COEN 352 SUMMER 2019 1 Tutorial Session 10
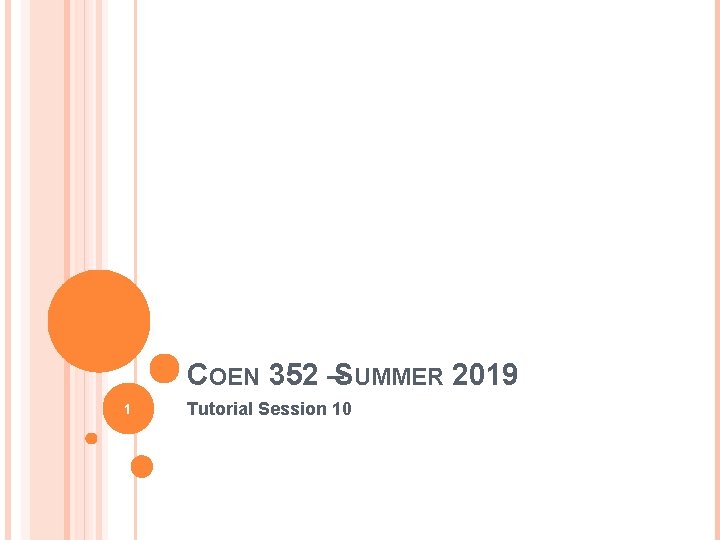
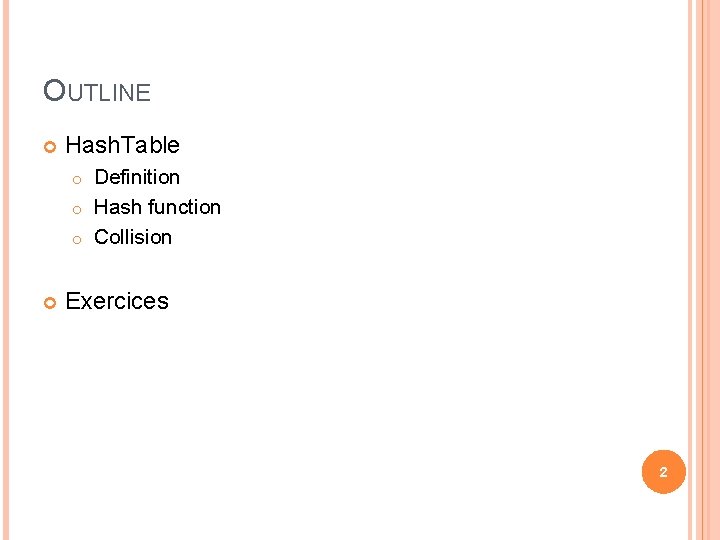
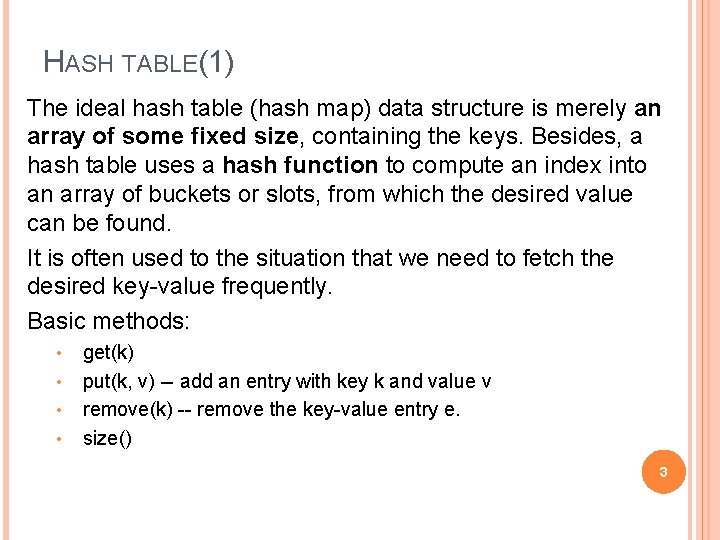
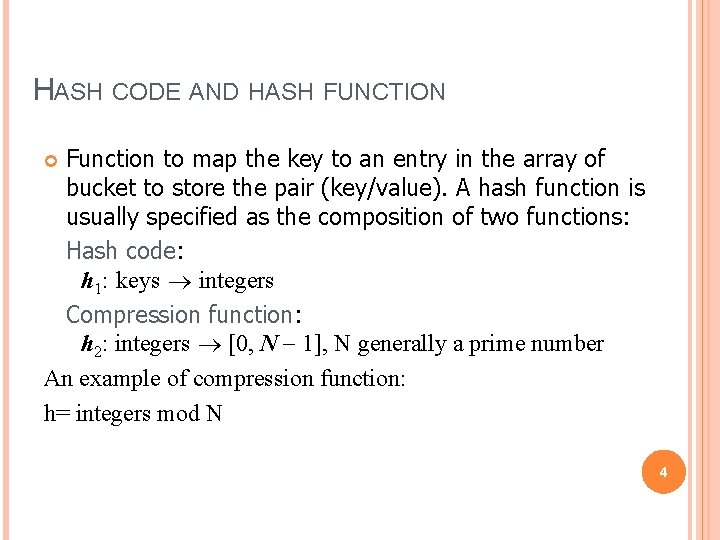
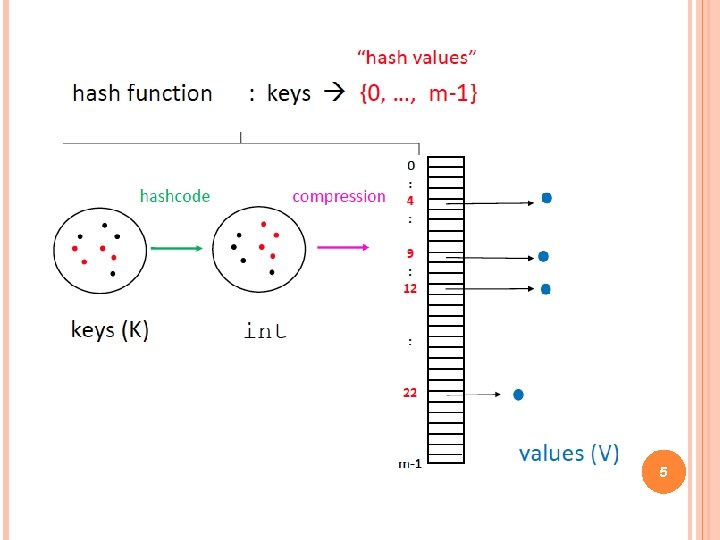
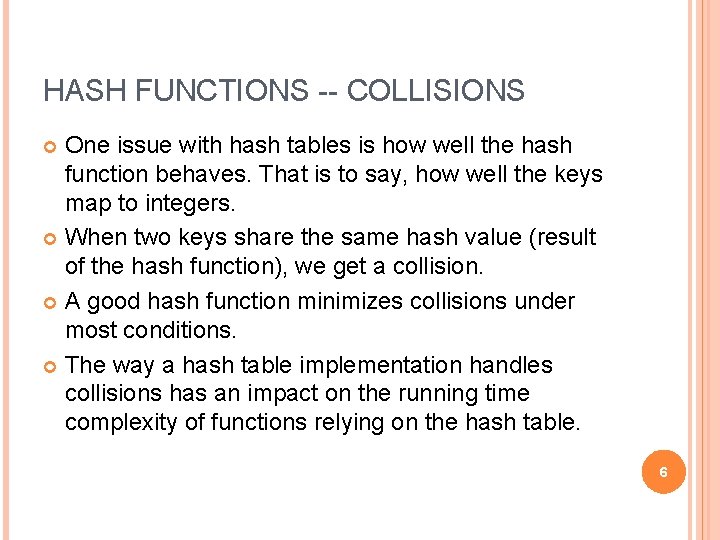
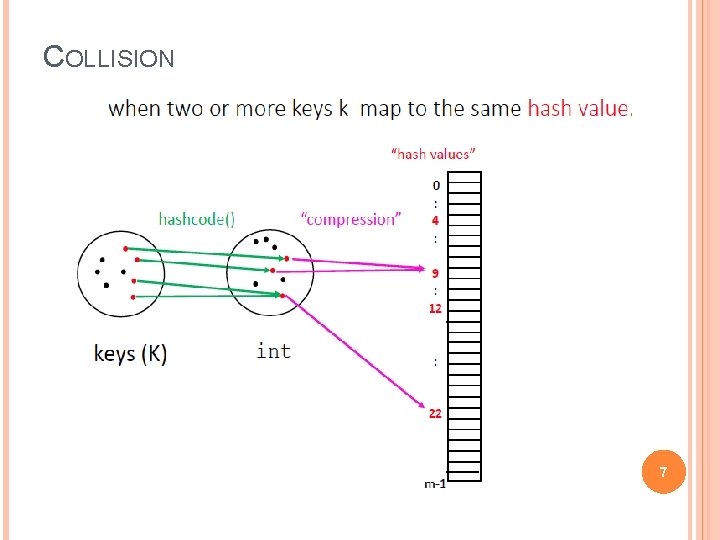
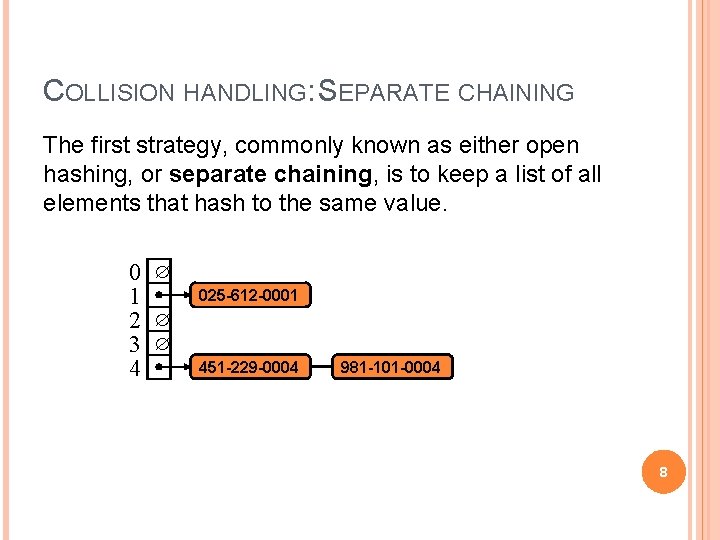
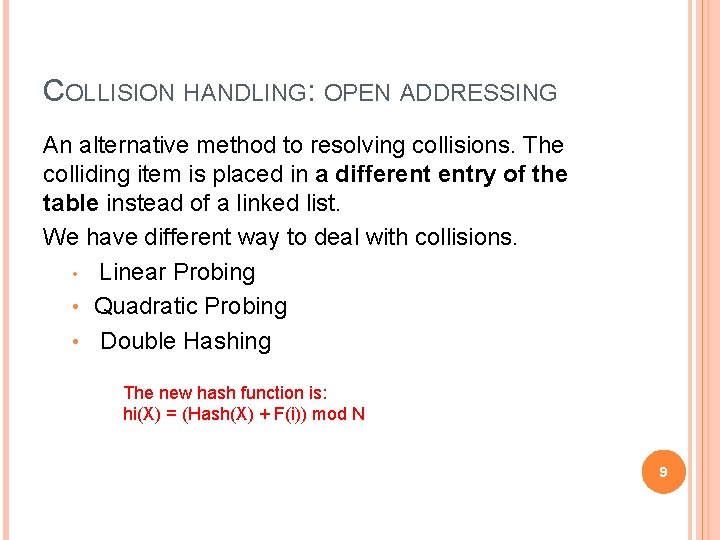
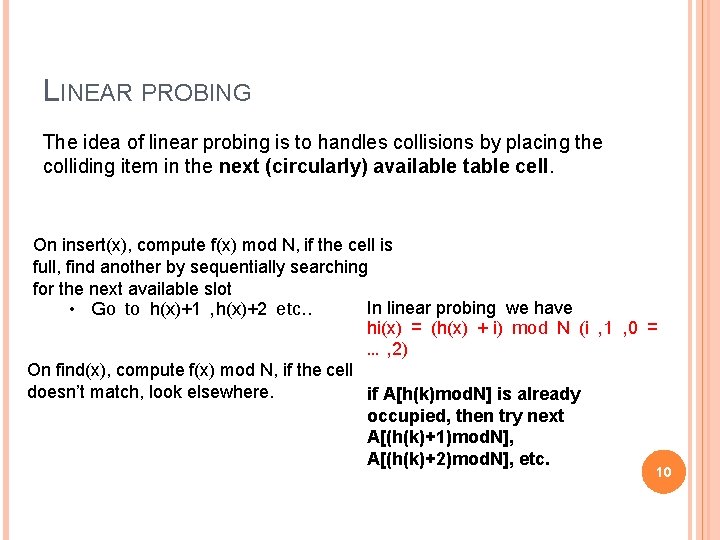
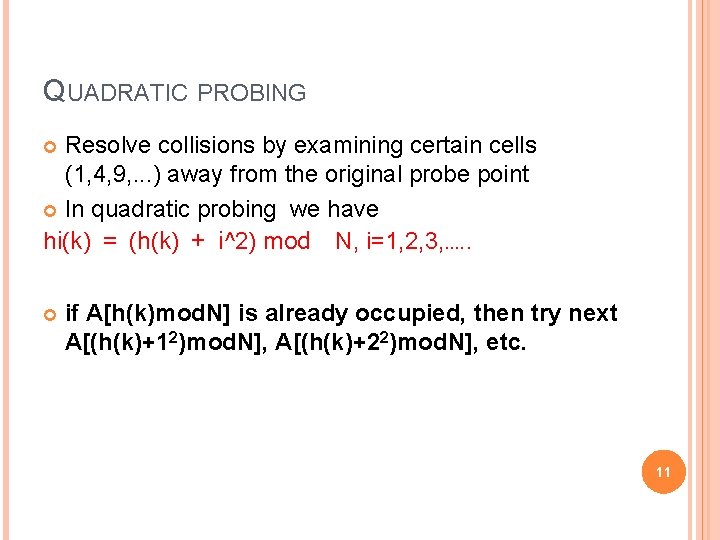
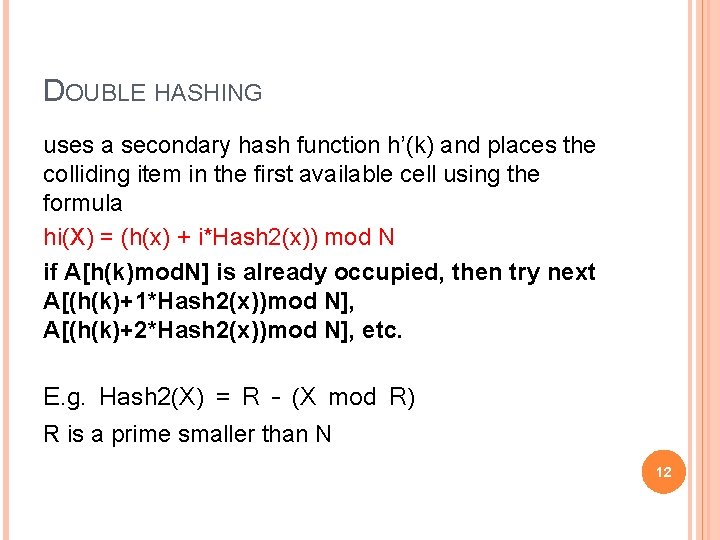
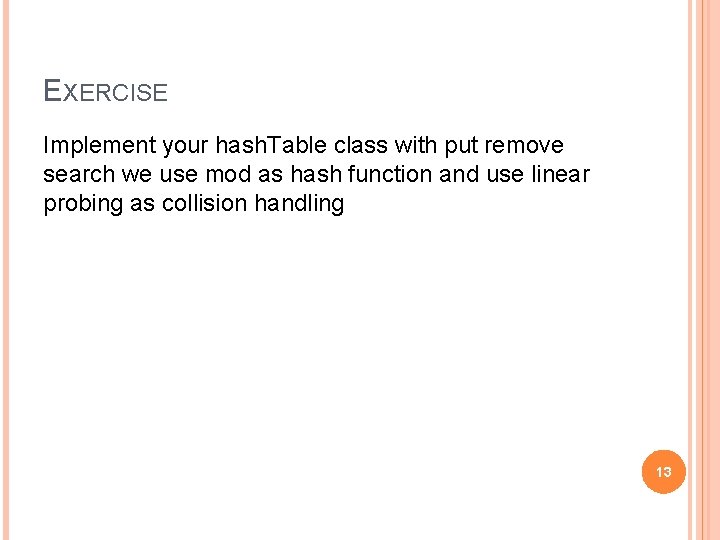
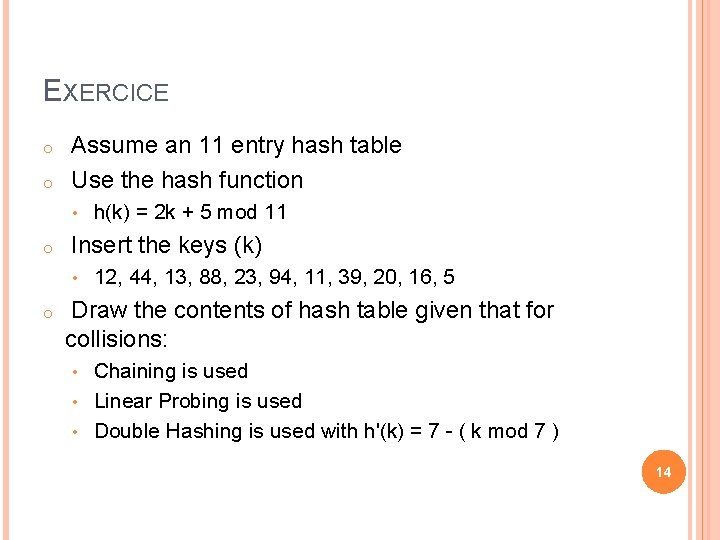
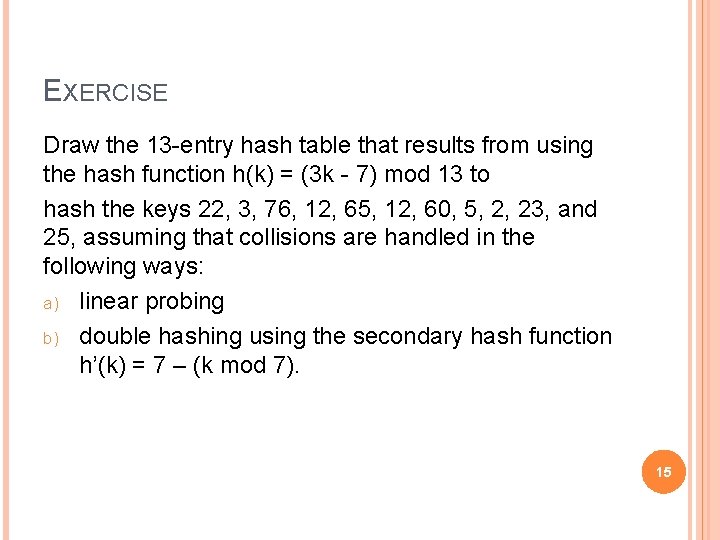
- Slides: 15
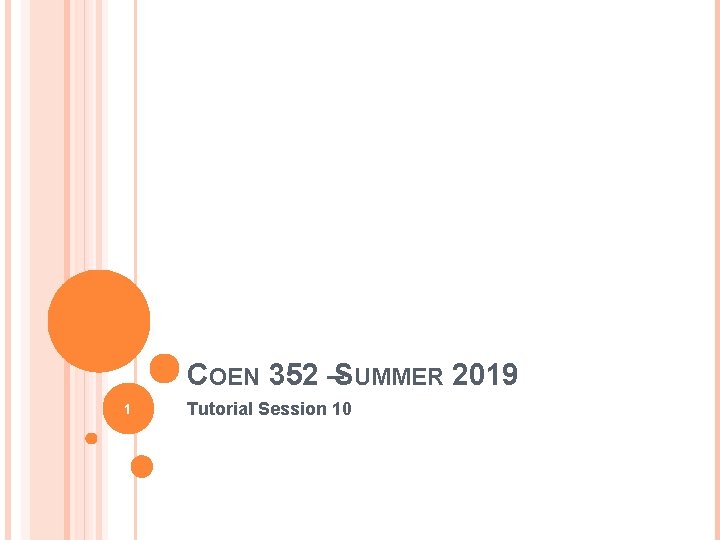
COEN 352 –SUMMER 2019 1 Tutorial Session 10
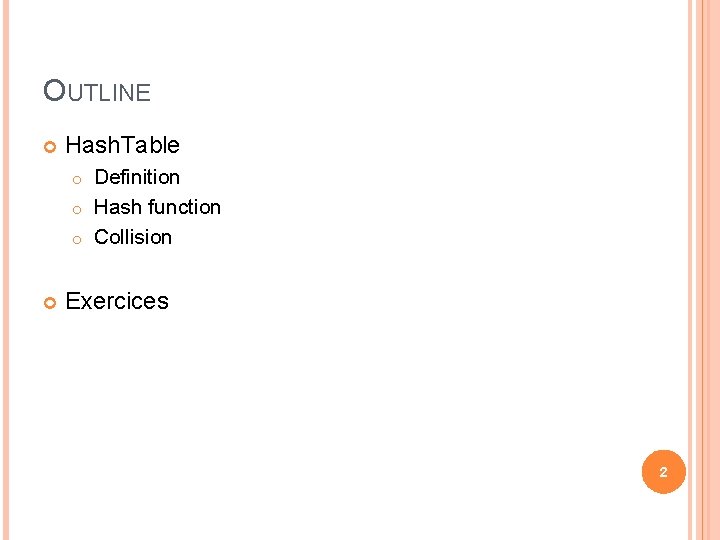
OUTLINE Hash. Table Definition o Hash function o Collision o Exercices 2
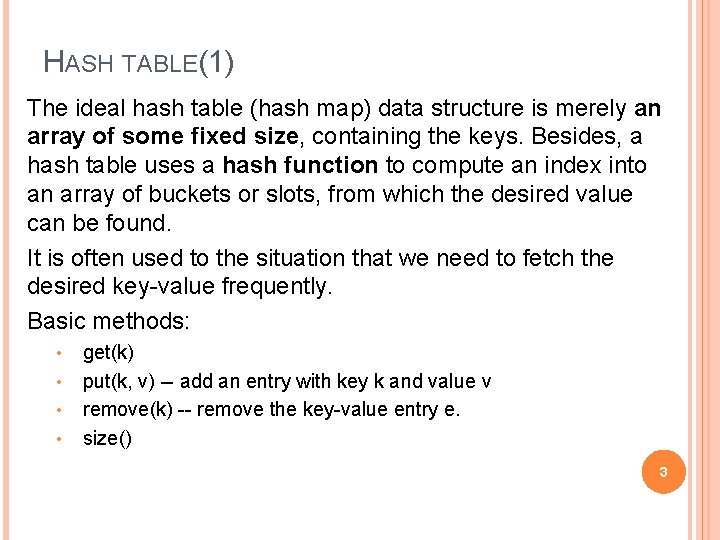
HASH TABLE(1) The ideal hash table (hash map) data structure is merely an array of some fixed size, containing the keys. Besides, a hash table uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found. It is often used to the situation that we need to fetch the desired key-value frequently. Basic methods: • • get(k) put(k, v) -- add an entry with key k and value v remove(k) -- remove the key-value entry e. size() 3
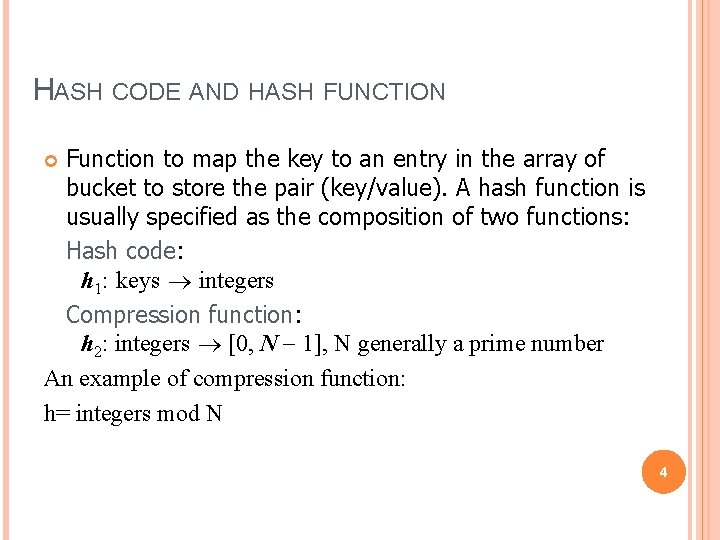
HASH CODE AND HASH FUNCTION Function to map the key to an entry in the array of bucket to store the pair (key/value). A hash function is usually specified as the composition of two functions: Hash code: h 1: keys integers Compression function: h 2: integers [0, N - 1], N generally a prime number An example of compression function: h= integers mod N 4
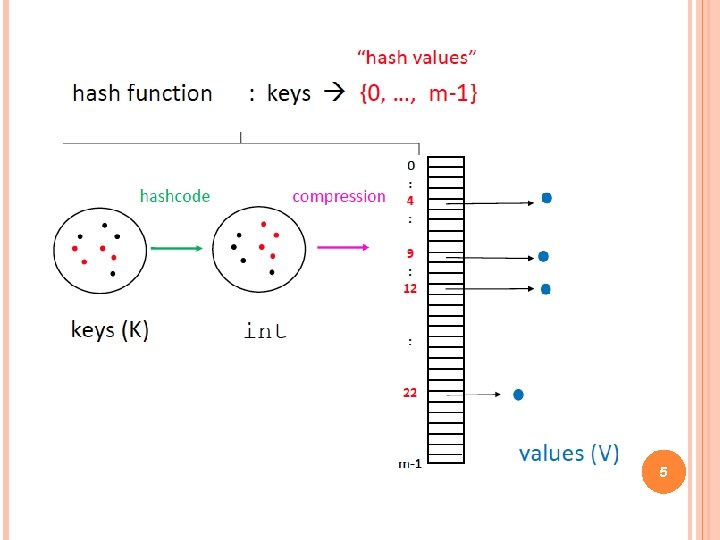
5
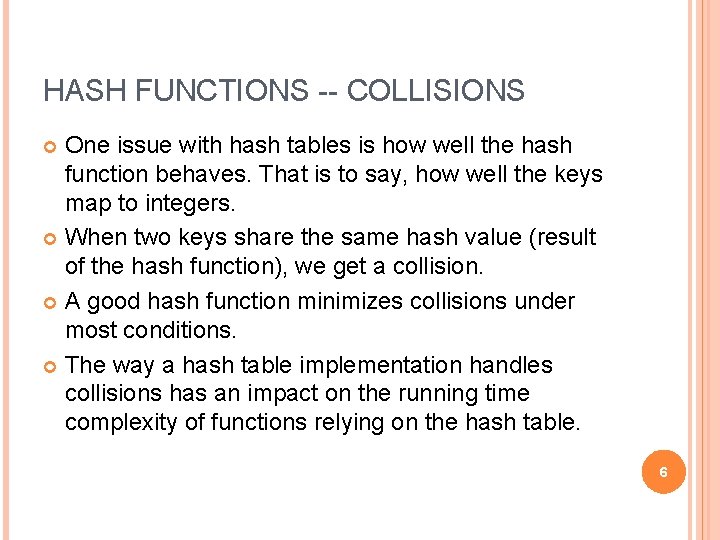
HASH FUNCTIONS -- COLLISIONS One issue with hash tables is how well the hash function behaves. That is to say, how well the keys map to integers. When two keys share the same hash value (result of the hash function), we get a collision. A good hash function minimizes collisions under most conditions. The way a hash table implementation handles collisions has an impact on the running time complexity of functions relying on the hash table. 6
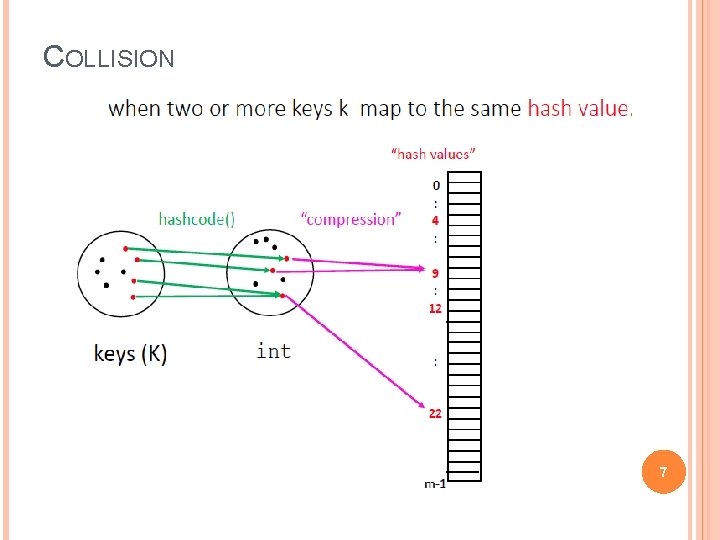
COLLISION 7
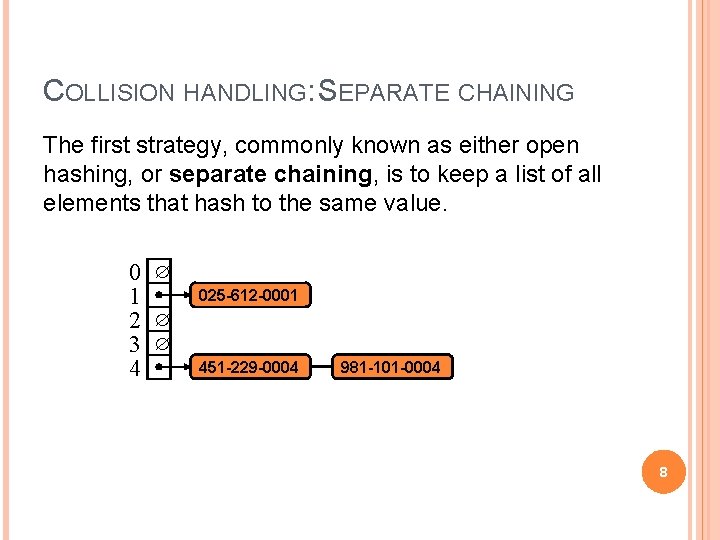
COLLISION HANDLING: SEPARATE CHAINING The first strategy, commonly known as either open hashing, or separate chaining, is to keep a list of all elements that hash to the same value. 0 1 2 3 4 025 -612 -0001 451 -229 -0004 981 -101 -0004 8
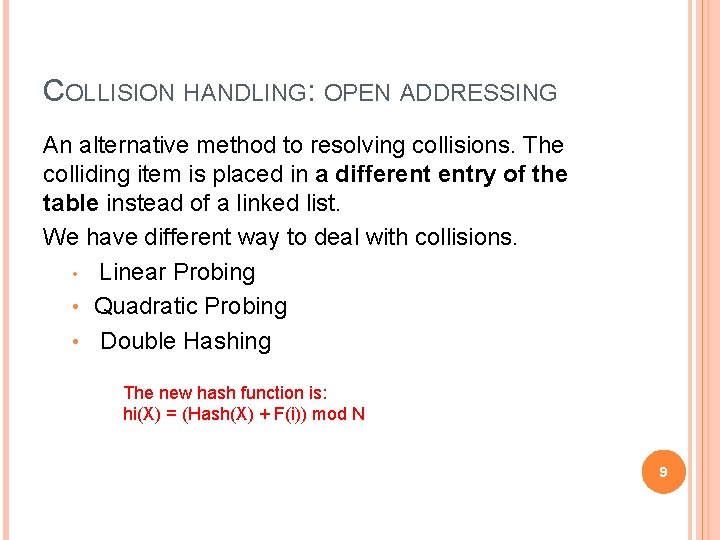
COLLISION HANDLING: OPEN ADDRESSING An alternative method to resolving collisions. The colliding item is placed in a different entry of the table instead of a linked list. We have different way to deal with collisions. • Linear Probing • Quadratic Probing • Double Hashing The new hash function is: hi(X) = (Hash(X) + F(i)) mod N 9
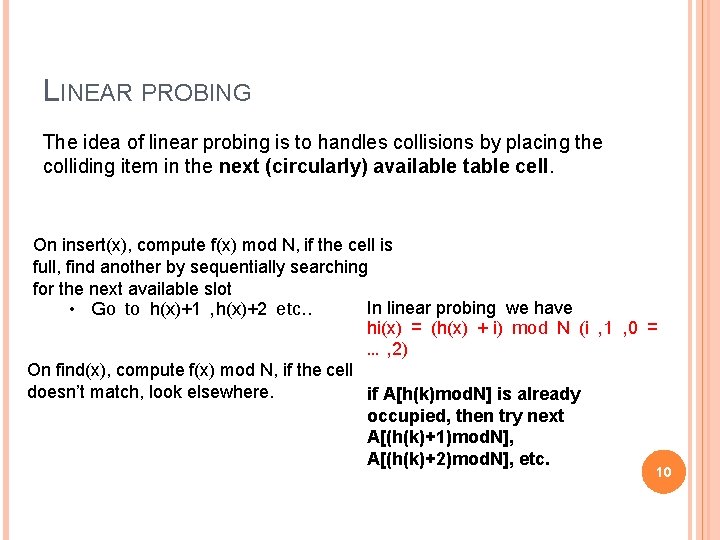
LINEAR PROBING The idea of linear probing is to handles collisions by placing the colliding item in the next (circularly) available table cell. On insert(x), compute f(x) mod N, if the cell is full, find another by sequentially searching for the next available slot In linear probing we have • Go to h(x)+1 , h(x)+2 etc. . hi(x) = (h(x) + i) mod N (i , 1 , 0 = … , 2) On find(x), compute f(x) mod N, if the cell doesn’t match, look elsewhere. if A[h(k)mod. N] is already occupied, then try next A[(h(k)+1)mod. N], A[(h(k)+2)mod. N], etc. 10
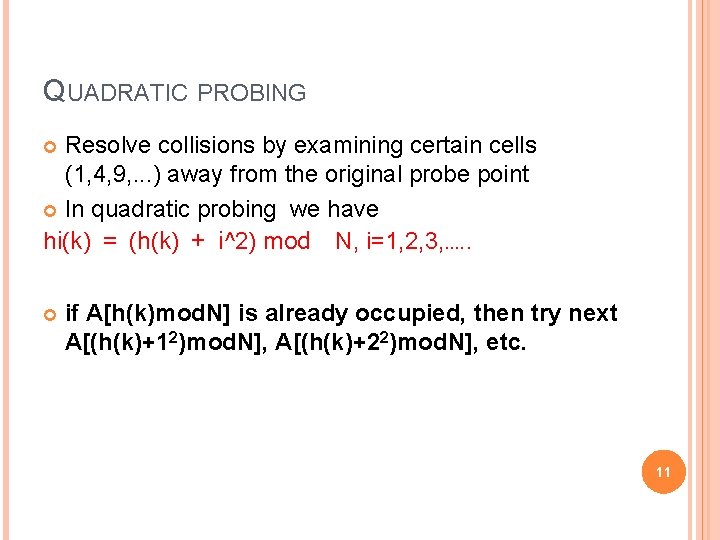
QUADRATIC PROBING Resolve collisions by examining certain cells (1, 4, 9, . . . ) away from the original probe point In quadratic probing we have hi(k) = (h(k) + i^2) mod N, i=1, 2, 3, …. . if A[h(k)mod. N] is already occupied, then try next A[(h(k)+12)mod. N], A[(h(k)+22)mod. N], etc. 11
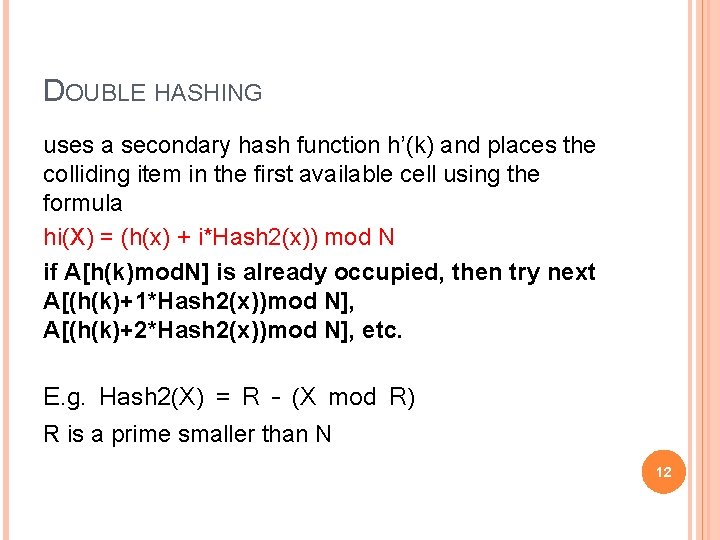
DOUBLE HASHING uses a secondary hash function h’(k) and places the colliding item in the first available cell using the formula hi(X) = (h(x) + i*Hash 2(x)) mod N if A[h(k)mod. N] is already occupied, then try next A[(h(k)+1*Hash 2(x))mod N], A[(h(k)+2*Hash 2(x))mod N], etc. E. g. Hash 2(X) = R – (X mod R) R is a prime smaller than N 12
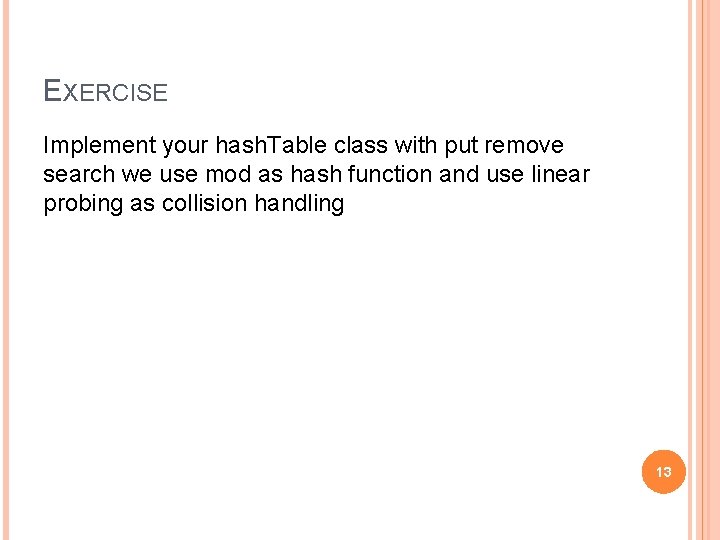
EXERCISE Implement your hash. Table class with put remove search we use mod as hash function and use linear probing as collision handling 13
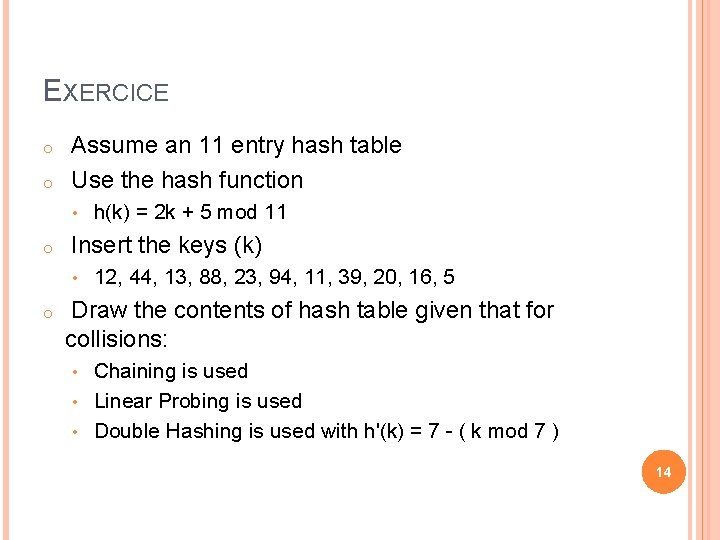
EXERCICE o o Assume an 11 entry hash table Use the hash function • o Insert the keys (k) • o h(k) = 2 k + 5 mod 11 12, 44, 13, 88, 23, 94, 11, 39, 20, 16, 5 Draw the contents of hash table given that for collisions: Chaining is used • Linear Probing is used • Double Hashing is used with h'(k) = 7 - ( k mod 7 ) • 14
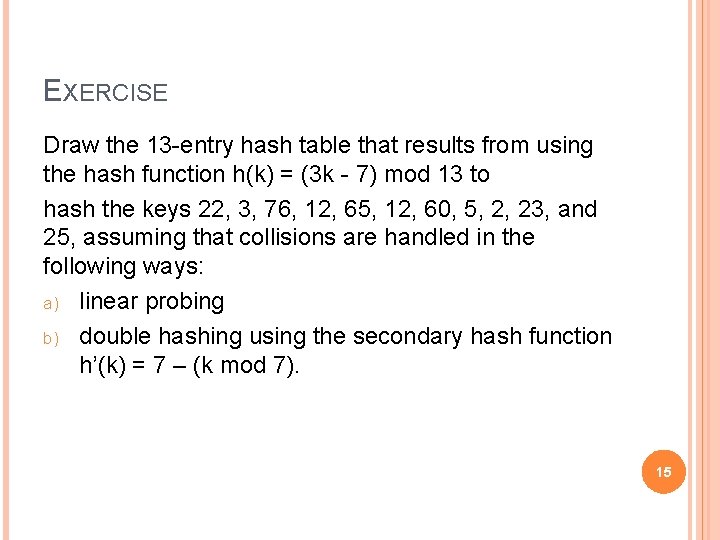
EXERCISE Draw the 13 -entry hash table that results from using the hash function h(k) = (3 k - 7) mod 13 to hash the keys 22, 3, 76, 12, 65, 12, 60, 5, 2, 23, and 25, assuming that collisions are handled in the following ways: a) linear probing b) double hashing using the secondary hash function h’(k) = 7 – (k mod 7). 15