COMP 2710 Software Construction Homework 5 Design and
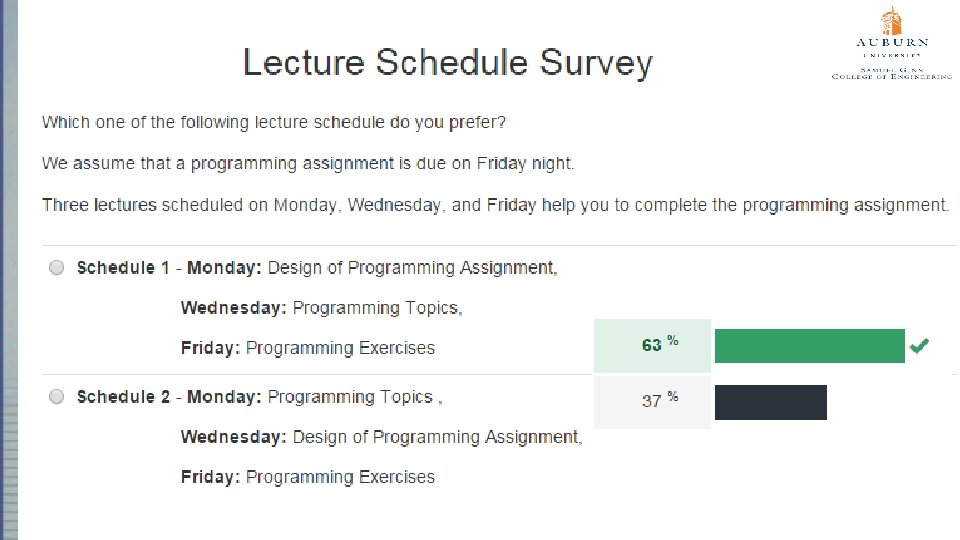
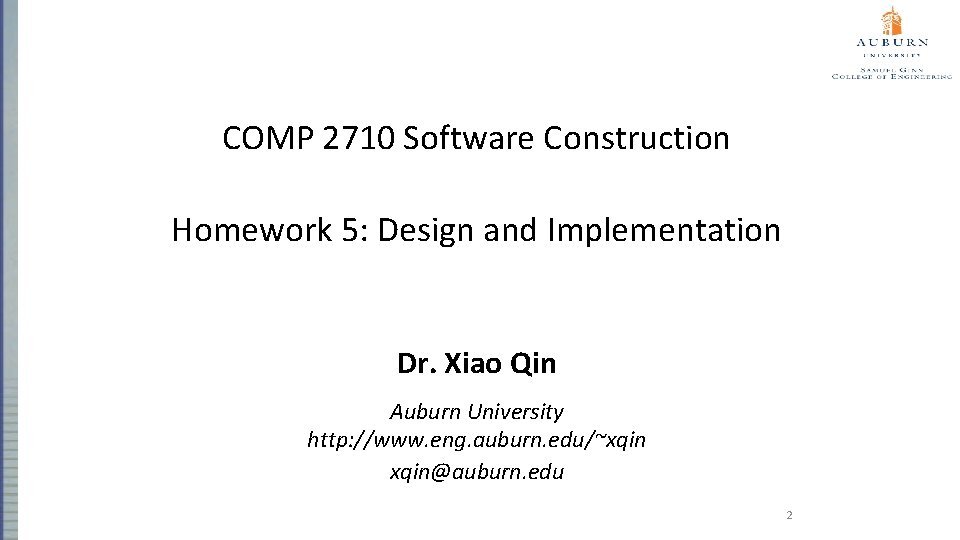
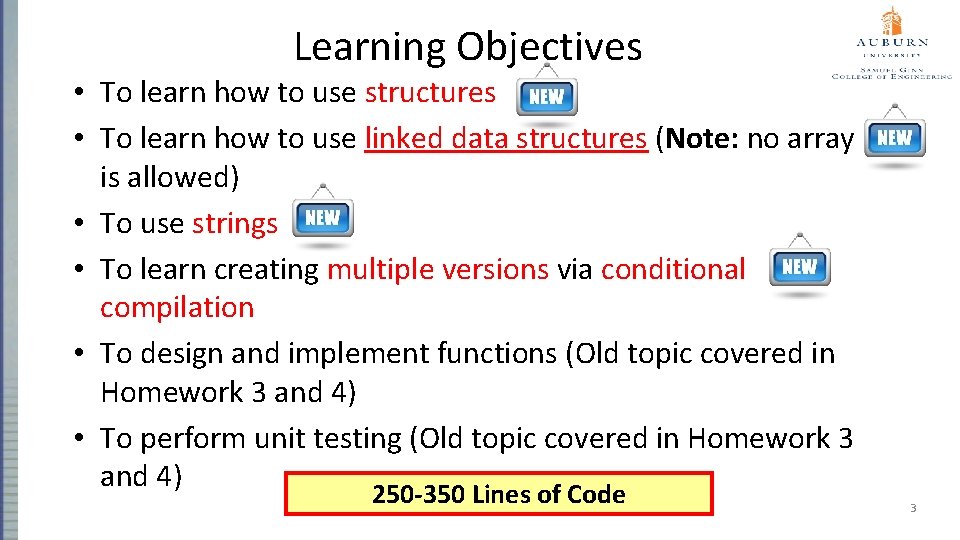
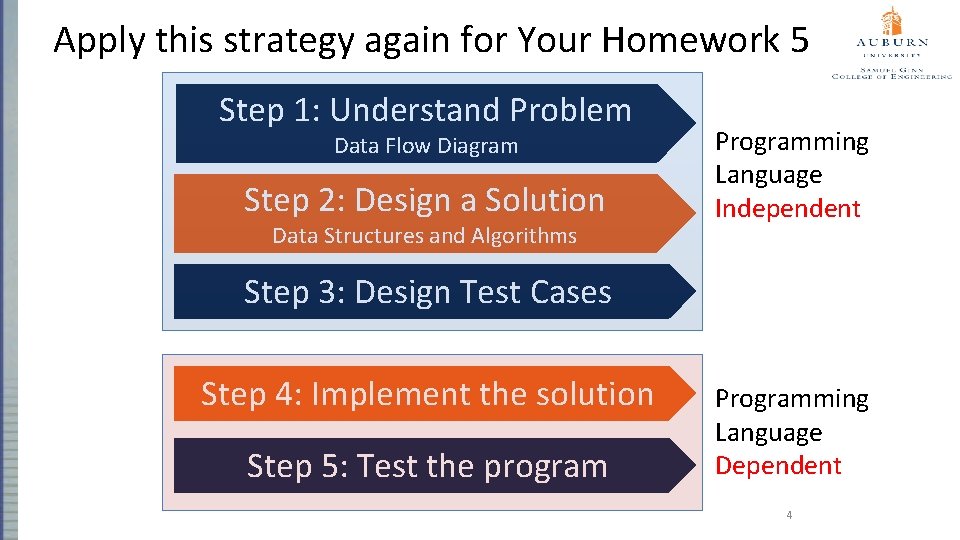
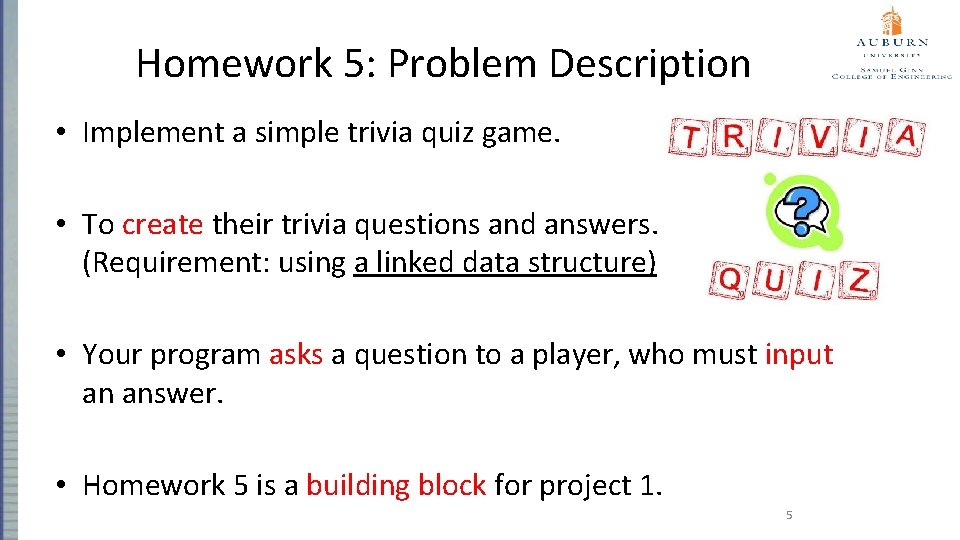
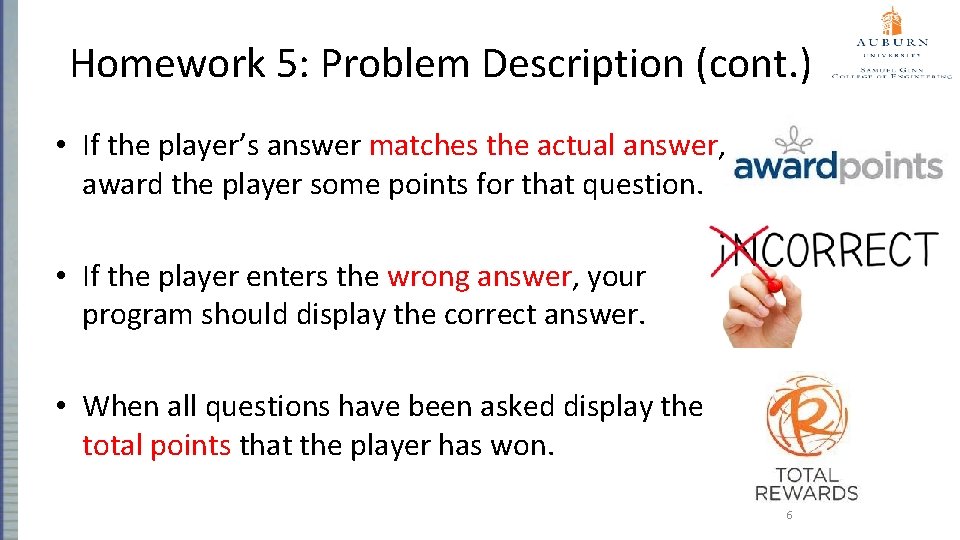
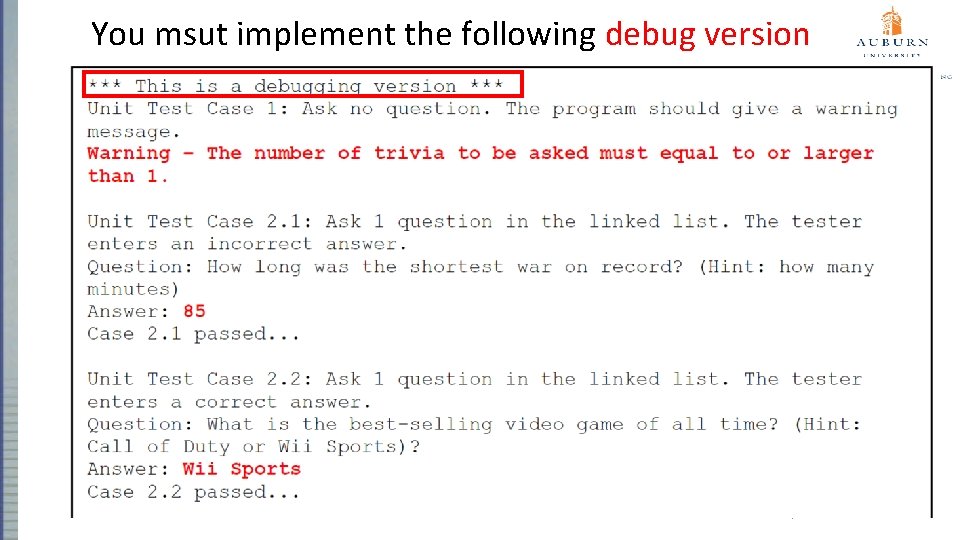
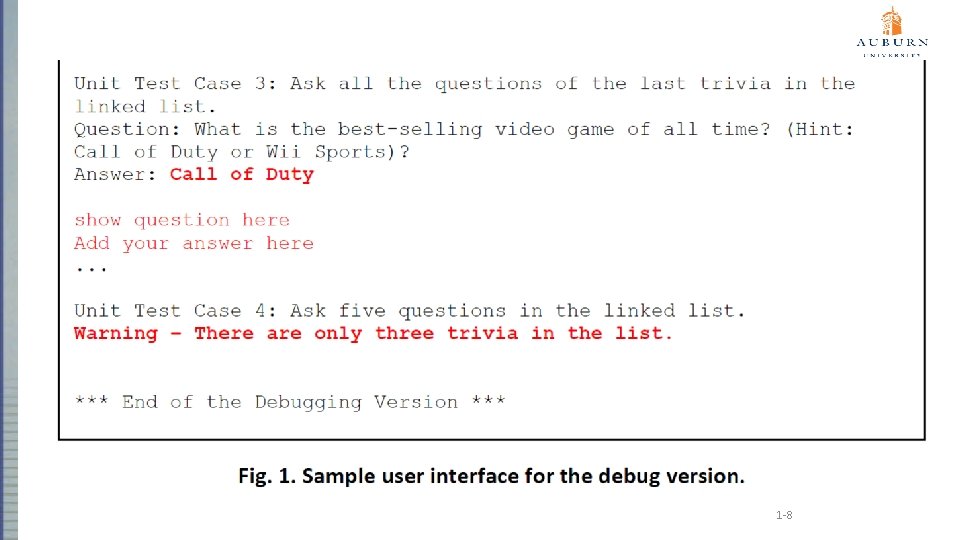
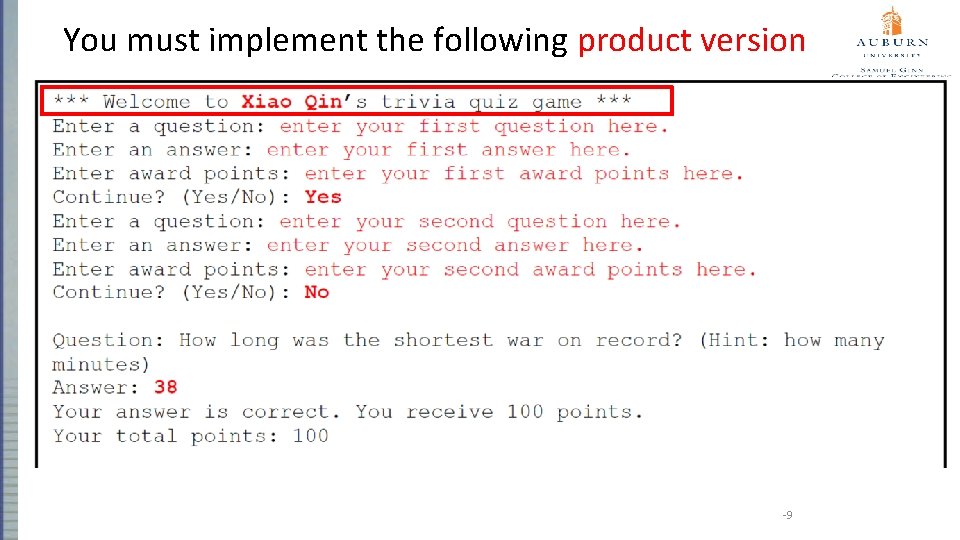
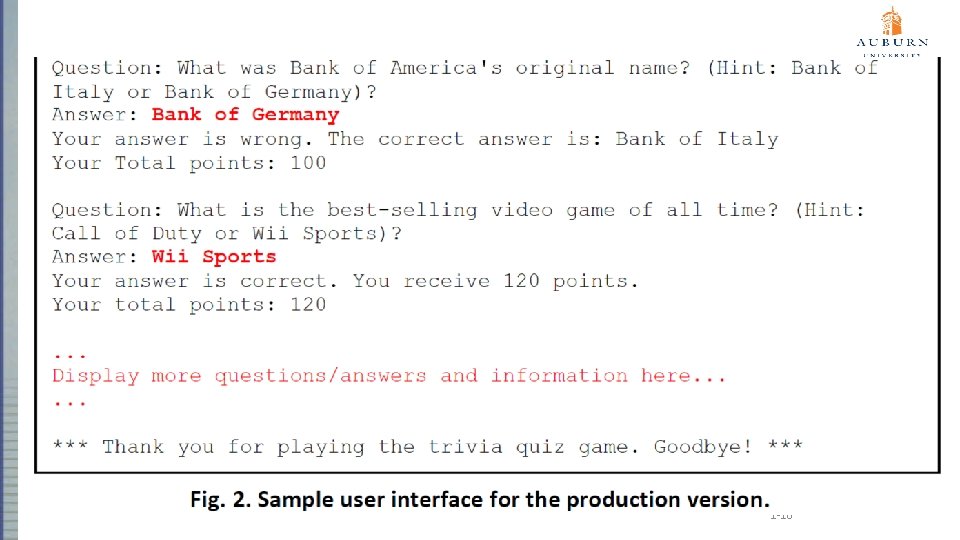
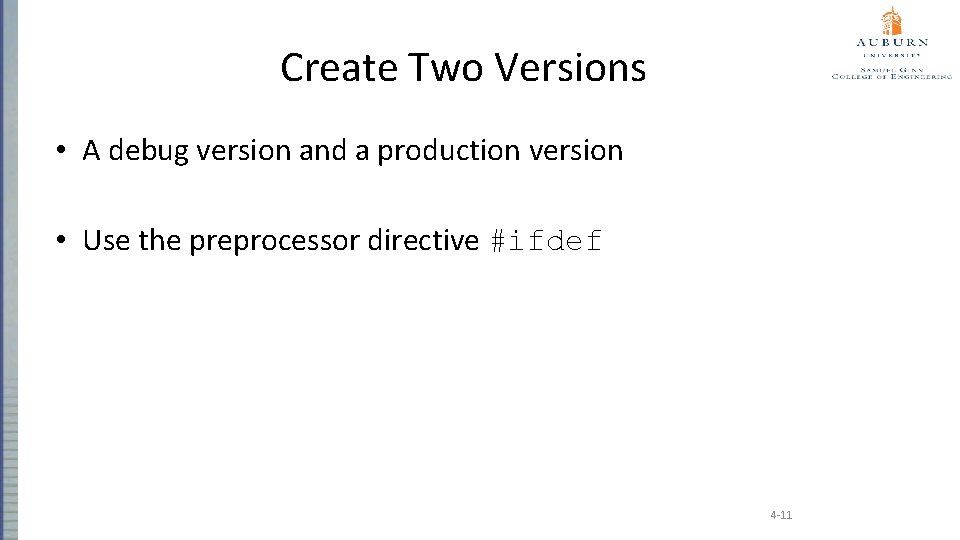
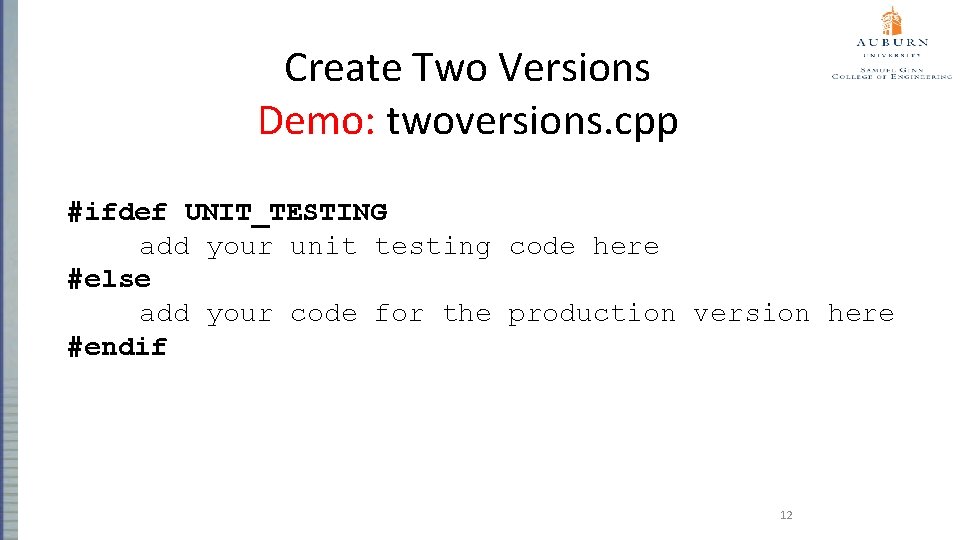
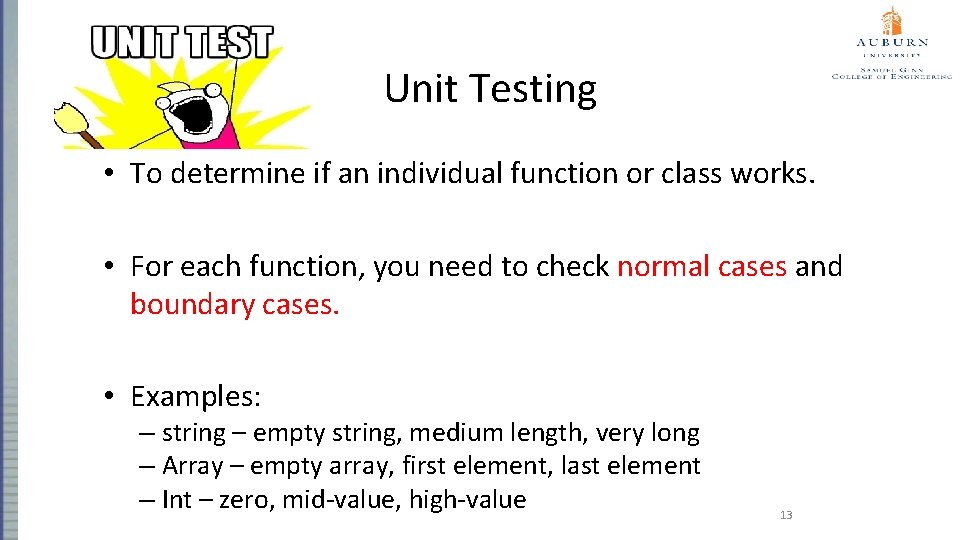
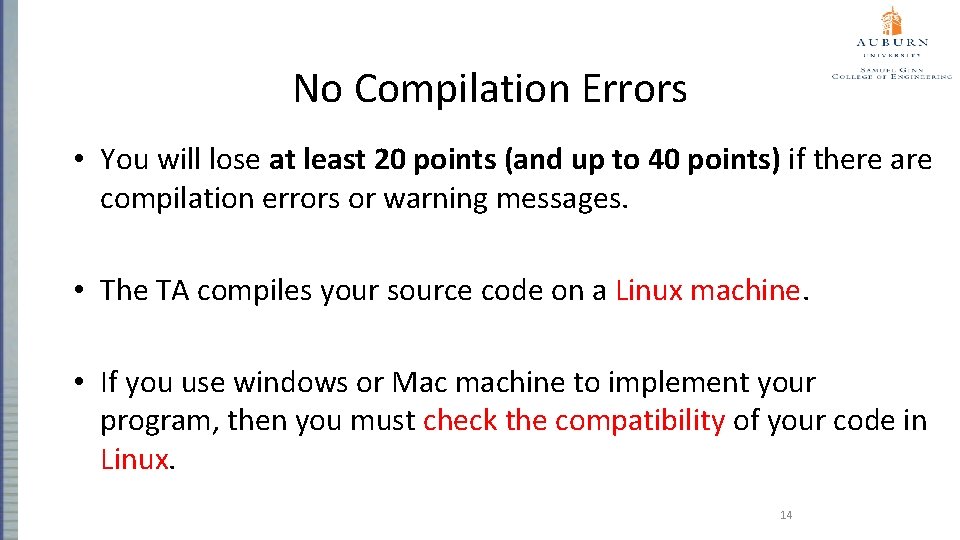
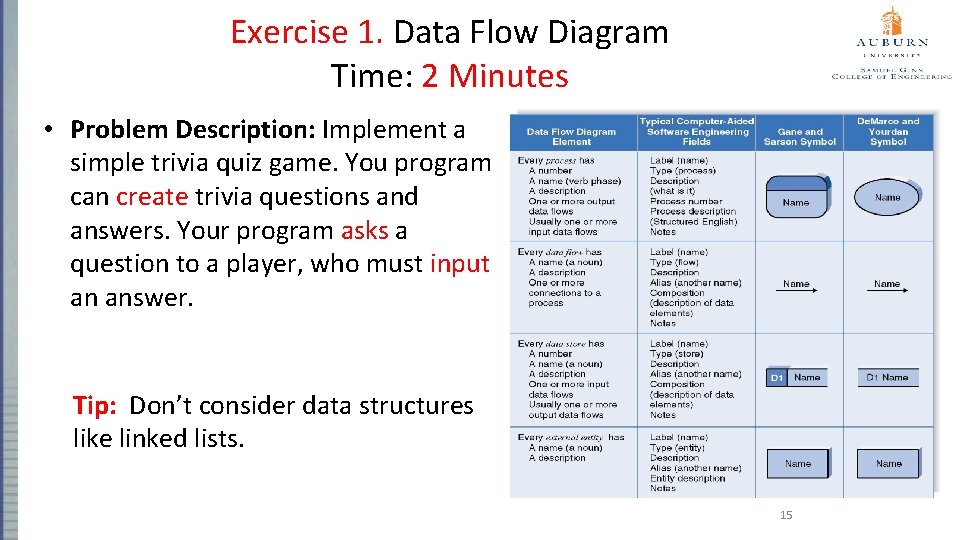
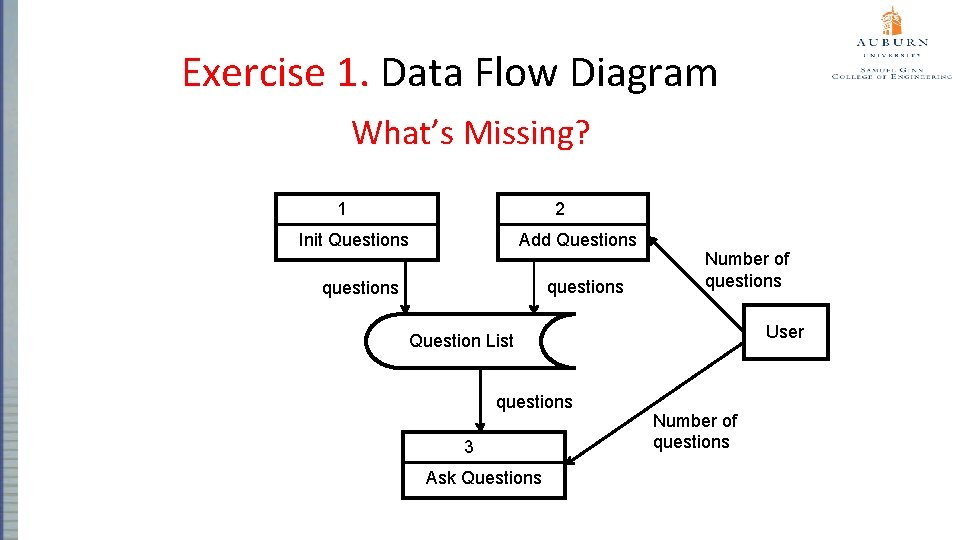
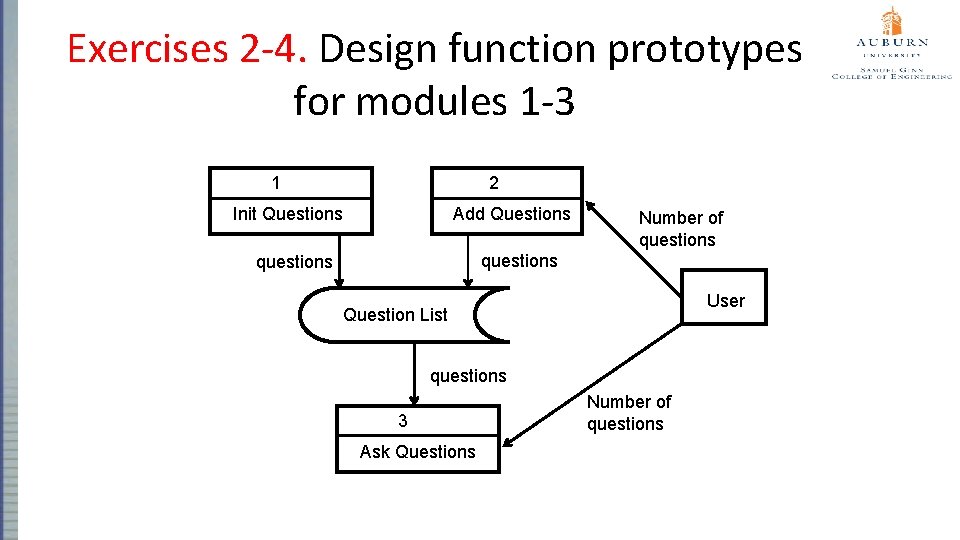
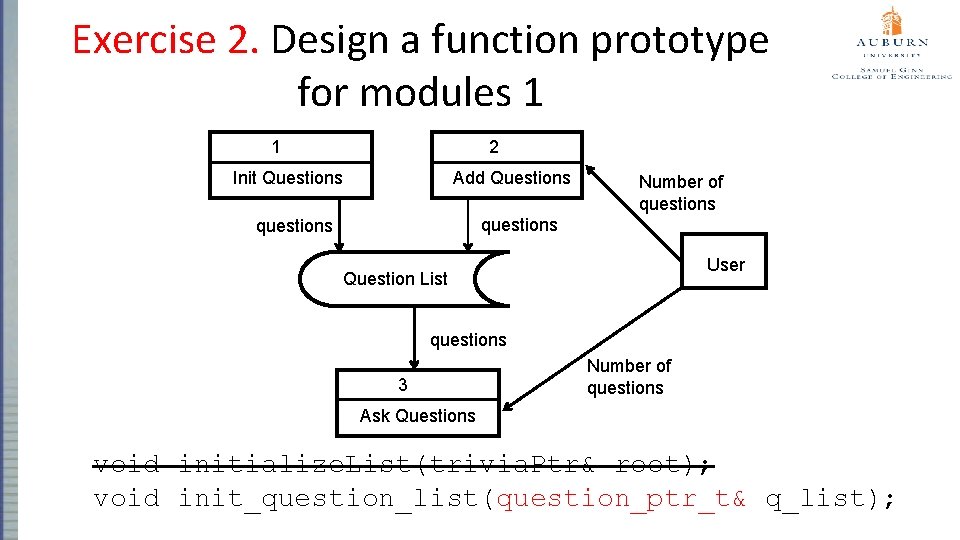
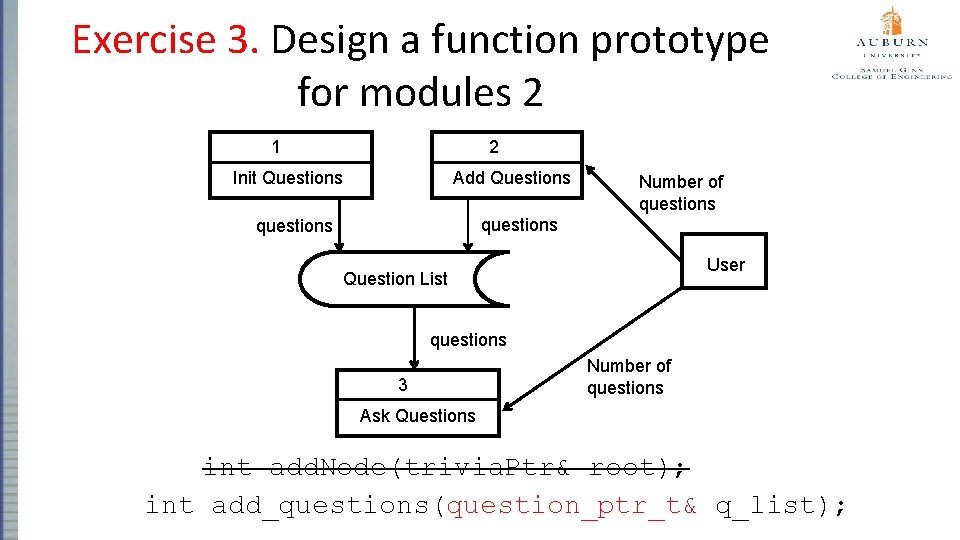
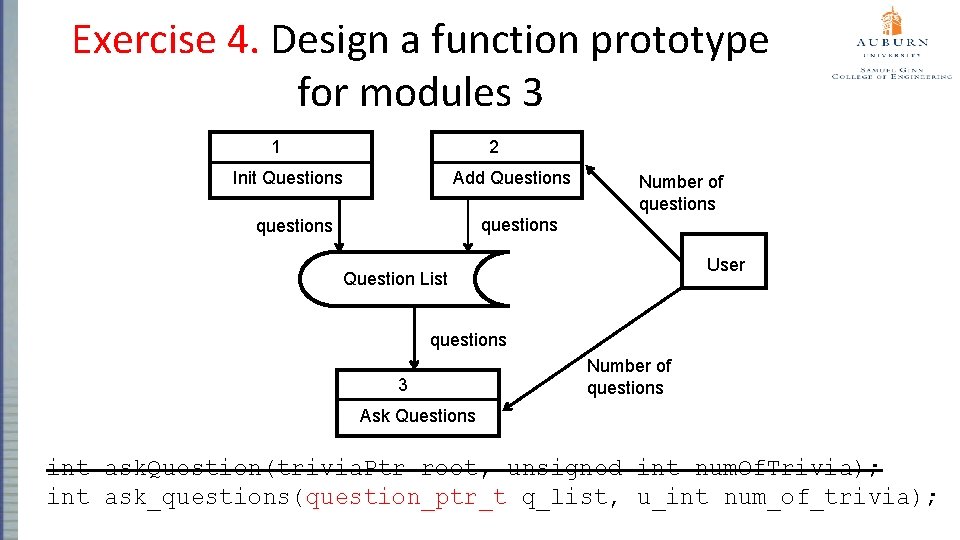
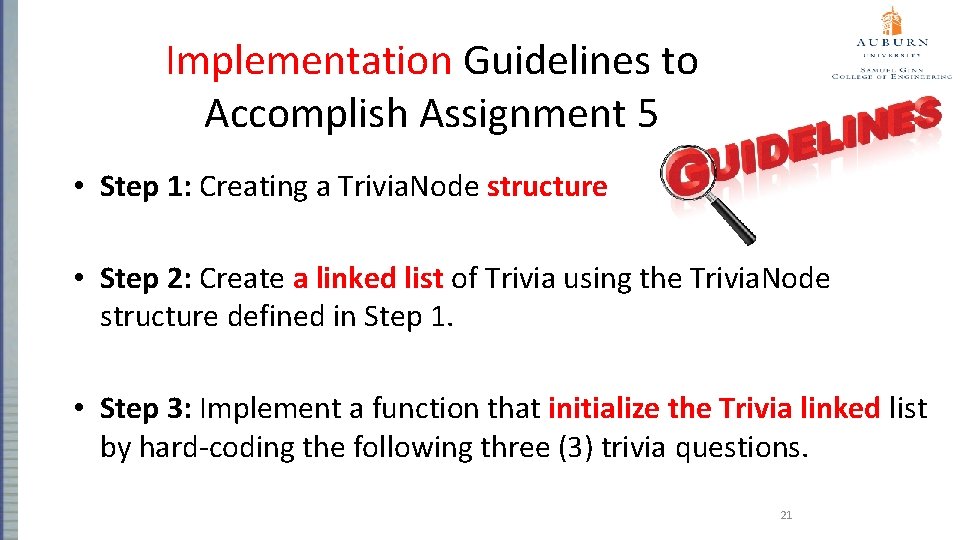
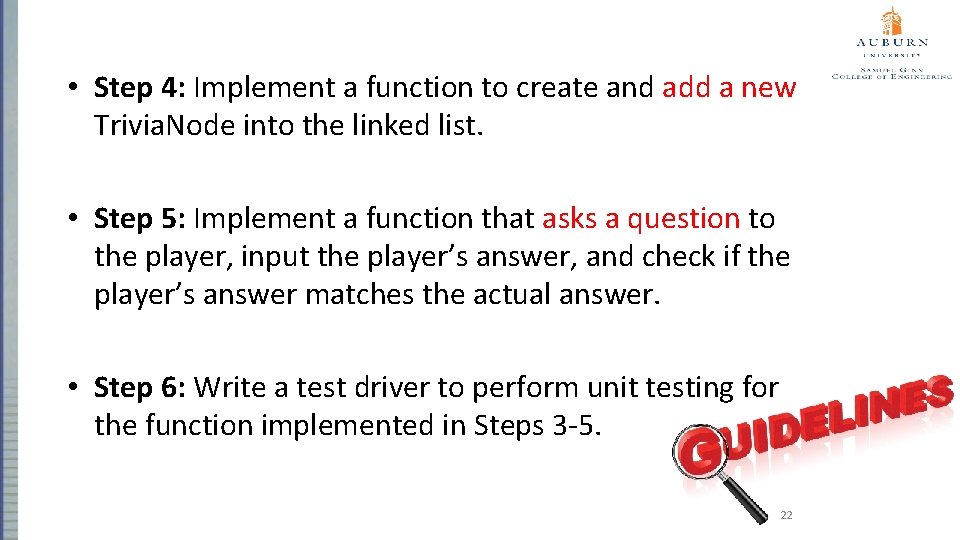
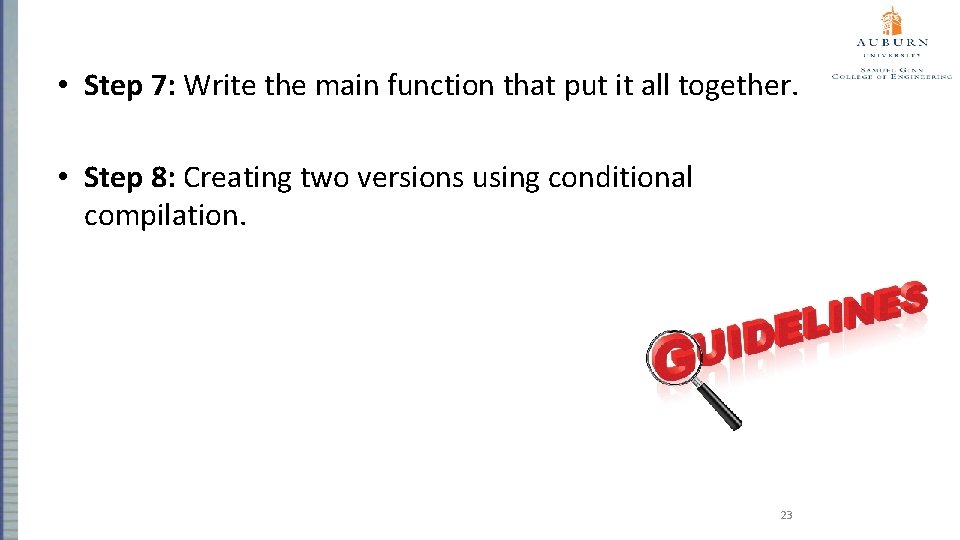
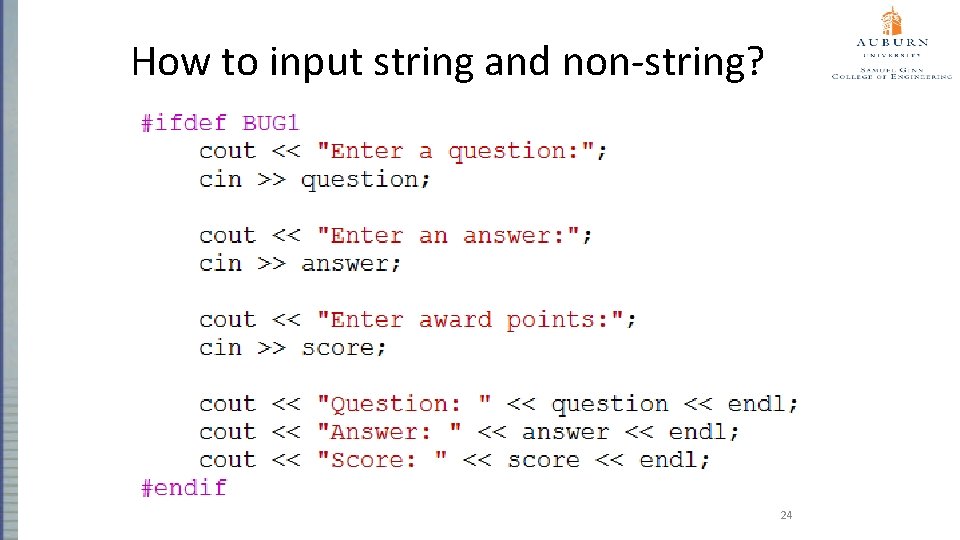
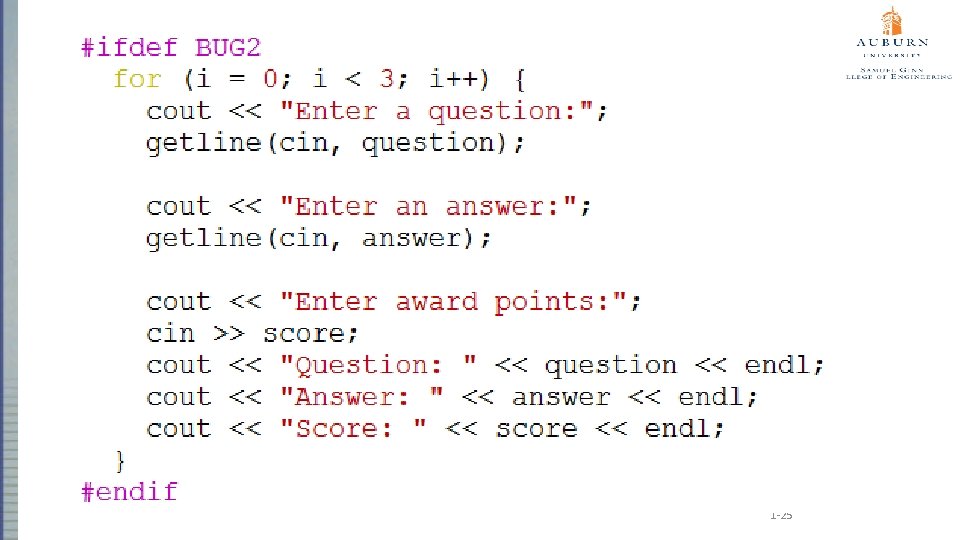
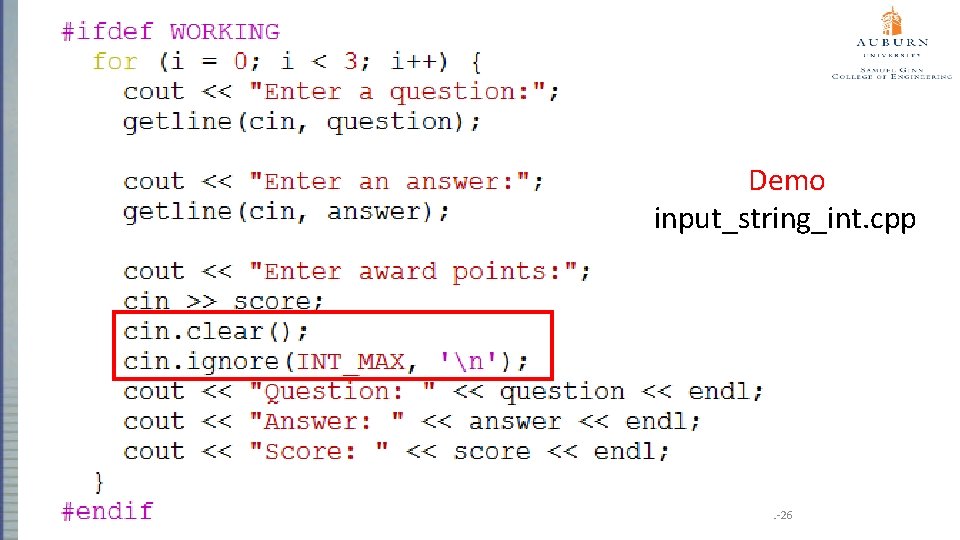
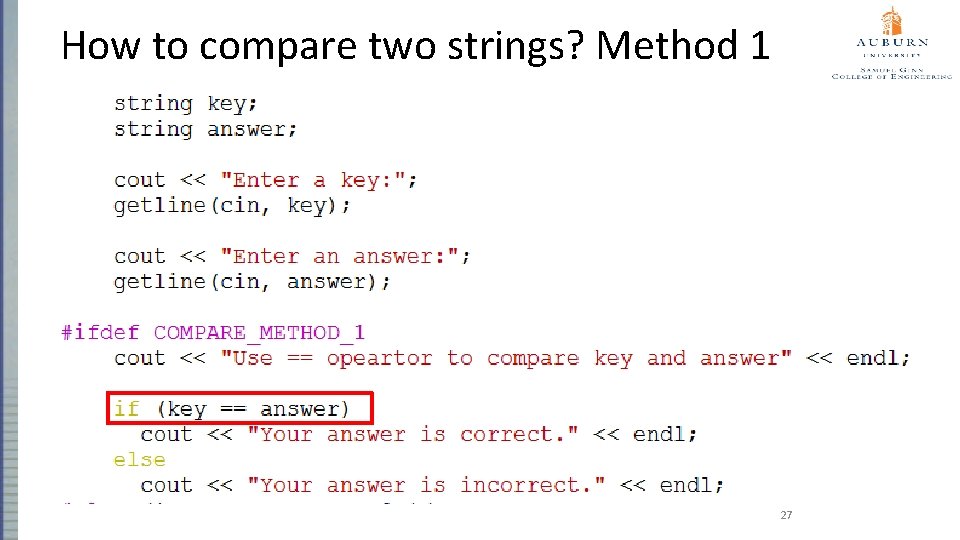
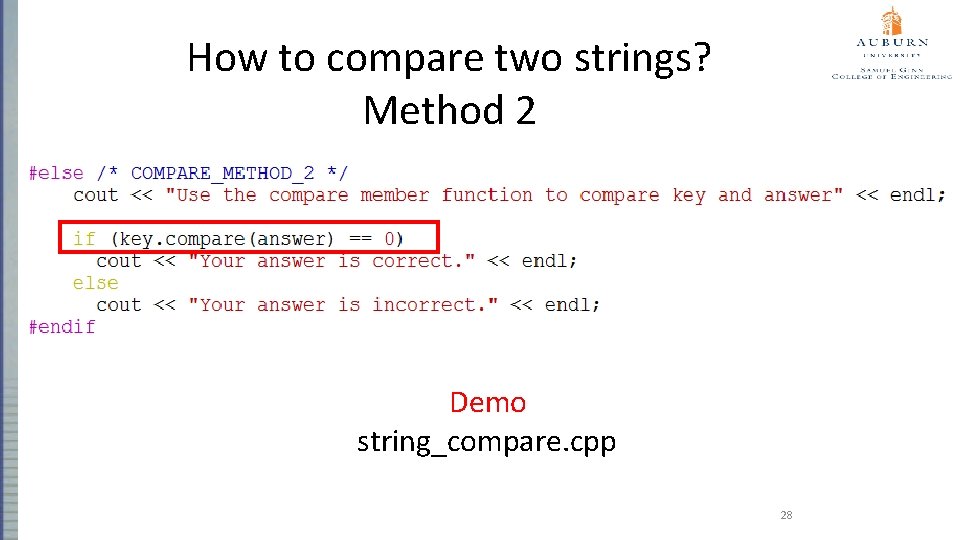
- Slides: 28
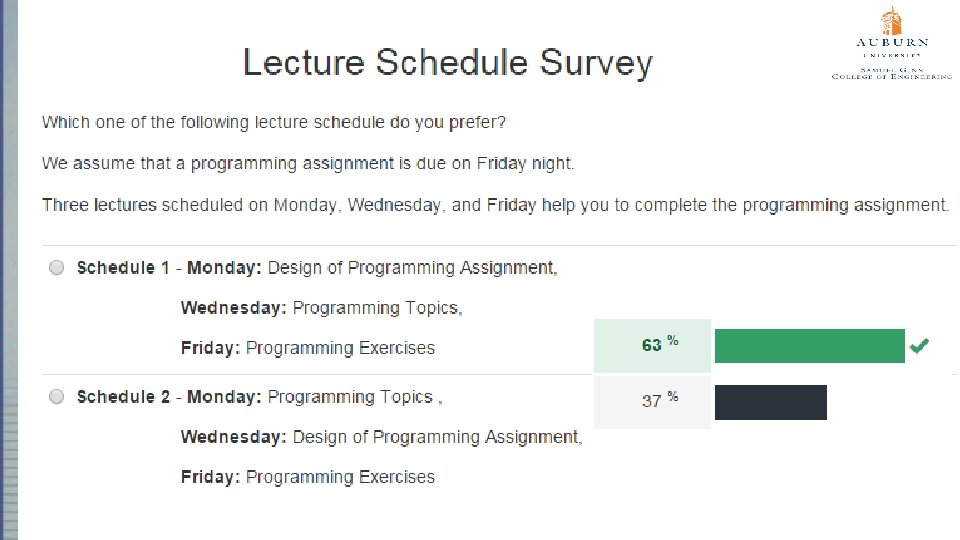
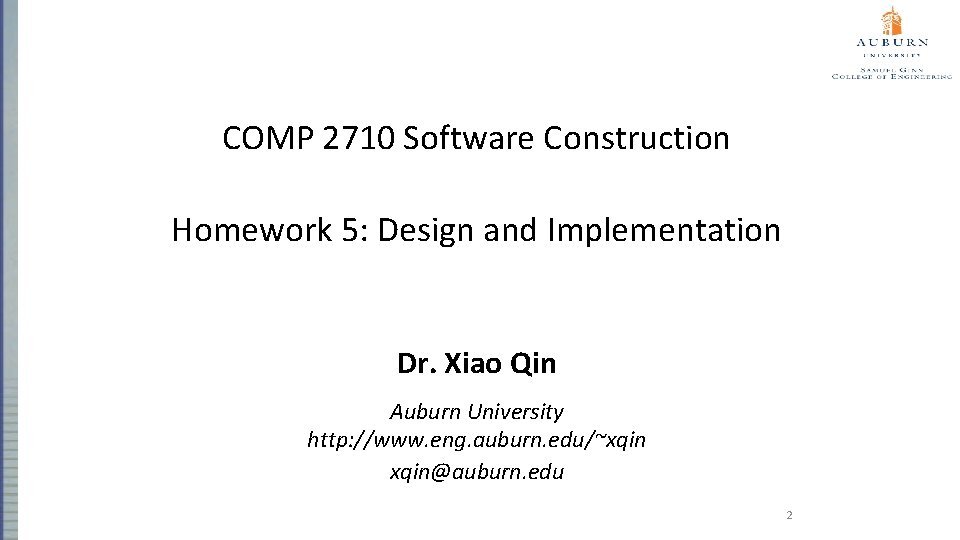
COMP 2710 Software Construction Homework 5: Design and Implementation Dr. Xiao Qin Auburn University http: //www. eng. auburn. edu/~xqin@auburn. edu 2
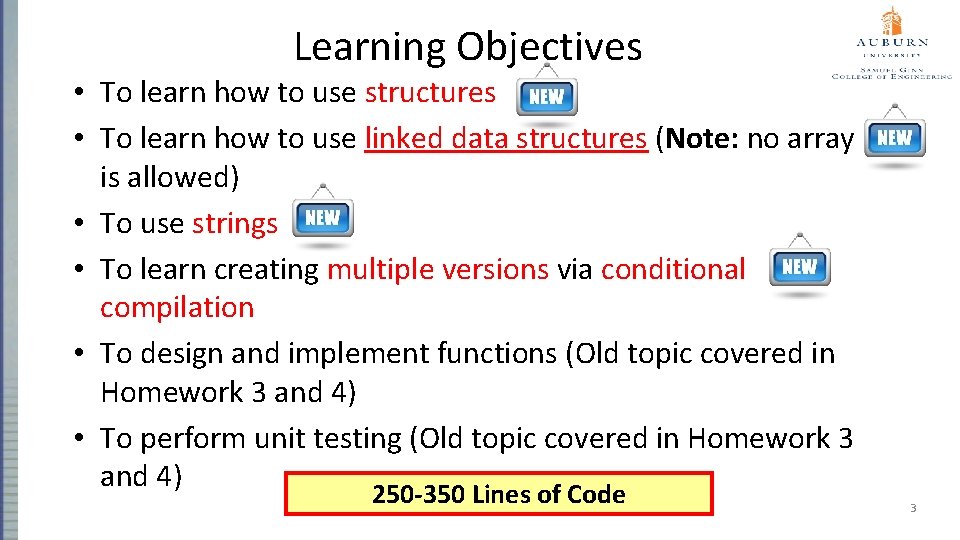
Learning Objectives • To learn how to use structures • To learn how to use linked data structures (Note: no array is allowed) • To use strings • To learn creating multiple versions via conditional compilation • To design and implement functions (Old topic covered in Homework 3 and 4) • To perform unit testing (Old topic covered in Homework 3 and 4) 250 -350 Lines of Code 3
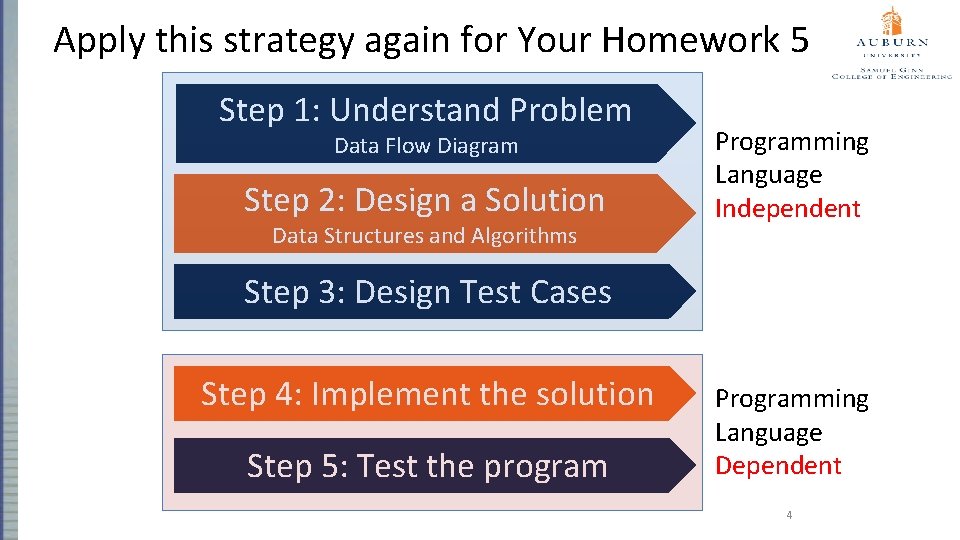
Apply this strategy again for Your Homework 5 Step 1: Understand Problem Data Flow Diagram Step 2: Design a Solution Data Structures and Algorithms Programming Language Independent Step 3: Design Test Cases Step 4: Implement the solution Step 5: Test the program Programming Language Dependent 4
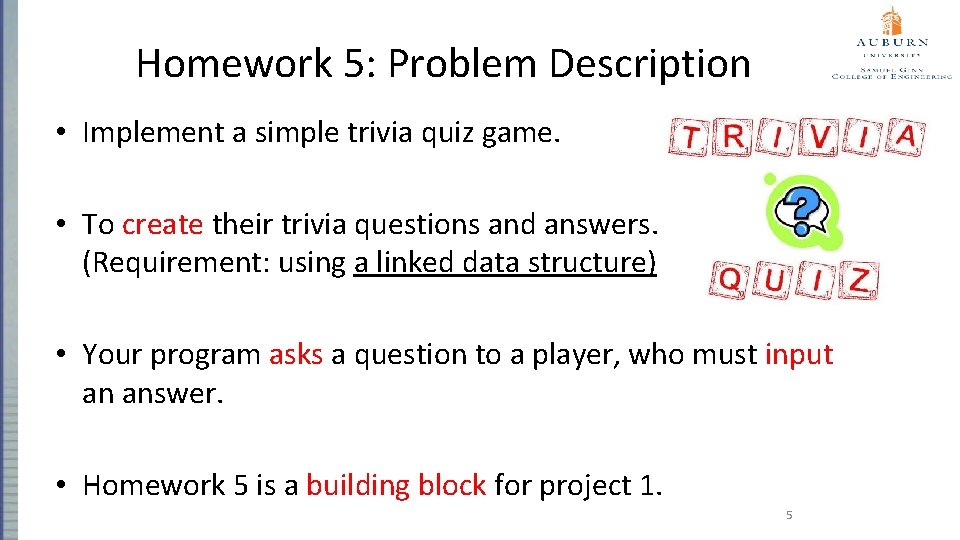
Homework 5: Problem Description • Implement a simple trivia quiz game. • To create their trivia questions and answers. (Requirement: using a linked data structure) • Your program asks a question to a player, who must input an answer. • Homework 5 is a building block for project 1. 5
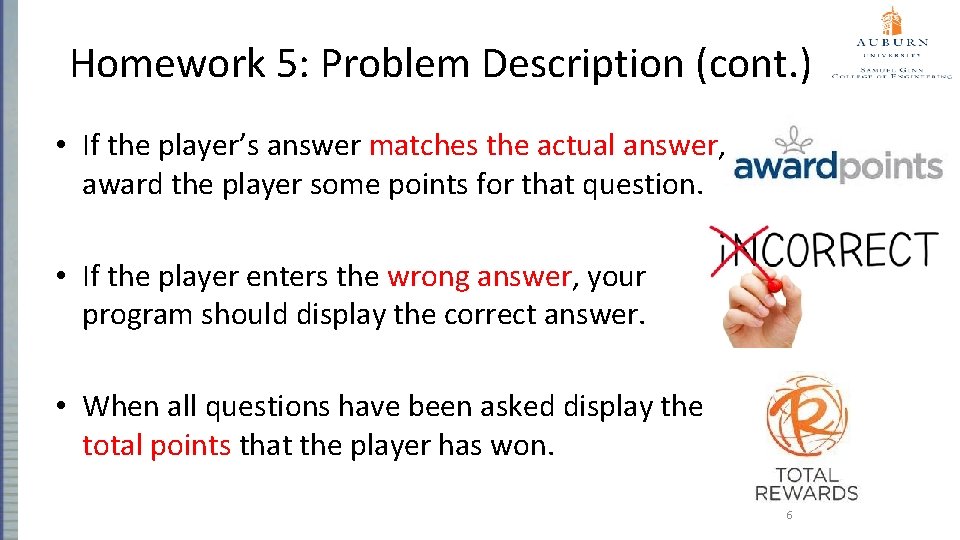
Homework 5: Problem Description (cont. ) • If the player’s answer matches the actual answer, award the player some points for that question. • If the player enters the wrong answer, your program should display the correct answer. • When all questions have been asked display the total points that the player has won. 6
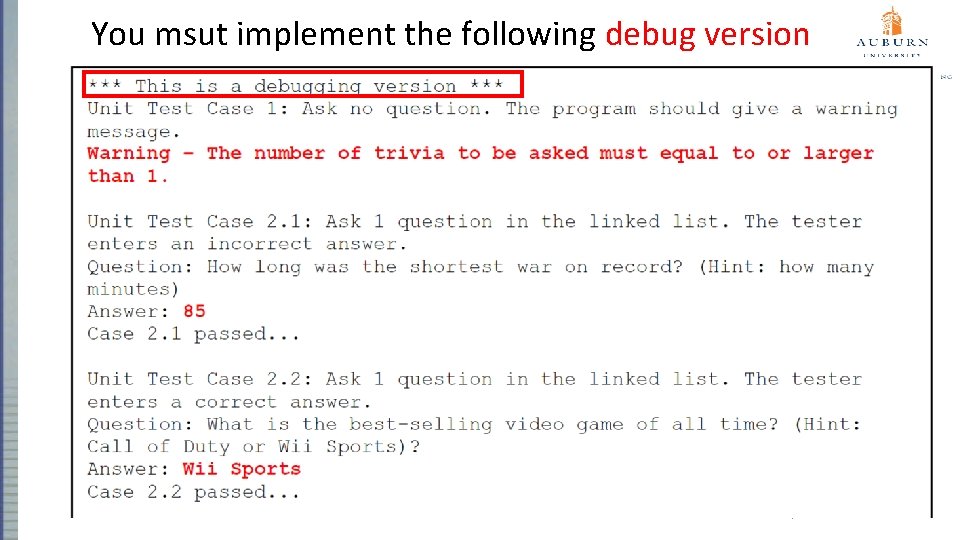
You msut implement the following debug version 7
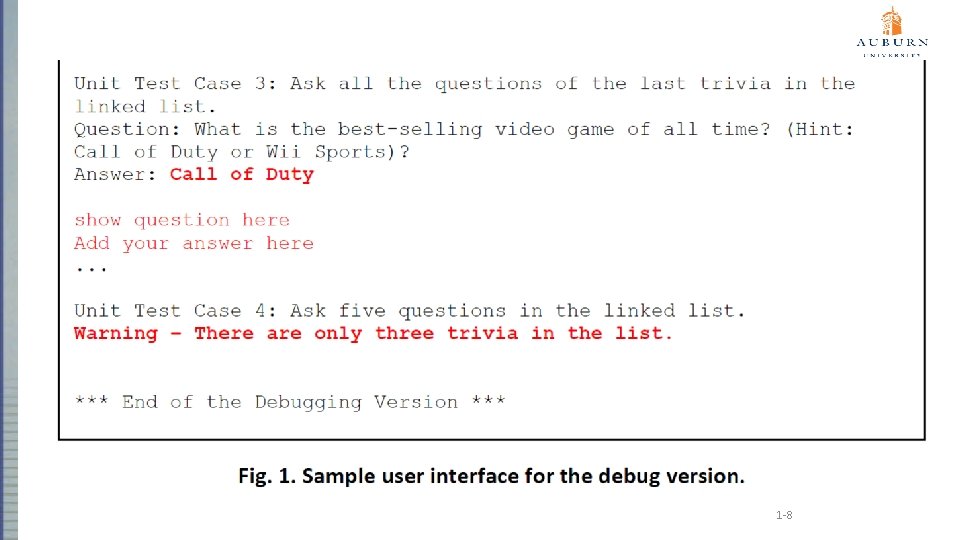
1 -8
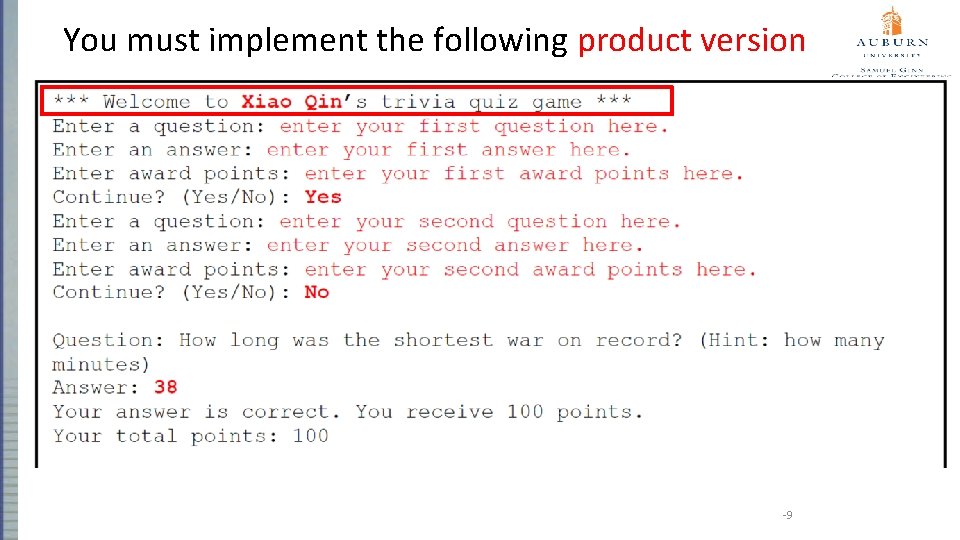
You must implement the following product version -9
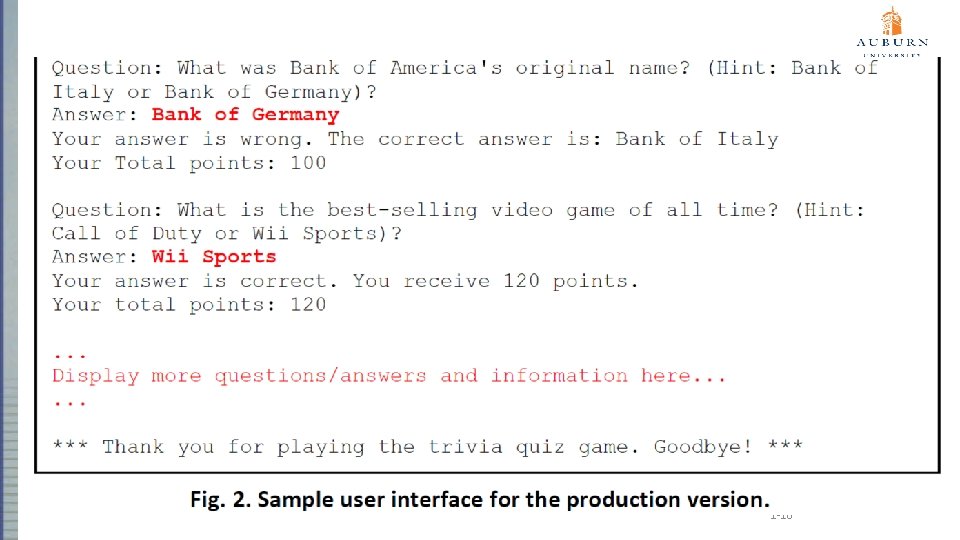
1 -10
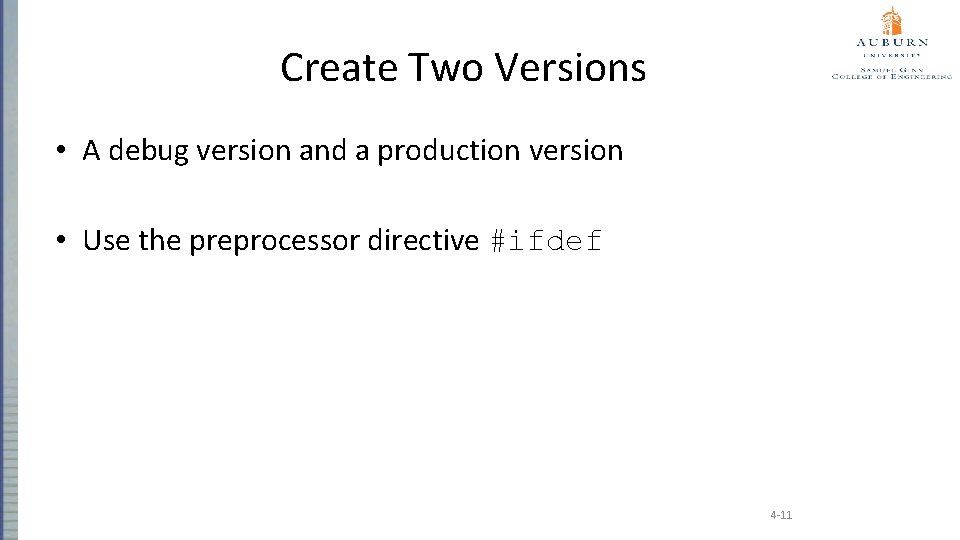
Create Two Versions • A debug version and a production version • Use the preprocessor directive #ifdef 4 -11
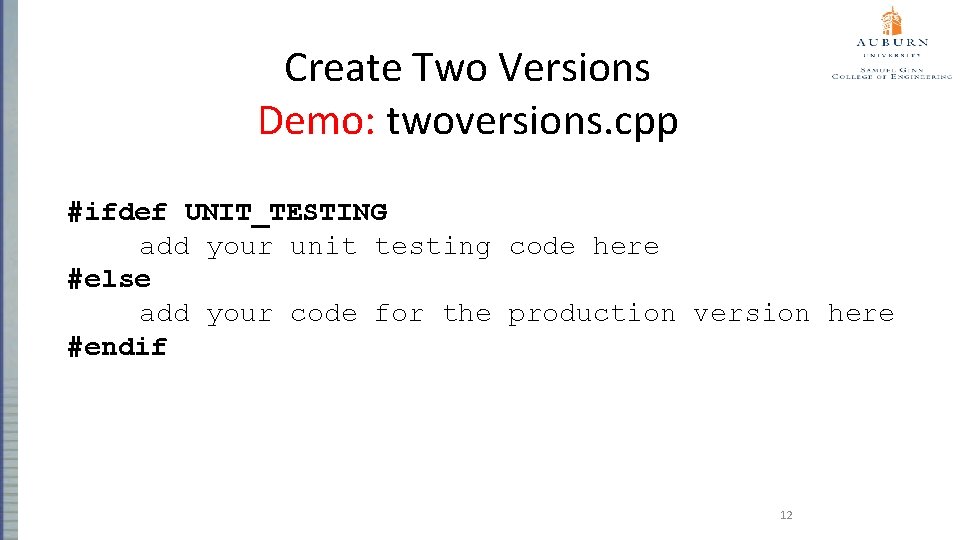
Create Two Versions Demo: twoversions. cpp #ifdef UNIT_TESTING add your unit testing code here #else add your code for the production version here #endif 12
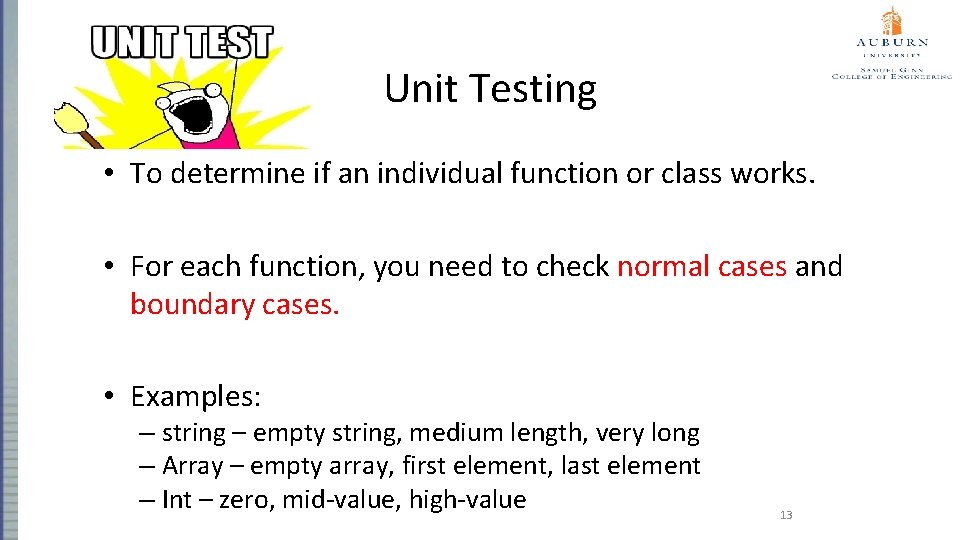
Unit Testing • To determine if an individual function or class works. • For each function, you need to check normal cases and boundary cases. • Examples: – string – empty string, medium length, very long – Array – empty array, first element, last element – Int – zero, mid-value, high-value 13
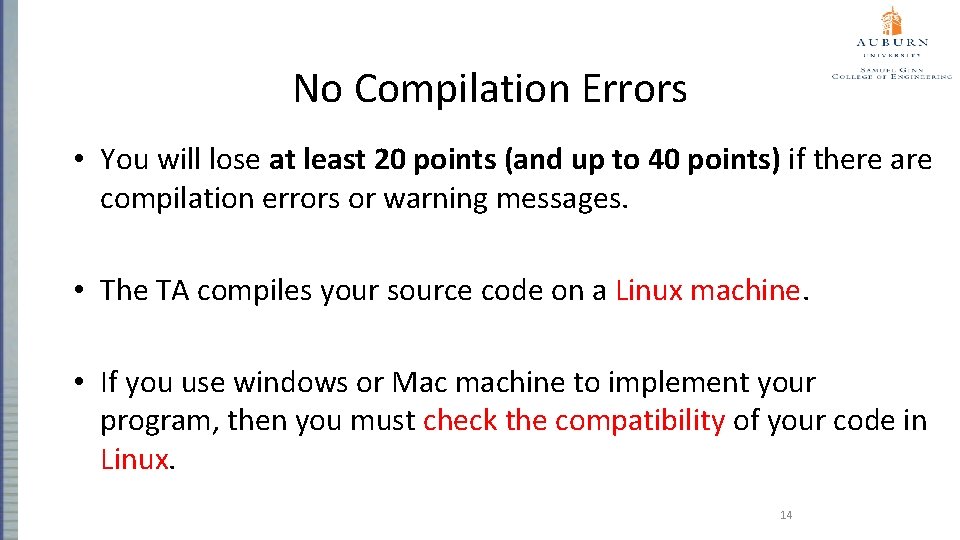
No Compilation Errors • You will lose at least 20 points (and up to 40 points) if there are compilation errors or warning messages. • The TA compiles your source code on a Linux machine. • If you use windows or Mac machine to implement your program, then you must check the compatibility of your code in Linux. 14
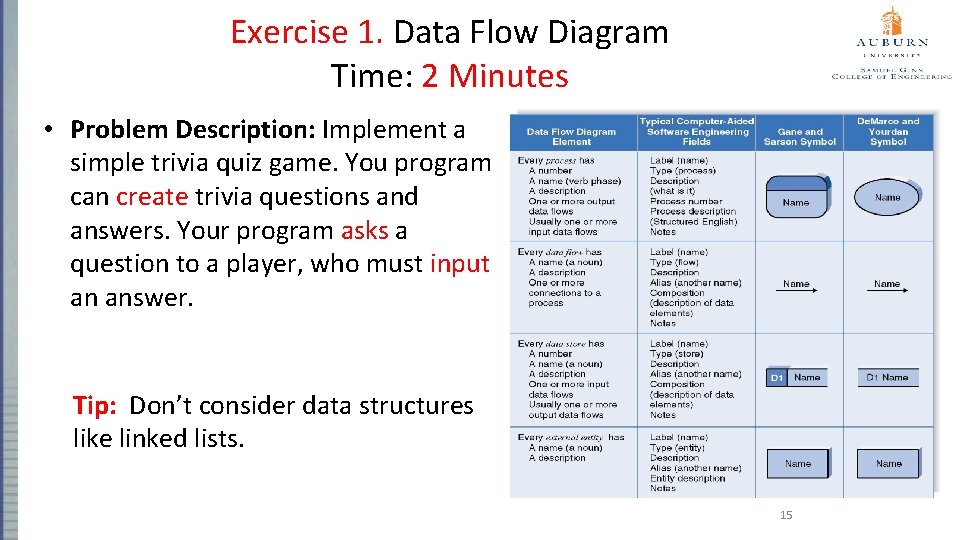
Exercise 1. Data Flow Diagram Time: 2 Minutes • Problem Description: Implement a simple trivia quiz game. You program can create trivia questions and answers. Your program asks a question to a player, who must input an answer. Tip: Don’t consider data structures like linked lists. 15
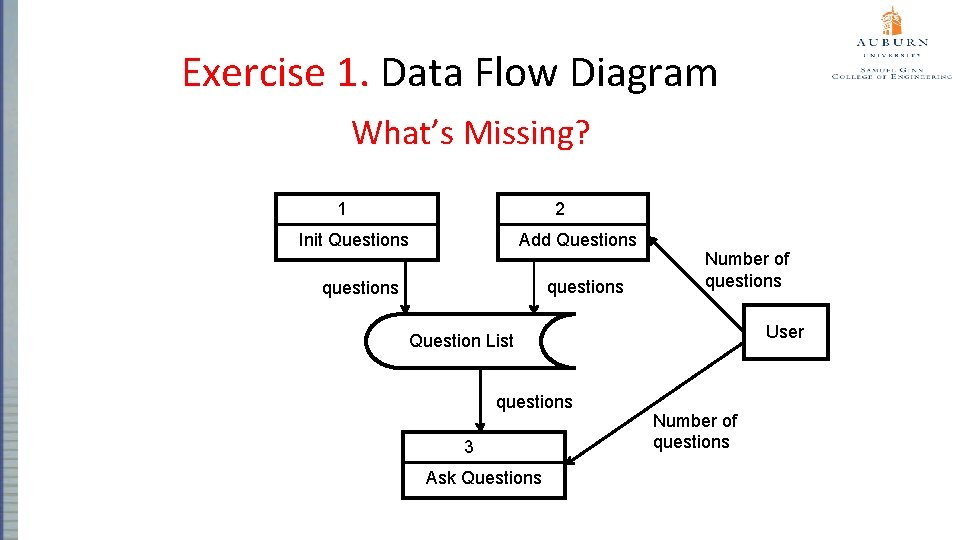
Exercise 1. Data Flow Diagram What’s Missing? 1 2 Add Questions Init Questions questions Number of questions User Question List questions 3 Ask Questions Number of questions
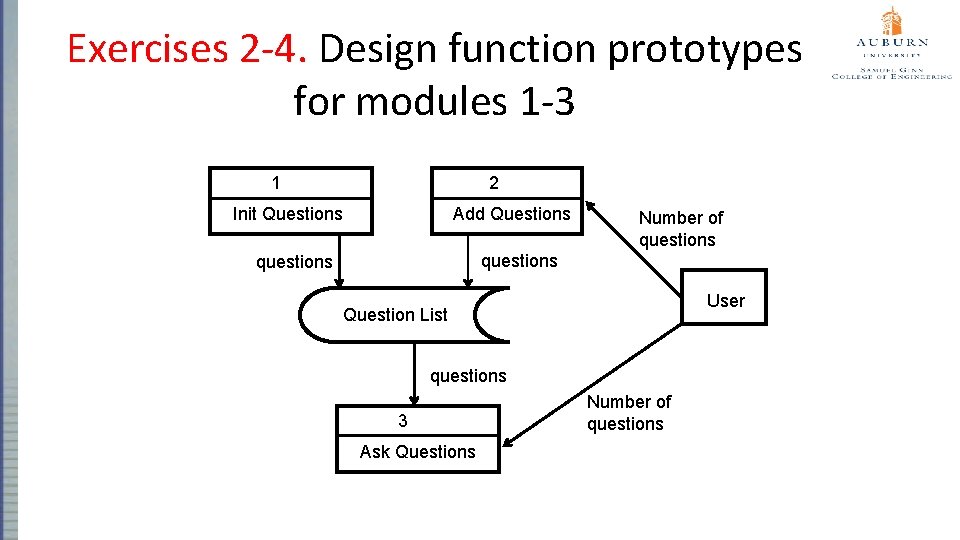
Exercises 2 -4. Design function prototypes for modules 1 -3 1 2 Add Questions Init Questions Number of questions User Question List questions 3 Ask Questions Number of questions
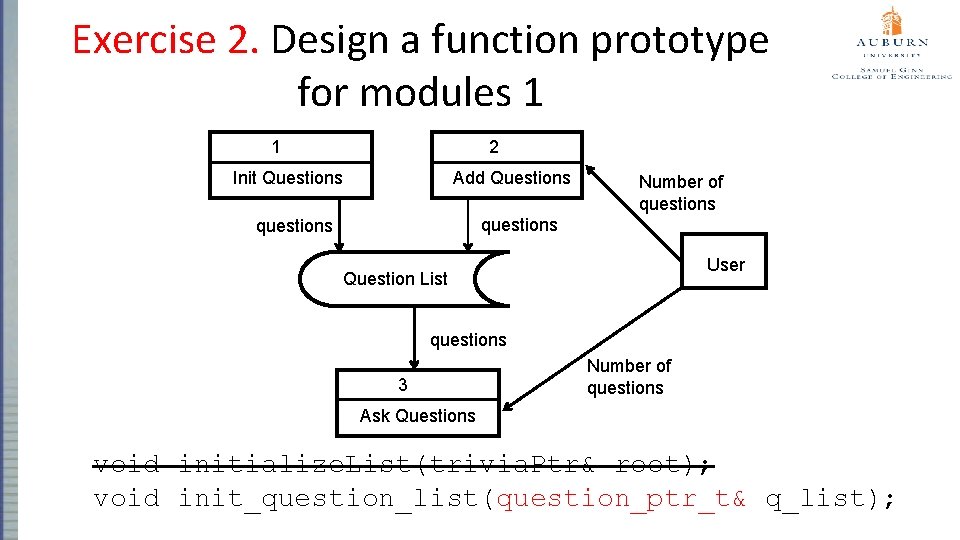
Exercise 2. Design a function prototype for modules 1 1 2 Add Questions Init Questions Number of questions User Question List questions 3 Number of questions Ask Questions void initialize. List(trivia. Ptr& root); void init_question_list(question_ptr_t& q_list);
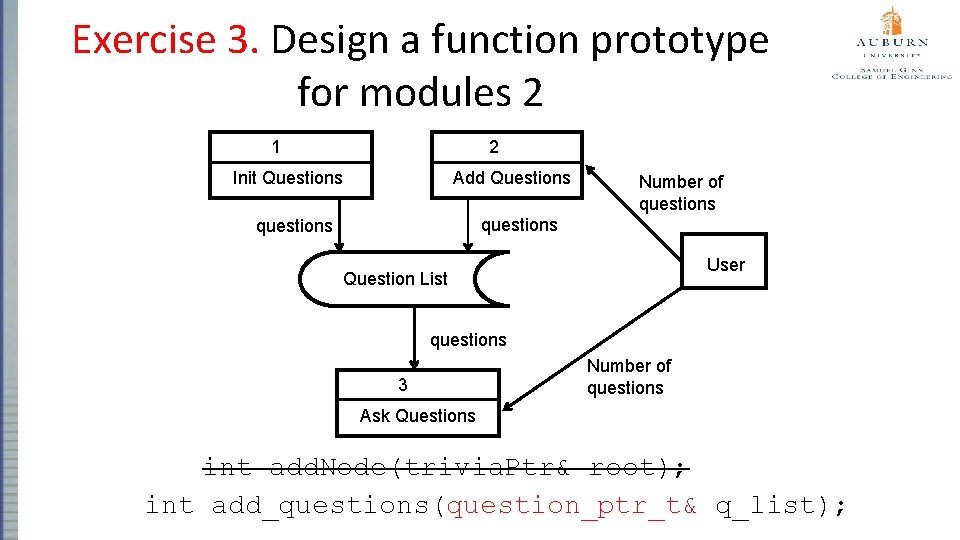
Exercise 3. Design a function prototype for modules 2 1 2 Add Questions Init Questions Number of questions User Question List questions 3 Number of questions Ask Questions int add. Node(trivia. Ptr& root); int add_questions(question_ptr_t& q_list);
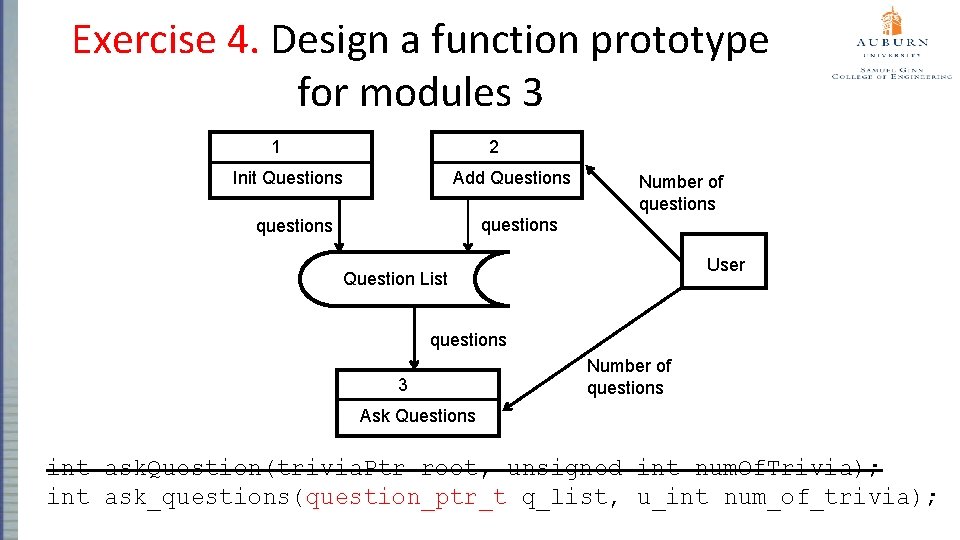
Exercise 4. Design a function prototype for modules 3 1 2 Add Questions Init Questions Number of questions User Question List questions 3 Number of questions Ask Questions int ask. Question(trivia. Ptr root, unsigned int num. Of. Trivia); int ask_questions(question_ptr_t q_list, u_int num_of_trivia);
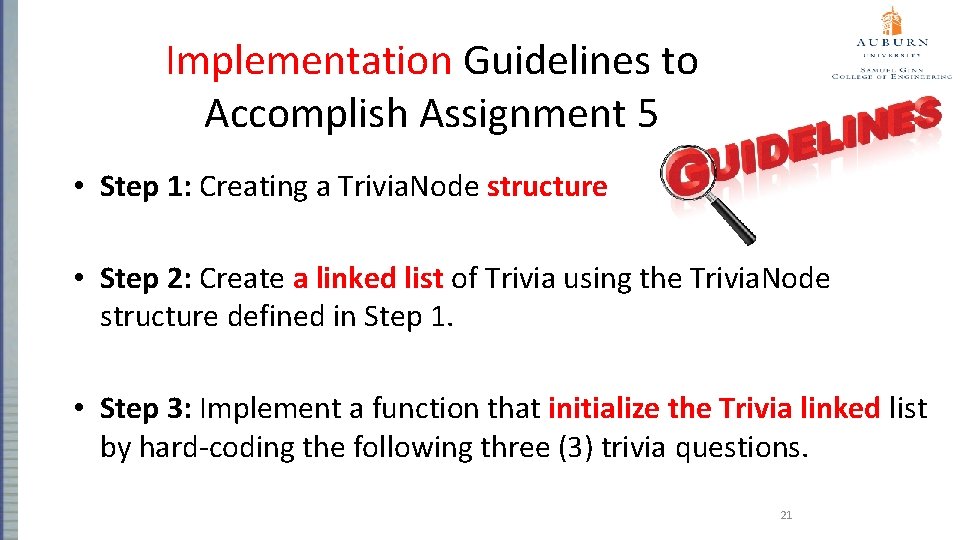
Implementation Guidelines to Accomplish Assignment 5 • Step 1: Creating a Trivia. Node structure • Step 2: Create a linked list of Trivia using the Trivia. Node structure defined in Step 1. • Step 3: Implement a function that initialize the Trivia linked list by hard-coding the following three (3) trivia questions. 21
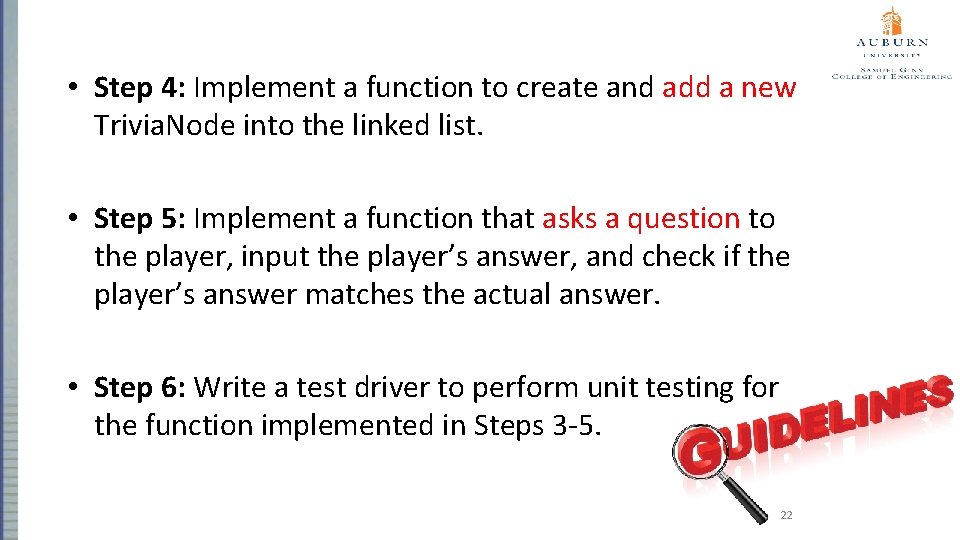
• Step 4: Implement a function to create and add a new Trivia. Node into the linked list. • Step 5: Implement a function that asks a question to the player, input the player’s answer, and check if the player’s answer matches the actual answer. • Step 6: Write a test driver to perform unit testing for the function implemented in Steps 3 -5. 22
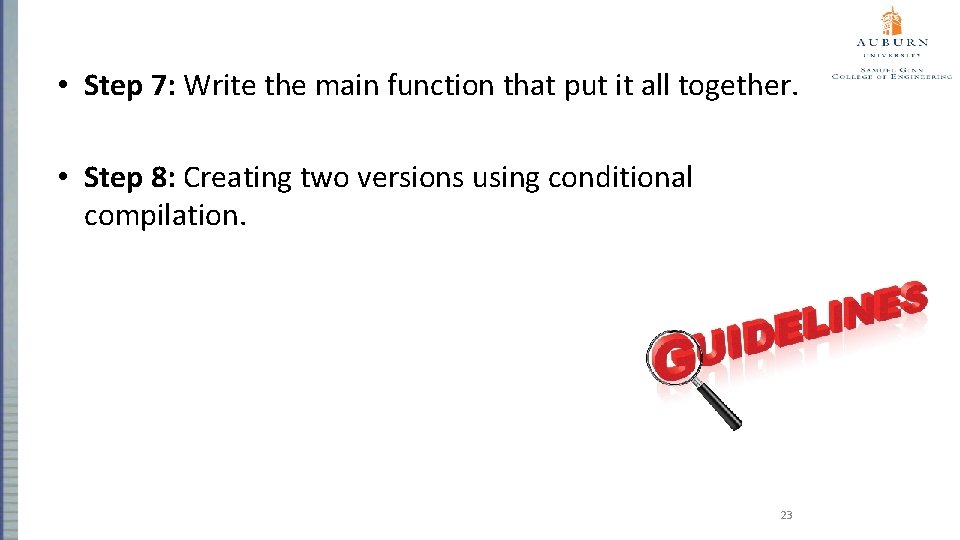
• Step 7: Write the main function that put it all together. • Step 8: Creating two versions using conditional compilation. 23
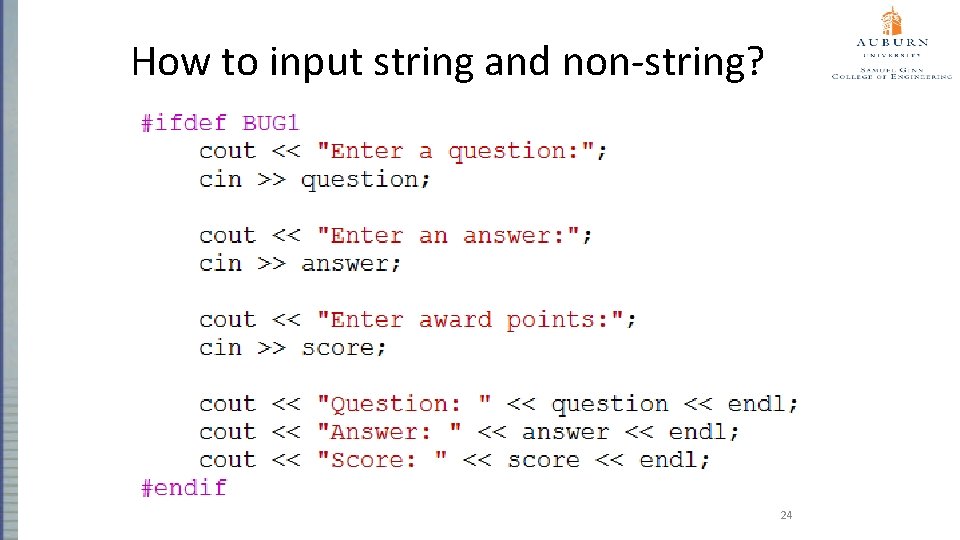
How to input string and non-string? 24
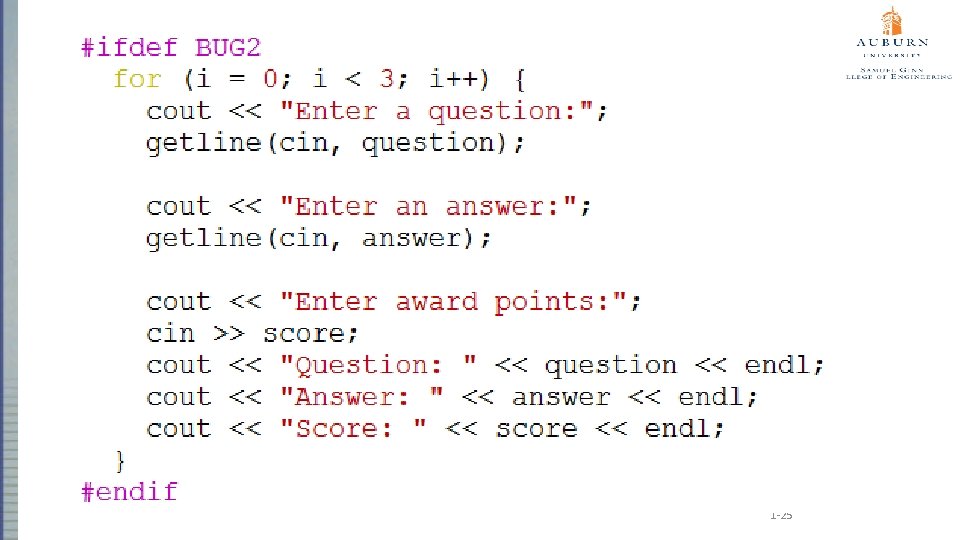
1 -25
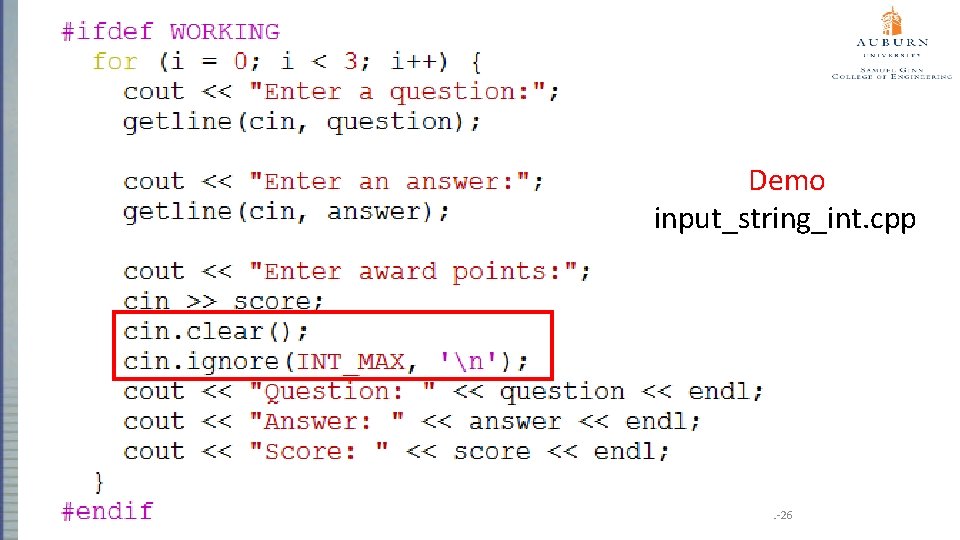
Demo input_string_int. cpp 1 -26
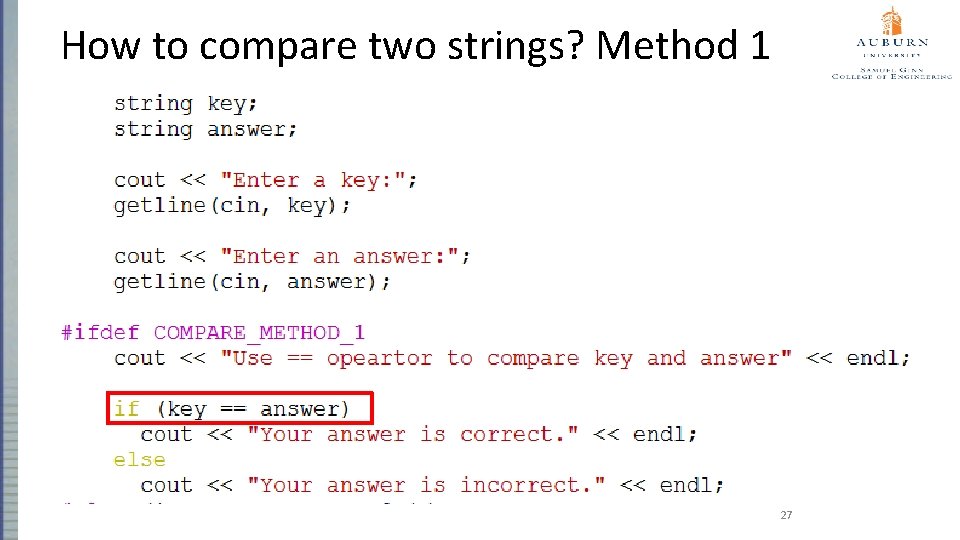
How to compare two strings? Method 1 27
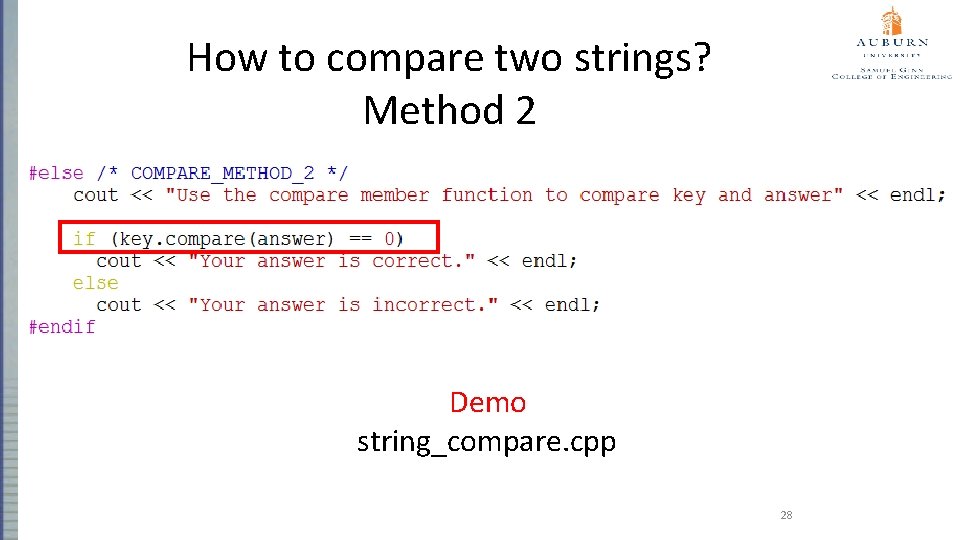
How to compare two strings? Method 2 Demo string_compare. cpp 28