COMP 14 03 Introduction to Programming Adrian Ilie
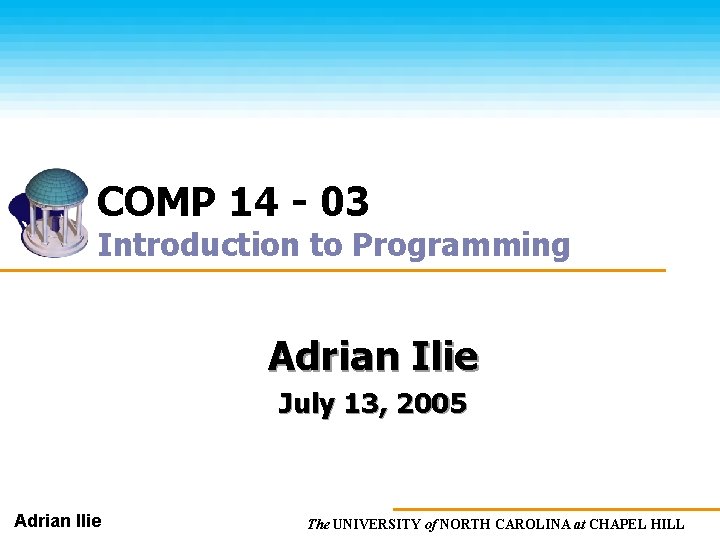
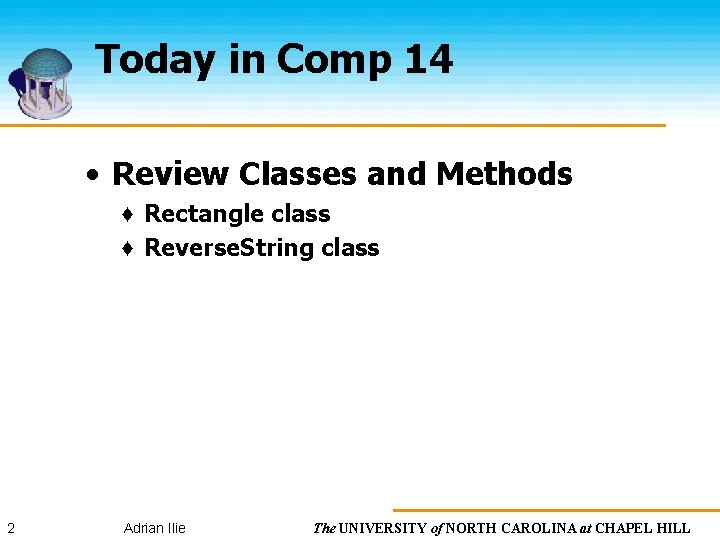
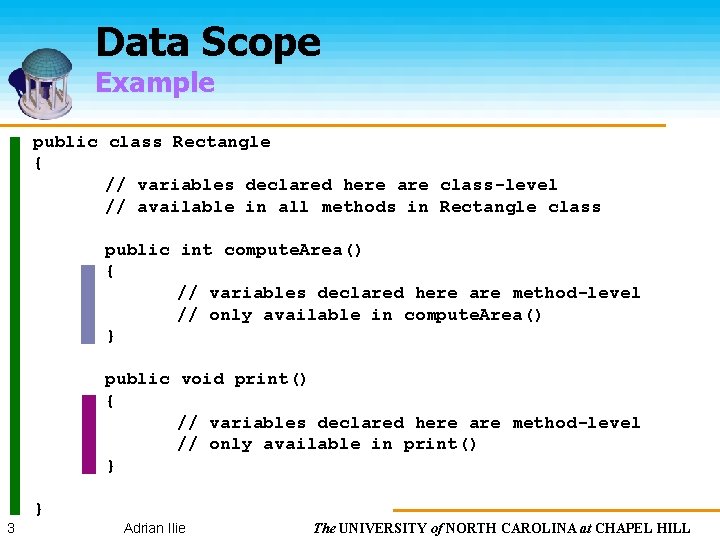
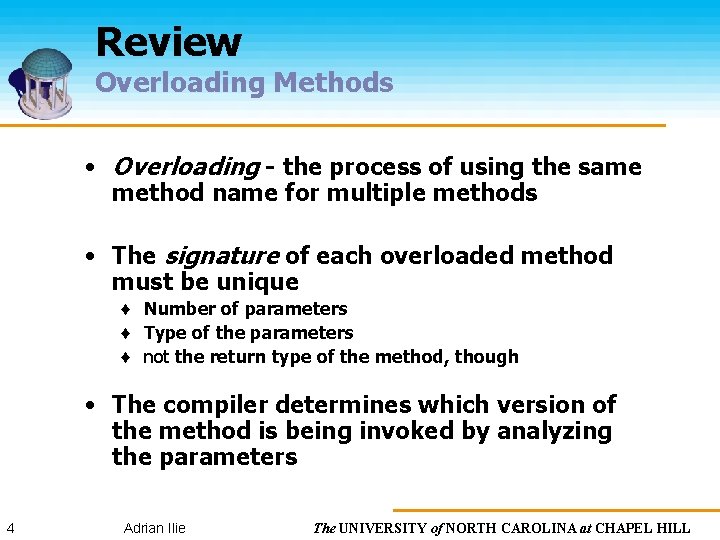
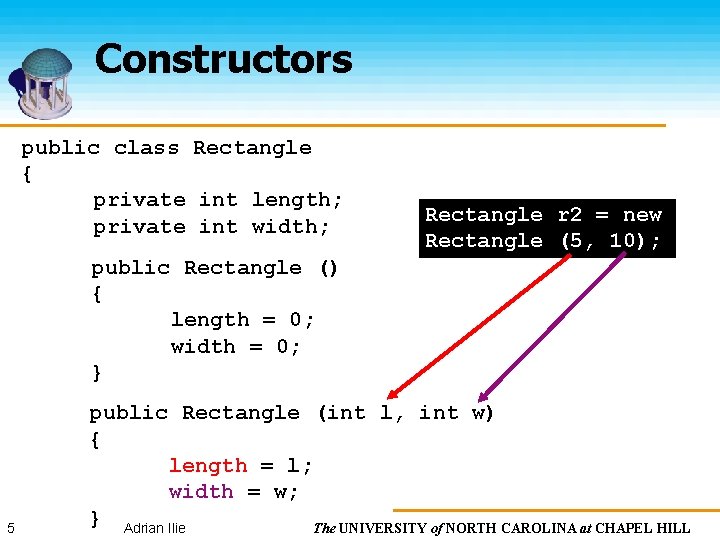
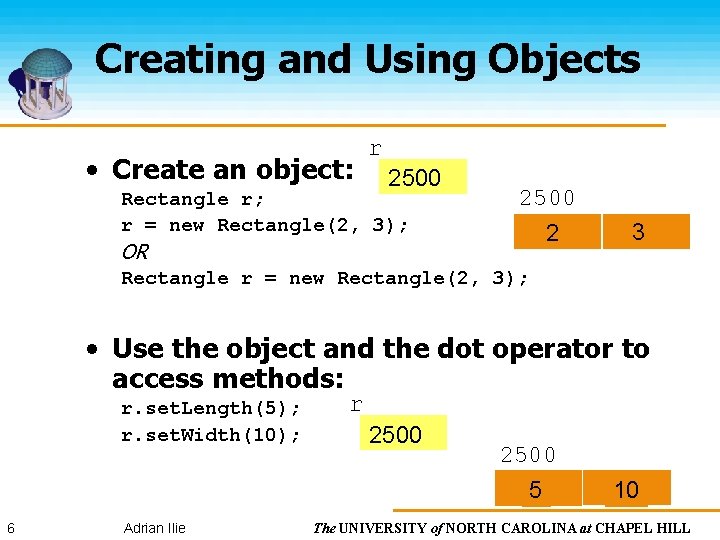
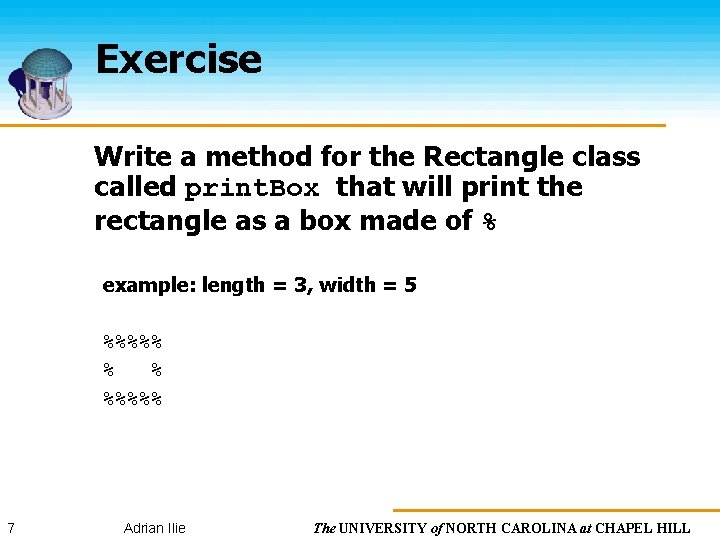
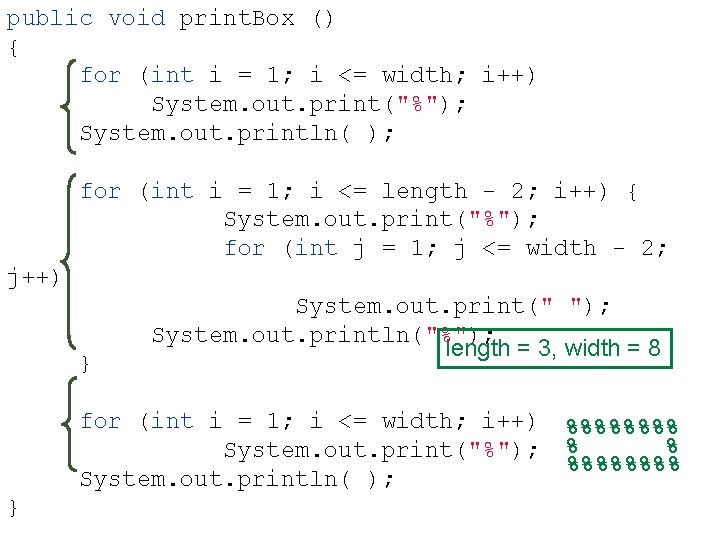
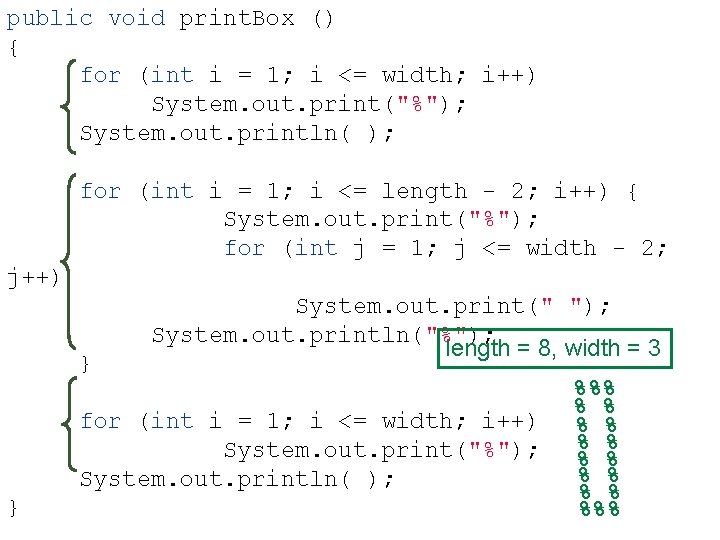
![Testing public class Rectangle. Tester { public static void main (String[] args) { Rectangle Testing public class Rectangle. Tester { public static void main (String[] args) { Rectangle](https://slidetodoc.com/presentation_image_h/2653b842746a912119404897b7ebf881/image-10.jpg)
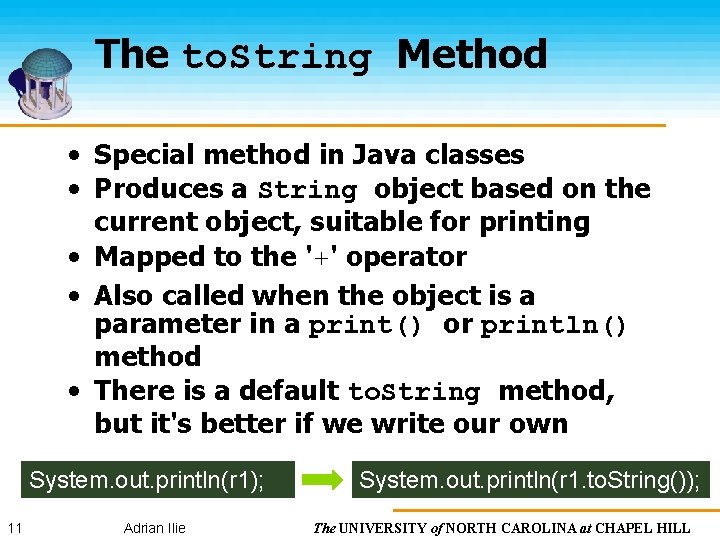
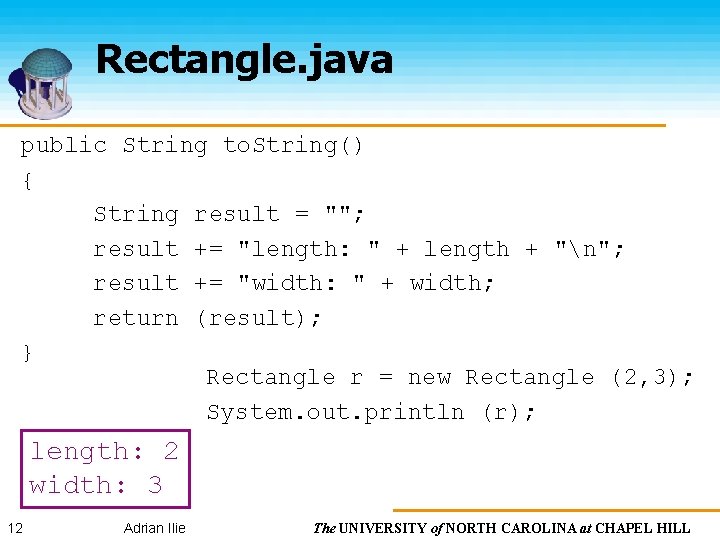
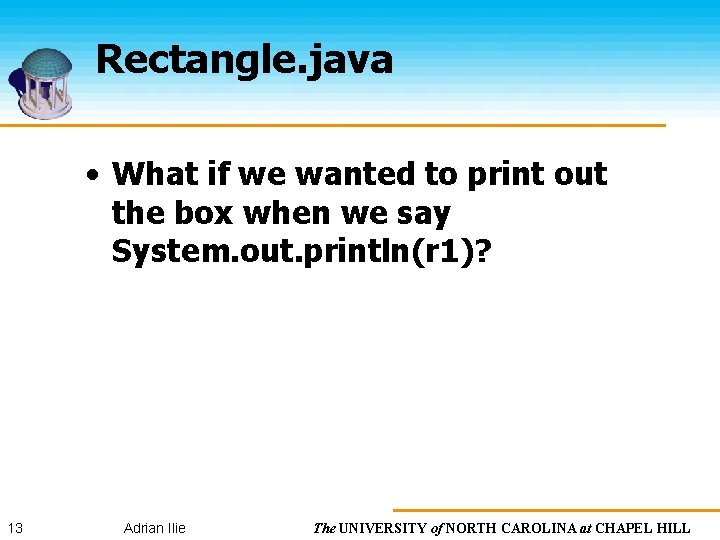
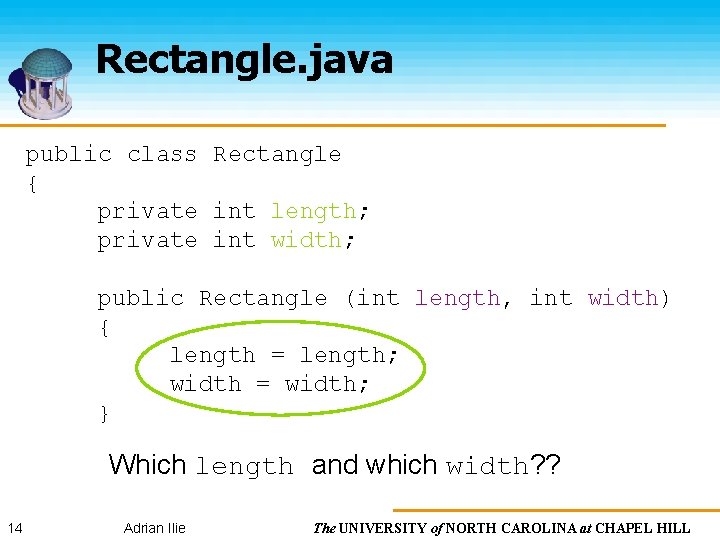
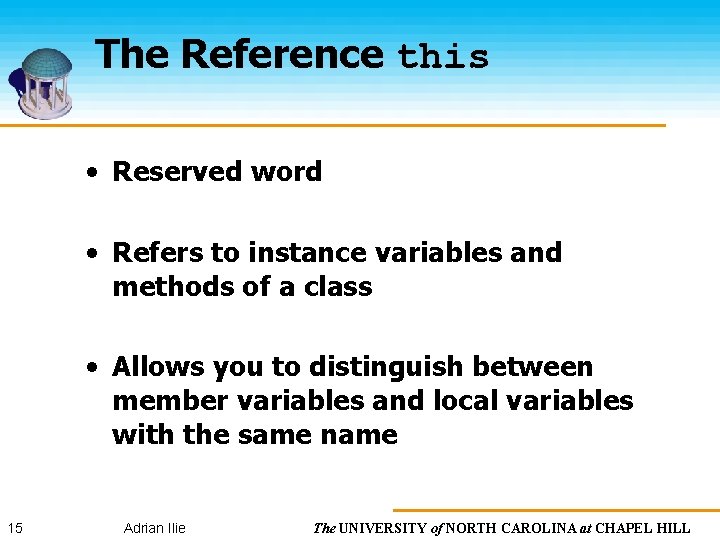
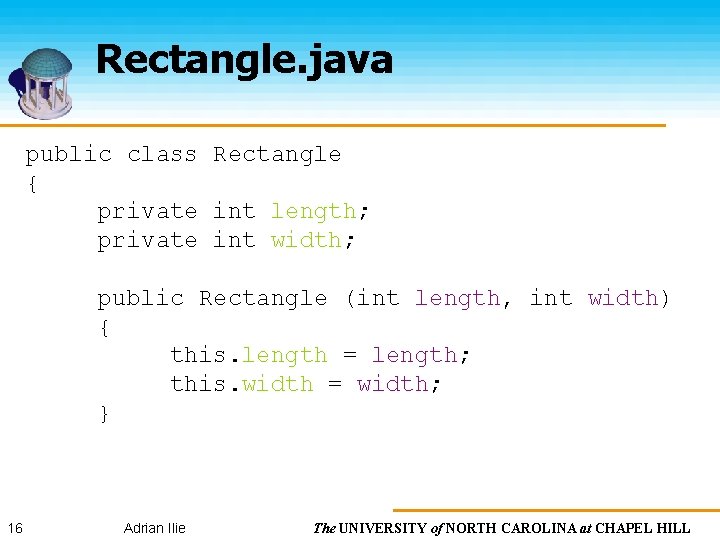
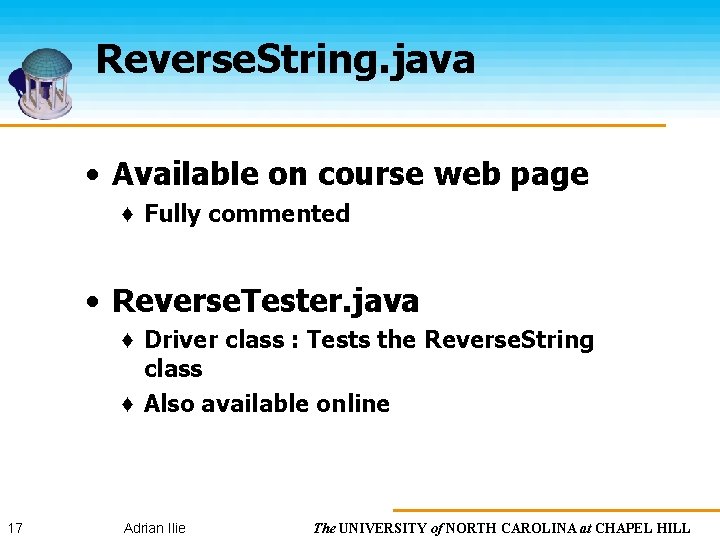
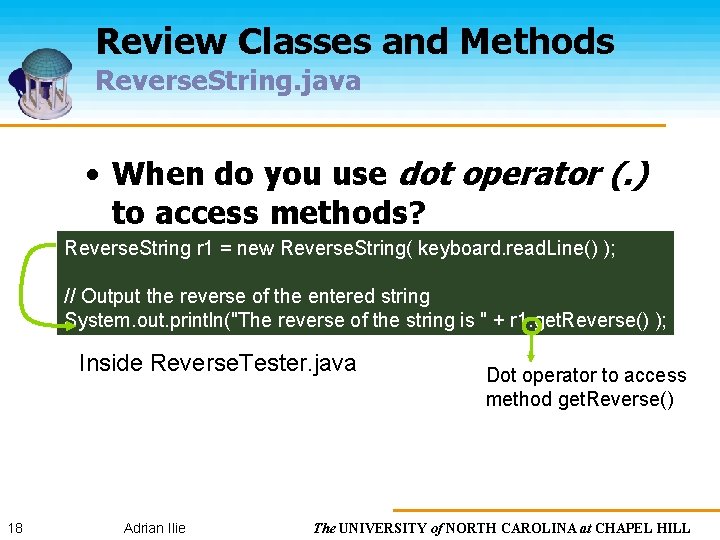
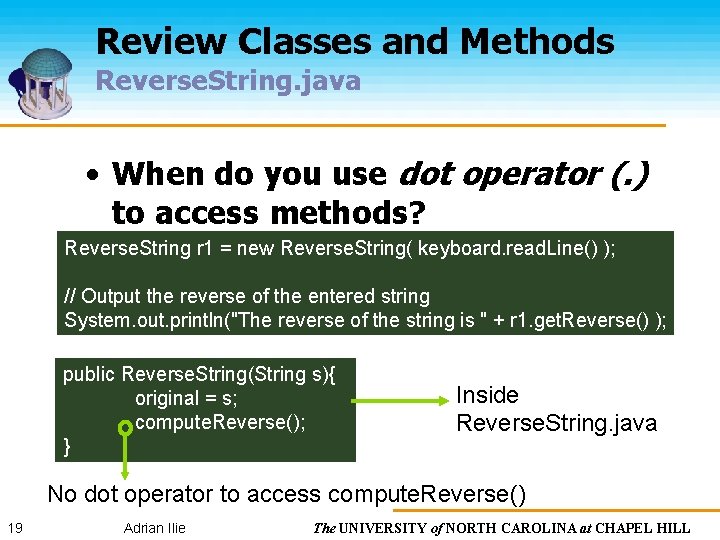
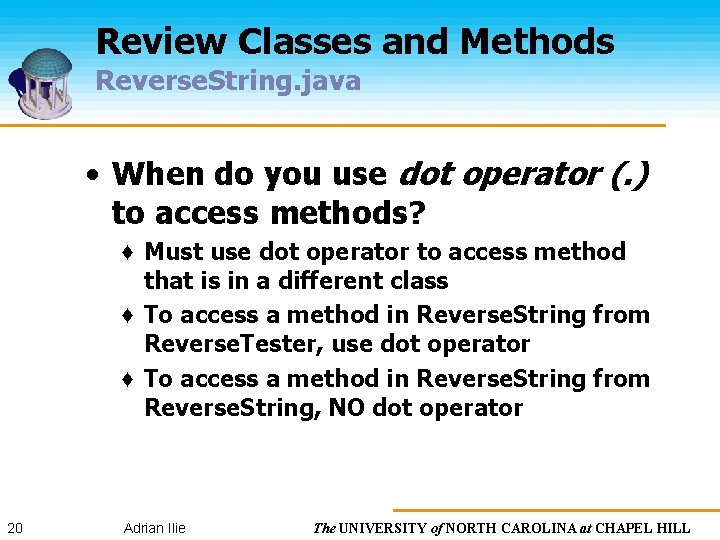
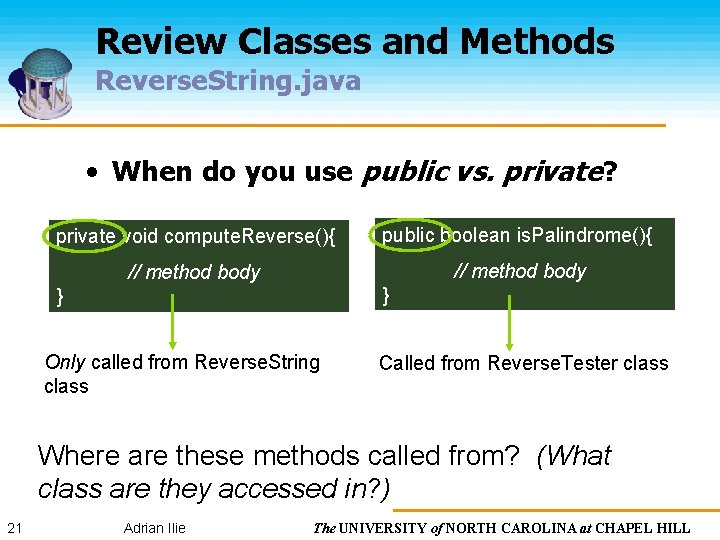
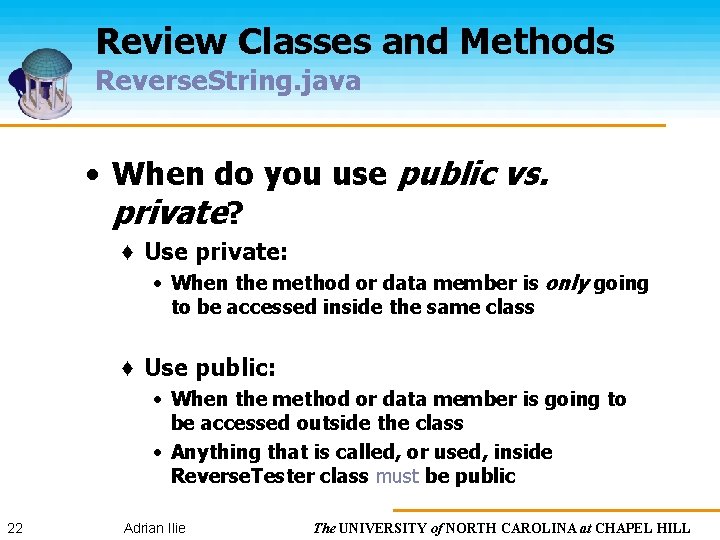
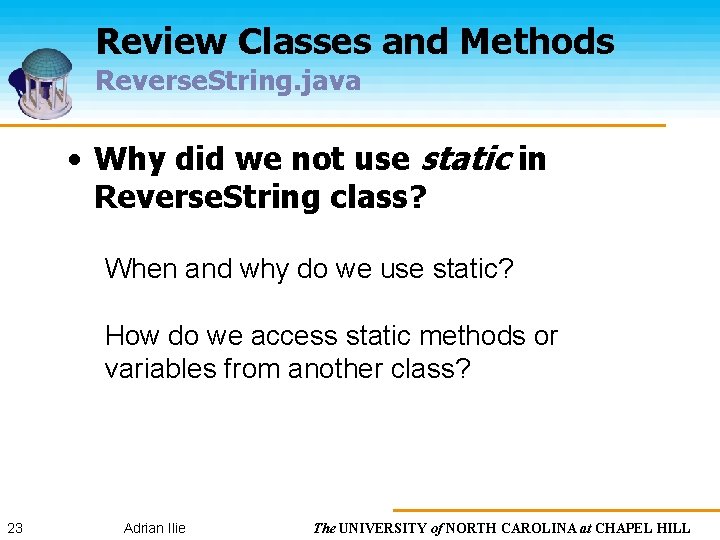
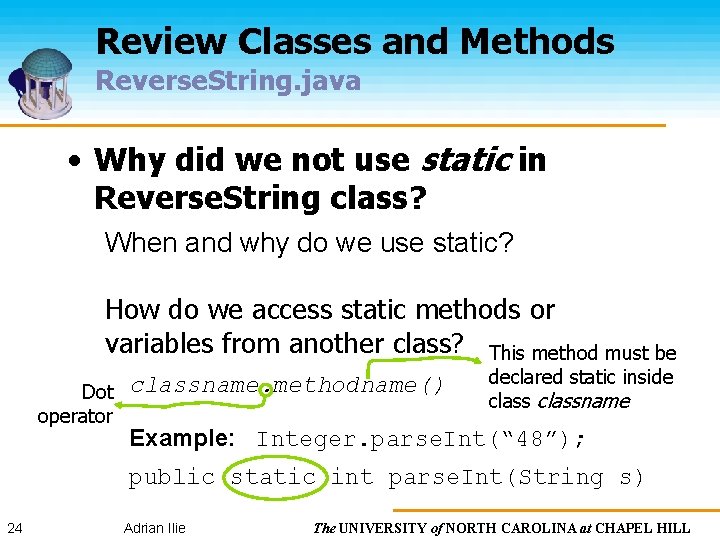
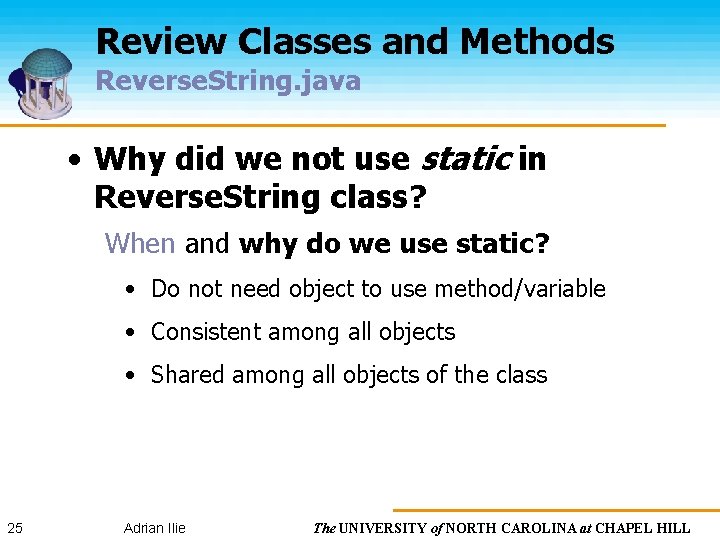
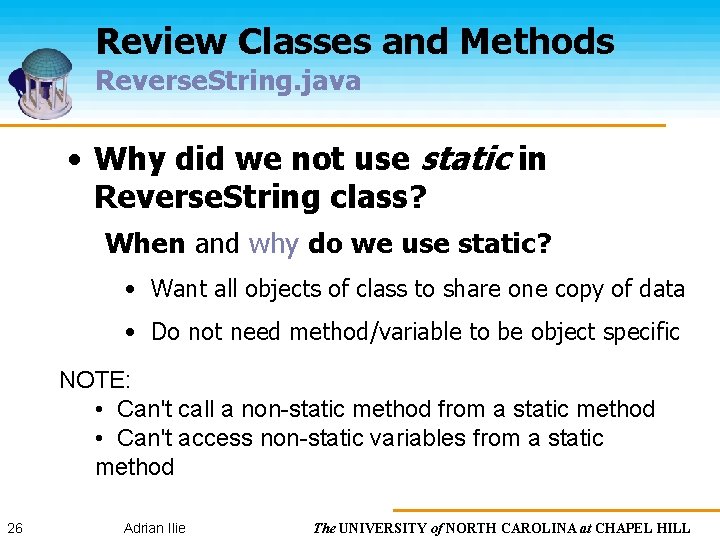
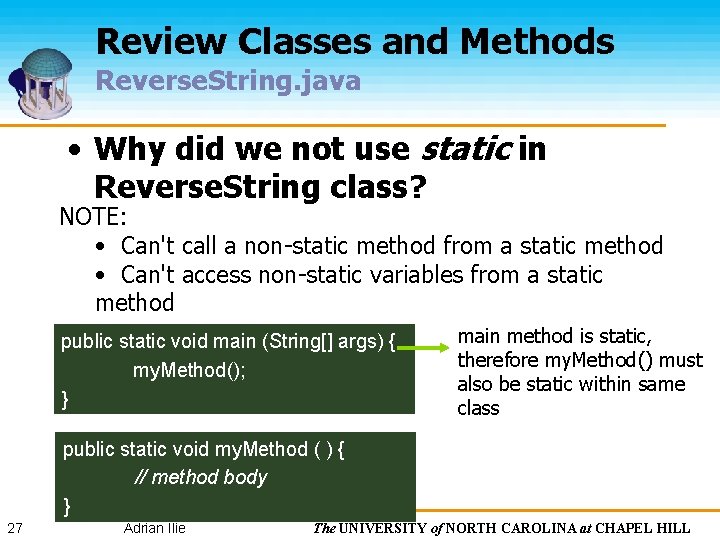
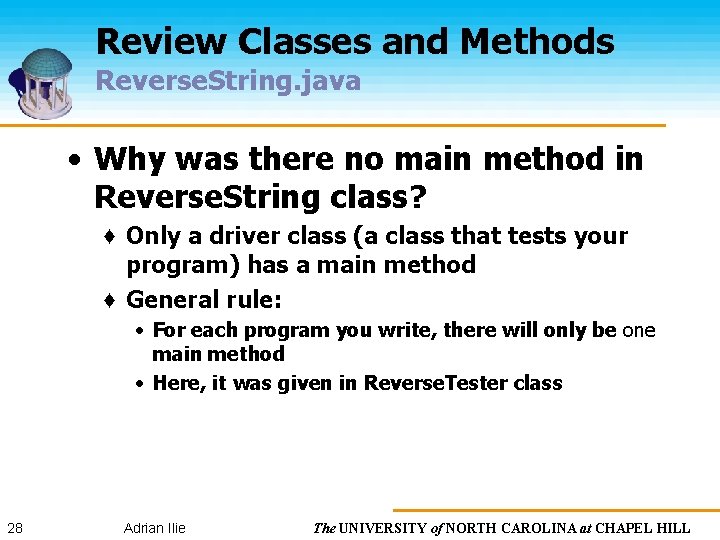
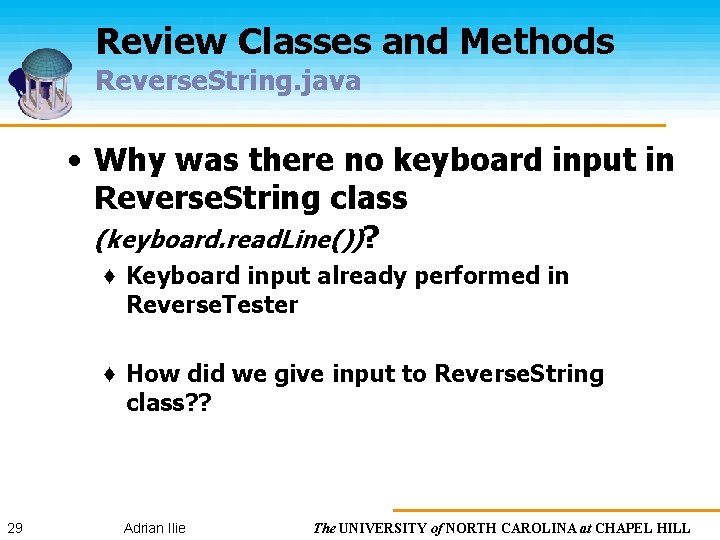
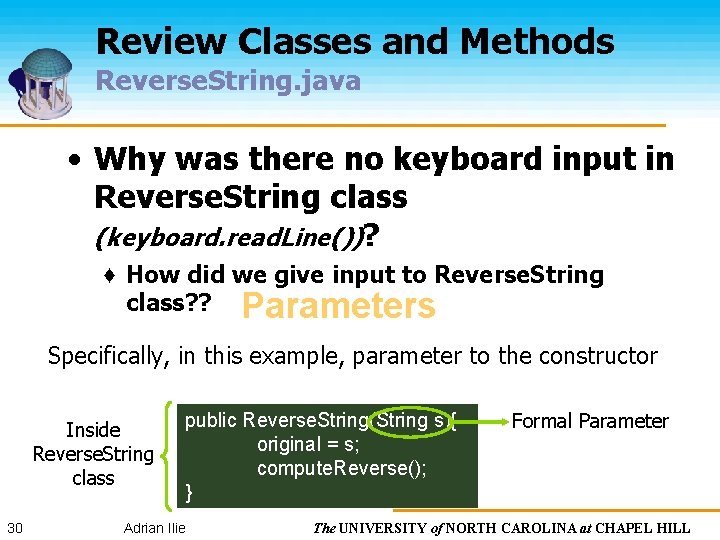
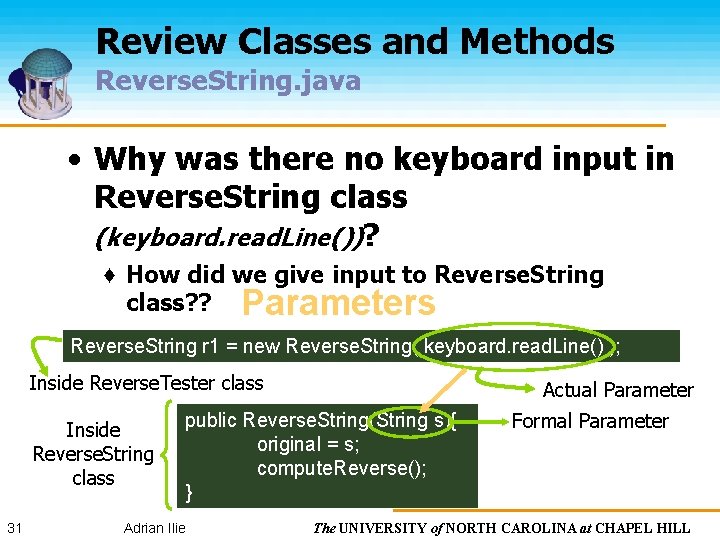
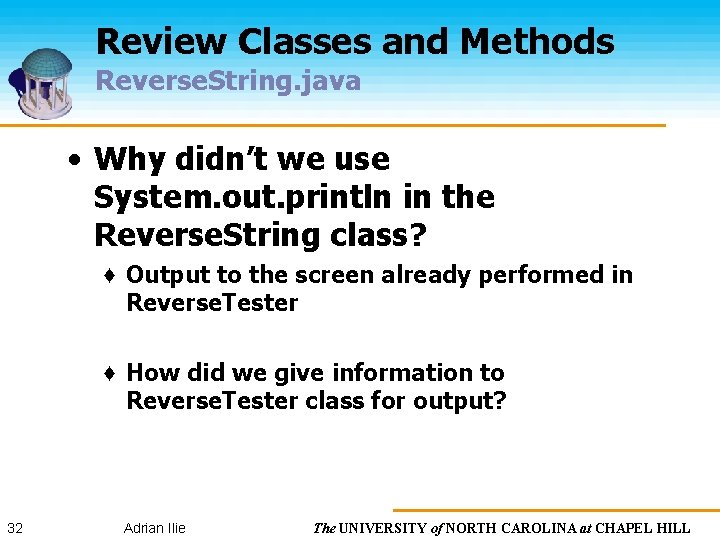
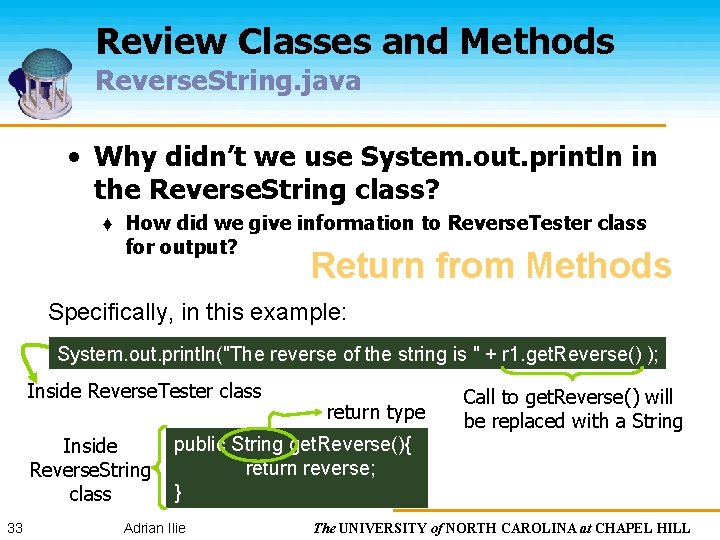
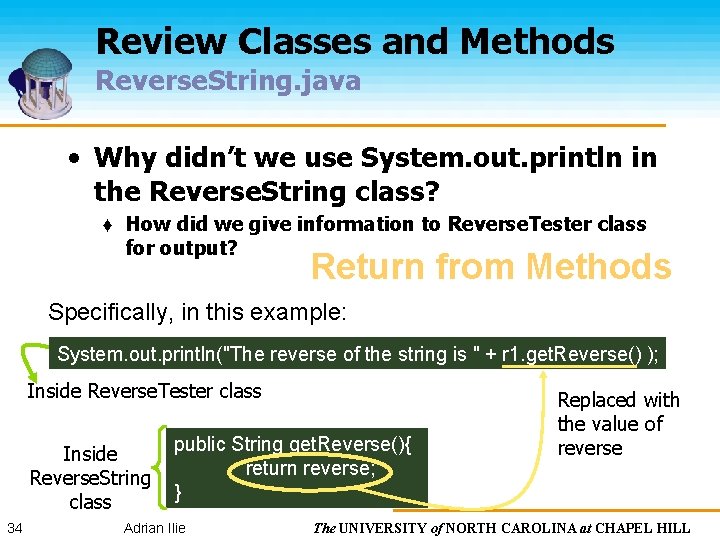
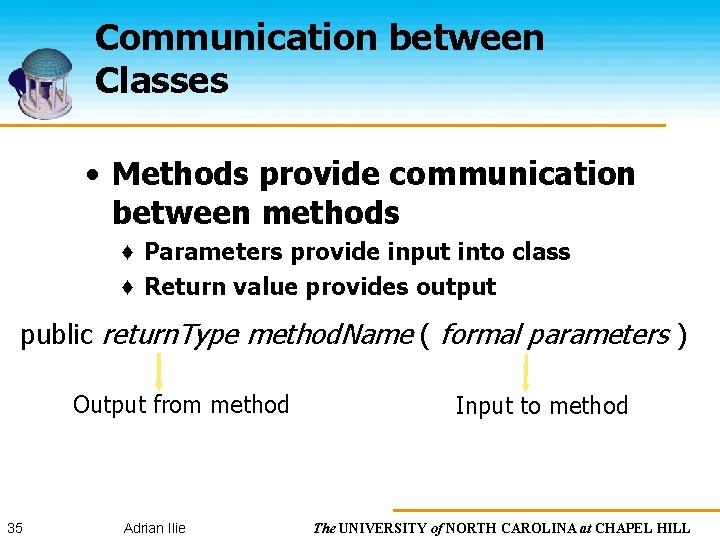
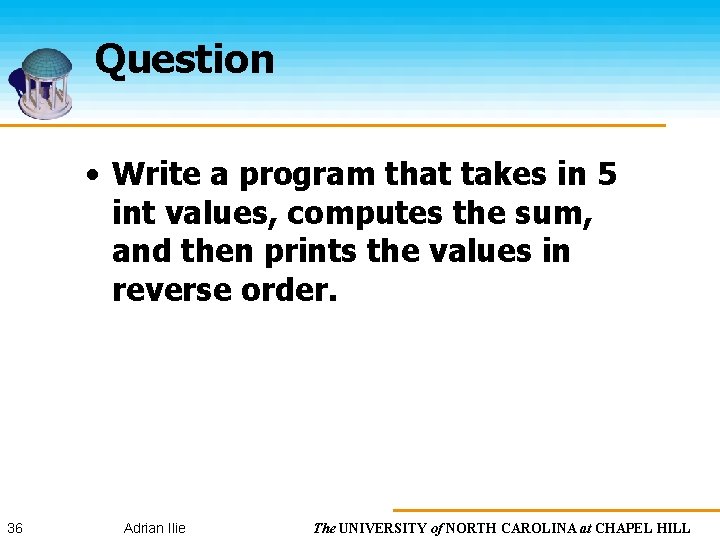
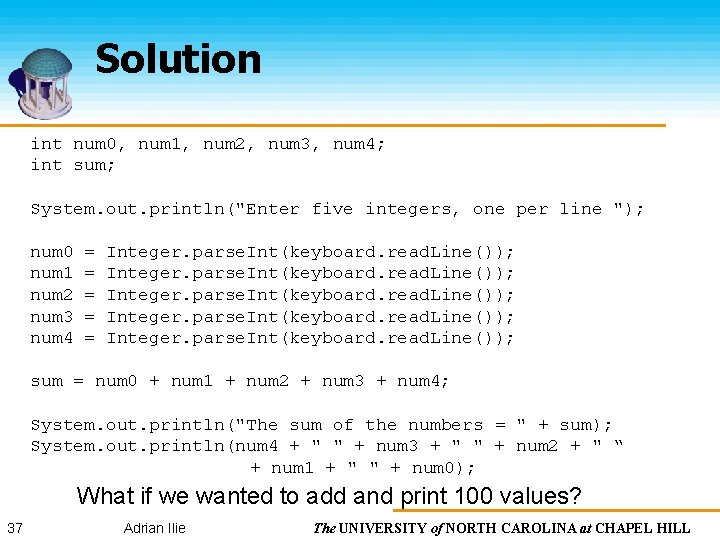
- Slides: 37
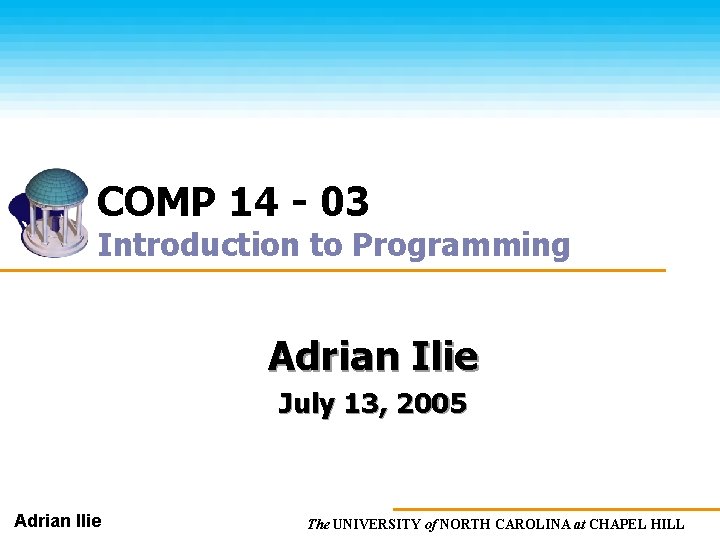
COMP 14 - 03 Introduction to Programming Adrian Ilie July 13, 2005 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
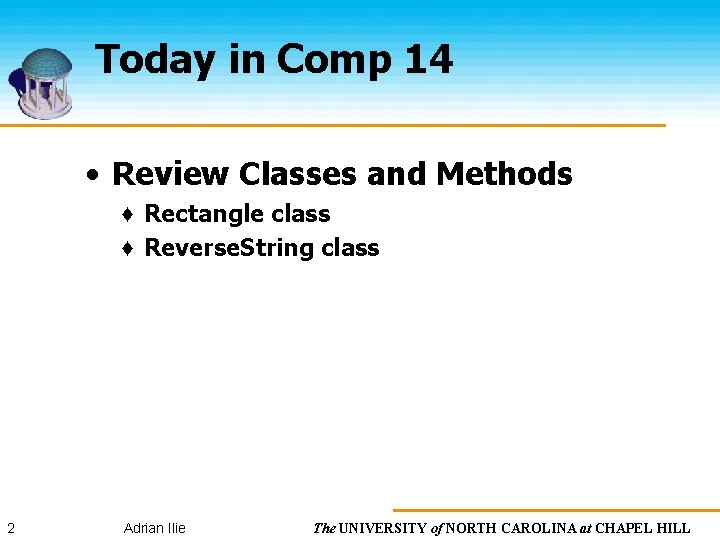
Today in Comp 14 • Review Classes and Methods ♦ Rectangle class ♦ Reverse. String class 2 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
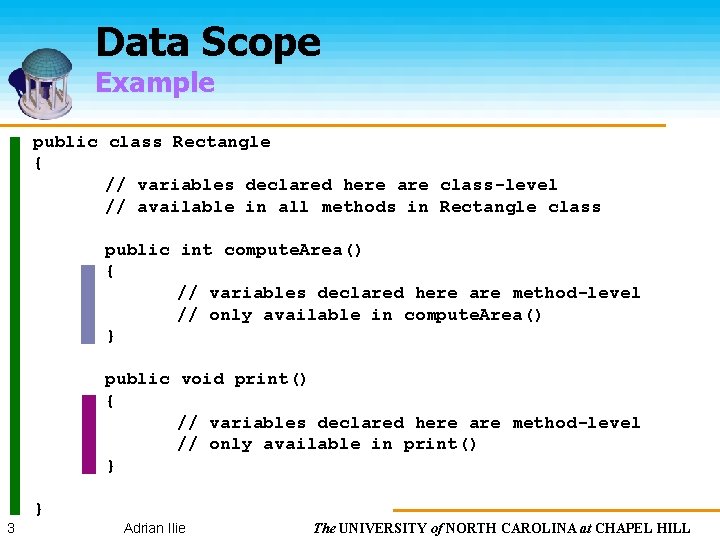
Data Scope Example public class Rectangle { // variables declared here are class-level // available in all methods in Rectangle class public int compute. Area() { // variables declared here are method-level // only available in compute. Area() } public void print() { // variables declared here are method-level // only available in print() } } 3 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
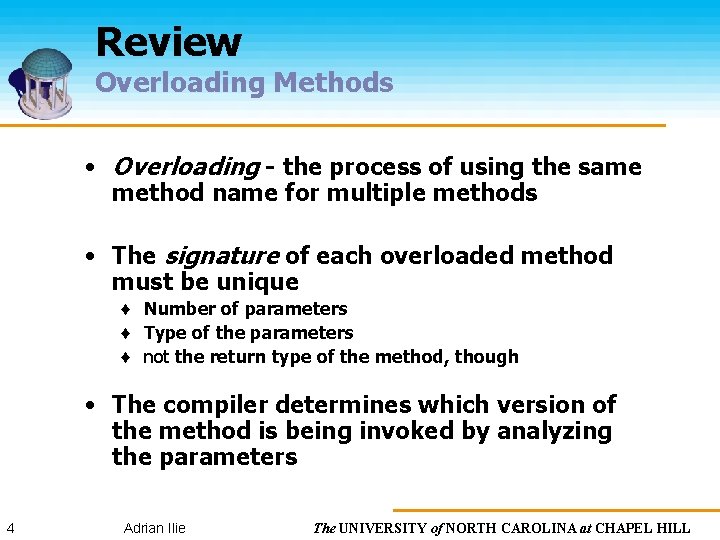
Review Overloading Methods • Overloading - the process of using the same method name for multiple methods • The signature of each overloaded method must be unique ♦ Number of parameters ♦ Type of the parameters ♦ not the return type of the method, though • The compiler determines which version of the method is being invoked by analyzing the parameters 4 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
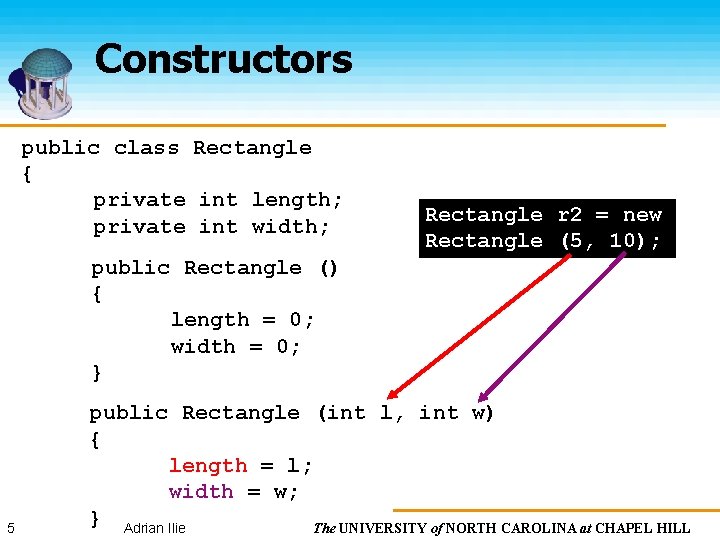
Constructors public class Rectangle { private int length; private int width; Rectangle r 2 = new Rectangle (5, 10); public Rectangle () { length = 0; width = 0; } 5 public Rectangle (int l, int w) { length = l; width = w; } Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
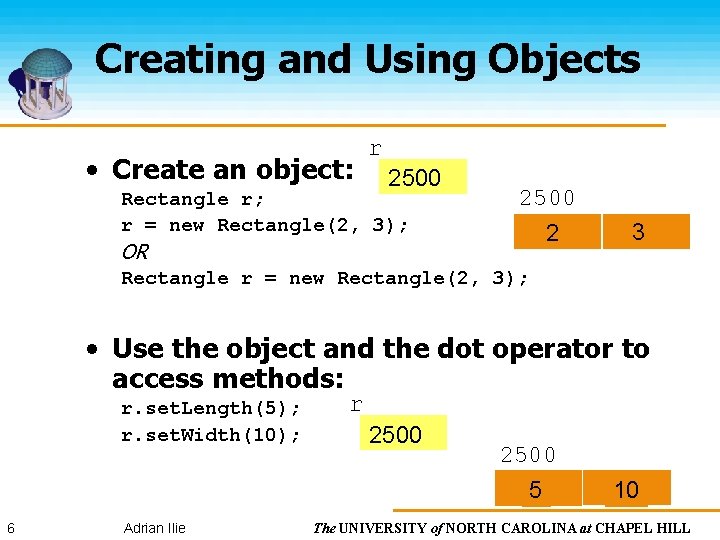
Creating and Using Objects • Create an object: r 2500 Rectangle r; r = new Rectangle(2, 3); OR 2500 2 3 Rectangle r = new Rectangle(2, 3); • Use the object and the dot operator to access methods: r. set. Length(5); r. set. Width(10); 6 Adrian Ilie r 2500 5 2 3 10 The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
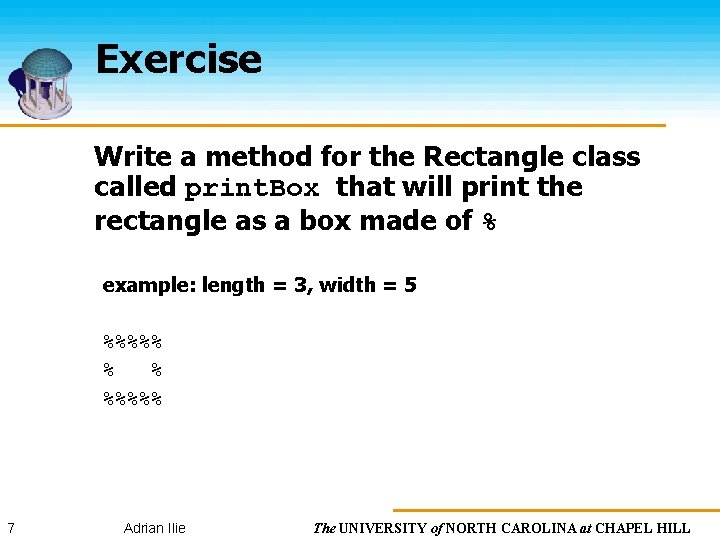
Exercise Write a method for the Rectangle class called print. Box that will print the rectangle as a box made of % example: length = 3, width = 5 %%%%% % % %%%%% 7 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
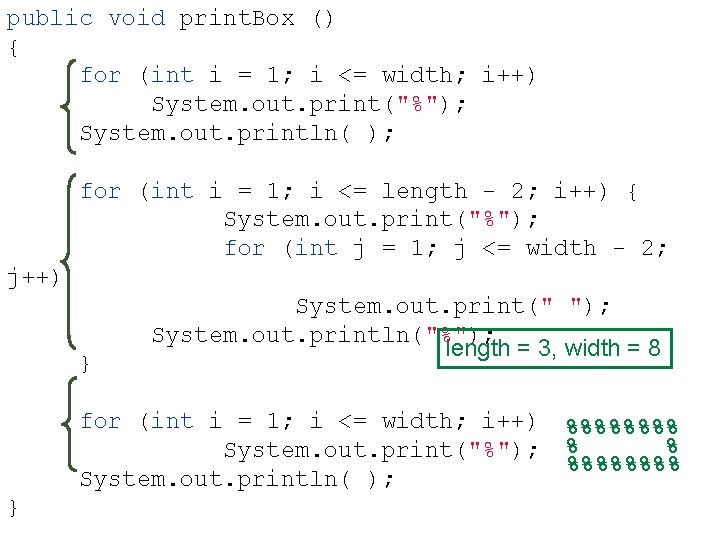
public void print. Box () { for (int i = 1; i <= width; i++) System. out. print("%"); System. out. println( ); for (int i = 1; i <= length - 2; i++) { System. out. print("%"); for (int j = 1; j <= width - 2; j++) } System. out. print(" "); System. out. println("%"); length = 3, width = 8 for (int i = 1; i <= width; i++) System. out. print("%"); System. out. println( ); } 8 Adrian Ilie %%%% % % %%%% The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
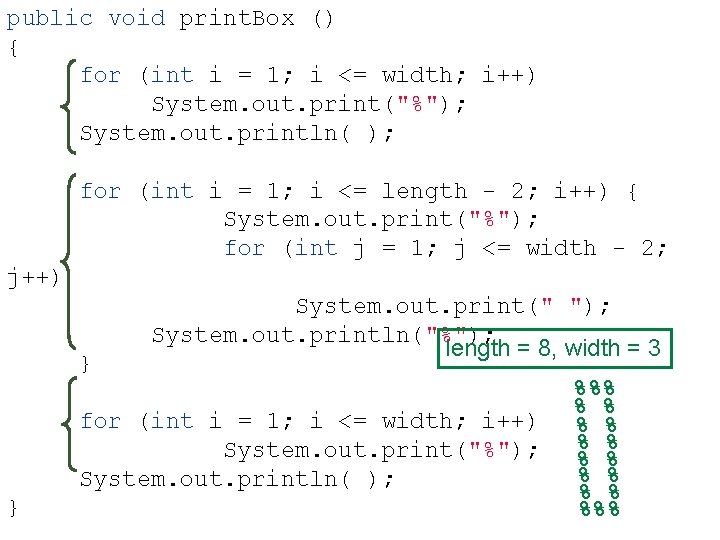
public void print. Box () { for (int i = 1; i <= width; i++) System. out. print("%"); System. out. println( ); for (int i = 1; i <= length - 2; i++) { System. out. print("%"); for (int j = 1; j <= width - 2; j++) } System. out. print(" "); System. out. println("%"); length = 8, width = 3 for (int i = 1; i <= width; i++) System. out. print("%"); System. out. println( ); } 9 Adrian Ilie %%% % % %%% The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
![Testing public class Rectangle Tester public static void main String args Rectangle Testing public class Rectangle. Tester { public static void main (String[] args) { Rectangle](https://slidetodoc.com/presentation_image_h/2653b842746a912119404897b7ebf881/image-10.jpg)
Testing public class Rectangle. Tester { public static void main (String[] args) { Rectangle r 1 = new Rectangle(); Rectangle r 2 = new Rectangle (20, 30); r 1. set. Width(5); r 1. set. Length(10); r 1. print(); What if we just wanted to r 2. print(); output r 1 and r 2 using System. out. println(r 1); System. out. println? System. out. println(r 2); } } // end of Rectangle. Tester class 10 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
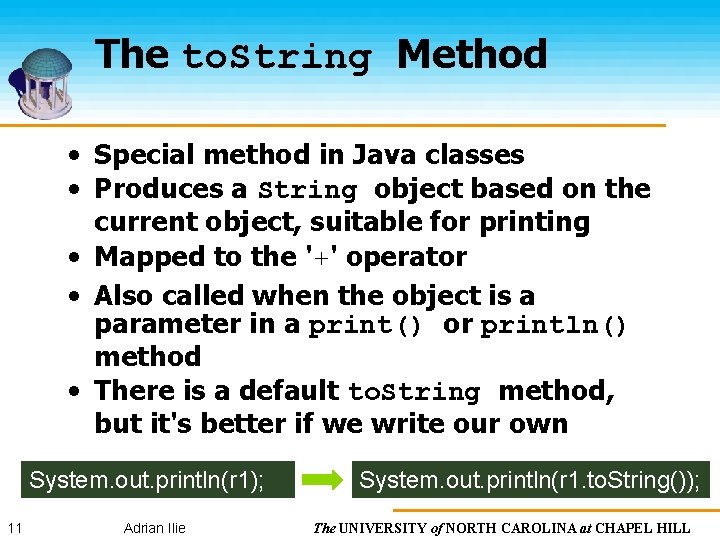
The to. String Method • Special method in Java classes • Produces a String object based on the current object, suitable for printing • Mapped to the '+' operator • Also called when the object is a parameter in a print() or println() method • There is a default to. String method, but it's better if we write our own System. out. println(r 1); 11 Adrian Ilie System. out. println(r 1. to. String()); The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
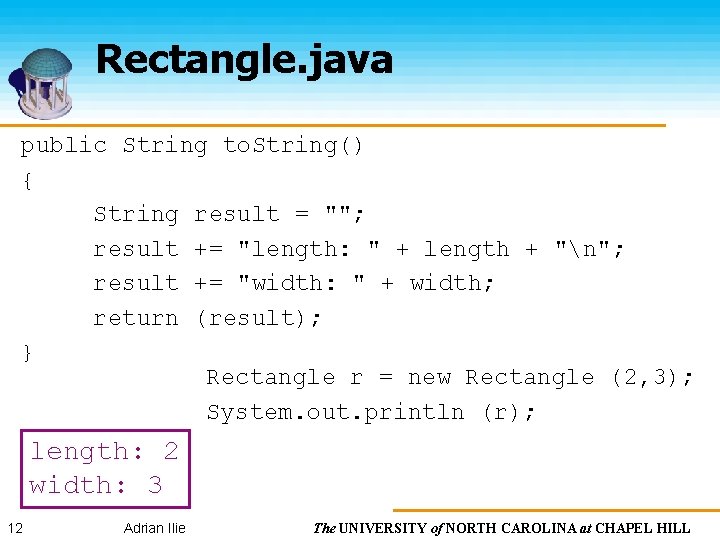
Rectangle. java public String to. String() { String result = ""; result += "length: " + length + "n"; result += "width: " + width; return (result); } Rectangle r = new Rectangle (2, 3); System. out. println (r); length: 2 width: 3 12 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
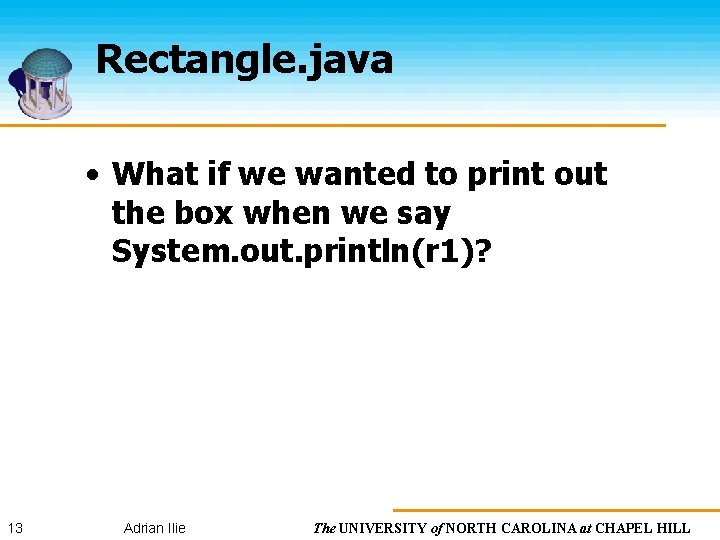
Rectangle. java • What if we wanted to print out the box when we say System. out. println(r 1)? 13 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
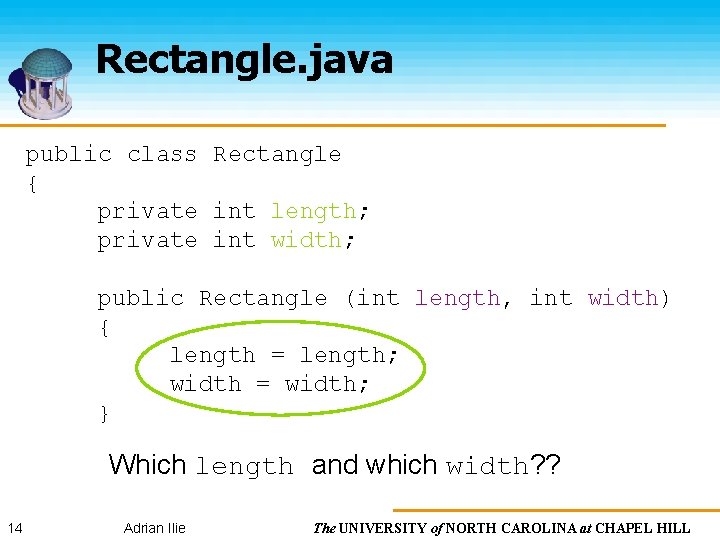
Rectangle. java public class Rectangle { private int length; private int width; public Rectangle (int length, int width) { length = length; width = width; } Which length and which width? ? 14 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
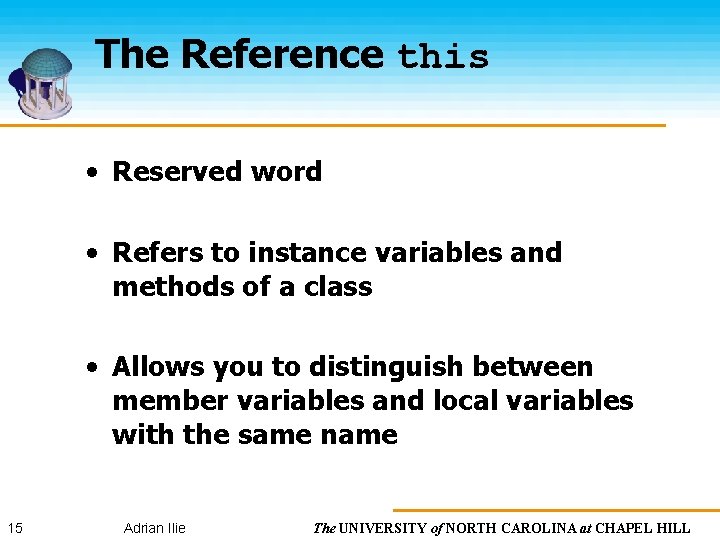
The Reference this • Reserved word • Refers to instance variables and methods of a class • Allows you to distinguish between member variables and local variables with the same name 15 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
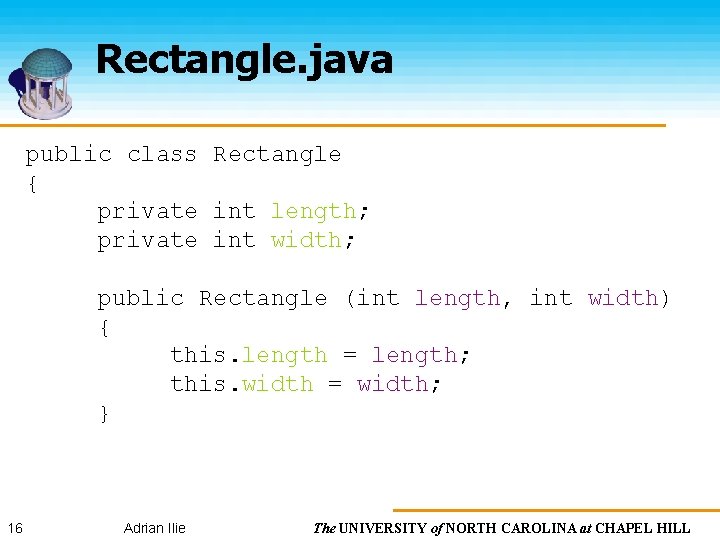
Rectangle. java public class Rectangle { private int length; private int width; public Rectangle (int length, int width) { this. length = length; this. width = width; } 16 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
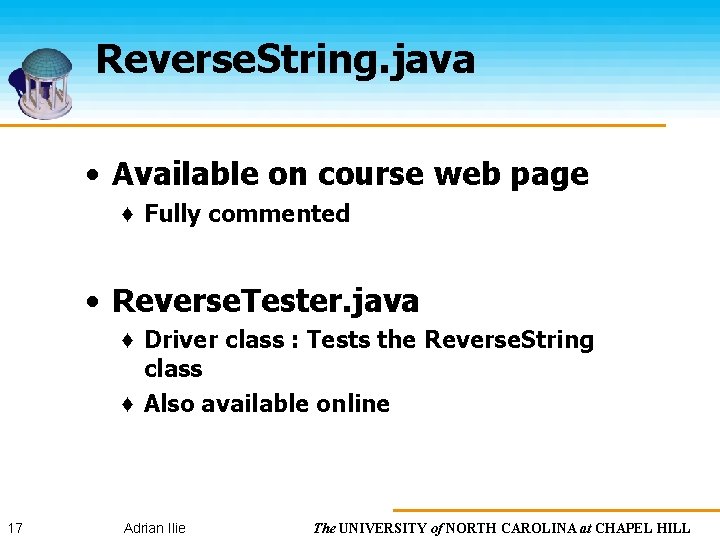
Reverse. String. java • Available on course web page ♦ Fully commented • Reverse. Tester. java ♦ Driver class : Tests the Reverse. String class ♦ Also available online 17 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
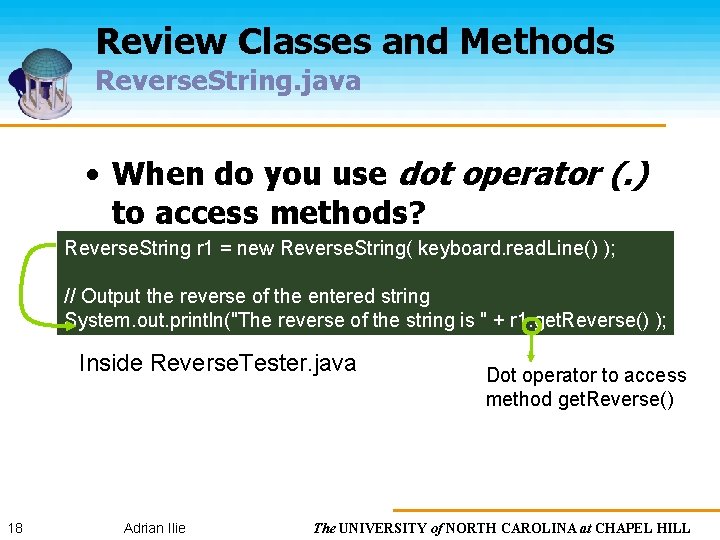
Review Classes and Methods Reverse. String. java • When do you use dot operator (. ) to access methods? Reverse. String r 1 = new Reverse. String( keyboard. read. Line() ); // Output the reverse of the entered string System. out. println("The reverse of the string is " + r 1. get. Reverse() ); Inside Reverse. Tester. java 18 Adrian Ilie Dot operator to access method get. Reverse() The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
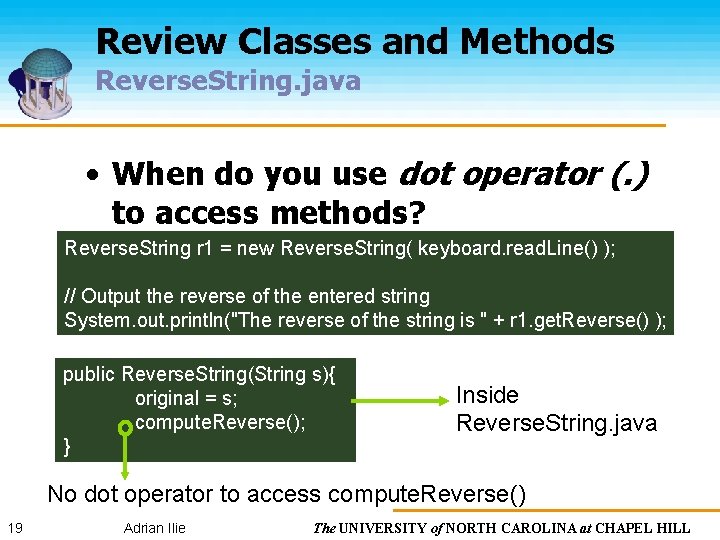
Review Classes and Methods Reverse. String. java • When do you use dot operator (. ) to access methods? Reverse. String r 1 = new Reverse. String( keyboard. read. Line() ); // Output the reverse of the entered string System. out. println("The reverse of the string is " + r 1. get. Reverse() ); public Reverse. String(String s){ original = s; compute. Reverse(); } Inside Reverse. String. java No dot operator to access compute. Reverse() 19 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
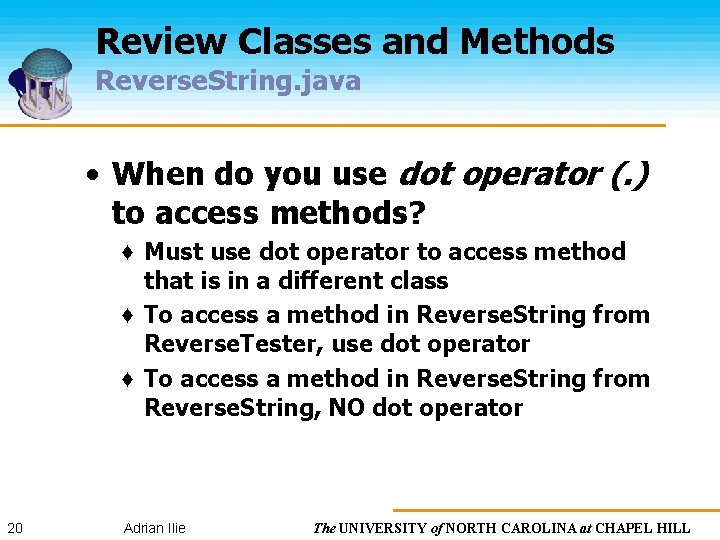
Review Classes and Methods Reverse. String. java • When do you use dot operator (. ) to access methods? ♦ Must use dot operator to access method that is in a different class ♦ To access a method in Reverse. String from Reverse. Tester, use dot operator ♦ To access a method in Reverse. String from Reverse. String, NO dot operator 20 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
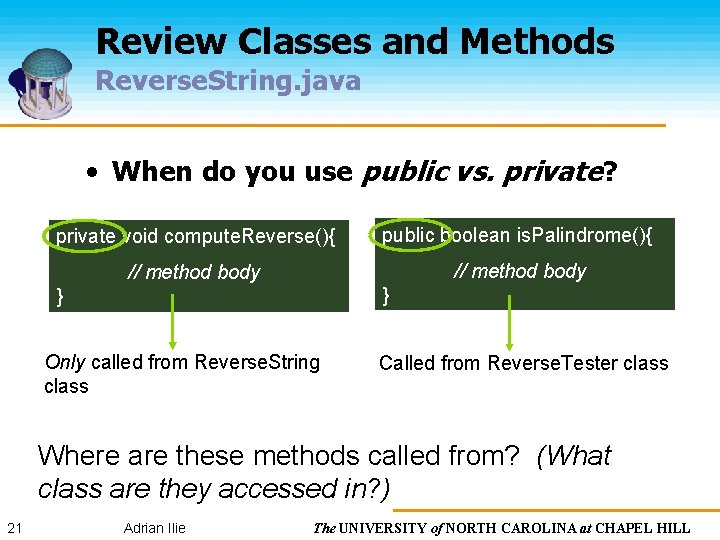
Review Classes and Methods Reverse. String. java • When do you use public vs. private? private void compute. Reverse(){ public boolean is. Palindrome(){ // method body } } Only called from Reverse. String class Called from Reverse. Tester class Where are these methods called from? (What class are they accessed in? ) 21 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
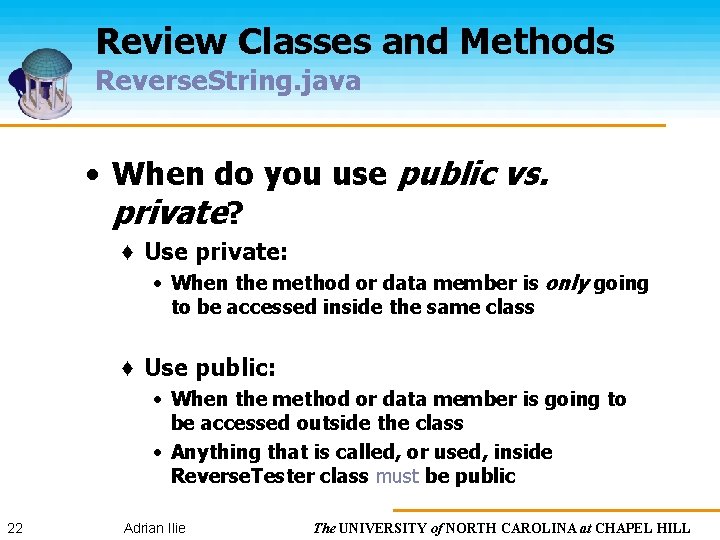
Review Classes and Methods Reverse. String. java • When do you use public vs. private? ♦ Use private: • When the method or data member is only going to be accessed inside the same class ♦ Use public: • When the method or data member is going to be accessed outside the class • Anything that is called, or used, inside Reverse. Tester class must be public 22 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
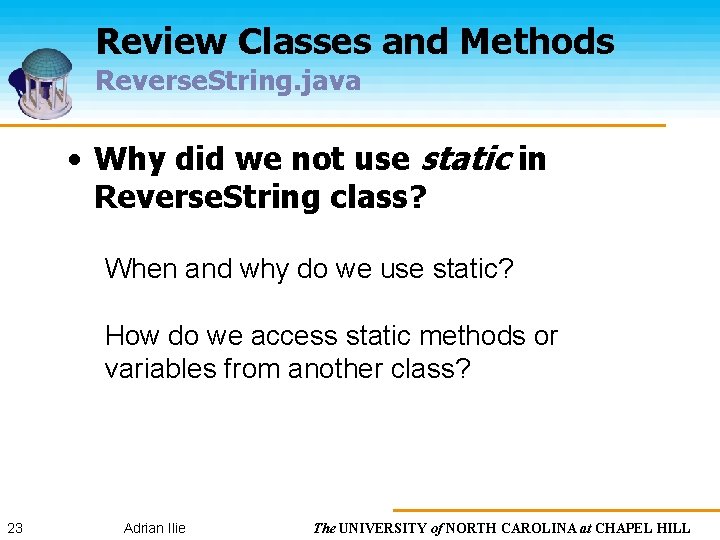
Review Classes and Methods Reverse. String. java • Why did we not use static in Reverse. String class? When and why do we use static? How do we access static methods or variables from another class? 23 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
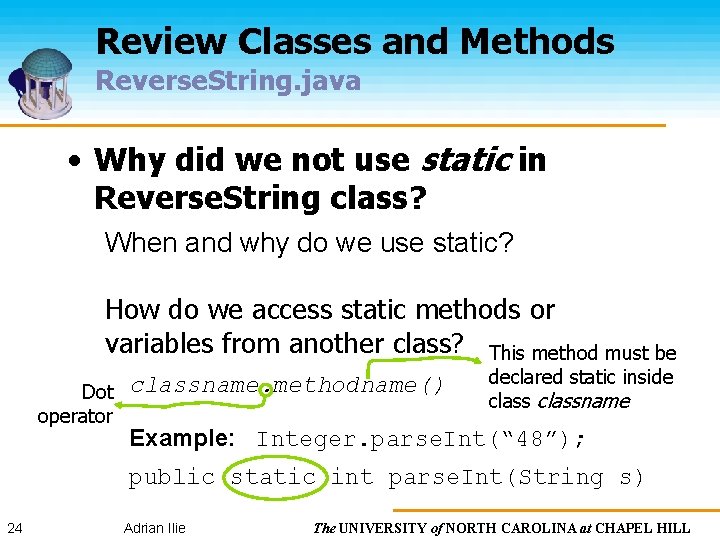
Review Classes and Methods Reverse. String. java • Why did we not use static in Reverse. String class? When and why do we use static? How do we access static methods or variables from another class? This method must be Dot classname. methodname() operator declared static inside classname Example: Integer. parse. Int(“ 48”); public static int parse. Int(String s) 24 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
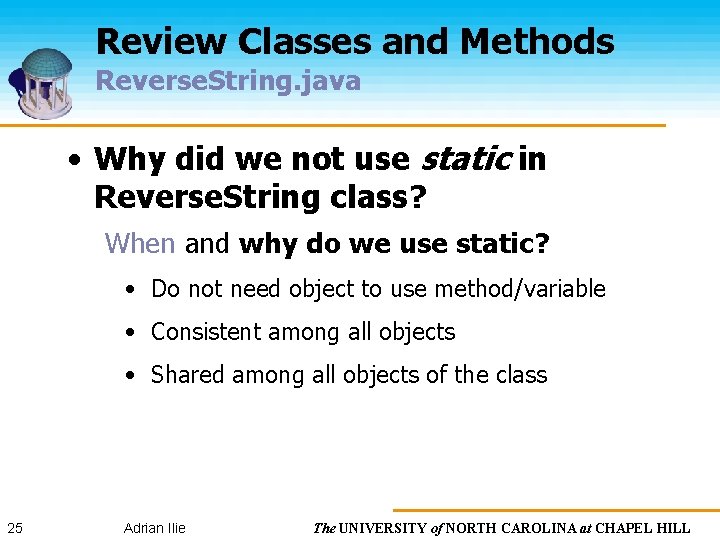
Review Classes and Methods Reverse. String. java • Why did we not use static in Reverse. String class? When and why do we use static? • Do not need object to use method/variable • Consistent among all objects • Shared among all objects of the class 25 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
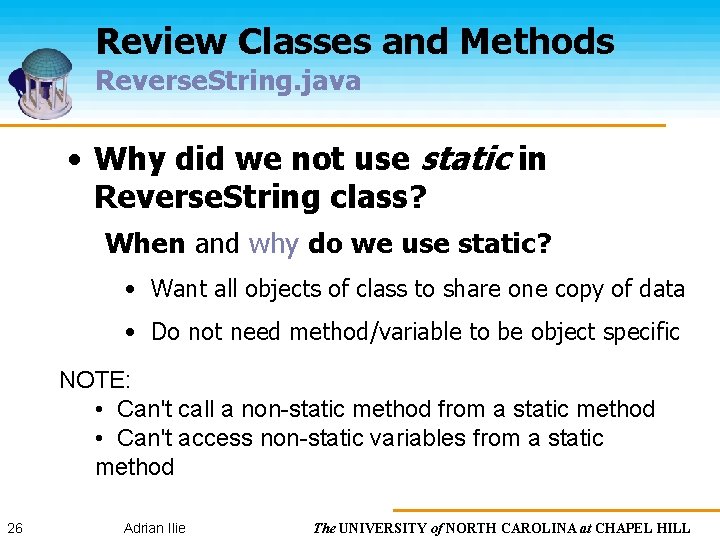
Review Classes and Methods Reverse. String. java • Why did we not use static in Reverse. String class? When and why do we use static? • Want all objects of class to share one copy of data • Do not need method/variable to be object specific NOTE: • Can't call a non-static method from a static method • Can't access non-static variables from a static method 26 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
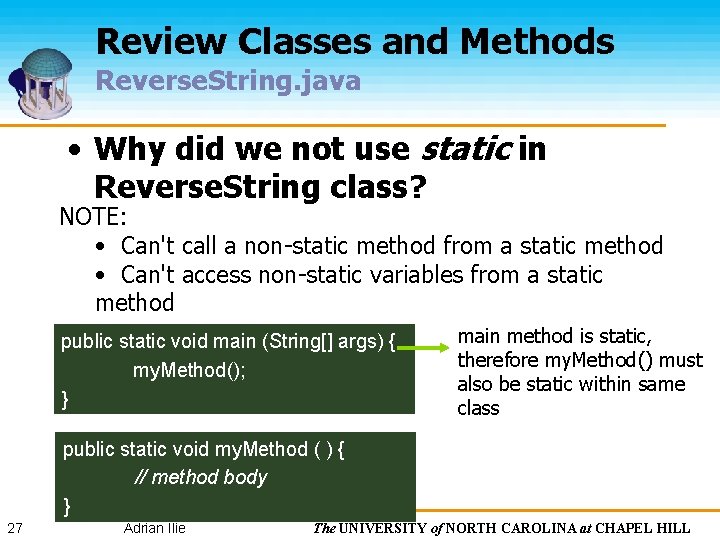
Review Classes and Methods Reverse. String. java • Why did we not use static in Reverse. String class? NOTE: • Can't call a non-static method from a static method • Can't access non-static variables from a static method public static void main (String[] args) { my. Method(); } main method is static, therefore my. Method() must also be static within same class public static void my. Method ( ) { // method body } 27 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
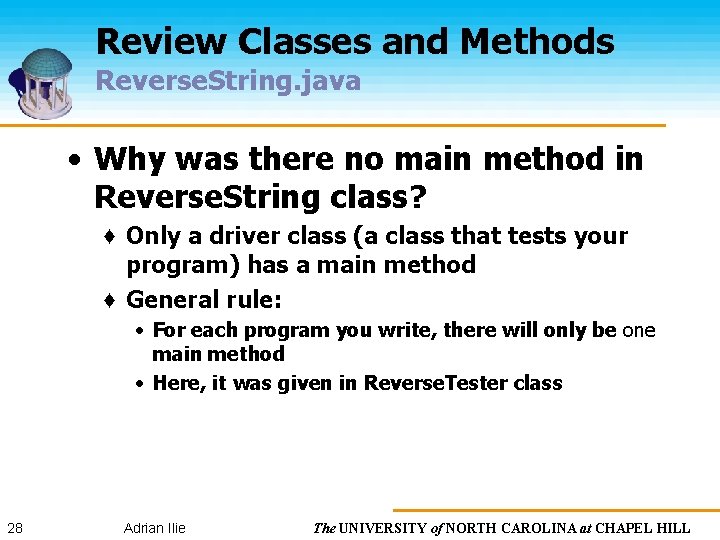
Review Classes and Methods Reverse. String. java • Why was there no main method in Reverse. String class? ♦ Only a driver class (a class that tests your program) has a main method ♦ General rule: • For each program you write, there will only be one main method • Here, it was given in Reverse. Tester class 28 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
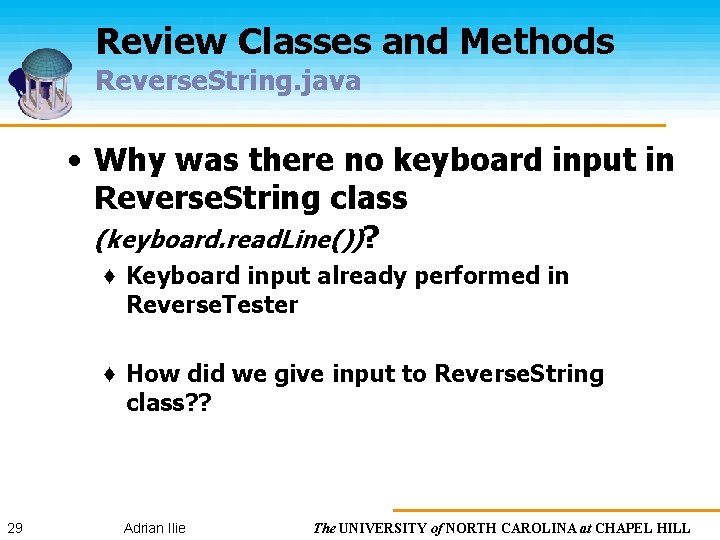
Review Classes and Methods Reverse. String. java • Why was there no keyboard input in Reverse. String class (keyboard. read. Line())? ♦ Keyboard input already performed in Reverse. Tester ♦ How did we give input to Reverse. String class? ? 29 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
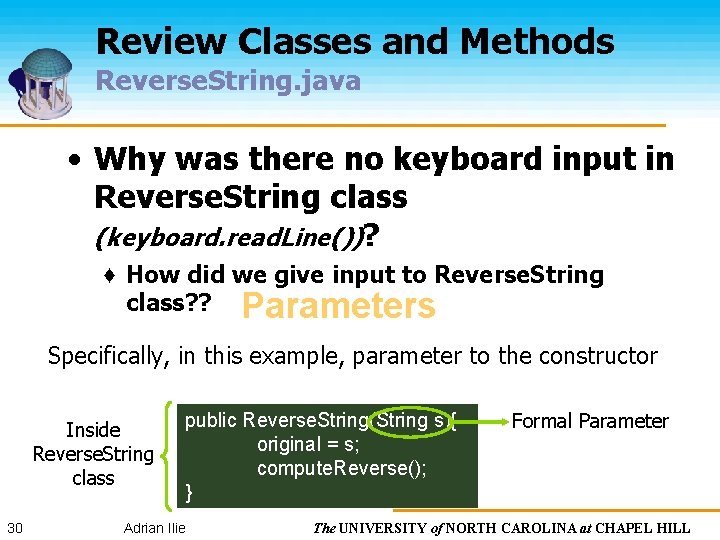
Review Classes and Methods Reverse. String. java • Why was there no keyboard input in Reverse. String class (keyboard. read. Line())? ♦ How did we give input to Reverse. String class? ? Parameters Specifically, in this example, parameter to the constructor Inside Reverse. String class 30 public Reverse. String(String s){ original = s; compute. Reverse(); } Adrian Ilie Formal Parameter The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
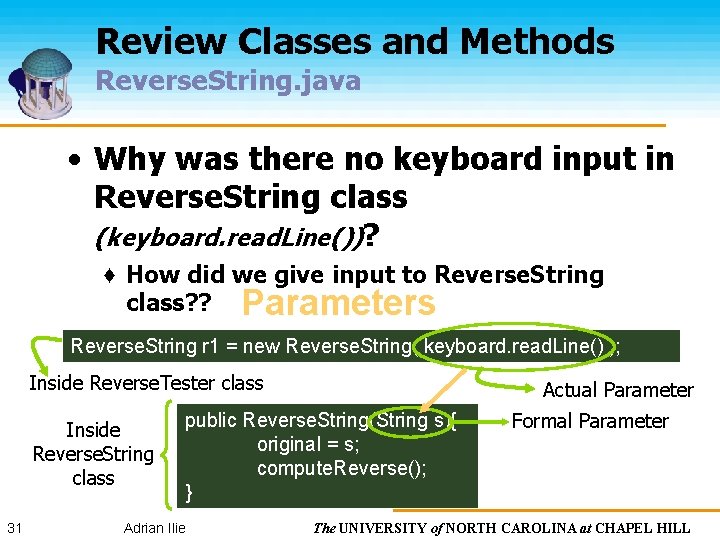
Review Classes and Methods Reverse. String. java • Why was there no keyboard input in Reverse. String class (keyboard. read. Line())? ♦ How did we give input to Reverse. String class? ? Parameters Reverse. String r 1 = new Reverse. String( keyboard. read. Line() ); Inside Reverse. Tester class Inside Reverse. String class 31 Actual Parameter public Reverse. String(String s){ original = s; compute. Reverse(); } Adrian Ilie Formal Parameter The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
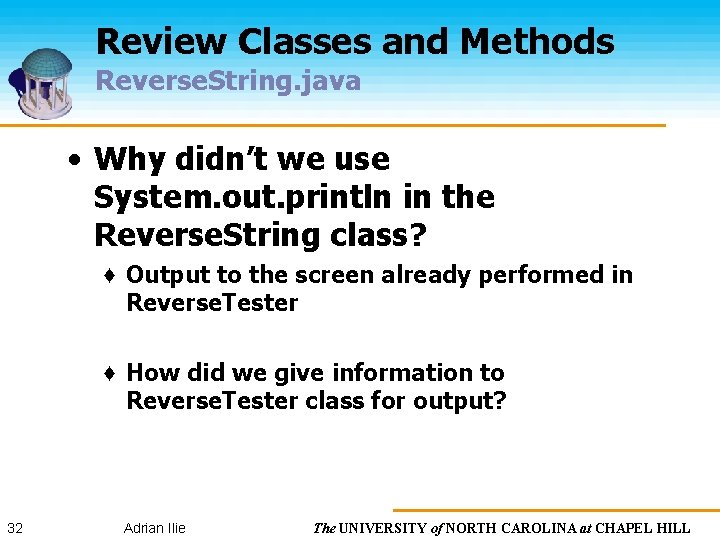
Review Classes and Methods Reverse. String. java • Why didn’t we use System. out. println in the Reverse. String class? ♦ Output to the screen already performed in Reverse. Tester ♦ How did we give information to Reverse. Tester class for output? 32 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
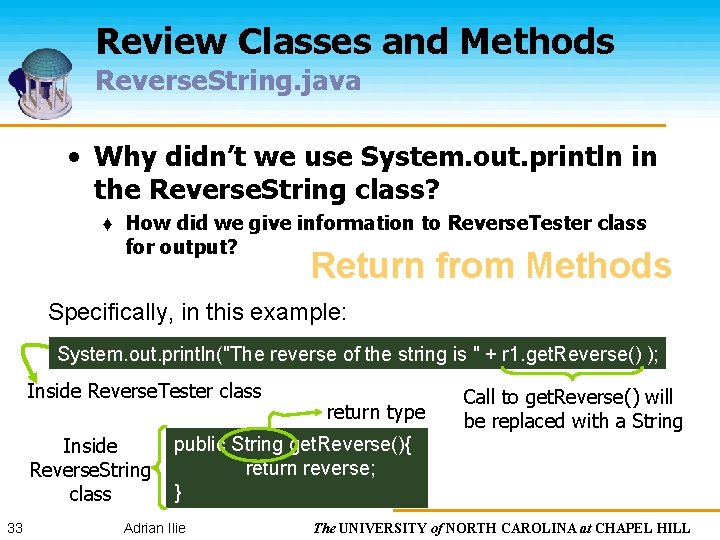
Review Classes and Methods Reverse. String. java • Why didn’t we use System. out. println in the Reverse. String class? ♦ How did we give information to Reverse. Tester class for output? Return from Methods Specifically, in this example: System. out. println("The reverse of the string is " + r 1. get. Reverse() ); Inside Reverse. Tester class Inside Reverse. String class 33 return type Call to get. Reverse() will be replaced with a String public String get. Reverse(){ return reverse; } Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
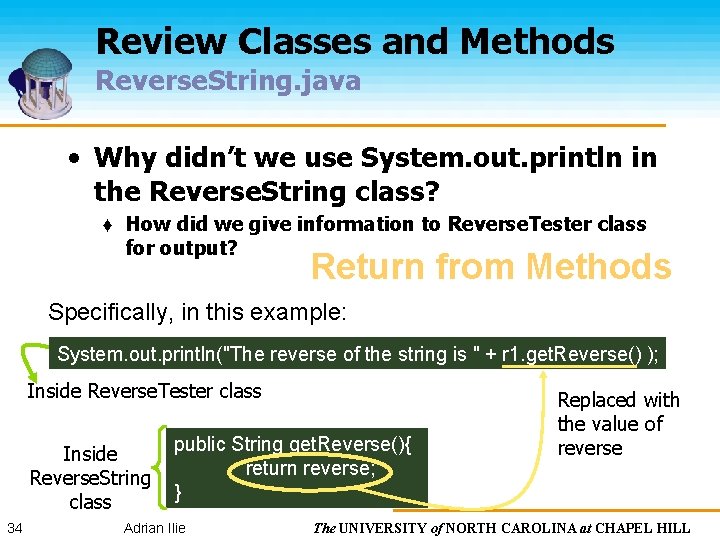
Review Classes and Methods Reverse. String. java • Why didn’t we use System. out. println in the Reverse. String class? ♦ How did we give information to Reverse. Tester class for output? Return from Methods Specifically, in this example: System. out. println("The reverse of the string is " + r 1. get. Reverse() ); Inside Reverse. Tester class Inside Reverse. String class 34 public String get. Reverse(){ return reverse; } Adrian Ilie Replaced with the value of reverse The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
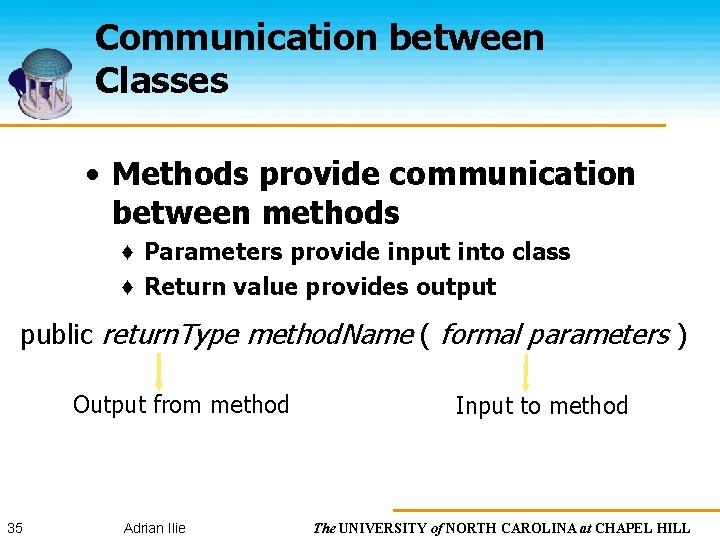
Communication between Classes • Methods provide communication between methods ♦ Parameters provide input into class ♦ Return value provides output public return. Type method. Name ( formal parameters ) Output from method 35 Adrian Ilie Input to method The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
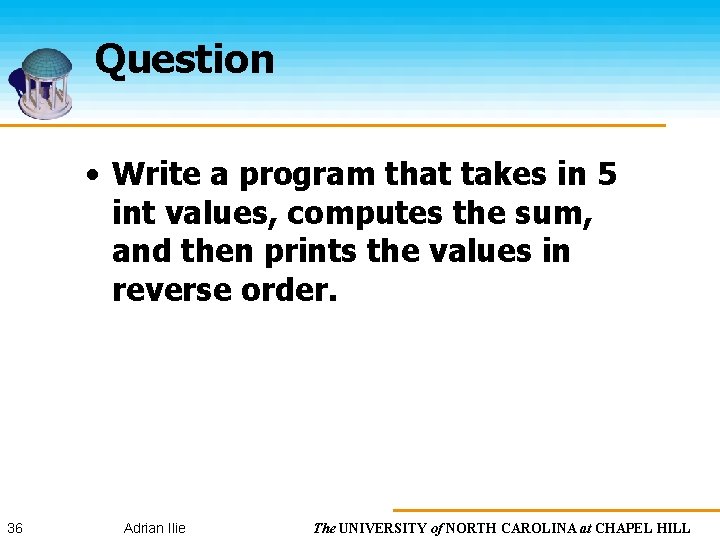
Question • Write a program that takes in 5 int values, computes the sum, and then prints the values in reverse order. 36 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL
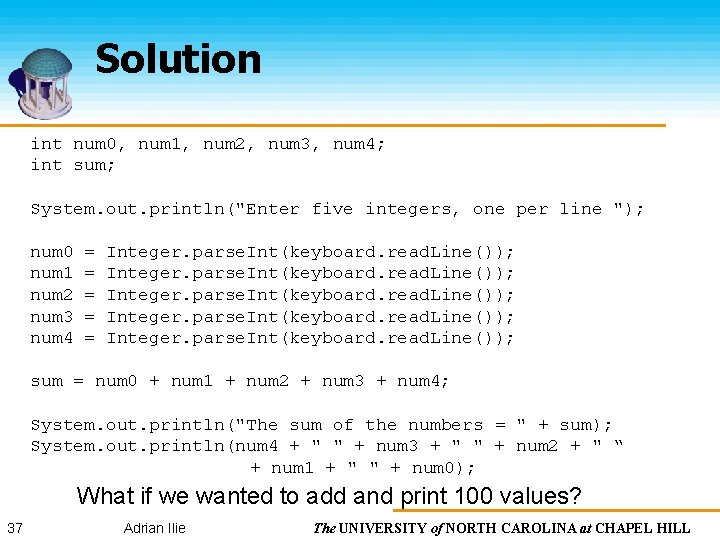
Solution int num 0, num 1, num 2, num 3, num 4; int sum; System. out. println("Enter five integers, one per line "); num 0 num 1 num 2 num 3 num 4 = = = Integer. parse. Int(keyboard. read. Line()); sum = num 0 + num 1 + num 2 + num 3 + num 4; System. out. println("The sum of the numbers = " + sum); System. out. println(num 4 + " " + num 3 + " " + num 2 + " “ + num 1 + " " + num 0); What if we wanted to add and print 100 values? 37 Adrian Ilie The UNIVERSITY of NORTH CAROLINA at CHAPEL HILL