Computer Architecture A Constructive Approach Implementing SMIPS Arvind
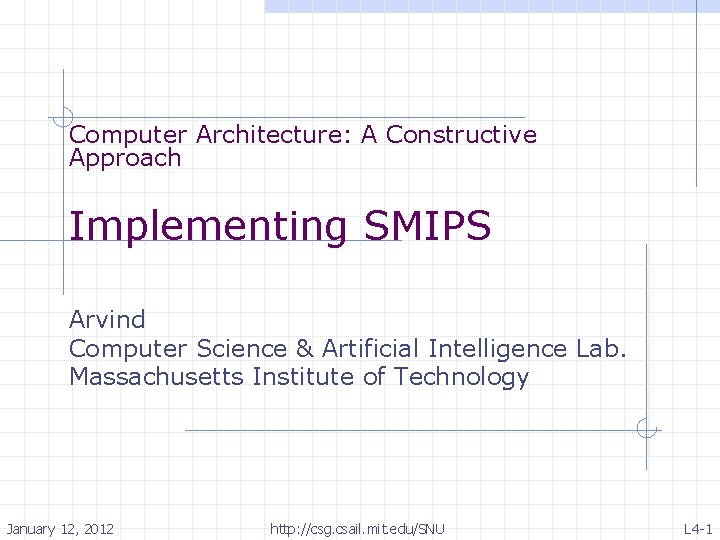
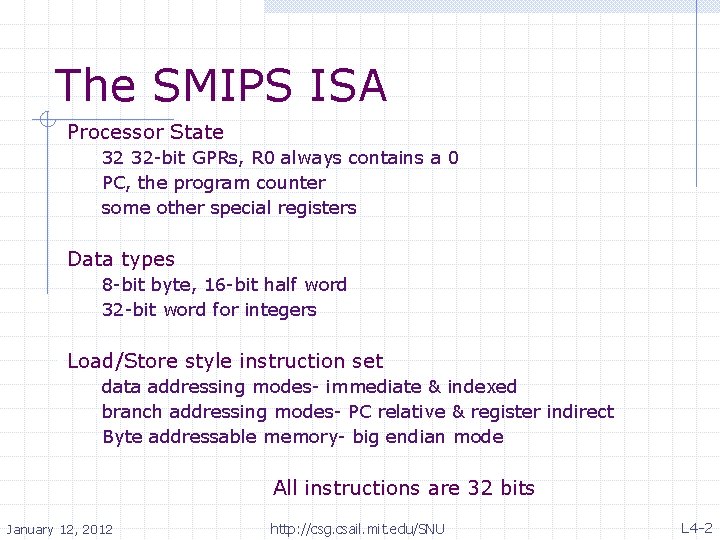
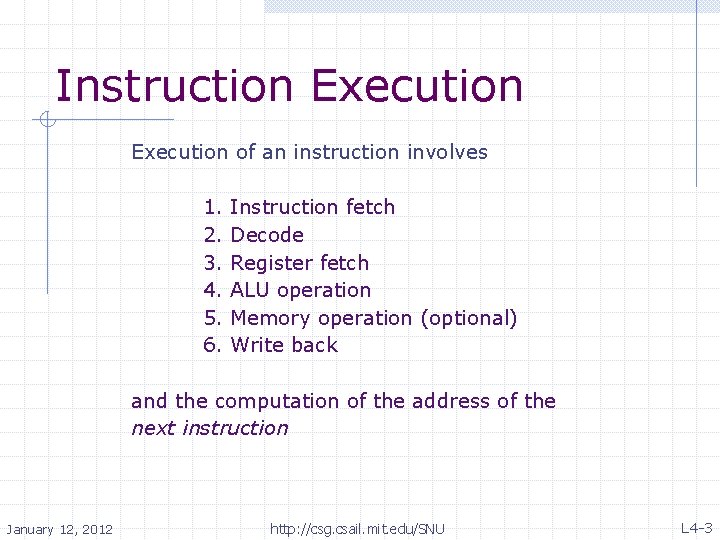
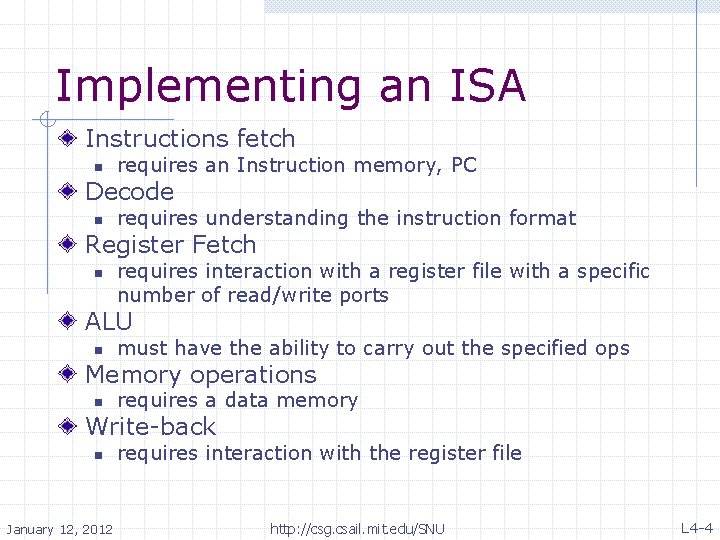
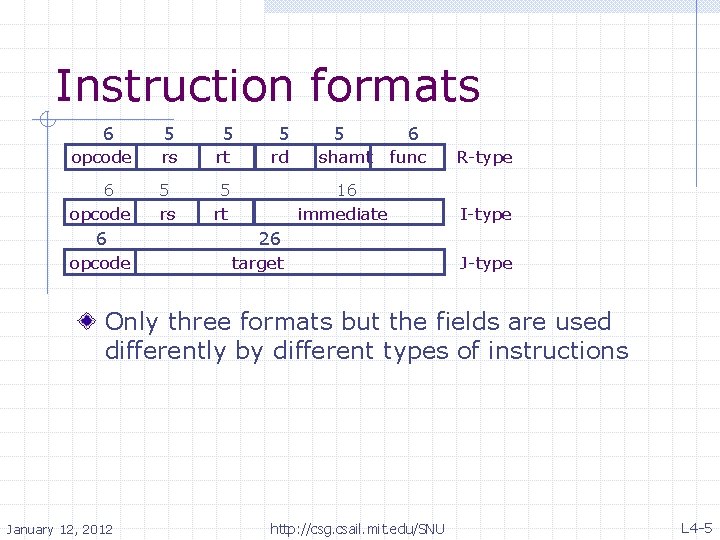
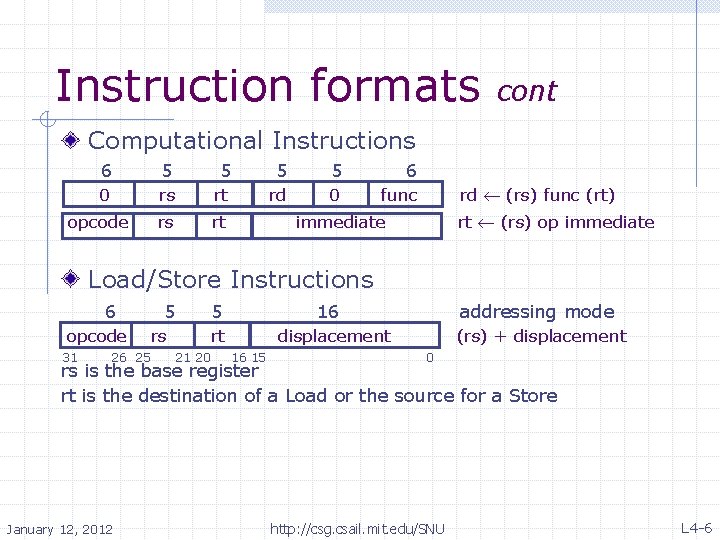
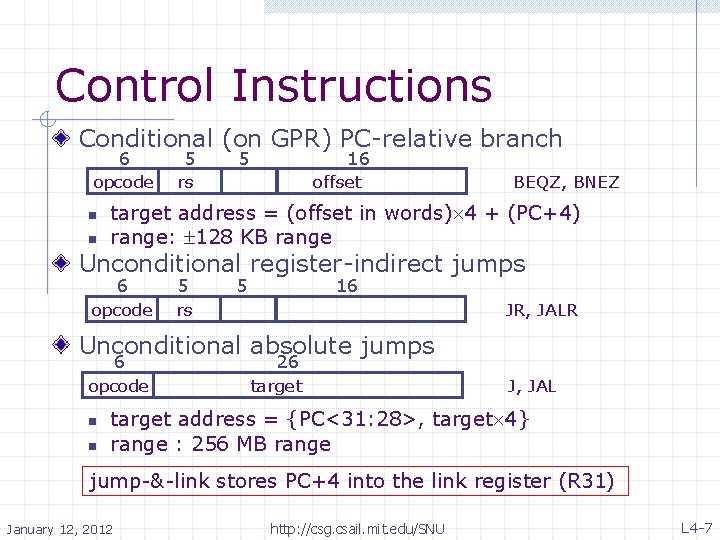
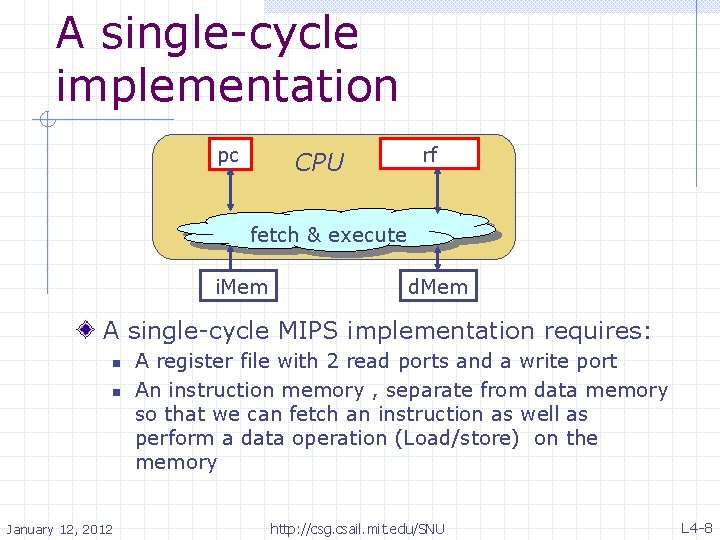
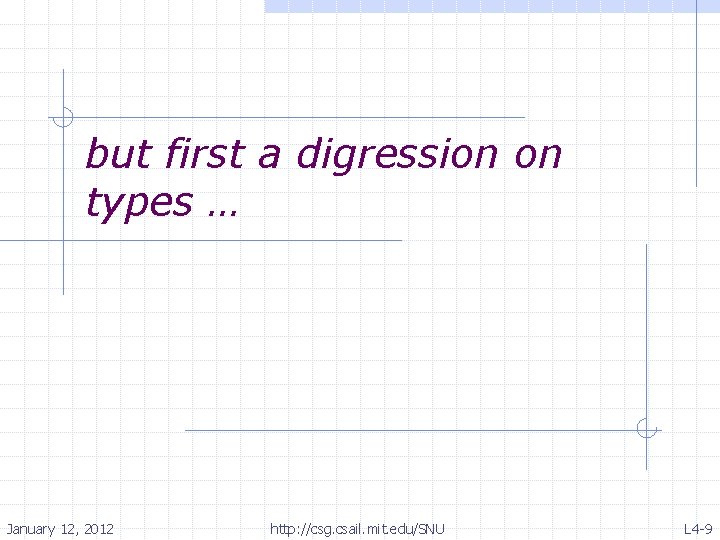
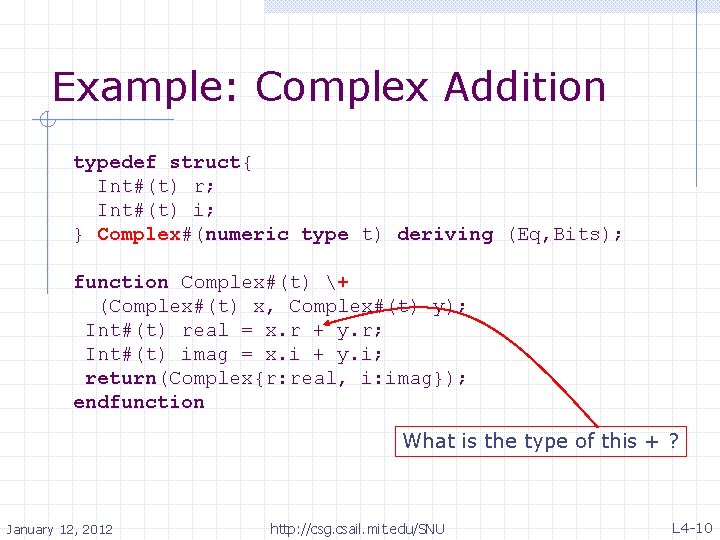
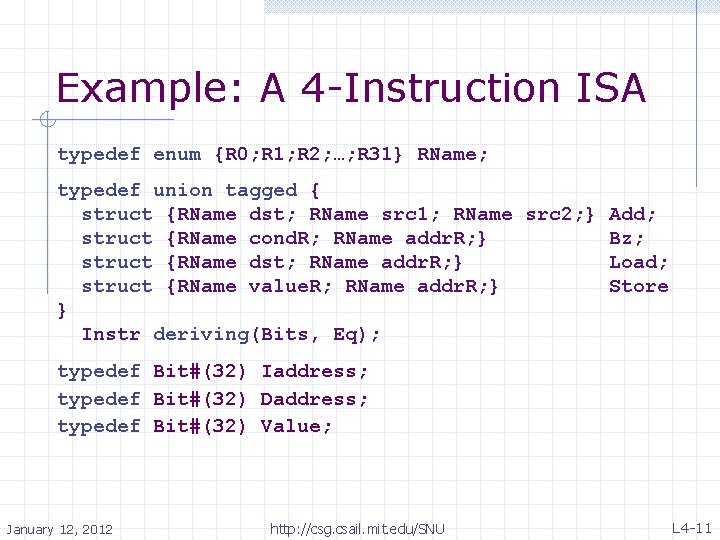
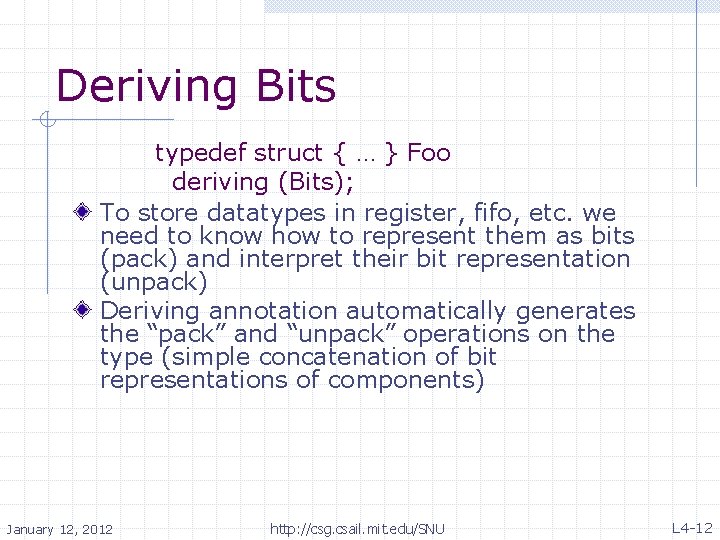
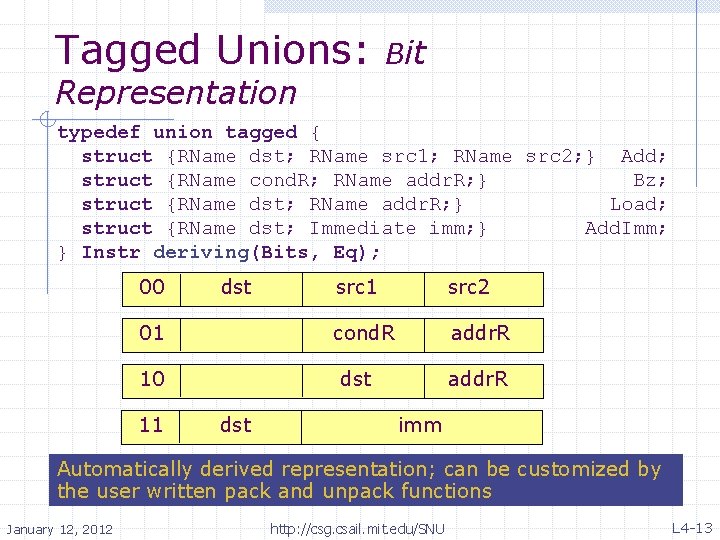
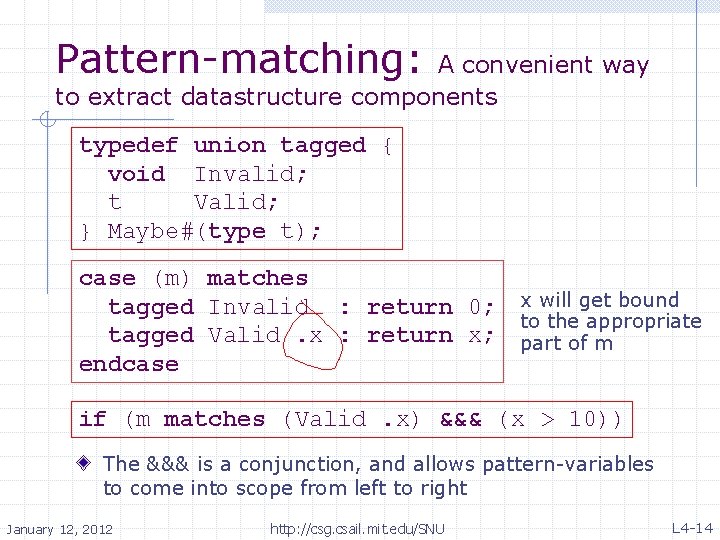
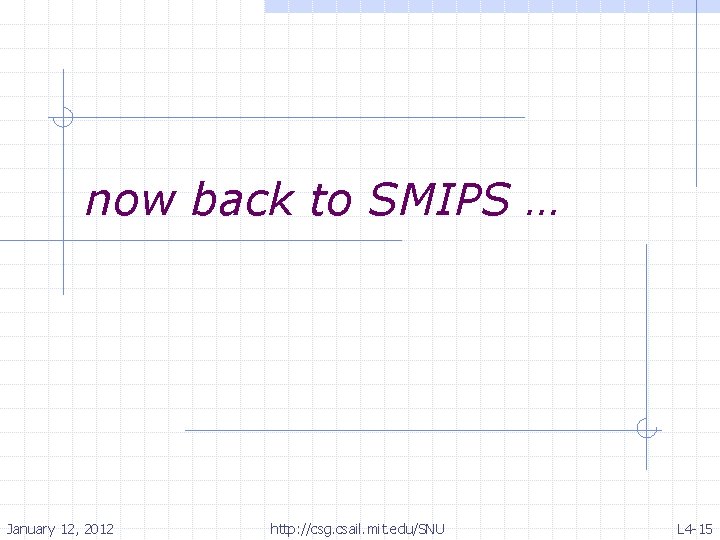
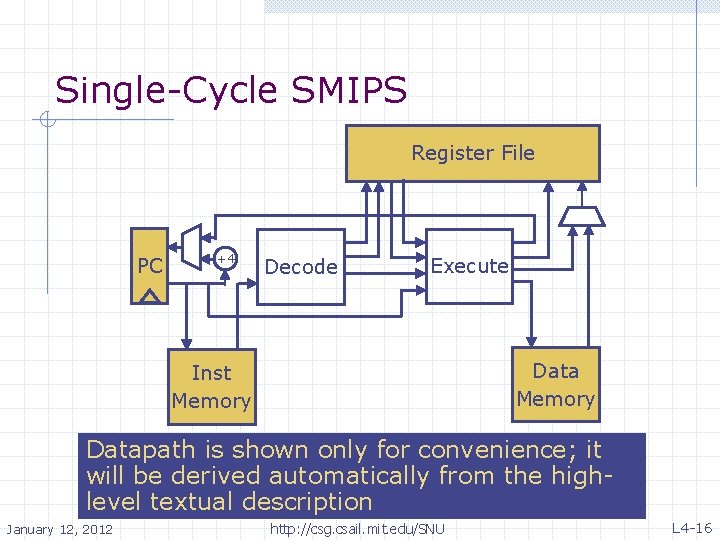
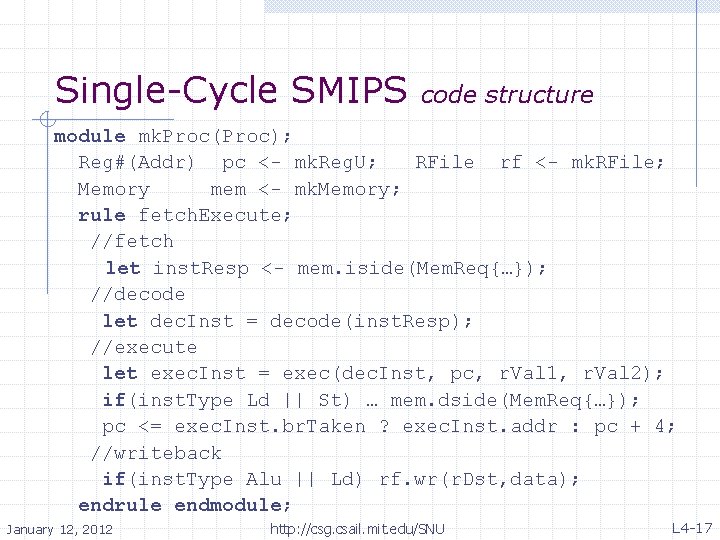
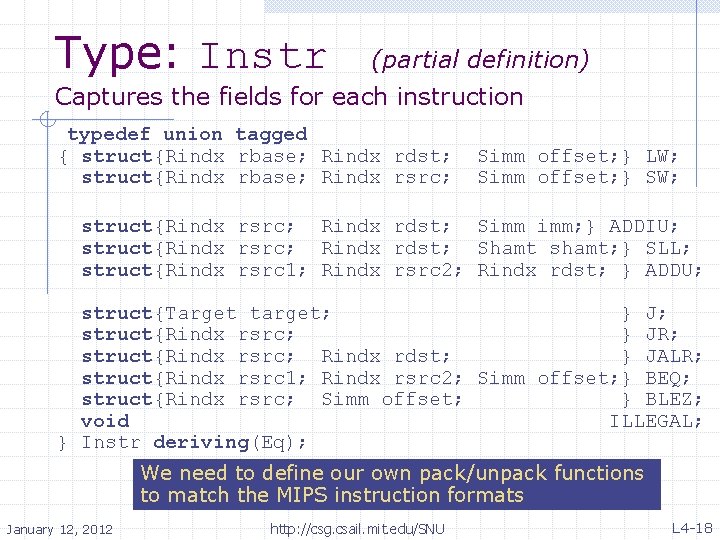
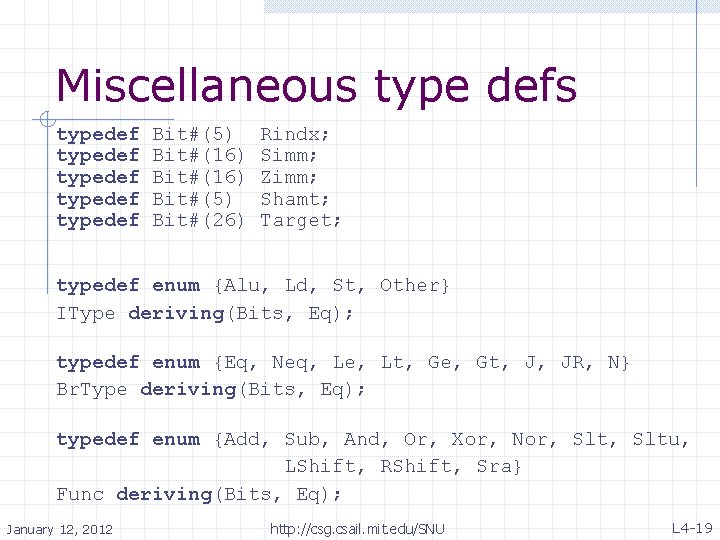
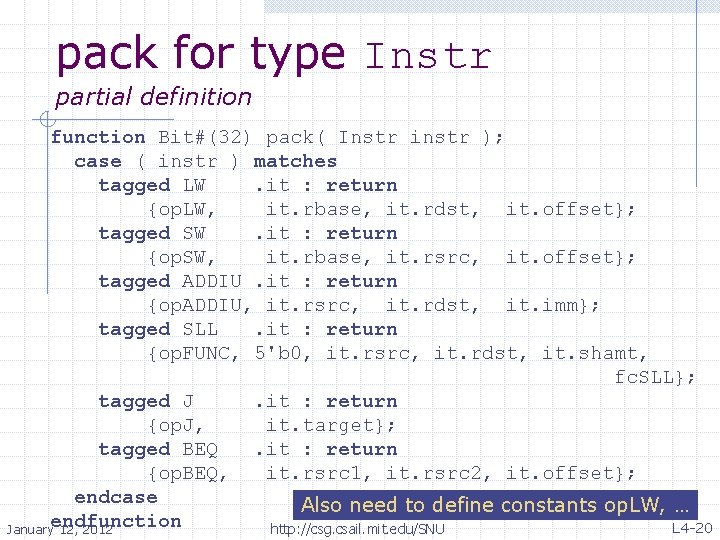
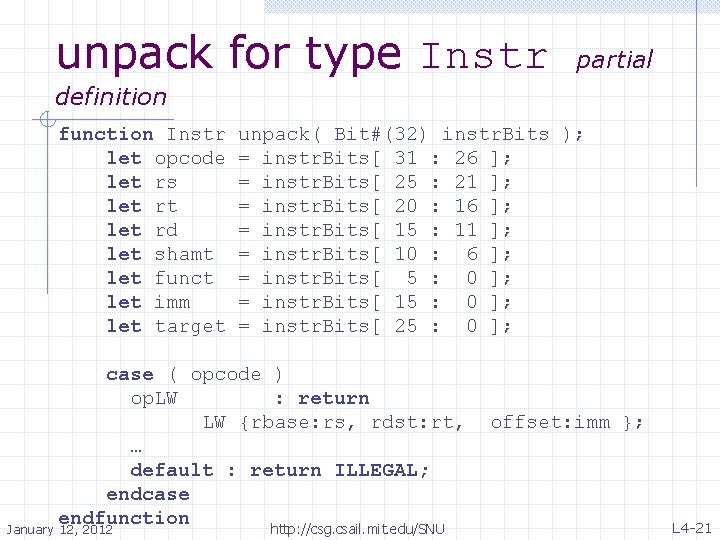
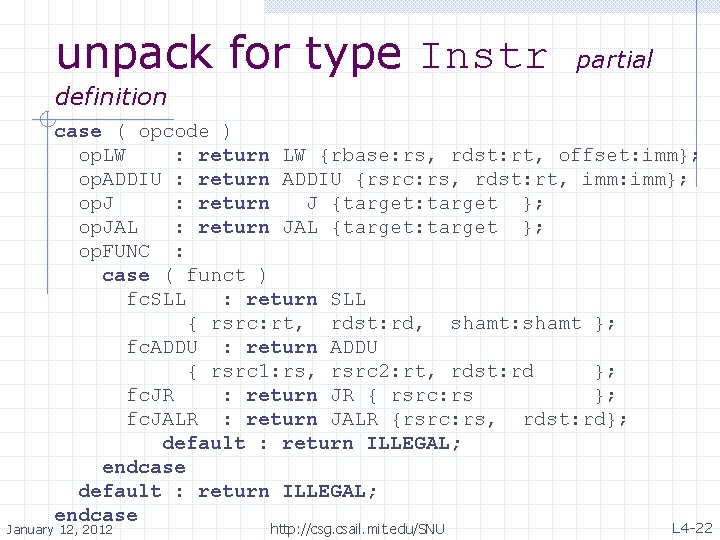
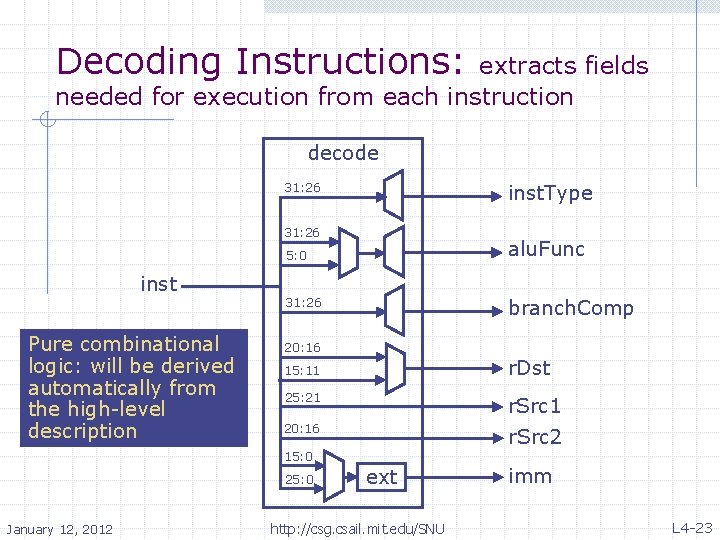
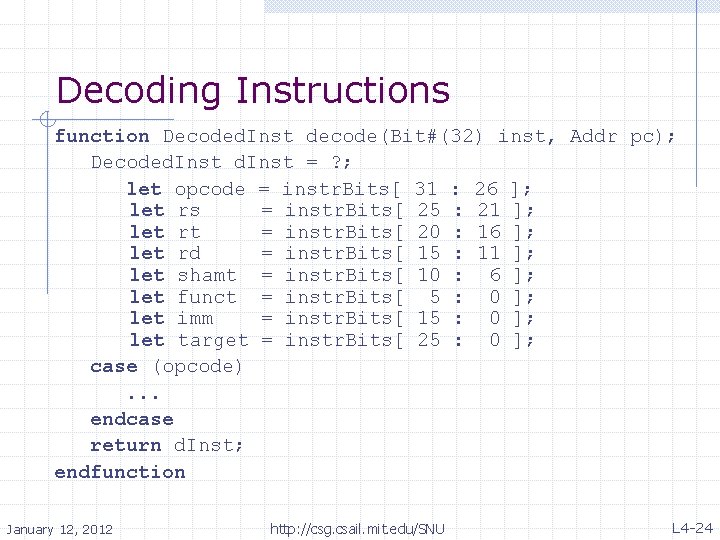
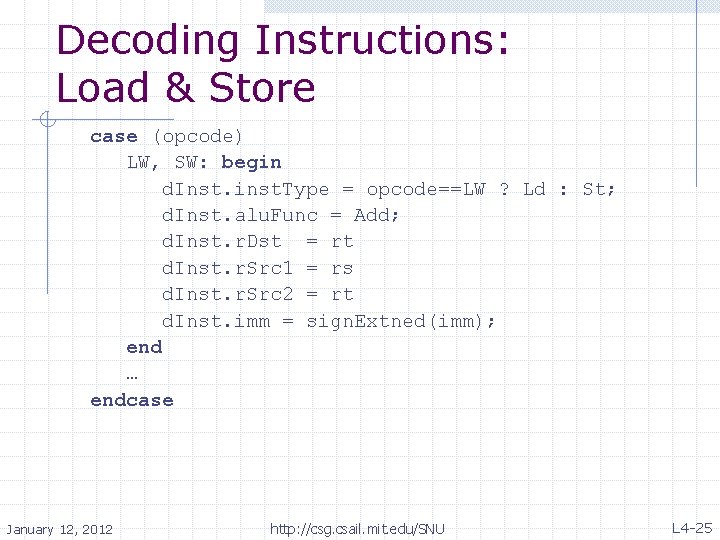
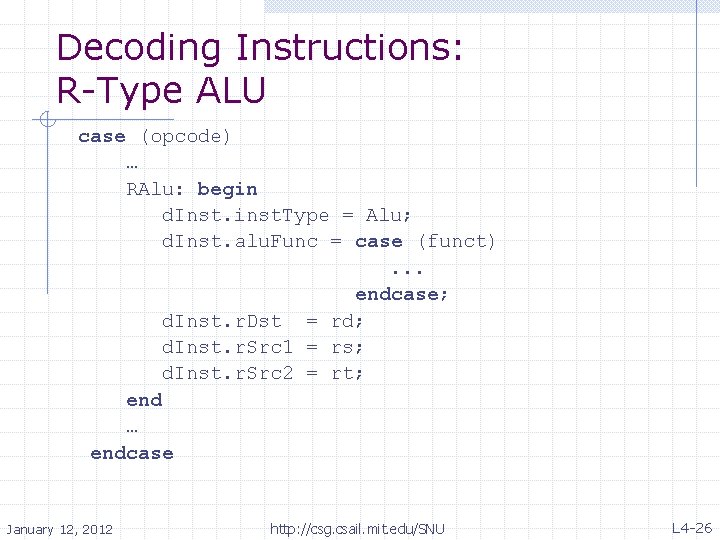
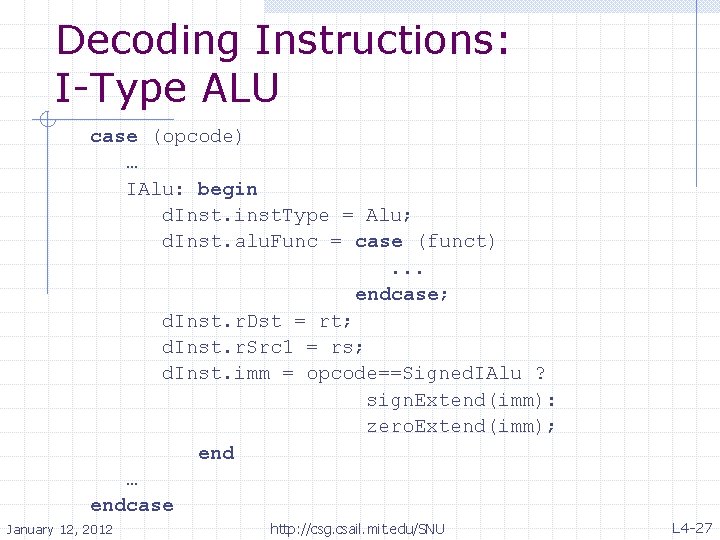
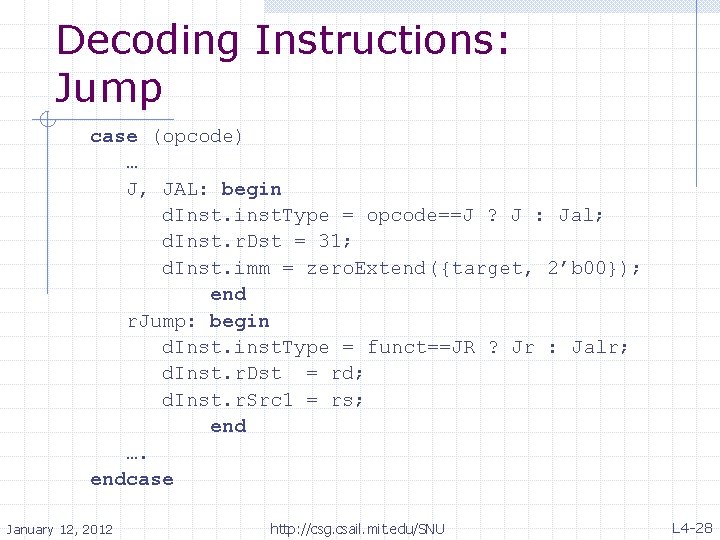
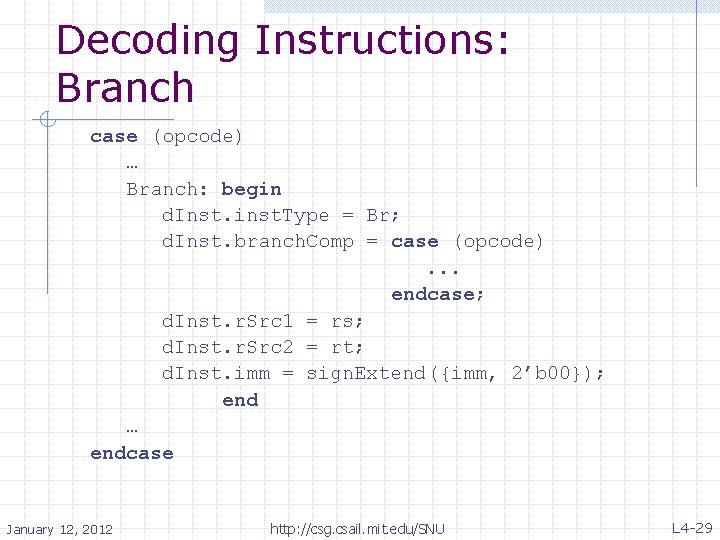
- Slides: 29
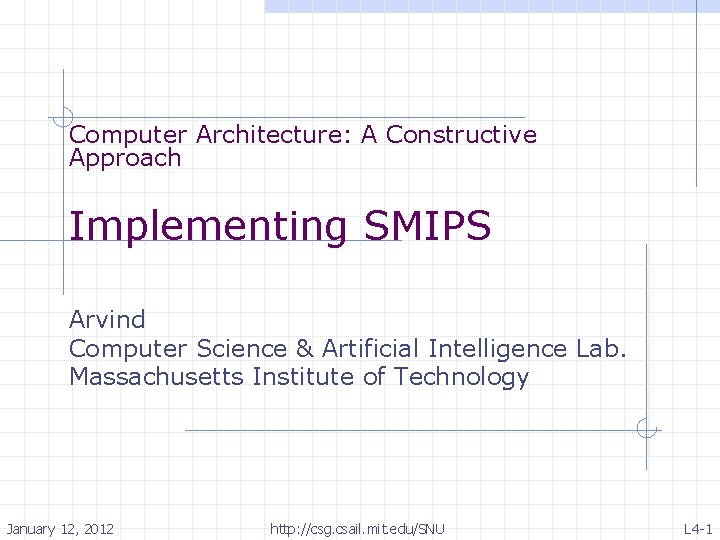
Computer Architecture: A Constructive Approach Implementing SMIPS Arvind Computer Science & Artificial Intelligence Lab. Massachusetts Institute of Technology January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -1
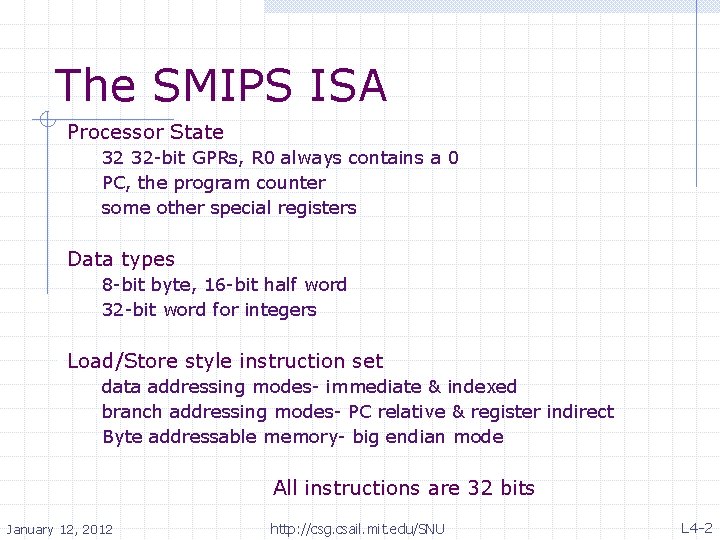
The SMIPS ISA • Processor State • 32 32 -bit GPRs, R 0 always contains a 0 • PC, the program counter • some other special registers • Data types • 8 -bit byte, 16 -bit half word • 32 -bit word for integers • Load/Store style instruction set • data addressing modes- immediate & indexed • branch addressing modes- PC relative & register indirect • Byte addressable memory- big endian mode • January 12, 2012 All instructions are 32 bits http: //csg. csail. mit. edu/SNU L 4 -2
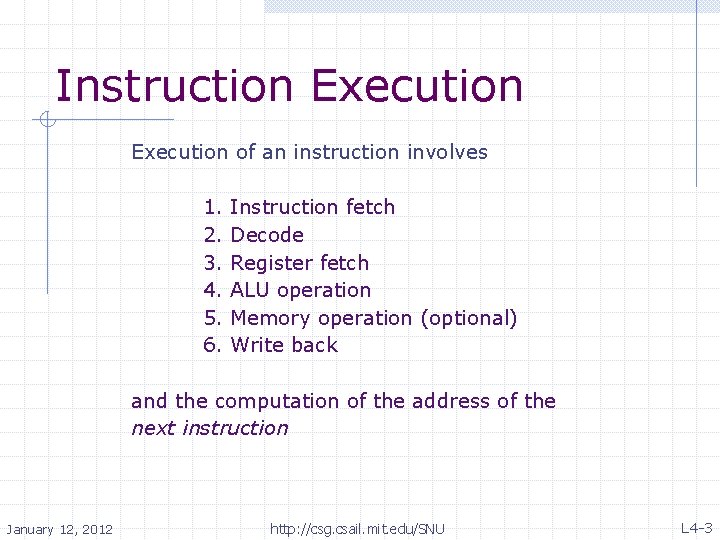
Instruction Execution • Execution of an instruction involves • 1. • 2. • 3. • 4. • 5. • 6. Instruction fetch Decode Register fetch ALU operation Memory operation (optional) Write back • and the computation of the address of the • next instruction January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -3
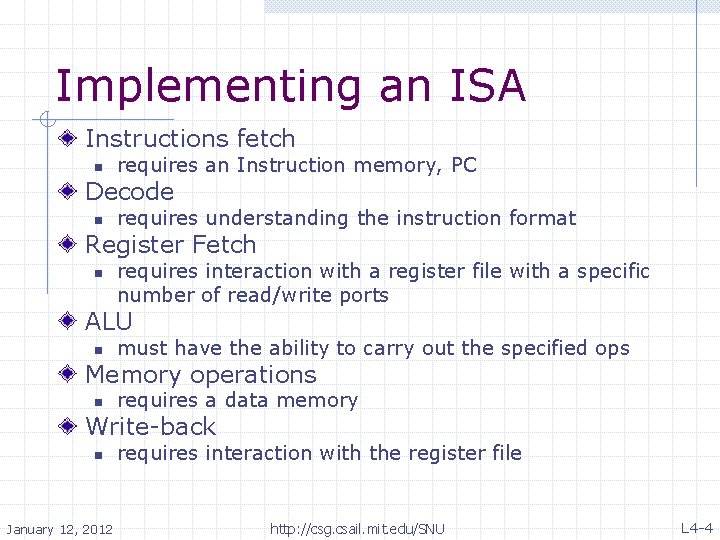
Implementing an ISA Instructions fetch n requires an Instruction memory, PC Decode n requires understanding the instruction format Register Fetch n requires interaction with a register file with a specific number of read/write ports ALU n must have the ability to carry out the specified ops Memory operations n requires a data memory Write-back n January 12, 2012 requires interaction with the register file http: //csg. csail. mit. edu/SNU L 4 -4
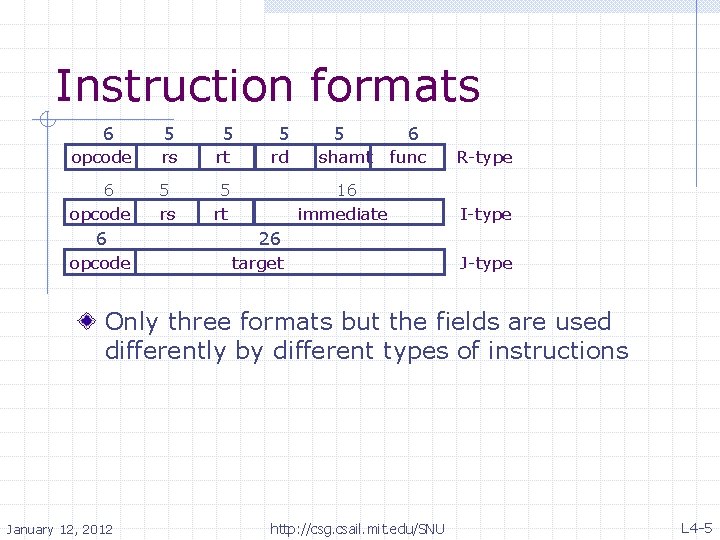
Instruction formats 6 opcode 5 5 rs rt rd 5 5 16 rs rt immediate shamt 6 func R-type I-type 26 target J-type Only three formats but the fields are used differently by different types of instructions January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -5
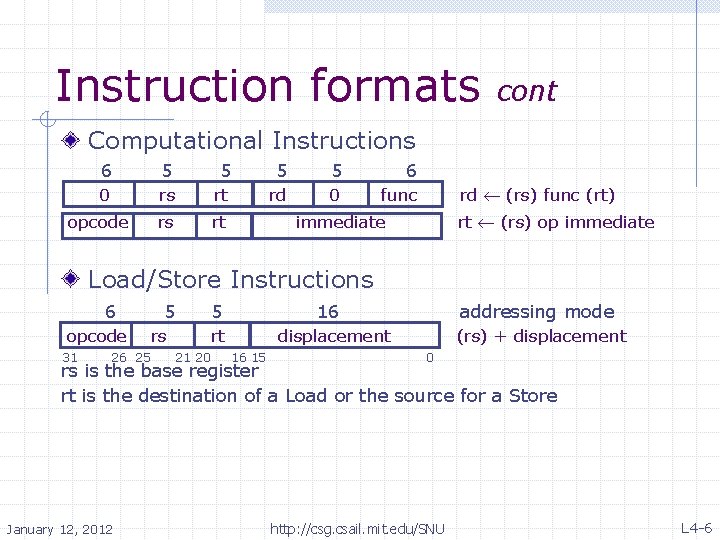
Instruction formats cont Computational Instructions 6 5 5 6 0 rs rt rd 0 func rs rt opcode rd (rs) func (rt) immediate rt (rs) op immediate Load/Store Instructions 6 opcode 31 26 25 5 5 rs 16 rt 21 20 addressing mode displacement 16 15 (rs) + displacement 0 rs is the base register rt is the destination of a Load or the source for a Store January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -6
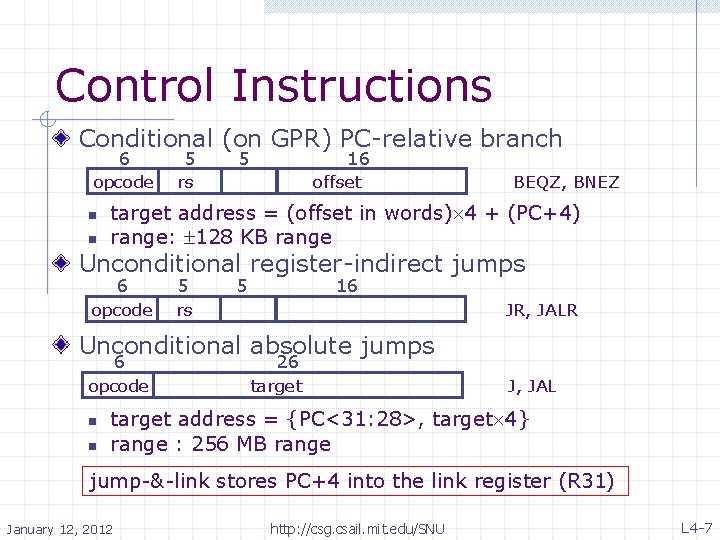
Control Instructions Conditional (on GPR) PC-relative branch 6 5 opcode rs n n 5 16 offset BEQZ, BNEZ target address = (offset in words) 4 + (PC+4) range: 128 KB range Unconditional register-indirect jumps 6 5 opcode rs 5 16 JR, JALR Unconditional absolute jumps 6 opcode n n 26 target J, JAL target address = {PC<31: 28>, target 4} range : 256 MB range jump-&-link stores PC+4 into the link register (R 31) January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -7
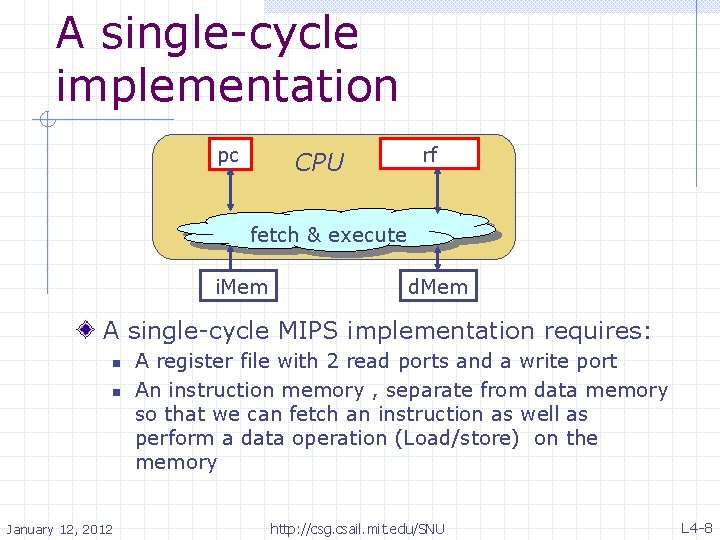
A single-cycle implementation pc CPU rf fetch & execute i. Mem d. Mem A single-cycle MIPS implementation requires: n n January 12, 2012 A register file with 2 read ports and a write port An instruction memory , separate from data memory so that we can fetch an instruction as well as perform a data operation (Load/store) on the memory http: //csg. csail. mit. edu/SNU L 4 -8
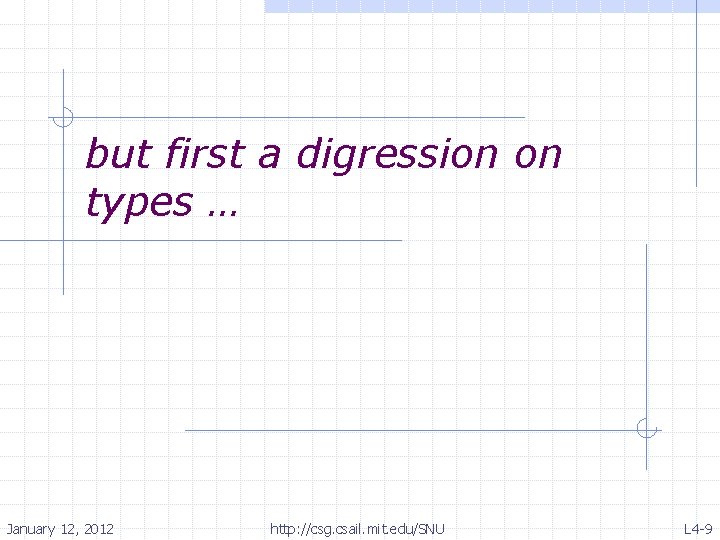
but first a digression on types … January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -9
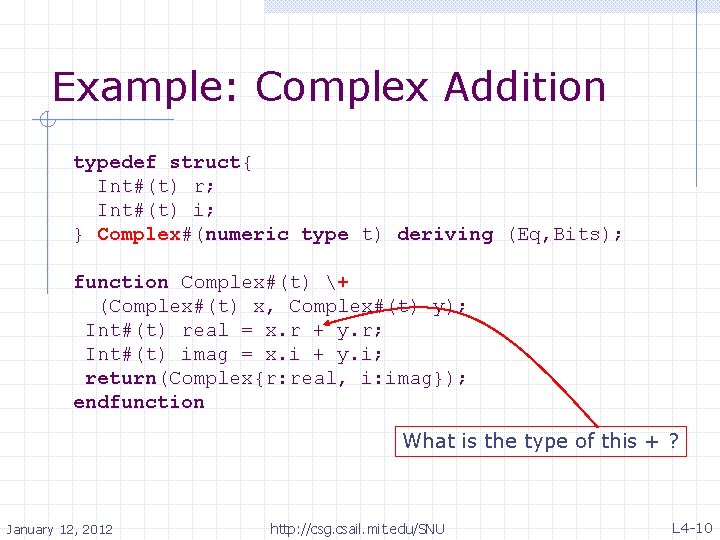
Example: Complex Addition typedef struct{ Int#(t) r; Int#(t) i; } Complex#(numeric type t) deriving (Eq, Bits); function Complex#(t) + (Complex#(t) x, Complex#(t) y); Int#(t) real = x. r + y. r; Int#(t) imag = x. i + y. i; return(Complex{r: real, i: imag}); endfunction What is the type of this + ? January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -10
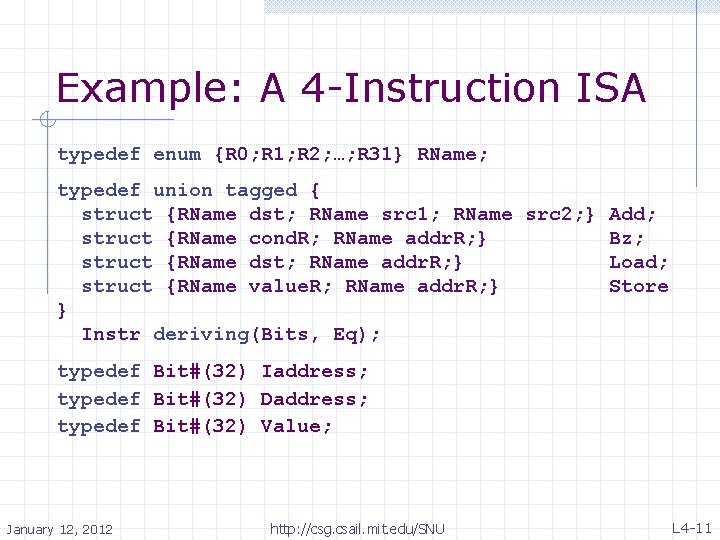
Example: A 4 -Instruction ISA typedef enum {R 0; R 1; R 2; …; R 31} RName; typedef union tagged { struct {RName dst; RName src 1; RName src 2; } Add; struct {RName cond. R; RName addr. R; } Bz; struct {RName dst; RName addr. R; } Load; struct {RName value. R; RName addr. R; } Store } Instr deriving(Bits, Eq); typedef Bit#(32) Iaddress; typedef Bit#(32) Daddress; typedef Bit#(32) Value; January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -11
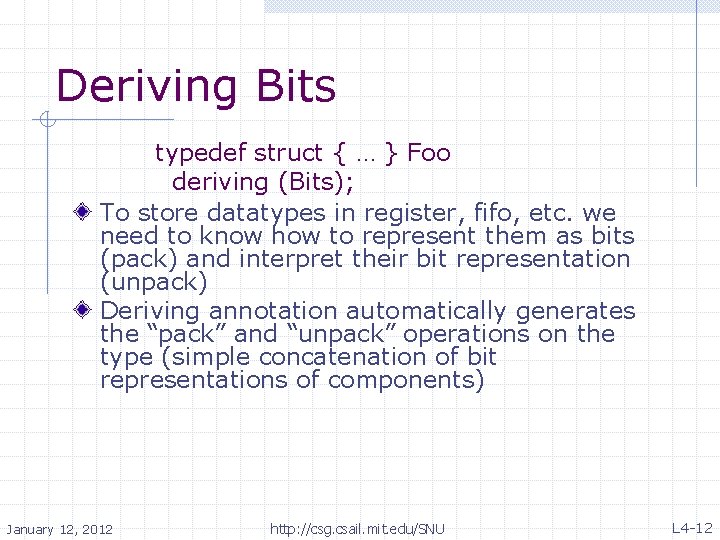
Deriving Bits typedef struct { … } Foo deriving (Bits); To store datatypes in register, fifo, etc. we need to know how to represent them as bits (pack) and interpret their bit representation (unpack) Deriving annotation automatically generates the “pack” and “unpack” operations on the type (simple concatenation of bit representations of components) January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -12
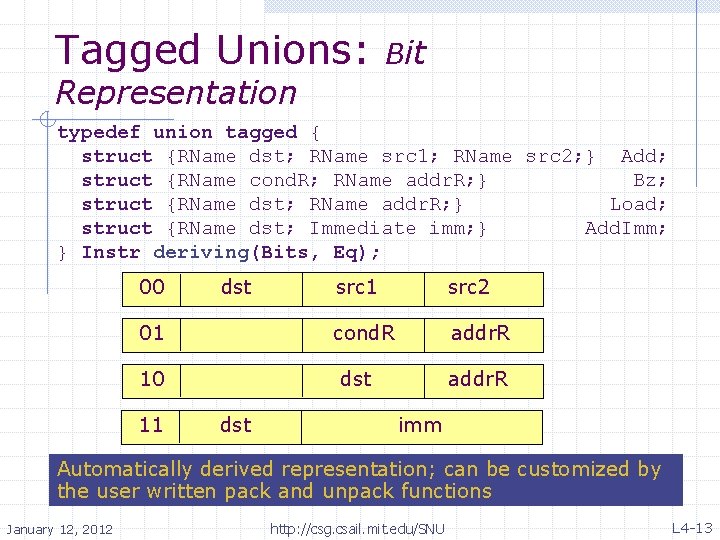
Tagged Unions: Bit Representation typedef union tagged { struct {RName dst; RName src 1; RName src 2; } Add; struct {RName cond. R; RName addr. R; } Bz; struct {RName dst; RName addr. R; } Load; struct {RName dst; Immediate imm; } Add. Imm; } Instr deriving(Bits, Eq); 00 dst 01 10 11 src 2 cond. R addr. R dst addr. R imm Automatically derived representation; can be customized by the user written pack and unpack functions January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -13
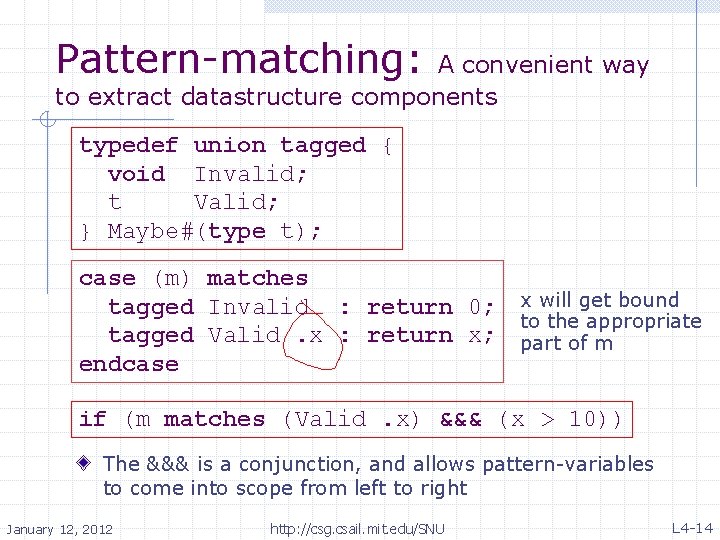
Pattern-matching: A convenient way to extract datastructure components typedef union tagged { void Invalid; t Valid; } Maybe#(type t); case (m) matches tagged Invalid : return 0; x will get bound to the appropriate tagged Valid. x : return x; part of m endcase if (m matches (Valid. x) &&& (x > 10)) The &&& is a conjunction, and allows pattern-variables to come into scope from left to right January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -14
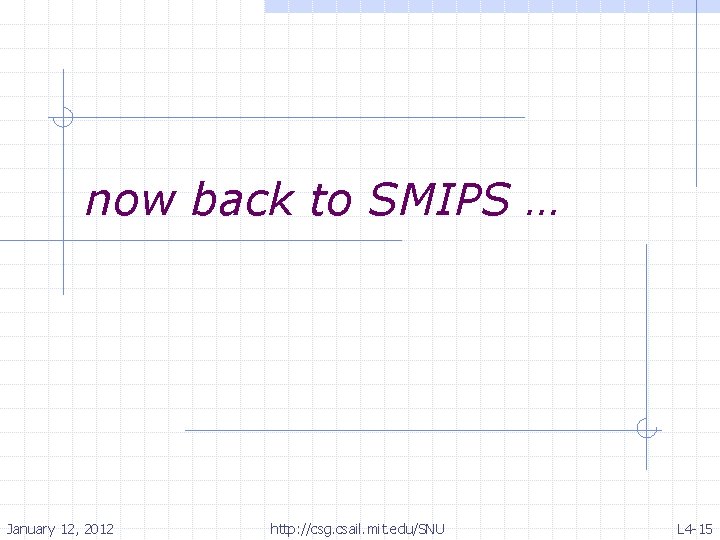
now back to SMIPS … January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -15
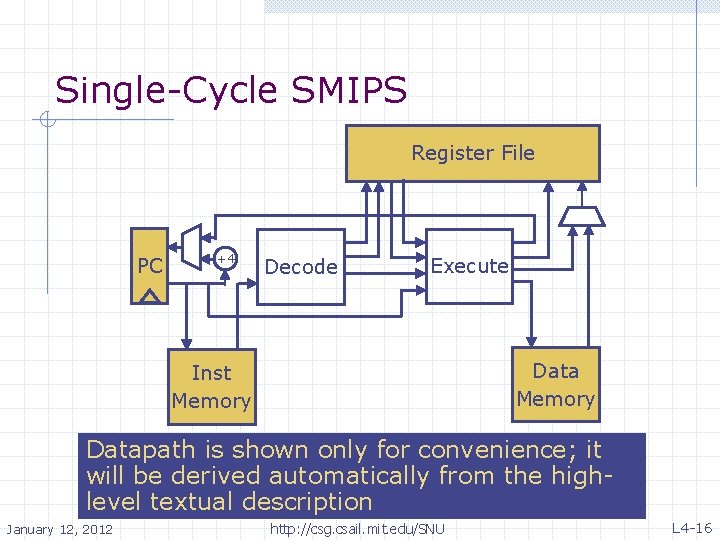
Single-Cycle SMIPS Register File PC +4 Decode Execute Data Memory Inst Memory Datapath is shown only for convenience; it will be derived automatically from the highlevel textual description January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -16
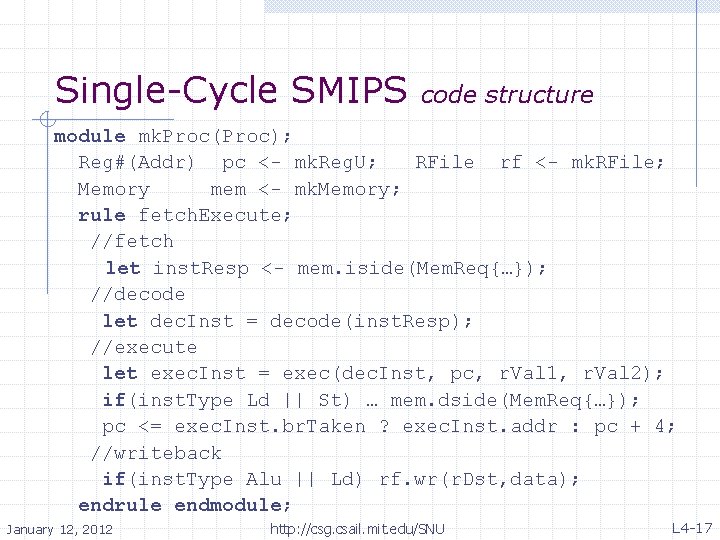
Single-Cycle SMIPS code structure module mk. Proc(Proc); Reg#(Addr) pc <- mk. Reg. U; RFile rf <- mk. RFile; Memory mem <- mk. Memory; rule fetch. Execute; //fetch let inst. Resp <- mem. iside(Mem. Req{…}); //decode let dec. Inst = decode(inst. Resp); //execute let exec. Inst = exec(dec. Inst, pc, r. Val 1, r. Val 2); if(inst. Type Ld || St) … mem. dside(Mem. Req{…}); pc <= exec. Inst. br. Taken ? exec. Inst. addr : pc + 4; //writeback if(inst. Type Alu || Ld) rf. wr(r. Dst, data); endrule endmodule; January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -17
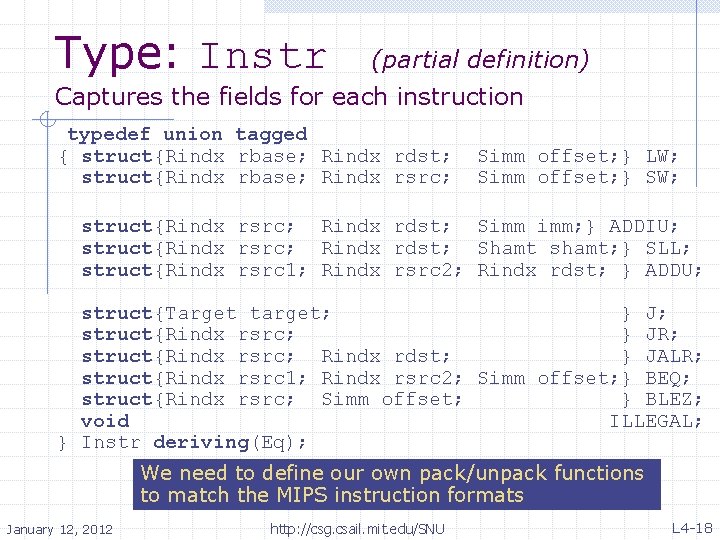
Type: Instr (partial definition) Captures the fields for each instruction • typedef union { struct{Rindx • struct{Rindx tagged rbase; Rindx rdst; rbase; Rindx rsrc; Simm offset; } LW; Simm offset; } SW; rsrc; Rindx rdst; Simm imm; } ADDIU; rsrc; Rindx rdst; Shamt shamt; } SLL; rsrc 1; Rindx rsrc 2; Rindx rdst; } ADDU; struct{Target target; } J; struct{Rindx rsrc; } JR; struct{Rindx rsrc; Rindx rdst; } JALR; struct{Rindx rsrc 1; Rindx rsrc 2; Simm offset; } BEQ; struct{Rindx rsrc; Simm offset; } BLEZ; void ILLEGAL; } Instr deriving(Eq); We need to define our own pack/unpack functions to match the MIPS instruction formats January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -18
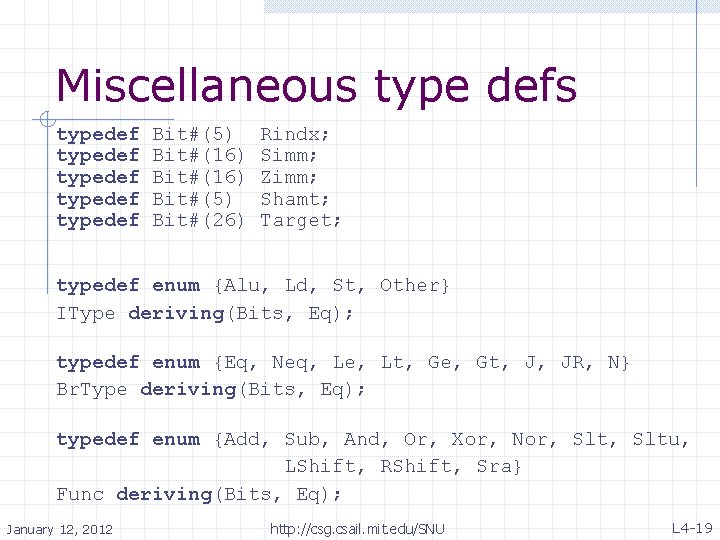
Miscellaneous type defs typedef typedef Bit#(5) Bit#(16) Bit#(5) Bit#(26) Rindx; Simm; Zimm; Shamt; Target; typedef enum {Alu, Ld, St, Other} IType deriving(Bits, Eq); typedef enum {Eq, Neq, Le, Lt, Ge, Gt, J, JR, N} Br. Type deriving(Bits, Eq); typedef enum {Add, Sub, And, Or, Xor, Nor, Sltu, LShift, RShift, Sra} Func deriving(Bits, Eq); January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -19
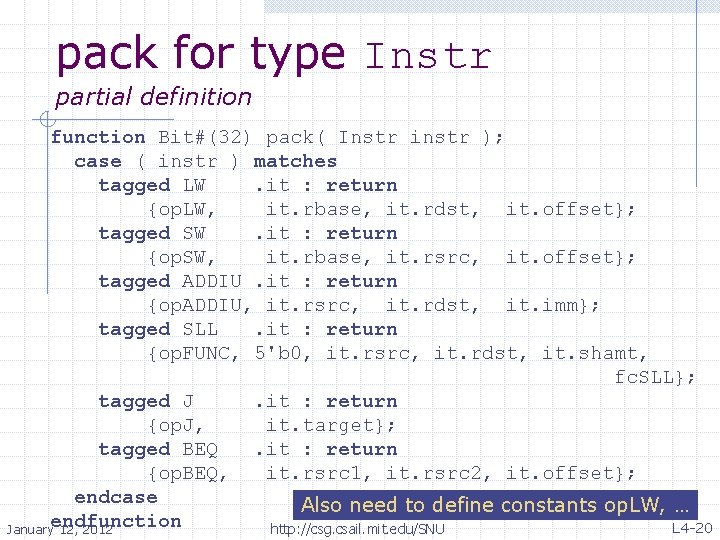
pack for type Instr partial definition function Bit#(32) pack( Instr instr ); case ( instr ) matches tagged LW. it : return {op. LW, it. rbase, it. rdst, it. offset}; tagged SW. it : return {op. SW, it. rbase, it. rsrc, it. offset}; tagged ADDIU. it : return {op. ADDIU, it. rsrc, it. rdst, it. imm}; tagged SLL. it : return {op. FUNC, 5'b 0, it. rsrc, it. rdst, it. shamt, fc. SLL}; tagged J. it : return {op. J, it. target}; tagged BEQ. it : return {op. BEQ, it. rsrc 1, it. rsrc 2, it. offset}; endcase Also need to define constants op. LW, … endfunction L 4 -20 http: //csg. csail. mit. edu/SNU January 12, 2012
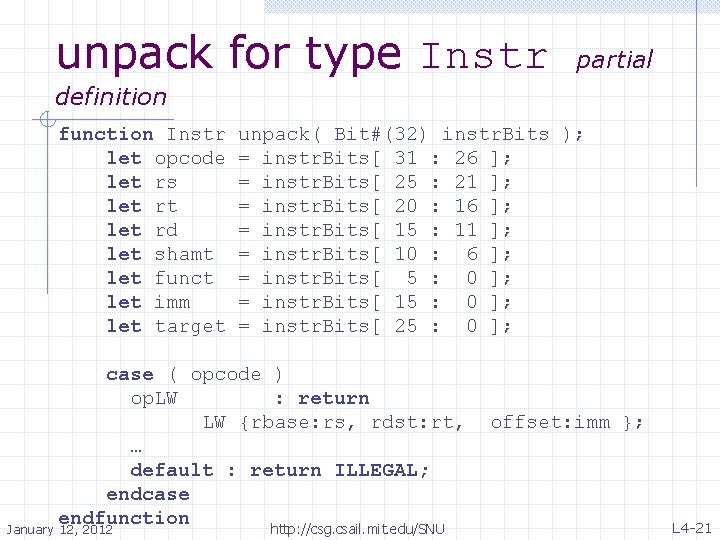
unpack for type Instr partial definition function Instr let opcode let rs let rt let rd let shamt let funct let imm let target unpack( Bit#(32) instr. Bits ); = instr. Bits[ 31 : 26 ]; = instr. Bits[ 25 : 21 ]; = instr. Bits[ 20 : 16 ]; = instr. Bits[ 15 : 11 ]; = instr. Bits[ 10 : 6 ]; = instr. Bits[ 5 : 0 ]; = instr. Bits[ 15 : 0 ]; = instr. Bits[ 25 : 0 ]; case ( opcode ) op. LW : return LW {rbase: rs, rdst: rt, … default : return ILLEGAL; endcase endfunction http: //csg. csail. mit. edu/SNU January 12, 2012 offset: imm }; L 4 -21
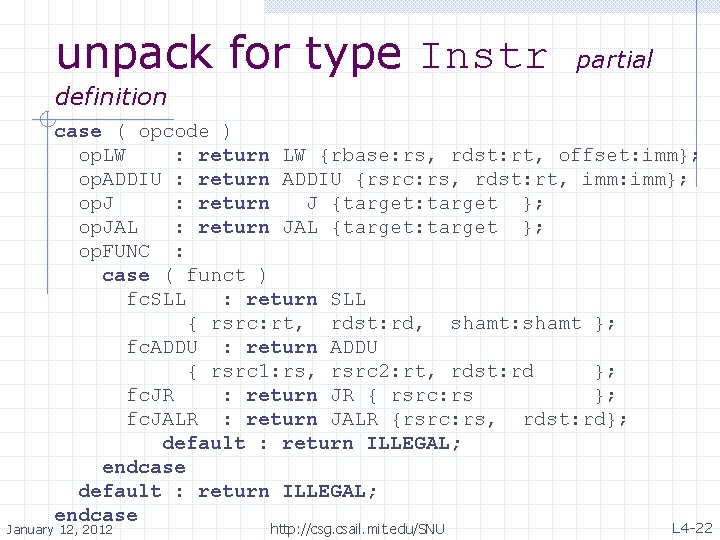
unpack for type Instr partial definition case ( opcode ) op. LW : return LW {rbase: rs, rdst: rt, offset: imm}; op. ADDIU : return ADDIU {rsrc: rs, rdst: rt, imm: imm}; op. J : return J {target: target }; op. JAL : return JAL {target: target }; op. FUNC : case ( funct ) fc. SLL : return SLL { rsrc: rt, rdst: rd, shamt: shamt }; fc. ADDU : return ADDU { rsrc 1: rs, rsrc 2: rt, rdst: rd }; fc. JR : return JR { rsrc: rs }; fc. JALR : return JALR {rsrc: rs, rdst: rd}; default : return ILLEGAL; endcase January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -22
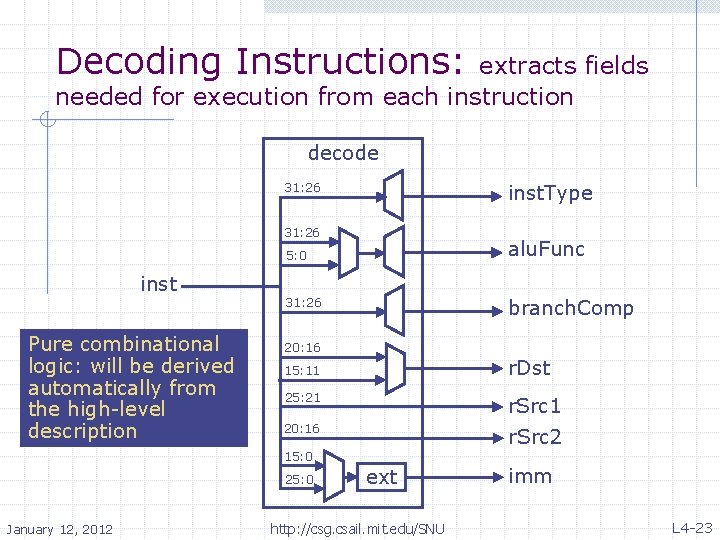
Decoding Instructions: extracts fields needed for execution from each instruction decode 31: 26 inst. Type 31: 26 alu. Func 5: 0 inst Pure combinational logic: will be derived automatically from the high-level description 31: 26 branch. Comp 20: 16 r. Dst 15: 11 25: 21 r. Src 1 20: 16 r. Src 2 15: 0 25: 0 January 12, 2012 ext http: //csg. csail. mit. edu/SNU imm L 4 -23
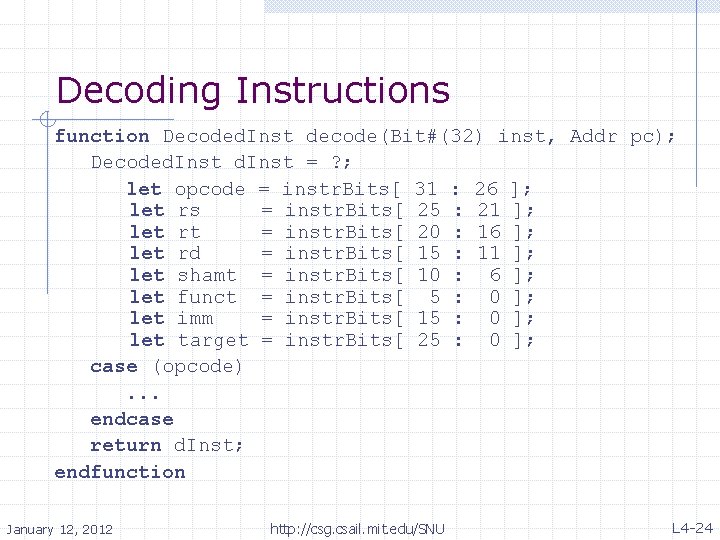
Decoding Instructions function Decoded. Inst decode(Bit#(32) inst, Addr pc); Decoded. Inst = ? ; let opcode = instr. Bits[ 31 : 26 ]; let rs = instr. Bits[ 25 : 21 ]; let rt = instr. Bits[ 20 : 16 ]; let rd = instr. Bits[ 15 : 11 ]; let shamt = instr. Bits[ 10 : 6 ]; let funct = instr. Bits[ 5 : 0 ]; let imm = instr. Bits[ 15 : 0 ]; let target = instr. Bits[ 25 : 0 ]; case (opcode). . . endcase return d. Inst; endfunction January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -24
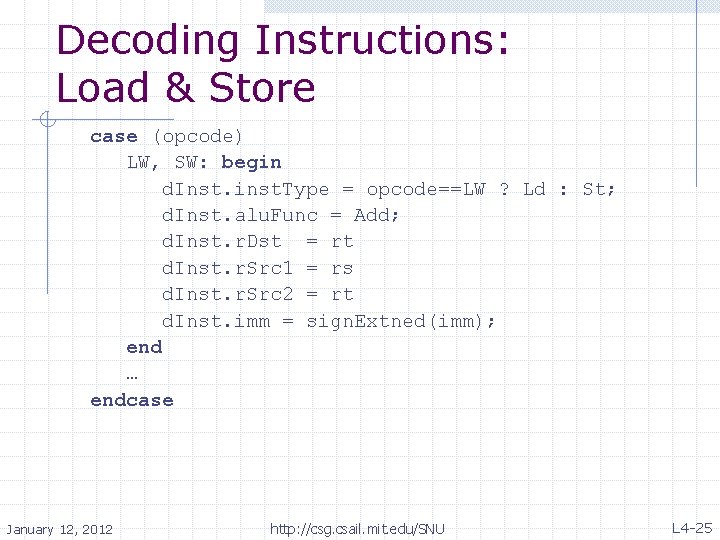
Decoding Instructions: Load & Store case (opcode) LW, SW: begin d. Inst. inst. Type = opcode==LW ? Ld : St; d. Inst. alu. Func = Add; d. Inst. r. Dst = rt d. Inst. r. Src 1 = rs d. Inst. r. Src 2 = rt d. Inst. imm = sign. Extned(imm); end … endcase January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -25
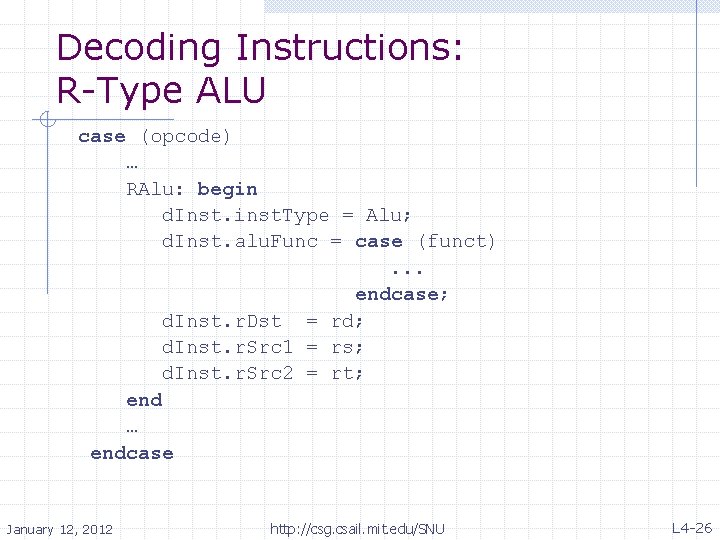
Decoding Instructions: R-Type ALU case (opcode) … RAlu: begin d. Inst. inst. Type = Alu; d. Inst. alu. Func = case (funct). . . endcase; d. Inst. r. Dst = rd; d. Inst. r. Src 1 = rs; d. Inst. r. Src 2 = rt; end … endcase January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -26
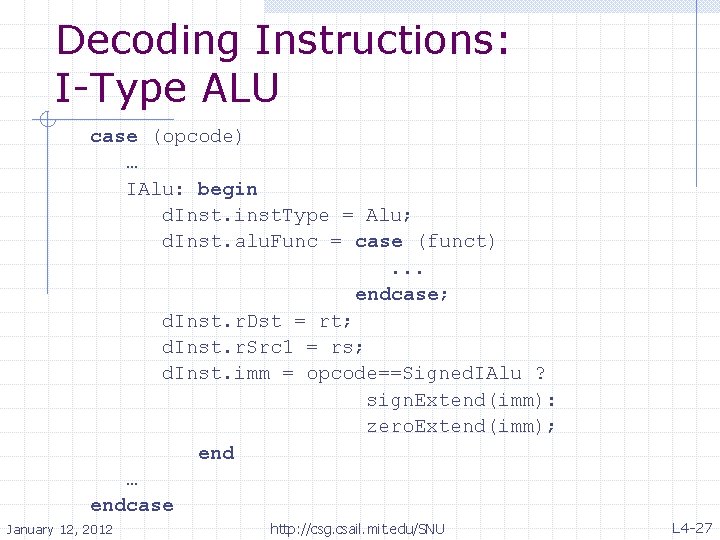
Decoding Instructions: I-Type ALU case (opcode) … IAlu: begin d. Inst. inst. Type = Alu; d. Inst. alu. Func = case (funct). . . endcase; d. Inst. r. Dst = rt; d. Inst. r. Src 1 = rs; d. Inst. imm = opcode==Signed. IAlu ? sign. Extend(imm): zero. Extend(imm); end … endcase January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -27
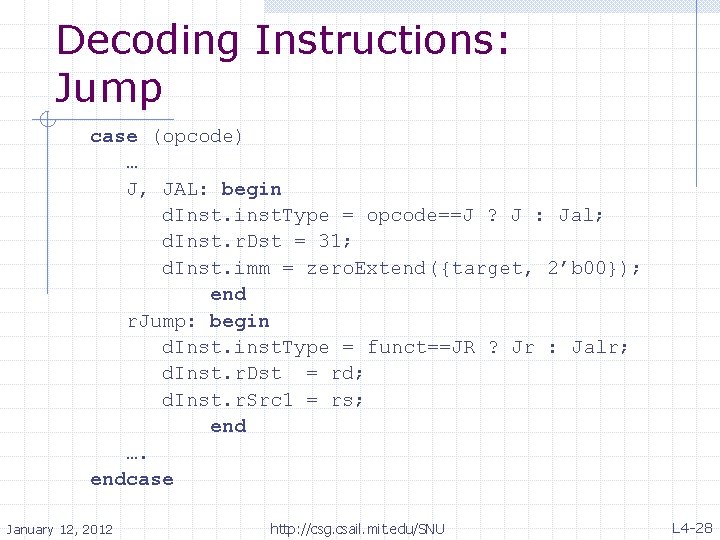
Decoding Instructions: Jump case (opcode) … J, JAL: begin d. Inst. inst. Type = opcode==J ? J : Jal; d. Inst. r. Dst = 31; d. Inst. imm = zero. Extend({target, 2’b 00}); end r. Jump: begin d. Inst. inst. Type = funct==JR ? Jr : Jalr; d. Inst. r. Dst = rd; d. Inst. r. Src 1 = rs; end …. endcase January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -28
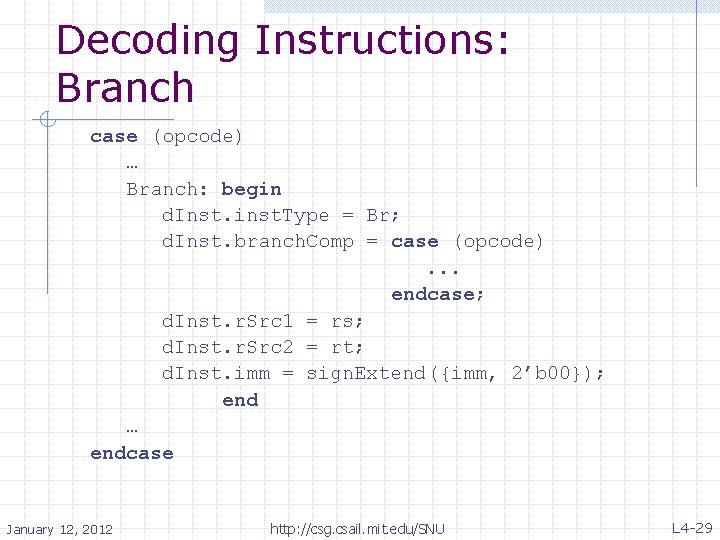
Decoding Instructions: Branch case (opcode) … Branch: begin d. Inst. inst. Type = Br; d. Inst. branch. Comp = case (opcode). . . endcase; d. Inst. r. Src 1 = rs; d. Inst. r. Src 2 = rt; d. Inst. imm = sign. Extend({imm, 2’b 00}); end … endcase January 12, 2012 http: //csg. csail. mit. edu/SNU L 4 -29