ClientSide programming with Java Script 2 Eventdriven programs
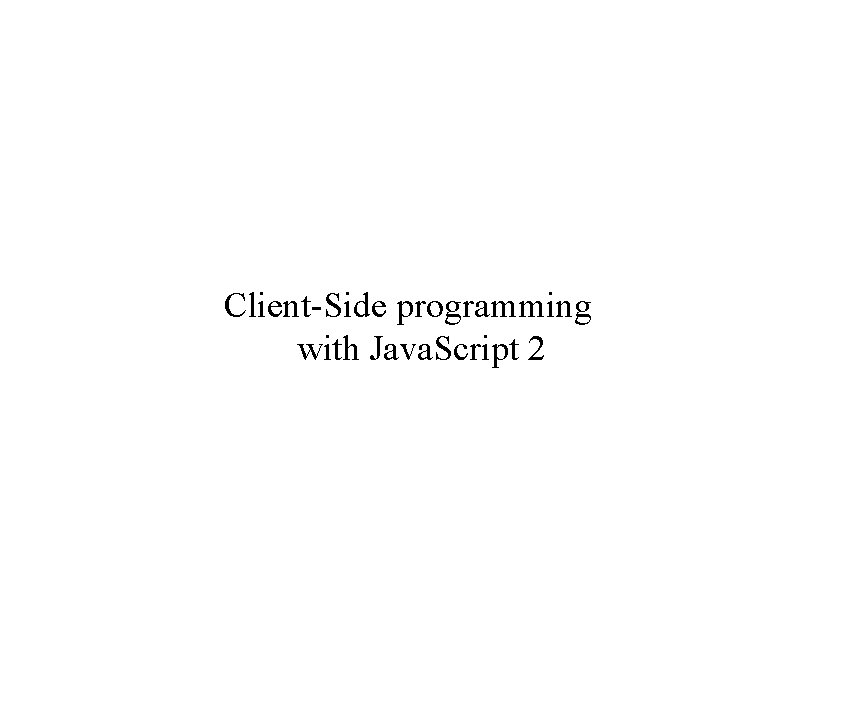
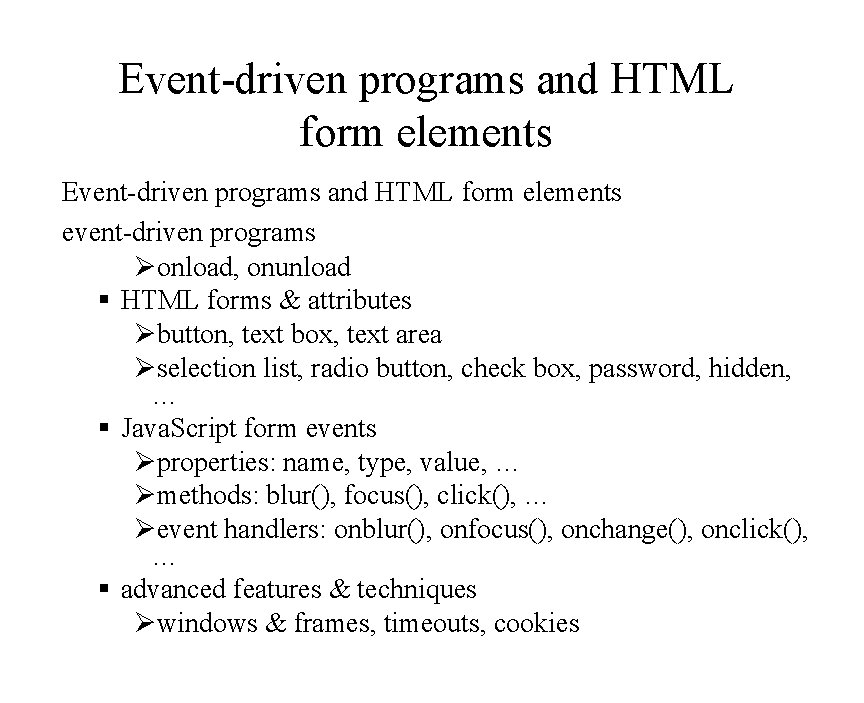
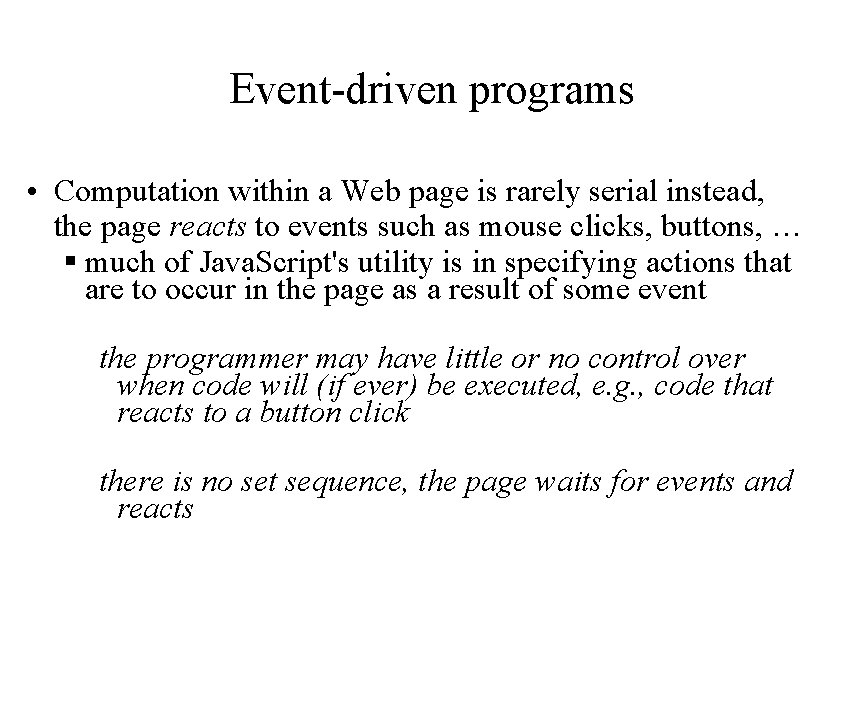
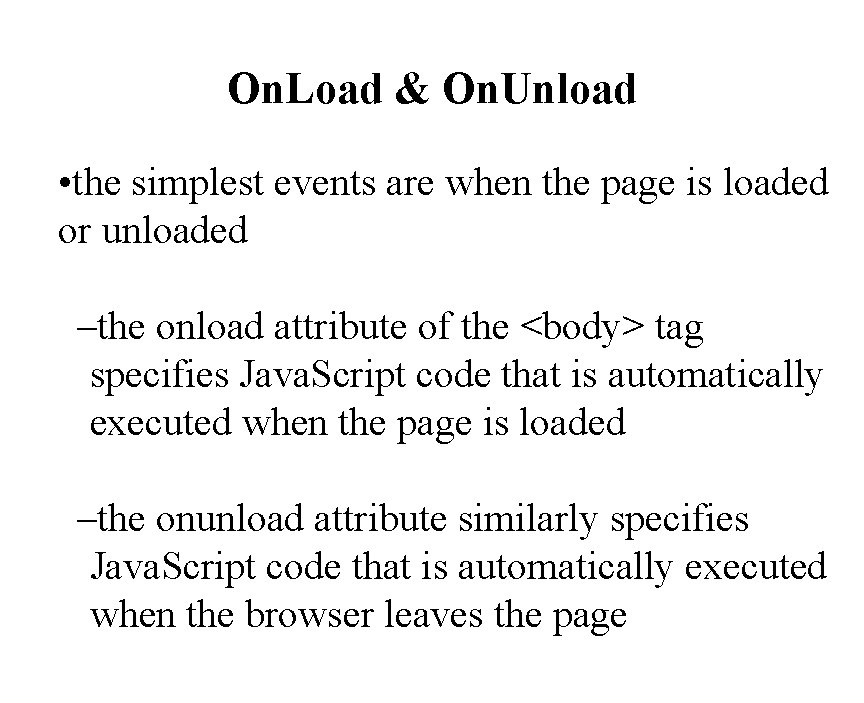
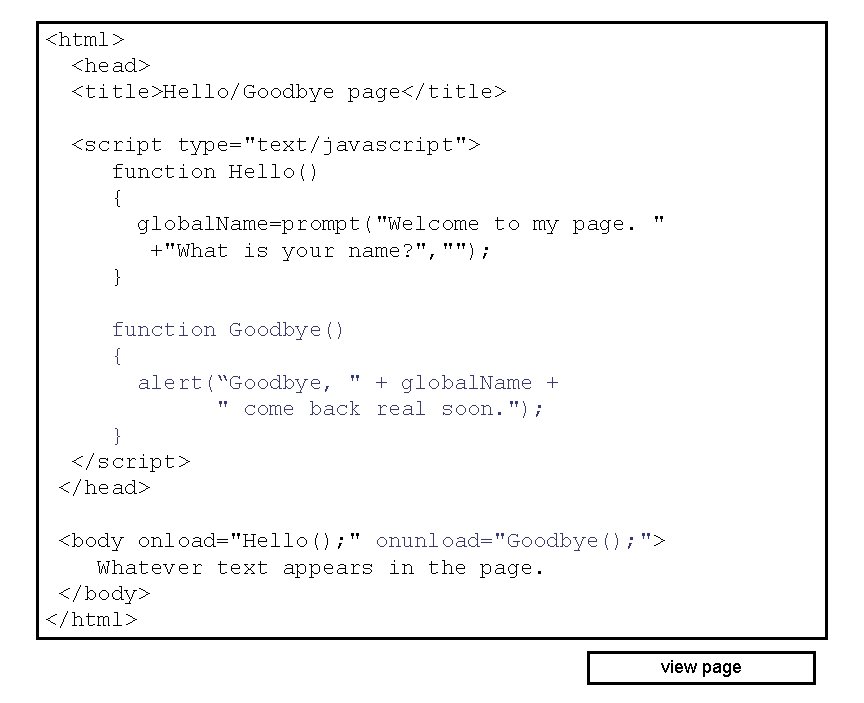
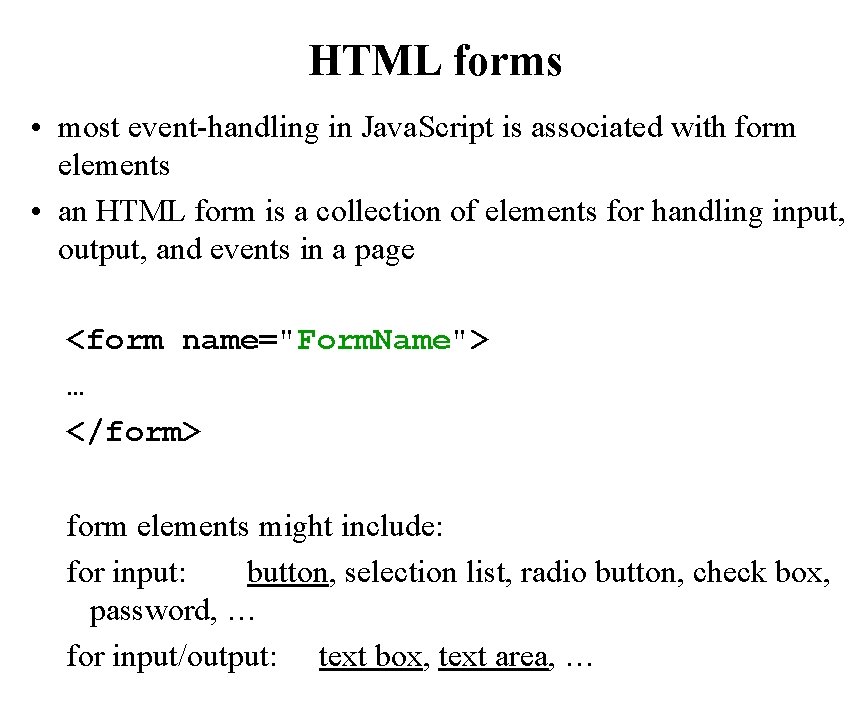
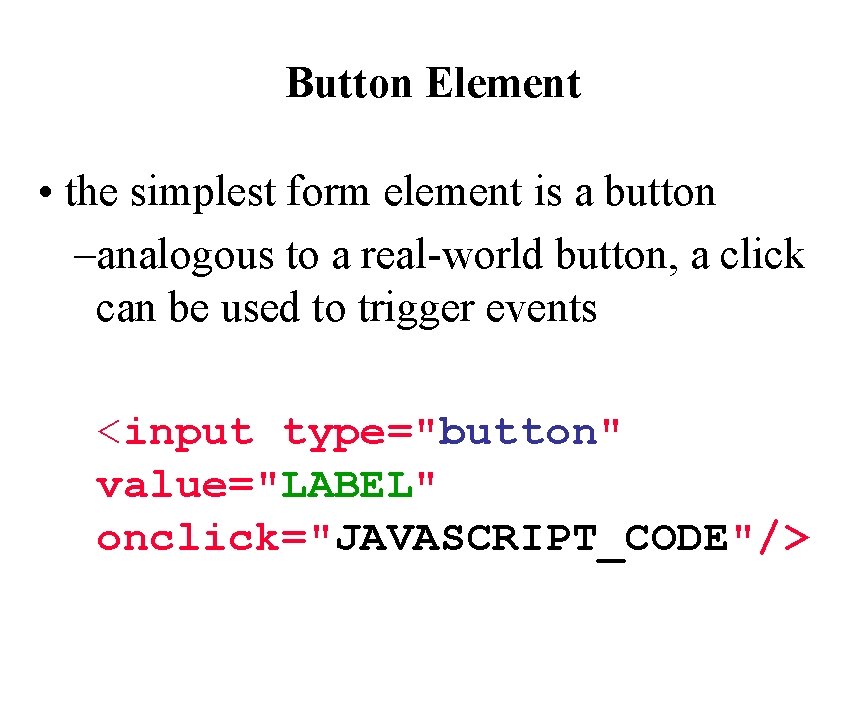
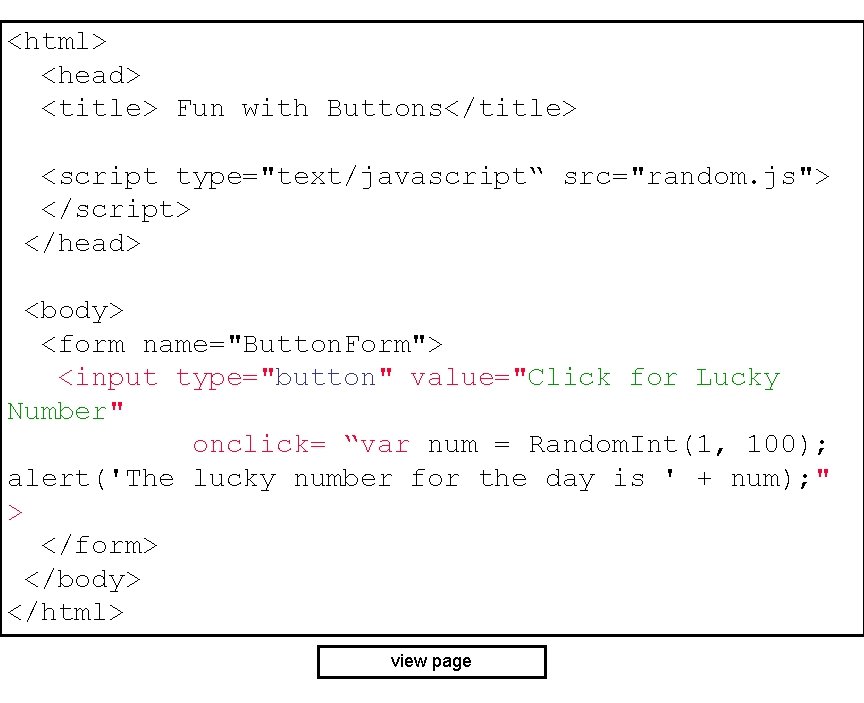
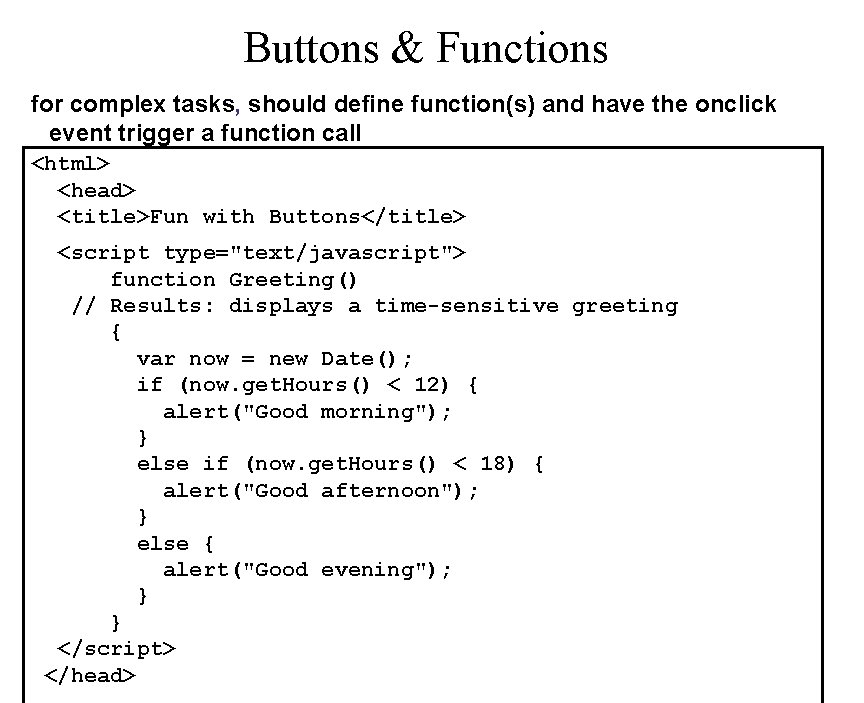
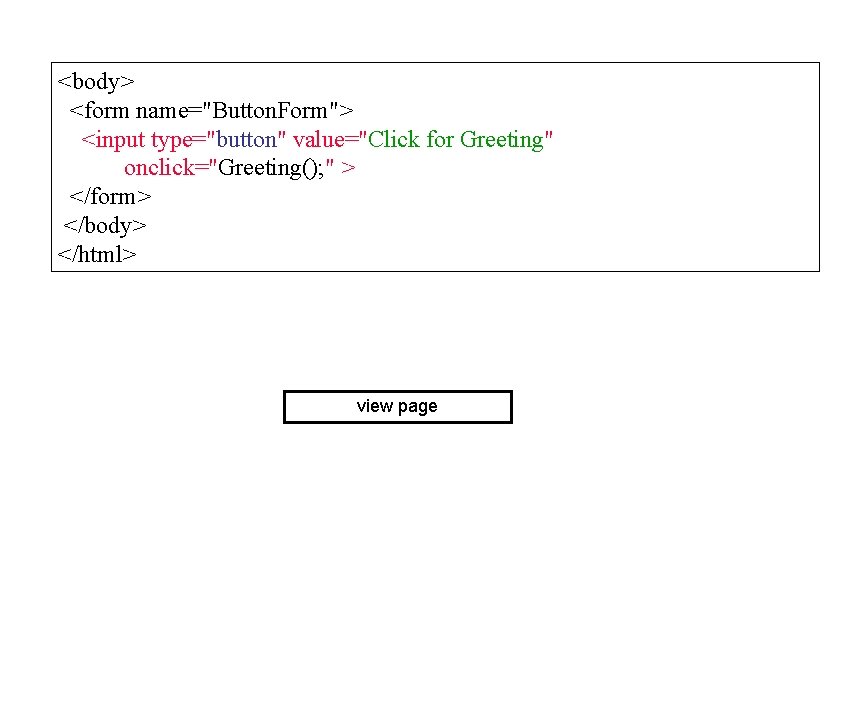
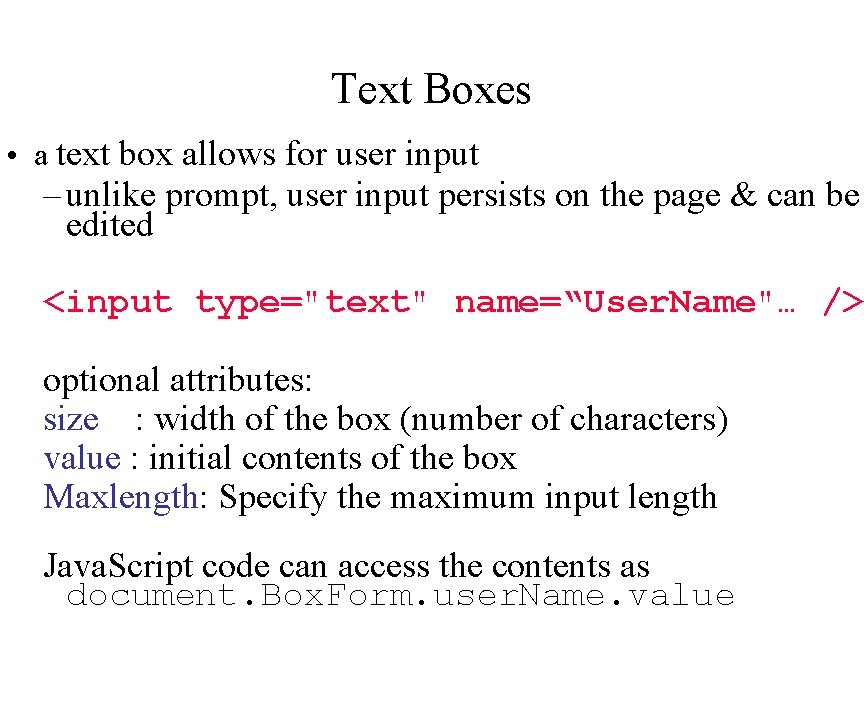
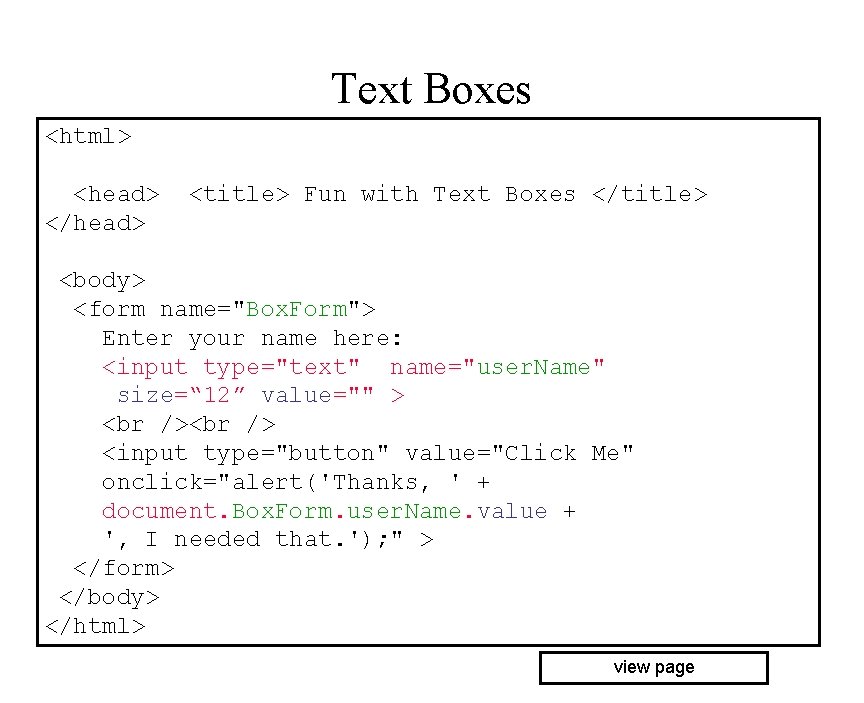
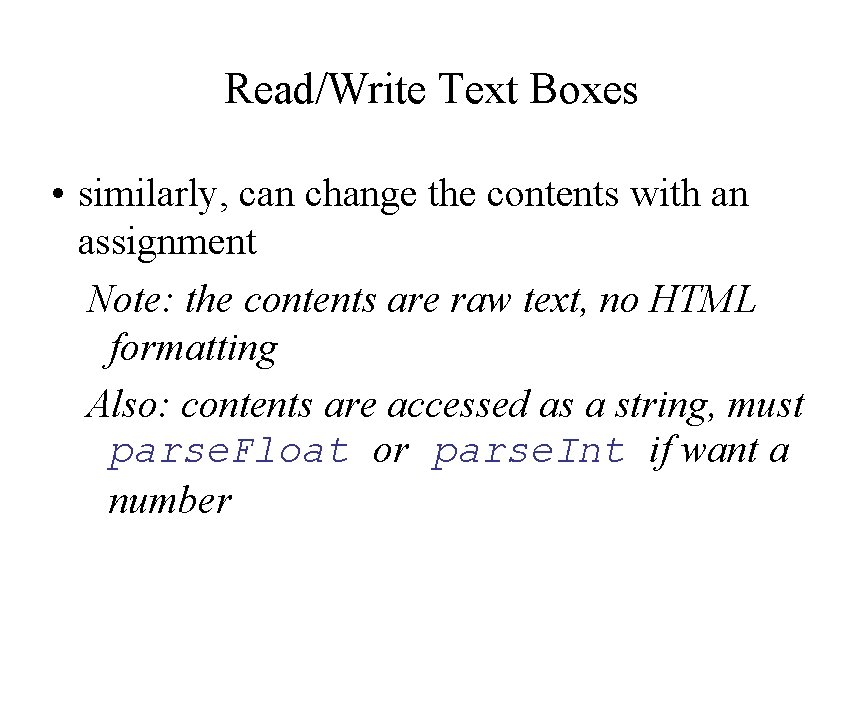
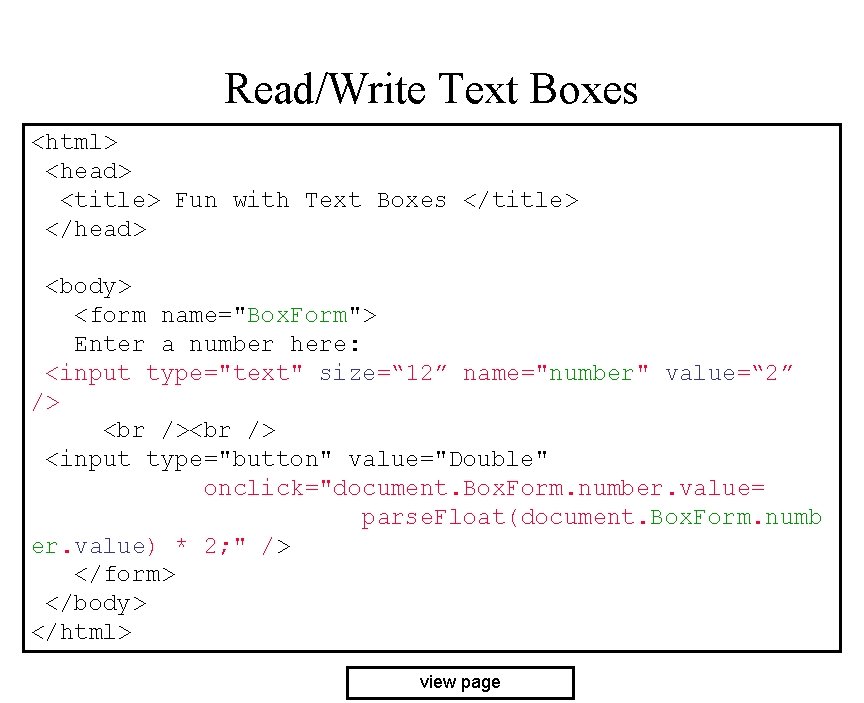
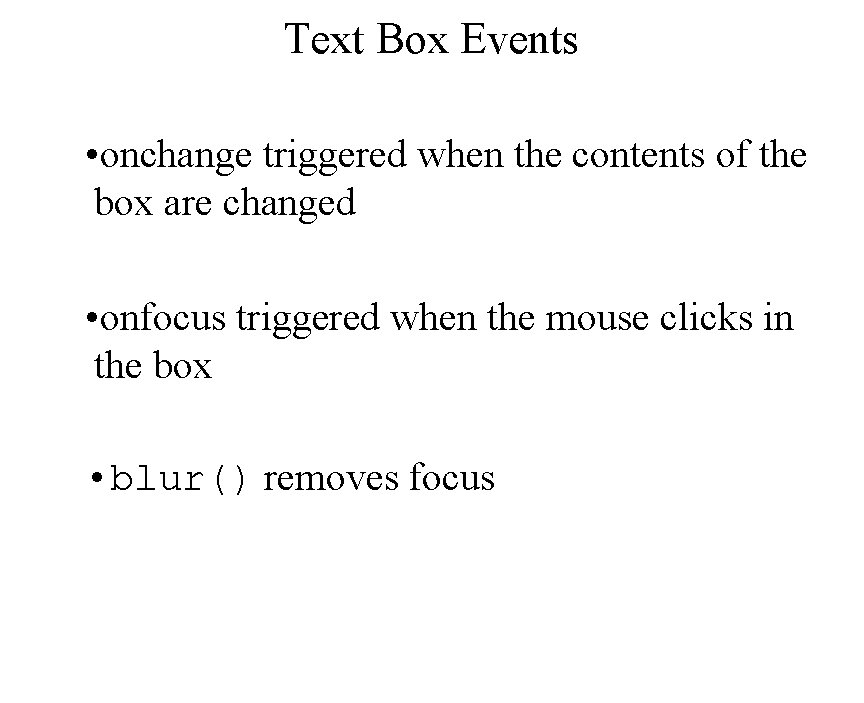
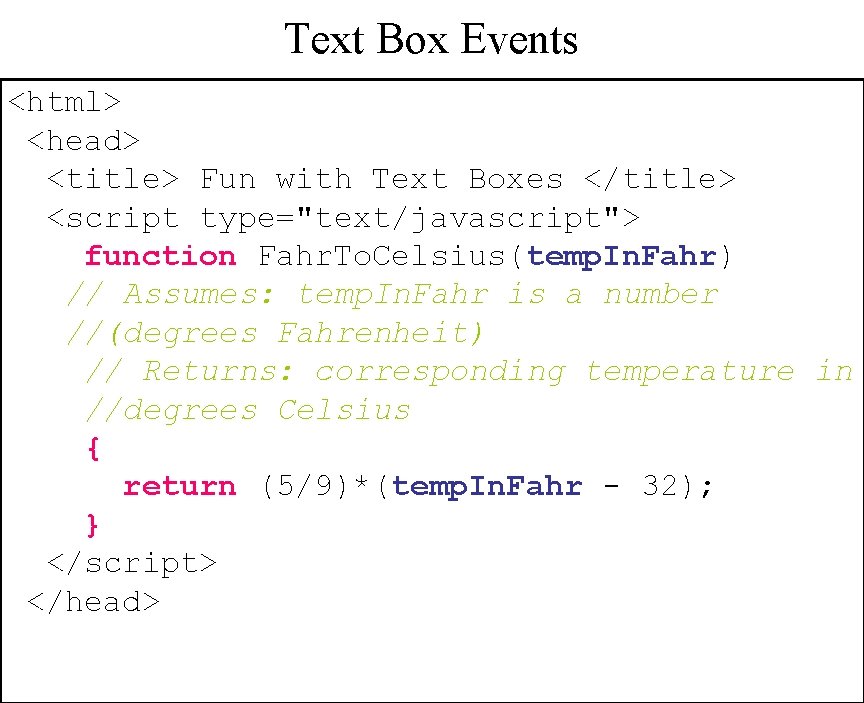
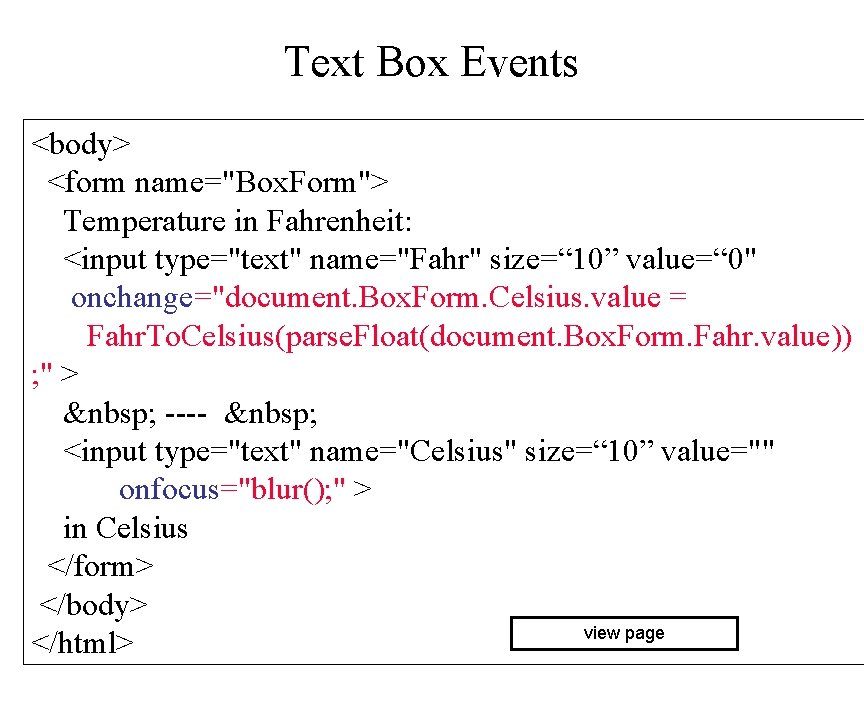
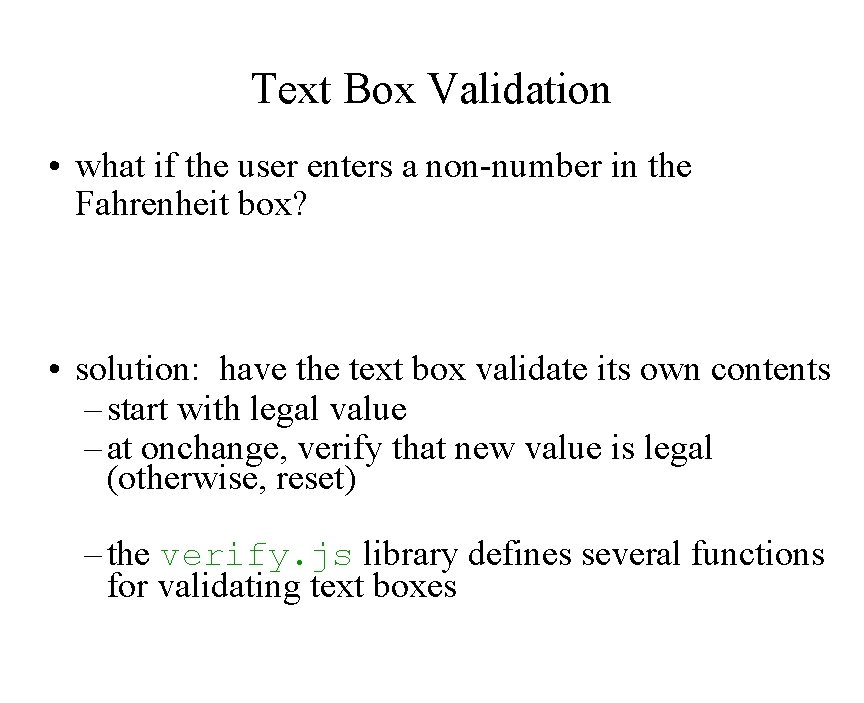
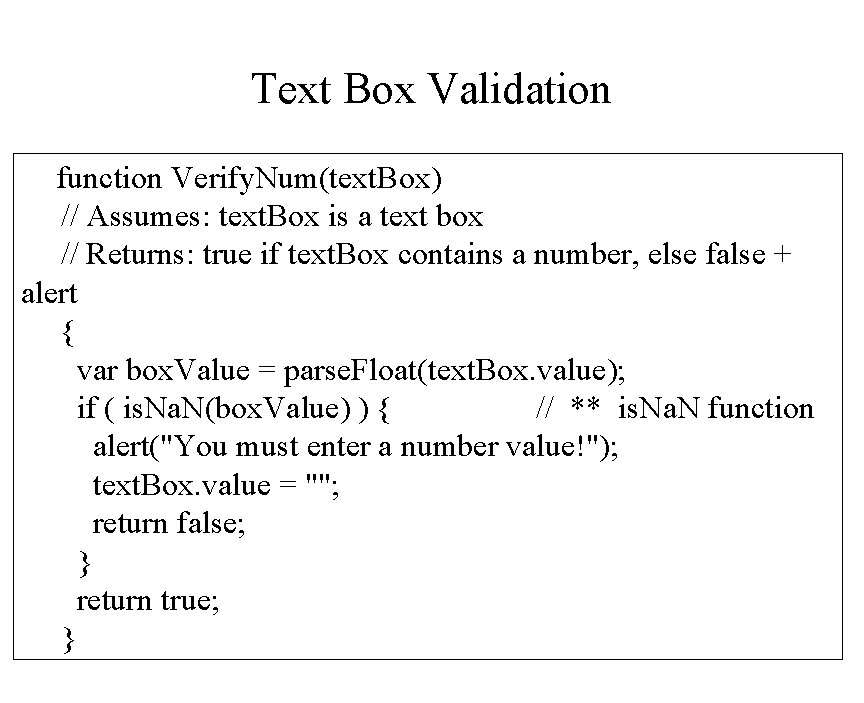
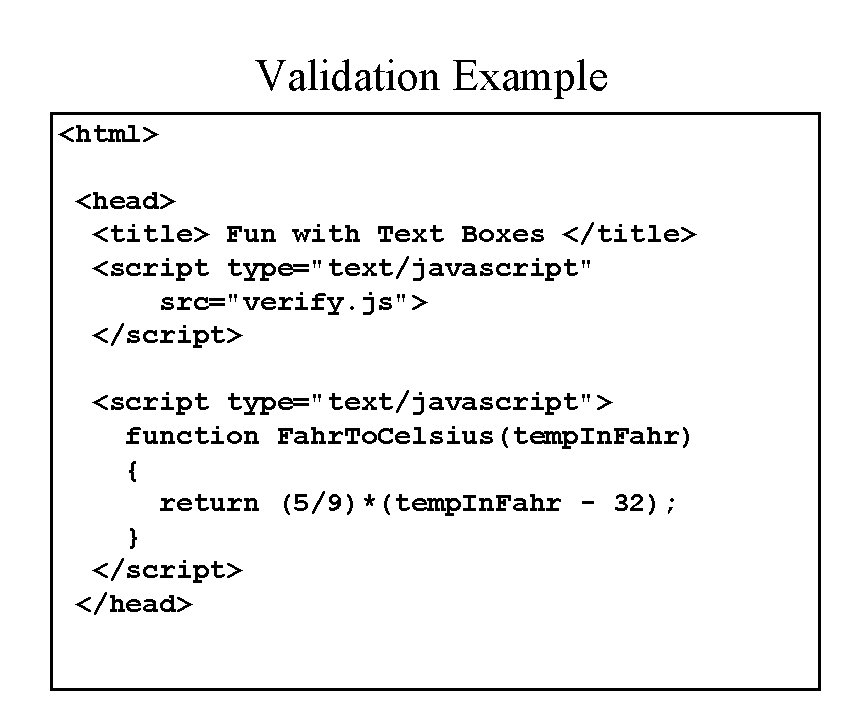
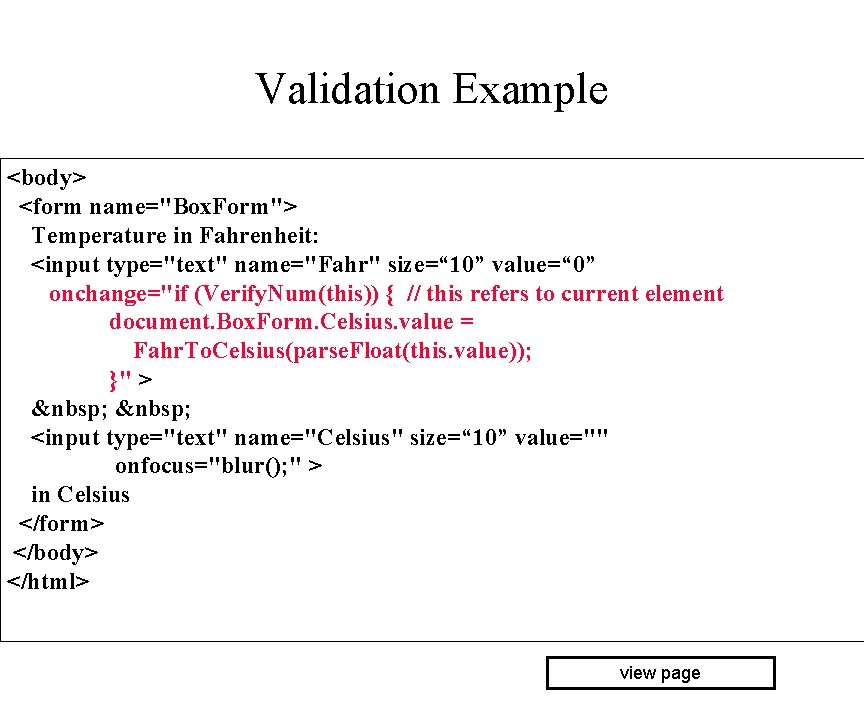
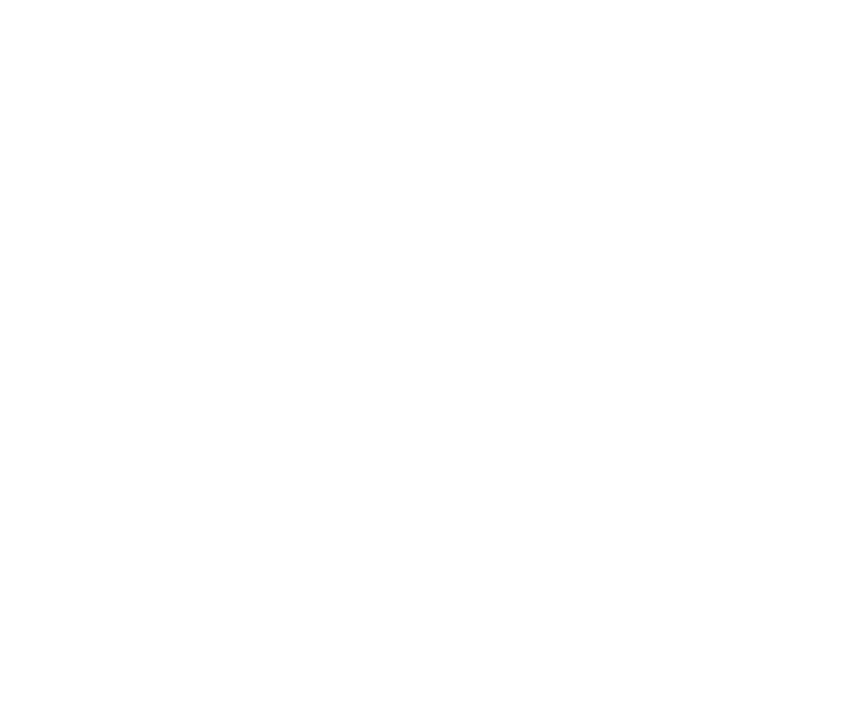
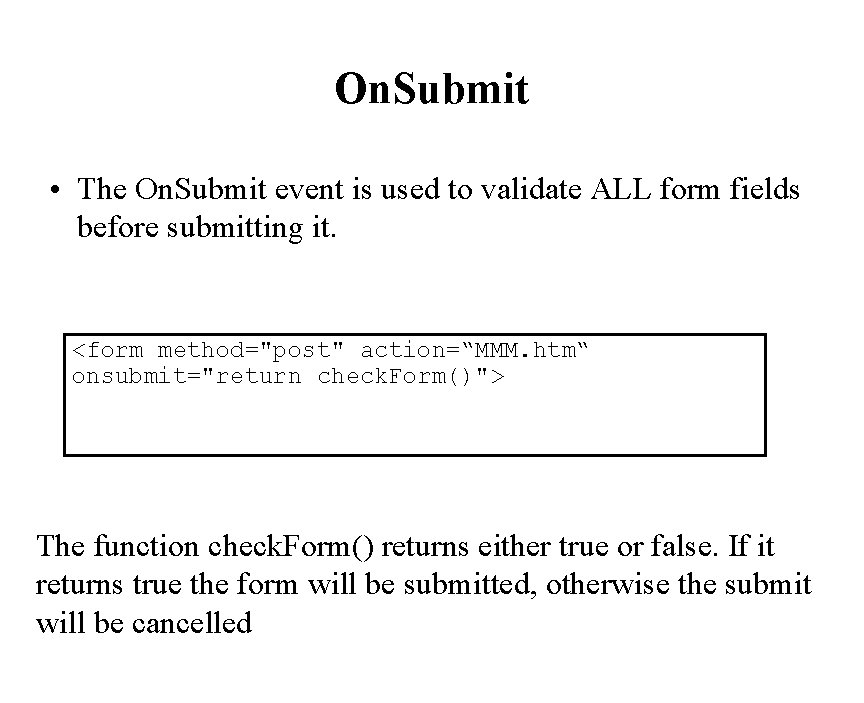
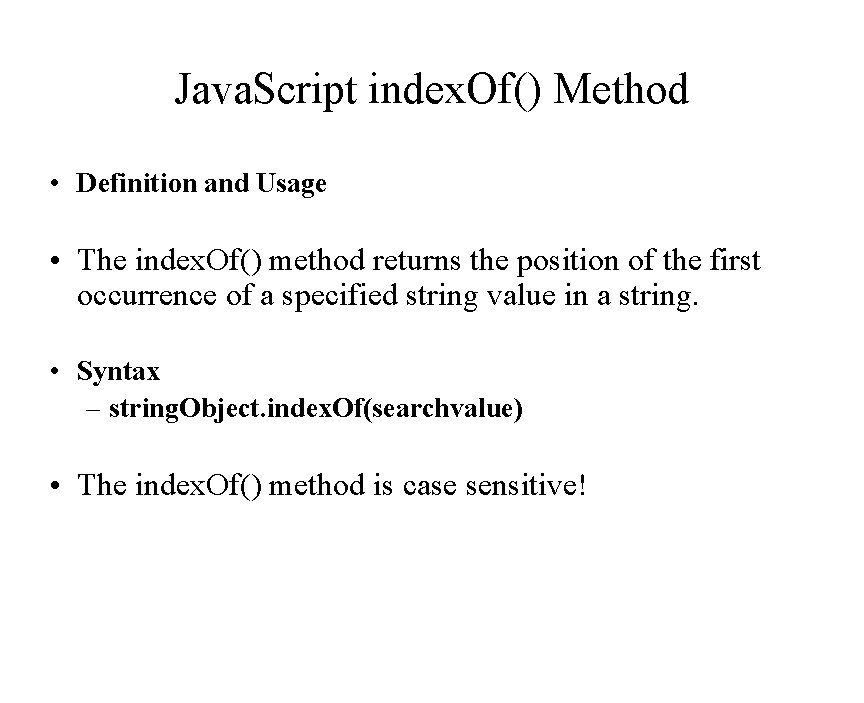
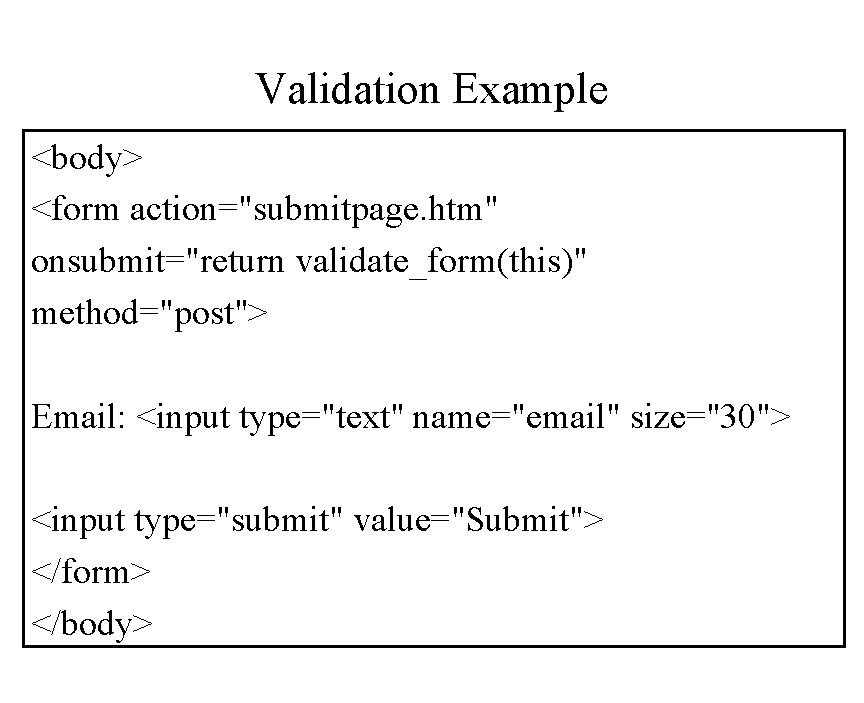
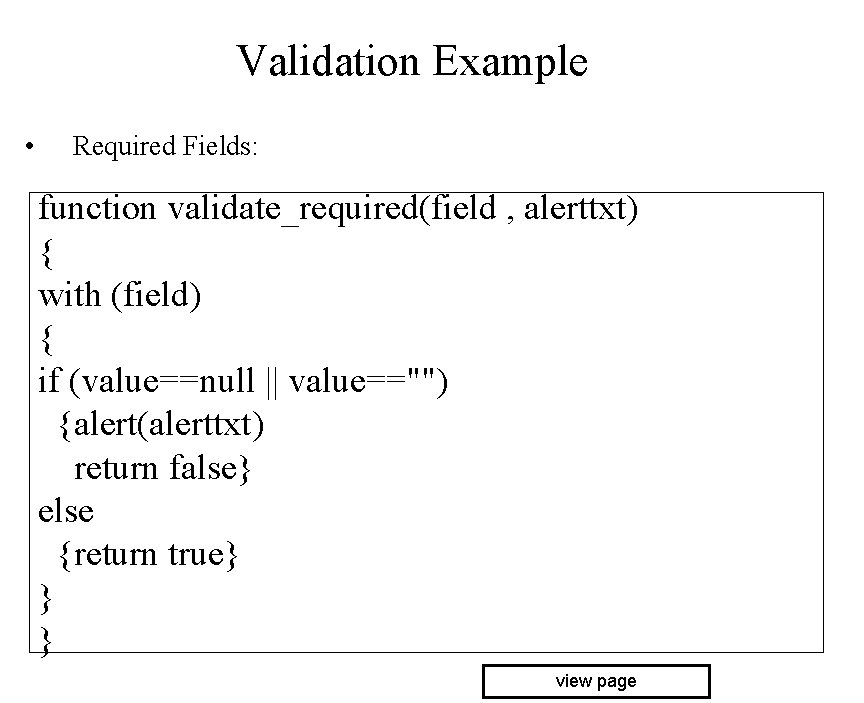
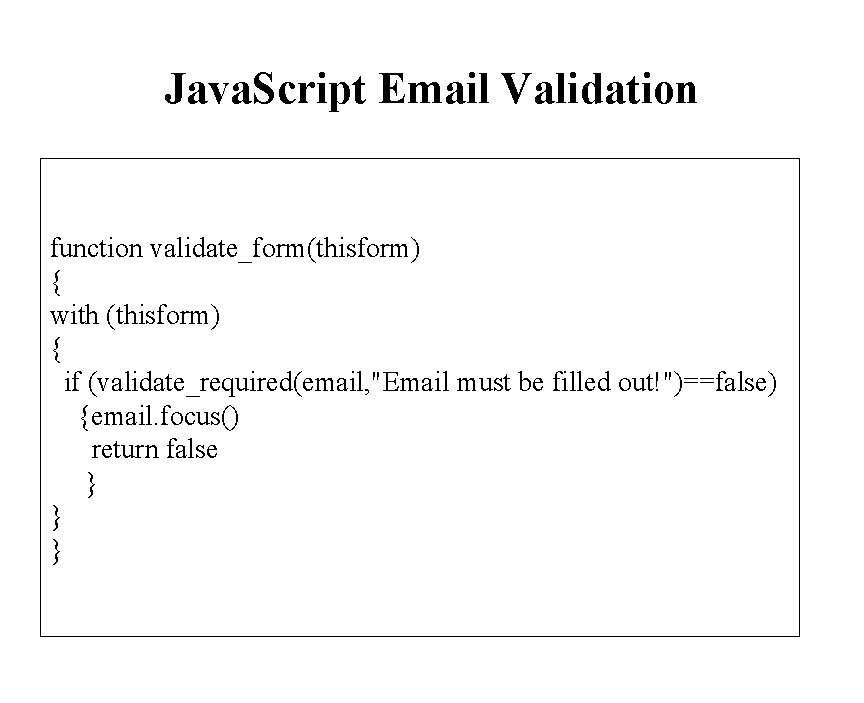
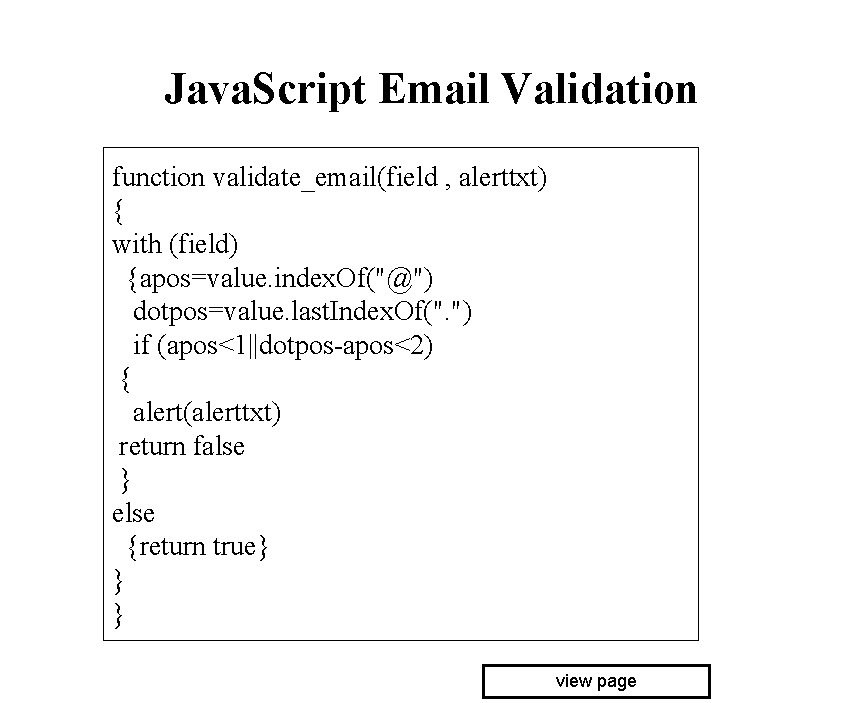
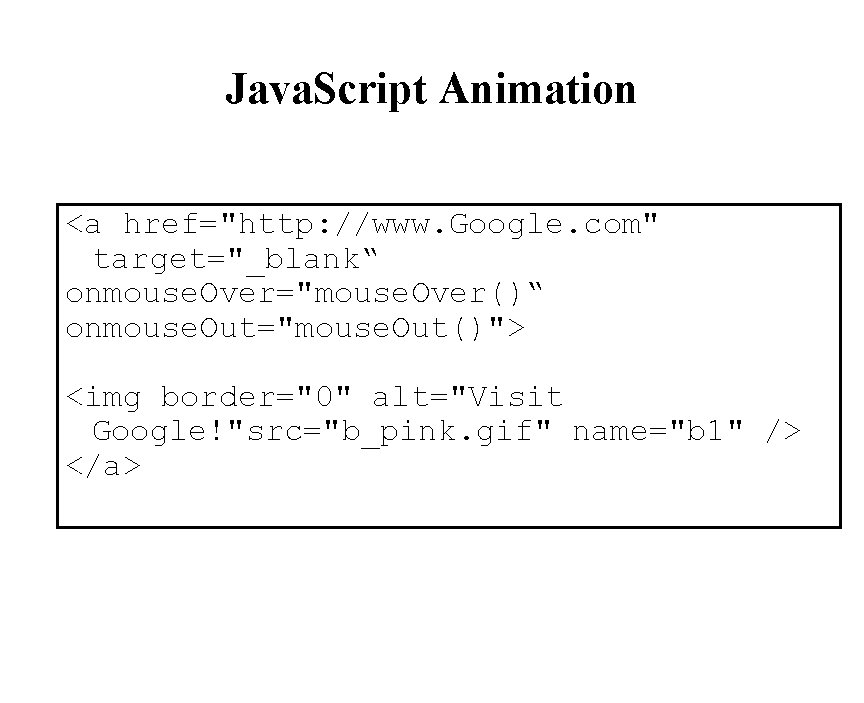
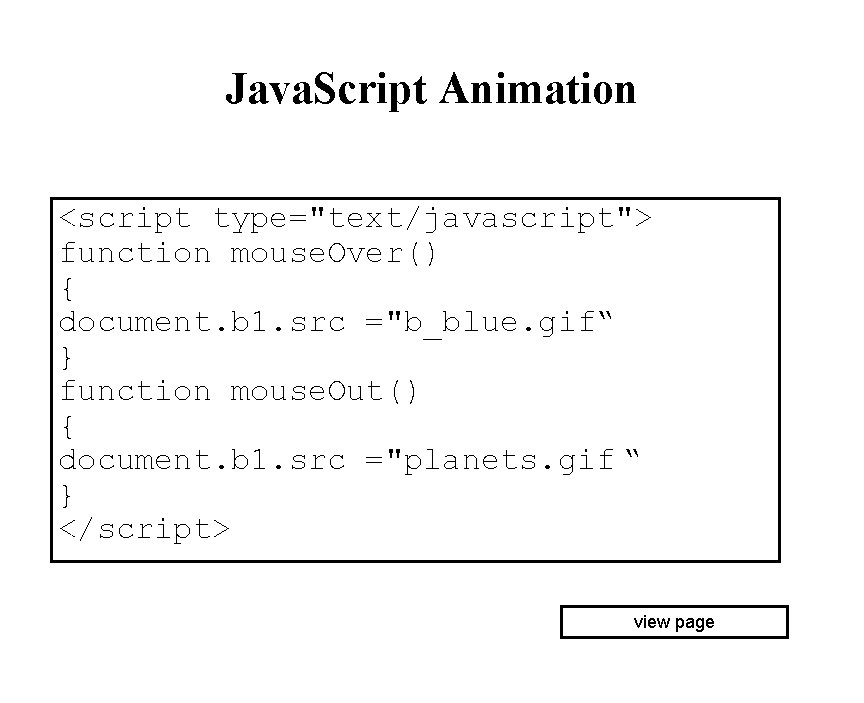
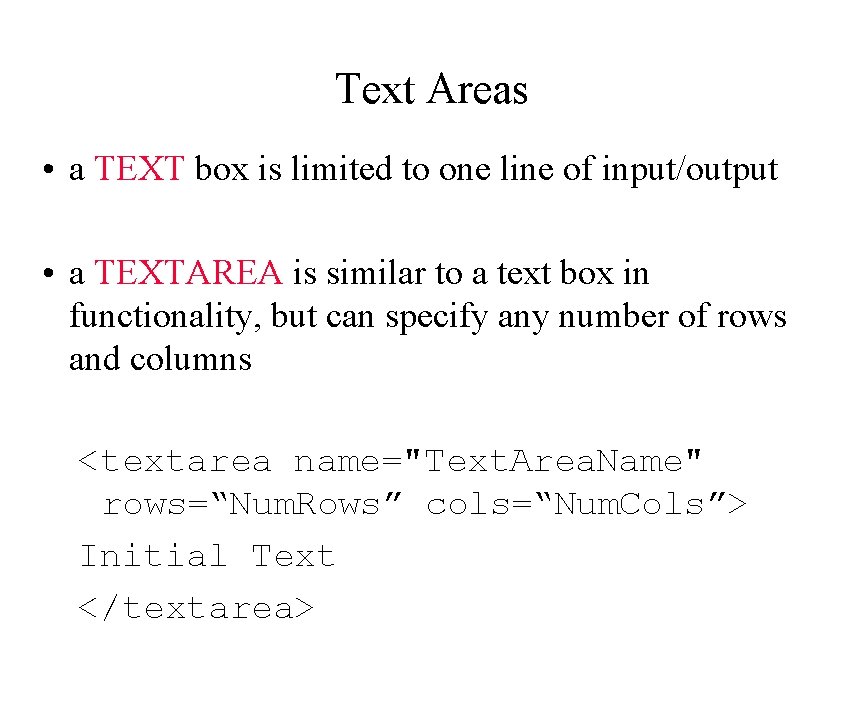
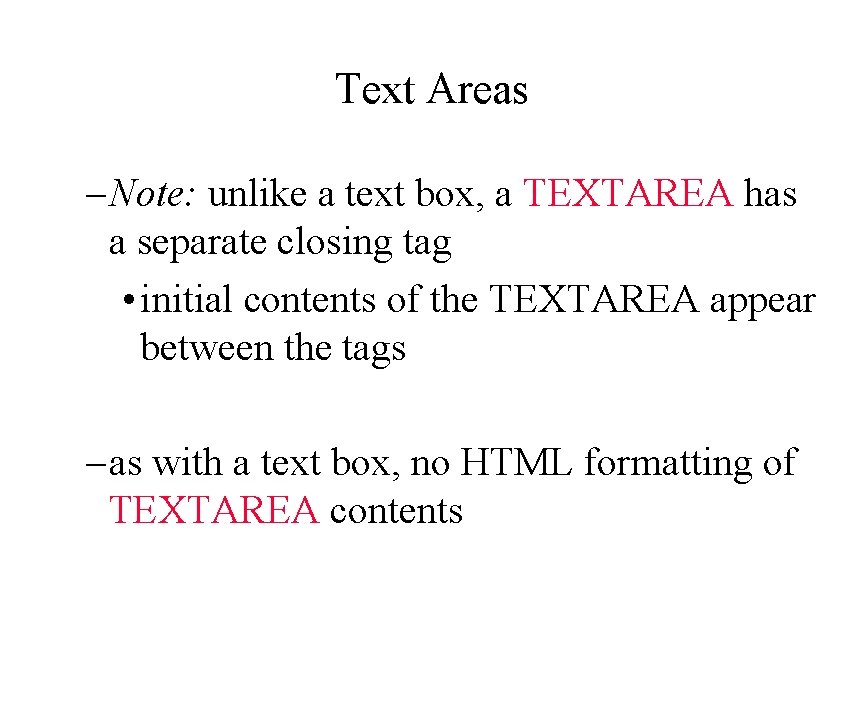
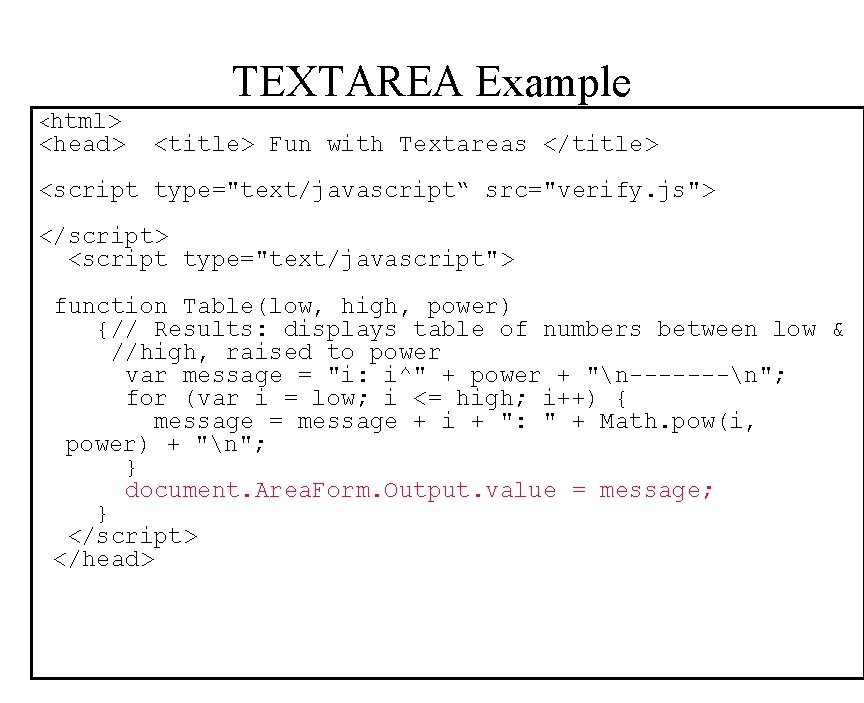
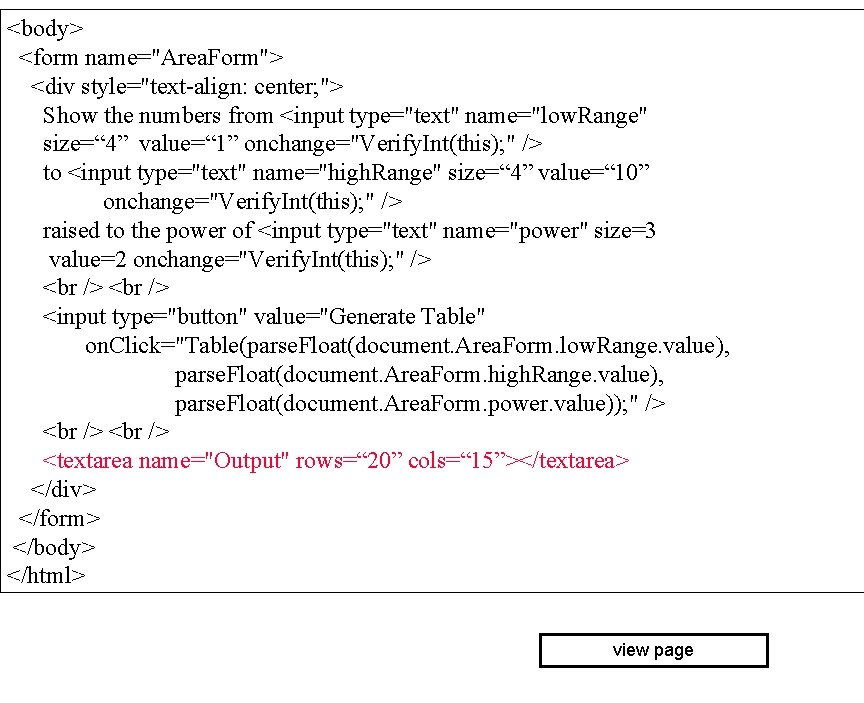
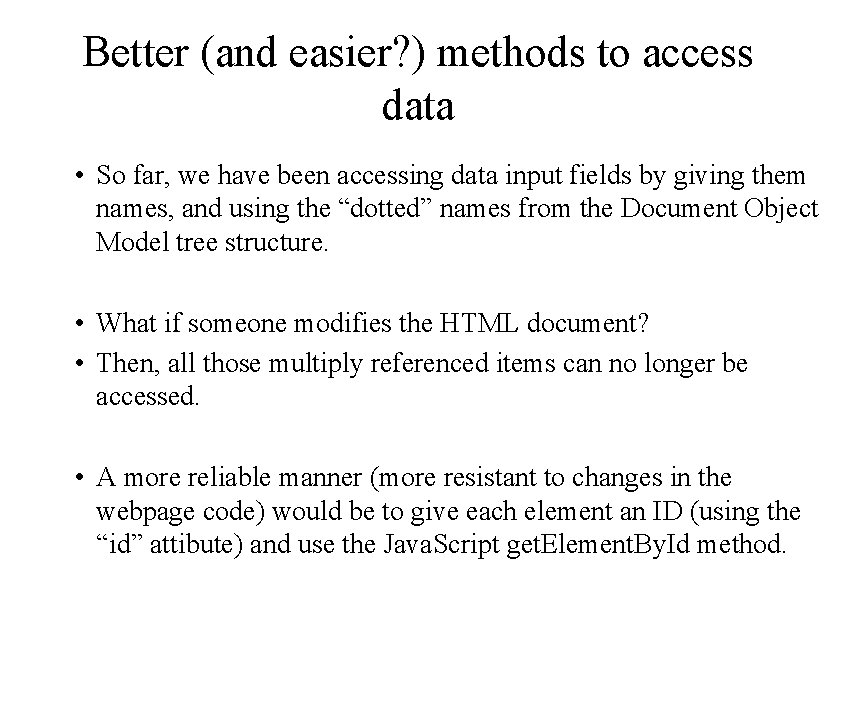
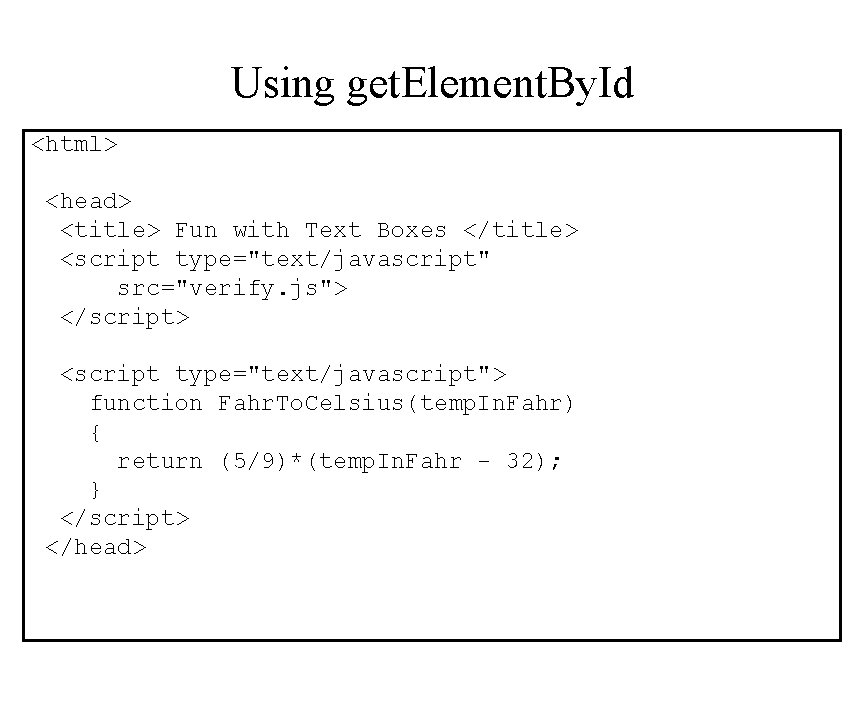
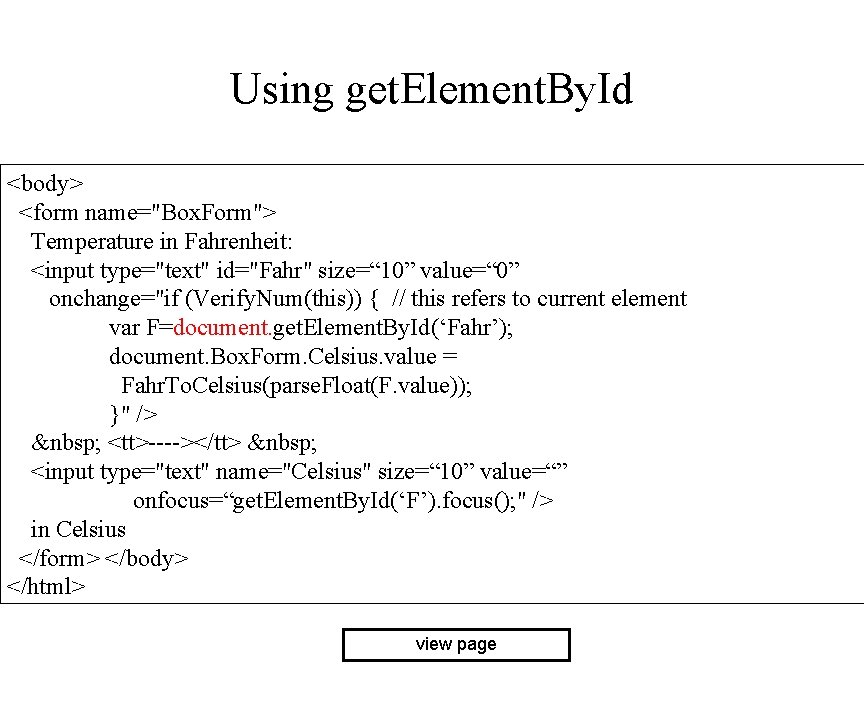
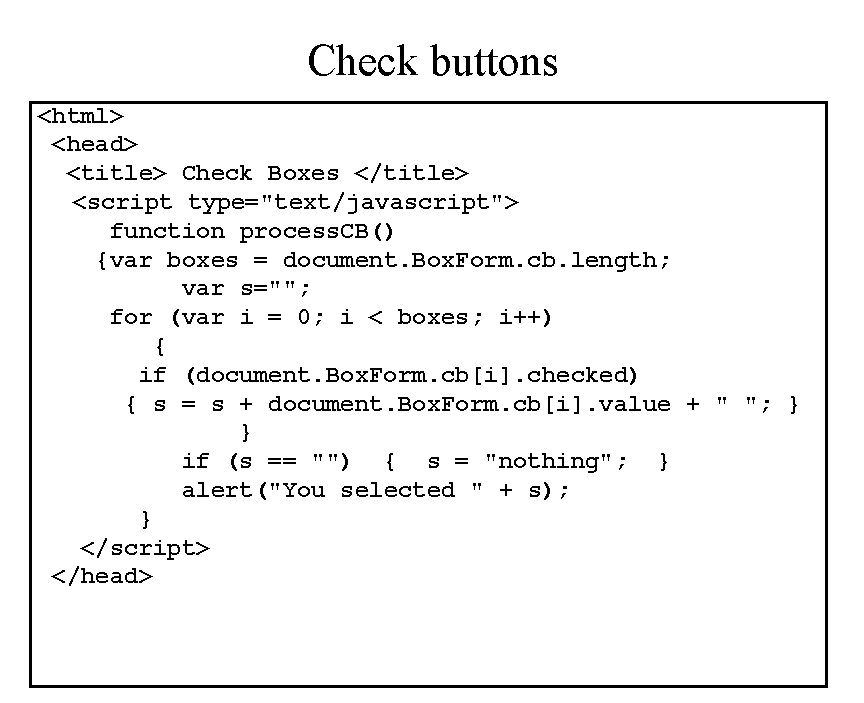
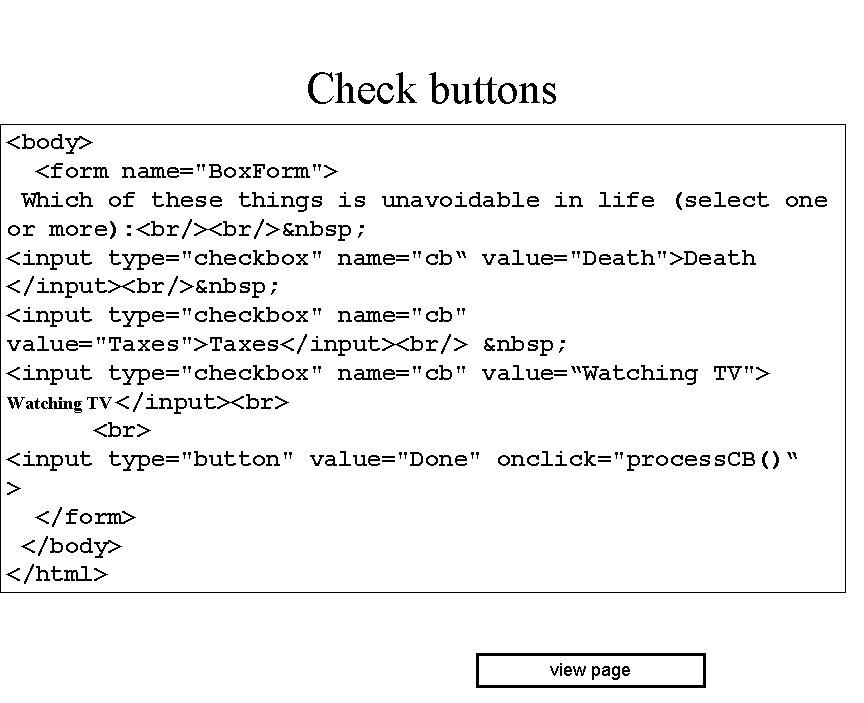
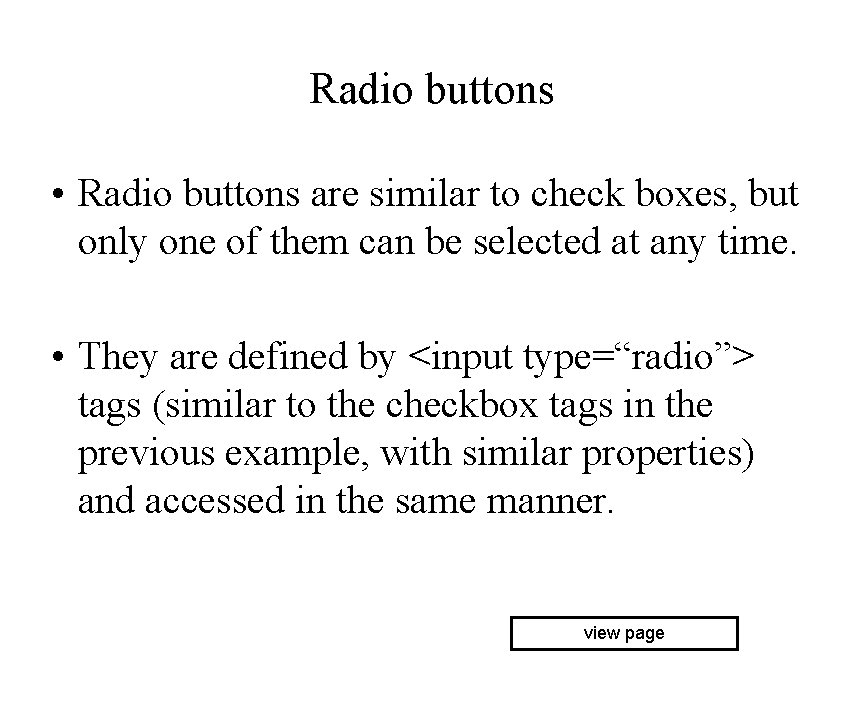
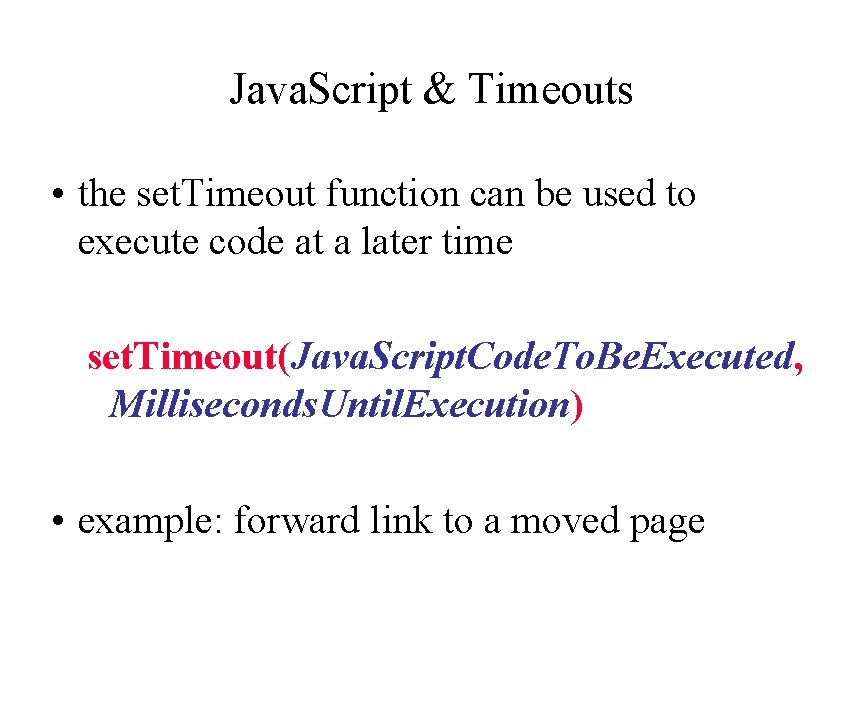
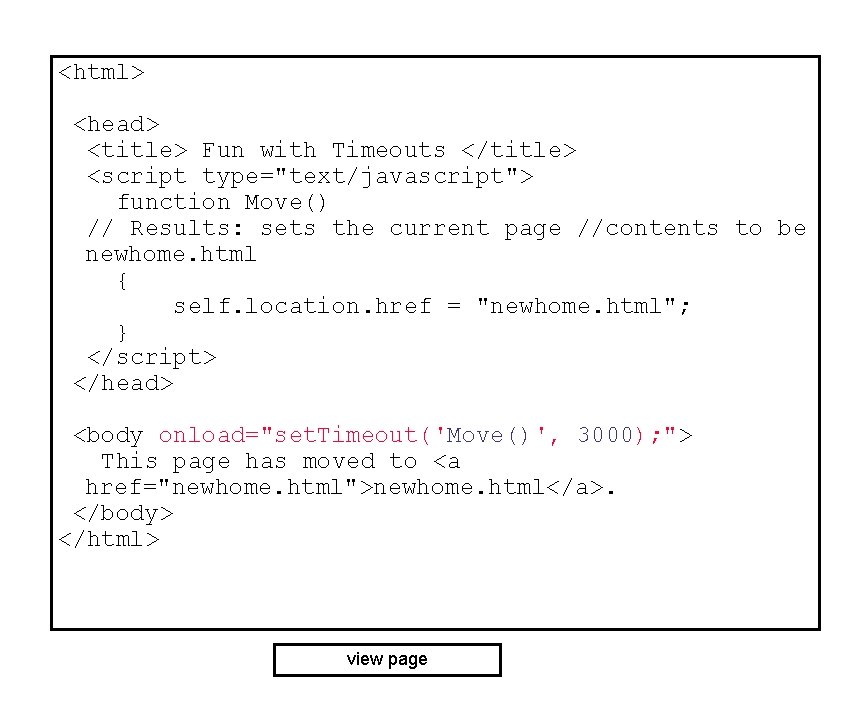
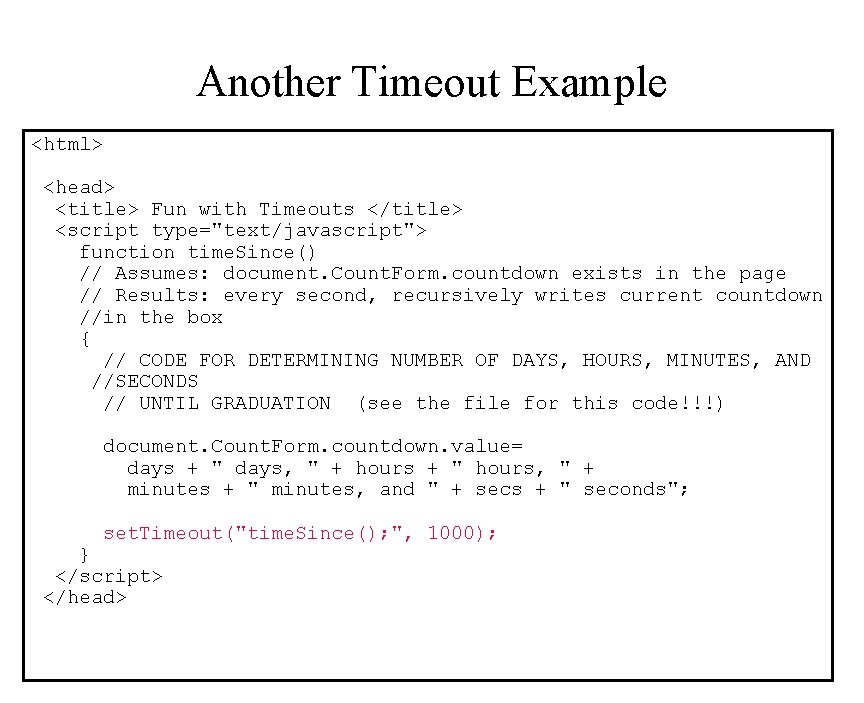
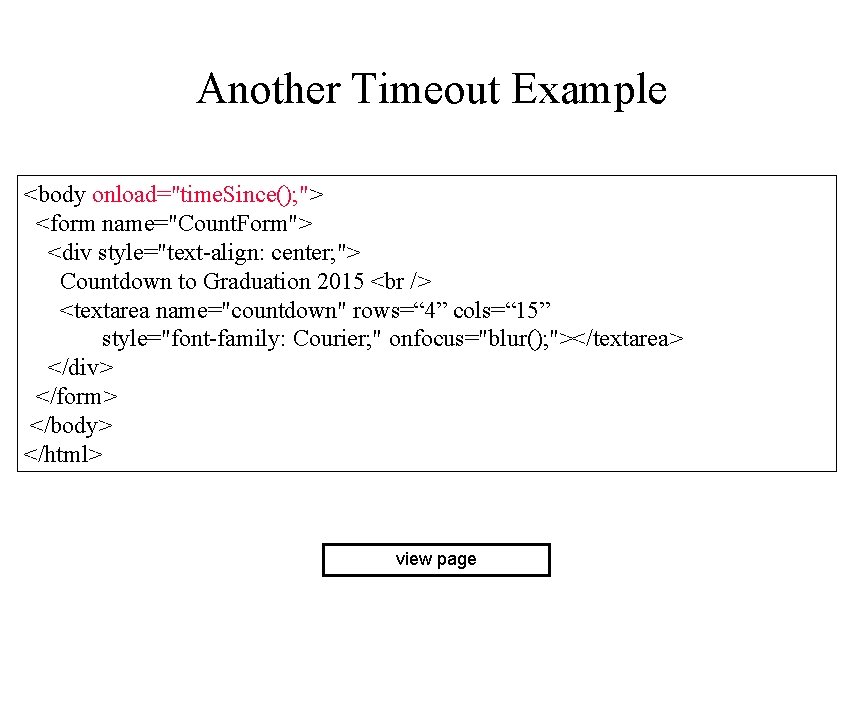
- Slides: 44
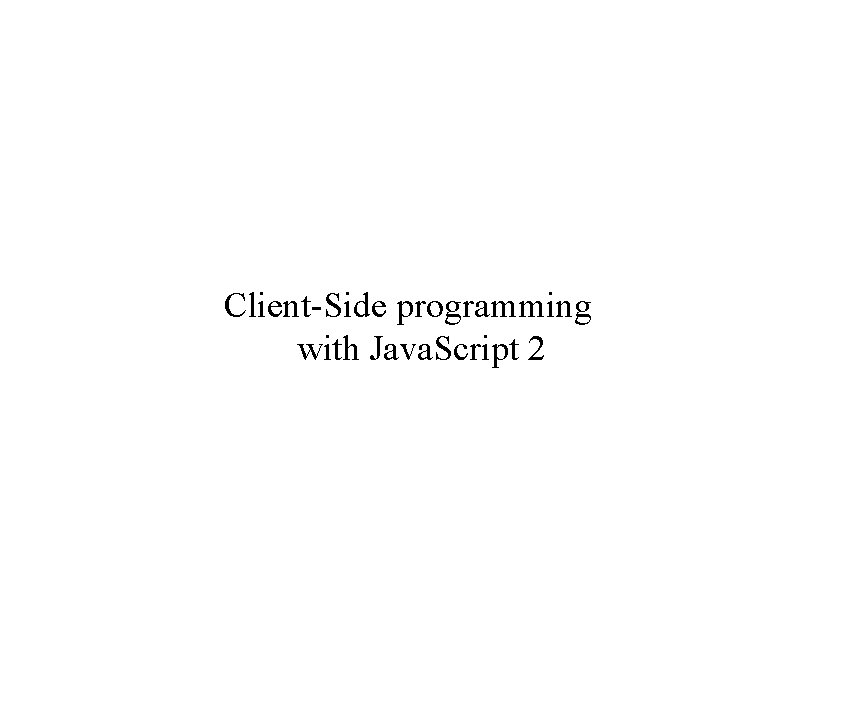
Client-Side programming with Java. Script 2
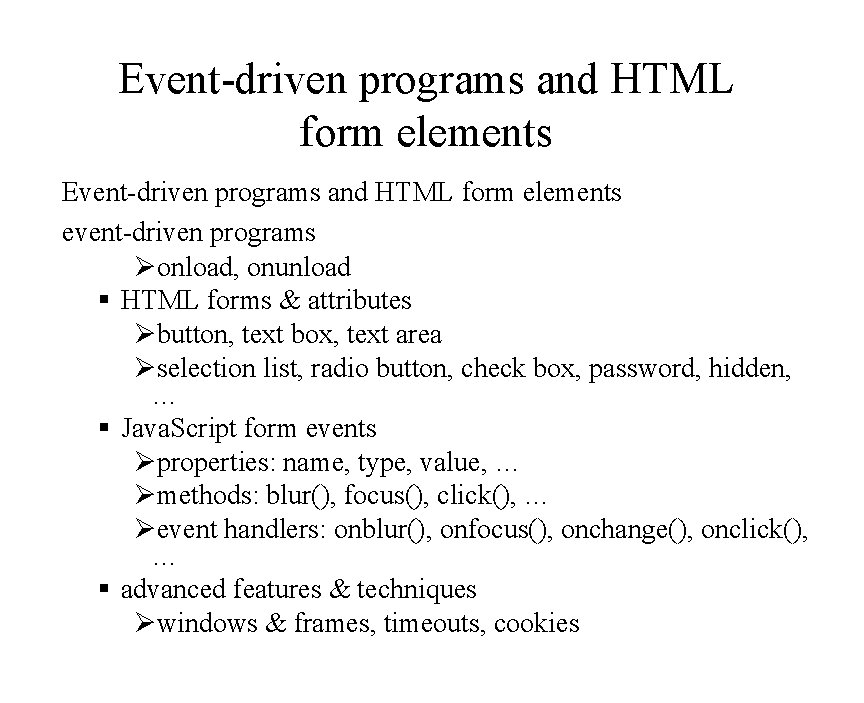
Event-driven programs and HTML form elements event-driven programs Øonload, onunload § HTML forms & attributes Øbutton, text box, text area Øselection list, radio button, check box, password, hidden, … § Java. Script form events Øproperties: name, type, value, … Ømethods: blur(), focus(), click(), … Øevent handlers: onblur(), onfocus(), onchange(), onclick(), … § advanced features & techniques Øwindows & frames, timeouts, cookies
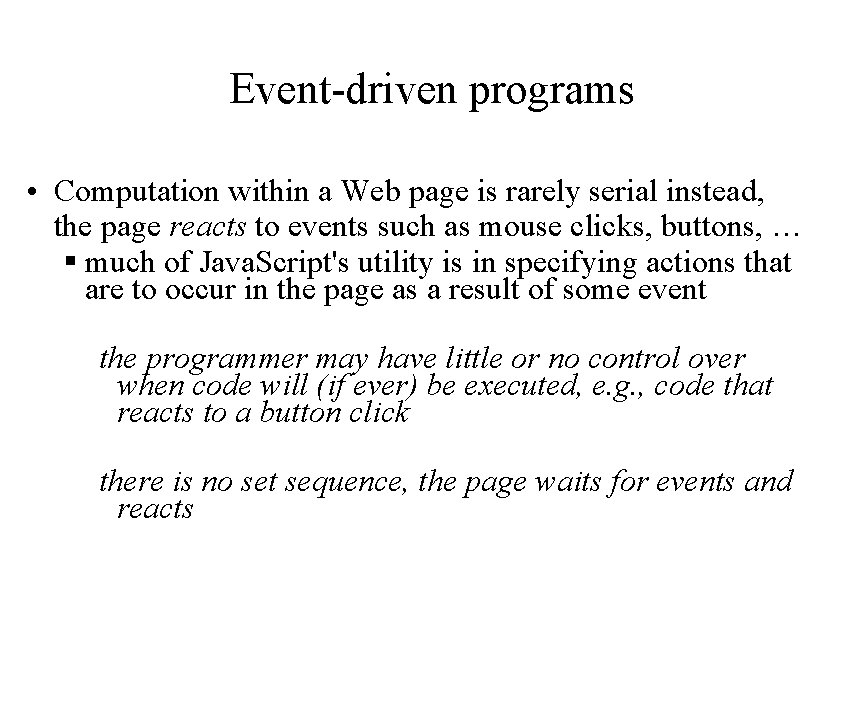
Event-driven programs • Computation within a Web page is rarely serial instead, the page reacts to events such as mouse clicks, buttons, … § much of Java. Script's utility is in specifying actions that are to occur in the page as a result of some event the programmer may have little or no control over when code will (if ever) be executed, e. g. , code that reacts to a button click there is no set sequence, the page waits for events and reacts
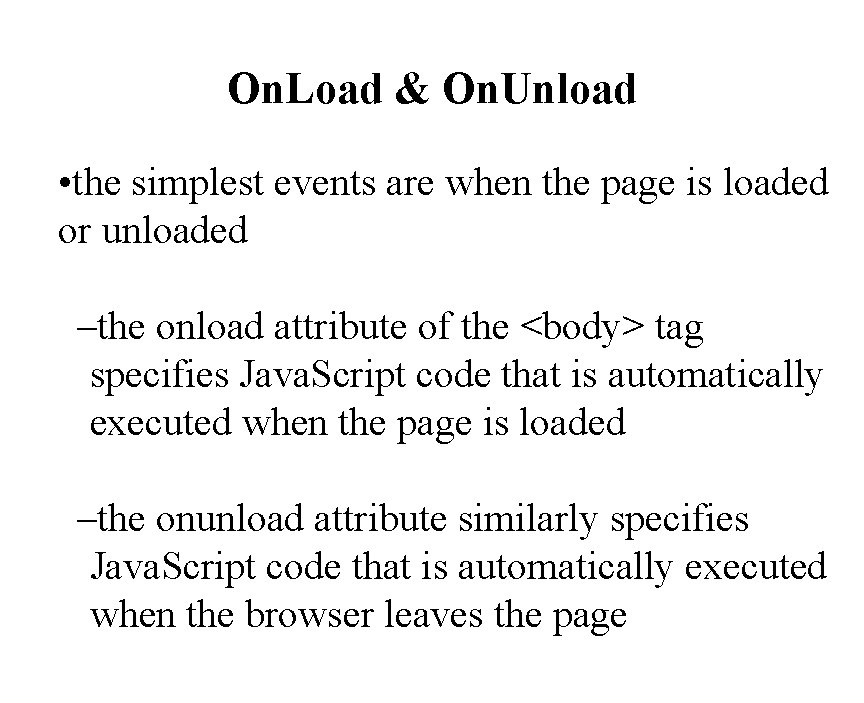
On. Load & On. Unload • the simplest events are when the page is loaded or unloaded –the onload attribute of the <body> tag specifies Java. Script code that is automatically executed when the page is loaded –the onunload attribute similarly specifies Java. Script code that is automatically executed when the browser leaves the page
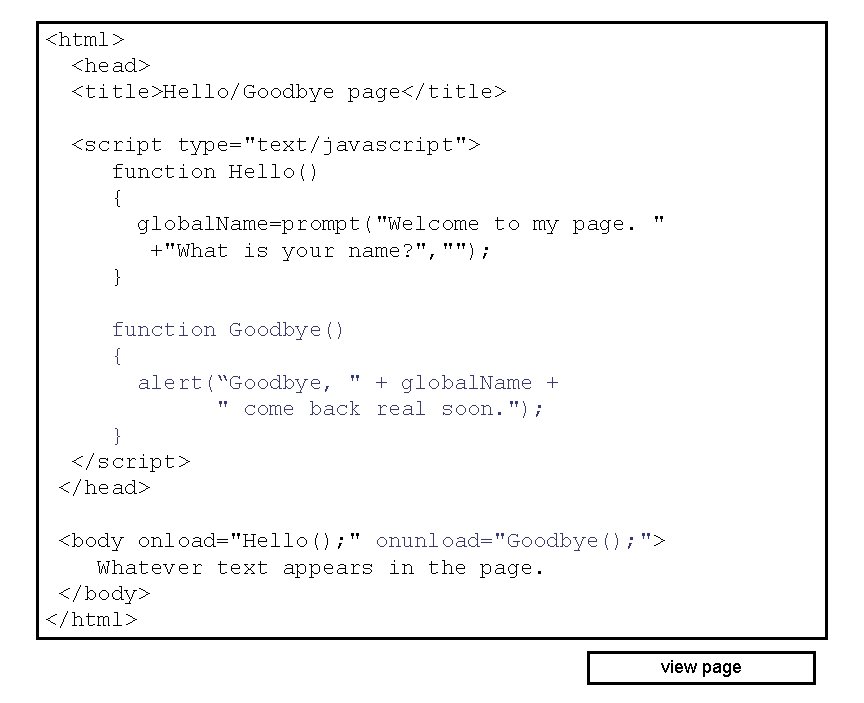
<html> <head> <title>Hello/Goodbye page</title> <script type="text/javascript"> function Hello() { global. Name=prompt("Welcome to my page. " +"What is your name? ", ""); } function Goodbye() { alert(“Goodbye, " + global. Name + " come back real soon. "); } </script> </head> <body onload="Hello(); " onunload="Goodbye(); "> Whatever text appears in the page. </body> </html> view page
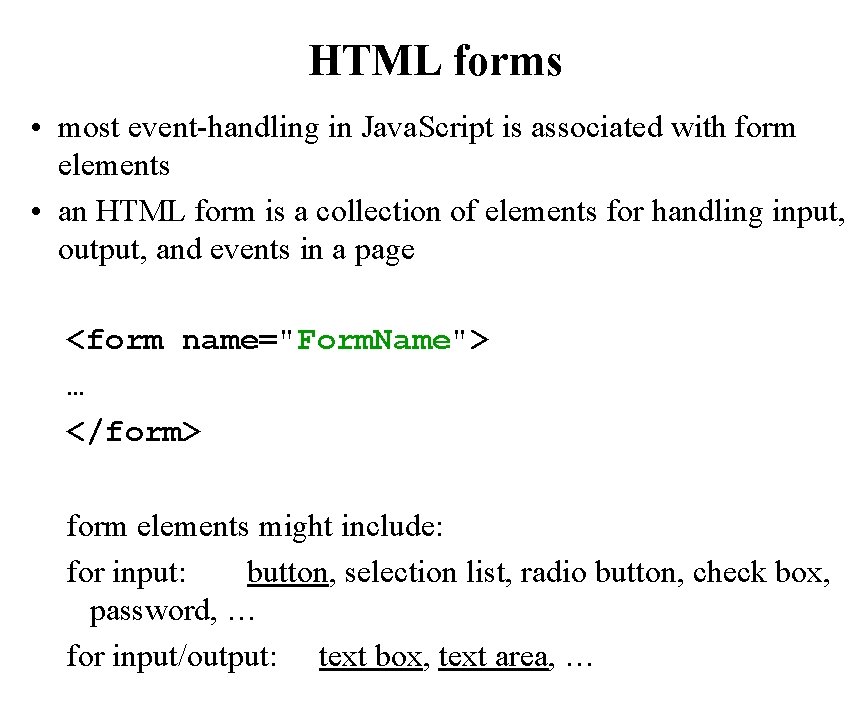
HTML forms • most event-handling in Java. Script is associated with form elements • an HTML form is a collection of elements for handling input, output, and events in a page <form name="Form. Name"> … </form> form elements might include: for input: button, selection list, radio button, check box, password, … for input/output: text box, text area, …
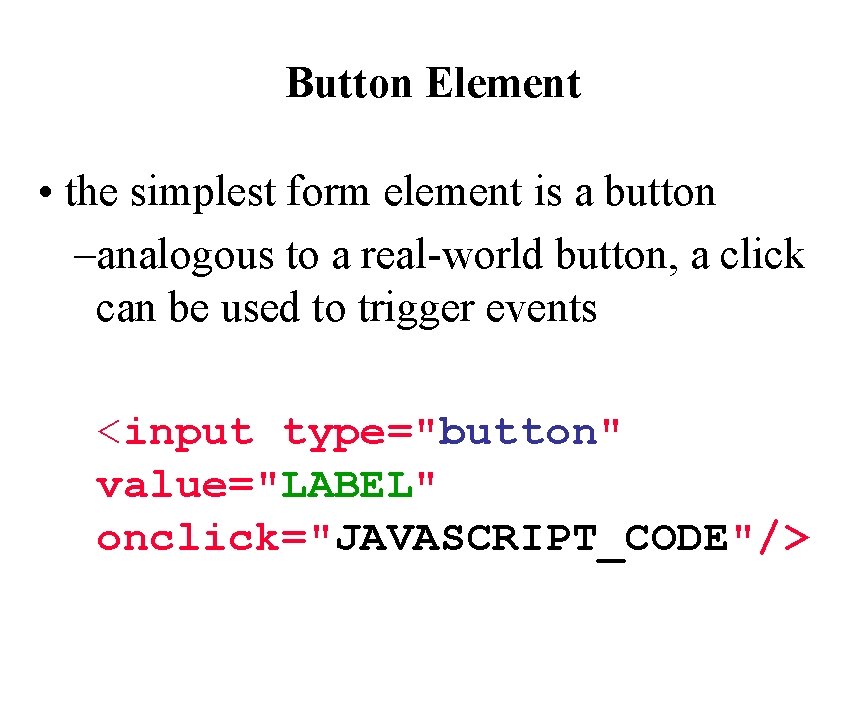
Button Element • the simplest form element is a button –analogous to a real-world button, a click can be used to trigger events <input type="button" value="LABEL" onclick="JAVASCRIPT_CODE"/>
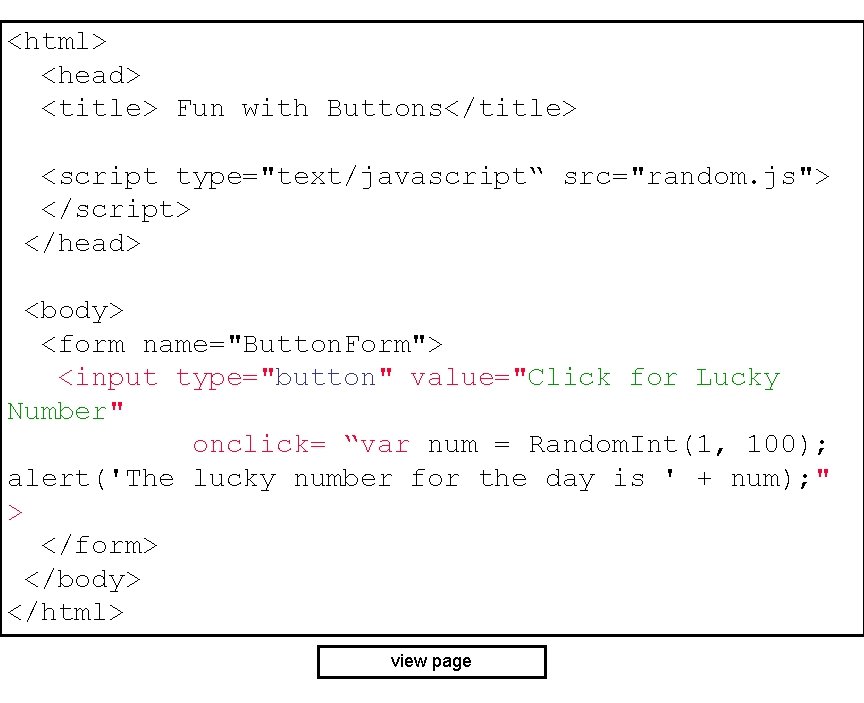
<html> <head> <title> Fun with Buttons</title> <script type="text/javascript“ src="random. js"> </script> </head> <body> <form name="Button. Form"> <input type="button" value="Click for Lucky Number" onclick= “var num = Random. Int(1, 100); alert('The lucky number for the day is ' + num); " > </form> </body> </html> view page
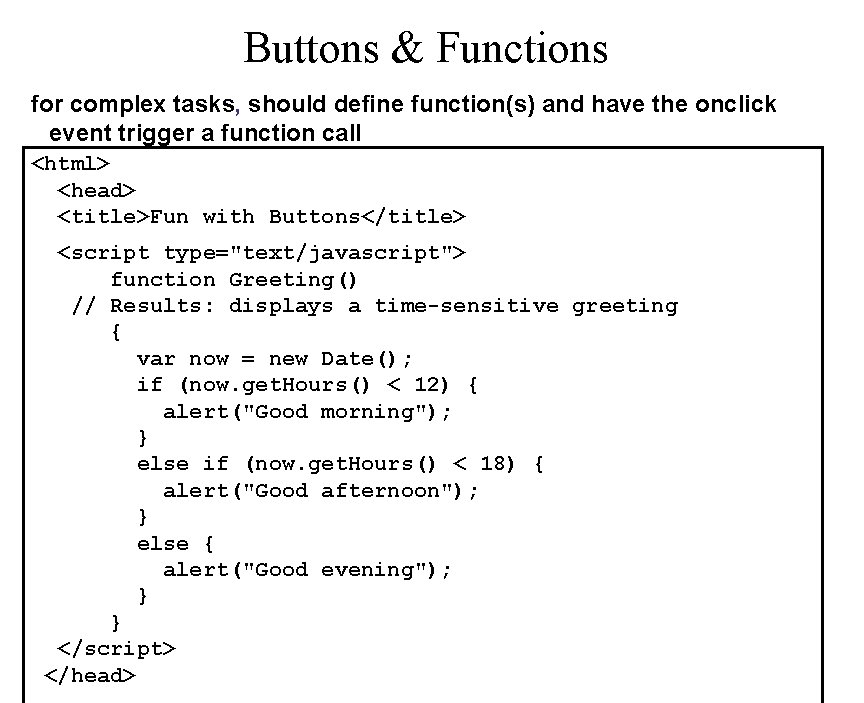
Buttons & Functions for complex tasks, should define function(s) and have the onclick event trigger a function call <html> <head> <title>Fun with Buttons</title> <script type="text/javascript"> function Greeting() // Results: displays a time-sensitive greeting { var now = new Date(); if (now. get. Hours() < 12) { alert("Good morning"); } else if (now. get. Hours() < 18) { alert("Good afternoon"); } else { alert("Good evening"); } } </script> </head>
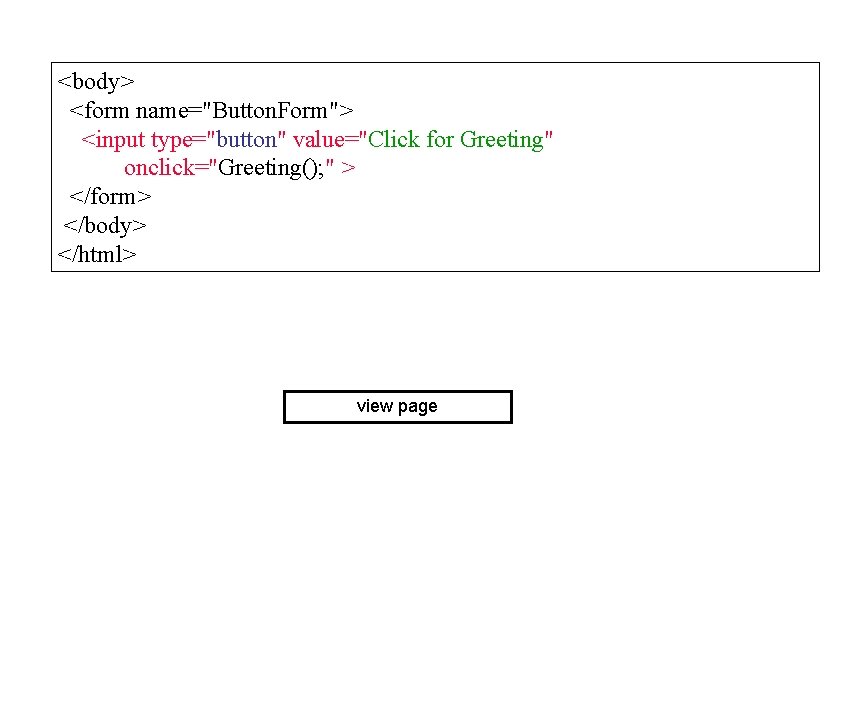
<body> <form name="Button. Form"> <input type="button" value="Click for Greeting" onclick="Greeting(); " > </form> </body> </html> view page
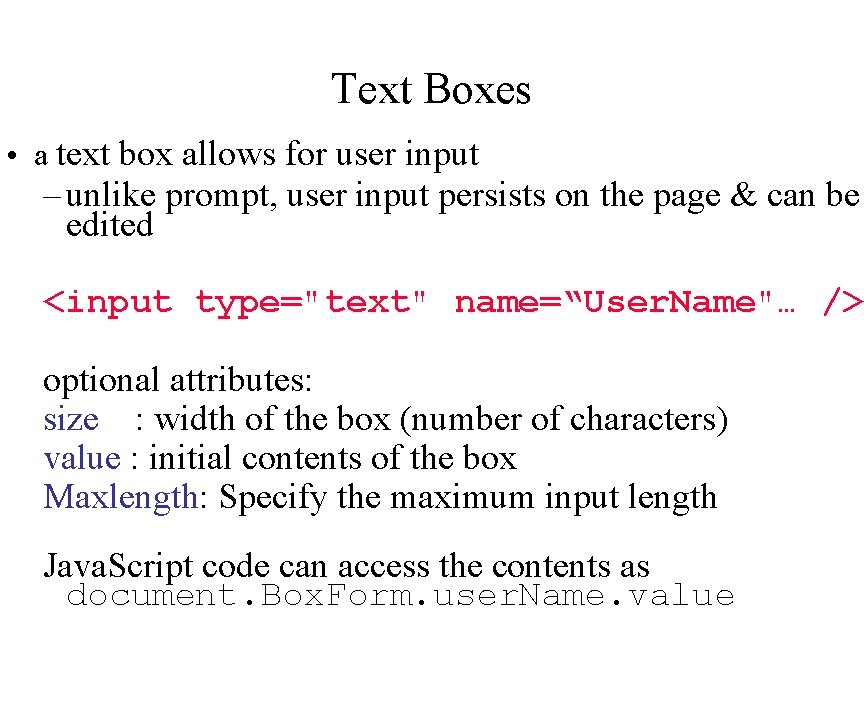
Text Boxes • a text box allows for user input – unlike prompt, user input persists on the page & can be edited <input type="text" name=“User. Name"… /> optional attributes: size : width of the box (number of characters) value : initial contents of the box Maxlength: Specify the maximum input length Java. Script code can access the contents as document. Box. Form. user. Name. value
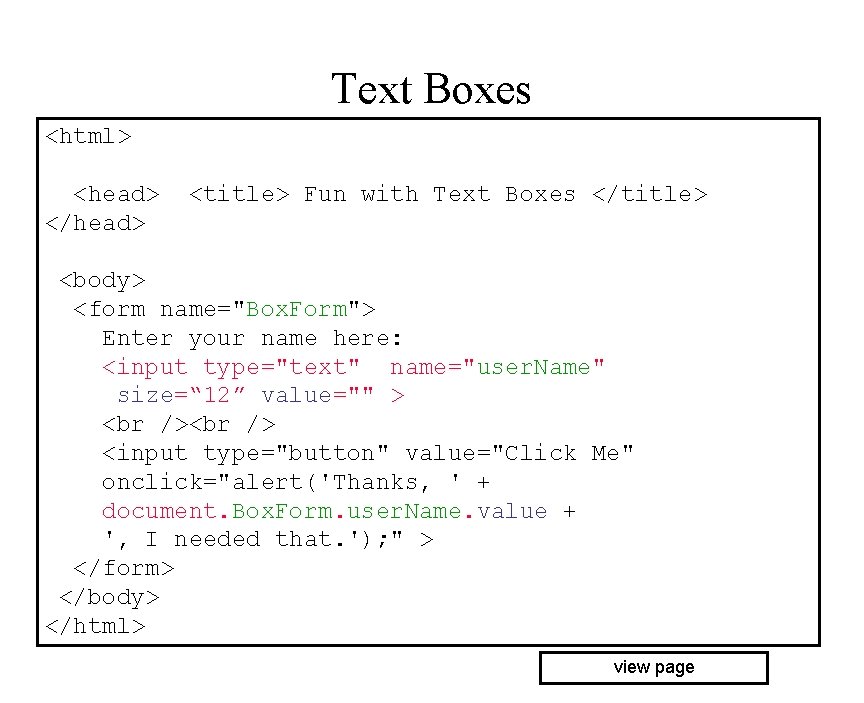
Text Boxes <html> <head> </head> <title> Fun with Text Boxes </title> <body> <form name="Box. Form"> Enter your name here: <input type="text" name="user. Name" size=“ 12” value="" > <input type="button" value="Click Me" onclick="alert('Thanks, ' + document. Box. Form. user. Name. value + ', I needed that. '); " > </form> </body> </html> view page
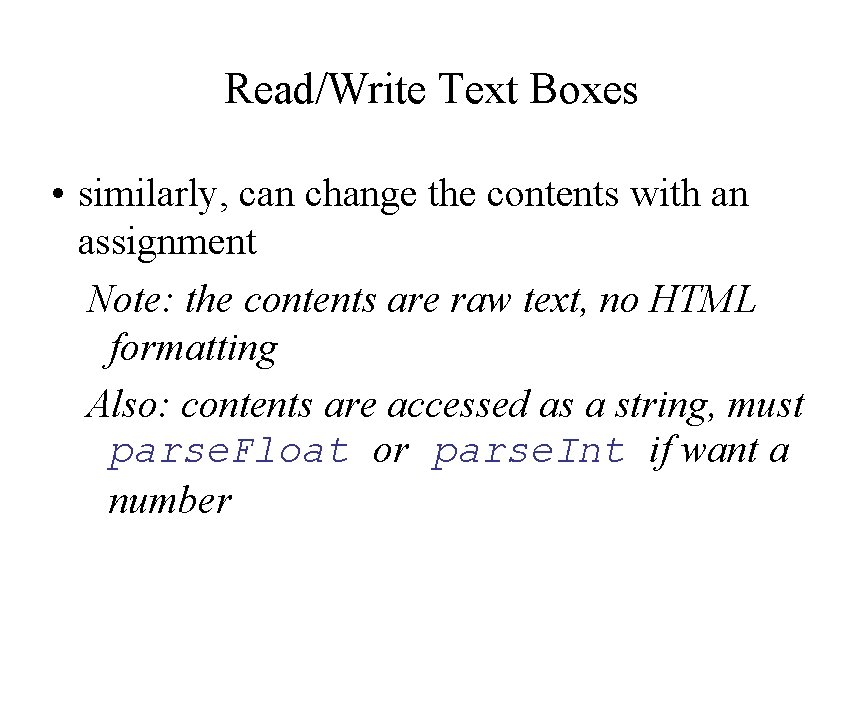
Read/Write Text Boxes • similarly, can change the contents with an assignment Note: the contents are raw text, no HTML formatting Also: contents are accessed as a string, must parse. Float or parse. Int if want a number
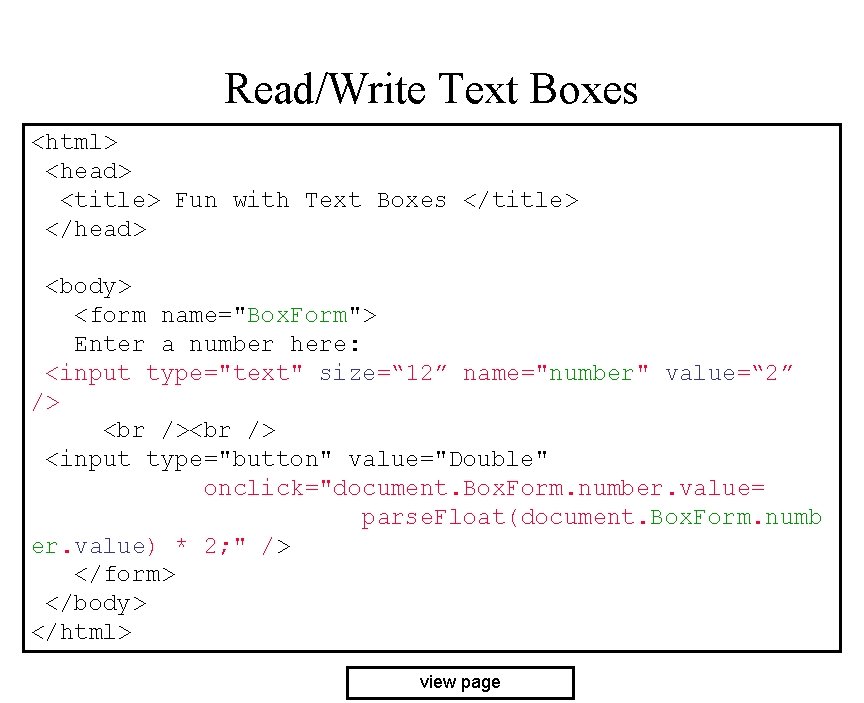
Read/Write Text Boxes <html> <head> <title> Fun with Text Boxes </title> </head> <body> <form name="Box. Form"> Enter a number here: <input type="text" size=“ 12” name="number" value=“ 2” /> <input type="button" value="Double" onclick="document. Box. Form. number. value= parse. Float(document. Box. Form. numb er. value) * 2; " /> </form> </body> </html> view page
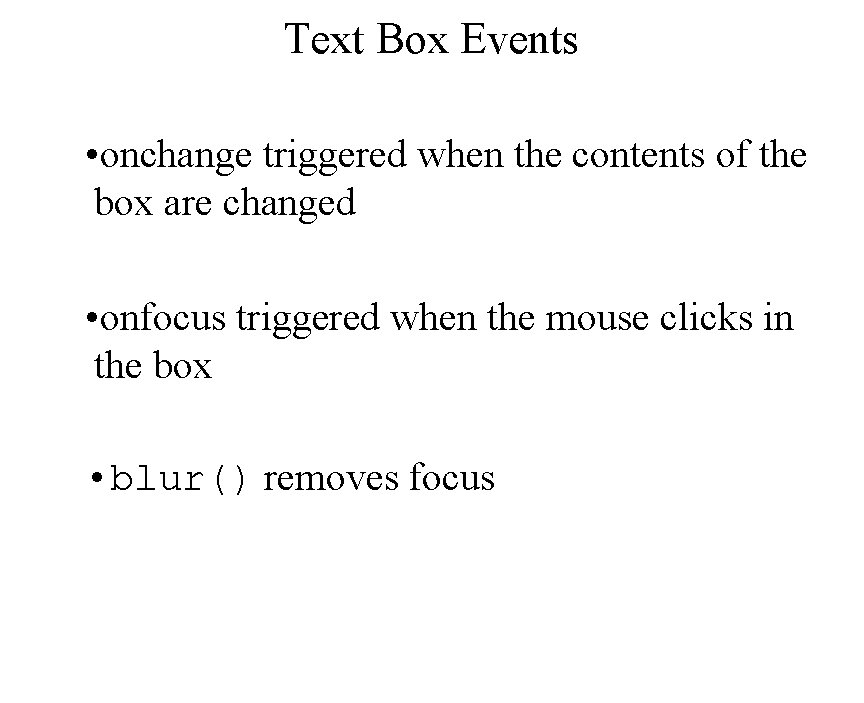
Text Box Events • onchange triggered when the contents of the box are changed • onfocus triggered when the mouse clicks in the box • blur() removes focus
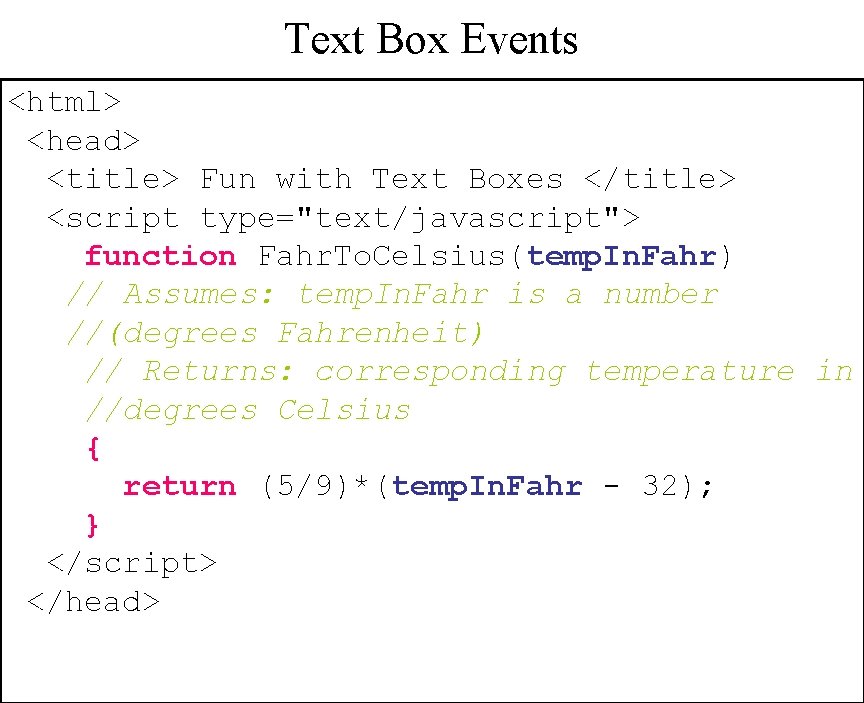
Text Box Events <html> <head> <title> Fun with Text Boxes </title> <script type="text/javascript"> function Fahr. To. Celsius(temp. In. Fahr) // Assumes: temp. In. Fahr is a number //(degrees Fahrenheit) // Returns: corresponding temperature in //degrees Celsius { return (5/9)*(temp. In. Fahr - 32); } </script> </head>
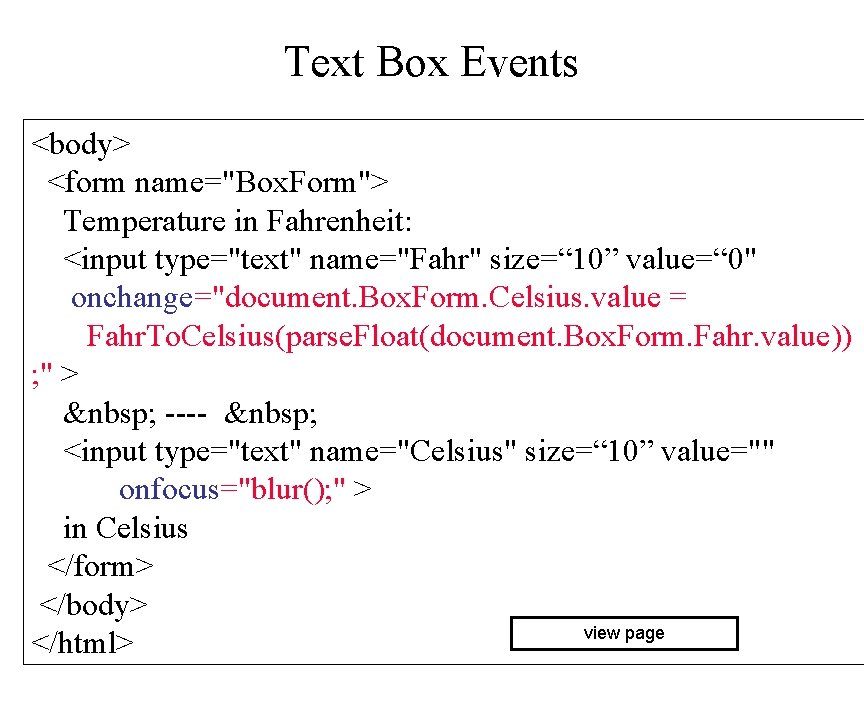
Text Box Events <body> <form name="Box. Form"> Temperature in Fahrenheit: <input type="text" name="Fahr" size=“ 10” value=“ 0" onchange="document. Box. Form. Celsius. value = Fahr. To. Celsius(parse. Float(document. Box. Form. Fahr. value)) ; " > ---- <input type="text" name="Celsius" size=“ 10” value="" onfocus="blur(); " > in Celsius </form> </body> view page </html>
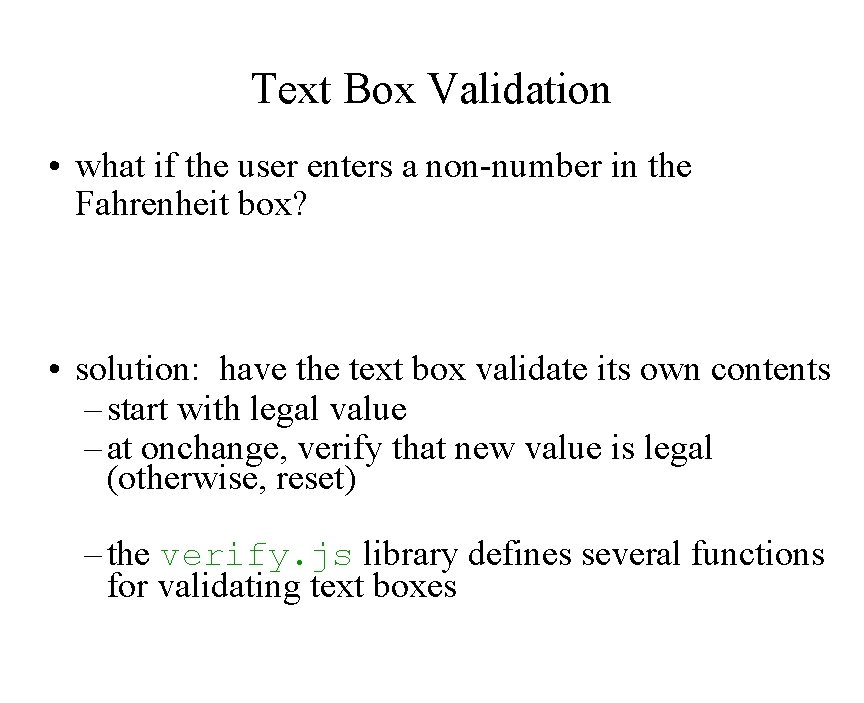
Text Box Validation • what if the user enters a non-number in the Fahrenheit box? • solution: have the text box validate its own contents – start with legal value – at onchange, verify that new value is legal (otherwise, reset) – the verify. js library defines several functions for validating text boxes
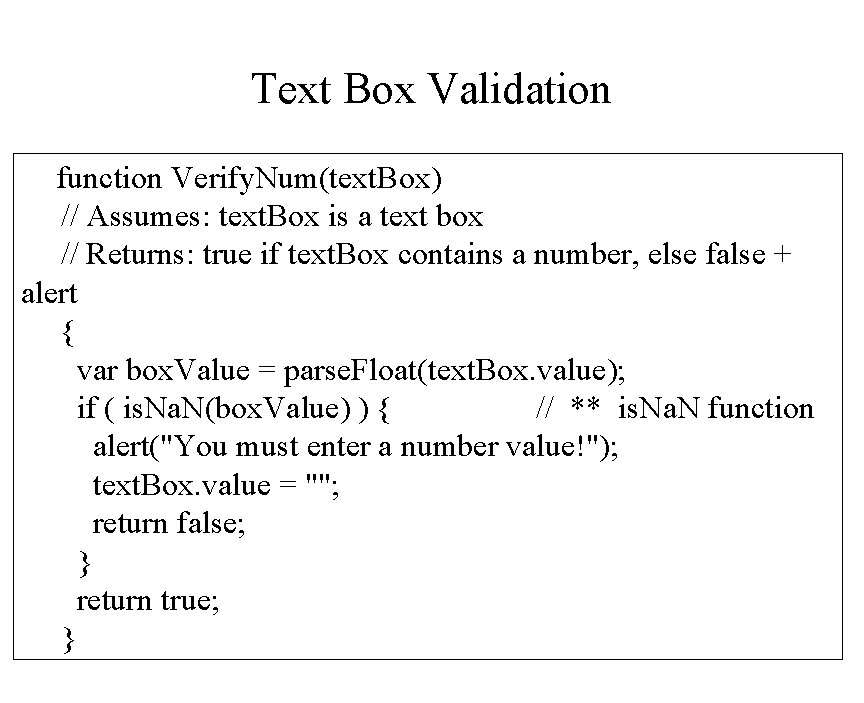
Text Box Validation function Verify. Num(text. Box) // Assumes: text. Box is a text box // Returns: true if text. Box contains a number, else false + alert { var box. Value = parse. Float(text. Box. value); if ( is. Na. N(box. Value) ) { // ** is. Na. N function alert("You must enter a number value!"); text. Box. value = ""; return false; } return true; }
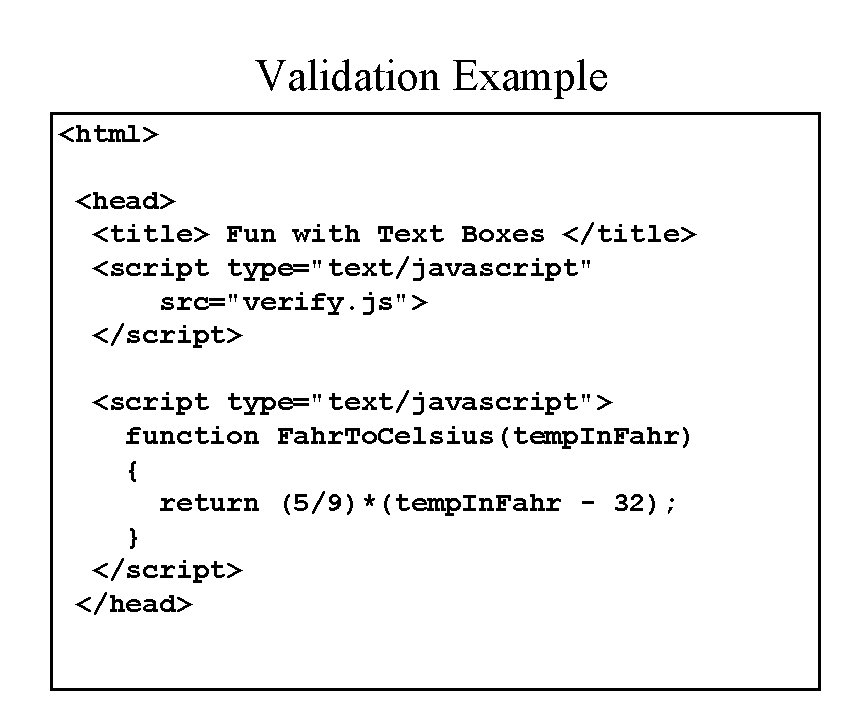
Validation Example <html> <head> <title> Fun with Text Boxes </title> <script type="text/javascript" src="verify. js"> </script> <script type="text/javascript"> function Fahr. To. Celsius(temp. In. Fahr) { return (5/9)*(temp. In. Fahr - 32); } </script> </head>
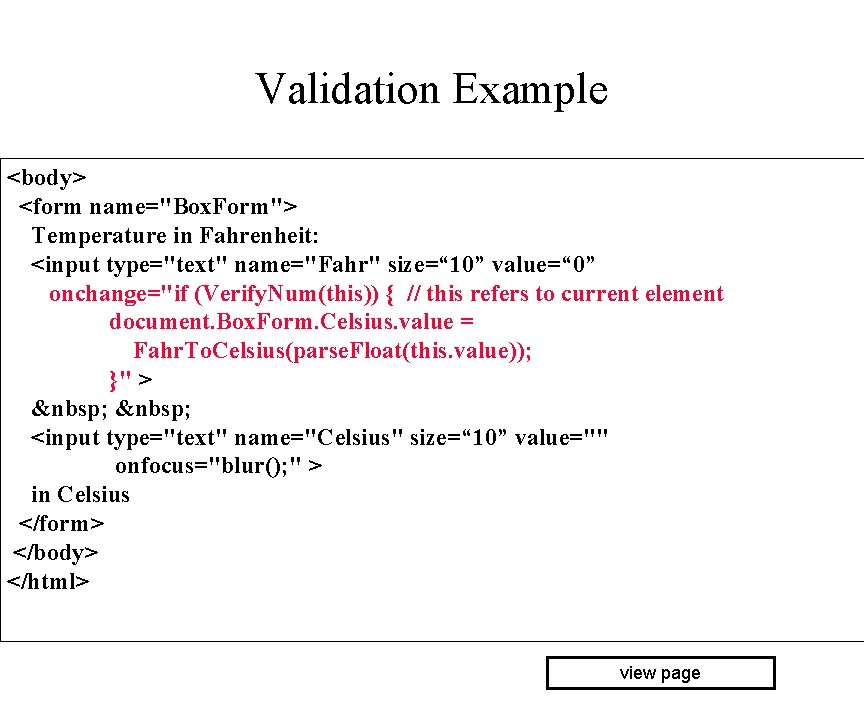
Validation Example <body> <form name="Box. Form"> Temperature in Fahrenheit: <input type="text" name="Fahr" size=“ 10” value=“ 0” onchange="if (Verify. Num(this)) { // this refers to current element document. Box. Form. Celsius. value = Fahr. To. Celsius(parse. Float(this. value)); }" > <input type="text" name="Celsius" size=“ 10” value="" onfocus="blur(); " > in Celsius </form> </body> </html> view page
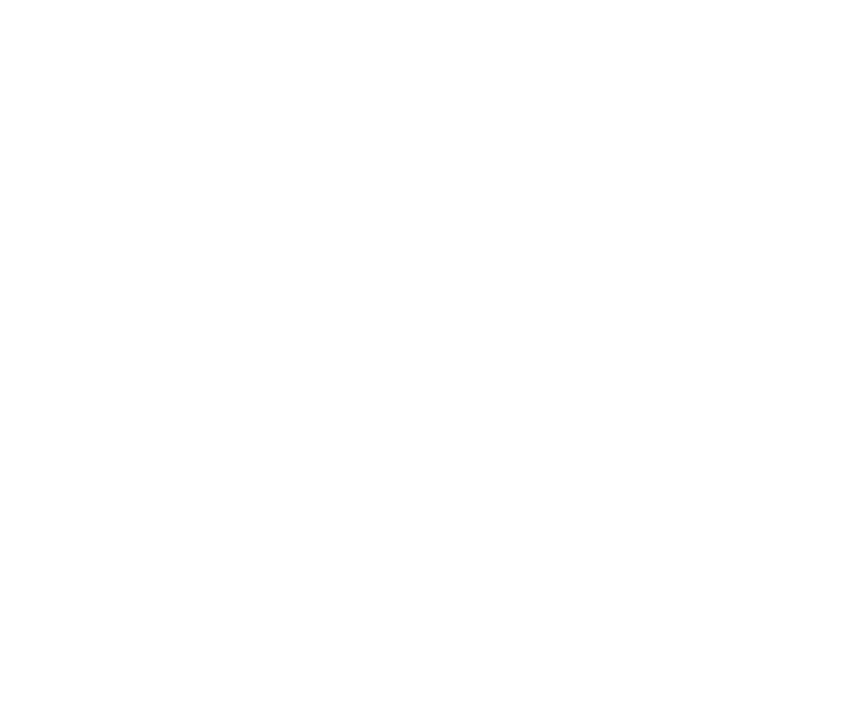
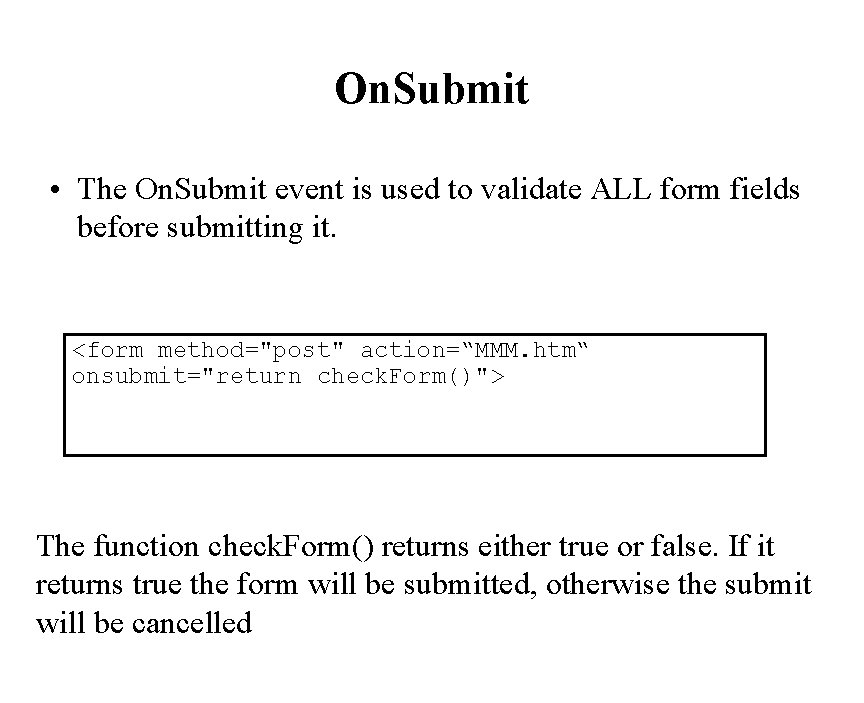
On. Submit • The On. Submit event is used to validate ALL form fields before submitting it. <form method="post" action=“MMM. htm“ onsubmit="return check. Form()"> The function check. Form() returns either true or false. If it returns true the form will be submitted, otherwise the submit will be cancelled
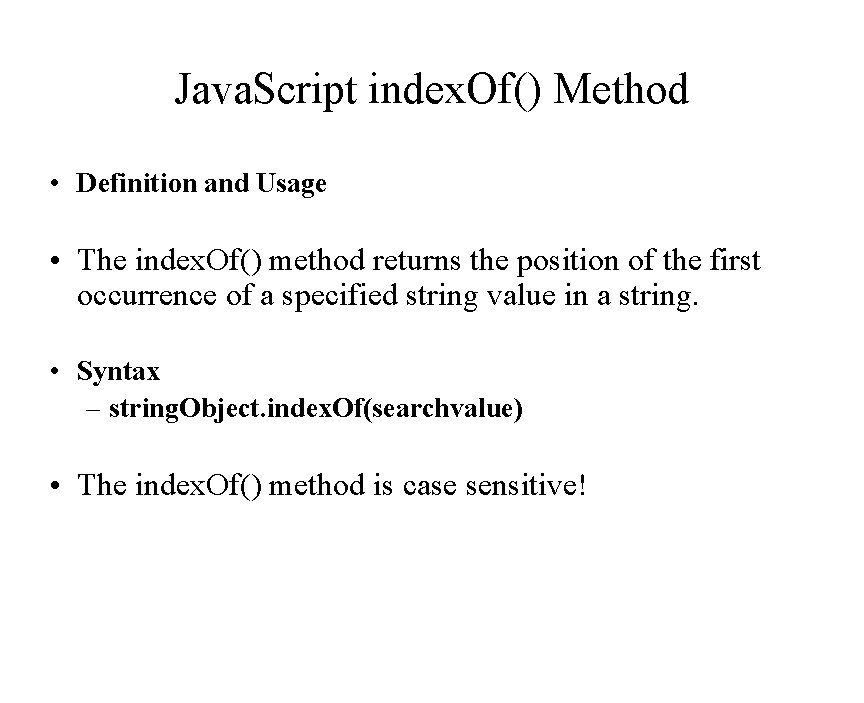
Java. Script index. Of() Method • Definition and Usage • The index. Of() method returns the position of the first occurrence of a specified string value in a string. • Syntax – string. Object. index. Of(searchvalue) • The index. Of() method is case sensitive!
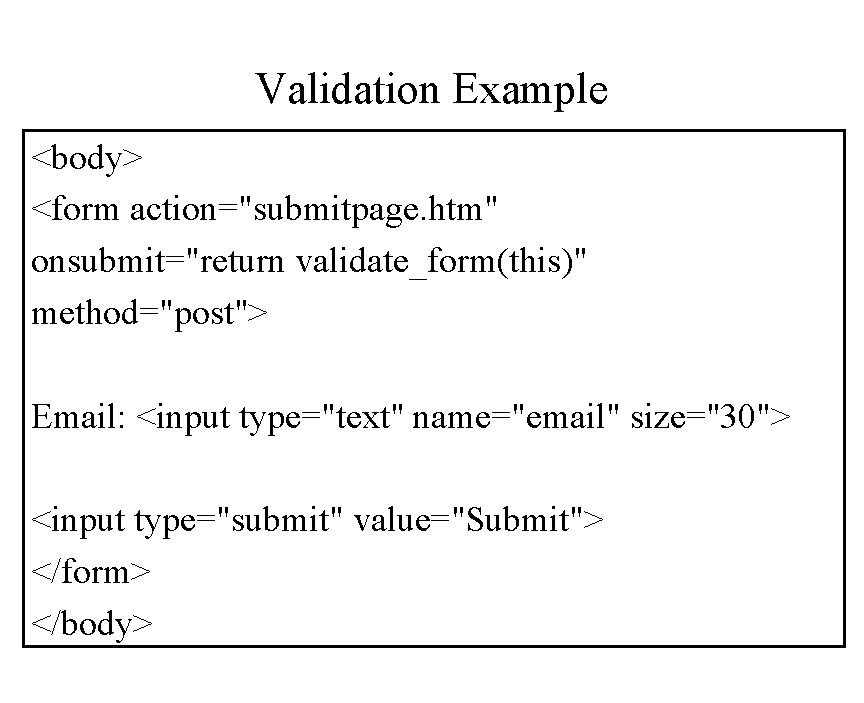
Validation Example <body> <form action="submitpage. htm" onsubmit="return validate_form(this)" method="post"> Email: <input type="text" name="email" size="30"> <input type="submit" value="Submit"> </form> </body>
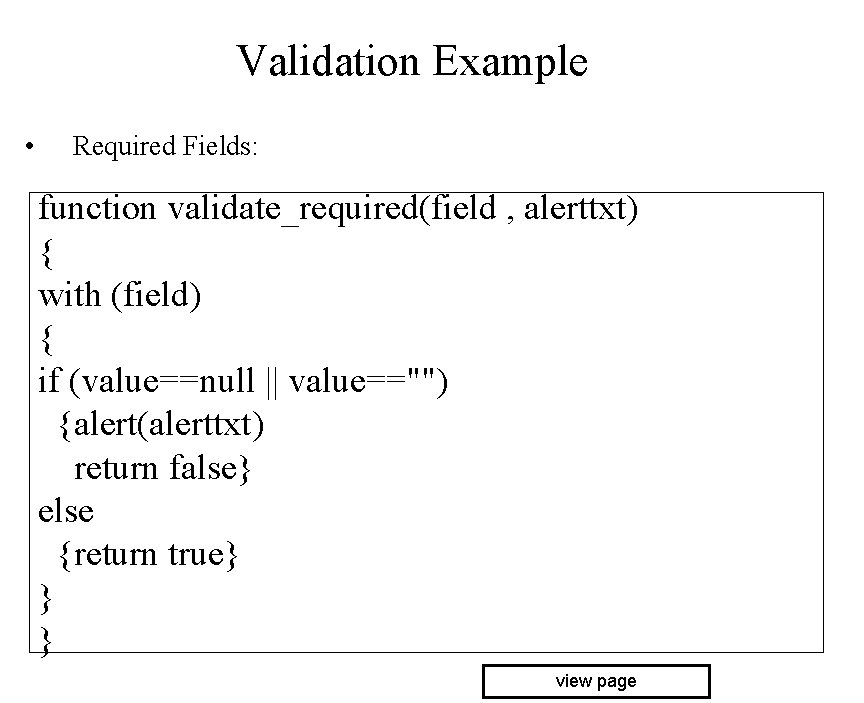
Validation Example • Required Fields: function validate_required(field , alerttxt) { with (field) { if (value==null || value=="") {alert(alerttxt) return false} else {return true} } } view page
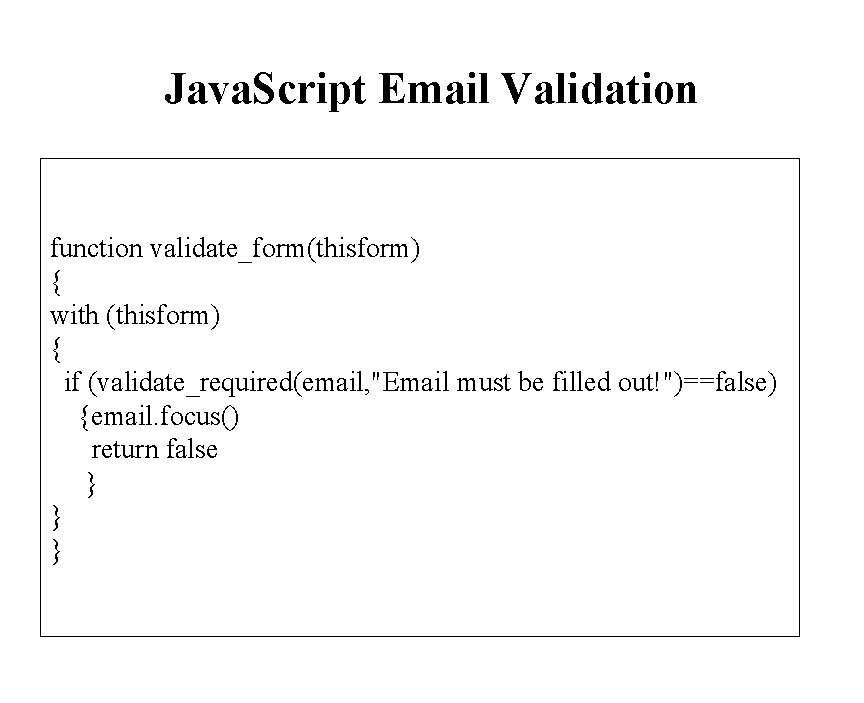
Java. Script Email Validation function validate_form(thisform) { with (thisform) { if (validate_required(email, "Email must be filled out!")==false) {email. focus() return false } } }
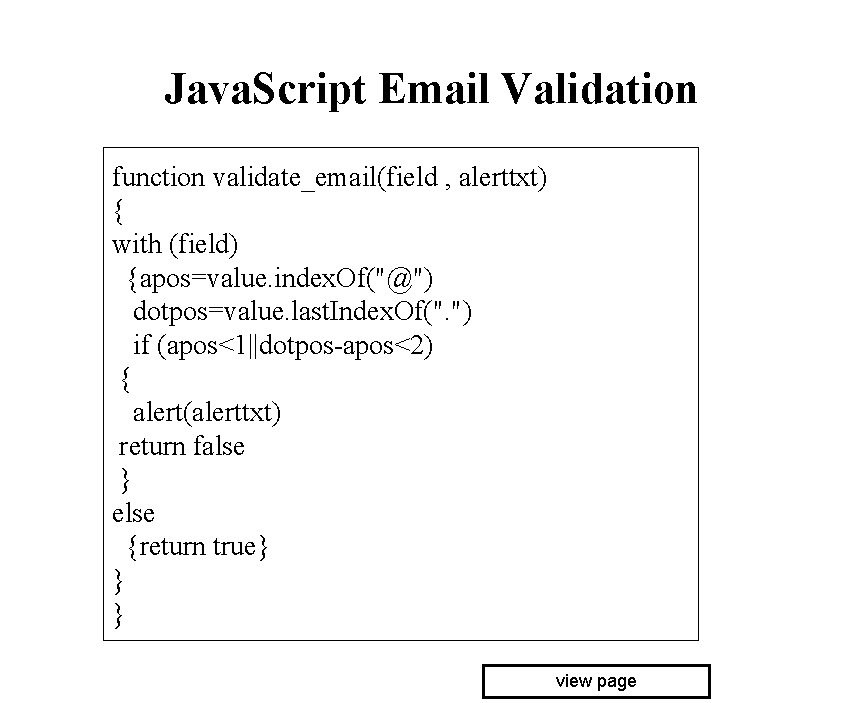
Java. Script Email Validation function validate_email(field , alerttxt) { with (field) {apos=value. index. Of("@") dotpos=value. last. Index. Of(". ") if (apos<1||dotpos-apos<2) { alert(alerttxt) return false } else {return true} } } view page
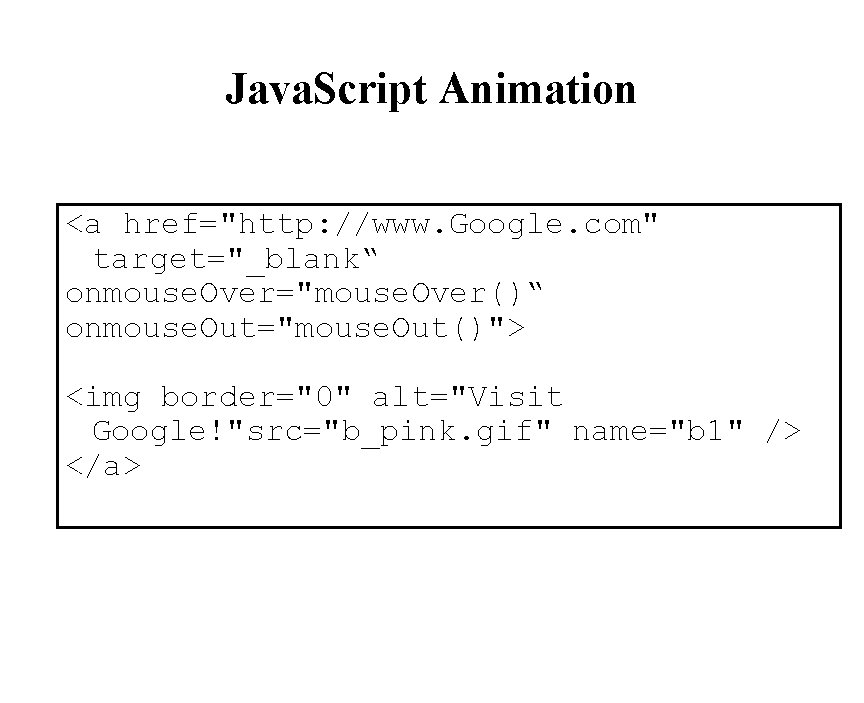
Java. Script Animation <a href="http: //www. Google. com" target="_blank“ onmouse. Over="mouse. Over()“ onmouse. Out="mouse. Out()"> <img border="0" alt="Visit Google!"src="b_pink. gif" name="b 1" /> </a>
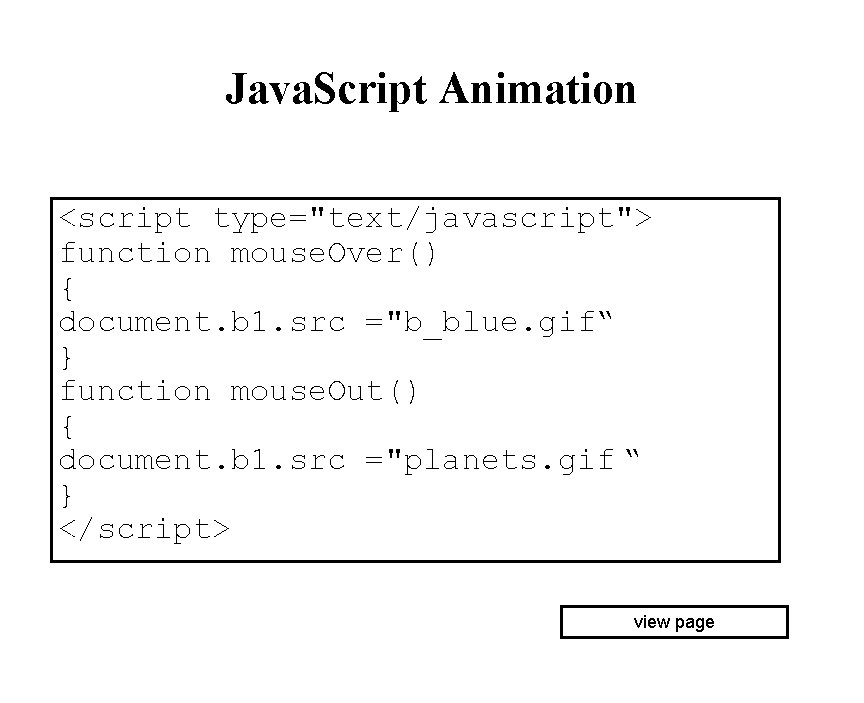
Java. Script Animation <script type="text/javascript"> function mouse. Over() { document. b 1. src ="b_blue. gif“ } function mouse. Out() { document. b 1. src ="planets. gif “ } </script> view page
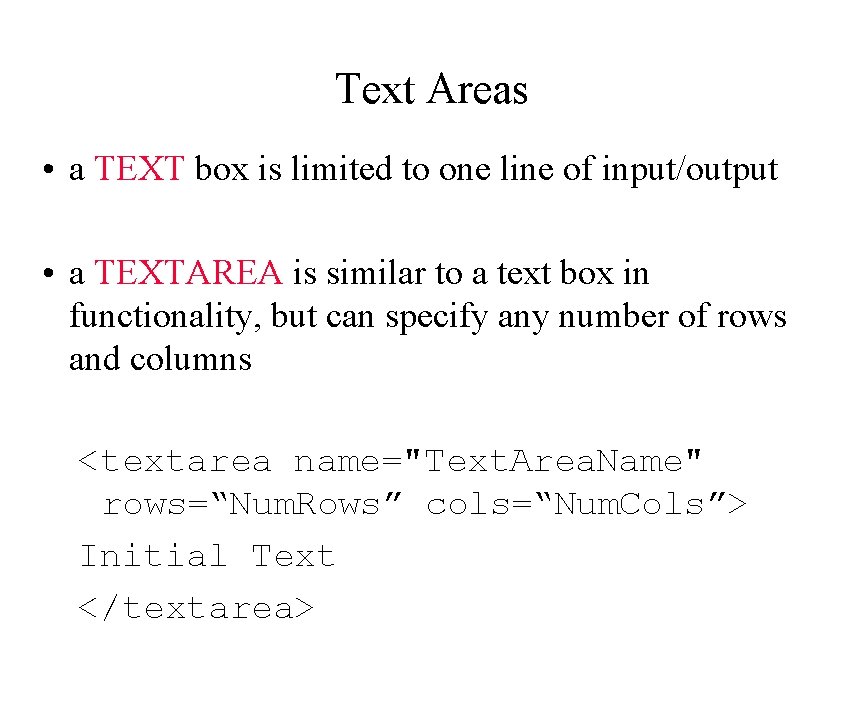
Text Areas • a TEXT box is limited to one line of input/output • a TEXTAREA is similar to a text box in functionality, but can specify any number of rows and columns <textarea name="Text. Area. Name" rows=“Num. Rows” cols=“Num. Cols”> Initial Text </textarea>
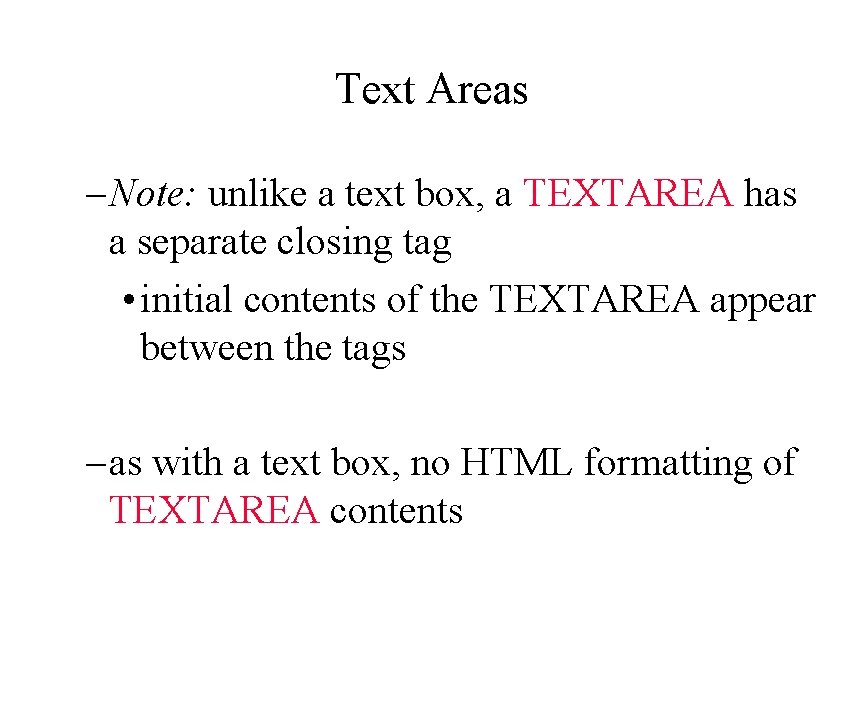
Text Areas – Note: unlike a text box, a TEXTAREA has a separate closing tag • initial contents of the TEXTAREA appear between the tags – as with a text box, no HTML formatting of TEXTAREA contents
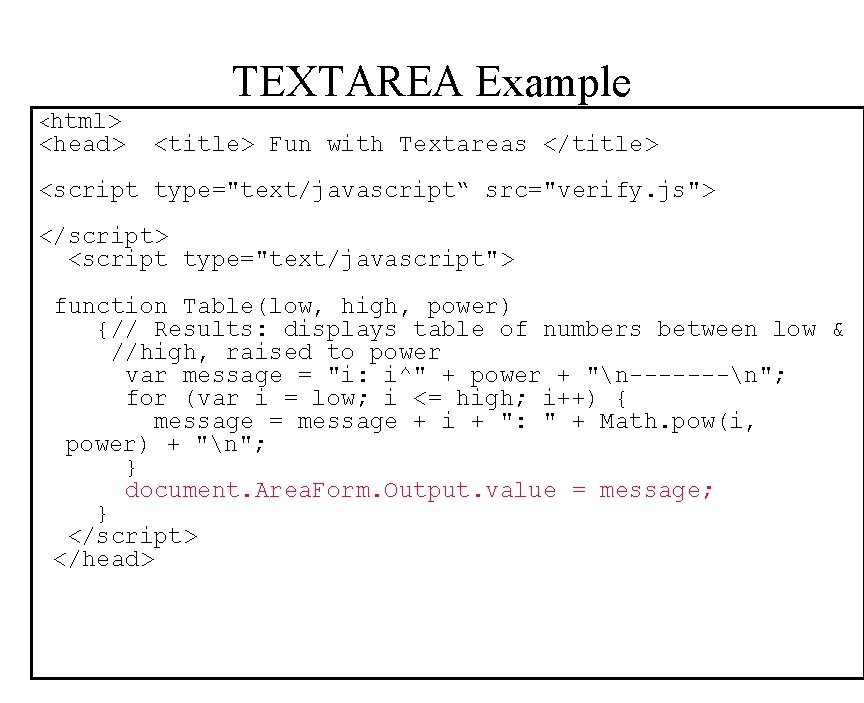
TEXTAREA Example <html> <head> <title> Fun with Textareas </title> <script type="text/javascript“ src="verify. js"> </script> <script type="text/javascript"> function Table(low, high, power) {// Results: displays table of numbers between low & //high, raised to power var message = "i: i^" + power + "n-------n"; for (var i = low; i <= high; i++) { message = message + i + ": " + Math. pow(i, power) + "n"; } document. Area. Form. Output. value = message; } </script> </head>
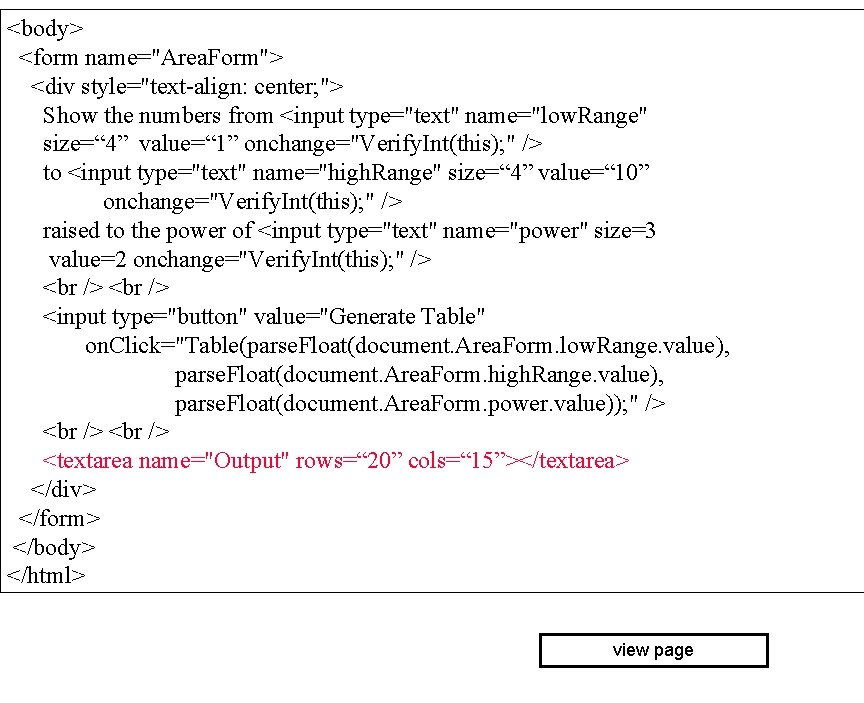
<body> <form name="Area. Form"> <div style="text-align: center; "> Show the numbers from <input type="text" name="low. Range" size=“ 4” value=“ 1” onchange="Verify. Int(this); " /> to <input type="text" name="high. Range" size=“ 4” value=“ 10” onchange="Verify. Int(this); " /> raised to the power of <input type="text" name="power" size=3 value=2 onchange="Verify. Int(this); " /> <input type="button" value="Generate Table" on. Click="Table(parse. Float(document. Area. Form. low. Range. value), parse. Float(document. Area. Form. high. Range. value), parse. Float(document. Area. Form. power. value)); " /> <textarea name="Output" rows=“ 20” cols=“ 15”></textarea> </div> </form> </body> </html> view page
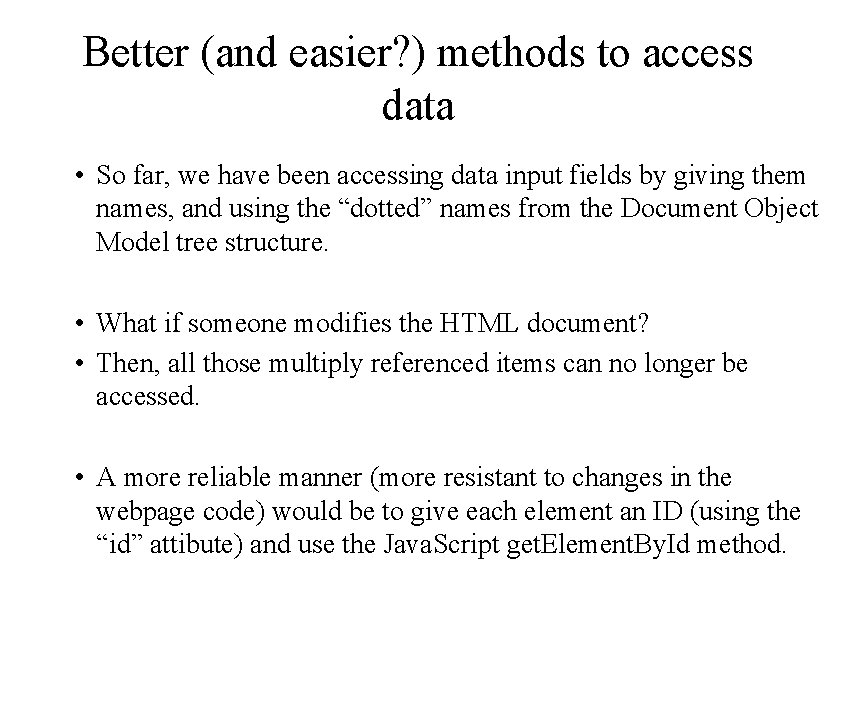
Better (and easier? ) methods to access data • So far, we have been accessing data input fields by giving them names, and using the “dotted” names from the Document Object Model tree structure. • What if someone modifies the HTML document? • Then, all those multiply referenced items can no longer be accessed. • A more reliable manner (more resistant to changes in the webpage code) would be to give each element an ID (using the “id” attibute) and use the Java. Script get. Element. By. Id method.
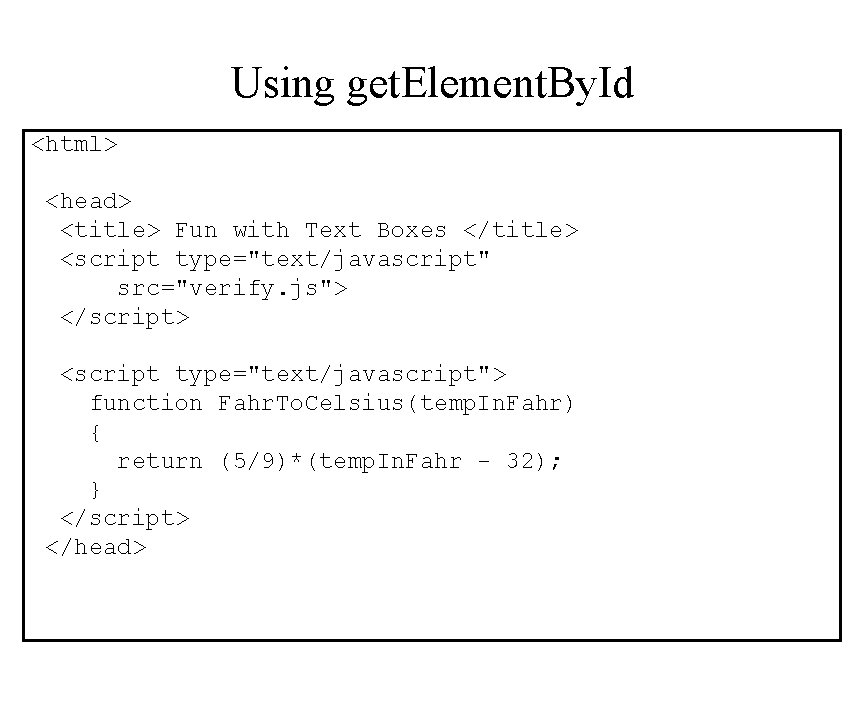
Using get. Element. By. Id <html> <head> <title> Fun with Text Boxes </title> <script type="text/javascript" src="verify. js"> </script> <script type="text/javascript"> function Fahr. To. Celsius(temp. In. Fahr) { return (5/9)*(temp. In. Fahr - 32); } </script> </head>
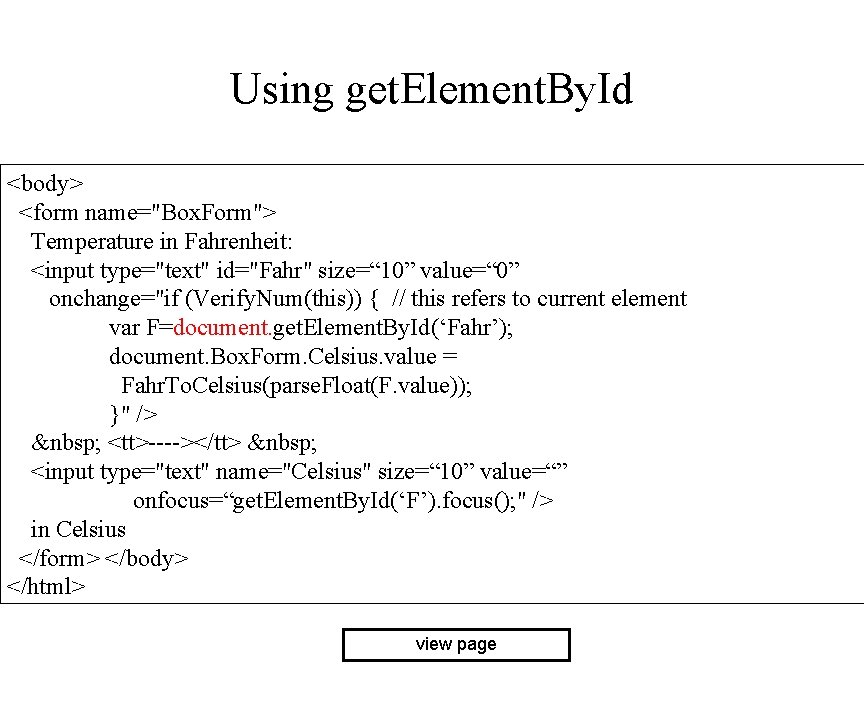
Using get. Element. By. Id <body> <form name="Box. Form"> Temperature in Fahrenheit: <input type="text" id="Fahr" size=“ 10” value=“ 0” onchange="if (Verify. Num(this)) { // this refers to current element var F=document. get. Element. By. Id(‘Fahr’); document. Box. Form. Celsius. value = Fahr. To. Celsius(parse. Float(F. value)); }" /> <tt>----></tt> <input type="text" name="Celsius" size=“ 10” value=“” onfocus=“get. Element. By. Id(‘F’). focus(); " /> in Celsius </form> </body> </html> view page
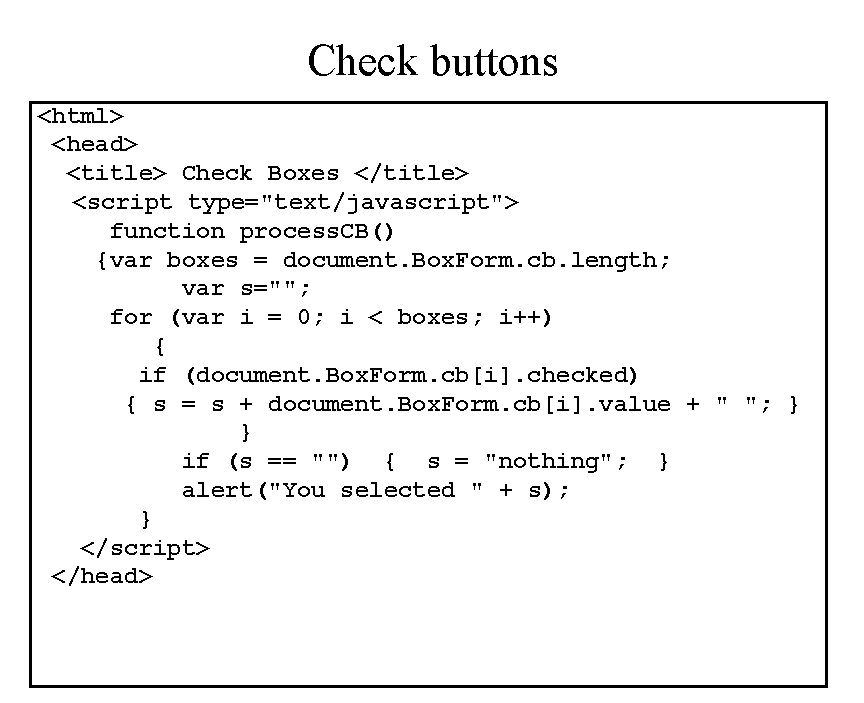
Check buttons <html> <head> <title> Check Boxes </title> <script type="text/javascript"> function process. CB() {var boxes = document. Box. Form. cb. length; var s=""; for (var i = 0; i < boxes; i++) { if (document. Box. Form. cb[i]. checked) { s = s + document. Box. Form. cb[i]. value + " "; } } if (s == "") { s = "nothing"; } alert("You selected " + s); } </script> </head>
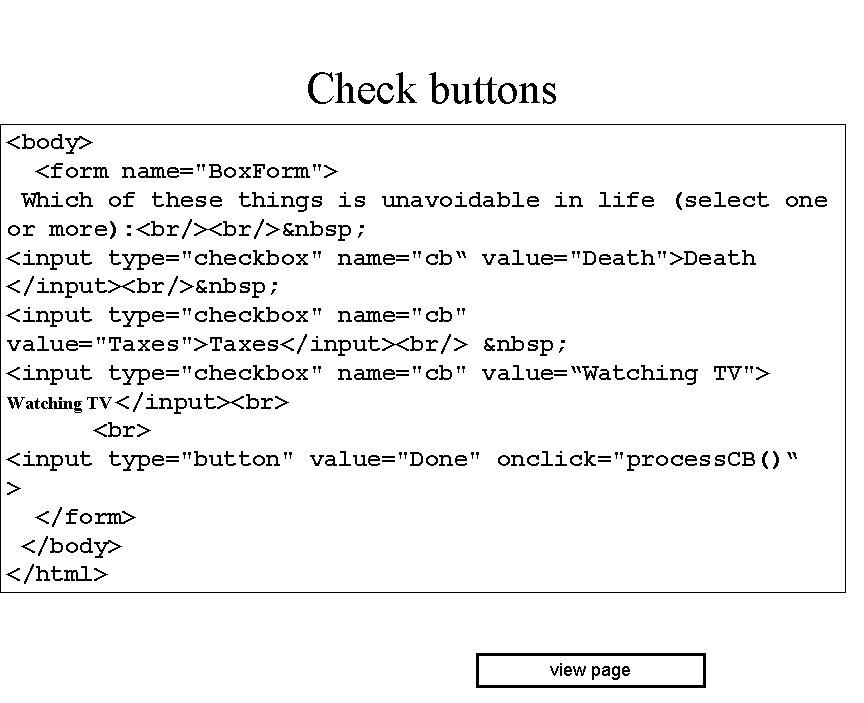
Check buttons <body> <form name="Box. Form"> Which of these things is unavoidable in life (select one or more): <br/> <input type="checkbox" name="cb“ value="Death">Death </input><br/> <input type="checkbox" name="cb" value="Taxes">Taxes</input><br/> <input type="checkbox" name="cb" value=“Watching TV"> Watching TV </input> <input type="button" value="Done" onclick="process. CB()“ > </form> </body> </html> view page
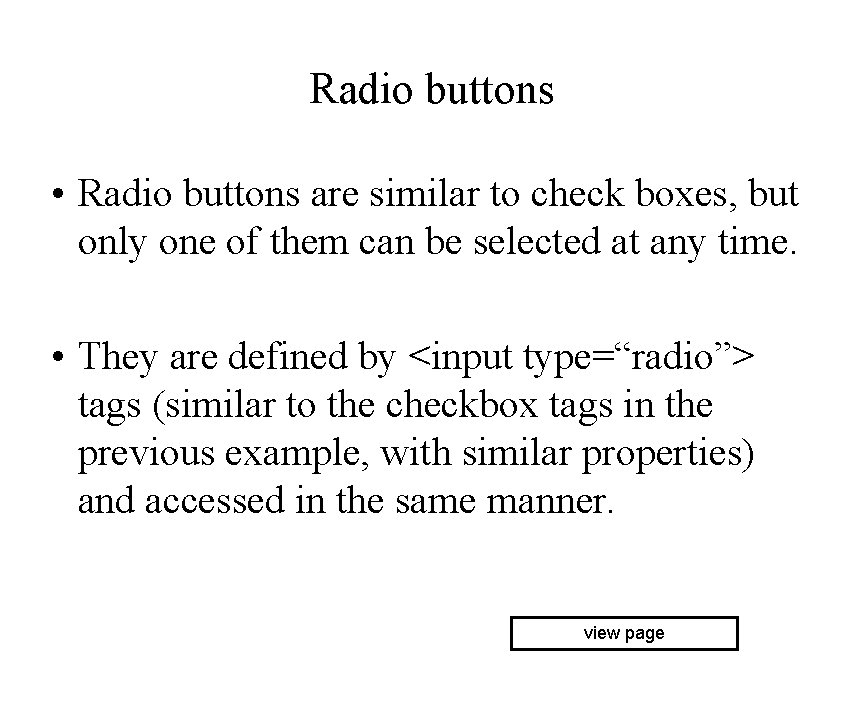
Radio buttons • Radio buttons are similar to check boxes, but only one of them can be selected at any time. • They are defined by <input type=“radio”> tags (similar to the checkbox tags in the previous example, with similar properties) and accessed in the same manner. view page
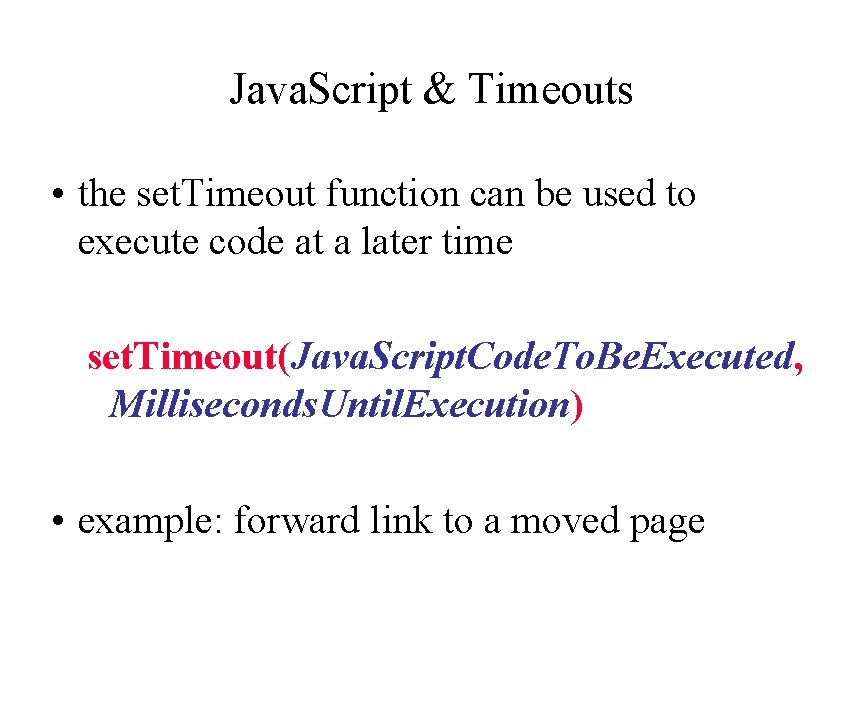
Java. Script & Timeouts • the set. Timeout function can be used to execute code at a later time set. Timeout(Java. Script. Code. To. Be. Executed, Milliseconds. Until. Execution) • example: forward link to a moved page
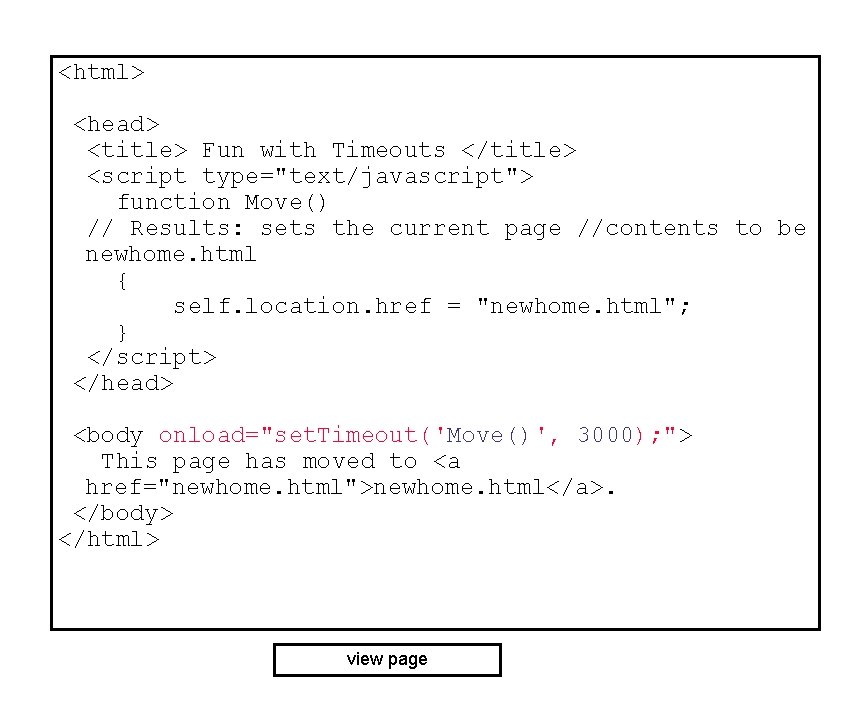
<html> <head> <title> Fun with Timeouts </title> <script type="text/javascript"> function Move() // Results: sets the current page //contents to be newhome. html { self. location. href = "newhome. html"; } </script> </head> <body onload="set. Timeout('Move()', 3000); "> This page has moved to <a href="newhome. html">newhome. html</a>. </body> </html> view page
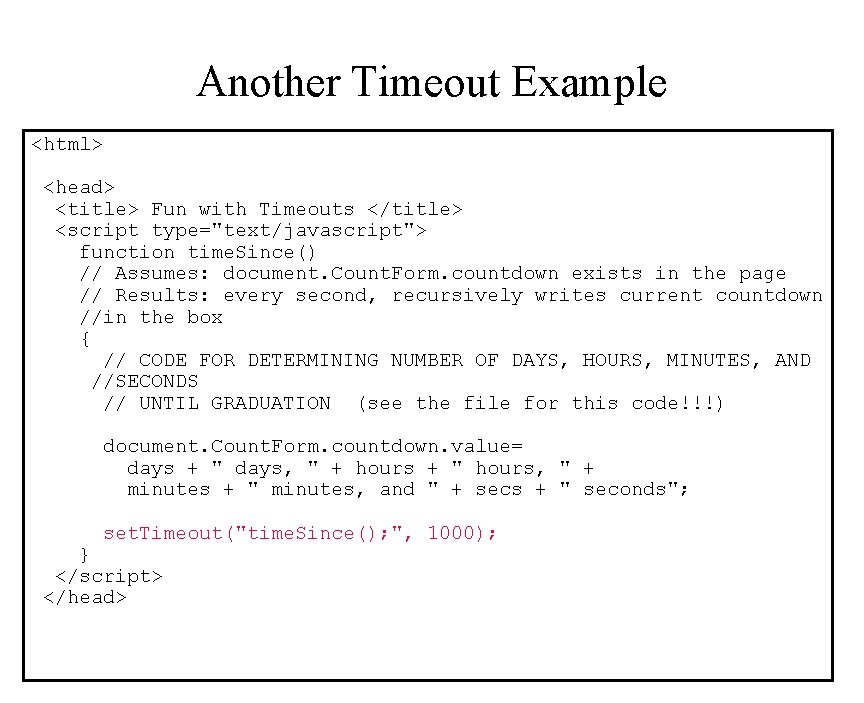
Another Timeout Example <html> <head> <title> Fun with Timeouts </title> <script type="text/javascript"> function time. Since() // Assumes: document. Count. Form. countdown exists in the page // Results: every second, recursively writes current countdown //in the box { // CODE FOR DETERMINING NUMBER OF DAYS, HOURS, MINUTES, AND //SECONDS // UNTIL GRADUATION (see the file for this code!!!) document. Count. Form. countdown. value= days + " days, " + hours + " hours, " + minutes + " minutes, and " + secs + " seconds"; set. Timeout("time. Since(); ", 1000); } </script> </head>
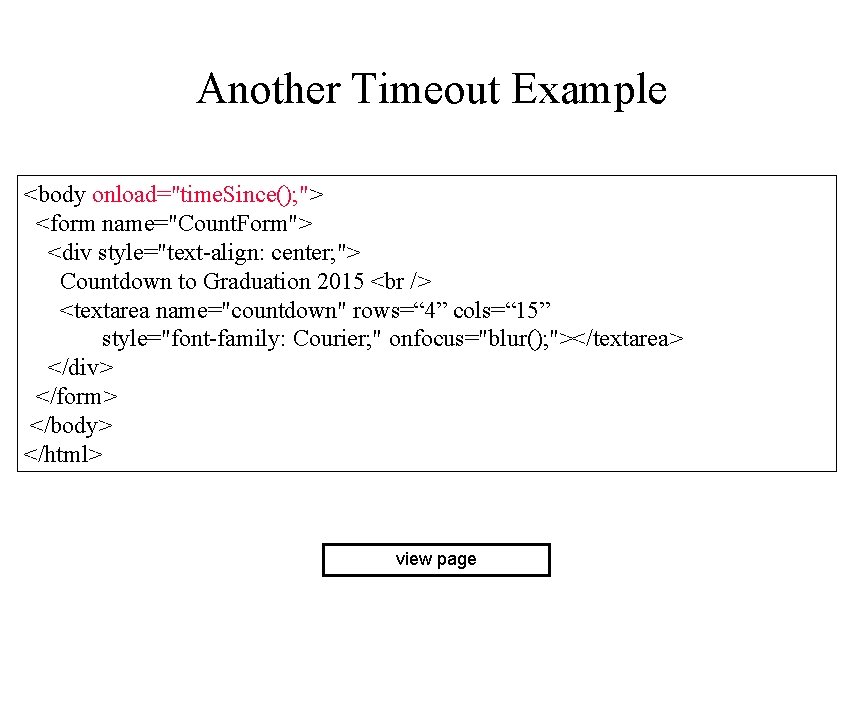
Another Timeout Example <body onload="time. Since(); "> <form name="Count. Form"> <div style="text-align: center; "> Countdown to Graduation 2015 <textarea name="countdown" rows=“ 4” cols=“ 15” style="font-family: Courier; " onfocus="blur(); "></textarea> </div> </form> </body> </html> view page