COMP 4426421 Compiler Design 1 Click to edit
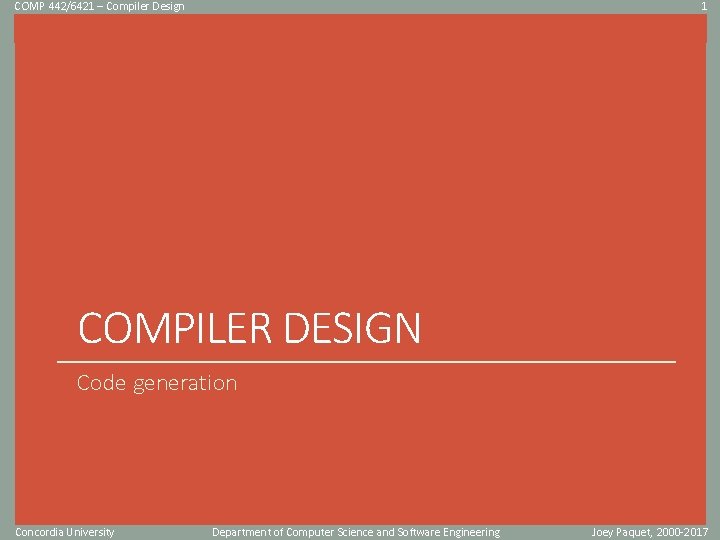
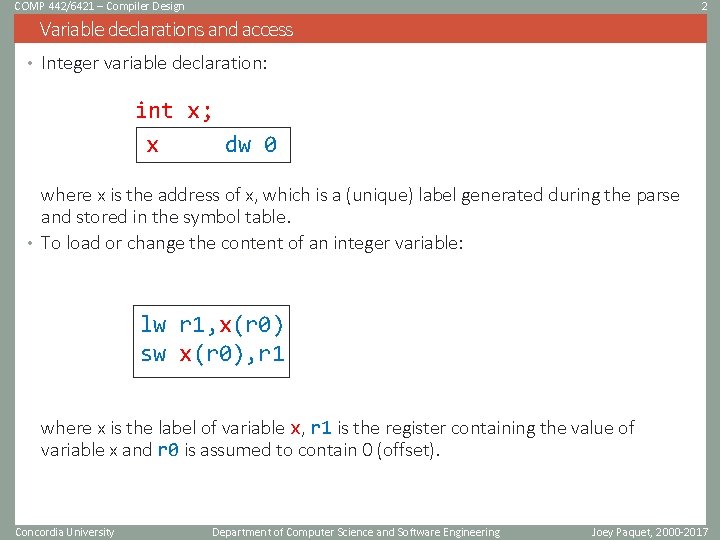
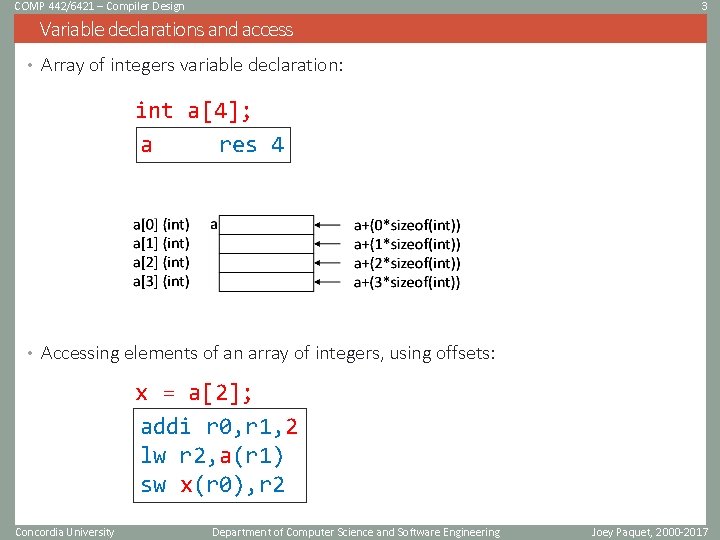
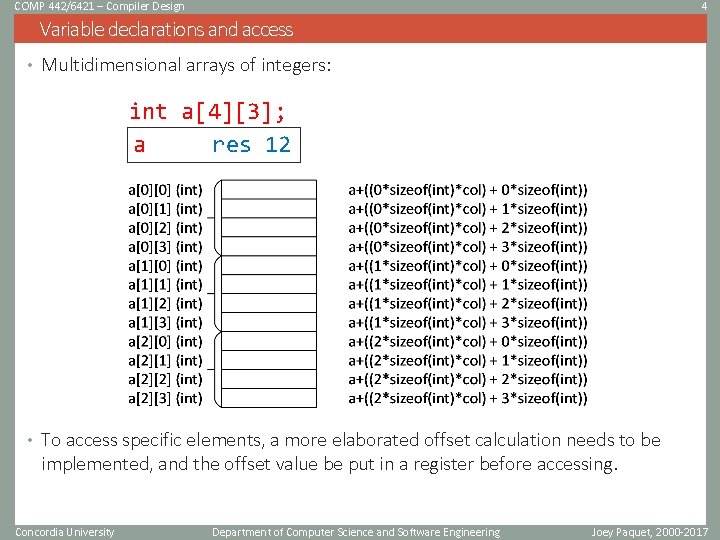
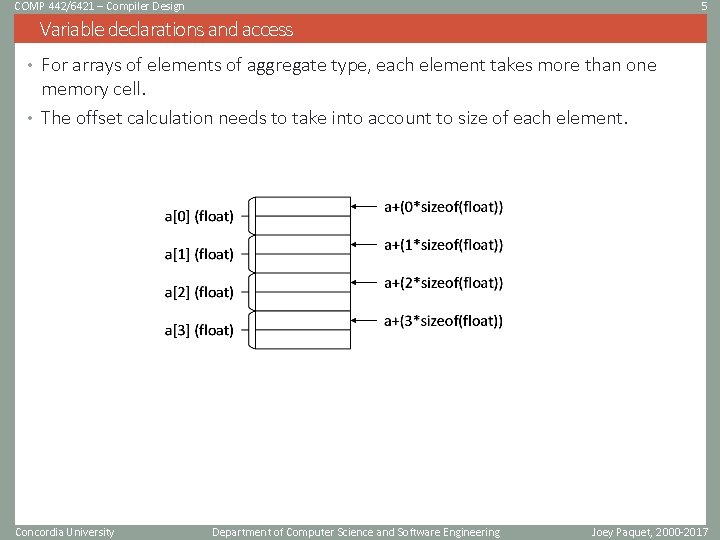
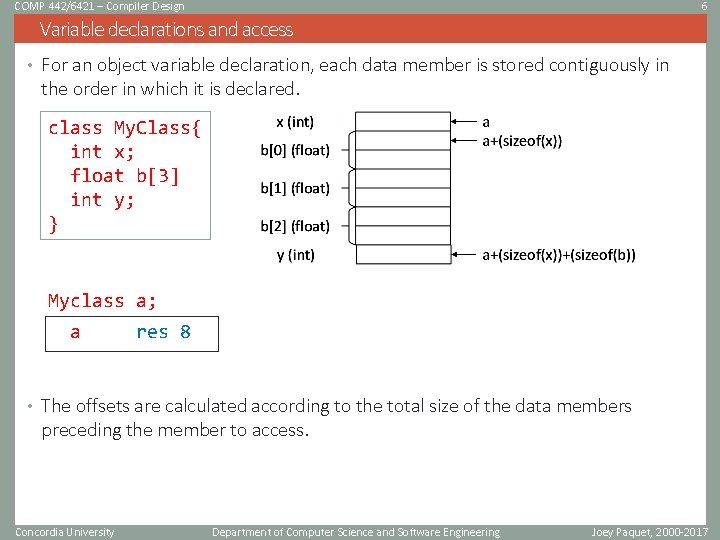
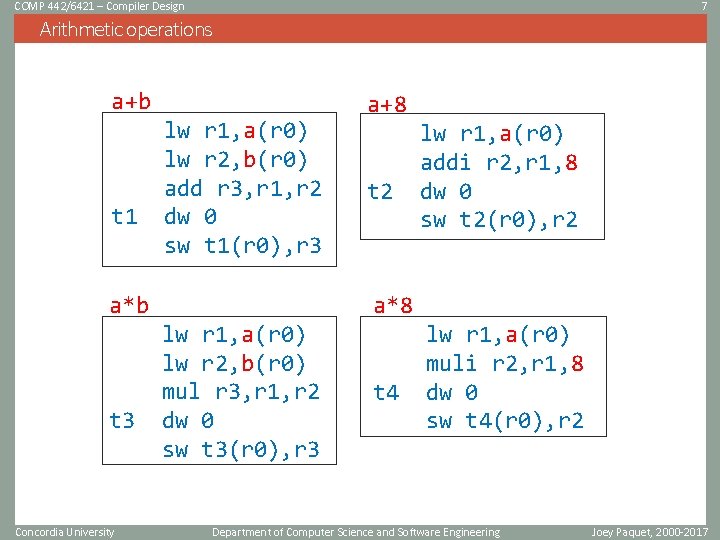
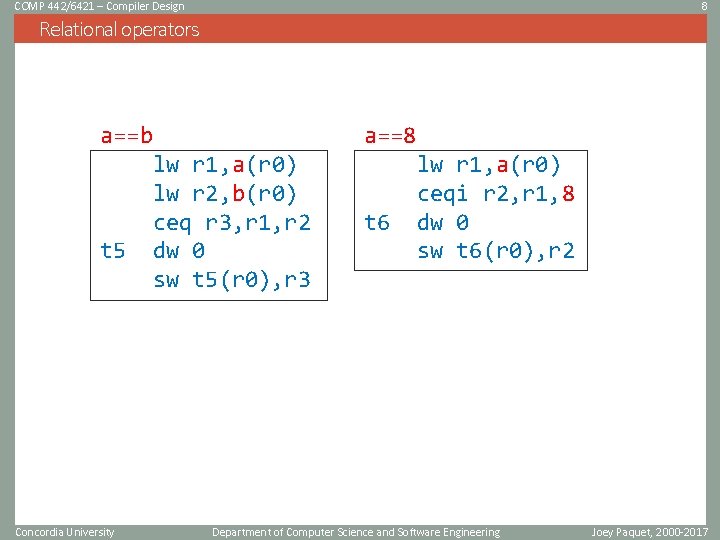
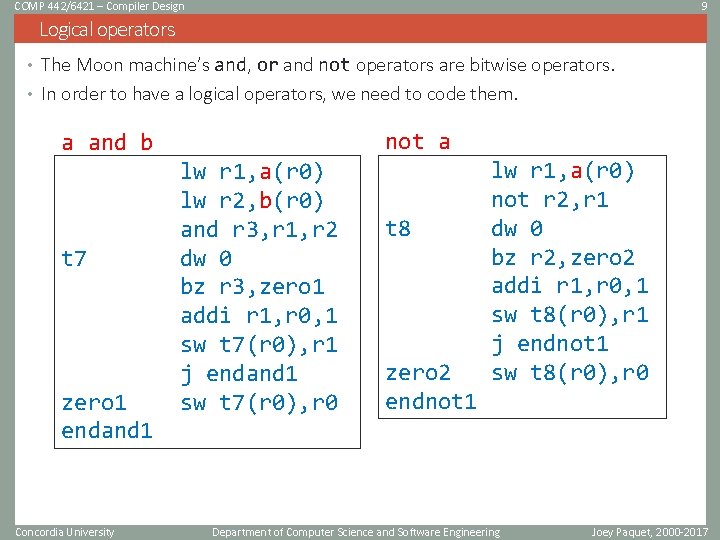
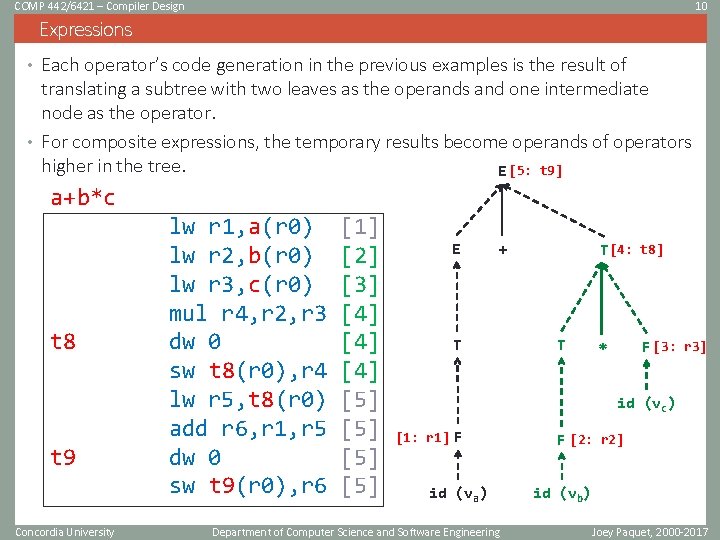
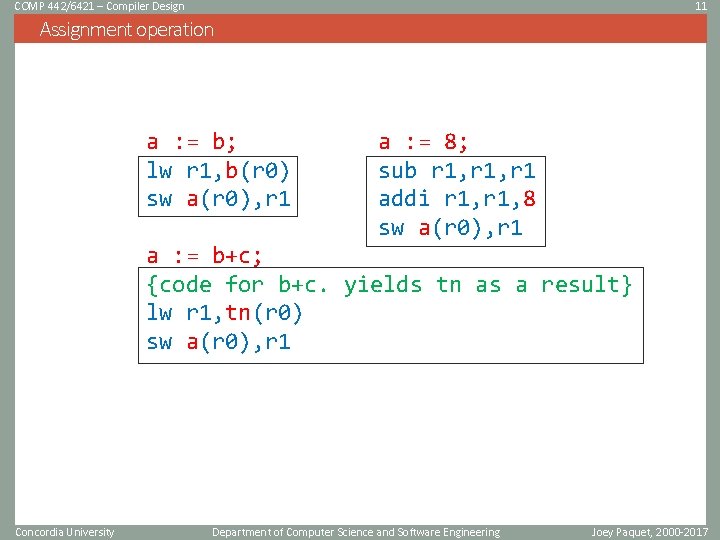
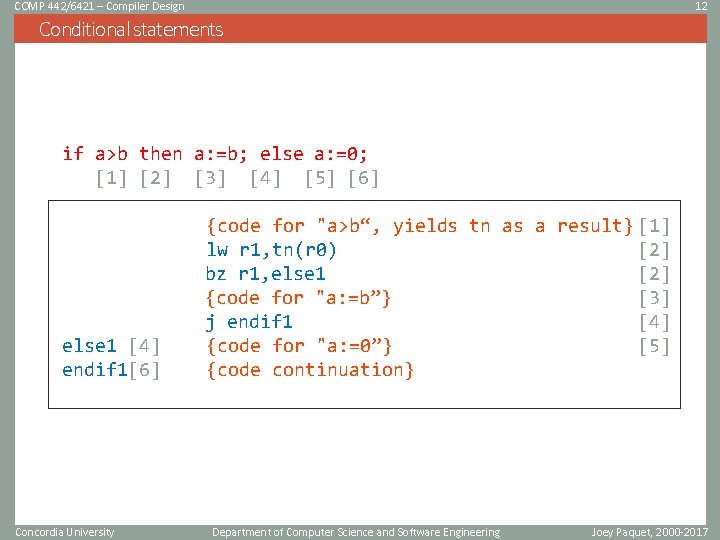
![COMP 442/6421 – Compiler Design 13 Loop statements while a<b [1] [2] do a: COMP 442/6421 – Compiler Design 13 Loop statements while a<b [1] [2] do a:](https://slidetodoc.com/presentation_image/2798495cc165ec8e808fa006b7f2778b/image-13.jpg)
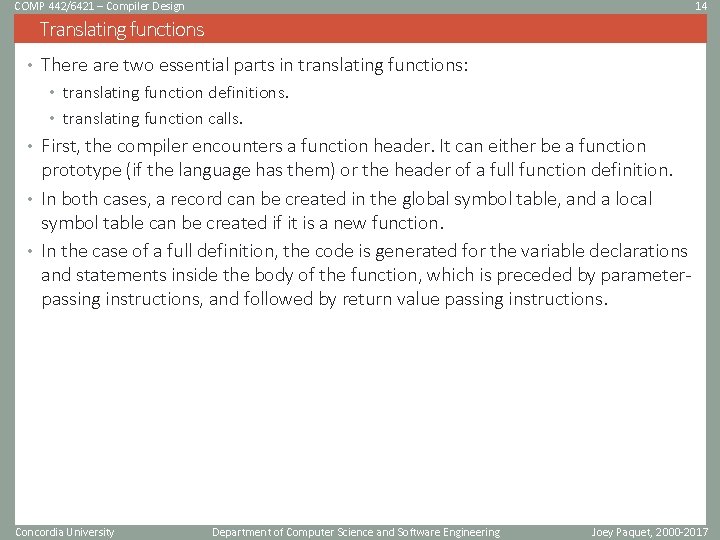
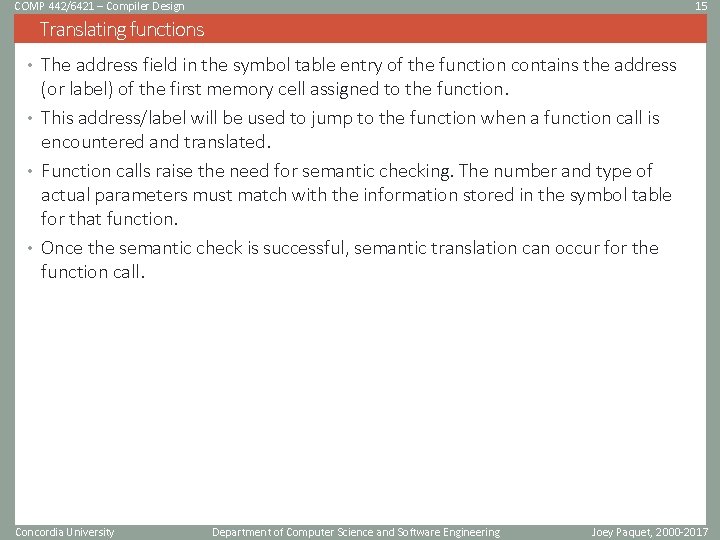
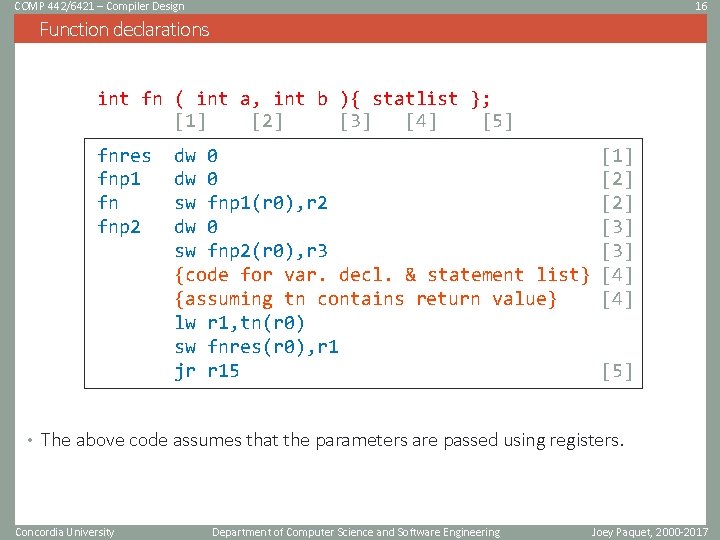
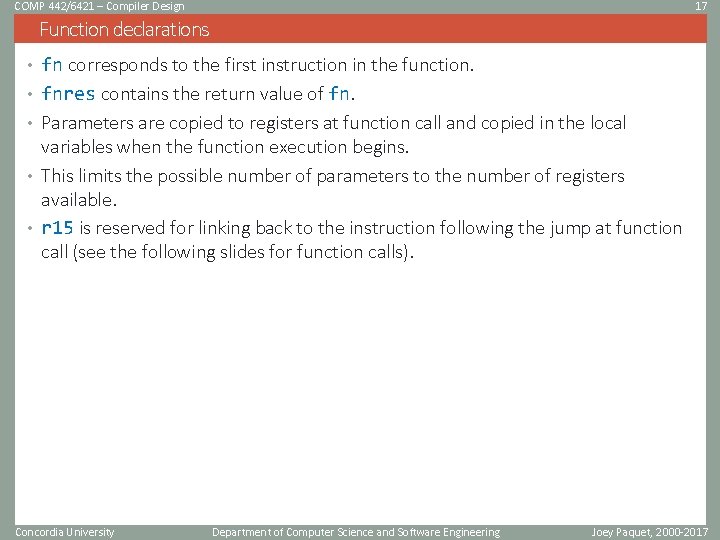
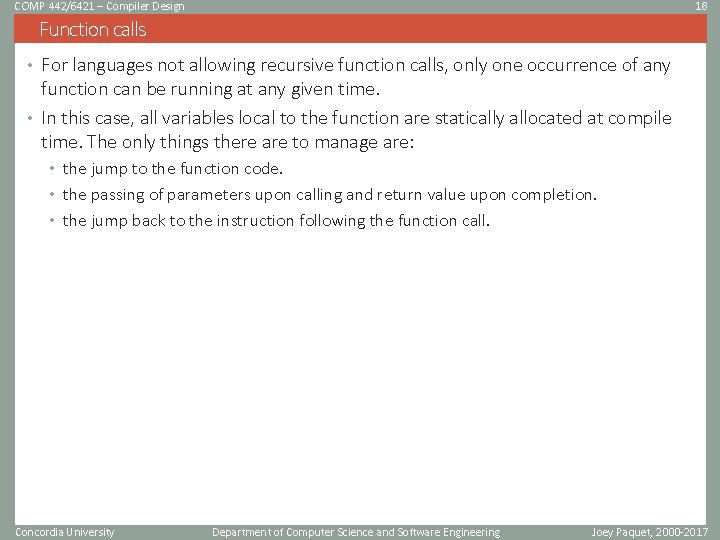
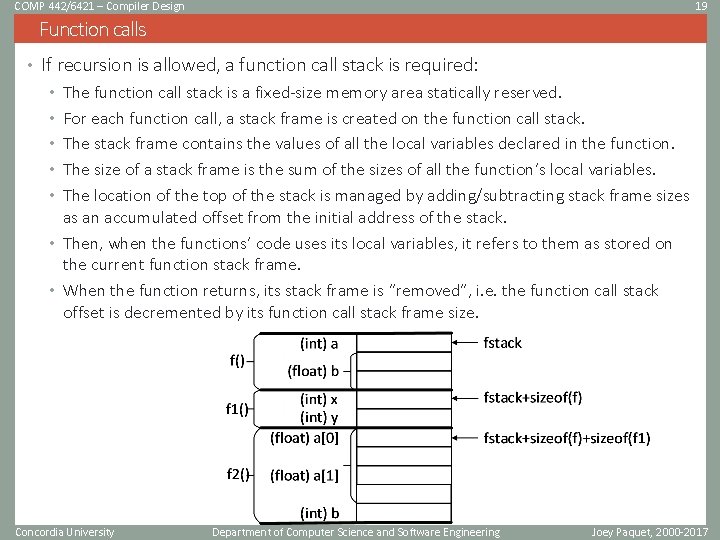
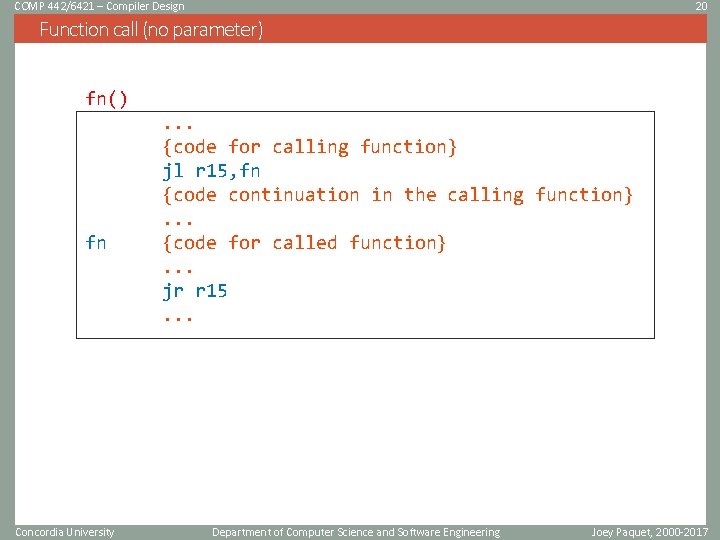
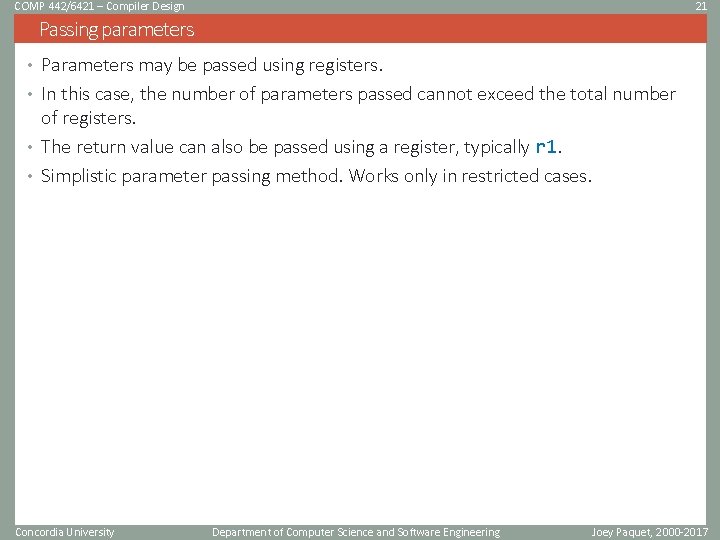
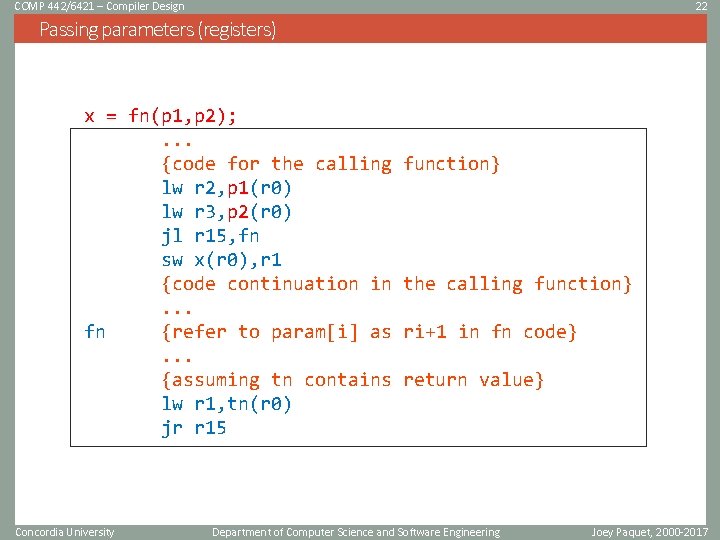
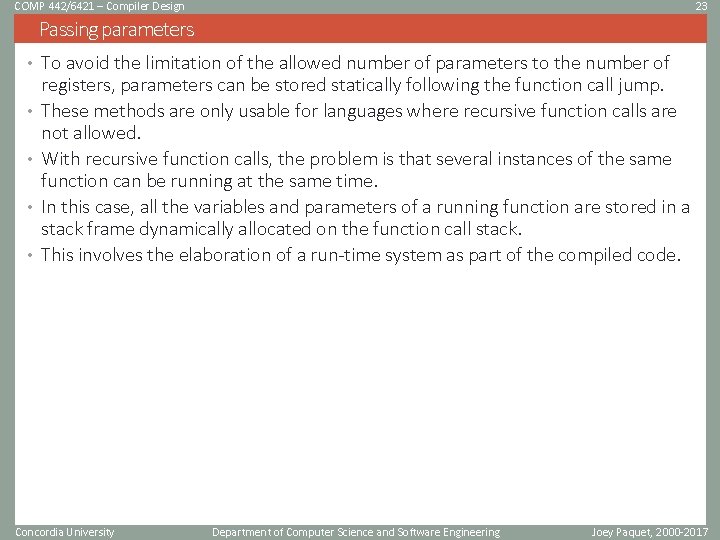
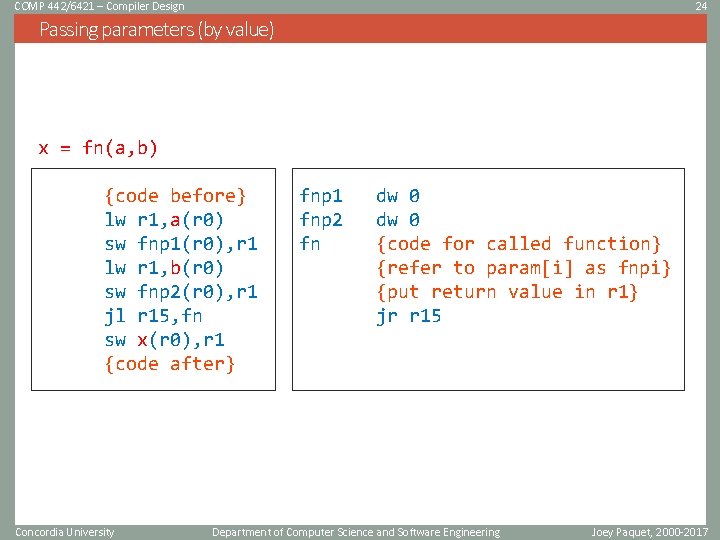
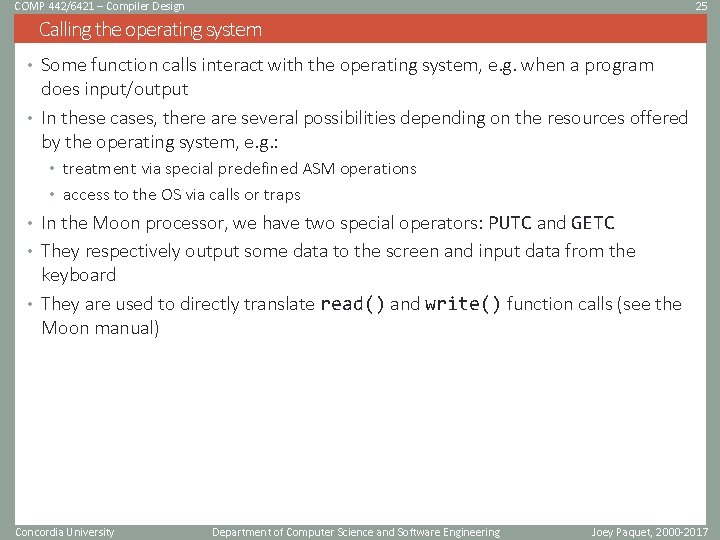
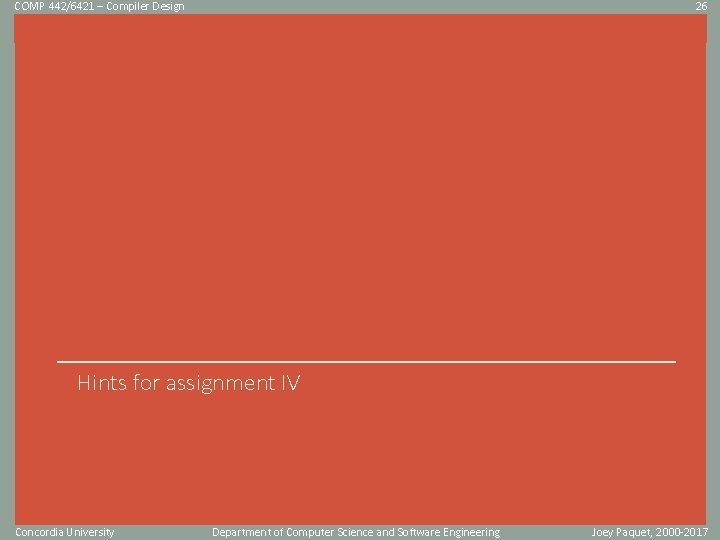
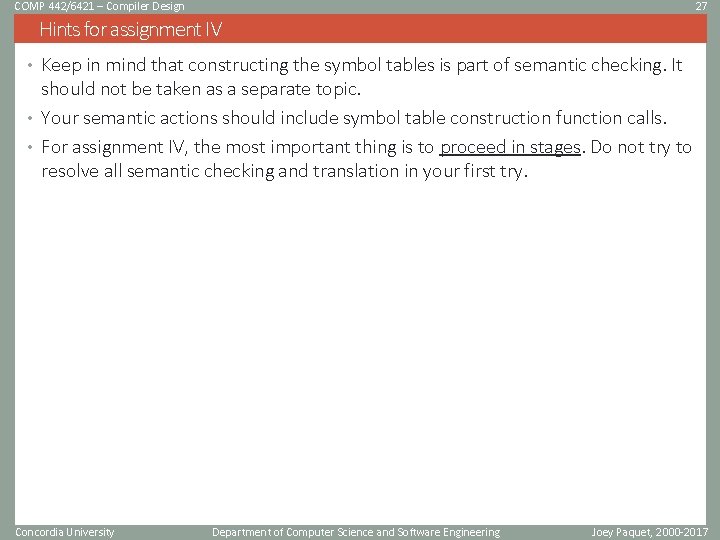
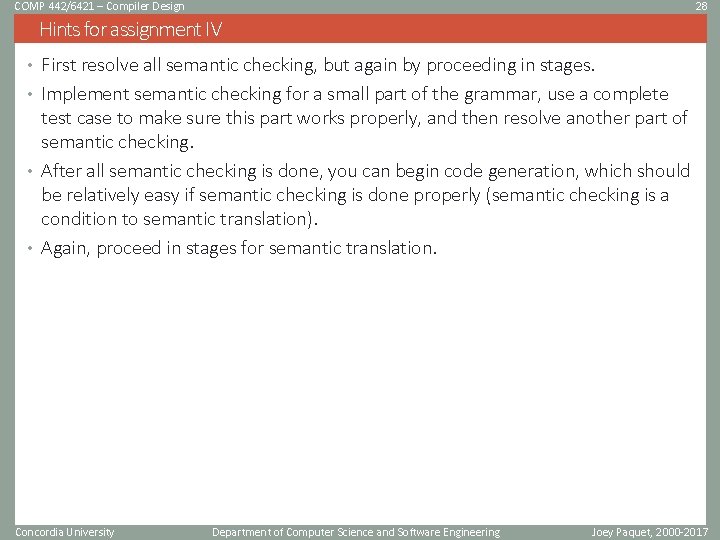
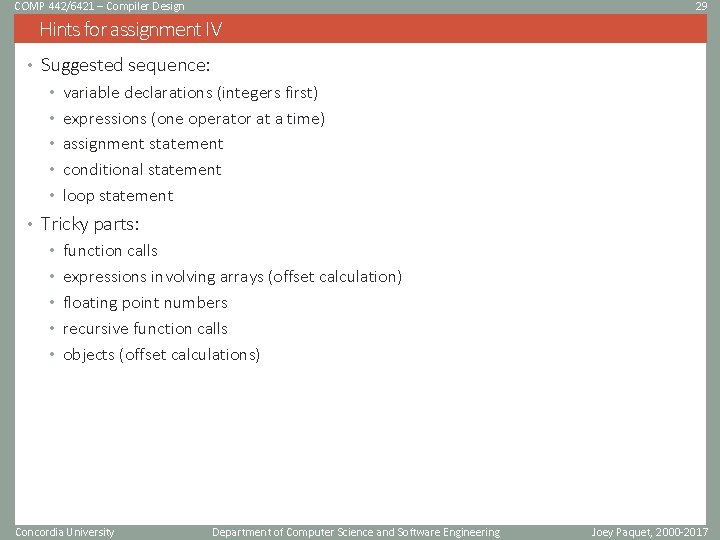
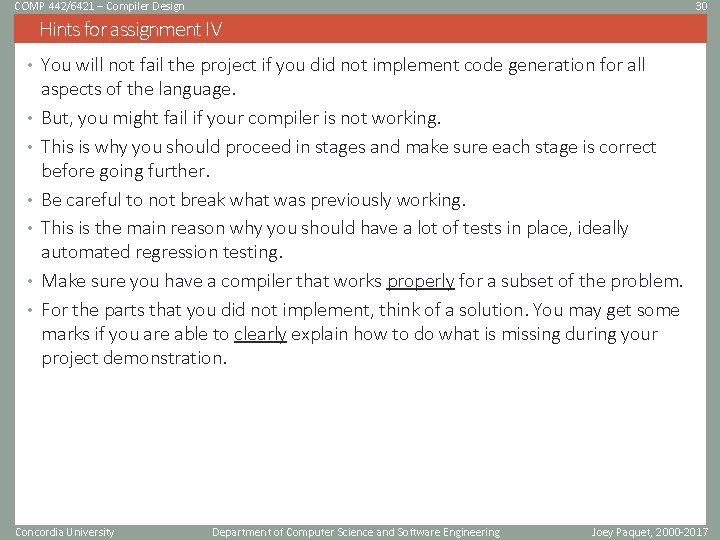
- Slides: 30
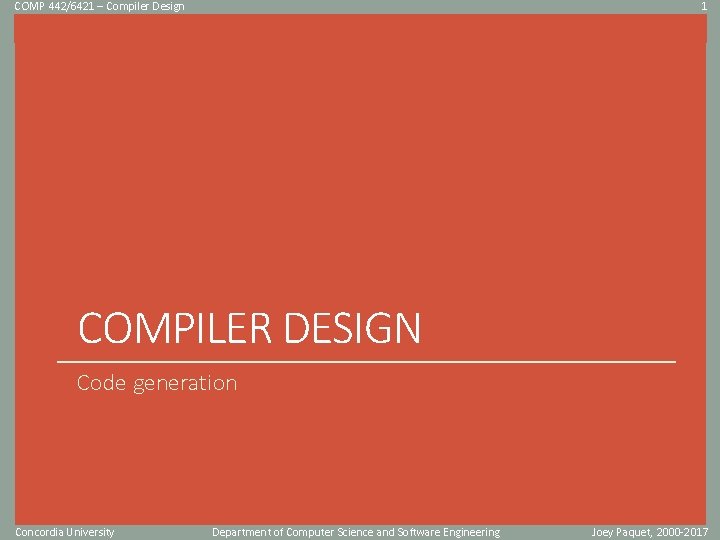
COMP 442/6421 – Compiler Design 1 Click to edit Master title style COMPILER DESIGN Code generation Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
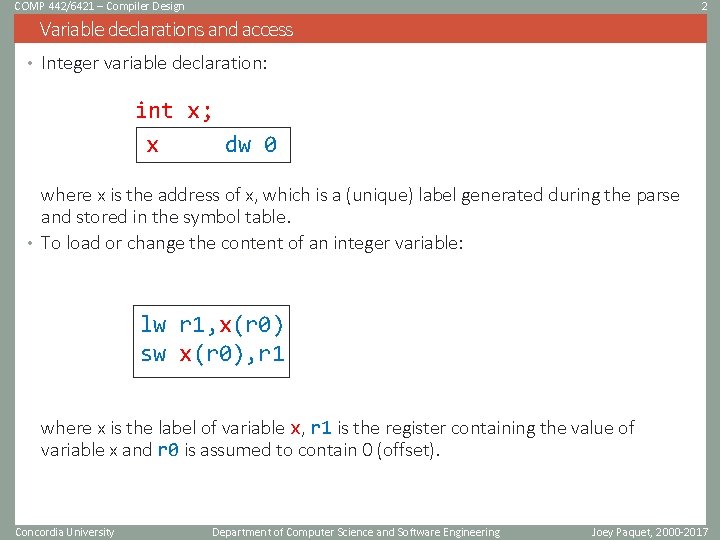
COMP 442/6421 – Compiler Design 2 Variable declarations and access • Integer variable declaration: int x; x dw 0 where x is the address of x, which is a (unique) label generated during the parse and stored in the symbol table. • To load or change the content of an integer variable: lw r 1, x(r 0) sw x(r 0), r 1 where x is the label of variable x, r 1 is the register containing the value of variable x and r 0 is assumed to contain 0 (offset). Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
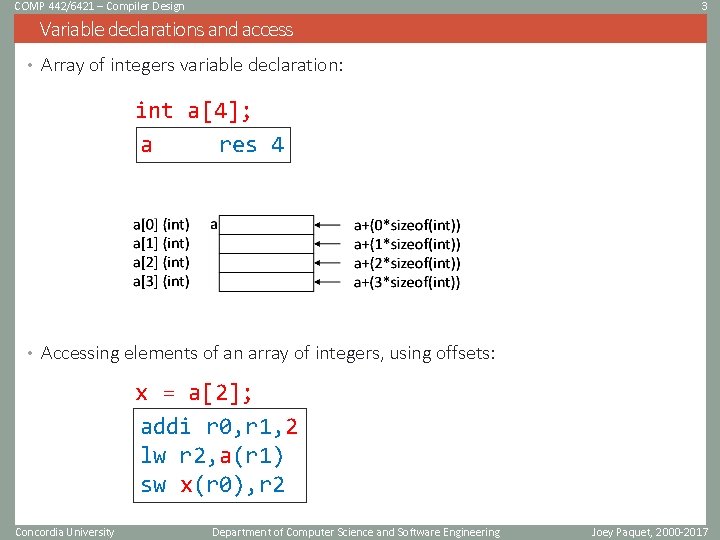
COMP 442/6421 – Compiler Design 3 Variable declarations and access • Array of integers variable declaration: int a[4]; a res 4 • Accessing elements of an array of integers, using offsets: x = a[2]; addi r 0, r 1, 2 lw r 2, a(r 1) sw x(r 0), r 2 Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
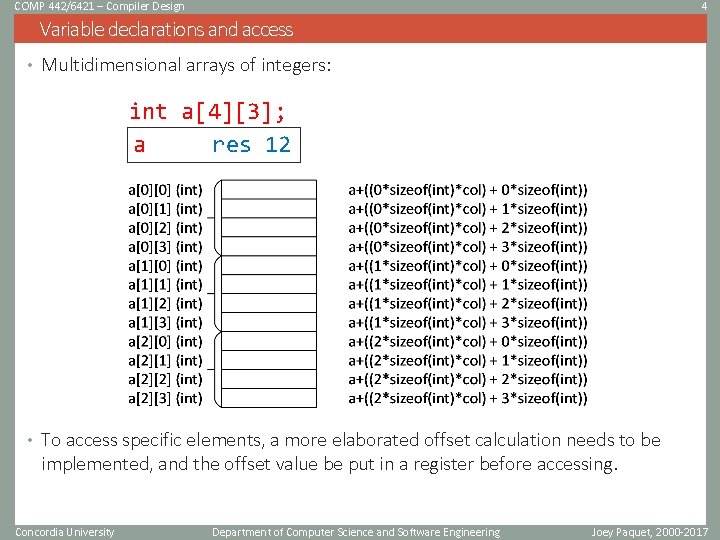
COMP 442/6421 – Compiler Design 4 Variable declarations and access • Multidimensional arrays of integers: int a[4][3]; a res 12 • To access specific elements, a more elaborated offset calculation needs to be implemented, and the offset value be put in a register before accessing. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
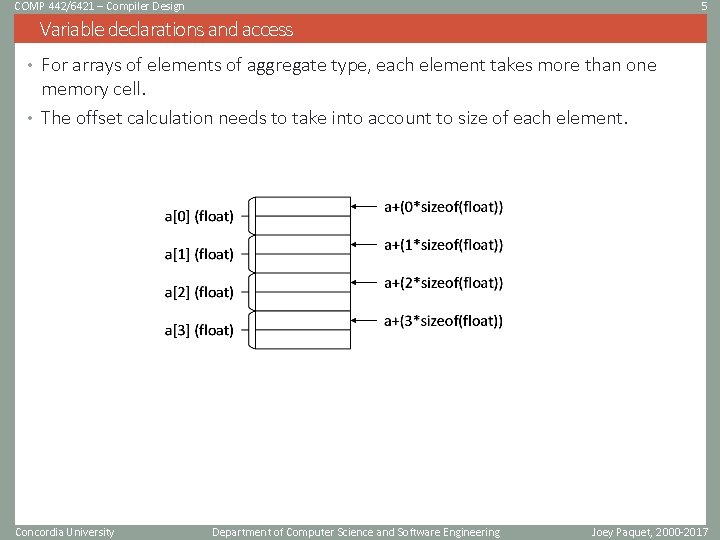
COMP 442/6421 – Compiler Design 5 Variable declarations and access • For arrays of elements of aggregate type, each element takes more than one memory cell. • The offset calculation needs to take into account to size of each element. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
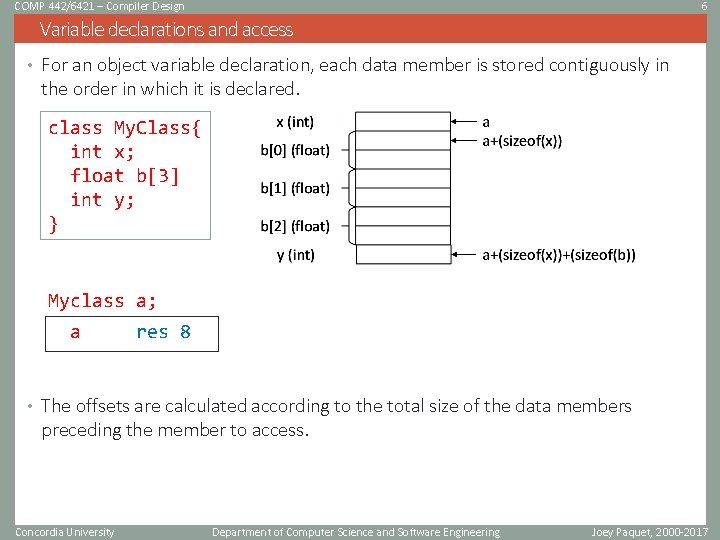
COMP 442/6421 – Compiler Design 6 Variable declarations and access • For an object variable declaration, each data member is stored contiguously in the order in which it is declared. class My. Class{ int x; float b[3] int y; } Myclass a; a res 8 • The offsets are calculated according to the total size of the data members preceding the member to access. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
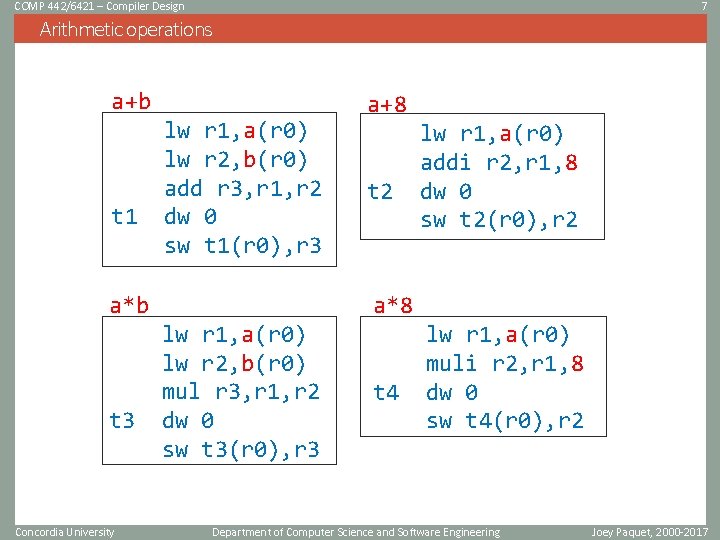
COMP 442/6421 – Compiler Design 7 Arithmetic operations a+b t 1 lw r 1, a(r 0) lw r 2, b(r 0) add r 3, r 1, r 2 dw 0 sw t 1(r 0), r 3 a*b t 3 Concordia University a+8 t 2 lw r 1, a(r 0) addi r 2, r 1, 8 dw 0 sw t 2(r 0), r 2 a*8 lw r 1, a(r 0) lw r 2, b(r 0) mul r 3, r 1, r 2 dw 0 sw t 3(r 0), r 3 t 4 lw r 1, a(r 0) muli r 2, r 1, 8 dw 0 sw t 4(r 0), r 2 Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
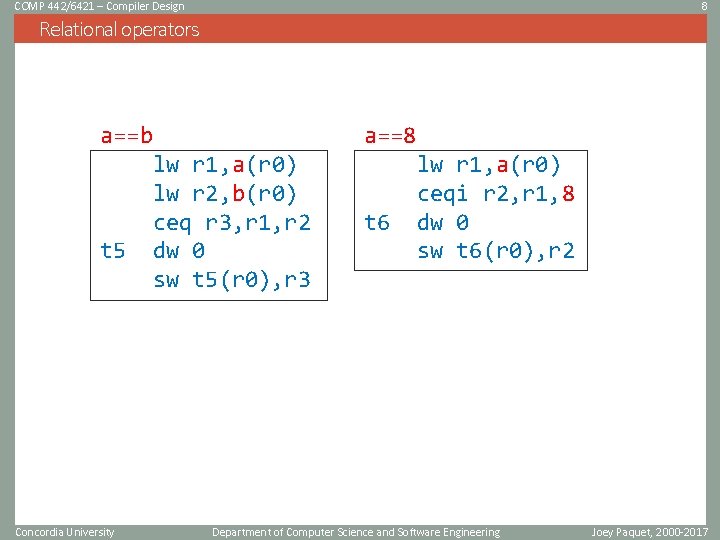
COMP 442/6421 – Compiler Design 8 Relational operators a==8 a==b t 5 Concordia University lw r 1, a(r 0) lw r 2, b(r 0) ceq r 3, r 1, r 2 dw 0 sw t 5(r 0), r 3 t 6 lw r 1, a(r 0) ceqi r 2, r 1, 8 dw 0 sw t 6(r 0), r 2 Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
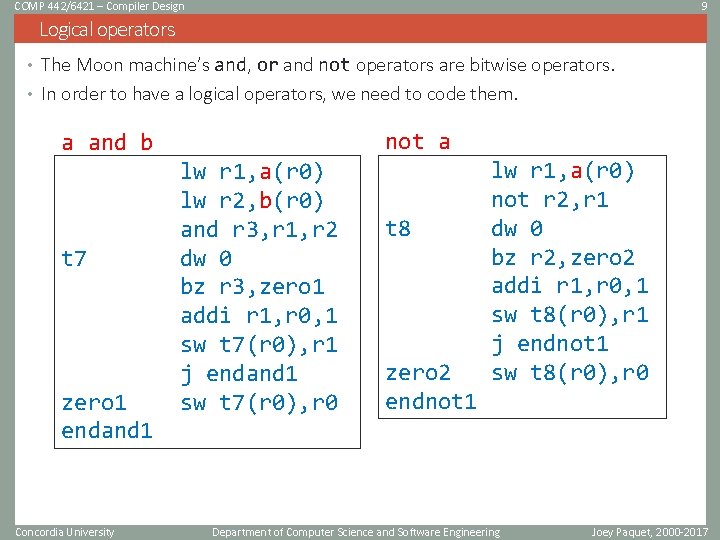
COMP 442/6421 – Compiler Design 9 Logical operators • The Moon machine’s and, or and not operators are bitwise operators. • In order to have a logical operators, we need to code them. not a a and b t 7 zero 1 endand 1 Concordia University lw r 1, a(r 0) lw r 2, b(r 0) and r 3, r 1, r 2 dw 0 bz r 3, zero 1 addi r 1, r 0, 1 sw t 7(r 0), r 1 j endand 1 sw t 7(r 0), r 0 t 8 zero 2 endnot 1 lw r 1, a(r 0) not r 2, r 1 dw 0 bz r 2, zero 2 addi r 1, r 0, 1 sw t 8(r 0), r 1 j endnot 1 sw t 8(r 0), r 0 Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
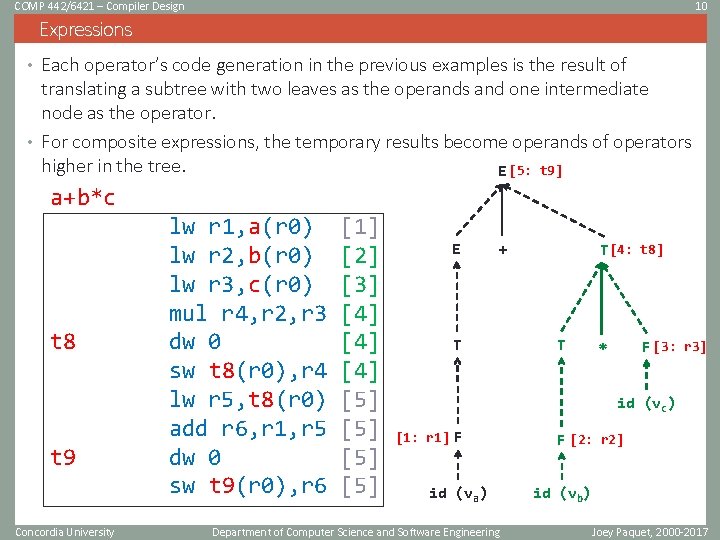
COMP 442/6421 – Compiler Design 10 Expressions • Each operator’s code generation in the previous examples is the result of translating a subtree with two leaves as the operands and one intermediate node as the operator. • For composite expressions, the temporary results become operands of operators higher in the tree. E [5: t 9] a+b*c t 8 t 9 Concordia University lw r 1, a(r 0) lw r 2, b(r 0) lw r 3, c(r 0) mul r 4, r 2, r 3 dw 0 sw t 8(r 0), r 4 lw r 5, t 8(r 0) add r 6, r 1, r 5 dw 0 sw t 9(r 0), r 6 [1] [2] [3] [4] [4] [5] [5] E + T T [4: t 8] T F [3: r 3] * id (vc) [1: r 1] F id (va) Department of Computer Science and Software Engineering F [2: r 2] id (vb) Joey Paquet, 2000 -2017
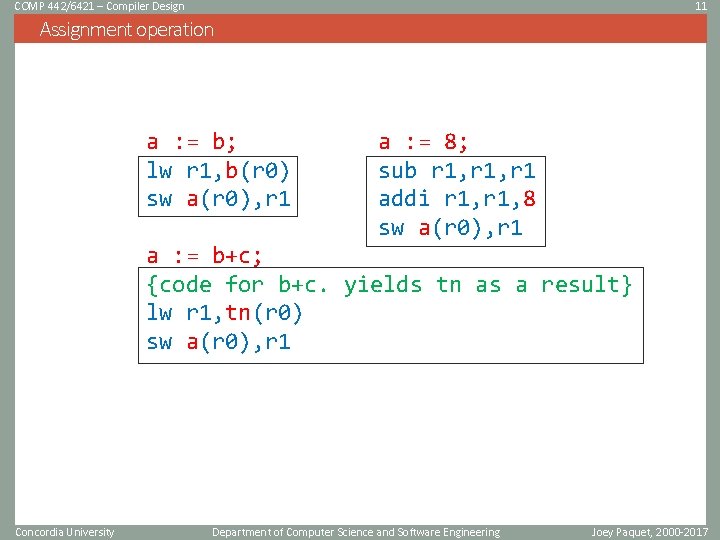
COMP 442/6421 – Compiler Design 11 Assignment operation a : = b; lw r 1, b(r 0) sw a(r 0), r 1 a : = 8; sub r 1, r 1 addi r 1, 8 sw a(r 0), r 1 a : = b+c; {code for b+c. yields tn as a result} lw r 1, tn(r 0) sw a(r 0), r 1 Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
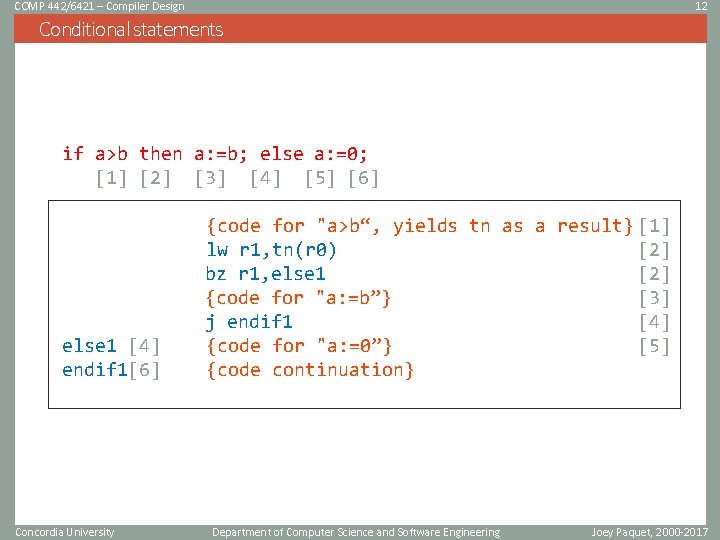
COMP 442/6421 – Compiler Design 12 Conditional statements if a>b then a: =b; else a: =0; [1] [2] [3] [4] [5] [6] else 1 [4] endif 1[6] Concordia University {code for "a>b“, yields tn as a result} [1] lw r 1, tn(r 0) [2] bz r 1, else 1 [2] {code for "a: =b”} [3] j endif 1 [4] {code for "a: =0”} [5] {code continuation} Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
![COMP 4426421 Compiler Design 13 Loop statements while ab 1 2 do a COMP 442/6421 – Compiler Design 13 Loop statements while a<b [1] [2] do a:](https://slidetodoc.com/presentation_image/2798495cc165ec8e808fa006b7f2778b/image-13.jpg)
COMP 442/6421 – Compiler Design 13 Loop statements while a<b [1] [2] do a: =a+1; [3] [4] [5] gowhile 1 [1] {code for "a<b". yields tn as a result} [2] lw r 1, tn(r 0) [3] bz r 1, endwhile 1 [3] {code for statblock (a: =a+1)} [4] j gowhile 1 [5] endwhile 1[5] {code continuation} Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
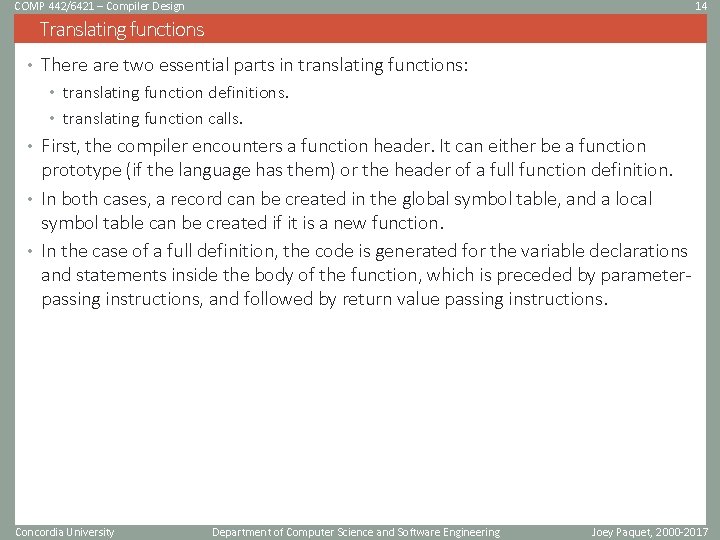
COMP 442/6421 – Compiler Design 14 Translating functions • There are two essential parts in translating functions: • translating function definitions. • translating function calls. • First, the compiler encounters a function header. It can either be a function prototype (if the language has them) or the header of a full function definition. • In both cases, a record can be created in the global symbol table, and a local symbol table can be created if it is a new function. • In the case of a full definition, the code is generated for the variable declarations and statements inside the body of the function, which is preceded by parameterpassing instructions, and followed by return value passing instructions. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
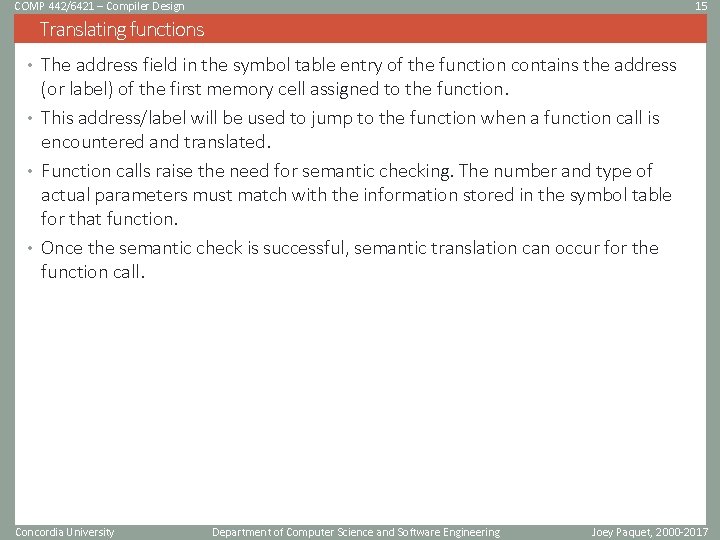
COMP 442/6421 – Compiler Design 15 Translating functions • The address field in the symbol table entry of the function contains the address (or label) of the first memory cell assigned to the function. • This address/label will be used to jump to the function when a function call is encountered and translated. • Function calls raise the need for semantic checking. The number and type of actual parameters must match with the information stored in the symbol table for that function. • Once the semantic check is successful, semantic translation can occur for the function call. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
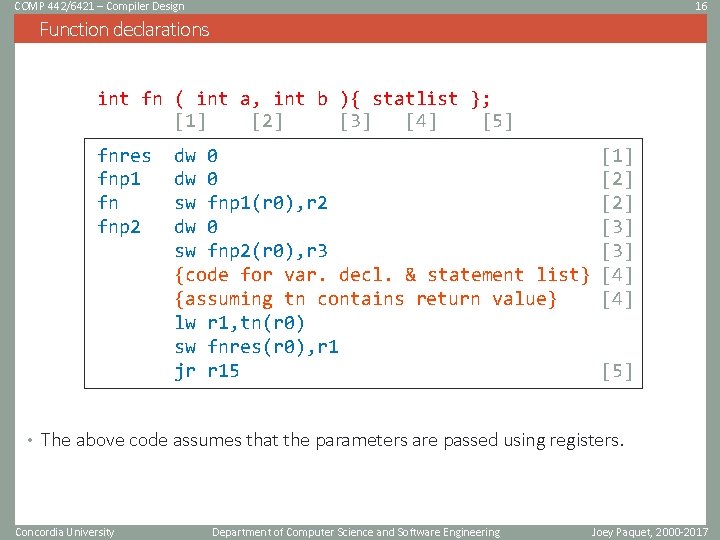
COMP 442/6421 – Compiler Design 16 Function declarations int fn ( int a, int b ){ statlist }; [1] [2] [3] [4] [5] fnres fnp 1 fn fnp 2 dw 0 sw fnp 1(r 0), r 2 dw 0 sw fnp 2(r 0), r 3 {code for var. decl. & statement list} {assuming tn contains return value} lw r 1, tn(r 0) sw fnres(r 0), r 1 jr r 15 [1] [2] [3] [4] [5] • The above code assumes that the parameters are passed using registers. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
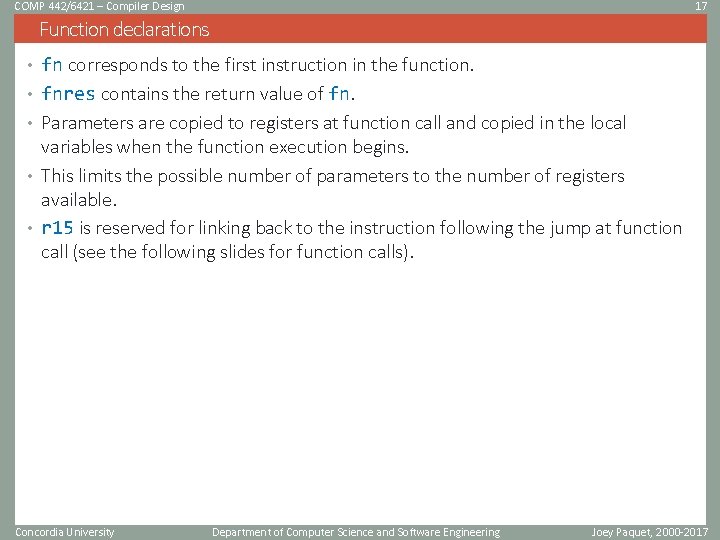
COMP 442/6421 – Compiler Design 17 Function declarations • fn corresponds to the first instruction in the function. • fnres contains the return value of fn. • Parameters are copied to registers at function call and copied in the local variables when the function execution begins. • This limits the possible number of parameters to the number of registers available. • r 15 is reserved for linking back to the instruction following the jump at function call (see the following slides for function calls). Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
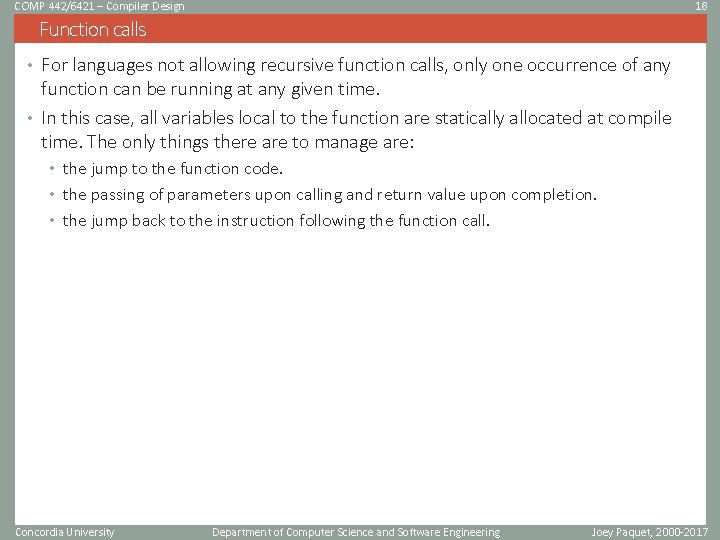
COMP 442/6421 – Compiler Design 18 Function calls • For languages not allowing recursive function calls, only one occurrence of any function can be running at any given time. • In this case, all variables local to the function are statically allocated at compile time. The only things there are to manage are: • the jump to the function code. • the passing of parameters upon calling and return value upon completion. • the jump back to the instruction following the function call. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
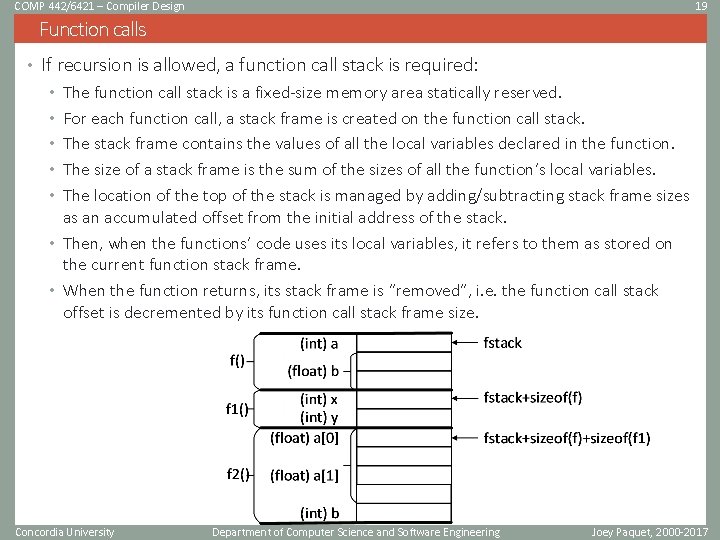
COMP 442/6421 – Compiler Design 19 Function calls • If recursion is allowed, a function call stack is required: • The function call stack is a fixed-size memory area statically reserved. • For each function call, a stack frame is created on the function call stack. • The stack frame contains the values of all the local variables declared in the function. • The size of a stack frame is the sum of the sizes of all the function’s local variables. • The location of the top of the stack is managed by adding/subtracting stack frame sizes as an accumulated offset from the initial address of the stack. • Then, when the functions’ code uses its local variables, it refers to them as stored on the current function stack frame. • When the function returns, its stack frame is “removed”, i. e. the function call stack offset is decremented by its function call stack frame size. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
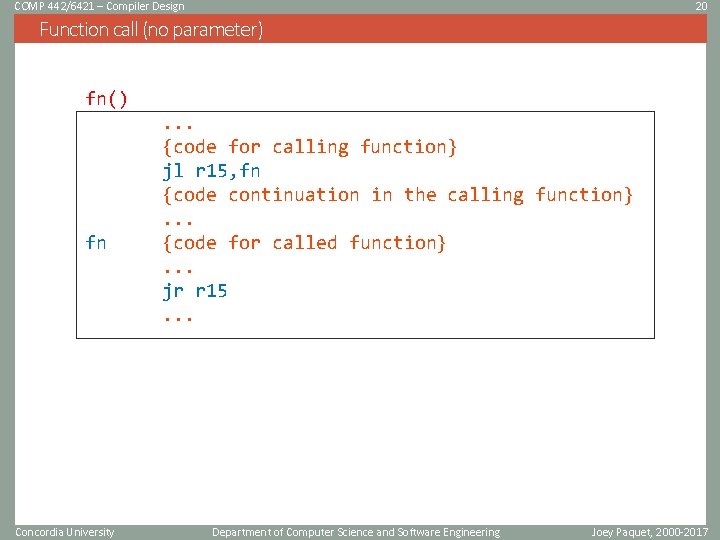
COMP 442/6421 – Compiler Design 20 Function call (no parameter) fn() fn Concordia University . . . {code for calling function} jl r 15, fn {code continuation in the calling function}. . . {code for called function}. . . jr r 15. . . Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
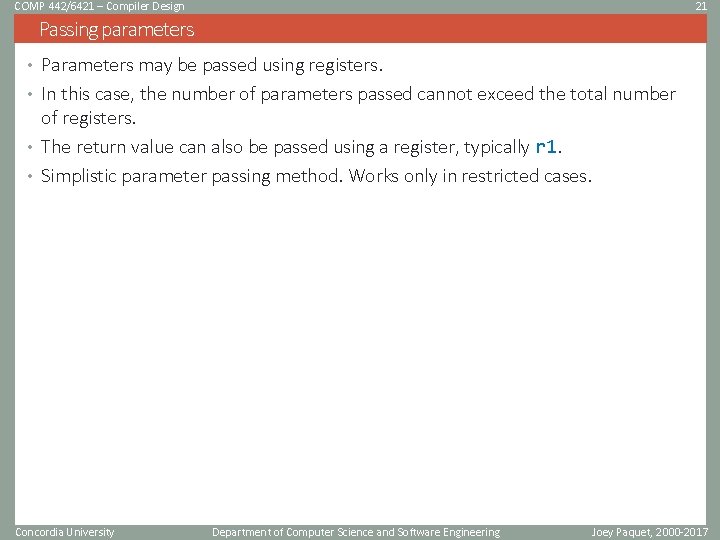
COMP 442/6421 – Compiler Design 21 Passing parameters • Parameters may be passed using registers. • In this case, the number of parameters passed cannot exceed the total number of registers. • The return value can also be passed using a register, typically r 1. • Simplistic parameter passing method. Works only in restricted cases. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
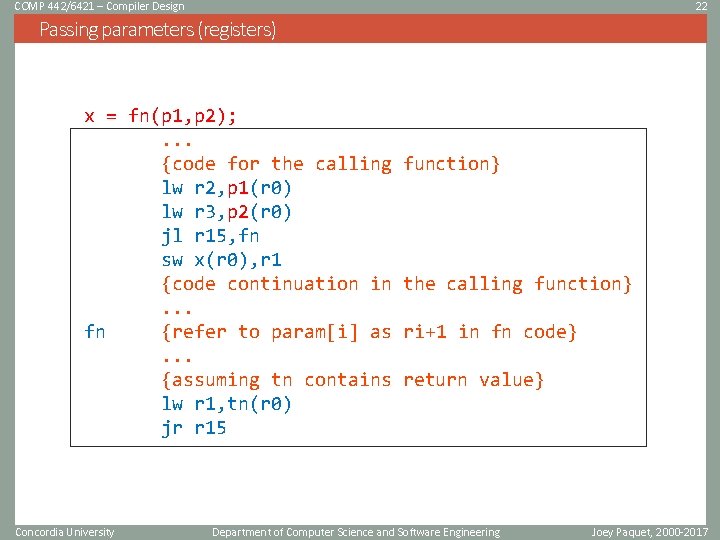
COMP 442/6421 – Compiler Design 22 Passing parameters (registers) x = fn(p 1, p 2); . . . {code for the calling lw r 2, p 1(r 0) lw r 3, p 2(r 0) jl r 15, fn sw x(r 0), r 1 {code continuation in. . . fn {refer to param[i] as. . . {assuming tn contains lw r 1, tn(r 0) jr r 15 Concordia University function} the calling function} ri+1 in fn code} return value} Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
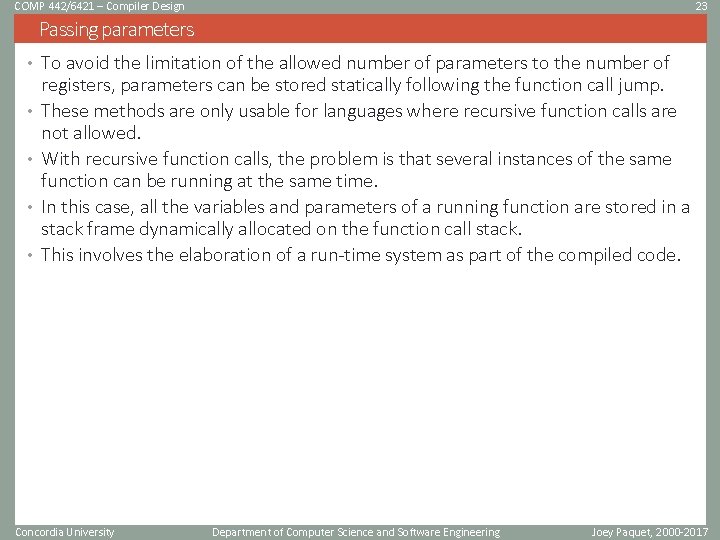
COMP 442/6421 – Compiler Design 23 Passing parameters • To avoid the limitation of the allowed number of parameters to the number of • • registers, parameters can be stored statically following the function call jump. These methods are only usable for languages where recursive function calls are not allowed. With recursive function calls, the problem is that several instances of the same function can be running at the same time. In this case, all the variables and parameters of a running function are stored in a stack frame dynamically allocated on the function call stack. This involves the elaboration of a run-time system as part of the compiled code. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
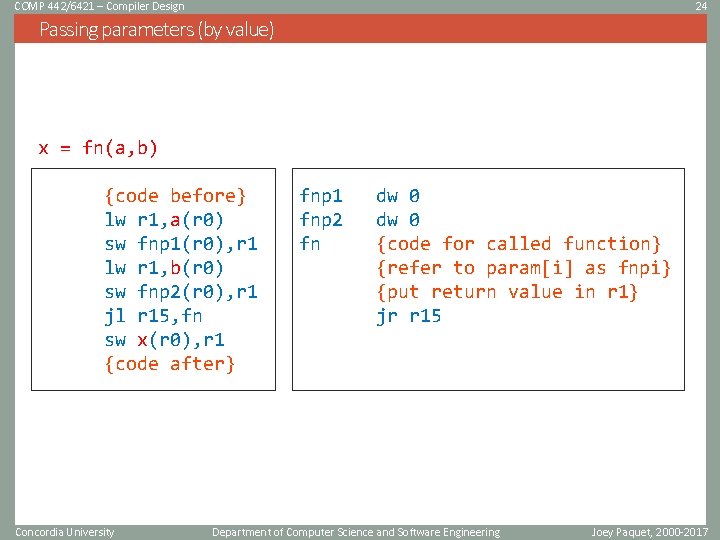
COMP 442/6421 – Compiler Design 24 Passing parameters (by value) x = fn(a, b) {code before} lw r 1, a(r 0) sw fnp 1(r 0), r 1 lw r 1, b(r 0) sw fnp 2(r 0), r 1 jl r 15, fn sw x(r 0), r 1 {code after} Concordia University fnp 1 fnp 2 fn dw 0 {code for called function} {refer to param[i] as fnpi} {put return value in r 1} jr r 15 Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
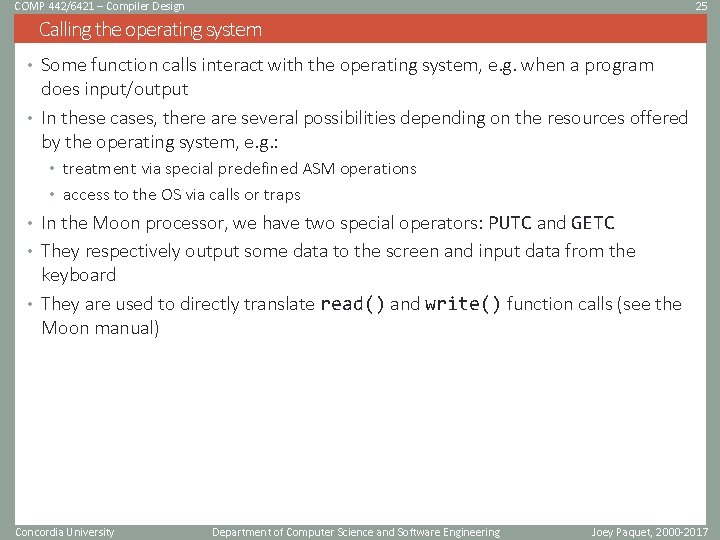
COMP 442/6421 – Compiler Design 25 Calling the operating system • Some function calls interact with the operating system, e. g. when a program does input/output • In these cases, there are several possibilities depending on the resources offered by the operating system, e. g. : • treatment via special predefined ASM operations • access to the OS via calls or traps • In the Moon processor, we have two special operators: PUTC and GETC • They respectively output some data to the screen and input data from the keyboard • They are used to directly translate read() and write() function calls (see the Moon manual) Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
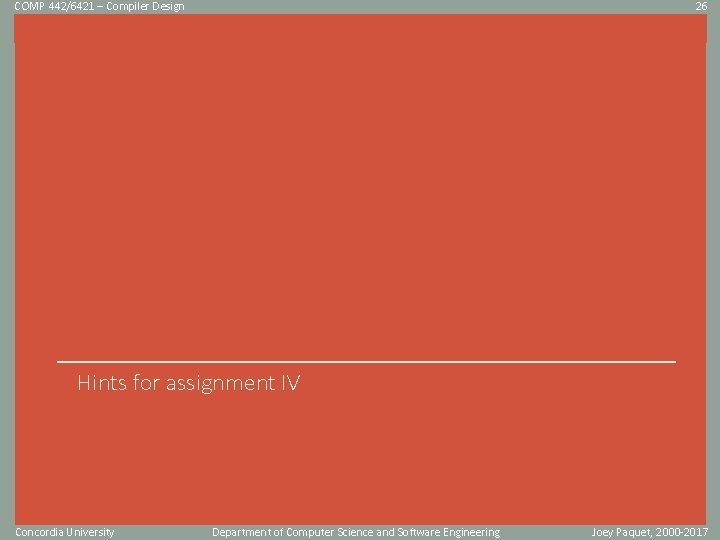
COMP 442/6421 – Compiler Design 26 Click to edit Master title style Hints for assignment IV Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
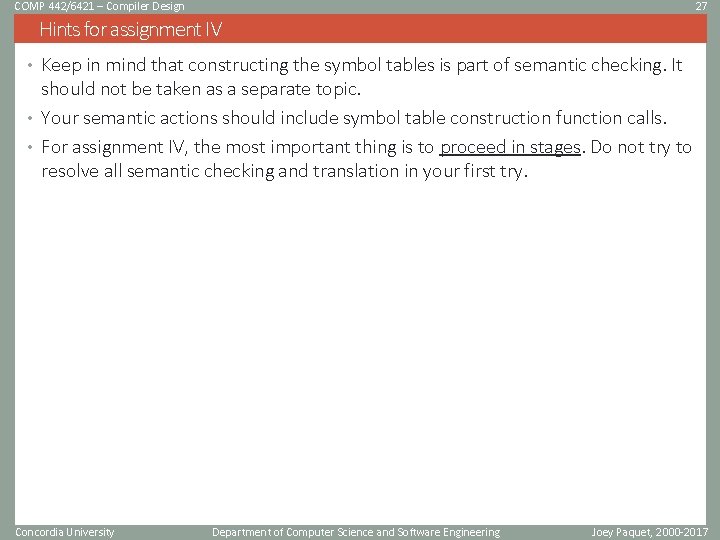
COMP 442/6421 – Compiler Design 27 Hints for assignment IV • Keep in mind that constructing the symbol tables is part of semantic checking. It should not be taken as a separate topic. • Your semantic actions should include symbol table construction function calls. • For assignment IV, the most important thing is to proceed in stages. Do not try to resolve all semantic checking and translation in your first try. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
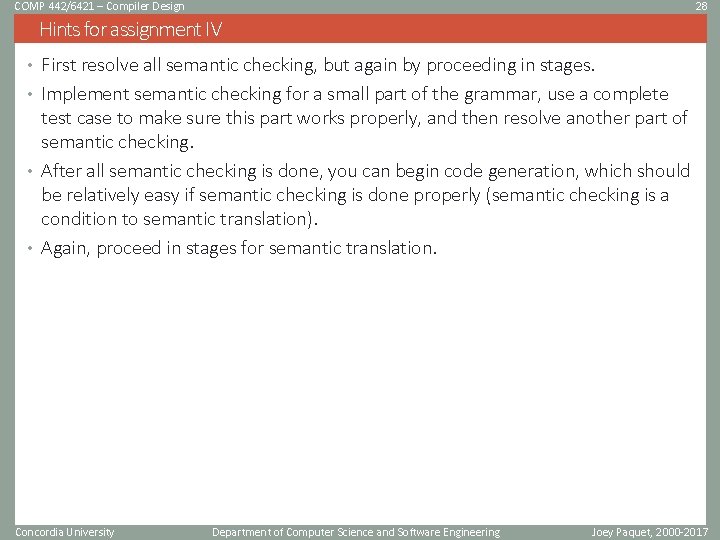
COMP 442/6421 – Compiler Design 28 Hints for assignment IV • First resolve all semantic checking, but again by proceeding in stages. • Implement semantic checking for a small part of the grammar, use a complete test case to make sure this part works properly, and then resolve another part of semantic checking. • After all semantic checking is done, you can begin code generation, which should be relatively easy if semantic checking is done properly (semantic checking is a condition to semantic translation). • Again, proceed in stages for semantic translation. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
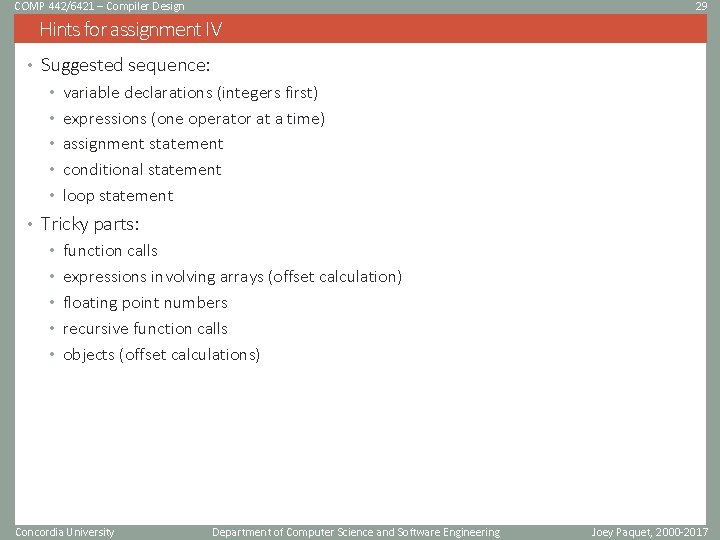
COMP 442/6421 – Compiler Design 29 Hints for assignment IV • Suggested sequence: • variable declarations (integers first) • expressions (one operator at a time) • assignment statement • conditional statement • loop statement • Tricky parts: • function calls • expressions involving arrays (offset calculation) • floating point numbers • recursive function calls • objects (offset calculations) Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017
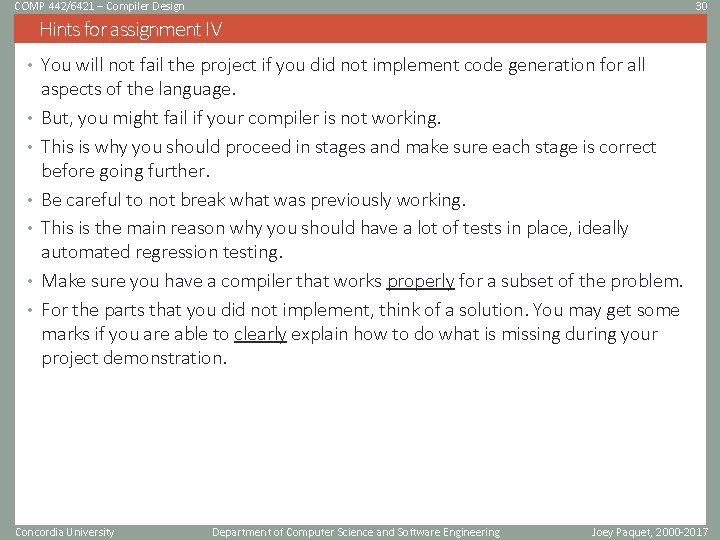
COMP 442/6421 – Compiler Design 30 Hints for assignment IV • You will not fail the project if you did not implement code generation for all • • • aspects of the language. But, you might fail if your compiler is not working. This is why you should proceed in stages and make sure each stage is correct before going further. Be careful to not break what was previously working. This is the main reason why you should have a lot of tests in place, ideally automated regression testing. Make sure you have a compiler that works properly for a subset of the problem. For the parts that you did not implement, think of a solution. You may get some marks if you are able to clearly explain how to do what is missing during your project demonstration. Concordia University Department of Computer Science and Software Engineering Joey Paquet, 2000 -2017