Classy Discussion Overloaded Methods Static Fields Static Methods
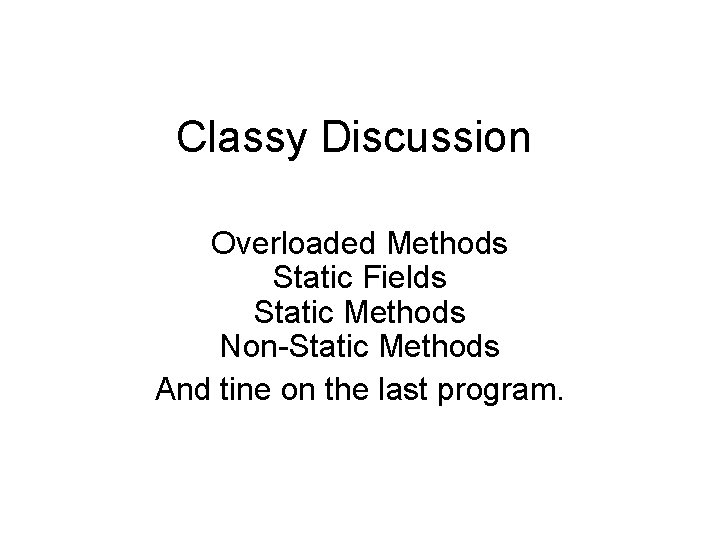
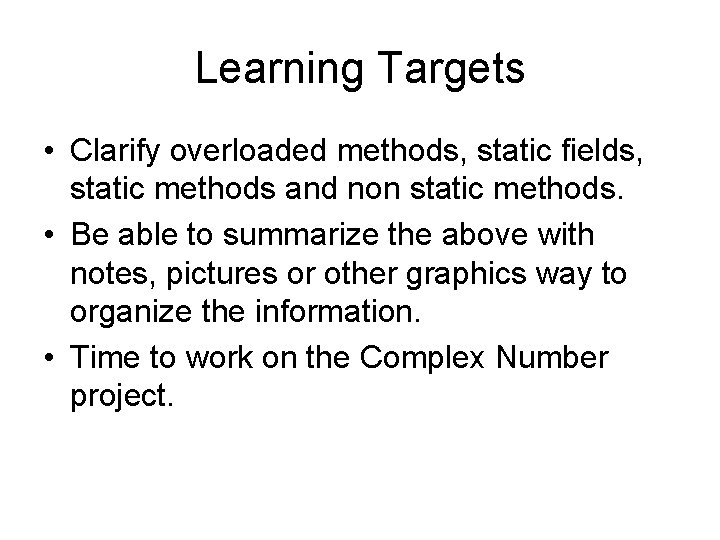
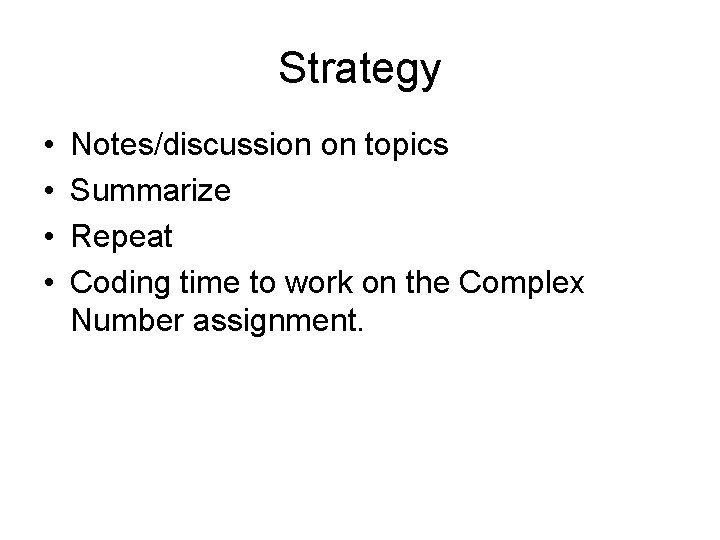
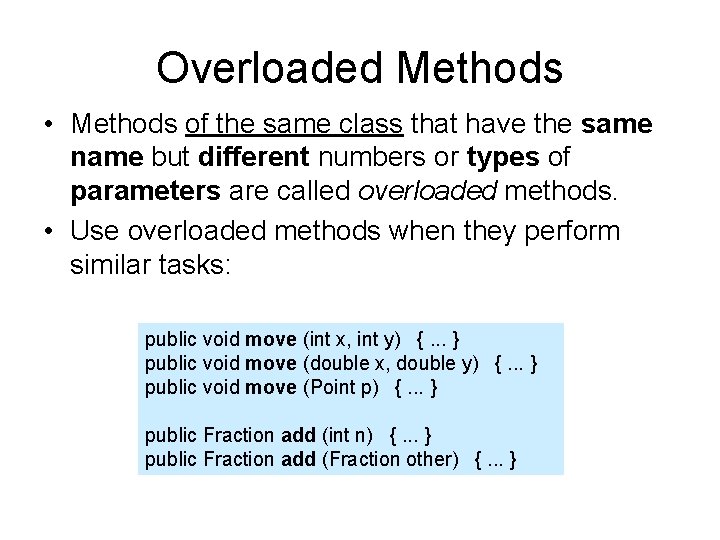
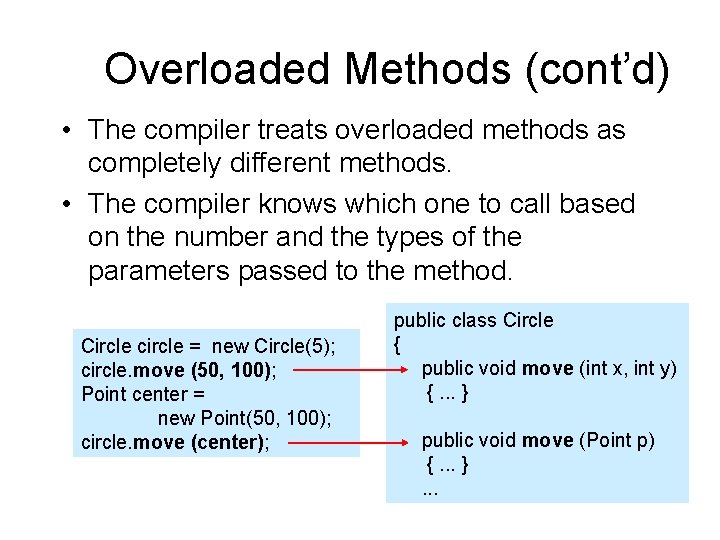
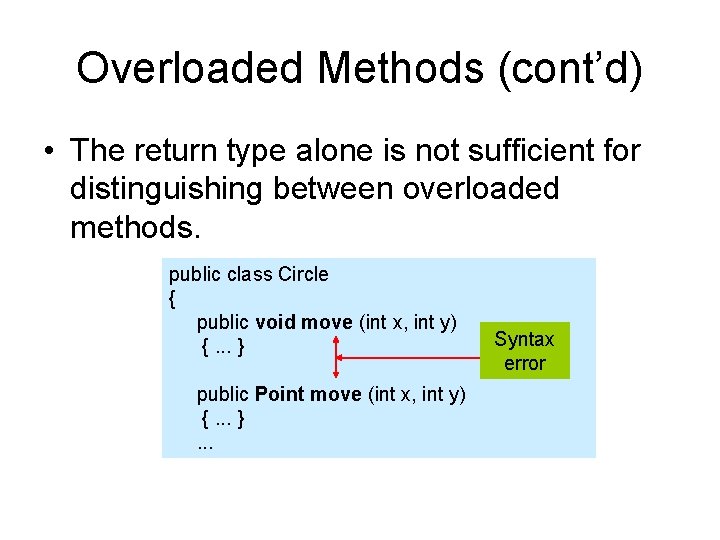
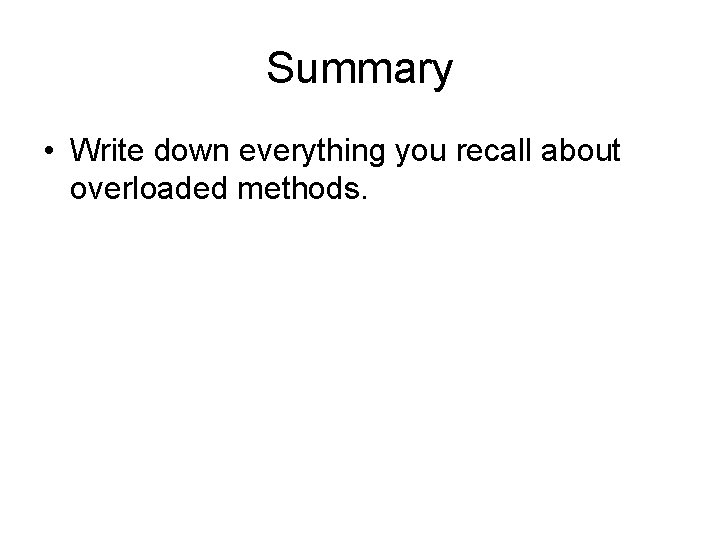
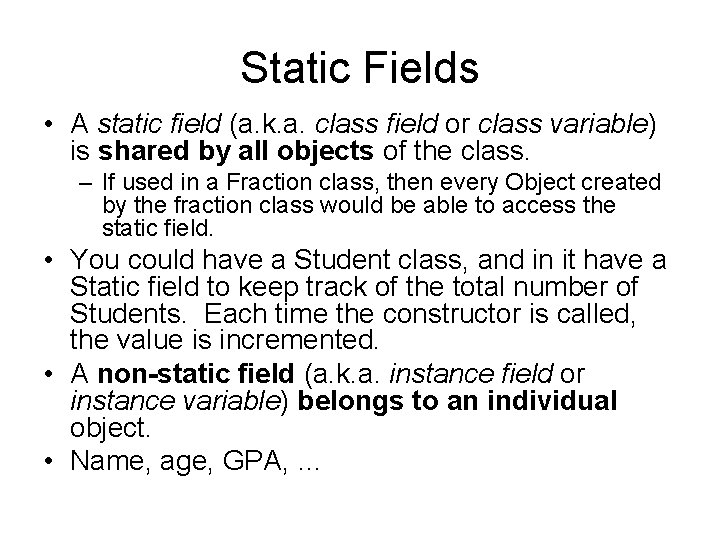
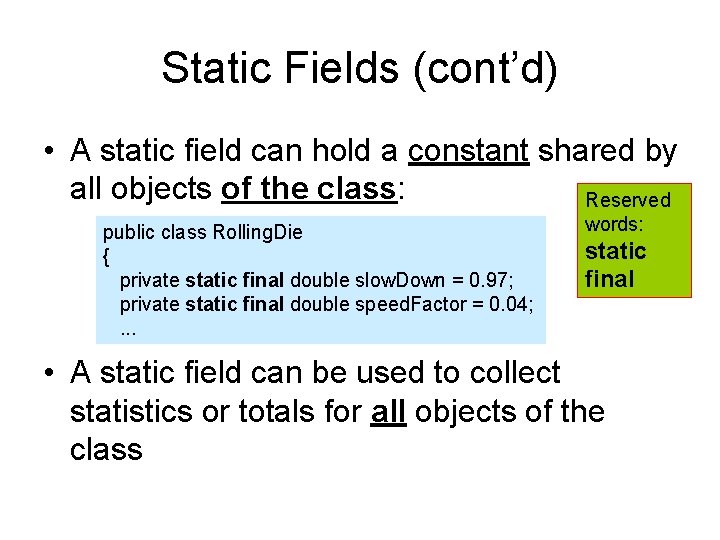
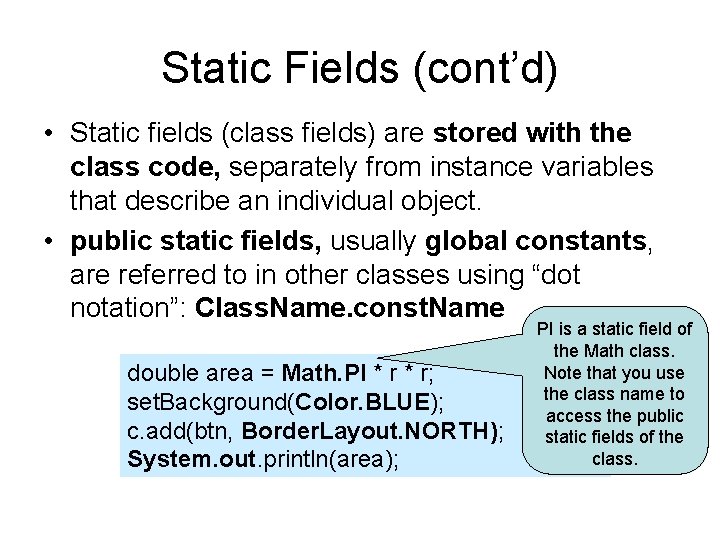
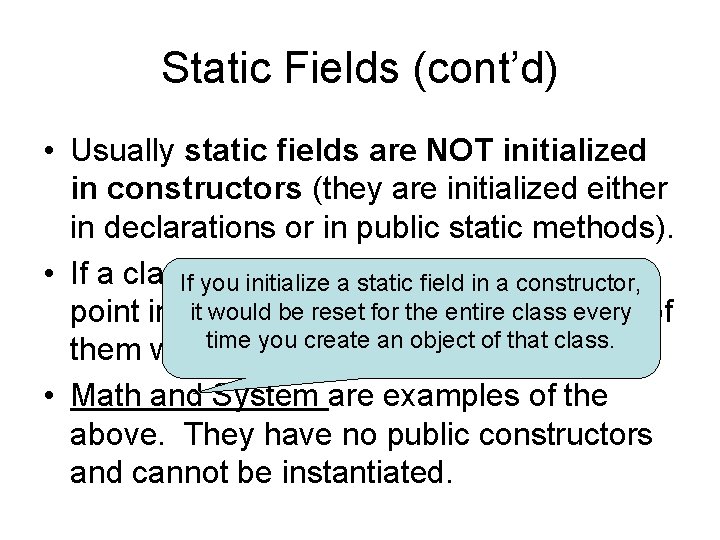
![Review public static void main (String [] args) 1. Consider the following class: { Review public static void main (String [] args) 1. Consider the following class: {](https://slidetodoc.com/presentation_image/0511e172a5eec60dd4e41292e4913f25/image-12.jpg)
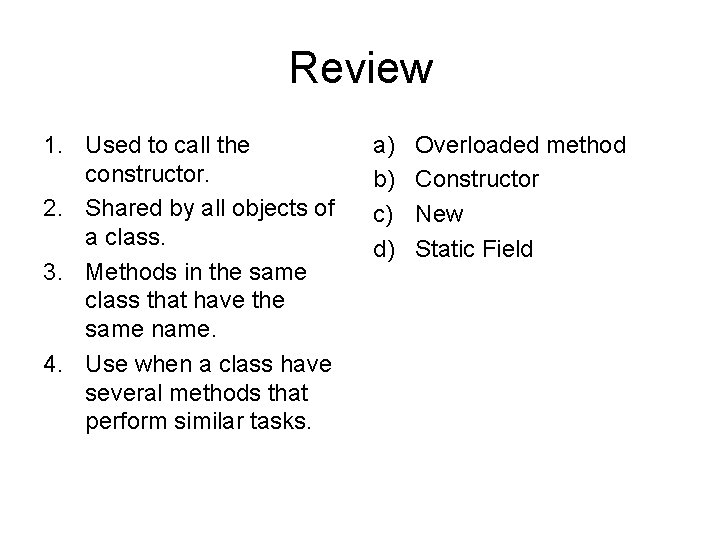
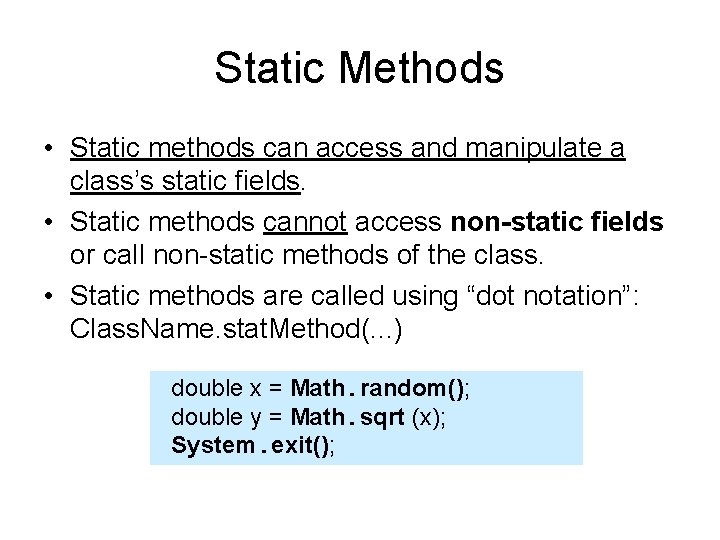
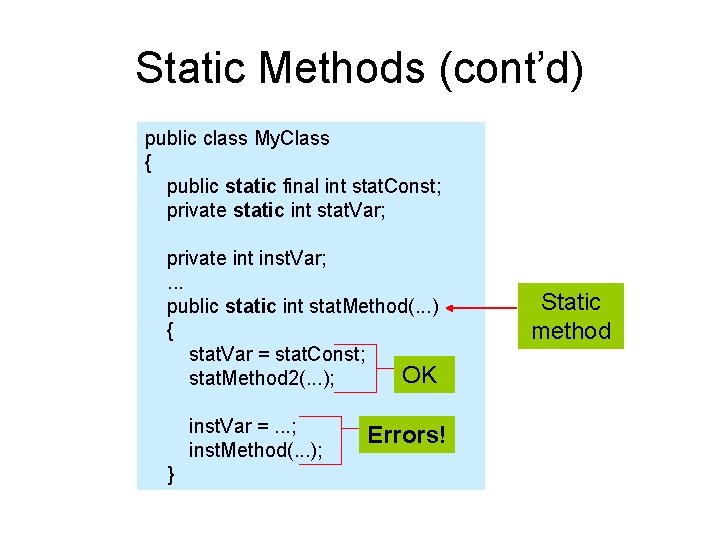
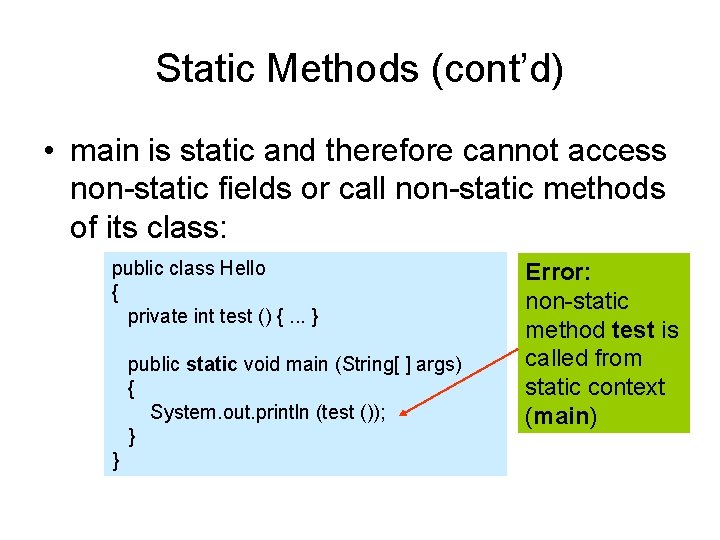
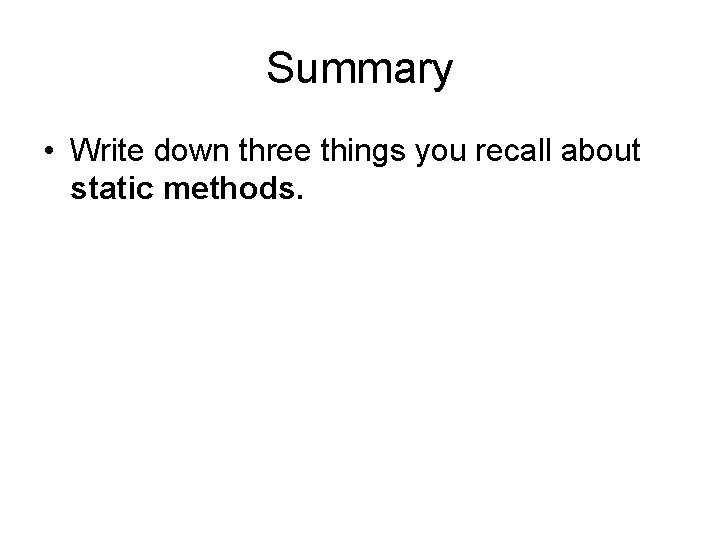
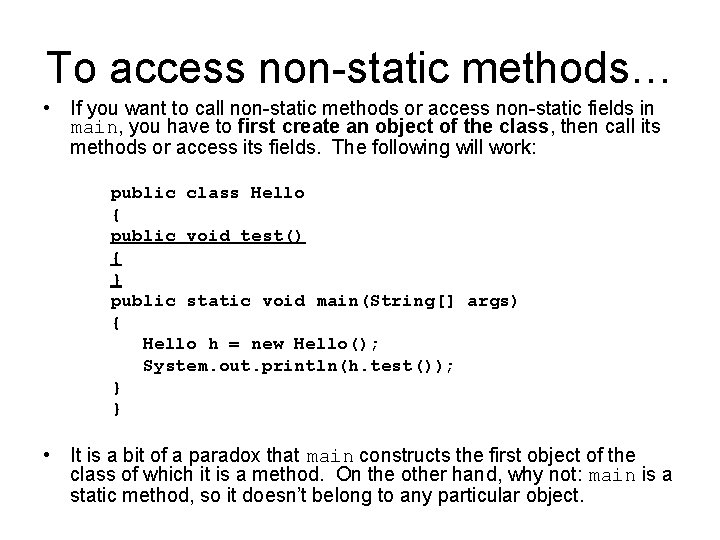
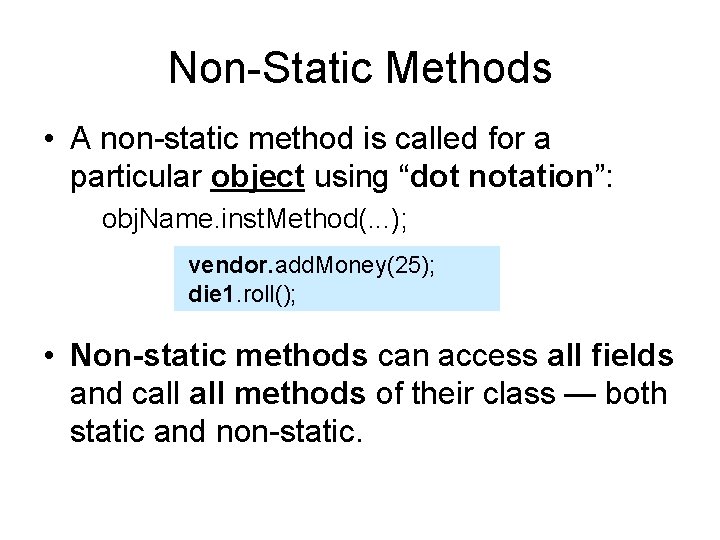
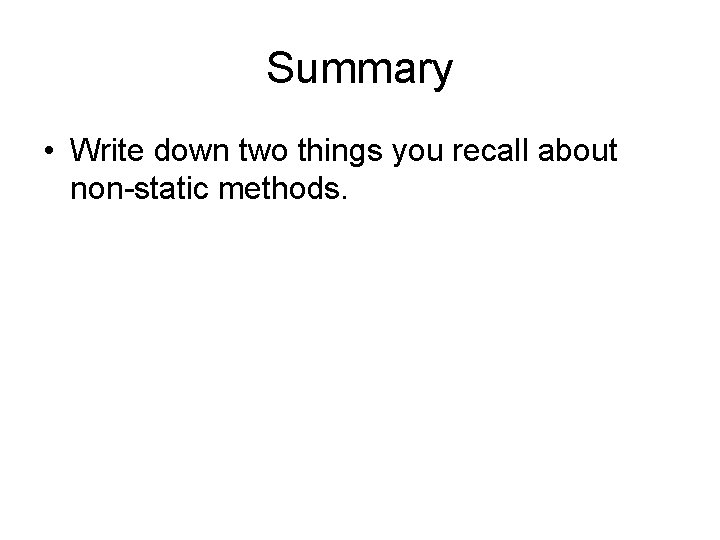
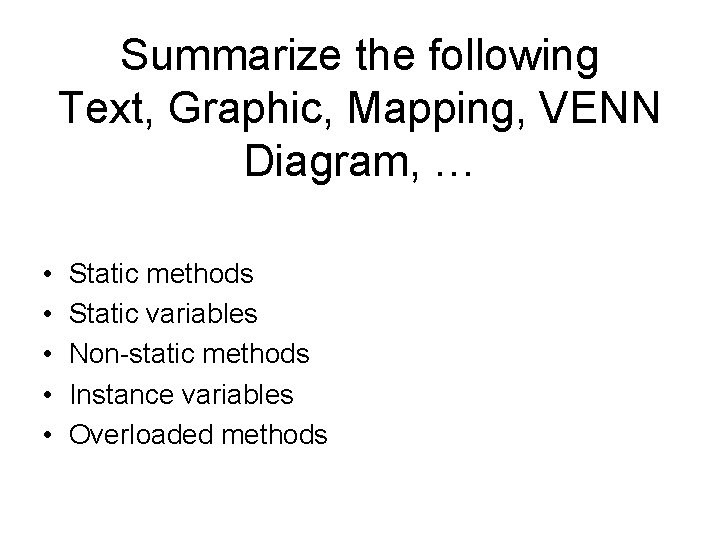
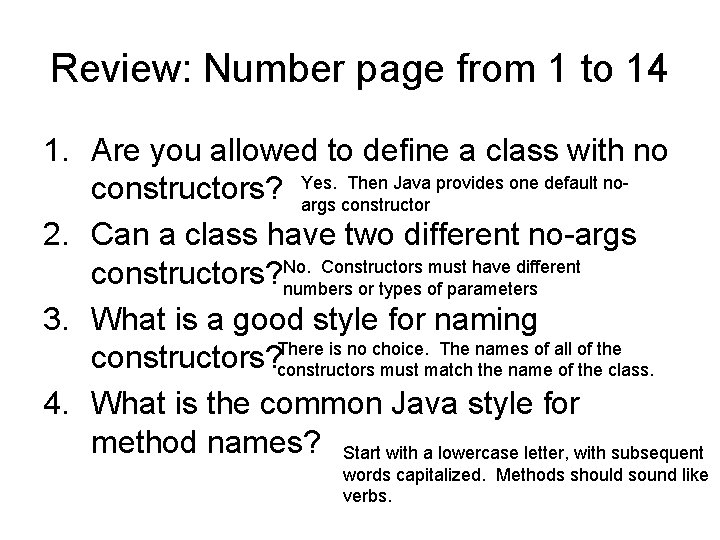
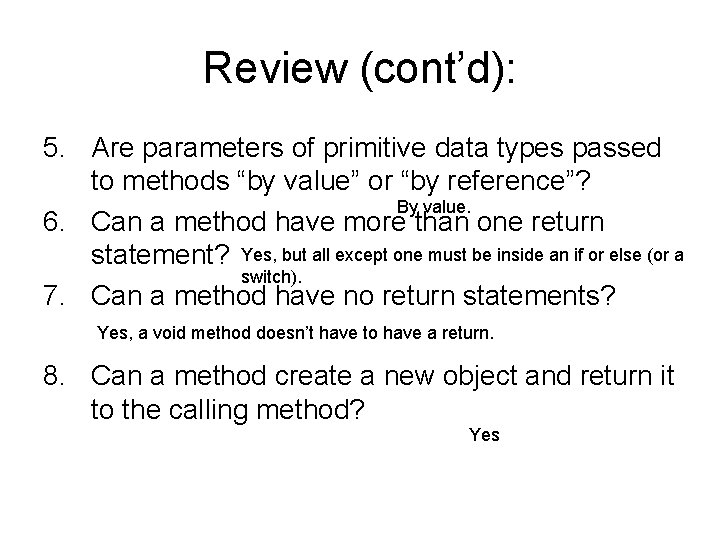
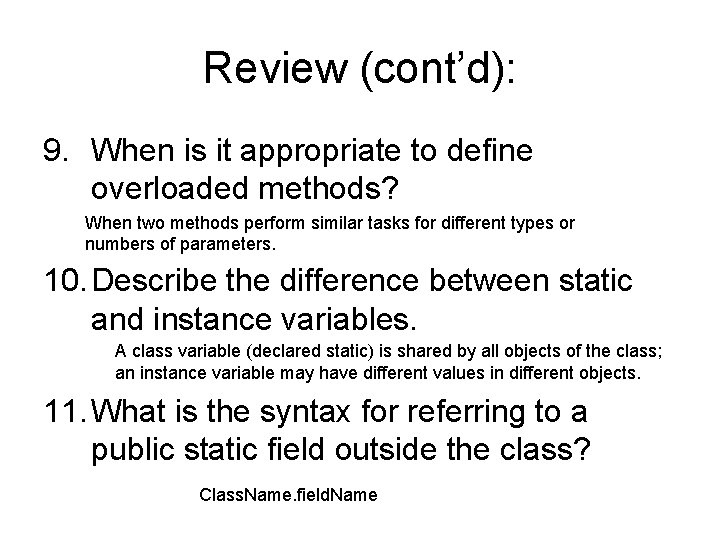
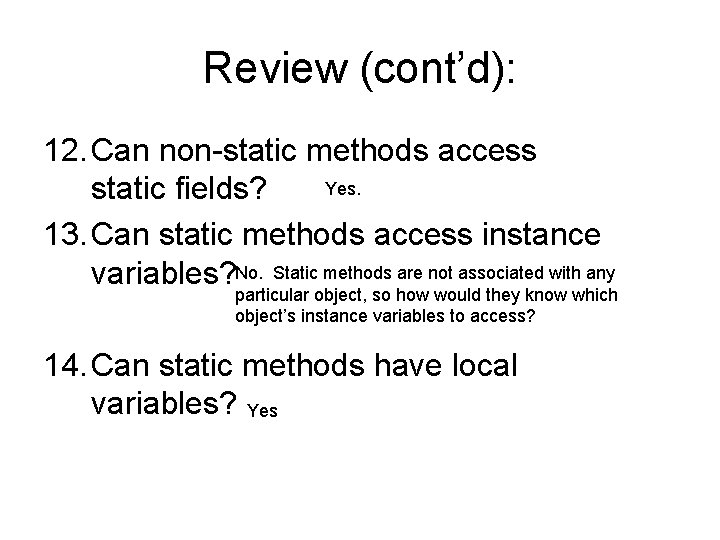
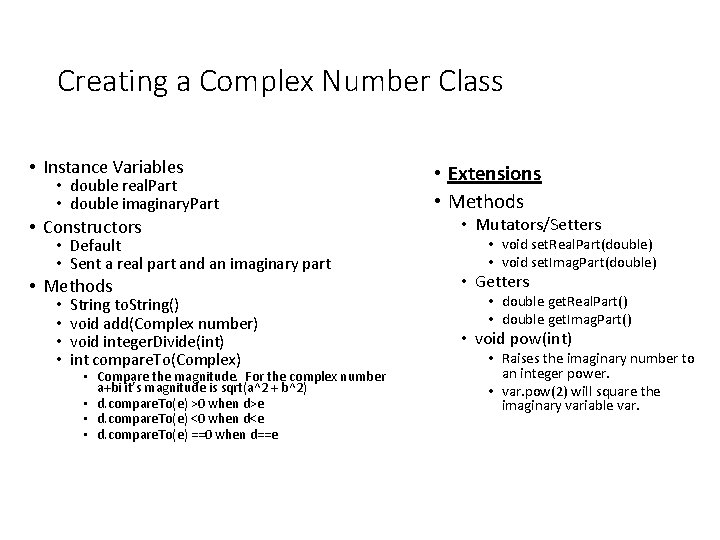
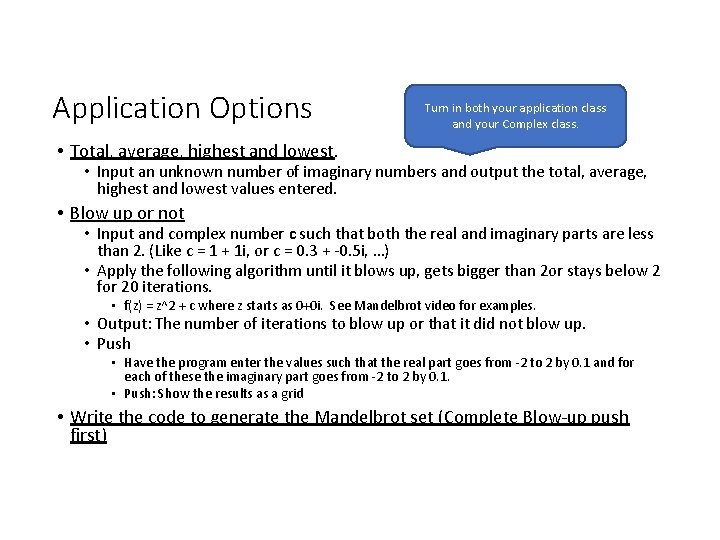
- Slides: 27
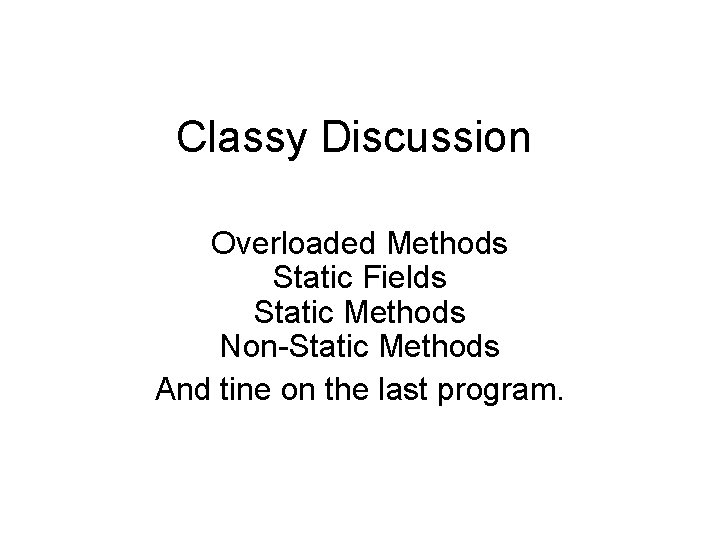
Classy Discussion Overloaded Methods Static Fields Static Methods Non-Static Methods And tine on the last program.
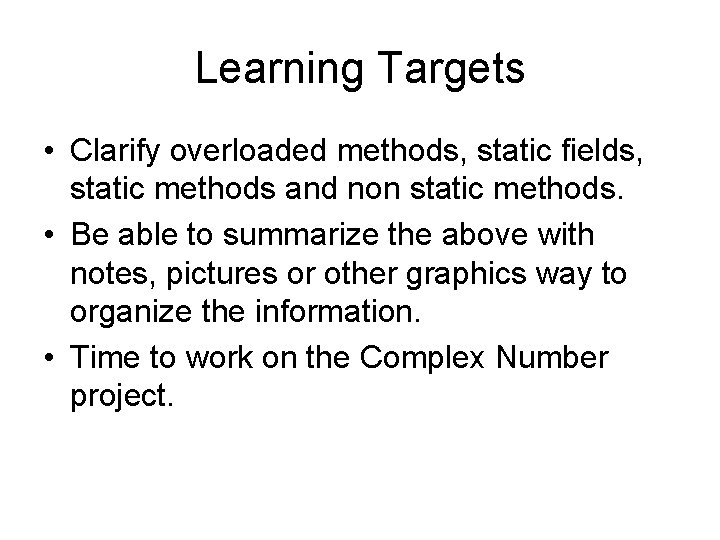
Learning Targets • Clarify overloaded methods, static fields, static methods and non static methods. • Be able to summarize the above with notes, pictures or other graphics way to organize the information. • Time to work on the Complex Number project.
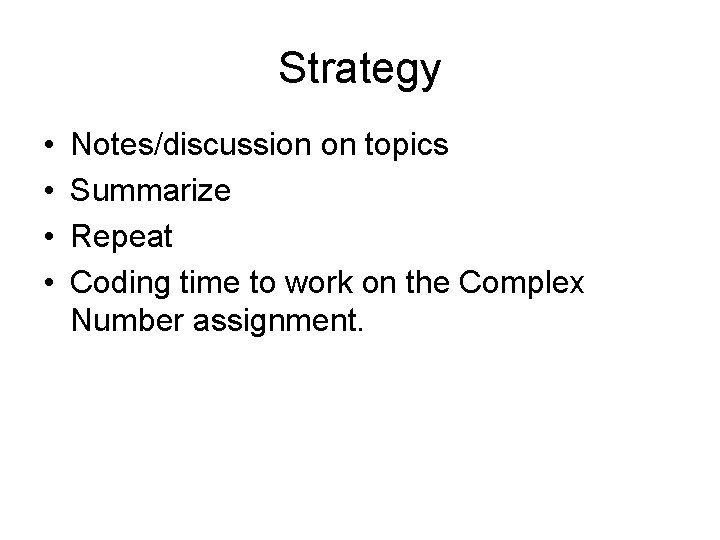
Strategy • • Notes/discussion on topics Summarize Repeat Coding time to work on the Complex Number assignment.
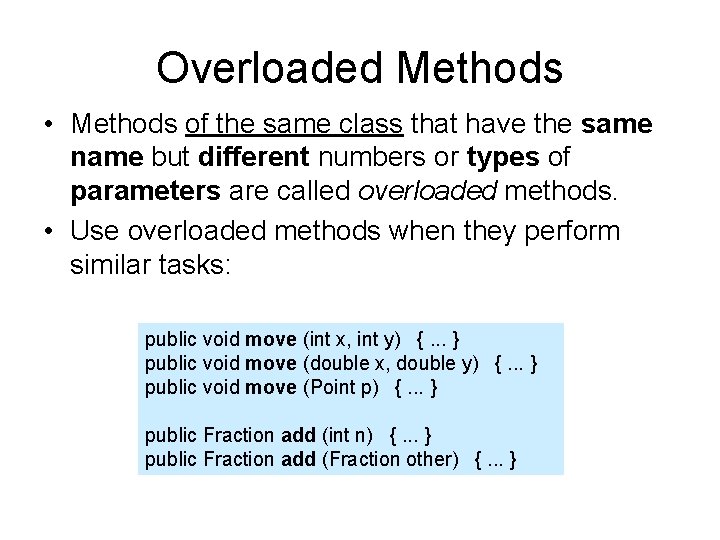
Overloaded Methods • Methods of the same class that have the same name but different numbers or types of parameters are called overloaded methods. • Use overloaded methods when they perform similar tasks: public void move (int x, int y) {. . . } public void move (double x, double y) {. . . } public void move (Point p) {. . . } public Fraction add (int n) {. . . } public Fraction add (Fraction other) {. . . }
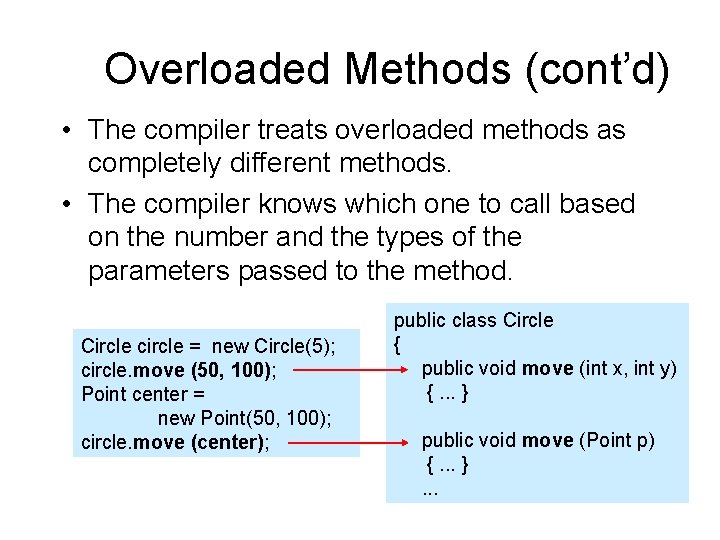
Overloaded Methods (cont’d) • The compiler treats overloaded methods as completely different methods. • The compiler knows which one to call based on the number and the types of the parameters passed to the method. Circle circle = new Circle(5); circle. move (50, 100); Point center = new Point(50, 100); circle. move (center); public class Circle { public void move (int x, int y) {. . . } public void move (Point p) {. . . }. . .
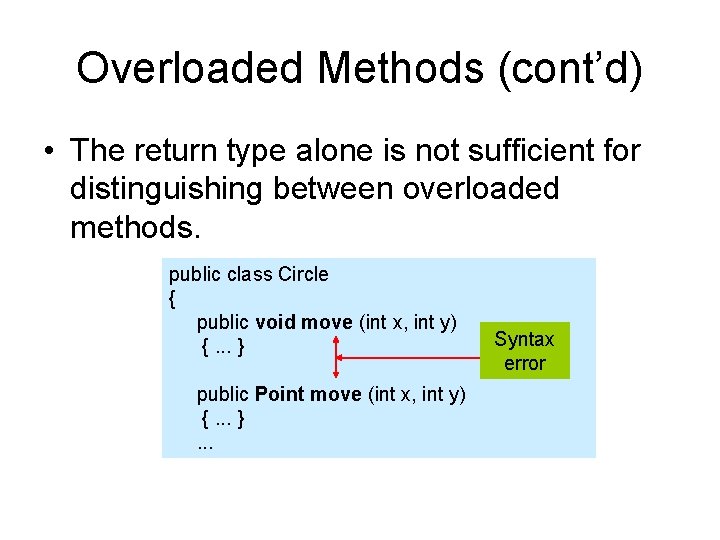
Overloaded Methods (cont’d) • The return type alone is not sufficient for distinguishing between overloaded methods. public class Circle { public void move (int x, int y) {. . . } public Point move (int x, int y) {. . . }. . . Syntax error
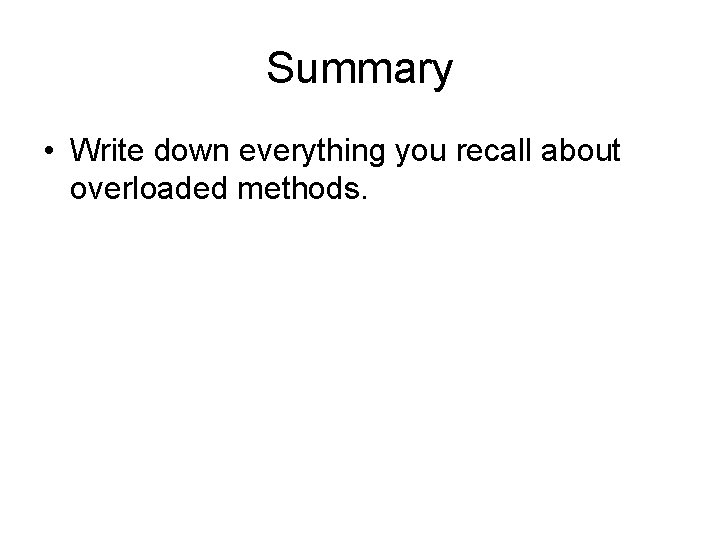
Summary • Write down everything you recall about overloaded methods.
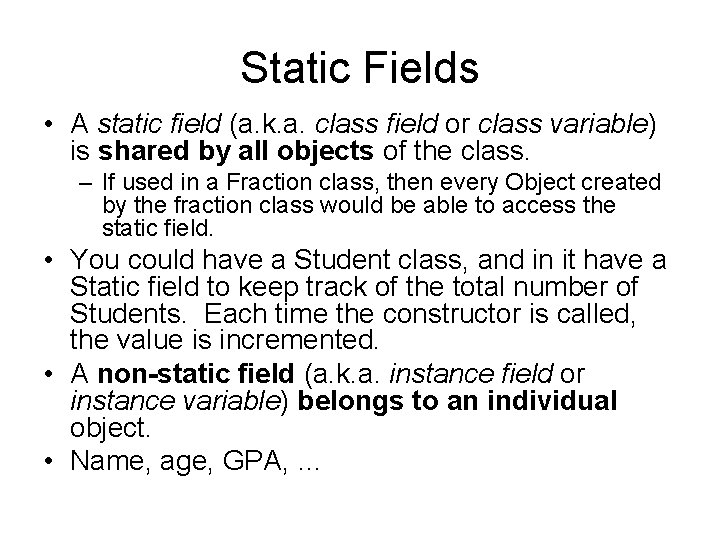
Static Fields • A static field (a. k. a. class field or class variable) is shared by all objects of the class. – If used in a Fraction class, then every Object created by the fraction class would be able to access the static field. • You could have a Student class, and in it have a Static field to keep track of the total number of Students. Each time the constructor is called, the value is incremented. • A non-static field (a. k. a. instance field or instance variable) belongs to an individual object. • Name, age, GPA, …
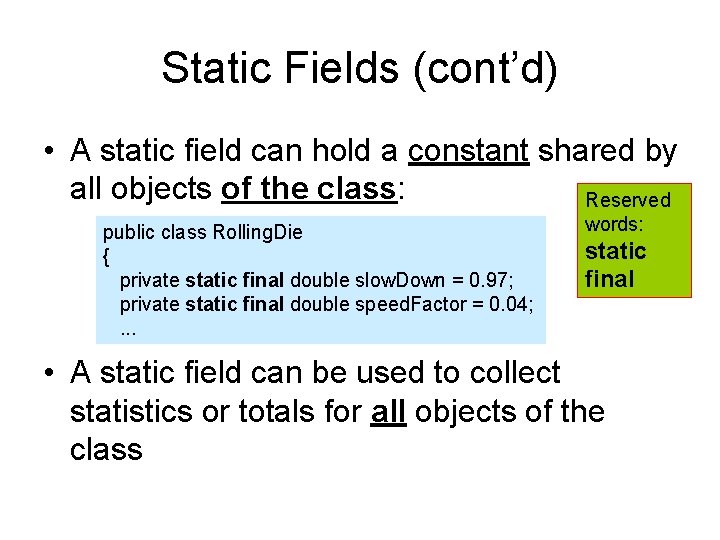
Static Fields (cont’d) • A static field can hold a constant shared by all objects of the class: Reserved public class Rolling. Die { private static final double slow. Down = 0. 97; private static final double speed. Factor = 0. 04; . . . words: static final • A static field can be used to collect statistics or totals for all objects of the class
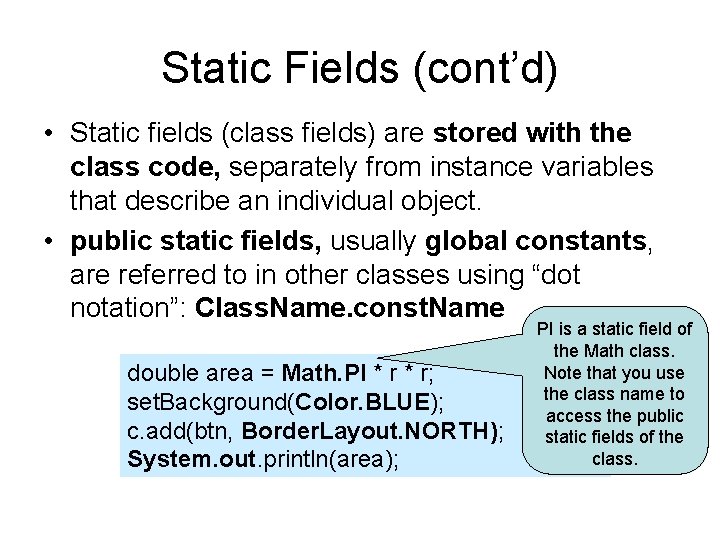
Static Fields (cont’d) • Static fields (class fields) are stored with the class code, separately from instance variables that describe an individual object. • public static fields, usually global constants, are referred to in other classes using “dot notation”: Class. Name. const. Name double area = Math. PI * r; set. Background(Color. BLUE); c. add(btn, Border. Layout. NORTH); System. out. println(area); PI is a static field of the Math class. Note that you use the class name to access the public static fields of the class.
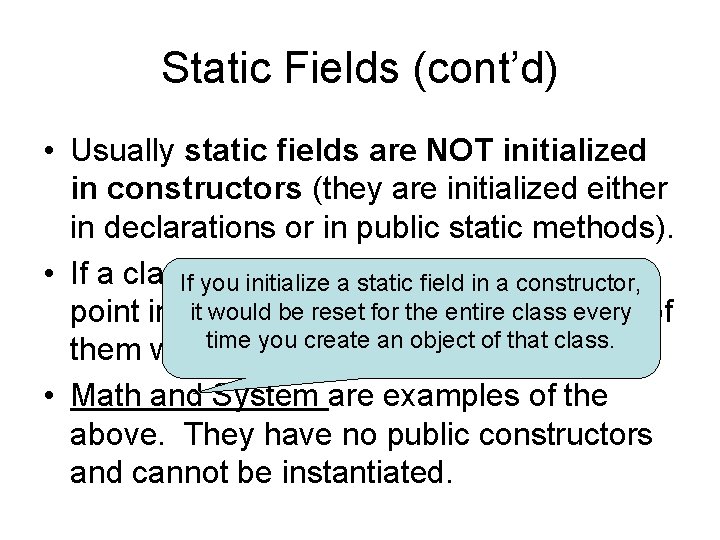
Static Fields (cont’d) • Usually static fields are NOT initialized in constructors (they are initialized either in declarations or in public static methods). • If a class. If you hasinitialize only static is no a static fields, field in athere constructor, it would beobjects reset for the point in creating of entire that classevery (all of time you create an object of that class. them would be identical). • Math and System are examples of the above. They have no public constructors and cannot be instantiated.
![Review public static void main String args 1 Consider the following class Review public static void main (String [] args) 1. Consider the following class: {](https://slidetodoc.com/presentation_image/0511e172a5eec60dd4e41292e4913f25/image-12.jpg)
Review public static void main (String [] args) 1. Consider the following class: { Identify. My. Parts a = new Identify. My. Parts(); Identify. My. Parts b = new Identify. My. Parts(); public class Identify. My. Parts { – public static int x = 7; – public int y = 3; } a. y = 5; b. y = 6; Identify. My. Parts. x = 1; b. x = 2; System. out. println("a. y = " + a. y); System. out. println("b. y = " + b. y); System. out. println("Identify. My. Parts. x = " + a. x); – a. What are the class variables? } – b. What are the instance variables? – c. What is the output from the following code: System. out. println("b. x = " + b. x);
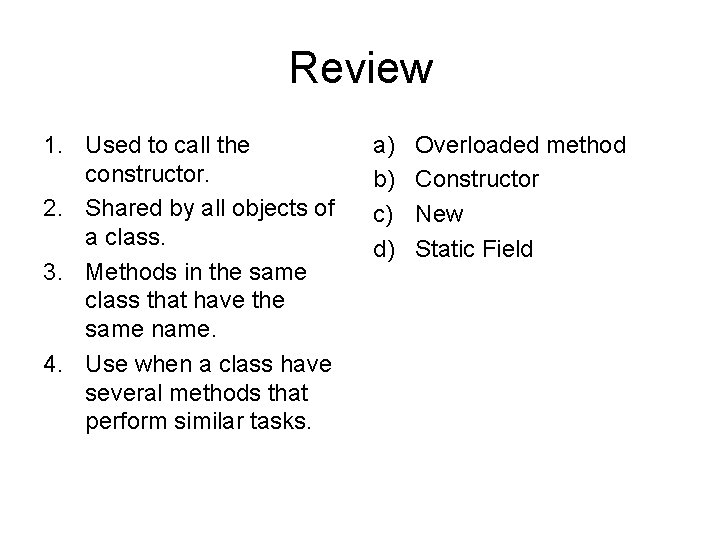
Review 1. Used to call the constructor. 2. Shared by all objects of a class. 3. Methods in the same class that have the same name. 4. Use when a class have several methods that perform similar tasks. a) b) c) d) Overloaded method Constructor New Static Field
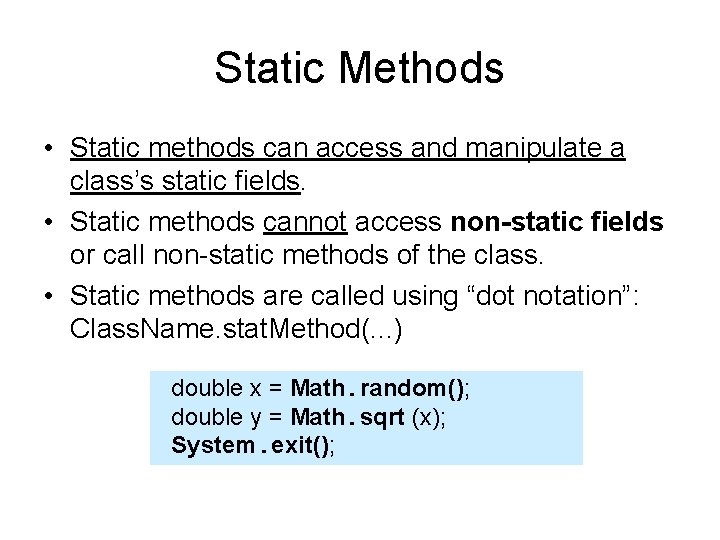
Static Methods • Static methods can access and manipulate a class’s static fields. • Static methods cannot access non-static fields or call non-static methods of the class. • Static methods are called using “dot notation”: Class. Name. stat. Method(. . . ) double x = Math. random(); double y = Math. sqrt (x); System. exit();
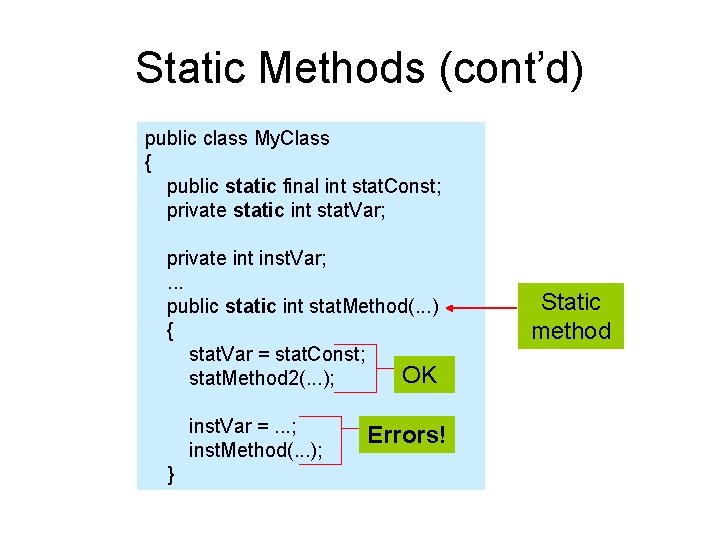
Static Methods (cont’d) public class My. Class { public static final int stat. Const; private static int stat. Var; private int inst. Var; . . . public static int stat. Method(. . . ) { stat. Var = stat. Const; OK stat. Method 2(. . . ); inst. Var =. . . ; inst. Method(. . . ); } Errors! Static method
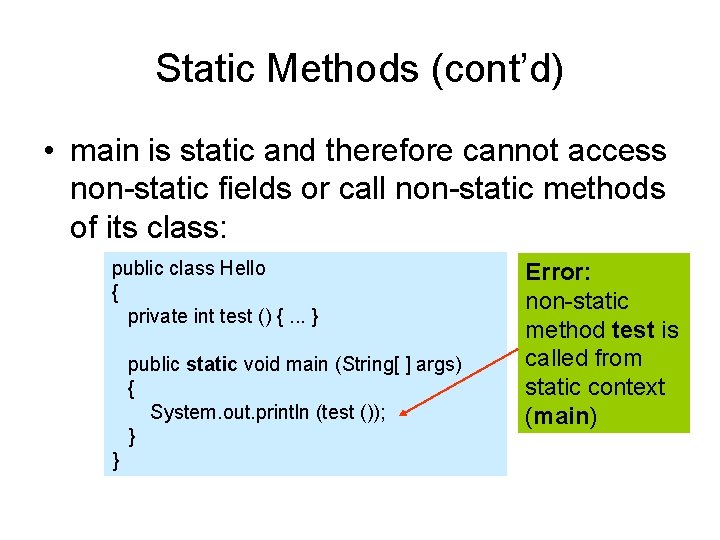
Static Methods (cont’d) • main is static and therefore cannot access non-static fields or call non-static methods of its class: public class Hello { private int test () {. . . } public static void main (String[ ] args) { System. out. println (test ()); } } Error: non-static method test is called from static context (main)
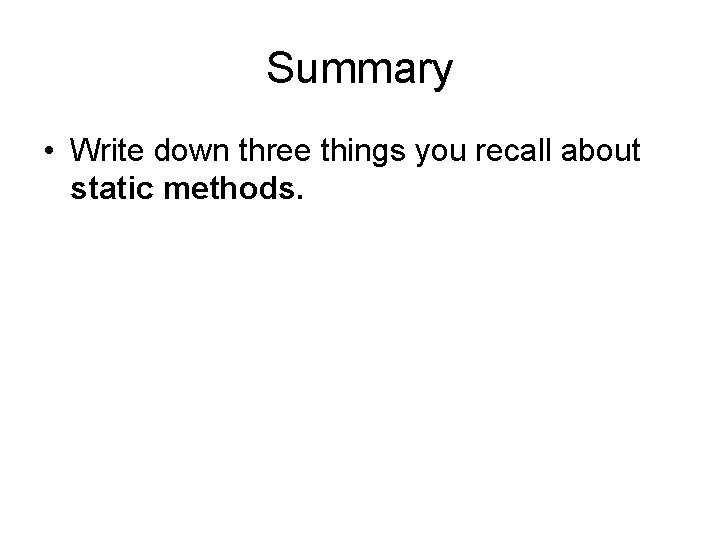
Summary • Write down three things you recall about static methods.
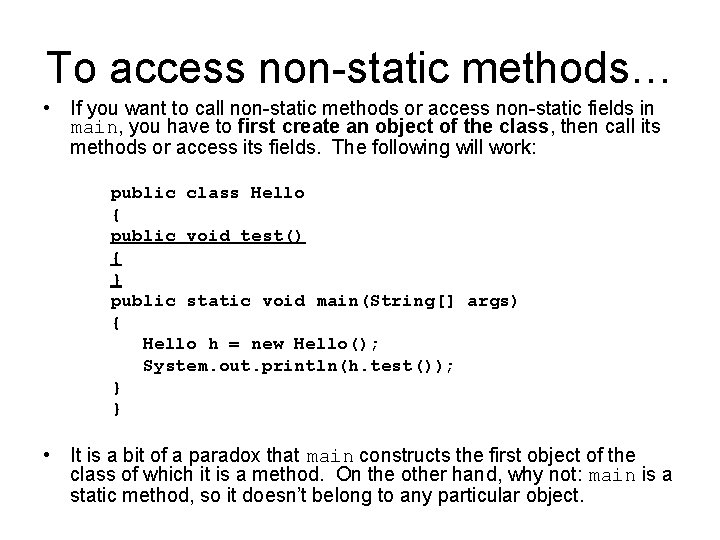
To access non-static methods… • If you want to call non-static methods or access non-static fields in main, you have to first create an object of the class, then call its methods or access its fields. The following will work: public class Hello { public void test() { } public static void main(String[] args) { Hello h = new Hello(); System. out. println(h. test()); } } • It is a bit of a paradox that main constructs the first object of the class of which it is a method. On the other hand, why not: main is a static method, so it doesn’t belong to any particular object.
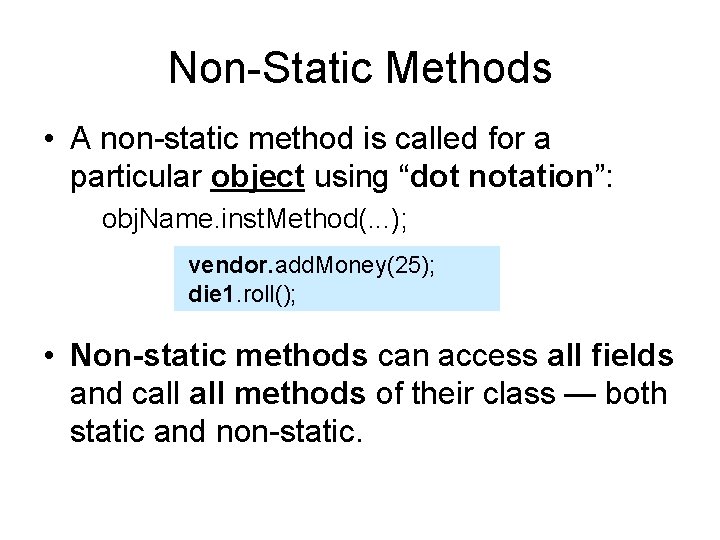
Non-Static Methods • A non-static method is called for a particular object using “dot notation”: obj. Name. inst. Method(. . . ); vendor. add. Money(25); die 1. roll(); • Non-static methods can access all fields and call methods of their class — both static and non-static.
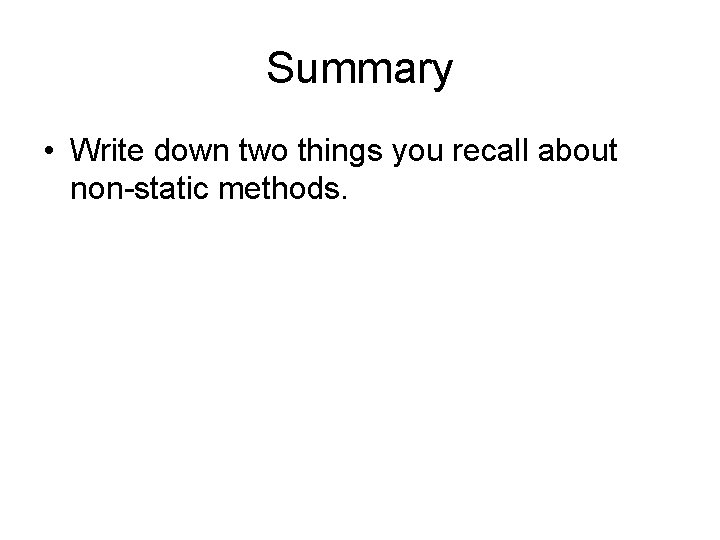
Summary • Write down two things you recall about non-static methods.
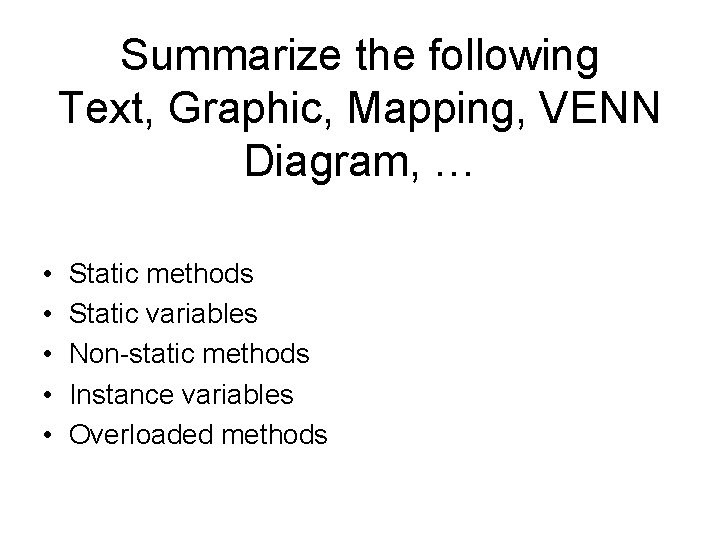
Summarize the following Text, Graphic, Mapping, VENN Diagram, … • • • Static methods Static variables Non-static methods Instance variables Overloaded methods
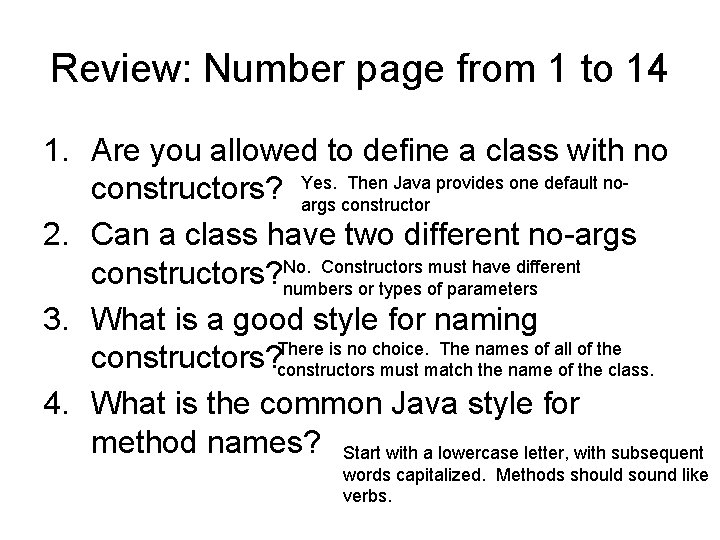
Review: Number page from 1 to 14 1. Are you allowed to define a class with no Then Java provides one default noconstructors? Yes. args constructor 2. Can a class have two different no-args Constructors must have different constructors? No. numbers or types of parameters 3. What is a good style for naming There is no choice. The names of all of the constructors? constructors must match the name of the class. 4. What is the common Java style for method names? Start with a lowercase letter, with subsequent words capitalized. Methods should sound like verbs.
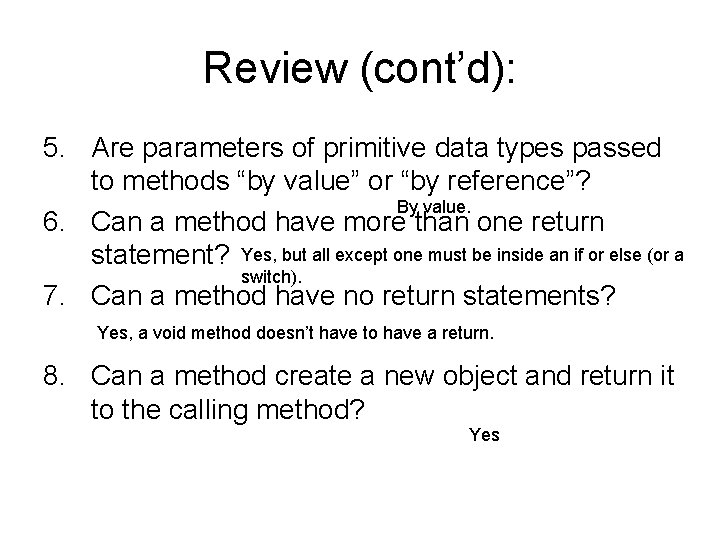
Review (cont’d): 5. Are parameters of primitive data types passed to methods “by value” or “by reference”? By value. 6. Can a method have more than one return statement? Yes, but all except one must be inside an if or else (or a switch). 7. Can a method have no return statements? Yes, a void method doesn’t have to have a return. 8. Can a method create a new object and return it to the calling method? Yes
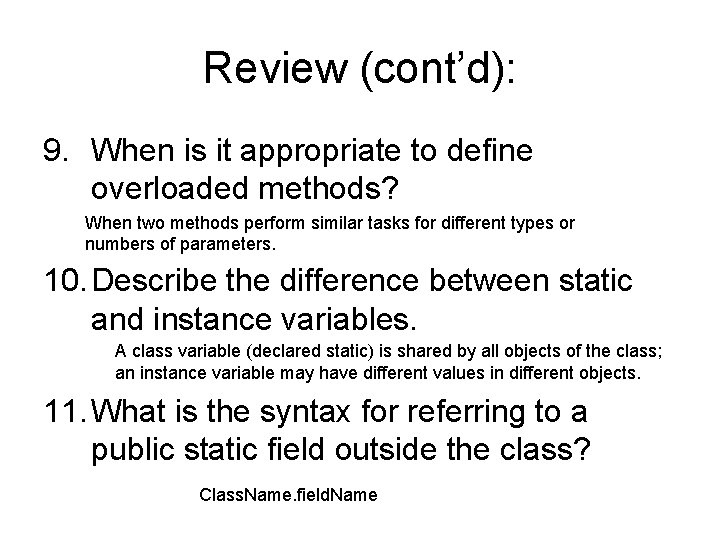
Review (cont’d): 9. When is it appropriate to define overloaded methods? When two methods perform similar tasks for different types or numbers of parameters. 10. Describe the difference between static and instance variables. A class variable (declared static) is shared by all objects of the class; an instance variable may have different values in different objects. 11. What is the syntax for referring to a public static field outside the class? Class. Name. field. Name
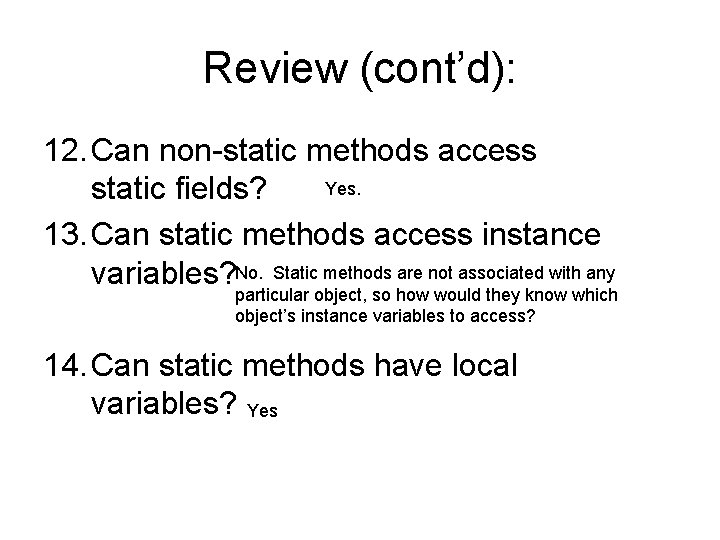
Review (cont’d): 12. Can non-static methods access Yes. static fields? 13. Can static methods access instance variables? No. Static methods are not associated with any particular object, so how would they know which object’s instance variables to access? 14. Can static methods have local variables? Yes
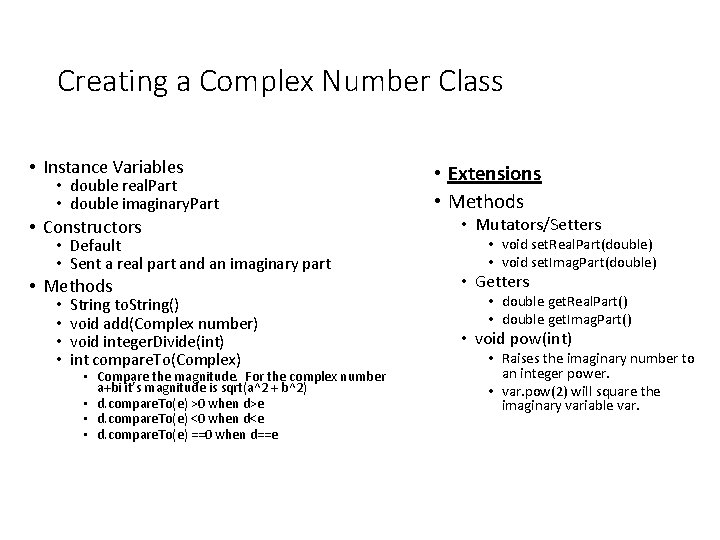
Creating a Complex Number Class • Instance Variables • double real. Part • double imaginary. Part • Extensions • Methods • Constructors • Mutators/Setters • Methods • Getters • Default • Sent a real part and an imaginary part • • String to. String() void add(Complex number) void integer. Divide(int) int compare. To(Complex) • Compare the magnitude. For the complex number a+bi it’s magnitude is sqrt(a^2 + b^2) • d. compare. To(e) >0 when d>e • d. compare. To(e) <0 when d<e • d. compare. To(e) ==0 when d==e • void set. Real. Part(double) • void set. Imag. Part(double) • double get. Real. Part() • double get. Imag. Part() • void pow(int) • Raises the imaginary number to an integer power. • var. pow(2) will square the imaginary variable var.
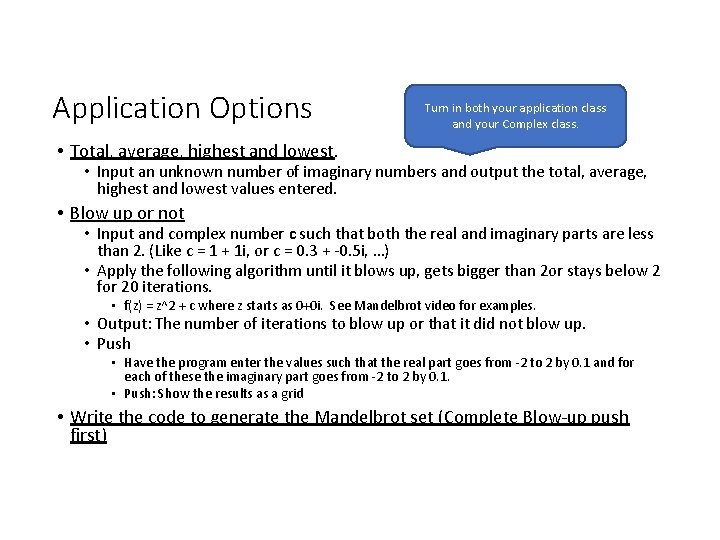
Application Options Turn in both your application class and your Complex class. • Total, average, highest and lowest. • Input an unknown number of imaginary numbers and output the total, average, highest and lowest values entered. • Blow up or not • Input and complex number c such that both the real and imaginary parts are less than 2. (Like c = 1 + 1 i, or c = 0. 3 + -0. 5 i, …) • Apply the following algorithm until it blows up, gets bigger than 2 or stays below 2 for 20 iterations. • f(z) = z^2 + c where z starts as 0+0 i. See Mandelbrot video for examples. • Output: The number of iterations to blow up or that it did not blow up. • Push • Have the program enter the values such that the real part goes from -2 to 2 by 0. 1 and for each of these the imaginary part goes from -2 to 2 by 0. 1. • Push: Show the results as a grid • Write the code to generate the Mandelbrot set (Complete Blow-up push first)