CMSC 202 Inheritance I Class Reuse with Inheritance
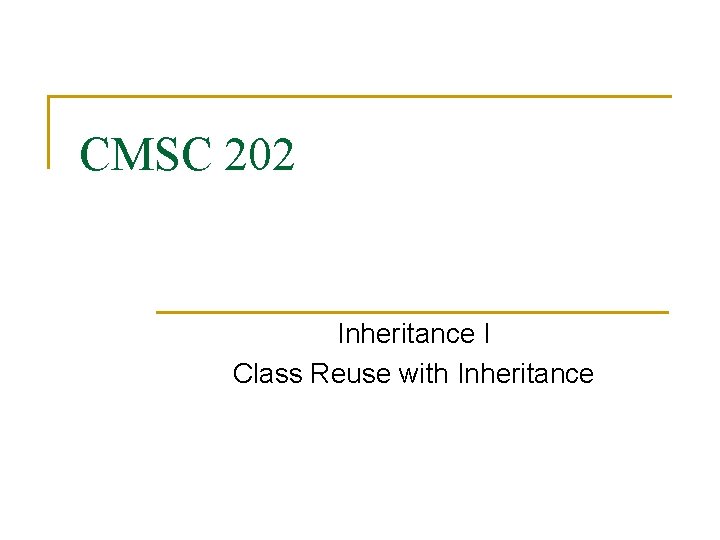
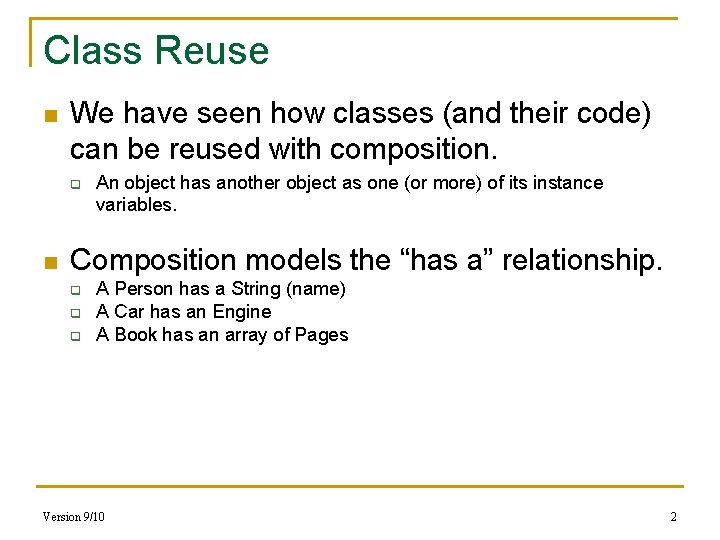
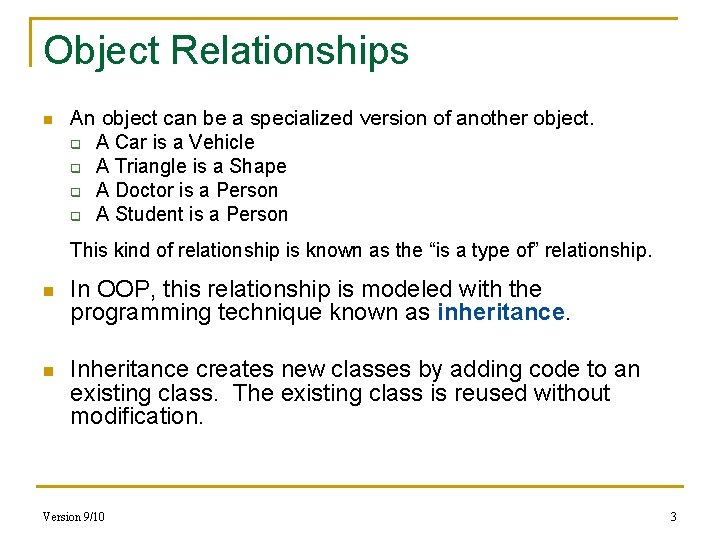
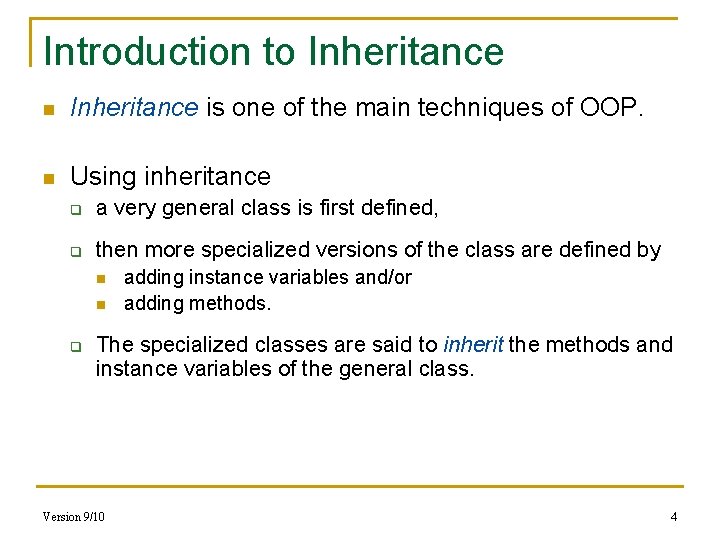
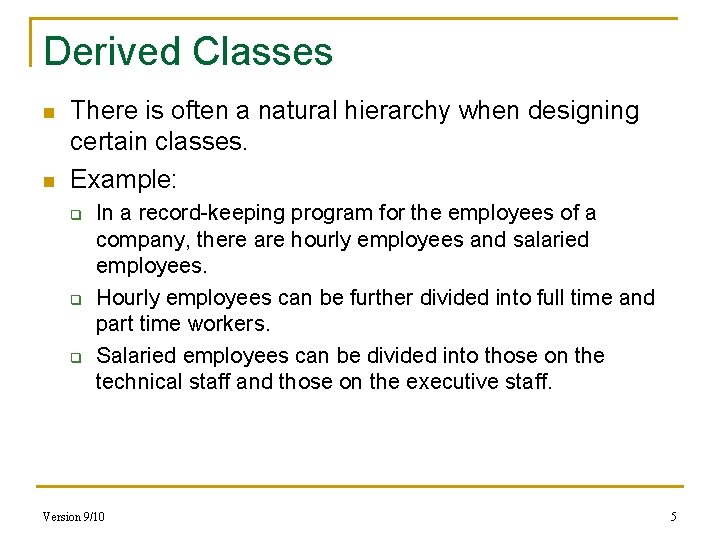
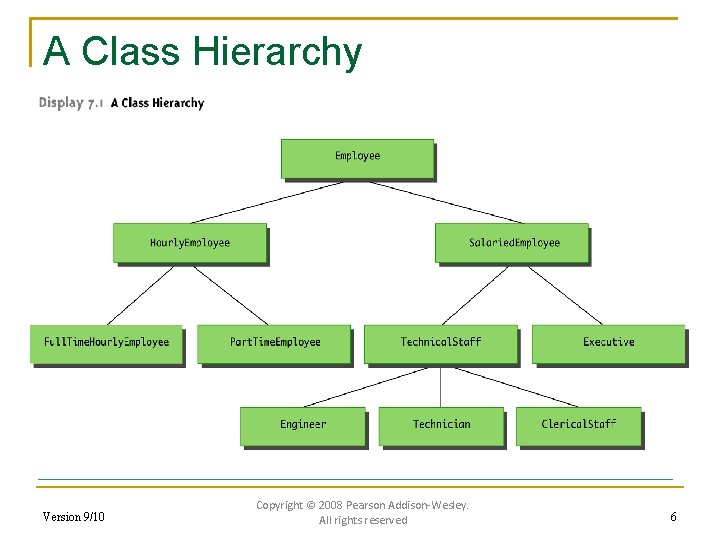
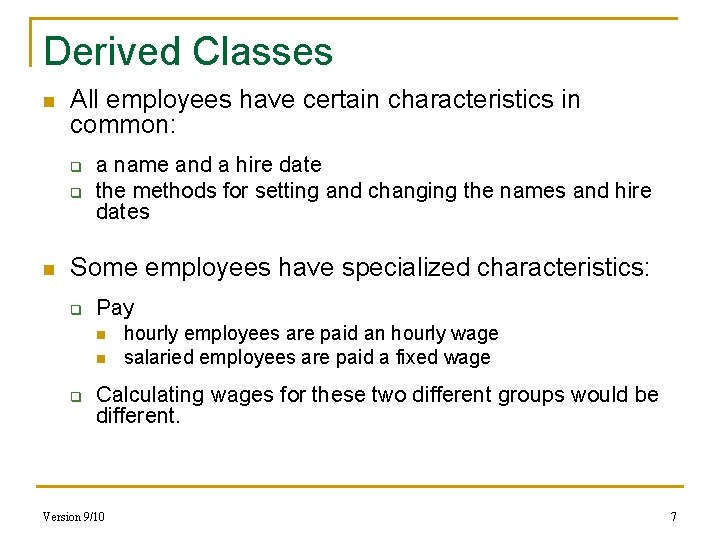
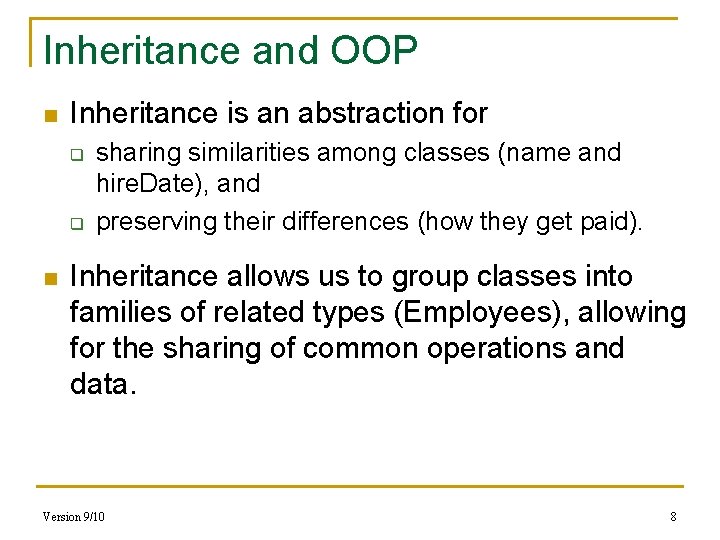
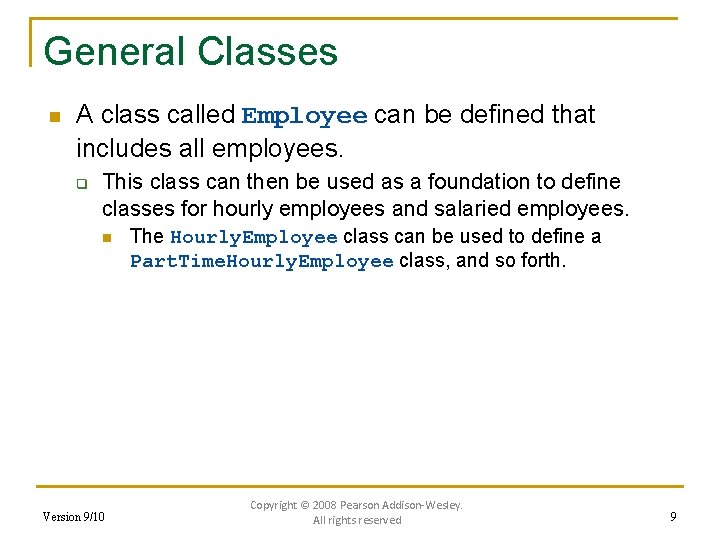
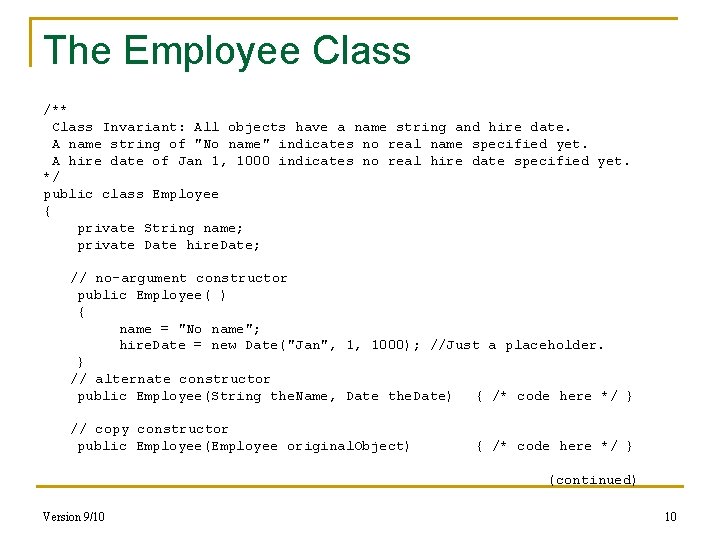
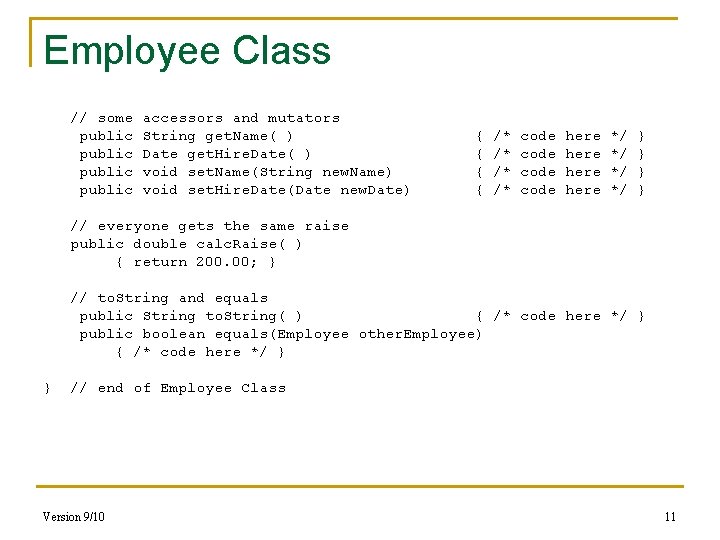
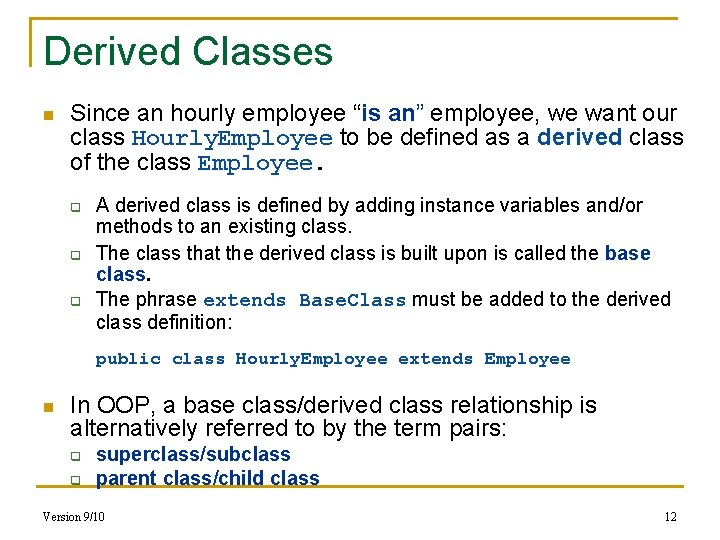
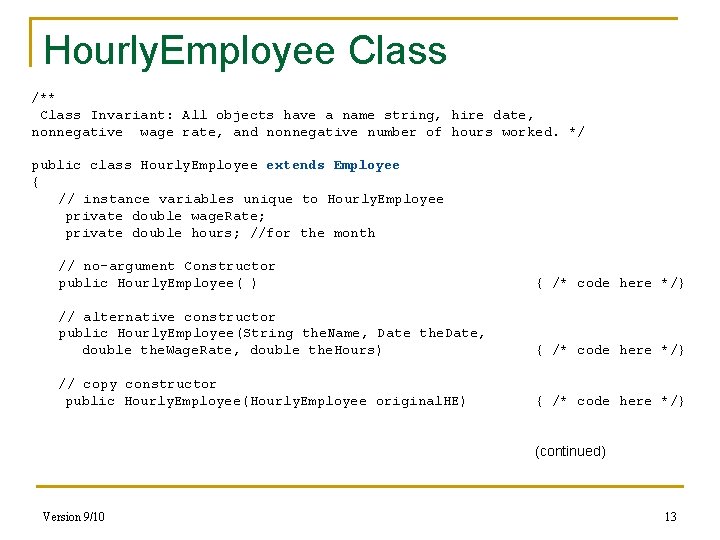
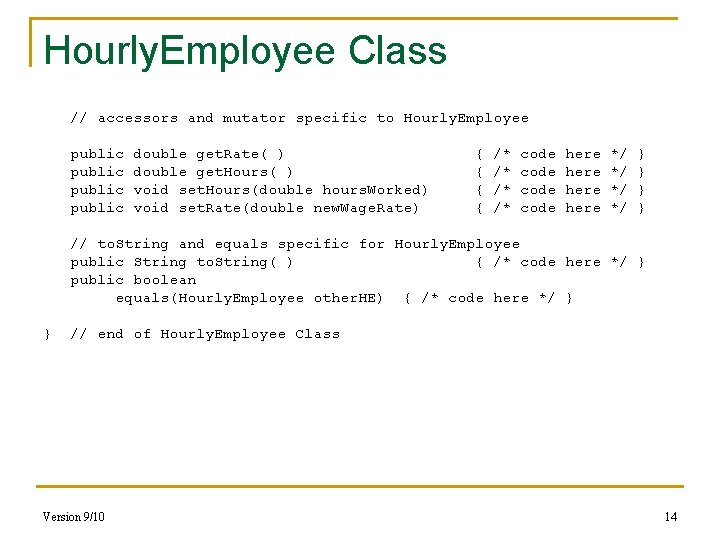
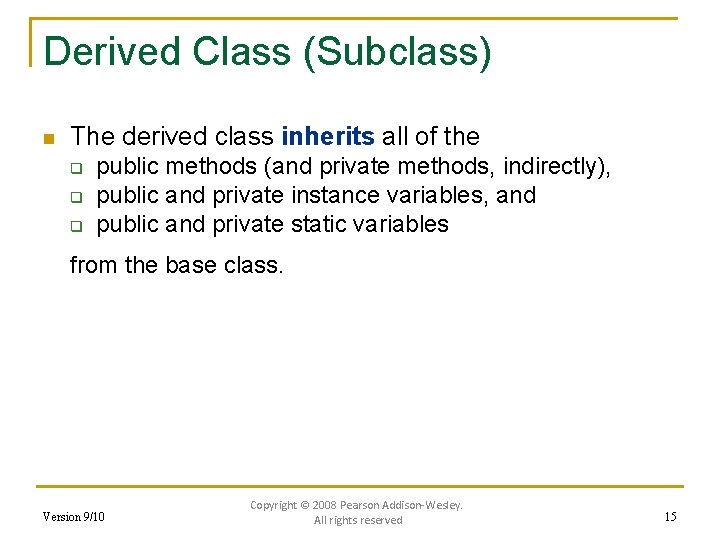
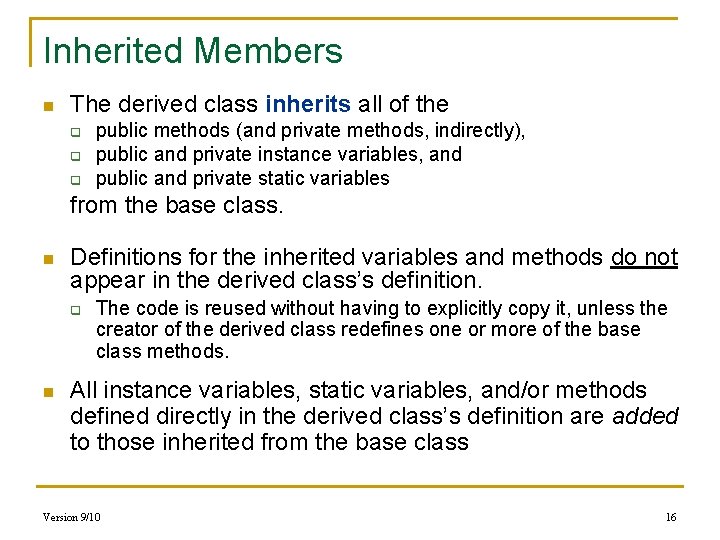
![Using Hourly. Employee public class Hourly. Employee. Example { public static void main(String[] args) Using Hourly. Employee public class Hourly. Employee. Example { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/adf2986af2dbaf16a0b00676867029f1/image-17.jpg)
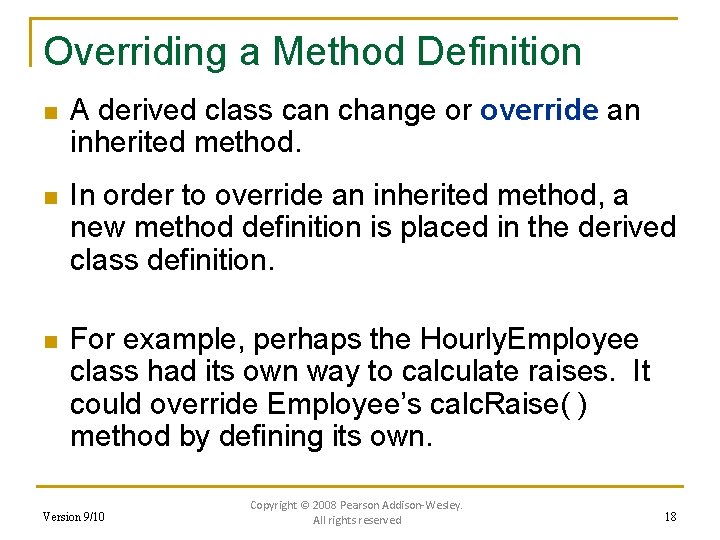
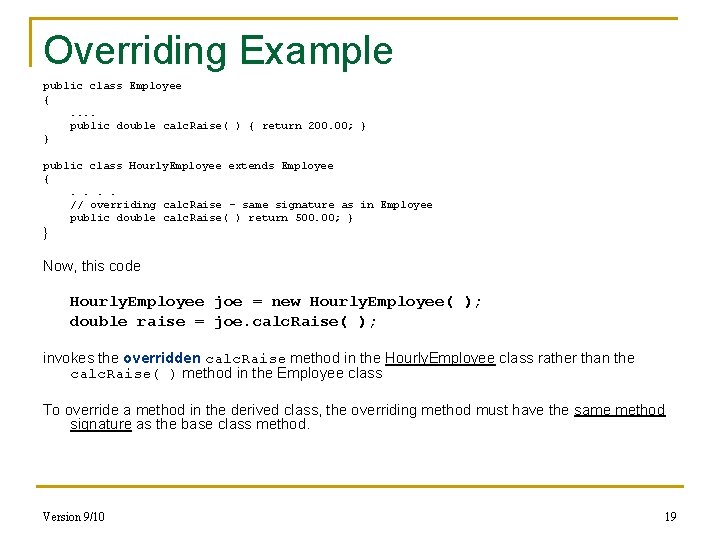
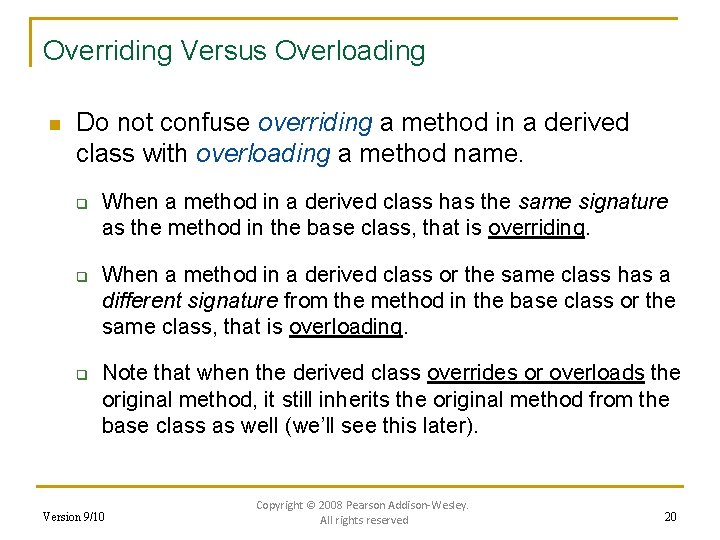
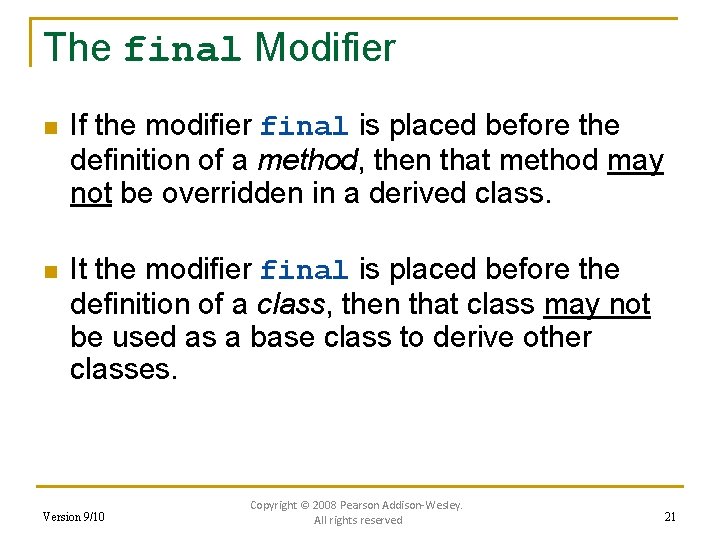
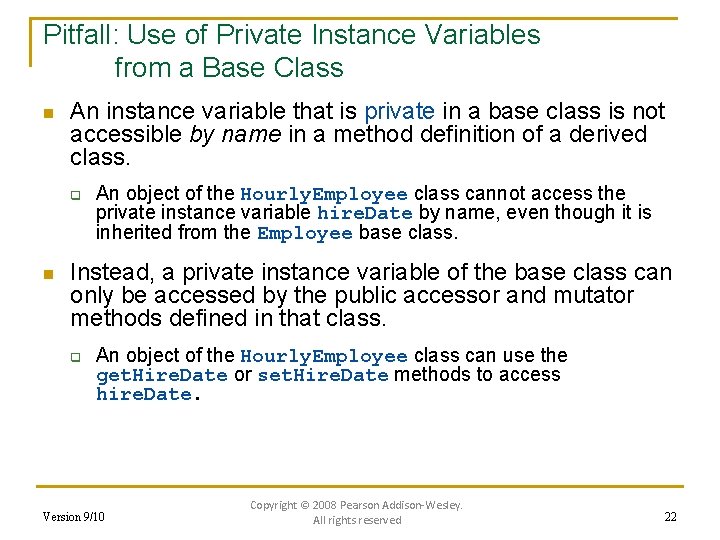
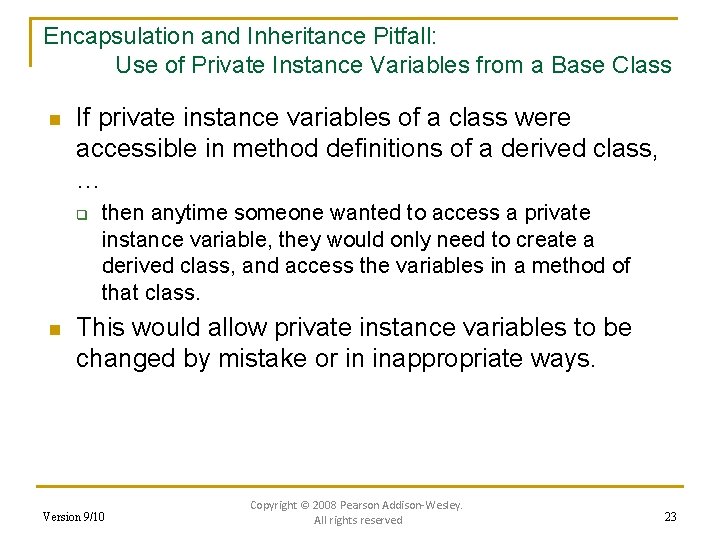
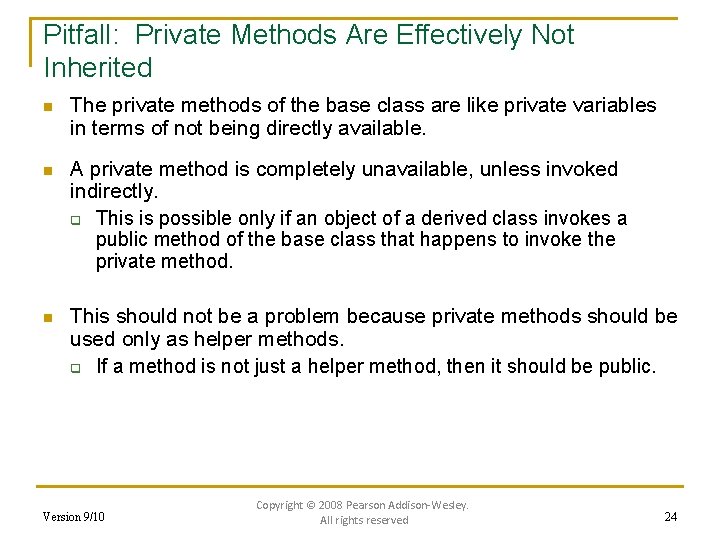
- Slides: 24
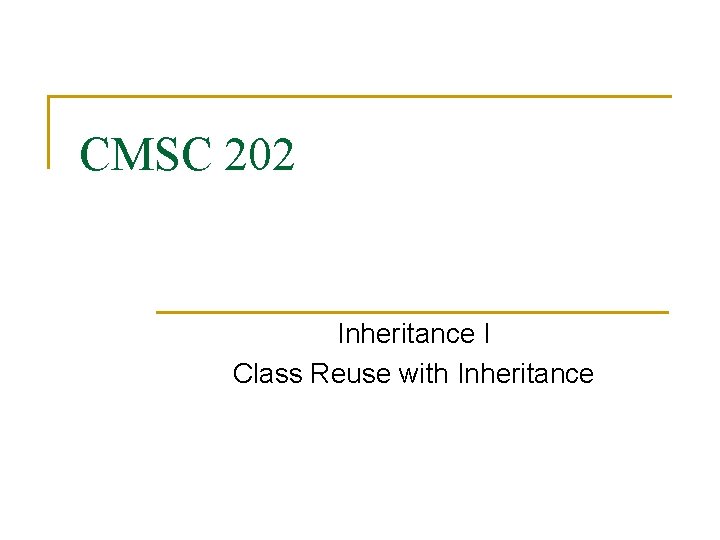
CMSC 202 Inheritance I Class Reuse with Inheritance
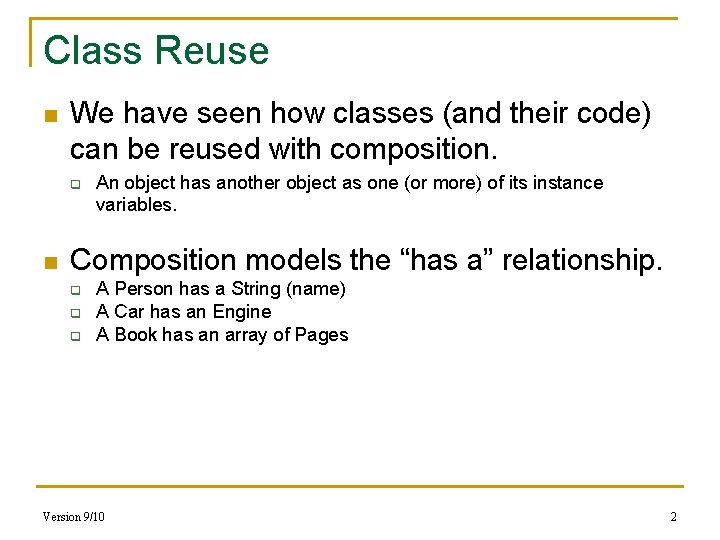
Class Reuse n We have seen how classes (and their code) can be reused with composition. q n An object has another object as one (or more) of its instance variables. Composition models the “has a” relationship. q q q A Person has a String (name) A Car has an Engine A Book has an array of Pages Version 9/10 2
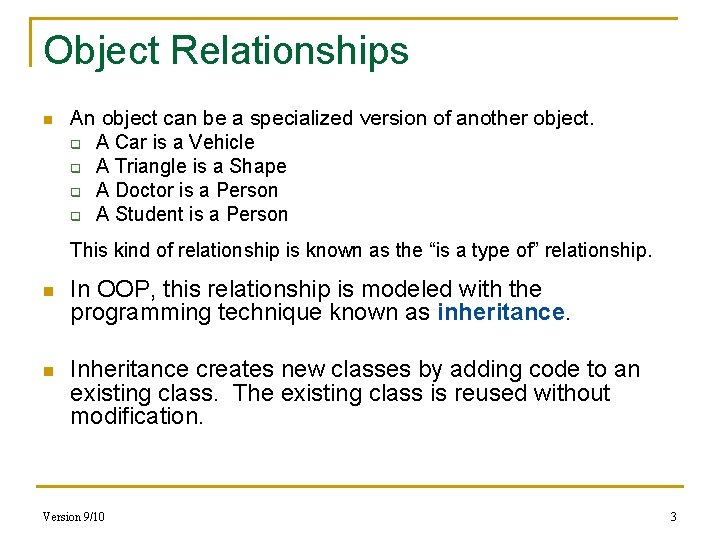
Object Relationships n An object can be a specialized version of another object. q A Car is a Vehicle q A Triangle is a Shape q A Doctor is a Person q A Student is a Person This kind of relationship is known as the “is a type of” relationship. n In OOP, this relationship is modeled with the programming technique known as inheritance. n Inheritance creates new classes by adding code to an existing class. The existing class is reused without modification. Version 9/10 3
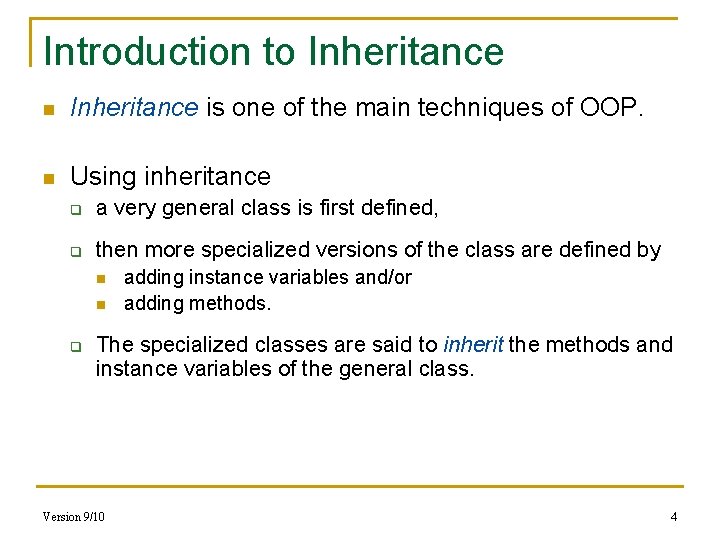
Introduction to Inheritance n Inheritance is one of the main techniques of OOP. n Using inheritance q a very general class is first defined, q then more specialized versions of the class are defined by n n q adding instance variables and/or adding methods. The specialized classes are said to inherit the methods and instance variables of the general class. Version 9/10 4
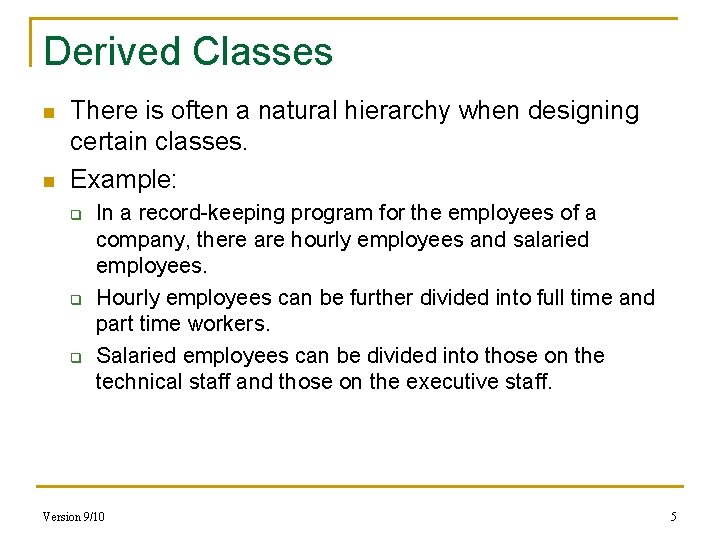
Derived Classes n n There is often a natural hierarchy when designing certain classes. Example: q q q In a record-keeping program for the employees of a company, there are hourly employees and salaried employees. Hourly employees can be further divided into full time and part time workers. Salaried employees can be divided into those on the technical staff and those on the executive staff. Version 9/10 5
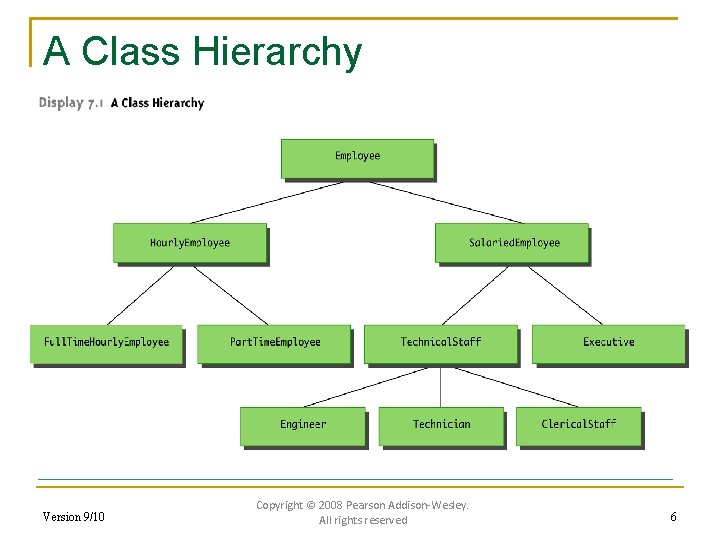
A Class Hierarchy Version 9/10 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 6
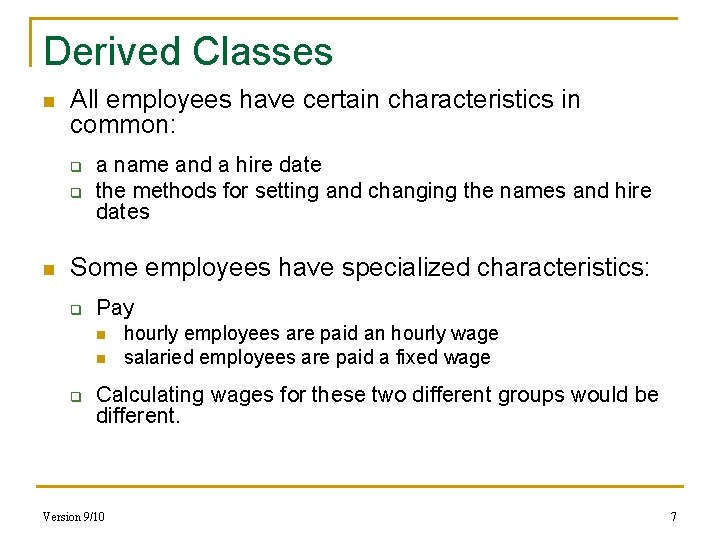
Derived Classes n All employees have certain characteristics in common: q q n a name and a hire date the methods for setting and changing the names and hire dates Some employees have specialized characteristics: q Pay n n q hourly employees are paid an hourly wage salaried employees are paid a fixed wage Calculating wages for these two different groups would be different. Version 9/10 7
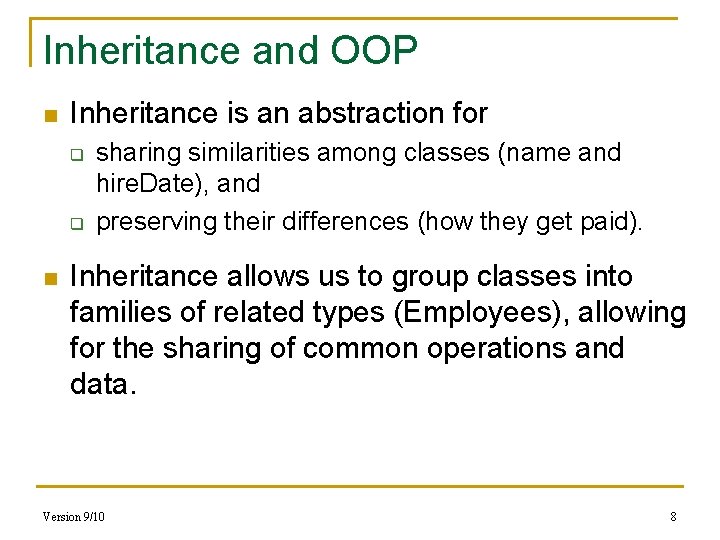
Inheritance and OOP n Inheritance is an abstraction for q q n sharing similarities among classes (name and hire. Date), and preserving their differences (how they get paid). Inheritance allows us to group classes into families of related types (Employees), allowing for the sharing of common operations and data. Version 9/10 8
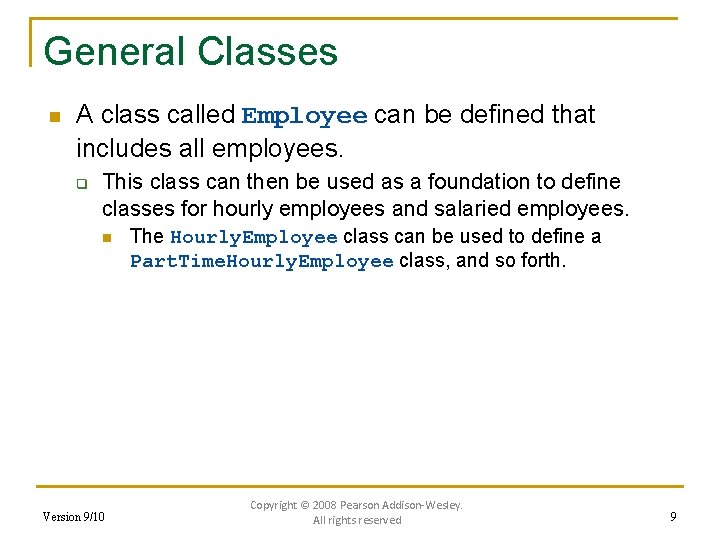
General Classes n A class called Employee can be defined that includes all employees. q This class can then be used as a foundation to define classes for hourly employees and salaried employees. n Version 9/10 The Hourly. Employee class can be used to define a Part. Time. Hourly. Employee class, and so forth. Copyright © 2008 Pearson Addison-Wesley. All rights reserved 9
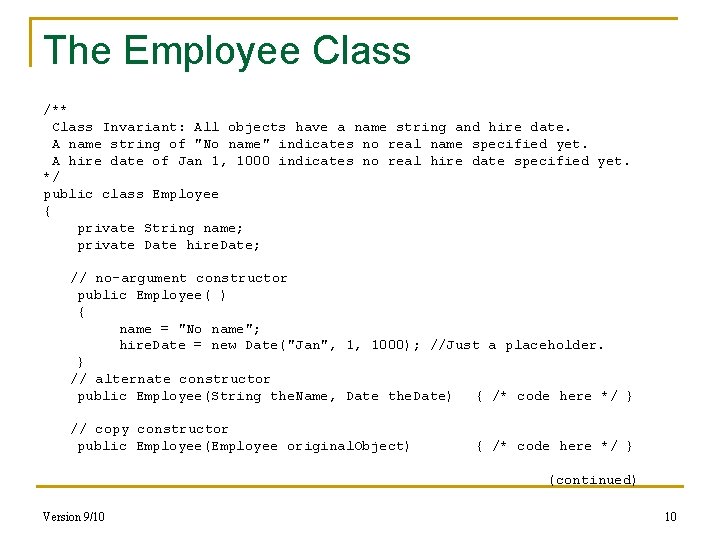
The Employee Class /** Class Invariant: All objects have a name string and hire date. A name string of "No name" indicates no real name specified yet. A hire date of Jan 1, 1000 indicates no real hire date specified yet. */ public class Employee { private String name; private Date hire. Date; // no-argument constructor public Employee( ) { name = "No name"; hire. Date = new Date("Jan", 1, 1000); //Just a placeholder. } // alternate constructor public Employee(String the. Name, Date the. Date) { /* code here */ } // copy constructor public Employee(Employee original. Object) { /* code here */ } (continued) Version 9/10 10
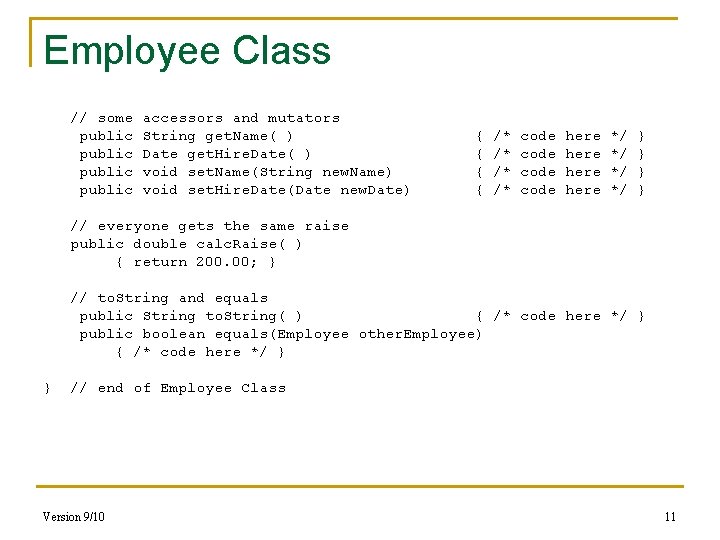
Employee Class // some public accessors and mutators String get. Name( ) Date get. Hire. Date( ) void set. Name(String new. Name) void set. Hire. Date(Date new. Date) { { /* /* code here */ */ } } // everyone gets the same raise public double calc. Raise( ) { return 200. 00; } // to. String and equals public String to. String( ) { /* code here */ } public boolean equals(Employee other. Employee) { /* code here */ } } // end of Employee Class Version 9/10 11
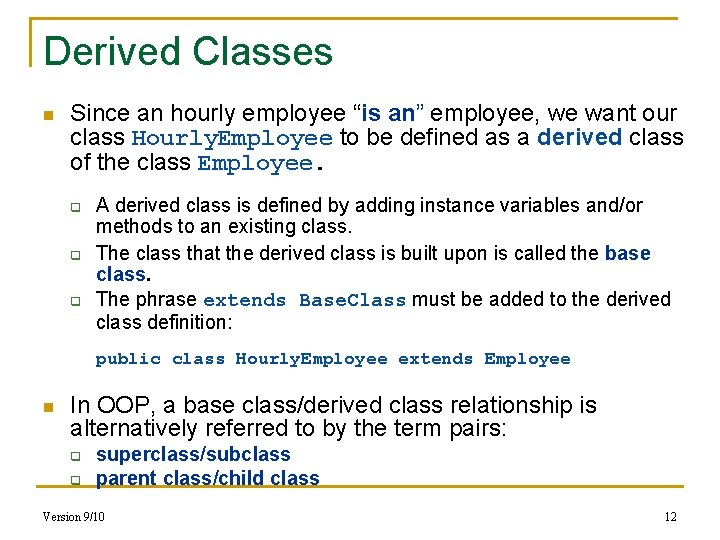
Derived Classes n Since an hourly employee “is an” employee, we want our class Hourly. Employee to be defined as a derived class of the class Employee. q q q A derived class is defined by adding instance variables and/or methods to an existing class. The class that the derived class is built upon is called the base class. The phrase extends Base. Class must be added to the derived class definition: public class Hourly. Employee extends Employee n In OOP, a base class/derived class relationship is alternatively referred to by the term pairs: q q superclass/subclass parent class/child class Version 9/10 12
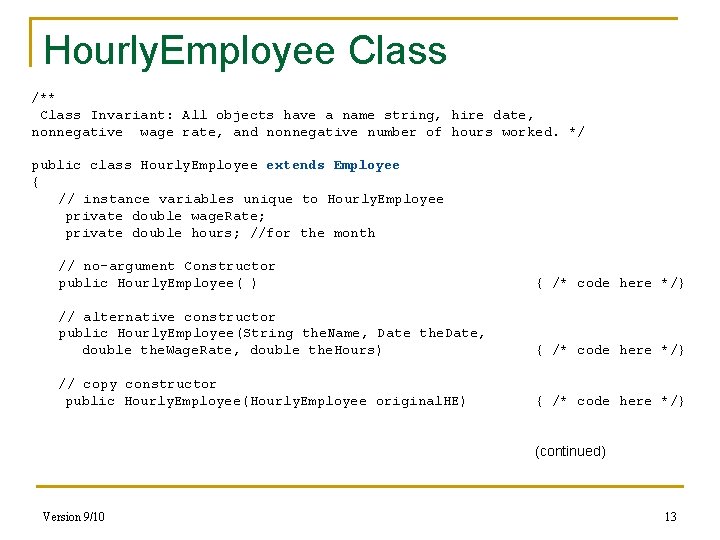
Hourly. Employee Class /** Class Invariant: All objects have a name string, hire date, nonnegative wage rate, and nonnegative number of hours worked. */ public class Hourly. Employee extends Employee { // instance variables unique to Hourly. Employee private double wage. Rate; private double hours; //for the month // no-argument Constructor public Hourly. Employee( ) { /* code here */} // alternative constructor public Hourly. Employee(String the. Name, Date the. Date, double the. Wage. Rate, double the. Hours) { /* code here */} // copy constructor public Hourly. Employee(Hourly. Employee original. HE) { /* code here */} (continued) Version 9/10 13
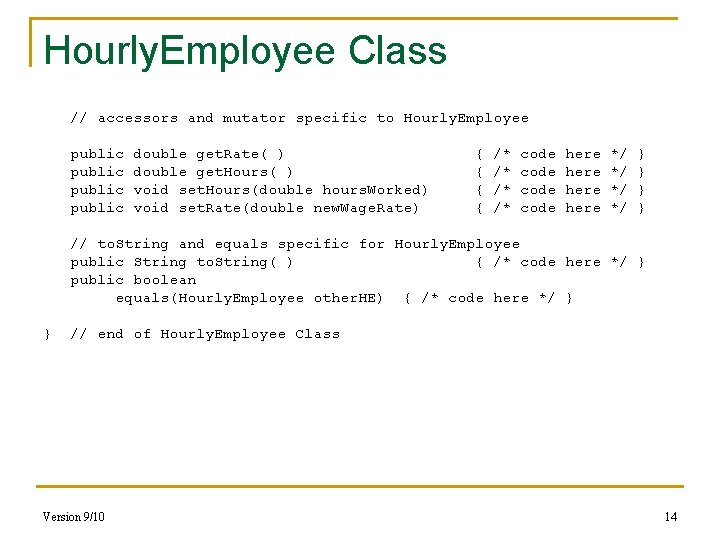
Hourly. Employee Class // accessors and mutator specific to Hourly. Employee public double get. Rate( ) double get. Hours( ) void set. Hours(double hours. Worked) void set. Rate(double new. Wage. Rate) { { /* /* code here */ */ } } // to. String and equals specific for Hourly. Employee public String to. String( ) { /* code here */ } public boolean equals(Hourly. Employee other. HE) { /* code here */ } } // end of Hourly. Employee Class Version 9/10 14
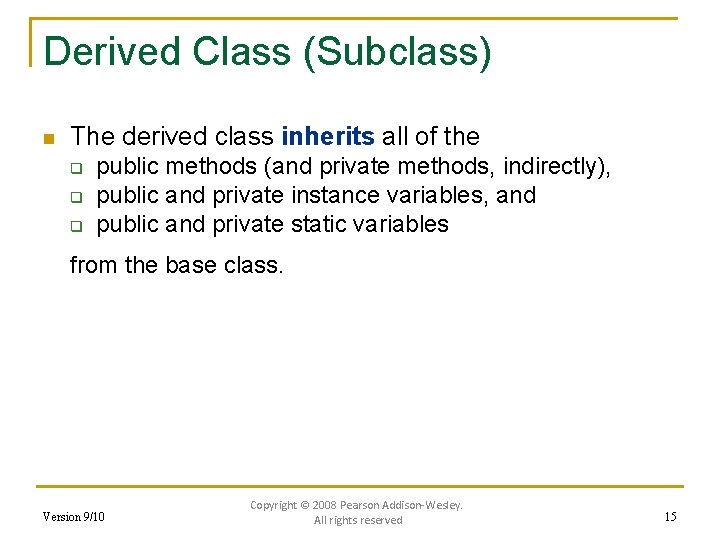
Derived Class (Subclass) n The derived class inherits all of the q q q public methods (and private methods, indirectly), public and private instance variables, and public and private static variables from the base class. Version 9/10 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 15
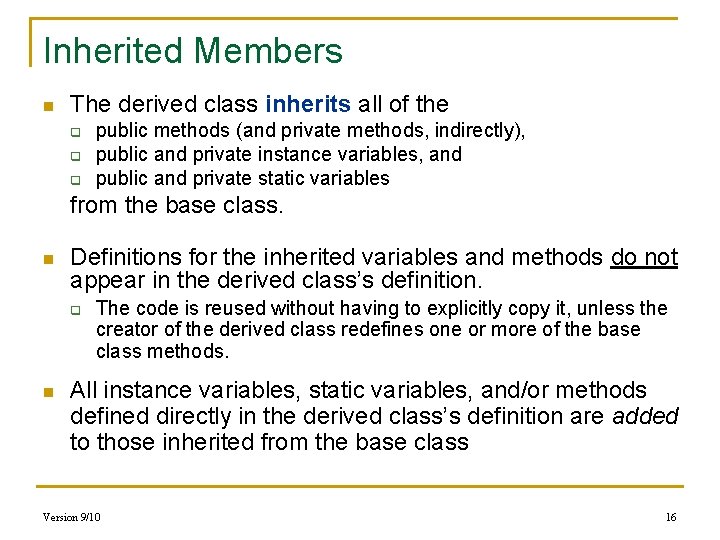
Inherited Members n The derived class inherits all of the q q q public methods (and private methods, indirectly), public and private instance variables, and public and private static variables from the base class. n Definitions for the inherited variables and methods do not appear in the derived class’s definition. q n The code is reused without having to explicitly copy it, unless the creator of the derived class redefines one or more of the base class methods. All instance variables, static variables, and/or methods defined directly in the derived class’s definition are added to those inherited from the base class Version 9/10 16
![Using Hourly Employee public class Hourly Employee Example public static void mainString args Using Hourly. Employee public class Hourly. Employee. Example { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/adf2986af2dbaf16a0b00676867029f1/image-17.jpg)
Using Hourly. Employee public class Hourly. Employee. Example { public static void main(String[] args) { Hourly. Employee joe = new Hourly. Employee("Joe Worker", new Date(1, 1, 2004), 50. 50, 160); // get. Name is defined in Employee System. out. println("joe's name is " + joe. get. Name( )); // set. Name is defined in Employee System. out. println("Changing joe's name to Josephine. "); joe. set. Name("Josephine"); // set. Rate is specific for Hourly. Employee System. out. println(“Giving Josephine a raise”); joe. set. Rate( 65. 00 ); // calc. Raise is defined in Employee double raise = joe. calc. Raise( ); System. out. println(“Joe’s raise is “ + raise ); } } Version 9/10 17
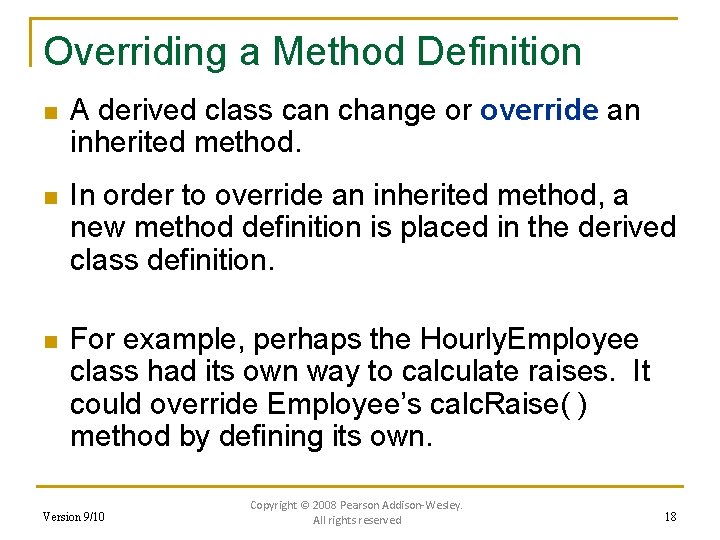
Overriding a Method Definition n A derived class can change or override an inherited method. n In order to override an inherited method, a new method definition is placed in the derived class definition. n For example, perhaps the Hourly. Employee class had its own way to calculate raises. It could override Employee’s calc. Raise( ) method by defining its own. Version 9/10 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 18
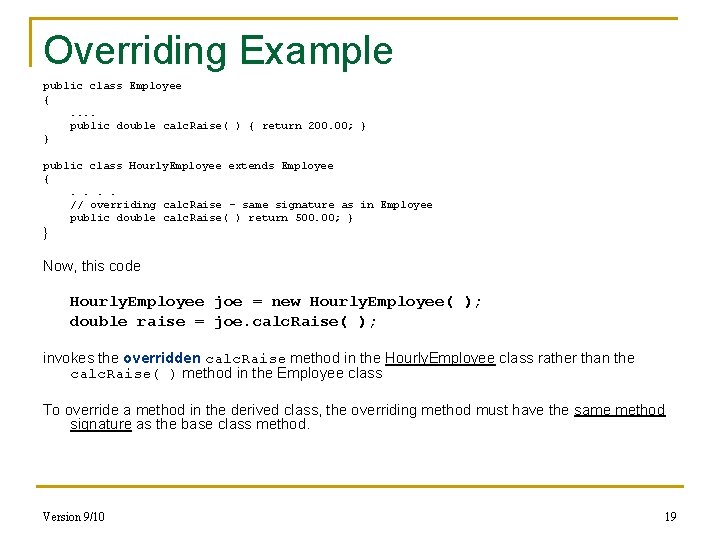
Overriding Example public class Employee {. . public double calc. Raise( ) { return 200. 00; } } public class Hourly. Employee extends Employee {. . // overriding calc. Raise – same signature as in Employee public double calc. Raise( ) return 500. 00; } } Now, this code Hourly. Employee joe = new Hourly. Employee( ); double raise = joe. calc. Raise( ); invokes the overridden calc. Raise method in the Hourly. Employee class rather than the calc. Raise( ) method in the Employee class To override a method in the derived class, the overriding method must have the same method signature as the base class method. Version 9/10 19
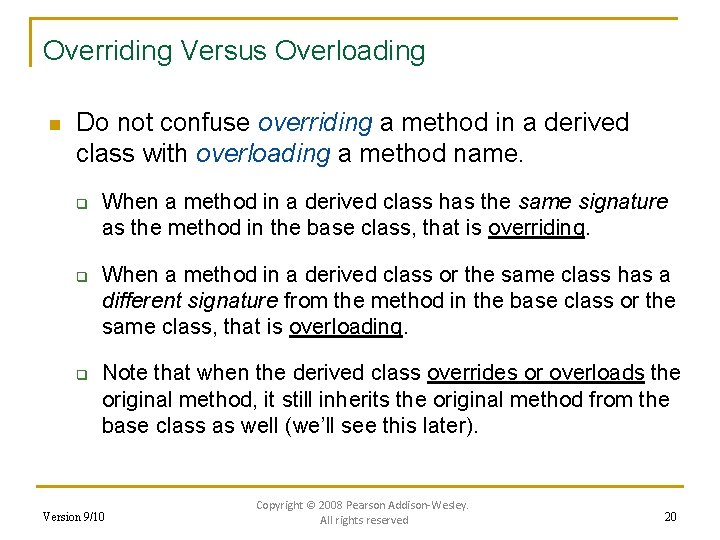
Overriding Versus Overloading n Do not confuse overriding a method in a derived class with overloading a method name. q q q When a method in a derived class has the same signature as the method in the base class, that is overriding. When a method in a derived class or the same class has a different signature from the method in the base class or the same class, that is overloading. Note that when the derived class overrides or overloads the original method, it still inherits the original method from the base class as well (we’ll see this later). Version 9/10 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 20
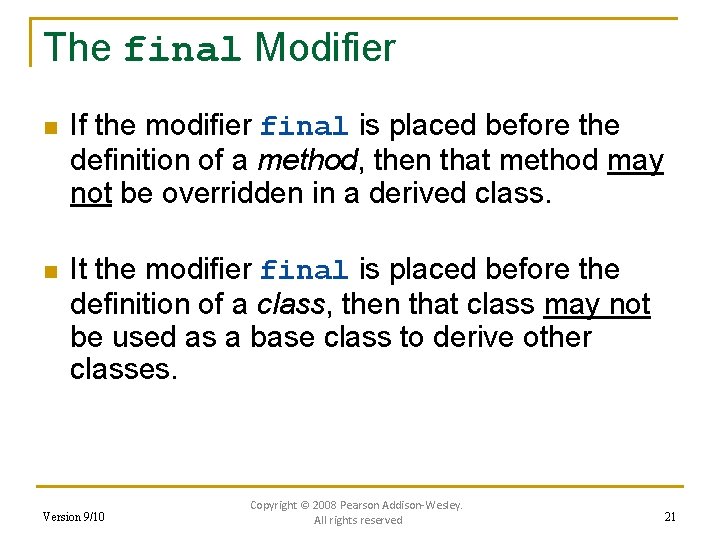
The final Modifier n If the modifier final is placed before the definition of a method, then that method may not be overridden in a derived class. n It the modifier final is placed before the definition of a class, then that class may not be used as a base class to derive other classes. Version 9/10 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 21
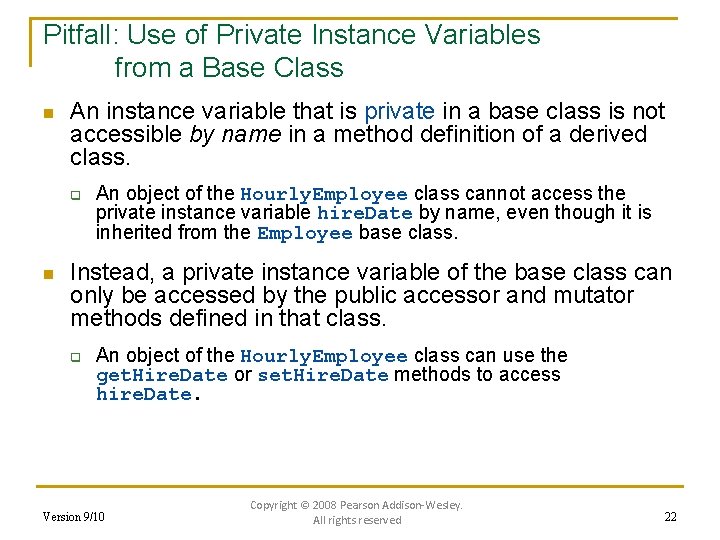
Pitfall: Use of Private Instance Variables from a Base Class n An instance variable that is private in a base class is not accessible by name in a method definition of a derived class. q n An object of the Hourly. Employee class cannot access the private instance variable hire. Date by name, even though it is inherited from the Employee base class. Instead, a private instance variable of the base class can only be accessed by the public accessor and mutator methods defined in that class. q An object of the Hourly. Employee class can use the get. Hire. Date or set. Hire. Date methods to access hire. Date. Version 9/10 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 22
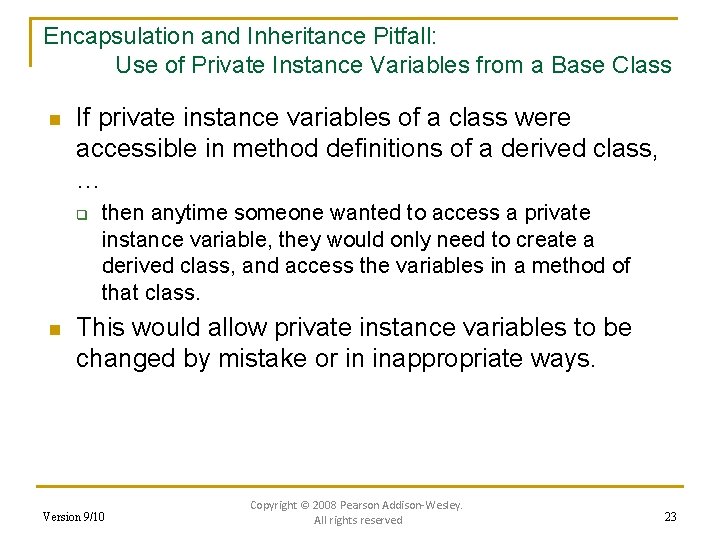
Encapsulation and Inheritance Pitfall: Use of Private Instance Variables from a Base Class n If private instance variables of a class were accessible in method definitions of a derived class, … q n then anytime someone wanted to access a private instance variable, they would only need to create a derived class, and access the variables in a method of that class. This would allow private instance variables to be changed by mistake or in inappropriate ways. Version 9/10 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 23
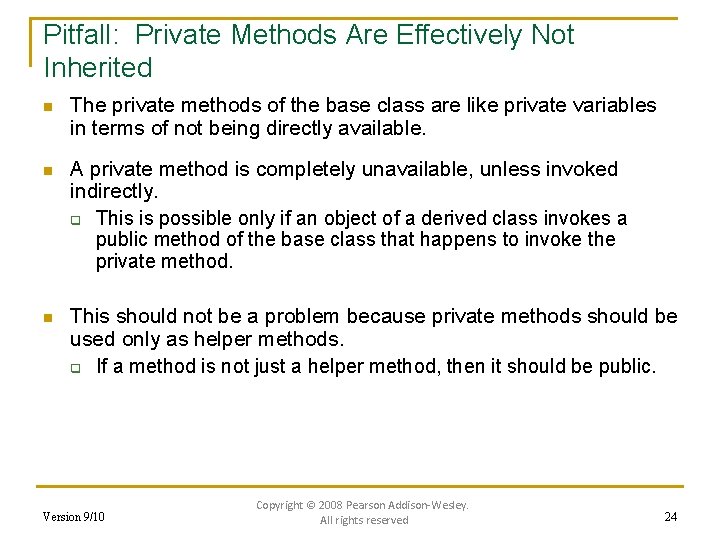
Pitfall: Private Methods Are Effectively Not Inherited n The private methods of the base class are like private variables in terms of not being directly available. n A private method is completely unavailable, unless invoked indirectly. q This is possible only if an object of a derived class invokes a public method of the base class that happens to invoke the private method. n This should not be a problem because private methods should be used only as helper methods. q If a method is not just a helper method, then it should be public. Version 9/10 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 24