COMP 3500 Introduction to Operating Systems Project 5
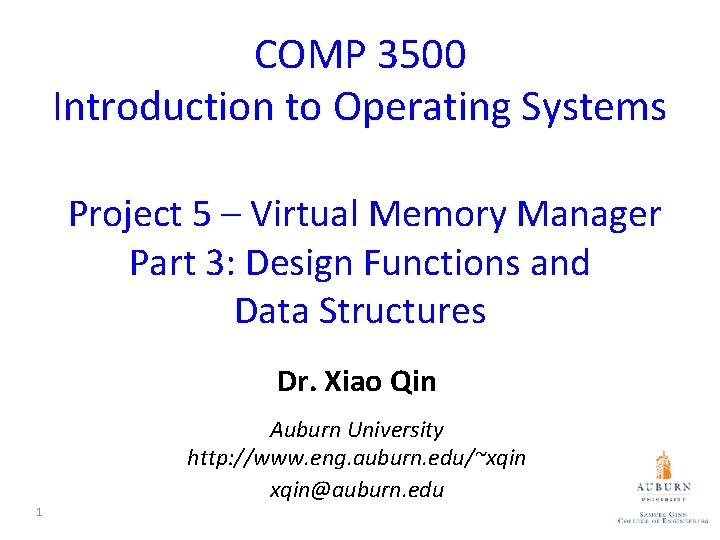
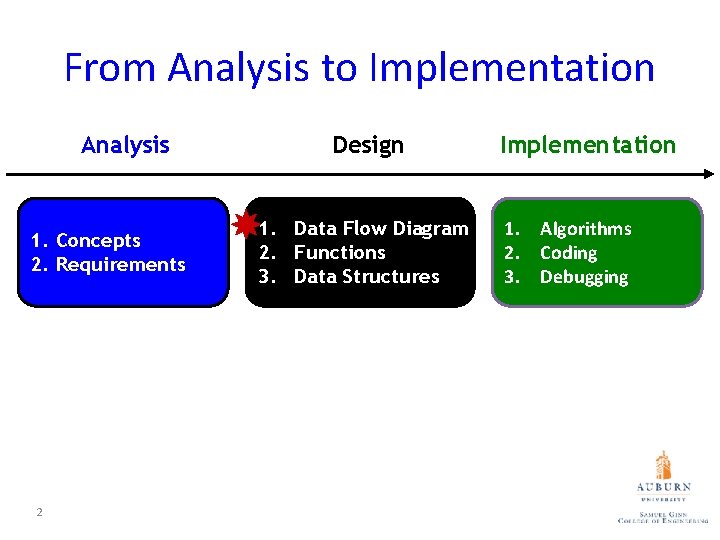
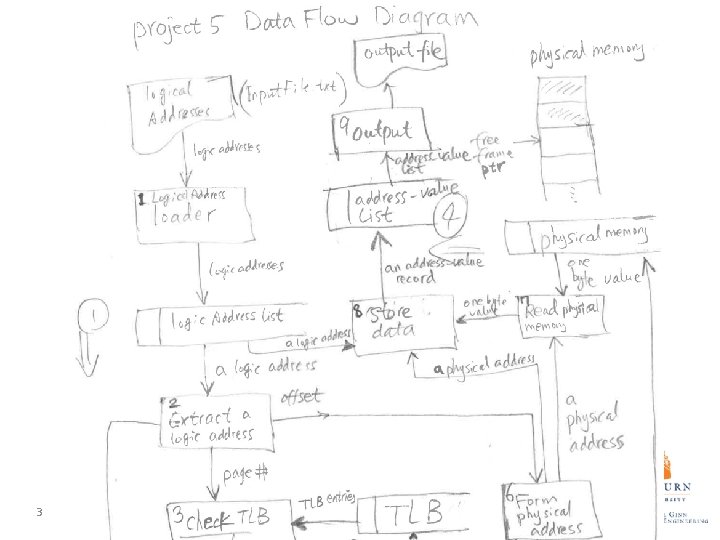
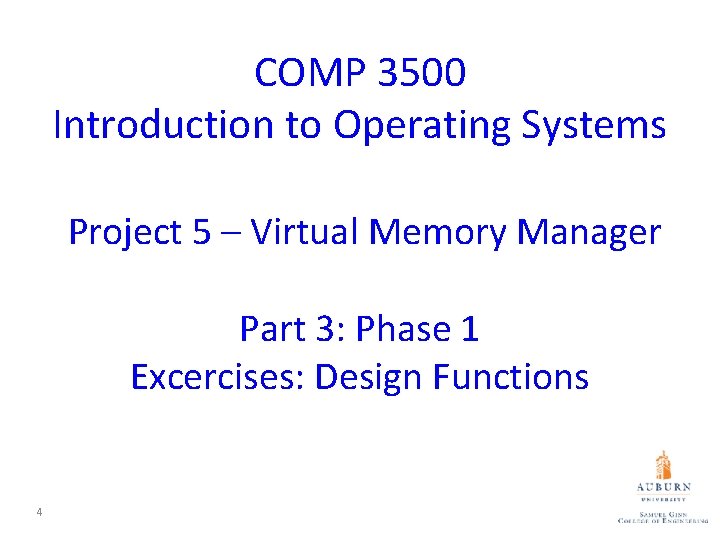
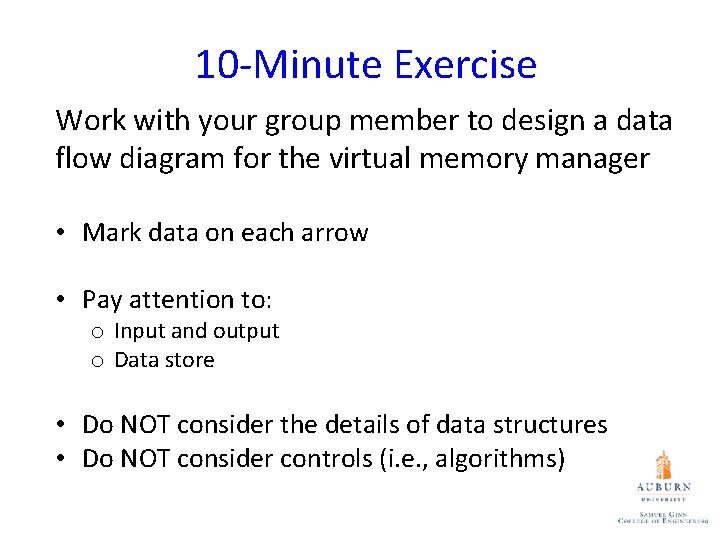
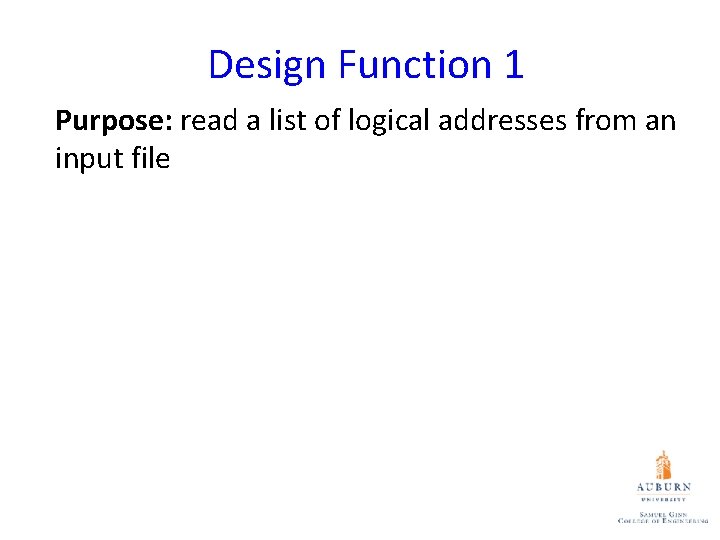
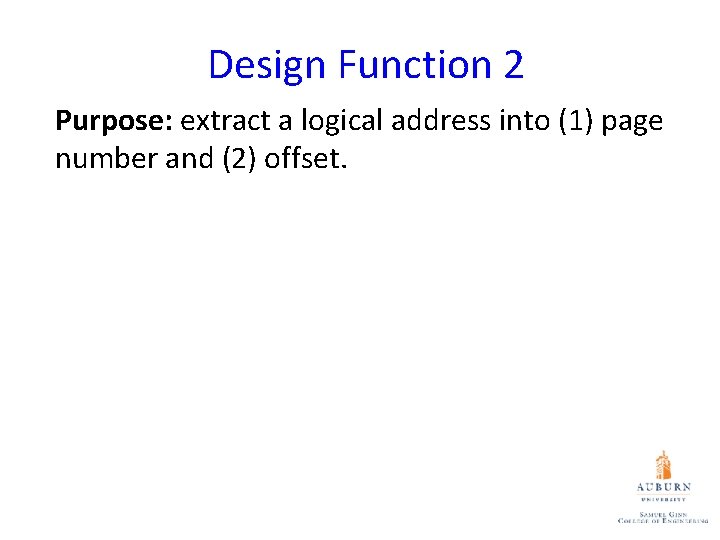
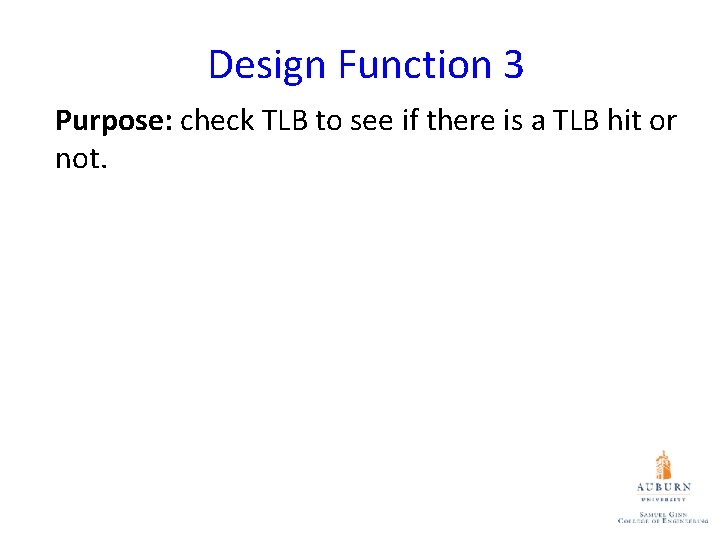
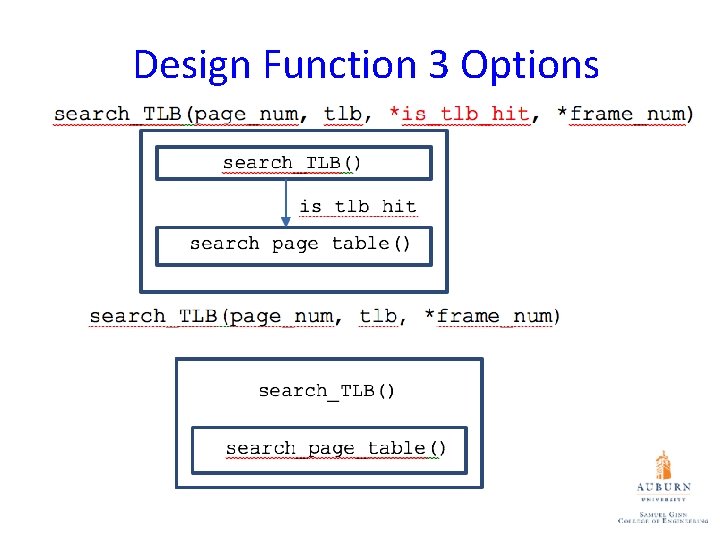
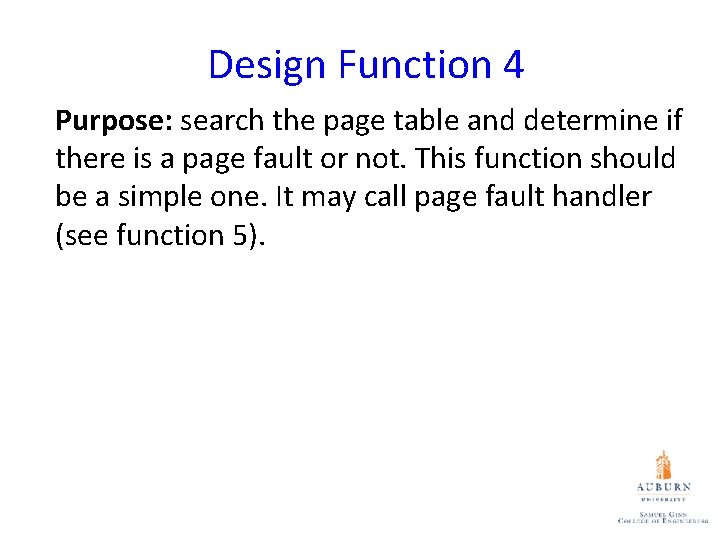
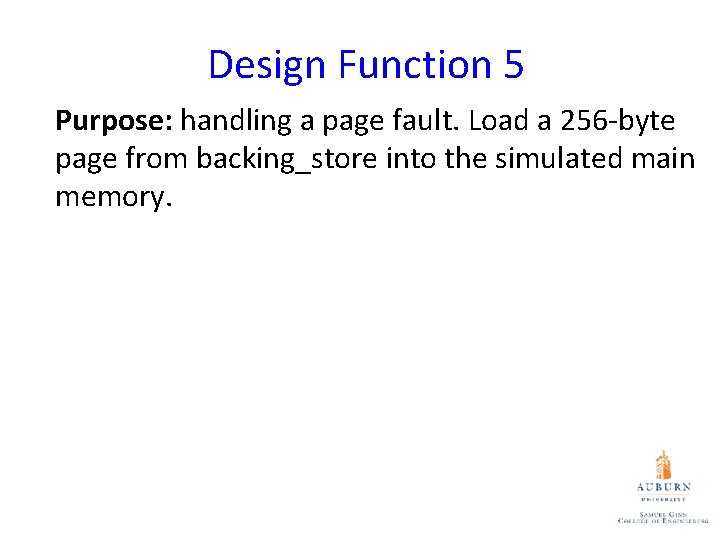
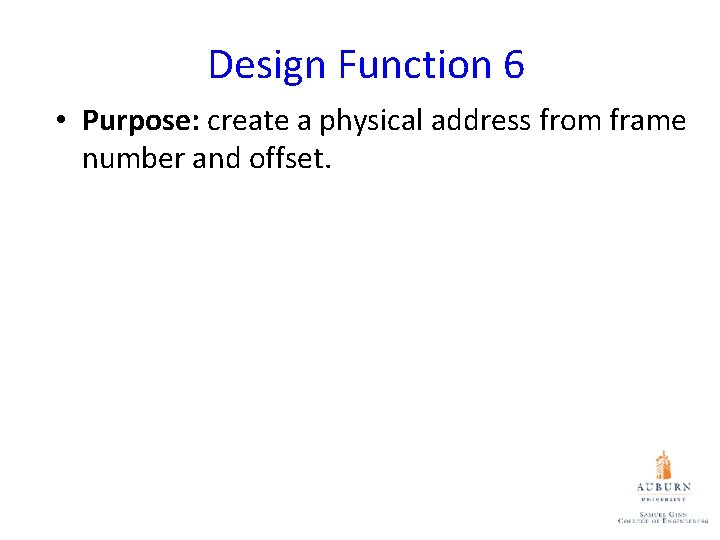
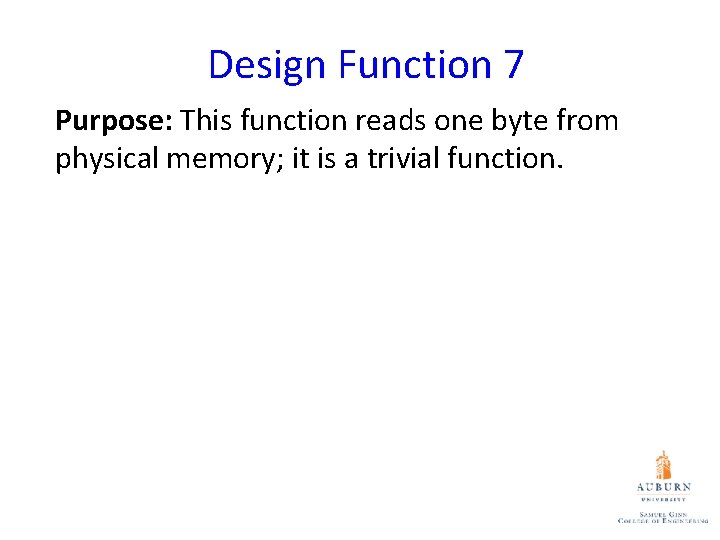
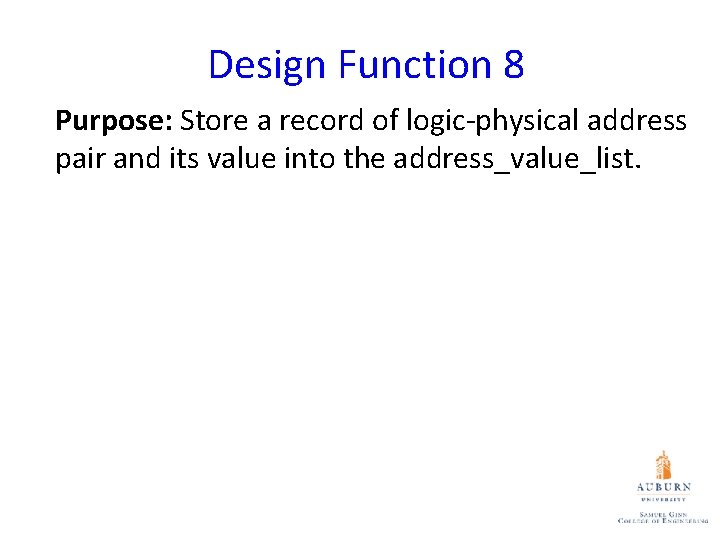
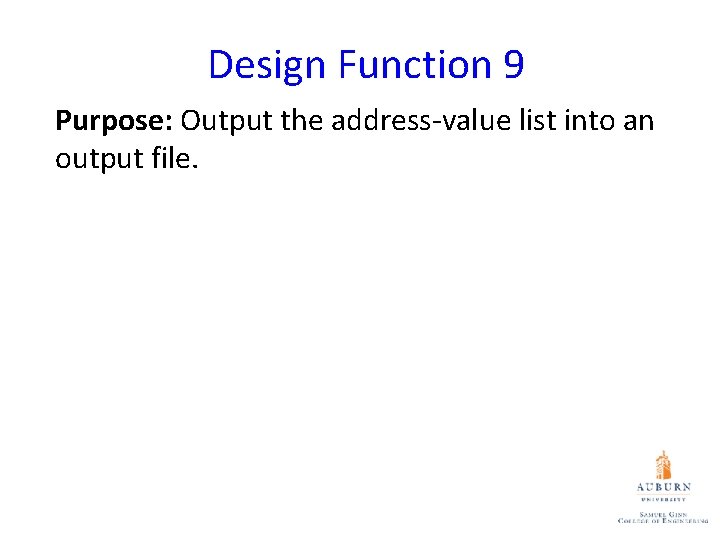
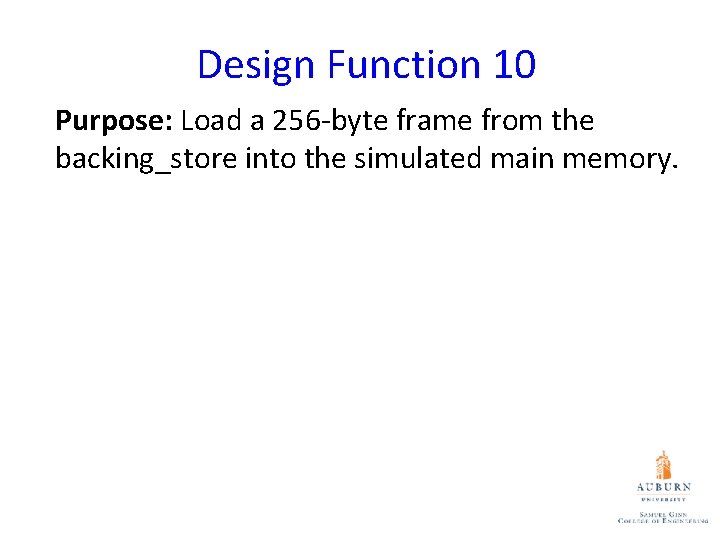
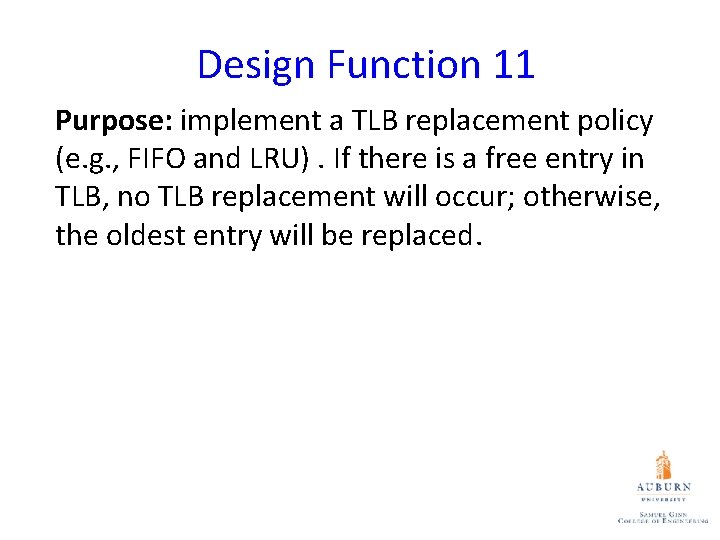
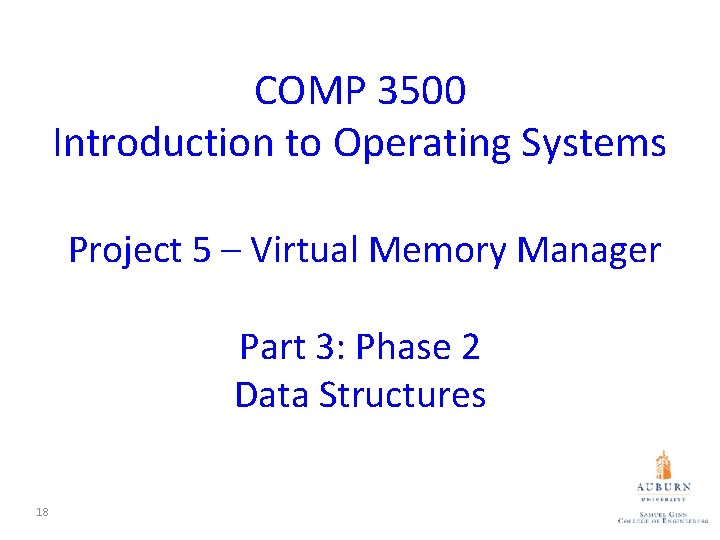
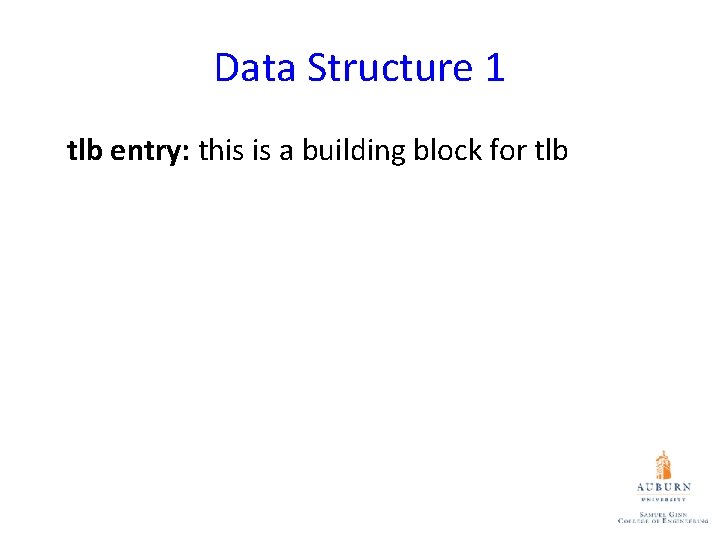
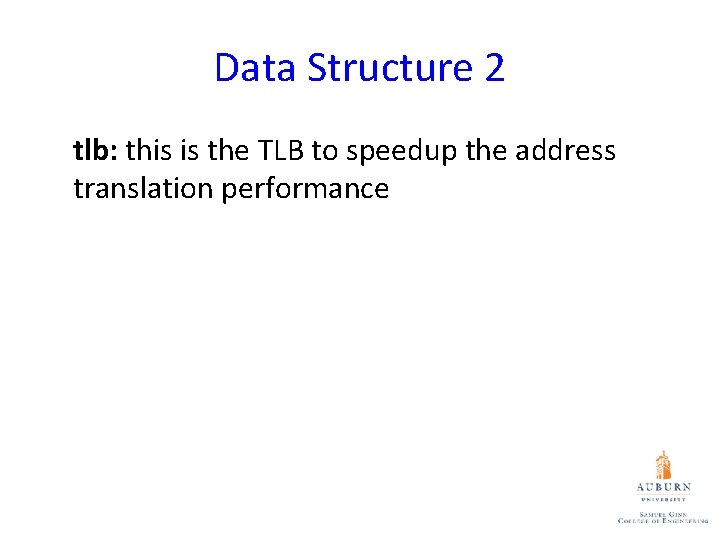
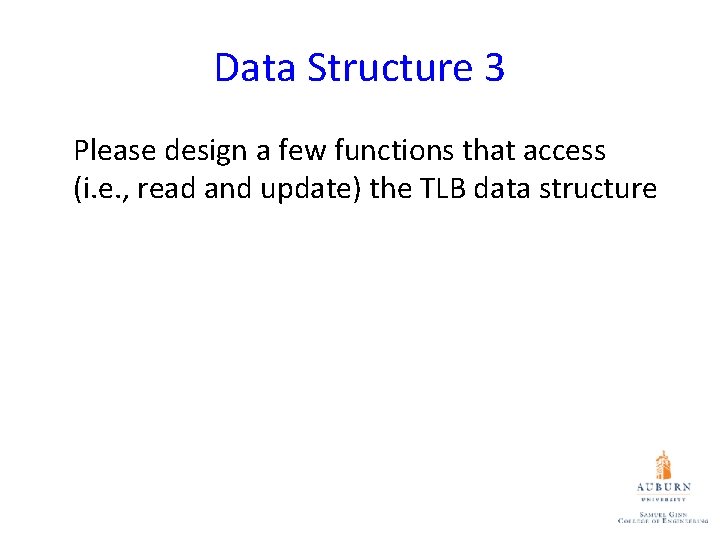
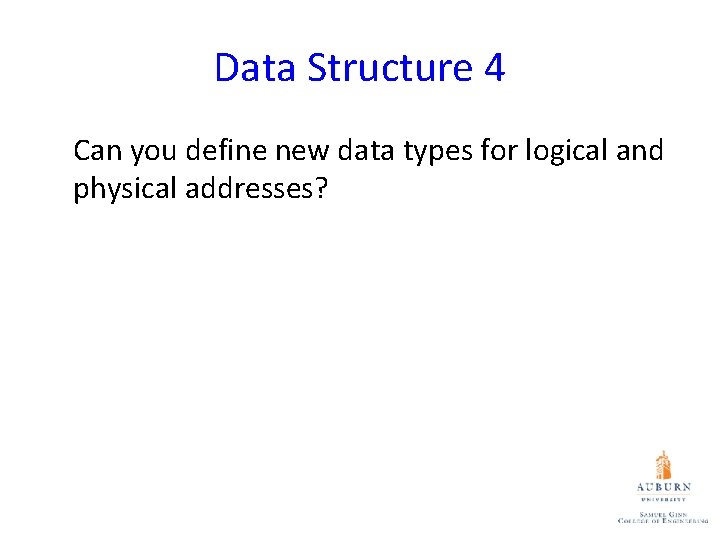
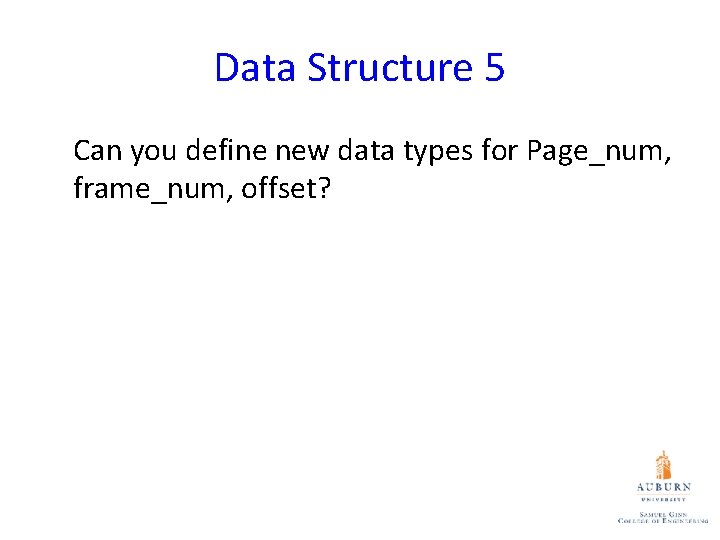
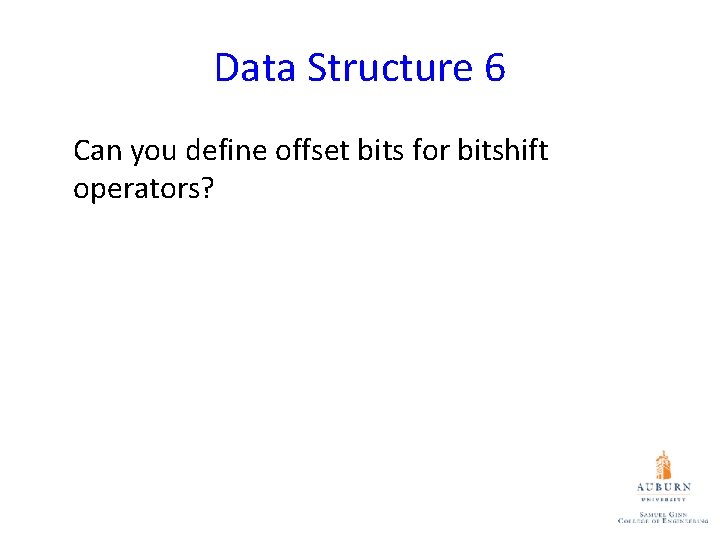
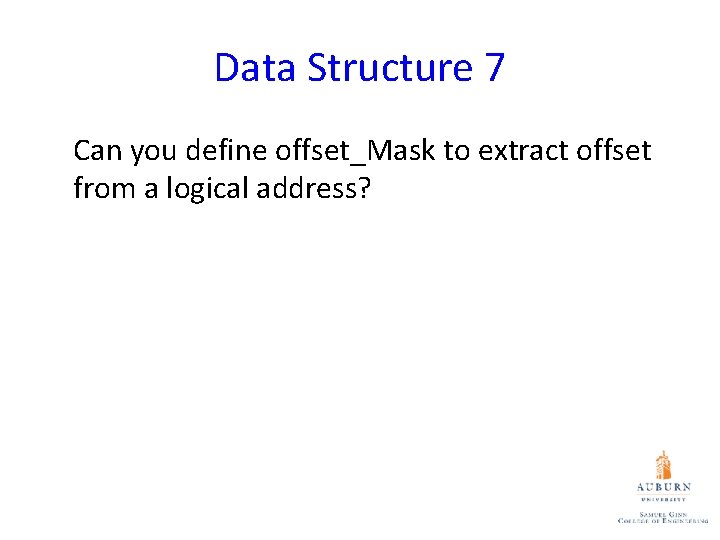
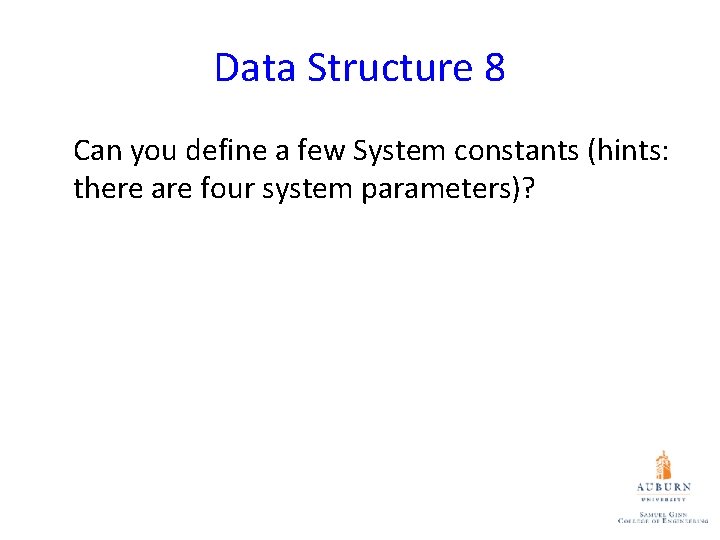
- Slides: 26
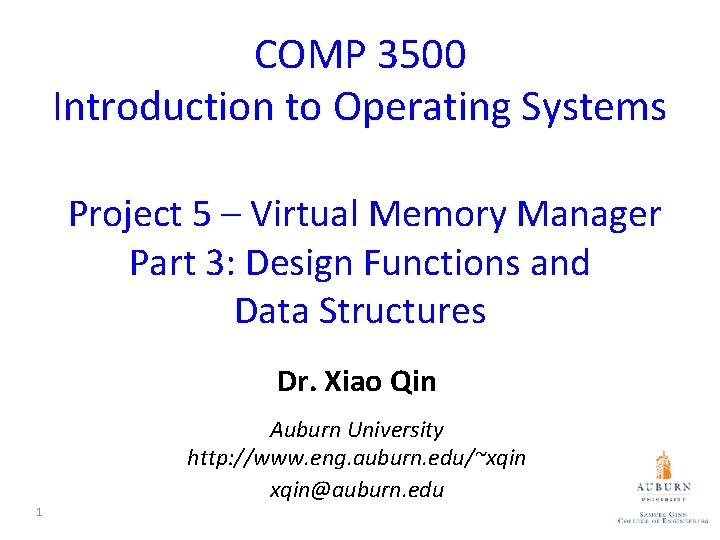
COMP 3500 Introduction to Operating Systems Project 5 – Virtual Memory Manager Part 3: Design Functions and Data Structures Dr. Xiao Qin Auburn University http: //www. eng. auburn. edu/~xqin@auburn. edu 1
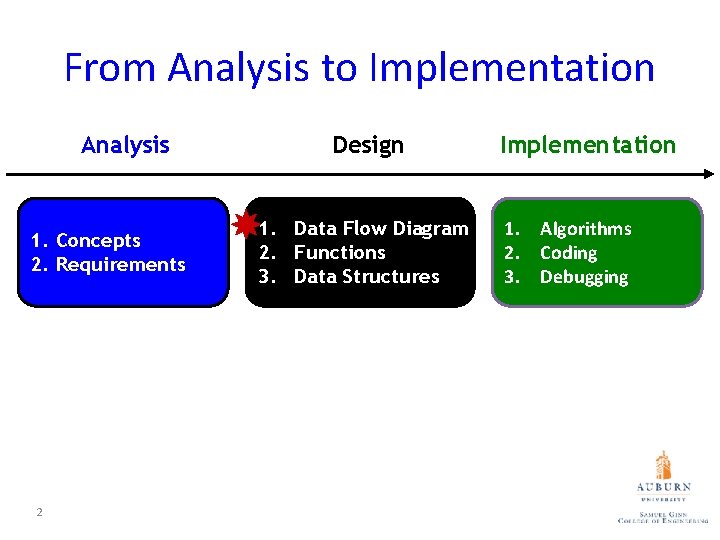
From Analysis to Implementation Analysis 1. Concepts 2. Requirements 2 Design 1. Data Flow Diagram 2. Functions 3. Data Structures Implementation 1. Algorithms 2. Coding 3. Debugging
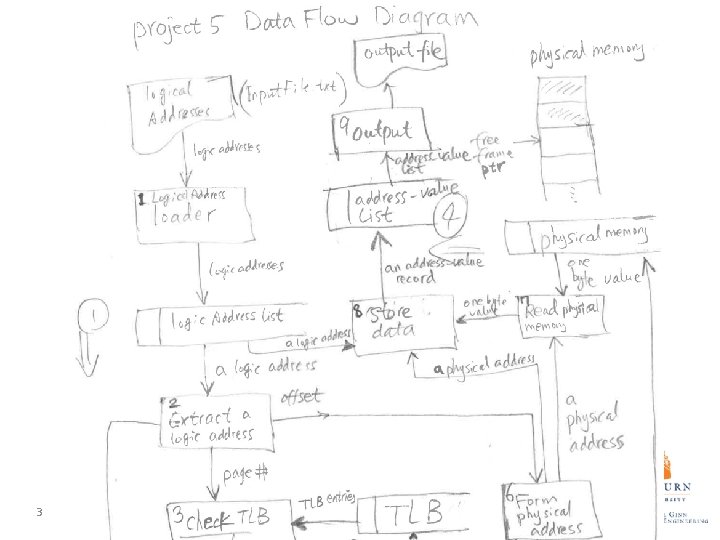
3
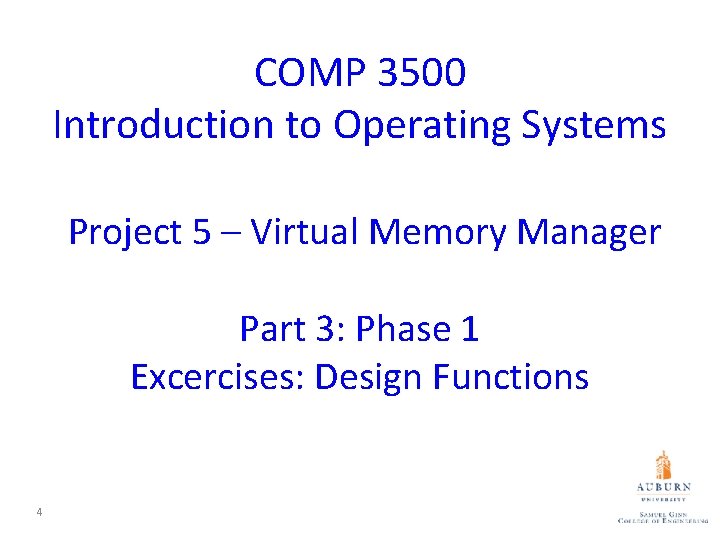
COMP 3500 Introduction to Operating Systems Project 5 – Virtual Memory Manager Part 3: Phase 1 Excercises: Design Functions 4
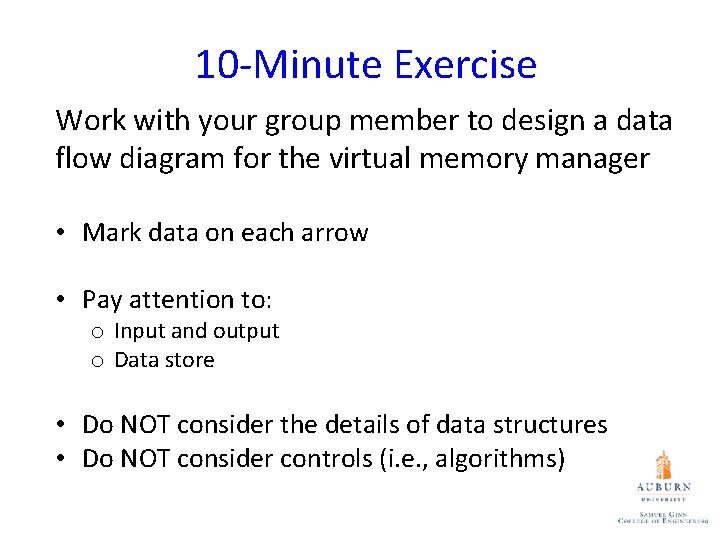
10 -Minute Exercise Work with your group member to design a data flow diagram for the virtual memory manager • Mark data on each arrow • Pay attention to: o Input and output o Data store • Do NOT consider the details of data structures • Do NOT consider controls (i. e. , algorithms)
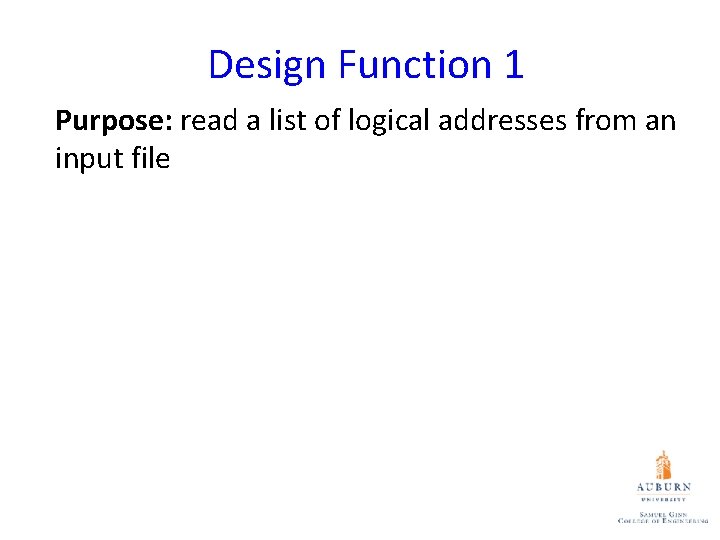
Design Function 1 Purpose: read a list of logical addresses from an input file
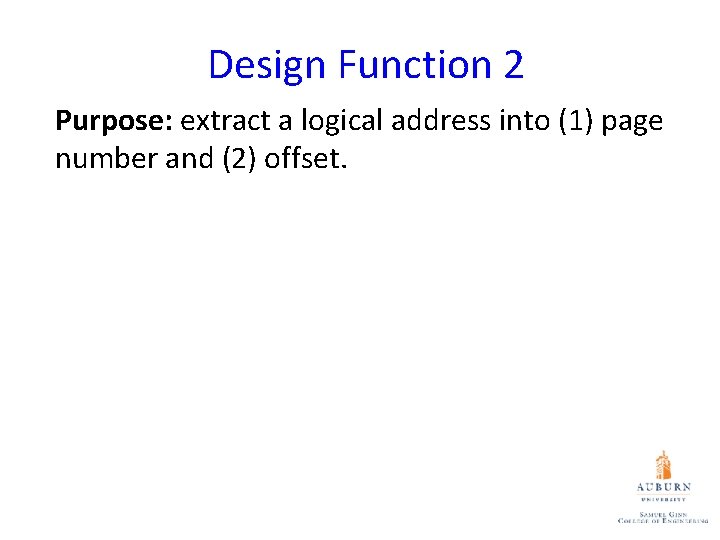
Design Function 2 Purpose: extract a logical address into (1) page number and (2) offset.
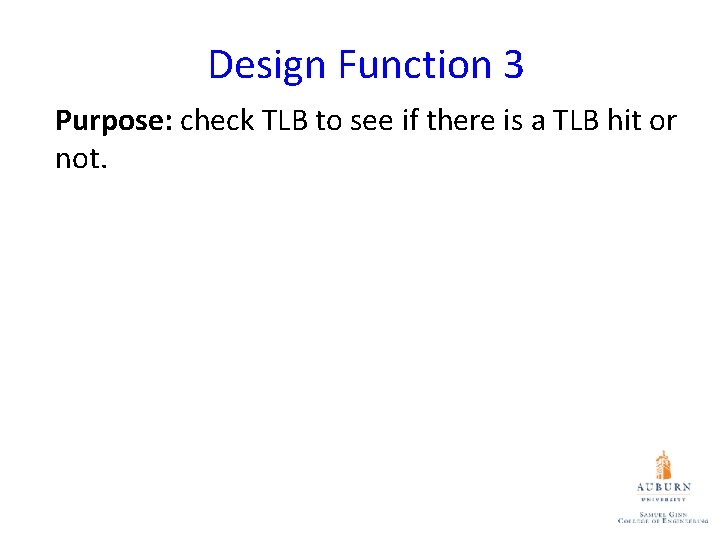
Design Function 3 Purpose: check TLB to see if there is a TLB hit or not.
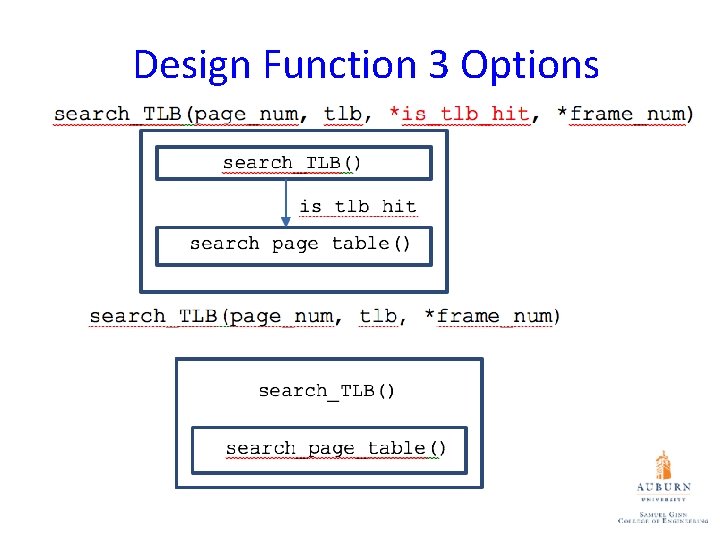
Design Function 3 Options
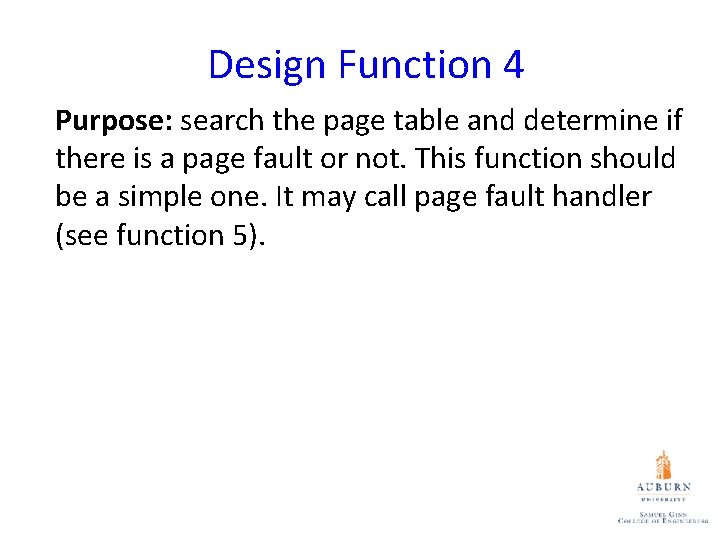
Design Function 4 Purpose: search the page table and determine if there is a page fault or not. This function should be a simple one. It may call page fault handler (see function 5).
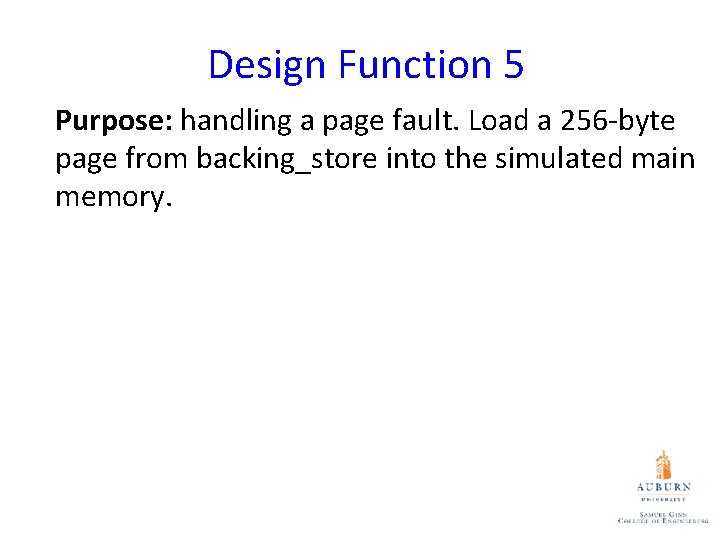
Design Function 5 Purpose: handling a page fault. Load a 256 -byte page from backing_store into the simulated main memory.
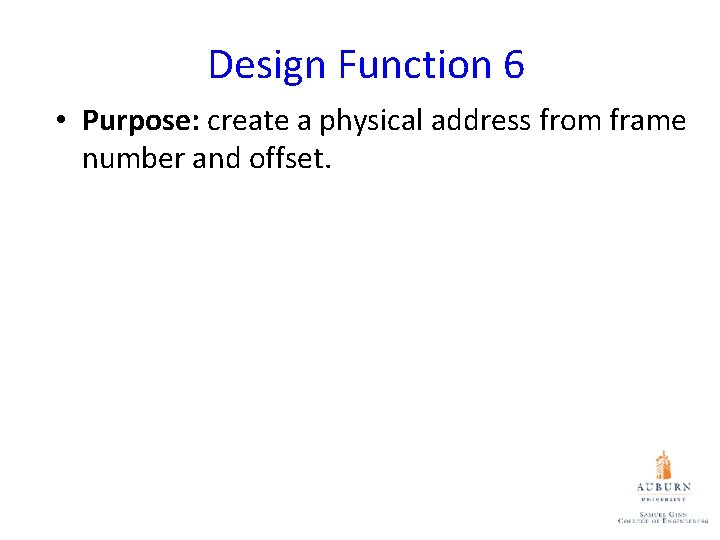
Design Function 6 • Purpose: create a physical address from frame number and offset.
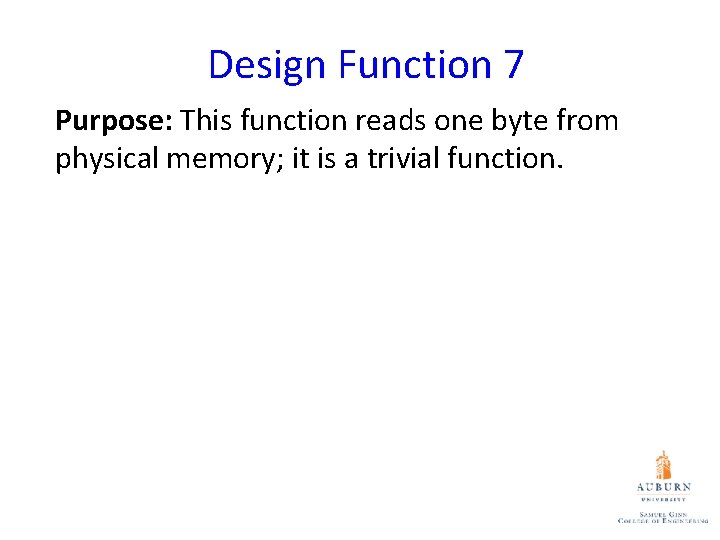
Design Function 7 Purpose: This function reads one byte from physical memory; it is a trivial function.
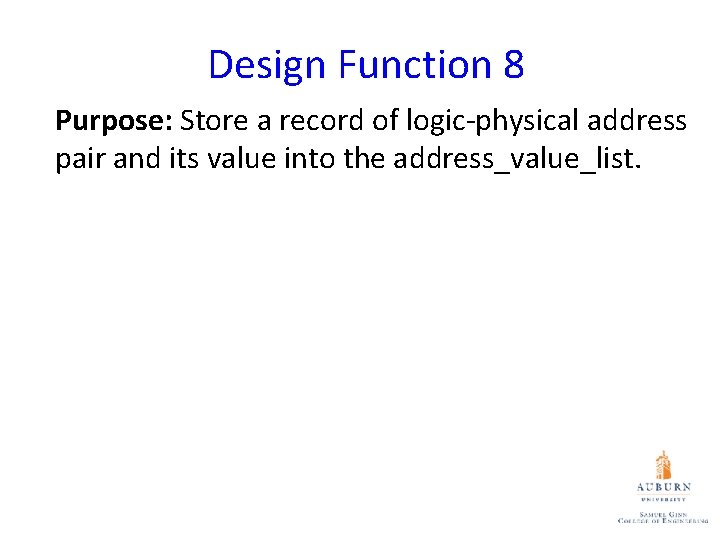
Design Function 8 Purpose: Store a record of logic-physical address pair and its value into the address_value_list.
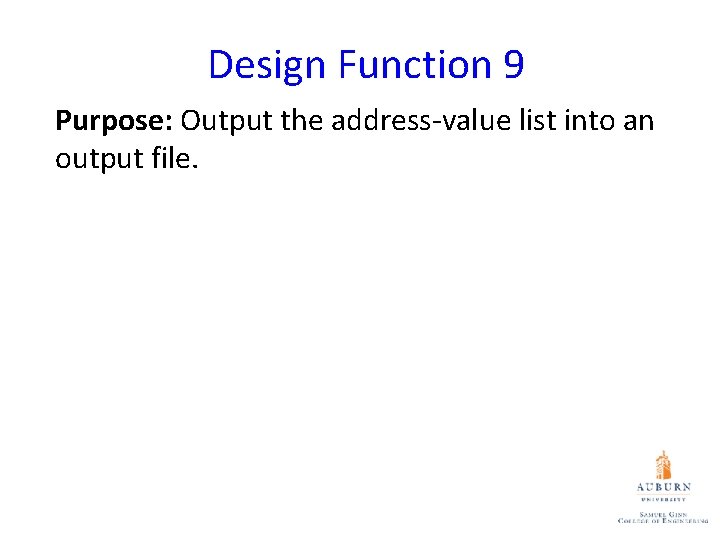
Design Function 9 Purpose: Output the address-value list into an output file.
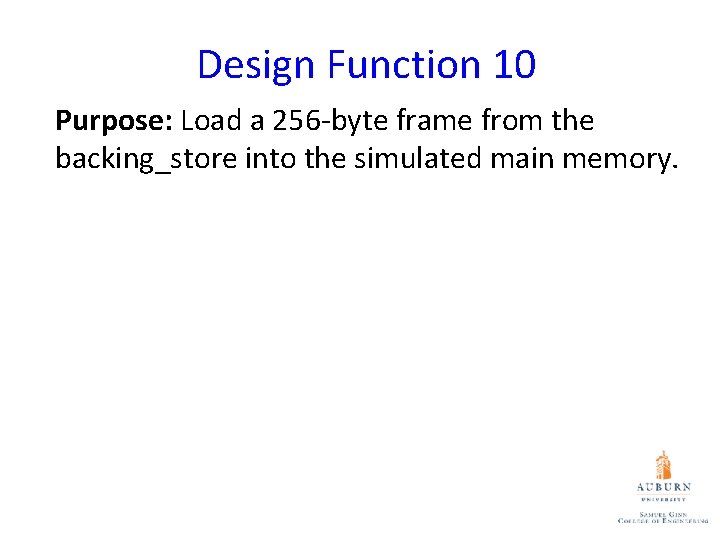
Design Function 10 Purpose: Load a 256 -byte frame from the backing_store into the simulated main memory.
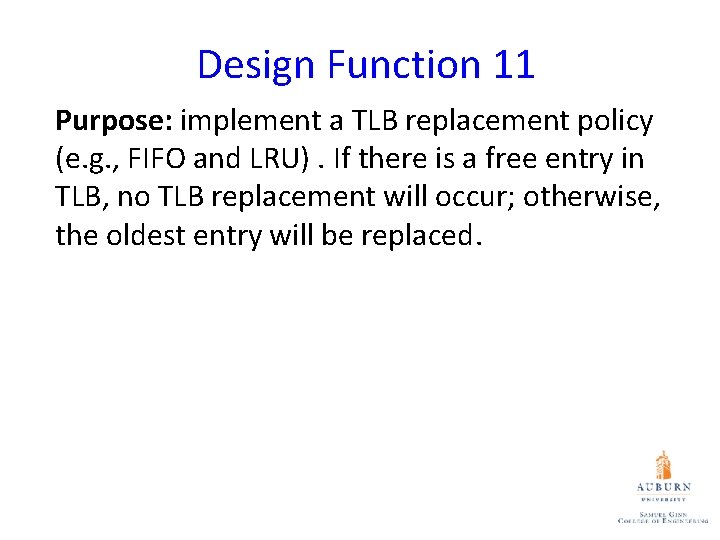
Design Function 11 Purpose: implement a TLB replacement policy (e. g. , FIFO and LRU). If there is a free entry in TLB, no TLB replacement will occur; otherwise, the oldest entry will be replaced.
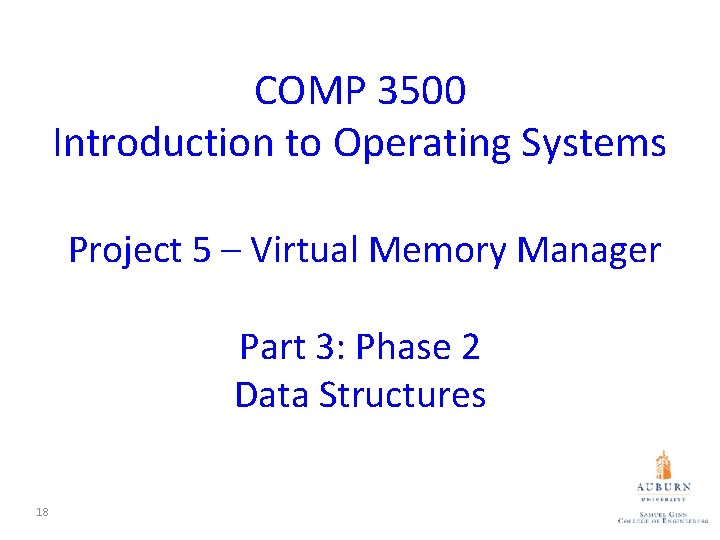
COMP 3500 Introduction to Operating Systems Project 5 – Virtual Memory Manager Part 3: Phase 2 Data Structures 18
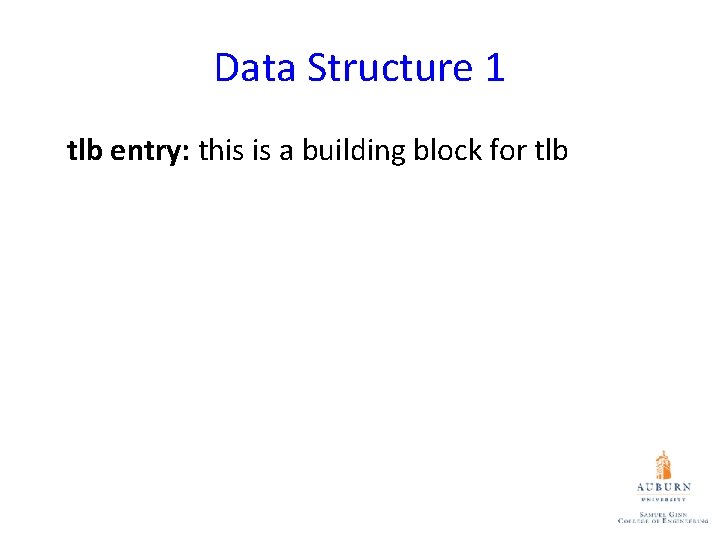
Data Structure 1 tlb entry: this is a building block for tlb
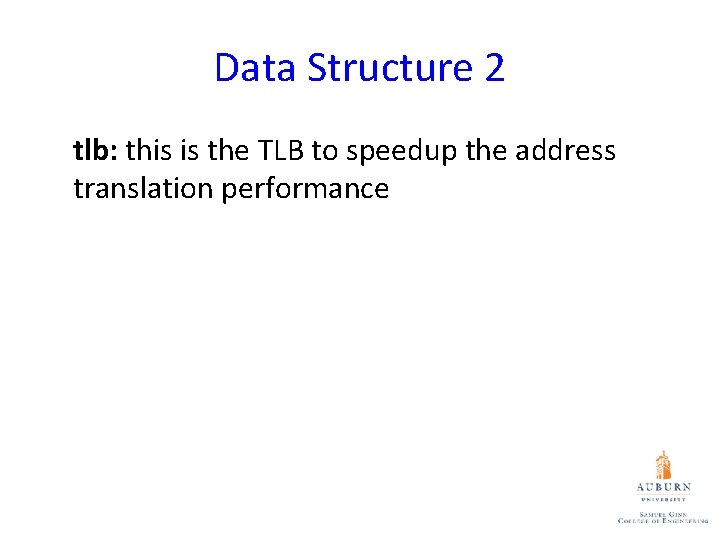
Data Structure 2 tlb: this is the TLB to speedup the address translation performance
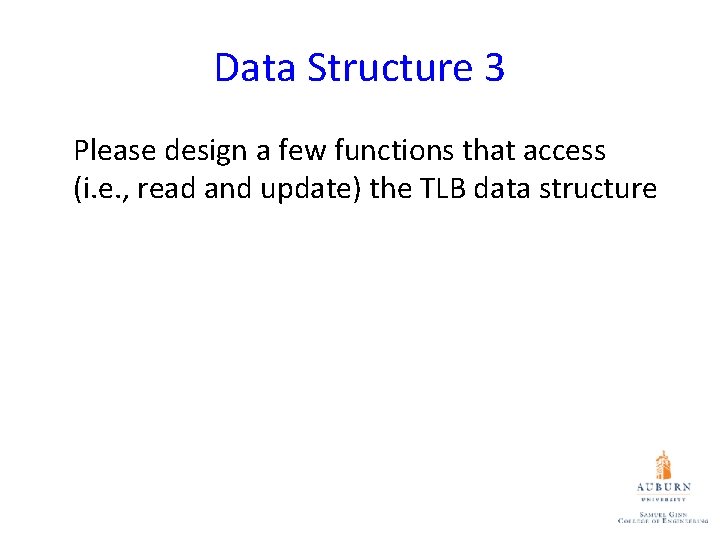
Data Structure 3 Please design a few functions that access (i. e. , read and update) the TLB data structure
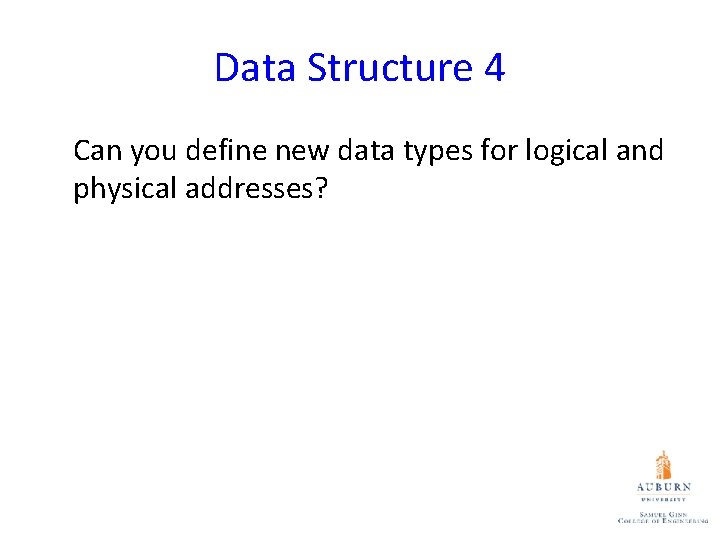
Data Structure 4 Can you define new data types for logical and physical addresses?
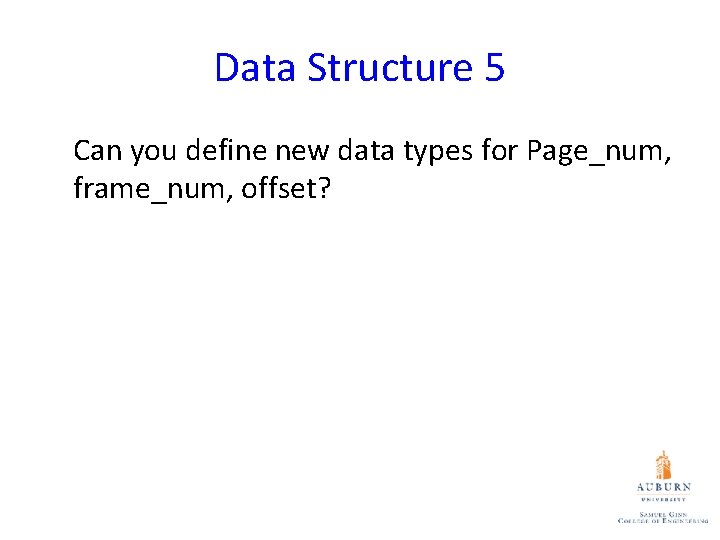
Data Structure 5 Can you define new data types for Page_num, frame_num, offset?
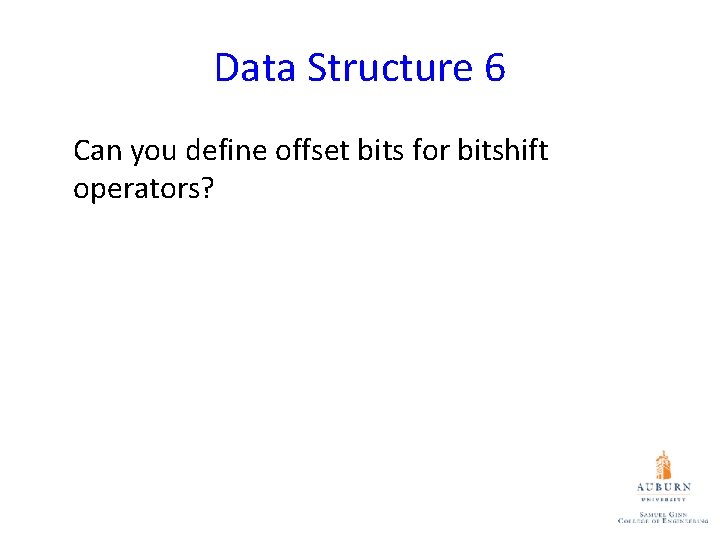
Data Structure 6 Can you define offset bits for bitshift operators?
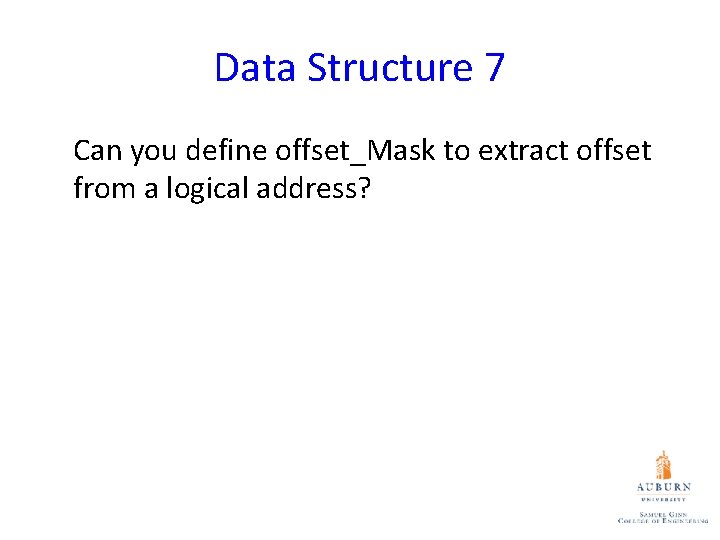
Data Structure 7 Can you define offset_Mask to extract offset from a logical address?
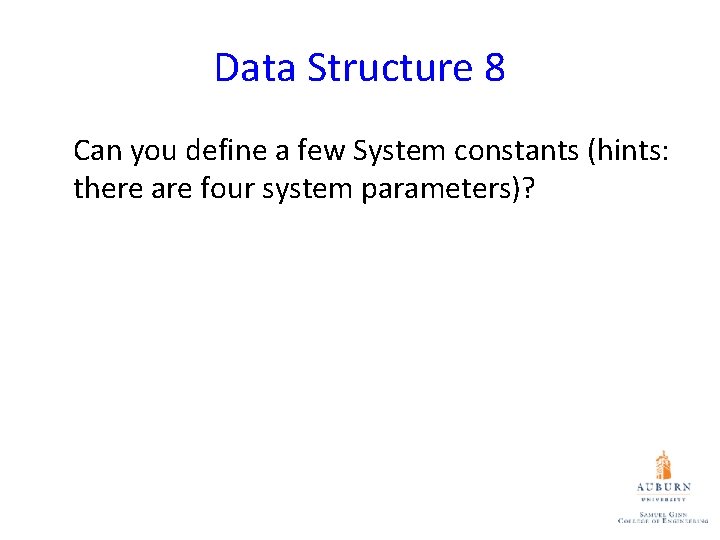
Data Structure 8 Can you define a few System constants (hints: there are four system parameters)?
Comp3500
Comp 3500
Dual mode in os
Bosch tronic 3500
3500 - 500
3500bce
The wheel 3500 bc
Philips lbb 3500
Athenian agora map
Bosch tronic heat
3500 + 1700
Egyptian
Monotheism
Ibm ts 3500
15000000/6000
Mesopotamia floodplain
Bosch tronic 3500
3750 stack
Proyecto andina
3500/3000
3 examples of operating system
Evolution of operating systems
Components of an operating system
Operating system components
Wsn operating systems
Arpaci dusseau operating systems
Operating systems lab