CO 1401 Program Design and Implementation Week 7
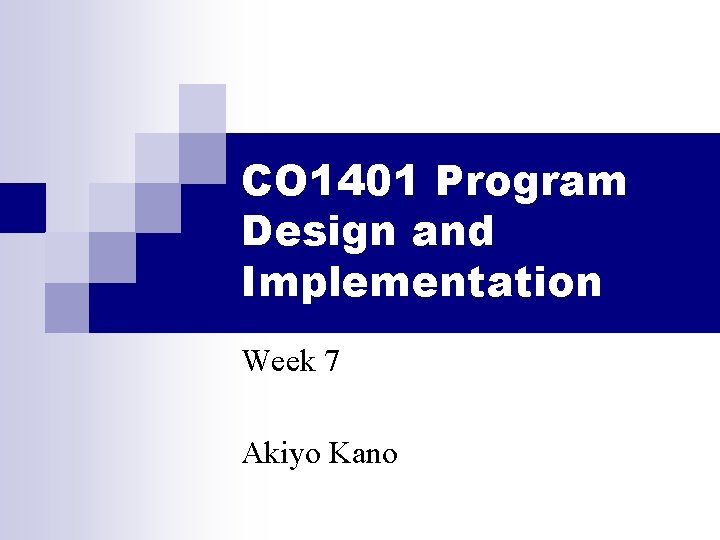
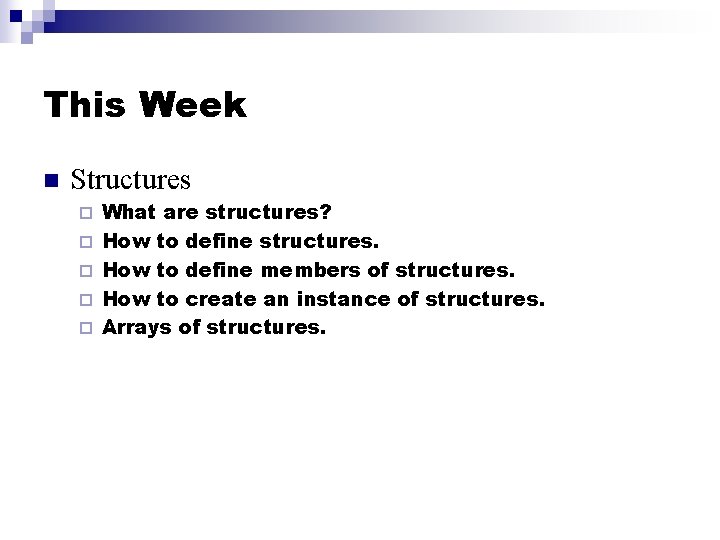
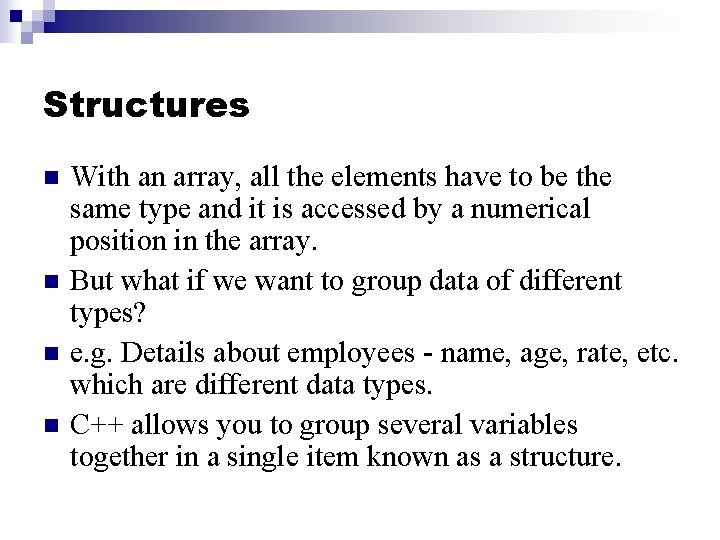
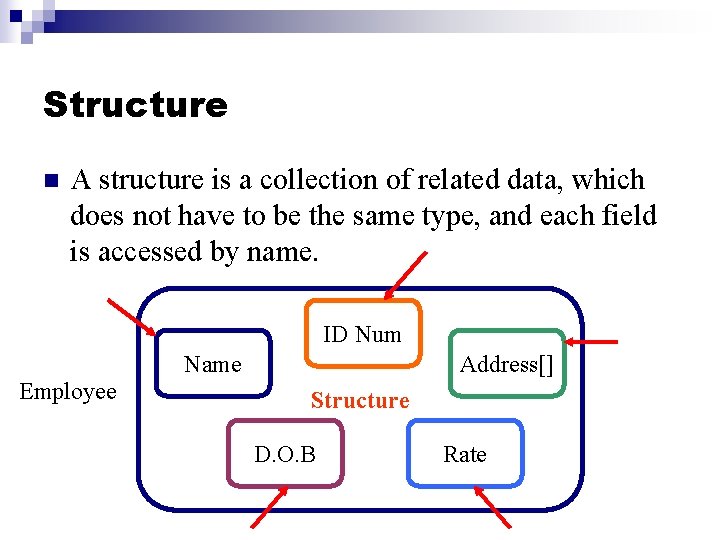
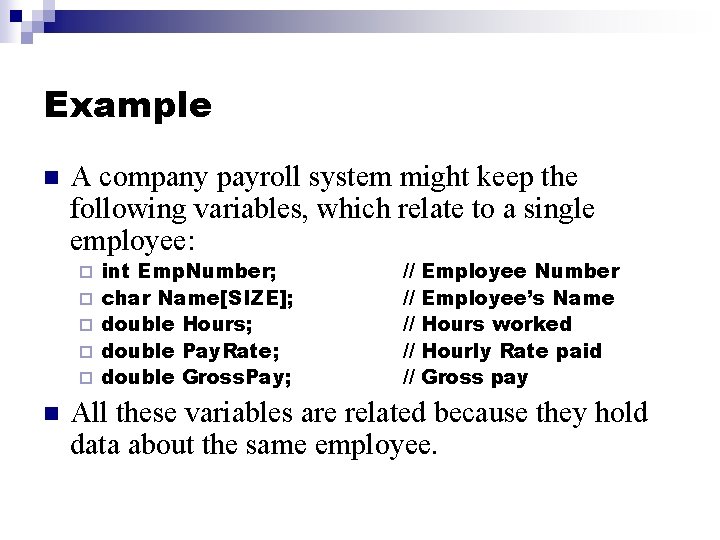
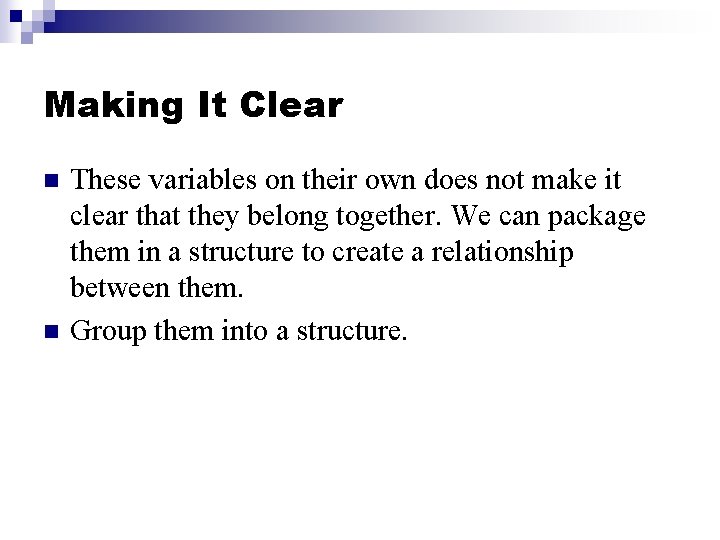
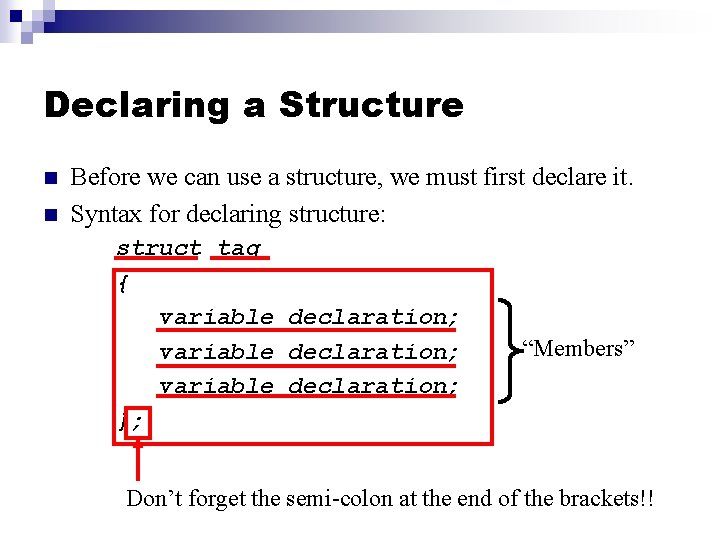
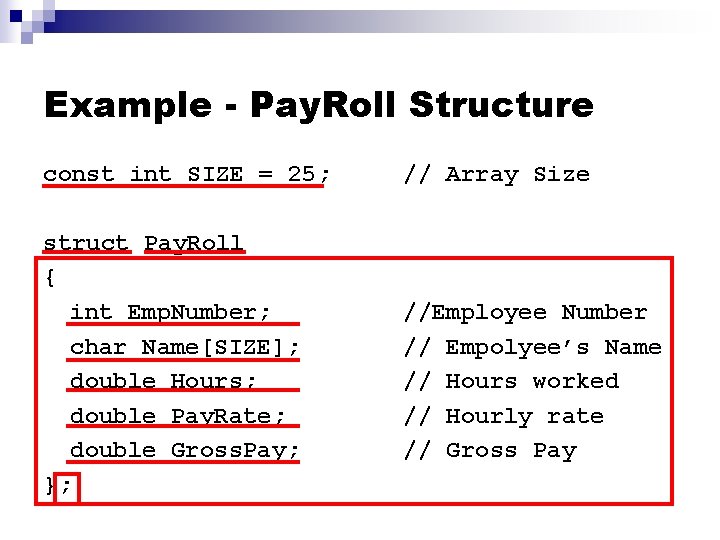
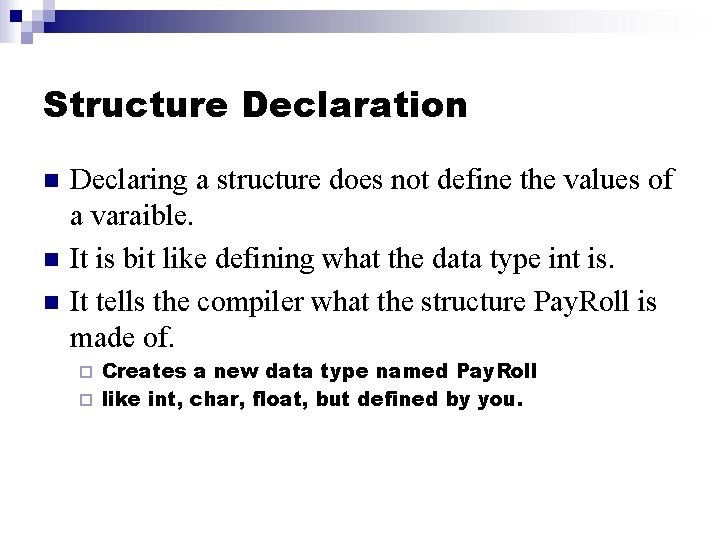
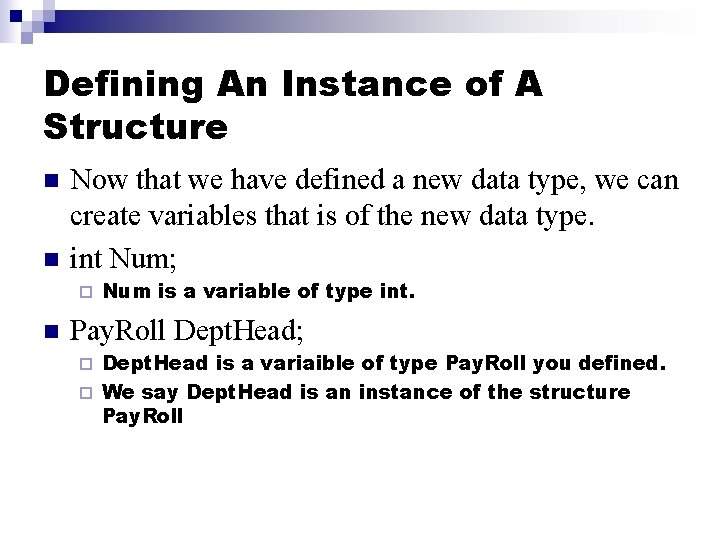
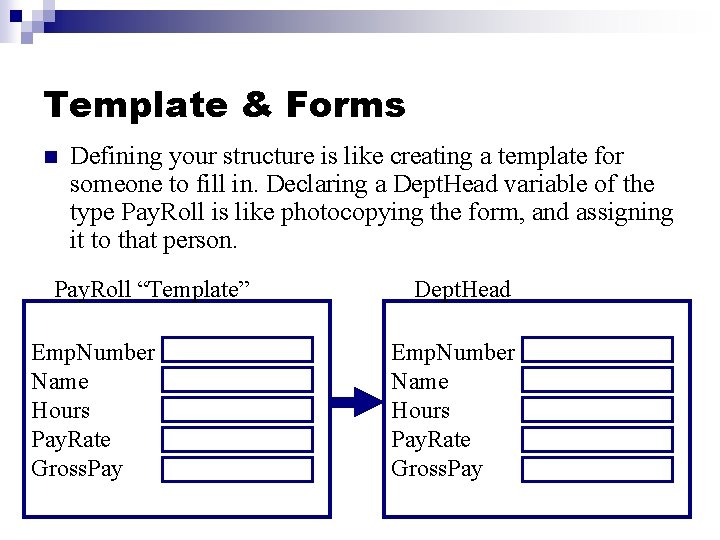
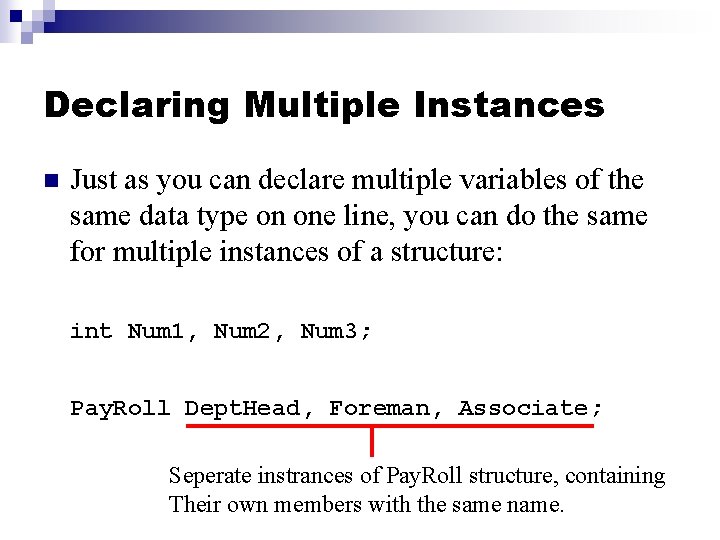
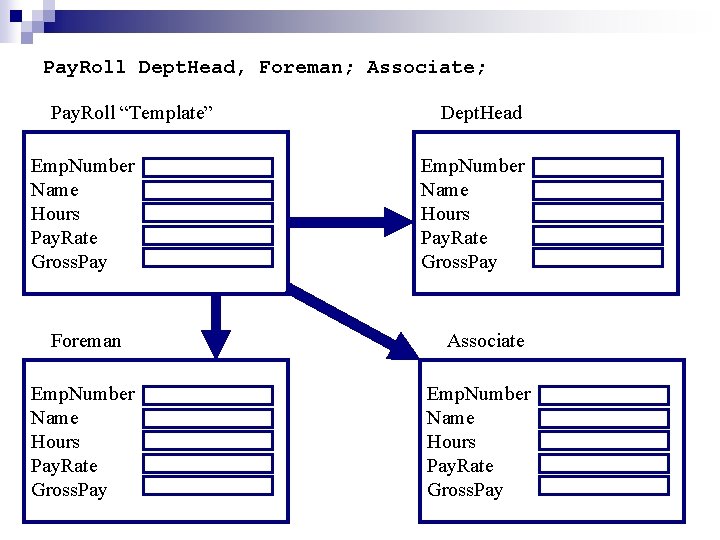
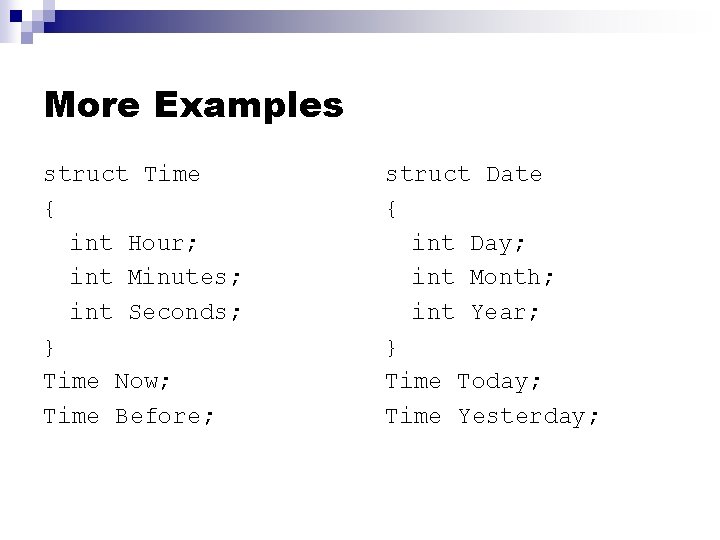
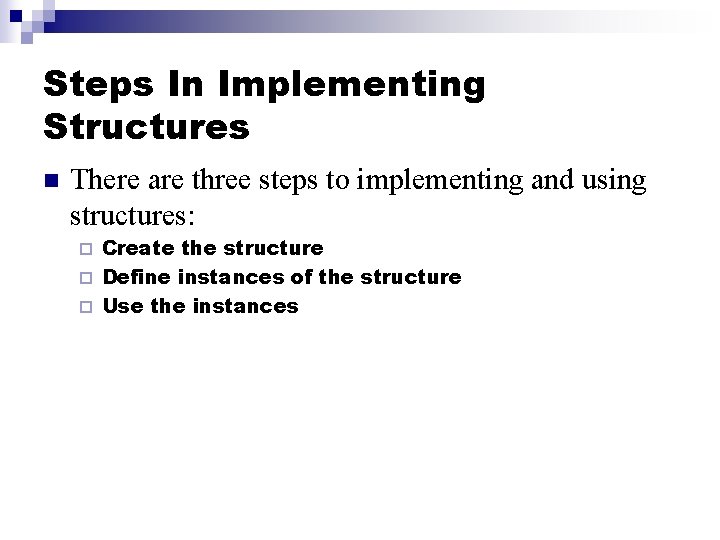
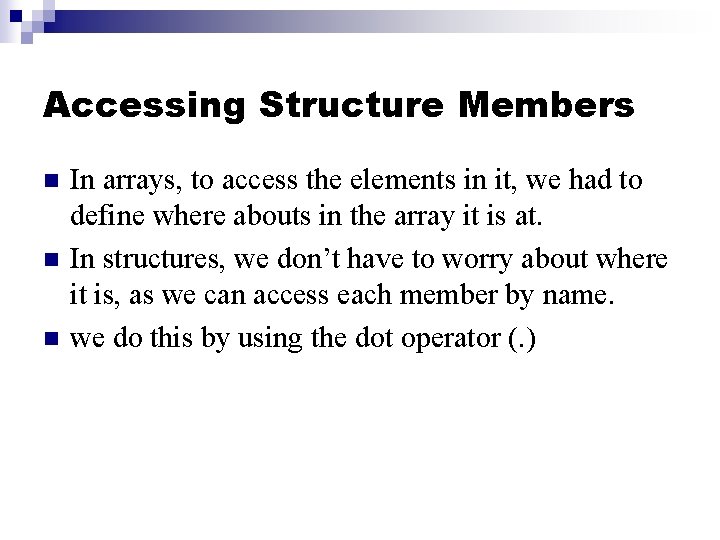
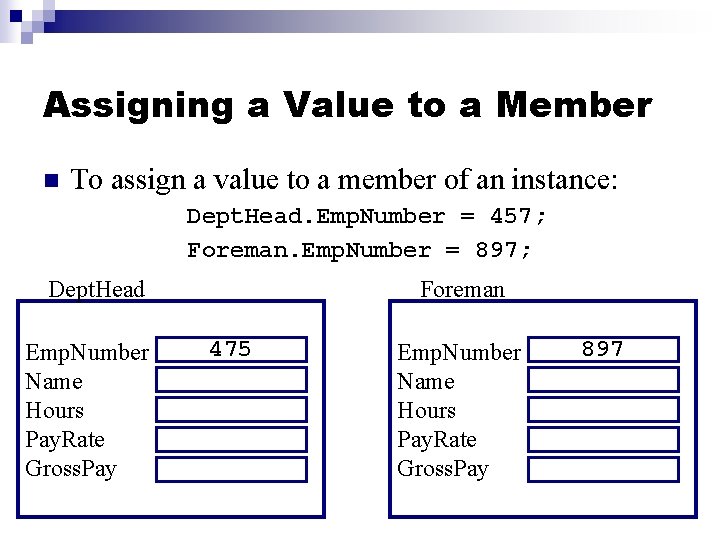
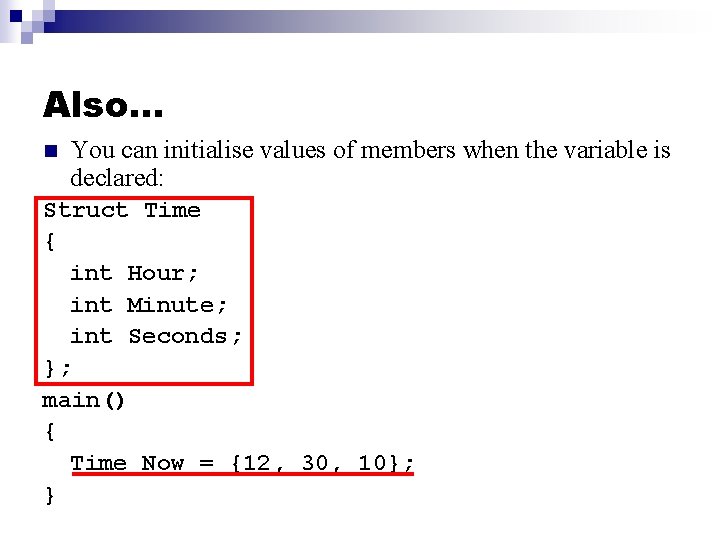
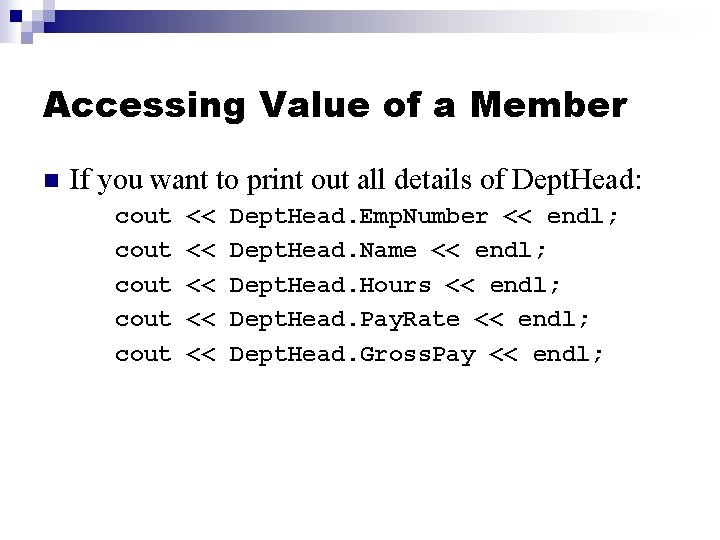
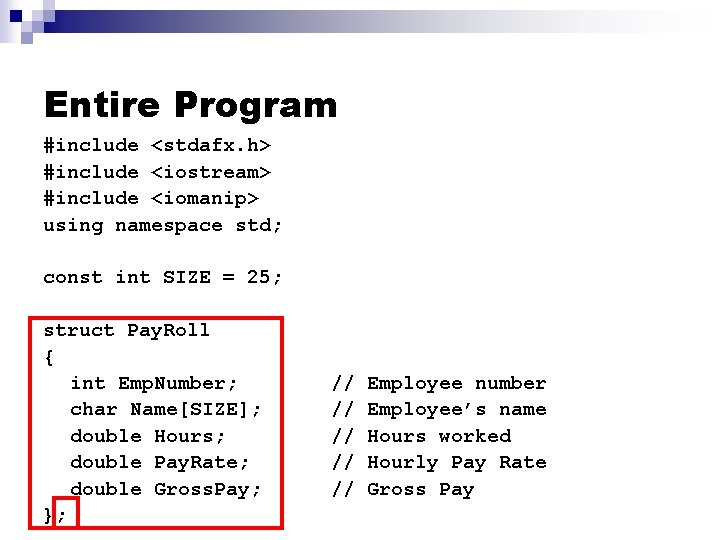
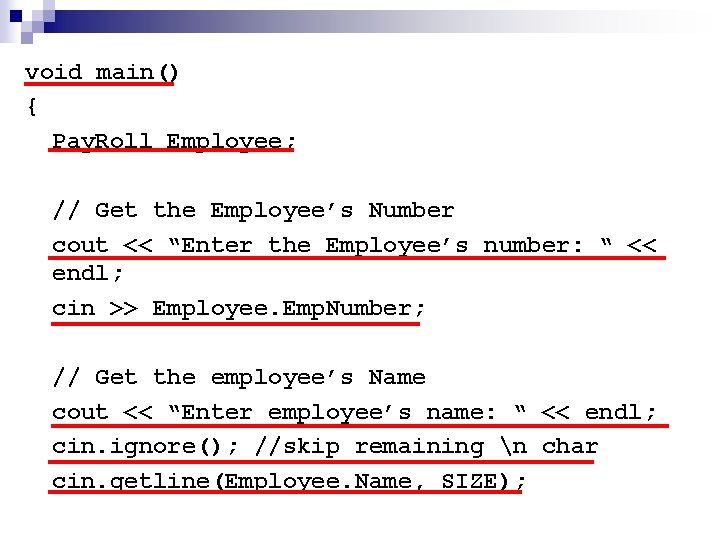
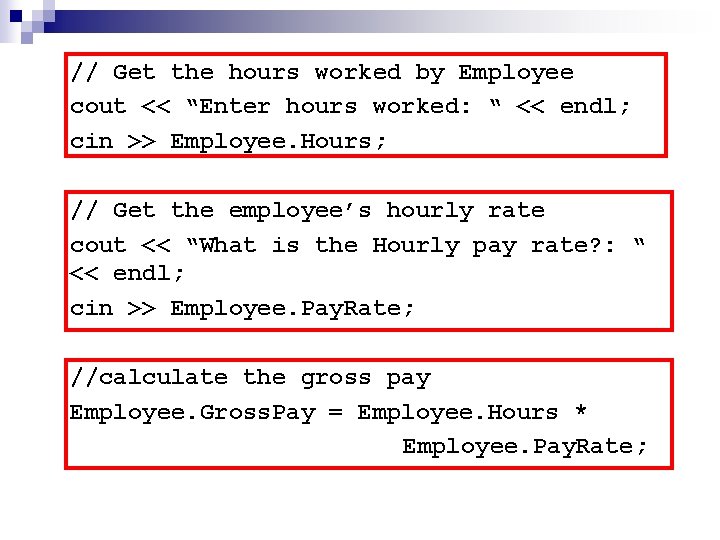
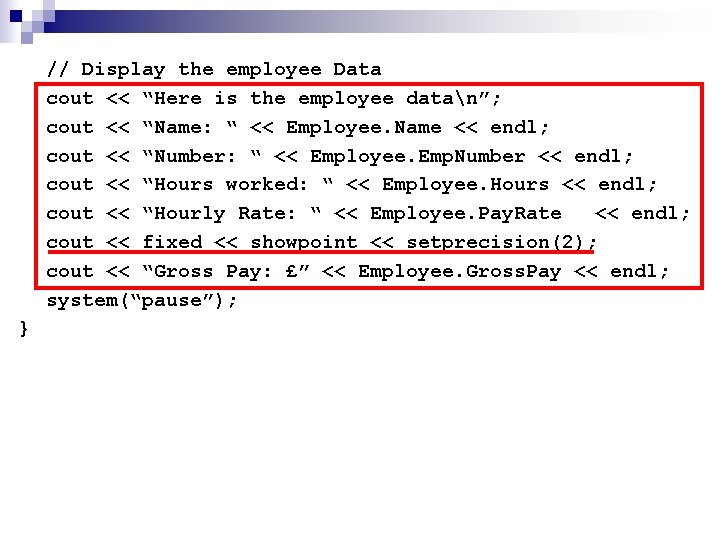
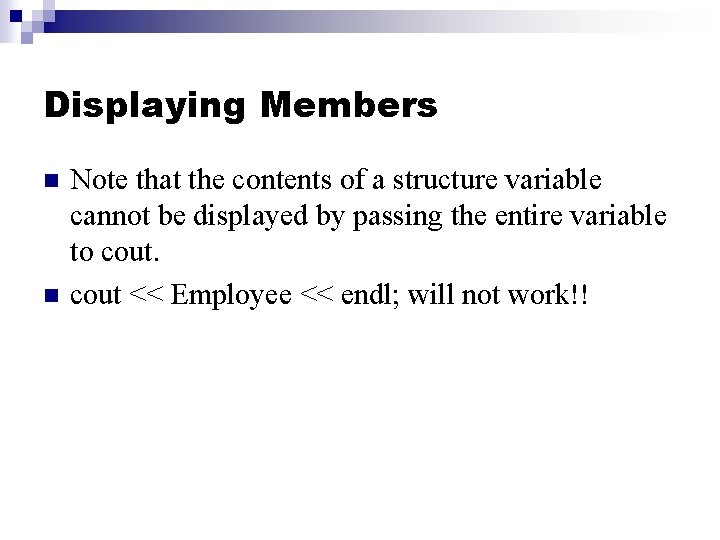
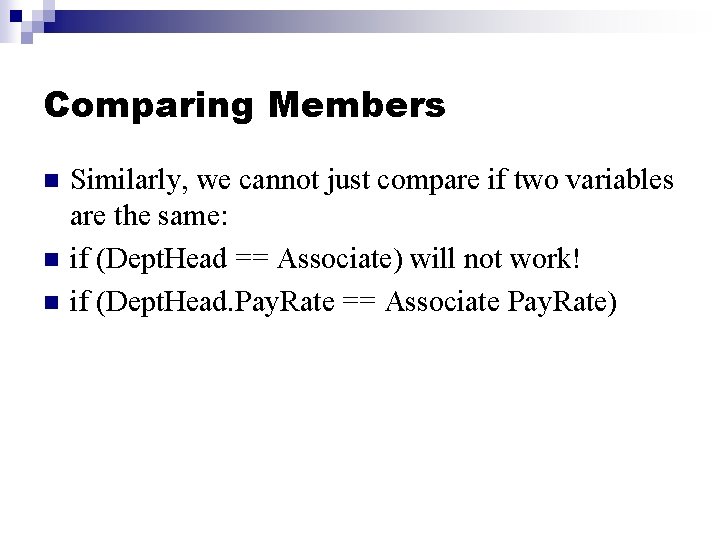
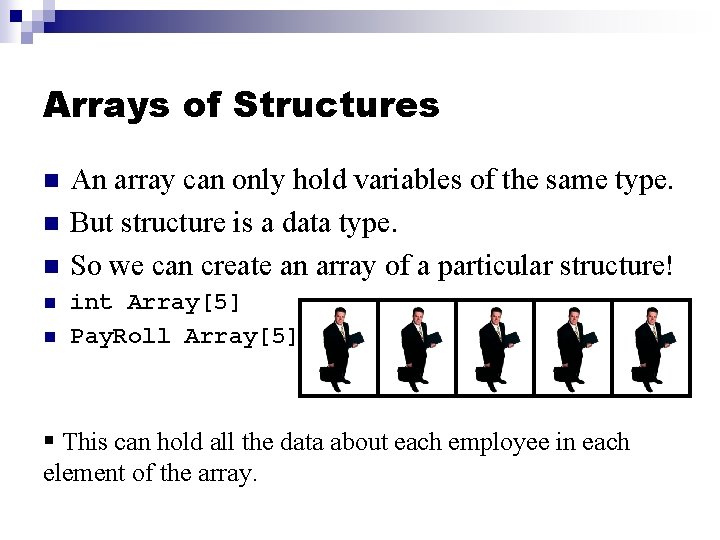
![Example - Book Info struct Book. Info { char Title[50]; char Author[30]; char Publisher[25]; Example - Book Info struct Book. Info { char Title[50]; char Author[30]; char Publisher[25];](https://slidetodoc.com/presentation_image_h/b1384de6f4e15c09982ed3cd0aac5a6a/image-27.jpg)
![Arrays of Structures n To find the title of the book in Book. List[5]: Arrays of Structures n To find the title of the book in Book. List[5]:](https://slidetodoc.com/presentation_image_h/b1384de6f4e15c09982ed3cd0aac5a6a/image-28.jpg)
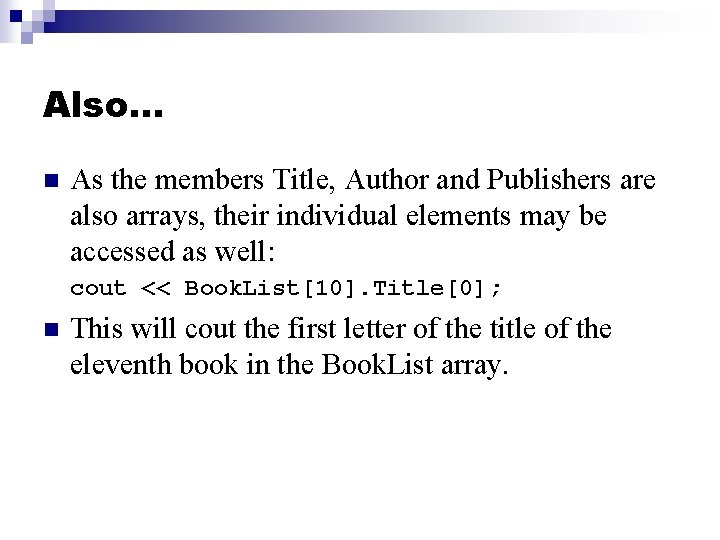
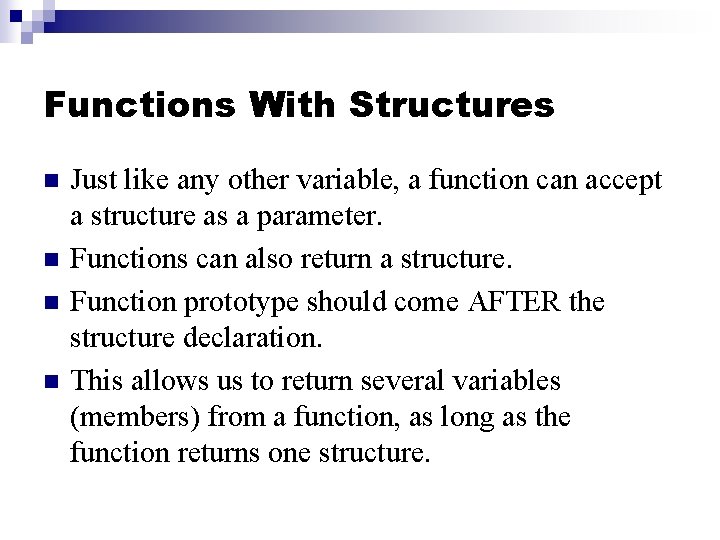
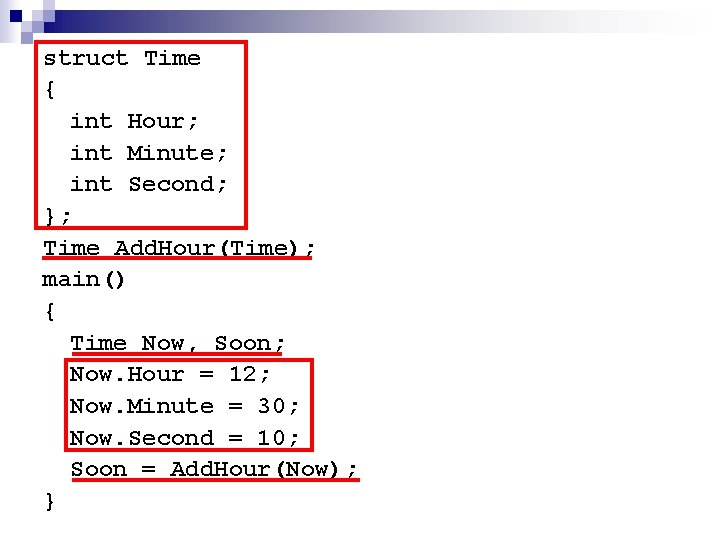
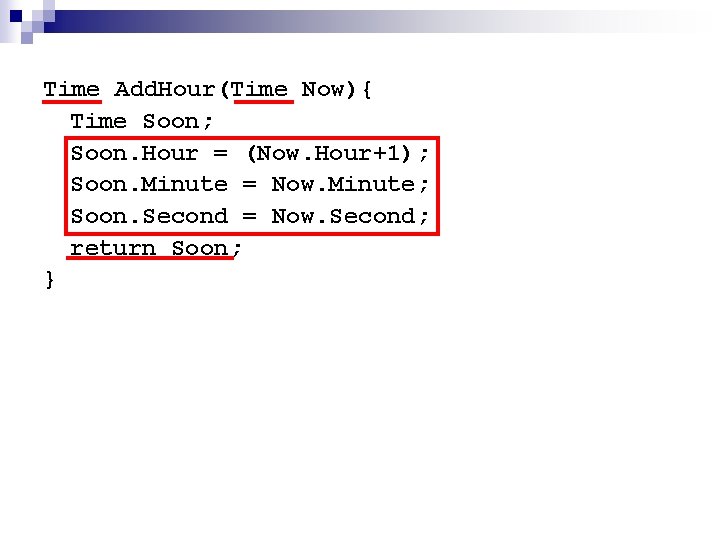
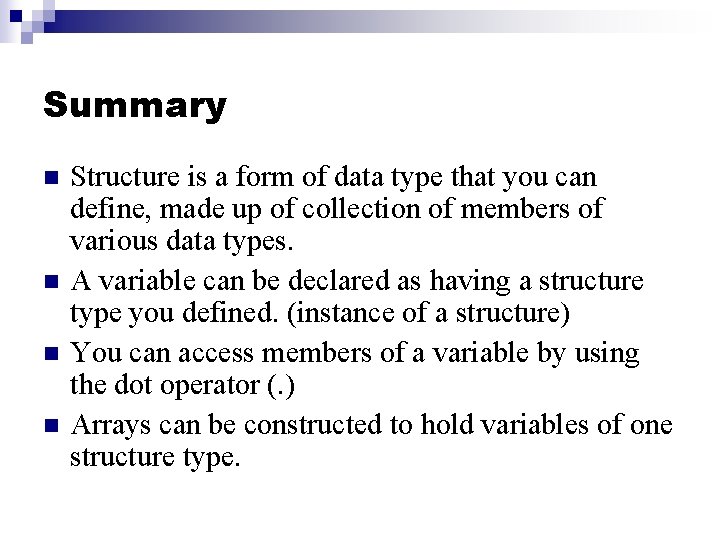
- Slides: 33
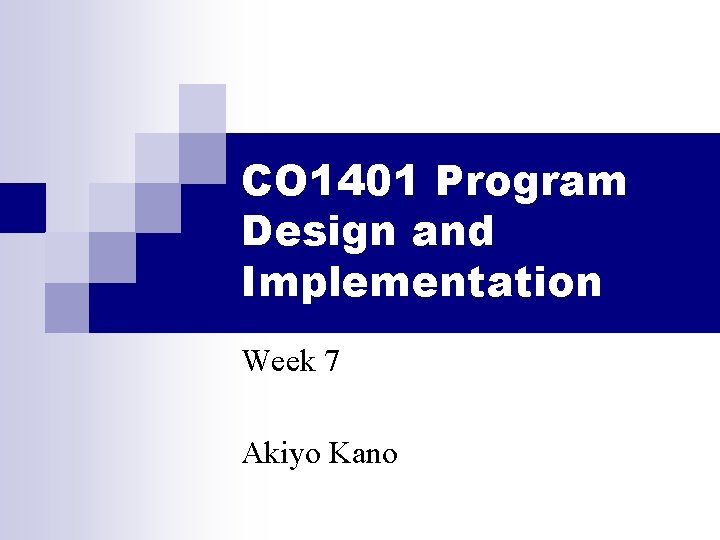
CO 1401 Program Design and Implementation Week 7 Akiyo Kano
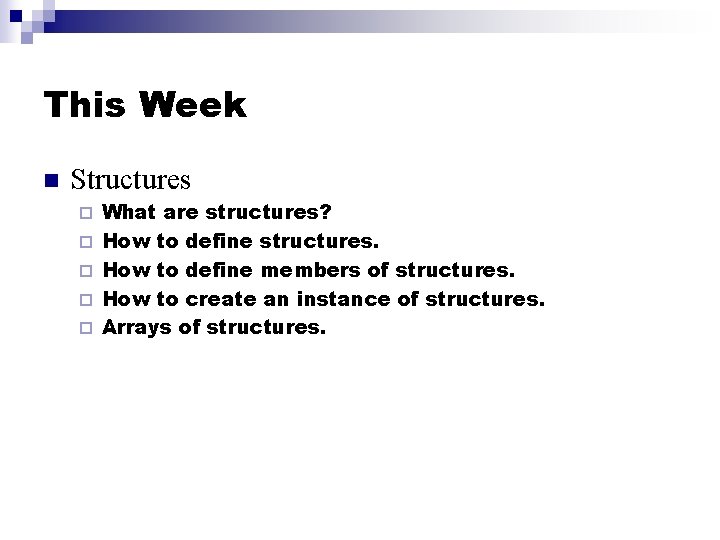
This Week n Structures ¨ ¨ ¨ What are structures? How to define structures. How to define members of structures. How to create an instance of structures. Arrays of structures.
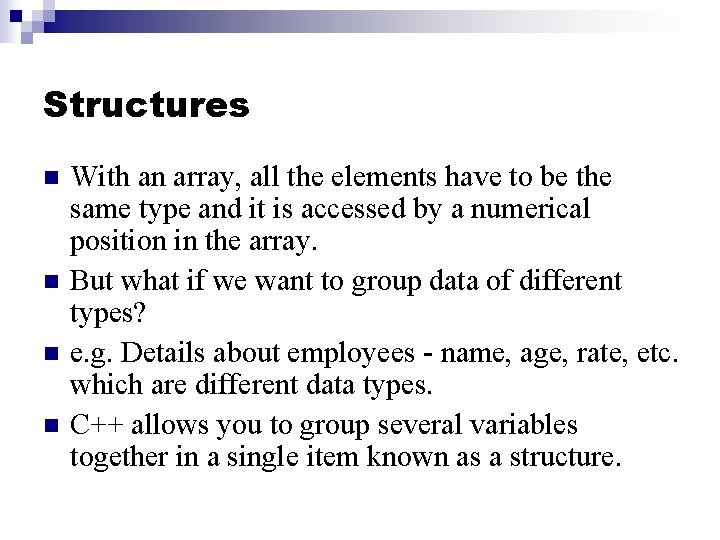
Structures n n With an array, all the elements have to be the same type and it is accessed by a numerical position in the array. But what if we want to group data of different types? e. g. Details about employees - name, age, rate, etc. which are different data types. C++ allows you to group several variables together in a single item known as a structure.
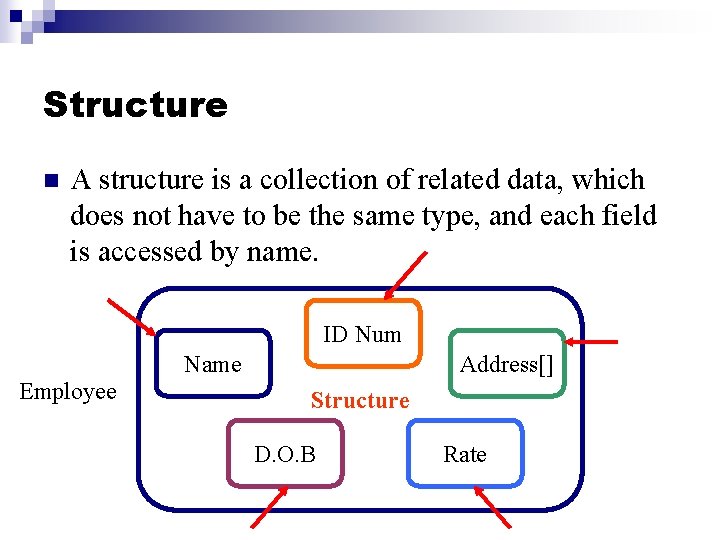
Structure n A structure is a collection of related data, which does not have to be the same type, and each field is accessed by name. ID Num Name Employee Address[] Structure D. O. B Rate
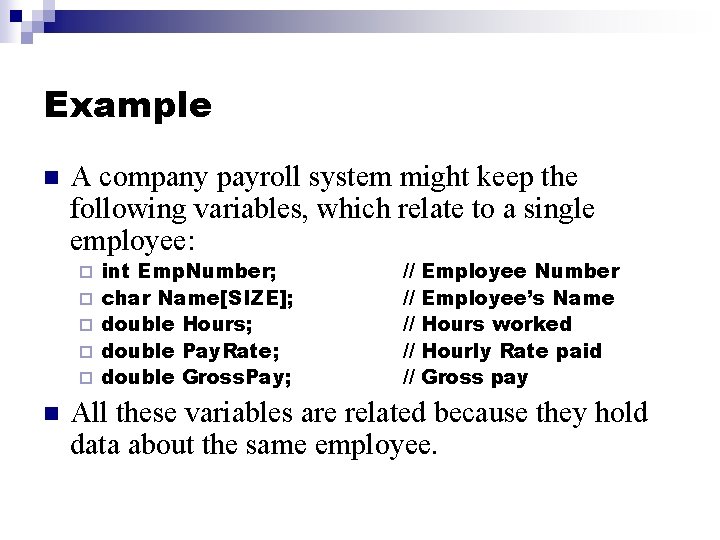
Example n A company payroll system might keep the following variables, which relate to a single employee: ¨ ¨ ¨ n int Emp. Number; char Name[SIZE]; double Hours; double Pay. Rate; double Gross. Pay; // // // Employee Number Employee’s Name Hours worked Hourly Rate paid Gross pay All these variables are related because they hold data about the same employee.
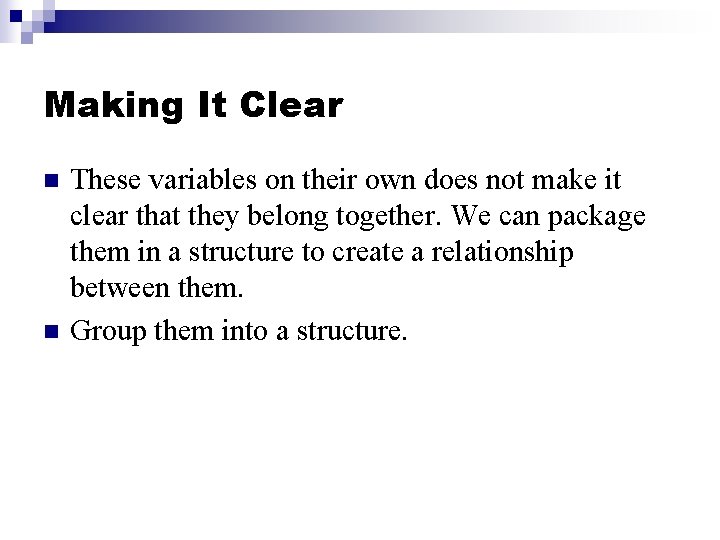
Making It Clear n n These variables on their own does not make it clear that they belong together. We can package them in a structure to create a relationship between them. Group them into a structure.
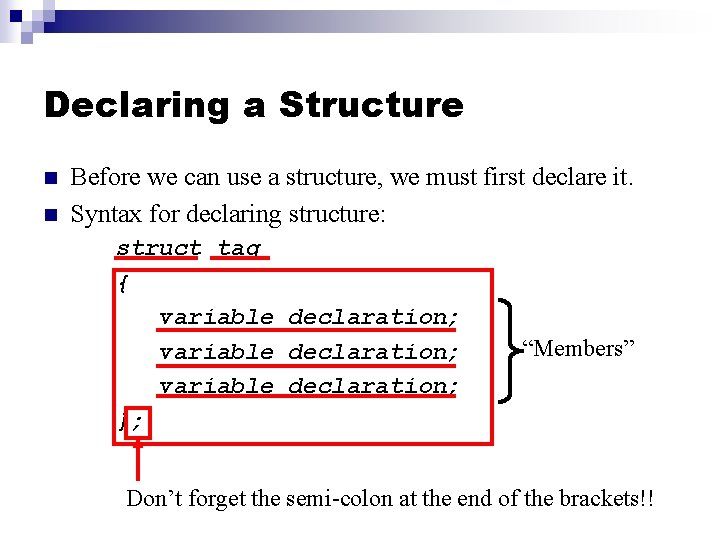
Declaring a Structure n n Before we can use a structure, we must first declare it. Syntax for declaring structure: struct tag { variable declaration; }; “Members” Don’t forget the semi-colon at the end of the brackets!!
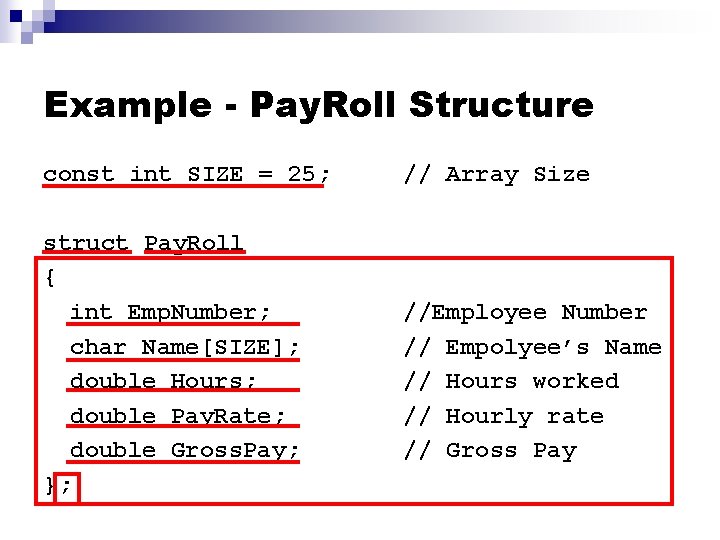
Example - Pay. Roll Structure const int SIZE = 25; struct Pay. Roll { int Emp. Number; char Name[SIZE]; double Hours; double Pay. Rate; double Gross. Pay; }; // Array Size //Employee Number // Empolyee’s Name // Hours worked // Hourly rate // Gross Pay
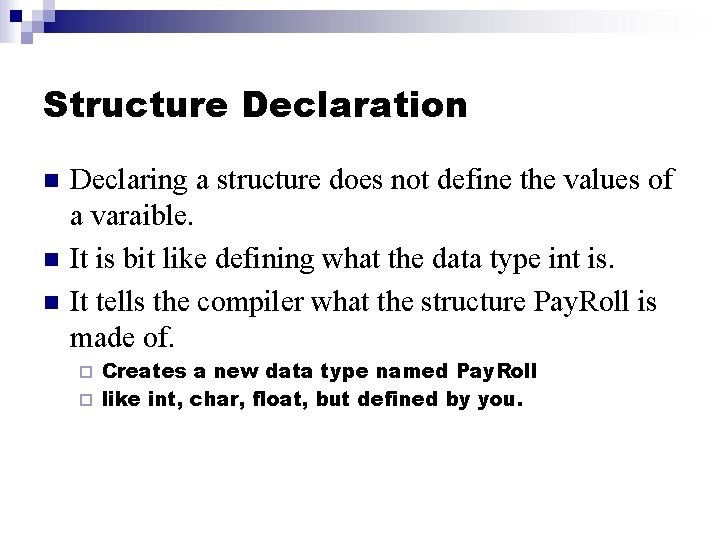
Structure Declaration n Declaring a structure does not define the values of a varaible. It is bit like defining what the data type int is. It tells the compiler what the structure Pay. Roll is made of. Creates a new data type named Pay. Roll ¨ like int, char, float, but defined by you. ¨
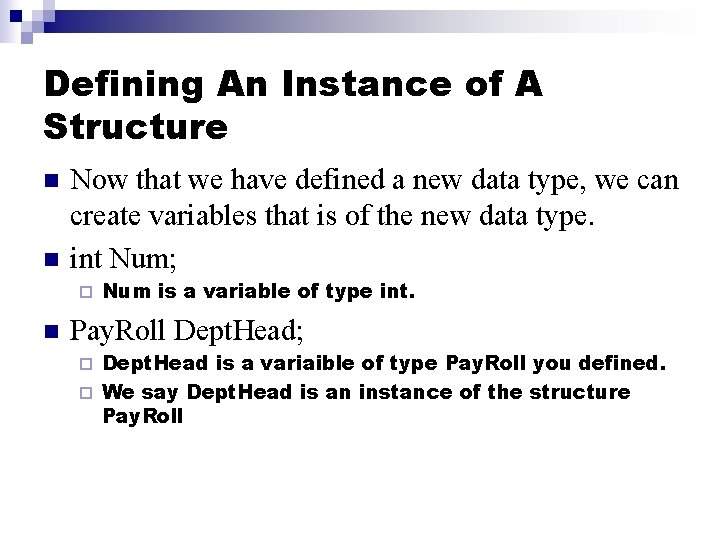
Defining An Instance of A Structure n n Now that we have defined a new data type, we can create variables that is of the new data type. int Num; ¨ n Num is a variable of type int. Pay. Roll Dept. Head; Dept. Head is a variaible of type Pay. Roll you defined. ¨ We say Dept. Head is an instance of the structure Pay. Roll ¨
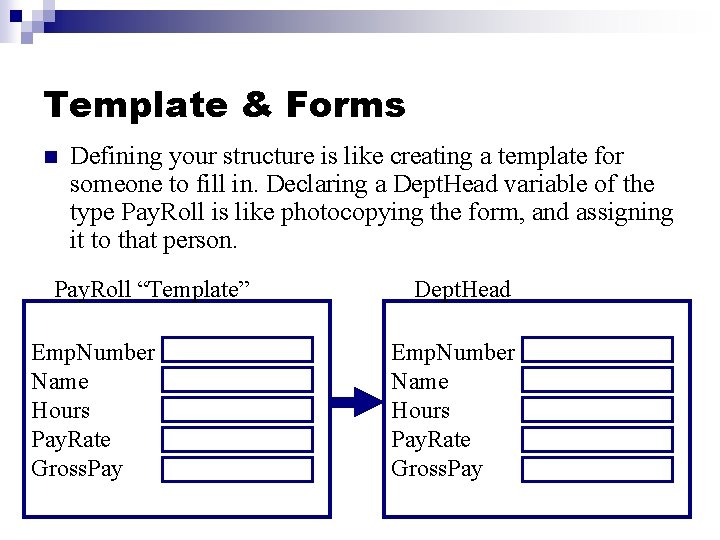
Template & Forms n Defining your structure is like creating a template for someone to fill in. Declaring a Dept. Head variable of the type Pay. Roll is like photocopying the form, and assigning it to that person. Pay. Roll “Template” Emp. Number Name Hours Pay. Rate Gross. Pay Dept. Head Emp. Number Name Hours Pay. Rate Gross. Pay
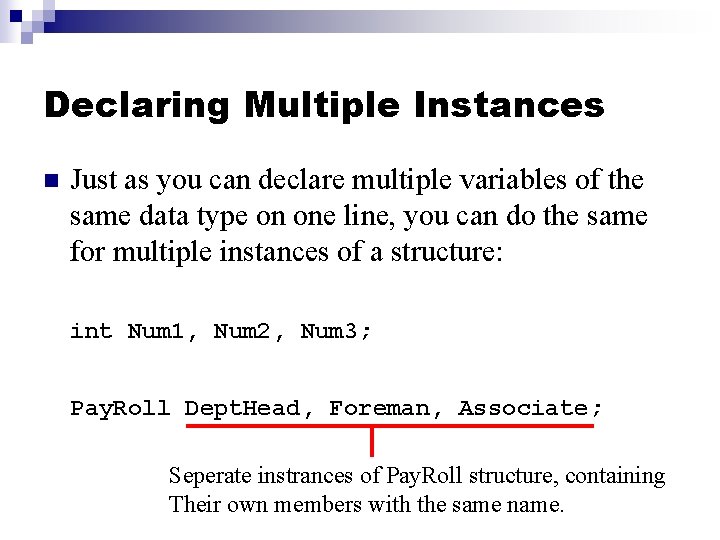
Declaring Multiple Instances n Just as you can declare multiple variables of the same data type on one line, you can do the same for multiple instances of a structure: int Num 1, Num 2, Num 3; Pay. Roll Dept. Head, Foreman, Associate; Seperate instrances of Pay. Roll structure, containing Their own members with the same name.
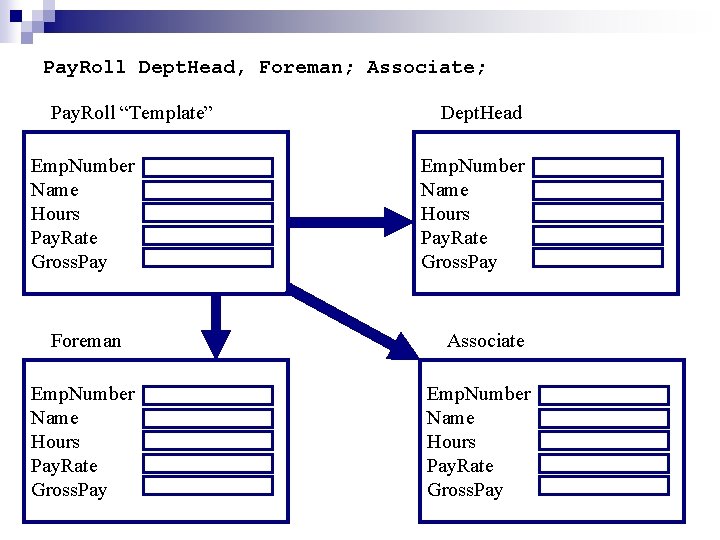
Pay. Roll Dept. Head, Foreman; Associate; Pay. Roll “Template” Emp. Number Name Hours Pay. Rate Gross. Pay Foreman Emp. Number Name Hours Pay. Rate Gross. Pay Dept. Head Emp. Number Name Hours Pay. Rate Gross. Pay Associate Emp. Number Name Hours Pay. Rate Gross. Pay
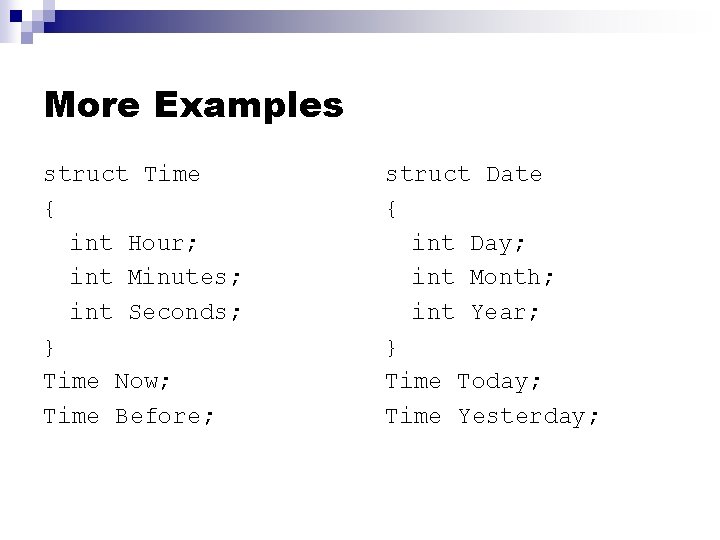
More Examples struct Time { int Hour; int Minutes; int Seconds; } Time Now; Time Before; struct Date { int Day; int Month; int Year; } Time Today; Time Yesterday;
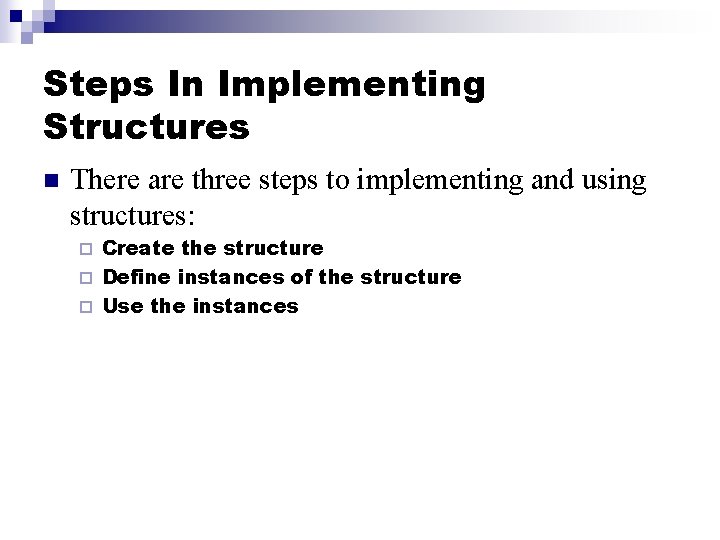
Steps In Implementing Structures n There are three steps to implementing and using structures: Create the structure ¨ Define instances of the structure ¨ Use the instances ¨
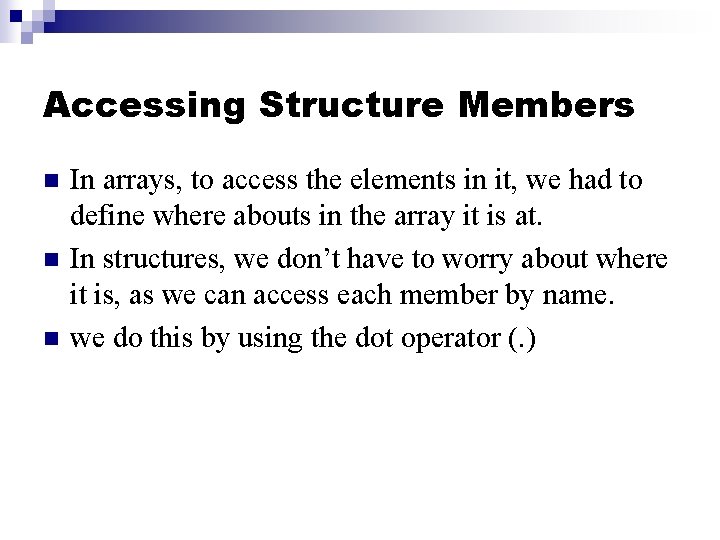
Accessing Structure Members n n n In arrays, to access the elements in it, we had to define where abouts in the array it is at. In structures, we don’t have to worry about where it is, as we can access each member by name. we do this by using the dot operator (. )
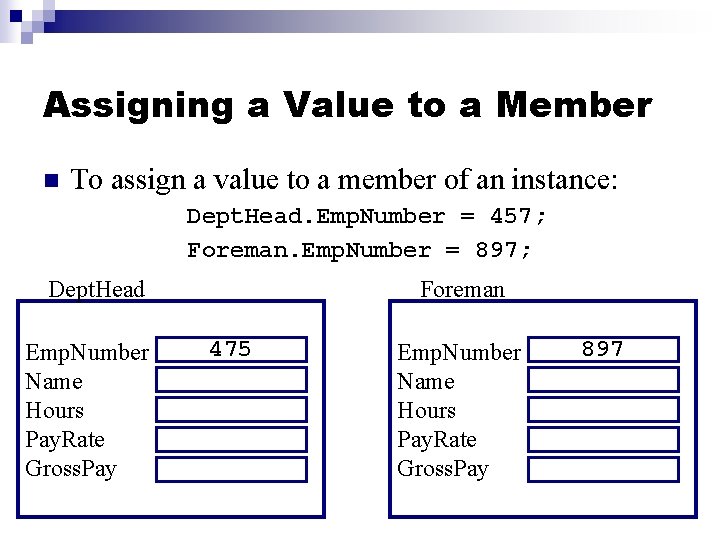
Assigning a Value to a Member n To assign a value to a member of an instance: Dept. Head. Emp. Number = 457; Foreman. Emp. Number = 897; Dept. Head Emp. Number Name Hours Pay. Rate Gross. Pay Foreman 475 Emp. Number Name Hours Pay. Rate Gross. Pay 897
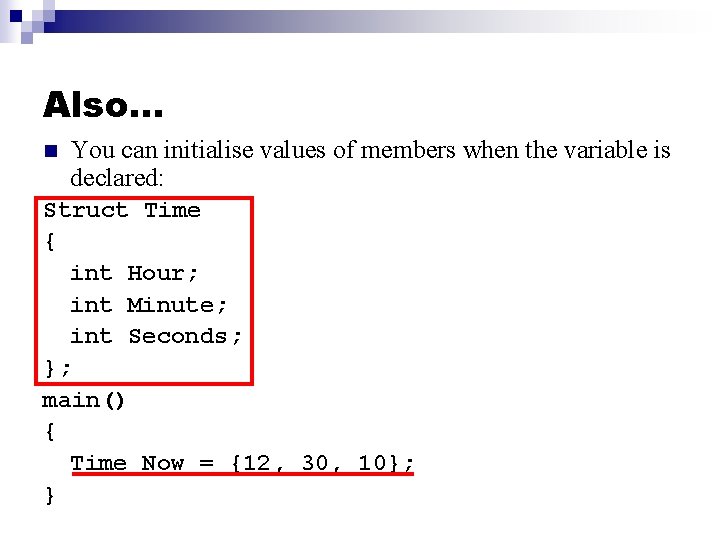
Also. . . n You can initialise values of members when the variable is declared: Struct Time { int Hour; int Minute; int Seconds; }; main() { Time Now = {12, 30, 10}; }
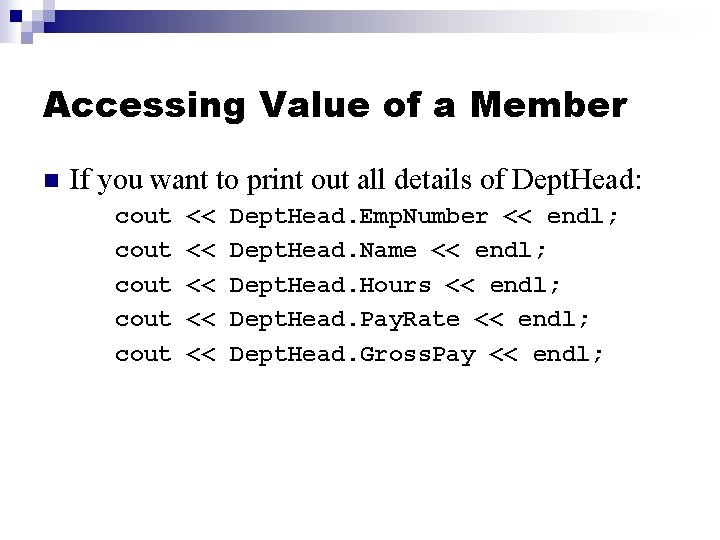
Accessing Value of a Member n If you want to print out all details of Dept. Head: cout cout << << << Dept. Head. Emp. Number << endl; Dept. Head. Name << endl; Dept. Head. Hours << endl; Dept. Head. Pay. Rate << endl; Dept. Head. Gross. Pay << endl;
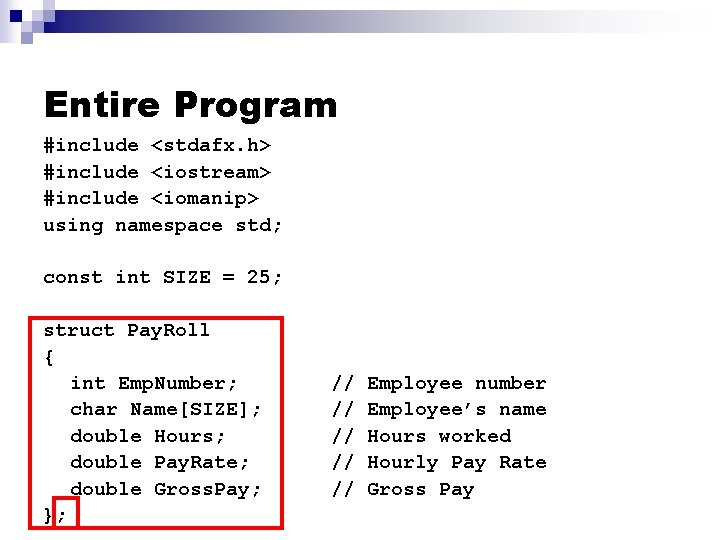
Entire Program #include <stdafx. h> #include <iostream> #include <iomanip> using namespace std; const int SIZE = 25; struct Pay. Roll { int Emp. Number; char Name[SIZE]; double Hours; double Pay. Rate; double Gross. Pay; }; // // // Employee number Employee’s name Hours worked Hourly Pay Rate Gross Pay
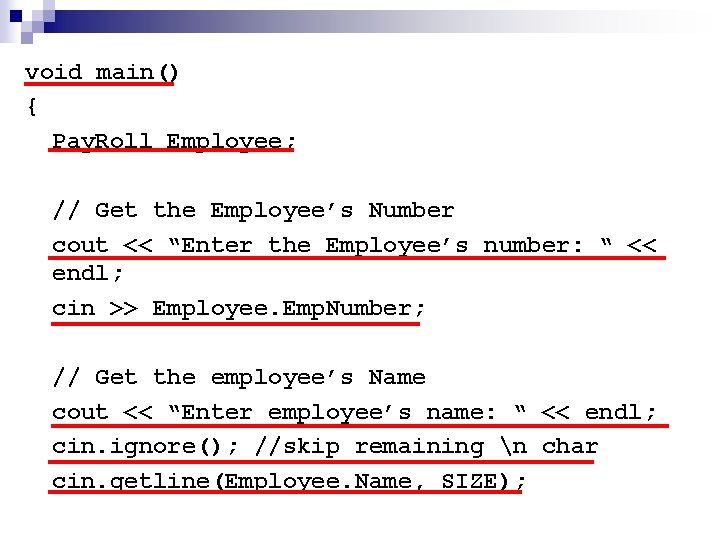
void main() { Pay. Roll Employee; // Get the Employee’s Number cout << “Enter the Employee’s number: “ << endl; cin >> Employee. Emp. Number; // Get the employee’s Name cout << “Enter employee’s name: “ << endl; cin. ignore(); //skip remaining n char cin. getline(Employee. Name, SIZE);
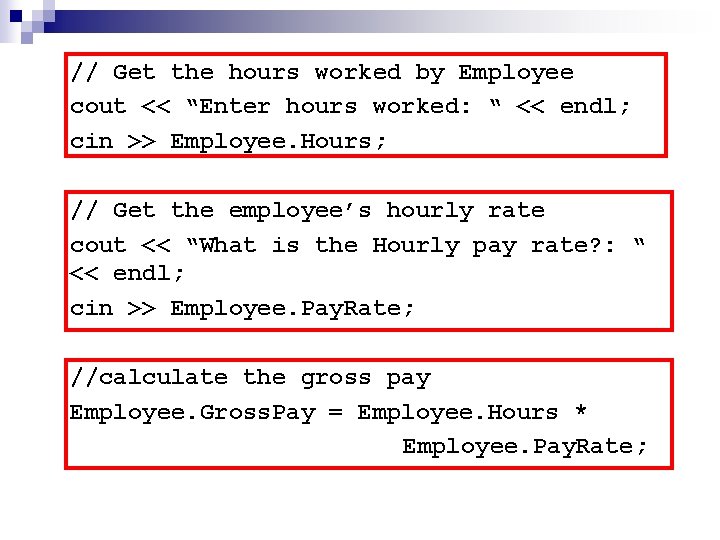
// Get the hours worked by Employee cout << “Enter hours worked: “ << endl; cin >> Employee. Hours; // Get the employee’s hourly rate cout << “What is the Hourly pay rate? : “ << endl; cin >> Employee. Pay. Rate; //calculate the gross pay Employee. Gross. Pay = Employee. Hours * Employee. Pay. Rate;
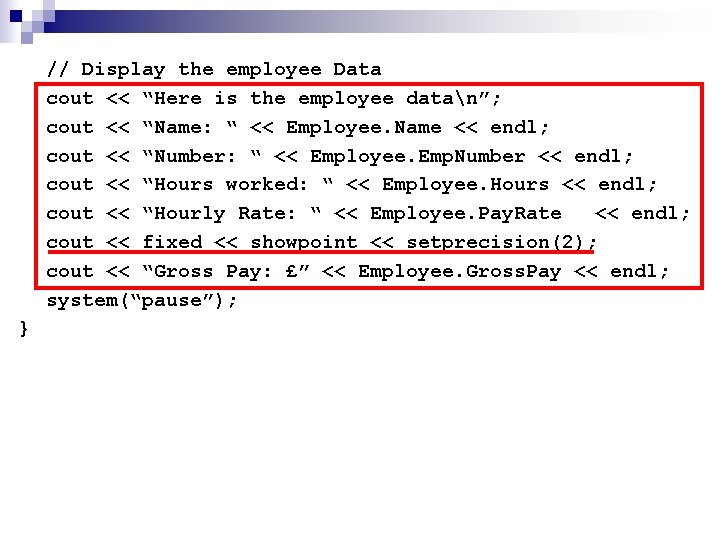
// Display the employee Data cout << “Here is the employee datan”; cout << “Name: “ << Employee. Name << endl; cout << “Number: “ << Employee. Emp. Number << endl; cout << “Hours worked: “ << Employee. Hours << endl; cout << “Hourly Rate: “ << Employee. Pay. Rate << endl; cout << fixed << showpoint << setprecision(2); cout << “Gross Pay: £” << Employee. Gross. Pay << endl; system(“pause”); }
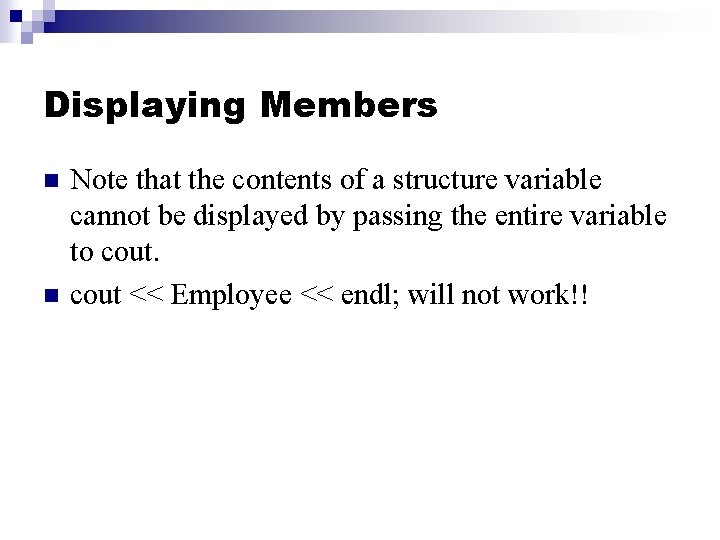
Displaying Members n n Note that the contents of a structure variable cannot be displayed by passing the entire variable to cout << Employee << endl; will not work!!
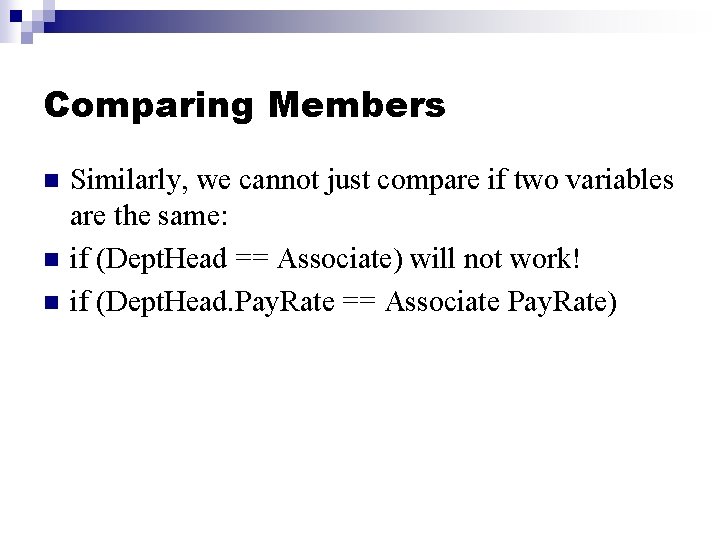
Comparing Members n n n Similarly, we cannot just compare if two variables are the same: if (Dept. Head == Associate) will not work! if (Dept. Head. Pay. Rate == Associate Pay. Rate)
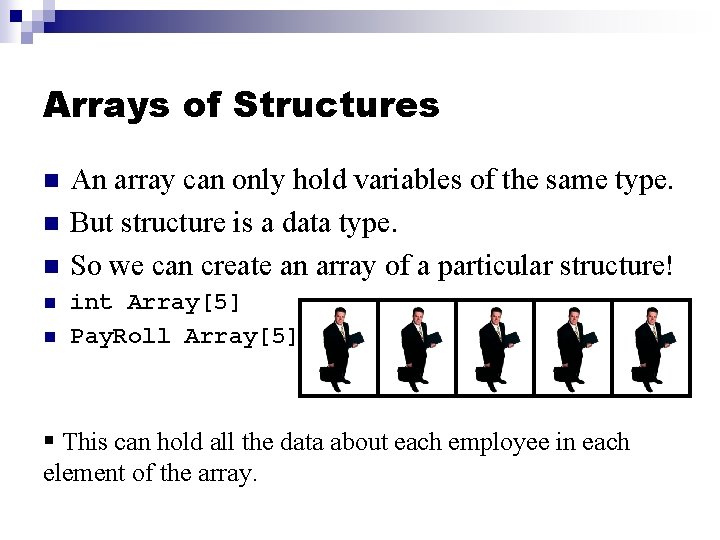
Arrays of Structures n n n An array can only hold variables of the same type. But structure is a data type. So we can create an array of a particular structure! int Array[5] Pay. Roll Array[5] § This can hold all the data about each employee in each element of the array.
![Example Book Info struct Book Info char Title50 char Author30 char Publisher25 Example - Book Info struct Book. Info { char Title[50]; char Author[30]; char Publisher[25];](https://slidetodoc.com/presentation_image_h/b1384de6f4e15c09982ed3cd0aac5a6a/image-27.jpg)
Example - Book Info struct Book. Info { char Title[50]; char Author[30]; char Publisher[25]; double price; }; Book. Info Book. List[20]; This array will hold information about 20 books
![Arrays of Structures n To find the title of the book in Book List5 Arrays of Structures n To find the title of the book in Book. List[5]:](https://slidetodoc.com/presentation_image_h/b1384de6f4e15c09982ed3cd0aac5a6a/image-28.jpg)
Arrays of Structures n To find the title of the book in Book. List[5]: Book. List[5]. title n To display all the info on all the books: for (int x=0; x < 20; x++) { cout << Book. List[x]. Title << endl; cout << Book. List[x]. Author << endl; cout << Book. List[x]. Publisher << endl; cout << Book. List[x]. Price << endl; }
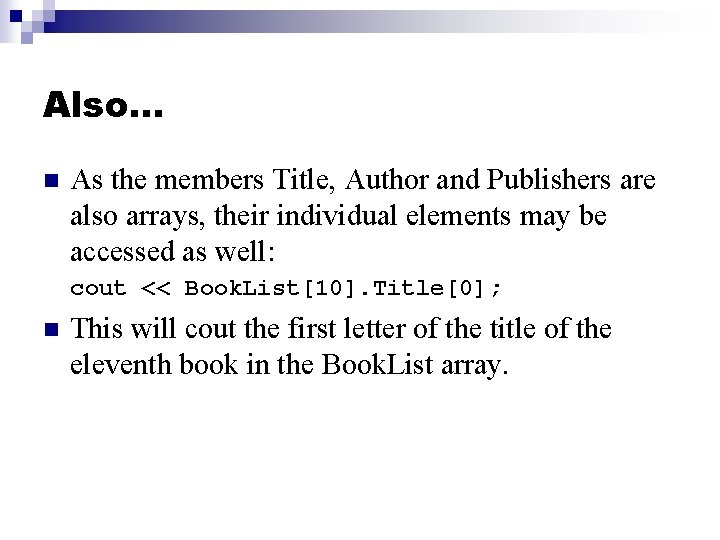
Also. . . n As the members Title, Author and Publishers are also arrays, their individual elements may be accessed as well: cout << Book. List[10]. Title[0]; n This will cout the first letter of the title of the eleventh book in the Book. List array.
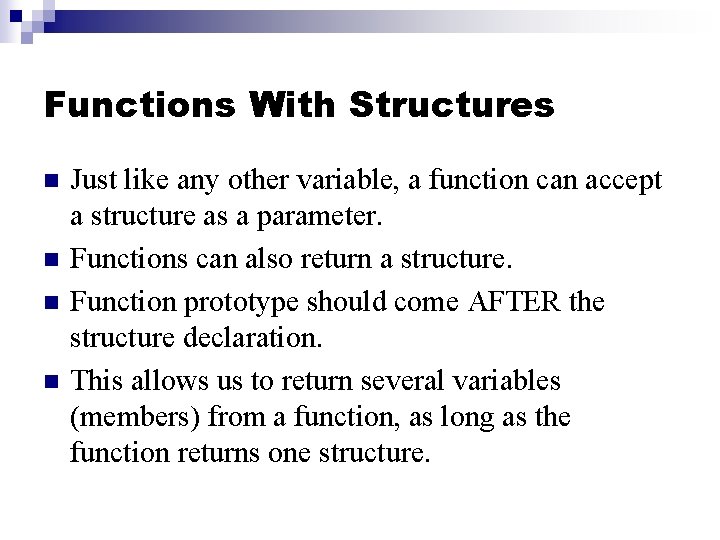
Functions With Structures n n Just like any other variable, a function can accept a structure as a parameter. Functions can also return a structure. Function prototype should come AFTER the structure declaration. This allows us to return several variables (members) from a function, as long as the function returns one structure.
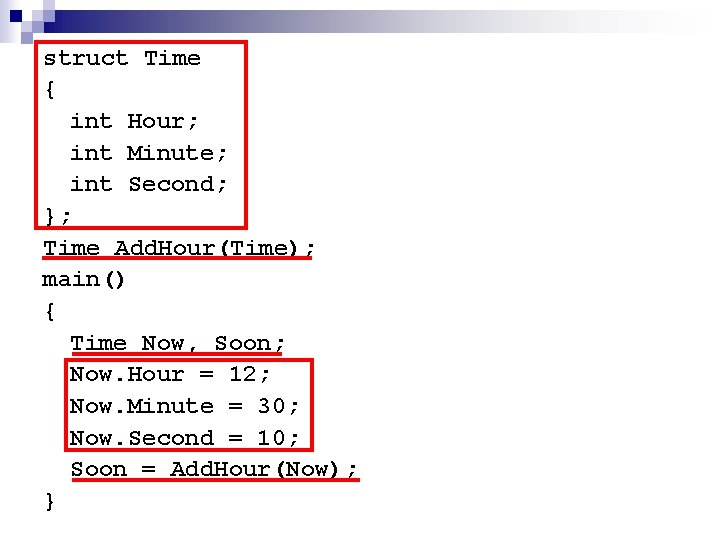
struct Time { int Hour; int Minute; int Second; }; Time Add. Hour(Time); main() { Time Now, Soon; Now. Hour = 12; Now. Minute = 30; Now. Second = 10; Soon = Add. Hour(Now); }
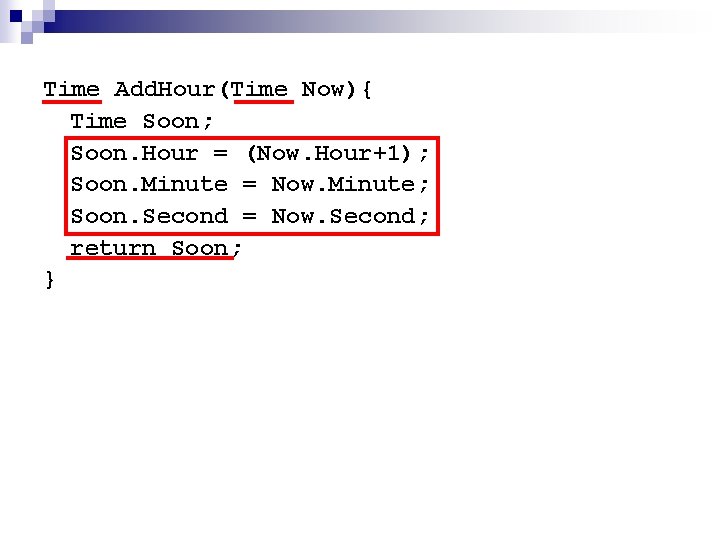
Time Add. Hour(Time Now){ Time Soon; Soon. Hour = (Now. Hour+1); Soon. Minute = Now. Minute; Soon. Second = Now. Second; return Soon; }
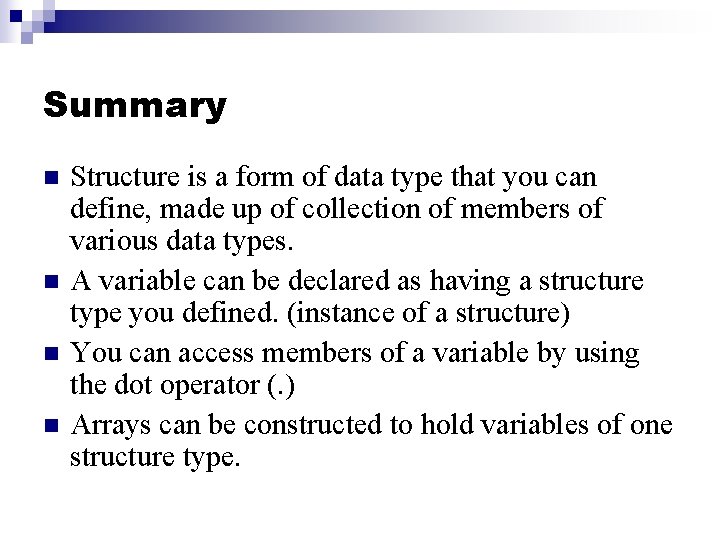
Summary n n Structure is a form of data type that you can define, made up of collection of members of various data types. A variable can be declared as having a structure type you defined. (instance of a structure) You can access members of a variable by using the dot operator (. ) Arrays can be constructed to hold variables of one structure type.