Composite Pattern Code Jim Fawcett CSE 776 Design
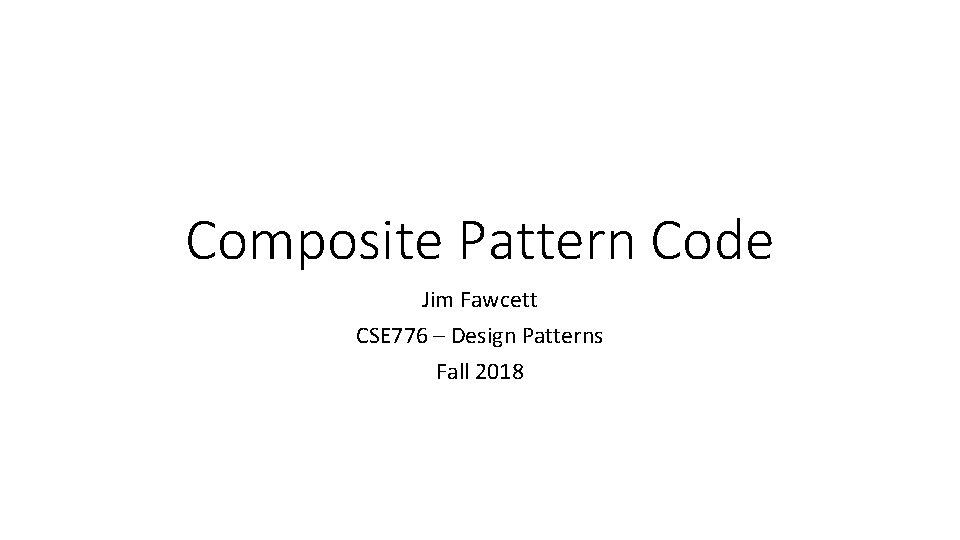
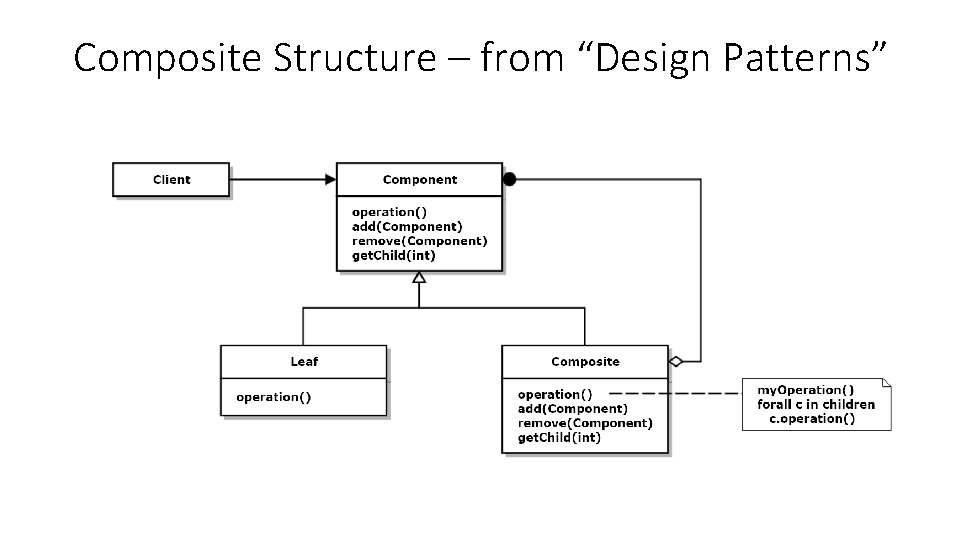
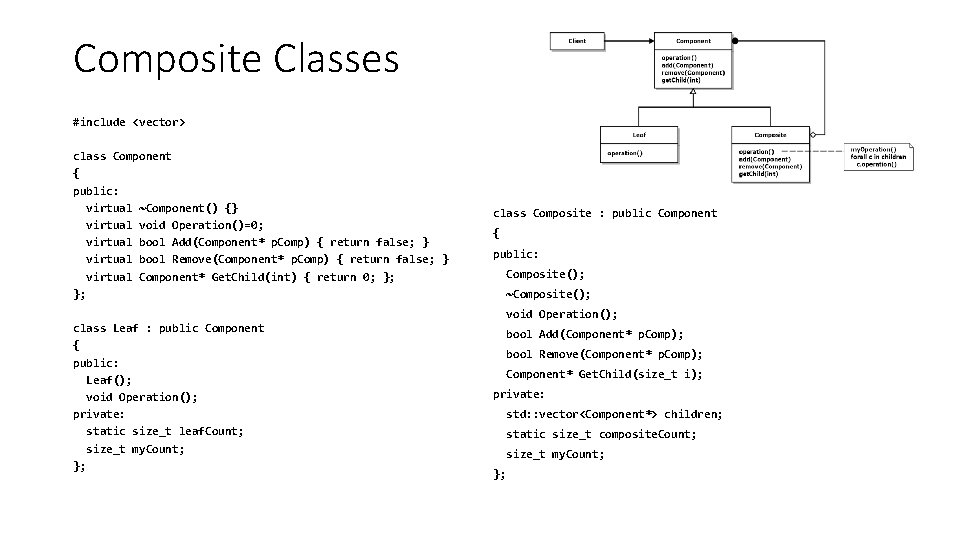
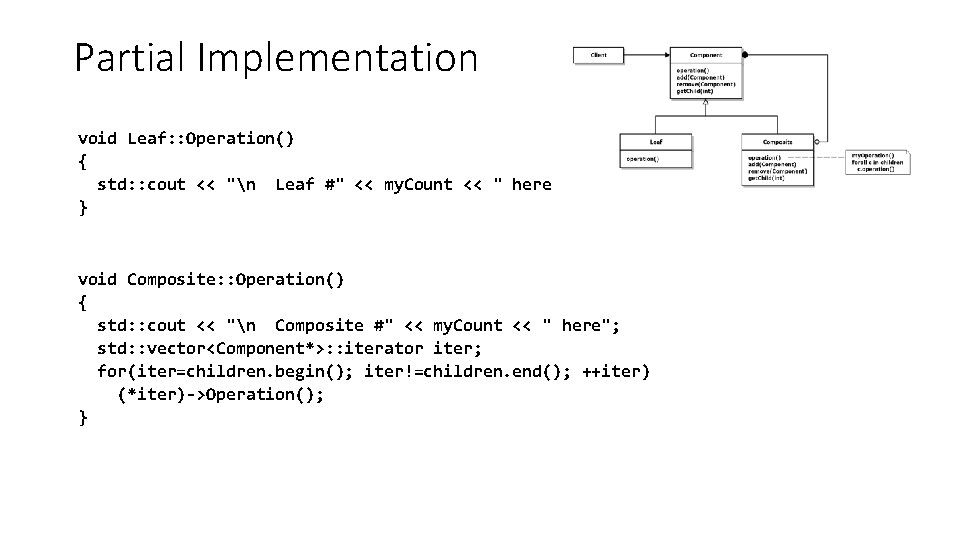
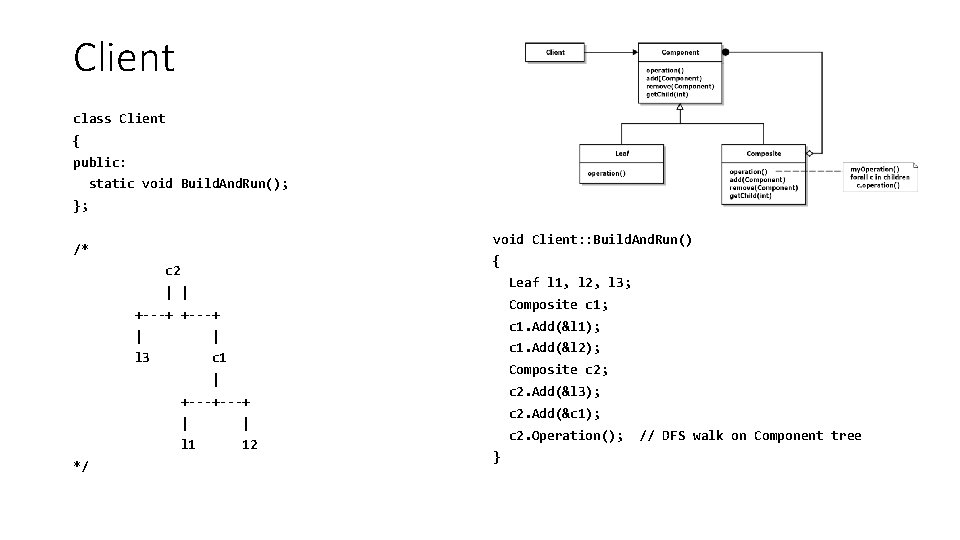
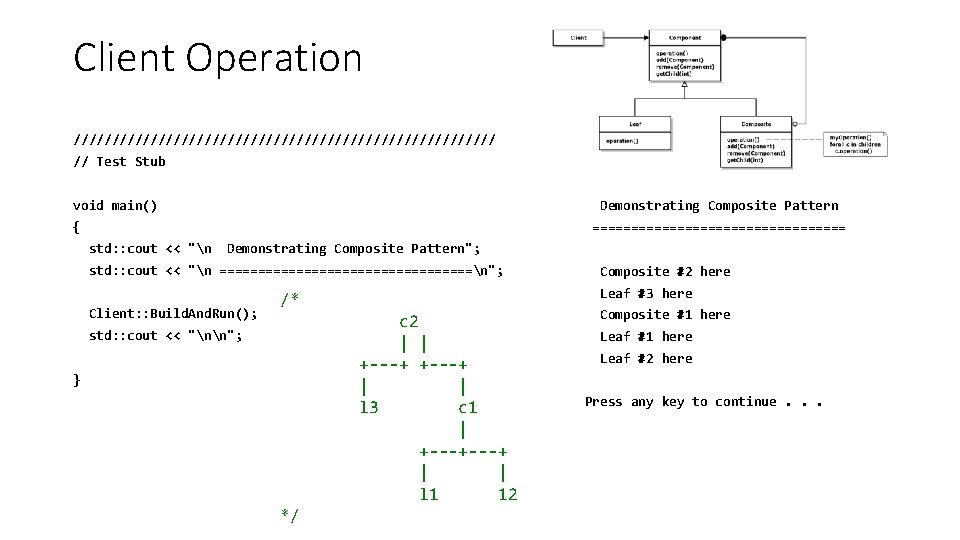
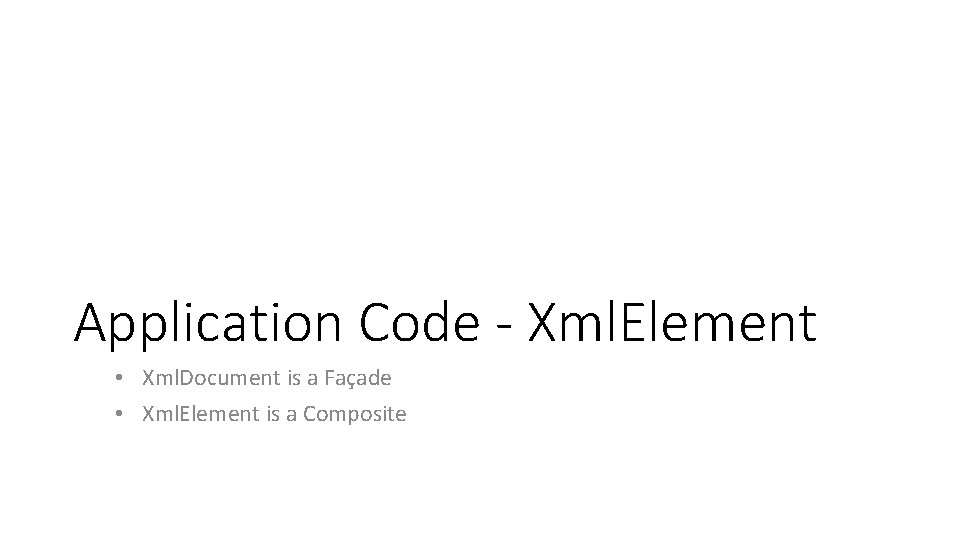
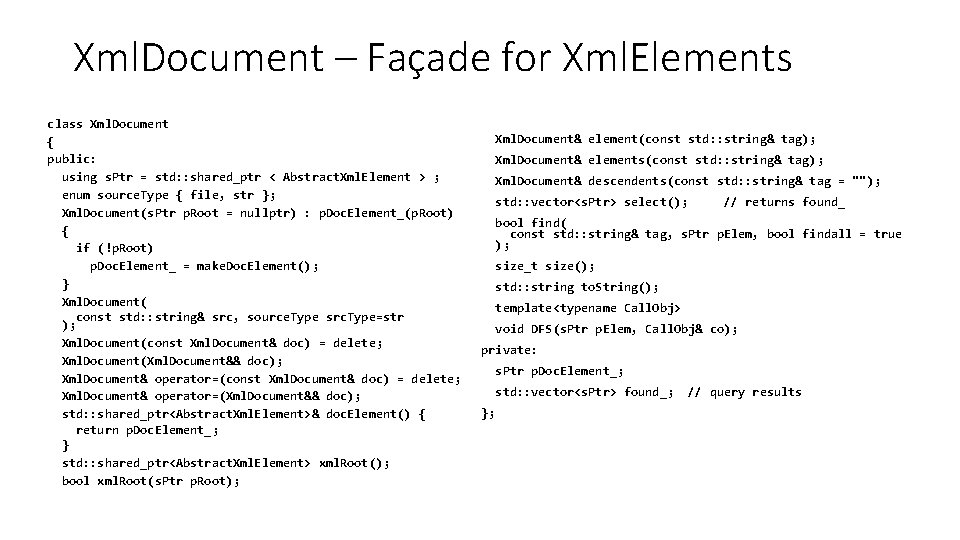
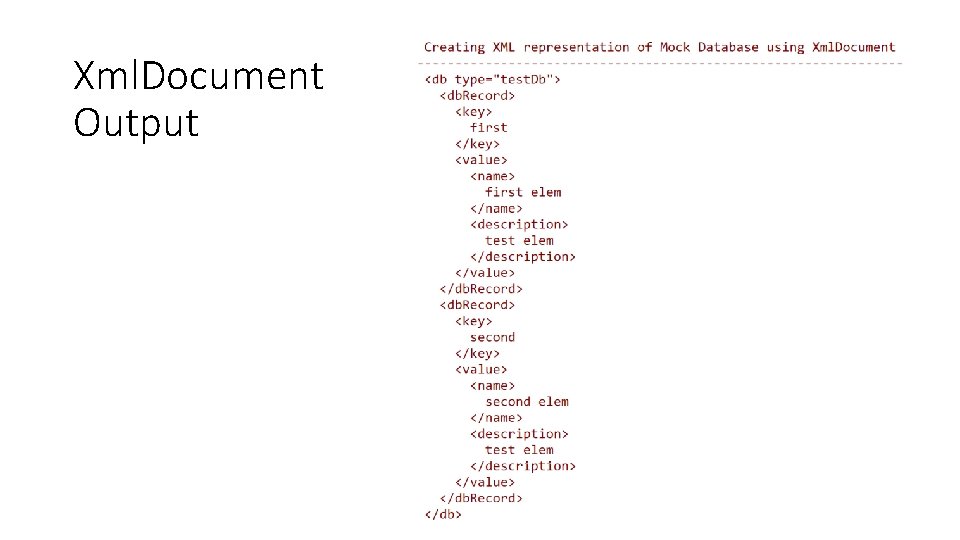
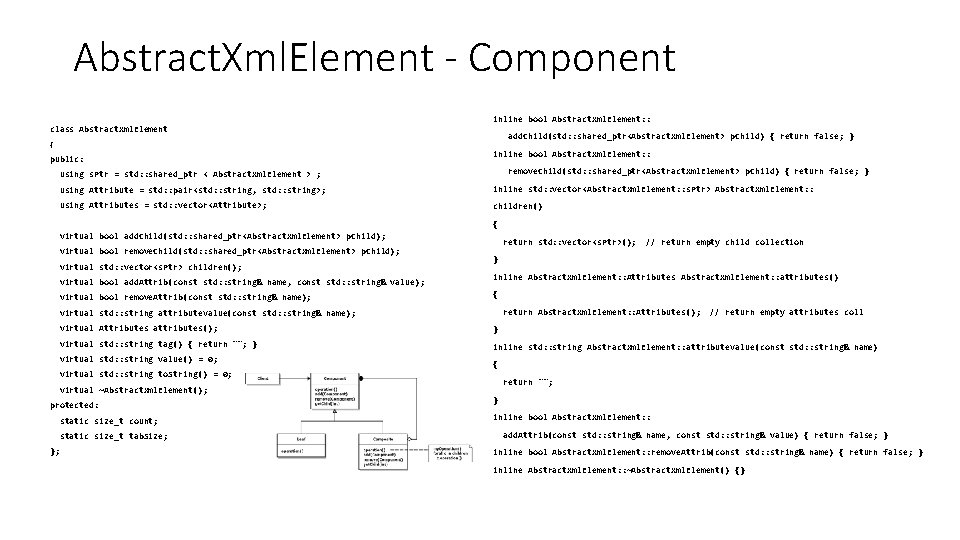
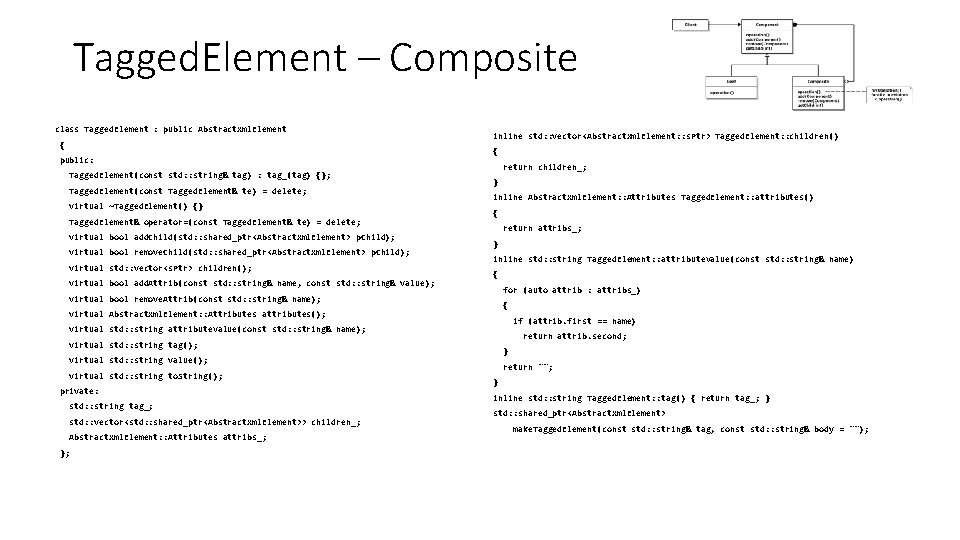
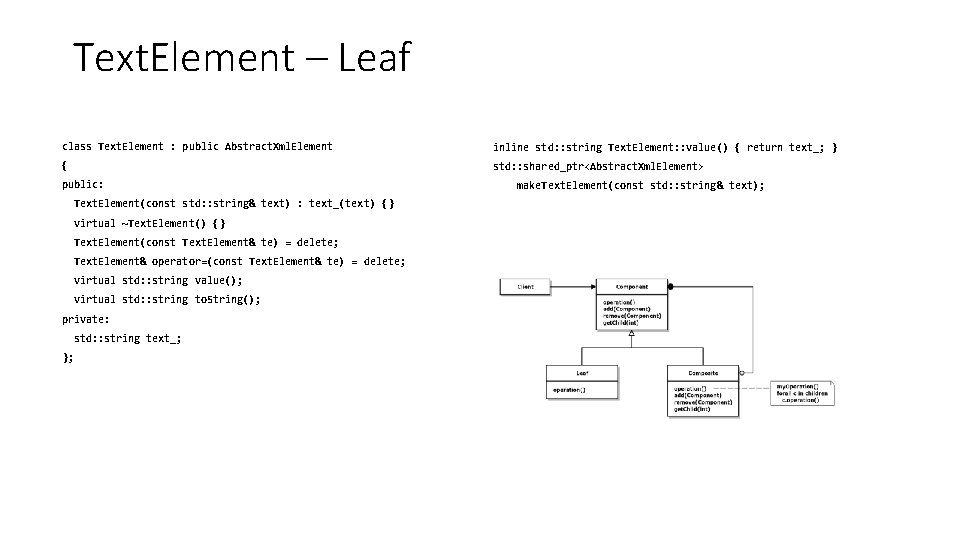
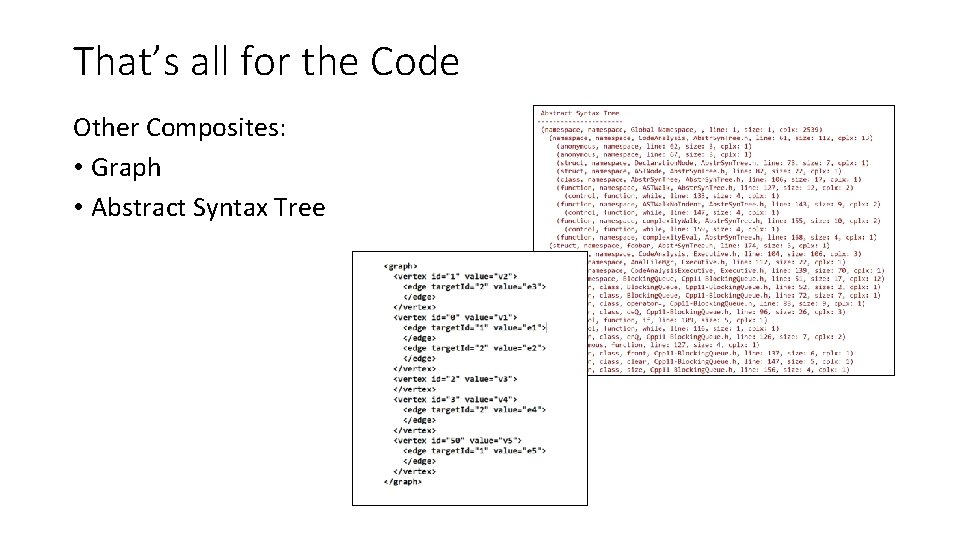
- Slides: 13
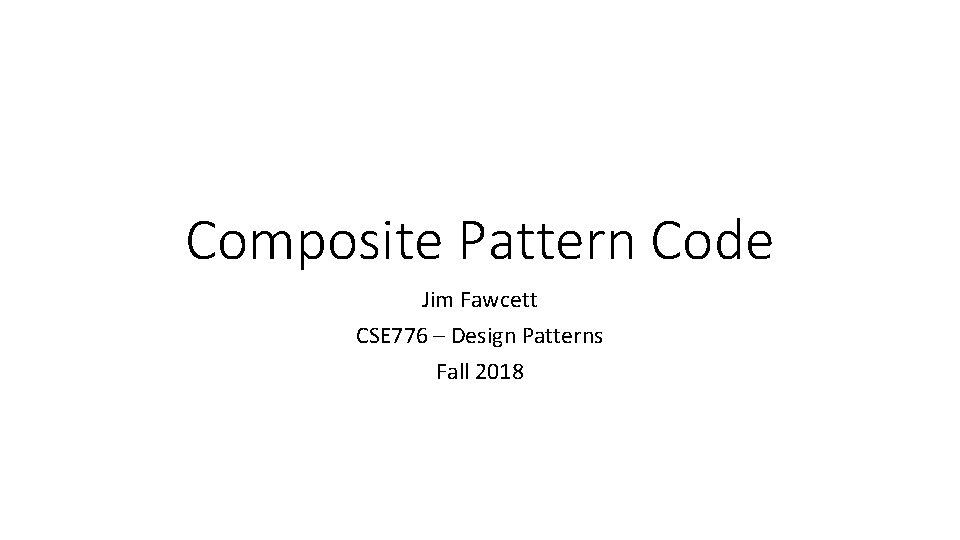
Composite Pattern Code Jim Fawcett CSE 776 – Design Patterns Fall 2018
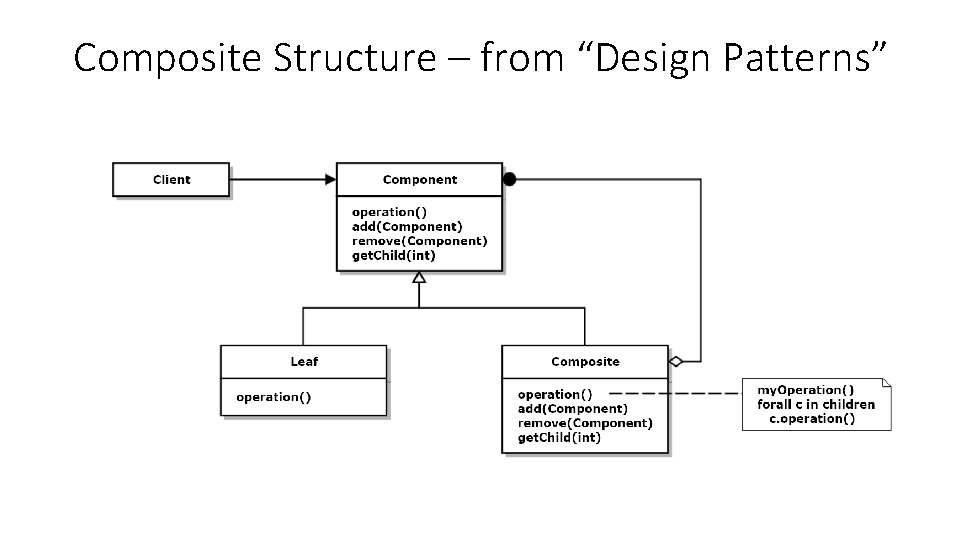
Composite Structure – from “Design Patterns”
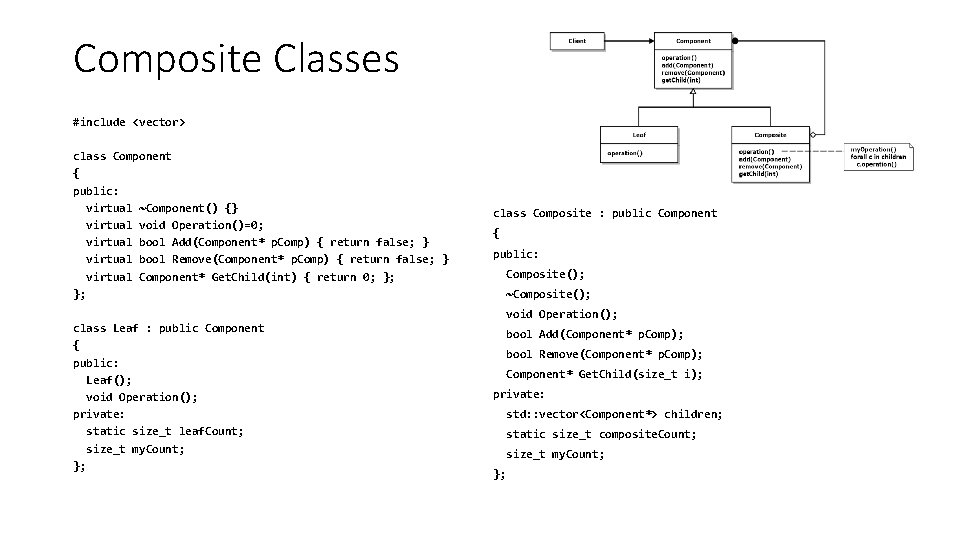
Composite Classes #include <vector> class Component { public: virtual ~Component() {} virtual void Operation()=0; virtual bool Add(Component* p. Comp) { return false; } virtual bool Remove(Component* p. Comp) { return false; } virtual Component* Get. Child(int) { return 0; }; }; class Composite : public Component { public: Composite(); ~Composite(); void Operation(); class Leaf : public Component { public: Leaf(); void Operation(); private: static size_t leaf. Count; size_t my. Count; }; bool Add(Component* p. Comp); bool Remove(Component* p. Comp); Component* Get. Child(size_t i); private: std: : vector<Component*> children; static size_t composite. Count; size_t my. Count; };
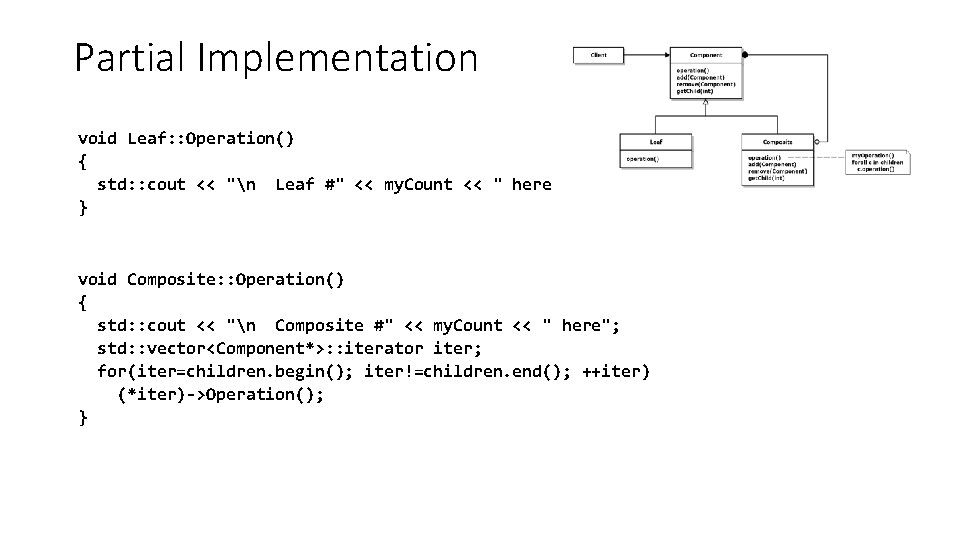
Partial Implementation void Leaf: : Operation() { std: : cout << "n Leaf #" << my. Count << " here"; } void Composite: : Operation() { std: : cout << "n Composite #" << my. Count << " here"; std: : vector<Component*>: : iterator iter; for(iter=children. begin(); iter!=children. end(); ++iter) (*iter)->Operation(); }
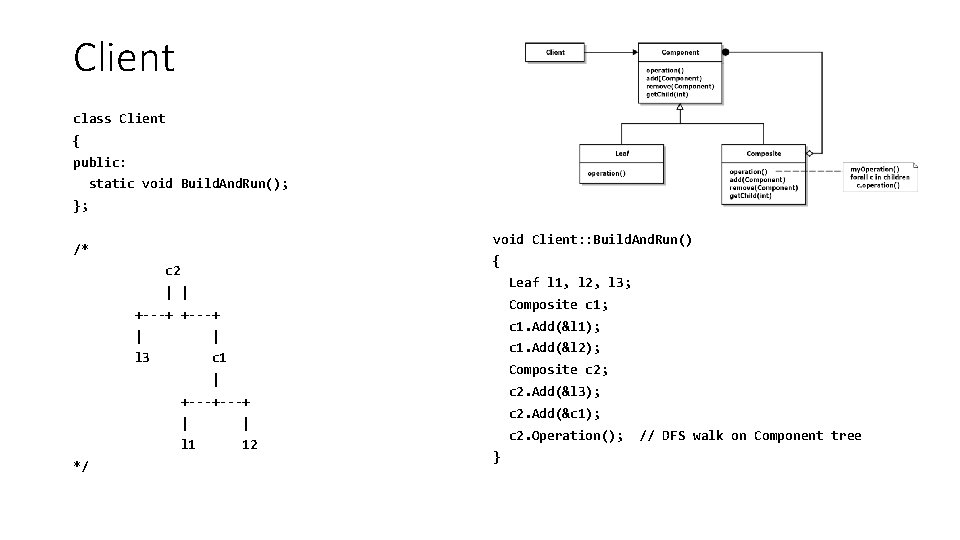
Client class Client { public: static void Build. And. Run(); }; void Client: : Build. And. Run() /* { c 2 Leaf l 1, l 2, l 3; | | Composite c 1; +---+ | | l 3 c 1. Add(&l 1); c 1. Add(&l 2); Composite c 2; | c 2. Add(&l 3); +---+ */ | | l 1 12 c 2. Add(&c 1); c 2. Operation(); } // DFS walk on Component tree
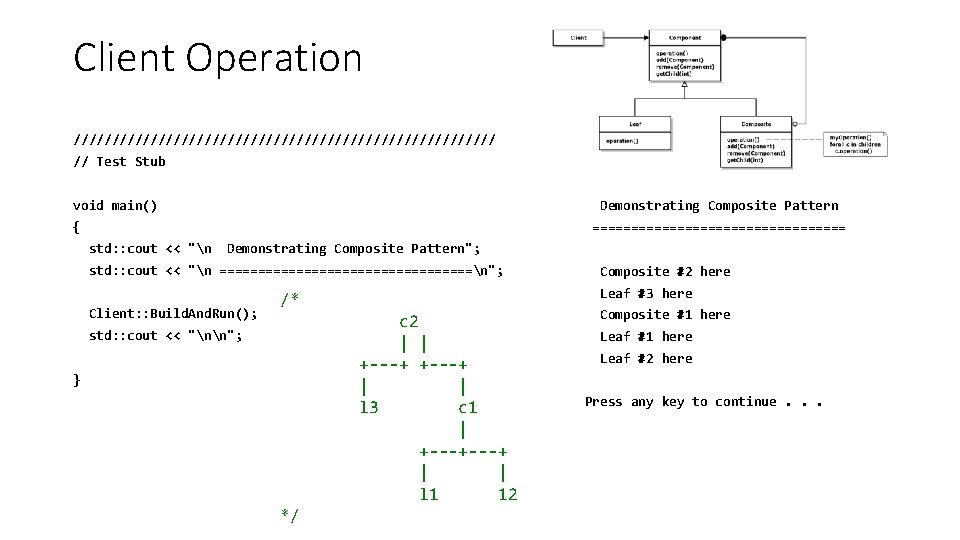
Client Operation //////////////////////////// // Test Stub void main() Demonstrating Composite Pattern { ================= std: : cout << "n Demonstrating Composite Pattern"; std: : cout << "n =================n"; Client: : Build. And. Run(); Leaf #3 here /* c 2 | | +---+ | | l 3 c 1 | +---+ | | l 1 12 std: : cout << "nn"; } */ Composite #2 here Composite #1 here Leaf #2 here Press any key to continue. . .
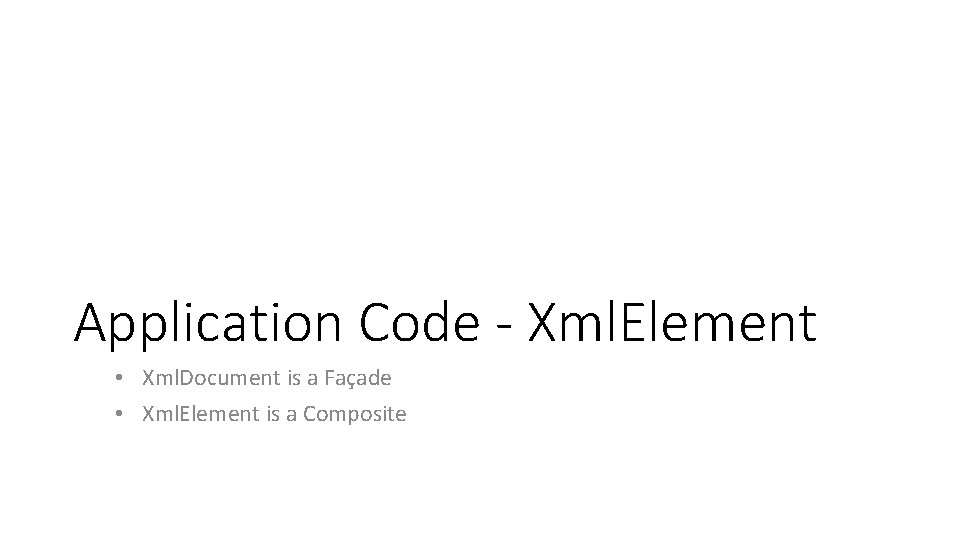
Application Code - Xml. Element • Xml. Document is a Façade • Xml. Element is a Composite
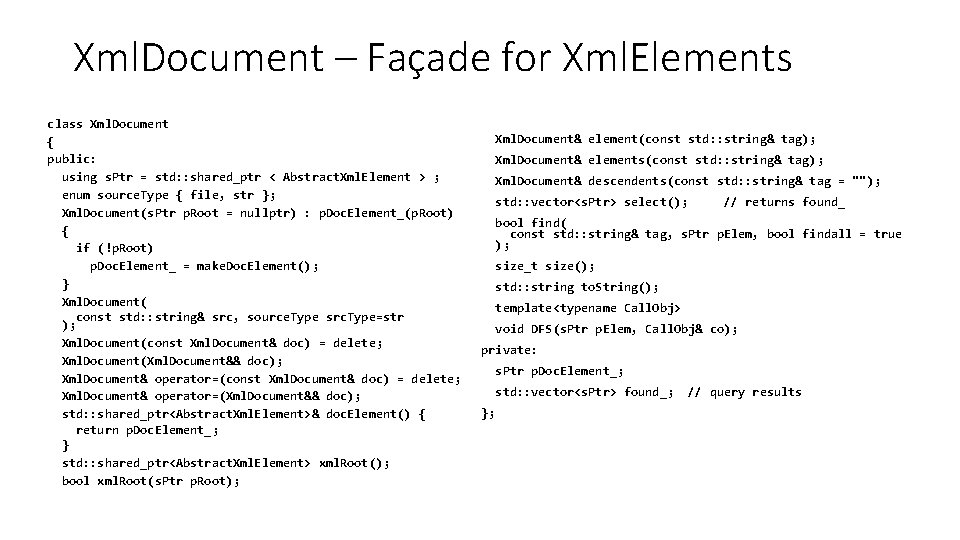
Xml. Document – Façade for Xml. Elements class Xml. Document { public: using s. Ptr = std: : shared_ptr < Abstract. Xml. Element > ; enum source. Type { file, str }; Xml. Document(s. Ptr p. Root = nullptr) : p. Doc. Element_(p. Root) { if (!p. Root) p. Doc. Element_ = make. Doc. Element(); } Xml. Document( const std: : string& src, source. Type src. Type=str ); Xml. Document(const Xml. Document& doc) = delete; Xml. Document(Xml. Document&& doc); Xml. Document& operator=(const Xml. Document& doc) = delete; Xml. Document& operator=(Xml. Document&& doc); std: : shared_ptr<Abstract. Xml. Element>& doc. Element() { return p. Doc. Element_; } std: : shared_ptr<Abstract. Xml. Element> xml. Root(); bool xml. Root(s. Ptr p. Root); Xml. Document& element(const std: : string& tag); Xml. Document& elements(const std: : string& tag); Xml. Document& descendents(const std: : string& tag = ""); std: : vector<s. Ptr> select(); // returns found_ bool find( const std: : string& tag, s. Ptr p. Elem, bool findall = true ); size_t size(); std: : string to. String(); template<typename Call. Obj> void DFS(s. Ptr p. Elem, Call. Obj& co); private: s. Ptr p. Doc. Element_; std: : vector<s. Ptr> found_; }; // query results
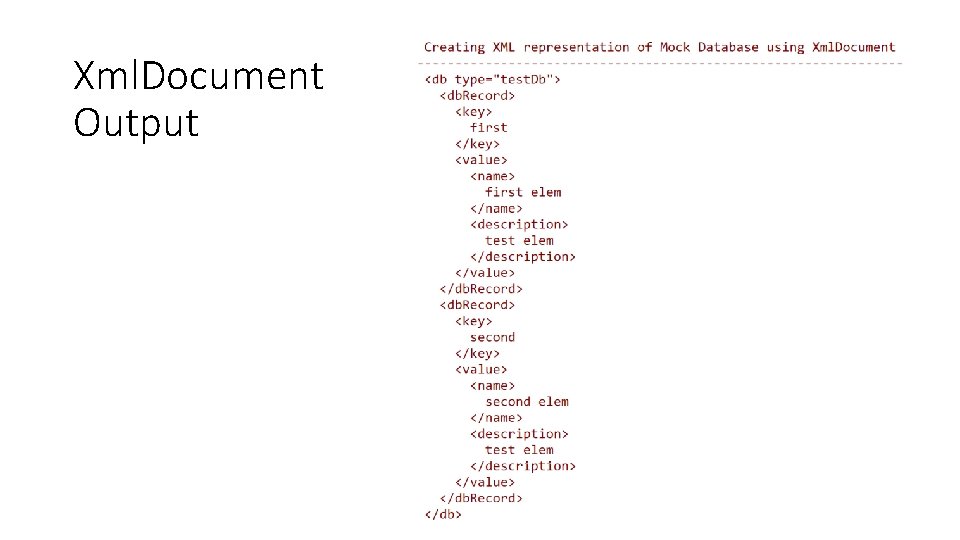
Xml. Document Output
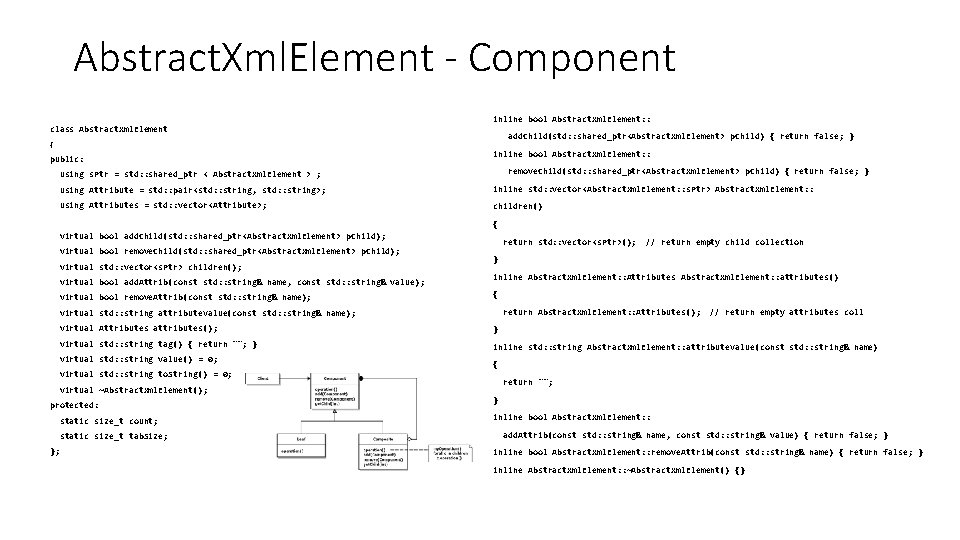
Abstract. Xml. Element - Component inline bool Abstract. Xml. Element: : class Abstract. Xml. Element { public: add. Child(std: : shared_ptr<Abstract. Xml. Element> p. Child) { return false; } inline bool Abstract. Xml. Element: : remove. Child(std: : shared_ptr<Abstract. Xml. Element> p. Child) { return false; } using s. Ptr = std: : shared_ptr < Abstract. Xml. Element > ; using Attribute = std: : pair<std: : string, std: : string>; inline std: : vector<Abstract. Xml. Element: : s. Ptr> Abstract. Xml. Element: : using Attributes = std: : vector<Attribute>; children() { virtual bool add. Child(std: : shared_ptr<Abstract. Xml. Element> p. Child); virtual bool remove. Child(std: : shared_ptr<Abstract. Xml. Element> p. Child); virtual std: : vector<s. Ptr> children(); virtual bool add. Attrib(const std: : string& name, const std: : string& value); virtual bool remove. Attrib(const std: : string& name); return std: : vector<s. Ptr>(); // return empty child collection } inline Abstract. Xml. Element: : Attributes Abstract. Xml. Element: : attributes() { return Abstract. Xml. Element: : Attributes(); virtual std: : string attribute. Value(const std: : string& name); // return empty attributes coll virtual Attributes attributes(); } virtual std: : string tag() { return ""; } inline std: : string Abstract. Xml. Element: : attribute. Value(const std: : string& name) virtual std: : string value() = 0; virtual std: : string to. String() = 0; virtual ~Abstract. Xml. Element(); protected: static size_t count; static size_t tab. Size; }; { return ""; } inline bool Abstract. Xml. Element: : add. Attrib(const std: : string& name, const std: : string& value) { return false; } inline bool Abstract. Xml. Element: : remove. Attrib(const std: : string& name) { return false; } inline Abstract. Xml. Element: : ~Abstract. Xml. Element() {}
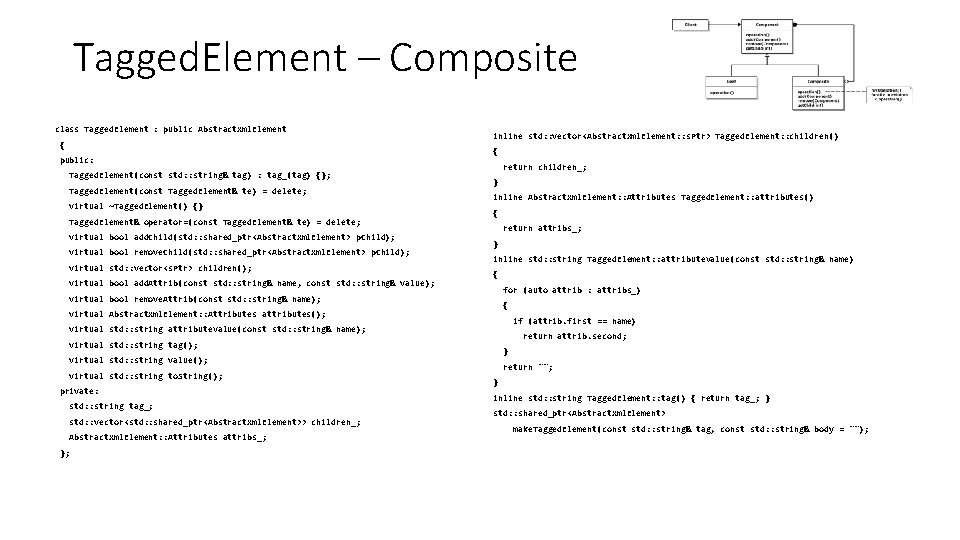
Tagged. Element – Composite class Tagged. Element : public Abstract. Xml. Element { public: Tagged. Element(const std: : string& tag) : tag_(tag) {}; Tagged. Element(const Tagged. Element& te) = delete; virtual ~Tagged. Element() {} Tagged. Element& operator=(const Tagged. Element& te) = delete; virtual bool add. Child(std: : shared_ptr<Abstract. Xml. Element> p. Child); virtual bool remove. Child(std: : shared_ptr<Abstract. Xml. Element> p. Child); virtual std: : vector<s. Ptr> children(); virtual bool add. Attrib(const std: : string& name, const std: : string& value); inline std: : vector<Abstract. Xml. Element: : s. Ptr> Tagged. Element: : children() { return children_; } inline Abstract. Xml. Element: : Attributes Tagged. Element: : attributes() { return attribs_; } inline std: : string Tagged. Element: : attribute. Value(const std: : string& name) { for (auto attrib : attribs_) virtual bool remove. Attrib(const std: : string& name); { virtual Abstract. Xml. Element: : Attributes attributes(); if (attrib. first == name) virtual std: : string attribute. Value(const std: : string& name); return attrib. second; virtual std: : string tag(); } virtual std: : string value(); virtual std: : string to. String(); private: std: : string tag_; std: : vector<std: : shared_ptr<Abstract. Xml. Element>> children_; Abstract. Xml. Element: : Attributes attribs_; }; return ""; } inline std: : string Tagged. Element: : tag() { return tag_; } std: : shared_ptr<Abstract. Xml. Element> make. Tagged. Element(const std: : string& tag, const std: : string& body = "");
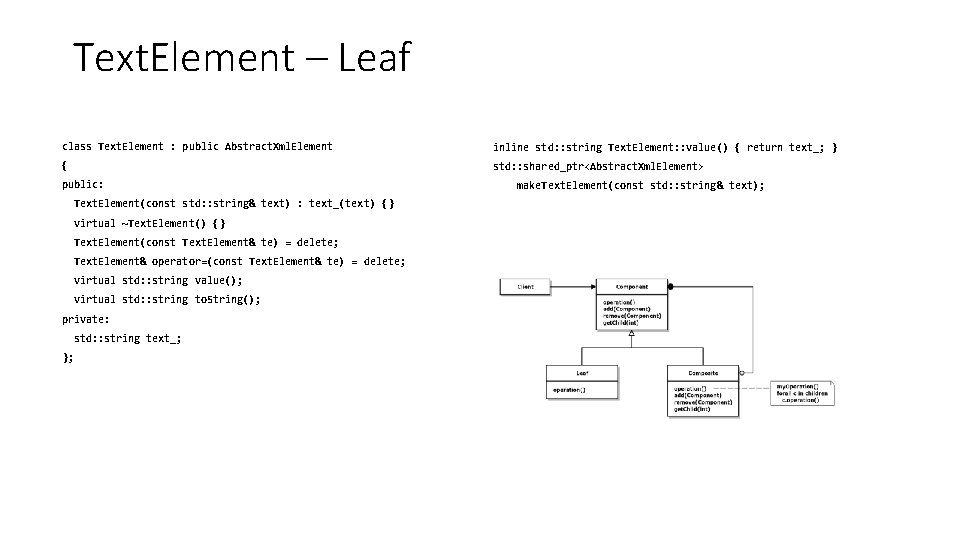
Text. Element – Leaf class Text. Element : public Abstract. Xml. Element inline std: : string Text. Element: : value() { return text_; } { std: : shared_ptr<Abstract. Xml. Element> public: Text. Element(const std: : string& text) : text_(text) {} virtual ~Text. Element() {} Text. Element(const Text. Element& te) = delete; Text. Element& operator=(const Text. Element& te) = delete; virtual std: : string value(); virtual std: : string to. String(); private: std: : string text_; }; make. Text. Element(const std: : string& text);
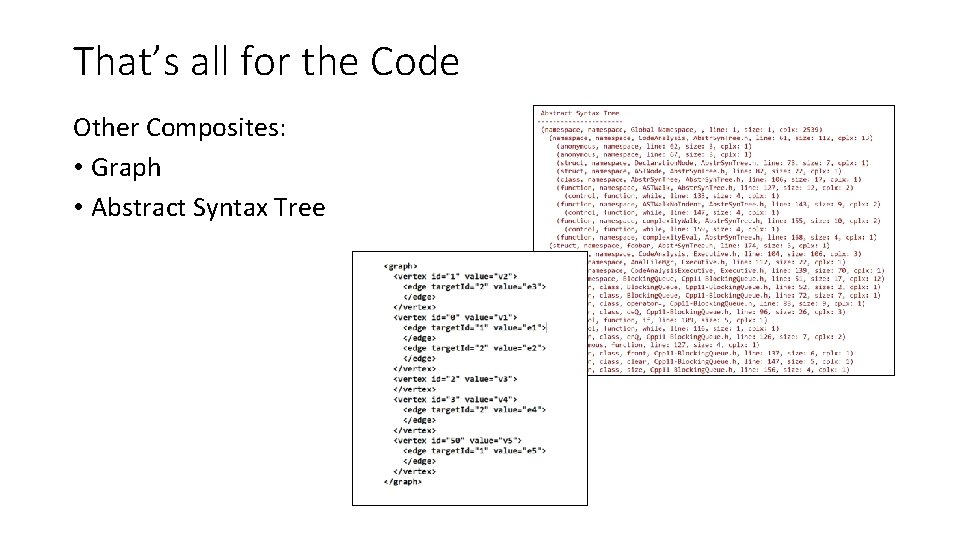
That’s all for the Code Other Composites: • Graph • Abstract Syntax Tree