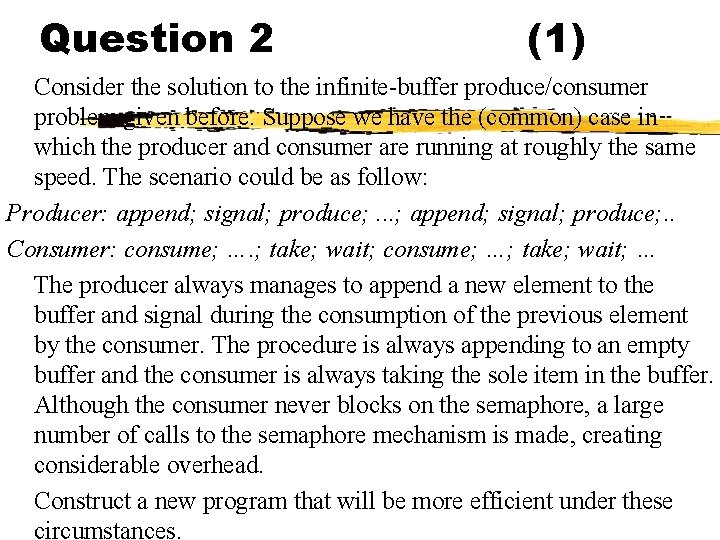
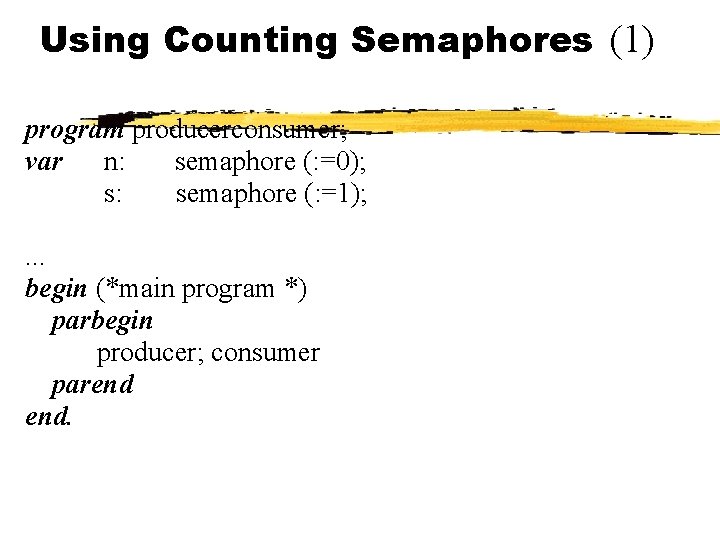
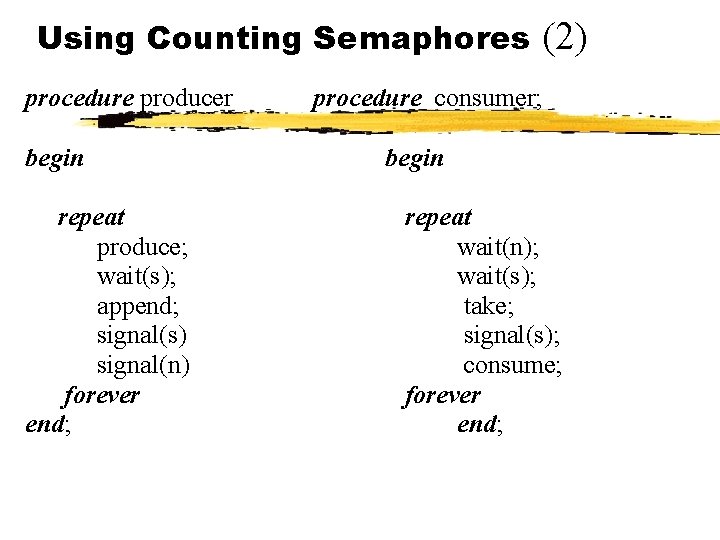
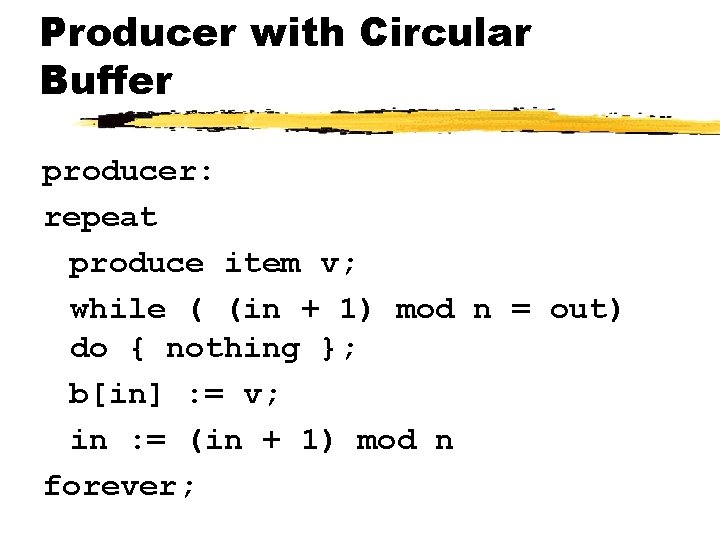
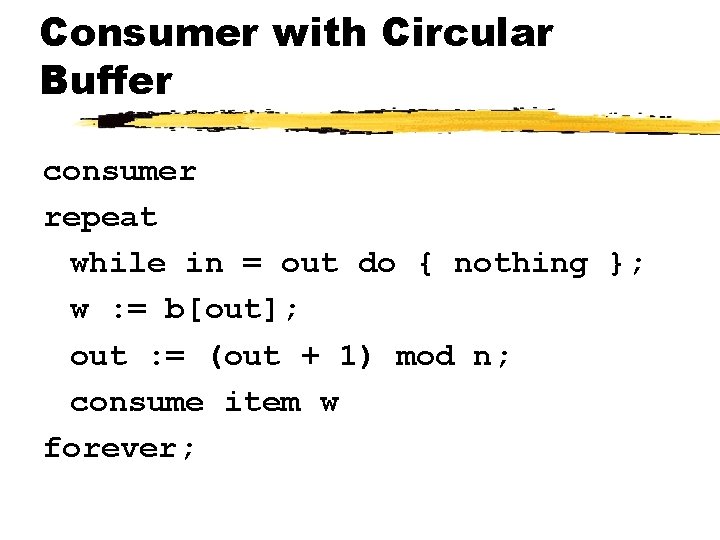
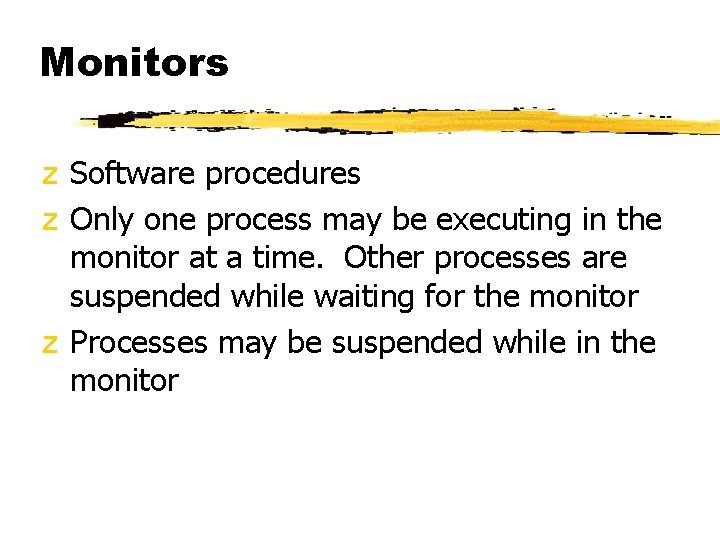
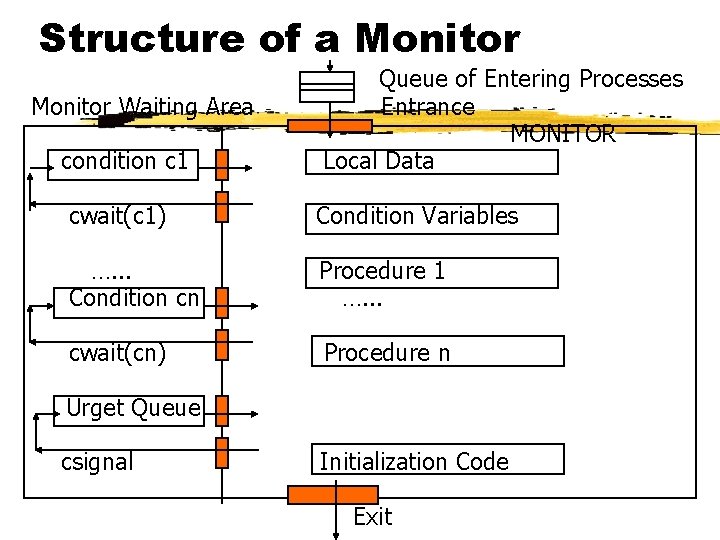
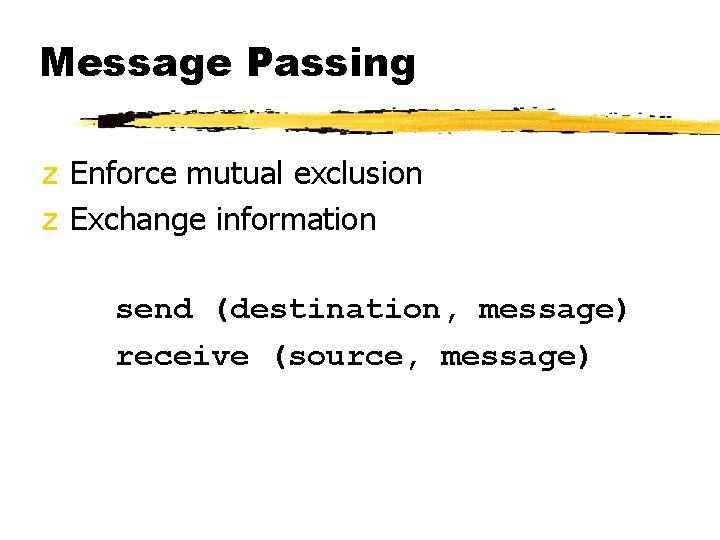
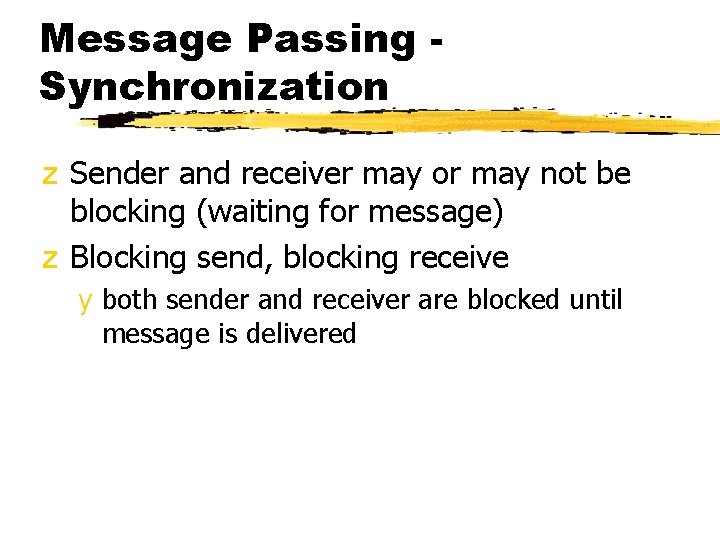
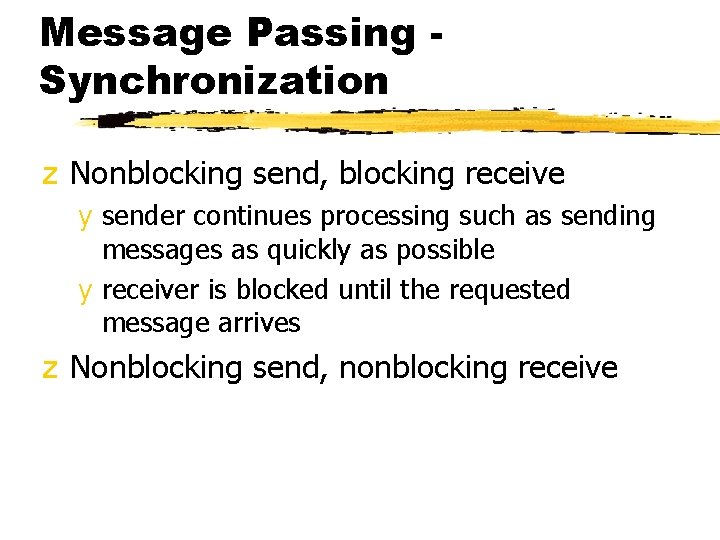
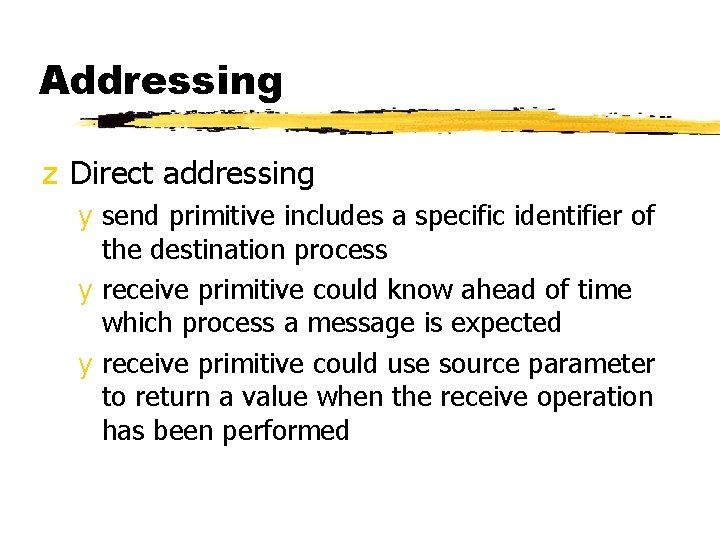
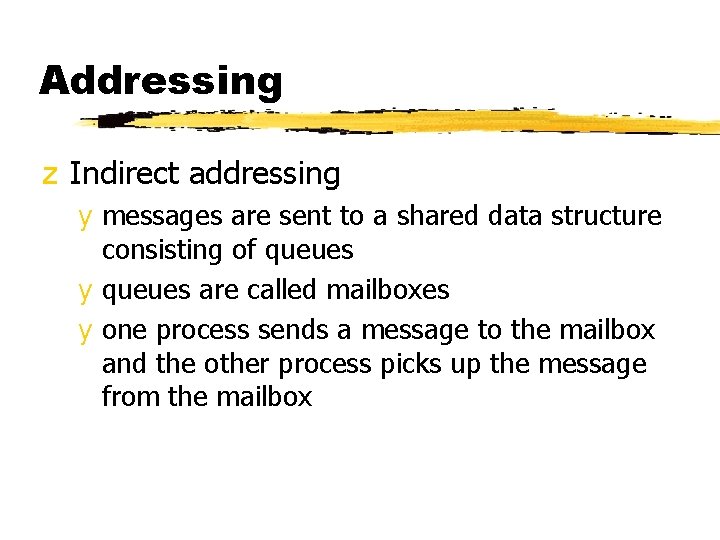
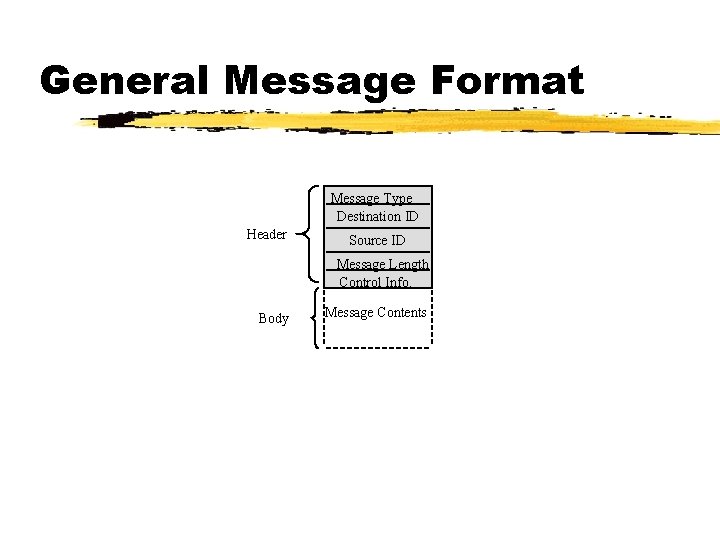
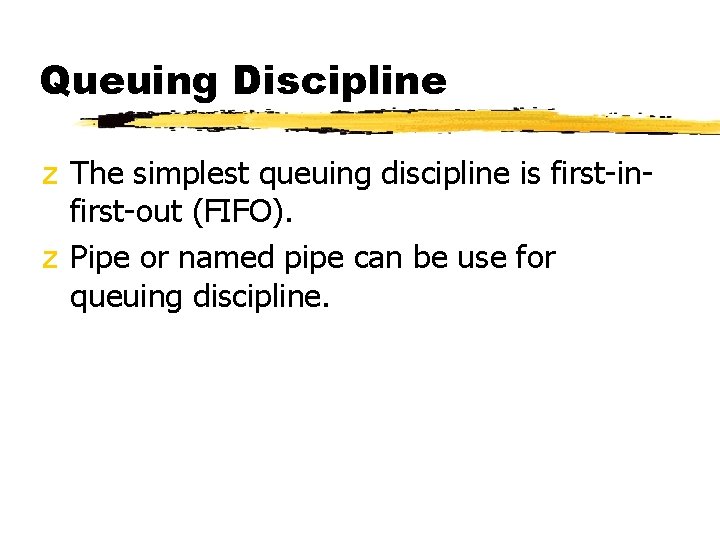
- Slides: 28
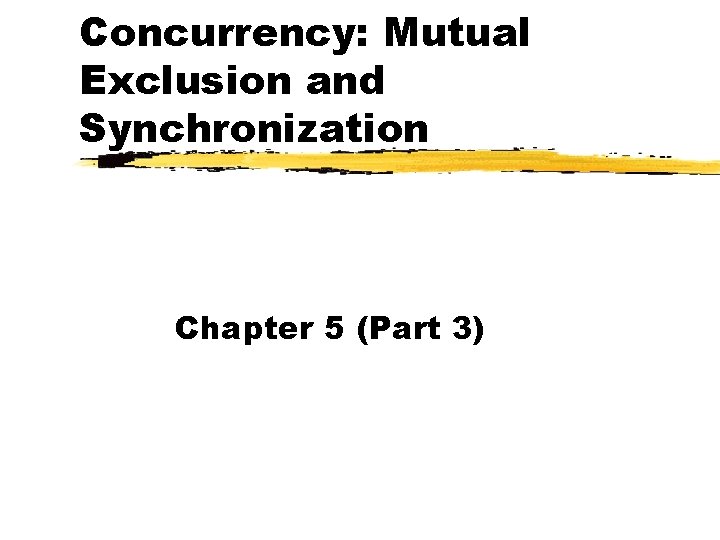
Concurrency: Mutual Exclusion and Synchronization Chapter 5 (Part 3)
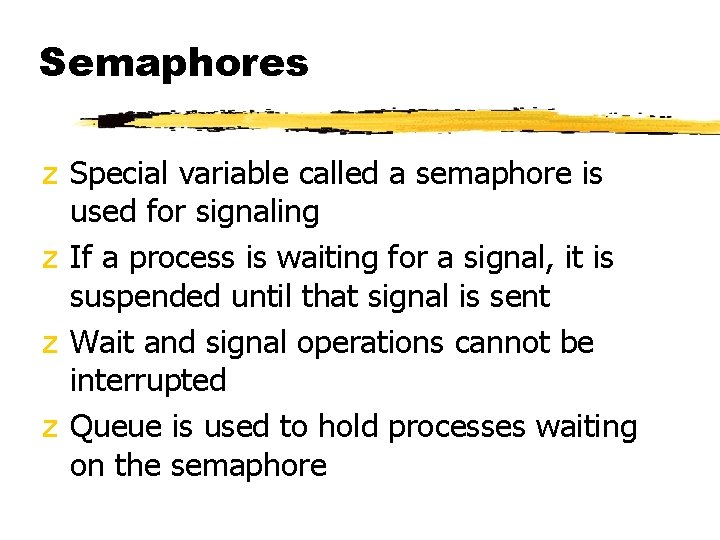
Semaphores z Special variable called a semaphore is used for signaling z If a process is waiting for a signal, it is suspended until that signal is sent z Wait and signal operations cannot be interrupted z Queue is used to hold processes waiting on the semaphore
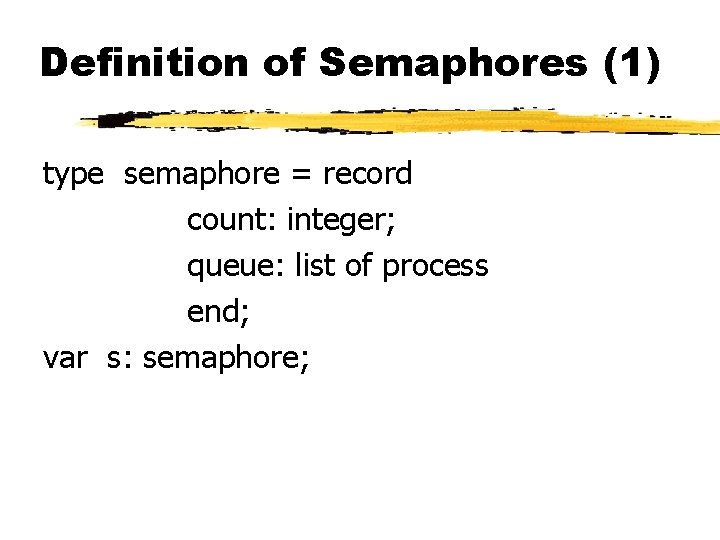
Definition of Semaphores (1) type semaphore = record count: integer; queue: list of process end; var s: semaphore;
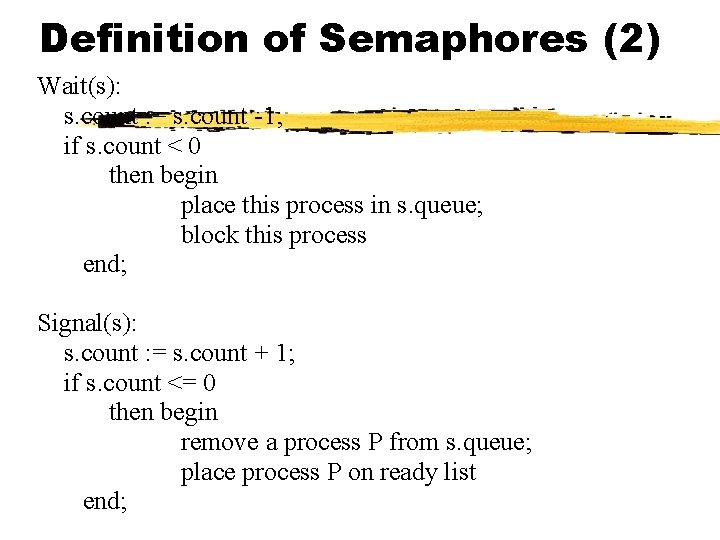
Definition of Semaphores (2) Wait(s): s. count : = s. count -1; if s. count < 0 then begin place this process in s. queue; block this process end; Signal(s): s. count : = s. count + 1; if s. count <= 0 then begin remove a process P from s. queue; place process P on ready list end;
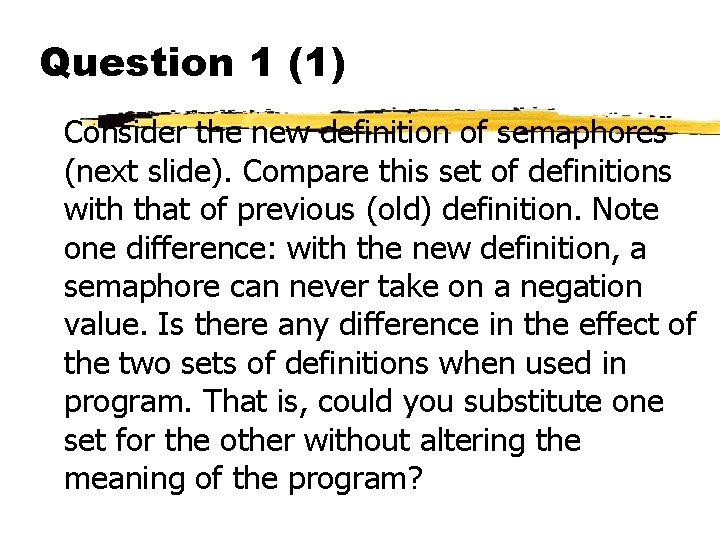
Question 1 (1) Consider the new definition of semaphores (next slide). Compare this set of definitions with that of previous (old) definition. Note one difference: with the new definition, a semaphore can never take on a negation value. Is there any difference in the effect of the two sets of definitions when used in program. That is, could you substitute one set for the other without altering the meaning of the program?
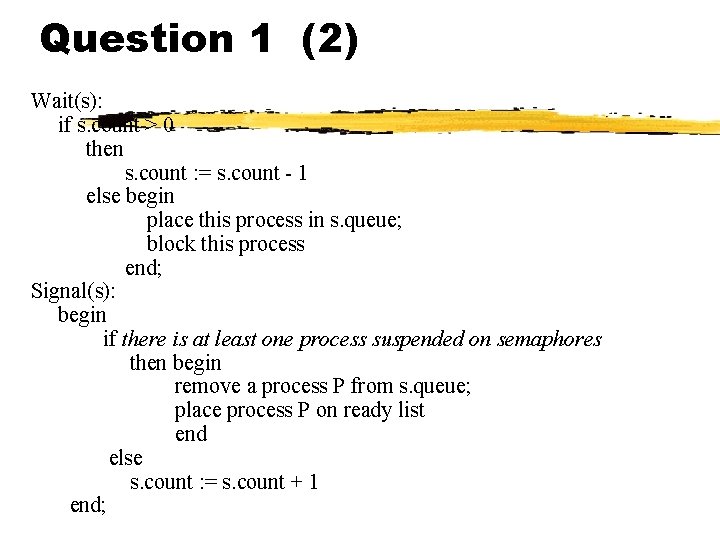
Question 1 (2) Wait(s): if s. count > 0 then s. count : = s. count - 1 else begin place this process in s. queue; block this process end; Signal(s): begin if there is at least one process suspended on semaphores then begin remove a process P from s. queue; place process P on ready list end else s. count : = s. count + 1 end;
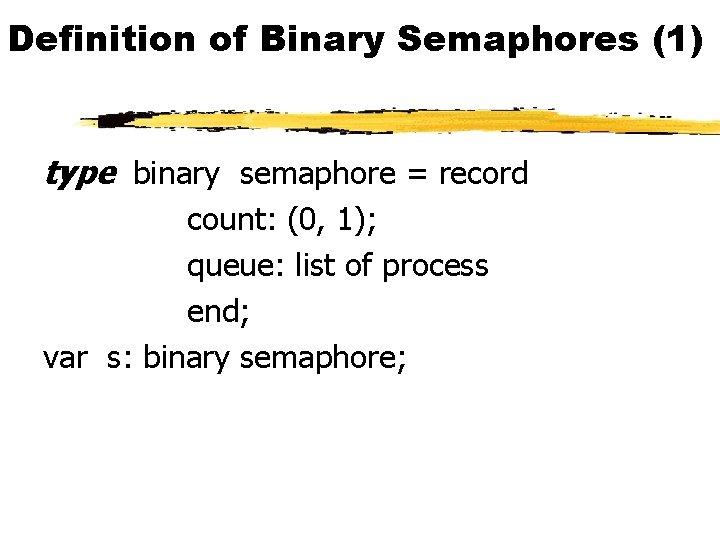
Definition of Binary Semaphores (1) type binary semaphore = record count: (0, 1); queue: list of process end; var s: binary semaphore;
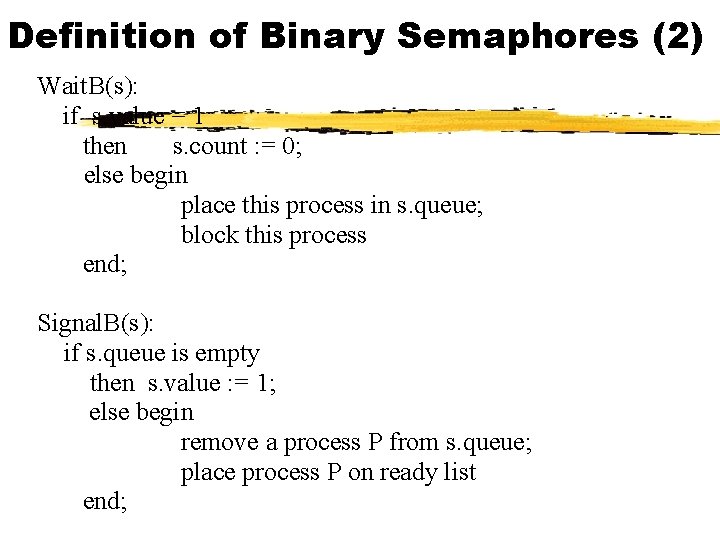
Definition of Binary Semaphores (2) Wait. B(s): if s. value = 1 then s. count : = 0; else begin place this process in s. queue; block this process end; Signal. B(s): if s. queue is empty then s. value : = 1; else begin remove a process P from s. queue; place process P on ready list end;
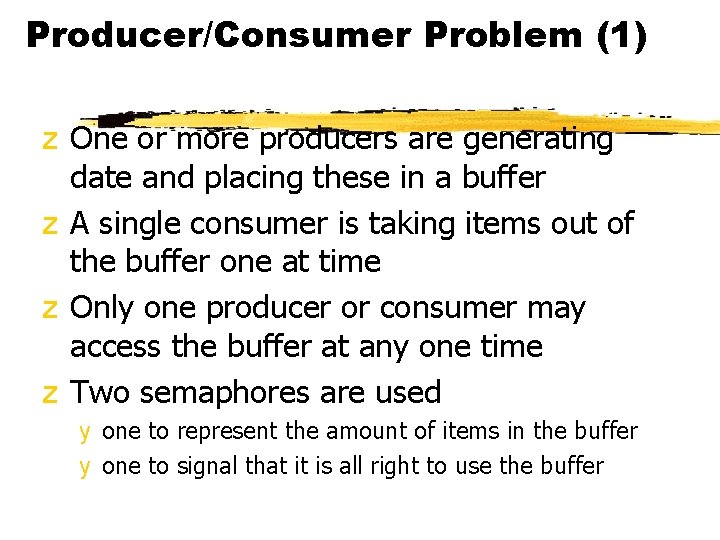
Producer/Consumer Problem (1) z One or more producers are generating date and placing these in a buffer z A single consumer is taking items out of the buffer one at time z Only one producer or consumer may access the buffer at any one time z Two semaphores are used y one to represent the amount of items in the buffer y one to signal that it is all right to use the buffer
![Producer Function ProducerConsumer Problem 2 producer repeat produce item v bin Producer Function - Producer/Consumer Problem (2) producer: repeat produce item v; b[in] : =](https://slidetodoc.com/presentation_image_h/c333faf5d760e5dbed2423dc0bfbe9d3/image-10.jpg)
Producer Function - Producer/Consumer Problem (2) producer: repeat produce item v; b[in] : = v; in : = in + 1 forever;
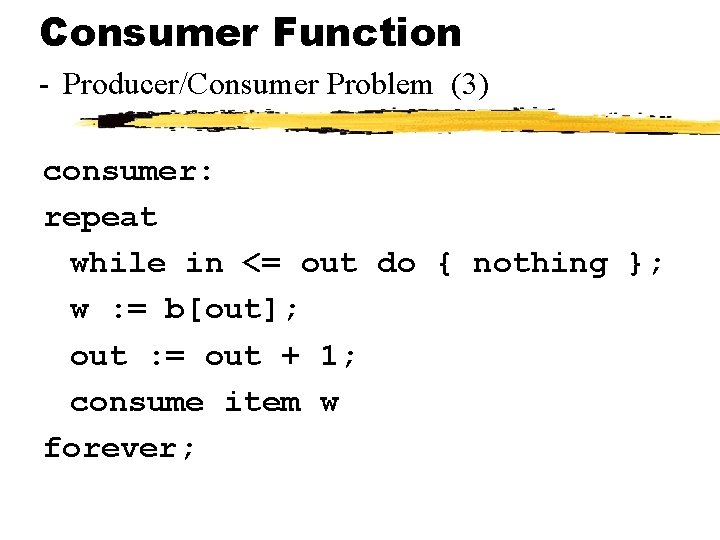
Consumer Function - Producer/Consumer Problem (3) consumer: repeat while in <= out do { nothing }; w : = b[out]; out : = out + 1; consume item w forever;
![Infinite Buffer for ProducerConsumer Problem ProducerConsumer Problem 4 b1 b2 b3 b4 b5 Infinite Buffer for Producer/Consumer Problem - Producer/Consumer Problem (4) b[1] b[2] b[3] b[4] b[5]](https://slidetodoc.com/presentation_image_h/c333faf5d760e5dbed2423dc0bfbe9d3/image-12.jpg)
Infinite Buffer for Producer/Consumer Problem - Producer/Consumer Problem (4) b[1] b[2] b[3] b[4] b[5] out . . in Note: shade area indicates portion of buffer that is occupied
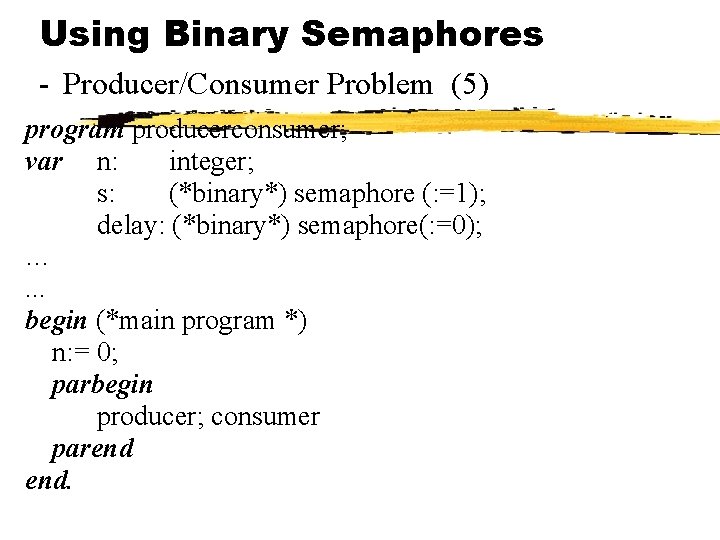
Using Binary Semaphores - Producer/Consumer Problem (5) program producerconsumer; var n: integer; s: (*binary*) semaphore (: =1); delay: (*binary*) semaphore(: =0); …. . . begin (*main program *) n: = 0; parbegin producer; consumer parend end.
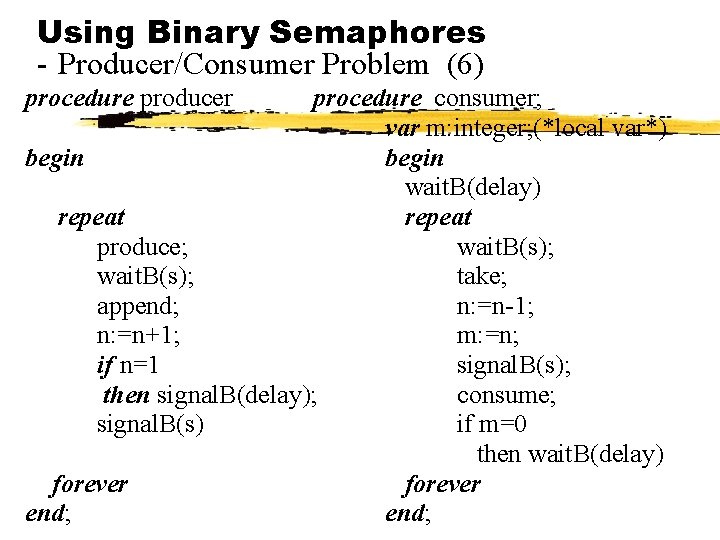
Using Binary Semaphores - Producer/Consumer Problem (6) procedure producer procedure consumer; var m: integer; (*local var*) begin wait. B(delay) repeat produce; wait. B(s); take; append; n: =n-1; n: =n+1; m: =n; if n=1 signal. B(s); then signal. B(delay); consume; signal. B(s) if m=0 then wait. B(delay) forever end;
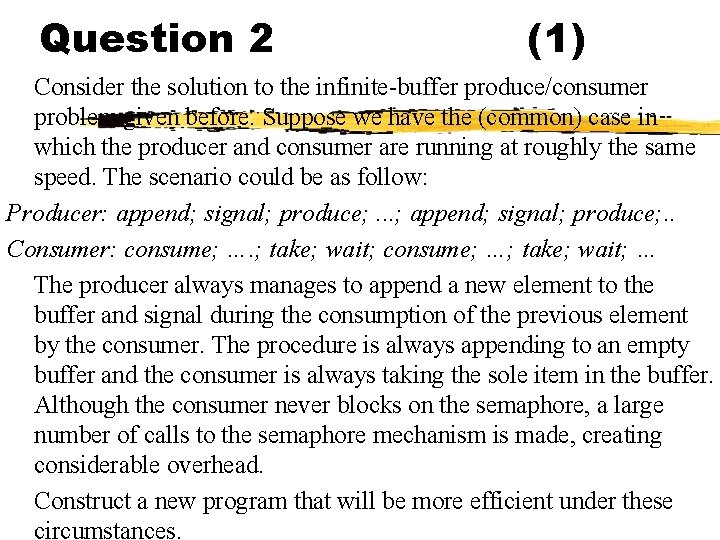
Question 2 (1) Consider the solution to the infinite-buffer produce/consumer problem given before. Suppose we have the (common) case in which the producer and consumer are running at roughly the same speed. The scenario could be as follow: Producer: append; signal; produce; . . . ; append; signal; produce; . . Consumer: consume; …. ; take; wait; consume; …; take; wait; … The producer always manages to append a new element to the buffer and signal during the consumption of the previous element by the consumer. The procedure is always appending to an empty buffer and the consumer is always taking the sole item in the buffer. Although the consumer never blocks on the semaphore, a large number of calls to the semaphore mechanism is made, creating considerable overhead. Construct a new program that will be more efficient under these circumstances.
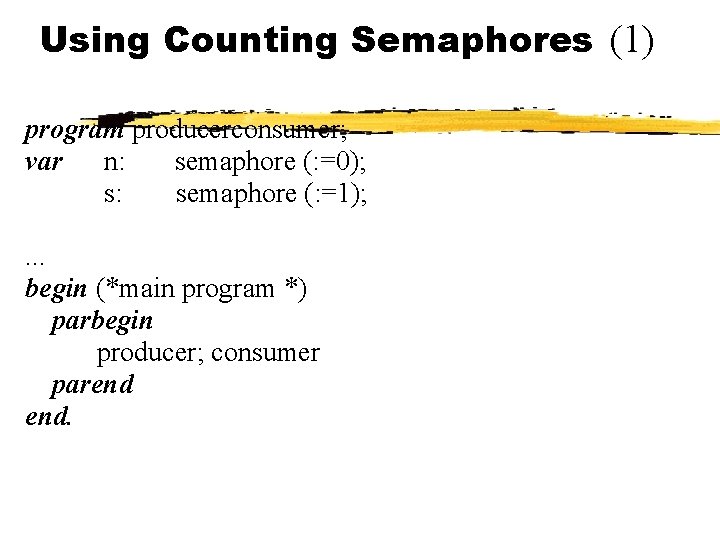
Using Counting Semaphores (1) program producerconsumer; var n: semaphore (: =0); s: semaphore (: =1); . . . begin (*main program *) parbegin producer; consumer parend end.
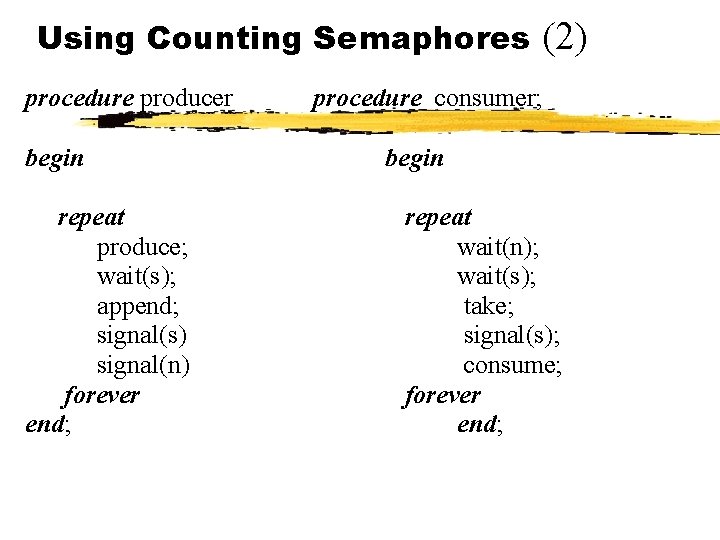
Using Counting Semaphores procedure producer begin repeat produce; wait(s); append; signal(s) signal(n) forever end; (2) procedure consumer; begin repeat wait(n); wait(s); take; signal(s); consume; forever end;
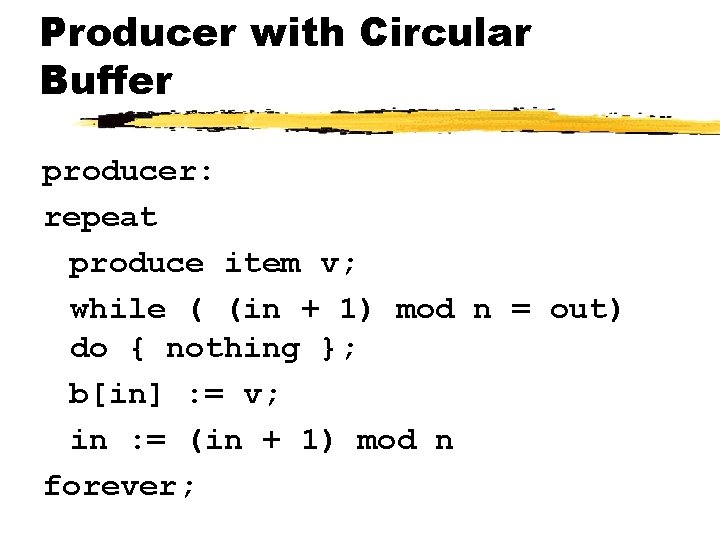
Producer with Circular Buffer producer: repeat produce item v; while ( (in + 1) mod n = out) do { nothing }; b[in] : = v; in : = (in + 1) mod n forever;
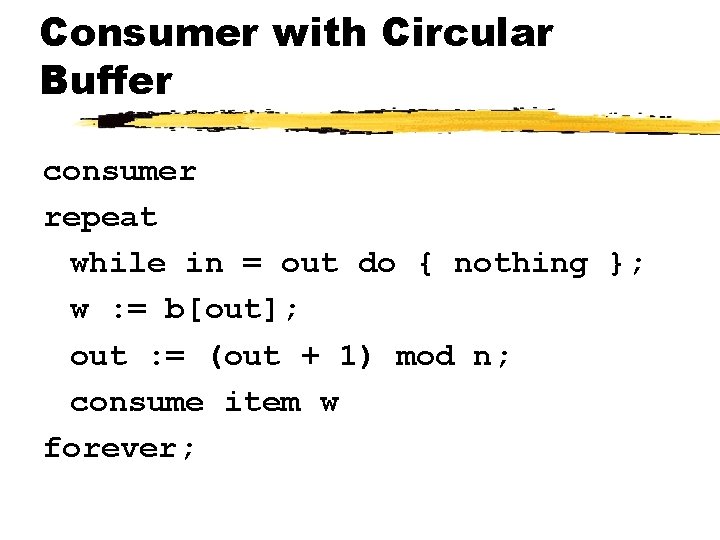
Consumer with Circular Buffer consumer repeat while in = out do { nothing }; w : = b[out]; out : = (out + 1) mod n; consume item w forever;
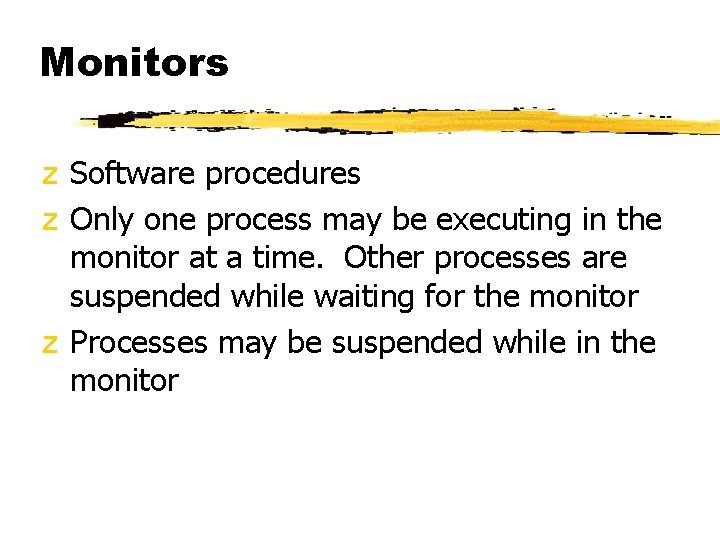
Monitors z Software procedures z Only one process may be executing in the monitor at a time. Other processes are suspended while waiting for the monitor z Processes may be suspended while in the monitor
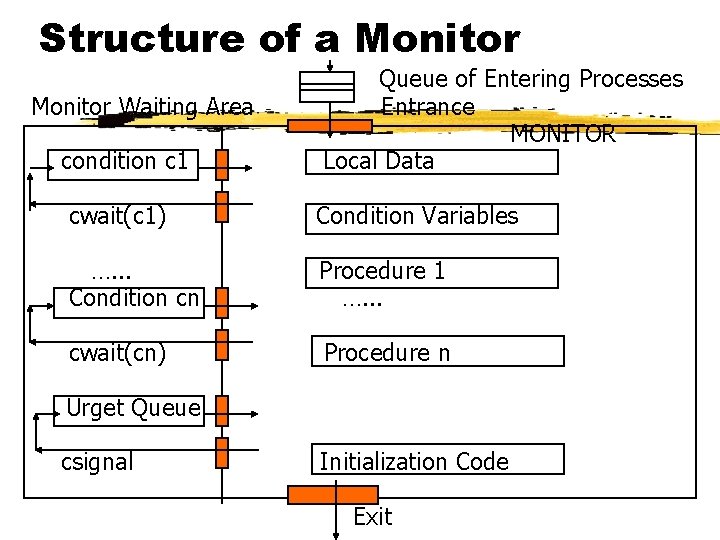
Structure of a Monitor Waiting Area condition c 1 Queue of Entering Processes Entrance MONITOR Local Data cwait(c 1) Condition Variables …. . . Condition cn Procedure 1 …. . . cwait(cn) Procedure n Urget Queue csignal Initialization Code Exit
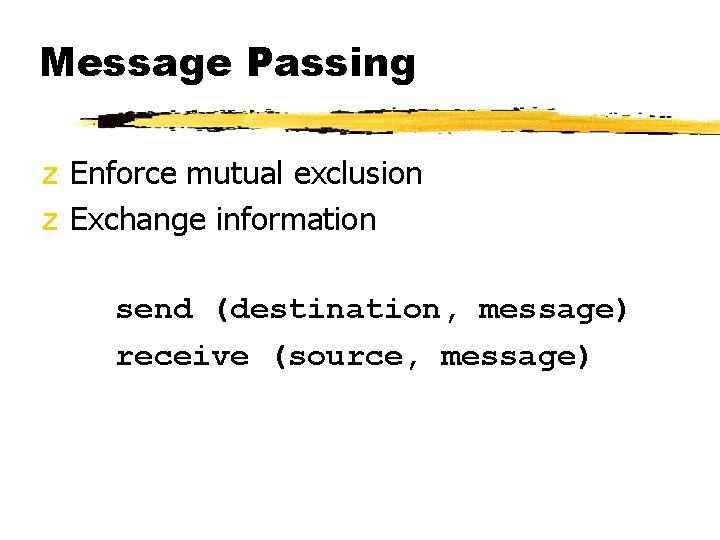
Message Passing z Enforce mutual exclusion z Exchange information send (destination, message) receive (source, message)
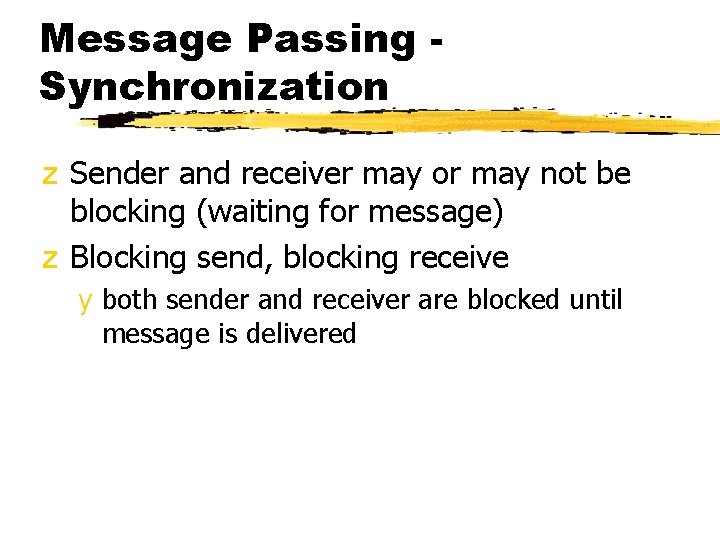
Message Passing Synchronization z Sender and receiver may or may not be blocking (waiting for message) z Blocking send, blocking receive y both sender and receiver are blocked until message is delivered
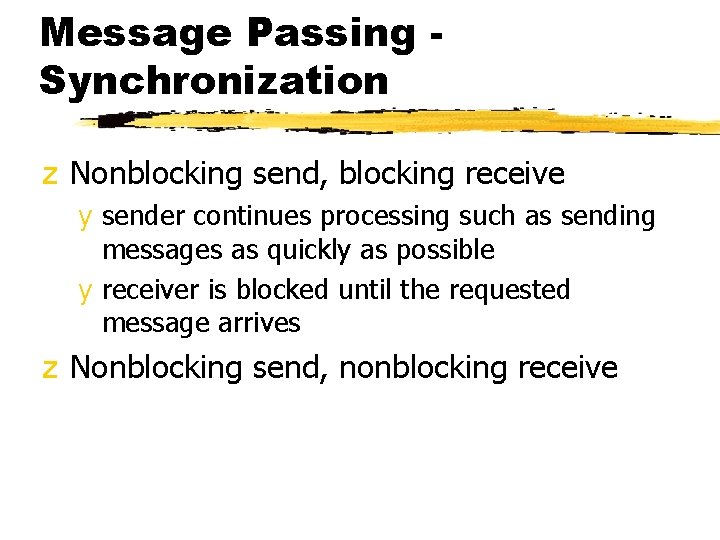
Message Passing Synchronization z Nonblocking send, blocking receive y sender continues processing such as sending messages as quickly as possible y receiver is blocked until the requested message arrives z Nonblocking send, nonblocking receive
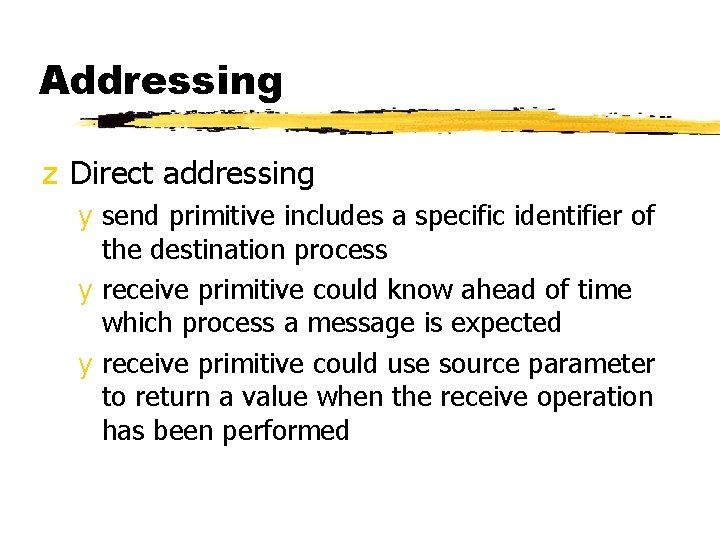
Addressing z Direct addressing y send primitive includes a specific identifier of the destination process y receive primitive could know ahead of time which process a message is expected y receive primitive could use source parameter to return a value when the receive operation has been performed
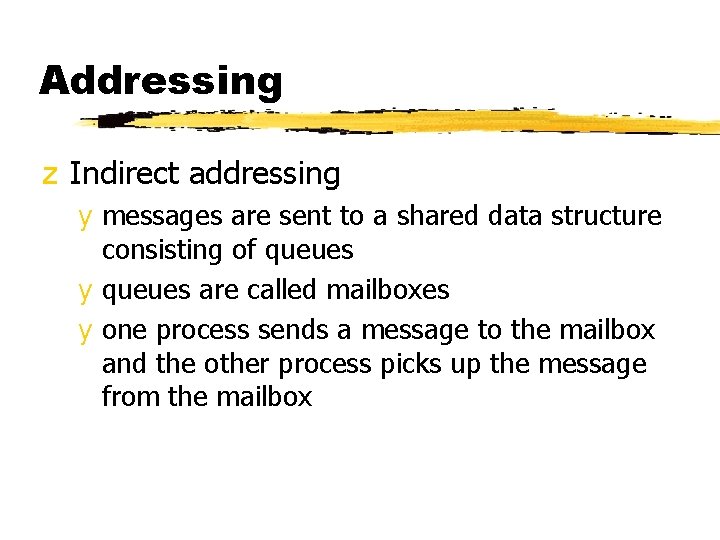
Addressing z Indirect addressing y messages are sent to a shared data structure consisting of queues y queues are called mailboxes y one process sends a message to the mailbox and the other process picks up the message from the mailbox
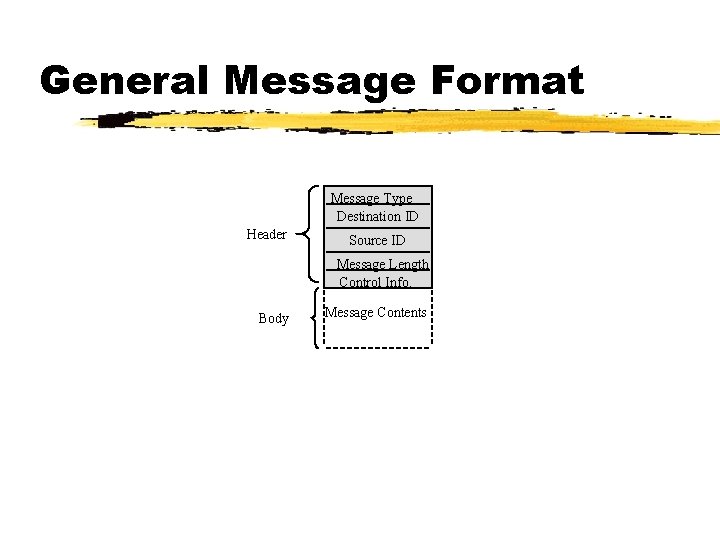
General Message Format Message Type Destination ID Header Source ID Message Length Control Info. Body Message Contents
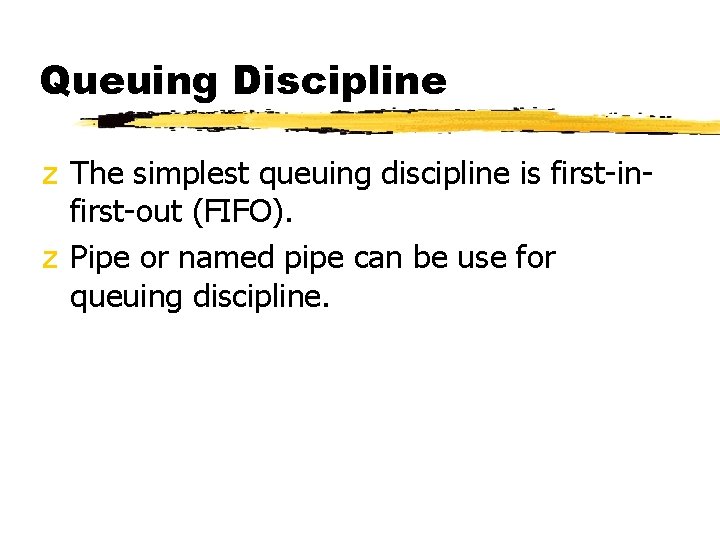
Queuing Discipline z The simplest queuing discipline is first-infirst-out (FIFO). z Pipe or named pipe can be use for queuing discipline.