Collections 4132010 Categorizing collections Static Size Dynamic Size
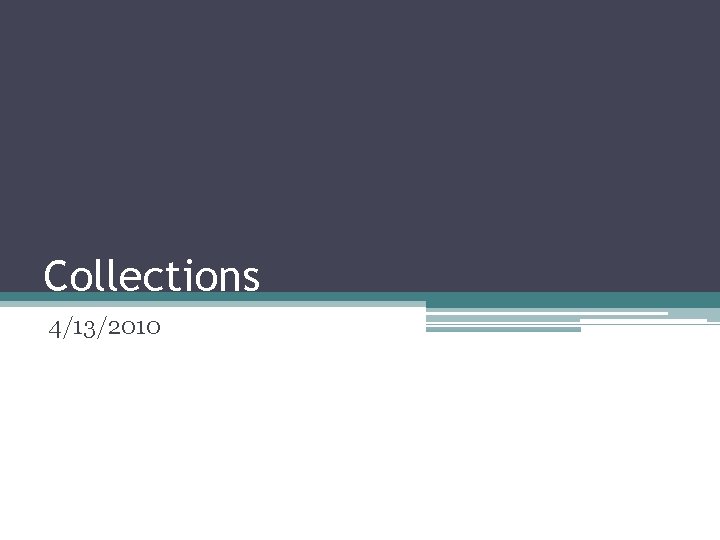
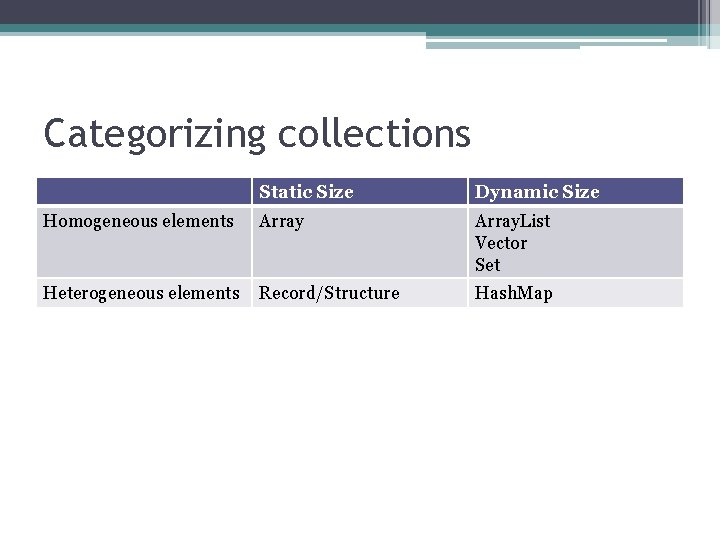
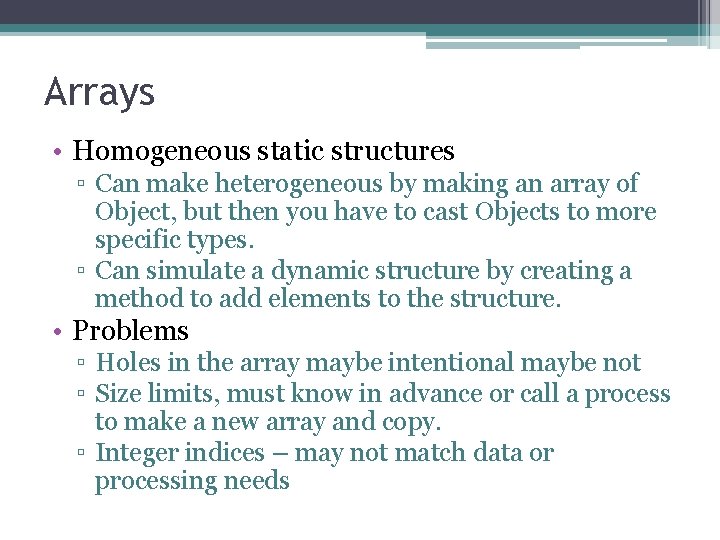
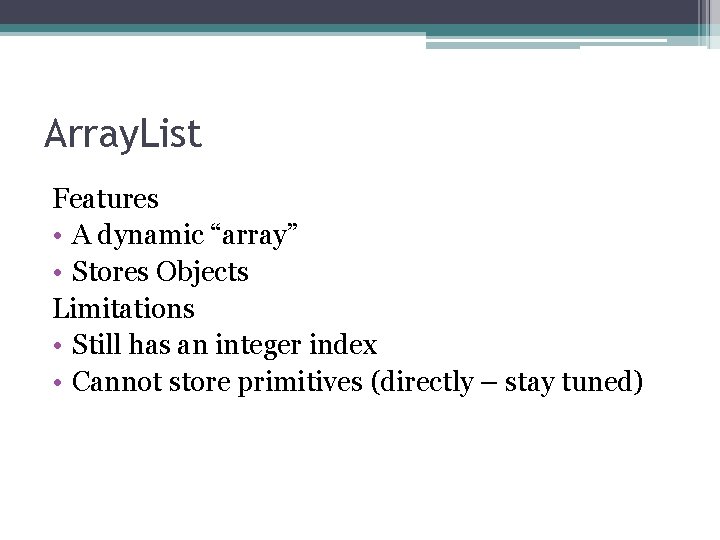
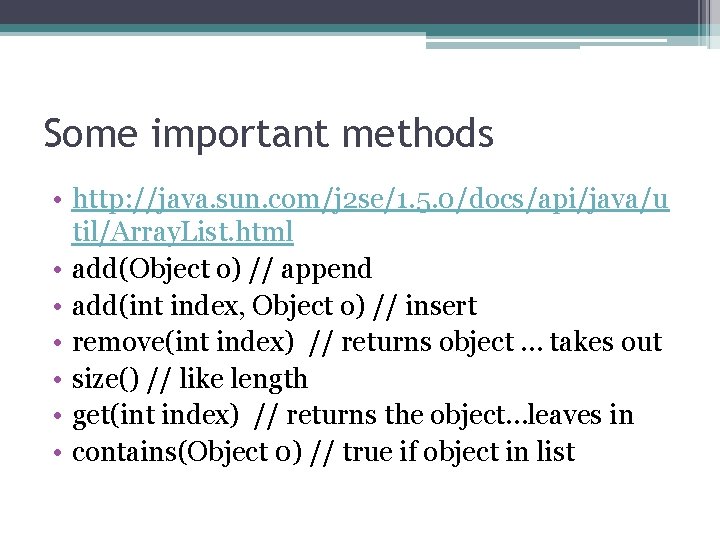
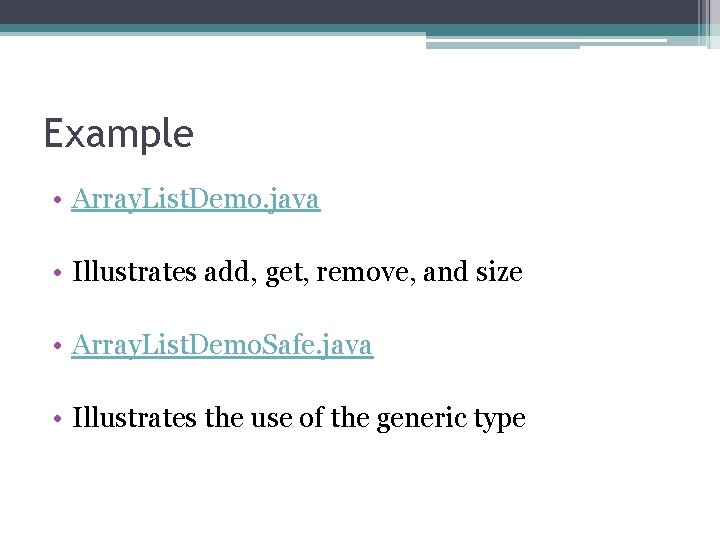
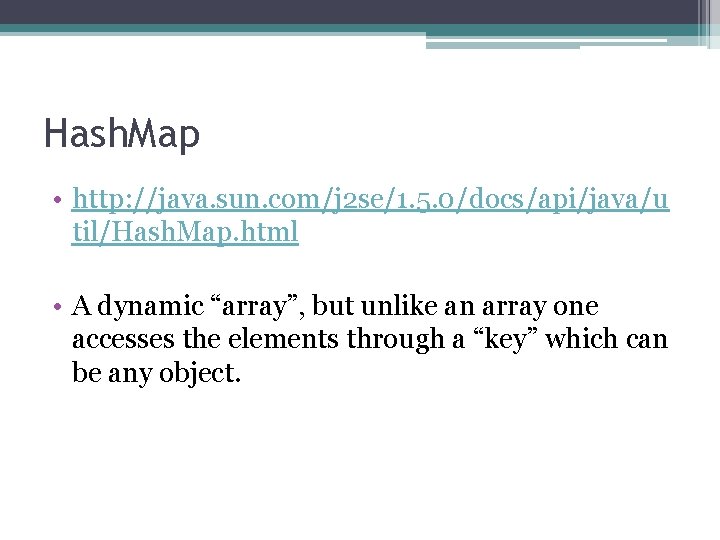
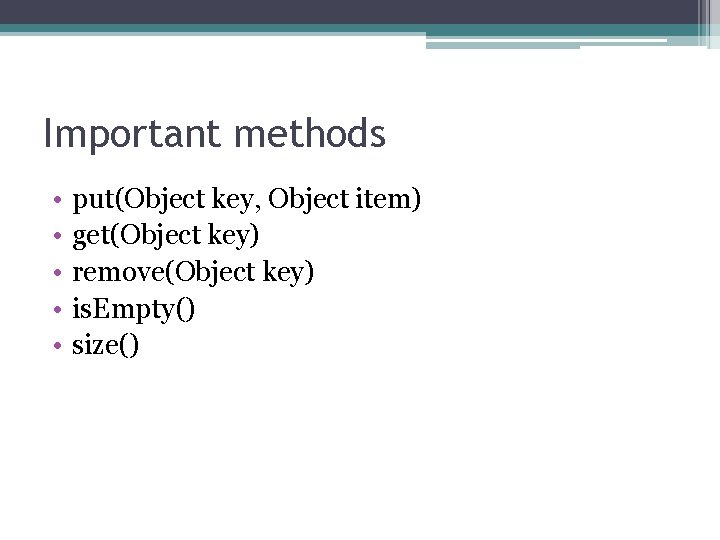
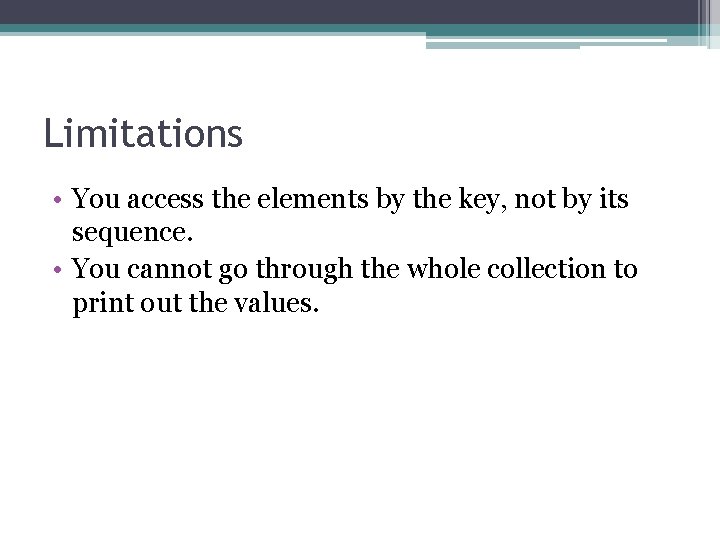
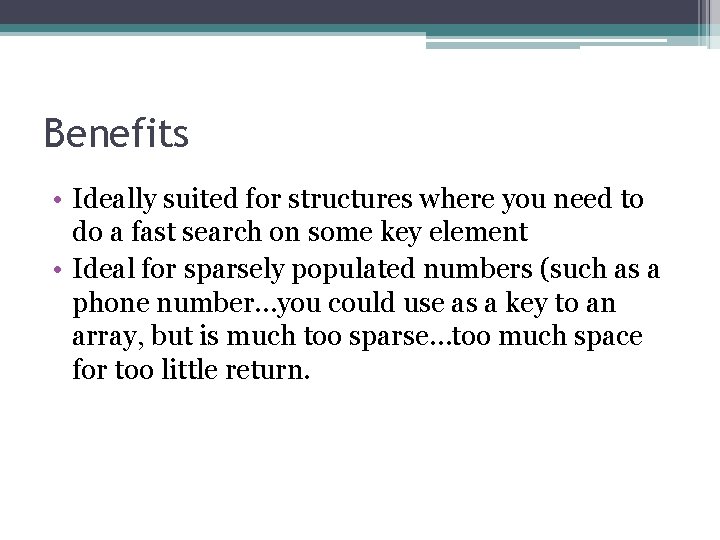
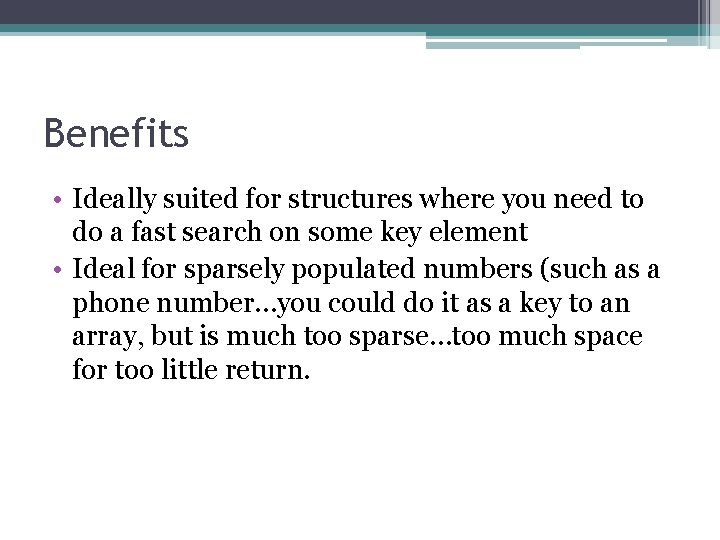
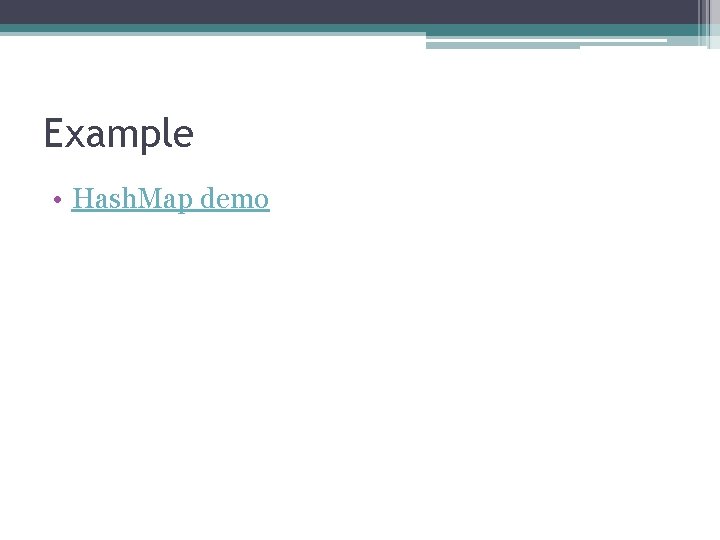
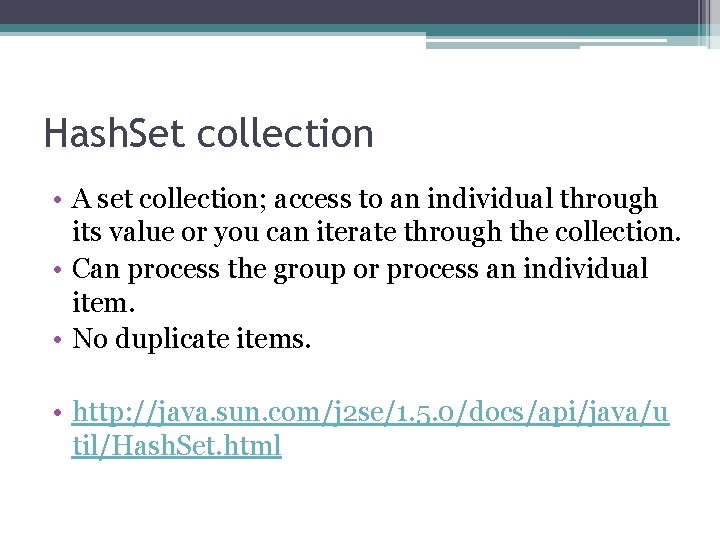
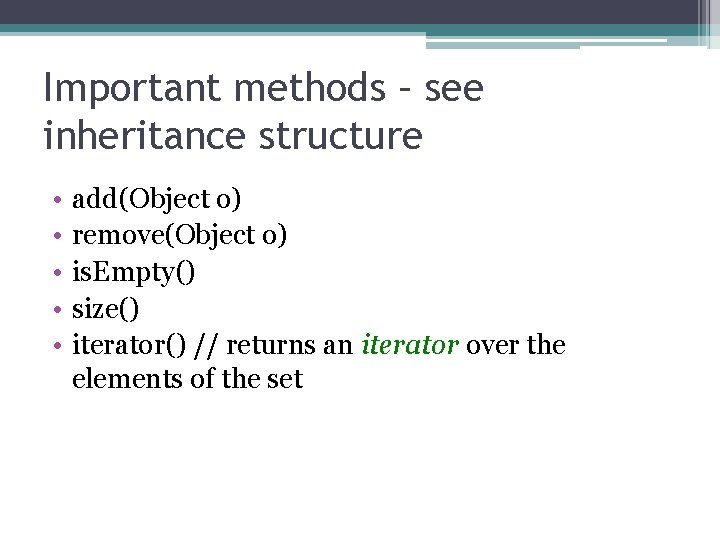
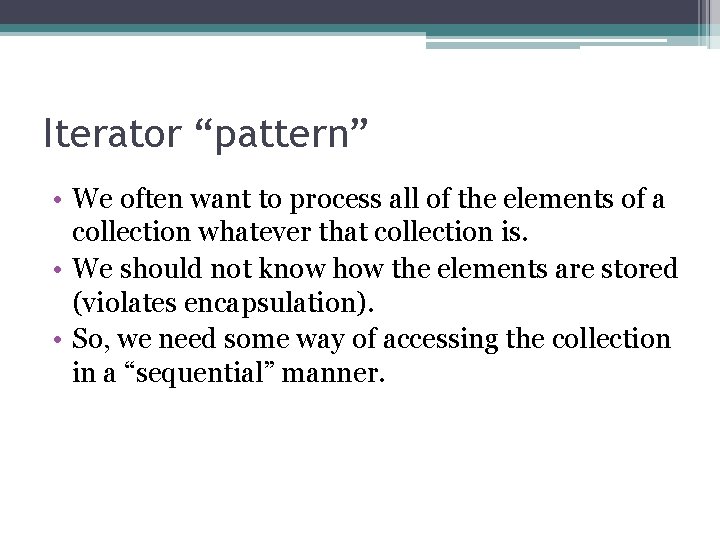
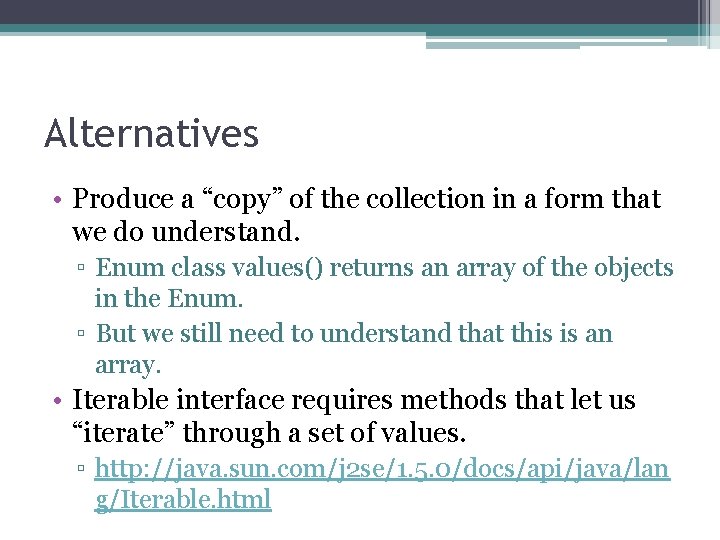
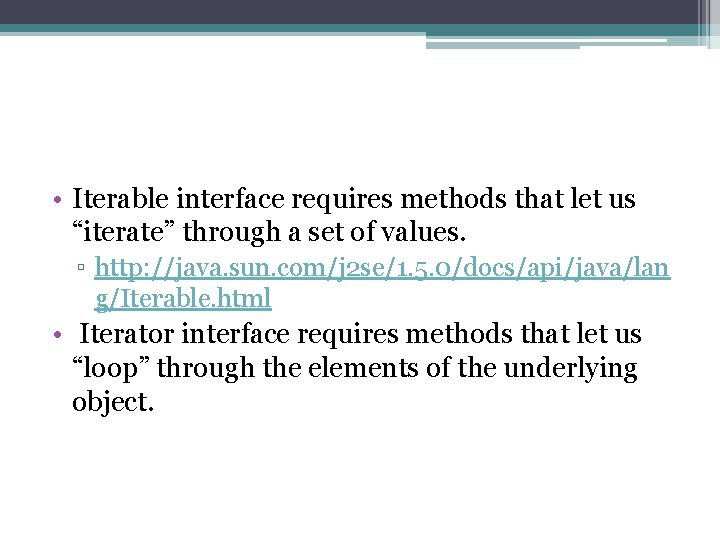
- Slides: 17
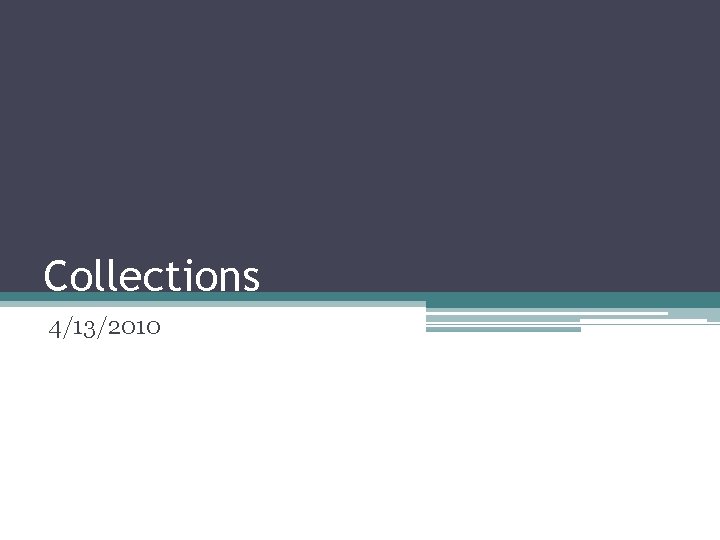
Collections 4/13/2010
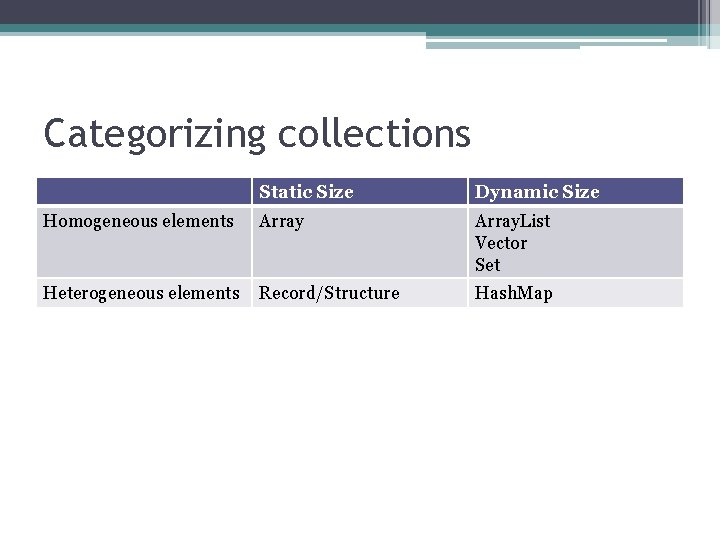
Categorizing collections Static Size Dynamic Size Homogeneous elements Array. List Vector Set Heterogeneous elements Record/Structure Hash. Map
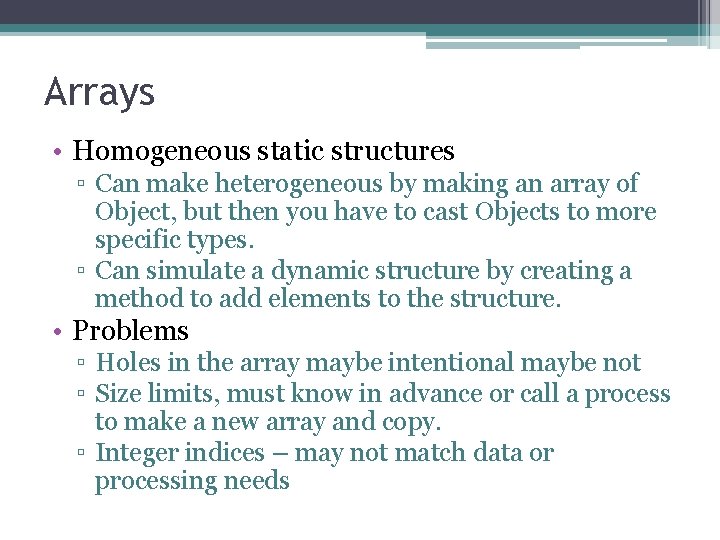
Arrays • Homogeneous static structures ▫ Can make heterogeneous by making an array of Object, but then you have to cast Objects to more specific types. ▫ Can simulate a dynamic structure by creating a method to add elements to the structure. • Problems ▫ Holes in the array maybe intentional maybe not ▫ Size limits, must know in advance or call a process to make a new array and copy. ▫ Integer indices – may not match data or processing needs
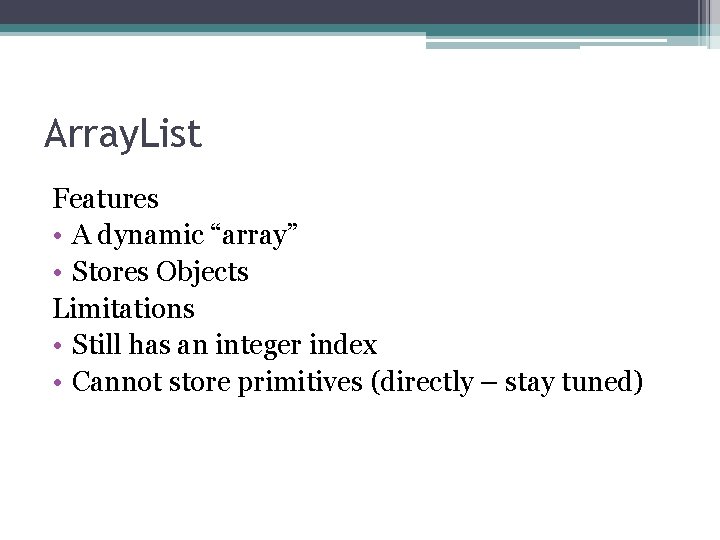
Array. List Features • A dynamic “array” • Stores Objects Limitations • Still has an integer index • Cannot store primitives (directly – stay tuned)
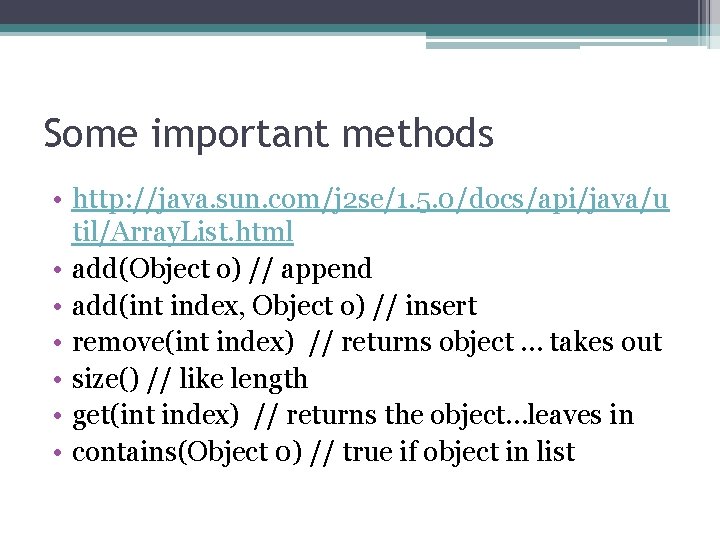
Some important methods • http: //java. sun. com/j 2 se/1. 5. 0/docs/api/java/u til/Array. List. html • add(Object o) // append • add(int index, Object o) // insert • remove(int index) // returns object … takes out • size() // like length • get(int index) // returns the object…leaves in • contains(Object 0) // true if object in list
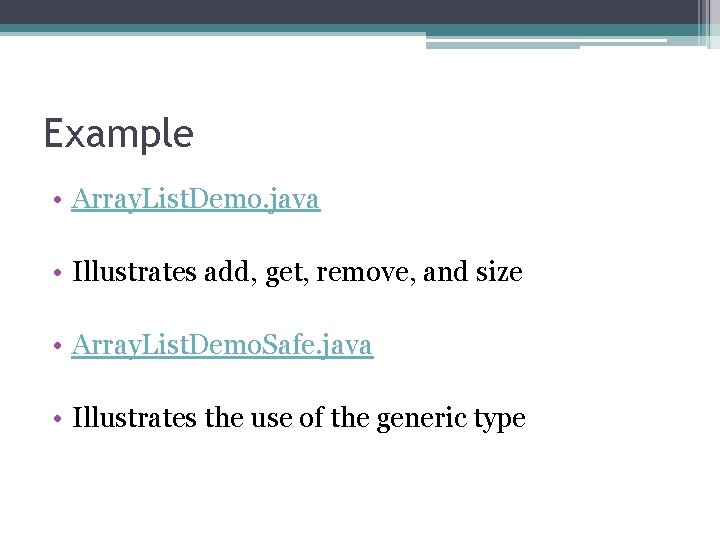
Example • Array. List. Demo. java • Illustrates add, get, remove, and size • Array. List. Demo. Safe. java • Illustrates the use of the generic type
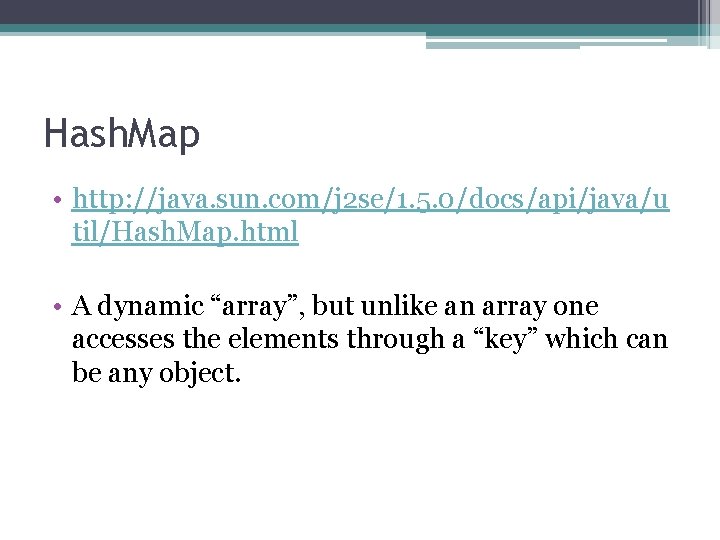
Hash. Map • http: //java. sun. com/j 2 se/1. 5. 0/docs/api/java/u til/Hash. Map. html • A dynamic “array”, but unlike an array one accesses the elements through a “key” which can be any object.
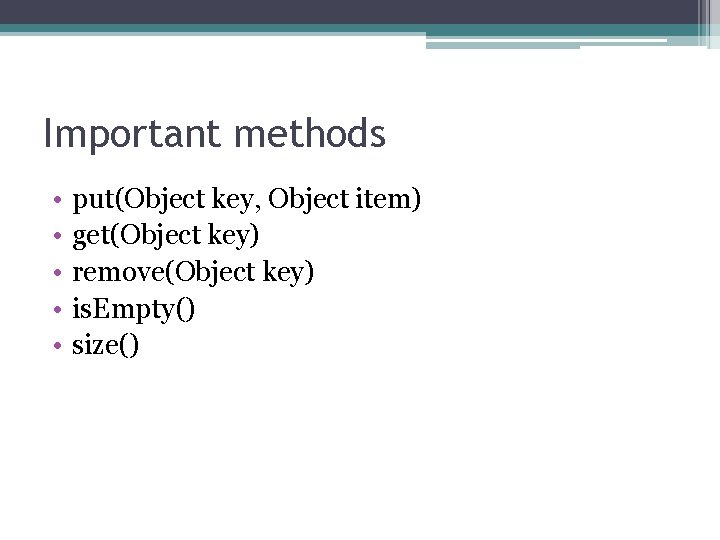
Important methods • • • put(Object key, Object item) get(Object key) remove(Object key) is. Empty() size()
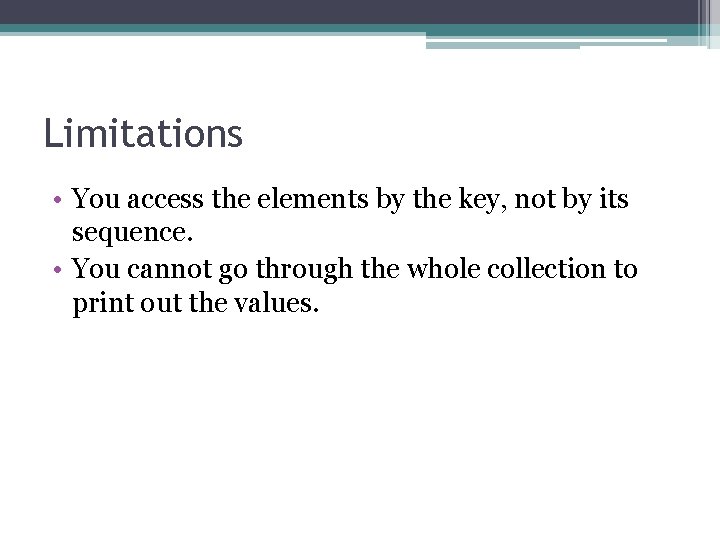
Limitations • You access the elements by the key, not by its sequence. • You cannot go through the whole collection to print out the values.
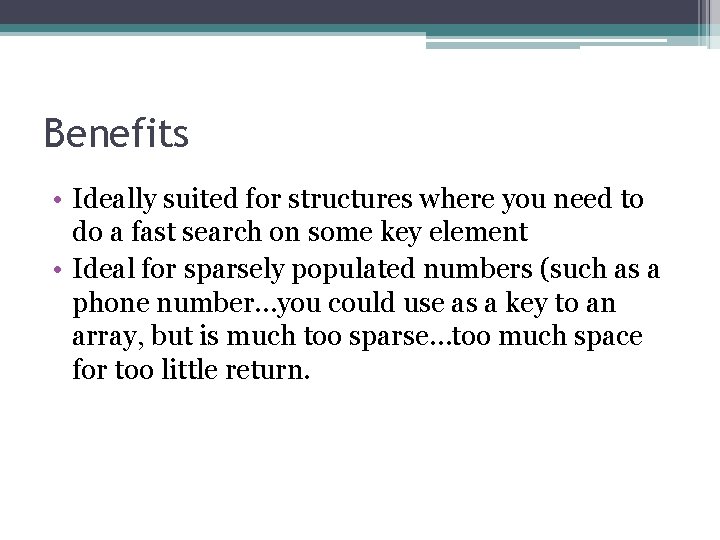
Benefits • Ideally suited for structures where you need to do a fast search on some key element • Ideal for sparsely populated numbers (such as a phone number…you could use as a key to an array, but is much too sparse…too much space for too little return.
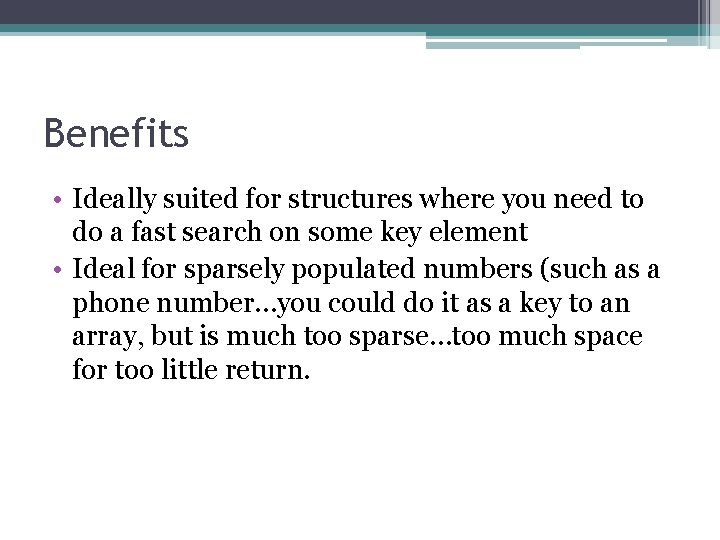
Benefits • Ideally suited for structures where you need to do a fast search on some key element • Ideal for sparsely populated numbers (such as a phone number…you could do it as a key to an array, but is much too sparse…too much space for too little return.
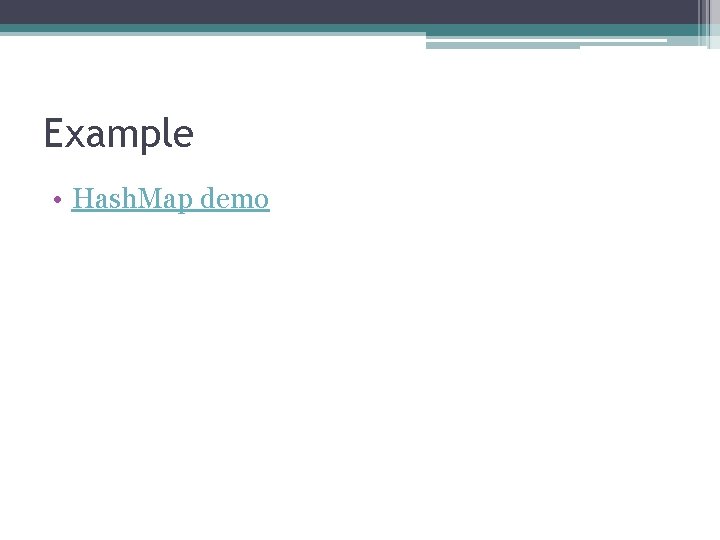
Example • Hash. Map demo
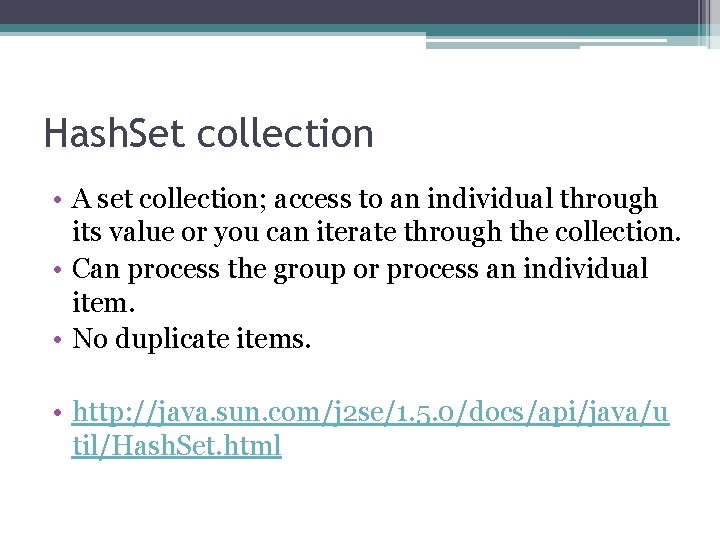
Hash. Set collection • A set collection; access to an individual through its value or you can iterate through the collection. • Can process the group or process an individual item. • No duplicate items. • http: //java. sun. com/j 2 se/1. 5. 0/docs/api/java/u til/Hash. Set. html
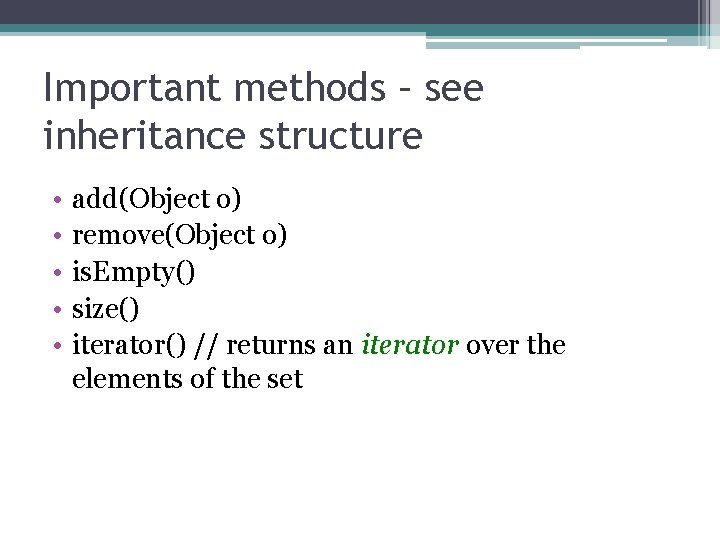
Important methods – see inheritance structure • • • add(Object o) remove(Object o) is. Empty() size() iterator() // returns an iterator over the elements of the set
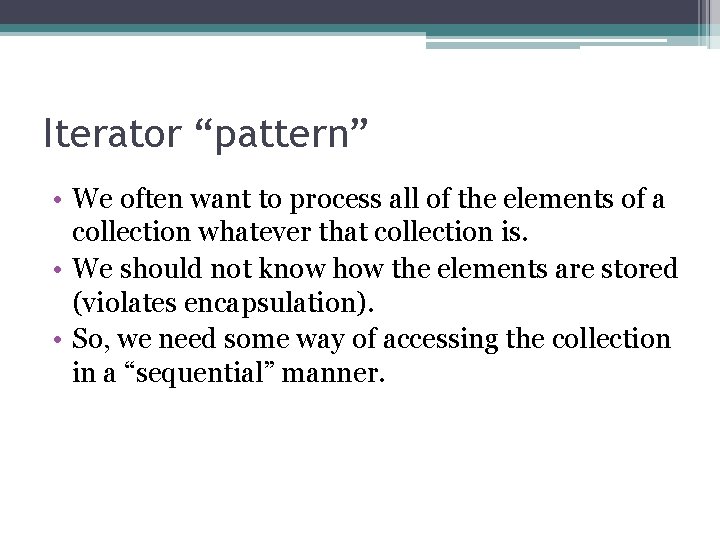
Iterator “pattern” • We often want to process all of the elements of a collection whatever that collection is. • We should not know how the elements are stored (violates encapsulation). • So, we need some way of accessing the collection in a “sequential” manner.
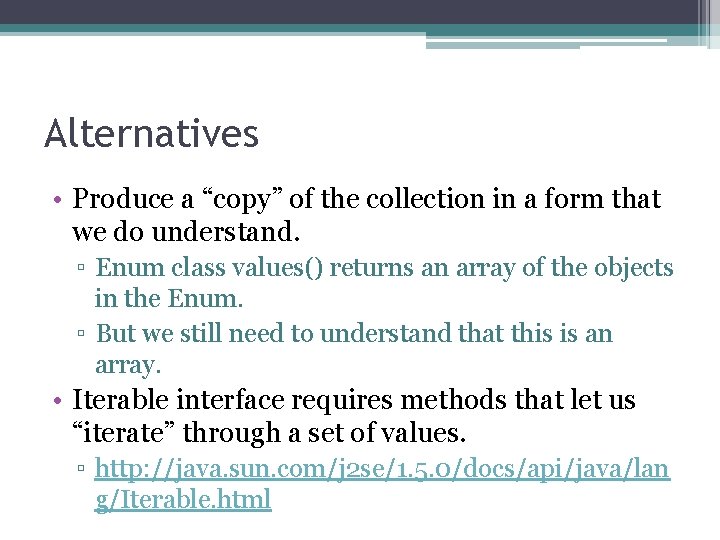
Alternatives • Produce a “copy” of the collection in a form that we do understand. ▫ Enum class values() returns an array of the objects in the Enum. ▫ But we still need to understand that this is an array. • Iterable interface requires methods that let us “iterate” through a set of values. ▫ http: //java. sun. com/j 2 se/1. 5. 0/docs/api/java/lan g/Iterable. html
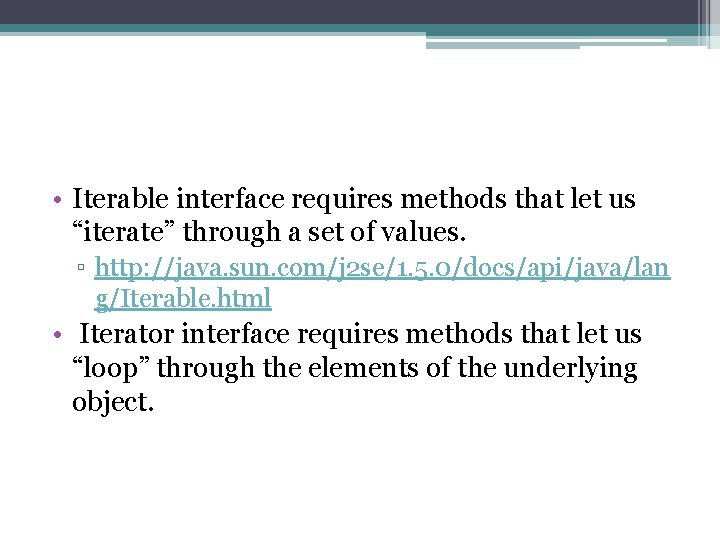
• Iterable interface requires methods that let us “iterate” through a set of values. ▫ http: //java. sun. com/j 2 se/1. 5. 0/docs/api/java/lan g/Iterable. html • Iterator interface requires methods that let us “loop” through the elements of the underlying object.
Are all the methods in the collections class static?
Objective-based categorizing projects is possible
Unit 2 materials technology
Categorizing equations
Categorizing different types of memory
Land biomes lesson outline answers
Introduction to ram
Static and dynamic binding
Static vs dynamic class loading
Monkeys paw irony
Static vs dynamic linking
Static and dynamic queue in data structure
Longitudinal stability of ship
Uml diagram
What is linking and loading
Symbols in things fall apart
Compiler type checking
Main character in great gatsby