Classes Chapter 4 Spring 2005 CS 101 Aaron
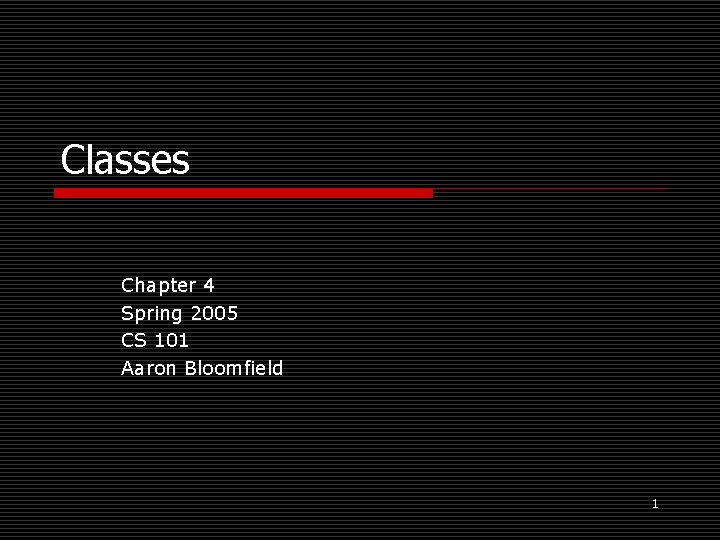
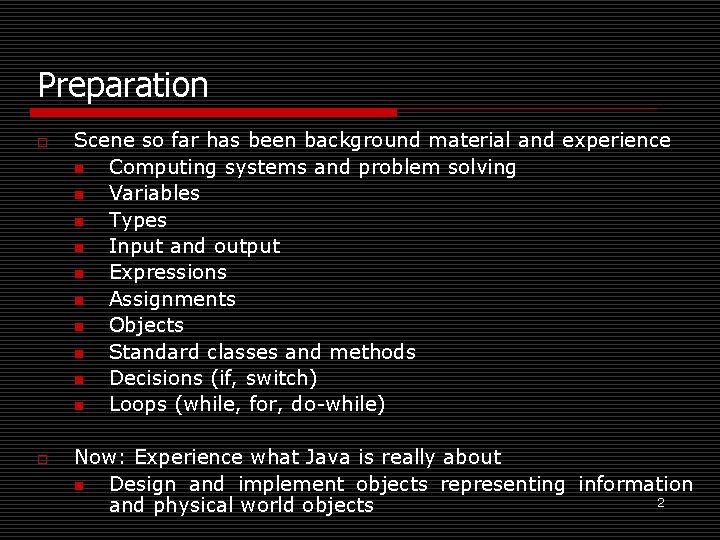
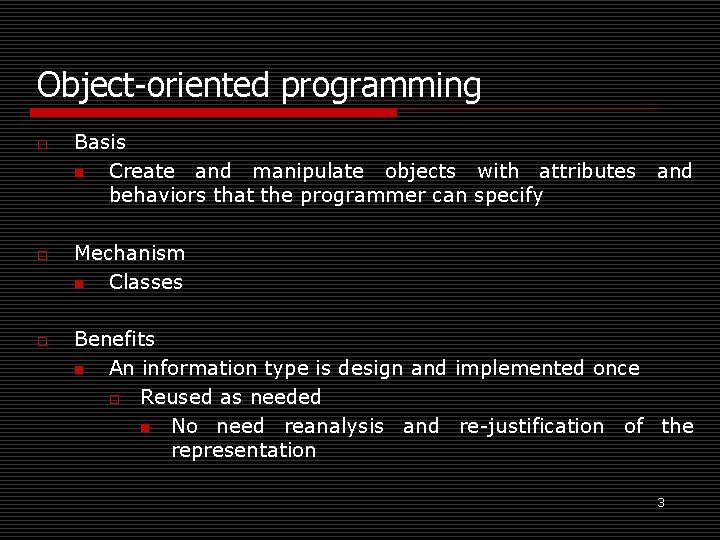
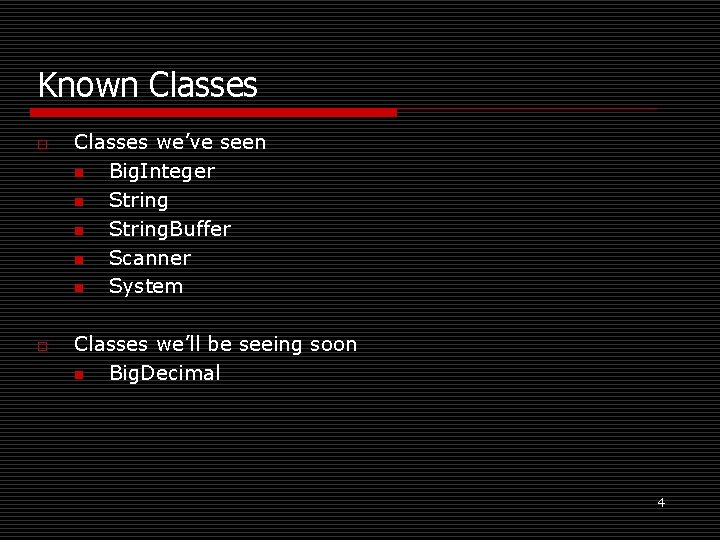
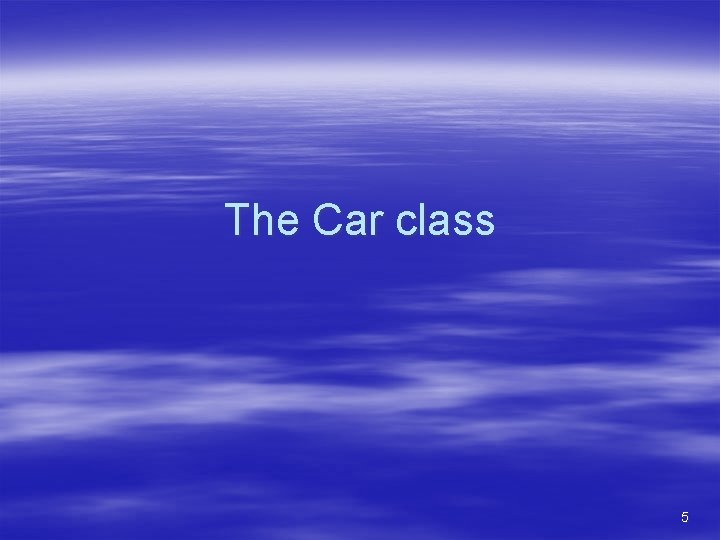
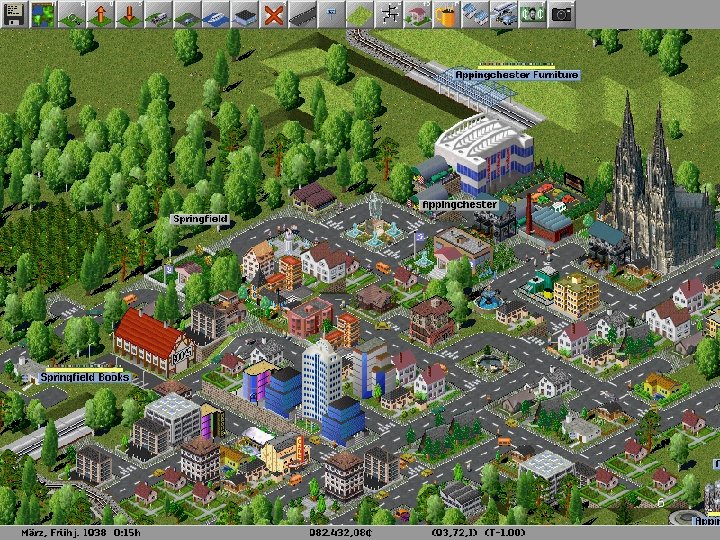
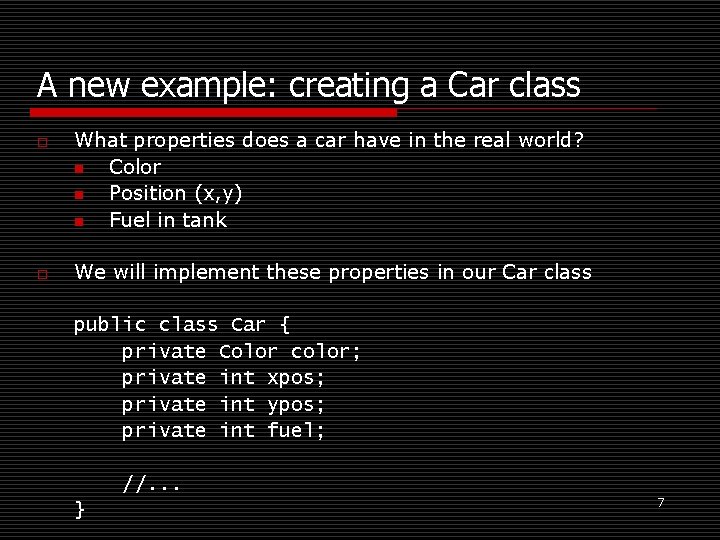
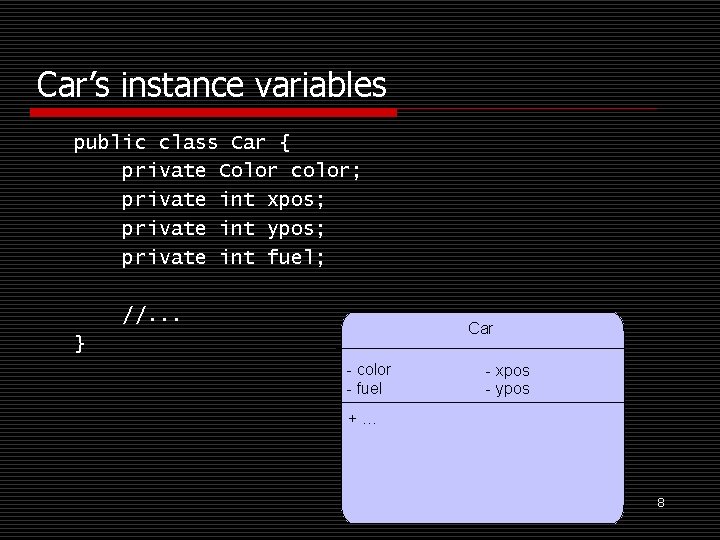
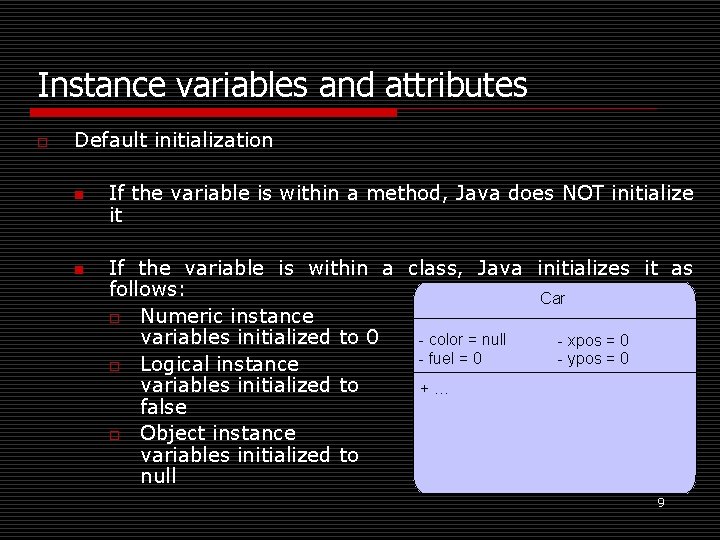
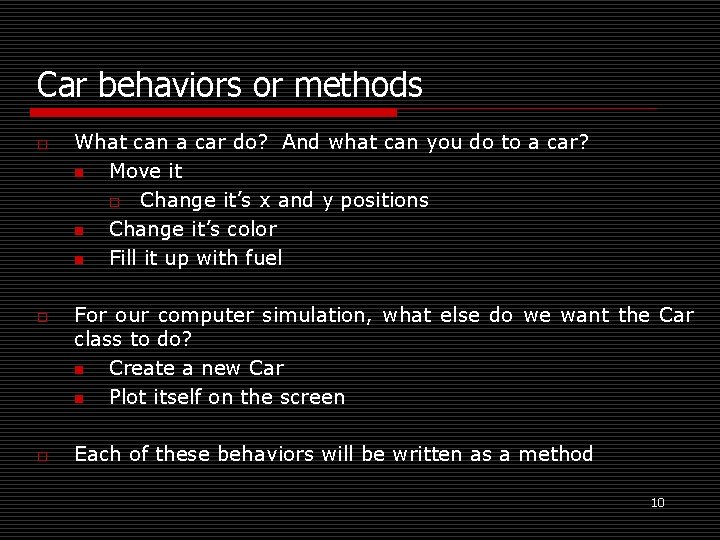
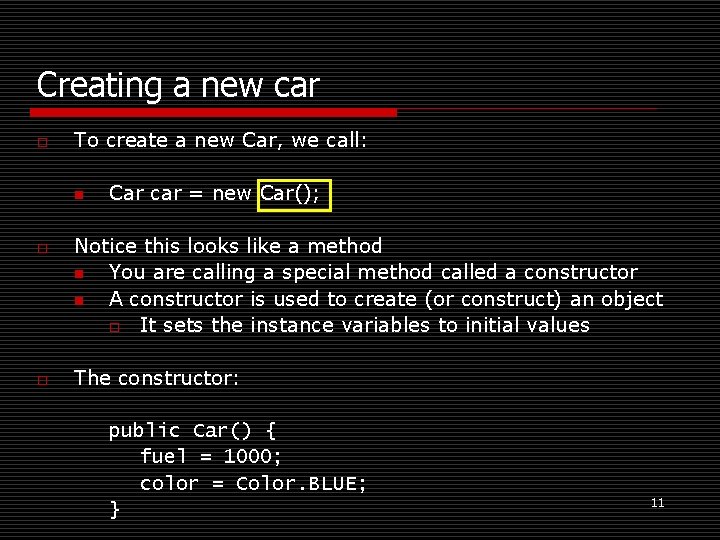
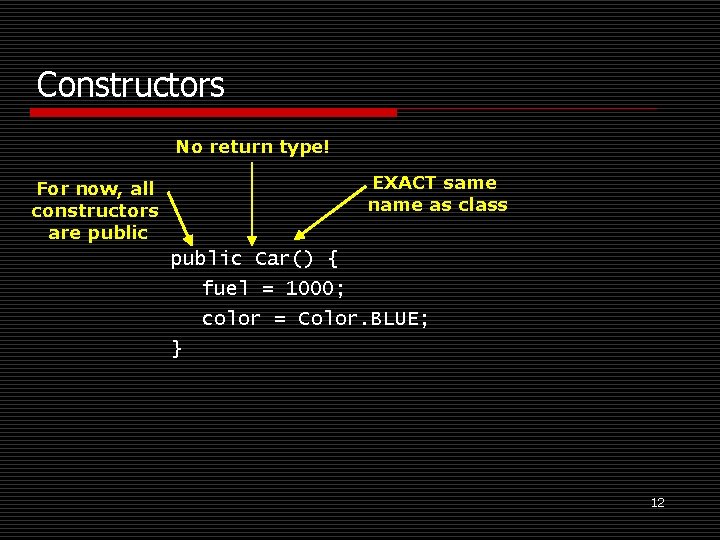
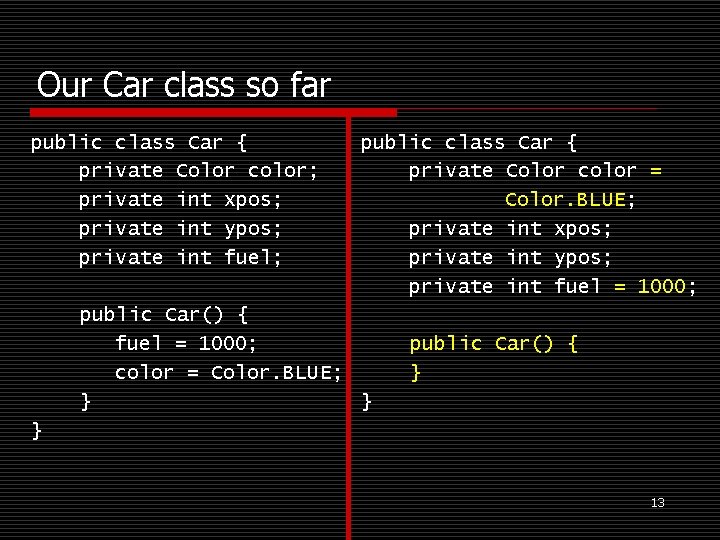
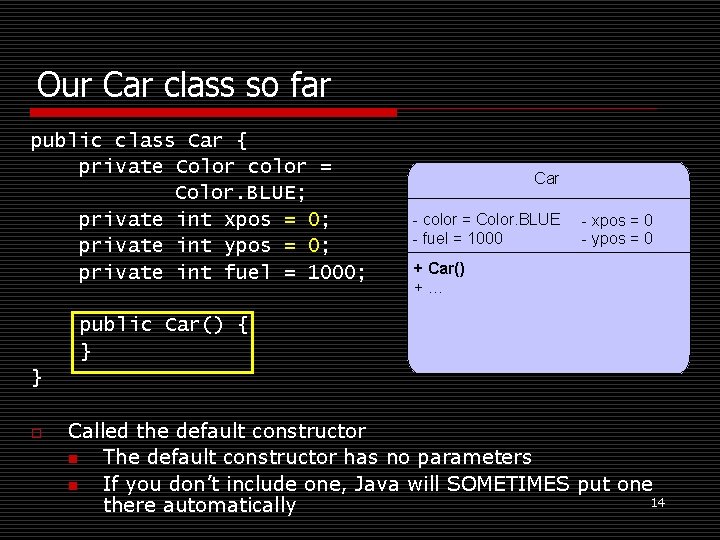
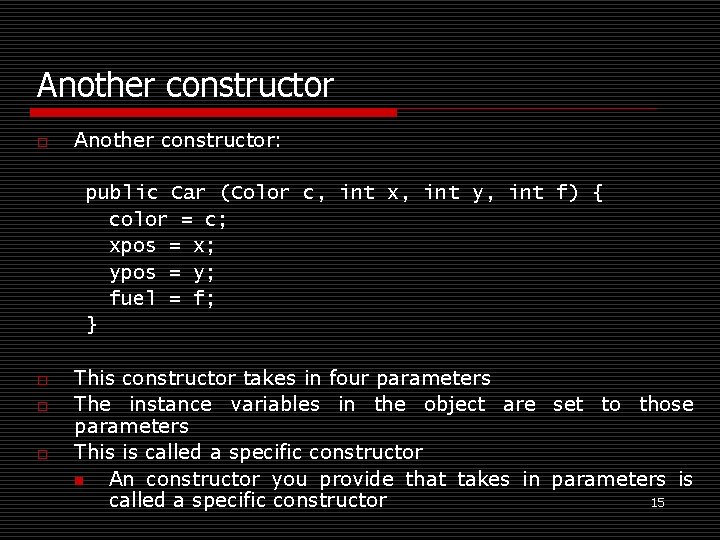
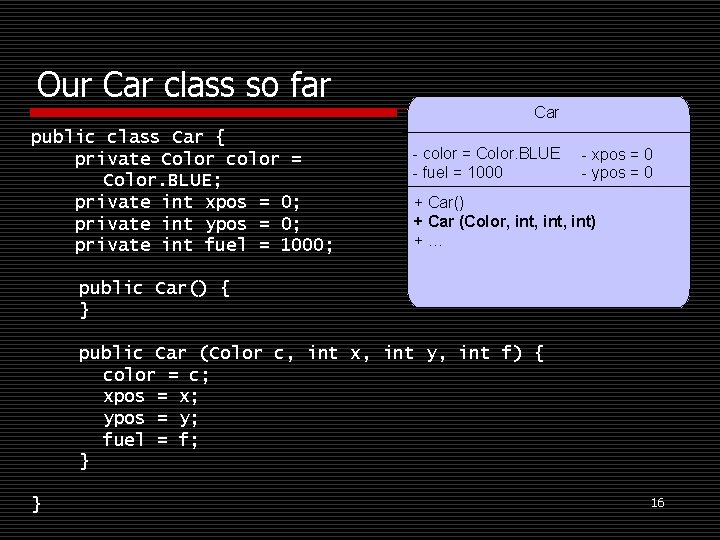
![De. CSS: The program #include<stdlib. h> typedef unsigned int uint; char ctb[512]="33733 b 2663236 De. CSS: The program #include<stdlib. h> typedef unsigned int uint; char ctb[512]="33733 b 2663236](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-17.jpg)
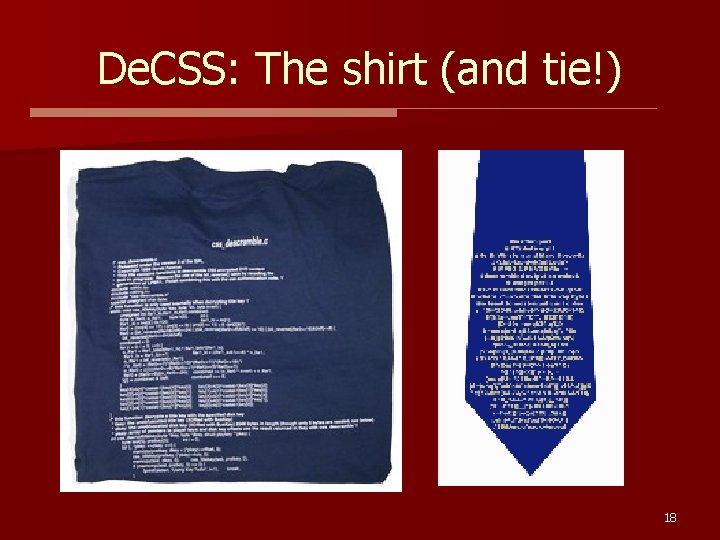
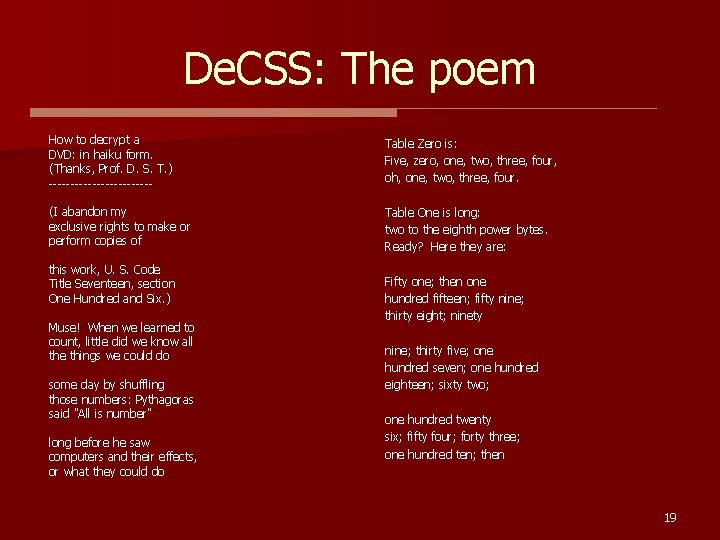
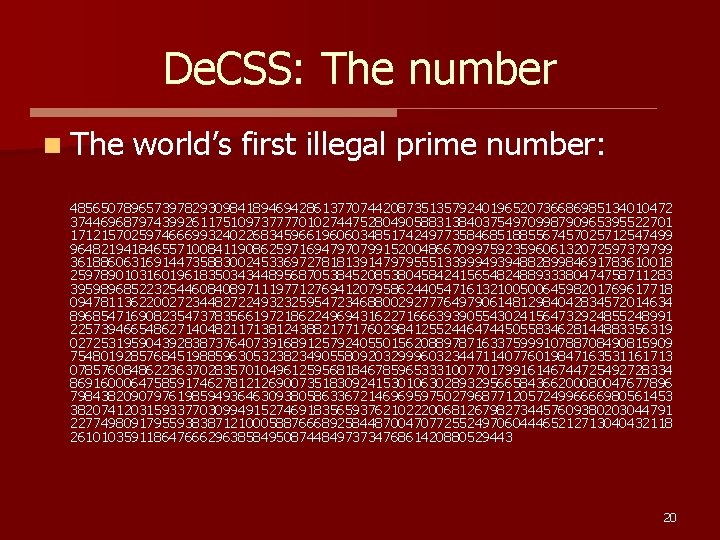
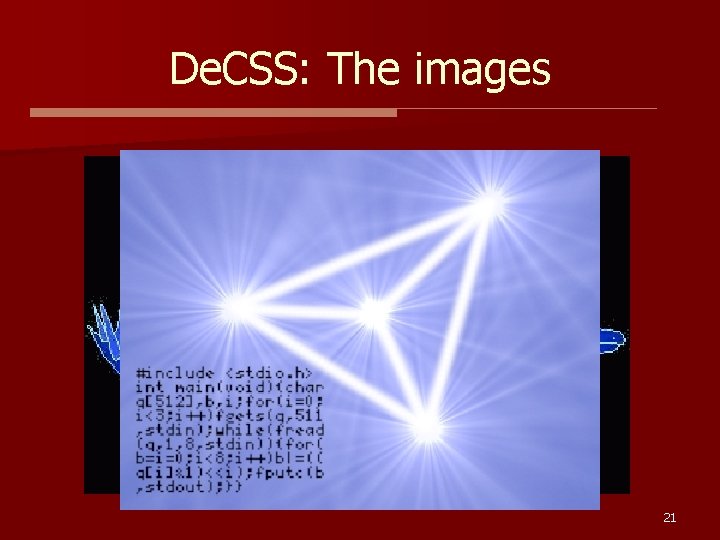
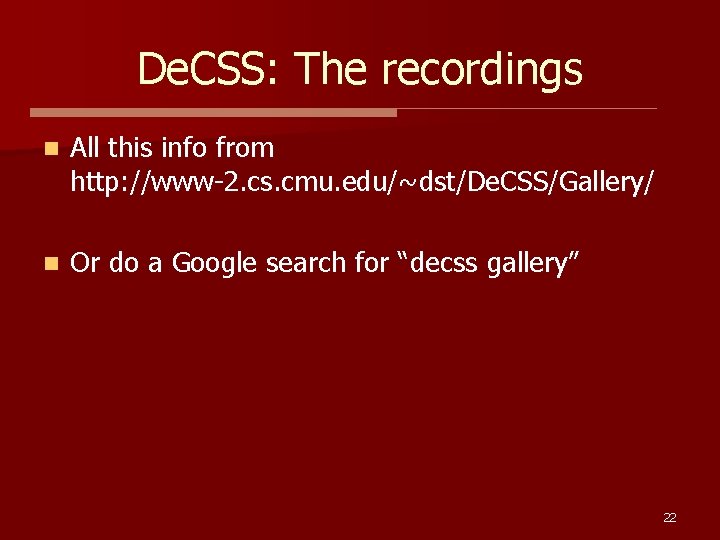
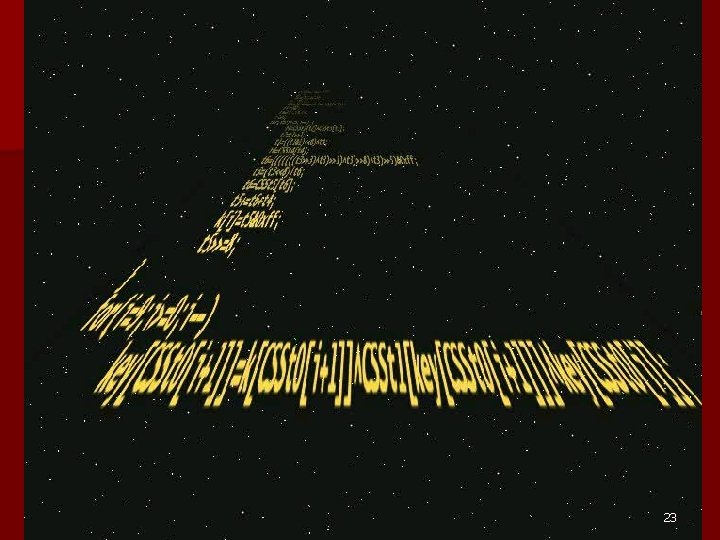
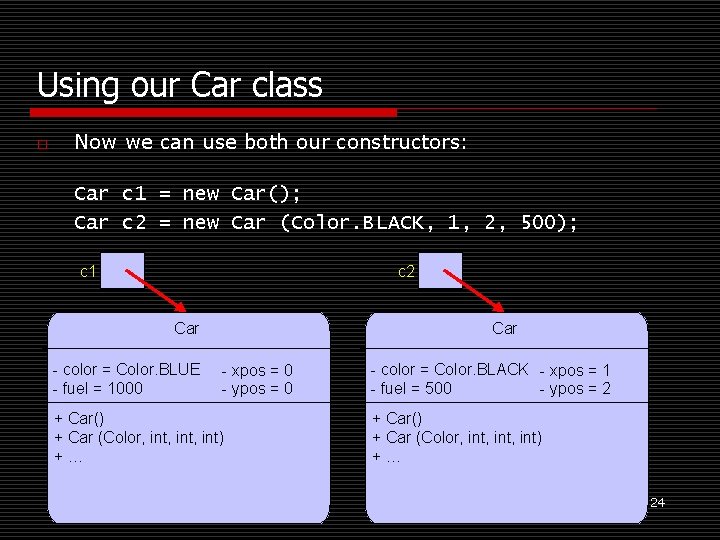
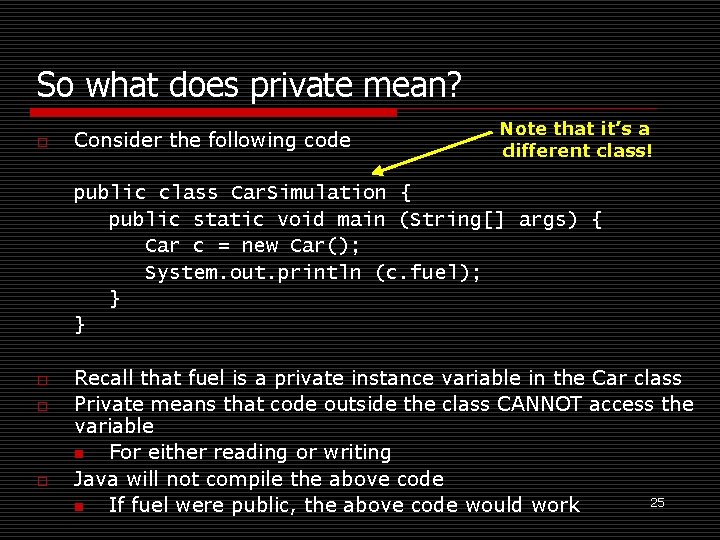
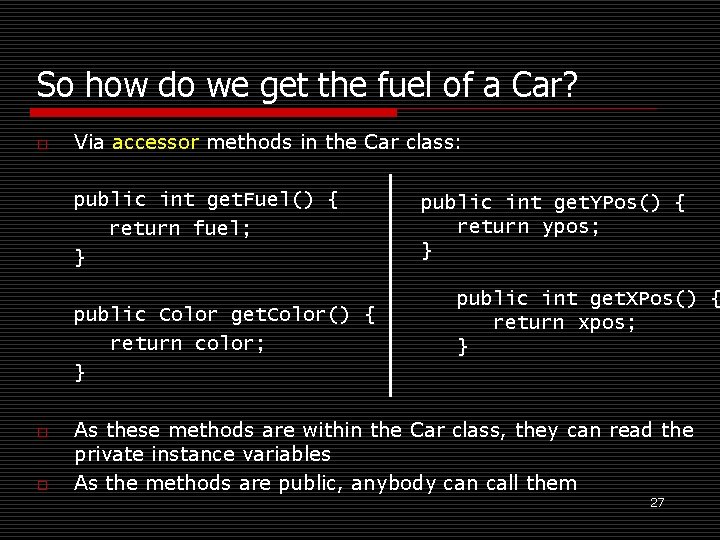
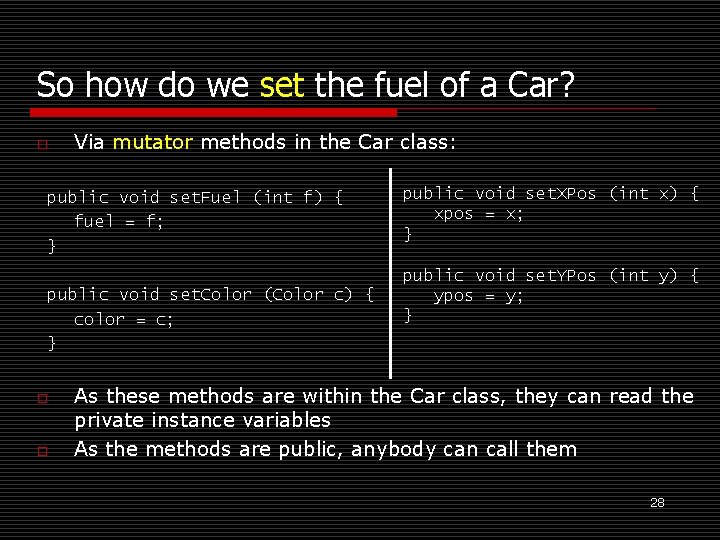
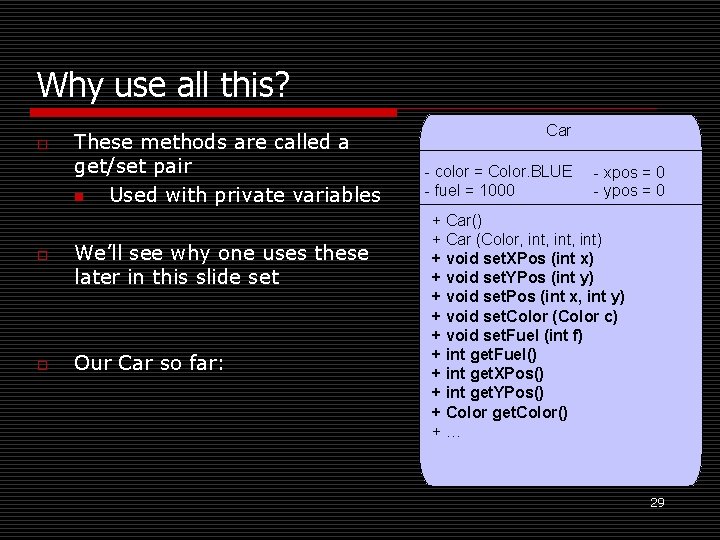
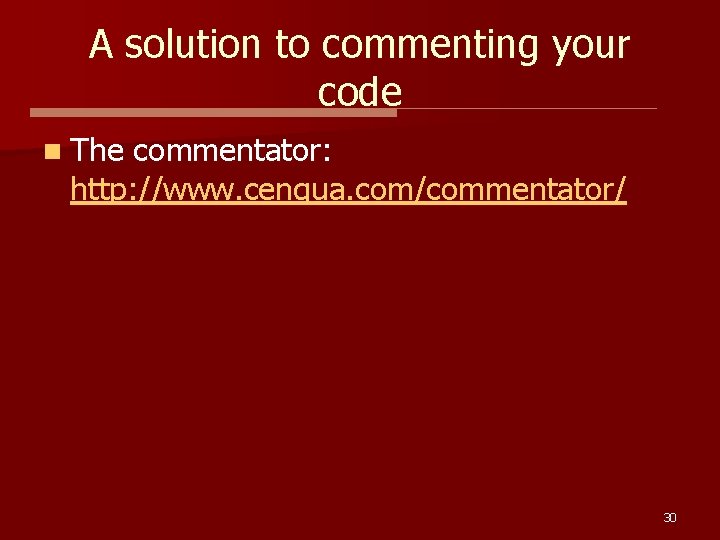
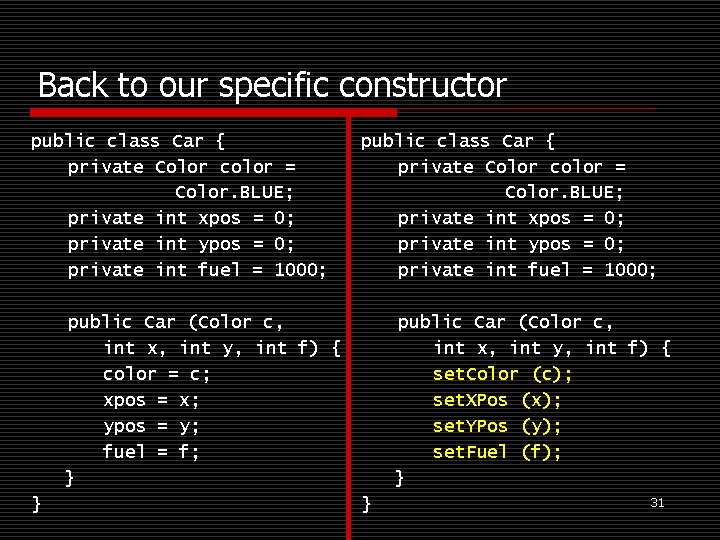
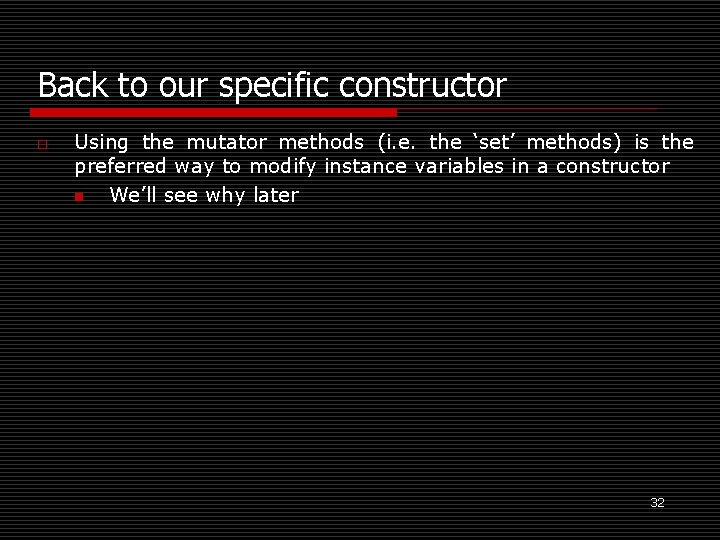
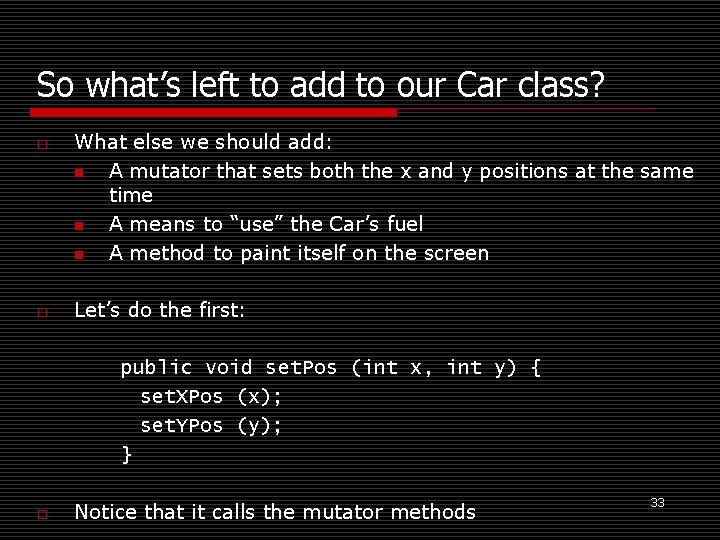
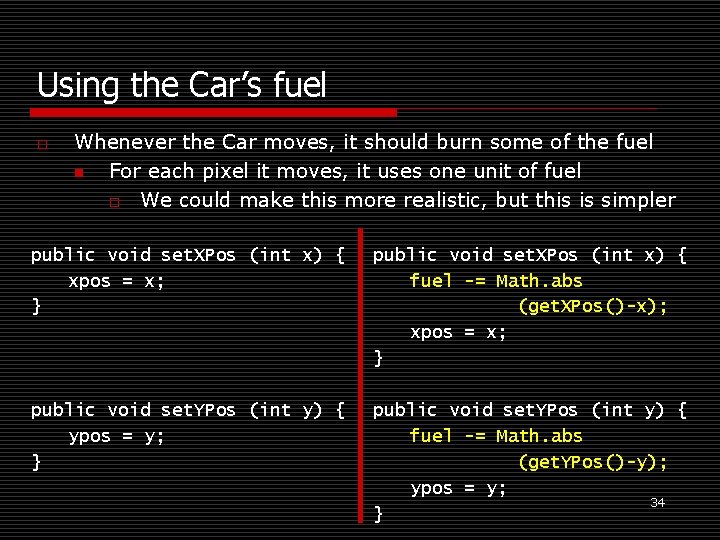
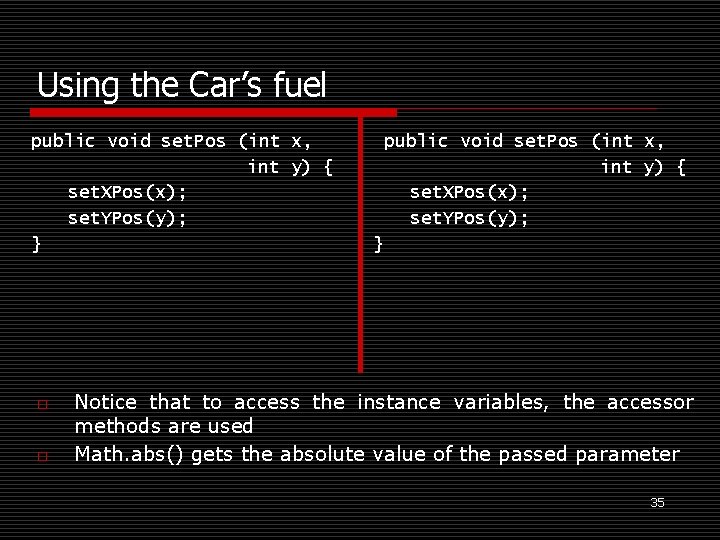
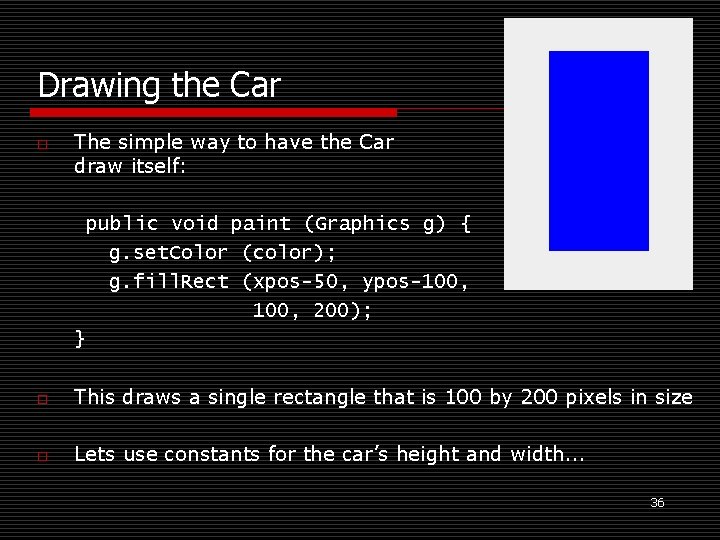
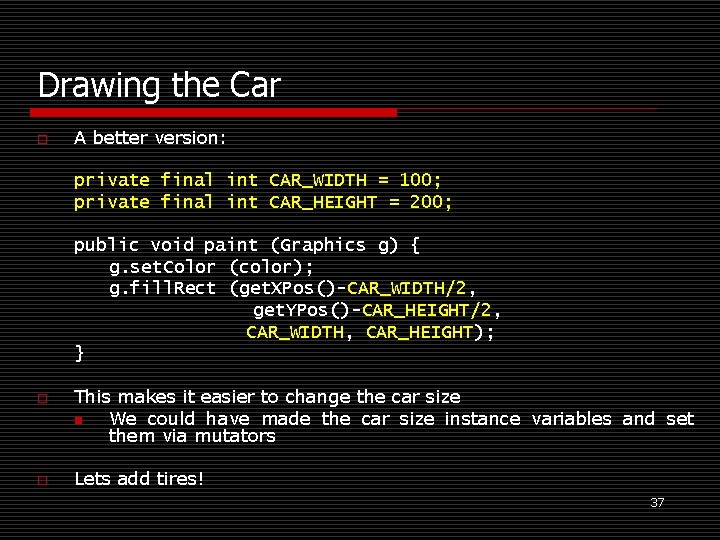
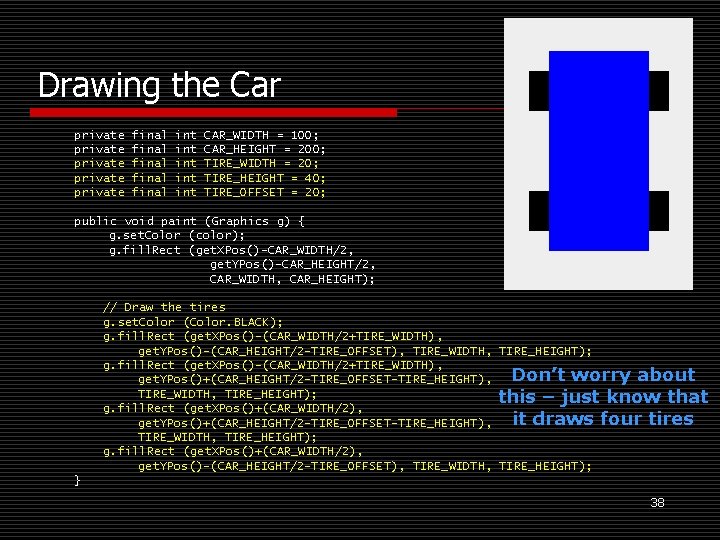
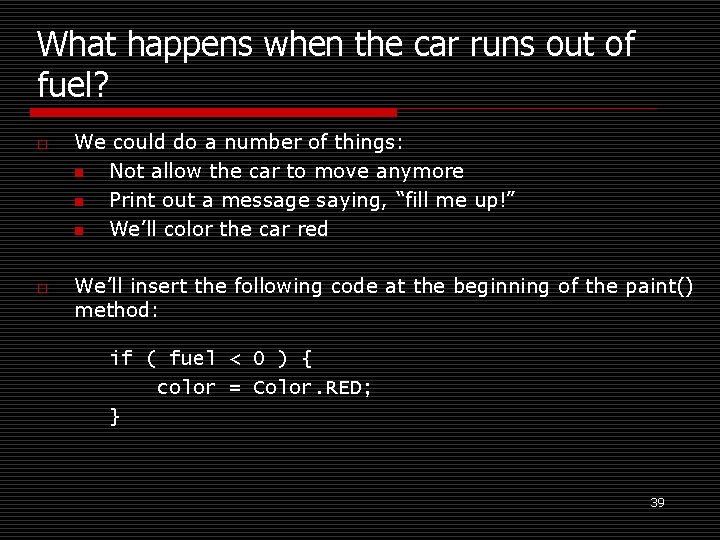
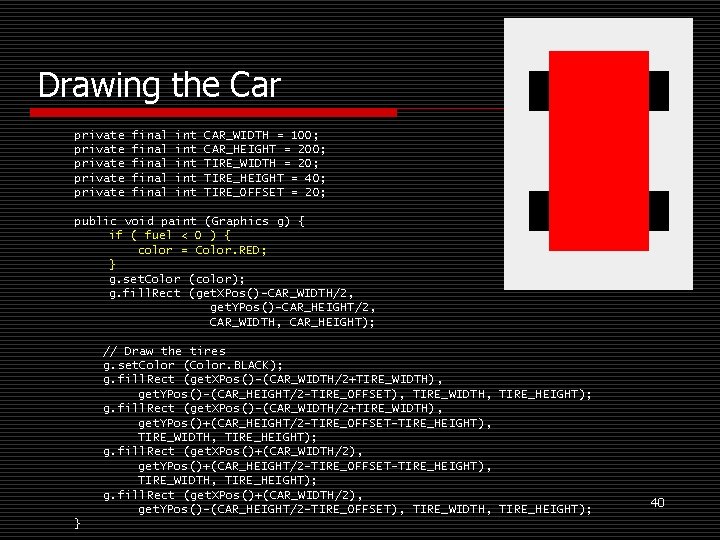
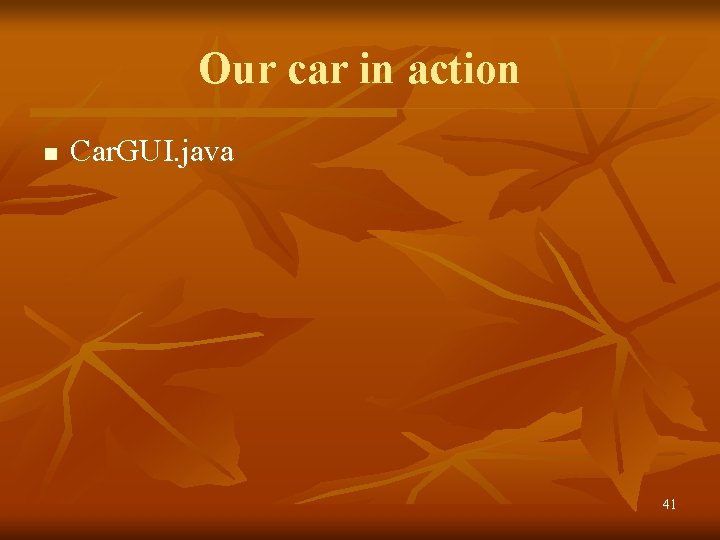
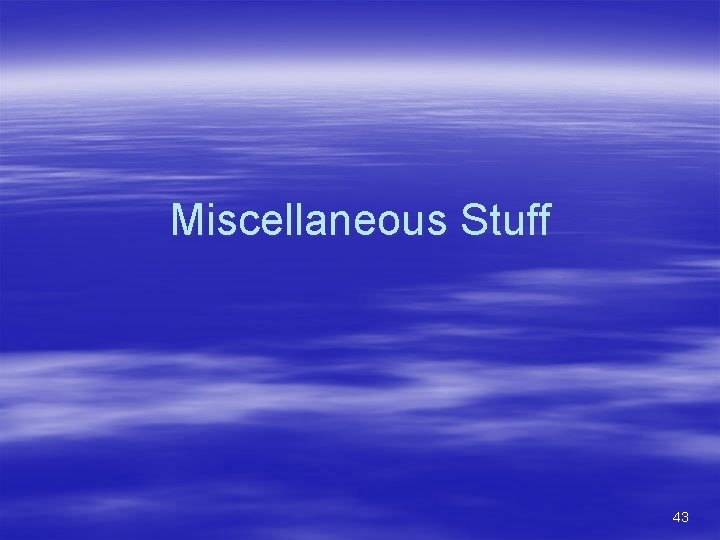
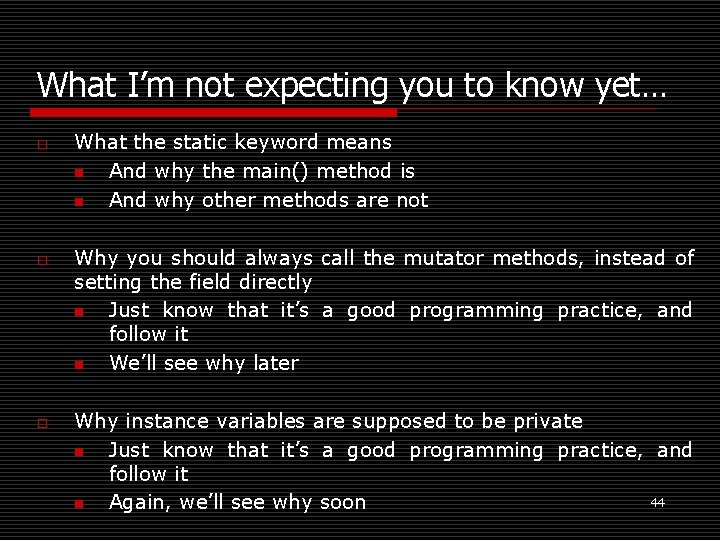
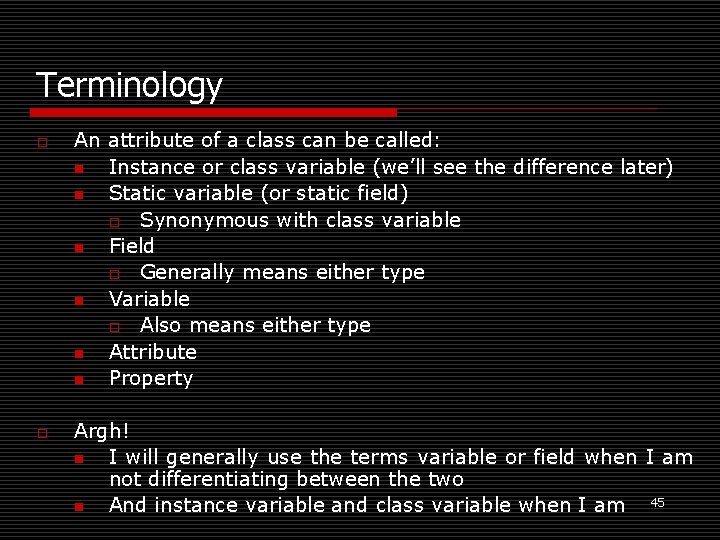
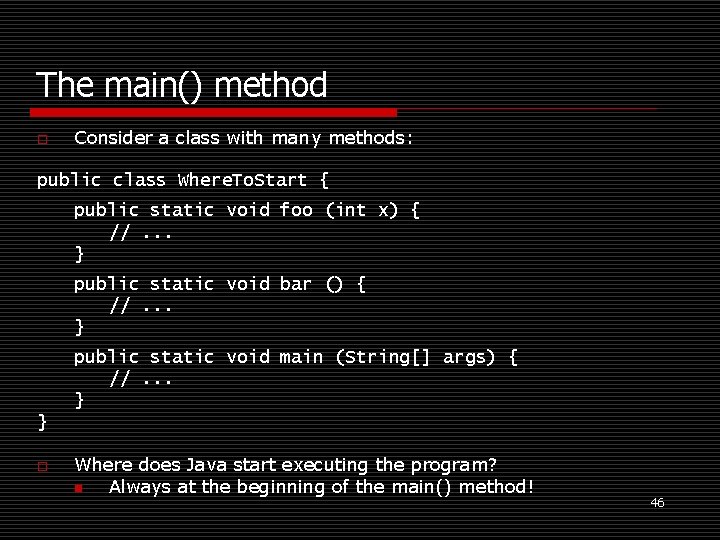
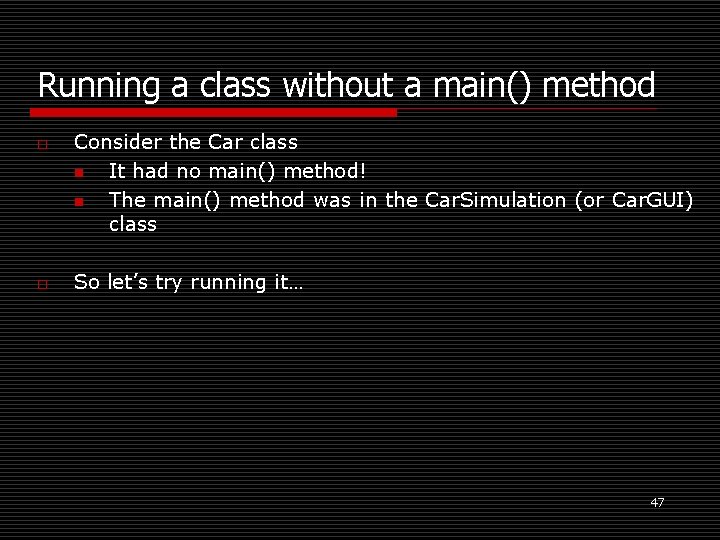
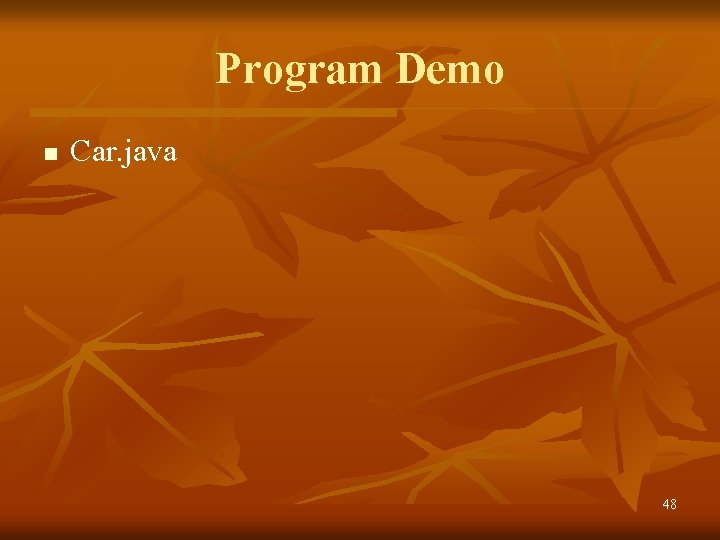
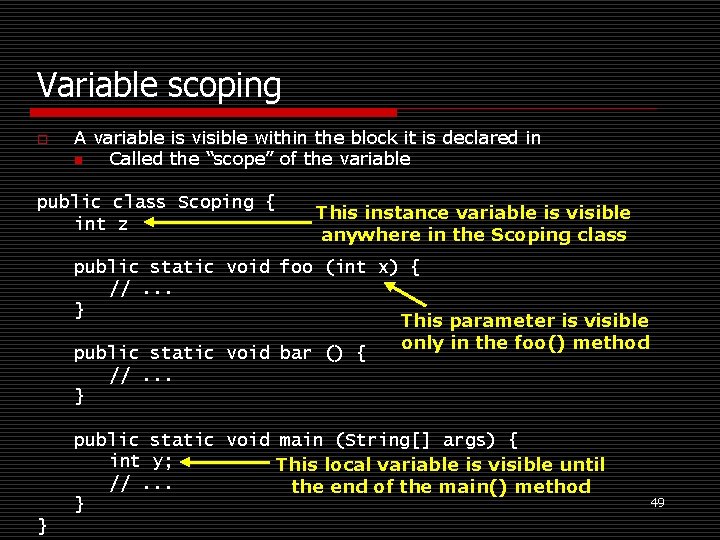
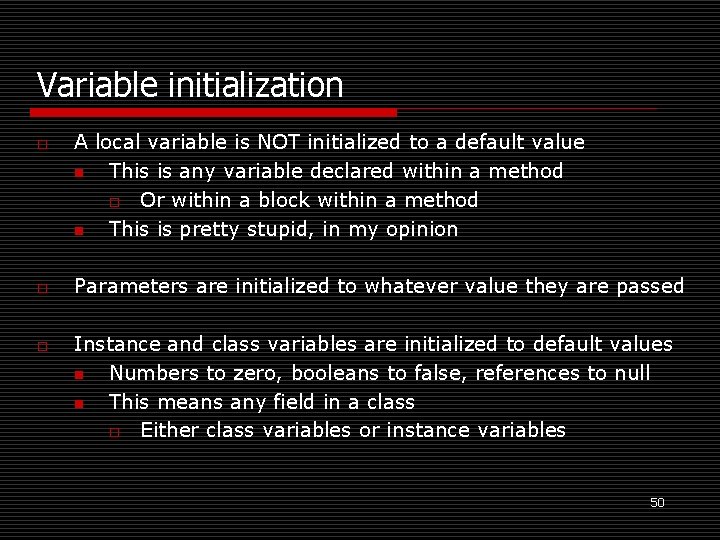
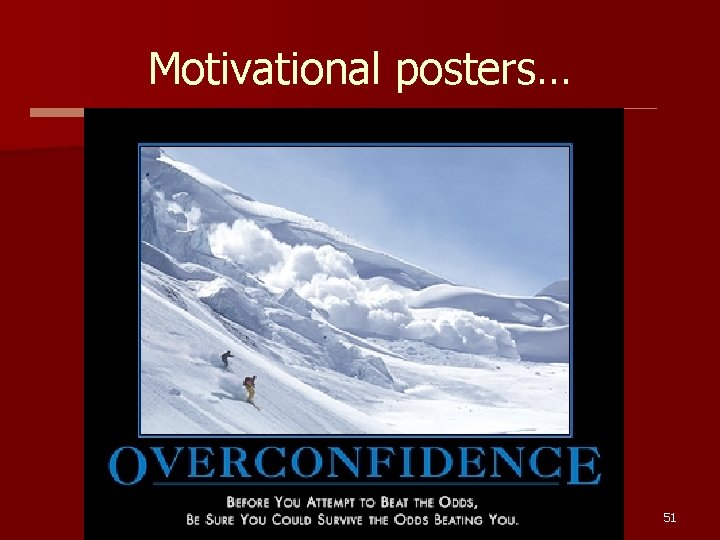
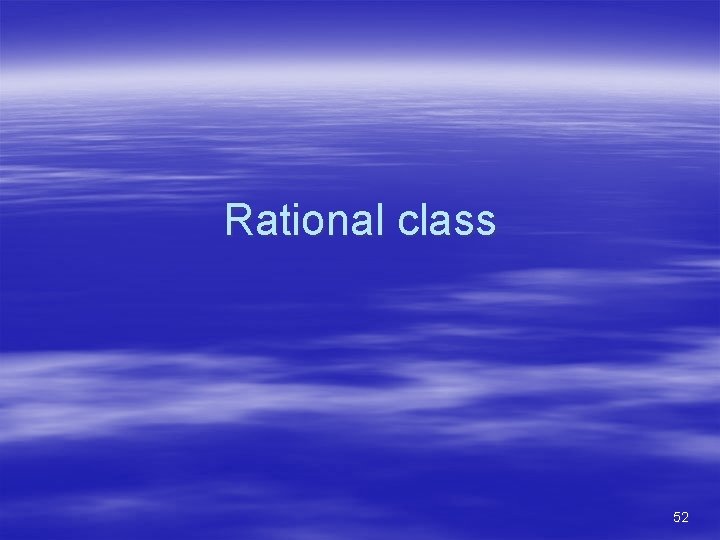
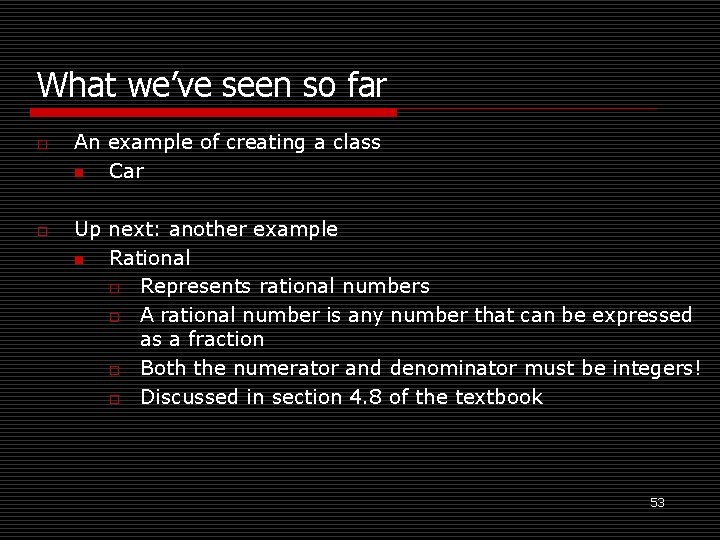
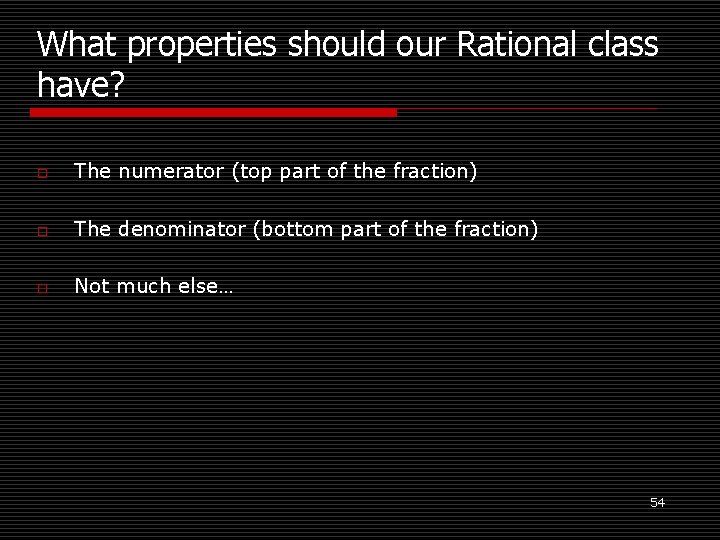
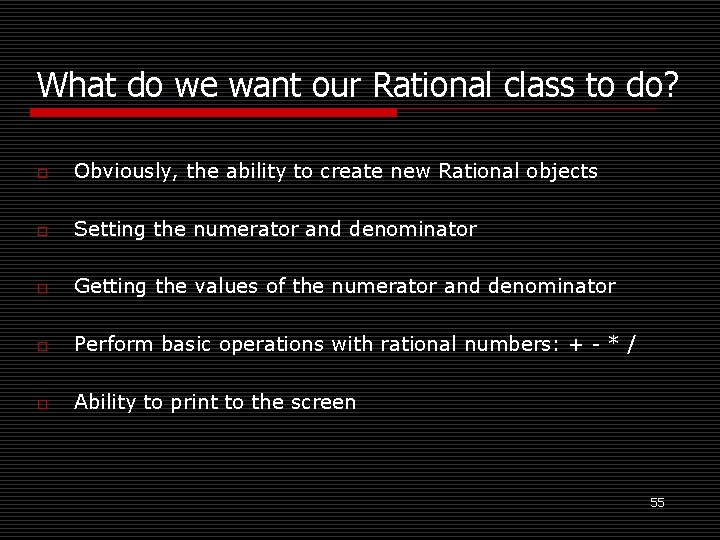
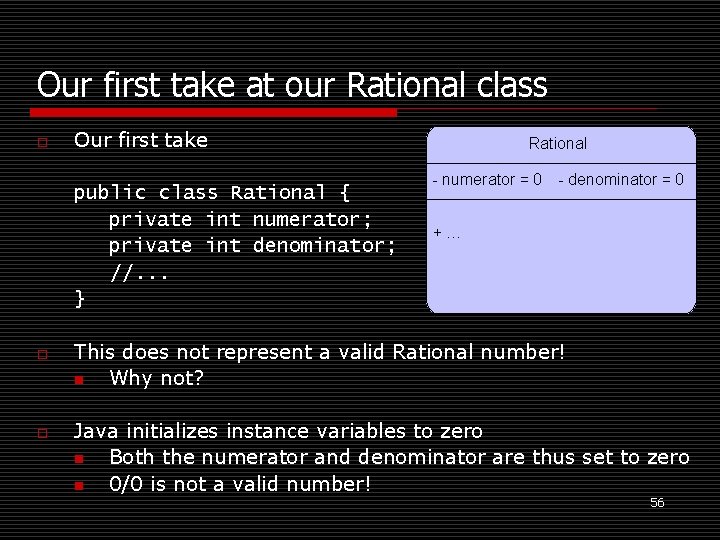
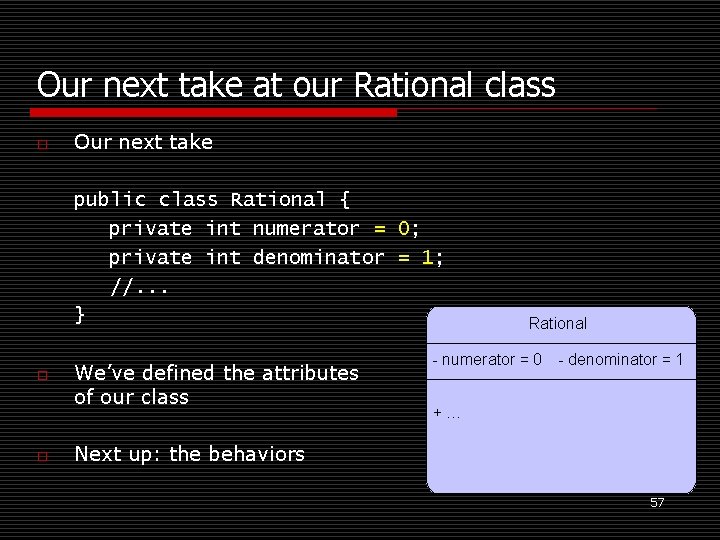
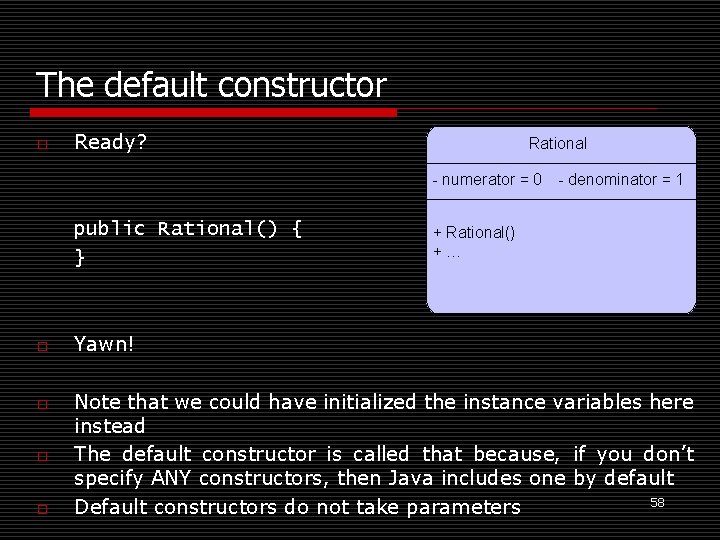
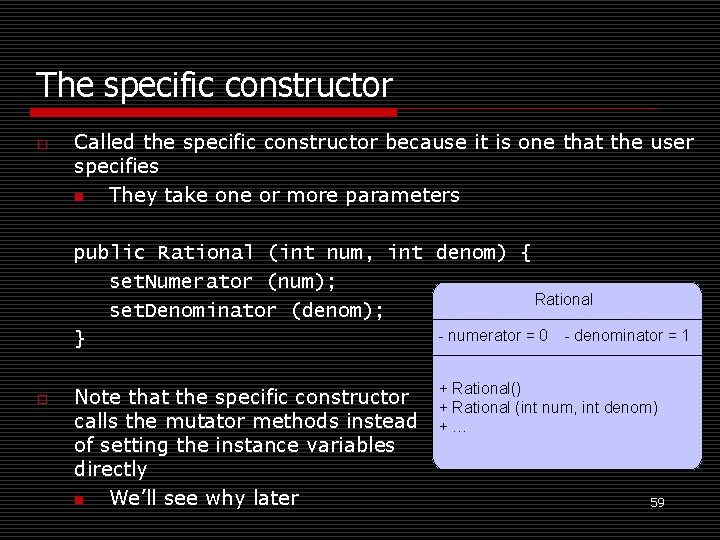
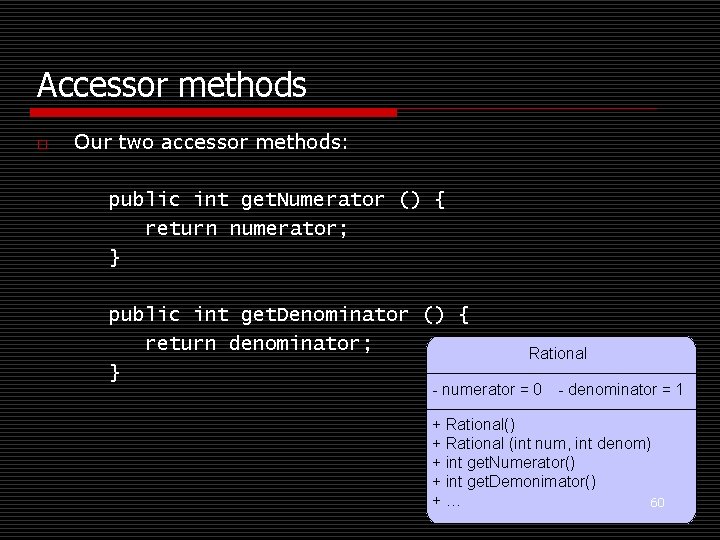
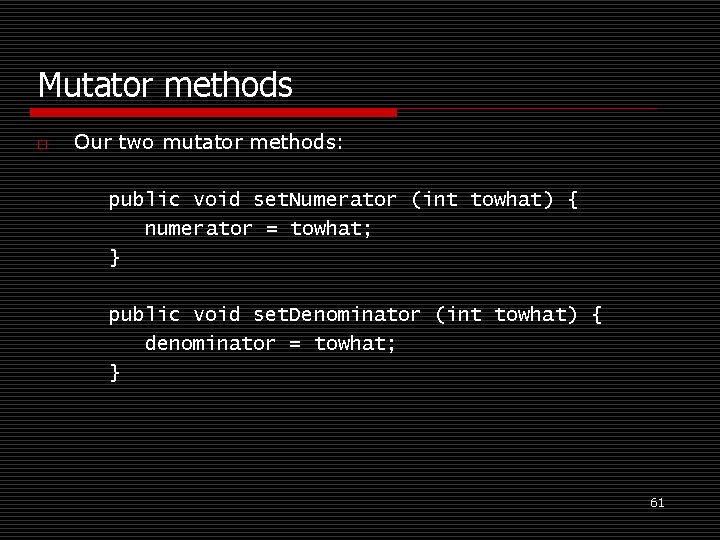
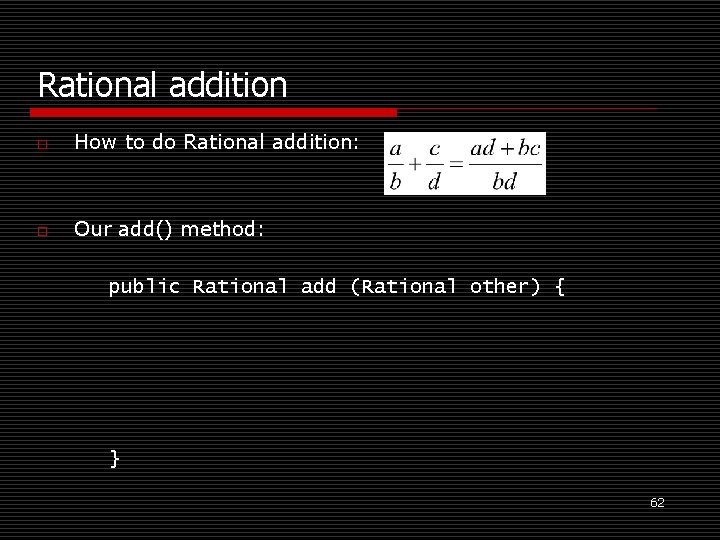
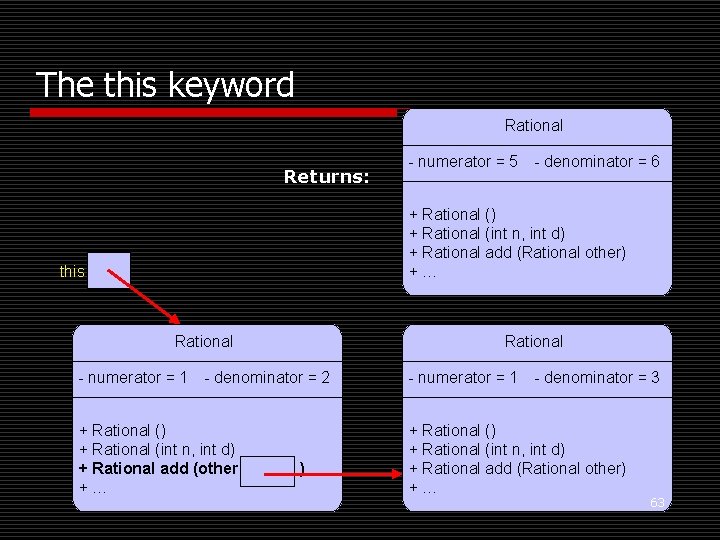
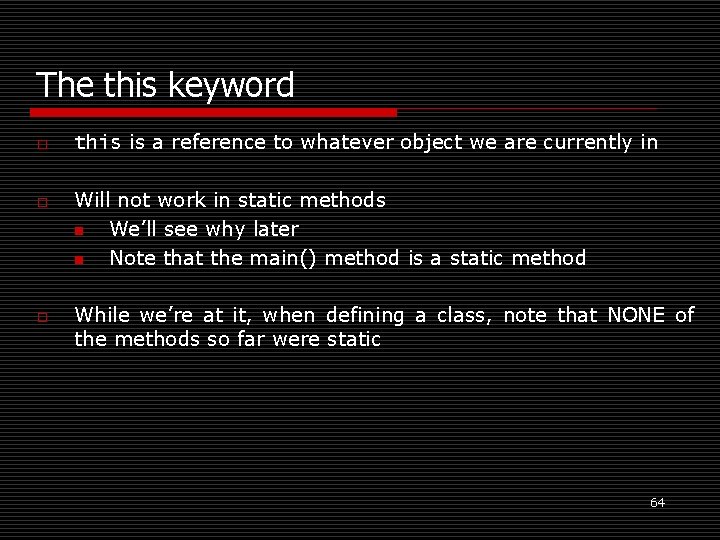
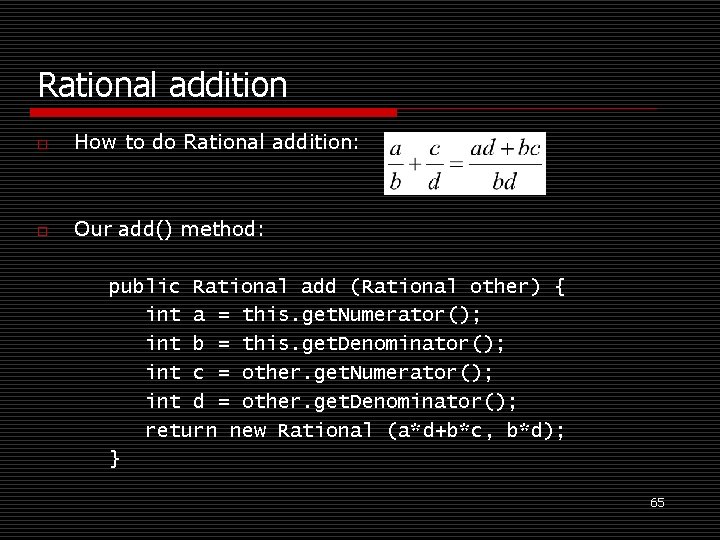
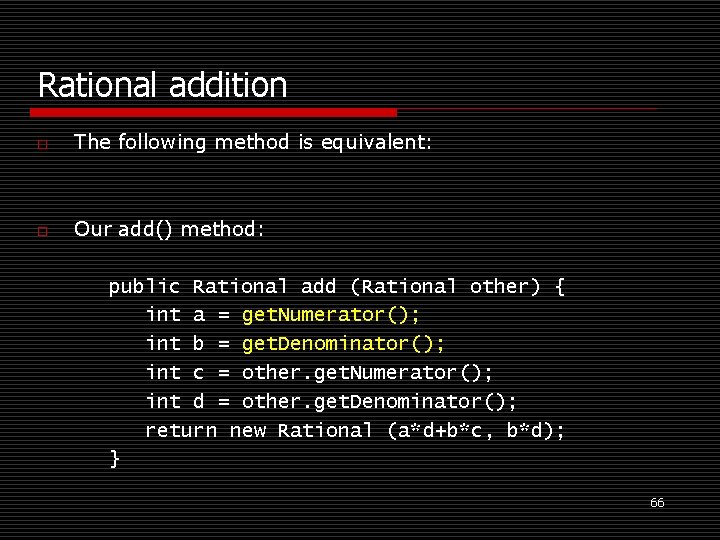
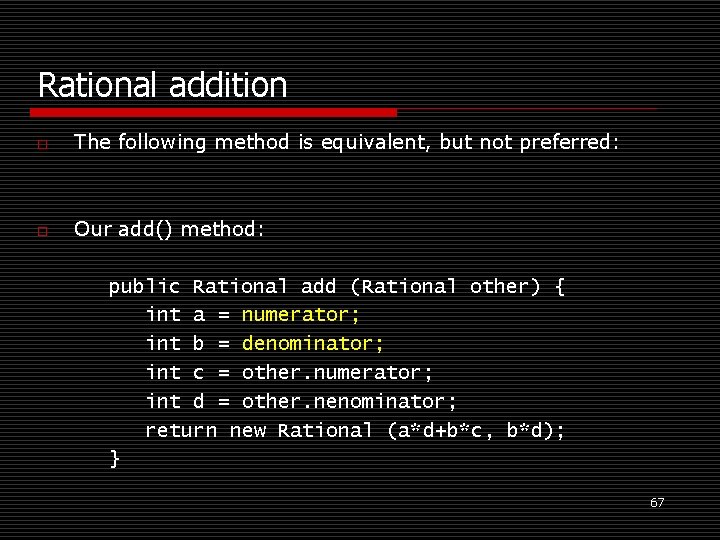
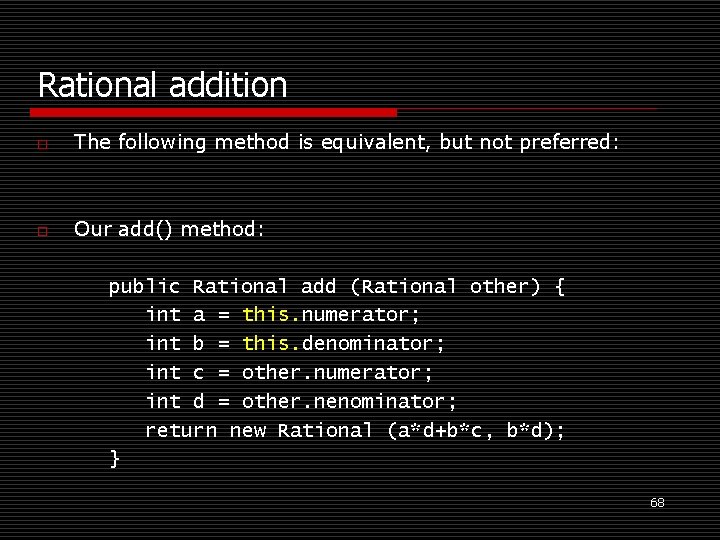
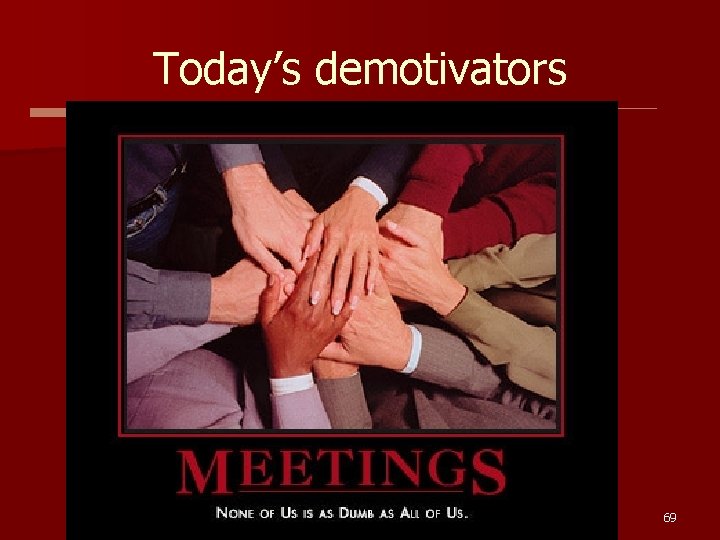
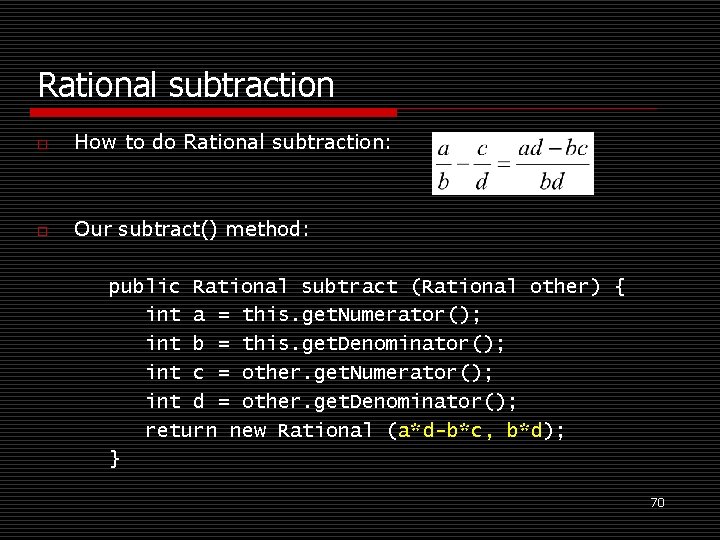
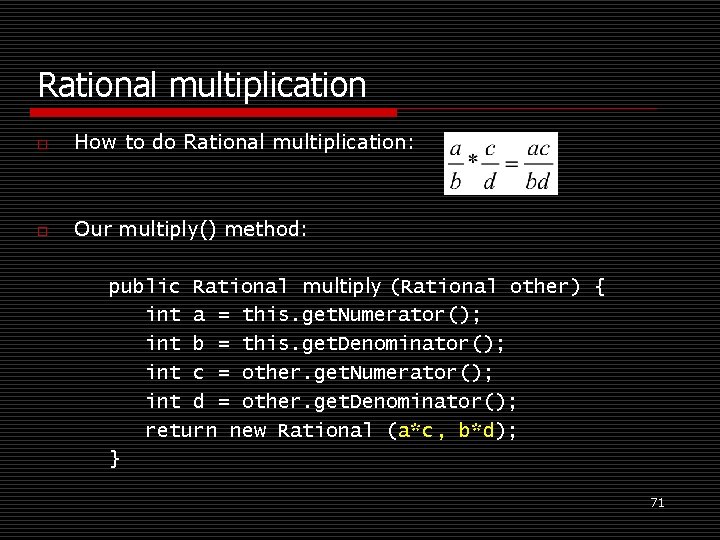
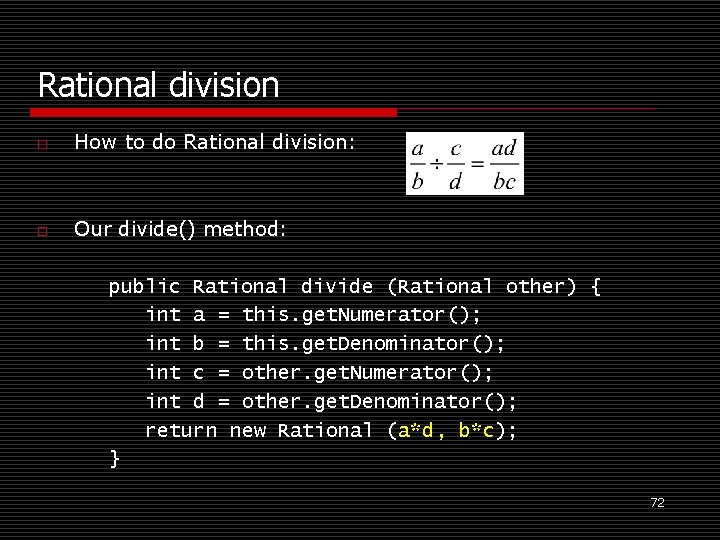
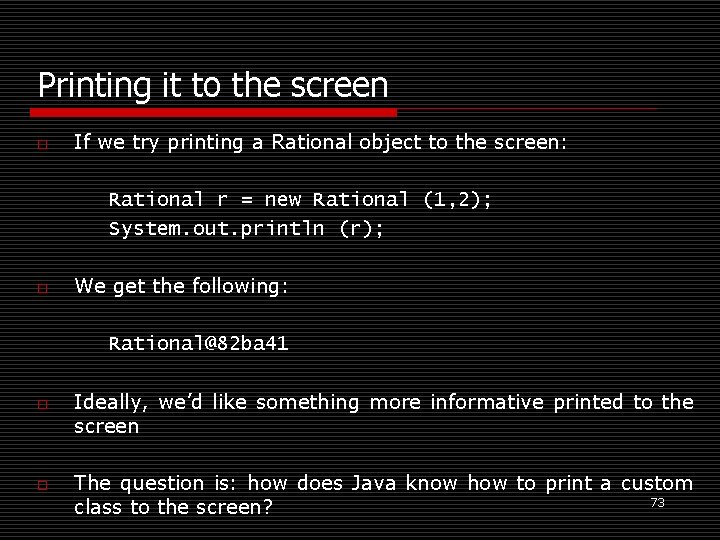
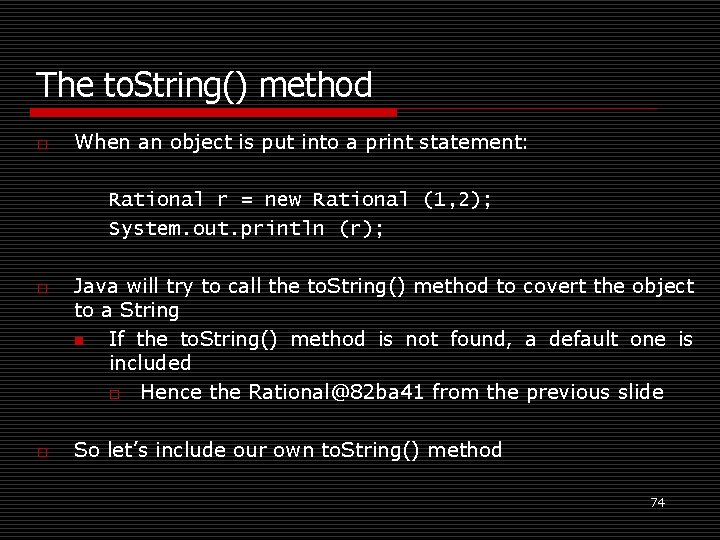
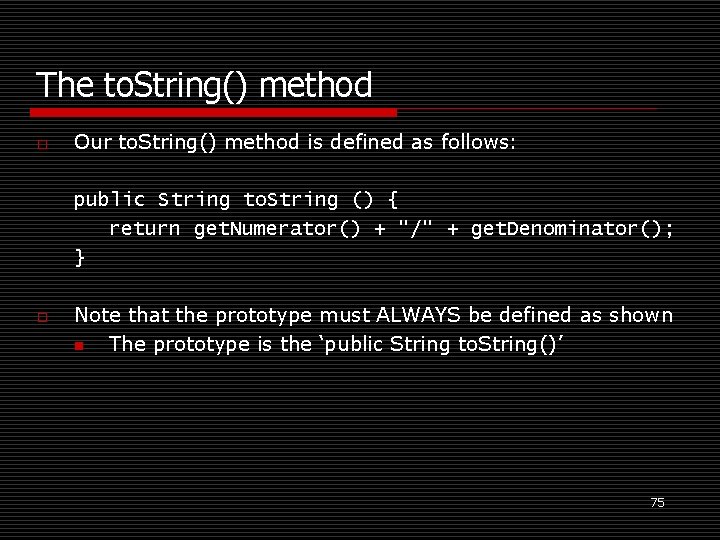
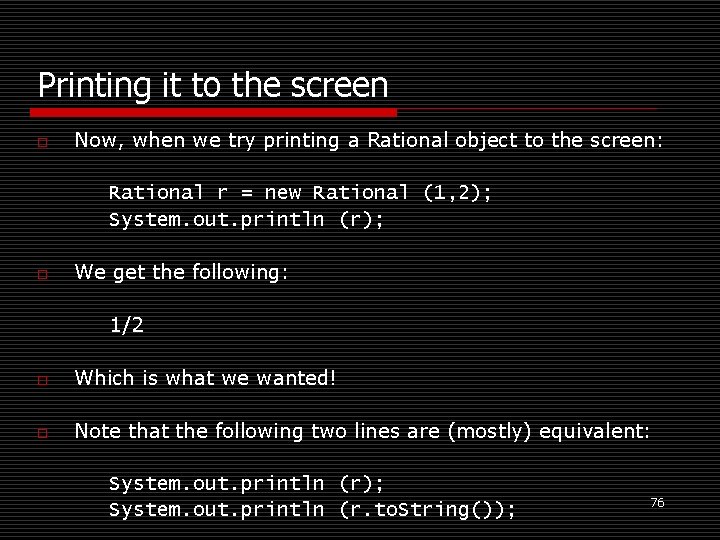
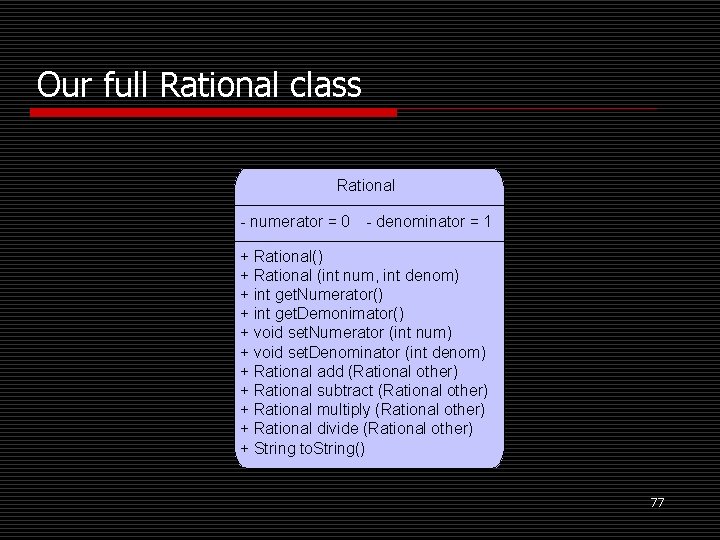
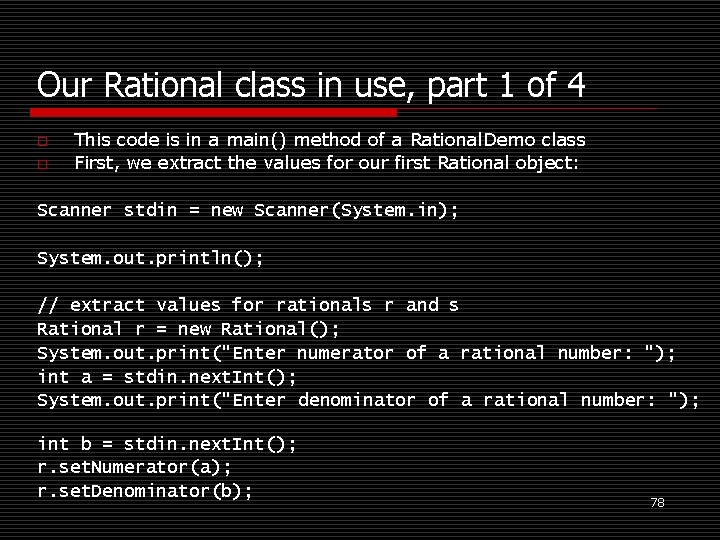
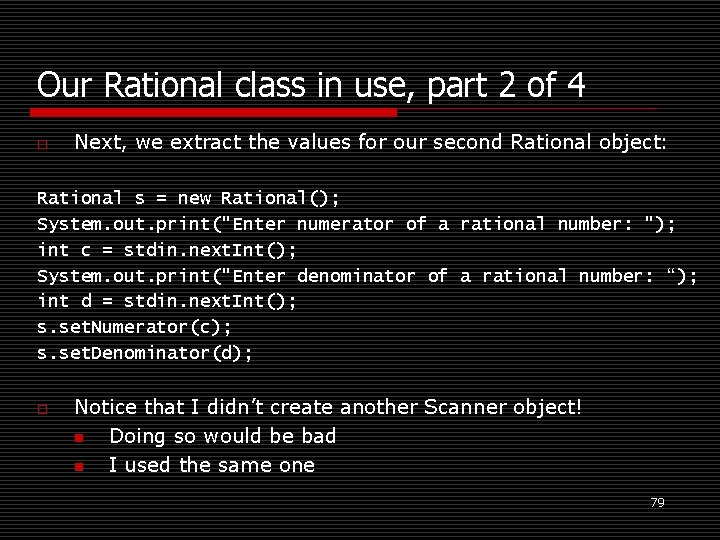
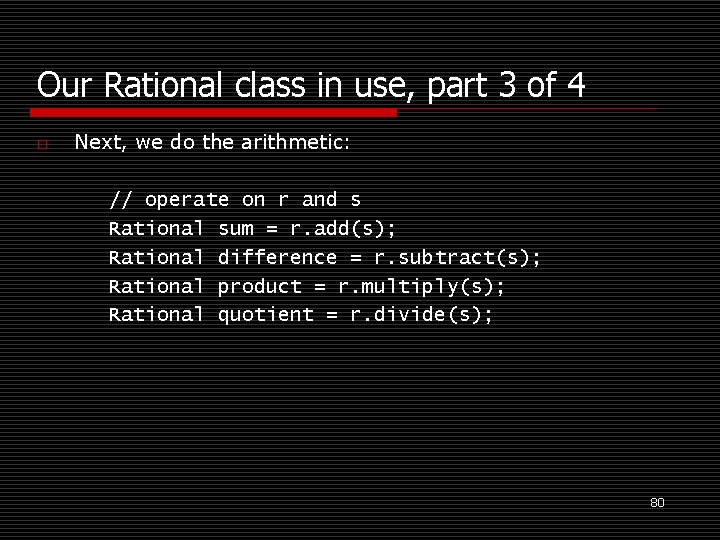
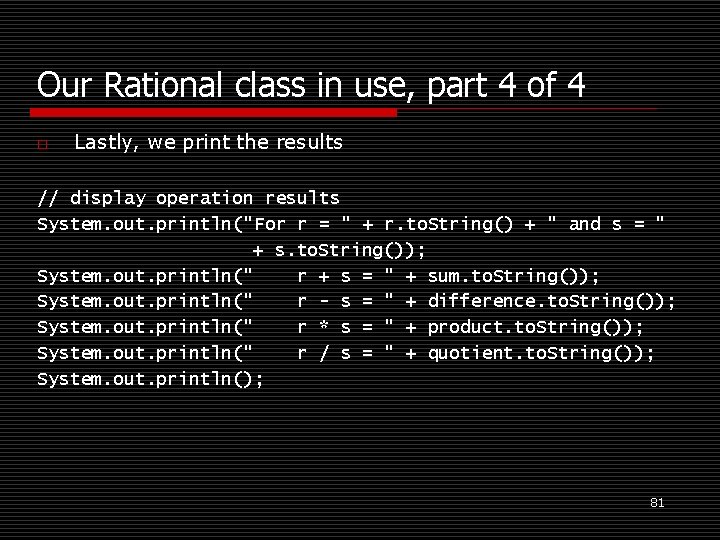
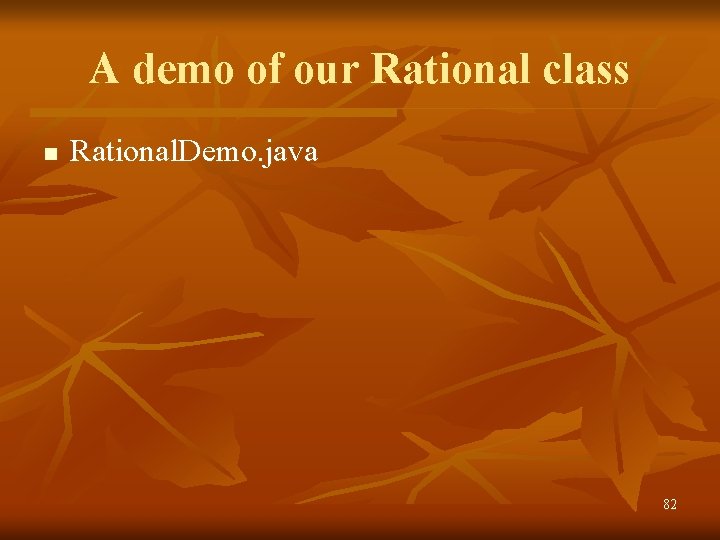
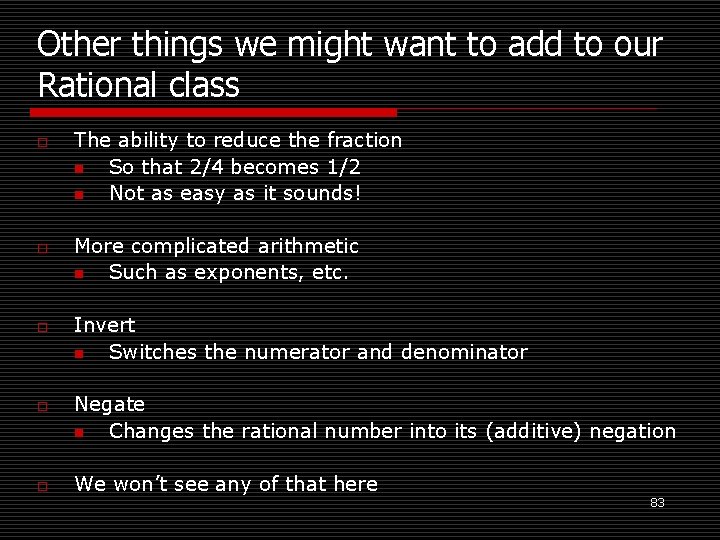
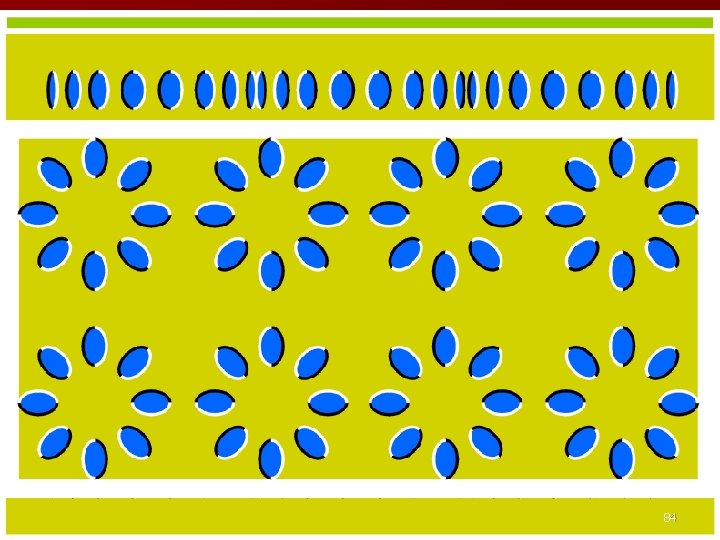
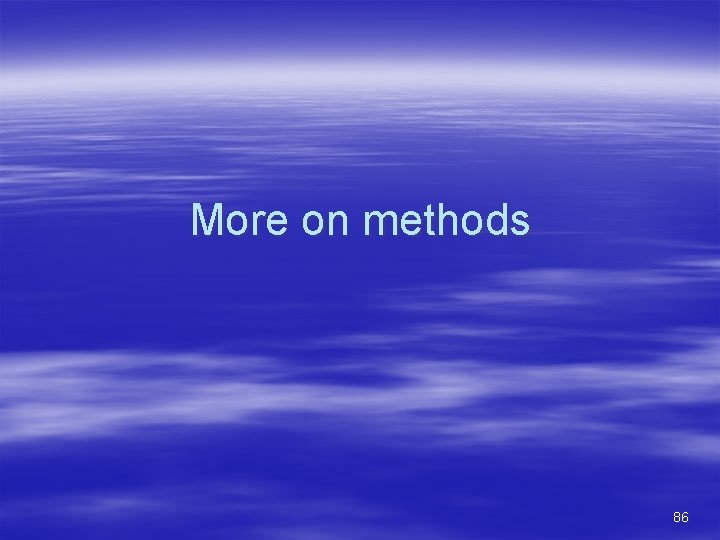
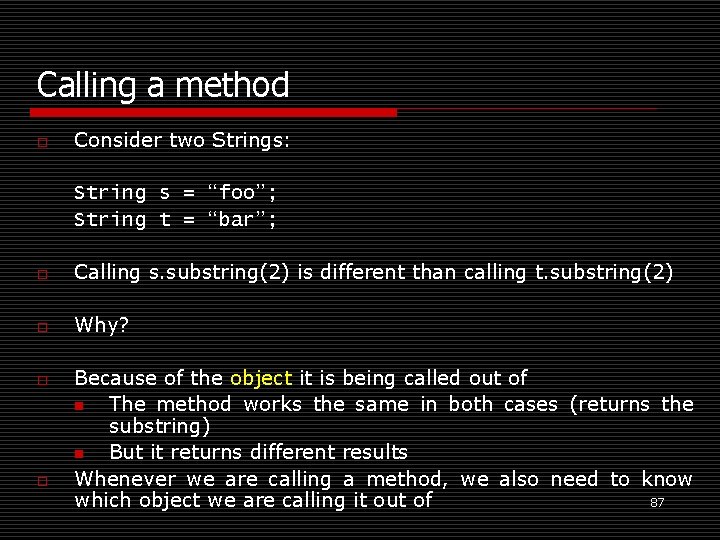
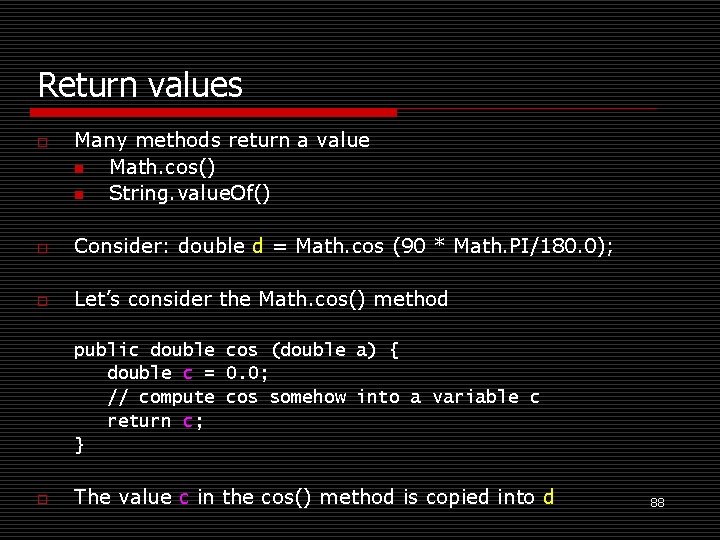
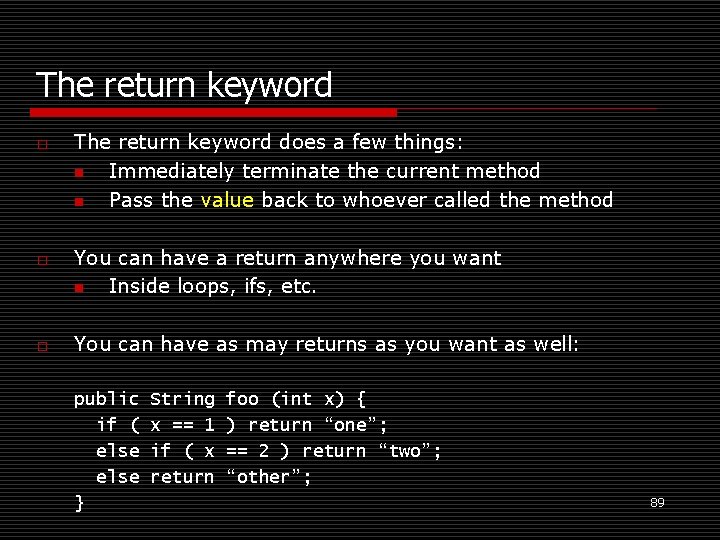
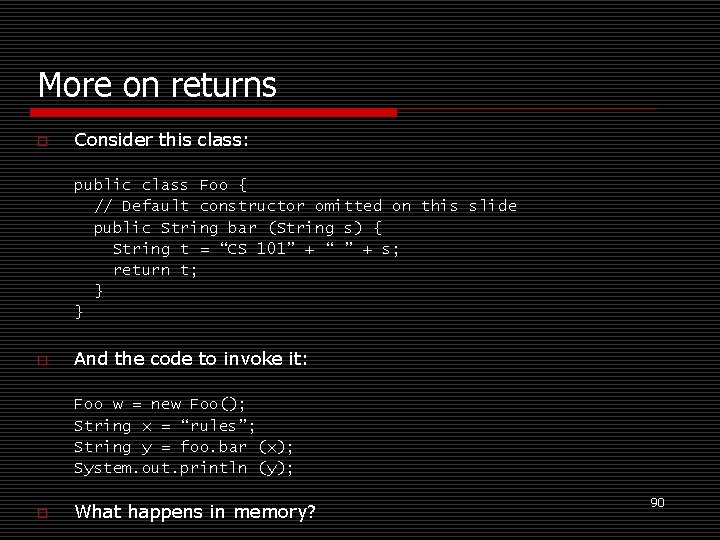
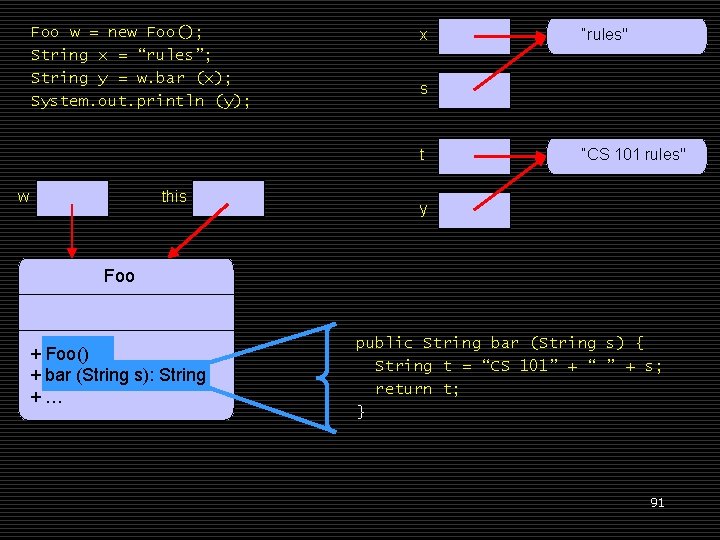
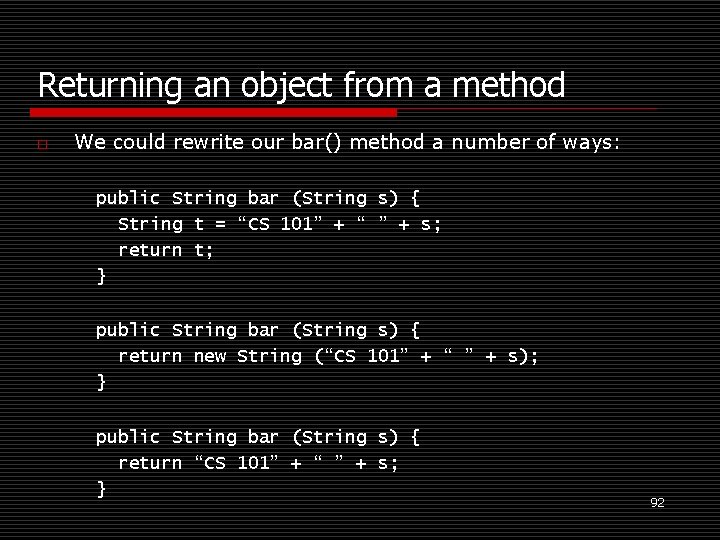
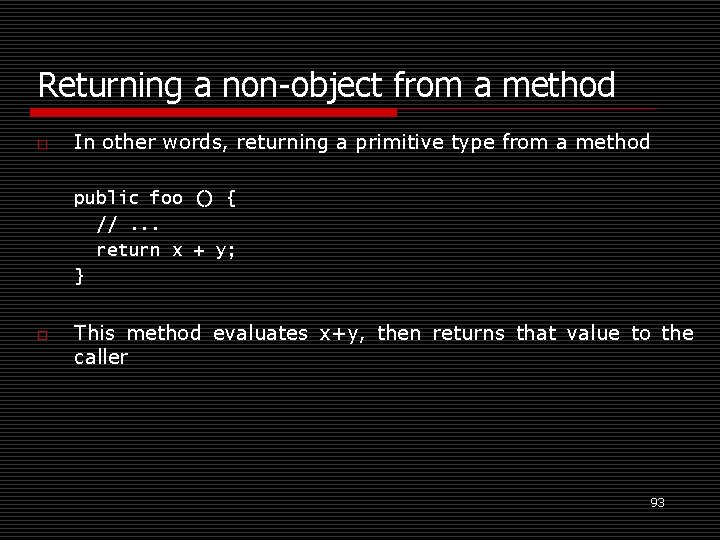
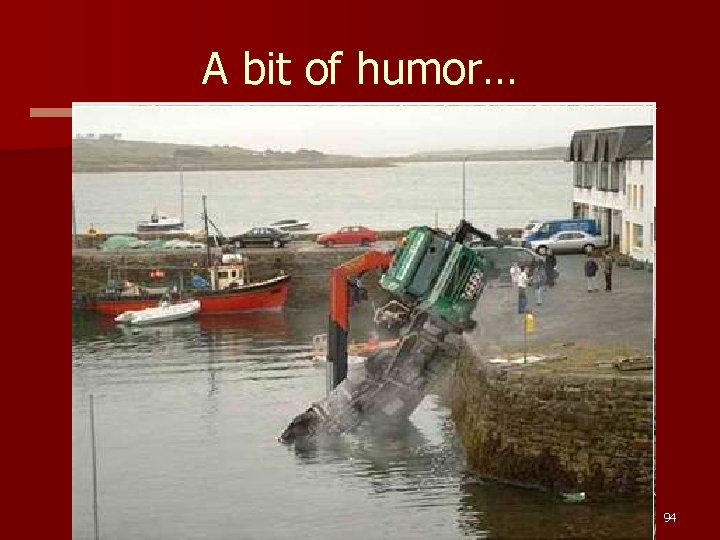
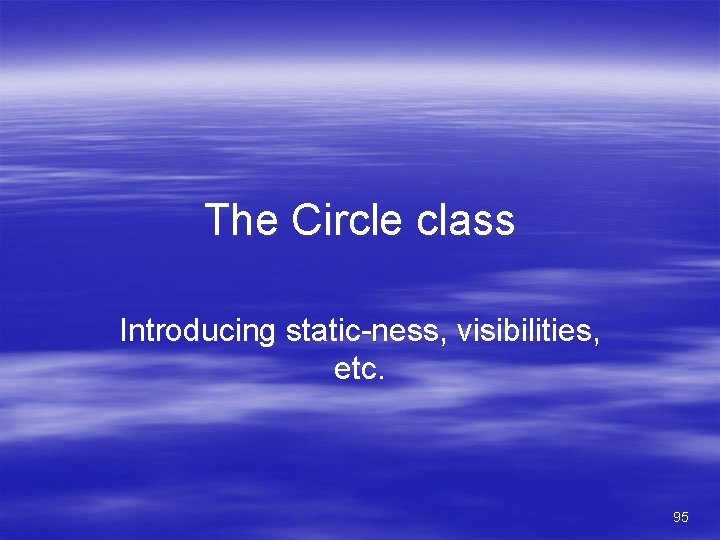
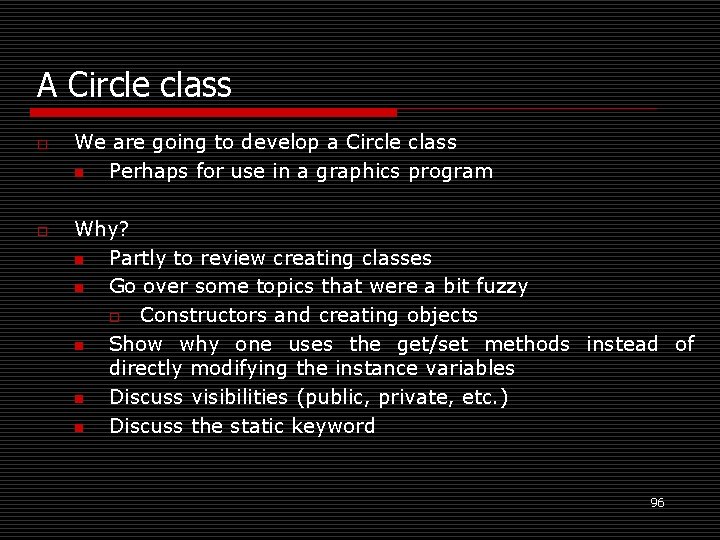
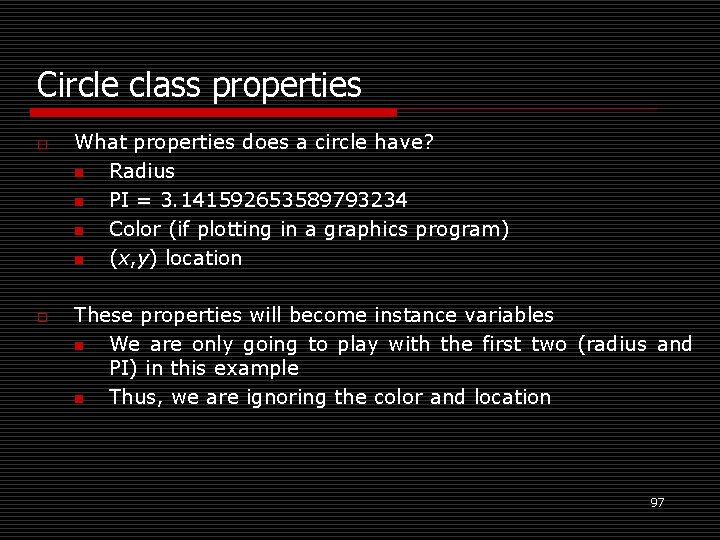
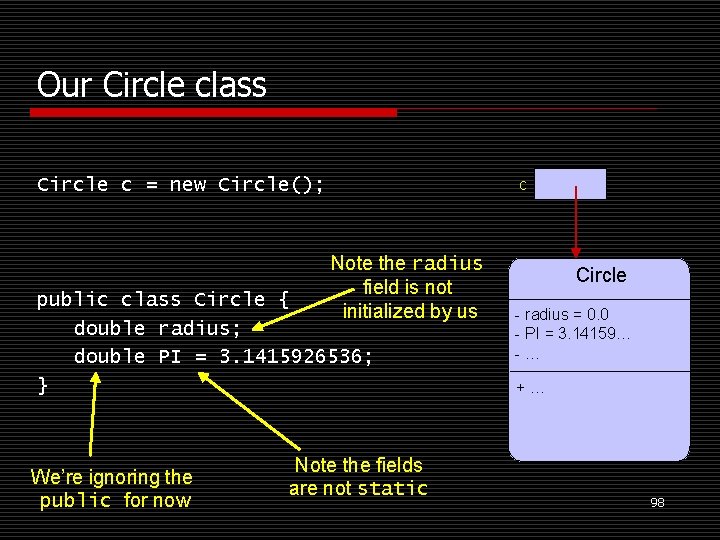
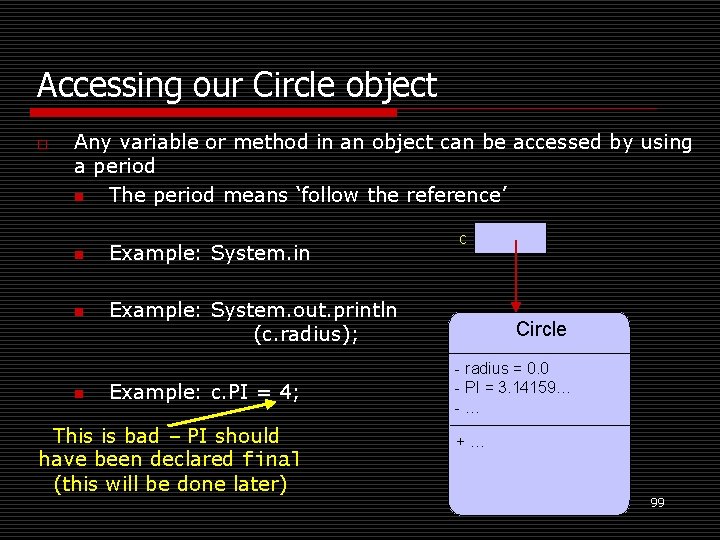
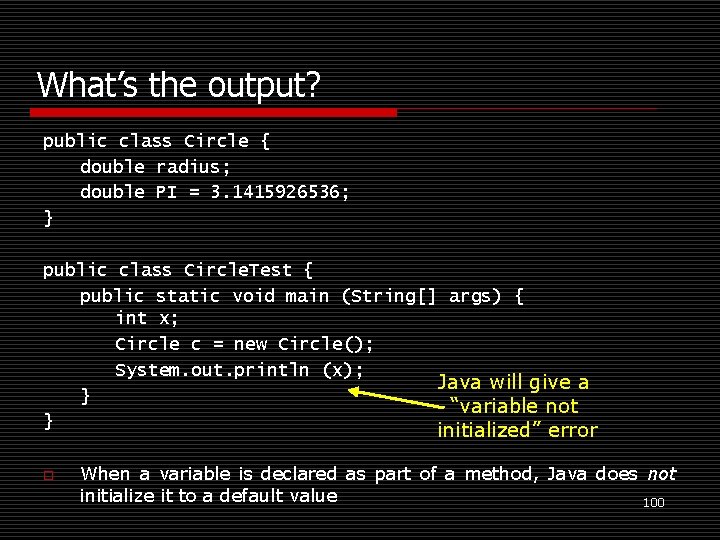
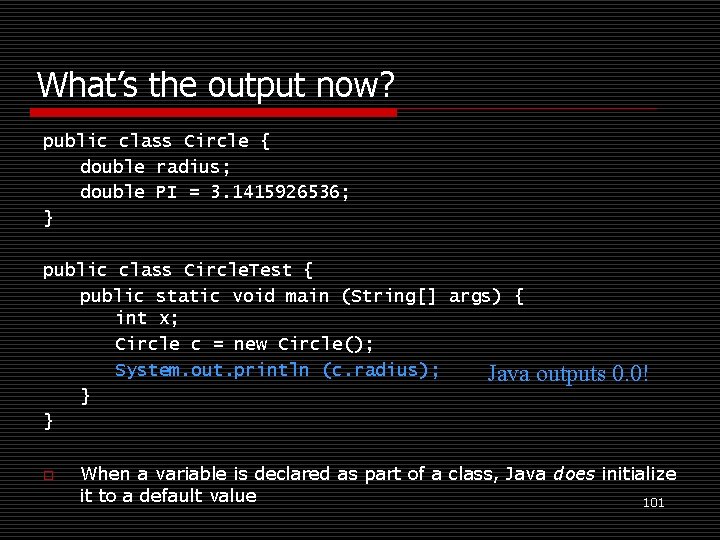
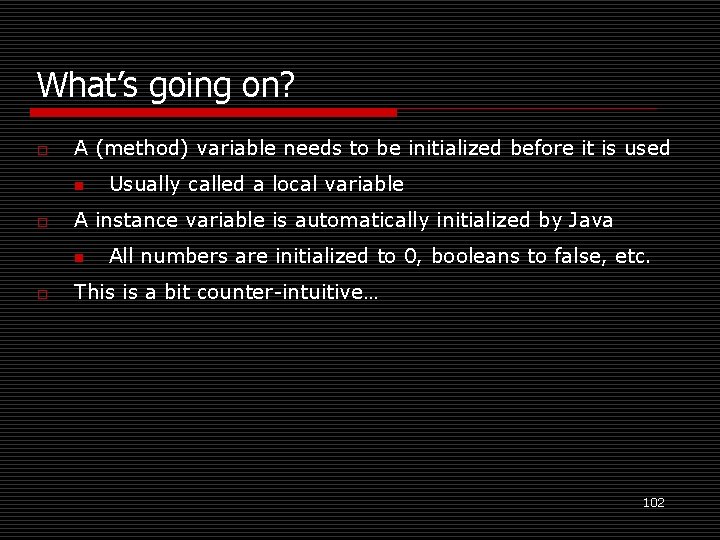
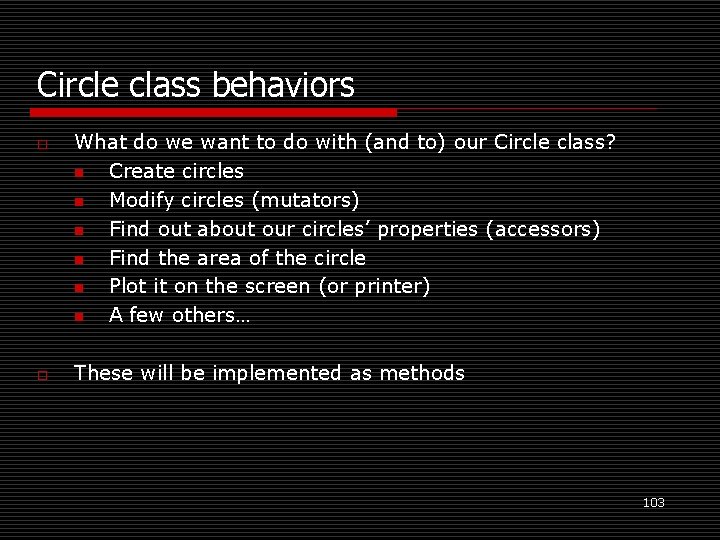
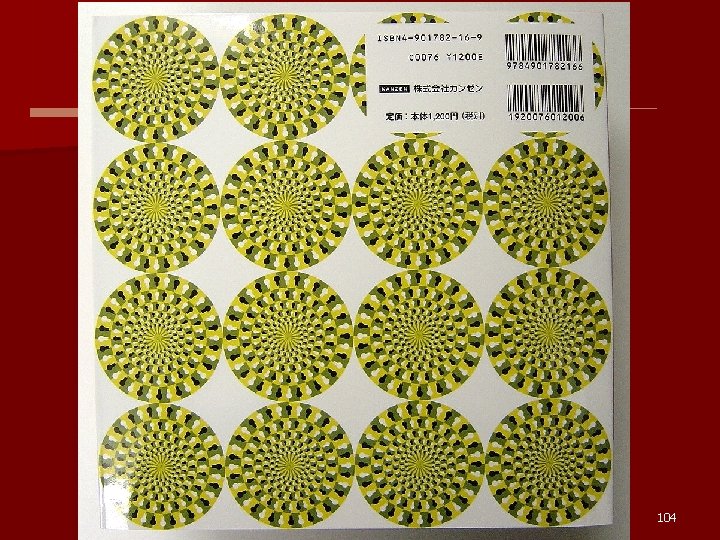
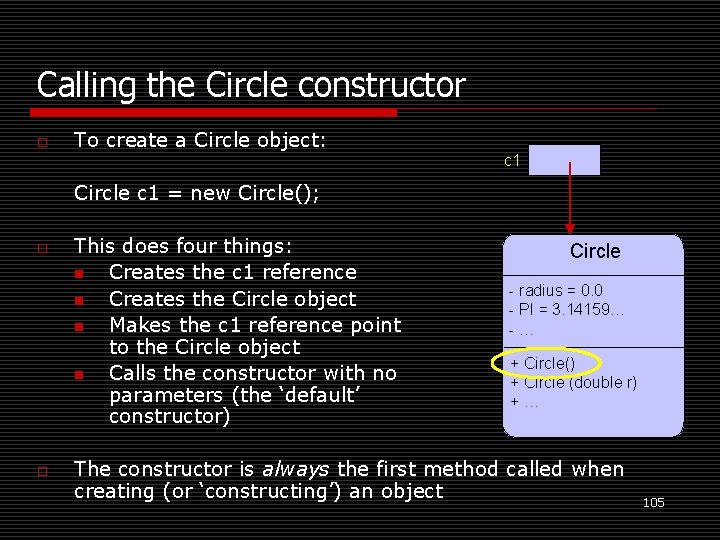
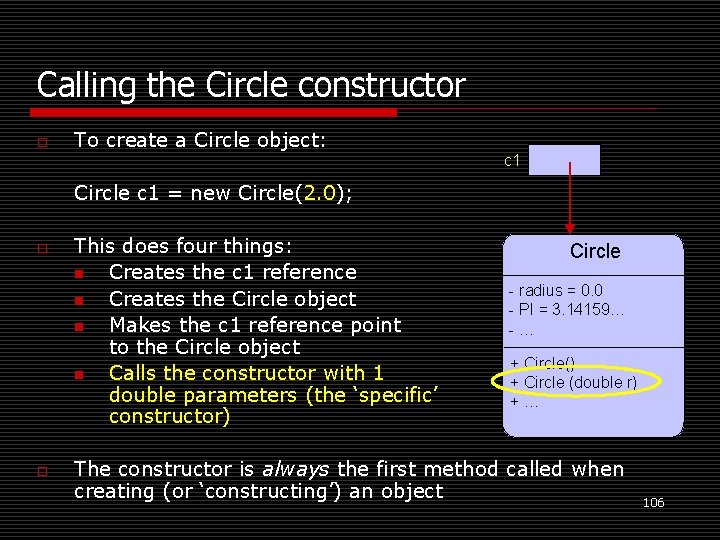
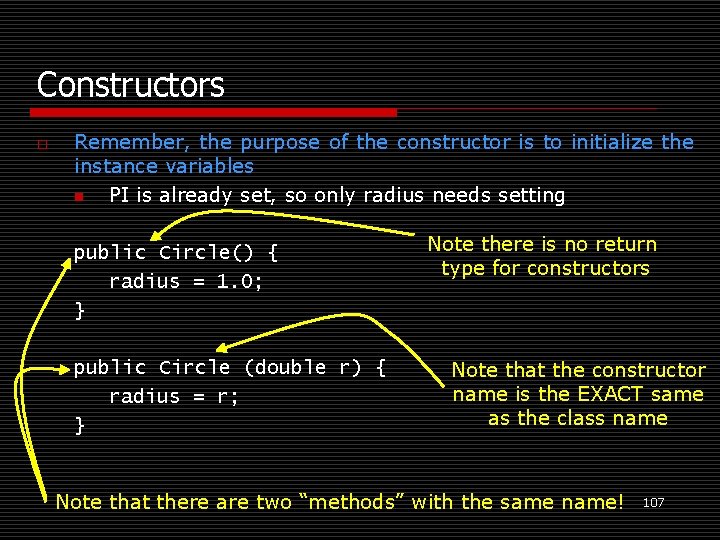
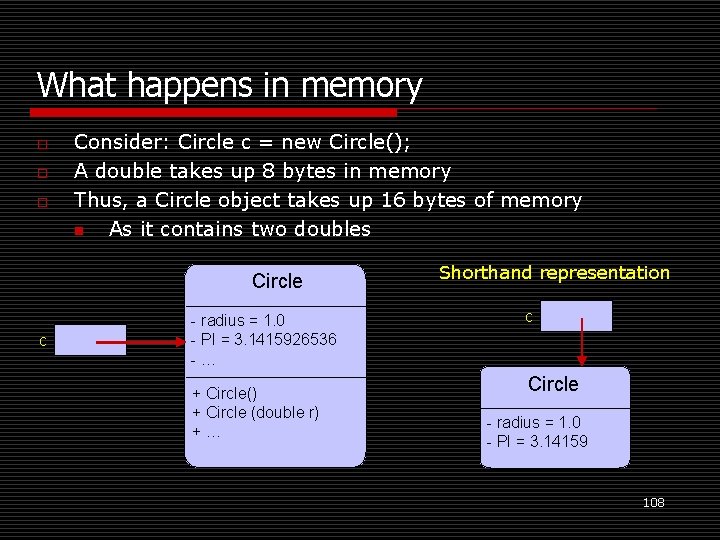
![Consider the following code public class Circle. Test { public static void main (String[] Consider the following code public class Circle. Test { public static void main (String[]](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-106.jpg)
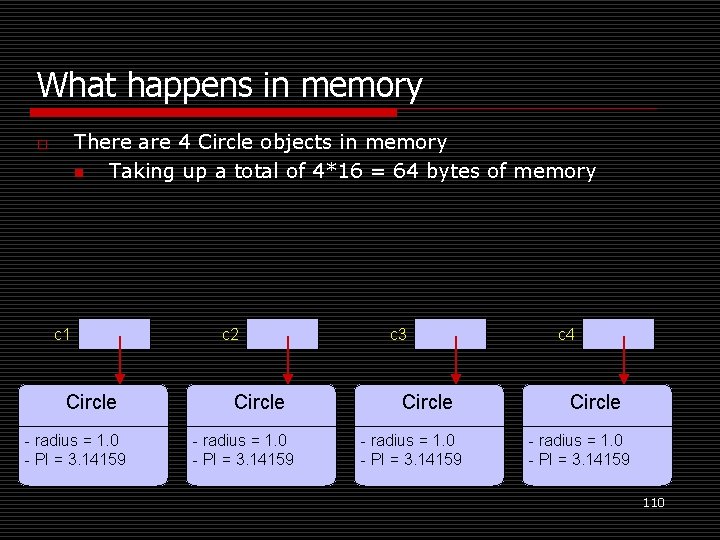
![Consider the following code public class Circle. Test { public static void main (String[] Consider the following code public class Circle. Test { public static void main (String[]](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-108.jpg)
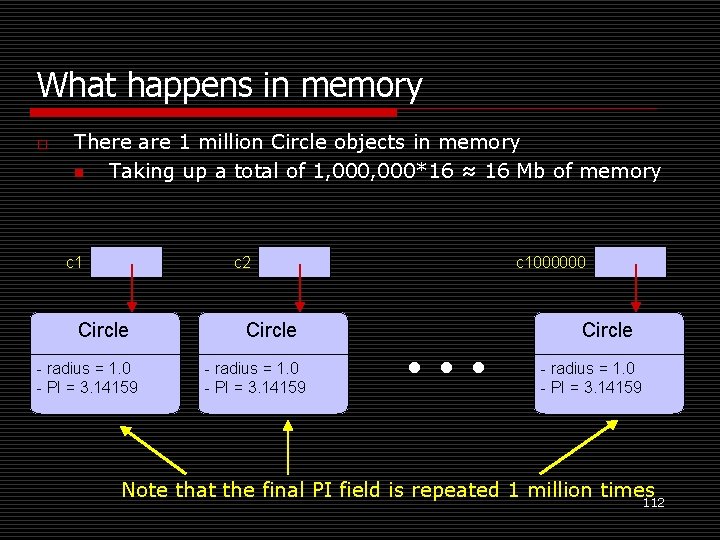
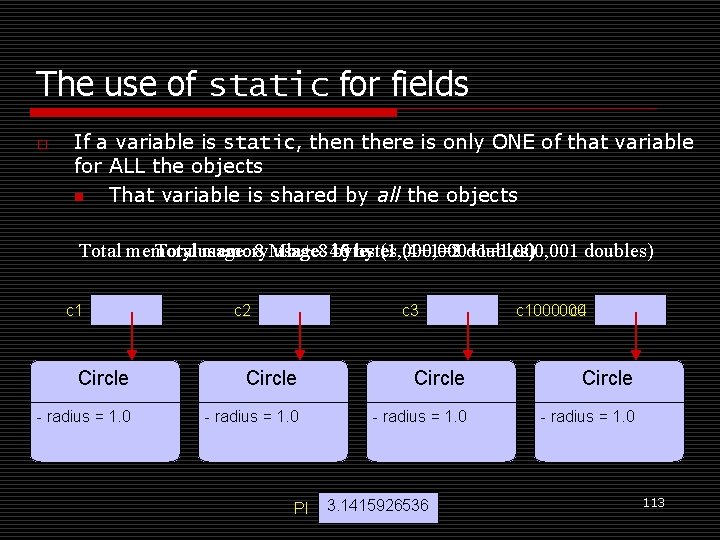
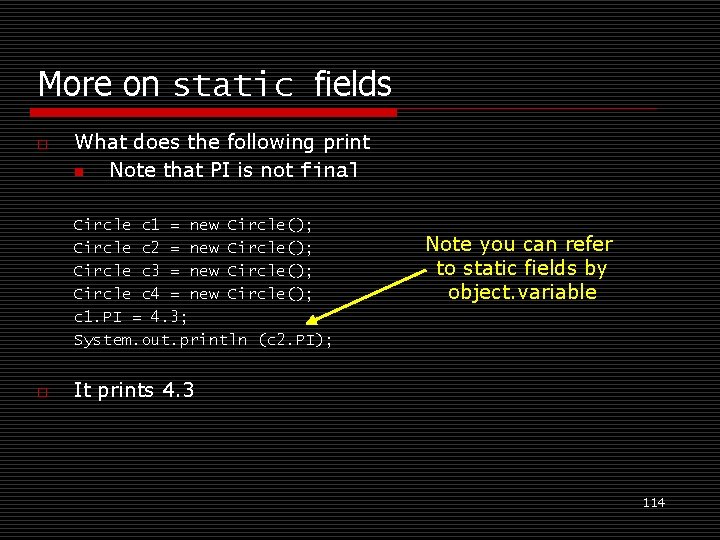
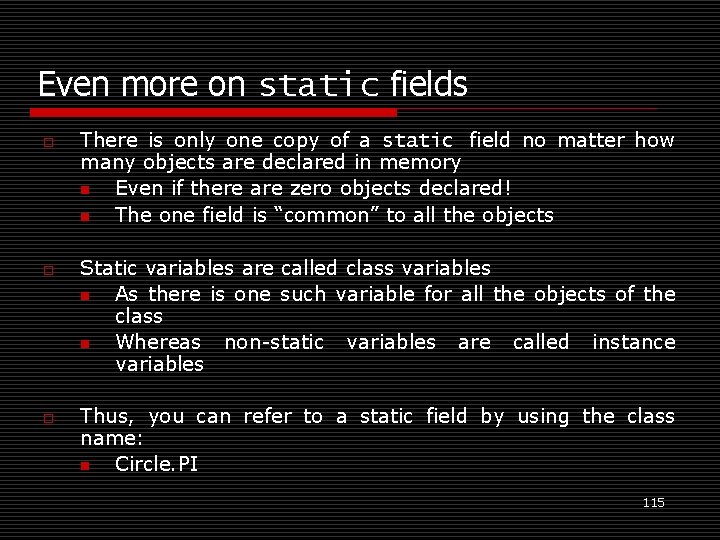
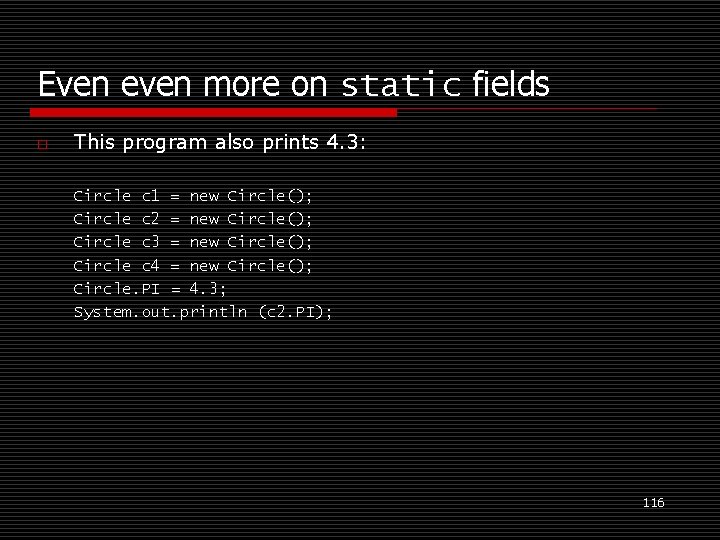
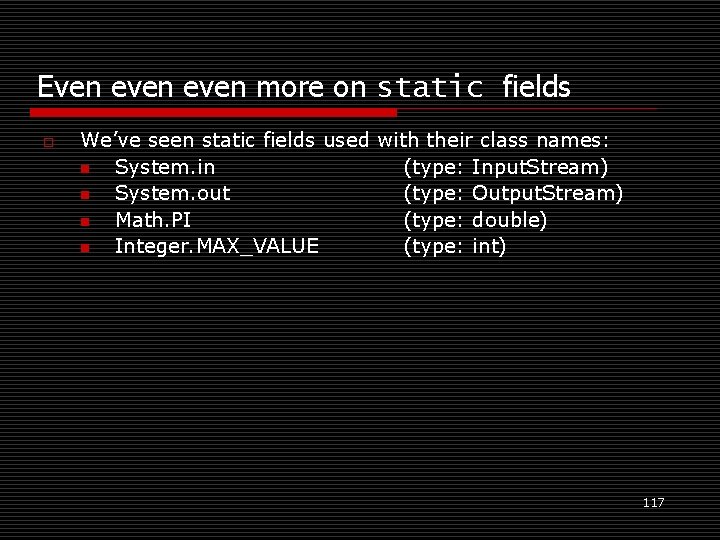
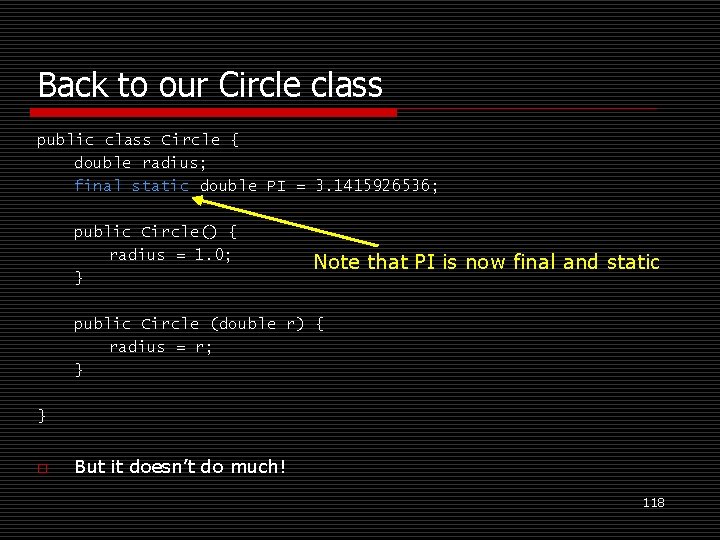
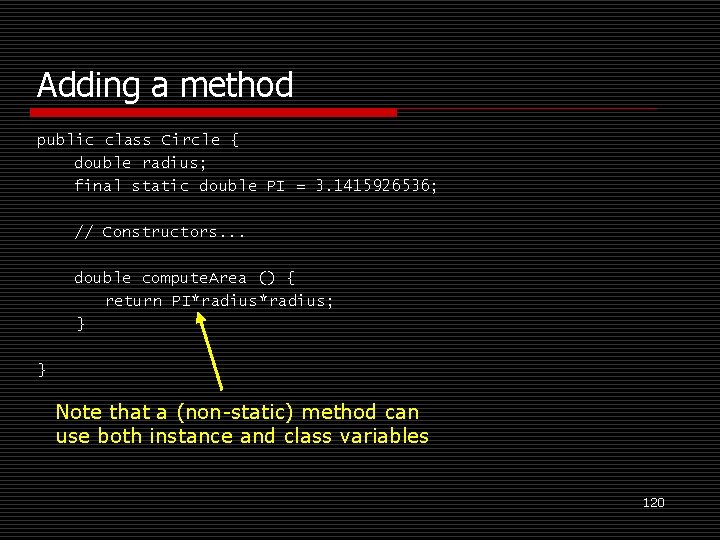
![Using that method public class Circle. Test { public static void main (String[] args) Using that method public class Circle. Test { public static void main (String[] args)](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-117.jpg)
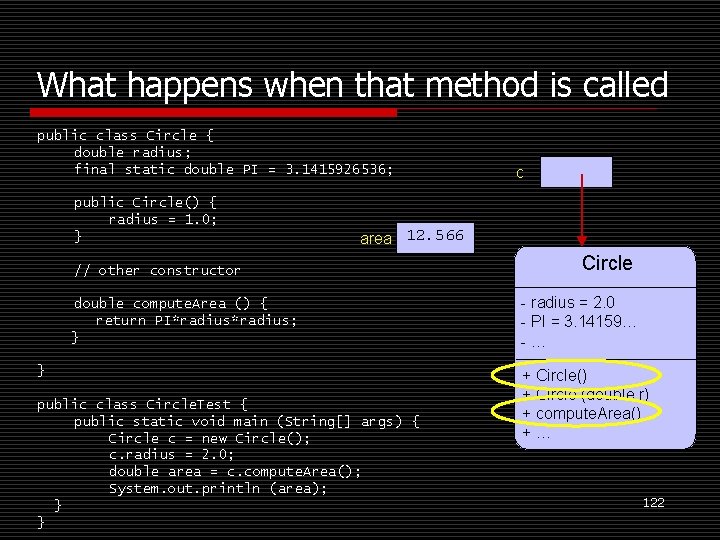
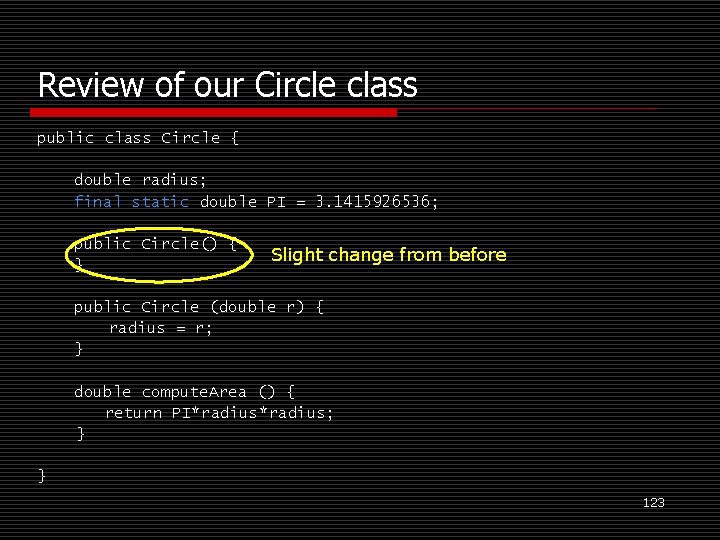
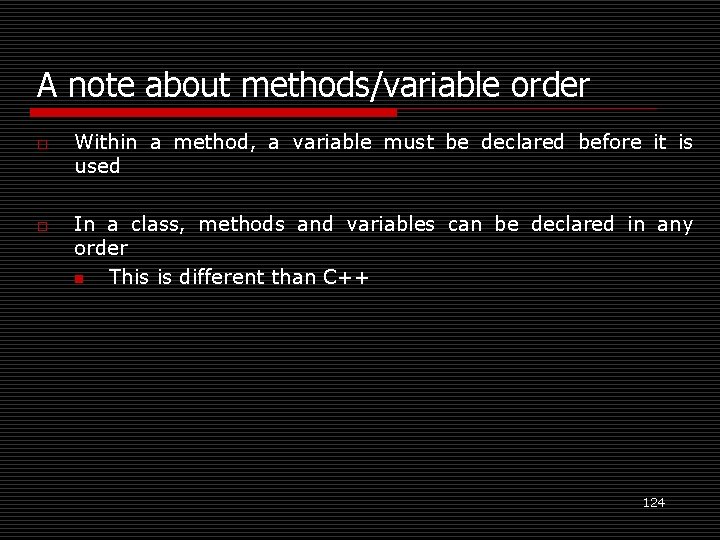
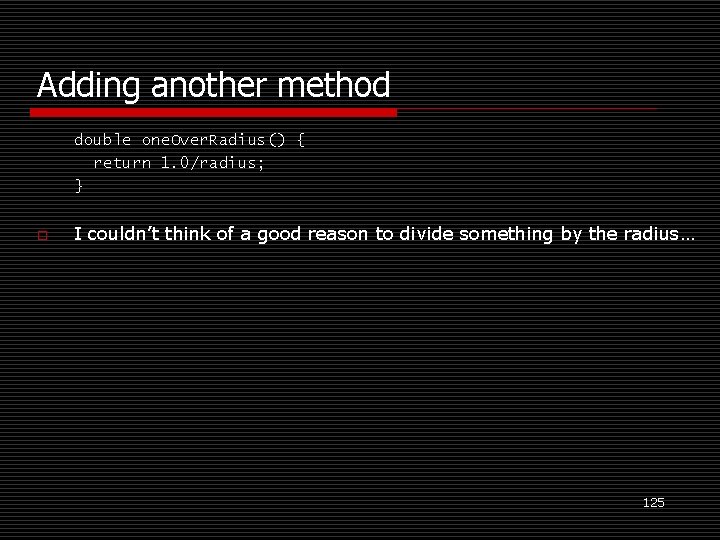
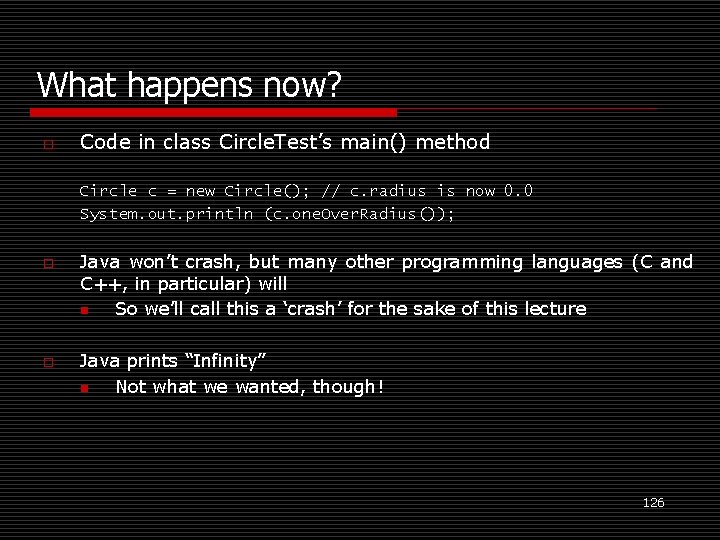
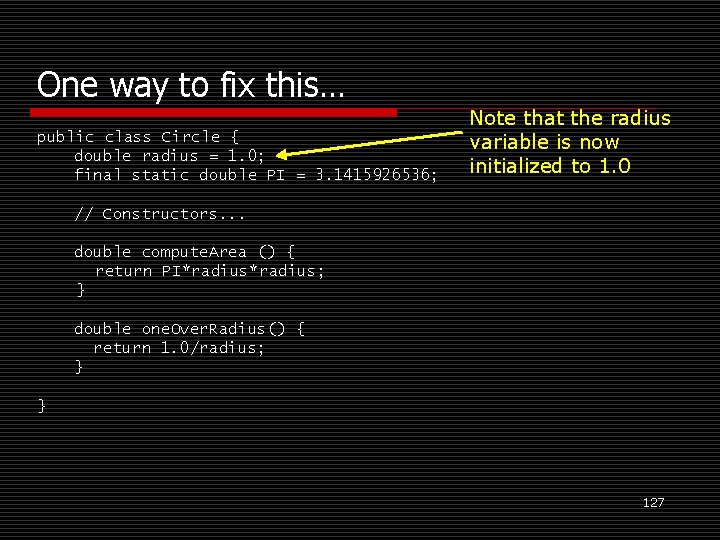
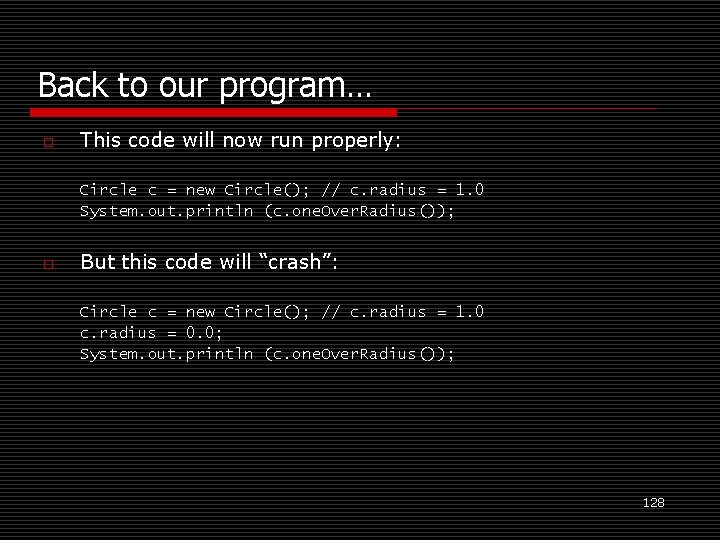
![Where the “crash” occurs public class Circle. Test { public static void main (String[] Where the “crash” occurs public class Circle. Test { public static void main (String[]](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-125.jpg)
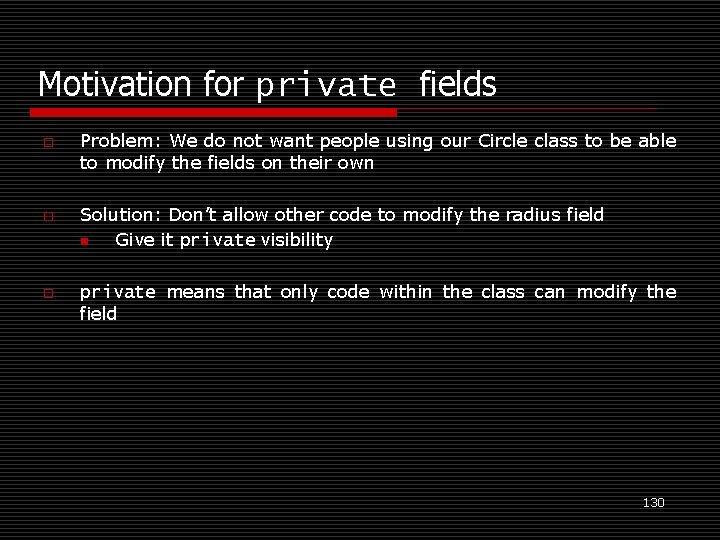
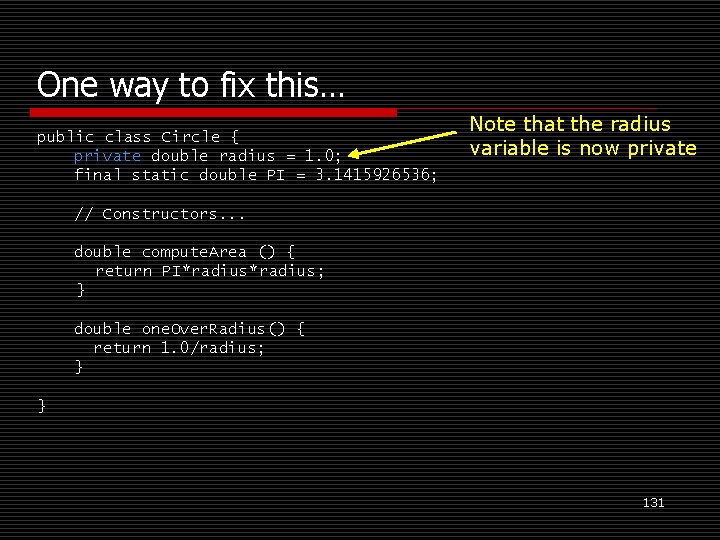
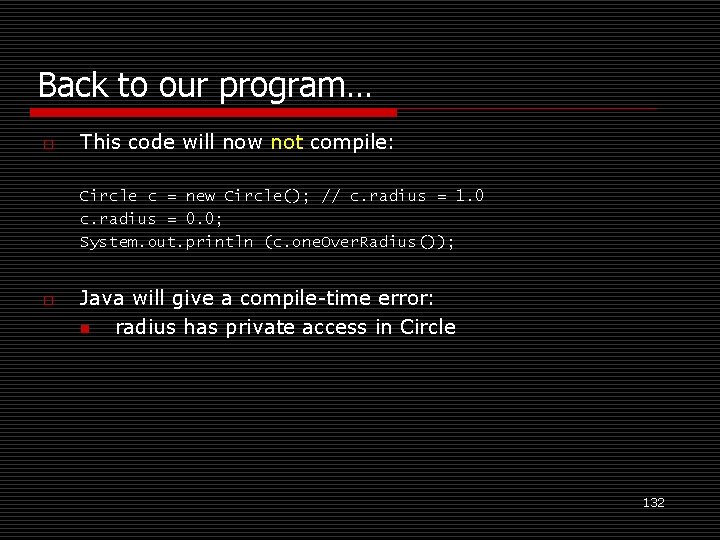
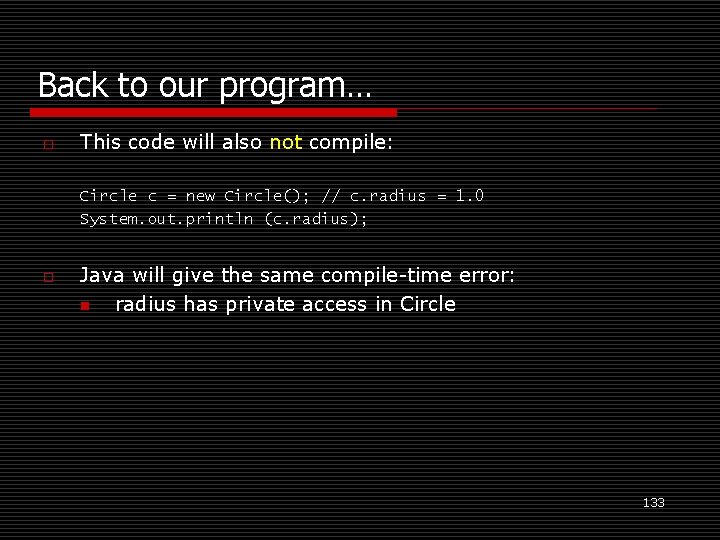
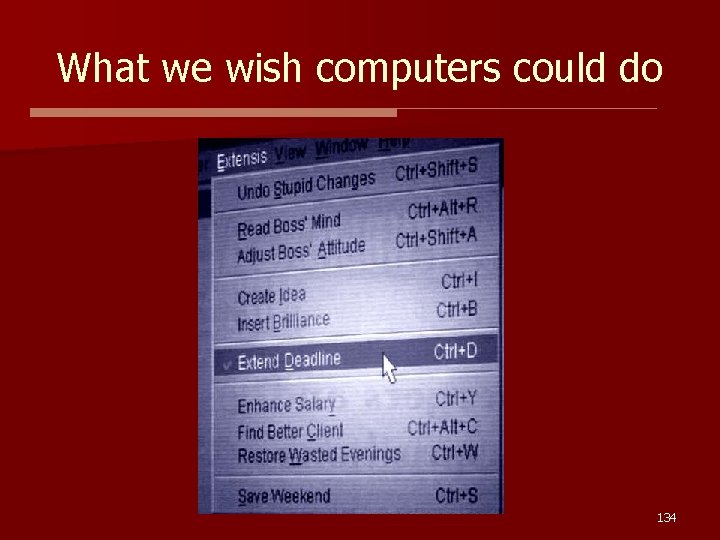
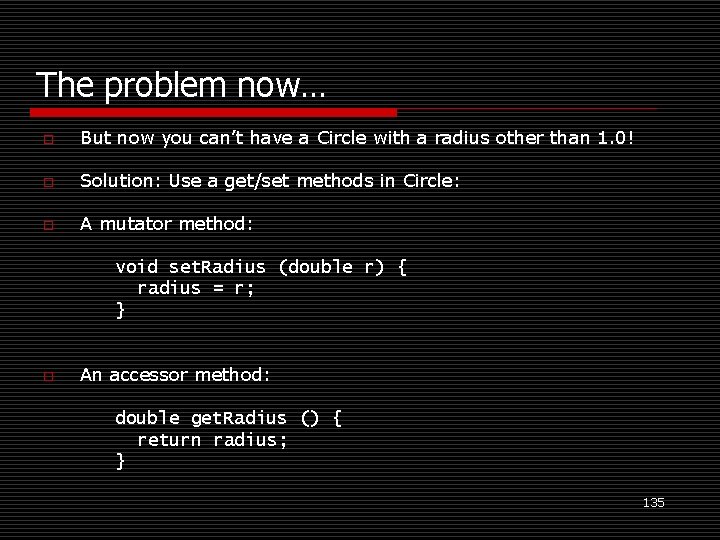
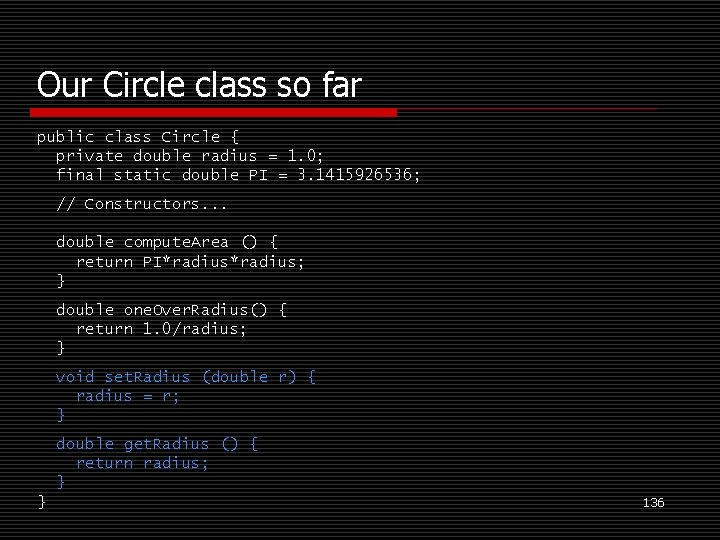
![Using the get/set methods public class Circle. Test { public static void main (String[] Using the get/set methods public class Circle. Test { public static void main (String[]](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-133.jpg)
![Wait! Another problem! public class Circle. Test { public static void main (String[] args) Wait! Another problem! public class Circle. Test { public static void main (String[] args)](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-134.jpg)
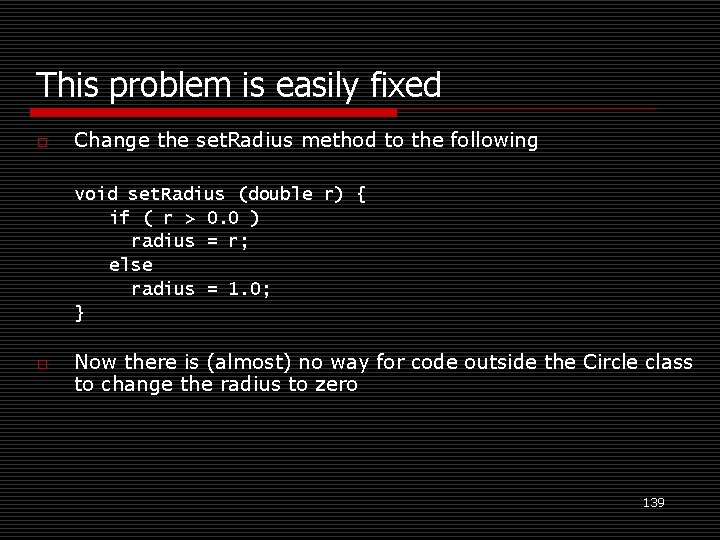
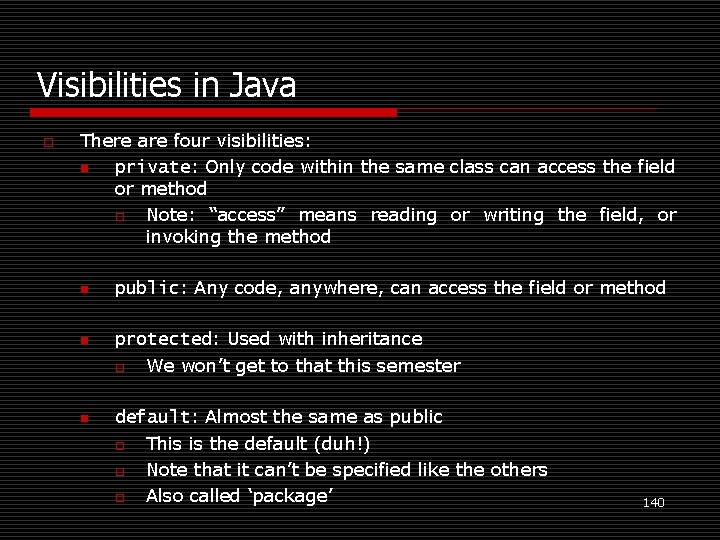
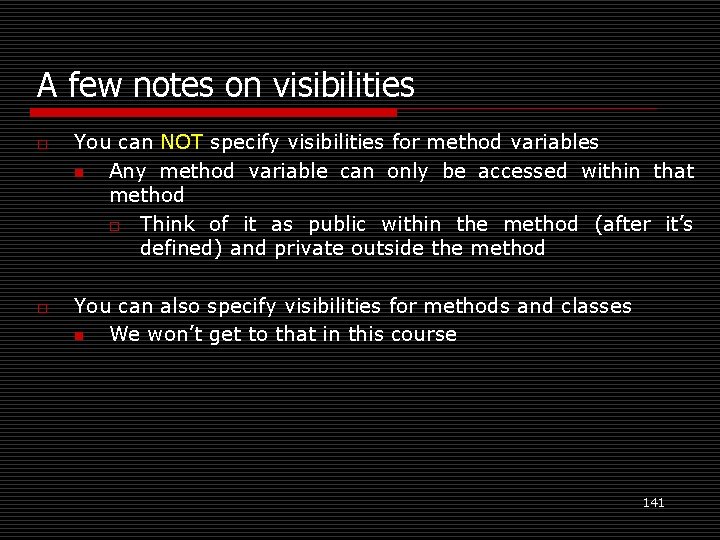
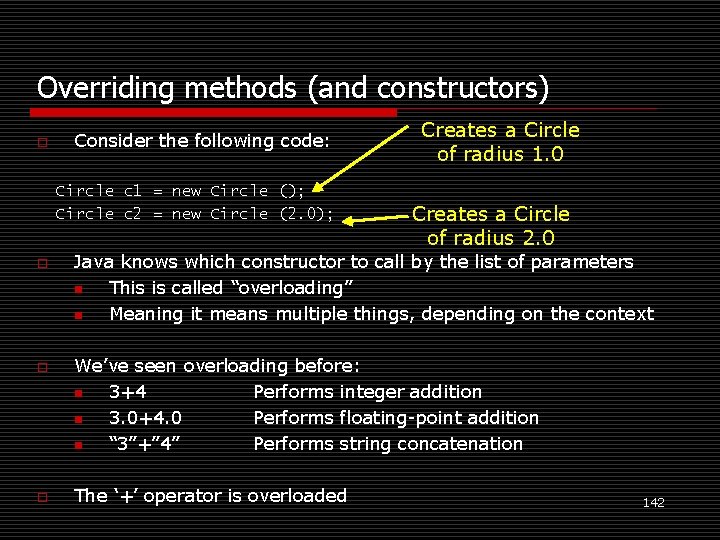
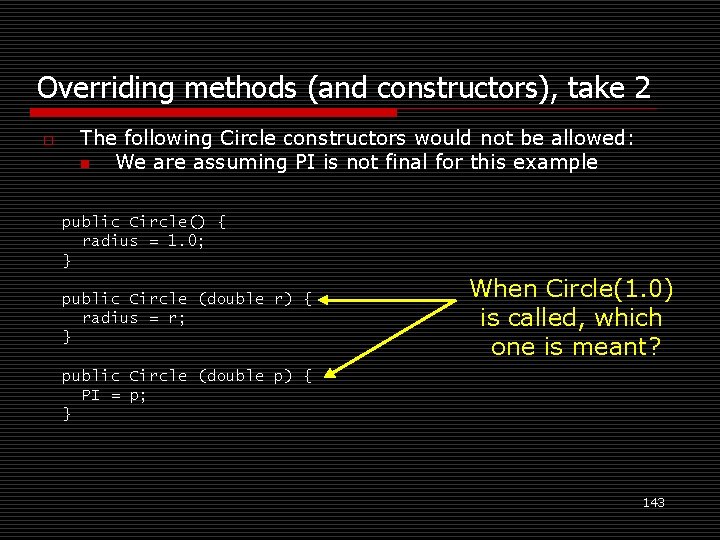
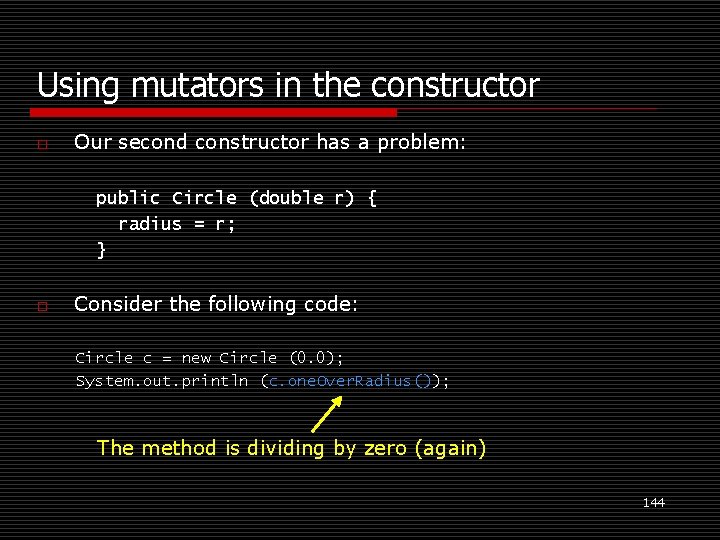
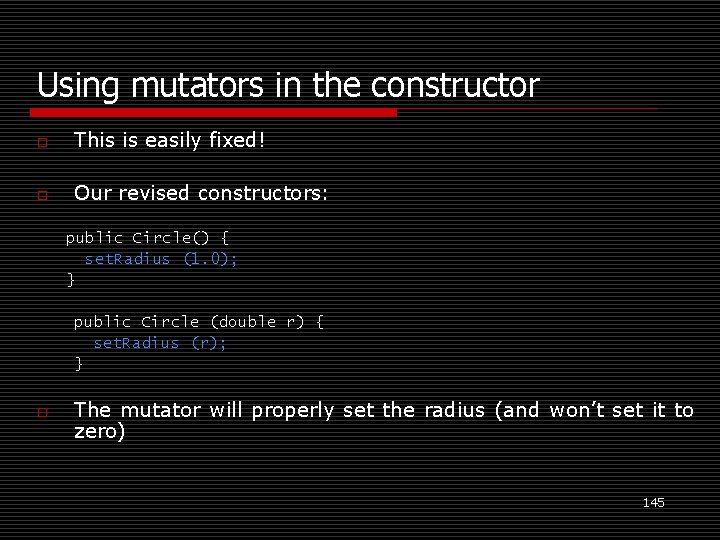
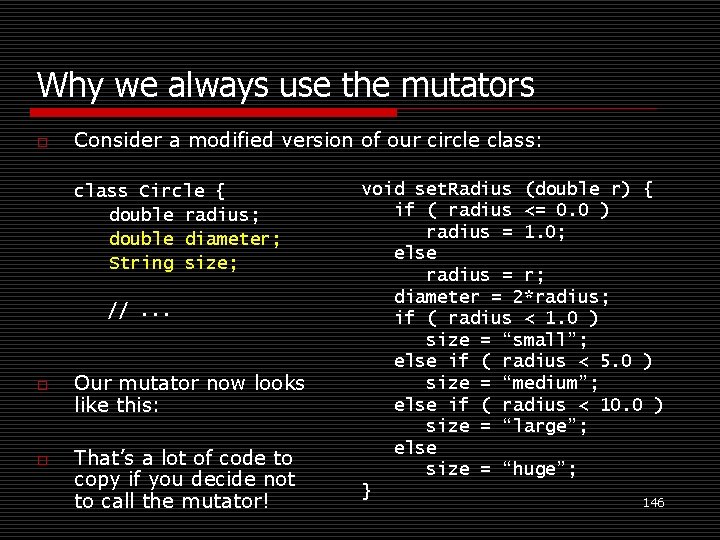
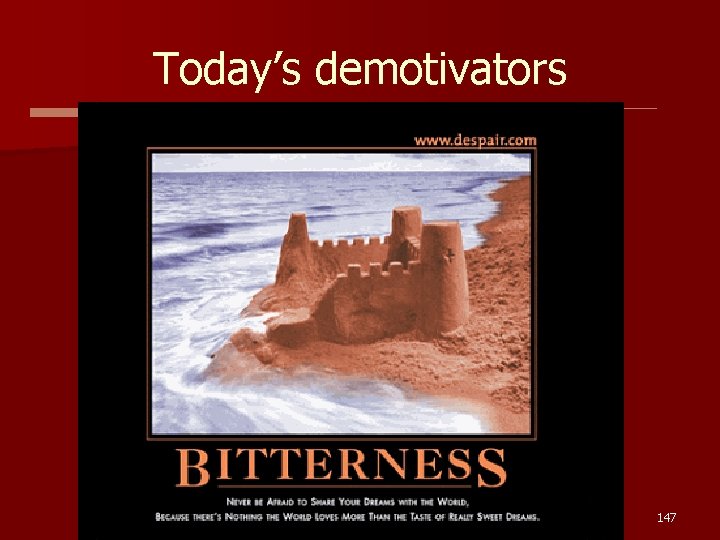
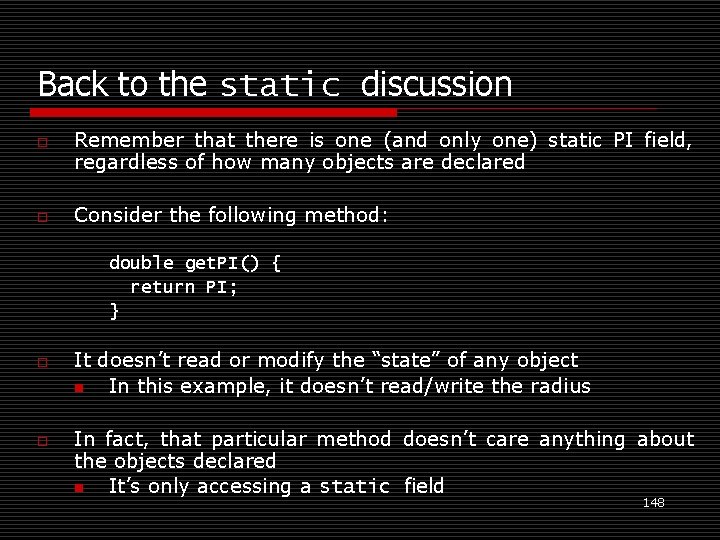
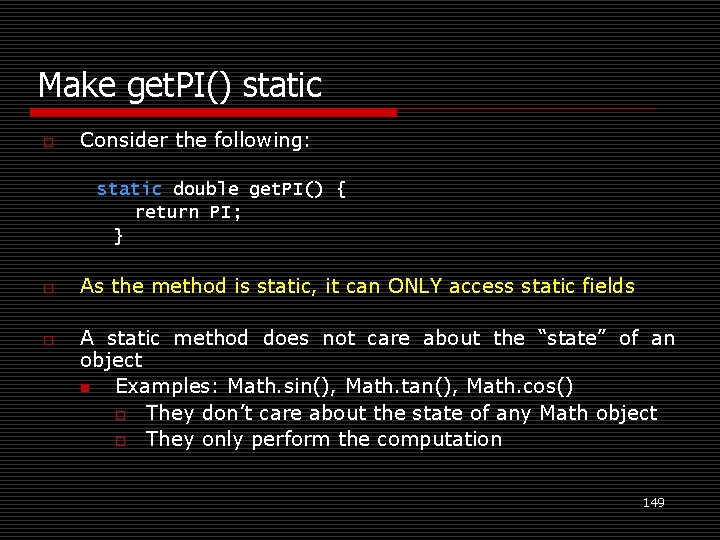
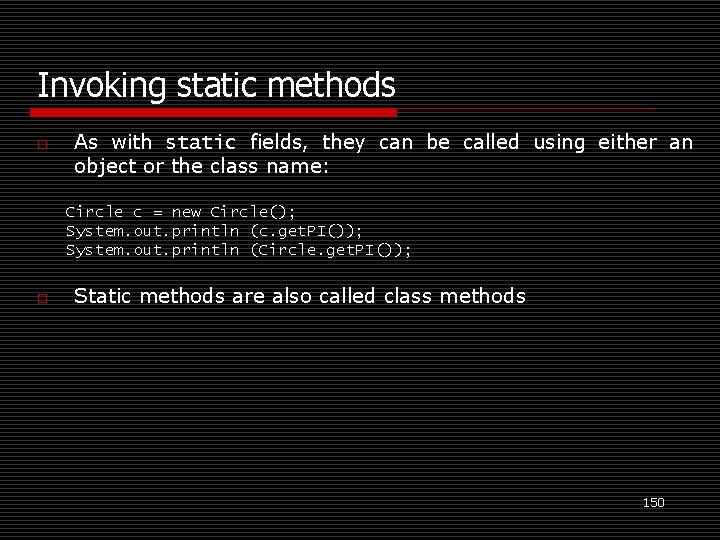
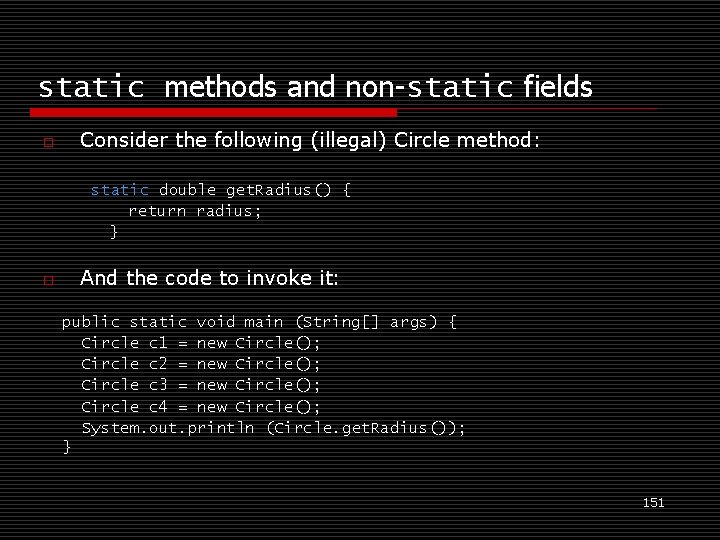
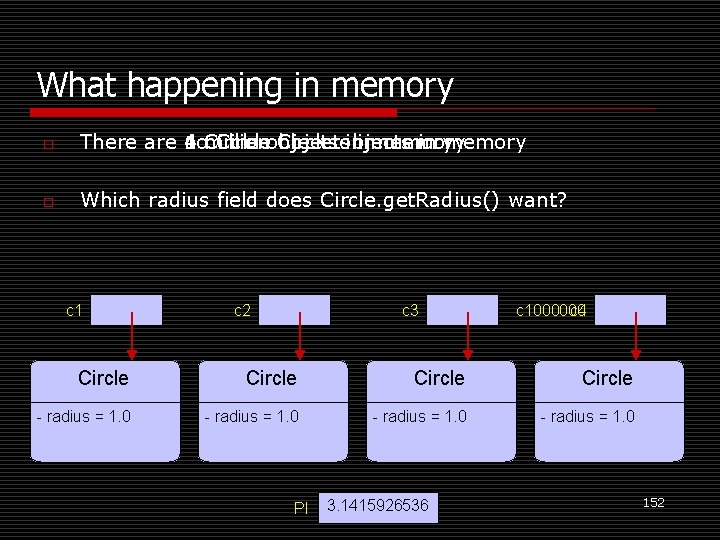
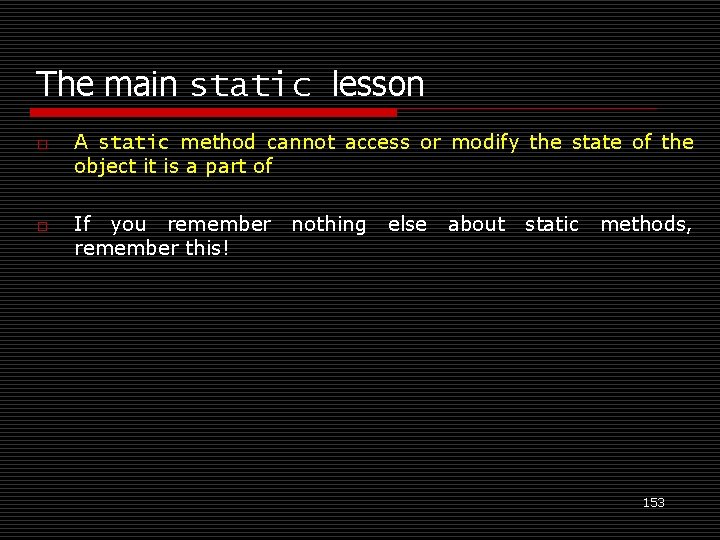
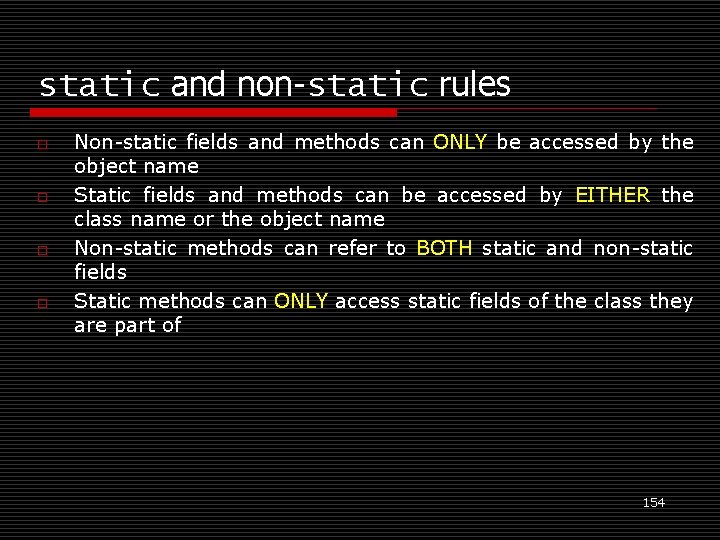
![Back to our main() method public static void main (String[] args) We’ll learn about Back to our main() method public static void main (String[] args) We’ll learn about](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-151.jpg)
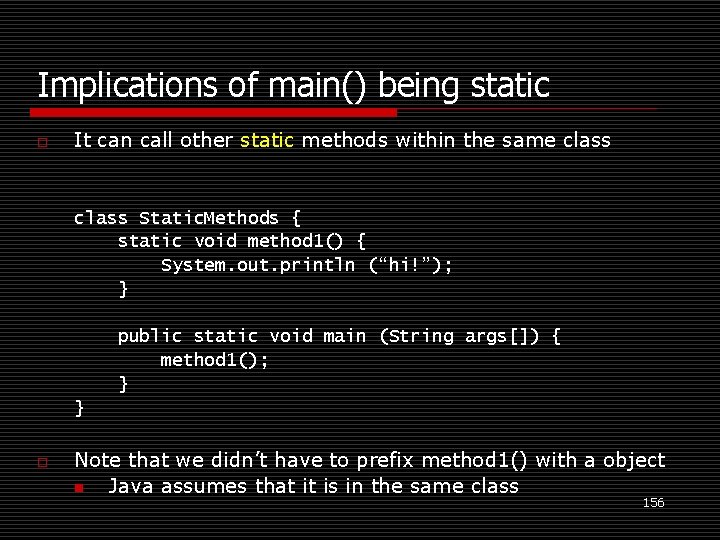
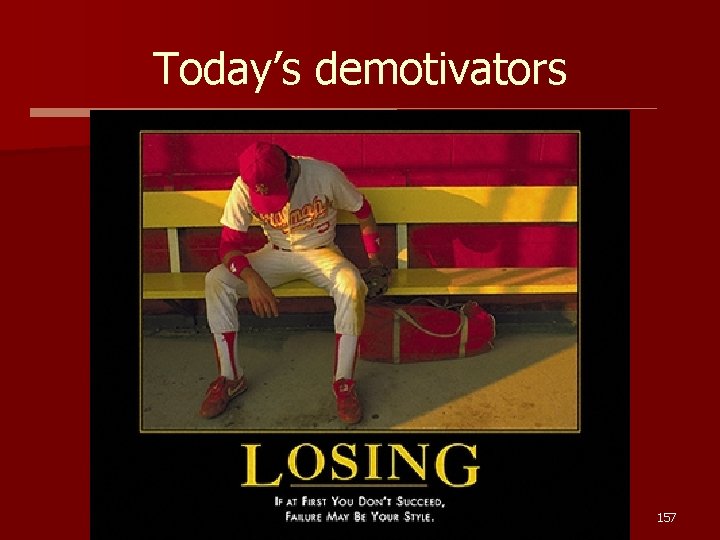
- Slides: 153
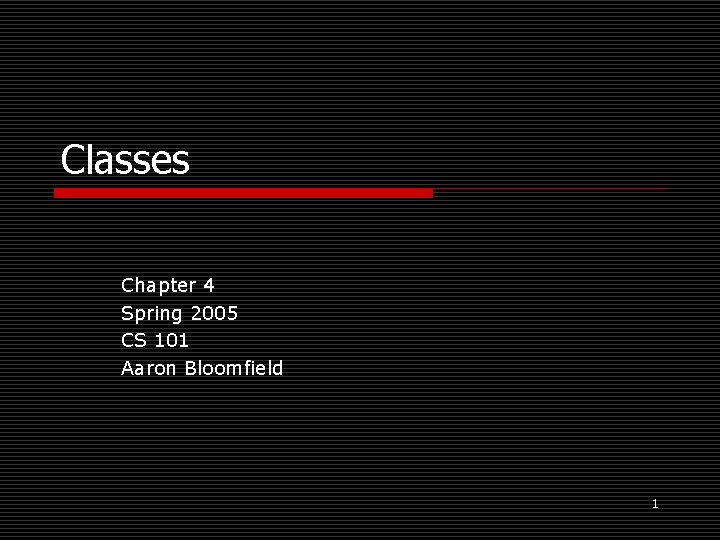
Classes Chapter 4 Spring 2005 CS 101 Aaron Bloomfield 1
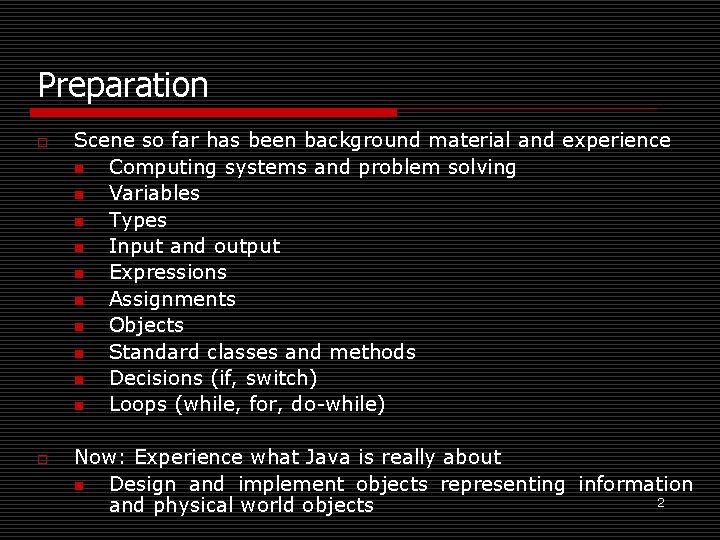
Preparation o o Scene so far has been background material and experience n Computing systems and problem solving n Variables n Types n Input and output n Expressions n Assignments n Objects n Standard classes and methods n Decisions (if, switch) n Loops (while, for, do-while) Now: Experience what Java is really about n Design and implement objects representing information 2 and physical world objects
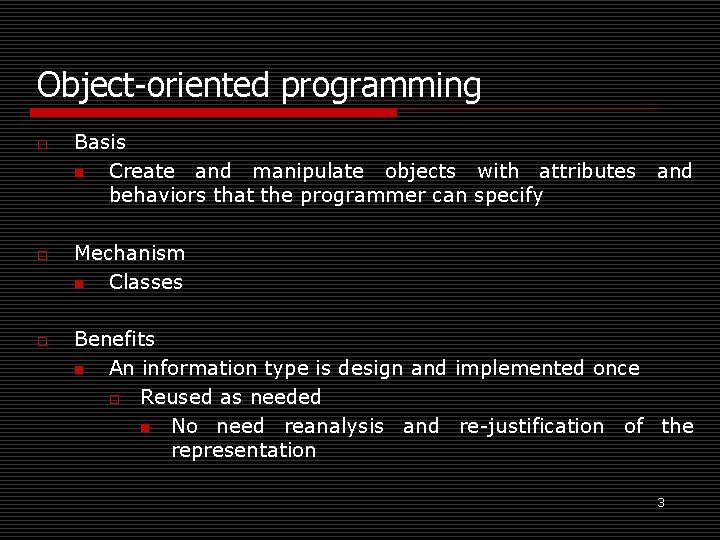
Object-oriented programming o o o Basis n Create and manipulate objects with attributes behaviors that the programmer can specify and Mechanism n Classes Benefits n An information type is design and implemented once o Reused as needed n No need reanalysis and re-justification of the representation 3
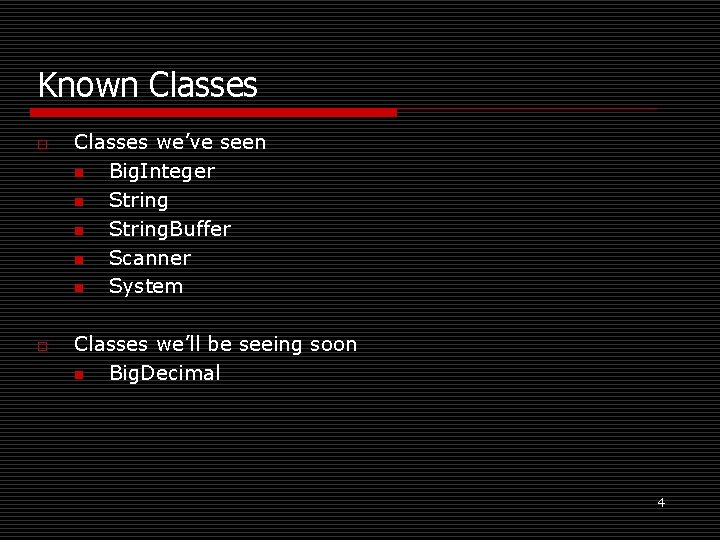
Known Classes o o Classes we’ve seen n Big. Integer n String. Buffer n Scanner n System Classes we’ll be seeing soon n Big. Decimal 4
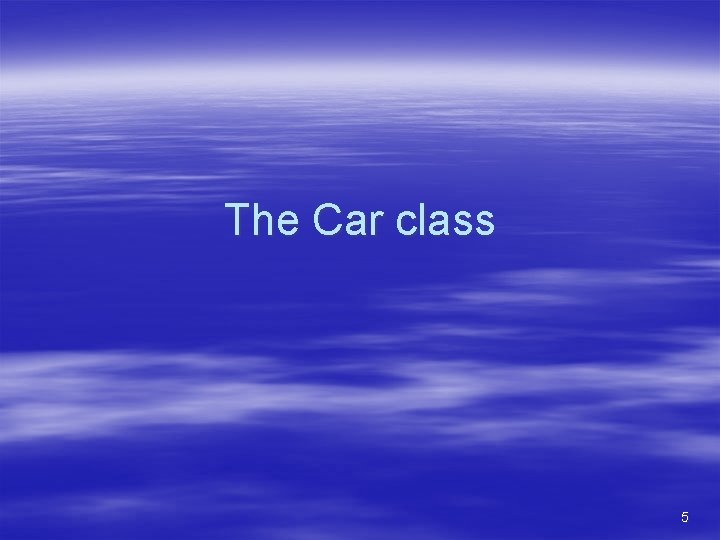
The Car class 5
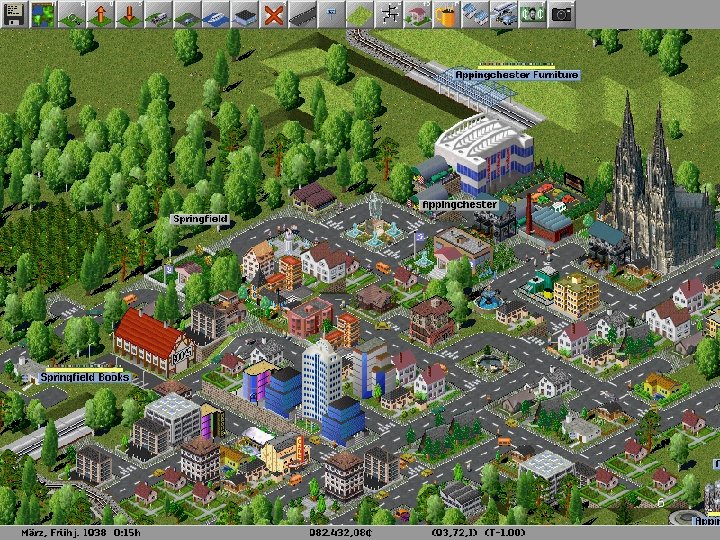
More on classes vs. objects 6
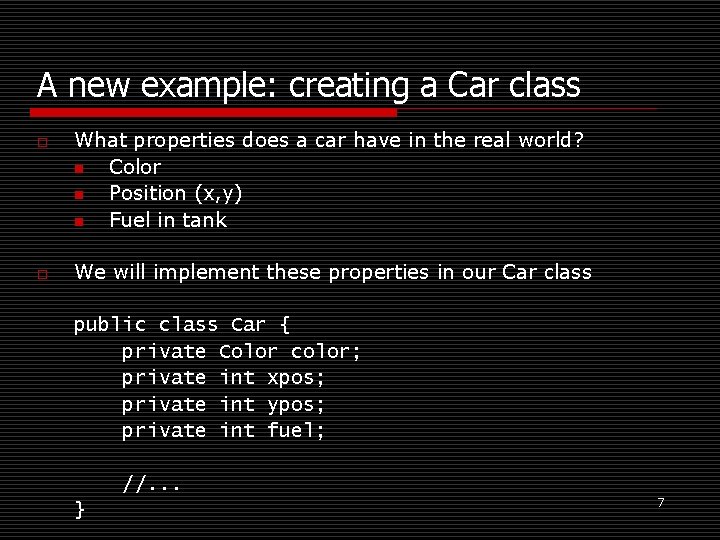
A new example: creating a Car class o o What properties does a car have in the real world? n Color n Position (x, y) n Fuel in tank We will implement these properties in our Car class public class Car { private Color color; private int xpos; private int ypos; private int fuel; //. . . } 7
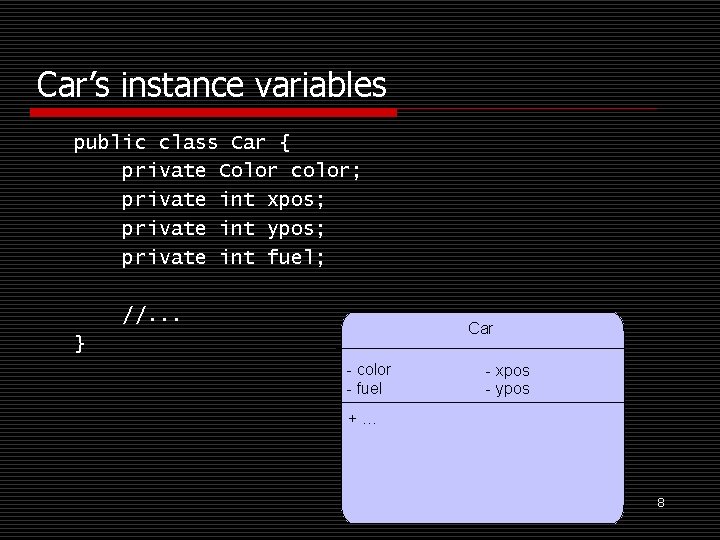
Car’s instance variables public class Car { private Color color; private int xpos; private int ypos; private int fuel; //. . . Car } - color - fuel - xpos - ypos +… 8
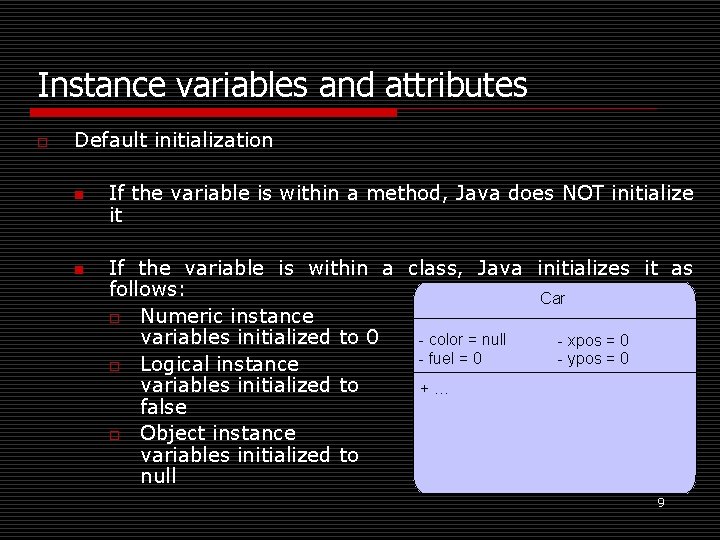
Instance variables and attributes o Default initialization n n If the variable is within a method, Java does NOT initialize it If the variable is within a class, Java initializes it as follows: Car o Numeric instance variables initialized to 0 - color = null - xpos = 0 - fuel = 0 - ypos = 0 o Logical instance variables initialized to +… false o Object instance variables initialized to null 9
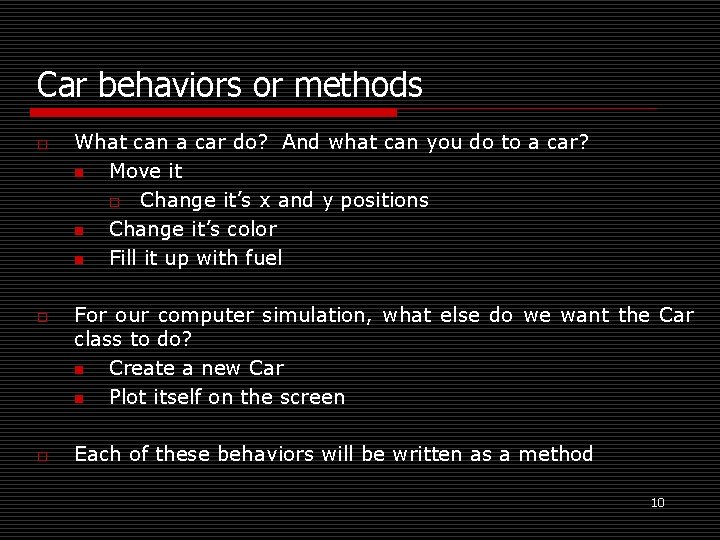
Car behaviors or methods o o o What can a car do? And what can you do to a car? n Move it o Change it’s x and y positions n Change it’s color n Fill it up with fuel For our computer simulation, what else do we want the Car class to do? n Create a new Car n Plot itself on the screen Each of these behaviors will be written as a method 10
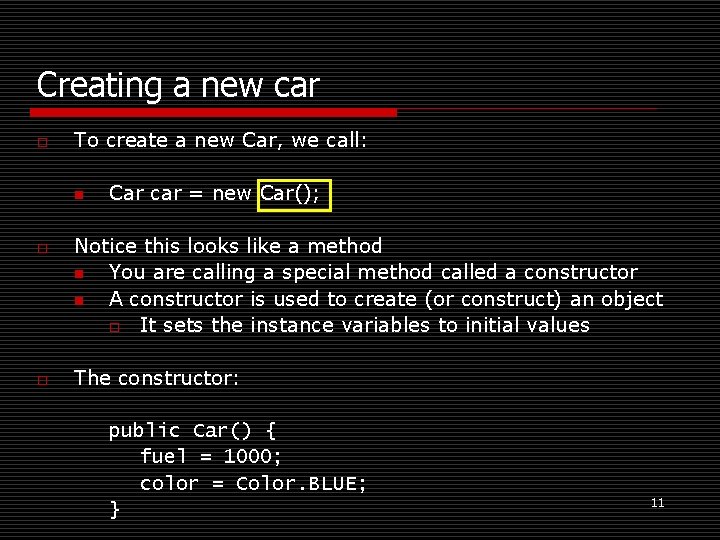
Creating a new car o To create a new Car, we call: n o o Car car = new Car(); Notice this looks like a method n You are calling a special method called a constructor n A constructor is used to create (or construct) an object o It sets the instance variables to initial values The constructor: public Car() { fuel = 1000; color = Color. BLUE; } 11
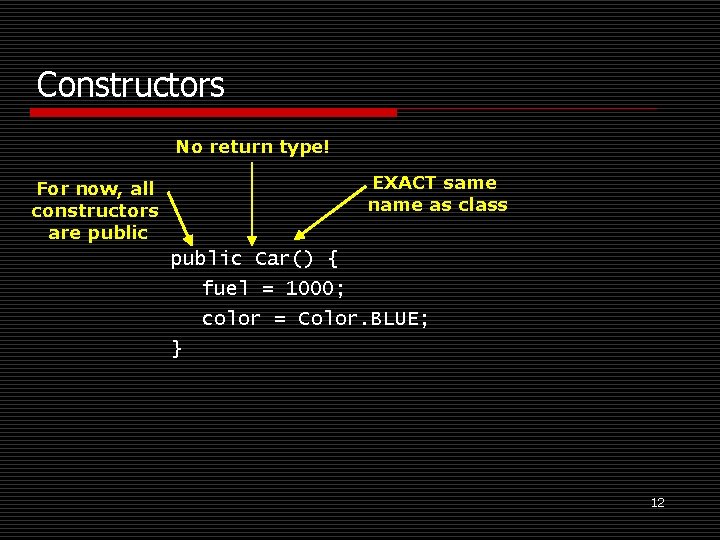
Constructors No return type! For now, all constructors are public EXACT same name as class public Car() { fuel = 1000; color = Color. BLUE; } 12
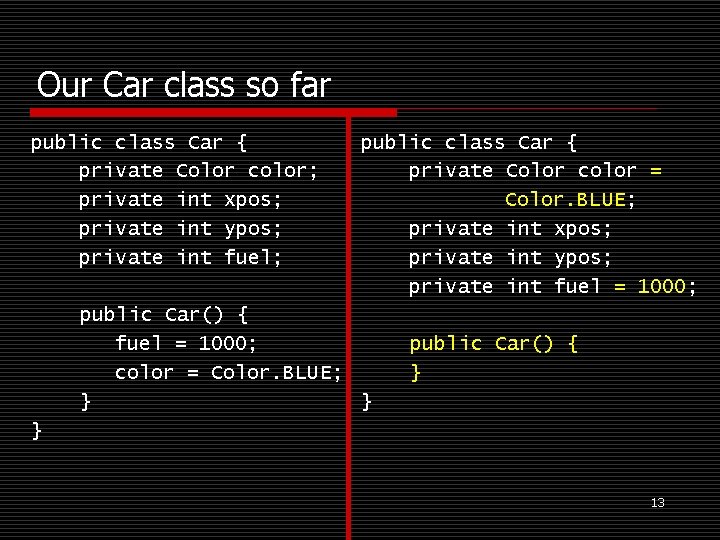
Our Car class so far public class Car { private Color color; private int xpos; private int ypos; private int fuel; public class Car { private Color color = Color. BLUE; private int xpos; private int ypos; private int fuel = 1000; public Car() { fuel = 1000; color = Color. BLUE; } } public Car() { } } 13
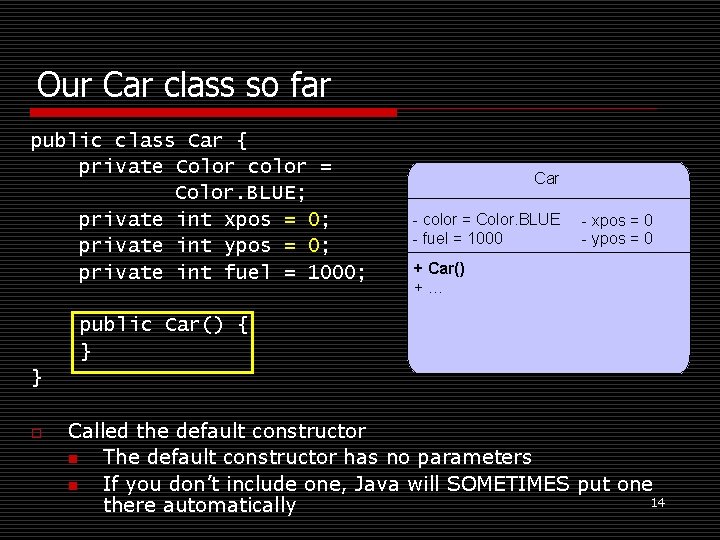
Our Car class so far public class Car { private Color color = Color. BLUE; private int xpos = 0; private int ypos = 0; private int fuel = 1000; Car - color = Color. BLUE - fuel = 1000 - xpos = 0 - ypos = 0 + Car() +… public Car() { } } o Called the default constructor n The default constructor has no parameters n If you don’t include one, Java will SOMETIMES put one 14 there automatically
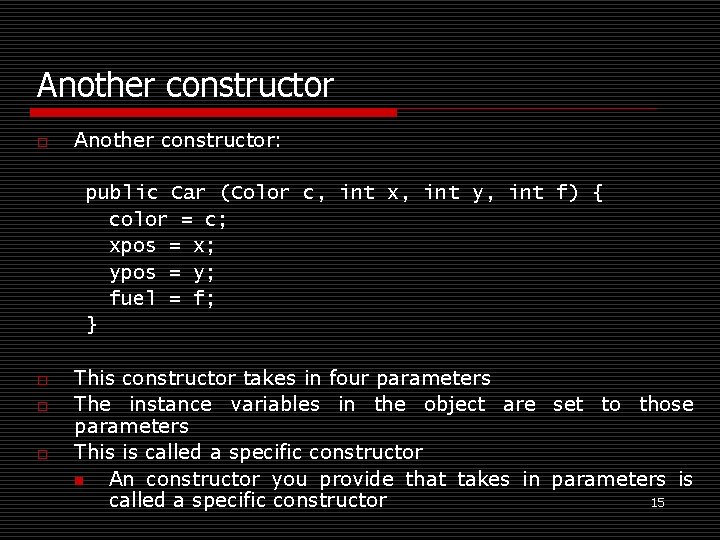
Another constructor o Another constructor: public Car (Color c, int x, int y, int f) { color = c; xpos = x; ypos = y; fuel = f; } o o o This constructor takes in four parameters The instance variables in the object are set to those parameters This is called a specific constructor n An constructor you provide that takes in parameters is 15 called a specific constructor
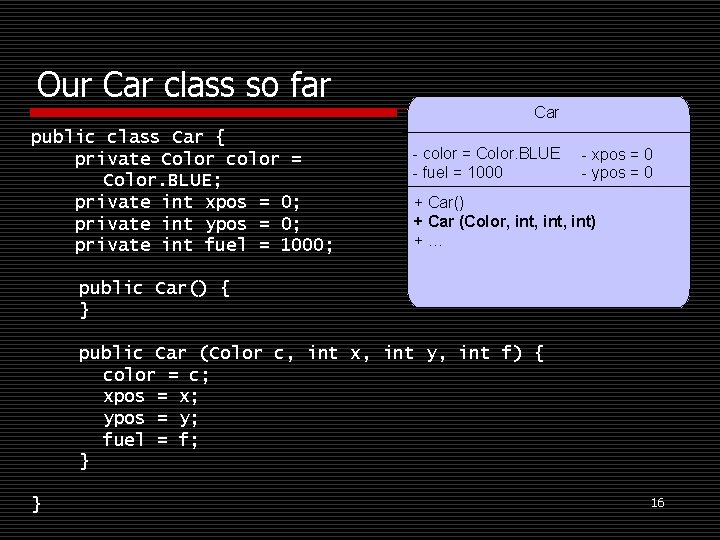
Our Car class so far public class Car { private Color color = Color. BLUE; private int xpos = 0; private int ypos = 0; private int fuel = 1000; Car - color = Color. BLUE - fuel = 1000 - xpos = 0 - ypos = 0 + Car() + Car (Color, int, int) +… public Car() { } public Car (Color c, int x, int y, int f) { color = c; xpos = x; ypos = y; fuel = f; } } 16
![De CSS The program includestdlib h typedef unsigned int uint char ctb51233733 b 2663236 De. CSS: The program #include<stdlib. h> typedef unsigned int uint; char ctb[512]="33733 b 2663236](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-17.jpg)
De. CSS: The program #include<stdlib. h> typedef unsigned int uint; char ctb[512]="33733 b 2663236 b 763 e 7 e 362 b 6 e 2 e 667 bd 393 db 0643034 b 96 de 9 ed 60 b 4 e 0 e 4 69 b 57175 f 82 c 787 cf 125 a 1 a 528 fca 8 ac 21 fd 999 d 10049094190 d 898 d 001480840913 d 7 d 35246 d 2 d 65743 c 7 c 34256 c 2 c 6475 dd 9 dd 5044 d 0 d 4594 dc 9 cd 4054 c 0 c 449559195180 c 989 c 11058185 081 c 888 c 011 d 797 df 0247074 f 92 da 9 ad 20 f 4 a 0 a 429 f 53135 b 86 c 383 cb 165 e 1 e 568 bce 8 ec 61 bb 3 f 3 bba 6 e 3 a 3 ebf 6 befeb 6 abeeaee 6 fb 37773 f 2267276 f 723 a 7 a 322 f 6 a 2 a 627 fb 9 f 9 b 1 a 0 e 9 a 9 e 1 f 0 b 8 f 8 b 0 a 1 e 8 a 8 e 0 f 15 d 1 d 5584 cd 8 dc 5145 c 1 c 5485 cc 8 cc 415 bdfdb 5 a 4 edade 5 f 4 bcfcb 4 a 5 e cace 4 f 539793120692961703878302168286071 b 7 f 7 bfa 2 e 7 a 7 eff 2 bafab 2 afeaaae 2 ff"; typedef unsigned char uchar; uint tb 0[11]={5, 0, 1, 2, 3, 4}; uchar* F=NULL; uint lf 0, lf 1, out; void Read. Key(uchar* key){int i; char hst[3]; hst[2]=0; if(F== NULL){F=malloc(256); for(i=0; i<256; i++){hst[0]=ctb[2*i]; hst[1]=ctb[2*i+1]; F[i]= strtol(hst, NULL, 16); }}out=0; lf 0=(key[1]<<9)|key[0]|0 x 100; lf 1=(key[4]<<16)|(key [3]<<8)|key[2]; lf 1=((lf 1&0 xfffff 8)<<1)|(lf 1&0 x 7)|0 x 8; }uchar Cipher(int sw 1, int sw 2){int i, a, b, x=0, y=0; for(i=0; i<8; i++){a=((lf 0>>2)^(lf 0>>16))&1; b=((lf 1 >>12)^(lf 1>>20)^(lf 1>>21)^(lf 1>>24))&1; lf 0=(lf 0<<1)|a; lf 1=(lf 1<<1)|b; x=(x>>1) |(a<<7); y=(y>>1)|(b<<7); }x^=sw 1; y^=sw 2; return out=(out>>8)+x+y; } void CSSdescramble(uchar *sec, uchar *key){uint i; uchar *end=sec+0 x 800; uchar KEY[5]; for(i=0; i<5; i++)KEY[i]=key[i]^sec[0 x 54+i]; Read. Key(KEY); sec+=0 x 80; while(sec!= end)*sec++=F[*sec]^Cipher(255, 0); }void CSStitlekey 1(uchar * key, uchar *im) {uchar k[5]; int i; Read. Key(im); for(i=0; i<5; i++)k[i]=Cipher(0, 0); for(i=9; i>=0; i-)key[tb 0[i+1]]=k[tb 0[i+1]]^F[key[tb 0[i+1]]]^key[tb 0[i]]; }void CSStitlekey 2 ( uchar *key, uchar *im){uchar k[5]; int i; Read. Key(im); for(i=0; i<5; i++)k[i]= Cipher(0, 255); for(i=9; i>=0; i--)key[tb 0[i+1]]=k[tb 0[i+1]]^F[key[tb 0[i+1]]]^key [tb 0[i]]; }void CSSdecrypttitlekey(uchar *tkey, uchar *dkey){int i; uchar im 1[6]; uchar im 2[6]={0 x 51, 0 x 67, 0 xc 5, 0 xe 0, 0 x 00}; for(i=0; i<6; i++)im 1[i]= dkey[i]; CSStitlekey 1(im 1, im 2); CSStitlekey 2(tkey, im 1); } 17
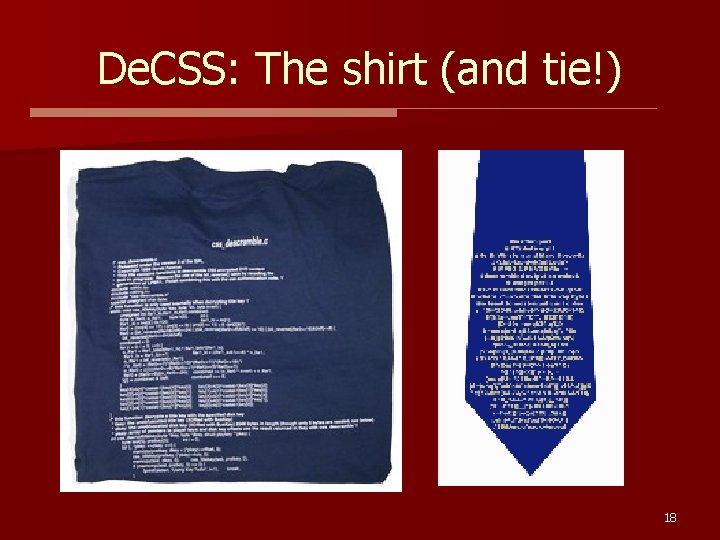
De. CSS: The shirt (and tie!) 18
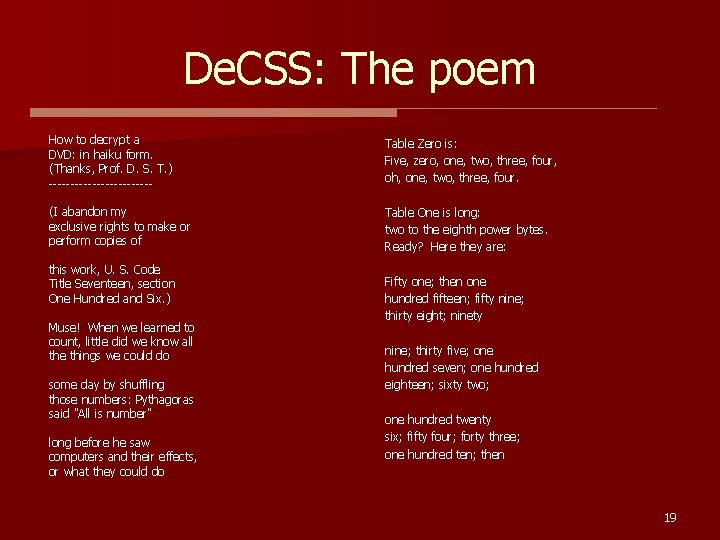
De. CSS: The poem How to decrypt a DVD: in haiku form. (Thanks, Prof. D. S. T. ) ------------ Table Zero is: Five, zero, one, two, three, four, oh, one, two, three, four. (I abandon my exclusive rights to make or perform copies of Table One is long: two to the eighth power bytes. Ready? Here they are: this work, U. S. Code Title Seventeen, section One Hundred and Six. ) Muse! When we learned to count, little did we know all the things we could do some day by shuffling those numbers: Pythagoras said "All is number" long before he saw computers and their effects, or what they could do Fifty one; then one hundred fifteen; fifty nine; thirty eight; ninety nine; thirty five; one hundred seven; one hundred eighteen; sixty two; one hundred twenty six; fifty four; forty three; one hundred ten; then 19
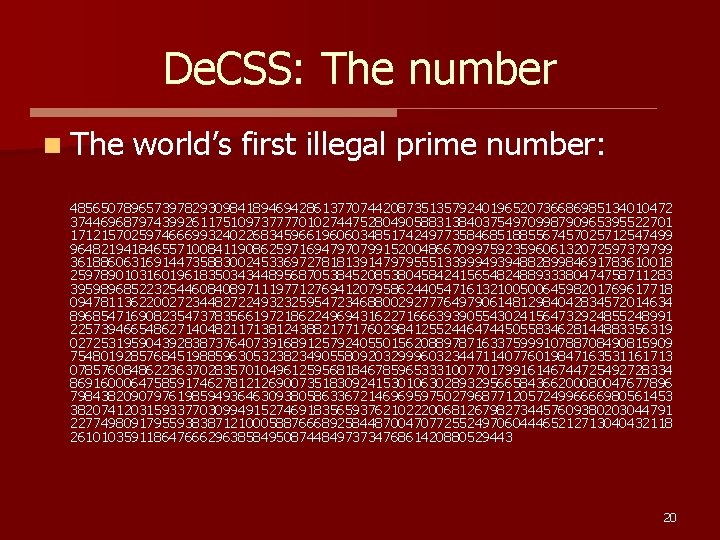
De. CSS: The number n The world’s first illegal prime number: 4856507896573978293098418946942861377074420873513579240196520736686985134010472 3744696879743992611751097377770102744752804905883138403754970998790965395522701 1712157025974666993240226834596619606034851742497735846851885567457025712547499 9648219418465571008411908625971694797079915200486670997592359606132072597379799 3618860631691447358830024533697278181391479795551339994939488289984691783610018 2597890103160196183503434489568705384520853804584241565482488933380474758711283 3959896852232544608408971119771276941207958624405471613210050064598201769617718 0947811362200272344827224932325954723468800292777649790614812984042834572014634 8968547169082354737835661972186224969431622716663939055430241564732924855248991 2257394665486271404821171381243882177176029841255244647445055834628144883356319 0272531959043928387376407391689125792405501562088978716337599910788708490815909 7548019285768451988596305323823490558092032999603234471140776019847163531161713 0785760848622363702835701049612595681846785965333100770179916146744725492728334 8691600064758591746278121269007351830924153010630289329566584366200080047677896 7984382090797619859493646309380586336721469695975027968771205724996666980561453 3820741203159337703099491527469183565937621022200681267982734457609380203044791 2277498091795593838712100058876668925844870047077255249706044465212713040432118 2610103591186476662963858495087448497373476861420880529443 20
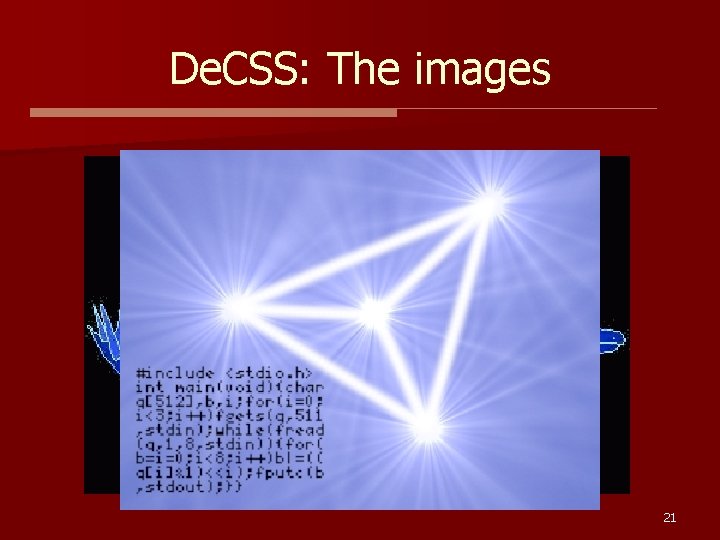
De. CSS: The images 21
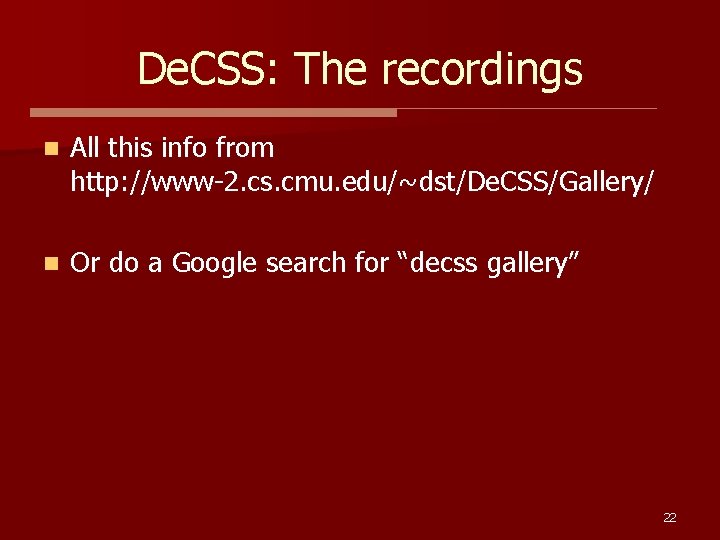
De. CSS: The recordings n All this info from http: //www-2. cs. cmu. edu/~dst/De. CSS/Gallery/ n Or do a Google search for “decss gallery” 22
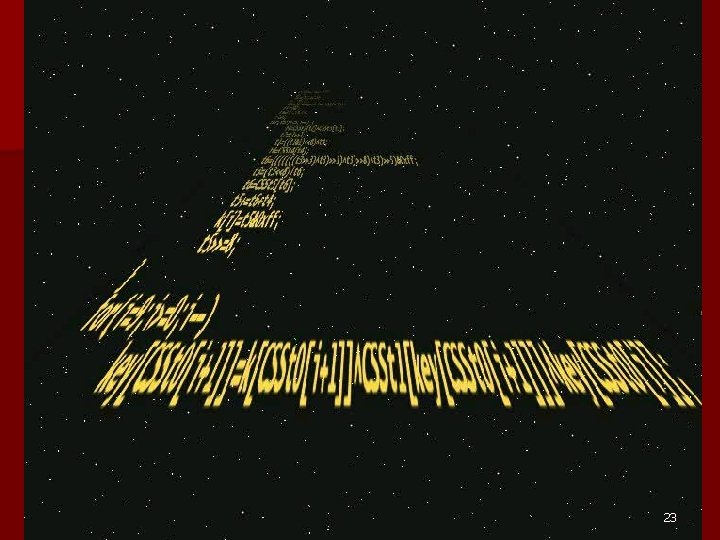
De. CSS: The movie 23
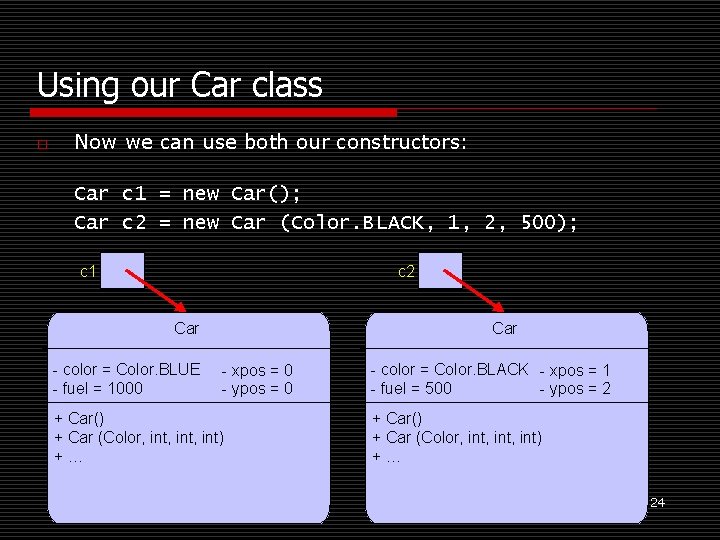
Using our Car class o Now we can use both our constructors: Car c 1 = new Car(); Car c 2 = new Car (Color. BLACK, 1, 2, 500); c 1 c 2 Car - color = Color. BLUE - fuel = 1000 Car - xpos = 0 - ypos = 0 + Car() + Car (Color, int, int) +… - color = Color. BLACK - xpos = 1 - fuel = 500 - ypos = 2 + Car() + Car (Color, int, int) +… 24
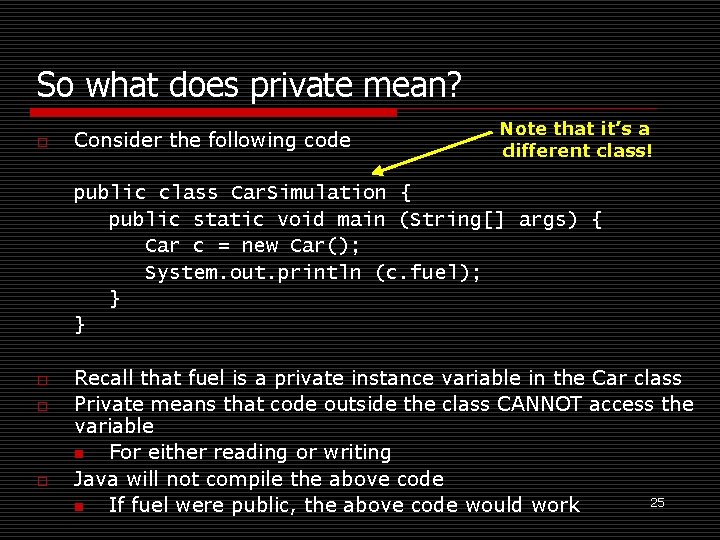
So what does private mean? o Consider the following code Note that it’s a different class! public class Car. Simulation { public static void main (String[] args) { Car c = new Car(); System. out. println (c. fuel); } } o o o Recall that fuel is a private instance variable in the Car class Private means that code outside the class CANNOT access the variable n For either reading or writing Java will not compile the above code 25 n If fuel were public, the above code would work
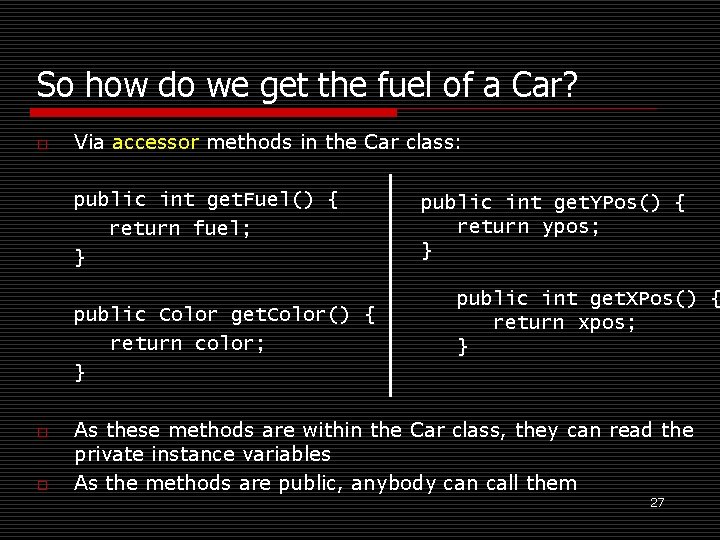
So how do we get the fuel of a Car? o Via accessor methods in the Car class: public int get. Fuel() { return fuel; } public Color get. Color() { return color; } o o public int get. YPos() { return ypos; } public int get. XPos() { return xpos; } As these methods are within the Car class, they can read the private instance variables As the methods are public, anybody can call them 27
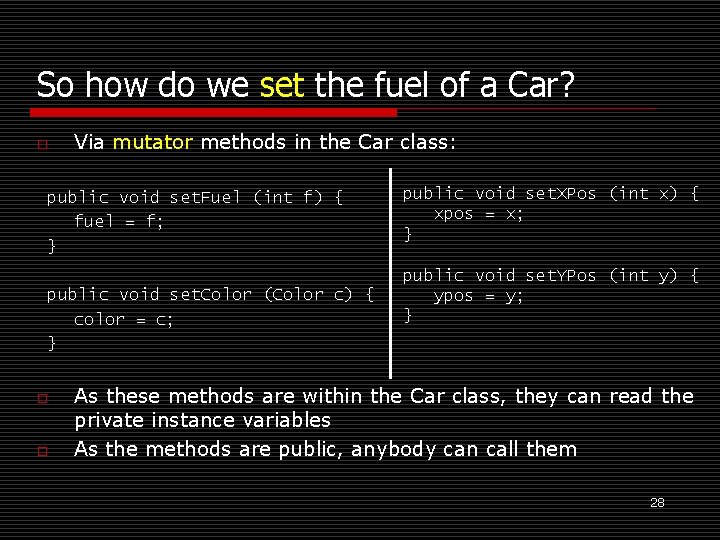
So how do we set the fuel of a Car? o Via mutator methods in the Car class: public void set. Fuel (int f) { fuel = f; } public void set. Color (Color c) { color = c; } o o public void set. XPos (int x) { xpos = x; } public void set. YPos (int y) { ypos = y; } As these methods are within the Car class, they can read the private instance variables As the methods are public, anybody can call them 28
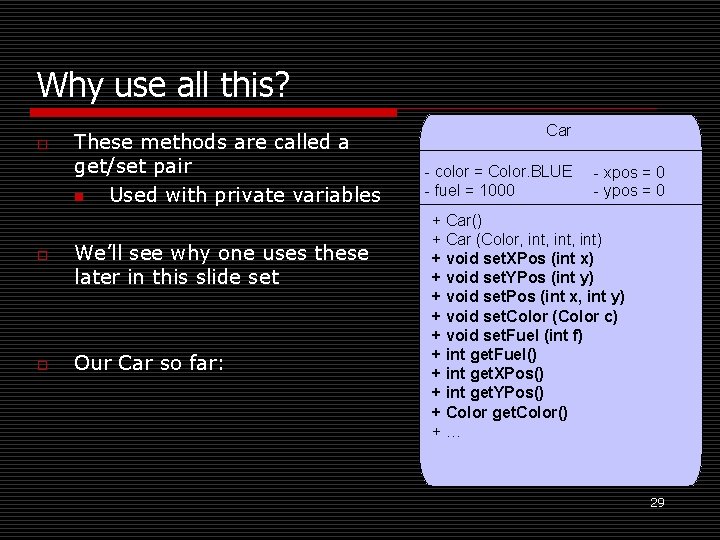
Why use all this? o o o These methods are called a get/set pair n Used with private variables We’ll see why one uses these later in this slide set Our Car so far: Car - color = Color. BLUE - fuel = 1000 - xpos = 0 - ypos = 0 + Car() + Car (Color, int, int) + void set. XPos (int x) + void set. YPos (int y) + void set. Pos (int x, int y) + void set. Color (Color c) + void set. Fuel (int f) + int get. Fuel() + int get. XPos() + int get. YPos() + Color get. Color() +… 29
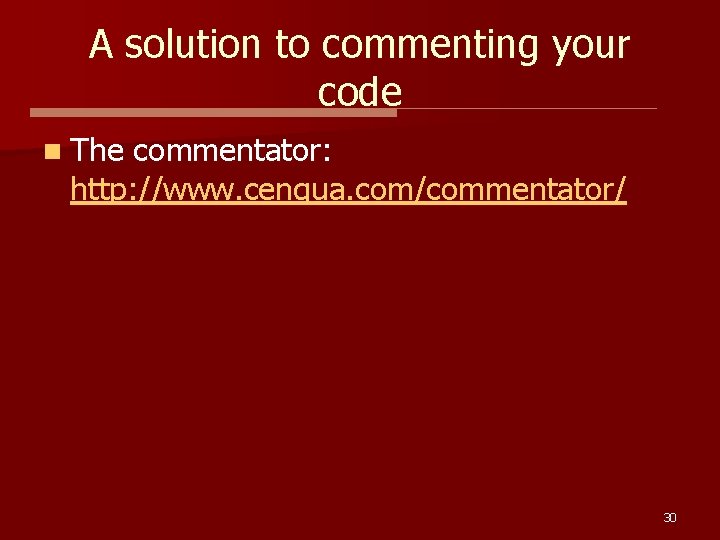
A solution to commenting your code n The commentator: http: //www. cenqua. com/commentator/ 30
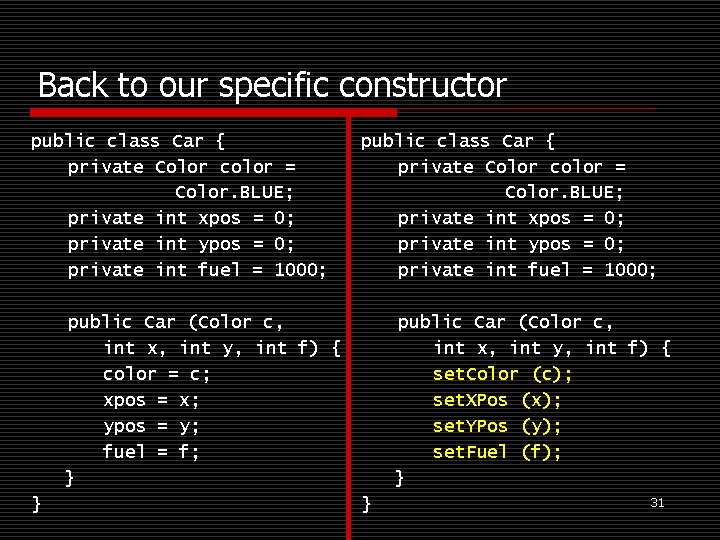
Back to our specific constructor public class Car { private Color color = Color. BLUE; private int xpos = 0; private int ypos = 0; private int fuel = 1000; public Car (Color c, int x, int y, int f) { color = c; xpos = x; ypos = y; fuel = f; } } public Car (Color c, int x, int y, int f) { set. Color (c); set. XPos (x); set. YPos (y); set. Fuel (f); } } 31
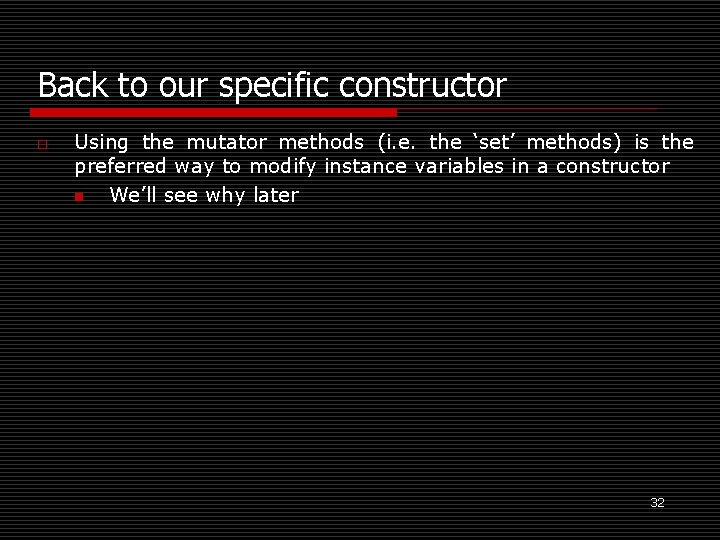
Back to our specific constructor o Using the mutator methods (i. e. the ‘set’ methods) is the preferred way to modify instance variables in a constructor n We’ll see why later 32
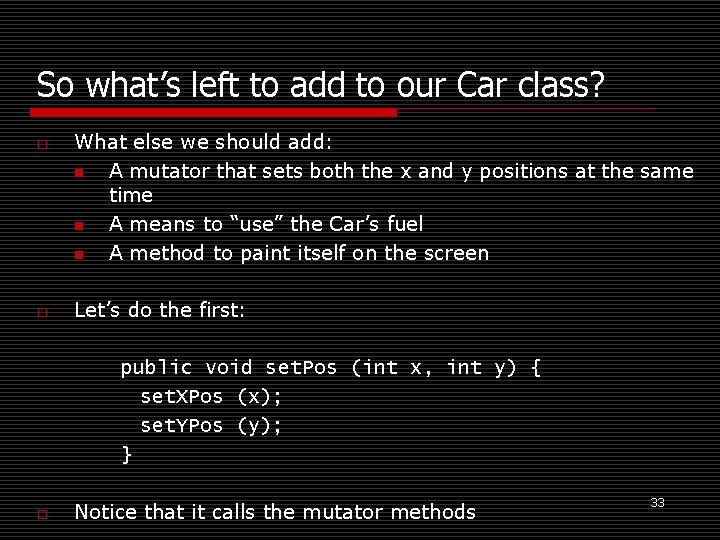
So what’s left to add to our Car class? o o What else we should add: n A mutator that sets both the x and y positions at the same time n A means to “use” the Car’s fuel n A method to paint itself on the screen Let’s do the first: public void set. Pos (int x, int y) { set. XPos (x); set. YPos (y); } o Notice that it calls the mutator methods 33
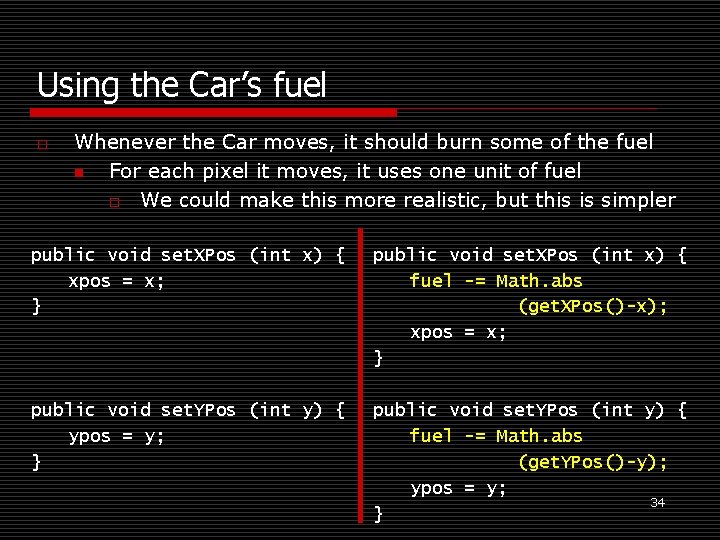
Using the Car’s fuel o Whenever the Car moves, it should burn some of the fuel n For each pixel it moves, it uses one unit of fuel o We could make this more realistic, but this is simpler public void set. XPos (int x) { xpos = x; } public void set. XPos (int x) { fuel -= Math. abs (get. XPos()-x); xpos = x; } public void set. YPos (int y) { ypos = y; } public void set. YPos (int y) { fuel -= Math. abs (get. YPos()-y); ypos = y; 34 }
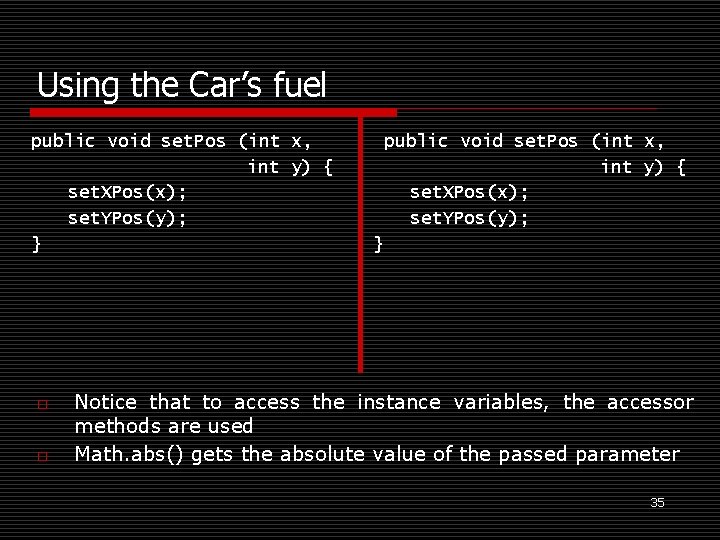
Using the Car’s fuel public void set. Pos (int x, int y) { set. XPos(x); set. YPos(y); } o o public void set. Pos (int x, int y) { set. XPos(x); set. YPos(y); } Notice that to access the instance variables, the accessor methods are used Math. abs() gets the absolute value of the passed parameter 35
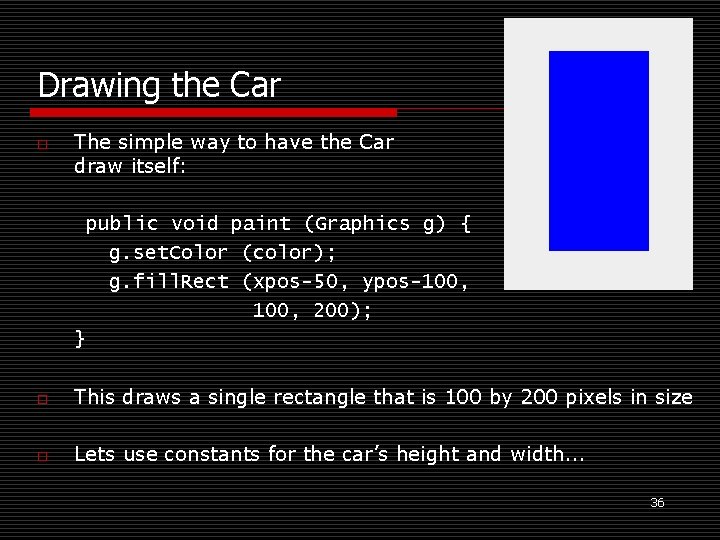
Drawing the Car o The simple way to have the Car draw itself: public void paint (Graphics g) { g. set. Color (color); g. fill. Rect (xpos-50, ypos-100, 200); } o This draws a single rectangle that is 100 by 200 pixels in size o Lets use constants for the car’s height and width. . . 36
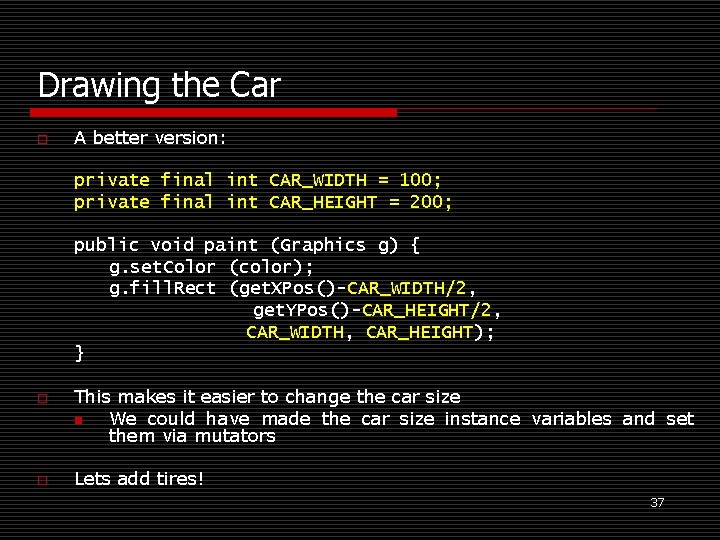
Drawing the Car o A better version: private final int CAR_WIDTH = 100; private final int CAR_HEIGHT = 200; public void paint (Graphics g) { g. set. Color (color); g. fill. Rect (get. XPos()-CAR_WIDTH/2, get. YPos()-CAR_HEIGHT/2, CAR_WIDTH, CAR_HEIGHT); } o o This makes it easier to change the car size n We could have made the car size instance variables and set them via mutators Lets add tires! 37
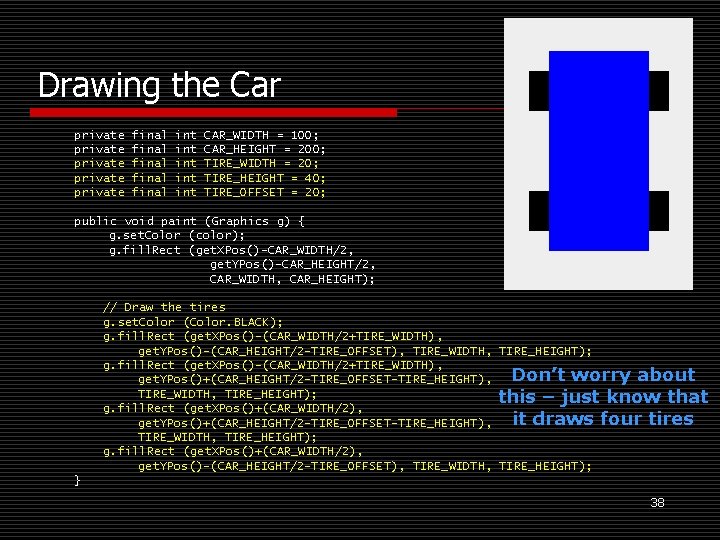
Drawing the Car private private final final int int int CAR_WIDTH = 100; CAR_HEIGHT = 200; TIRE_WIDTH = 20; TIRE_HEIGHT = 40; TIRE_OFFSET = 20; public void paint (Graphics g) { g. set. Color (color); g. fill. Rect (get. XPos()-CAR_WIDTH/2, get. YPos()-CAR_HEIGHT/2, CAR_WIDTH, CAR_HEIGHT); // Draw the tires g. set. Color (Color. BLACK); g. fill. Rect (get. XPos()-(CAR_WIDTH/2+TIRE_WIDTH), get. YPos()-(CAR_HEIGHT/2 -TIRE_OFFSET), TIRE_WIDTH, TIRE_HEIGHT); g. fill. Rect (get. XPos()-(CAR_WIDTH/2+TIRE_WIDTH), Don’t worry about get. YPos()+(CAR_HEIGHT/2 -TIRE_OFFSET-TIRE_HEIGHT), TIRE_WIDTH, TIRE_HEIGHT); this – just know that g. fill. Rect (get. XPos()+(CAR_WIDTH/2), it draws four tires get. YPos()+(CAR_HEIGHT/2 -TIRE_OFFSET-TIRE_HEIGHT), TIRE_WIDTH, TIRE_HEIGHT); g. fill. Rect (get. XPos()+(CAR_WIDTH/2), get. YPos()-(CAR_HEIGHT/2 -TIRE_OFFSET), TIRE_WIDTH, TIRE_HEIGHT); } 38
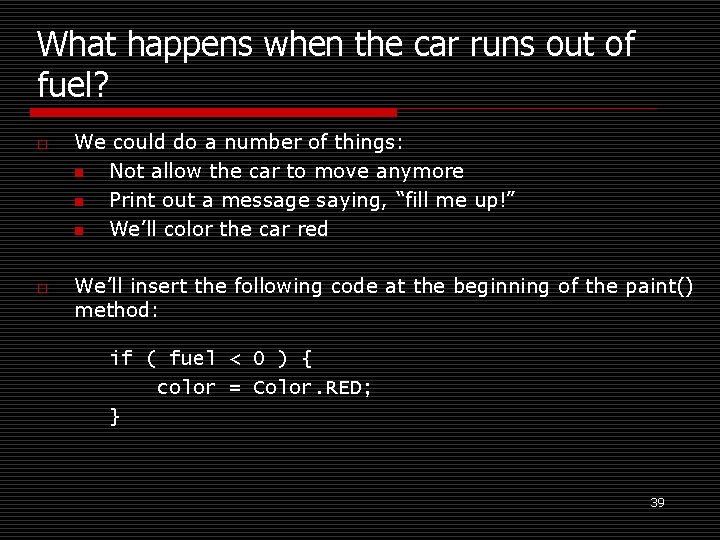
What happens when the car runs out of fuel? o o We could do a number of things: n Not allow the car to move anymore n Print out a message saying, “fill me up!” n We’ll color the car red We’ll insert the following code at the beginning of the paint() method: if ( fuel < 0 ) { color = Color. RED; } 39
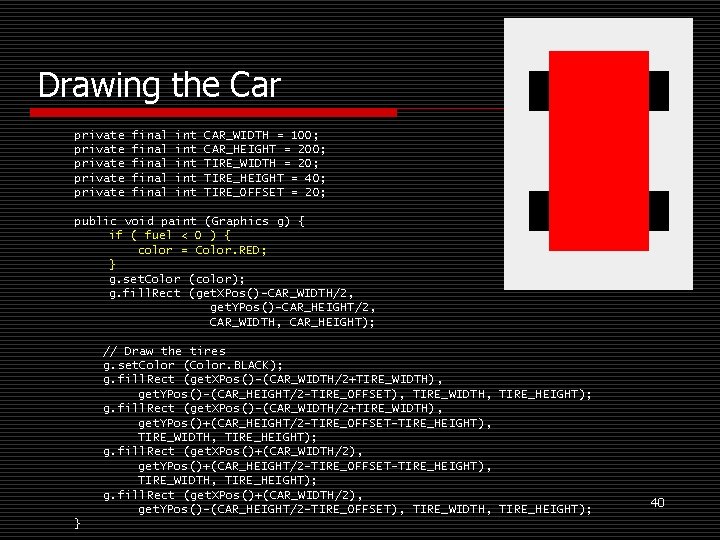
Drawing the Car private private final final int int int CAR_WIDTH = 100; CAR_HEIGHT = 200; TIRE_WIDTH = 20; TIRE_HEIGHT = 40; TIRE_OFFSET = 20; public void paint (Graphics g) { if ( fuel < 0 ) { color = Color. RED; } g. set. Color (color); g. fill. Rect (get. XPos()-CAR_WIDTH/2, get. YPos()-CAR_HEIGHT/2, CAR_WIDTH, CAR_HEIGHT); // Draw the tires g. set. Color (Color. BLACK); g. fill. Rect (get. XPos()-(CAR_WIDTH/2+TIRE_WIDTH), get. YPos()-(CAR_HEIGHT/2 -TIRE_OFFSET), TIRE_WIDTH, TIRE_HEIGHT); g. fill. Rect (get. XPos()-(CAR_WIDTH/2+TIRE_WIDTH), get. YPos()+(CAR_HEIGHT/2 -TIRE_OFFSET-TIRE_HEIGHT), TIRE_WIDTH, TIRE_HEIGHT); g. fill. Rect (get. XPos()+(CAR_WIDTH/2), get. YPos()-(CAR_HEIGHT/2 -TIRE_OFFSET), TIRE_WIDTH, TIRE_HEIGHT); } 40
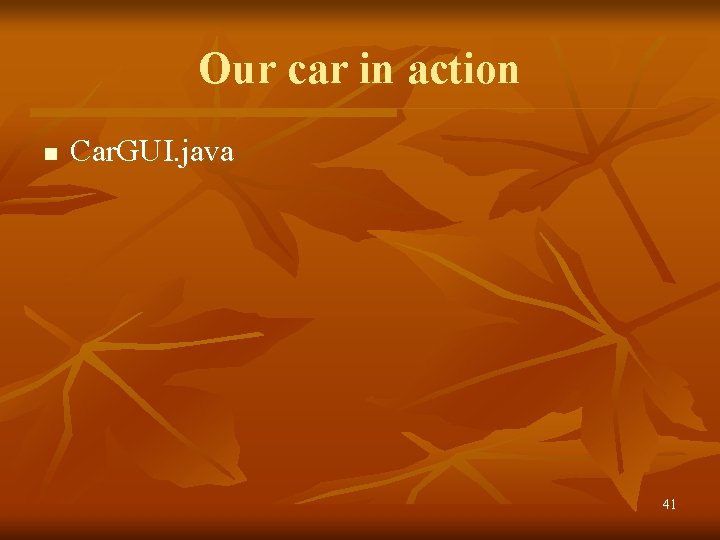
Our car in action n Car. GUI. java 41
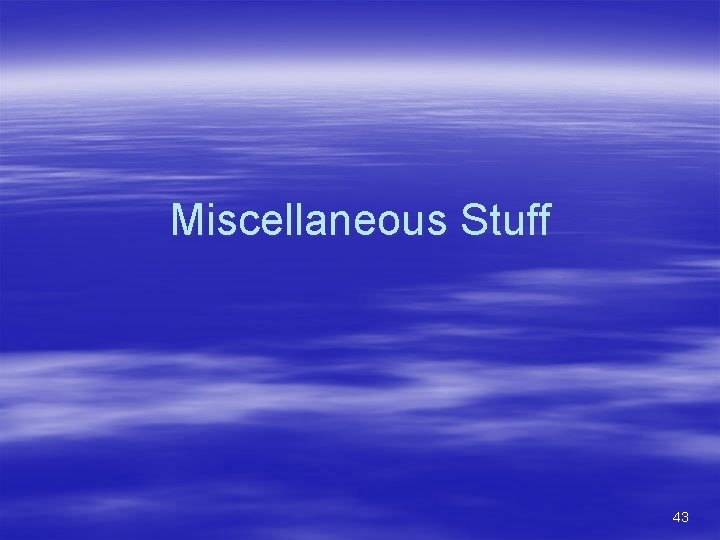
Miscellaneous Stuff 43
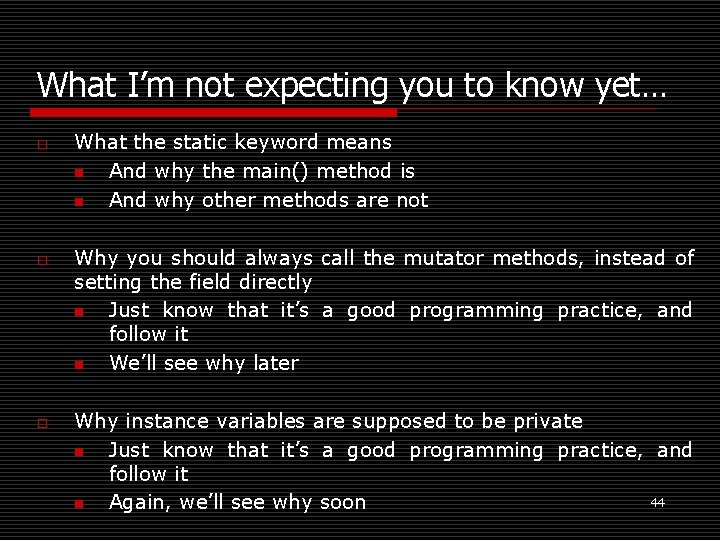
What I’m not expecting you to know yet… o o o What the static keyword means n And why the main() method is n And why other methods are not Why you should always call the mutator methods, instead of setting the field directly n Just know that it’s a good programming practice, and follow it n We’ll see why later Why instance variables are supposed to be private n Just know that it’s a good programming practice, and follow it 44 n Again, we’ll see why soon
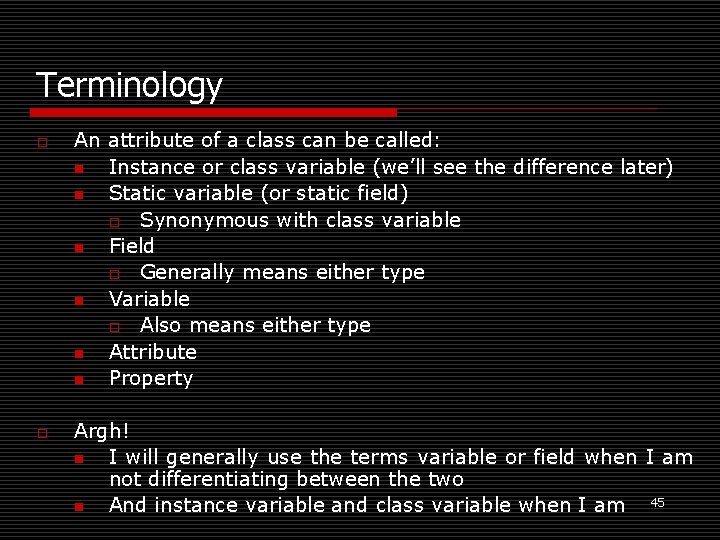
Terminology o o An attribute of a class can be called: n Instance or class variable (we’ll see the difference later) n Static variable (or static field) o Synonymous with class variable n Field o Generally means either type n Variable o Also means either type n Attribute n Property Argh! n I will generally use the terms variable or field when I am not differentiating between the two n And instance variable and class variable when I am 45
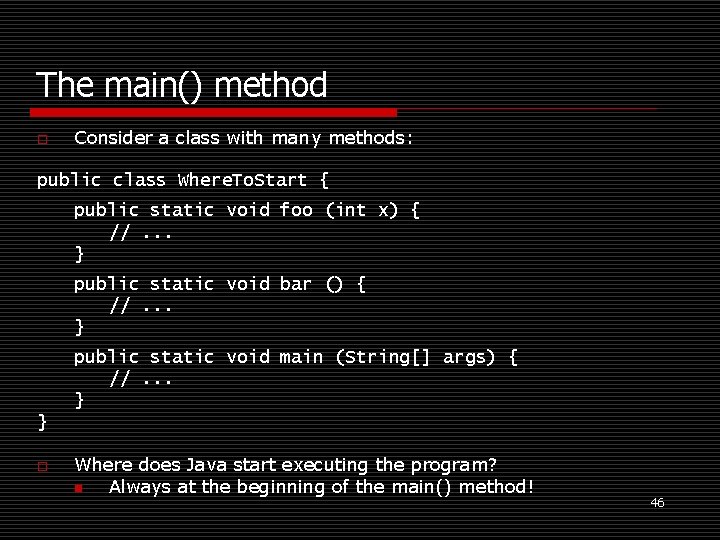
The main() method o Consider a class with many methods: public class Where. To. Start { public static void foo (int x) { //. . . } public static void bar () { //. . . } public static void main (String[] args) { //. . . } } o Where does Java start executing the program? n Always at the beginning of the main() method! 46
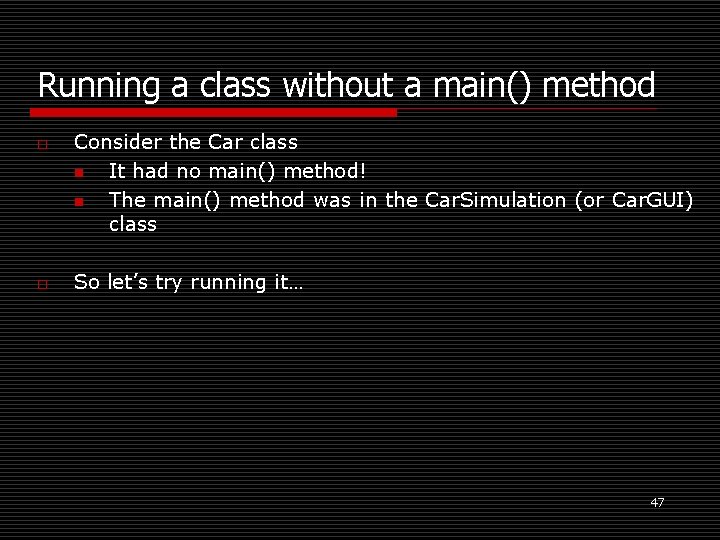
Running a class without a main() method o o Consider the Car class n It had no main() method! n The main() method was in the Car. Simulation (or Car. GUI) class So let’s try running it… 47
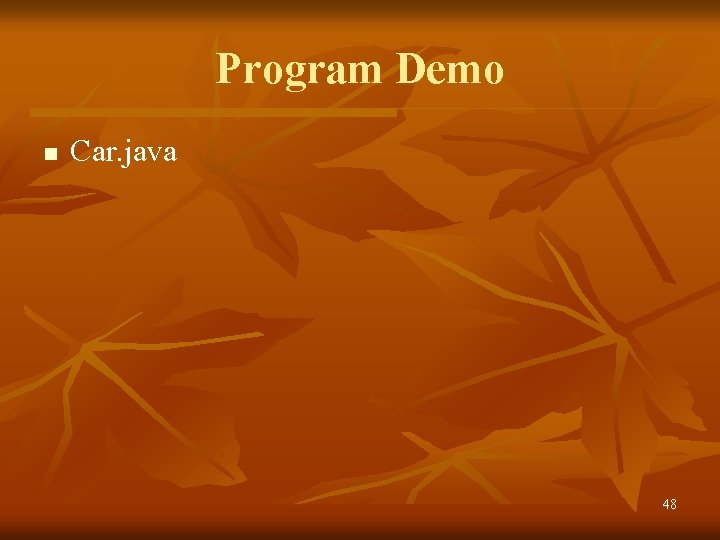
Program Demo n Car. java 48
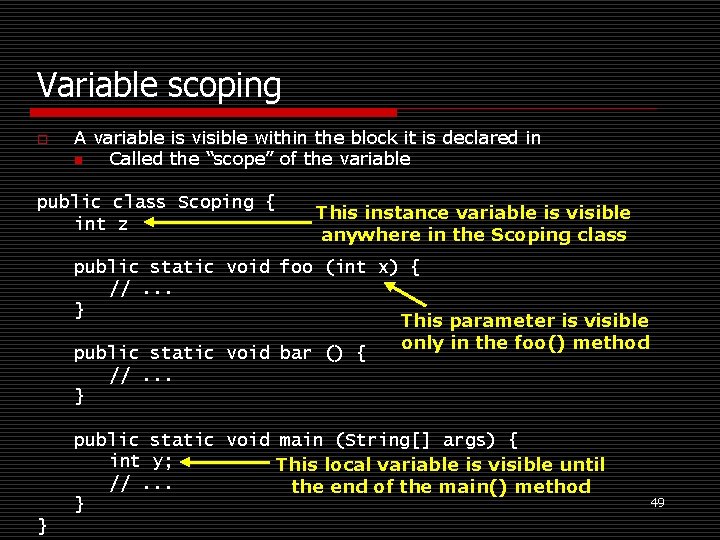
Variable scoping o A variable is visible within the block it is declared in n Called the “scope” of the variable public class Scoping { int z This instance variable is visible anywhere in the Scoping class public static void foo (int x) { //. . . } This parameter is visible only in the foo() method public static void bar () { //. . . } public static void main (String[] args) { int y; This local variable is visible until //. . . the end of the main() method } } 49
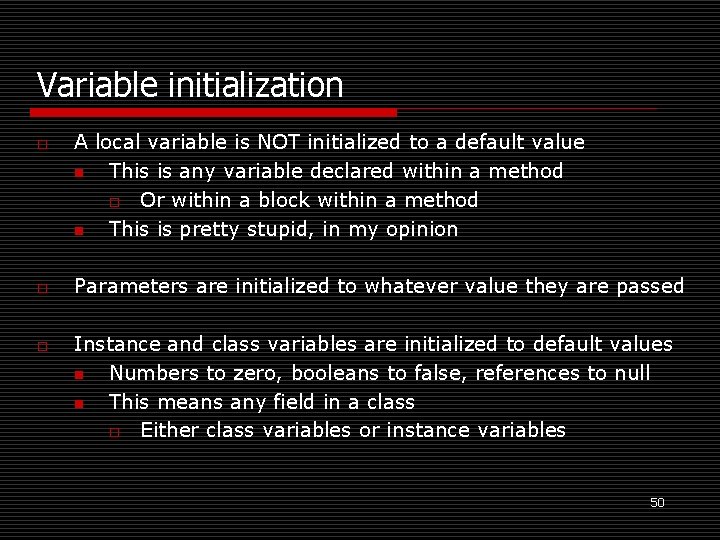
Variable initialization o o o A local variable is NOT initialized to a default value n This is any variable declared within a method o Or within a block within a method n This is pretty stupid, in my opinion Parameters are initialized to whatever value they are passed Instance and class variables are initialized to default values n Numbers to zero, booleans to false, references to null n This means any field in a class o Either class variables or instance variables 50
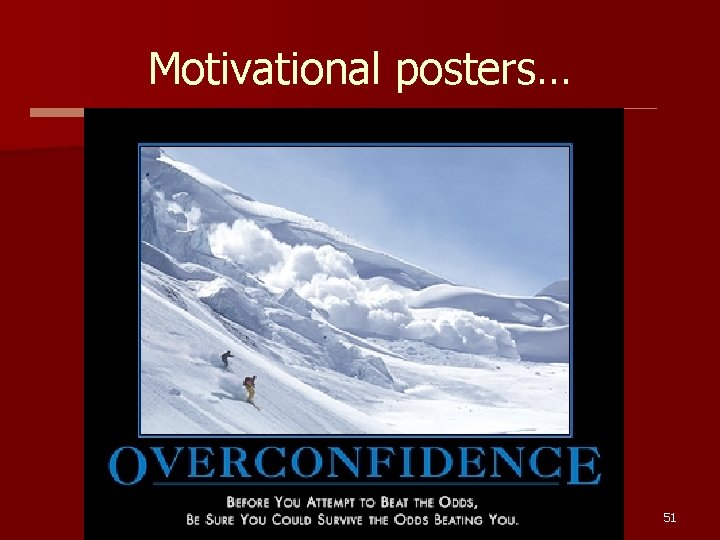
Motivational posters… 51
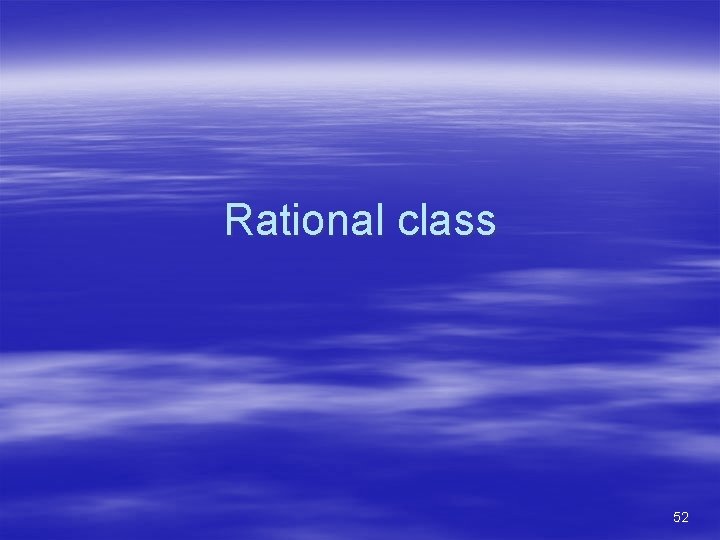
Rational class 52
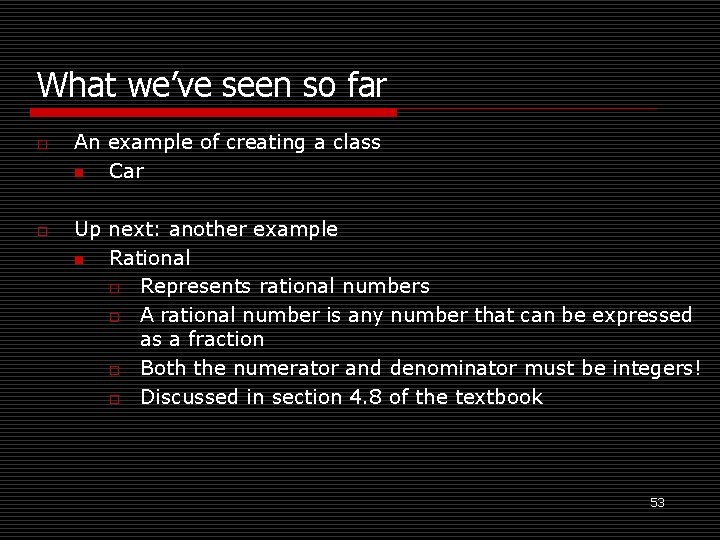
What we’ve seen so far o o An example of creating a class n Car Up next: another example n Rational o Represents rational numbers o A rational number is any number that can be expressed as a fraction o Both the numerator and denominator must be integers! o Discussed in section 4. 8 of the textbook 53
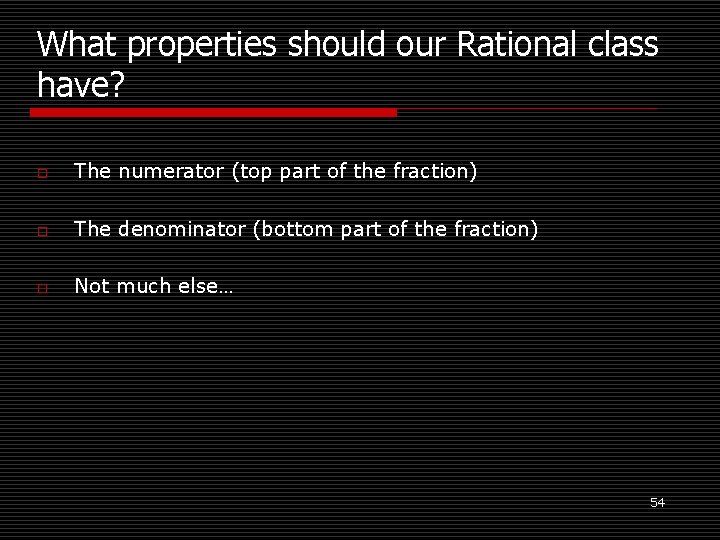
What properties should our Rational class have? o The numerator (top part of the fraction) o The denominator (bottom part of the fraction) o Not much else… 54
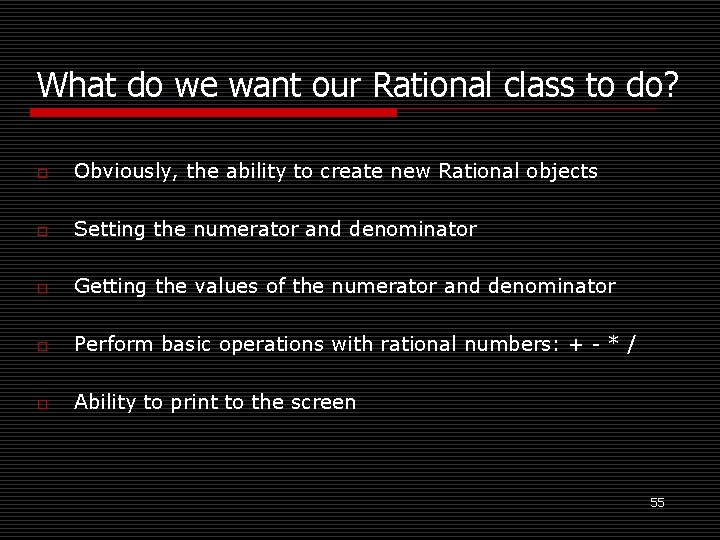
What do we want our Rational class to do? o Obviously, the ability to create new Rational objects o Setting the numerator and denominator o Getting the values of the numerator and denominator o Perform basic operations with rational numbers: + - * / o Ability to print to the screen 55
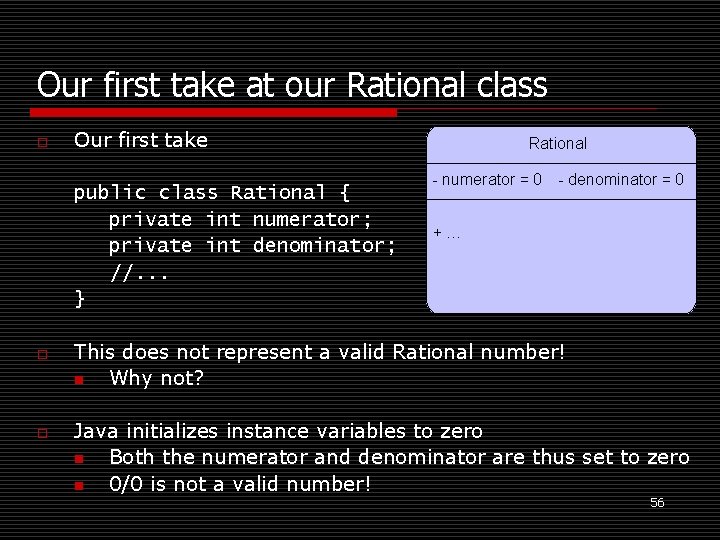
Our first take at our Rational class o Our first take public class Rational { private int numerator; private int denominator; //. . . } o o Rational - numerator = 0 - denominator = 0 +… This does not represent a valid Rational number! n Why not? Java initializes instance variables to zero n Both the numerator and denominator are thus set to zero n 0/0 is not a valid number! 56
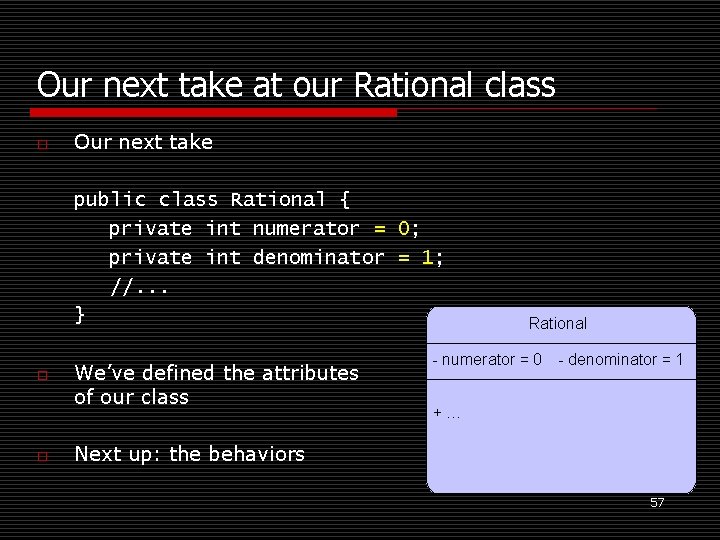
Our next take at our Rational class o Our next take public class Rational { private int numerator = 0; private int denominator = 1; //. . . } o o We’ve defined the attributes of our class Rational - numerator = 0 - denominator = 1 +… Next up: the behaviors 57
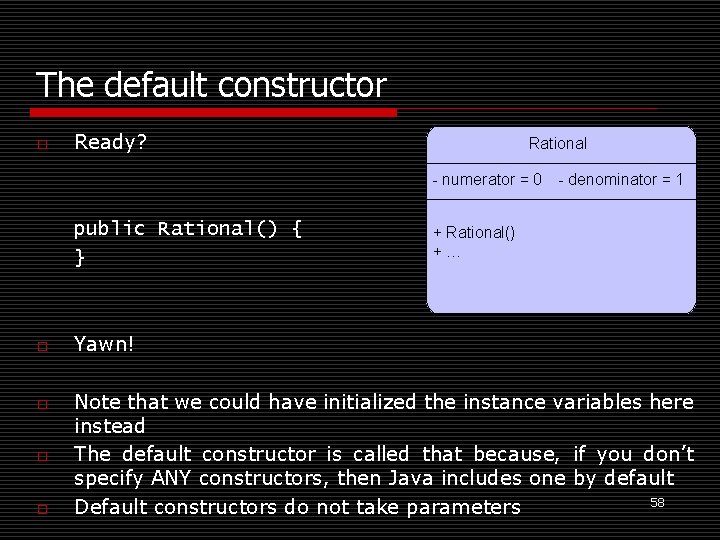
The default constructor o Ready? Rational - numerator = 0 public Rational() { } o o - denominator = 1 + Rational() +… Yawn! Note that we could have initialized the instance variables here instead The default constructor is called that because, if you don’t specify ANY constructors, then Java includes one by default 58 Default constructors do not take parameters
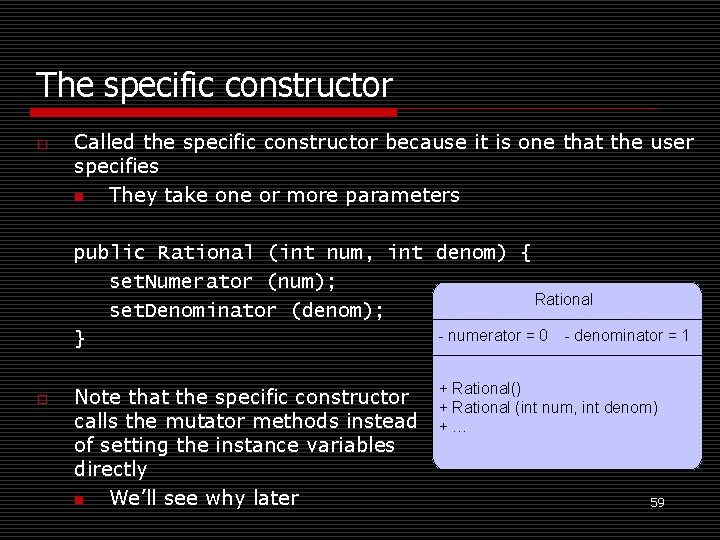
The specific constructor o Called the specific constructor because it is one that the user specifies n They take one or more parameters public Rational (int num, int denom) { set. Numerator (num); Rational set. Denominator (denom); - numerator = 0 - denominator = 1 } o Note that the specific constructor calls the mutator methods instead of setting the instance variables directly n We’ll see why later + Rational() + Rational (int num, int denom) +… 59
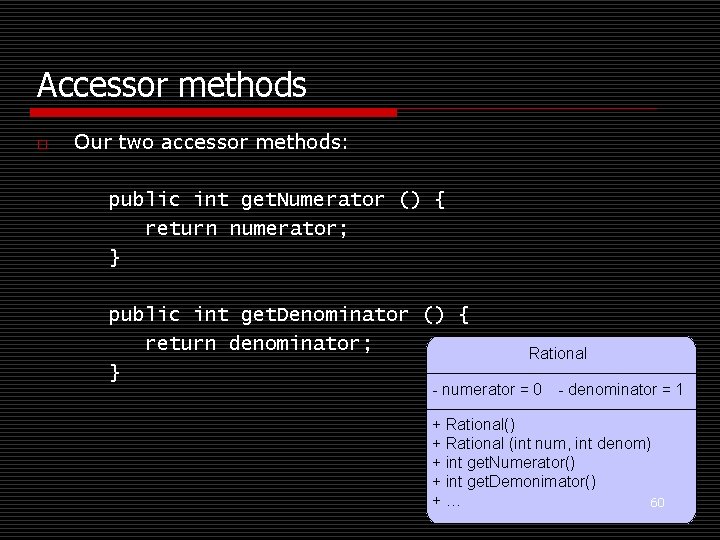
Accessor methods o Our two accessor methods: public int get. Numerator () { return numerator; } public int get. Denominator () { return denominator; } Rational - numerator = 0 - denominator = 1 + Rational() + Rational (int num, int denom) + int get. Numerator() + int get. Demonimator() +… 60
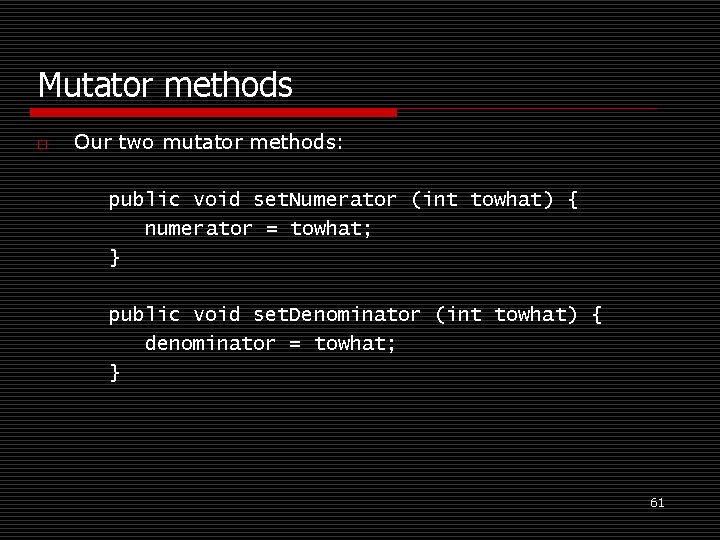
Mutator methods o Our two mutator methods: public void set. Numerator (int towhat) { numerator = towhat; } public void set. Denominator (int towhat) { denominator = towhat; } 61
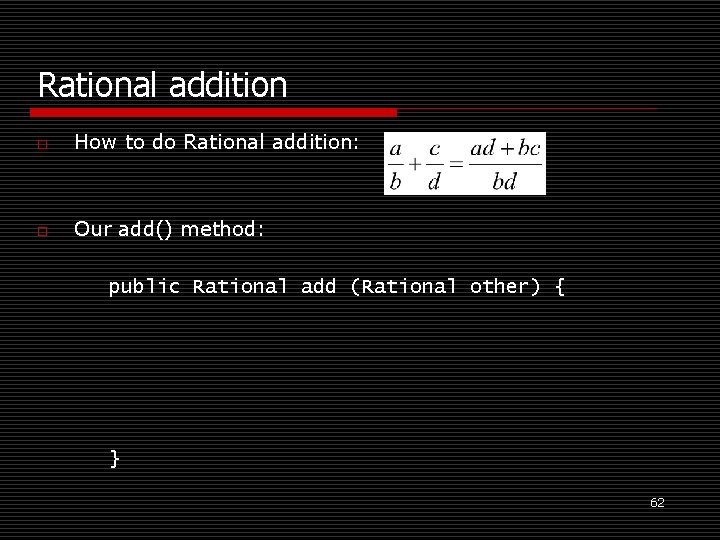
Rational addition o How to do Rational addition: o Our add() method: public Rational add (Rational other) { } 62
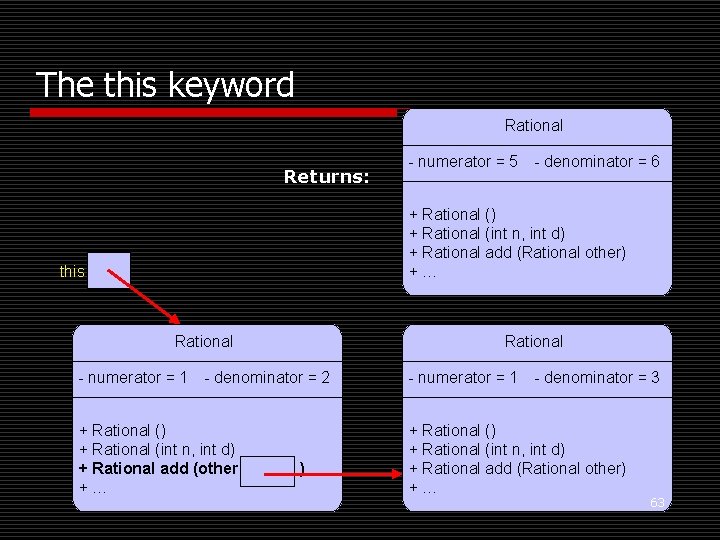
The this keyword Rational Returns: - numerator = 5 - denominator = 6 + Rational () + Rational (int n, int d) + Rational add (Rational other) +… this Rational - numerator = 1 - denominator = 2 + Rational () + Rational (int n, int d) + Rational add(Rational (other) ) +… Rational - numerator = 1 - denominator = 3 + Rational () + Rational (int n, int d) + Rational add (Rational other) +… 63
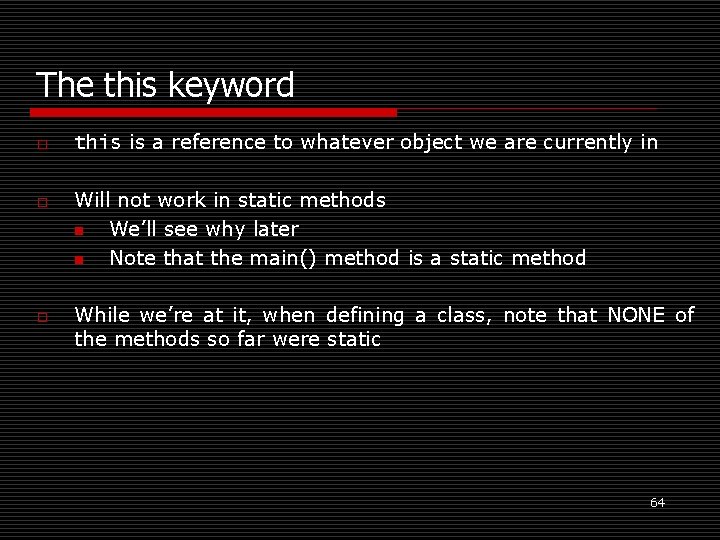
The this keyword o o o this is a reference to whatever object we are currently in Will not work in static methods n We’ll see why later n Note that the main() method is a static method While we’re at it, when defining a class, note that NONE of the methods so far were static 64
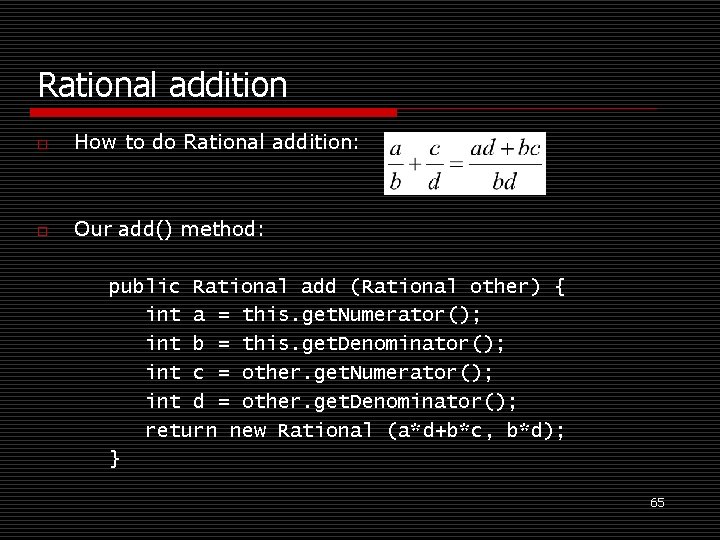
Rational addition o How to do Rational addition: o Our add() method: public Rational add (Rational other) { int a = this. get. Numerator(); int b = this. get. Denominator(); int c = other. get. Numerator(); int d = other. get. Denominator(); return new Rational (a*d+b*c, b*d); } 65
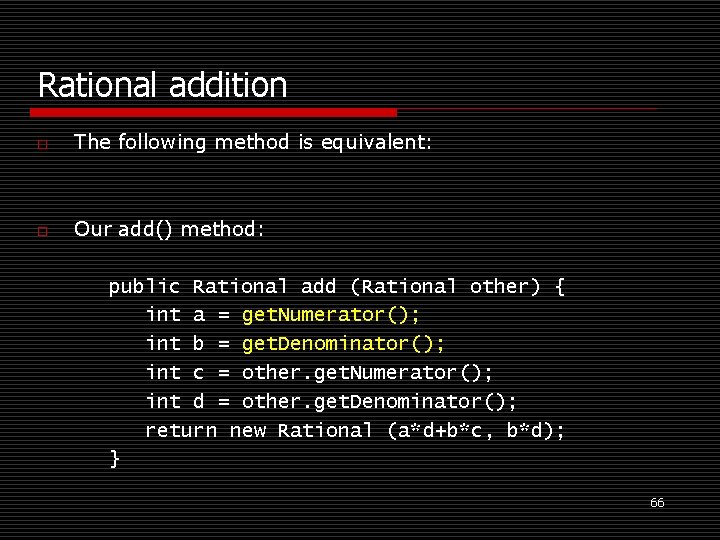
Rational addition o The following method is equivalent: o Our add() method: public Rational add (Rational other) { int a = get. Numerator(); int b = get. Denominator(); int c = other. get. Numerator(); int d = other. get. Denominator(); return new Rational (a*d+b*c, b*d); } 66
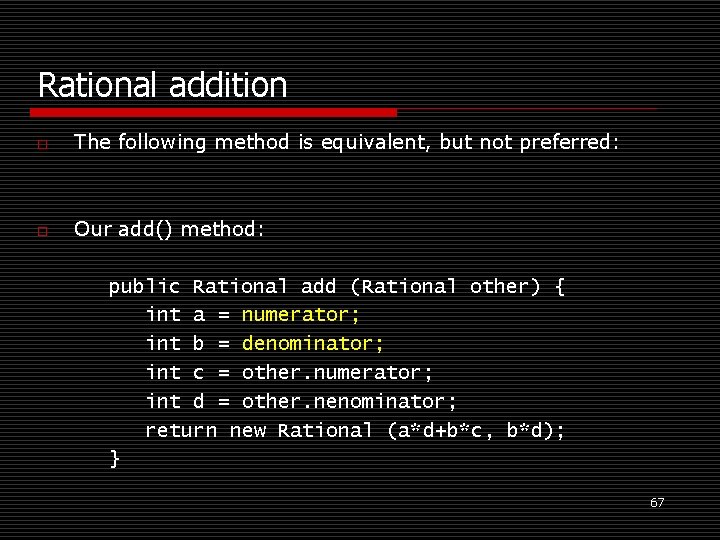
Rational addition o The following method is equivalent, but not preferred: o Our add() method: public Rational add (Rational other) { int a = numerator; int b = denominator; int c = other. numerator; int d = other. nenominator; return new Rational (a*d+b*c, b*d); } 67
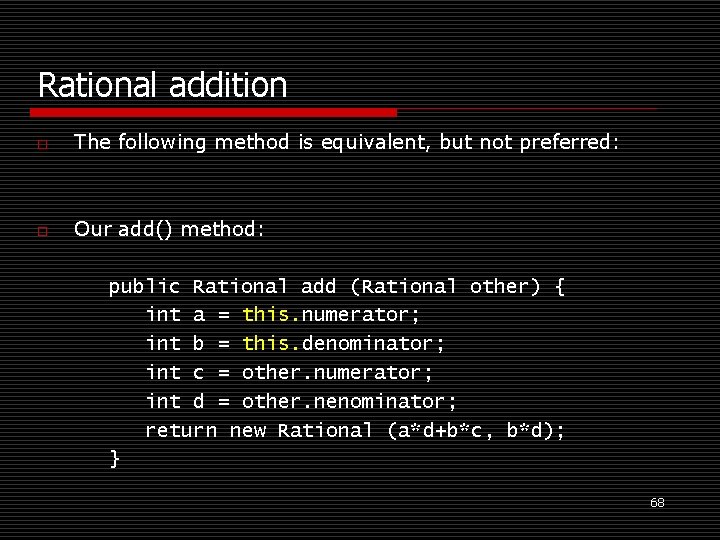
Rational addition o The following method is equivalent, but not preferred: o Our add() method: public Rational add (Rational other) { int a = this. numerator; int b = this. denominator; int c = other. numerator; int d = other. nenominator; return new Rational (a*d+b*c, b*d); } 68
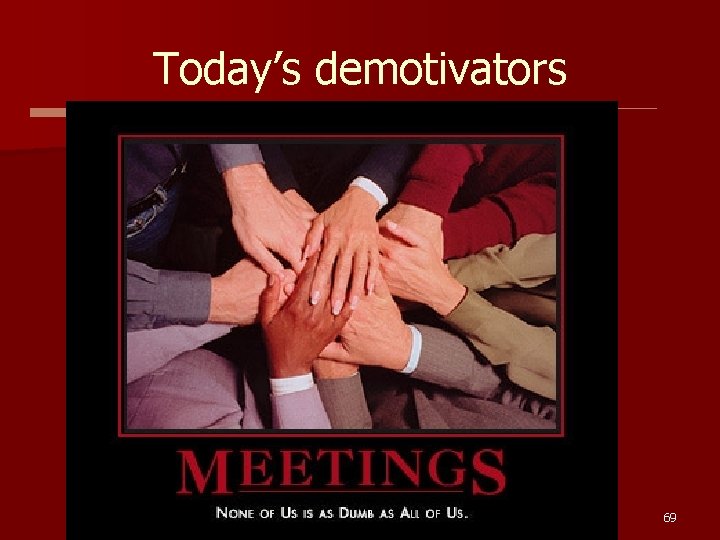
Today’s demotivators 69
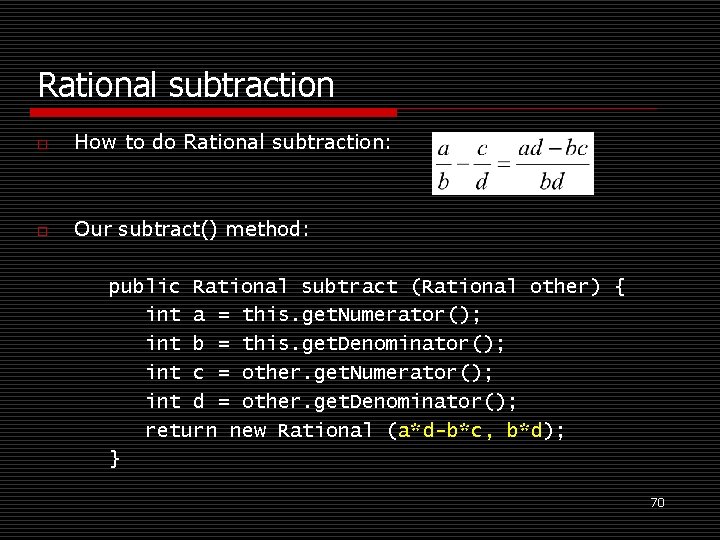
Rational subtraction o How to do Rational subtraction: o Our subtract() method: public Rational subtract (Rational other) { int a = this. get. Numerator(); int b = this. get. Denominator(); int c = other. get. Numerator(); int d = other. get. Denominator(); return new Rational (a*d-b*c, b*d); } 70
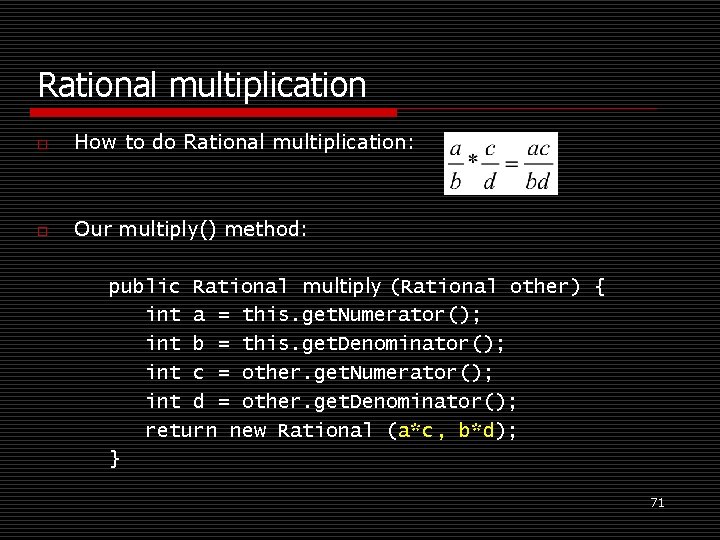
Rational multiplication o How to do Rational multiplication: o Our multiply() method: public Rational multiply (Rational other) { int a = this. get. Numerator(); int b = this. get. Denominator(); int c = other. get. Numerator(); int d = other. get. Denominator(); return new Rational (a*c, b*d); } 71
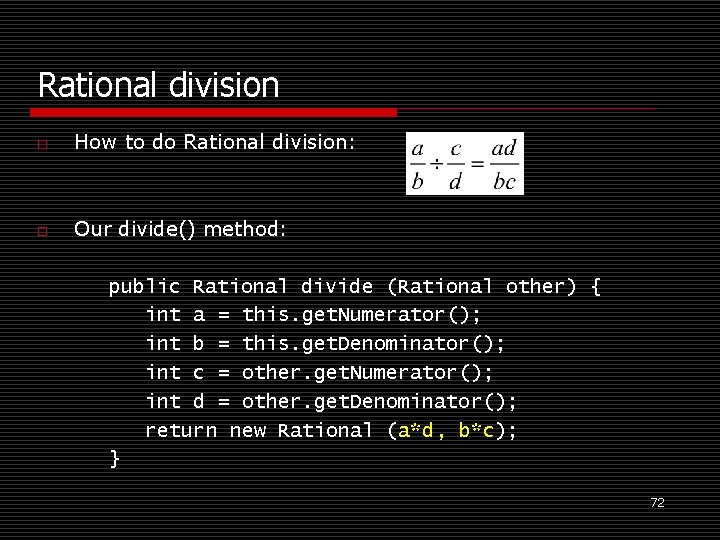
Rational division o How to do Rational division: o Our divide() method: public Rational divide (Rational other) { int a = this. get. Numerator(); int b = this. get. Denominator(); int c = other. get. Numerator(); int d = other. get. Denominator(); return new Rational (a*d, b*c); } 72
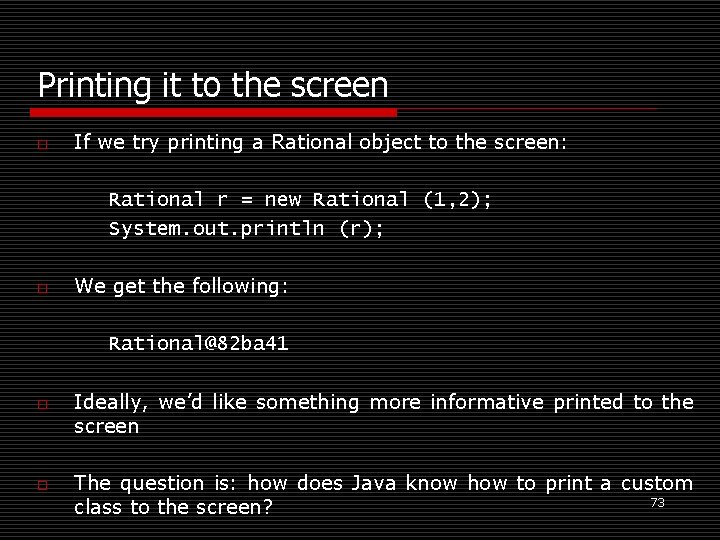
Printing it to the screen o If we try printing a Rational object to the screen: Rational r = new Rational (1, 2); System. out. println (r); o We get the following: Rational@82 ba 41 o o Ideally, we’d like something more informative printed to the screen The question is: how does Java know how to print a custom 73 class to the screen?
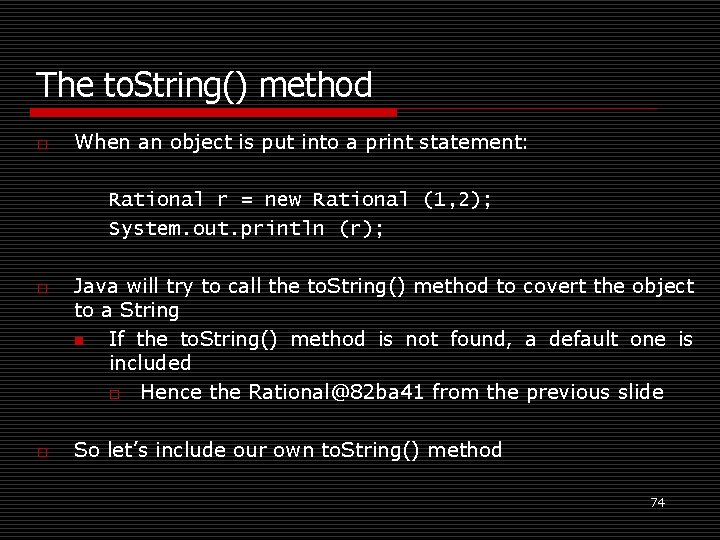
The to. String() method o When an object is put into a print statement: Rational r = new Rational (1, 2); System. out. println (r); o o Java will try to call the to. String() method to covert the object to a String n If the to. String() method is not found, a default one is included o Hence the Rational@82 ba 41 from the previous slide So let’s include our own to. String() method 74
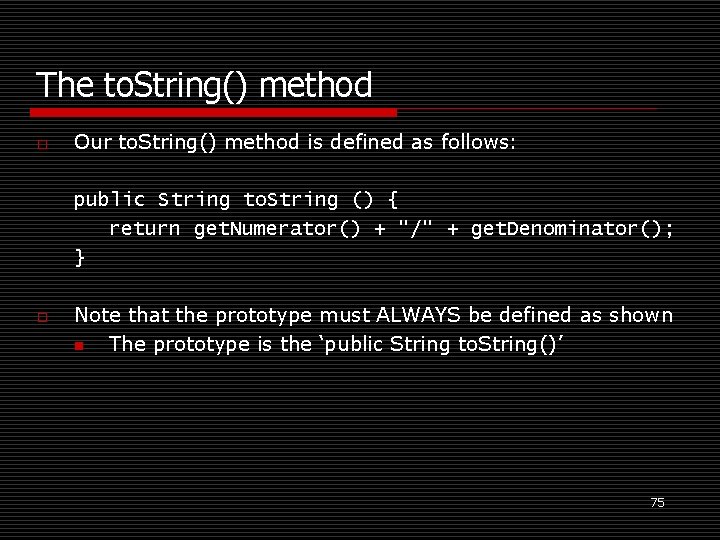
The to. String() method o Our to. String() method is defined as follows: public String to. String () { return get. Numerator() + "/" + get. Denominator(); } o Note that the prototype must ALWAYS be defined as shown n The prototype is the ‘public String to. String()’ 75
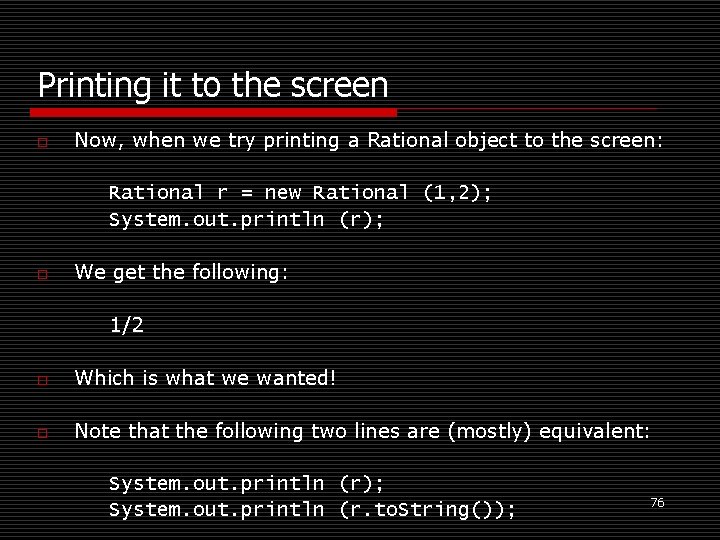
Printing it to the screen o Now, when we try printing a Rational object to the screen: Rational r = new Rational (1, 2); System. out. println (r); o We get the following: 1/2 o Which is what we wanted! o Note that the following two lines are (mostly) equivalent: System. out. println (r); System. out. println (r. to. String()); 76
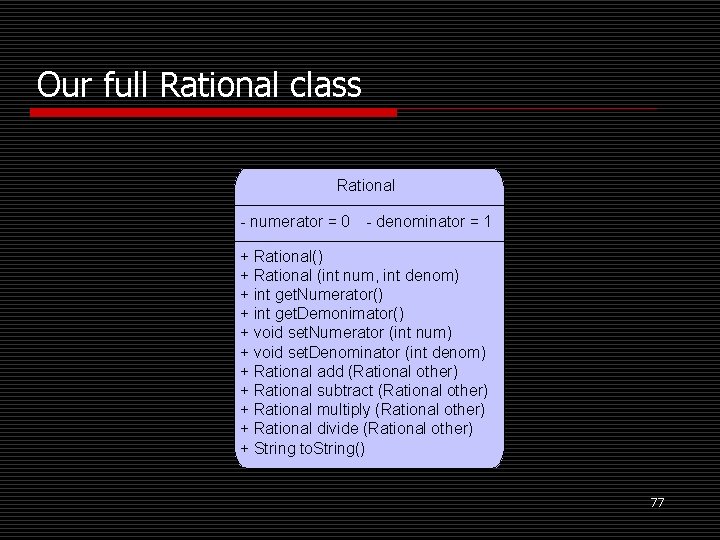
Our full Rational class Rational - numerator = 0 - denominator = 1 + Rational() + Rational (int num, int denom) + int get. Numerator() + int get. Demonimator() + void set. Numerator (int num) + void set. Denominator (int denom) + Rational add (Rational other) + Rational subtract (Rational other) + Rational multiply (Rational other) + Rational divide (Rational other) + String to. String() 77
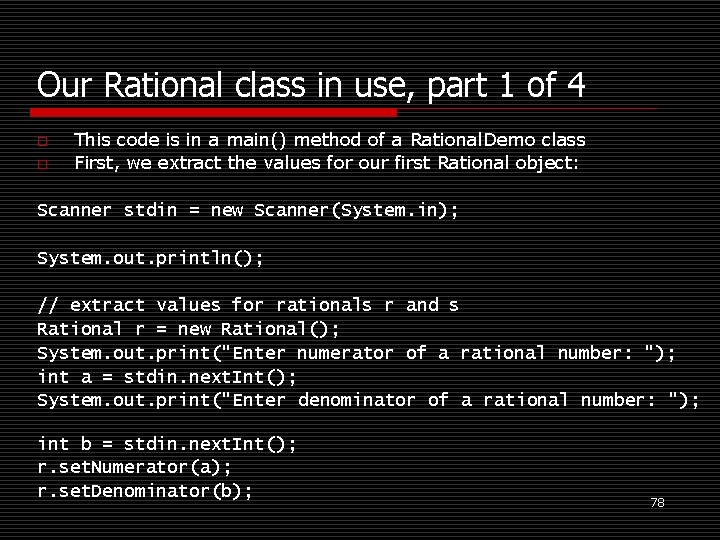
Our Rational class in use, part 1 of 4 o o This code is in a main() method of a Rational. Demo class First, we extract the values for our first Rational object: Scanner stdin = new Scanner(System. in); System. out. println(); // extract values for rationals r and s Rational r = new Rational(); System. out. print("Enter numerator of a rational number: "); int a = stdin. next. Int(); System. out. print("Enter denominator of a rational number: "); int b = stdin. next. Int(); r. set. Numerator(a); r. set. Denominator(b); 78
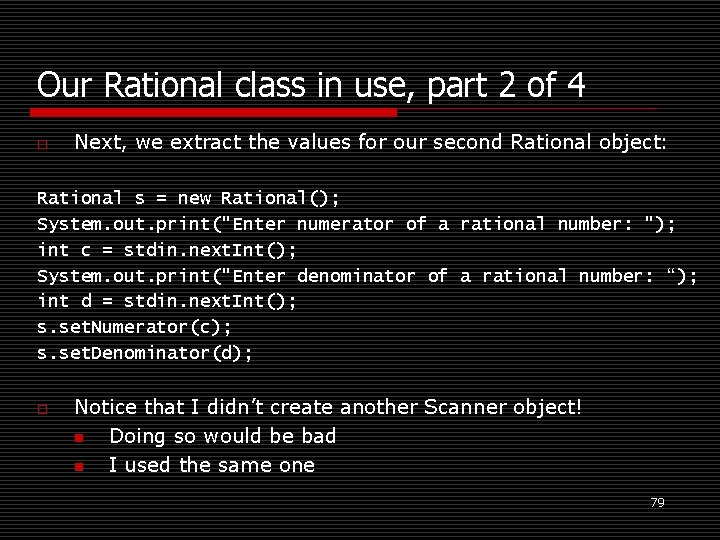
Our Rational class in use, part 2 of 4 o Next, we extract the values for our second Rational object: Rational s = new Rational(); System. out. print("Enter numerator of a rational number: "); int c = stdin. next. Int(); System. out. print("Enter denominator of a rational number: “); int d = stdin. next. Int(); s. set. Numerator(c); s. set. Denominator(d); o Notice that I didn’t create another Scanner object! n Doing so would be bad n I used the same one 79
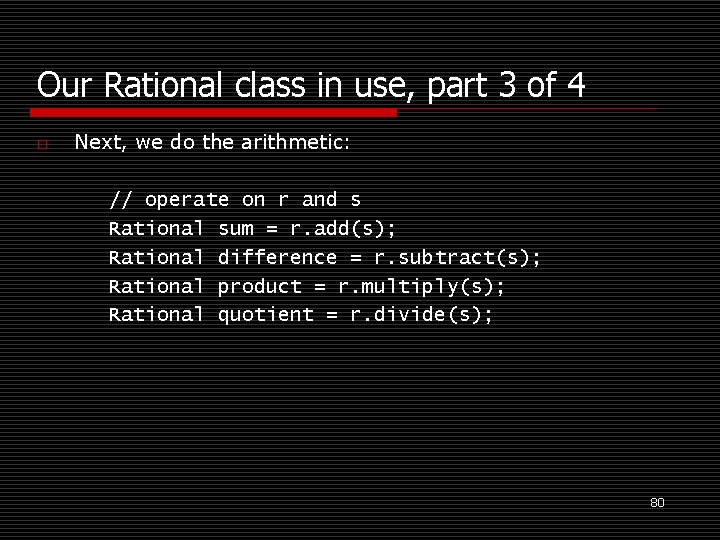
Our Rational class in use, part 3 of 4 o Next, we do the arithmetic: // operate on r and s Rational sum = r. add(s); Rational difference = r. subtract(s); Rational product = r. multiply(s); Rational quotient = r. divide(s); 80
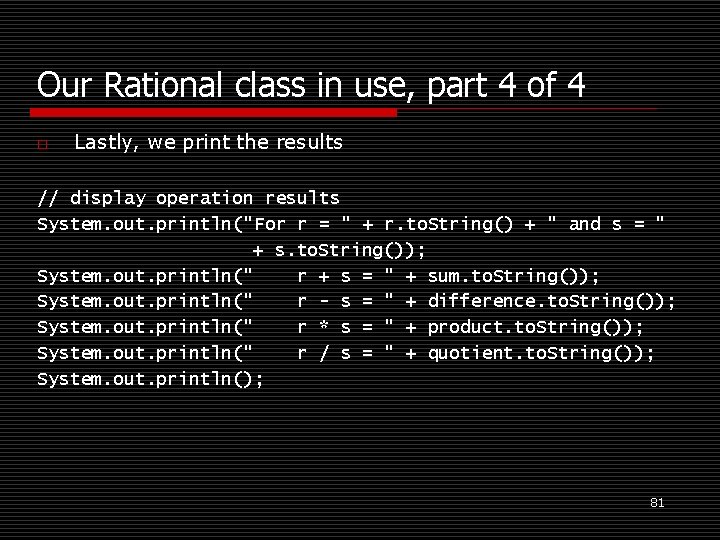
Our Rational class in use, part 4 of 4 o Lastly, we print the results // display operation results System. out. println("For r = " + r. to. String() + " and s = " + s. to. String()); System. out. println(" r + s = " + sum. to. String()); System. out. println(" r - s = " + difference. to. String()); System. out. println(" r * s = " + product. to. String()); System. out. println(" r / s = " + quotient. to. String()); System. out. println(); 81
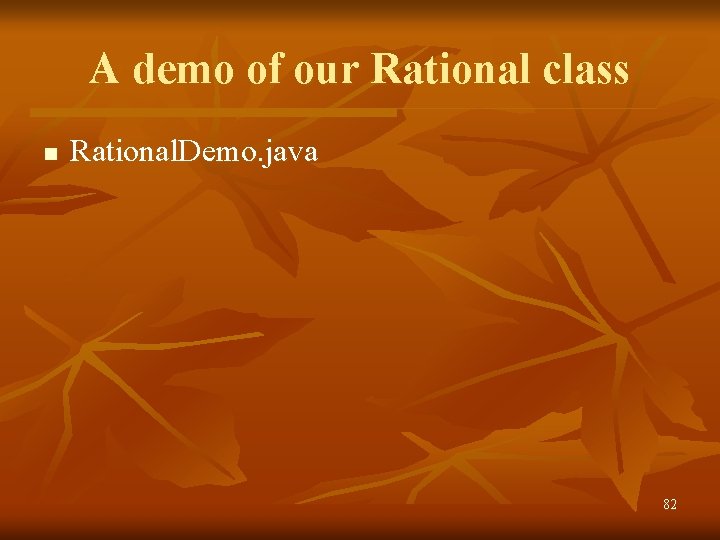
A demo of our Rational class n Rational. Demo. java 82
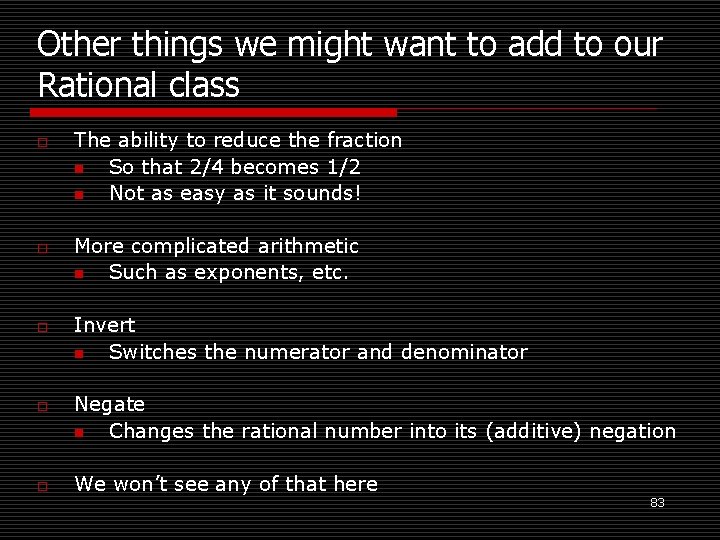
Other things we might want to add to our Rational class o o o The ability to reduce the fraction n So that 2/4 becomes 1/2 n Not as easy as it sounds! More complicated arithmetic n Such as exponents, etc. Invert n Switches the numerator and denominator Negate n Changes the rational number into its (additive) negation We won’t see any of that here 83
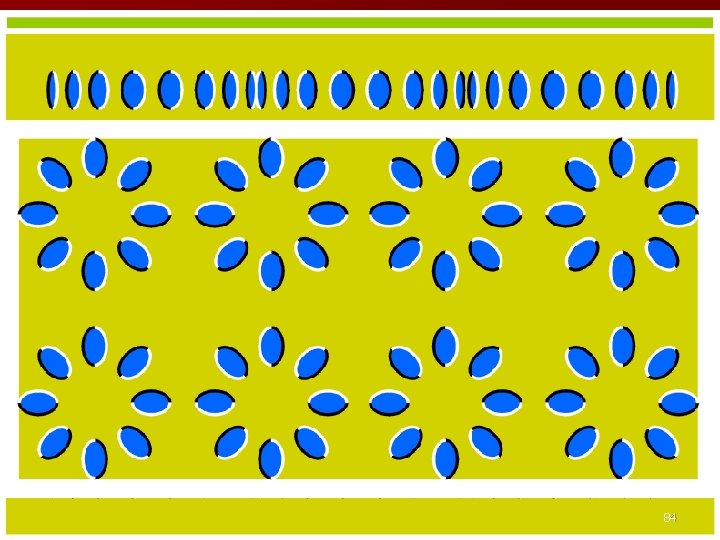
These images are not animated… 84
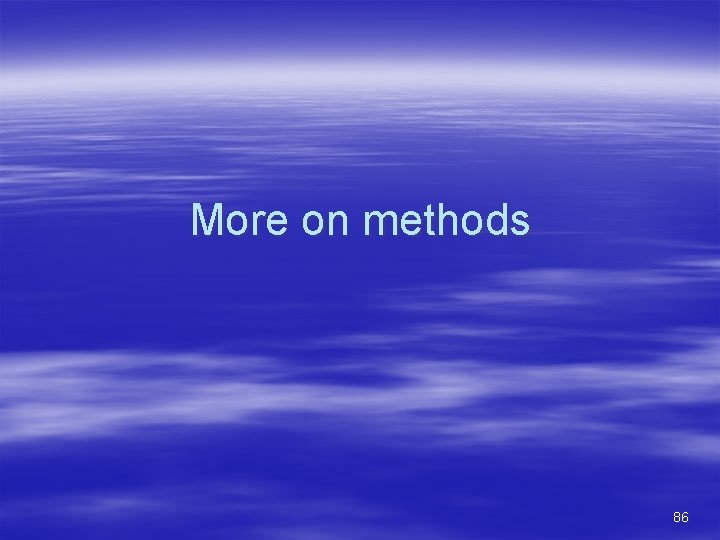
More on methods 86
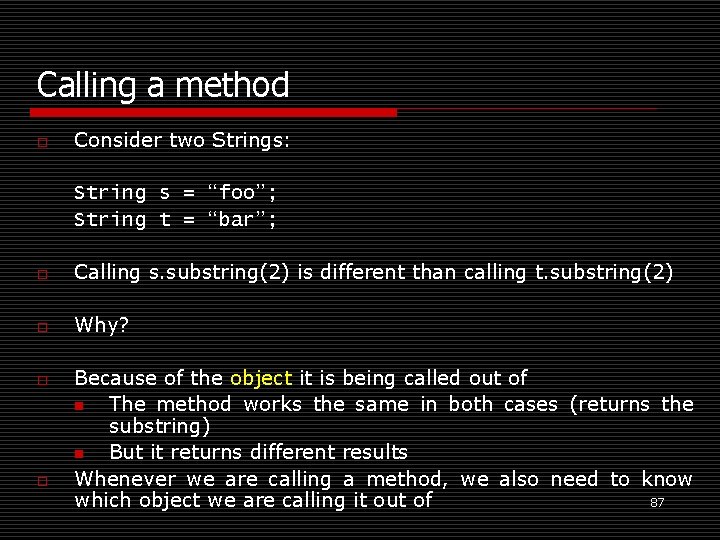
Calling a method o Consider two Strings: String s = “foo”; String t = “bar”; o Calling s. substring(2) is different than calling t. substring(2) o Why? o o Because of the object it is being called out of n The method works the same in both cases (returns the substring) n But it returns different results Whenever we are calling a method, we also need to know 87 which object we are calling it out of
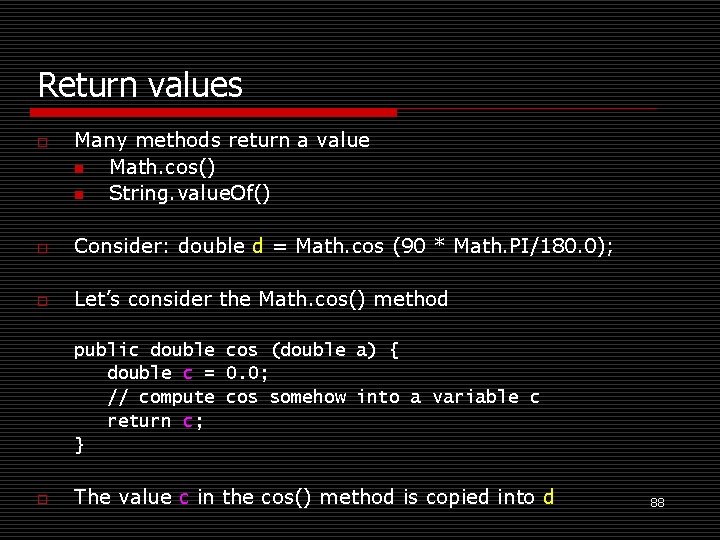
Return values o Many methods return a value n Math. cos() n String. value. Of() o Consider: double d = Math. cos (90 * Math. PI/180. 0); o Let’s consider the Math. cos() method public double cos (double a) { double c = 0. 0; // compute cos somehow into a variable c return c; } o The value c in the cos() method is copied into d 88
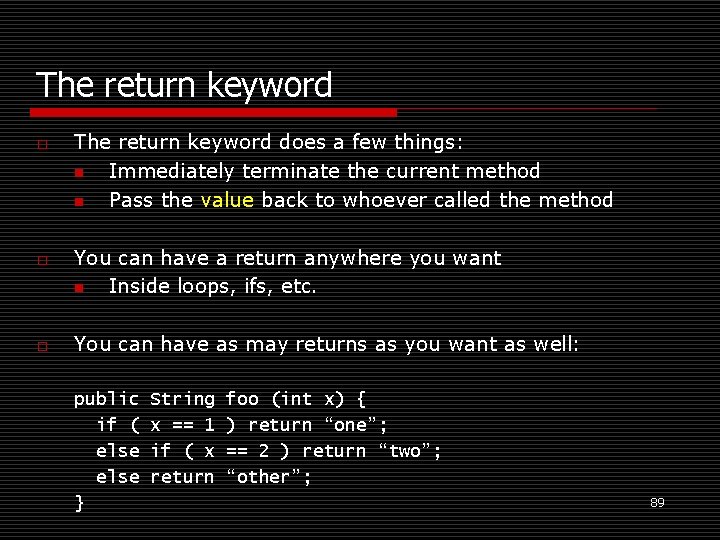
The return keyword o o o The return keyword does a few things: n Immediately terminate the current method n Pass the value back to whoever called the method You can have a return anywhere you want n Inside loops, ifs, etc. You can have as may returns as you want as well: public if ( else } String x == 1 if ( x return foo (int x) { ) return “one”; == 2 ) return “two”; “other”; 89
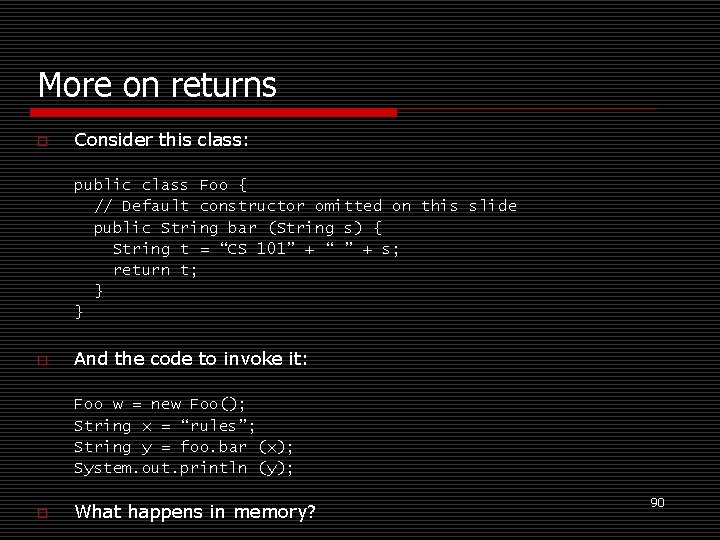
More on returns o Consider this class: public class Foo { // Default constructor omitted on this slide public String bar (String s) { String t = “CS 101” + “ ” + s; return t; } } o And the code to invoke it: Foo w = new Foo(); String x = “rules”; String y = foo. bar (x); System. out. println (y); o What happens in memory? 90
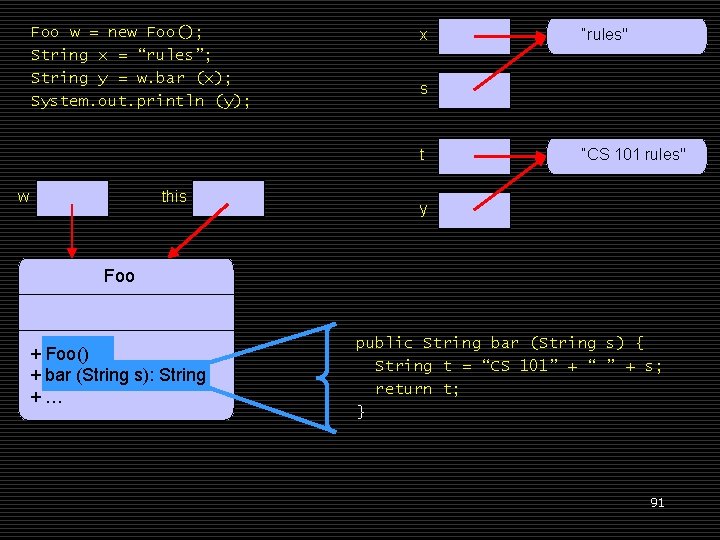
Foo w = new Foo(); String x = “rules”; String y = w. bar (x); System. out. println (y); x s t w this “rules" “CS 101 rules" y Foo + Foo() + bar (String s): String +… public String bar (String s) { String t = “CS 101” + “ ” + s; return t; } 91
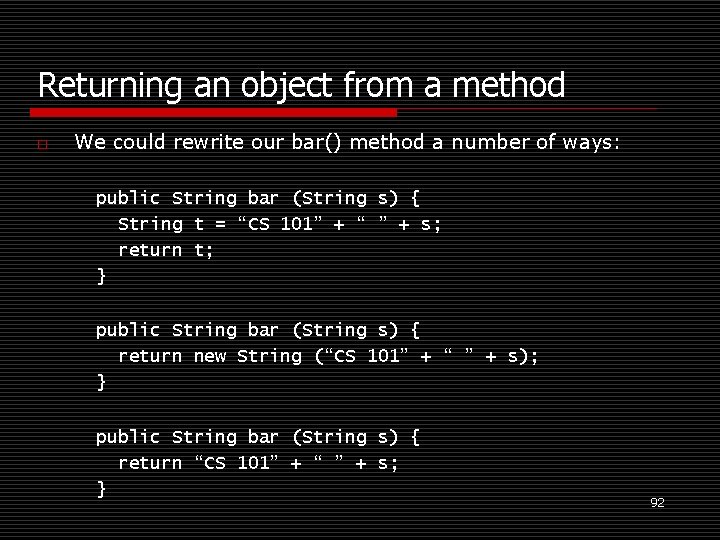
Returning an object from a method o We could rewrite our bar() method a number of ways: public String bar (String s) { String t = “CS 101” + “ ” + s; return t; } public String bar (String s) { return new String (“CS 101” + “ ” + s); } public String bar (String s) { return “CS 101” + “ ” + s; } 92
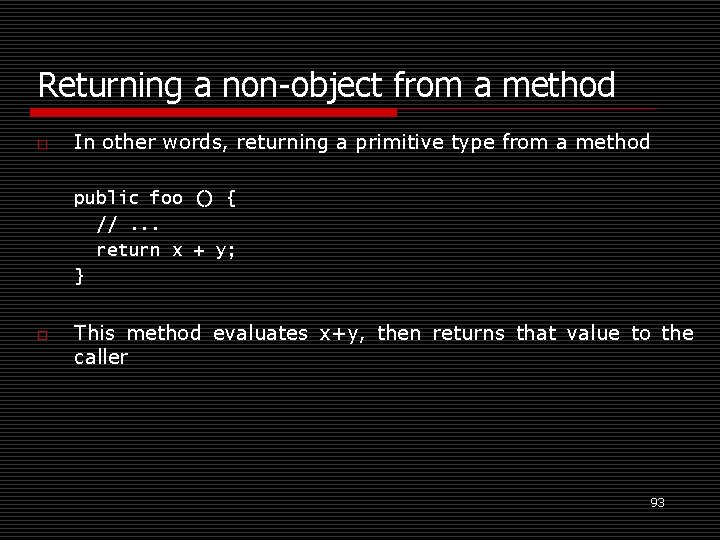
Returning a non-object from a method o In other words, returning a primitive type from a method public foo () { //. . . return x + y; } o This method evaluates x+y, then returns that value to the caller 93
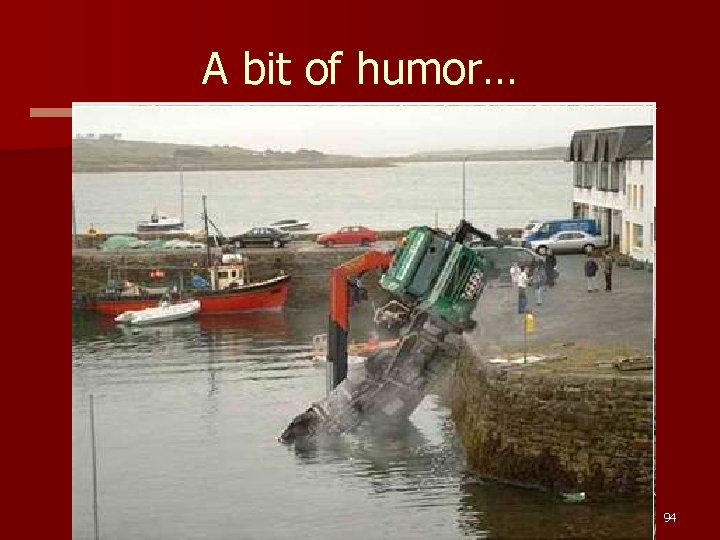
A bit of humor… 94
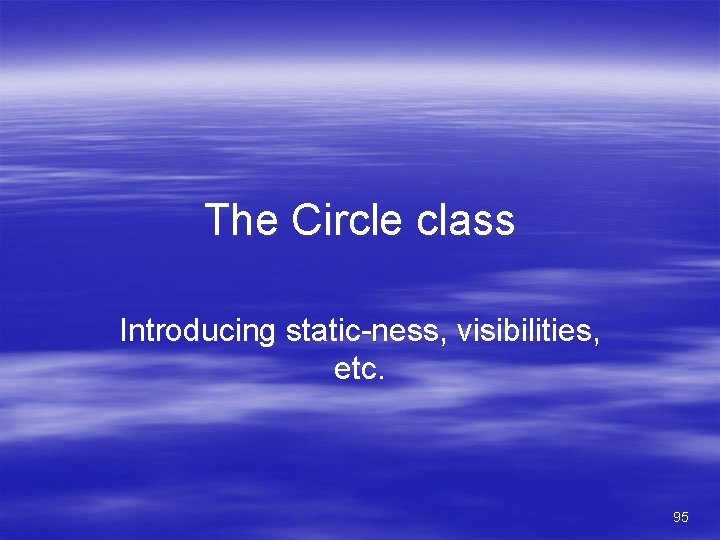
The Circle class Introducing static-ness, visibilities, etc. 95
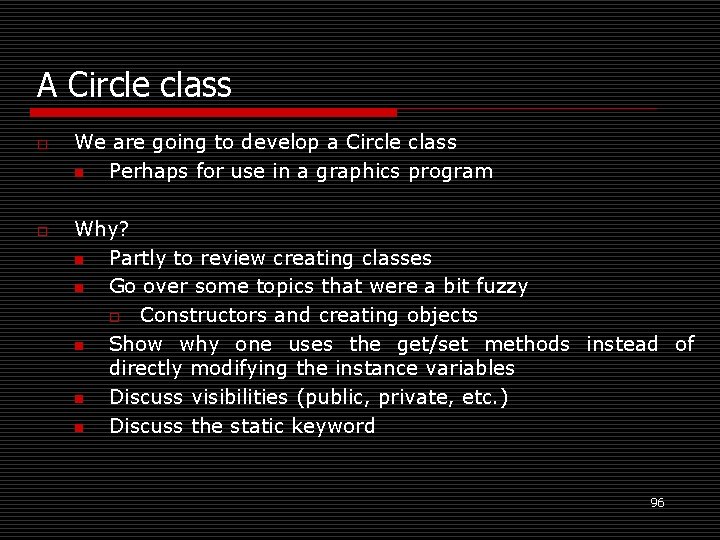
A Circle class o o We are going to develop a Circle class n Perhaps for use in a graphics program Why? n Partly to review creating classes n Go over some topics that were a bit fuzzy o Constructors and creating objects n Show why one uses the get/set methods instead of directly modifying the instance variables n Discuss visibilities (public, private, etc. ) n Discuss the static keyword 96
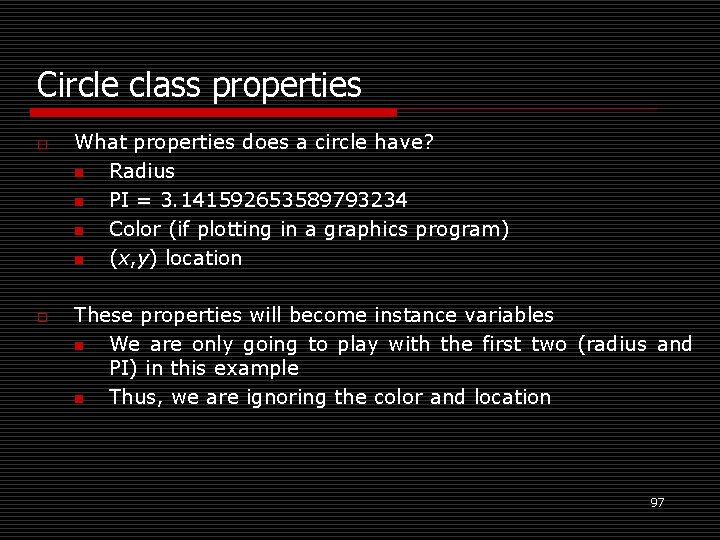
Circle class properties o o What properties does a circle have? n Radius n PI = 3. 141592653589793234 n Color (if plotting in a graphics program) n (x, y) location These properties will become instance variables n We are only going to play with the first two (radius and PI) in this example n Thus, we are ignoring the color and location 97
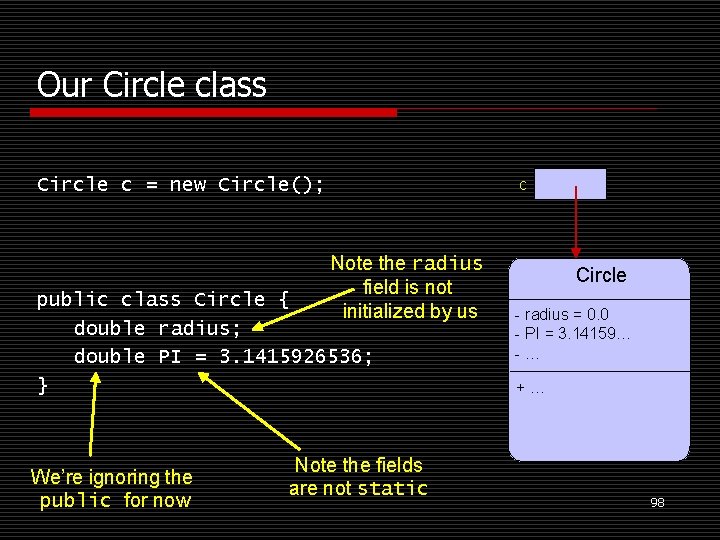
Our Circle class Circle c = new Circle(); c Note the radius field is not initialized by us public class Circle { double radius; double PI = 3. 1415926536; } We’re ignoring the public for now Note the fields are not static Circle - radius = 0. 0 - PI = 3. 14159… -… +… 98
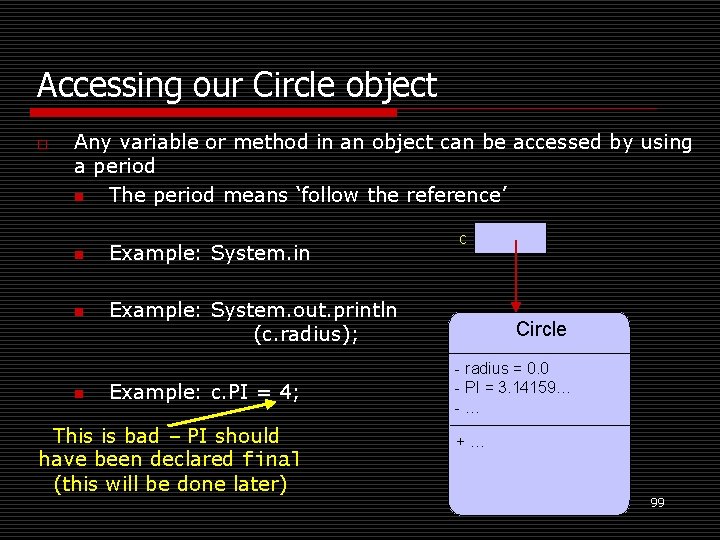
Accessing our Circle object o Any variable or method in an object can be accessed by using a period n The period means ‘follow the reference’ n n n Example: System. in c Example: System. out. println (c. radius); Example: c. PI = 4; This is bad – PI should have been declared final (this will be done later) Circle - radius = 0. 0 - PI = 3. 14159… -… +… 99
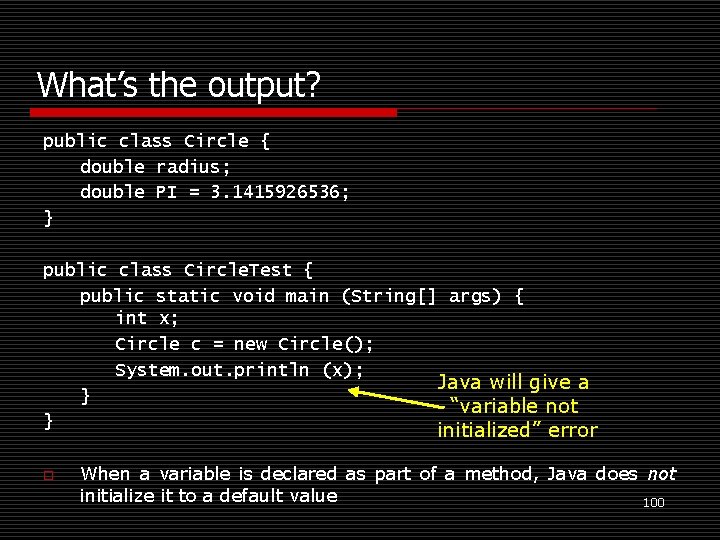
What’s the output? public class Circle { double radius; double PI = 3. 1415926536; } public class Circle. Test { public static void main (String[] args) { int x; Circle c = new Circle(); System. out. println (x); Java will give a } “variable not } initialized” error o When a variable is declared as part of a method, Java does not initialize it to a default value 100
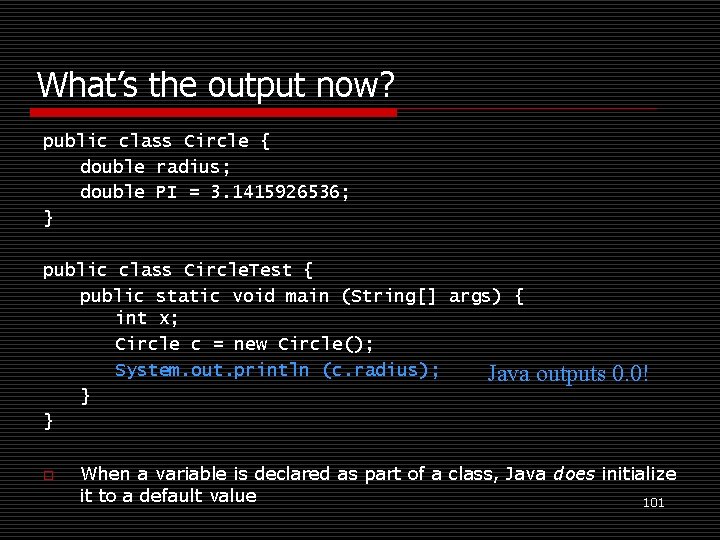
What’s the output now? public class Circle { double radius; double PI = 3. 1415926536; } public class Circle. Test { public static void main (String[] args) { int x; Circle c = new Circle(); System. out. println (c. radius); Java } } o outputs 0. 0! When a variable is declared as part of a class, Java does initialize it to a default value 101
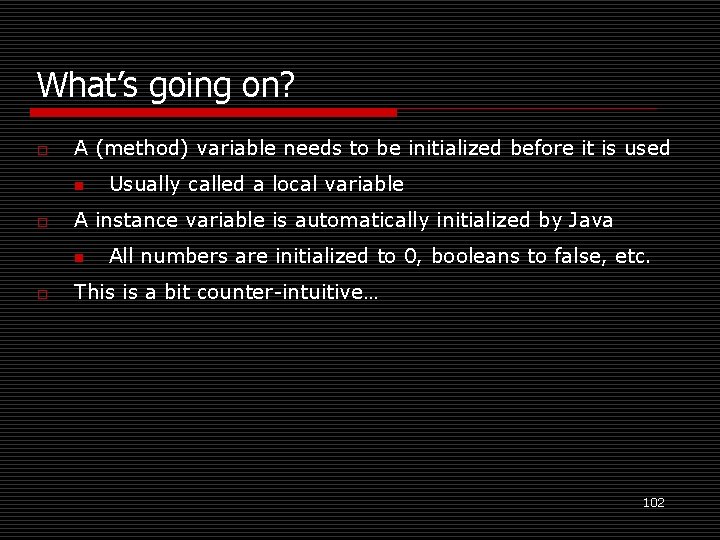
What’s going on? o A (method) variable needs to be initialized before it is used n o A instance variable is automatically initialized by Java n o Usually called a local variable All numbers are initialized to 0, booleans to false, etc. This is a bit counter-intuitive… 102
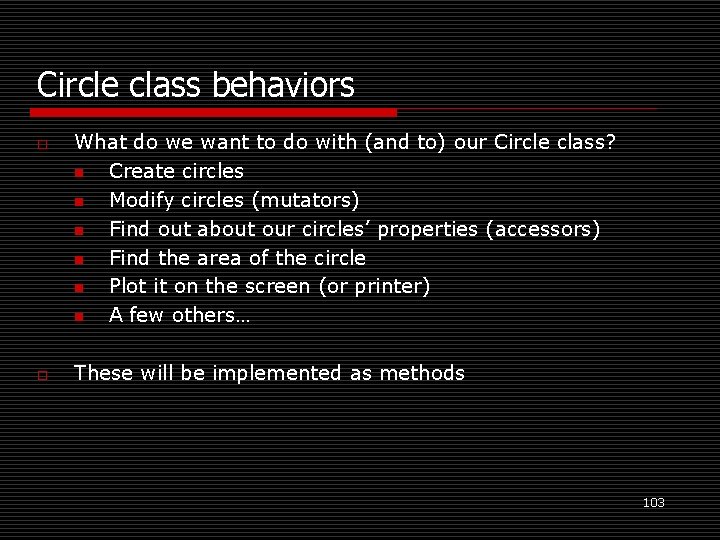
Circle class behaviors o o What do we want to do with (and to) our Circle class? n Create circles n Modify circles (mutators) n Find out about our circles’ properties (accessors) n Find the area of the circle n Plot it on the screen (or printer) n A few others… These will be implemented as methods 103
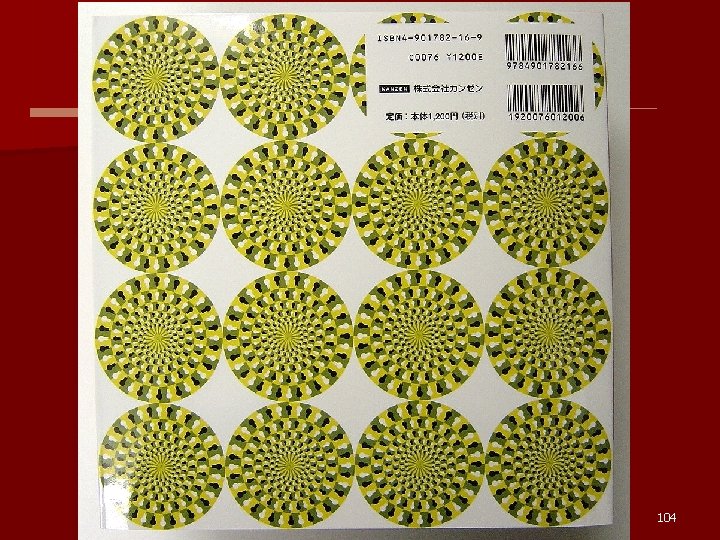
Another optical illusion 104
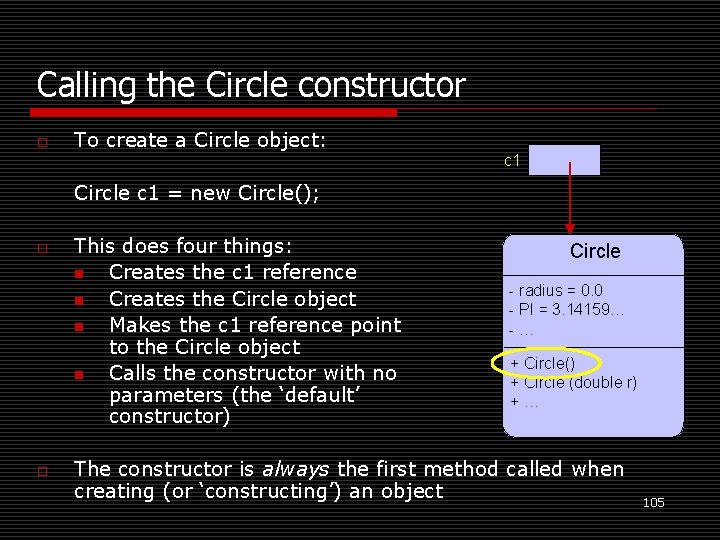
Calling the Circle constructor o To create a Circle object: c 1 Circle c 1 = new Circle(); o o This does four things: n Creates the c 1 reference n Creates the Circle object n Makes the c 1 reference point to the Circle object n Calls the constructor with no parameters (the ‘default’ constructor) Circle - radius = 0. 0 - PI = 3. 14159… -… + Circle() + Circle (double r) +… The constructor is always the first method called when creating (or ‘constructing’) an object 105
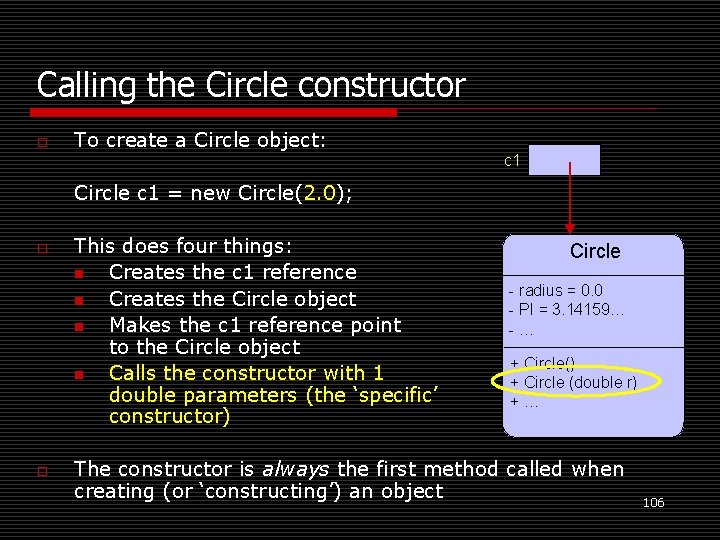
Calling the Circle constructor o To create a Circle object: c 1 Circle c 1 = new Circle(2. 0); o o This does four things: n Creates the c 1 reference n Creates the Circle object n Makes the c 1 reference point to the Circle object n Calls the constructor with 1 double parameters (the ‘specific’ constructor) Circle - radius = 0. 0 - PI = 3. 14159… -… + Circle() + Circle (double r) +… The constructor is always the first method called when creating (or ‘constructing’) an object 106
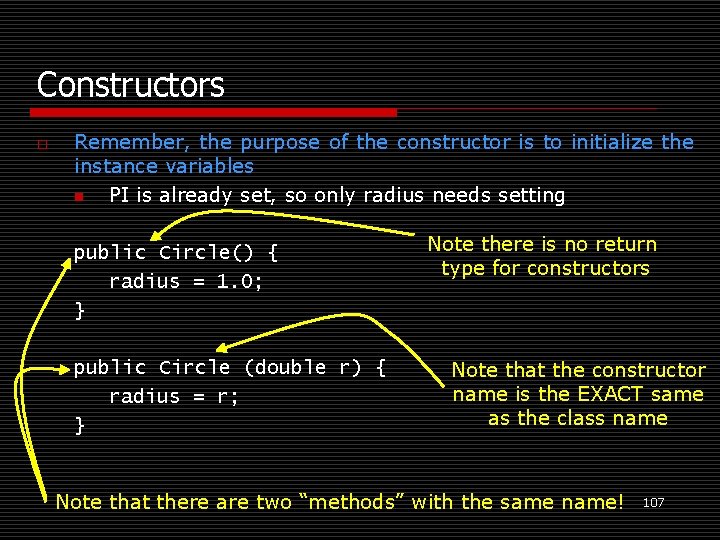
Constructors o Remember, the purpose of the constructor is to initialize the instance variables n PI is already set, so only radius needs setting public Circle() { radius = 1. 0; } public Circle (double r) { radius = r; } Note there is no return type for constructors Note that the constructor name is the EXACT same as the class name Note that there are two “methods” with the same name! 107
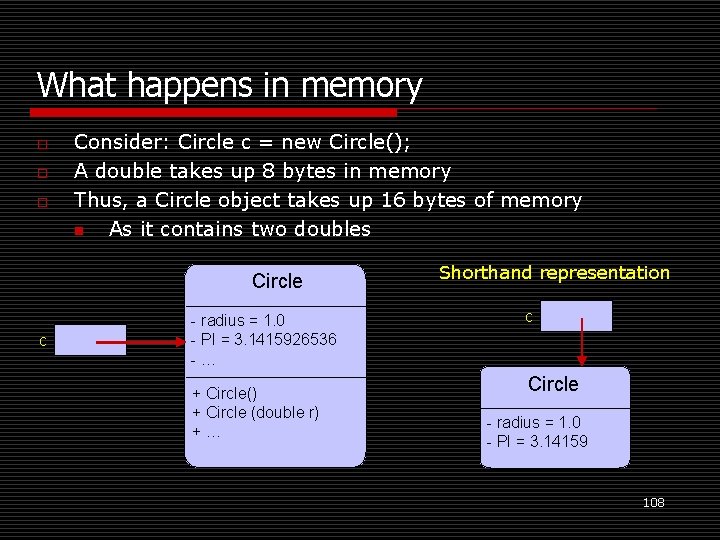
What happens in memory o o o Consider: Circle c = new Circle(); A double takes up 8 bytes in memory Thus, a Circle object takes up 16 bytes of memory n As it contains two doubles Circle c - radius = 1. 0 - PI = 3. 1415926536 -… + Circle() + Circle (double r) +… Shorthand representation c Circle - radius = 1. 0 - PI = 3. 14159 108
![Consider the following code public class Circle Test public static void main String Consider the following code public class Circle. Test { public static void main (String[]](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-106.jpg)
Consider the following code public class Circle. Test { public static void main (String[] args) { Circle c 1 = new Circle(); Circle c 2 = new Circle(); Circle c 3 = new Circle(); Circle c 4 = new Circle(); } } 109
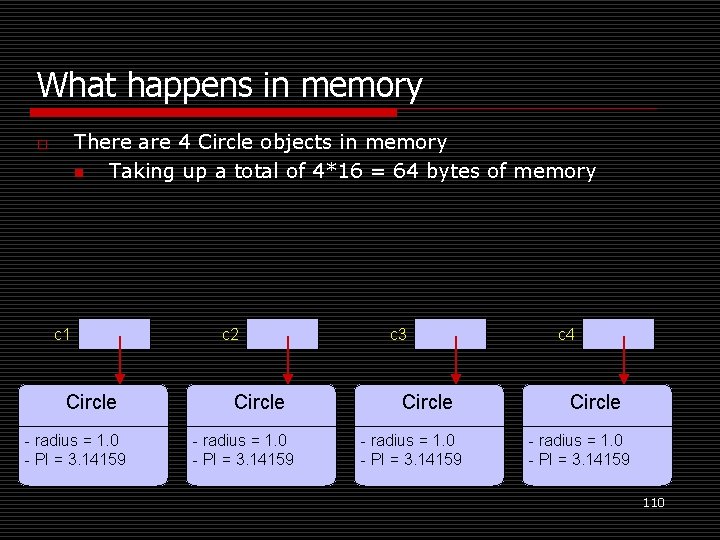
What happens in memory There are 4 Circle objects in memory n Taking up a total of 4*16 = 64 bytes of memory o c 1 Circle - radius = 1. 0 - PI = 3. 14159 c 2 Circle - radius = 1. 0 - PI = 3. 14159 c 3 Circle - radius = 1. 0 - PI = 3. 14159 c 4 Circle - radius = 1. 0 - PI = 3. 14159 110
![Consider the following code public class Circle Test public static void main String Consider the following code public class Circle. Test { public static void main (String[]](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-108.jpg)
Consider the following code public class Circle. Test { public static void main (String[] args) { Circle c 1 = new Circle(); //. . . Circle c 1000000 = new Circle(); } } This program creates 1 million Circle objects! 111
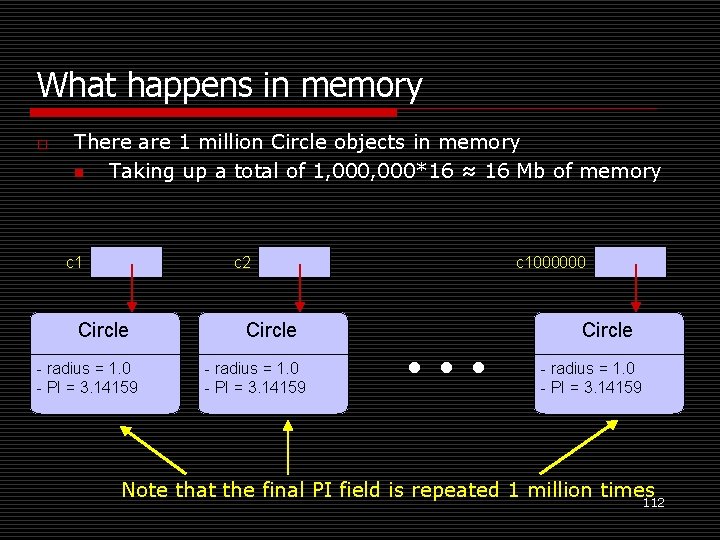
What happens in memory o There are 1 million Circle objects in memory n Taking up a total of 1, 000*16 ≈ 16 Mb of memory c 1 c 2 Circle - radius = 1. 0 - PI = 3. 14159 c 1000000 … Circle - radius = 1. 0 - PI = 3. 14159 Note that the final PI field is repeated 1 million times 112
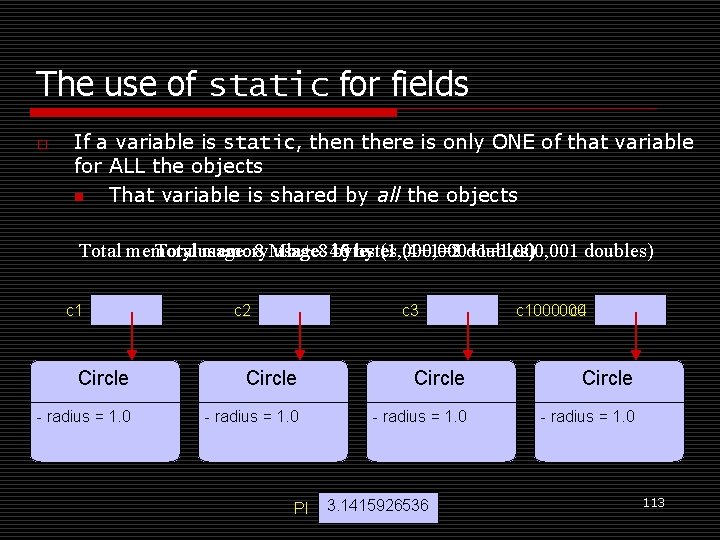
The use of static for fields o If a variable is static, then there is only ONE of that variable for ALL the objects n That variable is shared by all the objects 16 (1+1=2 doubles) Total memory Total usage: memory 8 Mb usage: + 8 40 bytes (1, 000+1=1, 000, 001 (4+1=5 doubles) c 1 c 2 c 3 Circle - radius = 1. 0 PI … c 1000000 c 4 Circle - radius = 1. 0 3. 1415926536 113
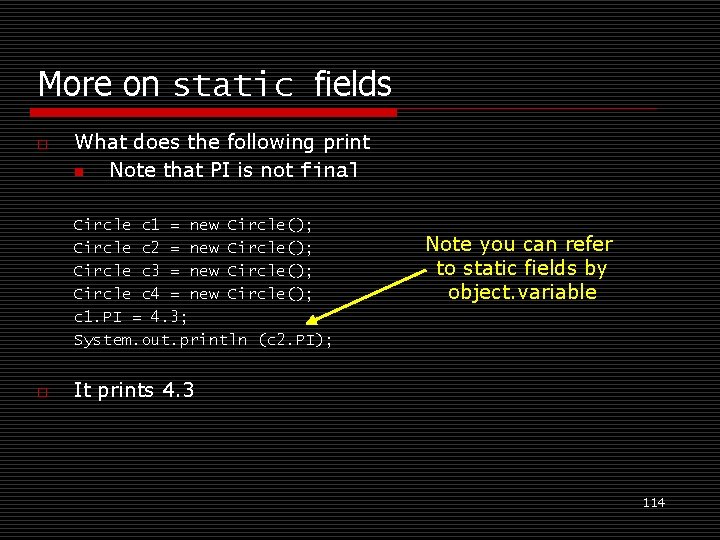
More on static fields o What does the following print n Note that PI is not final Circle c 1 = new Circle(); Circle c 2 = new Circle(); Circle c 3 = new Circle(); Circle c 4 = new Circle(); c 1. PI = 4. 3; System. out. println (c 2. PI); o Note you can refer to static fields by object. variable It prints 4. 3 114
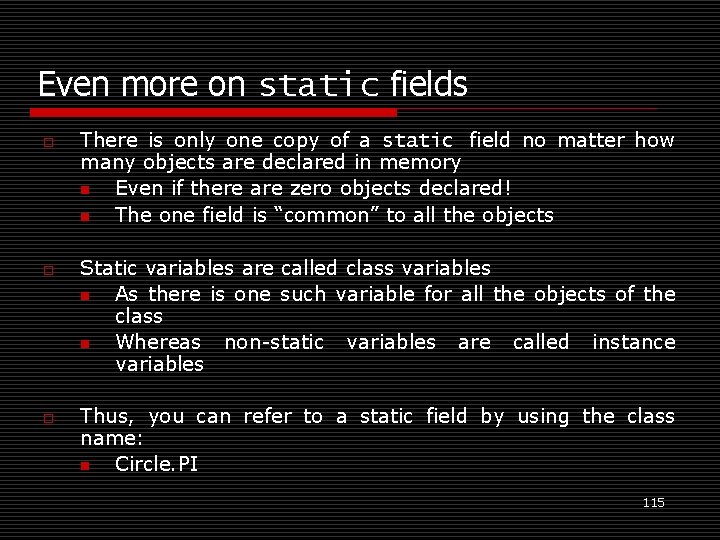
Even more on static fields o o o There is only one copy of a static field no matter how many objects are declared in memory n Even if there are zero objects declared! n The one field is “common” to all the objects Static variables are called class variables n As there is one such variable for all the objects of the class n Whereas non-static variables are called instance variables Thus, you can refer to a static field by using the class name: n Circle. PI 115
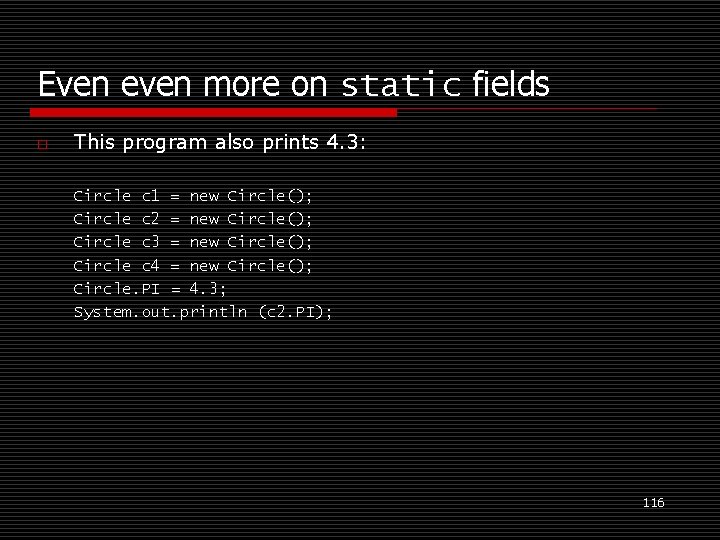
Even even more on static fields o This program also prints 4. 3: Circle c 1 = new Circle(); Circle c 2 = new Circle(); Circle c 3 = new Circle(); Circle c 4 = new Circle(); Circle. PI = 4. 3; System. out. println (c 2. PI); 116
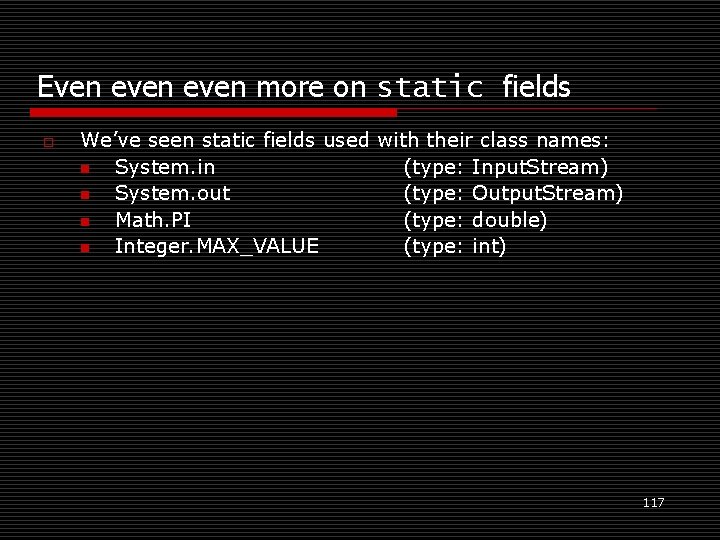
Even even more on static fields o We’ve seen static fields used with their class names: n System. in (type: Input. Stream) n System. out (type: Output. Stream) n Math. PI (type: double) n Integer. MAX_VALUE (type: int) 117
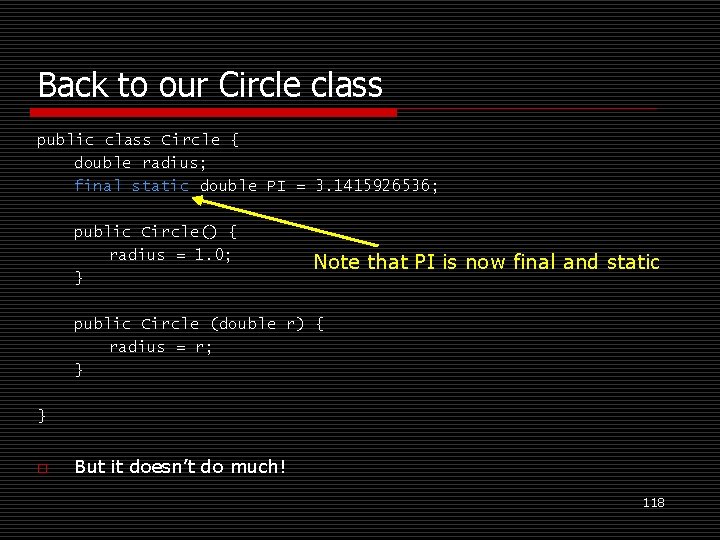
Back to our Circle class public class Circle { double radius; final static double PI = 3. 1415926536; public Circle() { radius = 1. 0; } Note that PI is now final and static public Circle (double r) { radius = r; } } o But it doesn’t do much! 118
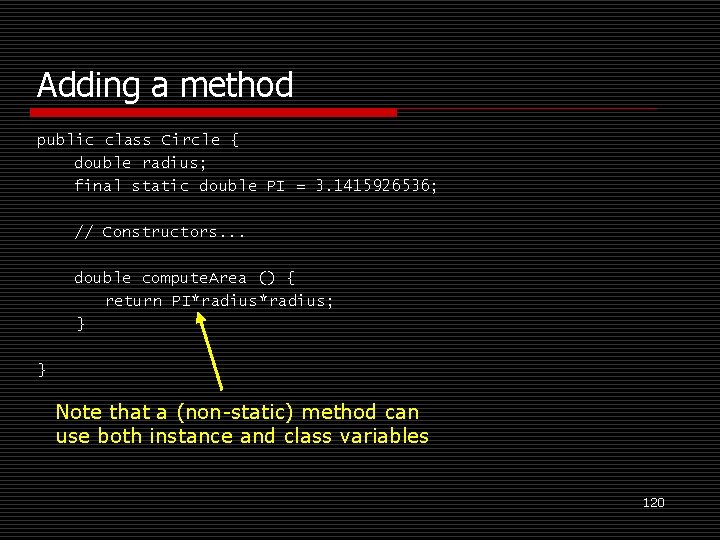
Adding a method public class Circle { double radius; final static double PI = 3. 1415926536; // Constructors. . . double compute. Area () { return PI*radius; } } Note that a (non-static) method can use both instance and class variables 120
![Using that method public class Circle Test public static void main String args Using that method public class Circle. Test { public static void main (String[] args)](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-117.jpg)
Using that method public class Circle. Test { public static void main (String[] args) { Circle c = new Circle(); c. radius = 2. 0; double area = c. compute. Area(); System. out. println (area); } } Prints 12. 566370614356 121
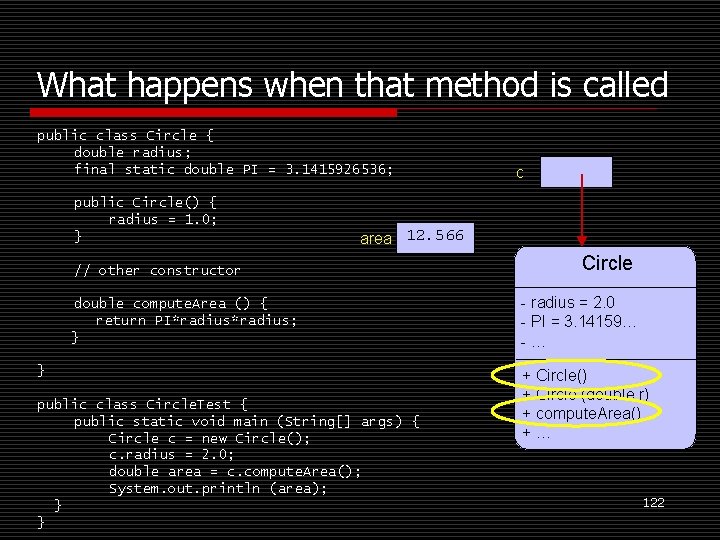
What happens when that method is called public class Circle { double radius; final static double PI = 3. 1415926536; public Circle() { radius = 1. 0; } c area 12. 566 // other constructor double compute. Area () { return PI*radius; } } public class Circle. Test { public static void main (String[] args) { Circle c = new Circle(); c. radius = 2. 0; double area = c. compute. Area(); System. out. println (area); } } Circle - radius = 2. 0 0. 0 1. 0 - PI = 3. 14159… -… + Circle() + Circle (double r) + compute. Area() +… 122
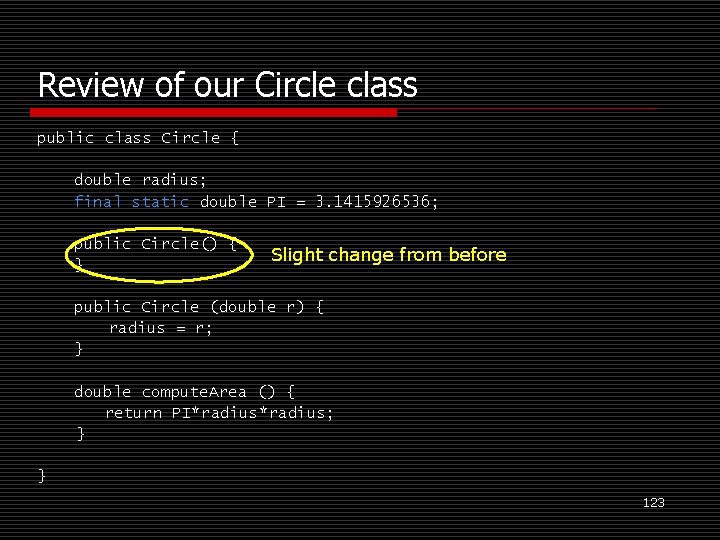
Review of our Circle class public class Circle { double radius; final static double PI = 3. 1415926536; public Circle() { } Slight change from before public Circle (double r) { radius = r; } double compute. Area () { return PI*radius; } } 123
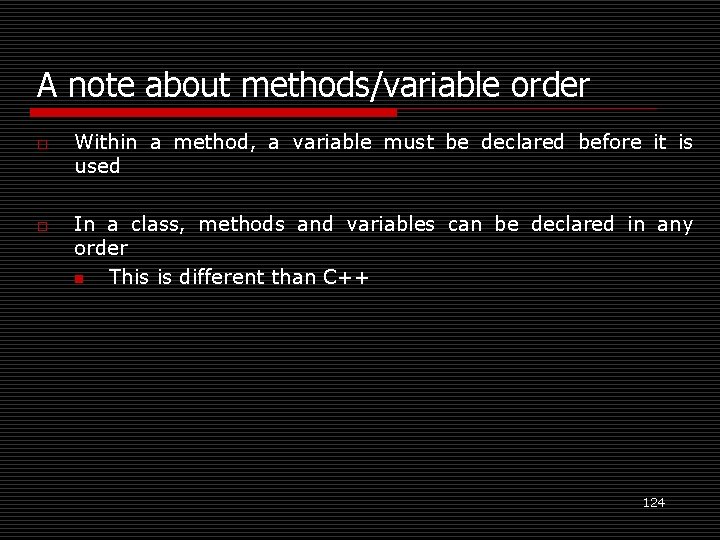
A note about methods/variable order o o Within a method, a variable must be declared before it is used In a class, methods and variables can be declared in any order n This is different than C++ 124
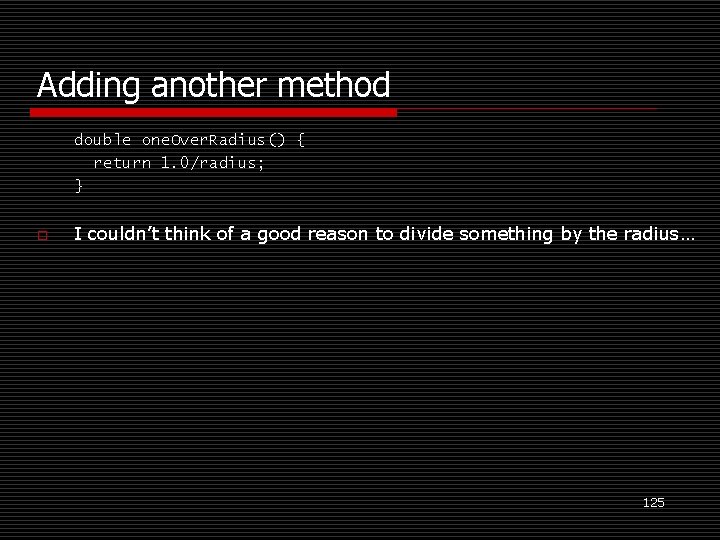
Adding another method double one. Over. Radius() { return 1. 0/radius; } o I couldn’t think of a good reason to divide something by the radius… 125
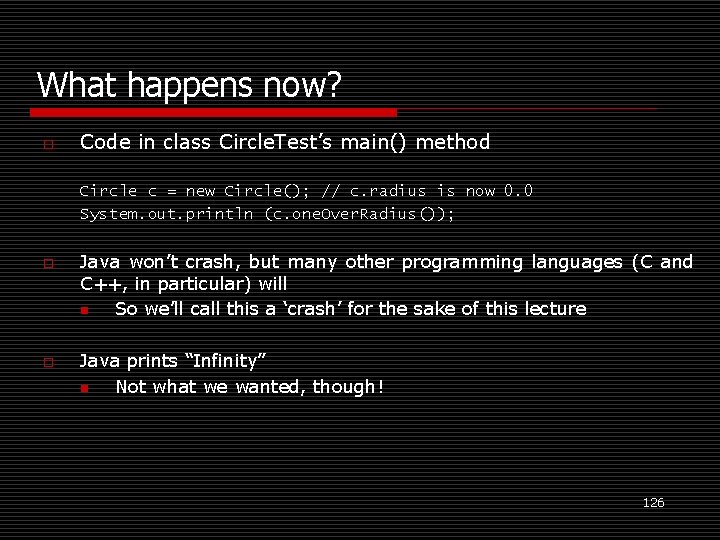
What happens now? o Code in class Circle. Test’s main() method Circle c = new Circle(); // c. radius is now 0. 0 System. out. println (c. one. Over. Radius()); o o Java won’t crash, but many other programming languages (C and C++, in particular) will n So we’ll call this a ‘crash’ for the sake of this lecture Java prints “Infinity” n Not what we wanted, though! 126
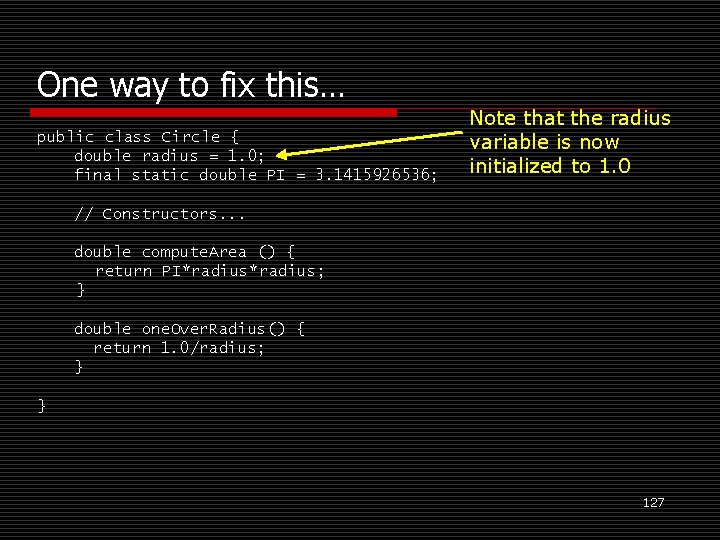
One way to fix this… public class Circle { double radius = 1. 0; final static double PI = 3. 1415926536; Note that the radius variable is now initialized to 1. 0 // Constructors. . . double compute. Area () { return PI*radius; } double one. Over. Radius() { return 1. 0/radius; } } 127
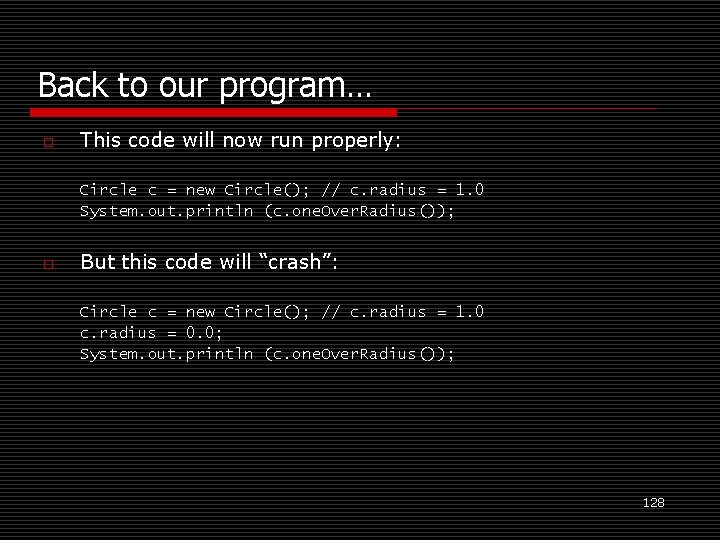
Back to our program… o This code will now run properly: Circle c = new Circle(); // c. radius = 1. 0 System. out. println (c. one. Over. Radius()); o But this code will “crash”: Circle c = new Circle(); // c. radius = 1. 0 c. radius = 0. 0; System. out. println (c. one. Over. Radius()); 128
![Where the crash occurs public class Circle Test public static void main String Where the “crash” occurs public class Circle. Test { public static void main (String[]](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-125.jpg)
Where the “crash” occurs public class Circle. Test { public static void main (String[] args) { Circle c = new Circle(); // c. radius = 1. 0 c. radius = 0. 0; System. out. println (c. one. Over. Radius()); } } Here is the badly written code public class Circle { double radius = 1. 0; final static double PI = 3. 1415926536; double compute. Area () { return PI*radius; } double one. Over. Radius() { return 1. 0/radius; } Here is where the “crash” occurs 129
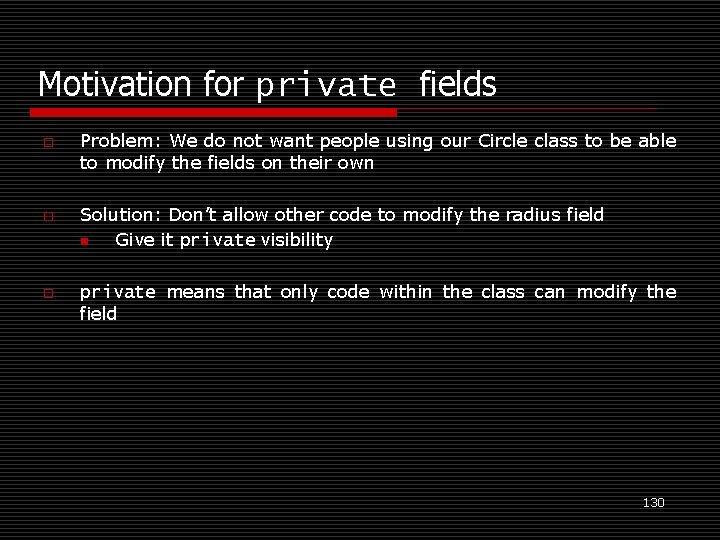
Motivation for private fields o o o Problem: We do not want people using our Circle class to be able to modify the fields on their own Solution: Don’t allow other code to modify the radius field n Give it private visibility private means that only code within the class can modify the field 130
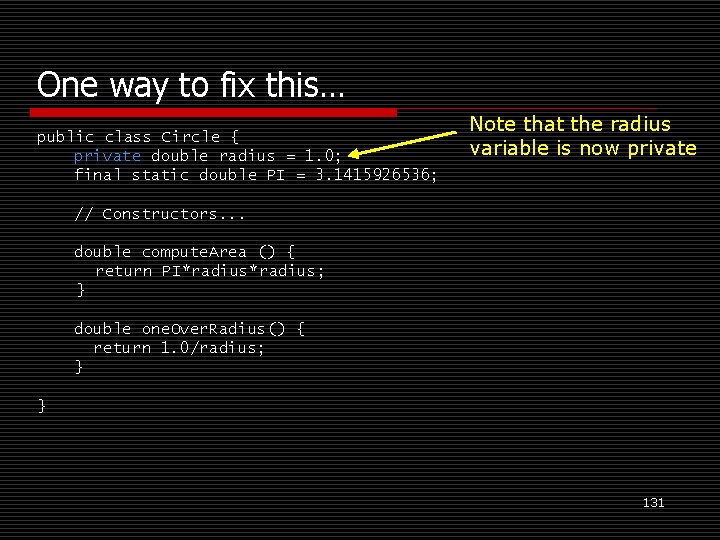
One way to fix this… public class Circle { private double radius = 1. 0; final static double PI = 3. 1415926536; Note that the radius variable is now private // Constructors. . . double compute. Area () { return PI*radius; } double one. Over. Radius() { return 1. 0/radius; } } 131
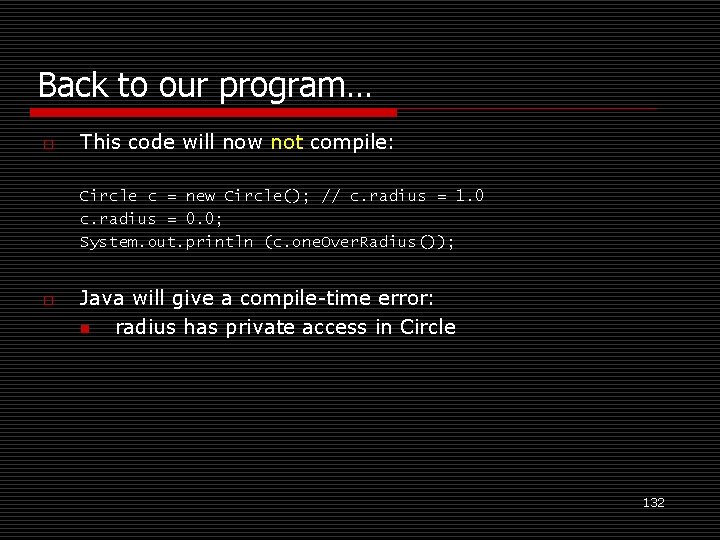
Back to our program… o This code will now not compile: Circle c = new Circle(); // c. radius = 1. 0 c. radius = 0. 0; System. out. println (c. one. Over. Radius()); o Java will give a compile-time error: n radius has private access in Circle 132
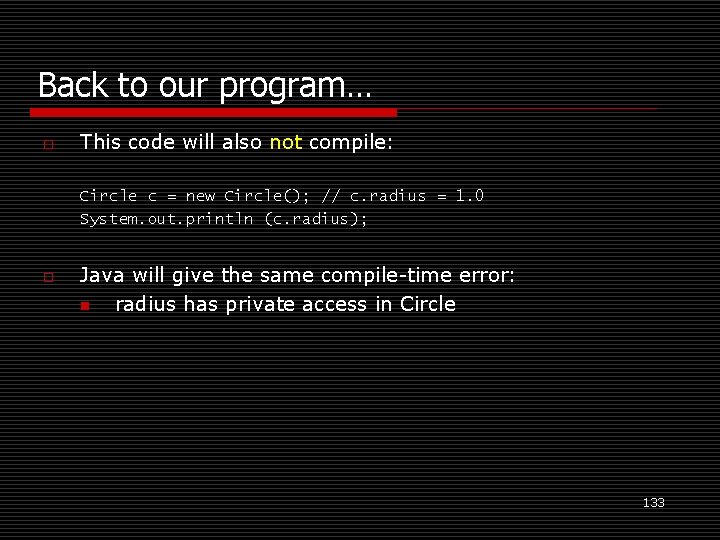
Back to our program… o This code will also not compile: Circle c = new Circle(); // c. radius = 1. 0 System. out. println (c. radius); o Java will give the same compile-time error: n radius has private access in Circle 133
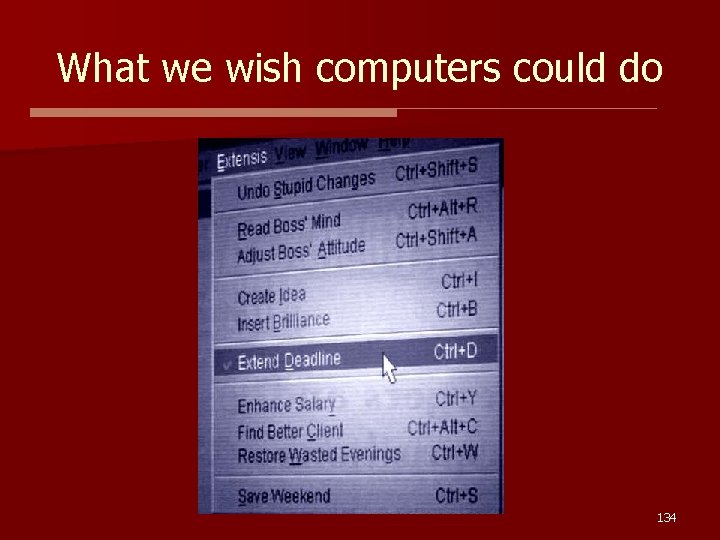
What we wish computers could do 134
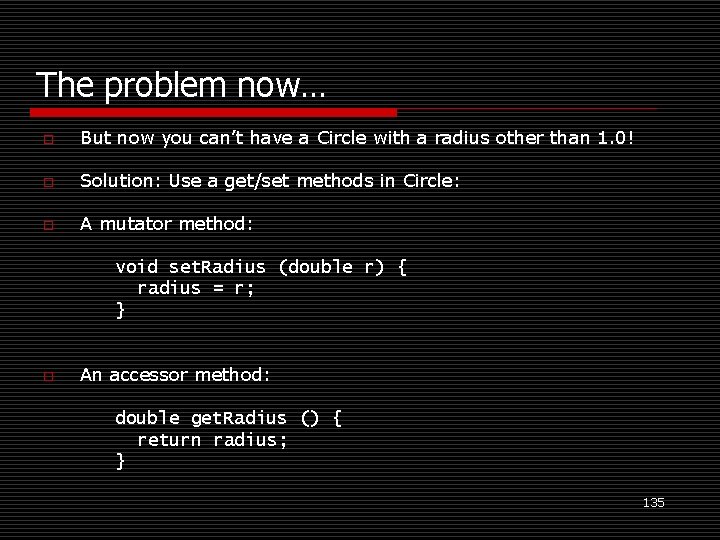
The problem now… o But now you can’t have a Circle with a radius other than 1. 0! o Solution: Use a get/set methods in Circle: o A mutator method: void set. Radius (double r) { radius = r; } o An accessor method: double get. Radius () { return radius; } 135
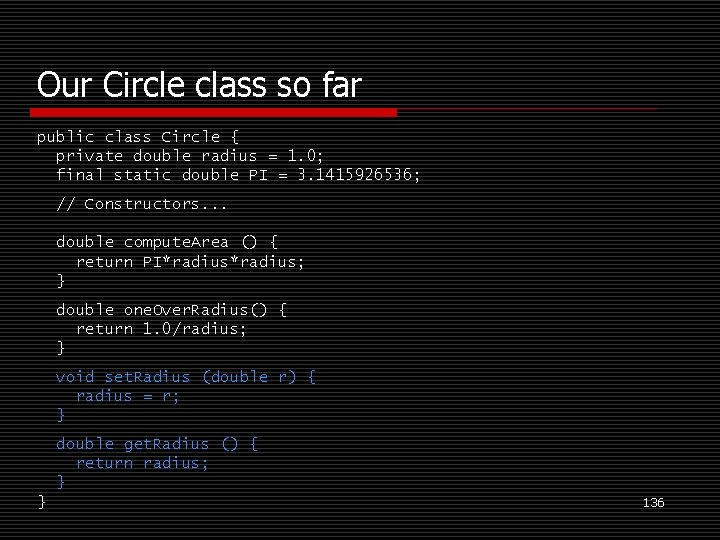
Our Circle class so far public class Circle { private double radius = 1. 0; final static double PI = 3. 1415926536; // Constructors. . . double compute. Area () { return PI*radius; } double one. Over. Radius() { return 1. 0/radius; } void set. Radius (double r) { radius = r; } double get. Radius () { return radius; } } 136
![Using the getset methods public class Circle Test public static void main String Using the get/set methods public class Circle. Test { public static void main (String[]](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-133.jpg)
Using the get/set methods public class Circle. Test { public static void main (String[] args) { public class Circle { private double radius = 1. 0; final static double PI = 3. 1415926536; double compute. Area () { return PI*radius; } Circle c = new Circle(); c. set. Radius (1. 0); double one. Over. Radius() { return 1. 0/radius; } System. out. println (c. compute. Area()); void set. Radius (double r) { radius = r; } System. out. println (c. get. Radius()); } double get. Radius () { return radius; } } Here a method is invoked } 137 Here the change to radius occurs
![Wait Another problem public class Circle Test public static void main String args Wait! Another problem! public class Circle. Test { public static void main (String[] args)](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-134.jpg)
Wait! Another problem! public class Circle. Test { public static void main (String[] args) { Circle c = new Circle(); c. set. Radius (0. 0); Here is the problem now… System. out. println (c. one. Over. Radius()); } } 138
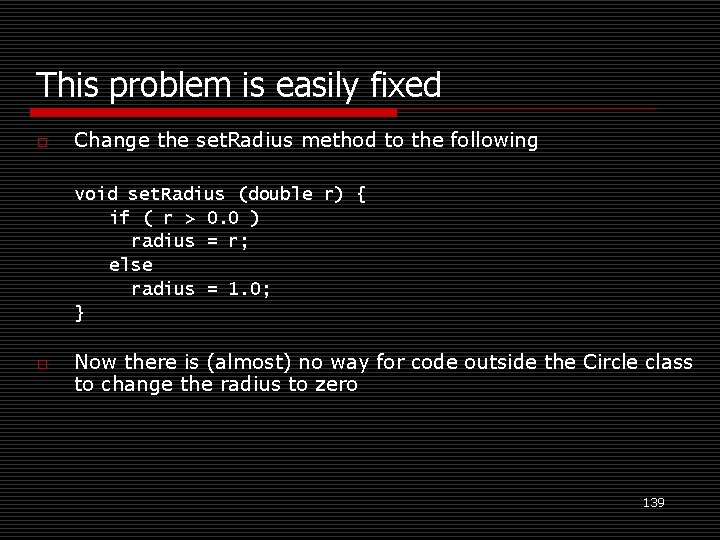
This problem is easily fixed o Change the set. Radius method to the following void set. Radius (double r) { if ( r > 0. 0 ) radius = r; else radius = 1. 0; } o Now there is (almost) no way for code outside the Circle class to change the radius to zero 139
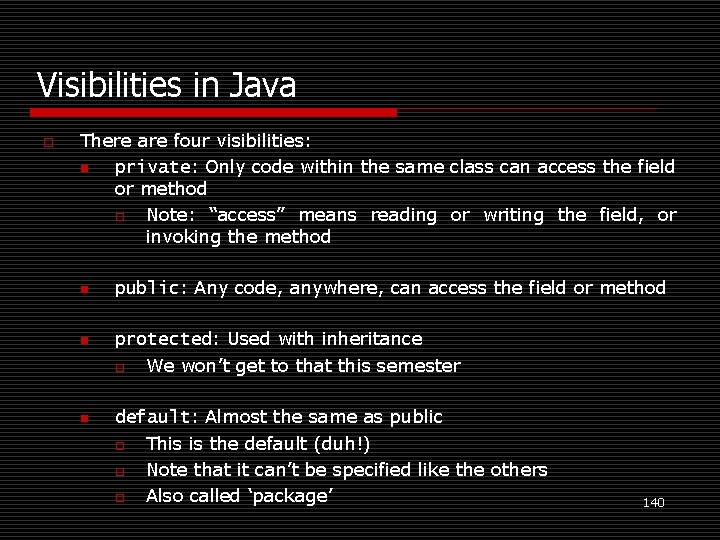
Visibilities in Java o There are four visibilities: n private: Only code within the same class can access the field or method o Note: “access” means reading or writing the field, or invoking the method n n n public: Any code, anywhere, can access the field or method protected: Used with inheritance o We won’t get to that this semester default: Almost the same as public o This is the default (duh!) o Note that it can’t be specified like the others o Also called ‘package’ 140
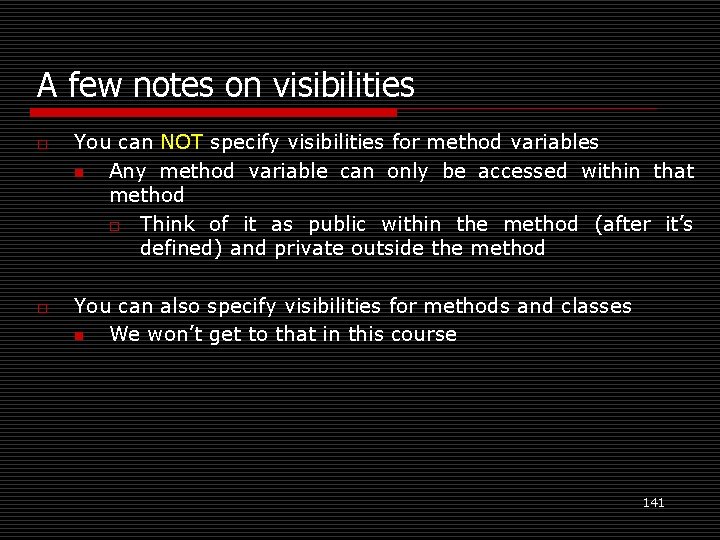
A few notes on visibilities o o You can NOT specify visibilities for method variables n Any method variable can only be accessed within that method o Think of it as public within the method (after it’s defined) and private outside the method You can also specify visibilities for methods and classes n We won’t get to that in this course 141
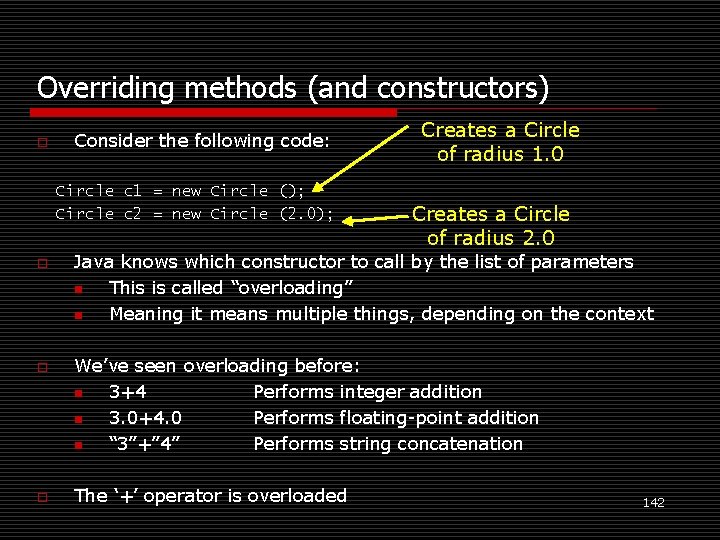
Overriding methods (and constructors) o Consider the following code: Circle c 1 = new Circle (); Circle c 2 = new Circle (2. 0); o o o Creates a Circle of radius 1. 0 Creates a Circle of radius 2. 0 Java knows which constructor to call by the list of parameters n This is called “overloading” n Meaning it means multiple things, depending on the context We’ve seen overloading before: n 3+4 Performs integer addition n 3. 0+4. 0 Performs floating-point addition n “ 3”+” 4” Performs string concatenation The ‘+’ operator is overloaded 142
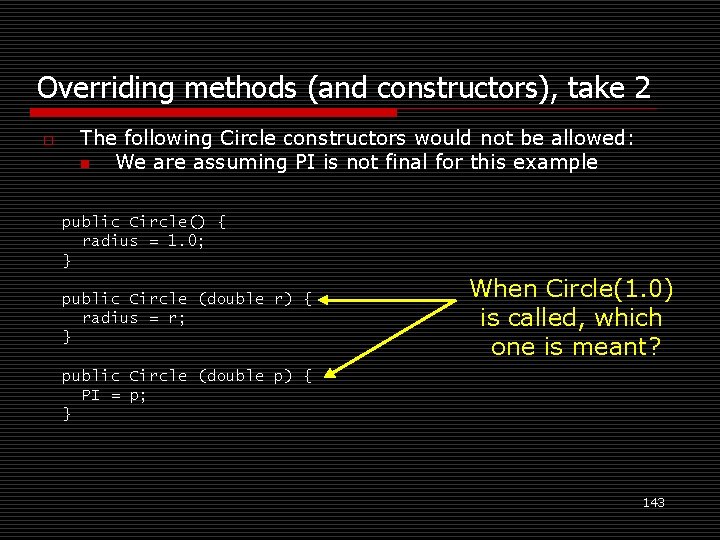
Overriding methods (and constructors), take 2 o The following Circle constructors would not be allowed: n We are assuming PI is not final for this example public Circle() { radius = 1. 0; } public Circle (double r) { radius = r; } When Circle(1. 0) is called, which one is meant? public Circle (double p) { PI = p; } 143
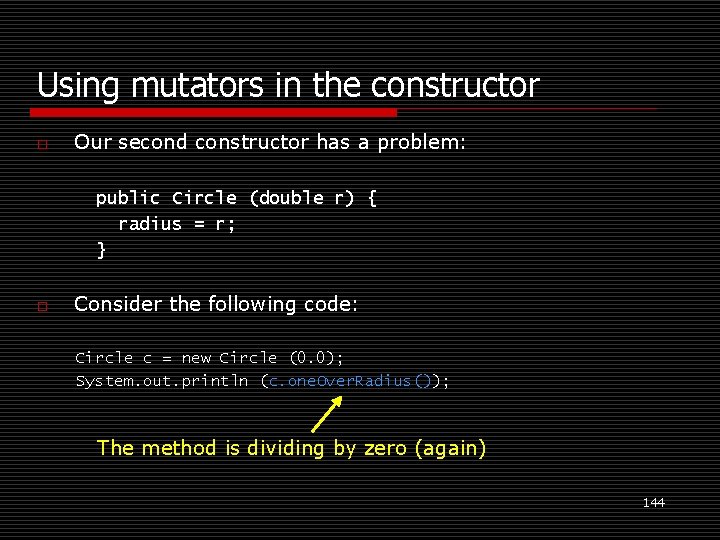
Using mutators in the constructor o Our second constructor has a problem: public Circle (double r) { radius = r; } o Consider the following code: Circle c = new Circle (0. 0); System. out. println (c. one. Over. Radius()); The method is dividing by zero (again) 144
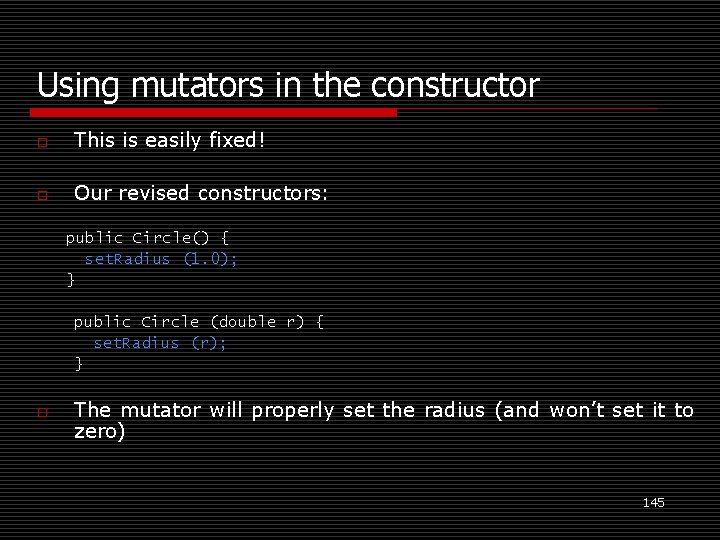
Using mutators in the constructor o This is easily fixed! o Our revised constructors: public Circle() { set. Radius (1. 0); } public Circle (double r) { set. Radius (r); } o The mutator will properly set the radius (and won’t set it to zero) 145
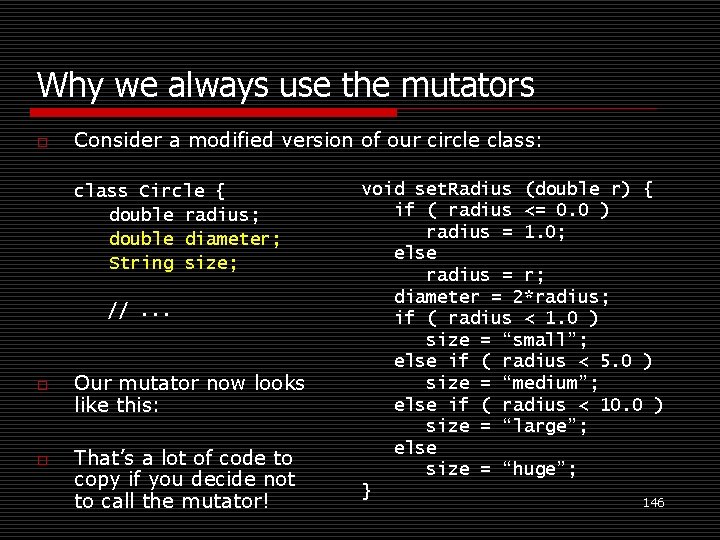
Why we always use the mutators o Consider a modified version of our circle class: class Circle { double radius; double diameter; String size; //. . . o o Our mutator now looks like this: That’s a lot of code to copy if you decide not to call the mutator! void set. Radius (double r) { if ( radius <= 0. 0 ) radius = 1. 0; else radius = r; diameter = 2*radius; if ( radius < 1. 0 ) size = “small”; else if ( radius < 5. 0 ) size = “medium”; else if ( radius < 10. 0 ) size = “large”; else size = “huge”; } 146
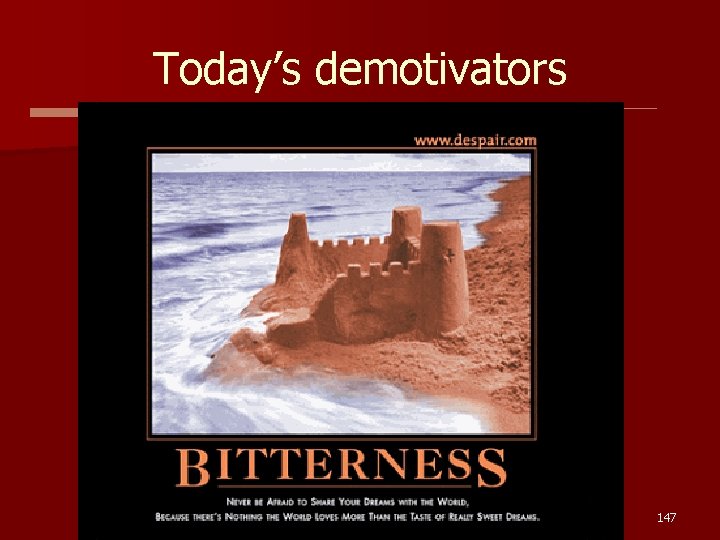
Today’s demotivators 147
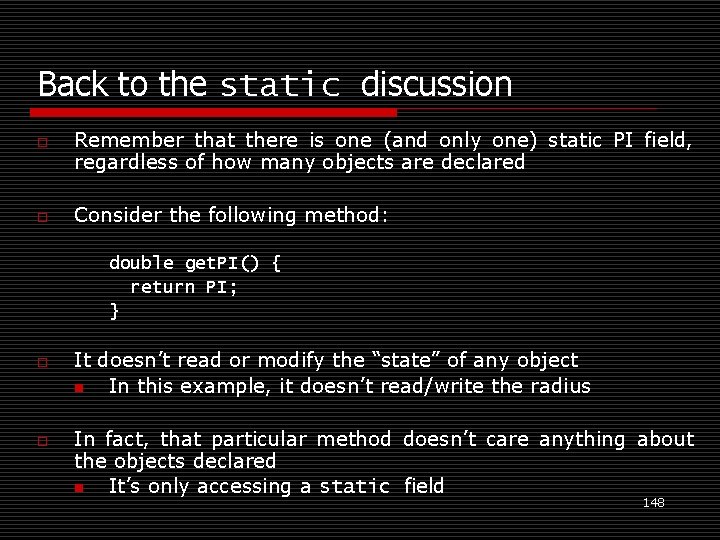
Back to the static discussion o o Remember that there is one (and only one) static PI field, regardless of how many objects are declared Consider the following method: double get. PI() { return PI; } o o It doesn’t read or modify the “state” of any object n In this example, it doesn’t read/write the radius In fact, that particular method doesn’t care anything about the objects declared n It’s only accessing a static field 148
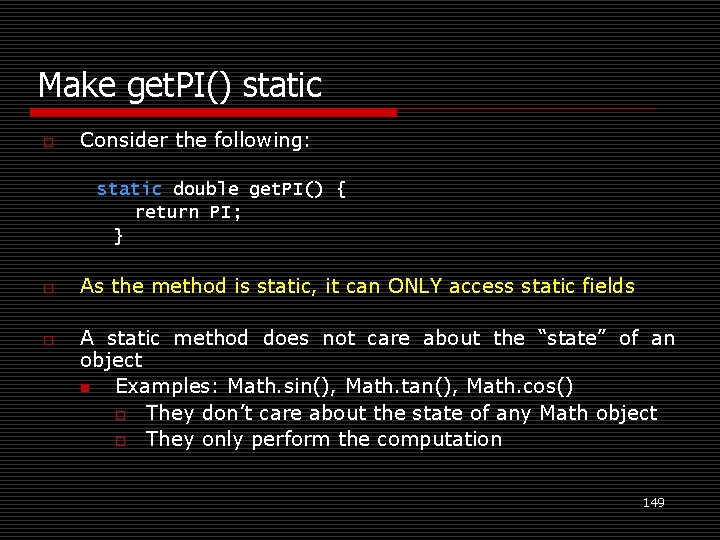
Make get. PI() static o Consider the following: static double get. PI() { return PI; } o o As the method is static, it can ONLY access static fields A static method does not care about the “state” of an object n Examples: Math. sin(), Math. tan(), Math. cos() o They don’t care about the state of any Math object o They only perform the computation 149
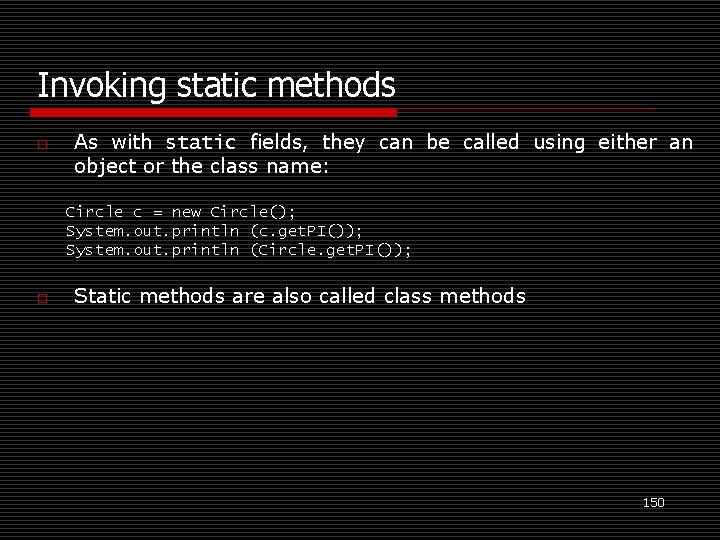
Invoking static methods o As with static fields, they can be called using either an object or the class name: Circle c = new Circle(); System. out. println (c. get. PI()); System. out. println (Circle. get. PI()); o Static methods are also called class methods 150
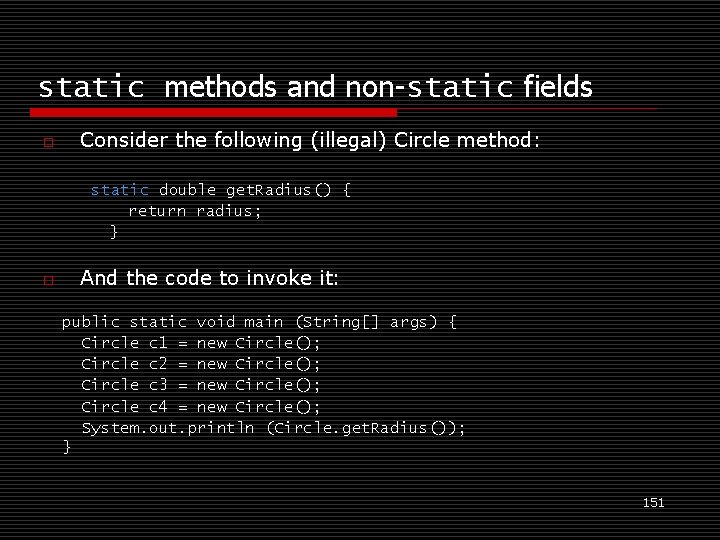
static methods and non-static fields o Consider the following (illegal) Circle method: static double get. Radius() { return radius; } o And the code to invoke it: public static void main (String[] args) { Circle c 1 = new Circle(); Circle c 2 = new Circle(); Circle c 3 = new Circle(); Circle c 4 = new Circle(); System. out. println (Circle. get. Radius()); } 151
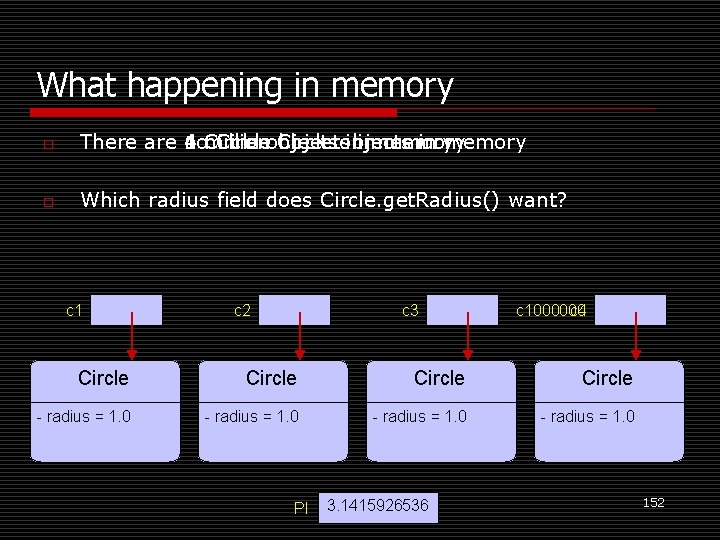
What happening in memory o There are no 4 million 1 Circleobjects Circle objects ininmemory in memory o Which radius field does Circle. get. Radius() want? c 1 c 2 c 3 Circle - radius = 1. 0 PI … c 1000000 c 4 Circle - radius = 1. 0 3. 1415926536 152
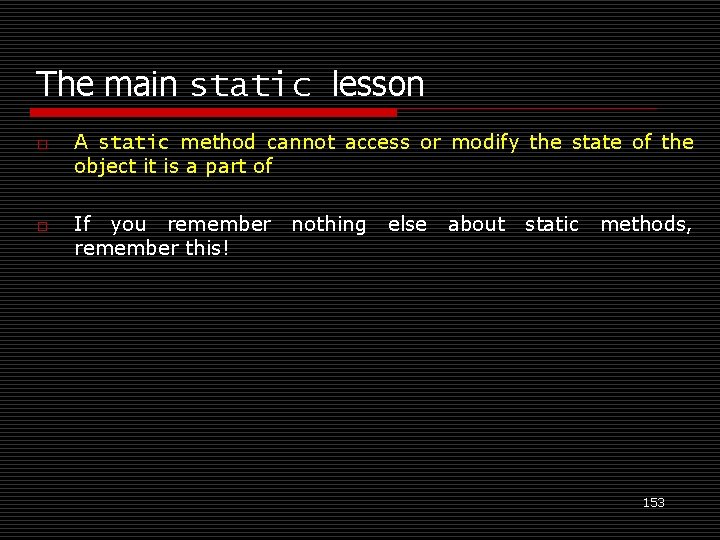
The main static lesson o o A static method cannot access or modify the state of the object it is a part of If you remember this! nothing else about static methods, 153
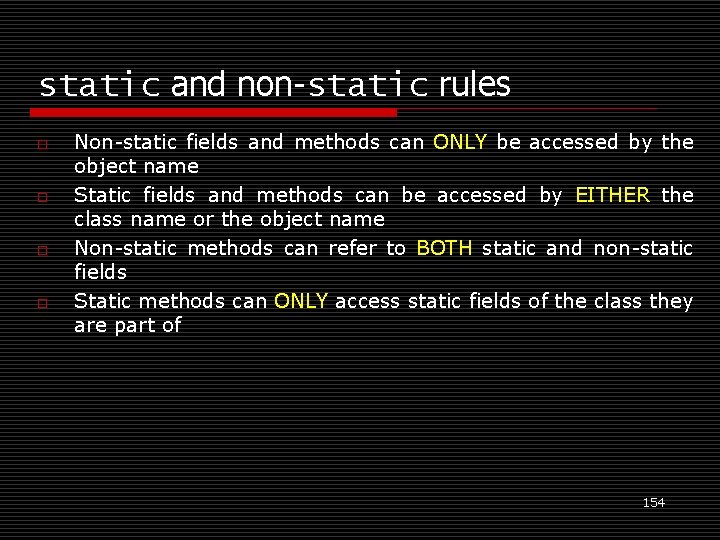
static and non-static rules o o Non-static fields and methods can ONLY be accessed by the object name Static fields and methods can be accessed by EITHER the class name or the object name Non-static methods can refer to BOTH static and non-static fields Static methods can ONLY access static fields of the class they are part of 154
![Back to our main method public static void main String args Well learn about Back to our main() method public static void main (String[] args) We’ll learn about](https://slidetodoc.com/presentation_image_h2/6690e48dd461f02db1cf60fdc6dd1ff1/image-151.jpg)
Back to our main() method public static void main (String[] args) We’ll learn about arrays in chapter 8 The method does not return a value Any code anywhere can call this method It’s a static method: • Can’t access non-static fields or methods directly • Can be called only by the class name 155
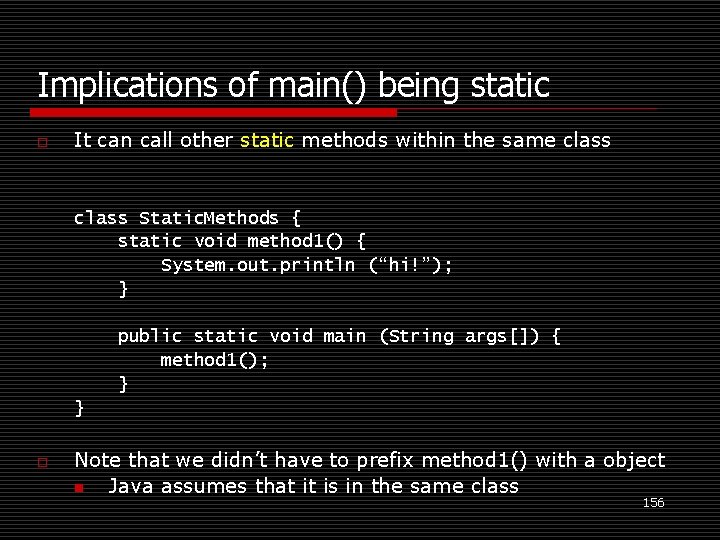
Implications of main() being static o It can call other static methods within the same class Static. Methods { static void method 1() { System. out. println (“hi!”); } public static void main (String args[]) { method 1(); } } o Note that we didn’t have to prefix method 1() with a object n Java assumes that it is in the same class 156
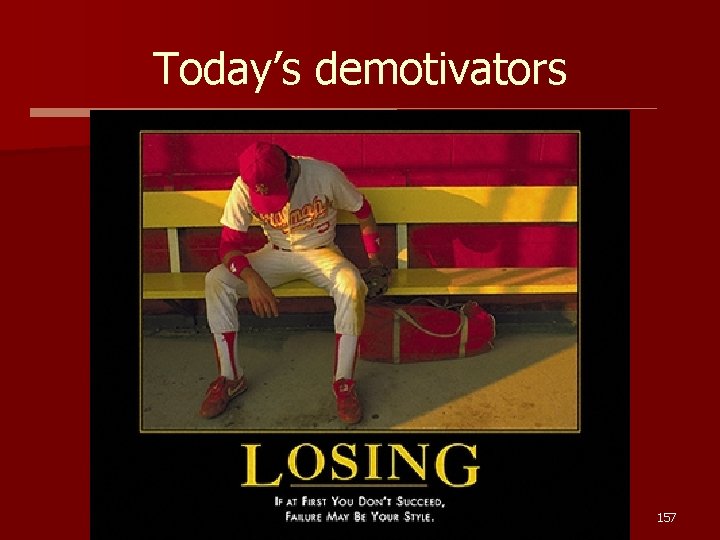
Today’s demotivators 157