COMP 103 Linked Lists 2 RECAPTODAY RECAP Linked
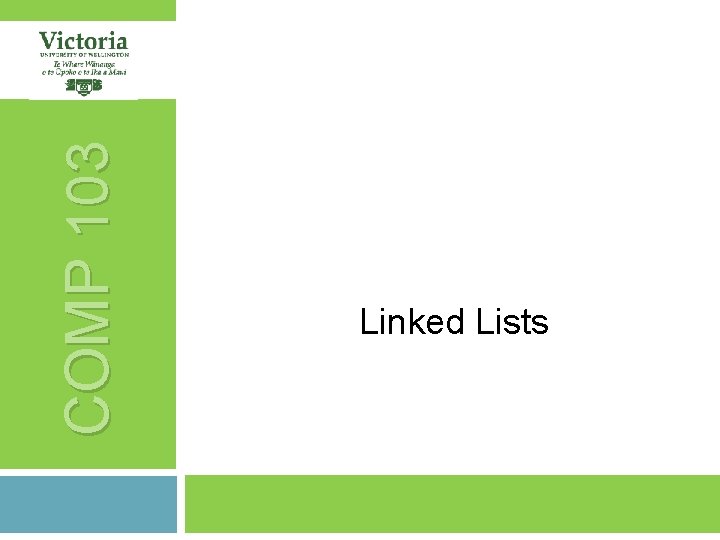
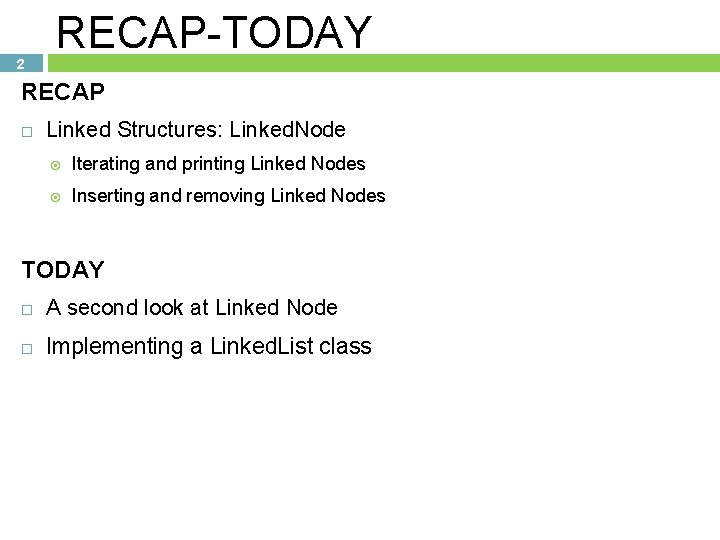
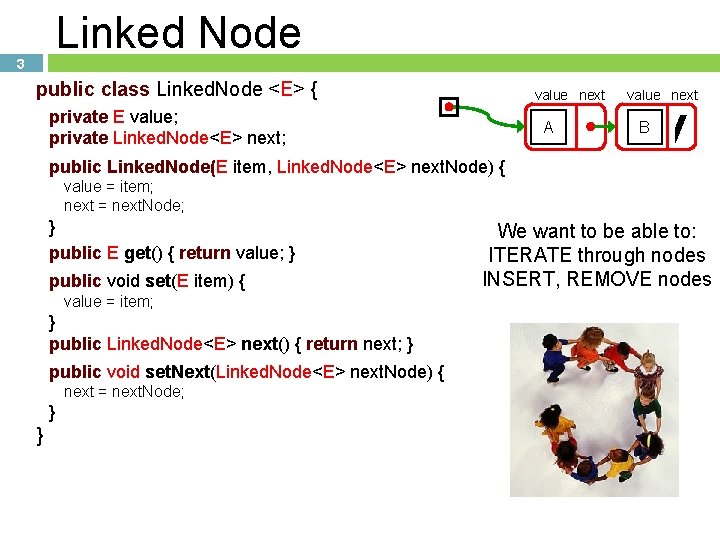
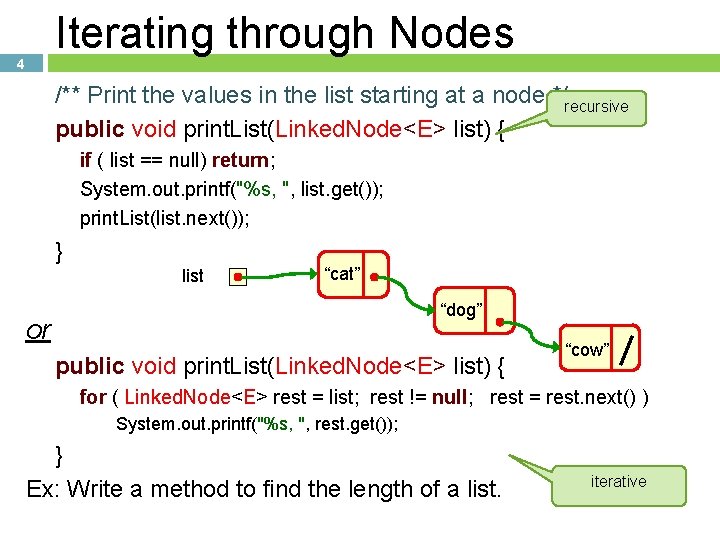
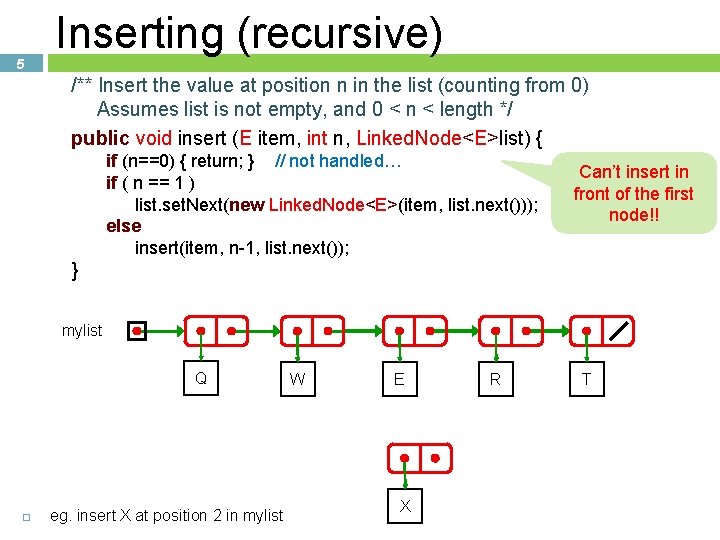
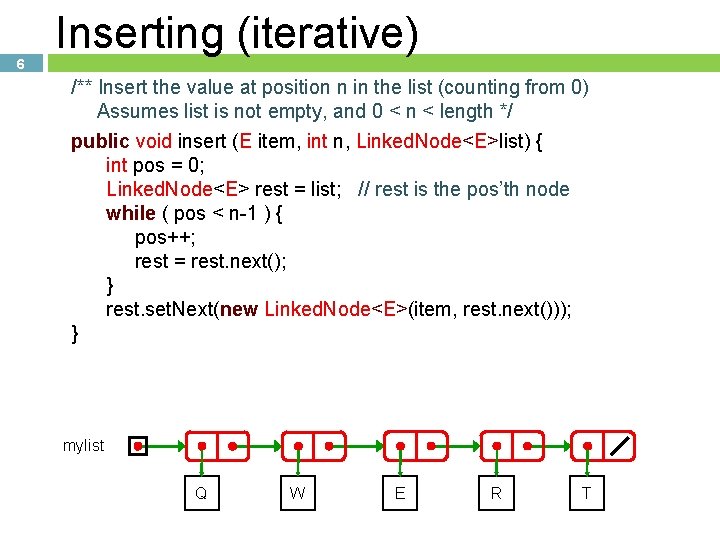
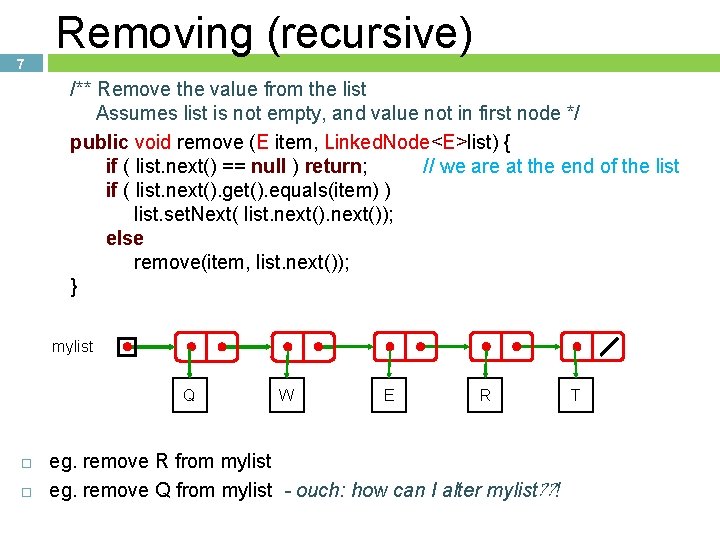
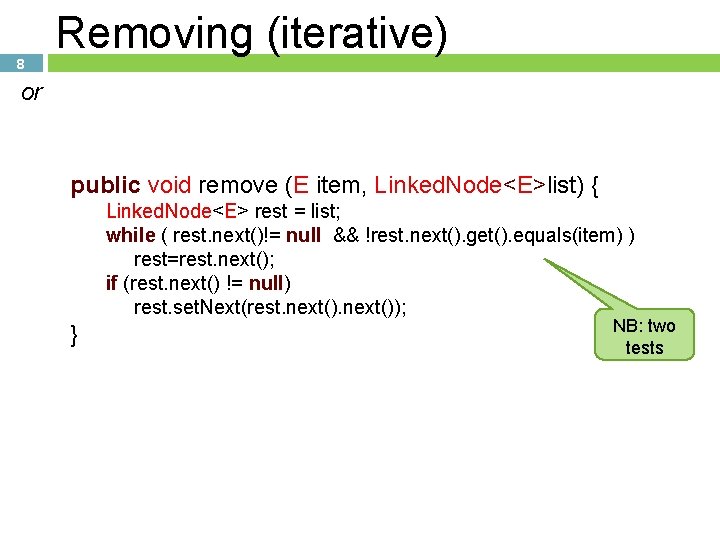
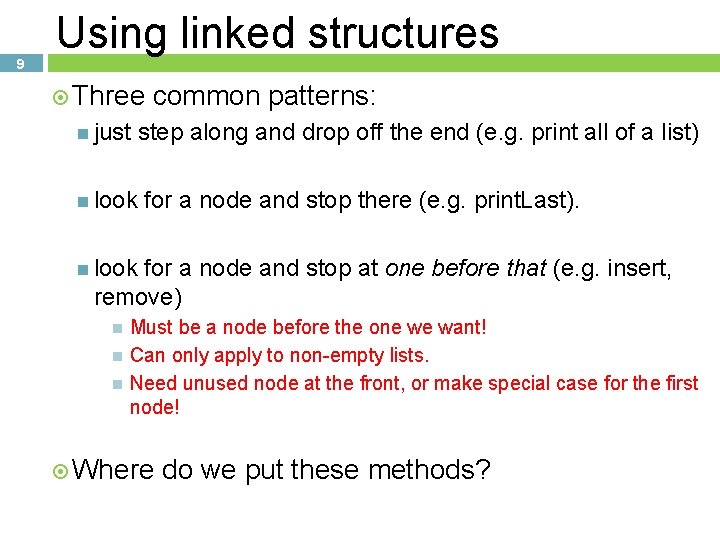
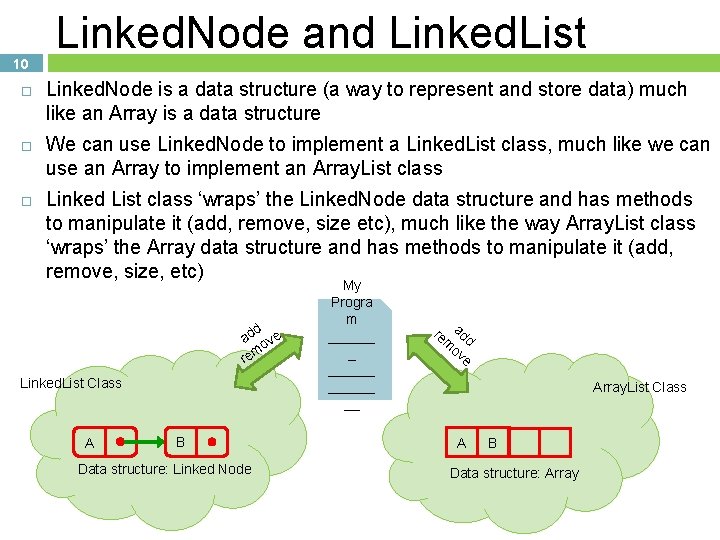
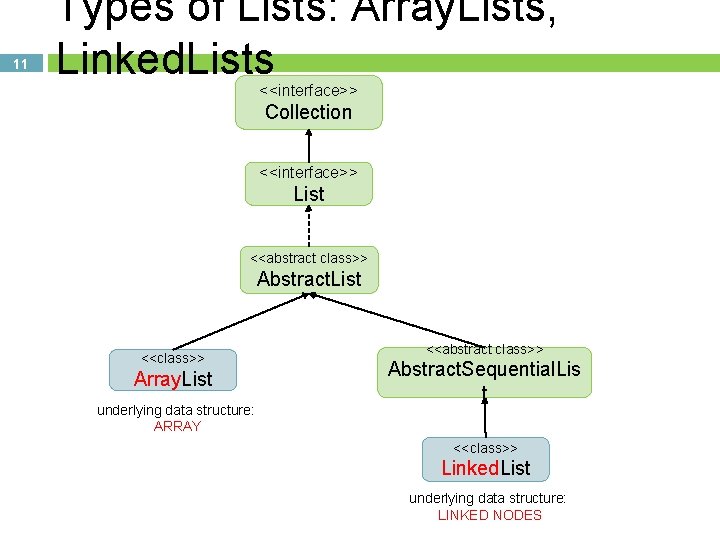
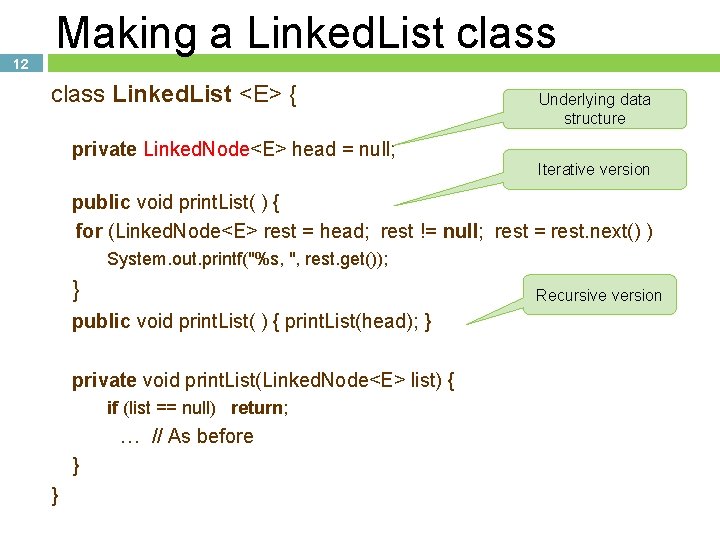
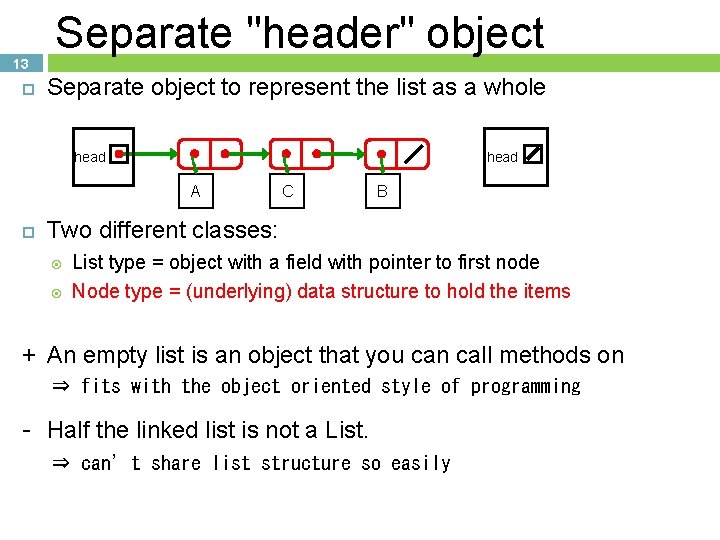
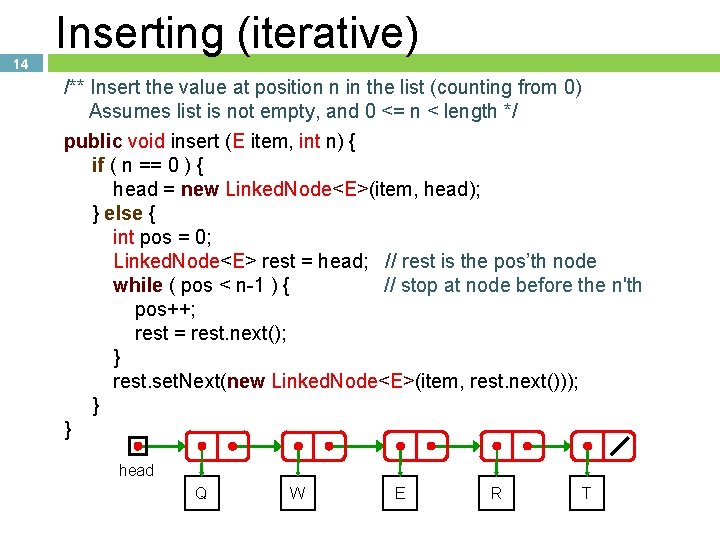
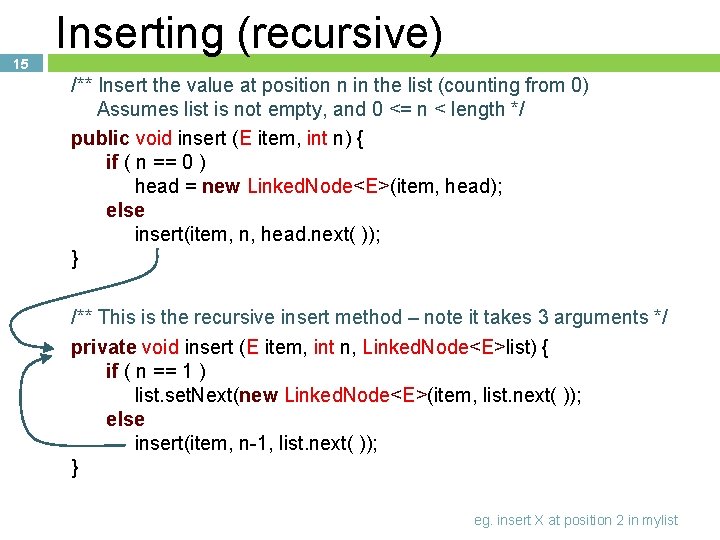
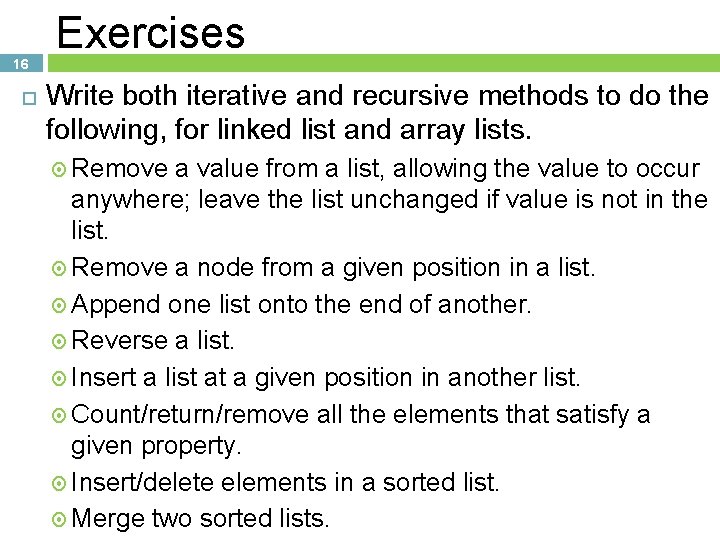
- Slides: 16
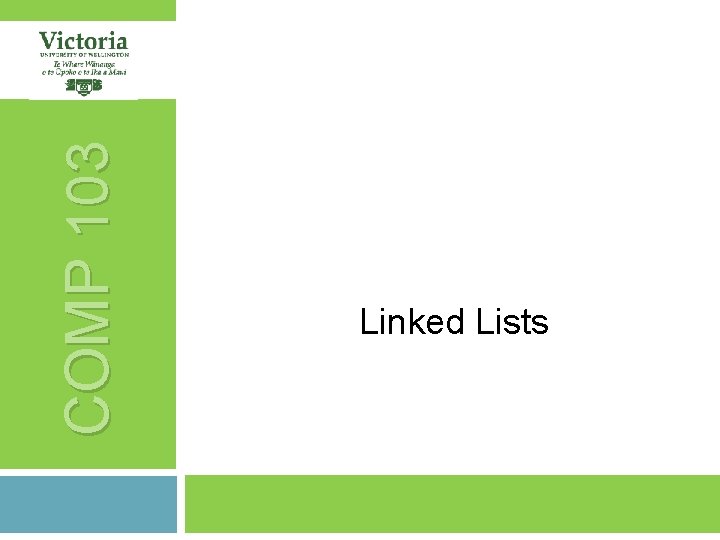
COMP 103 Linked Lists
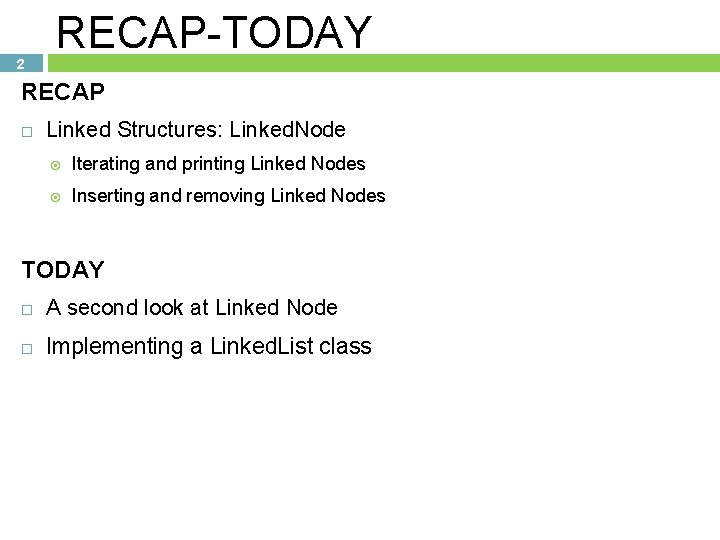
2 RECAP-TODAY RECAP Linked Structures: Linked. Node Iterating and printing Linked Nodes Inserting and removing Linked Nodes TODAY A second look at Linked Node Implementing a Linked. List class
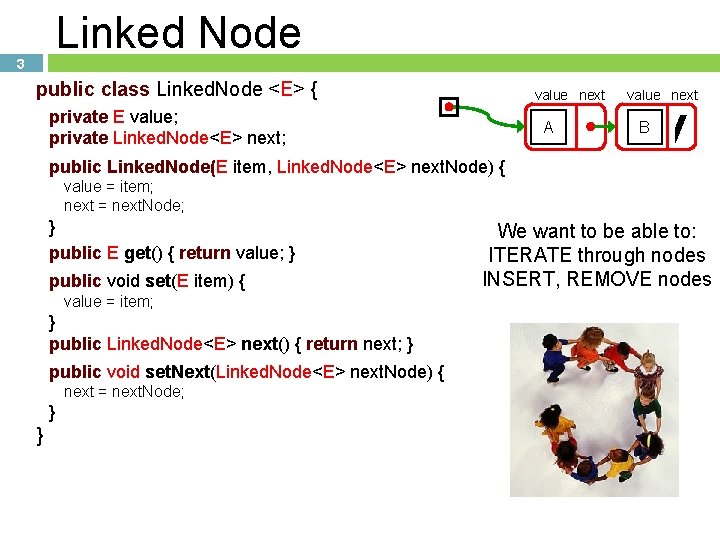
Linked Node 3 public class Linked. Node <E> { value next private E value; private Linked. Node<E> next; A value next B public Linked. Node(E item, Linked. Node<E> next. Node) { value = item; next = next. Node; } public E get() { return value; } public void set(E item) { value = item; } public Linked. Node<E> next() { return next; } public void set. Next(Linked. Node<E> next. Node) { next = next. Node; } } We want to be able to: ITERATE through nodes INSERT, REMOVE nodes
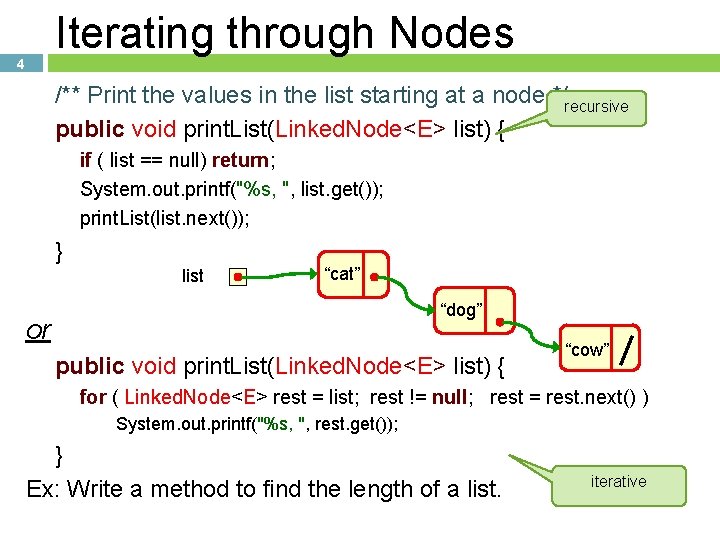
Iterating through Nodes 4 /** Print the values in the list starting at a node */recursive public void print. List(Linked. Node<E> list) { if ( list == null) return; System. out. printf("%s, ", list. get()); print. List(list. next()); } list “cat” “dog” or public void print. List(Linked. Node<E> list) { “cow” for ( Linked. Node<E> rest = list; rest != null; rest = rest. next() ) System. out. printf("%s, ", rest. get()); } Ex: Write a method to find the length of a list. iterative
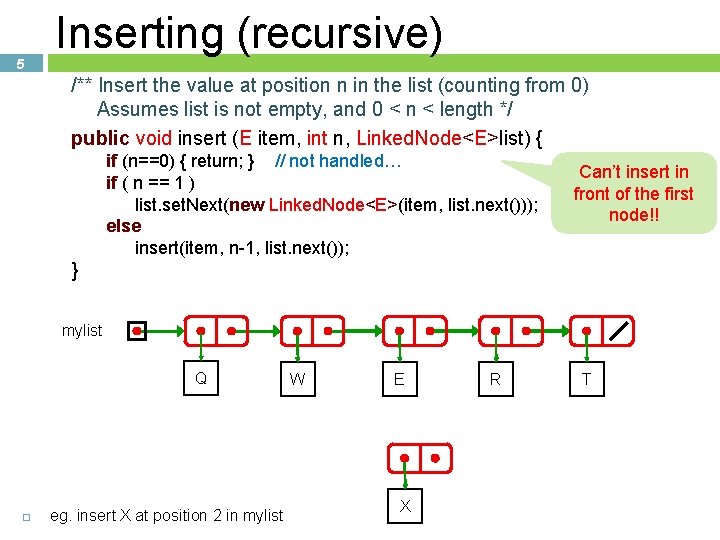
5 Inserting (recursive) /** Insert the value at position n in the list (counting from 0) Assumes list is not empty, and 0 < n < length */ public void insert (E item, int n, Linked. Node<E>list) { if (n==0) { return; } // not handled… if ( n == 1 ) list. set. Next(new Linked. Node<E>(item, list. next())); else insert(item, n-1, list. next()); Can’t insert in front of the first node!! } mylist Q eg. insert X at position 2 in mylist W E X R T
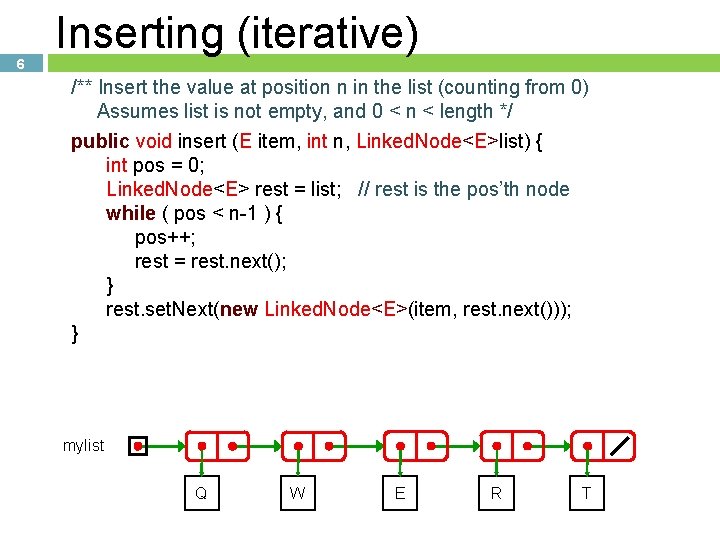
6 Inserting (iterative) /** Insert the value at position n in the list (counting from 0) Assumes list is not empty, and 0 < n < length */ public void insert (E item, int n, Linked. Node<E>list) { int pos = 0; Linked. Node<E> rest = list; // rest is the pos’th node while ( pos < n-1 ) { pos++; rest = rest. next(); } rest. set. Next(new Linked. Node<E>(item, rest. next())); } mylist Q W E R T
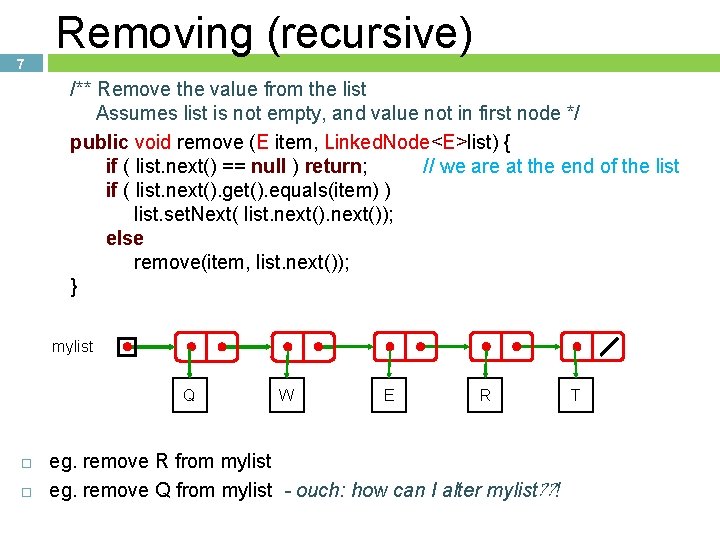
7 Removing (recursive) /** Remove the value from the list Assumes list is not empty, and value not in first node */ public void remove (E item, Linked. Node<E>list) { if ( list. next() == null ) return; // we are at the end of the list if ( list. next(). get(). equals(item) ) list. set. Next( list. next()); else remove(item, list. next()); } mylist Q W E R eg. remove R from mylist eg. remove Q from mylist - ouch: how can I alter mylist? ? ! T
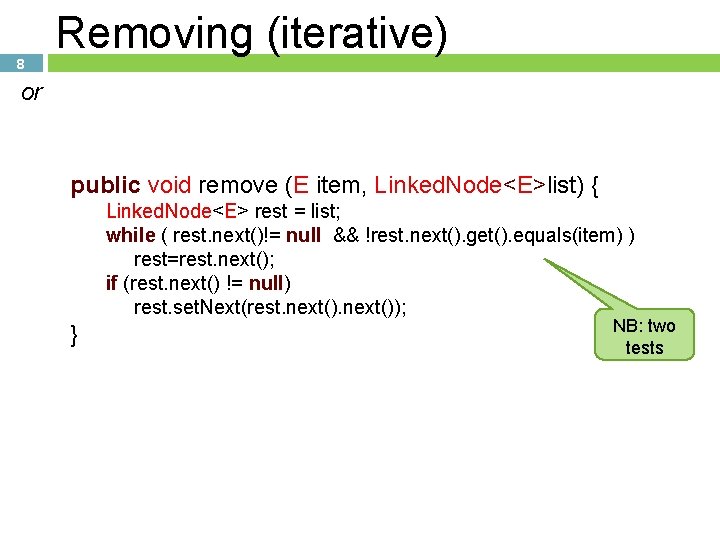
8 Removing (iterative) or public void remove (E item, Linked. Node<E>list) { Linked. Node<E> rest = list; while ( rest. next()!= null && !rest. next(). get(). equals(item) ) rest=rest. next(); if (rest. next() != null) rest. set. Next(rest. next()); } NB: two tests
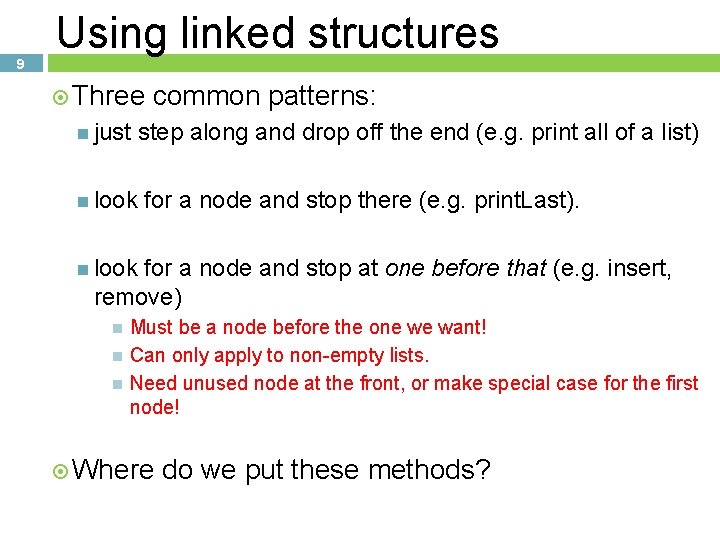
9 Using linked structures Three just common patterns: step along and drop off the end (e. g. print all of a list) look for a node and stop there (e. g. print. Last). look for a node and stop at one before that (e. g. insert, remove) Must be a node before the one we want! Can only apply to non-empty lists. Need unused node at the front, or make special case for the first node! Where do we put these methods?
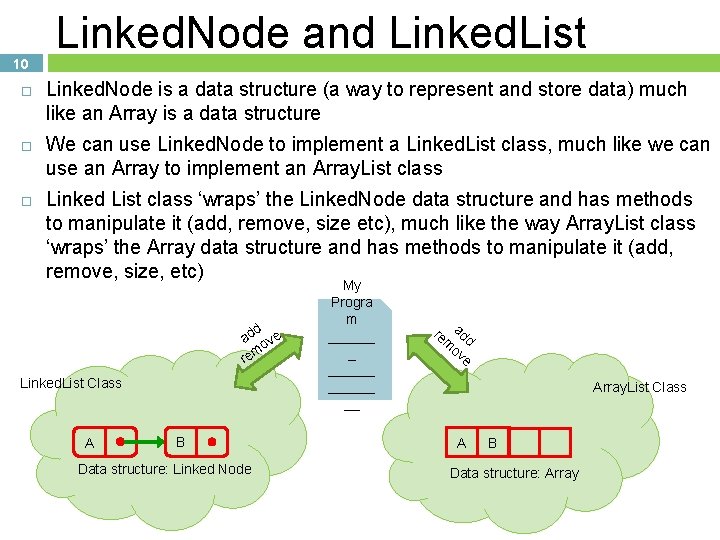
10 Linked. Node and Linked. List Linked. Node is a data structure (a way to represent and store data) much like an Array is a data structure We can use Linked. Node to implement a Linked. List class, much like we can use an Array to implement an Array. List class Linked List class ‘wraps’ the Linked. Node data structure and has methods to manipulate it (add, remove, size etc), much like the way Array. List class ‘wraps’ the Array data structure and has methods to manipulate it (add, remove, size, etc) d ad ove m re Linked. List Class A B Data structure: Linked Node My Progra m ______ __ re add m ov e Array. List Class A B Data structure: Array
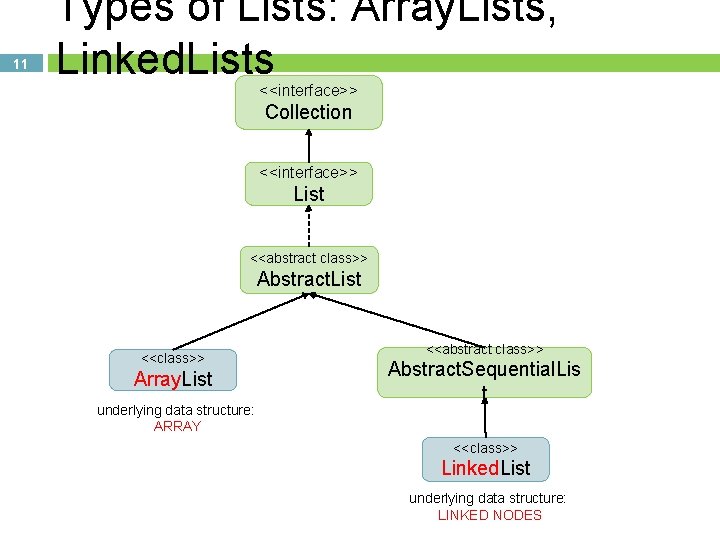
11 Types of Lists: Array. Lists, Linked. Lists <<interface>> Collection <<interface>> List <<abstract class>> Abstract. List <<class>> Array. List underlying data structure: ARRAY <<abstract class>> Abstract. Sequential. Lis t <<class>> Linked. List underlying data structure: LINKED NODES
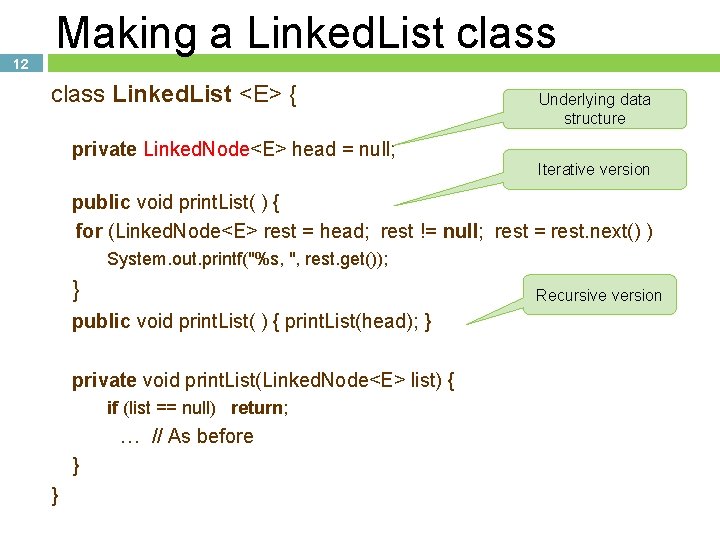
12 Making a Linked. List class Linked. List <E> { Underlying data structure private Linked. Node<E> head = null; Iterative version public void print. List( ) { for (Linked. Node<E> rest = head; rest != null; rest = rest. next() ) System. out. printf("%s, ", rest. get()); } Recursive version public void print. List( ) { print. List(head); } private void print. List(Linked. Node<E> list) { if (list == null) return; … // As before } }
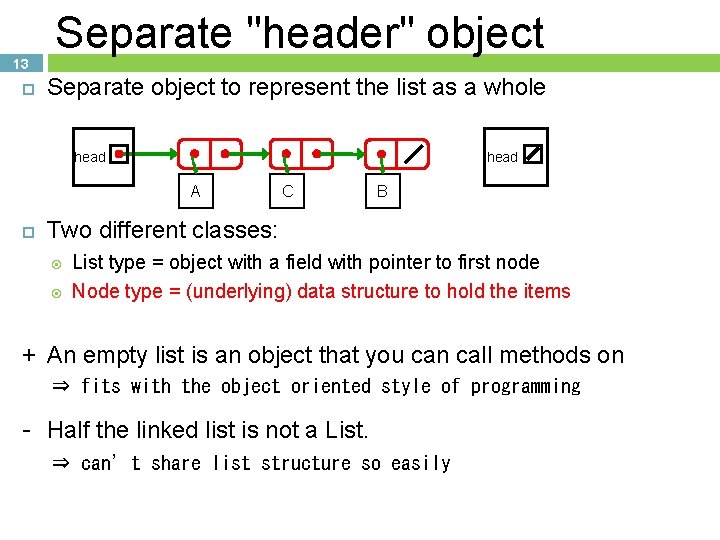
13 Separate "header" object Separate object to represent the list as a whole head A C B Two different classes: List type = object with a field with pointer to first node Node type = (underlying) data structure to hold the items + An empty list is an object that you can call methods on ⇒ fits with the object oriented style of programming - Half the linked list is not a List. ⇒ can’t share list structure so easily
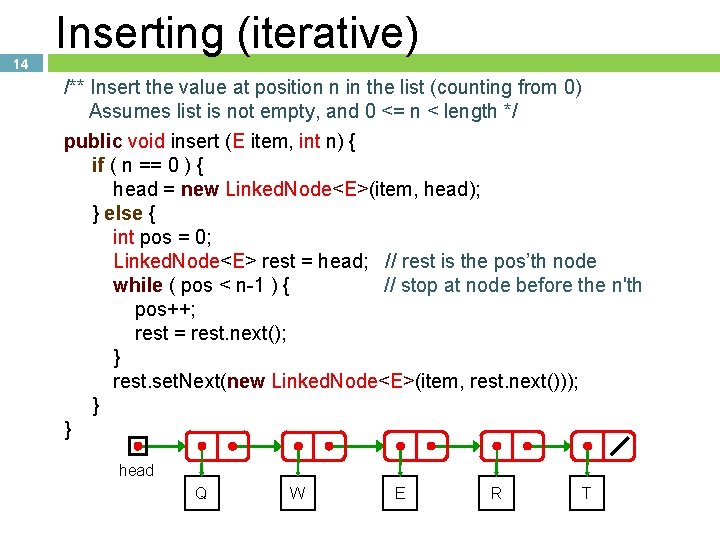
14 Inserting (iterative) /** Insert the value at position n in the list (counting from 0) Assumes list is not empty, and 0 <= n < length */ public void insert (E item, int n) { if ( n == 0 ) { head = new Linked. Node<E>(item, head); } else { int pos = 0; Linked. Node<E> rest = head; // rest is the pos’th node while ( pos < n-1 ) { // stop at node before the n'th pos++; rest = rest. next(); } rest. set. Next(new Linked. Node<E>(item, rest. next())); } } head Q W E R T
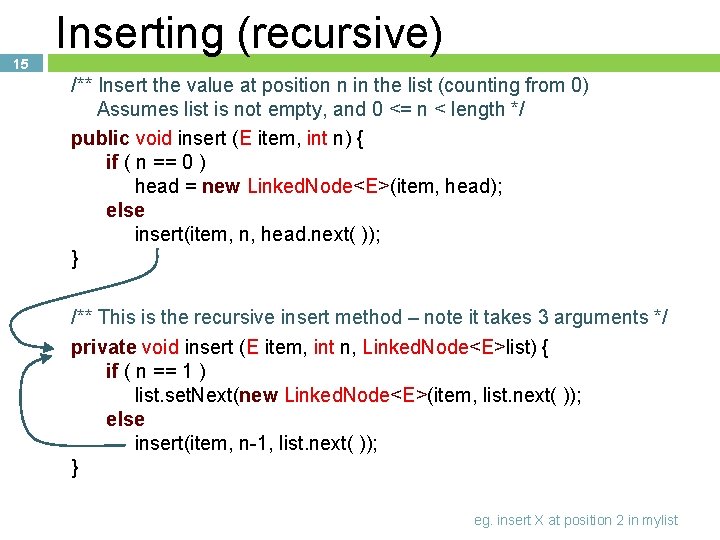
15 Inserting (recursive) /** Insert the value at position n in the list (counting from 0) Assumes list is not empty, and 0 <= n < length */ public void insert (E item, int n) { if ( n == 0 ) head = new Linked. Node<E>(item, head); else insert(item, n, head. next( )); } /** This is the recursive insert method – note it takes 3 arguments */ private void insert (E item, int n, Linked. Node<E>list) { if ( n == 1 ) list. set. Next(new Linked. Node<E>(item, list. next( )); else insert(item, n-1, list. next( )); } eg. insert X at position 2 in mylist
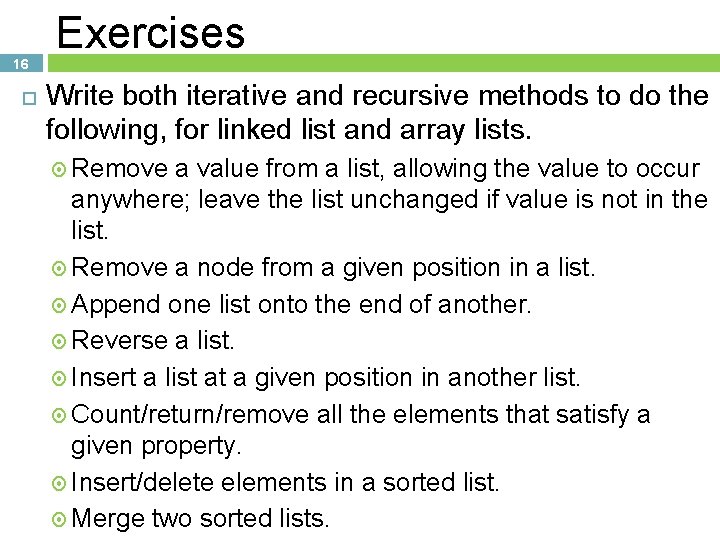
16 Exercises Write both iterative and recursive methods to do the following, for linked list and array lists. Remove a value from a list, allowing the value to occur anywhere; leave the list unchanged if value is not in the list. Remove a node from a given position in a list. Append one list onto the end of another. Reverse a list. Insert a list at a given position in another list. Count/return/remove all the elements that satisfy a given property. Insert/delete elements in a sorted list. Merge two sorted lists.