Compsci 101 Design Implementation Words Owen Astrachan Kristin
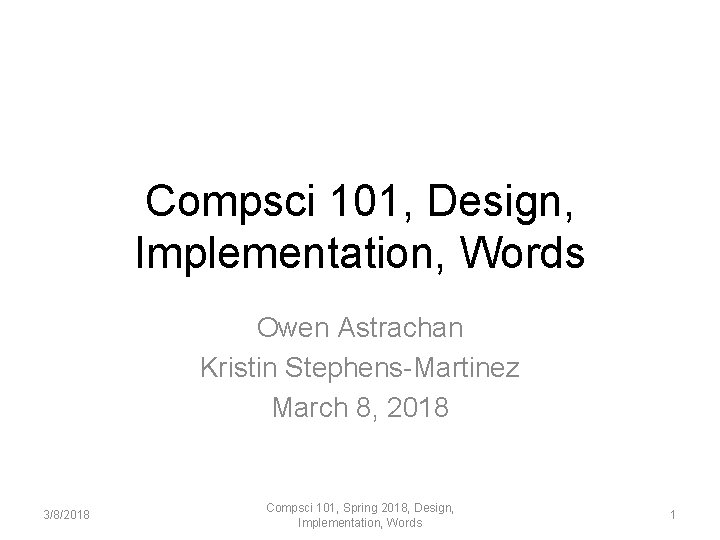
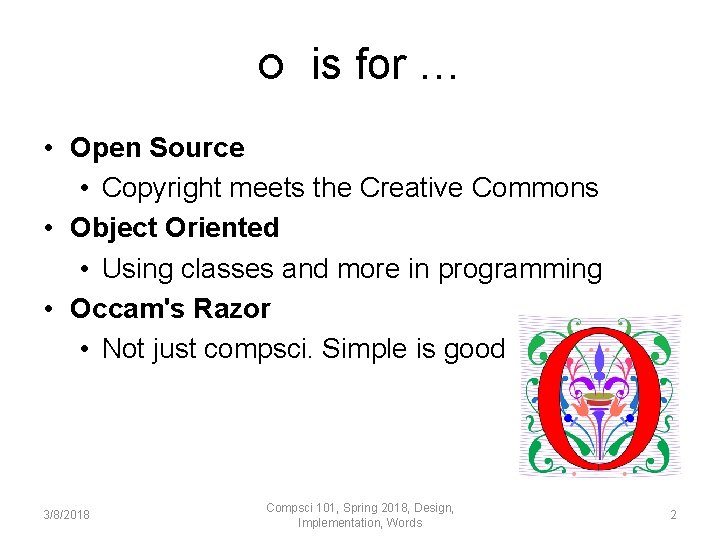
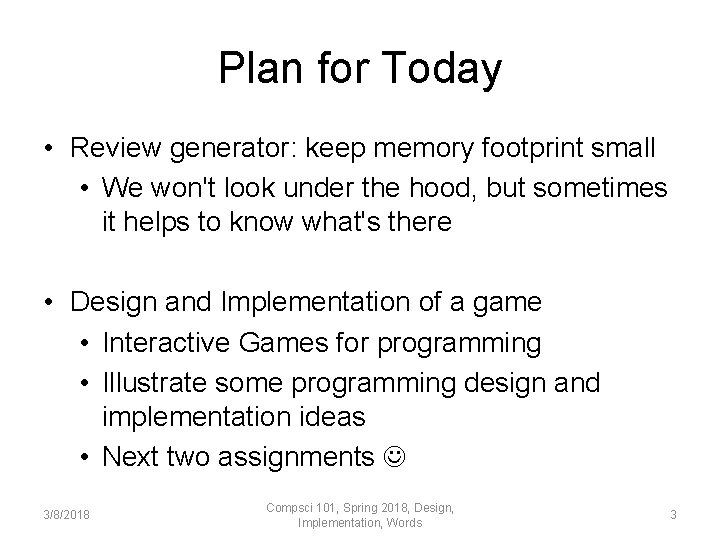
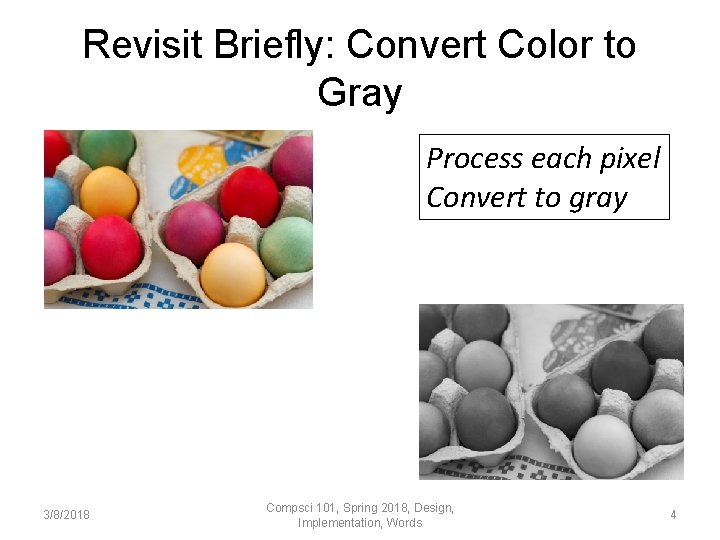
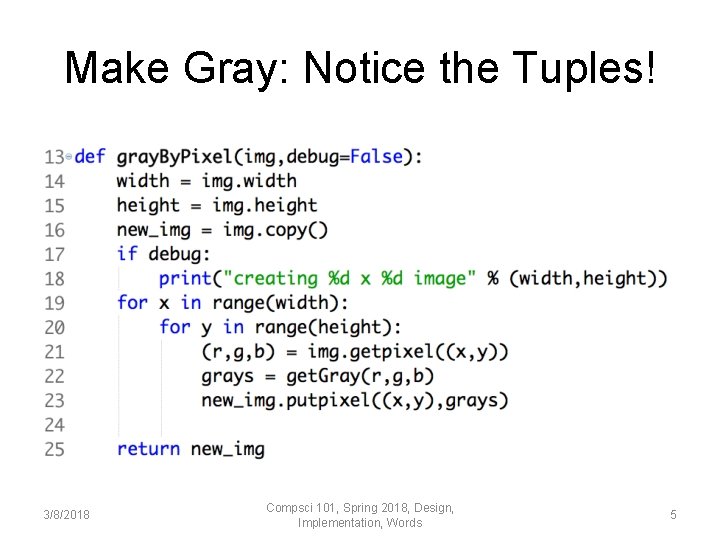
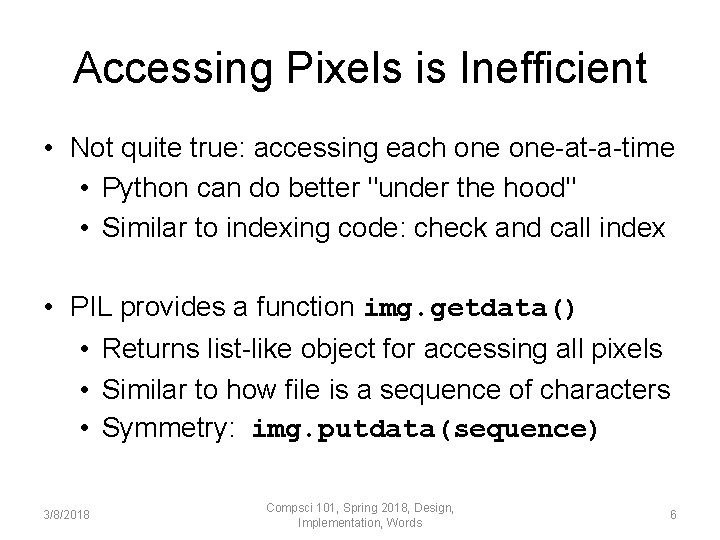
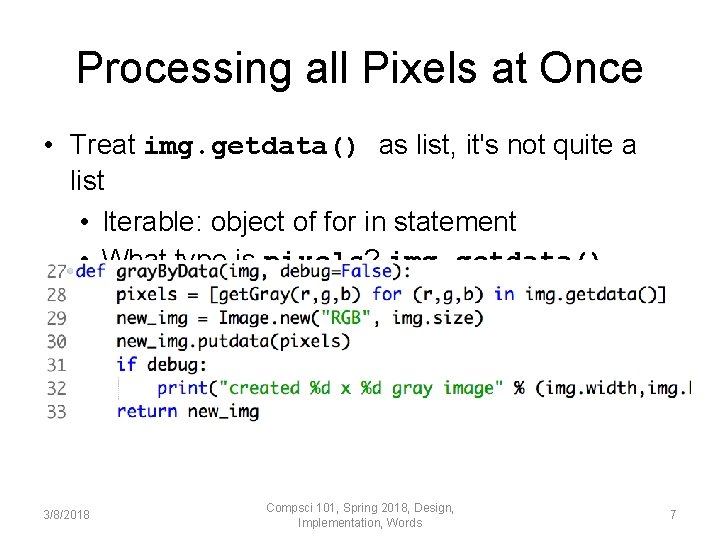
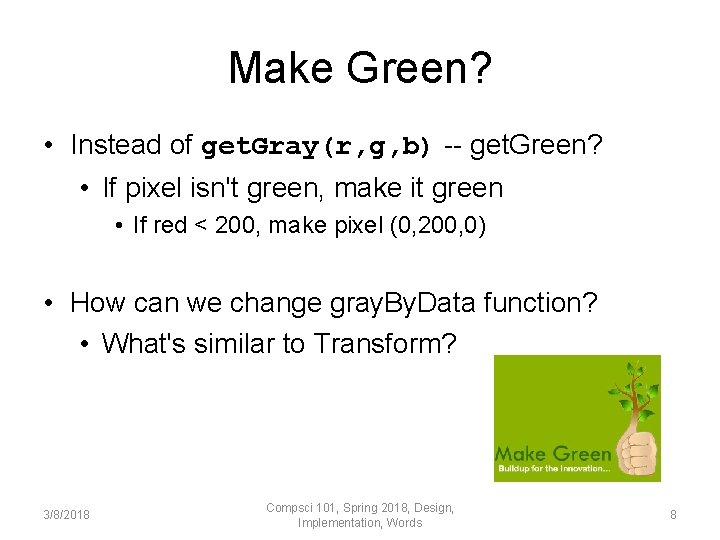
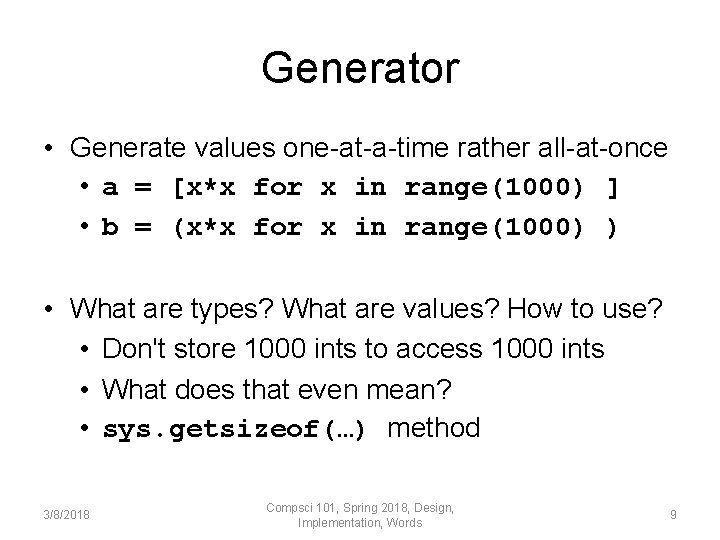
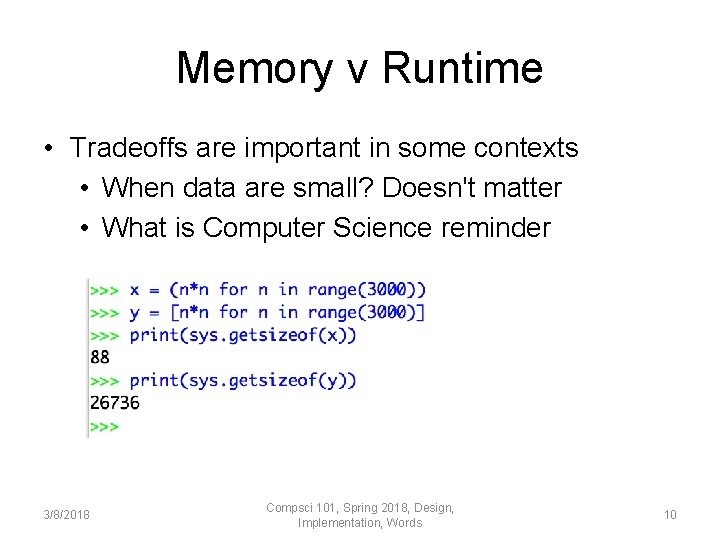
![Comprehensions • List comprehension • [w for w in words if len(w) > 20] Comprehensions • List comprehension • [w for w in words if len(w) > 20]](https://slidetodoc.com/presentation_image_h/2e51bd97cf411d2c0e341234c080f818/image-11.jpg)
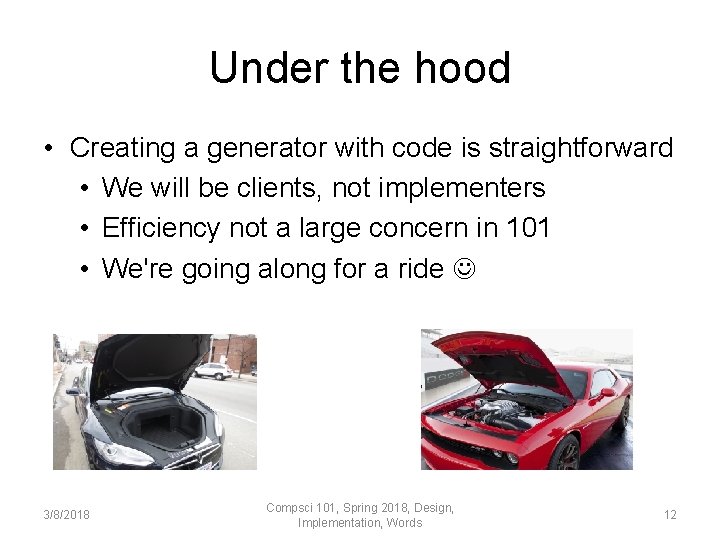
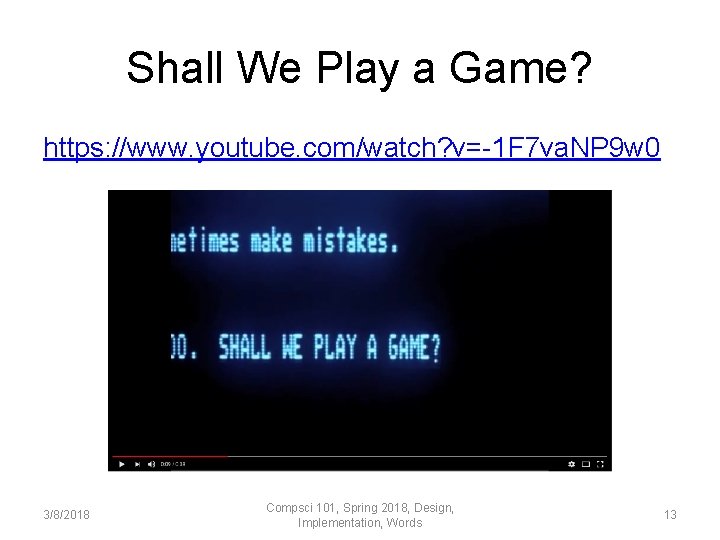
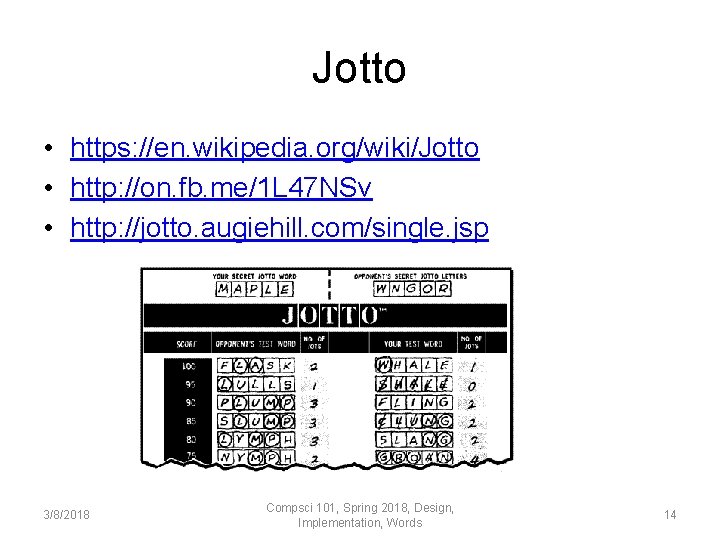
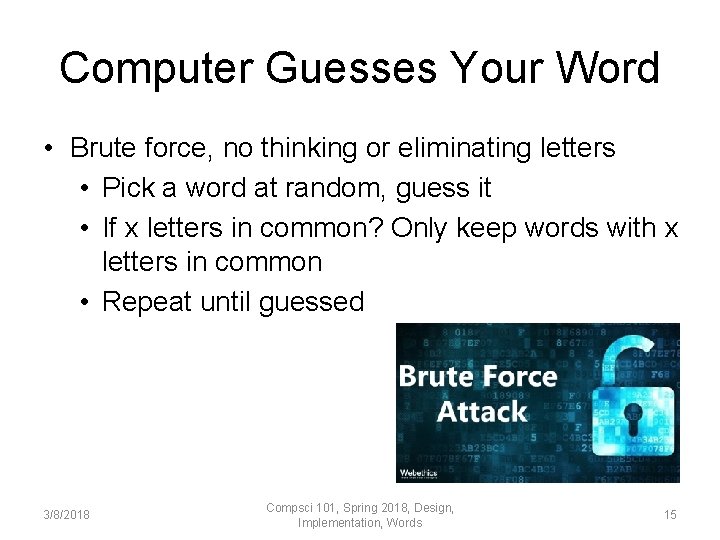
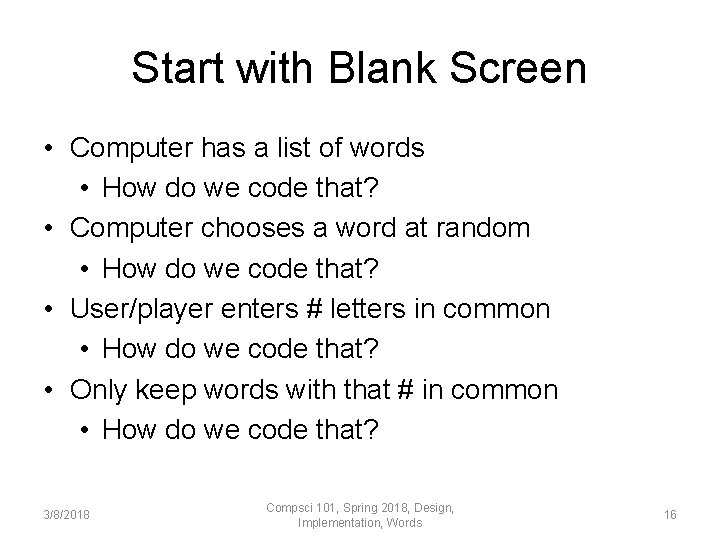
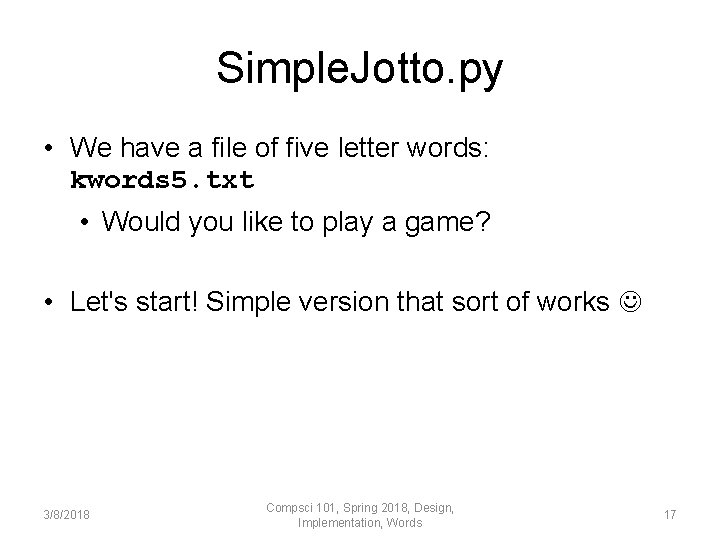
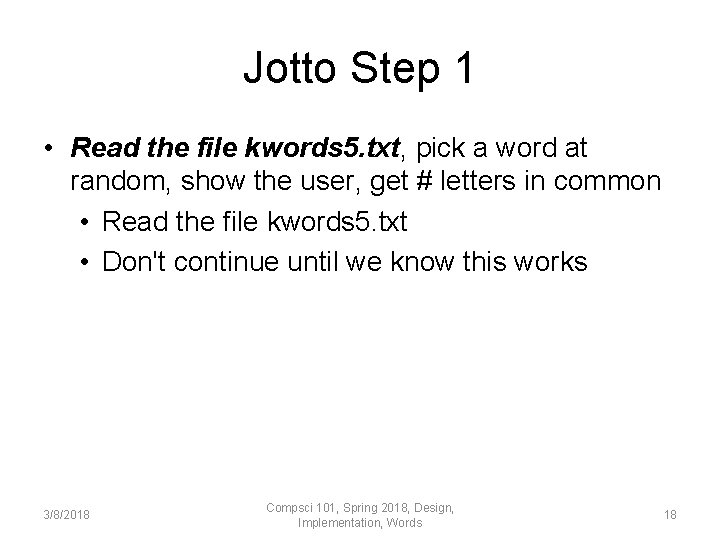
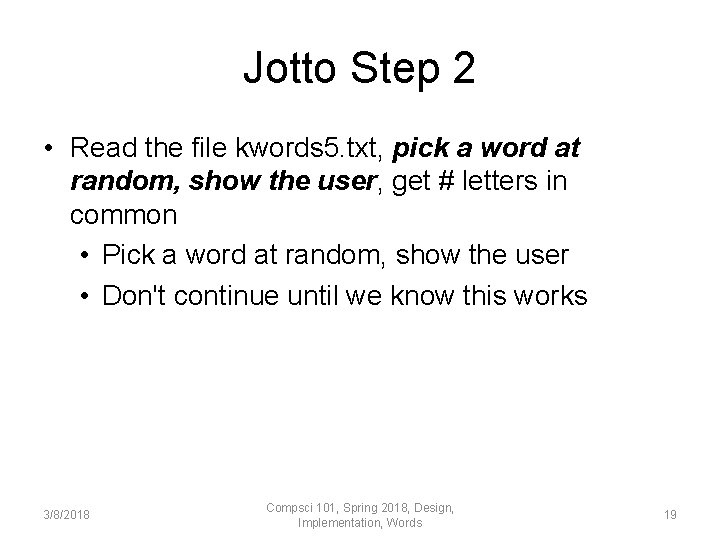
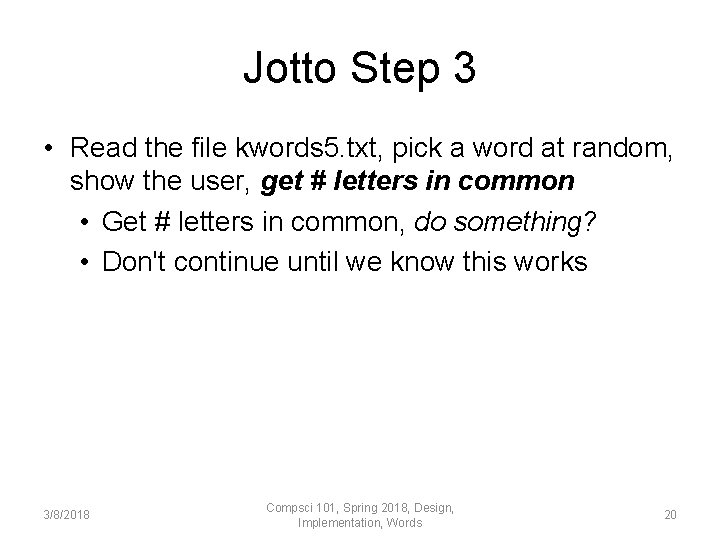
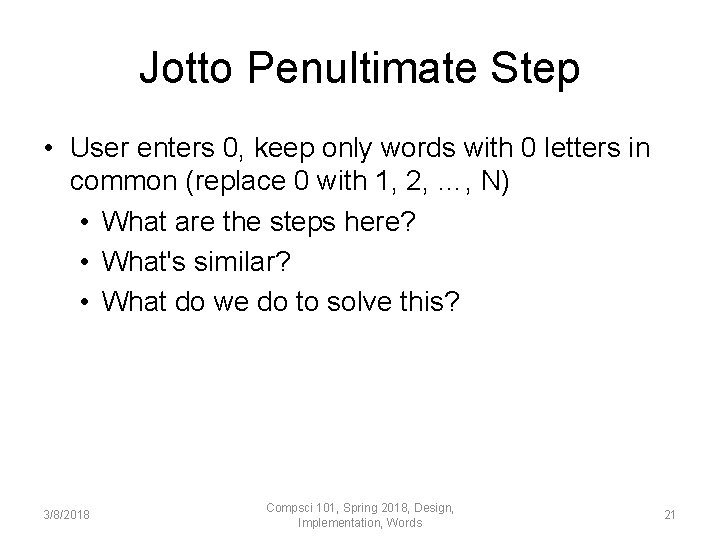
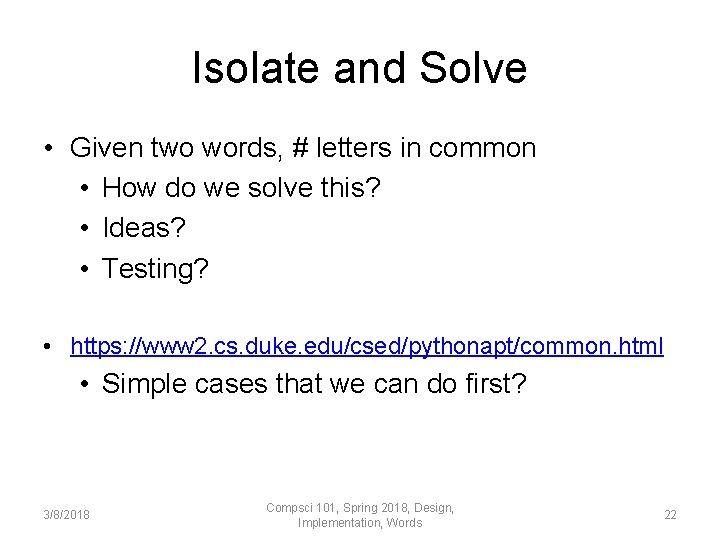
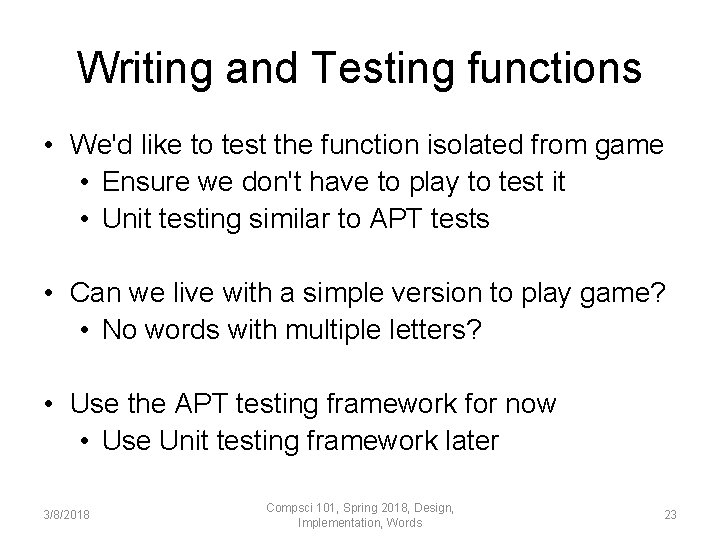
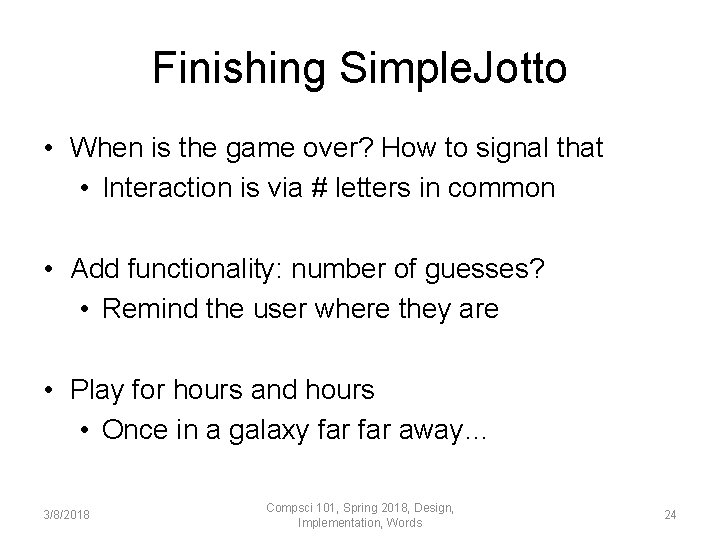
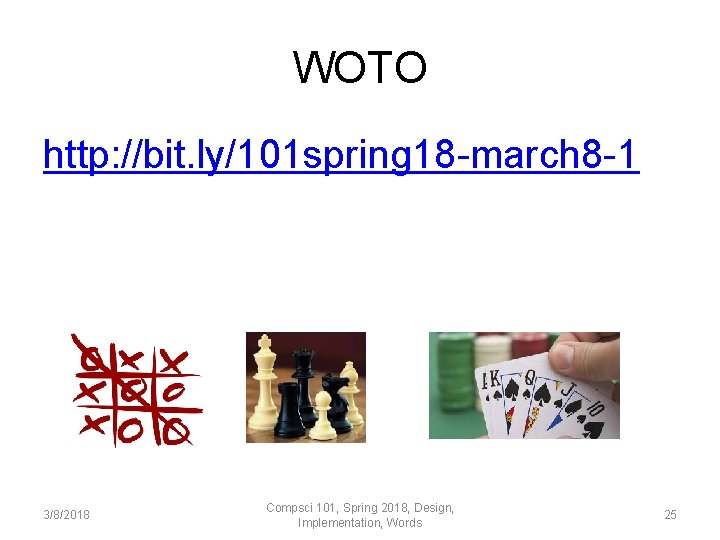
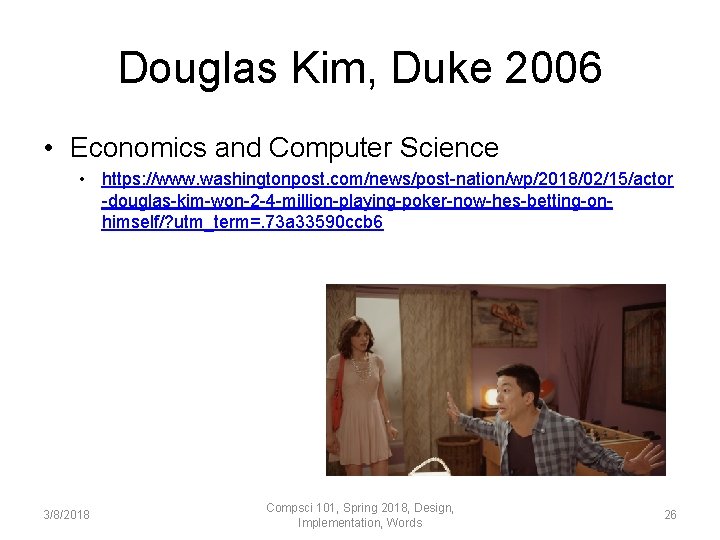
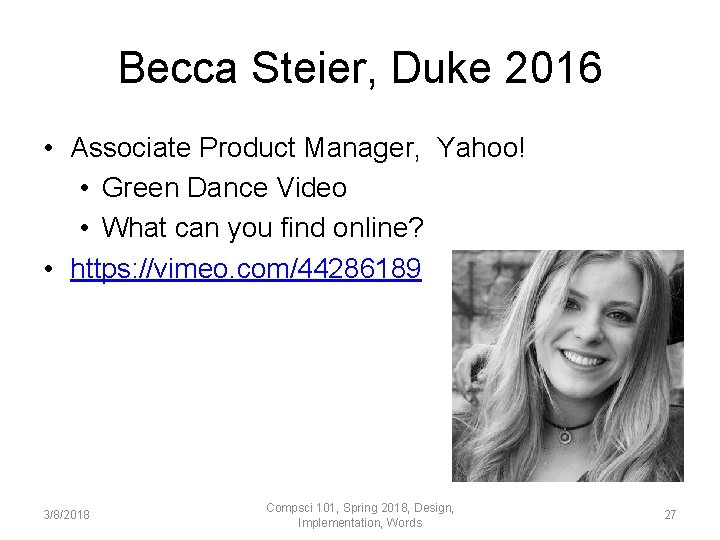
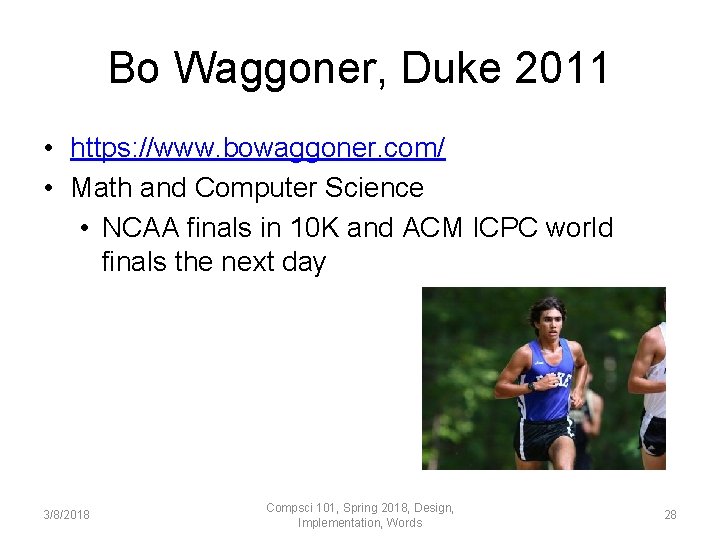
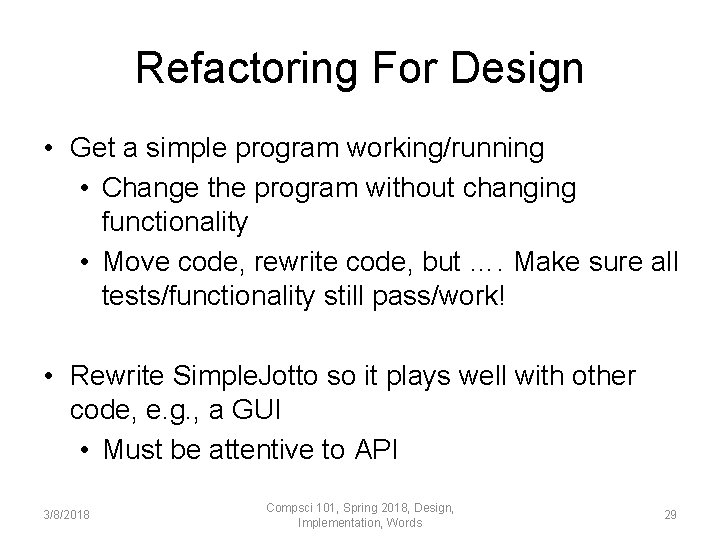
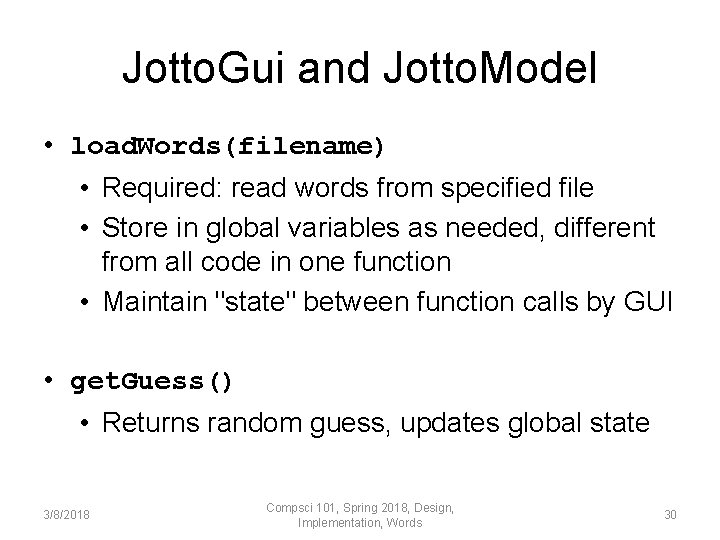
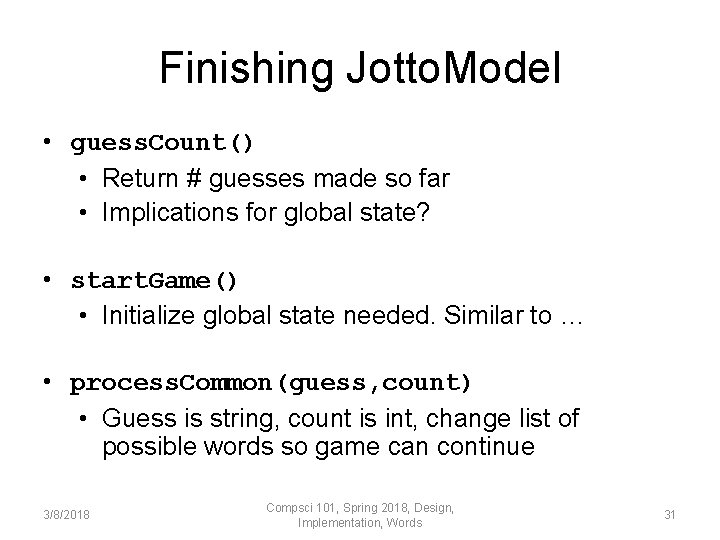
- Slides: 31
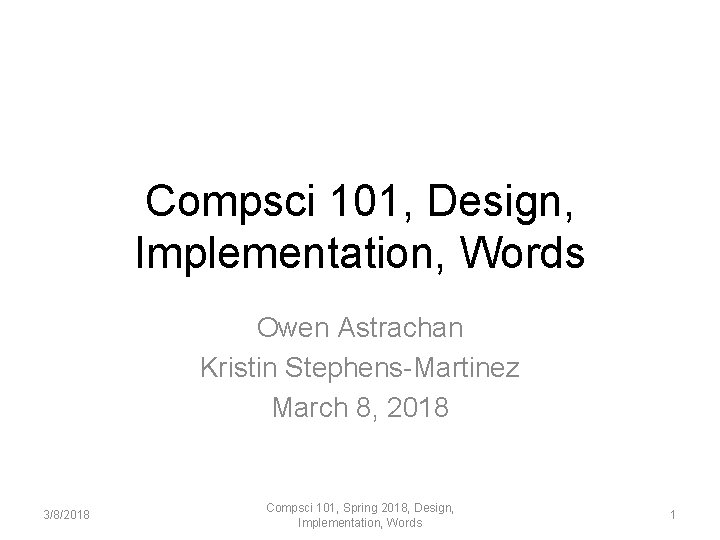
Compsci 101, Design, Implementation, Words Owen Astrachan Kristin Stephens-Martinez March 8, 2018 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 1
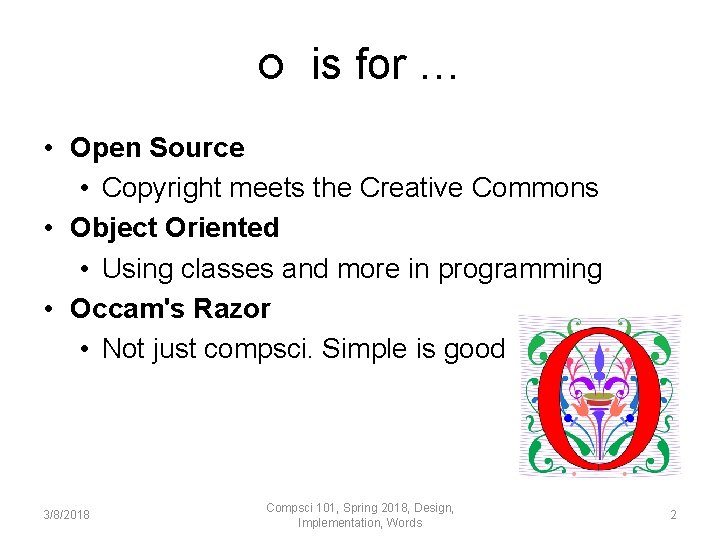
O is for … • Open Source • Copyright meets the Creative Commons • Object Oriented • Using classes and more in programming • Occam's Razor • Not just compsci. Simple is good 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 2
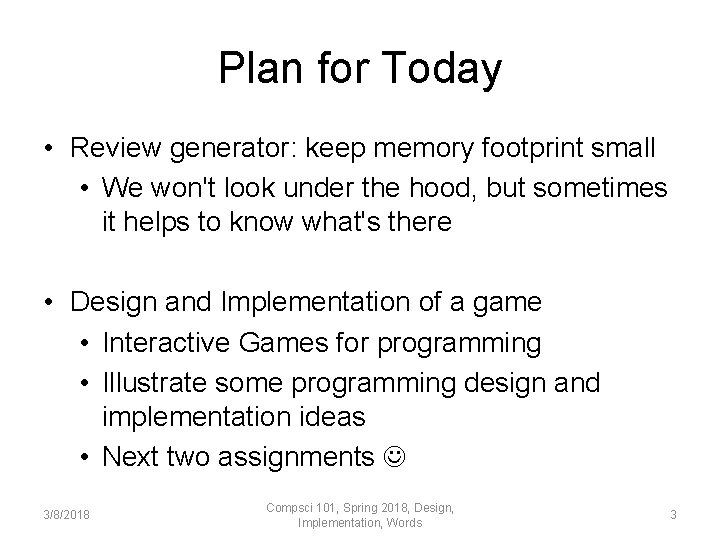
Plan for Today • Review generator: keep memory footprint small • We won't look under the hood, but sometimes it helps to know what's there • Design and Implementation of a game • Interactive Games for programming • Illustrate some programming design and implementation ideas • Next two assignments 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 3
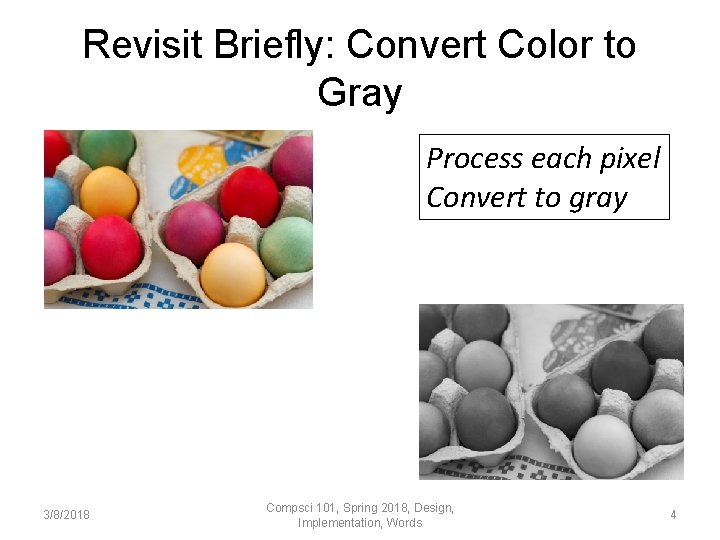
Revisit Briefly: Convert Color to Gray Process each pixel Convert to gray 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 4
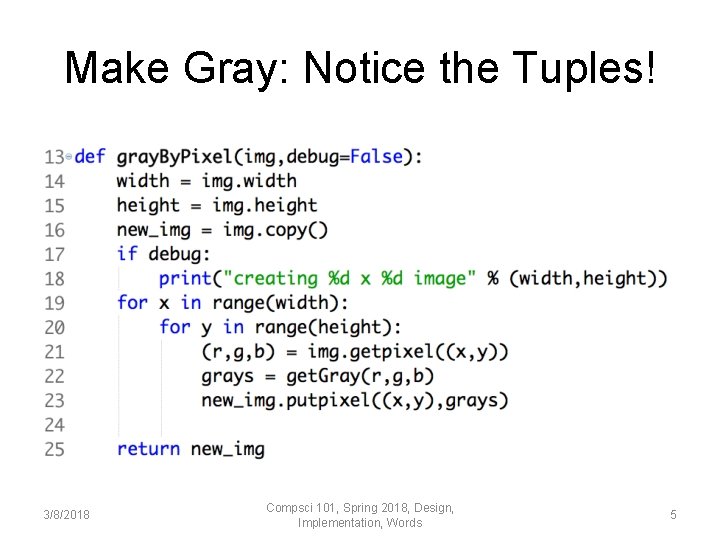
Make Gray: Notice the Tuples! 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 5
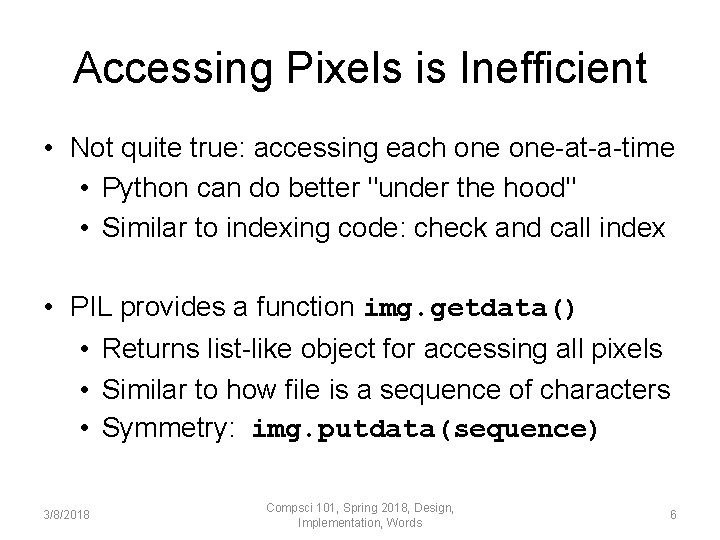
Accessing Pixels is Inefficient • Not quite true: accessing each one-at-a-time • Python can do better "under the hood" • Similar to indexing code: check and call index • PIL provides a function img. getdata() • Returns list-like object for accessing all pixels • Similar to how file is a sequence of characters • Symmetry: img. putdata(sequence) 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 6
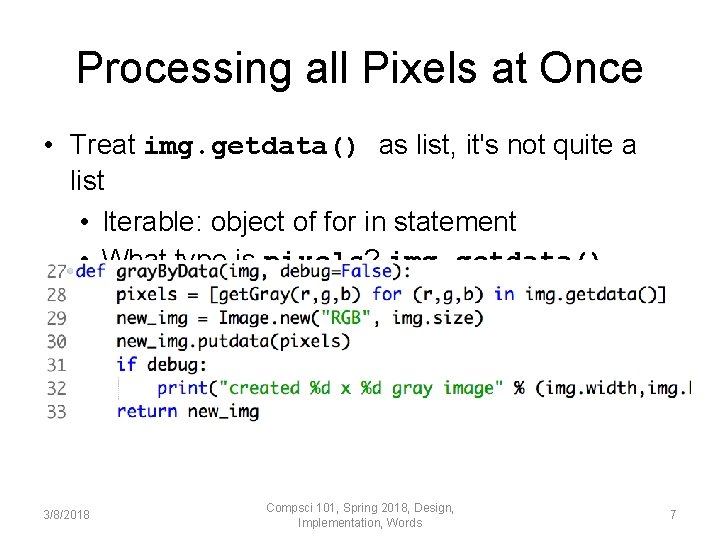
Processing all Pixels at Once • Treat img. getdata() as list, it's not quite a list • Iterable: object of for in statement • What type is pixels? img. getdata() 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 7
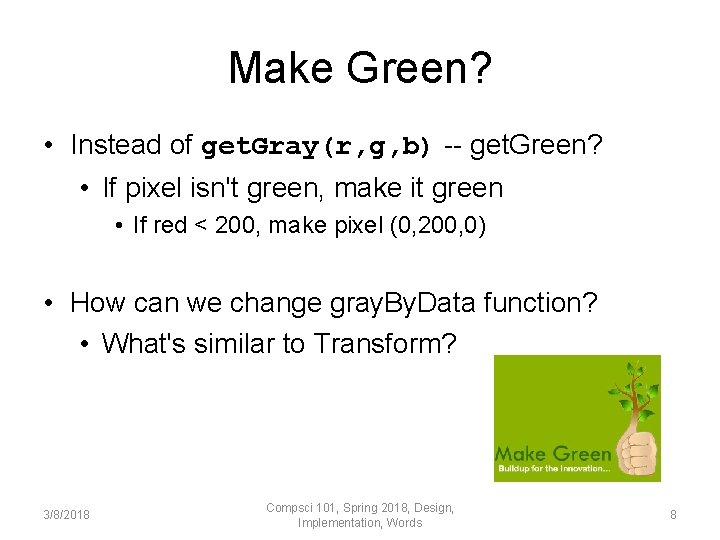
Make Green? • Instead of get. Gray(r, g, b) -- get. Green? • If pixel isn't green, make it green • If red < 200, make pixel (0, 200, 0) • How can we change gray. By. Data function? • What's similar to Transform? 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 8
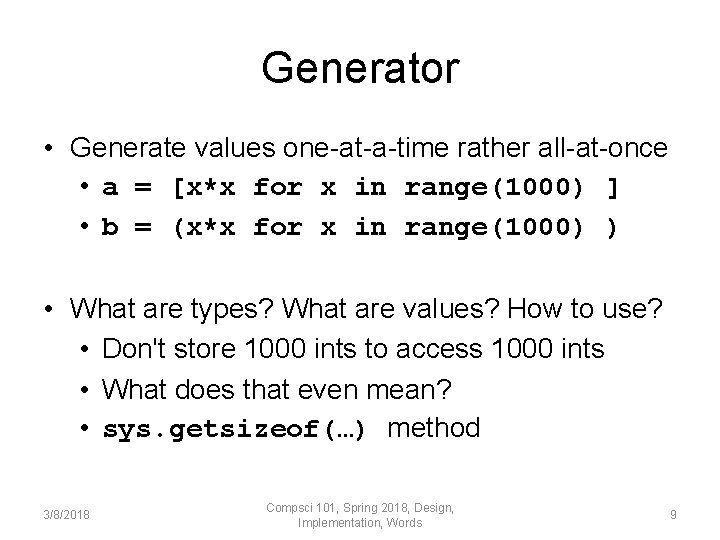
Generator • Generate values one-at-a-time rather all-at-once • a = [x*x for x in range(1000) ] • b = (x*x for x in range(1000) ) • What are types? What are values? How to use? • Don't store 1000 ints to access 1000 ints • What does that even mean? • sys. getsizeof(…) method 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 9
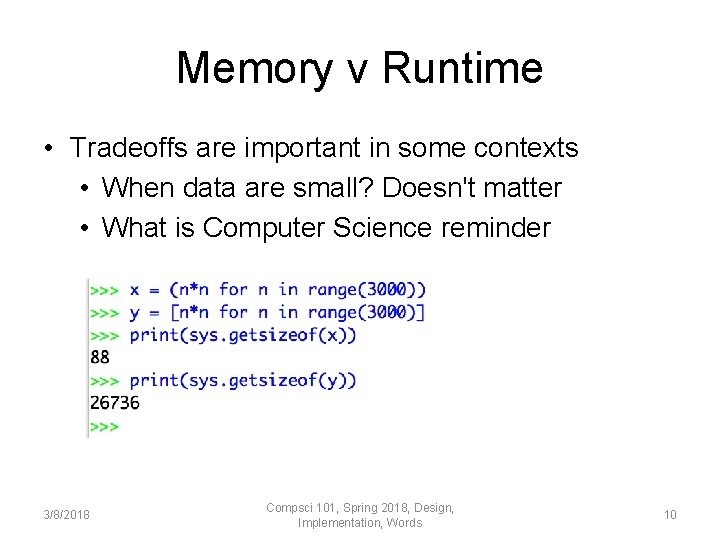
Memory v Runtime • Tradeoffs are important in some contexts • When data are small? Doesn't matter • What is Computer Science reminder 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 10
![Comprehensions List comprehension w for w in words if lenw 20 Comprehensions • List comprehension • [w for w in words if len(w) > 20]](https://slidetodoc.com/presentation_image_h/2e51bd97cf411d2c0e341234c080f818/image-11.jpg)
Comprehensions • List comprehension • [w for w in words if len(w) > 20] • Set comprehension • {w for w in words if len(w) > 20} • Generator • (w for w in words if len(w) > 20) 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 11
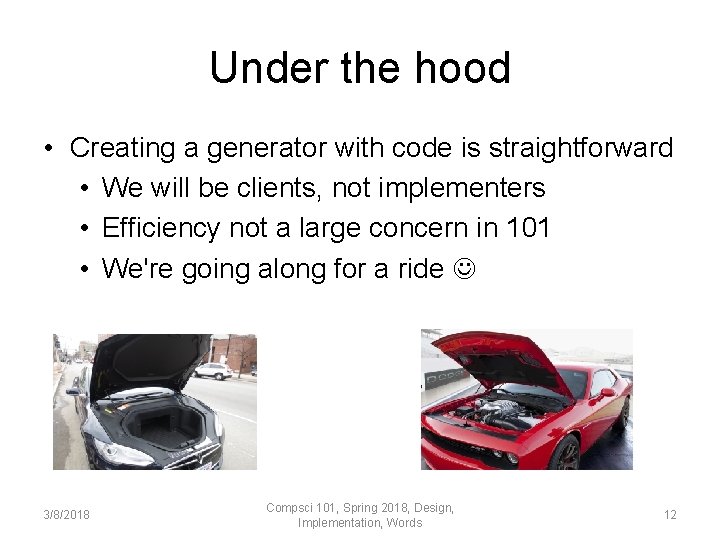
Under the hood • Creating a generator with code is straightforward • We will be clients, not implementers • Efficiency not a large concern in 101 • We're going along for a ride 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 12
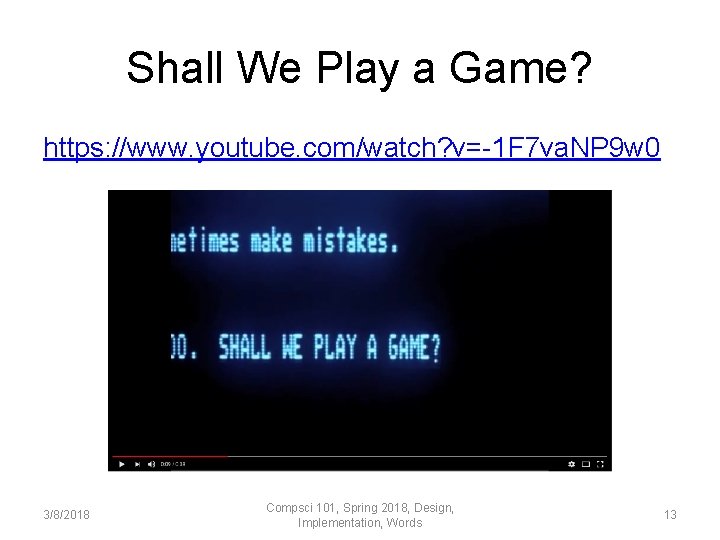
Shall We Play a Game? https: //www. youtube. com/watch? v=-1 F 7 va. NP 9 w 0 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 13
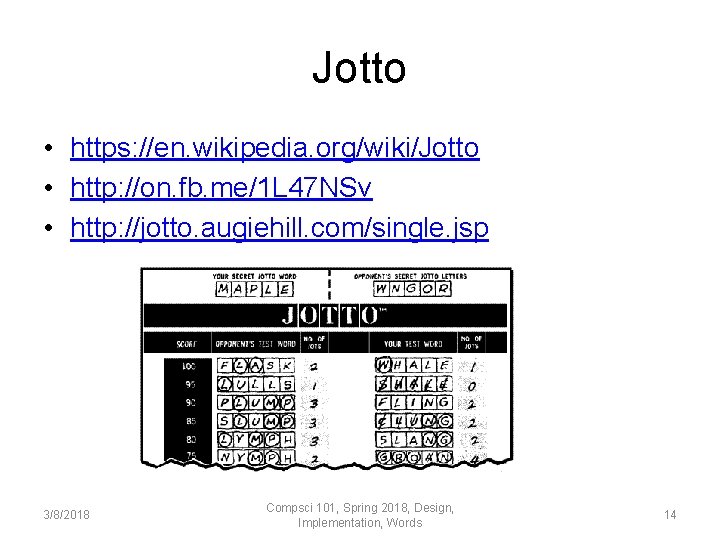
Jotto • https: //en. wikipedia. org/wiki/Jotto • http: //on. fb. me/1 L 47 NSv • http: //jotto. augiehill. com/single. jsp 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 14
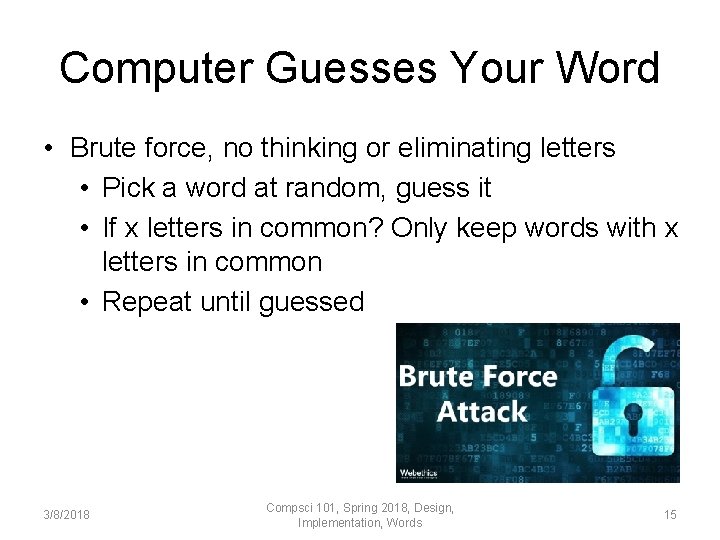
Computer Guesses Your Word • Brute force, no thinking or eliminating letters • Pick a word at random, guess it • If x letters in common? Only keep words with x letters in common • Repeat until guessed 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 15
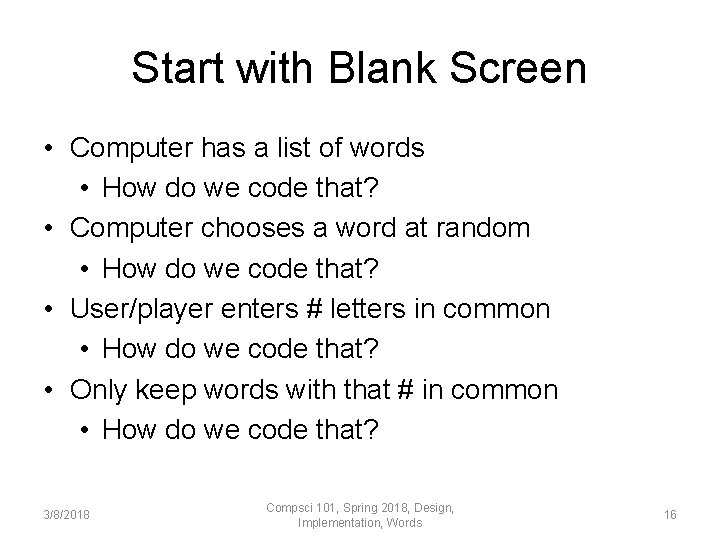
Start with Blank Screen • Computer has a list of words • How do we code that? • Computer chooses a word at random • How do we code that? • User/player enters # letters in common • How do we code that? • Only keep words with that # in common • How do we code that? 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 16
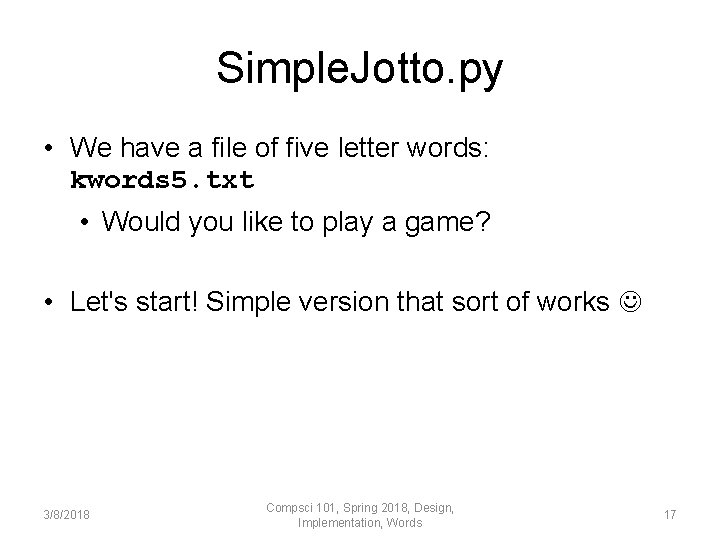
Simple. Jotto. py • We have a file of five letter words: kwords 5. txt • Would you like to play a game? • Let's start! Simple version that sort of works 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 17
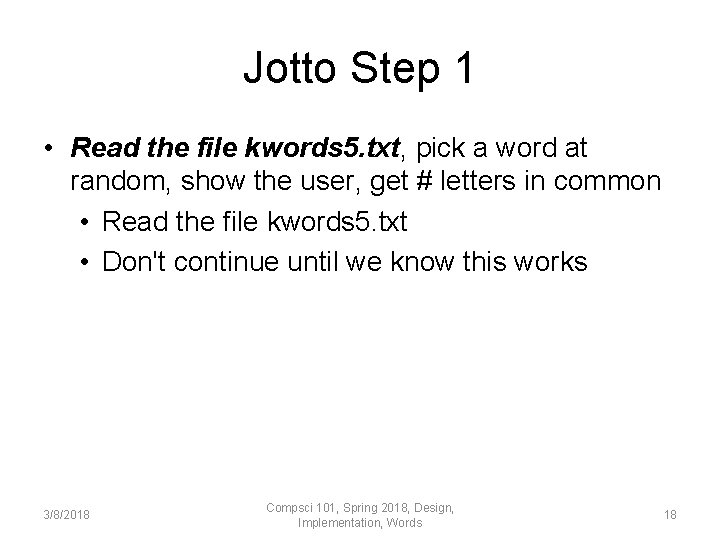
Jotto Step 1 • Read the file kwords 5. txt, pick a word at random, show the user, get # letters in common • Read the file kwords 5. txt • Don't continue until we know this works 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 18
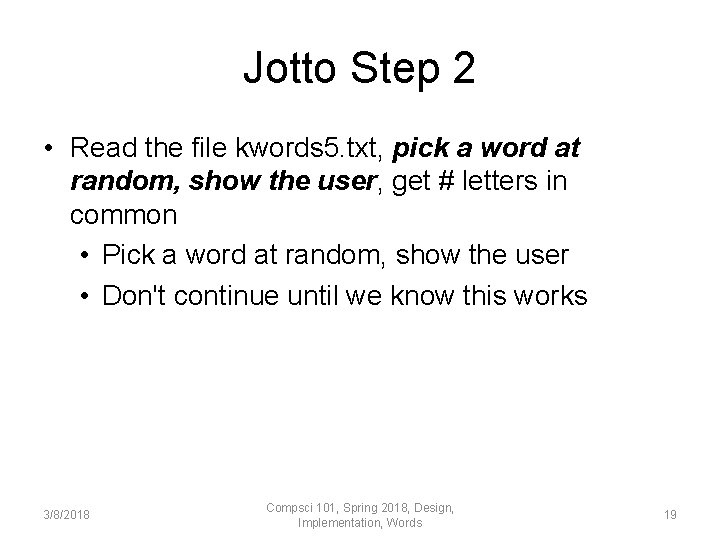
Jotto Step 2 • Read the file kwords 5. txt, pick a word at random, show the user, get # letters in common • Pick a word at random, show the user • Don't continue until we know this works 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 19
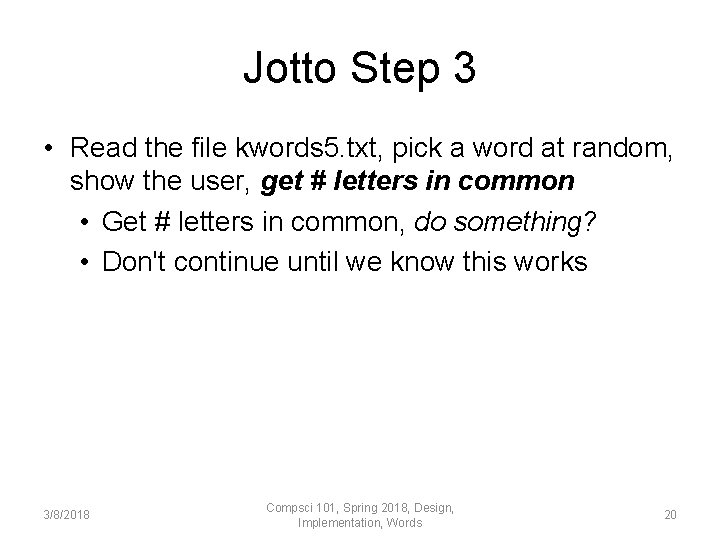
Jotto Step 3 • Read the file kwords 5. txt, pick a word at random, show the user, get # letters in common • Get # letters in common, do something? • Don't continue until we know this works 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 20
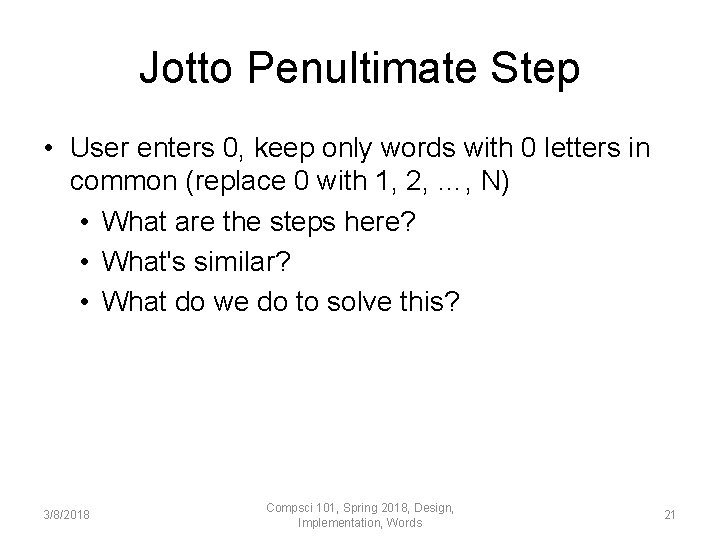
Jotto Penultimate Step • User enters 0, keep only words with 0 letters in common (replace 0 with 1, 2, …, N) • What are the steps here? • What's similar? • What do we do to solve this? 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 21
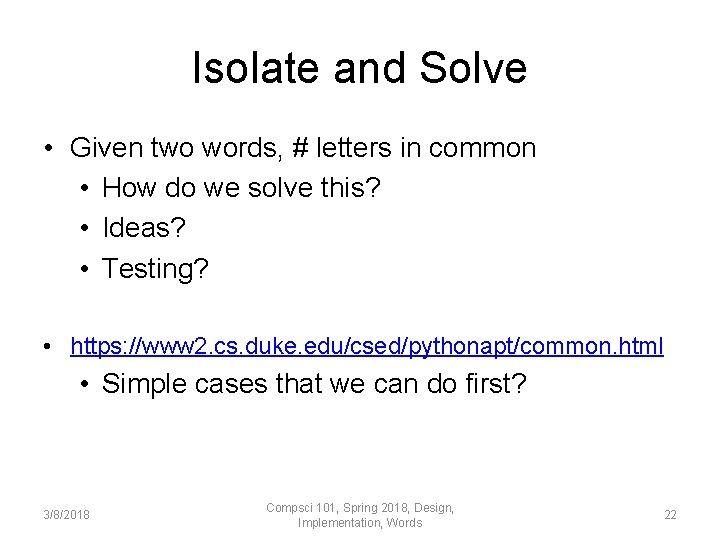
Isolate and Solve • Given two words, # letters in common • How do we solve this? • Ideas? • Testing? • https: //www 2. cs. duke. edu/csed/pythonapt/common. html • Simple cases that we can do first? 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 22
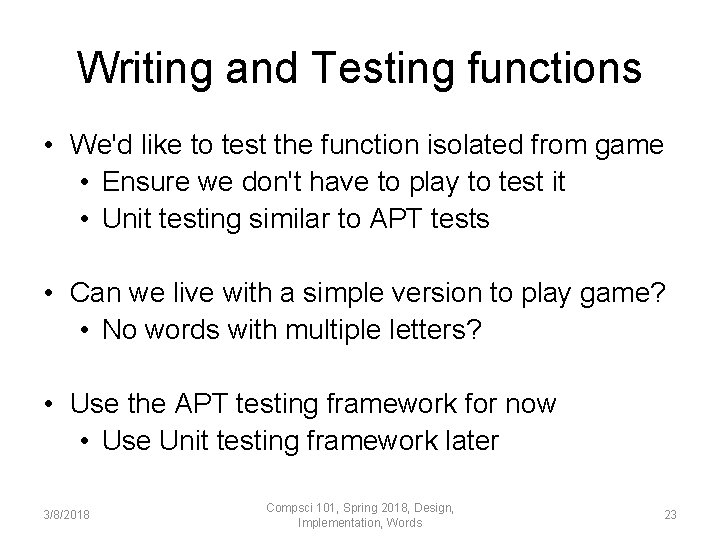
Writing and Testing functions • We'd like to test the function isolated from game • Ensure we don't have to play to test it • Unit testing similar to APT tests • Can we live with a simple version to play game? • No words with multiple letters? • Use the APT testing framework for now • Use Unit testing framework later 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 23
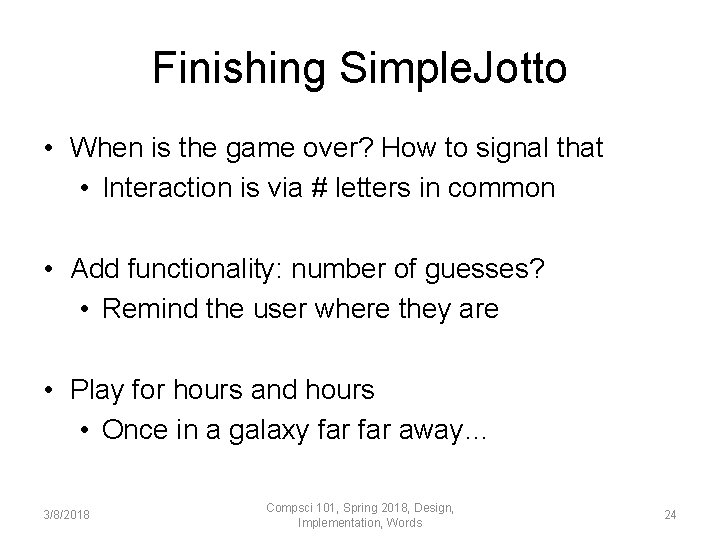
Finishing Simple. Jotto • When is the game over? How to signal that • Interaction is via # letters in common • Add functionality: number of guesses? • Remind the user where they are • Play for hours and hours • Once in a galaxy far away… 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 24
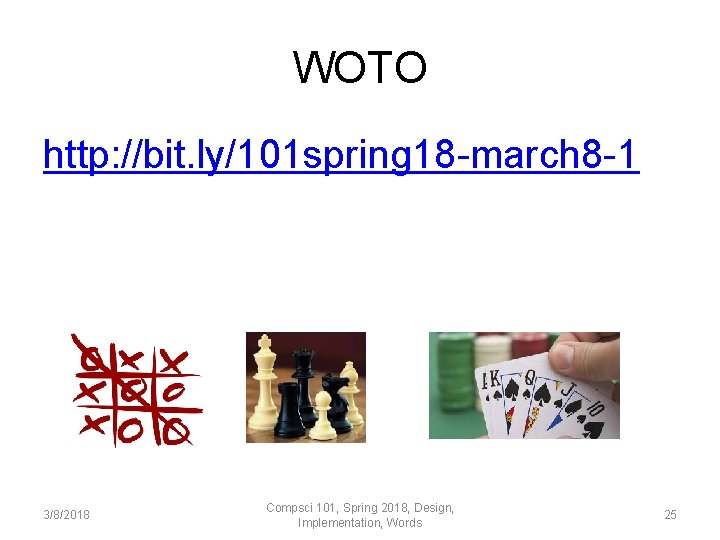
WOTO http: //bit. ly/101 spring 18 -march 8 -1 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 25
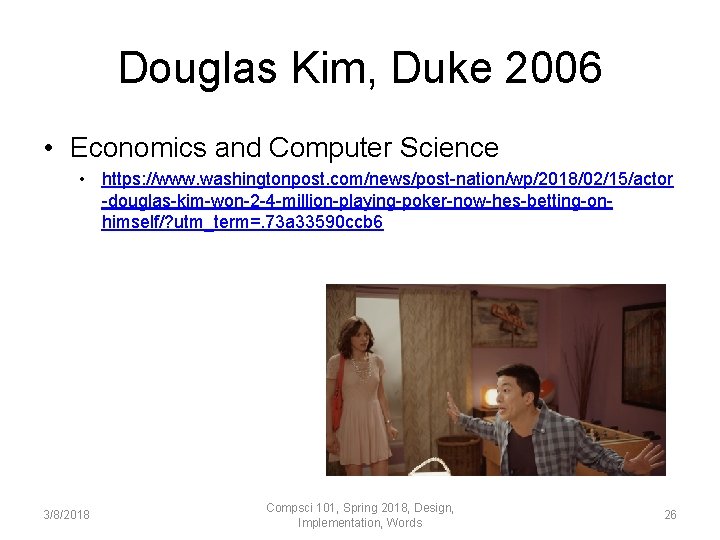
Douglas Kim, Duke 2006 • Economics and Computer Science • https: //www. washingtonpost. com/news/post-nation/wp/2018/02/15/actor -douglas-kim-won-2 -4 -million-playing-poker-now-hes-betting-onhimself/? utm_term=. 73 a 33590 ccb 6 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 26
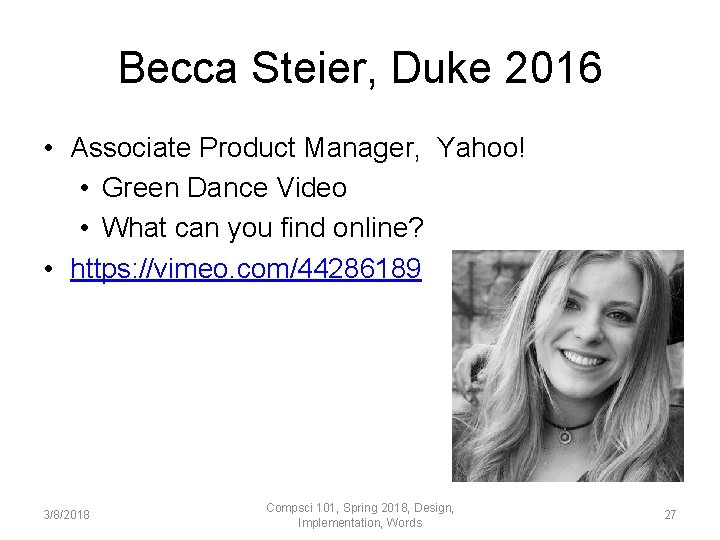
Becca Steier, Duke 2016 • Associate Product Manager, Yahoo! • Green Dance Video • What can you find online? • https: //vimeo. com/44286189 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 27
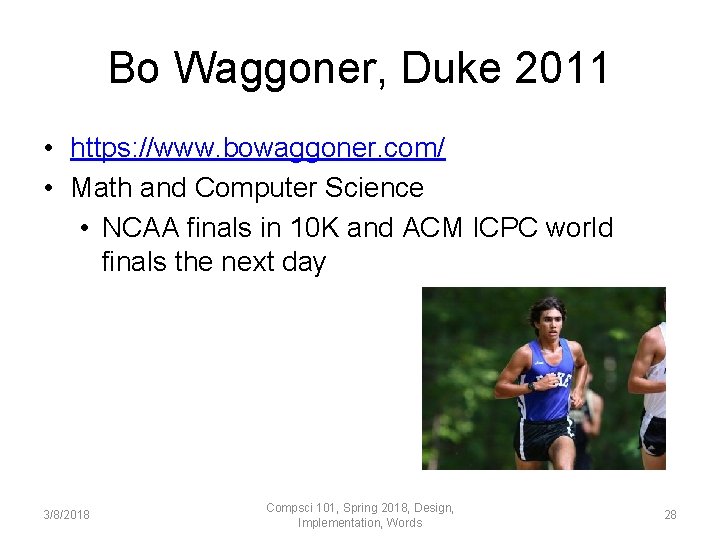
Bo Waggoner, Duke 2011 • https: //www. bowaggoner. com/ • Math and Computer Science • NCAA finals in 10 K and ACM ICPC world finals the next day 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 28
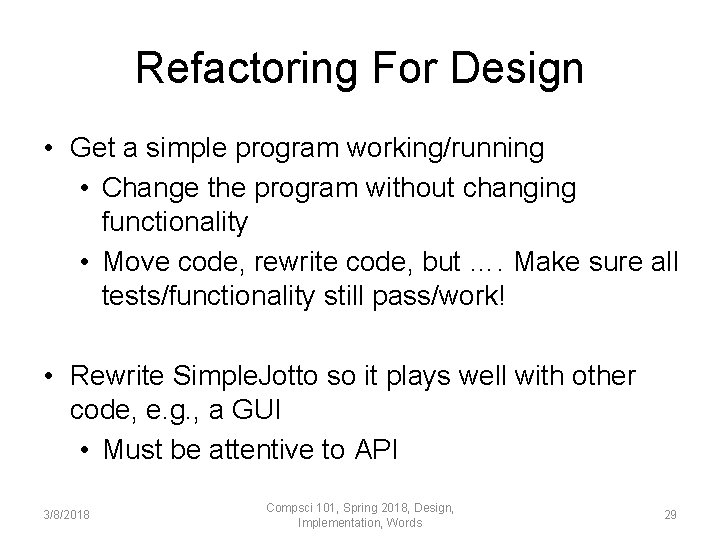
Refactoring For Design • Get a simple program working/running • Change the program without changing functionality • Move code, rewrite code, but …. Make sure all tests/functionality still pass/work! • Rewrite Simple. Jotto so it plays well with other code, e. g. , a GUI • Must be attentive to API 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 29
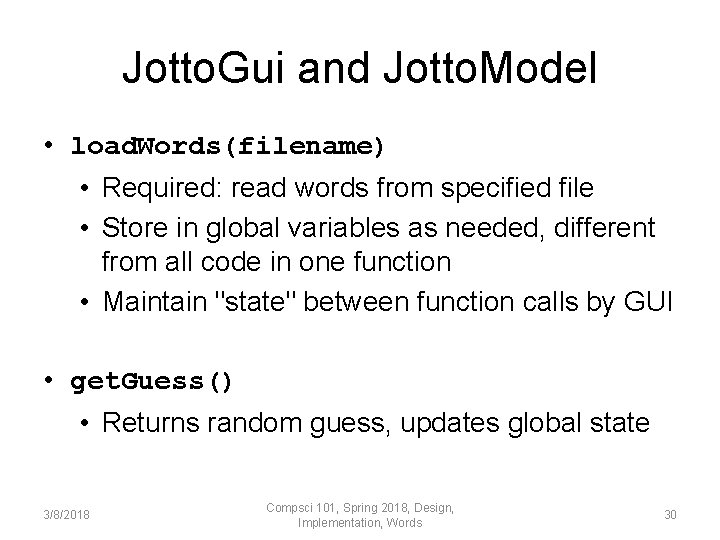
Jotto. Gui and Jotto. Model • load. Words(filename) • Required: read words from specified file • Store in global variables as needed, different from all code in one function • Maintain "state" between function calls by GUI • get. Guess() • Returns random guess, updates global state 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 30
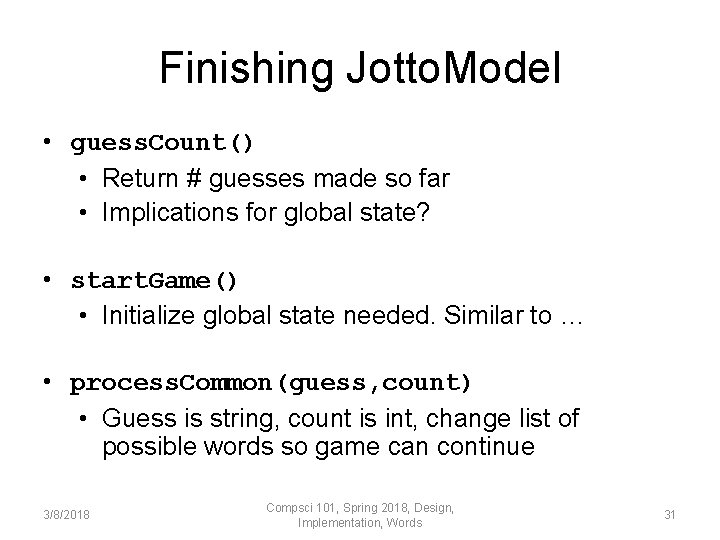
Finishing Jotto. Model • guess. Count() • Return # guesses made so far • Implications for global state? • start. Game() • Initialize global state needed. Similar to … • process. Common(guess, count) • Guess is string, count is int, change list of possible words so game can continue 3/8/2018 Compsci 101, Spring 2018, Design, Implementation, Words 31